自动化外文翻译
自动化专业外文翻译---模糊逻辑控制机器人走迷宫
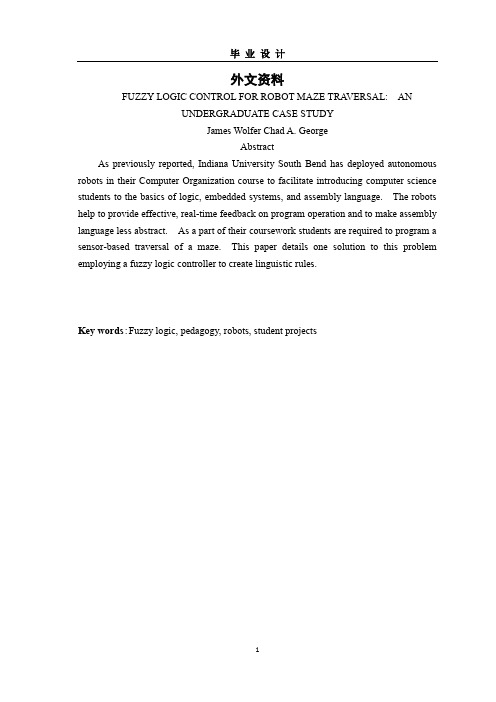
外文资料FUZZY LOGIC CONTROL FOR ROBOT MAZE TRA VERSAL: ANUNDERGRADUATE CASE STUDYJames Wolfer Chad A. GeorgeAbstractAs previously reported, Indiana University South Bend has deployed autonomous robots in their Computer Organization course to facilitate introducing computer science students to the basics of logic, embedded systems, and assembly language. The robots help to provide effective, real-time feedback on program operation and to make assembly language less abstract. As a part of their coursework students are required to program a sensor-based traversal of a maze. This paper details one solution to this problem employing a fuzzy logic controller to create linguistic rules.Key words:Fuzzy logic, pedagogy, robots, student projectsINTRODUCTIONAssembly language programming in a computer science environment is often taught using abstract exercises to illustrate concepts and encourage student proficiency.To augment this approach we have elected to provide hands-on, real-world experience to our students by introducing robots into our assembly language class.Observing the physical action of robots can generate valuable feedback and have real-world consequences – robots hitting walls make students instantly aware of program errors, for example.It also provides insight into the realities of physical machines such as motor control, sensor calibration, and noise. To help provide a meaningful experience for our computer organization students, we reviewed the course with the following objectives in mind:• Expand the experience of our students in a manner that enhances the student's insight, provides a hands-on, visual, environment for them to learn, and forms an integrated component for future classes.•Remove some of the abstraction inherent in the assembly language class. Specifically, to help enhance the error detection environment.• Provide a kinesthetic aspect to our pedagogy.• Build student expertise early in their program that could lead to research projects and advanced classroom activities later in their program. Specifically, in this case, to build expertise to support later coursework in intelligent systems and robotics.As one component in meeting these objectives we, in cooperation with the Computer Science department, the Intelligent Systems Laboratory, and the University Center for Excellence in Teaching, designed a robotics laboratory to support the assembly language portion of the computer organization class as described in [1].The balance of this report describes one example project resulting from this environment. Specifically, we describe the results of a student project developing an assembly language fuzzy engine, membership function creation, fuzzy controller, and resulting robot behavior in a Linux-based environment.We also describe subsequent software devlopment in C# under Windows, including graphical membership tuning, real-time display of sensor activation, and fuzzy controller system response. Collectively these tools allow for robust controller development, assemblylanguage support, and an environment suitable for effective classroom and publicdisplay.BACKGROUNDRobots have long been recognized for their potential educational utility, with examples ranging from abstract, simulated, robots, such as Karel[2] and Turtle[3] for teaching programming and geometry respectively, to competitive events such as robotic soccer tournaments[4].As the cost of robotics hardware has decreased their migration into the classroom has accelerated [5, 6]. Driven by the combined goals for this class and the future research objectives, as well as software availability, we chose to use off-the-shelf, Khepera II, robots from K-Team[7].SIMULATED ROBOT DIAGRAMThe K-Team Kephera II is a small, two-motor robot which uses differential wheel speed for steering. Figure 1 shows a functional diagram of the robot. In addition to thetwo motors it includes a series of eight infrared sensors, six along the “front” and two in the “back”of the robot. This robot also comes with an embedded system-call library, a variety of development tools, and the availability of several simulators. The embedded code in the Khepera robots includes a relatively simple, but adequate, command level interface which communicates with the host via a standard serial port. This allows students to write their programs using the host instruction set (Intel Pentium in this case), send commands, and receive responses such as sensor values, motor speed and relative wheel position.We also chose to provide a Linux-based programming environment to our students by adapting and remastering the Knoppix Linux distribution [9]. Our custom distribution supplemented Knoppix with modified simulators for the Khepera, the interface library (including source code),manuals, and assembler documentation. Collectively, this provides a complete development platform.The SIM Kheperasimulator[8] includes source code in C, and provides a workable subset of the native robot command language. It also has the ability to redirect input and output to the physical robot from the graphics display. Figure 2 shows the simulated Khepera robot in a maze environment and Figure 3 shows an actual Khepera in a physical maze. To provide a seamless interface to the simulator and robots we modified the original simulator to more effectively communicate through a pair of Linuxpipes, and we developed a small custom subroutine library callable from the student's assembly language programs.Assignments for the class range from initial C assignments to call the robot routines to assembly language assignments culminating in the robot traversing the maze. FUZZY CONTROLLEROne approach to robot control, fuzzy logic, attempts to encapsulate important aspects of human decision making. By forming a representation tolerant of vague, imprecise, ambiguous, and perhaps missing information fuzzy logic enhances the ability to deal with real-world problems. Furthermore, by empirically modeling a system engineering experience and intuition can be incorporated into a final design.Typical fuzzy controller design [10] consists of:• Defining the control objectives and criteria• Determining the input and output relationships• Creating fuzzy membership functions, along withsubsequent rules, to encapsulate a solution fromintput to output.• Apply necessary input/output conditioning• Test, evaluate, and tune the resulting system.Figure 4 illustrates the conversion from sensor input to a fuzzy-linguistic value. Given three fuzzy possibilities, …too close‟, …too far‟, and …just right‟, along with a sensor reading we can ascertain the degree to which the sensor reading belongs to each of these fuzzy terms. Note that while Figure 4 illustrates a triangular membership set, trapezoids and other shapes are also common.Once the inputs are mapped to their corresponding fuzzy sets the fuzzy attributes are used, expert system style, to trigger rules governing the consequent actions, in this case, of the robot.For example, a series of rules for a robot may include:• If left-sensor is too close and right sensor is too far then turn right.• If left sensor is just right and forward sensor is too far then drive straight.• If left sensor is too far and forward sensor is too far then turn left.• If forward sensor is close then turn right sharply.The logical operators …and‟, …or‟, and …not‟ are calculated as follows: …and‟ represents set intersection and is calculated as the minimum value, …or‟ is calculated as the maximum value or the union of the sets, and …not‟ finds the inverse of the set, calculated as 1.0-fitness.Once inputs have been processed and rules applied, the resulting fuzzy actions must be mapped to real-world control outputs. Figure 5 illustrates this process. Here output is computed as the coordinate of the centroid of the aggregate area of the individual membership sets along the horizontal axis.ASSEMBLY LANGUAGE IMPLEMENTATIONTwo implementations of the fuzzy robot controller were produced. The first was written in assembly language for the Intel cpu architecture under the Linux operating system, the second in C# under Windows to provide a visually intuitive interface for membership set design and public demonstration.Figure 6 shows an excerpt of pseudo-assembly language program. The actual program consists of approximately eight hundred lines of hand-coded assembly language. In the assembly language program subroutine calls are structured with parameters pushed onto the stack. Note that the code for pushing parameters has been edited from this example to conserve space and to illustrate the overall role of the controller. In this code-fragment the …open_pipes‟ routine establishes contact with the simulator or robot. Once communication is established, a continous loop obtains sensor values, encodes them as fuzzy inputs, interprets them through the rule base to linguistic output members which are then converted to control outputs which are sent to the robot. The bulk of the remaining code implements the fuzzy engine itself.FUZZY CONTROLLER MAIN LOOPMembership sets were manually defined to allow the robot to detect and track walls, avoid barriers, and negotiate void spaces in it field of operation. Using this controller, both the simulated robot and the actual Khepera successfully traversed a variety of maze configurations.ASSEMBLY LANGUAGE OBSERV ATIONSWhile implementing the input fuzzification and output defuzzification in assembly language was tedious compared with the same task in a high level language, the logic engine proved to be well suited to description in assembly language.The logic rules were defined in a type of psuedo-code using …and‟, …or‟, …not‟ as operators and using the fuzzy input and output membership sets as parameters. With the addition of input, output and flow control operators, the assembly language logic engine simply had to evaluate these psuedo-code expressions in order to map fuzzy inputs memberships to fuzzy output memberships.Other than storing the current membership fitness values from the inputfuzzyfication, the only data structure needed for the logic engine is a stack to hold intermediate calculations. This is convenient under assembly language since the CPUs stack is immediately available as well as the nescesary stack operators.There were seven commands implemented by the logic rule interpreter: IN, OUT, AND, OR, NOT, DONE, and EXIT.