java des加密方法
des加密算法java代码

des加密算法java代码1、DES加密算法Java实现(1)导入所需要的包:import javax.crypto.Cipher;import javax.crypto.SecretKey;import javax.crypto.SecretKeyFactory;import javax.crypto.spec.DESKeySpec;(2)定义加密算法:public class DesEncrypter {Cipher ecipher;Cipher dcipher;public DesEncrypter(String passPhrase) {try {// Create the keyDESKeySpec keySpec = newDESKeySpec(passPhrase.getBytes('UTF8'));SecretKeyFactory keyFactory =SecretKeyFactory.getInstance('DES');SecretKey key = keyFactory.generateSecret(keySpec); // Create the cipherecipher = Cipher.getInstance('DES');dcipher = Cipher.getInstance('DES');// Initialize the cipher for encryptionecipher.init(Cipher.ENCRYPT_MODE, key);// Initialize the same cipher for decryption dcipher.init(Cipher.DECRYPT_MODE, key);} catch (Exception e) {}}(3)定义加密函数:public String encrypt(String str) {try {// Encode the string into bytes using utf-8 byte[] utf8 = str.getBytes('UTF8');// Encryptbyte[] enc = ecipher.doFinal(utf8);// Encode bytes to base64 to get a stringreturn new sun.misc.BASE64Encoder().encode(enc);} catch (Exception e) {}return null;}(4)定义解密函数:// Decryptpublic String decrypt(String str) {try {// Decode base64 to get bytesbyte[] dec = newsun.misc.BASE64Decoder().decodeBuffer(str);// Decryptbyte[] utf8 = dcipher.doFinal(dec);// Decode using utf-8return new String(utf8, 'UTF8');} catch (Exception e) {}return null;}}(5)定义测试类,测试加密解之密是否正确:public class TestDes {public static void main(String[] args) {try {// Create encrypter/decrypter classDesEncrypter encrypter = new DesEncrypter('your key');// EncryptString encrypted = encrypter.encrypt('Don't tell anybody!');// DecryptString decrypted = encrypter.decrypt(encrypted);// Print out valuesSystem.out.println('Encrypted: ' + encrypted);System.out.println('Decrypted: ' + decrypted);} catch (Exception e) {}}}。
DES加密和解密java代码

DES加密和解密java代码在说DES加密算法之前,我们⾸先了解⼏个基本概念:1. 明⽂:明⽂是指没有经过加密的数据。
⼀般⽽⾔,明⽂都是等待传输的数据。
由于没有经过加密,明⽂很容易被识别与破解,因此在传输明⽂之前必须进⾏加密处理。
2. 密⽂:密⽂只是明⽂经过某种加密算法⽽得到的数据,通常密⽂的形式复杂难以识别及理解。
3. 密钥:密钥是⼀种参数,它是在明⽂转换为密⽂或将密⽂转换为明⽂的算法中输⼊的参数。
4. 对称加密:通信双⽅同时掌握⼀个密钥,加密解密都是由⼀个密钥完成的(即加密密钥等于解密密钥,加解密密钥可以相互推倒出来)。
双⽅通信前共同拟定⼀个密钥,不对第三⽅公开。
5. 分组加密:分组密码是将明⽂分成固定长度的组,每⼀组都采⽤同⼀密钥和算法进⾏加密,输出也是固定长度的密⽂。
好了了解这些以后,我们再来说DES加密算法。
DES 算法是⼀种常见的分组加密算法,由IBM公司在1971年提出。
DES 算法是分组加密算法的典型代表,同时也是应⽤最为⼴泛的对称加密算法。
下⾯我们详细介绍下:1.分组长度:DES 加密算法中,明⽂和密⽂为 64 位分组。
密钥的长度为 64 位,但是密钥的每个第⼋位设置为奇偶校验位,因此密钥的实际长度为56位。
2.加密流程:DES 加密算法⼤致分为 4 步:初始置换、⽣成⼦密钥、迭代过程、逆置换。
代码如下:需特别注意:不要使⽤JDK中⾃带的sun.misc.BASE64Decoder类去做BASE64,该类会在最后⾯多加换⾏。
⽽应使⽤apache中的mons.codec.binary.Base64这个类来做base64加密。
(待验证)import javax.crypto.Cipher;import javax.crypto.SecretKeyFactory;import javax.crypto.spec.DESKeySpec;import javax.crypto.spec.IvParameterSpec;import java.security.Key;import java.security.SecureRandom;import mons.codec.binary.Base64;public class DESUtil {// 偏移变量,固定占8位字节private final static String IV_PARAMETER = "12345678";// 字符编码public static final String CHARSET_UTF8 = "UTF-8";// 加密算法DESpublic static final String DES = "DES";// 电话本模式public static final String DES_ECB = "DES/ECB/PKCS5Padding";// 加密块链模式--推荐public static final String DES_CBC = "DES/CBC/PKCS5Padding";// 测试public static void main(String args[]) {// 待加密内容String str = "false";// 密码,长度要是8的倍数String password = "87654321";String result = DESUtil.encryptCBC(password, str);System.out.println("加密后:" + new String(result));// 直接将如上内容解密try {String decryResult = DESUtil.decryptCBC(password, result);System.out.println("解密后:" + new String(decryResult));} catch (Exception e1) {e1.printStackTrace();}}/*** ⽣成key** @param password* @return* @throws Exception*/private static Key generateKey(String password) throws Exception {DESKeySpec dks = new DESKeySpec(password.getBytes(CHARSET_UTF8));SecretKeyFactory keyFactory = SecretKeyFactory.getInstance(DES);return keyFactory.generateSecret(dks);}/*** DES加密字符串--ECB格式** @param password* 加密密码,长度不能够⼩于8位* @param data* 待加密字符串* @return加密后内容*/public static String encryptECB(String password, String data) {if (password == null || password.length() < 8) {throw new RuntimeException("加密失败,key不能⼩于8位");}if (data == null) {return null;}try {Key secretKey = generateKey(password);Cipher cipher = Cipher.getInstance(DES_ECB);cipher.init(Cipher.ENCRYPT_MODE, secretKey, new SecureRandom());byte[] bytes = cipher.doFinal(data.getBytes(CHARSET_UTF8));// JDK1.8及以上可直接使⽤Base64,JDK1.7及以下可以使⽤BASE64Encoder return new String(Base64.encodeBase64(bytes));} catch (Exception e) {e.printStackTrace();return data;}}/*** DES解密字符串--ECB格式** @param password* 解密密码,长度不能够⼩于8位* @param data* 待解密字符串* @return解密后内容*/public static String decryptECB(String password, String data)throws Exception {Key secretKey = generateKey(password);Cipher cipher = Cipher.getInstance(DES_ECB);cipher.init(Cipher.DECRYPT_MODE, secretKey, new SecureRandom());return new String(cipher.doFinal(Base64.decodeBase64(data.getBytes(CHARSET_UTF8))), CHARSET_UTF8);}/*** DES加密字符串-CBC加密格式** @param password* 加密密码,长度不能够⼩于8位* @param data* 待加密字符串* @return加密后内容*/public static String encryptCBC(String password, String data) {if (password == null || password.length() < 8) {throw new RuntimeException("加密失败,key不能⼩于8位");}if (data == null) {return null;}try {Key secretKey = generateKey(password);Cipher cipher = Cipher.getInstance(DES_CBC);IvParameterSpec spec = new IvParameterSpec(IV_PARAMETER.getBytes(CHARSET_UTF8));cipher.init(Cipher.ENCRYPT_MODE, secretKey, spec);byte[] bytes = cipher.doFinal(data.getBytes(CHARSET_UTF8));// JDK1.8及以上可直接使⽤Base64,JDK1.7及以下可以使⽤BASE64Encoder return new String(Base64.encodeBase64(bytes));} catch (Exception e) {e.printStackTrace();return data;}}/*** DES解密字符串--CBC格式** @param password* 解密密码,长度不能够⼩于8位* @param data* 待解密字符串* @return解密后内容*/public static String decryptCBC(String password, String data) throws Exception {Key secretKey = generateKey(password);Cipher cipher = Cipher.getInstance(DES_CBC);IvParameterSpec spec = new IvParameterSpec(IV_PARAMETER.getBytes(CHARSET_UTF8));cipher.init(Cipher.DECRYPT_MODE, secretKey, spec);return new String(cipher.doFinal(Base64.decodeBase64(data.getBytes(CHARSET_UTF8))), CHARSET_UTF8); }}。
Java实现DES加密解密

Java实现DES加密解密DES(Data Encryption Standard)是⼀种对称加密算法,所谓对称加密就是加密和解密都是使⽤同⼀个密钥加密原理:DES 使⽤⼀个 56 位的密钥以及附加的 8 位奇偶校验位,产⽣最⼤ 64 位的分组⼤⼩。
这是⼀个迭代的分组密码,使⽤称为 Feistel 的技术,其中将加密的⽂本块分成两半。
使⽤⼦密钥对其中⼀半应⽤循环功能,然后将输出与另⼀半进⾏"异或"运算;接着交换这两半,这⼀过程会继续下去,但最后⼀个循环不交换。
DES 使⽤ 16 个循环,使⽤异或,置换,代换,移位操作四种基本运算。
不过,DES已可破解,所以针对保密级别特别⾼的数据推荐使⽤⾮对称加密算法。
下⾯介绍基于Java实现的DES加解密⽅法,该⽅法同样适⽤于Android平台,使⽤的是JDK1.8。
public class DESUtil {/*** 偏移变量,固定占8位字节*/private final static String IV_PARAMETER = "12345678";/*** 密钥算法*/private static final String ALGORITHM = "DES";/*** 加密/解密算法-⼯作模式-填充模式*/private static final String CIPHER_ALGORITHM = "DES/CBC/PKCS5Padding";/*** 默认编码*/private static final String CHARSET = "utf-8";/*** ⽣成key** @param password* @return* @throws Exception*/private static Key generateKey(String password) throws Exception {DESKeySpec dks = new DESKeySpec(password.getBytes(CHARSET));SecretKeyFactory keyFactory = SecretKeyFactory.getInstance(ALGORITHM);return keyFactory.generateSecret(dks);}/*** DES加密字符串** @param password 加密密码,长度不能够⼩于8位* @param data 待加密字符串* @return 加密后内容*/public static String encrypt(String password, String data) {if (password== null || password.length() < 8) {throw new RuntimeException("加密失败,key不能⼩于8位");}if (data == null)return null;try {Key secretKey = generateKey(password);Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM);IvParameterSpec iv = new IvParameterSpec(IV_PARAMETER.getBytes(CHARSET));cipher.init(Cipher.ENCRYPT_MODE, secretKey, iv);byte[] bytes = cipher.doFinal(data.getBytes(CHARSET));//JDK1.8及以上可直接使⽤Base64,JDK1.7及以下可以使⽤BASE64Encoder//Android平台可以使⽤android.util.Base64return