•IN – reads the current fitness from an input membership set and places the value on the stack.•OUT – assigns the value on the top of the stack as the fitness value of an output membership set if it is greater than the existing fitness value for that set.•AND – performs the intersection operation by replacing the top two elements on the stack with the minimum element.•OR – performs the union operation by replace the top two elements on the stack with their maximum.•NOT – replaces the top value on the stack with its compliment.•DONE – pops the top value off the stack to prepare for the next rule•EXIT – signals the end of the logic rule definition and exits the interpreter.As an example the logic rule “If left-sensor is too close and right sensor is too far then turn right”, might be define d by the following fuzzy logic psuedo-code: IN, left_sensor[ TOO_CLOSE ]IN, right_sensor[ TOO_FAR ] ANDOUT, left_wheel[ FWD ]OUT, right_wheel[ STOP ]DONEEXITBy utilizing the existing CPU stack and implementing the logic engine as anpsuedo-code interpreter, the assembly language version is capable of handling arbitrarily complicated fuzzy rules composed of the simple logical operators provided. IMPLEMENTATIONWhile the assembly language programming was the original focus of the project, ultimately we felt that a more polished user interface was desirable for membership set design, fuzzy rule definition, and controller response monitoring. To provide these facilities the fuzzy controller was reimplemented in C# under Windows. through 10 illustrate the capabilities of the resulting software. Specifically, Figure 7 illustrates user interface for membership defination, in this case …near‟. Figure 8 illustrates theinterface for defining the actual fuzzy rules. Figure 9 profiles the output response with respect to a series of simulated inputs. Finally, real-time monitoring of the system is also implemented as illustrated in 10 which shows the robot sensor input values.Since the Khepera simulator was operating system specific, the C# program controls the robot directly. Again, the robot was successful at navigating the maze using a controller specified with this interface.SUMMARYTo summarize, we have developed a student-centric development environment for teaching assembly language programming. As one illustration of its potential we profiled a project implementing a fuzzy-logic engine and controller, along with a subsequent implementation in the C# programming language. Together these projects help to illustrate the viability of a robot-enhanced environment for assembly language programming.REFERENCES[1] Wolfer, J &Rababaah, H. R. A., “Creating a Hands-On Robot Environment for Teaching Assembly Language Programming”, Global Conference on Engineering and Technology Education, 2005[2] Pattic R.E., Karel the Robot: a gentle introduction to the art of programming, 2nd edition. Wiley, 1994[3] Abelson H. and diSessa A., Turtle geometry: the computer as a medium for exploring mathematics. MIT Press, 1996[4] Amirijoo M., Tesanovic A., and Nadjm-Tehrani S., “Raising motivation in real-time laboratories: the soccer scenario” in SIGCSE Technical Symposium on Computer Sciences Education, pp. 265-269, 2004.[5] Epp E.C., “Robot control and embedded systems on inexpensive linux platforms workshop,” in SIGCSE Technical Symposium on Computer Science Education, p. 505, 2004[6] Fagin B. and Merkle L., “Measuring the effectiveness of robots in teaching computer science,” in SIGCSE Technical Symposium on Computer Science Education, PP. 307-311, 2003.[7] K-Team Khepera Robots, , accessed 09/06/05.[8] Michel O., “Khepera Simulator package version 2.0: Freeware mobile robot simulator written at the university of nice Sophia-Antipolis by Olivier Michel. Downloadable from the world wide web. http://diwww.epfl.ch/lami/team/michel/khep-sim, accessed 09/06/05.[9] Knoppix Official Site, , accessed 09/06/05.[10] Earl Cox., The Fuzzy Systems Handbook, Academic Press, New York, 1999.模糊逻辑控制机器人走迷宫James Wolfer Chad A. George摘要美国印第安纳大学南本德已部署在他们的计算机组织课程自主机器人,以方便学生介绍计算机科学逻辑的基础知识,嵌入式系统和汇编语言。
自动化专业英语翻译

自动化专业英语翻译A: Electrical NetworksAn electrical circuit or network is composed of elements such as resistors, inductors,and capacitors connected together in some manner. If the network contains no energy sources, such as batteries or electrical generators, it is known as a passive network.On the other hand, if one or more energy sources are present, the resultant combination is an active network. In studying the behavior of an electrical network, we are interested in determining the voltages and currents that exist within the circuit. Since a network is composed of passive circuit elements, we must first define theelectrical characteristics of these elements.一个电路由一些元件,如电阻、电感和电容,以某种方式连接组成。
如果电路中不包括电源,如电池或者发电机,则称为无源网络。
另一方面,如果电路中有一个或多个电源,称为有源网络。
在研究电路的行为中,确定存在于电路中的电压和电流是我们感兴趣的。
由于一个电路网络由无源元件组成,我们必须首先定义这些元件的电的特性。
自动化专业常用英语词汇
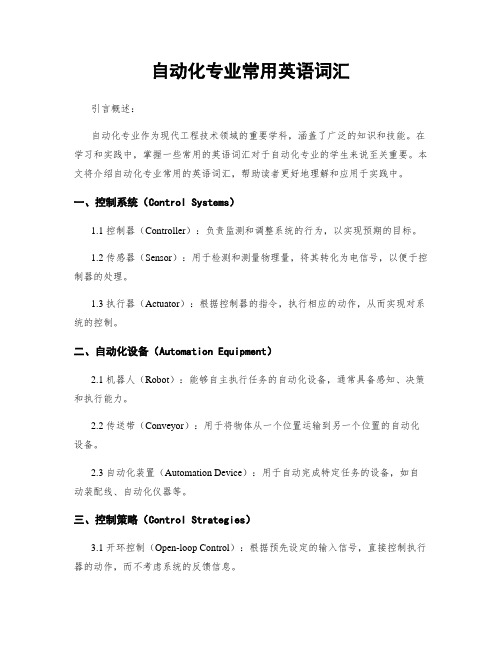
自动化专业常用英语词汇引言概述:自动化专业作为现代工程技术领域的重要学科,涵盖了广泛的知识和技能。
在学习和实践中,掌握一些常用的英语词汇对于自动化专业的学生来说至关重要。
本文将介绍自动化专业常用的英语词汇,帮助读者更好地理解和应用于实践中。
一、控制系统(Control Systems)1.1 控制器(Controller):负责监测和调整系统的行为,以实现预期的目标。
1.2 传感器(Sensor):用于检测和测量物理量,将其转化为电信号,以便于控制器的处理。
1.3 执行器(Actuator):根据控制器的指令,执行相应的动作,从而实现对系统的控制。
二、自动化设备(Automation Equipment)2.1 机器人(Robot):能够自主执行任务的自动化设备,通常具备感知、决策和执行能力。
2.2 传送带(Conveyor):用于将物体从一个位置运输到另一个位置的自动化设备。
2.3 自动化装置(Automation Device):用于自动完成特定任务的设备,如自动装配线、自动化仪器等。
三、控制策略(Control Strategies)3.1 开环控制(Open-loop Control):根据预先设定的输入信号,直接控制执行器的动作,而不考虑系统的反馈信息。
3.2 闭环控制(Closed-loop Control):根据系统的反馈信息,调整控制器的输出信号,以实现对系统的精确控制。
3.3 模糊控制(Fuzzy Control):基于模糊逻辑理论,将模糊的输入转化为模糊的输出,用于处理复杂的非线性系统。
四、通信协议(Communication Protocols)4.1 以太网(Ethernet):一种常用的局域网通信协议,用于实现设备之间的数据传输和通信。
4.2 控制网(ControlNet):一种用于工业自动化领域的网络通信协议,支持实时数据传输和设备控制。
4.3 无线通信(Wireless Communication):通过无线信号进行数据传输和通信的技术,如Wi-Fi、蓝牙等。
自动化专业英语全文翻译

《自动化专业英语教程》-王宏文主编-全文翻译PART 1Electrical and Electronic Engineering BasicsUNIT 1A Electrical Networks ————————————3B Three-phase CircuitsUNIT 2A The Operational Amplifier ———————————5B TransistorsUNIT 3A Logical Variables and Flip-flop ——————————8B Binary Number SystemUNIT 4A Power Semiconductor Devices ——————————11B Power Electronic ConvertersUNIT 5A Types of DC Motors —————————————15B Closed-loop Control of DC DriversUNIT 6A AC Machines ———————————————19B Induction Motor DriveUNIT 7A Electric Power System ————————————22B Power System AutomationPART 2Control TheoryUNIT 1A The World of Control ————————————27B The Transfer Function and the Laplace Transformation —————29 UNIT 2A Stability and the Time Response —————————30B Steady State—————————————————31 UNIT 3A The Root Locus —————————————32B The Frequency Response Methods: Nyquist Diagrams —————33 UNIT 4A The Frequency Response Methods: Bode Piots —————34B Nonlinear Control System 37UNIT 5 A Introduction to Modern Control Theory 38B State Equations 40UNIT 6 A Controllability, Observability, and StabilityB Optimum Control SystemsUNIT 7 A Conventional and Intelligent ControlB Artificial Neural NetworkPART 3 Computer Control TechnologyUNIT 1 A Computer Structure and Function 42B Fundamentals of Computer and Networks 43UNIT 2 A Interfaces to External Signals and Devices 44B The Applications of Computers 46UNIT 3 A PLC OverviewB PACs for Industrial Control, the Future of ControlUNIT 4 A Fundamentals of Single-chip Microcomputer 49B Understanding DSP and Its UsesUNIT 5 A A First Look at Embedded SystemsB Embedded Systems DesignPART 4 Process ControlUNIT 1 A A Process Control System 50B Fundamentals of Process Control 52UNIT 2 A Sensors and Transmitters 53B Final Control Elements and ControllersUNIT 3 A P Controllers and PI ControllersB PID Controllers and Other ControllersUNIT 4 A Indicating InstrumentsB Control PanelsPART 5 Control Based on Network and InformationUNIT 1 A Automation Networking Application AreasB Evolution of Control System ArchitectureUNIT 2 A Fundamental Issues in Networked Control SystemsB Stability of NCSs with Network-induced DelayUNIT 3 A Fundamentals of the Database SystemB Virtual Manufacturing—A Growing Trend in AutomationUNIT 4 A Concepts of Computer Integrated ManufacturingB Enterprise Resources Planning and BeyondPART 6 Synthetic Applications of Automatic TechnologyUNIT 1 A Recent Advances and Future Trends in Electrical Machine DriversB System Evolution in Intelligent BuildingsUNIT 2 A Industrial RobotB A General Introduction to Pattern RecognitionUNIT 3 A Renewable EnergyB Electric VehiclesUNIT 1A 电路电路或电网络由以某种方式连接的电阻器、电感器和电容器等元件组成。
自动化中英文对照表
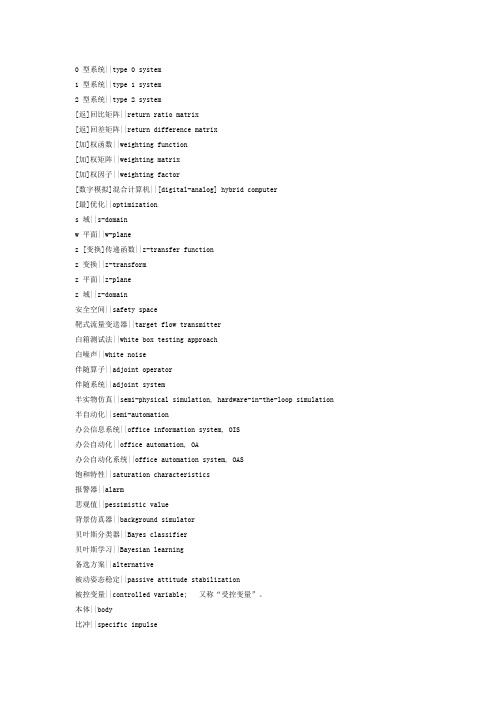
0 型系统||type 0 system1 型系统||type 1 system2 型系统||type 2 system[返]回比矩阵||return ratio matrix[返]回差矩阵||return difference matrix[加]权函数||weighting function[加]权矩阵||weighting matrix[加]权因子||weighting factor[数字模拟]混合计算机||[digital-analog] hybrid computer[最]优化||optimizations 域||s-domainw 平面||w-planez [变换]传递函数||z-transfer functionz 变换||z-transformz 平面||z-planez 域||z-domain安全空间||safety space靶式流量变送器||target flow transmitter白箱测试法||white box testing approach白噪声||white noise伴随算子||adjoint operator伴随系统||adjoint system半实物仿真||semi-physical simulation, hardware-in-the-loop simulation 半自动化||semi-automation办公信息系统||office information system, OIS办公自动化||office automation, OA办公自动化系统||office automation system, OAS饱和特性||saturation characteristics报警器||alarm悲观值||pessimistic value背景仿真器||background simulator贝叶斯分类器||Bayes classifier贝叶斯学习||Bayesian learning备选方案||alternative被动姿态稳定||passive attitude stabilization被控变量||controlled variable; 又称“受控变量”。
自动化专业-外文文献-英文文献-外文翻译-plc方面

1、外文原文(复印件)A: Fundamentals of Single-chip MicrocomputerTh e si ng le-ch i p mi cr oc om pu ter is t he c ul mi nat i on o f bo th t h e d ev el op me nt o f th e d ig it al com p ut er an d t he int e gr at ed ci rc ui ta r gu ab ly th e t ow m os t s i gn if ic ant i nv en ti on s o f t h e 20t h c en tu ry[1].Th es e to w t ype s o f a rc hi te ct ur e a re fo un d i n s i ng le—ch ip m i cr oc om pu te r。
S o me em pl oy th e s p li t p ro gr am/d at a me mo ry of t he H a rv ar d ar ch it ect u re, sh ow n in Fi g.3-5A—1,ot he r s fo ll ow t hep h il os op hy, wi del y a da pt ed f or ge n er al—pu rp os e c o mp ut er s an dm i cr op ro ce ss or s, of ma ki ng no lo gi c al di st in ct io n be tw ee n p ro gr am a n d da ta m em or y a s i n th e Pr in cet o n ar ch it ec tu re,sh ow n in F ig。
3-5A-2.In g en er al te r ms a s in gl e—ch i p mi cr oc om pu ter isc h ar ac te ri zed b y the i nc or po ra tio n of al l t he uni t s o f a co mp ut er i n to a s in gl e de v i ce,as s ho wn i n F ig3—5A—3。
自动化英语
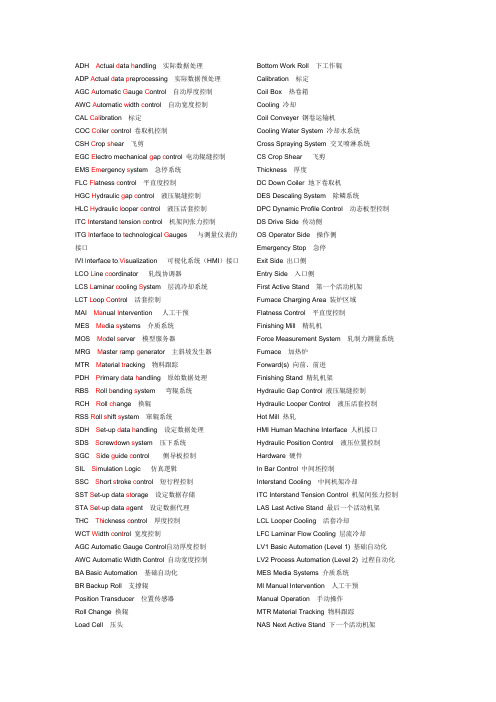
ADH A ctual d ata h andling 实际数据处理ADP A ctual d ata p reprocessing 实际数据预处理AGC A utomatic G auge C ontrol 自动厚度控制AWC A utomatic w idth c ontrol 自动宽度控制CAL Cal ibration 标定COC Co iler c ontrol 卷取机控制CSH C rop sh ear 飞剪EGC E lectro mechanical g ap c ontrol 电动辊缝控制EMS Em ergency s ystem 急停系统FLC Fl atness c ontrol 平直度控制HGC H ydraulic g ap c ontrol 液压辊缝控制HLC H ydraulic l ooper c ontrol 液压活套控制ITC I nterstand t ension c ontrol 机架间张力控制ITG I nterface to t echnological G auges 与测量仪表的接口IVI I nterface to Vi sualization 可视化系统(HMI)接口LCO L ine co ordinator 轧线协调器LCS L aminar c ooling S ystem 层流冷却系统LCT L oop C on t rol 活套控制MAI Ma nual I ntervention 人工干预MES Me dia s ystems 介质系统MOS Mo del s erver 模型服务器MRG M aster r amp g enerator 主斜坡发生器MTR M aterial tr acking 物料跟踪PDH P rimary d ata h andling 原始数据处理RBS R oll b ending s ystem 弯辊系统RCH R oll ch ange 换辊RSS R oll s hift s ystem 窜辊系统SDH S et-up d ata h andling 设定数据处理SDS S crew d own s ystem 压下系统SGC S ide g uide c ontrol 侧导板控制SIL Si mulation L ogic 仿真逻辑SSC S hort s troke c ontrol 短行程控制SST S et-up data st orage 设定数据存储STA S e t-up data a gent 设定数据代理THC Th ickness c ontrol 厚度控制WCT W idth c on t rol 宽度控制AGC Automatic Gauge Control自动厚度控制AWC Automatic Width Control 自动宽度控制BA Basic Automation 基础自动化BR Backup Roll 支撑辊Position Transducer 位置传感器Roll Change 换辊Load Cell 压头Bottom Work Roll 下工作辊Calibration 标定Coil Box 热卷箱Cooling 冷却Coil Conveyer 钢卷运输机Cooling Water System 冷却水系统Cross Spraying System 交叉喷淋系统CS Crop Shear 飞剪Thickness 厚度DC Down Coiler 地下卷取机DES Descaling System 除鳞系统DPC Dynamic Profile Control 动态板型控制DS Drive Side 传动侧OS Operator Side 操作侧Emergency Stop 急停Exit Side 出口侧Entry Side 入口侧First Active Stand 第一个活动机架Furnace Charging Area 装炉区域Flatness Control 平直度控制Finishing Mill 精轧机Force Measurement System 轧制力测量系统Furnace 加热炉Forward(s) 向前、前进Finishing Stand 精轧机架Hydraulic Gap Control 液压辊缝控制Hydraulic Looper Control 液压活套控制Hot Mill 热轧HMI Human Machine Interface 人机接口Hydraulic Position Control 液压位置控制Hardware 硬件In Bar Control 中间坯控制Interstand Cooling 中间机架冷却ITC Interstand Tension Control 机架间张力控制LAS Last Active Stand 最后一个活动机架LCL Looper Cooling 活套冷却LFC Laminar Flow Cooling 层流冷却LV1 Basic Automation (Level 1) 基础自动化LV2 Process Automation (Level 2) 过程自动化MES Media Systems 介质系统MI Manual Intervention 人工干预Manual Operation 手动操作MTR Material Tracking 物料跟踪NAS Next Active Stand 下一个活动机架OLS Oil Lubrication System 润滑系统Operation Mode 操作模式Profile and Flatness Control 板型和平直度控制Pressure Measurement System 压力测量系统Profile Control 板型控制Pinch Roll Unit 夹送辊单元Pulpit 操作台Quick Stop 快停Reset 复位Roll Change 换辊Runout Table 输出辊道Request 请求Roughing Mill 粗轧机Roll Shifting Systems 窜辊系统Roller Table 辊道Roughing Stand (Rougher) 粗轧机架SDS Screwdown System 压下系统SG Side Guides 侧导板Position Measurement System 位置测量系统SSC Short Stroke Control 短行程控制Strip Tracking 带钢跟踪Servo-Valve 伺服阀Swivel 旋转Software 软件Slab Weighing System 板坯称重系统Top Backup Roll 上支撑辊Tension System 张力系统THC Thickness Control 厚度控制TWR Top Work Roll 上工作辊Work Roll Balancing 工作辊平衡Work Roll Cooling 工作辊冷却Weighing Machine 称重机Work Roll 工作辊WRB Work Roll Balancing/Bending System 工作辊平衡/弯辊系统Work Roll Change 工作辊换辊Work Roll Positive Bending 工作辊正弯辊WRS Work Roll Shifting System 工作辊窜辊系统Roll Cooling and Interstand Cooling 轧辊冷却和中间机架冷却Tension Measurement System 张力测量系统Compensation 补偿Setpoint 设定值Manual intervention 人工干预Lead factor 超前因数Lag factor 滞后因数Increase 增加decrease 减小pass through operation mode 直通操作模式Furnace加热炉descaler 除鳞箱enter table入口辊道bar reject device 中间坯剔除装置rollertable辊道work roll 工作辊backup roll 支撑辊mill stand 轧机机架sensor 传感器position transducer 位置传感器pressure transducer 压力传感器valve 阀operator panel(OP) 操作面板main control desk 主控台rougher 粗轧机edger 立辊轧机strip带钢entry side guide入口侧导板entry chute with deflector roll入口导槽及偏转辊bending device弯曲设备coiling station卷取站forming roll成型辊pusher推卷器stabilizer稳定器peeler开卷器uncoiling station开卷站heat preservation side guide保湿侧导板holdback pin开尾销pinch roll unit夹送辊单元hold back roll缓冲辊local desk就地操作台limit switch极限开关coil box热卷箱pass schedules轧制表push button按钮cradle roll 托卷辊Oscillate 摆动scrap废料spray nozzle 喷嘴stand zeroing机架调零mechanical 机械的synchronize同步hydraulic cylinder 液压缸Proportional valve 比例阀cylinder 液压缸remote 远程conveyor 运输机Coil discharge 卸卷pulse 脉冲telegram 报文Ethernet-bus 以太网总线mandrel outboard bearing 芯轴外支撑even passes 偶道次odd passes 奇道次。
自动化外文参考文献(精选120个最新)
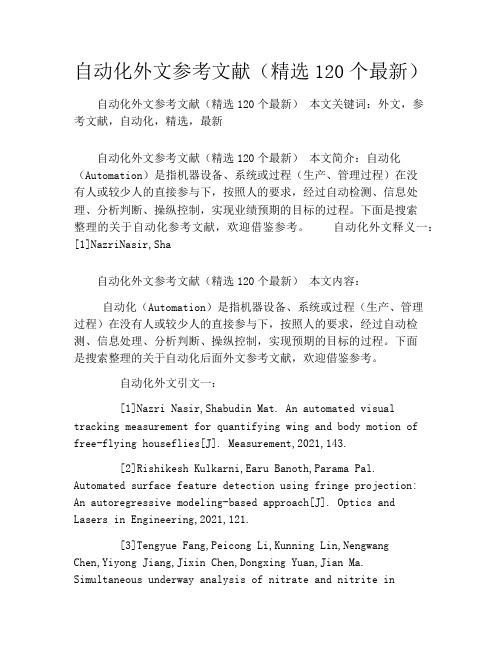
自动化外文参考文献(精选120个最新)自动化外文参考文献(精选120个最新)本文关键词:外文,参考文献,自动化,精选,最新自动化外文参考文献(精选120个最新)本文简介:自动化(Automation)是指机器设备、系统或过程(生产、管理过程)在没有人或较少人的直接参与下,按照人的要求,经过自动检测、信息处理、分析判断、操纵控制,实现业绩预期的目标的过程。
下面是搜索整理的关于自动化参考文献,欢迎借鉴参考。
自动化外文释义一:[1]NazriNasir,Sha自动化外文参考文献(精选120个最新)本文内容:自动化(Automation)是指机器设备、系统或过程(生产、管理过程)在没有人或较少人的直接参与下,按照人的要求,经过自动检测、信息处理、分析判断、操纵控制,实现预期的目标的过程。
下面是搜索整理的关于自动化后面外文参考文献,欢迎借鉴参考。
自动化外文引文一:[1]Nazri Nasir,Shabudin Mat. An automated visual tracking measurement for quantifying wing and body motion of free-flying houseflies[J]. Measurement,2021,143.[2]Rishikesh Kulkarni,Earu Banoth,Parama Pal. Automated surface feature detection using fringe projection: An autoregressive modeling-based approach[J]. Optics and Lasers in Engineering,2021,121.[3]Tengyue Fang,Peicong Li,Kunning Lin,NengwangChen,Yiyong Jiang,Jixin Chen,Dongxing Yuan,Jian Ma. Simultaneous underway analysis of nitrate and nitrite inestuarine and coastal waters using an automated integrated syringe-pump-based environmental-water analyzer[J]. Analytica Chimica Acta,2021,1076.[4]Shengfeng Chen,Jian Liu,Xiaosong Zhang,XinyuSuo,Enhui Lu,Jilong Guo,Jianxun Xi. Development ofpositioning system for Nuclear-fuel rod automated assembly[J]. Robotics and Computer Integrated Manufacturing,2021,61.[5]Cheng-Ta Lee,Yu-Ching Lee,Albert Y. Chen. In-building automated external defibrillator location planning and assessment through building information models[J]. Automation in Construction,2021,106.[6]Torgeir Aleti,Jason I. Pallant,Annamaria Tuan,Tom van Laer. Tweeting with the Stars: Automated Text Analysis of the Effect of Celebrity Social Media ications on ConsumerWord of Mouth[J]. Journal of Interactive Marketing,2021,48.[7]Daniel Bacioiu,Geoff Melton,MayorkinosPapaelias,Rob Shaw. Automated defect classification of SS304 TIG welding process using visible spectrum camera and machine learning[J]. NDT and E International,2021,107.[8]Marcus von der Au,Max Schwinn,KatharinaKuhlmeier,Claudia Büchel,Bj?rn Meermann. Development of an automated on-line purification HPLC single cell-ICP-MS approach for fast diatom analysis[J]. Analytica ChimicaActa,2021,1077.[9]Jitendra Mehar,Ajam Shekh,Nethravathy M. U.,R. Sarada,Vikas Singh Chauhan,Sandeep Mudliar. Automation ofpilot-scale open raceway pond: A case study of CO 2 -fed pHcontrol on Spirulina biomass, protein and phycocyanin production[J]. Journal of CO2 Utilization,2021,33.[10]John T. Sloop,Henry J.B. Bonilla,TinaHarville,Bradley T. Jones,George L. Donati. Automated matrix-matching calibration using standard dilution analysis withtwo internal standards and a simple three-port mixing chamber[J]. Talanta,2021,205.[11]Daniel J. Spade,Cathy Yue Bai,ChristyLambright,Justin M. Conley,Kim Boekelheide,L. Earl Gray. Corrigendum to “Validation of an automated counting procedure for phthalate-induced testicular multinucleated germ cells” [Toxicol. Lett. 290 (2021) 55–61][J]. Toxicology Letters,2021,313.[12]Christian P. Janssen,Shamsi T. Iqbal,Andrew L. Kun,Stella F. Donker. Interrupted by my car? Implications of interruption and interleaving research for automatedvehicles[J]. International Journal of Human - Computer Studies,2021,130.[13]Seunguk Lee,Si Kuan Thio,Sung-Yong Park,Sungwoo Bae. An automated 3D-printed smartphone platform integrated with optoelectrowetting (OEW) microfluidic chip for on-site monitoring of viable algae in water[J]. Harmful Algae,2021,88.