new String(Base64.getEncoder().encode(bytes));} catch (Exception e) {e.printStackTrace();return data;}}/*** DES解密字符串** @param password 解密密码,长度不能够⼩于8位* @param data 待解密字符串* @return 解密后内容*/public static String decrypt(String password, String data) {if (password== null || password.length() < 8) {throw new RuntimeException("加密失败,key不能⼩于8位");}if (data == null)return null;try {Key secretKey = generateKey(password);Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM);IvParameterSpec iv = new IvParameterSpec(IV_PARAMETER.getBytes(CHARSET));cipher.init(Cipher.DECRYPT_MODE, secretKey, iv);return new String(cipher.doFinal(Base64.getDecoder().decode(data.getBytes(CHARSET))), CHARSET); } catch (Exception e) {e.printStackTrace();return data;}}/*** DES加密⽂件** @param srcFile 待加密的⽂件* @param destFile 加密后存放的⽂件路径* @return 加密后的⽂件路径*/public static String encryptFile(String password, String srcFile, String destFile) {if (password== null || password.length() < 8) {throw new RuntimeException("加密失败,key不能⼩于8位");}try {IvParameterSpec iv = new IvParameterSpec(IV_PARAMETER.getBytes(CHARSET));Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM);cipher.init(Cipher.ENCRYPT_MODE, generateKey(key), iv);InputStream is = new FileInputStream(srcFile);OutputStream out = new FileOutputStream(destFile);CipherInputStream cis = new CipherInputStream(is, cipher);byte[] buffer = new byte[1024];int r;while ((r = cis.read(buffer)) > 0) {out.write(buffer, 0, r);}cis.close();is.close();out.close();return destFile;} catch (Exception ex) {ex.printStackTrace();}return null;}/*** DES解密⽂件** @param srcFile 已加密的⽂件* @param destFile 解密后存放的⽂件路径* @return 解密后的⽂件路径*/public static String decryptFile(String password, String srcFile, String destFile) {if (password== null || password.length() < 8) {throw new RuntimeException("加密失败,key不能⼩于8位");}try {File file = new File(destFile);if (!file.exists()) {file.getParentFile().mkdirs();file.createNewFile();}IvParameterSpec iv = new IvParameterSpec(IV_PARAMETER.getBytes(CHARSET));Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM);cipher.init(Cipher.DECRYPT_MODE, generateKey(key), iv);InputStream is = new FileInputStream(srcFile);OutputStream out = new FileOutputStream(destFile);CipherOutputStream cos = new CipherOutputStream(out, cipher);byte[] buffer = new byte[1024];int r;while ((r = is.read(buffer)) >= 0) {cos.write(buffer, 0, r);}cos.close();is.close();out.close();return destFile;} catch (Exception ex) { ex.printStackTrace(); }return null;}}。
java加密解密算法desededesdiffie-hellman的使用
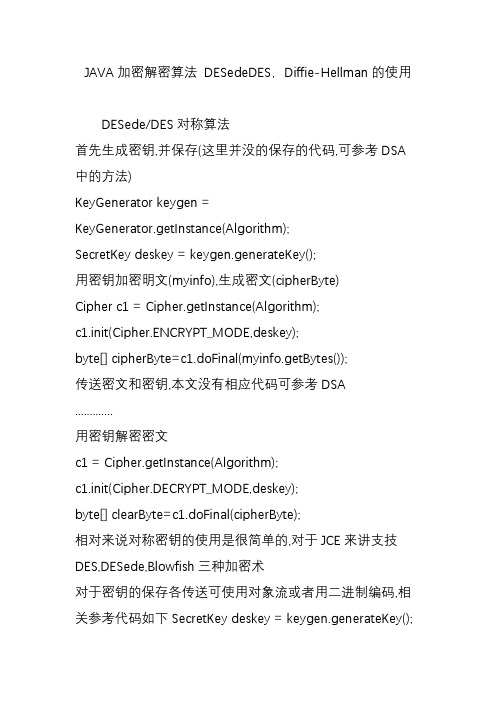
JAVA加密解密算法DESedeDES,Diffie-Hellman的使用DESede/DES对称算法首先生成密钥,并保存(这里并没的保存的代码,可参考DSA 中的方法)KeyGenerator keygen =KeyGenerator.getInstance(Algorithm);SecretKey deskey = keygen.generateKey();用密钥加密明文(myinfo),生成密文(cipherByte)Cipher c1 = Cipher.getInstance(Algorithm);c1.init(Cipher.ENCRYPT_MODE,deskey);byte[] cipherByte=c1.doFinal(myinfo.getBytes());传送密文和密钥,本文没有相应代码可参考DSA .............用密钥解密密文c1 = Cipher.getInstance(Algorithm);c1.init(Cipher.DECRYPT_MODE,deskey);byte[] clearByte=c1.doFinal(cipherByte);相对来说对称密钥的使用是很简单的,对于JCE来讲支技DES,DESede,Blowfish三种加密术对于密钥的保存各传送可使用对象流或者用二进制编码,相关参考代码如下SecretKey deskey =keygen.generateKey(); byte[]desEncode=deskey.getEncoded();javax.crypto.spec.SecretKeySpec destmp=newjavax.crypto.spec.SecretKeySpec(desEncode,Algorithm); SecretKey mydeskey=destmp;相关APIKeyGenerator 在DSA中已经说明,在添加JCE后在instance进可以如下参数DES,DESede,Blowfish,HmacMD5,HmacSHA1javax.crypto.Cipher 加/解密器public static final Cipher getInstance(ng.String transformation)throws java.security.NoSuchAlgorithmException, NoSuchPaddingException返回一个指定方法的Cipher对象参数:transformation 方法名(可用DES,DESede,Blowfish) public final void init(int opmode, java.security.Key key) throws java.security.InvalidKeyException用指定的密钥和模式初始化Cipher对象参数:opmode 方式(ENCRYPT_MODE, DECRYPT_MODE, WRAP_MODE,UNWRAP_MODE)key 密钥public final byte[] doFinal(byte[] input)throws ng.IllegalStateException, IllegalBlockSizeException,BadPaddingException对input内的串,进行编码处理,返回处理后二进制串,是返回解密文还是加解文由init时的opmode决定注意:本方法的执行前如果有update,是对updat和本次input 全部处理,否则是本inout的内容/*安全程序DESede/DES 测试*/import java.security.*;import javax.crypto.*;public class testdes {public static void main(String[]args){ testdes my=new testdes();my.run(); }public void run() {//添加新安全算法,如果用JCE就要把它添加进去Security.addProvider(newcom.sun.crypto.provider.SunJCE());StringAlgorithm="DES"; //定义加密算法,可用DES,DESede,BlowfishString myinfo="要加密的信息";try { //生成密钥KeyGenerator keygen = KeyGenerator.getInstance(Algorithm); SecretKey deskey = keygen.generateKey(); //加密System.out.println("加密前的二进串:"+byte2hex(myinfo.getBytes())); System.out.println("加密前的信息:"+myinfo); Cipher c1 =Cipher.getInstance(Algorithm);c1.init(Cipher.ENCRYPT_MODE,deskey); byte[] cipherByte=c1.doFinal(myinfo.getBytes());System.out.println("加密后的二进串:"+byte2hex(cipherByte)); //解密c1 =Cipher.getInstance(Algorithm);c1.init(Cipher.DECRYPT_MODE,deskey); byte[] clearByte=c1.doFinal(cipherByte); System.out.println("解密后的二进串:"+byte2hex(clearByte));System.out.println("解密后的信息:"+(newString(clearByte))); } catch(java.security.NoSuchAlgorithmException e1){e1.printStackTrace();} catch(javax.crypto.NoSuchPaddingException e2){e2.printStackTrace();} catch (ng.Exception e3) {e3.printStackTrace();} } public String byte2hex(byte[] b) //二行制转字符串{ String hs=""; String stmp=""; for (int n=0;n2.5. Diffie-Hellman密钥一致协议公开密钥密码体制的奠基人Diffie和Hellman所提出的"指数密钥一致协议"(Exponential Key Agreement Protocol),该协议不要求别的安全性先决条件,允许两名用户在公开媒体上交换信息以生成"一致"的,可以共享的密钥。
Java 加密解密之对称加密算法DESede

Java 加密解密之对称加密算法DESede本文转自网络DESede即三重DES加密算法,也被称为3DES或者Triple DES。
使用三(或两)个不同的密钥对数据块进行三次(或两次)DES加密(加密一次要比进行普通加密的三次要快)。
三重DES的强度大约和112-bit的密钥强度相当。
通过迭代次数的提高了安全性,但同时也造成了加密效率低的问题。
正因DESede算法效率问题,AES算法诞生了。
(详见:Java 加密解密之对称加密算法AES)到目前为止,还没有人给出攻击三重DES的有效方法。
对其密钥空间中密钥进行蛮干搜索,那么由于空间太大,这实际上是不可行的。
若用差分攻击的方法,相对于单一DES来说复杂性以指数形式增长。
三重DES有四种模型(a)DES-EEE3,使用三个不同密钥,顺序进行三次加密变换。
(b)DES-EDE3,使用三个不同密钥,依次进行加密-解密-加密变换。
(c)DES-EEE2,其中密钥K1=K3,顺序进行三次加密变换。
(d)DES-EDE2,其中密钥K1=K3,依次进行加密-解密-加密变换。
JDK对DESede算法的支持密钥长度:112位/168位工作模式:ECB/CBC/PCBC/CTR/CTS/CFB/CFB8 to CFB128/OFB/OBF8 to OFB128填充方式:Nopadding/PKCS5Padding/ISO10126Padding/工作模式和填充方式请参考:JAVA加密解密基础十六进制工具类Hex.java,见:java byte数组与十六进制字符串互转DESede加密解密的java实现:DESede.javaJava代码1.import java.security.Key;2.3.import javax.crypto.Cipher;4.import javax.crypto.KeyGenerator;。
DES加密算法代码
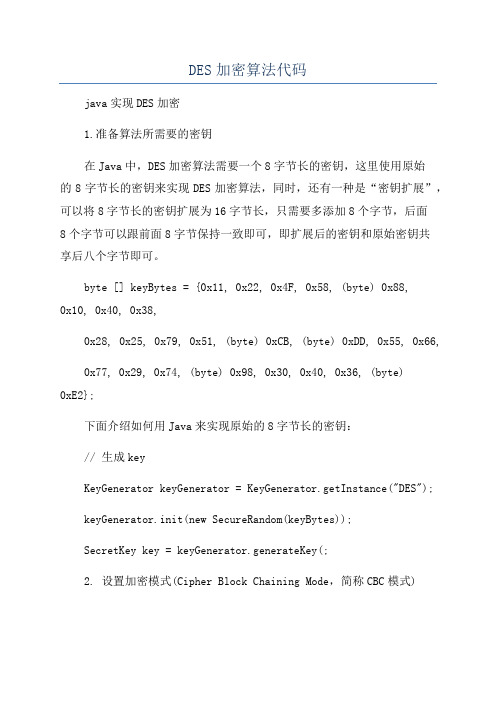
DES加密算法代码java实现DES加密1.准备算法所需要的密钥在Java中,DES加密算法需要一个8字节长的密钥,这里使用原始的8字节长的密钥来实现DES加密算法,同时,还有一种是“密钥扩展”,可以将8字节长的密钥扩展为16字节长,只需要多添加8个字节,后面8个字节可以跟前面8字节保持一致即可,即扩展后的密钥和原始密钥共享后八个字节即可。
byte [] keyBytes = {0x11, 0x22, 0x4F, 0x58, (byte) 0x88,0x10, 0x40, 0x38,0x28, 0x25, 0x79, 0x51, (byte) 0xCB, (byte) 0xDD, 0x55, 0x66, 0x77, 0x29, 0x74, (byte) 0x98, 0x30, 0x40, 0x36, (byte)0xE2};下面介绍如何用Java来实现原始的8字节长的密钥:// 生成keyKeyGenerator keyGenerator = KeyGenerator.getInstance("DES");keyGenerator.init(new SecureRandom(keyBytes));SecretKey key = keyGenerator.generateKey(;2. 设置加密模式(Cipher Block Chaining Mode,简称CBC模式)在Java中,DES加密算法可以支持三种不同的加密模式:ECB,CBC,CFB。
其中ECB模式为最原始的模式,它不需要任何附加的参数,而CBC模式需要一个8字节的初始向量(Initial Vector,IV)参数,CFB模式需要一个1字节或8字节的变量参数,它们用来增加加密的强度。
这里,我们使用CBC模式,它是最常用的DES加密模式,下面是设置CBC模式所需要的参数:// 创建Cipher对象,指定其支持的DES算法Cipher cipher = Cipher.getInstance("DES/CBC/PKCS5Padding");// 用密匙初始化Cipher对象IvParameterSpec param = new IvParameterSpec(iv);cipher.init(Cipher.ENCRYPT_MODE, key,param);3.加密。
DES加密算法的JAVA实现
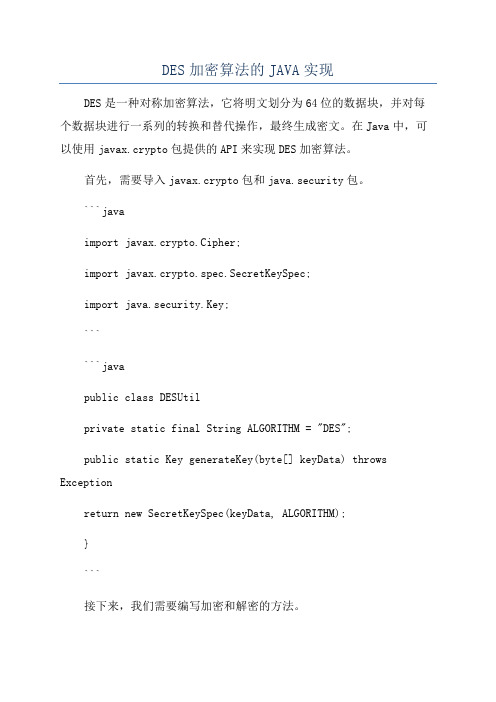
DES加密算法的JAVA实现DES是一种对称加密算法,它将明文划分为64位的数据块,并对每个数据块进行一系列的转换和替代操作,最终生成密文。
在Java中,可以使用javax.crypto包提供的API来实现DES加密算法。
首先,需要导入javax.crypto包和java.security包。