[14]Yuxia Duan,Shicai Liu,Caiqi Hu,Junqi Hu,Hai Zhang,Yiqian Yan,Ning Tao,Cunlin Zhang,Xavier Maldague,Qiang Fang,Clemente Ibarra-Castanedo,Dapeng Chen,Xiaoli Li,Jianqiao Meng. Automated defect classification in infrared thermography based on a neural network[J]. NDT and E International,2021,107.[15]Alex M. Pagnozzi,Jurgen Fripp,Stephen E. Rose. Quantifying deep grey matter atrophy using automated segmentation approaches: A systematic review of structural MRI studies[J]. NeuroImage,2021,201.[16]Jin Ye,Zhihong Xuan,Bing Zhang,Yu Wu,LiLi,Songshan Wang,Gang Xie,Songxue Wang. Automated analysis of ochratoxin A in cereals and oil by iaffinity magnetic beads coupled to UPLC-FLD[J]. Food Control,2021,104.[17]Anne Bech Risum,Rasmus Bro. Using deep learning to evaluate peaks in chromatographic data[J].Talanta,2021,204.[18]Faris Elghaish,Sepehr Abrishami,M. Reza Hosseini,Soliman Abu-Samra,Mark Gaterell. Integrated project delivery with BIM: An automated EVM-based approach[J]. Automation in Construction,2021,106.[19]Carl J. Pearson,Michael Geden,Christopher B. Mayhorn. Who's the real expert here? Pedigree's unique bias on trust between human and automated advisers[J]. Applied Ergonomics,2021,81.[20]Vibhas Mishra,Dani?l M.J. Peeters,Mostafa M. Abdalla. Stiffness and buckling analysis of variablestiffness laminates including the effect of automated fibre placement defects[J]. Composite Structures,2021,226.[21]Jenny S. Wesche,Andreas Sonderegger. When computers take the lead: The automation of leadership[J]. Computers in Human Behavior,2021,101.[22]Murat Ayaz,Hüseyin Yüksel. Design of a new cost-efficient automation system for gas leak detection in industrial buildings[J]. Energy & Buildings,2021,200.[23]Stefan A. Mann,Juliane Heide,Thomas Knott,Razvan Airini,Florin Bogdan Epureanu,Alexandru-FlorianDeftu,Antonia-Teona Deftu,Beatrice Mihaela Radu,Bogdan Amuzescu. Recording of multiple ion current components and action potentials in human induced pluripotent stem cell-derived cardiomyocytes via automated patch-clamp[J]. Journal of Pharmacological and Toxicological Methods,2021,100.[24]Rhar? de Almeida Cardoso,Alexandre Cury,Flavio Barbosa. Automated real-time damage detection strategy using raw dynamic measurements[J]. Engineering Structures,2021,196.[25]Mengmeng Zhong,Tielong Wang,Chengdu Qi,Guilong Peng,Meiling Lu,Jun Huang,Lee Blaney,Gang Yu. Automated online solid-phase extraction liquid chromatography tandem mass spectrometry investigation for simultaneous quantification of per- and polyfluoroalkyl substances, pharmaceuticals and personal care products, and organophosphorus flame retardants in environmental waters[J]. Journal of Chromatography A,2021,1602.[26]Pau Climent-Pér ez,Susanna Spinsante,Alex Mihailidis,Francisco Florez-Revuelta. A review on video-based active and assisted living technologies for automated lifelogging[J]. Expert Systems With Applications,2021,139.[27]William Snyder,Marisa Patti,Vanessa Troiani. An evaluation of automated tracing for orbitofrontal cortexsulcogyral pattern typing[J]. Journal of Neuroscience Methods,2021,326.[28]Juan Manuel Davila Delgado,LukumonOyedele,Anuoluwapo Ajayi,Lukman Akanbi,OlugbengaAkinade,Muhammad Bilal,Hakeem Owolabi. Robotics and automated systems in construction: Understanding industry-specific challenges for adoption[J]. Journal of Building Engineering,2021,26.[29]Mohamed Taher Alrefaie,Stever Summerskill,Thomas W Jackon. In a heart beat: Using driver’s physiological changes to determine the quality of a takeover in highly automated vehicles[J]. Accident Analysis andPrevention,2021,131.[30]Tawseef Ayoub Shaikh,Rashid Ali. Automated atrophy assessment for Alzheimer's disease diagnosis from brain MRI images[J]. Magnetic Resonance Imaging,2021,62.自动化外文参考文献二:[31]Vaanathi Sundaresan,Giovanna Zamboni,Campbell Le Heron,Peter M. Rothwell,Masud Husain,Marco Battaglini,Nicola De Stefano,Mark Jenkinson,Ludovica Griffanti. Automatedlesion segmentation with BIANCA: Impact of population-level features, classification algorithm and locally adaptive thresholding[J]. NeuroImage,2021,202.[32]Ho-Jun Suk,Edward S. Boyden,Ingrid van Welie. Advances in the automation of whole-cell patch clamp technology[J]. Journal of Neuroscience Methods,2021,326.[33]Ivana Duznovic,Mathias Diefenbach,Mubarak Ali,Tom Stein,Markus Biesalski,Wolfgang Ensinger. Automated measuring of mass transport through synthetic nanochannels functionalized with polyelectrolyte porous networks[J]. Journal of Membrane Science,2021,591.[34]James A.D. Cameron,Patrick Savoie,Mary E.Kaye,Erik J. Scheme. Design considerations for the processing system of a CNN-based automated surveillance system[J]. Expert Systems With Applications,2021,136.[35]Ebrahim Azadniya,Gertrud E. Morlock. Automated piezoelectric spraying of biological and enzymatic assays for effect-directed analysis of planar chromatograms[J]. Journal of Chromatography A,2021,1602.[36]Lilla Z?llei,Camilo Jaimes,Elie Saliba,P. Ellen Grant,Anastasia Yendiki. TRActs constrained by UnderLying INfant anatomy (TRACULInA): An automated probabilistic tractography tool with anatomical priors for use in the newborn brain[J]. NeuroImage,2021,199.[37]Kate?ina Fikarová,David J. Cocovi-Solberg,María Rosende,Burkhard Horstkotte,Hana Sklená?ová,Manuel Miró. A flow-based platform hyphenated to on-line liquid chromatography for automatic leaching tests of chemical additives from microplastics into seawater[J]. Journal of Chromatography A,2021,1602.[38]Darko ?tern,Christian Payer,Martin Urschler. Automated age estimation from MRI volumes of the hand[J]. Medical Image Analysis,2021,58.[39]Jacques Blum,Holger Heumann,Eric Nardon,Xiao Song. Automating the design of tokamak experiment scenarios[J]. Journal of Computational Physics,2021,394.[40]Elton F. de S. Soares,Carlos Alberto V.Campos,Sidney C. de Lucena. Online travel mode detection method using automated machine learning and feature engineering[J]. Future Generation Computer Systems,2021,101.[41]M. Marouli,S. Pommé. Autom ated optical distance measurements for counting at a defined solid angle[J].Applied Radiation and Isotopes,2021,153.[42]Yi Dai,Zhen-Hua Yu,Jian-Bo Zhan,Bao-Shan Yue,Jiao Xie,Hao Wang,Xin-Sheng Chai. Determination of starch gelatinization temperatures by an automated headspace gas chromatography[J]. Journal of Chromatography A,2021,1602.[43]Marius Tarp?,Tobias Friis,Peter Olsen,MartinJuul,Christos Georgakis,Rune Brincker. Automated reduction of statistical errors in the estimated correlation functionmatrix for operational modal analysis[J]. Mechanical Systems and Signal Processing,2021,132.[44]Wenxia Dai,Bisheng Yang,Xinlian Liang,ZhenDong,Ronggang Huang,Yunsheng Wang,Wuyan Li. Automated fusionof forest airborne and terrestrial point clouds throughcanopy density analysis[J]. ISPRS Journal of Photogrammetry and Remote Sensing,2021,156.[45]Jyh-Haur Woo,Marcus Ang,Hla Myint Htoon,Donald Tan. Descemet Membrane Endothelial Keratoplasty Versus Descemet Stripping Automated Endothelial Keratoplasty andPenetrating Keratoplasty[J]. American Journal of Ophthalmology,2021,207.[46]F. Wilde,S. Marsen,T. Stange,D. Moseev,J.W. Oosterbeek,H.P. Laqua,R.C. Wolf,K. Avramidis,G.Gantenbein,I.Gr. Pagonakis,S. Illy,J. Jelonnek,M.K. Thumm,W7-X team. Automated mode recovery for gyrotrons demonstrated at Wendelstein 7-X[J]. Fusion Engineering and Design,2021,148.[47]Andrew Kozbial,Lekhana Bhandary,Shashi K. Murthy. Effect of yte seeding density on dendritic cell generation in an automated perfusion-based culture system[J]. Biochemical Engineering Journal,2021,150.[48]Wen-Hao Su,Steven A. Fennimore,David C. Slaughter. Fluorescence imaging for rapid monitoring of translocation behaviour of systemic markers in snap beans for automatedcrop/weed discrimination[J]. Biosystems Engineering,2021,186.[49]Ki-Taek Lim,Dinesh K. Patel,Hoon Se,JanghoKim,Jong Hoon Chung. A fully automated bioreactor system for precise control of stem cell proliferation anddifferentiation[J]. Biochemical Engineering Journal,2021,150.[50]Mitchell L. Cunningham,Michael A. Regan,Timothy Horberry,Kamal Weeratunga,Vinayak Dixit. Public opinion about automated vehicles in Australia: Results from a large-scale national survey[J]. Transportation Research Part A,2021,129.[51]Yi Xie,Qiaobei You,Pingyang Dai,Shuyi Wang,Peiyi Hong,Guokun Liu,Jun Yu,Xilong Sun,Yongming Zeng. How to achieve auto-identification in Raman analysis by spectral feature extraction & Adaptive Hypergraph[J].Spectrochimica Acta Part A: Molecular and Biomolecular Spectroscopy,2021,222.[52]Ozal Yildirim,Muhammed Talo,Betul Ay,Ulas Baran Baloglu,Galip Aydin,U. Rajendra Acharya. Automated detection of diabetic subject using pre-trained 2D-CNN models with frequency spectrum images extracted from heart ratesignals[J]. Computers in Biology and Medicine,2021,113.[53]Marius Kern,Laura Tusa,Thomas Lei?ner,Karl Gerald van den Boogaart,Jens Gutzmer. Optimal sensor selection for sensor-based sorting based on automated mineralogy data[J]. Journal of Cleaner Production,2021,234.[54]Karim Keddadouche,Régis Braucher,Didier L.Bourlès,Mélanie Baroni,Valéry Guillou,La?titia Léanni,Georges Auma?tre. Design and performance of an automated chemical extraction bench for the preparation of 10 Be and 26 Al targets to be analyzed by accelerator mass spectrometry[J]. Nuclear Inst. and Methods in Physics Research, B,2021,456.[55]Christian P. Janssen,Stella F. Donker,Duncan P. Brumby,Andrew L. Kun. History and future of human-automation interaction[J]. International Journal of Human - Computer Studies,2021,131.[56]Victoriya Orlovskaya,Olga Fedorova,Michail Nadporojskii,Raisa Krasikova. A fully automated azeotropic drying free synthesis of O -(2-[ 18 F]fluoroethyl)- l -tyrosine ([ 18 F]FET) using tetrabutylammonium tosylate[J]. Applied Radiation and Isotopes,2021,152.[57]Dinesh Krishnamoorthy,Kjetil Fjalestad,Sigurd Skogestad. Optimal operation of oil and gas production usingsimple feedback control structures[J]. Control Engineering Practice,2021,91.[58]Nick Oliver,Thomas Calvard,Kristina Poto?nik. Safe limits, mindful organizing and loss of control in commercial aviation[J]. Safety Science,2021,120.[59]Bo Sui,Nils Lubbe,Jonas B?rgman. A clustering approach to developing car-to-two-wheeler test scenarios for the assessment of Automated Emergency Braking in China using in-depth Chinese crash data[J]. Accident Analysis and Prevention,2021,132.[60]Ji-Seok Yoon,Eun Young Choi,Maliazurina Saad,Tae-Sun Choi. Automated integrated system for stained neuron detection: An end-to-end framework with a high negative predictive rate[J]. Computer Methods and Programs in Biomedicine,2021,180.自动化外文参考文献八:[61]Min Wang,Barbara E. Glick-Wilson,Qi-Huang Zheng. Facile fully automated radiosynthesis and quality control of O -(2-[ 18 F]fluoroethyl)- l -tyrosine ([ 18 F]FET) for human brain tumor imaging[J]. Applied Radiation andIsotopes,2021,154.[62]Fabian Pütz,Finbarr Murphy,Martin Mullins,LisaO'Malley. Connected automated vehicles and insurance: Analysing future market-structure from a business ecosystem perspective[J]. Technology in Society,2021,59.[63]Victoria A. Banks,Neville A. Stanton,Katherine L. Plant. Who is responsible for automated driving? A macro-level insight into automated driving in the United Kingdom using the Risk Management Framework and Social NetworkAnalysis[J]. Applied Ergonomics,2021,81.[64]Yingjun Ye,Xiaohui Zhang,Jian Sun. Automated vehicle’s behavior decision making using deep reinforcement learning and high-fidelity simulation environment[J]. Transportation Research Part C,2021,107.[65]Hasan Alkaf,Jameleddine Hassine,TahaBinalialhag,Daniel Amyot. An automated change impact analysis approach for User Requirements Notation models[J]. TheJournal of Systems & Software,2021,157.[66]Zonghua Luo,Jiwei Gu,Robert C. Dennett,Gregory G. Gaehle,Joel S. Perlmutter,Delphine L. Chen,Tammie L.S. Benzinger,Zhude Tu. Automated production of a sphingosine-1 phosphate receptor 1 (S1P1) PET radiopharmaceutical [ 11C]CS1P1 for human use[J]. Applied Radiation andIsotopes,2021,152.[67]Sarfraz Qureshi,Wu Jiacheng,Jeroen Anton van Kan. Automated alignment and focusing system for nuclear microprobes[J]. Nuclear Inst. and Methods in Physics Research, B,2021,456.[68]Srikanth Sagar Bangaru,Chao Wang,MarwaHassan,Hyun Woo Jeon,Tarun Ayiluri. Estimation of the degreeof hydration of concrete through automated machine learning based microstructure analysis – A study on effect of image magnification[J]. Advanced Engineering Informatics,2021,42.[69]Fang Tengyue,Li Peicong,Lin Kunning,Chen Nengwang,Jiang Yiyong,Chen Jixin,Yuan Dongxing,Ma Jian. Simultaneous underway analysis of nitrate and nitrite in estuarine and coastal waters using an automated integrated syringe-pump-based environmental-water analyzer.[J]. Analytica chimica acta,2021,1076.[70]Ramos Inês I,Carl Peter,Schneider RudolfJ,Segundo Marcela A. Automated lab-on-valve sequential injection ELISA for determination of carbamazepine.[J]. Analytica chimica acta,2021,1076.[71]Au Marcus von der,Schwinn Max,Kuhlmeier Katharina,Büchel Claudia,Meermann Bj?rn. Development of an automated on-line purification HPLC single cell-ICP-MS approach for fast diatom analysis.[J]. Analytica chimica acta,2021,1077.[72]Risum Anne Bech,Bro Rasmus. Using deep learning to evaluate peaks in chromatographic data.[J].Talanta,2021,204.[73]Spade Daniel J,Bai Cathy Yue,LambrightChristy,Conley Justin M,Boekelheide Kim,Gray L Earl. Corrigendum to "Validation of an automated counting procedure for phthalate-induced testicular multinucleated germ cells" [Toxicol. Lett. 290 (2021) 55-61].[J]. Toxicologyletters,2021,313.[74]Zhong Mengmeng,Wang Tielong,Qi Chengdu,Peng Guilong,Lu Meiling,Huang Jun,Blaney Lee,Yu Gang. Automated online solid-phase extraction liquid chromatography tandem mass spectrometry investigation for simultaneousquantification of per- and polyfluoroalkyl substances, pharmaceuticals and personal care products, and organophosphorus flame retardants in environmental waters.[J]. Journal of chromatography. A,2021,1602.[75]Stein Christopher J,Reiher Markus. autoCAS: A Program for Fully Automated MulticonfigurationalCalculations.[J]. Journal of computationalchemistry,2021,40(25).[76]Alrefaie Mohamed Taher,Summerskill Stever,Jackon Thomas W. In a heart beat: Using driver's physiological changes to determine the quality of a takeover in highly automated vehicles.[J]. Accident; analysis andprevention,2021,131.[77]Shaikh Tawseef Ayoub,Ali Rashid. Automatedatrophy assessment for Alzheimer's disease diagnosis frombrain MRI images.[J]. Magnetic resonance imaging,2021,62.[78]Xie Yi,You Qiaobei,Dai Pingyang,Wang Shuyi,Hong Peiyi,Liu Guokun,Yu Jun,Sun Xilong,Zeng Yongming. How to achieve auto-identification in Raman analysis by spectral feature extraction & Adaptive Hypergraph.[J]. Spectrochimica acta. Part A, Molecular and biomolecular spectroscopy,2021,222.[79]Azadniya Ebrahim,Morlock Gertrud E. Automated piezoelectric spraying of biological and enzymatic assays for effect-directed analysis of planar chromatograms.[J]. Journal of chromatography. A,2021,1602.[80]Fikarová Kate?ina,Cocovi-Solberg David J,Rosende María,Horstkotte Burkhard,Sklená?ová Hana,Miró Manuel. Aflow-based platform hyphenated to on-line liquid chromatography for automatic leaching tests of chemical additives from microplastics into seawater.[J]. Journal of chromatography. A,2021,1602.[81]Moitra Dipanjan,Mandal Rakesh Kr. Automated AJCC (7th edition) staging of non-small cell lung cancer (NSCLC) using deep convolutional neural network (CNN) and recurrent neural network (RNN).[J]. Health information science and systems,2021,7(1).[82]Ramos-Payán María. Liquid - Phase microextraction and electromembrane extraction in millifluidic devices:A tutorial.[J]. Analytica chimica acta,2021,1080.[83]Z?llei Lilla,Jaimes Camilo,Saliba Elie,Grant P Ellen,Yendiki Anastasia. TRActs constrained by UnderLying INfant anatomy (TRACULInA): An automated probabilistic tractography tool with anatomical priors for use in the newborn brain.[J]. NeuroImage,2021,199.[84]Sedghi Gamechi Zahra,Bons Lidia R,Giordano Marco,Bos Daniel,Budde Ricardo P J,Kofoed Klaus F,Pedersen Jesper Holst,Roos-Hesselink Jolien W,de Bruijne Marleen. Automated 3D segmentation and diameter measurement of the thoracic aorta on non-contrast enhanced CT.[J]. European radiology,2021,29(9).[85]Smith Claire,Galland Barbara C,de Bruin Willemijn E,Taylor Rachael W. Feasibility of Automated Cameras to Measure Screen Use in Adolescents.[J]. American journal of preventive medicine,2021,57(3).[86]Lambert Marie-?ve,Arsenault Julie,AudetPascal,Delisle Benjamin,D'Allaire Sylvie. Evaluating an automated clustering approach in a perspective of ongoing surveillance of porcine reproductive and respiratory syndrome virus (PRRSV) field strains.[J]. Infection, genetics and evolution : journal of molecular epidemiology and evolutionary genetics in infectious diseases,2021,73.[87]Slanetz Priscilla J. Does Computer-aided Detection Help in Interpretation of Automated Breast US?[J]. Radiology,2021,292(3).[88]Sander Laura,Pezold Simon,Andermatt Simon,Amann Michael,Meier Dominik,Wendebourg Maria J,Sinnecker Tim,Radue Ernst-Wilhelm,Naegelin Yvonne,Granziera Cristina,Kappos Ludwig,Wuerfel Jens,Cattin Philippe,Schlaeger Regina. Accurate, rapid and reliable, fully automated MRI brainstem segmentation for application in multiple sclerosis and neurodegenerative diseases.[J]. Human brainmapping,2021,40(14).[89]Pajkossy Péter,Sz?ll?si ?gnes,Racsmány Mihály. Retrieval practice decreases processing load of recall: Evidence revealed by pupillometry.[J]. International journal of psychophysiology : official journal of the International Organization of Psychophysiology,2021,143.[90]Kaiser Eric A,Igdalova Aleksandra,Aguirre Geoffrey K,Cucchiara Brett. A web-based, branching logic questionnaire for the automated classification ofmigraine.[J]. Cephalalgia : an international journal of headache,2021,39(10).自动化外文参考文献四:[91]Kim Jin Ju,Park Younhee,Choi Dasom,Kim Hyon Suk. Performance Evaluation of a New Automated Chemiluminescent Ianalyzer-Based Interferon-Gamma Releasing Assay AdvanSure I3 in Comparison With the QuantiFERON-TB Gold In-Tube Assay.[J]. Annals of laboratory medicine,2021,40(1).[92]Yang Shanling,Gao Xican,Liu Liwen,Shu Rui,Yan Jingru,Zhang Ge,Xiao Yao,Ju Yan,Zhao Ni,Song Hongping. Performance and Reading Time of Automated Breast US with or without Computer-aided Detection.[J]. Radiology,2021,292(3).[93]Hung Andrew J,Chen Jian,Ghodoussipour Saum,OhPaul J,Liu Zequn,Nguyen Jessica,Purushotham Sanjay,Gill Inderbir S,Liu Yan. A deep-learning model using automated performance metrics and clinical features to predict urinary continence recovery after robot-assisted radical prostatectomy.[J]. BJU international,2021,124(3).[94]Kim Ryan S,Kim Gene. Double Descemet Stripping Automated Endothelial Keratoplasty (DSAEK): Secondary DSAEK Without Removal of the Failed Primary DSAEK Graft.[J]. Ophthalmology,2021,126(9).[95]Sargent Alexandra,Theofanous Ioannis,Ferris Sarah. Improving laboratory workflow through automated pre-processing of SurePath specimens for human papillomavirus testing with the Abbott RealTime assay.