```javaimport javax.crypto.Cipher;import javax.crypto.spec.SecretKeySpec;import java.security.Key;``````javapublic class DESUtilprivate static final String ALGORITHM = "DES";public static Key generateKey(byte[] keyData) throws Exceptionreturn new SecretKeySpec(keyData, ALGORITHM);}```接下来,我们需要编写加密和解密的方法。
```javapublic class DESUtil//...public static byte[] encrypt(byte[] data, Key key) throws ExceptionCipher cipher = Cipher.getInstance(ALGORITHM);cipher.init(Cipher.ENCRYPT_MODE, key);return cipher.doFinal(data);}public static byte[] decrypt(byte[] encryptedData, Key key) throws ExceptionCipher cipher = Cipher.getInstance(ALGORITHM);cipher.init(Cipher.DECRYPT_MODE, key);return cipher.doFinal(encryptedData);}```在上述代码中,encrypt方法用于将明文数据加密成密文,decrypt 方法用于将密文数据解密成明文。
DES加密算法详细原理以及Java代码实现
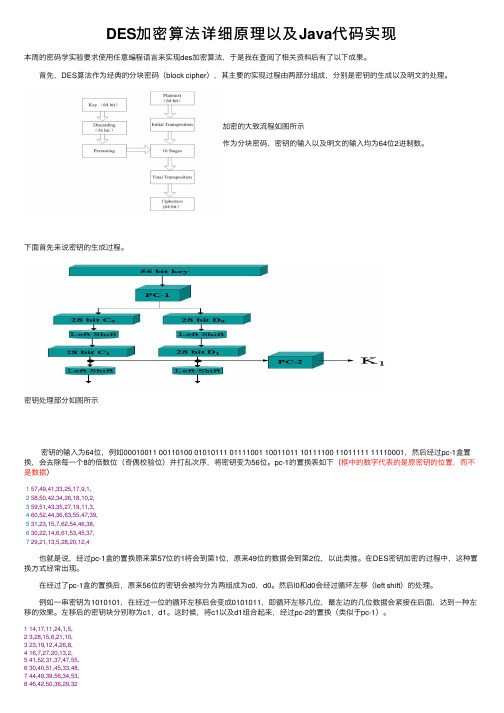
DES加密算法详细原理以及Java代码实现本周的密码学实验要求使⽤任意编程语⾔来实现des加密算法,于是我在查阅了相关资料后有了以下成果。
⾸先,DES算法作为经典的分块密码(block cipher),其主要的实现过程由两部分组成,分别是密钥的⽣成以及明⽂的处理。
加密的⼤致流程如图所⽰作为分块密码,密钥的输⼊以及明⽂的输⼊均为64位2进制数。
下⾯⾸先来说密钥的⽣成过程。
密钥处理部分如图所⽰ 密钥的输⼊为64位,例如00010011 00110100 01010111 01111001 10011011 10111100 11011111 11110001,然后经过pc-1盒置换,会去除每⼀个8的倍数位(奇偶校验位)并打乱次序,将密钥变为56位。
pc-1的置换表如下(框中的数字代表的是原密钥的位置,⽽不是数据)157,49,41,33,25,17,9,1,258,50,42,34,26,18,10,2,359,51,43,35,27,19,11,3,460,52,44,36,63,55,47,39,531,23,15,7,62,54,46,38,630,22,14,6,61,53,45,37,729,21,13,5,28,20,12,4 也就是说,经过pc-1盒的置换原来第57位的1将会到第1位,原来49位的数据会到第2位,以此类推。
在DES密钥加密的过程中,这种置换⽅式经常出现。
在经过了pc-1盒的置换后,原来56位的密钥会被均分为两组成为c0,d0。
然后l0和d0会经过循环左移(left shift)的处理。
例如⼀串密钥为1010101,在经过⼀位的循环左移后会变成0101011,即循环左移⼏位,最左边的⼏位数据会紧接在后⾯,达到⼀种左移的效果。
左移后的密钥块分别称为c1,d1。
这时候,将c1以及d1组合起来,经过pc-2的置换(类似于pc-1)。
114,17,11,24,1,5,23,28,15,6,21,10,323,19,12,4,26,8,416,7,27,20,13,2,541,52,31,37,47,55,630,40,51,45,33,48,744,49,39,56,34,53,846,42,50,36,29,32 经过了pc-2盒的置换后,原本56位的密钥会变为48位。
S-DES加密算法的JAVA实现

周胜安(200620109286) 计算机科学与工程研一(2)班S-DES加密算法的JAVA实现一、实验要求(1)简述S-DES的加密算法的主要思想(2)实现的主要步骤,及各个步骤的主要思想及示意图(3)给出程序的分析,运行结果和程序的效率分析(4)通过编程实现S-DES加密算法,了解DES加密的原理,也加深对现代对称密码的理解。
二、算法思想•加密算法的数学表示:密文=IP-1fk2(SW(fk1(IP(明文)))))其中K1=P8(移位(P10(密钥K)))K2=P8(移位(移位(P10(密钥K))))• 解密算法的数学表示:明文=IP-1(fk1(SW(fk2(IP(密文)))))S-DES 加密方法示意图:S-DES算法涉及加密、密钥产生、解密三个部分。
流程图(每个函数的算法见源代码)三、算法流程框图、各模块说明实验是用JAVA实现的(1)密钥的产生密钥产生的示意图:密钥的产生算法涉及五个函数定义(1)初始置换P10 其中P10的定义为:P10(k1,k2,…,k10)=(k3,k5,k2,k7,k4,k10,k1,k9,k8,k6)(2)LS-1操作:对密钥的左右两组循环左移一位(密钥分成5位一组,共2组)(3)P8置换产生Key1。
其中P8的定义为:P8(k1,k2,…,k10)=(k6,k3,k7,k4,k8,k5,k10,k9)(4)LS-2操作: :对密钥的左右两组循环左移两位.(5) P8置换产生Key2(2) S-DES 的加密过程示意图:加密算法涉及五个函数:(1)初始置换IP(initial permutation)。
其中P8的定义为:IP(k1,k2,…,k8)=(k2,k6,k3,k1,k4,k8,k5,k7)(2)复合函数fk,它是由密钥K确定的,具有置换和代替的运算。
函数fk,是加密方案中的最重要部分,它可表示为:fk(L,R)=(L F(R,SK),R)其中L,R为8位输入, 左右各为4位, F为从4位集到4位集的一个映射, 并不要求是1-1的。
java实现DES加密算法
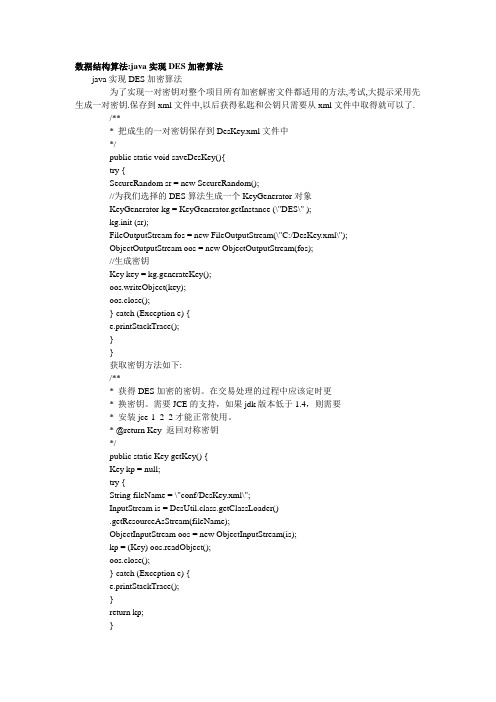
数据结构算法:java实现DES加密算法java实现DES加密算法为了实现一对密钥对整个项目所有加密解密文件都适用的方法,考试,大提示采用先生成一对密钥.保存到xml文件中,以后获得私匙和公钥只需要从xml文件中取得就可以了./*** 把成生的一对密钥保存到DesKey.xml文件中*/public static void saveDesKey(){try {SecureRandom sr = new SecureRandom();//为我们选择的DES算法生成一个KeyGenerator对象KeyGenerator kg = KeyGenerator.getInstance (\"DES\" );kg.init (sr);FileOutputStream fos = new FileOutputStream(\"C:/DesKey.xml\");ObjectOutputStream oos = new ObjectOutputStream(fos);//生成密钥Key key = kg.generateKey();oos.writeObject(key);oos.close();} catch (Exception e) {e.printStackTrace();}}获取密钥方法如下:/*** 获得DES加密的密钥。
在交易处理的过程中应该定时更* 换密钥。
需要JCE的支持,如果jdk版本低于1.4,则需要* 安装jce-1_2_2才能正常使用。
* @return Key 返回对称密钥*/public static Key getKey() {Key kp = null;try {String fileName = \"conf/DesKey.xml\";InputStream is = DesUtil.class.getClassLoader().getResourceAsStream(fileName);ObjectInputStream oos = new ObjectInputStream(is);kp = (Key) oos.readObject();oos.close();} catch (Exception e) {e.printStackTrace();}return kp;}文件采用DES算法加密文件/*** 文件file进行加密并保存目标文件destFile中* @param file* 要加密的文件如c:/test/srcFile.txt* @param destFile* 加密后存放的文件名如c:/加密后文件.txt*/public static void encrypt(String file, String destFile) throws Exception { Cipher cipher = Cipher.getInstance(\"DES\");cipher.init(Cipher.ENCRYPT_MODE, getKey());InputStream is = new FileInputStream(file);OutputStream out = new FileOutputStream(dest); CipherInputStream cis = new CipherInputStream(is, cipher);byte[] buffer = new byte[1024];int r;while ((r = cis.read(buffer)) > 0) {out.write(buffer, 0, r);}cis.close();is.close();out.close();}文件采用DES算法解密文件/*** 文件file进行加密并保存目标文件destFile中* @param file* 已加密的文件如c:/加密后文件.txt* @param destFile* 解密后存放的文件名如c:/ test/解密后文件.txt*/public static void decrypt(String file, String dest) throws Exception { Cipher cipher = Cipher.getInstance(\"DES\");cipher.init(Cipher.DECRYPT_MODE, getKey());InputStream is = new FileInputStream(file);OutputStream out = new FileOutputStream(dest); CipherOutputStream cos = new CipherOutputStream(out, cipher);byte[] buffer = new byte[1024];int r;while ((r = is.read(buffer)) >= 0) {cos.write(buffer, 0, r);}cos.close();out.close();is.close(); }。
JAVADES加密(DESECBPKCS5Padding)和C#加密对应设置
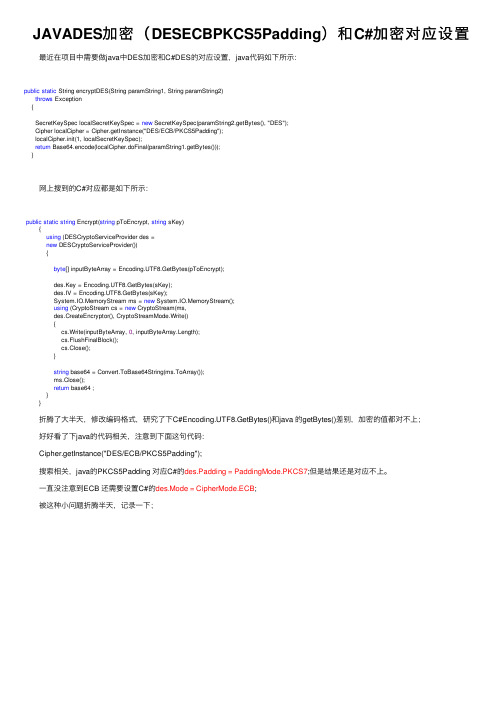
JAVADES加密(DESECBPKCS5Padding)和C#加密对应设置 最近在项⽬中需要做java中DES加密和C#DES的对应设置,java代码如下所⽰:public static String encryptDES(String paramString1, String paramString2)throws Exception{SecretKeySpec localSecretKeySpec = new SecretKeySpec(paramString2.getBytes(), "DES");Cipher localCipher = Cipher.getInstance("DES/ECB/PKCS5Padding");localCipher.init(1, localSecretKeySpec);return Base64.encode(localCipher.doFinal(paramString1.getBytes()));} ⽹上搜到的C#对应都是如下所⽰:public static string Encrypt(string pToEncrypt, string sKey){using (DESCryptoServiceProvider des =new DESCryptoServiceProvider()){byte[] inputByteArray = Encoding.UTF8.GetBytes(pToEncrypt);des.Key = Encoding.UTF8.GetBytes(sKey);des.IV = Encoding.UTF8.GetBytes(sKey);System.IO.MemoryStream ms = new System.IO.MemoryStream();using (CryptoStream cs = new CryptoStream(ms,des.CreateEncryptor(), CryptoStreamMode.Write)){cs.Write(inputByteArray, 0, inputByteArray.Length);cs.FlushFinalBlock();cs.Close();}string base64 = Convert.ToBase64String(ms.ToArray());ms.Close();return base64 ;}} 折腾了⼤半天,修改编码格式,研究了下C#Encoding.UTF8.GetBytes()和java 的getBytes()差别,加密的值都对不上; 好好看了下java的代码相关,注意到下⾯这句代码: Cipher.getInstance("DES/ECB/PKCS5Padding"); 搜索相关,java的PKCS5Padding 对应C#的des.Padding = PaddingMode.PKCS7;但是结果还是对应不上。
Des加密(js+java结果一致)
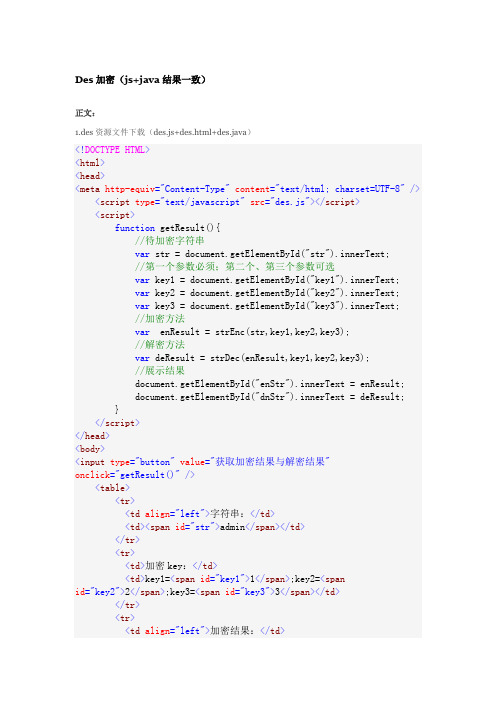
Des加密(js+java结果一致)正文:1.des资源文件下载(des.js+des.html+des.java)<!DOCTYPE HTML><html><head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8"/> <script type="text/javascript" src="des.js"></script><script>function getResult(){//待加密字符串var str = document.getElementById("str").innerText;//第一个参数必须;第二个、第三个参数可选var key1 = document.getElementById("key1").innerText;var key2 = document.getElementById("key2").innerText;var key3 = document.getElementById("key3").innerText;//加密方法var enResult = strEnc(str,key1,key2,key3);//解密方法var deResult = strDec(enResult,key1,key2,key3);//展示结果document.getElementById("enStr").innerText = enResult; document.getElementById("dnStr").innerText = deResult; }</script></head><body><input type="button" value="获取加密结果与解密结果"onclick="getResult()"/><table><tr><td align="left">字符串:</td><td><span id="str">admin</span></td></tr><tr><td>加密key:</td><td>key1=<span id="key1">1</span>;key2=<spanid="key2">2</span>;key3=<span id="key3">3</span></td></tr><tr><td align="left">加密结果:</td><td align="left"><label id = "enStr"></label></td></tr><tr><td align="left">解密结果:</td><td align="left"><label id = "dnStr"></label></td></tr><table></body></html>4.java文件(des.java)package com.zz.test;import java.util.ArrayList;import java.util.List;public class Des {public Des() {}public static void main(String[] args) {Des desObj = new Des();String key1 = "1";String key2 = "2";String key3 = "3";String data = "admin";String str = desObj.strEnc(data, key1, key2, key3);System.out.println(str);String