[J]. Cytopathology : official journal of the British Society for Clinical Cytology,2021,30(5).[96]Saba Tanzila. Automated lung nodule detection and classification based on multiple classifiers voting.[J]. Microscopy research and technique,2021,82(9).[97]Ivan D. Welsh,Jane R. Allison. Automated simultaneous assignment of bond orders and formal charges[J]. Journal of Cheminformatics,2021,11(1).[98]Willem Jespers,MauricioEsguerra,Johan ?qvist,Hugo Gutiérrez-de-Terán. QligFEP: an automated workflow for small molecule free energycalculations in Q[J]. Journal of Cheminformatics,2021,11(1).[99]Manav Raj,Robert Seamans. Primer on artificial intelligence and robotics[J]. Journal of OrganizationDesign,2021,8(1).[100]Yvette Pronk,Peter Pilot,Justus M.Brinkman,Ronald J. Heerwaarden,Walter Weegen. Response rate and costs for automated patient-reported outcomes collection alone compared to combined automated and manual collection[J]. Journal of Patient-Reported Outcomes,2021,3(1).[101]Tristan Martin,Ana?s Moyon,Cyril Fersing,Evan Terrier,Aude Gouillet,Fabienne Giraud,BenjaminGuillet,Philippe Garrigue. Have you looked for “stranger things” in your automated PET dose dispensing system? A process and operators qualification scheme[J]. EJNMMI Radiopharmacy and Chemistry,2021,4(1).[102]Manuel Peuster,Michael Marchetti,Ger ardo García de Blas,Holger Karl. Automated testing of NFV orchestrators against carrier-grade multi-PoP scenarios using emulation-based smoke testing[J]. EURASIP Journal on Wireless ications and Networking,2021,2021(1).[103]R. Ferrús,O. Sallent,J. Pérez-Romero,R. Agustí. On the automation of RAN slicing provisioning: solution framework and applicability examples[J]. EURASIP Journal on Wireless ications and Networking,2021,2021(1).[104]Duo Li,Peter Wagner. Impacts of gradual automated vehicle penetration on motorway operation: a comprehensive evaluation[J]. European Transport Research Review,2021,11(1).[105]Abel Gómez,Ricardo J. Rodríguez,María-Emilia Cambronero,Valentín Valero. Profiling the publish/subscribe paradigm for automated analysis using colored Petri nets[J]. Software & Systems Modeling,2021,18(5).[106]Dipanjan Moitra,Rakesh Kr. Mandal. Automated AJCC (7th edition) staging of non-small cell lung cancer (NSCLC) using deep convolutional neural network (CNN) and recurrent neural network (RNN)[J]. Health Information Science and Systems,2021,7(1).[107]Marta D’Alonzo,Laura Martincich,Agnese Fenoglio,Valentina Giannini,Lisa Cellini,ViolaLiberale,Nicoletta Biglia. Nipple-sparing mastectomy: external validation of a three-dimensional automated method to predict nipple occult tumour involvement on preoperative breast MRI[J]. European Radiology Experimental,2021,3(1).[108]N. V. Dozmorov,A. S. Bogomolov,A. V. Baklanov. An Automated Apparatus for Measuring Spectral Dependences ofthe Mass Spectra and Velocity Map Images of Photofragments[J]. Instruments and Experimental Techniques,2021,62(4).[109]Zhiqiang Sun,Bingzhao Gao,Jiaqi Jin,Kazushi Sanada. Modelling, Analysis and Simulation of a Novel Automated Manual Transmission with Gearshift Assistant Mechanism[J]. International Journal of Automotive Technology,2021,20(5).[110]Andrés Vega,Mariano Córdoba,Mauricio Castro-Franco,Mónica Balzarini. Protocol for automating errorremoval from yield maps[J]. Precision Agriculture,2021,20(5).[111]Bethany L. Lussier,DaiWai M. Olson,Venkatesh Aiyagari. Automated Pupillometry in Neurocritical Care: Research and Practice[J]. Current Neurology and Neuroscience Reports,2021,19(10).[112] B. Haskali,Peter D. Roselt,David Binns,Amit Hetsron,Stan Poniger,Craig A. Hutton,Rodney J. Hicks. Automated preparation of clinical grade [ 68 Ga]Ga-DOTA-CP04, a cholecystokinin-2 receptor agonist, using iPHASE MultiSyn synthesis platform[J]. EJNMMI Radiopharmacy andChemistry,2021,4(1).[113]Ju Hyun Ahn,Minho Na,Sungkwan Koo,HyunsooChun,Inhwan Kim,Jong Won Hur,Jae Hyuk Lee,Jong G. Ok. Development of a fully automated desktop chemical vapor deposition system for programmable and controlled carbon nanotube growth[J]. Micro and Nano Systems Letters,2021,7(1).[114]Kamellia Shahi,Brenda Y. McCabe,Arash Shahi. Framework for Automated Model-Based e-Permitting System forMunicipal Jurisdictions[J]. Journal of Management in Engineering,2021,35(6).[115]Ahmed Khalafallah,Yasmin Shalaby. Change Orders: Automating Comparative Data Analysis and Controlling Impacts in Public Projects[J]. Journal of Construction Engineering and Management,2021,145(11).[116]José ?. Martínez-Huertas,OlgaJastrzebska,Ricardo Olmos,José A. León. Automated summary evaluation with inbuilt rubric method: An alternative to constructed responses and multiple-choice testsassessments[J]. Assessment & Evaluation in Higher Education,2021,44(7).[117]Samsonov,Koshel,Walther,Jenny. Automated placement of supplementary contour lines[J]. International Journal of Geographical Information Science,2021,33(10).[118]Veronika V. Odintsova,Peter J. Roetman,Hill F. Ip,René Pool,Camiel M. Van der Laan,Klodiana-DaphneTona,Robert R.J.M. Vermeiren,Dorret I. Boomsma. Genomics of human aggression: current state of genome-wide studies and an automated systematic review tool[J]. PsychiatricGenetics,2021,29(5).[119]Sebastian Eggert,Dietmar W Hutmacher. In vitro disease models 4.0 via automation and high-throughput processing[J]. Biofabrication,2021,11(4).[120]Asad Mahmood,Faizan Ahmad,Zubair Shafiq,Padmini Srinivasan,Fareed Zaffar. A Girl Has No Name: Automated Authorship Obfuscation using Mutant-X[J]. Proceedings on Privacy Enhancing Technologies,2021,2021(4).。
自动化专业翻译必备词汇

acceleration transducer加速度传感器acceptance testing验收测试accessibility可及性accumulated error累积误差AC-DC-AC frequency converter交-直-交变频器AC (alternating current) electric drive交流电子传动active attitude stabilization主动姿态稳定actuator驱动器,执行机构adaline线性适应元adaptation layer适应层adaptive telemeter system适应遥测系统adjoint operator伴随算子admissible error容许误差aggregation matrix集结矩阵AHP (analytic hierarchy process)层次分析法amplifying element放大环节analog-digital conversion模数转换annunciator信号器antenna pointing control天线指向控制anti-integral windup抗积分饱卷aperiodic decomposition非周期分解a posteriori estimate后验估计approximate reasoning近似推理a priori estimate先验估计articulated robot关节型机器人assignment problem配置问题,分配问题associative memory model联想记忆模型associatron联想机asymptotic stability渐进稳定性attained pose drift实际位姿漂移attitude acquisition姿态捕获AOCS (attritude and orbit control system)姿态轨道控制系统attitude angular velocity姿态角速度attitude disturbance姿态扰动attitude maneuver姿态机动attractor吸引子augment ability可扩充性augmented system增广系统automatic manual station自动-手动操作器automaton自动机autonomous system自治系统backlash characteristics间隙特性base coordinate system基座坐标系Bayes classifier贝叶斯分类器bearing alignment方位对准bellows pressure gauge波纹管压力表benefit-cost analysis收益成本分析bilinear system双线性系统biocybernetics生物控制论biological feedback system生物反馈系统black box testing approach黑箱测试法blind search盲目搜索block diagonalization块对角化Boltzman machine玻耳兹曼机bottom-up development自下而上开发boundary value analysis边界值分析brainstorming method头脑风暴法breadth-first search广度优先搜索butterfly valve蝶阀CAE (computer aided engineering)计算机辅助工程CAM (computer aided manufacturing)计算机辅助制造Camflex valve偏心旋转阀canonical state variable规范化状态变量capacitive displacement transducer电容式位移传感器capsule pressure gauge膜盒压力表CARD计算机辅助研究开发Cartesian robot直角坐标型机器人cascade compensation串联补偿catastrophe theory突变论centrality集中性chained aggregation链式集结chaos混沌characteristic locus特征轨迹chemical propulsion化学推进calrity清晰性classical information pattern经典信息模式classifier分类器clinical control system临床控制系统closed loop pole闭环极点closed loop transfer function闭环传递函数cluster analysis聚类分析coarse-fine control粗-精控制cobweb model蛛网模型coefficient matrix系数矩阵cognitive science认知科学cognitron认知机coherent system单调关联系统combination decision组合决策combinatorial explosion组合爆炸combined pressure and vacuum gauge压力真空表command pose指令位姿companion matrix相伴矩阵compartmental model房室模型compatibility相容性,兼容性compensating network补偿网络compensation补偿,矫正compliance柔顺,顺应composite control组合控制computable general equilibrium model可计算一般均衡模型conditionally instability条件不稳定性configuration组态connectionism连接机制connectivity连接性conservative system守恒系统consistency一致性constraint condition约束条件consumption function消费函数context-free grammar上下文无关语法continuous discrete event hybrid system simulation连续离散事件混合系统仿真continuous duty连续工作制control accuracy控制精度control cabinet控制柜controllability index可控指数controllable canonical form可控规范型[control] plant控制对象,被控对象controlling instrument控制仪表control moment gyro控制力矩陀螺control panel控制屏,控制盘control synchro控制[式]自整角机control system synthesis控制系统综合control time horizon控制时程cooperative game合作对策coordinability condition可协调条件coordination strategy协调策略coordinator协调器corner frequency转折频率costate variable共态变量cost-effectiveness analysis费用效益分析coupling of orbit and attitude轨道和姿态耦合critical damping临界阻尼critical stability临界稳定性cross-over frequency穿越频率,交越频率current source inverter电流[源]型逆变器cut-off frequency截止频率cybernetics控制论cyclic remote control循环遥控cylindrical robot圆柱坐标型机器人damped oscillation阻尼振荡damper阻尼器damping ratio阻尼比data acquisition数据采集data encryption数据加密data preprocessing数据预处理data processor数据处理器DC generator-motor set drive直流发电机-电动机组传动D controller微分控制器decentrality分散性decentralized stochastic control分散随机控制decision space决策空间decision support system决策支持系统decomposition-aggregation approach分解集结法decoupling parameter解耦参数deductive-inductive hybrid modeling method演绎与归纳混合建模法delayed telemetry延时遥测derivation tree导出树derivative feedback微分反馈describing function描述函数desired value希望值despinner消旋体destination目的站detector检出器deterministic automaton确定性自动机deviation偏差deviation alarm偏差报警器DFD数据流图diagnostic model诊断模型diagonally