dec = desObj.strDec(str, key1, key2, key3);System.out.println(dec);}/*** DES加密/解密** @Copyright Copyright (c) 2006* @author Guapo* @see DESCore*//** encrypt the string to string made up of hex return the encrypted string*/public String strEnc(String data, String firstKey, String secondKey, String thirdKey) {int leng = data.length();String encData = "";List firstKeyBt = null, secondKeyBt = null, thirdKeyBt = null;int firstLength = 0, secondLength = 0, thirdLength = 0;if (firstKey != null && firstKey != "") {firstKeyBt = getKeyBytes(firstKey);firstLength = firstKeyBt.size();}if (secondKey != null && secondKey != "") {secondKeyBt = getKeyBytes(secondKey);secondLength = secondKeyBt.size();}if (thirdKey != null && thirdKey != "") {thirdKeyBt = getKeyBytes(thirdKey);thirdLength = thirdKeyBt.size();}if (leng > 0) {if (leng < 4) {int[] bt = strToBt(data);int[] encByte = null;if (firstKey != null && firstKey != "" && secondKey != null&& secondKey != "" && thirdKey != null&& thirdKey != "") {int[] tempBt;int x, y, z;tempBt = bt;for (x = 0; x < firstLength; x++) {tempBt = enc(tempBt, (int[])firstKeyBt.get(x));}for (y = 0; y < secondLength; y++) {tempBt = enc(tempBt, (int[])secondKeyBt.get(y));}for (z = 0; z < thirdLength; z++) {tempBt = enc(tempBt, (int[])thirdKeyBt.get(z));}} else {if(firstKey != null&& firstKey != "" && secondKey != null&& secondKey != "") {int[] tempBt;int x, y;tempBt = bt;for (x = 0; x < firstLength; x++) {tempBt = enc(tempBt, (int[])firstKeyBt.get(x));}for (y = 0; y < secondLength; y++) {tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));}encByte = tempBt;} else {if (firstKey != null && firstKey != "") {int[] tempBt;int x = 0;tempBt = bt;for (x = 0; x < firstLength; x++) {tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));}encByte = tempBt;}}}encData = bt64ToHex(encByte);} else {int iterator = (leng / 4);int remainder = leng % 4;int i = 0;for (i = 0; i < iterator; i++) {String tempData = data.substring(i * 4 + 0, i * 4 + 4);int[] tempByte = strToBt(tempData);int[] encByte = null;if(firstKey != null&& firstKey != "" && secondKey != null&& secondKey != "" && thirdKey != null&& thirdKey != "") {int x, y, z;tempBt = tempByte;for (x = 0; x < firstLength; x++) {tempBt = enc(tempBt, (int[])firstKeyBt.get(x));}for (y = 0; y < secondLength; y++) {tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));}for (z = 0; z < thirdLength; z++) {tempBt = enc(tempBt, (int[])thirdKeyBt.get(z));}encByte = tempBt;} else {if (firstKey != null && firstKey != ""&& secondKey != null&& secondKey != "") {int[] tempBt;int x, y;tempBt = tempByte;for (x = 0; x < firstLength; x++) {tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));}for (y = 0; y < secondLength; y++) {tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));}encByte = tempBt;} else {if (firstKey != null && firstKey != "") {int[] tempBt;int x;tempBt = tempByte;for (x = 0; x < firstLength; x++) { tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));}encByte = tempBt;}}}encData += bt64ToHex(encByte);}if (remainder > 0) {String remainderData = data.substring(iterator * 4 + 0,leng);int[] tempByte = strToBt(remainderData);int[] encByte = null;if(firstKey != null&& firstKey != "" && secondKey != null&& secondKey != "" && thirdKey != null&& thirdKey != "") {int[] tempBt;int x, y, z;tempBt = tempByte;for (x = 0; x < firstLength; x++) {tempBt = enc(tempBt, (int[])firstKeyBt.get(x));}for (y = 0; y < secondLength; y++) {tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));}for (z = 0; z < thirdLength; z++) {tempBt = enc(tempBt, (int[])thirdKeyBt.get(z));}encByte = tempBt;} else {if (firstKey != null && firstKey != ""&& secondKey != null&& secondKey != "") {int[] tempBt;int x, y;tempBt = tempByte;for (x = 0; x < firstLength; x++) {tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));}for (y = 0; y < secondLength; y++) {tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));}encByte = tempBt;} else {if (firstKey != null && firstKey != "") {int[] tempBt;int x;tempBt = tempByte;for (x = 0; x < firstLength; x++) { tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));}encByte = tempBt;}}}encData += bt64ToHex(encByte);}}}return encData;}/** decrypt the encrypted string to the original string** return the original string*/public String strDec(String data, String firstKey, String secondKey, String thirdKey) {int leng = data.length();String decStr = "";List firstKeyBt = null, secondKeyBt = null, thirdKeyBt = null;int firstLength = 0, secondLength = 0, thirdLength = 0;if (firstKey != null && firstKey != "") {firstKeyBt = getKeyBytes(firstKey);firstLength = firstKeyBt.size();}if (secondKey != null && secondKey != "") {secondKeyBt = getKeyBytes(secondKey);secondLength = secondKeyBt.size();}if (thirdKey != null && thirdKey != "") {thirdKeyBt = getKeyBytes(thirdKey);thirdLength = thirdKeyBt.size();}int iterator = leng / 16;int i = 0;for (i = 0; i < iterator; i++) {String tempData = data.substring(i * 16 + 0, i * 16 + 16); String strByte = hexToBt64(tempData);int[] intByte = new int[64];int j = 0;for (j = 0; j < 64; j++) {intByte[j] = Integer.parseInt(strByte.substring(j, j + 1));}int[] decByte = null;if (firstKey != null && firstKey != "" && secondKey != null && secondKey != "" && thirdKey != null&& thirdKey != "") {int[] tempBt;int x, y, z;tempBt = intByte;for (x = thirdLength - 1; x >= 0; x--) {tempBt = dec(tempBt, (int[]) thirdKeyBt.get(x)); }for (y = secondLength - 1; y >= 0; y--) {tempBt = dec(tempBt, (int[]) secondKeyBt.get(y)); }for (z = firstLength - 1; z >= 0; z--) {tempBt = dec(tempBt, (int[]) firstKeyBt.get(z)); }decByte = tempBt;} else {if (firstKey != null && firstKey != "" && secondKey != null&& secondKey != "") {int[] tempBt;int x, y, z;tempBt = intByte;for (x = secondLength - 1; x >= 0; x--) {tempBt = dec(tempBt, (int[])secondKeyBt.get(x));}for (y = firstLength - 1; y >= 0; y--) {tempBt = dec(tempBt, (int[])firstKeyBt.get(y));}decByte = tempBt;} else {if (firstKey != null && firstKey != "") {int[] tempBt;int x, y, z;tempBt = intByte;for (x = firstLength - 1; x >= 0; x--) {tempBt = dec(tempBt, (int[])firstKeyBt.get(x));}decByte = tempBt;}}}decStr += byteToString(decByte);}return decStr;}/** chang the string into the bit array** return bit array(it's length % 64 = 0)*/public List getKeyBytes(String key) {List keyBytes = new ArrayList();int leng = key.length();int iterator = (leng / 4);int remainder = leng % 4;int i = 0;for (i = 0; i < iterator; i++) {keyBytes.add(i, strToBt(key.substring(i * 4 + 0, i * 4 + 4))); }if (remainder > 0) {// keyBytes[i] = strToBt(key.substring(i*4+0,leng));keyBytes.add(i, strToBt(key.substring(i * 4 + 0, leng))); }return keyBytes;}/** chang the string(it's length <= 4) into the bit array ** return bit array(it's length = 64)*/public int[] strToBt(String str) {int leng = str.length();int[] bt = new int[64];if (leng < 4) {int i = 0, j = 0, p = 0, q = 0;for (i = 0; i < leng; i++) {int k = str.charAt(i);for (j = 0; j < 16; j++) {int pow = 1, m = 0;for (m = 15; m > j; m--) {pow *= 2;}// bt.set(16*i+j,""+(k/pow)%2));bt[16 * i + j] = (k / pow) % 2;}}for (p = leng; p < 4; p++) {int k = 0;for (q = 0; q < 16; q++) {int pow = 1, m = 0;for (m = 15; m > q; m--) {pow *= 2;}// bt[16*p+q]=parseInt(k/pow)%2;// bt.add(16*p+q,""+((k/pow)%2));bt[16 * p + q] = (k / pow) % 2;}}} else {for (int i = 0; i < 4; i++) {int k = str.charAt(i);for (int j = 0; j < 16; j++) {int pow = 1;for (int m = 15; m > j; m--) {pow *= 2;}// bt[16*i+j]=parseInt(k/pow)%2;// bt.add(16*i+j,""+((k/pow)%2));bt[16 * i + j] = (k / pow) % 2;}}}return bt;}/** chang the bit(it's length = 4) into the hex** return hex*/public String bt4ToHex(String binary) {String hex = "";if (binary.equalsIgnoreCase("0000")) {hex = "0";} else if (binary.equalsIgnoreCase("0001")) { hex = "1";} else if (binary.equalsIgnoreCase("0010")) { hex = "2";} else if (binary.equalsIgnoreCase("0011")) { hex = "3";} else if (binary.equalsIgnoreCase("0100")) { hex = "4";} else if (binary.equalsIgnoreCase("0101")) { hex = "5";} else if (binary.equalsIgnoreCase("0110")) { hex = "6";} else if (binary.equalsIgnoreCase("0111")) { hex = "7";} else if (binary.equalsIgnoreCase("1000")) { hex = "8";} else if (binary.equalsIgnoreCase("1001")) { hex = "9";} else if (binary.equalsIgnoreCase("1010")) { hex = "A";} else if (binary.equalsIgnoreCase("1011")) { hex = "B";} else if (binary.equalsIgnoreCase("1100")) { hex = "C";} else if (binary.equalsIgnoreCase("1101")) { hex = "D";} else if (binary.equalsIgnoreCase("1110")) { hex = "E";} else if (binary.equalsIgnoreCase("1111")) { hex = "F";}return hex;}/** chang the hex into the bit(it's length = 4) ** return the bit(it's length = 4)*/public String hexToBt4(String hex) {String binary = "";if (hex.equalsIgnoreCase("0")) {binary = "0000";} else if (hex.equalsIgnoreCase("1")) {binary = "0001";}if (hex.equalsIgnoreCase("2")) {binary = "0010";}if (hex.equalsIgnoreCase("3")) {binary = "0011";}if (hex.equalsIgnoreCase("4")) {binary = "0100";}if (hex.equalsIgnoreCase("5")) {binary = "0101";}if (hex.equalsIgnoreCase("6")) {binary = "0110";}if (hex.equalsIgnoreCase("7")) {binary = "0111";}if (hex.equalsIgnoreCase("8")) {binary = "1000";}if (hex.equalsIgnoreCase("9")) {binary = "1001";}if (hex.equalsIgnoreCase("A")) {binary = "1010";}if (hex.equalsIgnoreCase("B")) {binary = "1011";}if (hex.equalsIgnoreCase("C")) {binary = "1100";}if (hex.equalsIgnoreCase("D")) {binary = "1101";}if (hex.equalsIgnoreCase("E")) {binary = "1110";}if (hex.equalsIgnoreCase("F")) {binary = "1111";}return binary;}/** chang the bit(it's length = 64) into the string ** return string*/public String byteToString(int[] byteData) {String str = "";for (int i = 0; i < 4; i++) {int count = 0;for (int j = 0; j < 16; j++) {int pow = 1;for (int m = 15; m > j; m--) {pow *= 2;}count += byteData[16 * i + j] * pow; }if (count != 0) {str += "" + (char) (count);}}return str;}public String bt64ToHex(int[] byteData) {String hex = "";for (int i = 0; i < 16; i++) {String bt = "";for (int j = 0; j < 4; j++) {bt += byteData[i * 4 + j];}hex += bt4ToHex(bt);}return hex;}public String hexToBt64(String hex) {String binary = "";for (int i = 0; i < 16; i++) {binary += hexToBt4(hex.substring(i, i + 1));}return binary;}/** the 64 bit des core arithmetic*/public int[] enc(int[] dataByte, int[] keyByte) {int[][] keys = generateKeys(keyByte);int[] ipByte = initPermute(dataByte);int[] ipLeft = new int[32];int[] ipRight = new int[32];int[] tempLeft = new int[32];int i = 0, j = 0, k = 0, m = 0, n = 0;for (k = 0; k < 32; k++) {ipLeft[k] = ipByte[k];ipRight[k] = ipByte[32 + k];}for (i = 0; i < 16; i++) {for (j = 0; j < 32; j++) {tempLeft[j] = ipLeft[j];ipLeft[j] = ipRight[j];}int[] key = new int[48];for (m = 0; m < 48; m++) {key[m] = keys[i][m];}int[] tempRight = xor(pPermute(sBoxPermute(xor(expandPermute(ipRight), key))), tempLeft);for (n = 0; n < 32; n++) {ipRight[n] = tempRight[n];}}int[] finalData = new int[64];for (i = 0; i < 32; i++) {finalData[i] = ipRight[i];finalData[32 + i] = ipLeft[i];}return finallyPermute(finalData);}public int[] dec(int[] dataByte, int[] keyByte) {int[][] keys = generateKeys(keyByte);int[] ipByte = initPermute(dataByte);int[] ipLeft = new int[32];int[] ipRight = new int[32];int[] tempLeft = new int[32];int i = 0, j = 0, k = 0, m = 0, n = 0;for (k = 0; k < 32; k++) {ipLeft[k] = ipByte[k];ipRight[k] = ipByte[32 + k];}for (i = 15; i >= 0; i--) {for (j = 0; j < 32; j++) {tempLeft[j] = ipLeft[j];ipLeft[j] = ipRight[j];}int[] key = new int[48];for (m = 0; m < 48; m++) {key[m] = keys[i][m];}int[] tempRight = xor(pPermute(sBoxPermute(xor(expandPermute(ipRight), key))), tempLeft);for (n = 0; n < 32; n++) {ipRight[n] = tempRight[n];}}int[] finalData = new int[64];for (i = 0; i < 32; i++) {finalData[i] = ipRight[i];finalData[32 + i] = ipLeft[i];}return finallyPermute(finalData);}public int[] initPermute(int[] originalData) {int[] ipByte = new int[64];int i = 0, m = 1, n = 0, j, k;for (i = 0, m = 1, n = 0; i < 4; i++, m += 2, n += 2) { for (j = 7, k = 0; j >= 0; j--, k++) {ipByte[i * 8 + k] = originalData[j * 8 + m];ipByte[i * 8 + k + 32] = originalData[j * 8 + n]; }}return ipByte;}public int[] expandPermute(int[] rightData) {int[] epByte = new int[48];int i, j;for (i = 0; i < 8; i++) {if (i == 0) {epByte[i * 6 + 0] = rightData[31];} else {epByte[i * 6 + 0] = rightData[i * 4 - 1];}epByte[i * 6 + 1] = rightData[i * 4 + 0];epByte[i * 6 + 2] = rightData[i * 4 + 1];epByte[i * 6 + 3] = rightData[i * 4 + 2];epByte[i * 6 + 4] = rightData[i * 4 + 3];if (i == 7) {epByte[i * 6 + 5] = rightData[0];} else {epByte[i * 6 + 5] = rightData[i * 4 + 4];}}return epByte;}public int[] xor(int[] byteOne, int[] byteTwo) {// var xorByte = new Array(byteOne.length);// for(int i = 0;i < byteOne.length; i ++){// xorByte[i] = byteOne[i] ^ byteTwo[i];// }// return xorByte;int[] xorByte = new int[byteOne.length];for (int i = 0; i < byteOne.length; i++) {xorByte[i] = byteOne[i] ^ byteTwo[i];}return xorByte;}public int[] sBoxPermute(int[] expandByte) {// var sBoxByte = new Array(32);int[] sBoxByte = new int[32];String binary = "";int[][] s1 = {{ 14, 4, 13, 1, 2, 15, 11, 8, 3, 10, 6, 12, 5, 9, 0, 7 }, { 0, 15, 7, 4, 14, 2, 13, 1, 10, 6, 12, 11, 9, 5, 3, 8 }, { 4, 1, 14, 8, 13, 6, 2, 11, 15, 12, 9, 7, 3, 10, 5, 0 }, { 15, 12, 8, 2, 4, 9, 1, 7, 5, 11, 3, 14, 10, 0, 6, 13 } };/* Table - s2 */int[][] s2 = {{ 15, 1, 8, 14, 6, 11, 3, 4, 9, 7, 2, 13, 12, 0, 5, 10 }, { 3, 13, 4, 7, 15, 2, 8, 14, 12, 0, 1, 10, 6, 9, 11, 5 }, { 0, 14, 7, 11, 10, 4, 13, 1, 5, 8, 12, 6, 9, 3, 2, 15 }, { 13, 8, 10, 1, 3, 15, 4, 2, 11, 6, 7, 12, 0, 5, 14, 9 } };/* Table - s3 */int[][] s3 = {{ 10, 0, 9, 14, 6, 3, 15, 5, 1, 13, 12, 7, 11, 4, 2, 8 }, { 13, 7, 0, 9, 3, 4, 6, 10, 2, 8, 5, 14, 12, 11, 15, 1 }, { 13, 6, 4, 9, 8, 15, 3, 0, 11, 1, 2, 12, 5, 10, 14, 7 }, { 1, 10, 13, 0, 6, 9, 8, 7, 4, 15, 14, 3, 11, 5, 2, 12 } };/* Table - s4 */int[][] s4 = {{ 7, 13, 14, 3, 0, 6, 9, 10, 1, 2, 8, 5, 11, 12, 4, 15 }, { 13, 8, 11, 5, 6, 15, 0, 3, 4, 7, 2, 12, 1, 10, 14, 9 }, { 10, 6, 9, 0, 12, 11, 7, 13, 15, 1, 3, 14, 5, 2, 8, 4 }, { 3, 15, 0, 6, 10, 1, 13, 8, 9, 4, 5, 11, 12, 7, 2, 14 } };/* Table - s5 */int[][] s5 = {{ 2, 12, 4, 1, 7, 10, 11, 6, 8, 5, 3, 15, 13, 0, 14, 9 }, { 14, 11, 2, 12, 4, 7, 13, 1, 5, 0, 15, 10, 3, 9, 8, 6 }, { 4, 2, 1, 11, 10, 13, 7, 8, 15, 9, 12, 5, 6, 3, 0, 14 },{ 11, 8, 12, 7, 1, 14, 2, 13, 6, 15, 0, 9, 10, 4, 5, 3 } };/* Table - s6 */int[][] s6 = {{ 12, 1, 10, 15, 9, 2, 6, 8, 0, 13, 3, 4, 14, 7, 5, 11 }, { 10, 15, 4, 2, 7, 12, 9, 5, 6, 1, 13, 14, 0, 11, 3, 8 }, { 9, 14, 15, 5, 2, 8, 12, 3, 7, 0, 4, 10, 1, 13, 11, 6 }, { 4, 3, 2, 12, 9, 5, 15, 10, 11, 14, 1, 7, 6, 0, 8, 13 } };/* Table - s7 */int[][] s7 = {{ 4, 11, 2, 14, 15, 0, 8, 13, 3, 12, 9, 7, 5, 10, 6, 1 }, { 13, 0, 11, 7, 4, 9, 1, 10, 14, 3, 5, 12, 2, 15, 8, 6 }, { 1, 4, 11, 13, 12, 3, 7, 14, 10, 15, 6, 8, 0, 5, 9, 2 }, { 6, 11, 13, 8, 1, 4, 10, 7, 9, 5, 0, 15, 14, 2, 3, 12 } };/* Table - s8 */int[][] s8 = {{ 13, 2, 8, 4, 6, 15, 11, 1, 10, 9, 3, 14, 5, 0, 12, 7 }, { 1, 15, 13, 8, 10, 3, 7, 4, 12, 5, 6, 11, 0, 14, 9, 2 }, { 7, 11, 4, 1, 9, 12, 14, 2, 0, 6, 10, 13, 15, 3, 5, 8 }, { 2, 1, 14, 7, 4, 10, 8, 13, 15, 12, 9, 0, 3, 5, 6, 11 } };for (int m = 0; m < 8; m++) {int i = 0, j = 0;i = expandByte[m * 6 + 0] * 2 + expandByte[m * 6 + 