dominant matrix对角主导矩阵diaphragm pressure gauge膜片压力表difference equation model差分方程模型differential dynamical system微分动力学系统differential game微分对策differential pressure level meter差压液位计differential pressure transmitter差压变送器differential transformer displacement transducer差动变压器式位移传感器differentiation element微分环节digital filer数字滤波器digital signal processing数字信号处理digitization数字化digitizer数字化仪dimension transducer尺度传感器direct coordination直接协调disaggregation解裂discoordination失协调discrete event dynamic system离散事件动态系统discrete system simulation language离散系统仿真语言discriminant function判别函数displacement vibration amplitude transducer位移振幅传感器dissipative structure耗散结构distributed parameter control system分布参数控制系统distrubance扰动disturbance compensation扰动补偿diversity多样性divisibility可分性domain knowledge领域知识dominant pole主导极点dose-response model剂量反应模型dual modulation telemetering system双重调制遥测系统dual principle对偶原理dual spin stabilization双自旋稳定duty ratio负载比dynamic braking能耗制动dynamic characteristics动态特性dynamic deviation动态偏差dynamic error coefficient动态误差系数dynamic exactness动它吻合性dynamic input-output model动态投入产出模型econometric model计量经济模型economic cybernetics经济控制论economic effectiveness经济效益economic evaluation经济评价economic index经济指数economic indicator经济指标eddy current thickness meter电涡流厚度计effectiveness有效性effectiveness theory效益理论elasticity of demand需求弹性electric actuator电动执行机构electric conductance levelmeter电导液位计electric drive control gear电动传动控制设备electric hydraulic converter电-液转换器electric pneumatic converter电-气转换器electrohydraulic servo vale电液伺服阀electromagnetic flow transducer电磁流量传感器electronic batching scale电子配料秤electronic belt conveyor scale电子皮带秤electronic hopper scale电子料斗秤elevation仰角emergency stop异常停止empirical distribution经验分布endogenous variable内生变量equilibrium growth均衡增长equilibrium point平衡点equivalence partitioning等价类划分ergonomics工效学error误差error-correction parsing纠错剖析estimate估计量estimation theory估计理论evaluation technique评价技术event chain事件链evolutionary system进化系统exogenous variable外生变量expected characteristics希望特性external disturbance外扰[next]fact base事实failure diagnosis故障诊断fast mode快变模态feasibility study可行性研究feasible coordination可行协调feasible region可行域feature detection特征检测feature extraction特征抽取feedback compensation反馈补偿feedforward path前馈通路field bus现场总线finite automaton有限自动机FIP (factory information protocol)工厂信息协议first order predicate logic一阶谓词逻辑fixed sequence manipulator固定顺序机械手fixed set point control定值控制FMS (flexible manufacturing system)柔性制造系统flow sensor/transducer流量传感器flow transmitter流量变送器fluctuation涨落forced oscillation强迫振荡formal language theory形式语言理论formal neuron形式神经元forward path正向通路forward reasoning正向推理fractal分形体,分维体frequency converter变频器frequency domain model reduction method频域模型降阶法frequency response频域响应full order observer全阶观测器functional decomposition功能分解FES (functional electrical stimulation)功能电刺激functional simularity功能相似fuzzy logic模糊逻辑game tree对策树gate valve闸阀general equilibrium theory一般均衡理论generalized least squares estimation广义最小二乘估计generation function生成函数geomagnetic torque地磁力矩geometric similarity几何相似gimbaled wheel框架轮global asymptotic stability全局渐进稳定性global optimum全局最优globe valve球形阀goal coordination method目标协调法grammatical inference文法推断graphic search图搜索gravity gradient torque重力梯度力矩group technology成组技术guidance system制导系统gyro drift rate陀螺漂移率gyrostat陀螺体Hall displacement transducer霍尔式位移传感器hardware-in-the-loop simulation半实物仿真harmonious deviation和谐偏差harmonious strategy和谐策略heuristic inference启发式推理hidden oscillation隐蔽振荡hierarchical chart层次结构图hierarchical planning递阶规划hierarchical control递阶控制homeostasis内稳态homomorphic model同态系统horizontal decomposition横向分解hormonal control内分泌控制hydraulic step motor液压步进马达hypercycle theory超循环理论I controller积分控制器identifiability可辨识性IDSS (intelligent decision support system)智能决策支持系统image recognition图像识别impulse冲量impulse function冲击函数,脉冲函数inching点动incompatibility principle不相容原理incremental motion control增量运动控制index of merit品质因数inductive force transducer电感式位移传感器inductive modeling method归纳建模法industrial automation工业自动化inertial attitude sensor惯性姿态敏感器inertial coordinate system惯性坐标系inertial wheel惯性轮inference engine推理机infinite dimensional system无穷维系统information acquisition信息采集infrared gas analyzer红外线气体分析器inherent nonlinearity固有非线性inherent regulation固有调节initial deviation初始偏差initiator发起站injection attitude入轨姿势input-output model投入产出模型instability不稳定性instruction level language指令级语言integral of absolute value of error criterion绝对误差积分准则integral of squared error criterion平方误差积分准则integral performance criterion积分性能准则integration instrument积算仪器integrity整体性intelligent terminal智能终端interacted system互联系统,关联系统interactive prediction approach互联预估法,关联预估法interconnection互联intermittent duty断续工作制internal disturbance内扰ISM (interpretive structure modeling)解释结构建模法invariant embedding principle不变嵌入原理inventory theory库伦论inverse Nyquist diagram逆奈奎斯特图inverter逆变器investment decision投资决策isomorphic model同构模型iterative coordination迭代协调jet propulsion喷气推进job-lot control分批控制joint关节Kalman-Bucy filer卡尔曼-布西滤波器knowledge accomodation知识顺应knowledge acquisition知识获取knowledge assimilation知识同化KBMS (knowledge base management system)知识库管理系统knowledge representation知识表达ladder diagram梯形图lag-lead compensation滞后超前补偿Lagrange duality拉格朗日对偶性Laplace transform拉普拉斯变换large scale system大系统lateral inhibition network侧抑制网络least cost input最小成本投入least squares criterion最小二乘准则level switch物位开关libration damping天平动阻尼limit cycle极限环linearization technique线性化方法linear motion electric drive直线运动电气传动linear motion valve直行程阀linear programming线性规划LQR (linear quadratic regulator problem)线性二次调节器问题load cell称重传感器local asymptotic stability局部渐近稳定性local optimum局部最优log magnitude-phase diagram对数幅相图long term memory长期记忆lumped parameter model集总参数模型Lyapunov theorem of asymptotic stability李雅普诺夫渐近稳定性定理macro-economic system宏观经济系统magnetic dumping磁卸载magnetoelastic weighing cell磁致弹性称重传感器magnitude-frequency characteristic幅频特性magnitude margin幅值裕度magnitude scale factor幅值比例尺manipulator机械手man-machine coordination人机协调manual station手动操作器MAP (manufacturing automation protocol)制造自动化协议marginal effectiveness边际效益Mason's gain formula梅森增益公式master station主站matching criterion匹配准则maximum likelihood estimation最大似然估计maximum overshoot最大超调量maximum principle极大值原理mean-square error criterion均方误差准则mechanism model机理模型meta-knowledge元知识metallurgical automation冶金自动化minimal realization最小实现minimum phase system最小相位系统minimum variance estimation最小方差估计minor loop副回路missile-target relative movement simulator弹体-目标相对运动仿真器modal aggregation模态集结modal transformation模态变换MB (model base)模型库model confidence模型置信度model fidelity模型逼真度model reference adaptive control system模型参考适应控制系统model verification模型验证modularization模块化MEC (most economic control)最经济控制motion space可动空间MTBF (mean time between failures)平均故障间隔时间MTTF (mean time to failures)平均无故障时间multi-attributive utility function多属性效用函数multicriteria多重判据multilevel hierarchical structure多级递阶结构multiloop control多回路控制multi-objective decision多目标决策multistate logic多态逻辑multistratum hierarchical control多段递阶控制multivariable control system多变量控制系统myoelectric control肌电控制Nash optimality纳什最优性natural language generation自然语言生成nearest-neighbor最近邻necessity measure必然性侧度negative feedback负反馈neural assembly神经集合neural network computer神经网络计算机Nichols chart尼科尔斯图noetic science思维科学noncoherent system非单调关联系统noncooperative game非合作博弈nonequilibrium state非平衡态nonlinear element非线性环节nonmonotonic logic非单调逻辑nonparametric training非参数训练nonreversible electric drive不可逆电气传动nonsingular perturbation非奇异摄动non-stationary random process非平稳随机过程nuclear radiation levelmeter核辐射物位计nutation sensor章动敏感器Nyquist stability criterion奈奎斯特稳定判据[next]objective function目标函数observability index可观测指数observable canonical form可观测规范型on-line assistance在线帮助on-off control通断控制open loop pole开环极点operational research model运筹学模型optic fiber tachometer光纤式转速表optimal trajectory最优轨迹optimization technique最优化技术orbital rendezvous轨道交会orbit gyrocompass轨道陀螺罗盘orbit perturbation轨道摄动order parameter序参数orientation control定向控制originator始发站oscillating period振荡周期output prediction method输出预估法oval wheel flowmeter椭圆齿轮流量计overall design总体设计overdamping过阻尼overlapping decomposition交叠分解Pade approximation帕德近似Pareto optimality帕雷托最优性passive attitude stabilization被动姿态稳定path repeatability路径可重复性pattern primitive模式基元PR (pattern recognition)模式识别P control比例控制器peak time峰值时间penalty function method罚函数法perceptron感知器periodic duty周期工作制perturbation theory摄动理论pessimistic value悲观值phase locus相轨迹phase trajectory相轨迹phase lead相位超前photoelectric tachometric transducer光电式转速传感器phrase-structure grammar短句结构文法physical symbol system物理符号系统piezoelectric force transducer压电式力传感器playback robot示教再现式机器人PLC (programmable logic controller)可编程序逻辑控制器plug braking反接制动plug valve旋塞阀pneumatic actuator气动执行机构point-to-point control点位控制polar robot极坐标型机器人pole assignment极点配置pole-zero cancellation零极点相消polynomial input多项式输入portfolio theory投资搭配理论pose overshoot位姿过调量position measuring instrument位置测量仪posentiometric displacement transducer电位器式位移传感器positive feedback正反馈power system automation电力系统自动化predicate logic谓词逻辑pressure gauge with electric contact电接点压力表pressure transmitter压力变送器price coordination价格协调primal coordination主协调primary frequency zone主频区PCA (principal component analysis)主成分分析法principle of turnpike大道原理priority优先级process-oriented simulation面向过程的仿真production budget生产预算production rule产生式规则profit forecast利润预测PERT (program evaluation and review technique)计划评审技术program set station程序设定操作器proportional control比例控制proportional plus derivative controller比例微分控制器protocol engineering协议工程prototype原型pseudo random sequence伪随机序列pseudo-rate-increment control伪速率增量控制pulse duration脉冲持续时间pulse frequency modulation control system脉冲调频控制系统pulse width modulation control system脉冲调宽控制系统PWM inverter脉宽调制逆变器pushdown automaton下推自动机QC (quality control)质量管理quadratic