5];j = expandByte[m * 6 + 1] * 2 * 2 * 2 + expandByte[m * 6 + 2] * 2* 2 + expandByte[m * 6 + 3] * 2 + expandByte[m * 6 + 4];switch (m) {case 0:binary = getBoxBinary(s1[i][j]);break;case 1:binary = getBoxBinary(s2[i][j]);break;case 2:binary = getBoxBinary(s3[i][j]);break;case 3:binary = getBoxBinary(s4[i][j]);break;case 4:binary = getBoxBinary(s5[i][j]);break;case 5:binary = getBoxBinary(s6[i][j]);break;case 6:binary = getBoxBinary(s7[i][j]);break;case 7:binary = getBoxBinary(s8[i][j]);break;}sBoxByte[m * 4 + 0] = Integer.parseInt(binary.substring(0, 1));sBoxByte[m * 4 + 1] = Integer.parseInt(binary.substring(1, 2));sBoxByte[m * 4 + 2] = Integer.parseInt(binary.substring(2, 3));sBoxByte[m * 4 + 3] = Integer.parseInt(binary.substring(3, 4));}return sBoxByte;}public int[] pPermute(int[] sBoxByte) {int[] pBoxPermute = new int[32];pBoxPermute[0] = sBoxByte[15];pBoxPermute[1] = sBoxByte[6];pBoxPermute[2] = sBoxByte[19];pBoxPermute[3] = sBoxByte[20];pBoxPermute[4] = sBoxByte[28];pBoxPermute[5] = sBoxByte[11];pBoxPermute[6] = sBoxByte[27];pBoxPermute[7] = sBoxByte[16];pBoxPermute[8] = sBoxByte[0];pBoxPermute[9] = sBoxByte[14];pBoxPermute[10] = sBoxByte[22];pBoxPermute[11] = sBoxByte[25];pBoxPermute[12] = sBoxByte[4];pBoxPermute[13] = sBoxByte[17];pBoxPermute[14] = sBoxByte[30];pBoxPermute[15] = sBoxByte[9];pBoxPermute[16] = sBoxByte[1];pBoxPermute[17] = sBoxByte[7];pBoxPermute[18] = sBoxByte[23];pBoxPermute[19] = sBoxByte[13];pBoxPermute[20] = sBoxByte[31];pBoxPermute[21] = sBoxByte[26];pBoxPermute[22] = sBoxByte[2];pBoxPermute[23] = sBoxByte[8];pBoxPermute[24] = sBoxByte[18];pBoxPermute[25] = sBoxByte[12];pBoxPermute[26] = sBoxByte[29];pBoxPermute[27] = sBoxByte[5];pBoxPermute[28] = sBoxByte[21];pBoxPermute[29] = sBoxByte[10];pBoxPermute[30] = sBoxByte[3];pBoxPermute[31] = sBoxByte[24];return pBoxPermute;}public int[] finallyPermute(int[] endByte) { int[] fpByte = new int[64];fpByte[0] = endByte[39];fpByte[1] = endByte[7];fpByte[2] = endByte[47];fpByte[3] = endByte[15];fpByte[4] = endByte[55];fpByte[5] = endByte[23];fpByte[6] = endByte[63];fpByte[7] = endByte[31];fpByte[8] = endByte[38];fpByte[9] = endByte[6];fpByte[10] = endByte[46];fpByte[11] = endByte[14];fpByte[12] = endByte[54];fpByte[13] = endByte[22];fpByte[14] = endByte[62];fpByte[15] = endByte[30];fpByte[16] = endByte[37];fpByte[17] = endByte[5];fpByte[18] = endByte[45];fpByte[19] = endByte[13];fpByte[20] = endByte[53];fpByte[21] = endByte[21];fpByte[22] = endByte[61];fpByte[23] = endByte[29];fpByte[24] = endByte[36];fpByte[25] = endByte[4];fpByte[26] = endByte[44];fpByte[27] = endByte[12];fpByte[28] = endByte[52];fpByte[29] = endByte[20];fpByte[30] = endByte[60];fpByte[31] = endByte[28];fpByte[32] = endByte[35];fpByte[33] = endByte[3];fpByte[34] = endByte[43];fpByte[35] = endByte[11];fpByte[36] = endByte[51];fpByte[37] = endByte[19];fpByte[38] = endByte[59];fpByte[39] = endByte[27];fpByte[40] = endByte[34];fpByte[41] = endByte[2];fpByte[42] = endByte[42];fpByte[43] = endByte[10];fpByte[44] = endByte[50];fpByte[45] = endByte[18];fpByte[46] = endByte[58];fpByte[47] = endByte[26];fpByte[48] = endByte[33];fpByte[49] = endByte[1];fpByte[50] = endByte[41];fpByte[51] = endByte[9];fpByte[52] = endByte[49];fpByte[53] = endByte[17];fpByte[54] = endByte[57];fpByte[55] = endByte[25];fpByte[56] = endByte[32];fpByte[57] = endByte[0];fpByte[58] = endByte[40];fpByte[59] = endByte[8];fpByte[60] = endByte[48];fpByte[61] = endByte[16];fpByte[62] = endByte[56];fpByte[63] = endByte[24];return fpByte;}public String getBoxBinary(int i) { String binary = "";switch (i) {case 0:binary = "0000";break;case 1:binary = "0001";break;case 2:binary = "0010";break;case 3:binary = "0011";break;case 4:binary = "0100";break;case 5:binary = "0101";break;case 6:binary = "0110";break;case 7:binary = "0111";break;case 8:binary = "1000";break;case 9:binary = "1001";break;case 10:binary = "1010";break;case 11:binary = "1011";break;case 12:binary = "1100";break;case 13:binary = "1101";break;case 14:break;case 15:binary = "1111";break;}return binary;}/** generate 16 keys for xor**/public int[][] generateKeys(int[] keyByte) {int[] key = new int[56];int[][] keys = new int[16][48];// keys[ 0] = new Array();// keys[ 1] = new Array();// keys[ 2] = new Array();// keys[ 3] = new Array();// keys[ 4] = new Array();// keys[ 5] = new Array();// keys[ 6] = new Array();// keys[ 7] = new Array();// keys[ 8] = new Array();// keys[ 9] = new Array();// keys[10] = new Array();// keys[11] = new Array();// keys[12] = new Array();// keys[13] = new Array();// keys[14] = new Array();// keys[15] = new Array();int[] loop = new int[] { 1, 1, 2, 2, 2, 2, 2, 2, 1, 2, 2, 2, 2, 2, 2, 1 };for (int i = 0; i < 7; i++) {for (int j = 0, k = 7; j < 8; j++, k--) {key[i * 8 + j] = keyByte[8 * k + i];}}int i = 0;for (i = 0; i < 16; i++) {int tempRight = 0;for (int j = 0; j < loop[i]; j++) { tempLeft = key[0];tempRight = key[28];for (int k = 0; k < 27; k++) { key[k] = key[k + 1];key[28 + k] = key[29 + k]; }key[27] = tempLeft;key[55] = tempRight;}// var tempKey = new Array(48);int[] tempKey = new int[48];tempKey[0] = key[13];tempKey[1] = key[16];tempKey[2] = key[10];tempKey[3] = key[23];tempKey[4] = key[0];tempKey[5] = key[4];tempKey[6] = key[2];tempKey[7] = key[27];tempKey[8] = key[14];tempKey[9] = key[5];tempKey[10] = key[20];tempKey[11] = key[9];tempKey[12] = key[22];tempKey[13] = key[18];tempKey[14] = key[11];tempKey[15] = key[3];tempKey[16] = key[25];tempKey[17] = key[7];tempKey[18] = key[15];tempKey[19] = key[6];tempKey[20] = key[26];tempKey[21] = key[19];tempKey[22] = key[12];tempKey[23] = key[1];tempKey[24] = key[40];tempKey[25] = key[51];tempKey[26] = key[30];tempKey[27] = key[36];tempKey[28] = key[46];tempKey[29] = key[54];。
JAVA使用DES加密解密
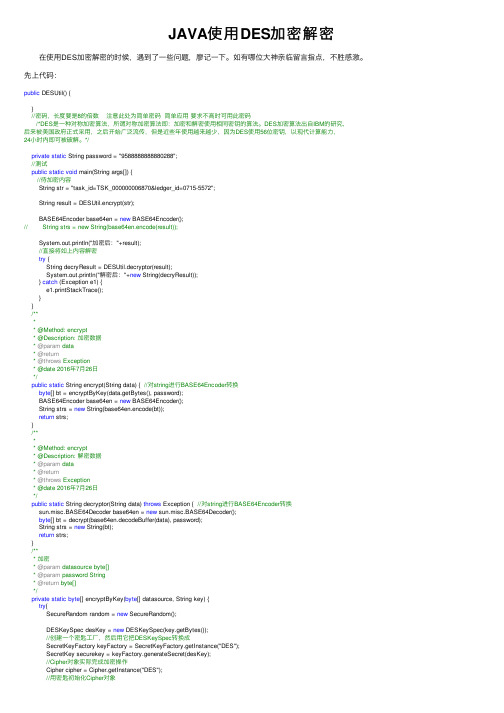
JAVA使⽤DES加密解密 在使⽤DES加密解密的时候,遇到了⼀些问题,廖记⼀下。
如有哪位⼤神亲临留⾔指点,不胜感激。
先上代码:public DESUtil() {}//密码,长度要是8的倍数注意此处为简单密码简单应⽤要求不⾼时可⽤此密码 /*DES是⼀种对称加密算法,所谓对称加密算法即:加密和解密使⽤相同密钥的算法。
DES加密算法出⾃IBM的研究,后来被美国政府正式采⽤,之后开始⼴泛流传,但是近些年使⽤越来越少,因为DES使⽤56位密钥,以现代计算能⼒,24⼩时内即可被破解。
*/private static String password = "9588888888880288";//测试public static void main(String args[]) {//待加密内容String str = "task_id=TSK_000000006870&ledger_id=0715-5572";String result = DESUtil.encrypt(str);BASE64Encoder base64en = new BASE64Encoder();// String strs = new String(base64en.encode(result));System.out.println("加密后:"+result);//直接将如上内容解密try {String decryResult = DESUtil.decryptor(result);System.out.println("解密后:"+new String(decryResult));} catch (Exception e1) {e1.printStackTrace();}}/**** @Method: encrypt* @Description: 加密数据* @param data* @return* @throws Exception* @date 2016年7⽉26⽇*/public static String encrypt(String data) { //对string进⾏BASE64Encoder转换byte[] bt = encryptByKey(data.getBytes(), password);BASE64Encoder base64en = new BASE64Encoder();String strs = new String(base64en.encode(bt));return strs;}/**** @Method: encrypt* @Description: 解密数据* @param data* @return* @throws Exception* @date 2016年7⽉26⽇*/public static String decryptor(String data) throws Exception { //对string进⾏BASE64Encoder转换sun.misc.BASE64Decoder base64en = new sun.misc.BASE64Decoder();byte[] bt = decrypt(base64en.decodeBuffer(data), password);String strs = new String(bt);return strs;}/*** 加密* @param datasource byte[]* @param password String* @return byte[]*/private static byte[] encryptByKey(byte[] datasource, String key) {try{SecureRandom random = new SecureRandom();DESKeySpec desKey = new DESKeySpec(key.getBytes());//创建⼀个密匙⼯⼚,然后⽤它把DESKeySpec转换成SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES");SecretKey securekey = keyFactory.generateSecret(desKey);//Cipher对象实际完成加密操作Cipher cipher = Cipher.getInstance("DES");//⽤密匙初始化Cipher对象cipher.init(Cipher.ENCRYPT_MODE, securekey, random);//现在,获取数据并加密//正式执⾏加密操作return cipher.doFinal(datasource);}catch(Throwable e){e.printStackTrace();}return null;}/*** 解密* @param src byte[]* @param password String* @return byte[]* @throws Exception*/private static byte[] decrypt(byte[] src, String key) throws Exception {// DES算法要求有⼀个可信任的随机数源SecureRandom random = new SecureRandom();// 创建⼀个DESKeySpec对象DESKeySpec desKey = new DESKeySpec(key.getBytes());// 创建⼀个密匙⼯⼚SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES");// 将DESKeySpec对象转换成SecretKey对象SecretKey securekey = keyFactory.generateSecret(desKey);// Cipher对象实际完成解密操作Cipher cipher = Cipher.getInstance("DES");// ⽤密匙初始化Cipher对象cipher.init(Cipher.DECRYPT_MODE, securekey, random);// 真正开始解密操作return cipher.doFinal(src);}解密过程中总有各种异常,有的说 SecureRandom 有问题需要换个⽅式⽣产随机数。
在JAVA中使用DES算法
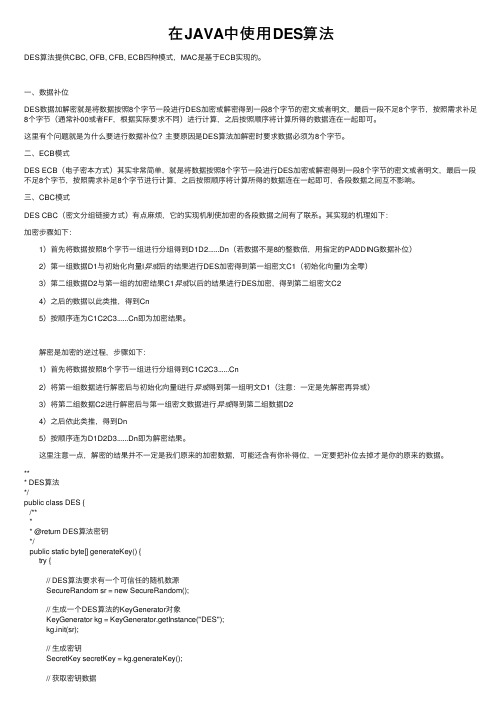
在JAVA中使⽤DES算法DES算法提供CBC, OFB, CFB, ECB四种模式,MAC是基于ECB实现的。
⼀、数据补位DES数据加解密就是将数据按照8个字节⼀段进⾏DES加密或解密得到⼀段8个字节的密⽂或者明⽂,最后⼀段不⾜8个字节,按照需求补⾜8个字节(通常补00或者FF,根据实际要求不同)进⾏计算,之后按照顺序将计算所得的数据连在⼀起即可。
这⾥有个问题就是为什么要进⾏数据补位?主要原因是DES算法加解密时要求数据必须为8个字节。
⼆、ECB模式DES ECB(电⼦密本⽅式)其实⾮常简单,就是将数据按照8个字节⼀段进⾏DES加密或解密得到⼀段8个字节的密⽂或者明⽂,最后⼀段不⾜8个字节,按照需求补⾜8个字节进⾏计算,之后按照顺序将计算所得的数据连在⼀起即可,各段数据之间互不影响。
三、CBC模式DES CBC(密⽂分组链接⽅式)有点⿇烦,它的实现机制使加密的各段数据之间有了联系。
其实现的机理如下:加密步骤如下:1)⾸先将数据按照8个字节⼀组进⾏分组得到D1D2......Dn(若数据不是8的整数倍,⽤指定的PADDING数据补位)2)第⼀组数据D1与初始化向量I异或后的结果进⾏DES加密得到第⼀组密⽂C1(初始化向量I为全零)3)第⼆组数据D2与第⼀组的加密结果C1异或以后的结果进⾏DES加密,得到第⼆组密⽂C24)之后的数据以此类推,得到Cn5)按顺序连为即为加密结果。
解密是加密的逆过程,步骤如下:1)⾸先将数据按照8个字节⼀组进⾏分组得到2)将第⼀组数据进⾏解密后与初始化向量I进⾏异或得到第⼀组明⽂D1(注意:⼀定是先解密再异或)3)将第⼆组数据C2进⾏解密后与第⼀组密⽂数据进⾏异或得到第⼆组数据D24)之后依此类推,得到Dn5)按顺序连为D1D2D3......Dn即为解密结果。
这⾥注意⼀点,解密的结果并不⼀定是我们原来的加密数据,可能还含有你补得位,⼀定要把补位去掉才是你的原来的数据。
java实现DES加密解密算法

java实现DES加密解密算法DES算法的⼊⼝参数有三个:Key、Data、Mode。
其中Key为8个字节共64位,是DES算法的⼯作密钥;Data也为8个字节64位,是要被加密或被解密的数据;Mode为DES的⼯作⽅式,有两种:加密或解密。
DES算法是这样⼯作的:如Mode为加密,则⽤Key 去把数据Data进⾏加密,⽣成Data的密码形式(64位)作为DES的输出结果;如 Mode为解密,则⽤Key去把密码形式的数据Data解密,还原为Data的明码形式(64位)作为DES的输出结果。
在通信⽹络的两端,双⽅约定⼀致的Key,在通信的源点⽤Key对核⼼数据进⾏DES加密,然后以密码形式在公共通信⽹(如电话⽹)中传输到通信⽹络的终点,数据到达⽬的地后,⽤同样的 Key对密码数据进⾏解密,便再现了明码形式的核⼼数据。
这样,便保证了核⼼数据(如PIN、MAC等)在公共通信⽹中传输的安全性和可靠性。
通过定期在通信⽹络的源端和⽬的端同时改⽤新的Key,便能更进⼀步提⾼数据的保密性,这正是现在⾦融交易⽹络的流⾏做法。
下⾯是具体代码:(切记切记字符串转字节或字节转字符串时⼀定要加上编码,否则可能出现乱码)1import java.io.IOException;2import java.security.SecureRandom;34import javax.crypto.Cipher;5import javax.crypto.SecretKey;6import javax.crypto.SecretKeyFactory;7import javax.crypto.spec.DESKeySpec;89import sun.misc.BASE64Decoder;10import sun.misc.BASE64Encoder;1112/**13 * DES加密解密算法14 *15 * @author lifq16 * @date 2015-3-17 上午10:12:1117*/18public class DesUtil {1920private final static String DES = "DES";21private final static String ENCODE = "GBK";22private final static String defaultKey = "test1234";2324public static void main(String[] args) throws Exception {25 String data = "测试ss";26// System.err.println(encrypt(data, key));27// System.err.println(decrypt(encrypt(data, key), key));28 System.out.println(encrypt(data));29 System.out.println(decrypt(encrypt(data)));3031 }3233/**34 * 使⽤默认key 加密35 *36 * @return String37 * @author lifq38 * @date 2015-3-17 下午02:46:4339*/41byte[] bt = encrypt(data.getBytes(ENCODE), defaultKey.getBytes(ENCODE));42 String strs = new BASE64Encoder().encode(bt);43return strs;44 }4546/**47 * 使⽤默认key 解密48 *49 * @return String50 * @author lifq51 * @date 2015-3-17 下午02:49:5252*/53public static String decrypt(String data) throws IOException, Exception {54if (data == null)55return null;56 BASE64Decoder decoder = new BASE64Decoder();57byte[] buf = decoder.decodeBuffer(data);58byte[] bt = decrypt(buf, defaultKey.getBytes(ENCODE));59return new String(bt, ENCODE);60 }6162/**63 * Description 根据键值进⾏加密64 *65 * @param data66 * @param key67 * 加密键byte数组68 * @return69 * @throws Exception70*/71public static String encrypt(String data, String key) throws Exception {72byte[] bt = encrypt(data.getBytes(ENCODE), defaultKey.getBytes(ENCODE));73 String strs = new BASE64Encoder().encode(bt);74return strs;75 }7677/**78 * Description 根据键值进⾏解密79 *80 * @param data81 * @param key82 * 加密键byte数组83 * @return84 * @throws IOException85 * @throws Exception86*/87public static String decrypt(String data, String key) throws IOException,88 Exception {89if (data == null)90return null;91 BASE64Decoder decoder = new BASE64Decoder();92byte[] buf = decoder.decodeBuffer(data);93byte[] bt = decrypt(buf, key.getBytes(ENCODE));94return new String(bt, ENCODE);95 }9698 * Description 根据键值进⾏加密99 *100 * @param data101 * @param key102 * 加密键byte数组103 * @return104 * @throws Exception105*/106private static byte[] encrypt(byte[] data, byte[] key) throws Exception { 107// ⽣成⼀个可信任的随机数源108 SecureRandom sr = new SecureRandom();109110// 从原始密钥数据创建DESKeySpec对象111 DESKeySpec dks = new DESKeySpec(key);112113// 创建⼀个密钥⼯⼚,然后⽤它把DESKeySpec转换成SecretKey对象114 SecretKeyFactory keyFactory = SecretKeyFactory.getInstance(DES); 115 SecretKey securekey = keyFactory.generateSecret(dks);116117// Cipher对象实际完成加密操作118 Cipher cipher = Cipher.getInstance(DES);119120// ⽤密钥初始化Cipher对象121 cipher.init(Cipher.ENCRYPT_MODE, securekey, sr);122123return cipher.doFinal(data);124 }125126/**127 * Description 根据键值进⾏解密128 *129 * @param data130 * @param key131 * 加密键byte数组132 * @return133 * @throws Exception134*/135private static byte[] decrypt(byte[] data, byte[] key) throws Exception { 136// ⽣成⼀个可信任的随机数源137 SecureRandom sr = new SecureRandom();138139// 从原始密钥数据创建DESKeySpec对象140 DESKeySpec dks = new DESKeySpec(key);141142// 创建⼀个密钥⼯⼚,然后⽤它把DESKeySpec转换成SecretKey对象143 SecretKeyFactory keyFactory = SecretKeyFactory.getInstance(DES); 144 SecretKey securekey = keyFactory.generateSecret(dks);145146// Cipher对象实际完成解密操作147 Cipher cipher = Cipher.getInstance(DES);148149// ⽤密钥初始化Cipher对象150 cipher.init(Cipher.DECRYPT_MODE, securekey, sr);151152return cipher.doFinal(data);153 }。
Java使用Hutool实现AES、DES加密解密的方法

Java使⽤Hutool实现AES、DES加密解密的⽅法在Java世界中,AES、DES加密解密需要使⽤Cipher对象构建加密解密系统,Hutool中对这⼀对象做再包装,简化了加密解密过程。
介绍AES和DES同属对称加密算法,数据发信⽅将明⽂(原始数据)和加密密钥⼀起经过特殊加密算法处理后,使其变成复杂的加密密⽂发送出去。
收信⽅收到密⽂后,若想解读原⽂,则需要使⽤加密⽤过的密钥及相同算法的逆算法对密⽂进⾏解密,才能使其恢复成可读明⽂。
在对称加密算法中,使⽤的密钥只有⼀个,发收信双⽅都使⽤这个密钥对数据进⾏加密和解密,这就要求解密⽅事先必须知道加密密钥。
在Java世界中,AES、DES加密解密需要使⽤Cipher对象构建加密解密系统,Hutool中对这⼀对象做再包装,简化了加密解密过程。
引⼊Hutool<dependency><groupId>com.xiaoleilu</groupId><artifactId>hutool-all</artifactId><version>3.0.9</version></dependency>使⽤AES加密解密String content = "test中⽂";//随机⽣成密钥byte[] key = SecureUtil.generateKey(SymmetricAlgorithm.AES.getValue()).getEncoded();//构建AES aes = SecureUtil.aes(key);//加密byte[] encrypt = aes.encrypt(content);//解密byte[] decrypt = aes.decrypt(encrypt);//加密为16进制表⽰String encryptHex = des.encryptHex(content);//解密为原字符串String decryptStr = des.decryptStr(encryptHex);DES加密解密DES的使⽤⽅式与AES基本⼀致String content = "test中⽂";//随机⽣成密钥byte[] key = SecureUtil.generateKey(SymmetricAlgorithm.DES.getValue()).getEncoded();//构建DES des = SecureUtil.des(key);//加密解密byte[] encrypt = des.encrypt(content);byte[] decrypt = des.decrypt(encrypt);//加密为16进制,解密为原字符串String encryptHex = des.encryptHex(content);String decryptStr = des.decryptStr(encryptHex);更多Hutool中针对JDK⽀持的所有对称加密算法做了封装,封装为SymmetricCrypto类,AES和DES两个类是此类的简化表⽰。
java相关加密解密方法

java相关加密解密方法Java加密解密方法是保护数据安全的重要手段,本文将详细介绍几种常见的Java加密解密方法。
一、对称加密算法对称加密算法是一种使用相同的密钥进行加密和解密的算法。
这种加密方式简单高效,但存在密钥管理的问题,因为所有用户都必须知道密钥。
在Java中,常用的对称加密算法有DES、3DES、AES等。
1. DES:Data Encryption Standard,数据加密标准,是一种使用56位密钥的对称块密码算法。
在Java中,我们可以使用javax.crypto.Cipher类来实现DES 加密解密。
2. 3DES:Triple Data Encryption Algorithm,三重数据加密算法,是DES的增强版本,使用三个不同的56位密钥进行三次加密。
在Java中,我们同样可以使用Cipher类来实现3DES加密解密。
3. AES:Advanced Encryption Standard,高级加密标准,是一种使用128、192或256位密钥的对称块密码算法。
在Java中,我们可以使用Cipher类来实现AES加密解密。
二、非对称加密算法非对称加密算法是一种使用一对密钥(公钥和私钥)进行加密和解密的算法。
公钥可以公开给所有人,而私钥需要保密。
在Java中,常用的非对称加密算法有RSA、DSA等。
1. RSA:Rivest-Shamir-Adleman,一种基于大数因子分解难题的非对称加密算法。
在Java中,我们可以使用java.security.KeyPairGenerator类生成RSA密钥对,然后使用Cipher类进行RSA加密解密。
2. DSA:Digital Signature Algorithm,数字签名算法,是一种基于整数有限域离散对数难题的非对称加密算法。
在Java中,我们可以使用KeyPairGenerator类生成DSA密钥对,然后使用Signature类进行DSA签名和验证。
java后台加密解密方法

java后台加密解密方法Java后台加密解密方法在当今互联网安全领域中具有重要意义。
为了保护数据的安全,各种加密算法应运而生。
本文将介绍几种常见的Java后台加密解密方法,包括RSA、AES、DES和SM2。
1.RSA加密解密原理及实现RSA是一种非对称加密算法,其公钥和私钥是成对存在的。
使用公钥加密后的数据只能通过私钥进行解密。
在Java中,我们可以使用KeyPairGenerator生成一对公私钥,然后使用RSAPrivateKey对数据进行解密。
2.AES加密解密原理及实现AES是一种对称加密算法,加密和解密过程使用相同的密钥。
在Java中,我们可以使用Java的加密库如Java Cryptography Extension (JCE)实现AES 加密解密。
3.DES加密解密原理及实现DES是一种对称加密算法,其加密过程和解密过程使用不同的密钥。
在Java中,我们可以使用Java Cryptography Extension (JCE)实现DES加密解密。
4.SM2加密解密原理及实现SM2是一种国密算法,具有非对称加密和对称加密的特点。
在前端,我们可以使用SM2加密请求参数,然后在后台使用对应的私钥进行解密。
在Java 中,我们可以使用Hutool库实现SM2加密解密。
5.总结:选择合适的加密解密方法及注意事项在实际应用中,选择合适的加密解密方法至关重要。
需要考虑数据安全性、算法复杂度、性能和兼容性等因素。
此外,还需注意以下几点:- 加密解密算法应根据实际需求进行选择,如对称加密算法适用于加密大量数据,非对称加密算法适用于加密少量数据;- 加密密钥和解密密钥应妥善保管,避免泄露;- 在传输加密数据时,应注意防范中间人攻击,可以使用SSL/TLS等安全协议进行保护;- 定期更新和升级加密算法,以应对潜在的安全威胁。
本文介绍了Java后台加密解密方法,包括RSA、AES、DES和SM2。
java加密解密desutil、tripledesutil
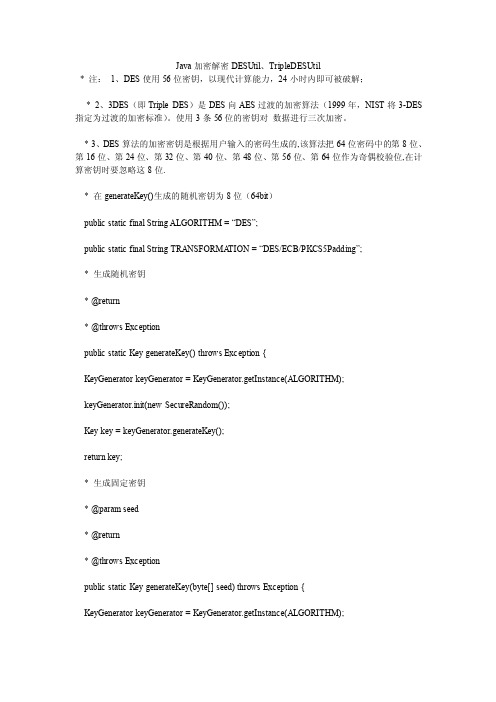
Java加密解密DESUtil、TripleDESUtil* 注:1、DES使用56位密钥,以现代计算能力,24小时内即可被破解;* 2、3DES(即Triple DES)是DES向AES过渡的加密算法(1999年,NIST将3-DES 指定为过渡的加密标准)。
使用3条56位的密钥对数据进行三次加密。
* 3、DES算法的加密密钥是根据用户输入的密码生成的,该算法把64位密码中的第8位、第16位、第24位、第32位、第40位、第48位、第56位、第64位作为奇偶校验位,在计算密钥时要忽略这8位.* 在generateKey()生成的随机密钥为8位(64bit)public static final String ALGORITHM = “DES”;public static final String TRANSFORMA TION = “DES/ECB/PKCS5Padding”;* 生成随机密钥* @return* @throws Exceptionpublic static Key generateKey() throws Exception {KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM);keyGenerator.init(new SecureRandom());Key key = keyGenerator.generateKey();return key;* 生成固定密钥* @param seed* @return* @throws Exceptionpublic static Key generateKey(byte[] seed) throws Exception {KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM);keyGenerator.init(new SecureRandom(seed));Key key = keyGenerator.generateKey();return key;* 生成固定密钥* @param password* @return* @throws Exceptionpublic static Key generateKey(String password) throws Exception {return generateKey(password.getBytes());* 执行加密* @param content* @param key 长度必须为8位,即64bit* @return* @throws Exceptionpublic static byte[] encrypt(byte[] content, byte[] key) throws Exception {Cipher cipher = Cipher.getInstance(TRANSFORMA TION);cipher.init(Cipher.ENCRYPT_MODE, new SecretKeySpec(key, ALGORITHM)); byte[] output = cipher.doFinal(content);return output;* 执行加密* @param content* @param password* @return* @throws Exceptionpublic static byte[] encrypt(byte[] content, String password) throws Exception { Cipher cipher = Cipher.getInstance(TRANSFORMA TION);cipher.init(Cipher.ENCRYPT_MODE, generateKey(password));byte[] output = cipher.doFinal(content);return output;* 执行解密* @param content* @param key 长度必须为8位,即64bit* @return* @throws Exceptionpublic static byte[] decrypt(byte[] content, byte[] key) throws Exception {Cipher cipher = Cipher.getInstance(TRANSFORMA TION);cipher.init(Cipher.DECRYPT_MODE, new SecretKeySpec(key, ALGORITHM)); byte[] output = cipher.doFinal(content);return output;* 执行解密* @param content* @param password* @return* @throws Exceptionpublic static byte[] decrypt(byte[] content, String password) throws Exception {Cipher cipher = Cipher.getInstance(TRANSFORMA TION);cipher.init(Cipher.DECRYPT_MODE, generateKey(password));byte[] output = cipher.doFinal(content);return output;public static void main(String[] args) throws Exception {System.out.println(Arrays.toString(encrypt(“DES使用56位密钥,以现代计算能力”.getBytes(), “012345”)));System.out.println(new String(decrypt(encrypt(“DES使用56位密钥,以现代计算能力”.getBytes(), “012345”), “012345”)));System.out.println(Arrays.toString(encrypt(“DES使用56位密钥,以现代计算能力”.getBytes(), “01234567”.getBytes())));System.out.println(new String(decrypt(encrypt(“DES使用56位密钥,以现代计算能力”.getBytes(), “01234567”.getBytes()), “01234567”.getBytes())));* 控制台输出:* [117, -109, -80, -75, 51, -86, -57, -109, -94, 58, 38, 94, 39, -107, -60, 65, -122, -7, -124, 2, -23, -30, -98, -64, 90, 26, 15, 82, 84, 102, -108, -3, -68, -4, 110, -86, -106, 19, -65, -110, 2, 15, 49, -79, -98, 38, -39, 6]* DES使用56位密钥,以现代计算能力* [-6, 63, -15, 73, -28, 85, 125, 64, 6, 55, -63, 99, 114, 21, 108, -49, 19, 11, -15, -126, 36, -92, 62, 112, -40, 64, -102, 127, -94, -53, -89, 33, 72, -20, -126, -90, 105, -37, -68, -46, -61, -36, 5, -103, 27, 32, 84, 28]* DES使用56位密钥,以现代计算能力[Java]代码import java.security.Key;import java.security.SecureRandom;import java.util.Arrays;import javax.crypto.Cipher;import javax.crypto.KeyGenerator;import javax.crypto.spec.SecretKeySpec;public sta tic final String ALGORITHM = “DESede”;public static final String TRANSFORMA TION = “DESede/ECB/PKCS5Padding”; * 生成随机密钥* @return* @throws Exceptionpublic static Key generateKey() throws Exception {KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM);keyGenerator.init(new SecureRandom());Key key = keyGenerator.generateKey();return key;* 生成固定密钥* @param seed* @return* @throws Exceptionpublic static Key generateKey(byte[] seed) throws Exception {KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM);keyGenerator.init(new SecureRandom(seed));Key key = keyGenerator.generateKey();return key;* 生成固定密钥* @param password* @return* @throws Exceptionpublic static Key generateKey(String password) throws Exception {return generateKey(password.getBytes());* 执行加密* @param content* @param key 长度必须为8位,即64bit* @return* @throws Exceptionpublic static byte[] encrypt(byte[] content, byte[] key) throws Exception {Cipher cipher = Cipher.getInstance(TRANSFORMA TION);cipher.init(Cipher.ENCRYPT_MODE, new SecretKeySpec(key, ALGORITHM)); byte[] output = cipher.doFinal(content);return output;* 执行加密* @param content* @param password* @return* @throws Exceptionpublic static byte[] encrypt(byte[] content, String password) throws Exception { Cipher cipher = Cipher.getInstance(TRANSFORMA TION);cipher.init(Cipher.ENCRYPT_MODE, generateKey(password));byte[] output = cipher.doFinal(content);return output;* 执行解密* @param content* @param key 长度必须为8位,即64bit* @return* @throws Exceptionpublic static byte[] decrypt(byte[] content, byte[] key) throws Exception {Cipher cipher = Cipher.getInstance(TRANSFORMA TION);cipher.init(Cipher.DECRYPT_MODE, new SecretKeySpec(key, ALGORITHM)); byte[] output = cipher.doFinal(content);return output;* 执行解密* @param content* @param password* @return* @throws Exceptionpublic static byte[] decrypt(byte[] content, String password) throws Exception {Cipher cipher = Cipher.getInstance(TRANSFORMA TION);cipher.init(Cipher.DECRYPT_MODE, generateKey(password));byte[] output = cipher.doFinal(content);return output;public static void main(String[] args) throws Exception {System.out.println(Arrays.toString(encrypt(“使用3条56位的密钥对数据进行三次加密。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
java des加密方法
Java中的DES加密方法是一种对称加密算法,它使用一个共享的密钥来加密和解密数据。
DES算法的密钥长度为56位,但由于其已被认为容易被破解,因此现在已经不再被广泛使用。
在Java中,我们可以使用Java Cryptography Extension (JCE)中的javax.crypto包中的类来执行DES加密和解密操作。
我们可以使用javax.crypto.KeyGenerator类来生成一个DES密钥,然后使用javax.crypto.Cipher类来执行加密和解密操作。
使用DES加密和解密数据的基本流程如下:
1. 创建一个javax.crypto.KeyGenerator对象,并使用DES算法初始化它。
2. 调用KeyGenerator的generateKey()方法生成一个密钥。
3. 创建一个javax.crypto.Cipher对象,并使用密钥初始化它。
4. 调用Cipher的doFinal()方法,将需要加密或解密的数据作为参数传递给它。
5. 将加密或解密后的数据作为结果返回。
在进行DES加密和解密操作时,我们需要注意以下几点:
1. 密钥长度必须为8个字节,即56位。
2. 加密和解密的数据必须是8个字节的倍数,如果不足8个字节,则需要使用填充方法填充。
3. 加密和解密使用的密钥必须相同,否则解密将失败。
在实际应用中,我们可以使用DES加密算法来保护敏感数据的安
全性,例如密码、信用卡号码等。
同时,我们也需要采取其他措施来保护数据传输的安全,例如使用SSL/TLS协议。