performance index二次型性能指标qualitative physical model定性物理模型quantized noise量化噪声quasilinear characteristics准线性特性queuing theory排队论radio frequency sensor射频敏感器ramp function斜坡函数random disturbance随机扰动random process随机过程rate integrating gyro速率积分陀螺ratio station比值操作器reachability可达性reaction wheel control反作用轮控制realizability可实现性,能实现性real time telemetry实时遥测receptive field感受野rectangular robot直角坐标型机器人rectifier整流器recursive estimation递推估计reduced order observer降阶观测器redundant information冗余信息reentry control再入控制regenerative braking回馈制动,再生制动regional planning model区域规划模型regulating device调节装载regulation调节relational algebra关系代数relay characteristic继电器特性remote manipulator遥控操作器remote regulating遥调remote set point adjuster远程设定点调整器rendezvous and docking交会和对接reproducibility再现性resistance thermometer sensor热电阻resolution principle归结原理resource allocation资源分配response curve响应曲线return difference matrix回差矩阵return ratio matrix回比矩阵reverberation回响reversible electric drive可逆电气传动revolute robot关节型机器人revolution speed transducer转速传感器rewriting rule重写规则rigid spacecraft dynamics刚性航天动力学risk decision风险分析robotics机器人学robot programming language机器人编程语言robust control鲁棒控制robustness鲁棒性roll gap measuring instrument辊缝测量仪root locus根轨迹roots flowmeter腰轮流量计rotameter浮子流量计,转子流量计rotary eccentric plug valve偏心旋转阀rotary motion valve角行程阀rotating transformer旋转变压器Routh approximation method劳思近似判据routing problem路径问题sampled-data control system采样控制系统sampling control system采样控制系统saturation characteristics饱和特性scalar Lyapunov function标量李雅普诺夫函数SCARA (selective compliance assembly robot arm)平面关节型机器人scenario analysis method情景分析法scene analysis物景分析s-domain s域self-operated controller自力式控制器self-organizing system自组织系统self-reproducing system自繁殖系统self-tuning control自校正控制semantic network语义网络semi-physical simulation半实物仿真sensing element敏感元件sensitivity analysis灵敏度分析sensory control感觉控制sequential decomposition顺序分解sequential least squares estimation序贯最小二乘估计servo control伺服控制,随动控制servomotor伺服马达settling time过渡时间sextant六分仪short term planning短期计划short time horizon coordination短时程协调signal detection and estimation信号检测和估计signal reconstruction信号重构similarity相似性simulated interrupt仿真中断simulation block diagram仿真框图simulation experiment仿真实验simulation velocity仿真速度simulator仿真器single axle table单轴转台single degree of freedom gyro单自由度陀螺single level process单级过程single value nonlinearity单值非线性singular attractor奇异吸引子singular perturbation奇异摄动sink汇点slaved system受役系统slower-than-real-time simulation欠实时仿真slow subsystem慢变子系统socio-cybernetics社会控制论socioeconomic system社会经济系统software psychology软件心理学solar array pointing control太阳帆板指向控制solenoid valve电磁阀source源点specific impulse比冲speed control system调速系统spin axis自旋轴spinner自旋体stability criterion稳定性判据stability limit稳定极限stabilization镇定,稳定Stackelberg decision theory施塔克尔贝格决策理论state equation model状态方程模型state space description状态空间描述static characteristics curve静态特性曲线station accuracy定点精度stationary random process平稳随机过程statistical analysis统计分析statistic pattern recognition统计模式识别steady state deviation稳态偏差steady state error coefficient稳态误差系数step-by-step control步进控制step function阶跃函数stepwise refinement逐步精化stochastic finite automaton随机有限自动机strain gauge load cell应变式称重传感器strategic function策略函数strongly coupled system强耦合系统subjective probability主观频率suboptimality次优性supervised training监督学习supervisory computer control system计算机监控系统sustained oscillation自持振荡swirlmeter旋进流量计switching point切换点symbolic processing符号处理synaptic plasticity突触可塑性synergetics协同学syntactic analysis句法分析system assessment系统评价systematology系统学system homomorphism系统同态system isomorphism系统同构system engineering系统工程tachometer转速表target flow transmitter靶式流量变送器task cycle作业周期teaching programming示教编程telemechanics远动学telemetering system of frequency division type频分遥测系统telemetry遥测teleological system目的系统teleology目的论temperature transducer温度传感器template base模版库tensiometer张力计texture纹理theorem proving定理证明therapy model治疗模型thermocouple热电偶thermometer温度计thickness meter厚度计three-axis attitude stabilization三轴姿态稳定three state controller三位控制器thrust vector control system推力矢量控制系统thruster推力器time constant时间常数time-invariant system定常系统,非时变系统time schedule controller时序控制器time-sharing control分时控制time-varying parameter时变参数top-down testing自上而下测试topological structure拓扑结构TQC (total quality control)全面质量管理tracking error跟踪误差trade-off analysis权衡分析transfer function matrix传递函数矩阵transformation grammar转换文法transient deviation瞬态偏差transient process过渡过程transition diagram转移图transmissible pressure gauge电远传压力表transmitter变送器trend analysis趋势分析triple modulation telemetering system三重调制遥测系统turbine flowmeter涡轮流量计Turing machine图灵机two-time scale system双时标系统ultrasonic levelmeter超声物位计unadjustable speed electric drive非调速电气传动unbiased estimation无偏估计underdamping欠阻尼uniformly asymptotic stability一致渐近稳定性uninterrupted duty不间断工作制,长期工作制unit circle单位圆unit testing单元测试unsupervised learing非监督学习upper level problem上级问题urban planning城市规划utility function效用函数value engineering价值工程variable gain可变增益,可变放大系数variable structure control system变结构控制vector Lyapunov function向量李雅普诺夫函数velocity error coefficient速度误差系数velocity transducer速度传感器vertical decomposition纵向分解vibrating wire force transducer振弦式力传感器vibrometer振动计viscous damping粘性阻尼voltage source inverter电压源型逆变器vortex precession flowmeter旋进流量计vortex shedding flowmeter涡街流量计WB (way base)方法库weighing cell称重传感器weighting factor权因子weighting method加权法Whittaker-Shannon sampling theorem惠特克-香农采样定理Wiener filtering维纳滤波work station for computer aided design计算机辅助设计工作站w-plane w平面zero-based budget零基预算zero-input response零输入响应zero-state response零状态响应zero sum game model零和对策模型z-transform z变换。
自动化专业英语IntroductionstoOptimalControlSystems课件

专业英语
12
2. 译成汉语的联合复句
He made notes as he was listening to the lecture. (时间状语从句译成汉语的 并列关系)
他边听课,边做记录。
2024年8月5日2时7分
专业英语
13
3. 译成汉语的简单句 When I make a speech, I get nervous. 我演讲时总是有些紧张。
7. compromise n.妥协,和解
8. tractable adj.易驾驭的
9. payload n.有效载荷[负载]
10. vehicle n.车辆,运载工具
11. residual
adj. 剩余的,残留的
12. propellant adj.推进的
n. 推进物,火药
13. velocity n.速度,速率,迅速
optimal control system within limits imposed by physical constraints是并 列宾语。连词that引导的宾语从句作介 词in的宾语。
2024年8月5日2时7分
专业英语
5
(2) In solving problems of optimal control systems, we may have the goal of finding a rule for determining the present control decision, subject to certain constraints which will minimize some measure of a deviation from ideal behavior.
电气工程与其自动化专业_外文文献_英文文献_外文翻译_plc方面

1、外文原文A: Fundamentals of Single-chip MicrocomputerTh e si ng le -c hi p mic ro co mput er i s t he c ul mi na ti on of both t h e de ve lo pmen t o f t he d ig it al co m pu te r an d th e i n te gr at ed c i rc ui t a rg ua bl y t h e to w mos t s ig ni f ic an t i nv en ti on s of t he 20th c e nt ur y [1].Th es e t ow ty pe s of ar ch it ec tu re a re fo un d i n s in gle -ch i p m i cr oc ompu te r. So me em pl oy t he spl i t pr og ra m/da ta memory o f th e Ha rv ar d ar ch it ect ure , sh own in Fi g.3-5A-1, o th ers fo ll ow t he ph il os op hy , wi del y a da pt ed f or ge ner al -pur po se co m pu te rs a nd m i cr op ro ce ss or s, o f maki ng n o log i ca l di st in ct ion be tw ee n pr og ra m an d d at a memory a s i n t he P r in ce to n ar ch ite c tu re , sh own i n F ig.3-5A-2.In g en er al te r ms a s in gl e -chi p m ic ro co mput er i sc h ar ac te ri zed by t he i nc or po ra ti on of a ll t he un it s of a co mputer i n to a s in gl e d ev i ce , as s ho wn in Fi g3-5A-3.Fig.3-5A-1 A Harvard typeProgrammemory DatamemoryCPU Input&Outputunitmemory CPU Input&OutputunitFig.3-5A-2. A conventional Princeton computerReset Interrupts PowerFig3-5A-3. Principal features of a microcomputerRead only memory (ROM).R OM i s us ua ll y f or th e p erm an ent, no n-vo la ti le s tor age o f an a pp lic ati on s pr og ra m .Man ym i cr oc ompu te rs an d m ar e in te nd e d f or hi gh -v ol ume a ppl ic at ions an d he nc e t he eco nomic al m an uf act ure o f th e de vic es re qu ir es t h at t he co nt en t s of t he pr og ra m mem or y b e co mm it t ed pe rm ane ntly du ri ng t he m an ufa c tu re o f ch ip s .Cl ea rl y, t hi s i mpl ie s a r i go ro us a pp ro ach to R OM c od e de ve l op ment s in ce ch ang es c an not be mad e af te r manu f ac tu re .Th is d ev elo pmen t pr oc ess ma y in vo lv e emul at io n us in g a so ph is ti ca te d d eve lo pmen t sy ste m w it h a ha rd ware e mula tio n c ap ab il it y as wel l as t he u se o f po werf ul s o ft ware t oo ls.Some m an uf act ure rs p ro vi de ad d it io na l ROM opt i on s byi n cl ud in g i n th eir r ange d ev ic es wi t h (or i nt en de d f or u se wit h)us er p ro gr ammable memory. Th e sim ple st o f th es e i s u su al lyde vi ce w hi ch c an o per at e in a mi cro pro ce ss or mod e b y u si ng s ome of t he i np ut /o utp ut li ne s as a n a ddr es s an d da ta b us f or ac ce ss in g ex te rna l m emor y. T hi s t y pe o f de vi ce ca n b eh av eExternalTimingcomponents System clock Timer/ CounterSerial I/OPrarallelI/ORAMROMCPUf u nc ti on al ly a s t he si ng le ch ip mi cr oc ompu te r fro m w hi ch it is de ri ve d al be it wi t h re st ri ct ed I/O a nd a m od if ied ex te rn alc i rc ui t. Th e u se o f th es e dev ic es i s c ommon e ve n i n pr od uc ti on c i rc ui ts wh ere t he vo lu me do es no t j us tif y t h e dev el opmen t costsof c us to m o n-ch i p ROM[2];t he re c a n s ti ll be a s ig nif i ca nt sa vingi n I/O an d o th er c hip s c ompa re d t o a co nv en ti on al mi c ro pr oc es sor ba se d ci rc ui t. Mo r e ex ac t re pl ace m en t fo r RO M dev i ce s ca n be ob ta in ed i n th e f orm o f va ri an ts wit h 'p ig gy-b ack'EPRO M(Er as ab le pr o gr ammabl e RO M )s oc ke ts o r d ev ic e s wi th EP ROM i n st ea d of ROM 。
自动化专业中英文对照外文翻译文献
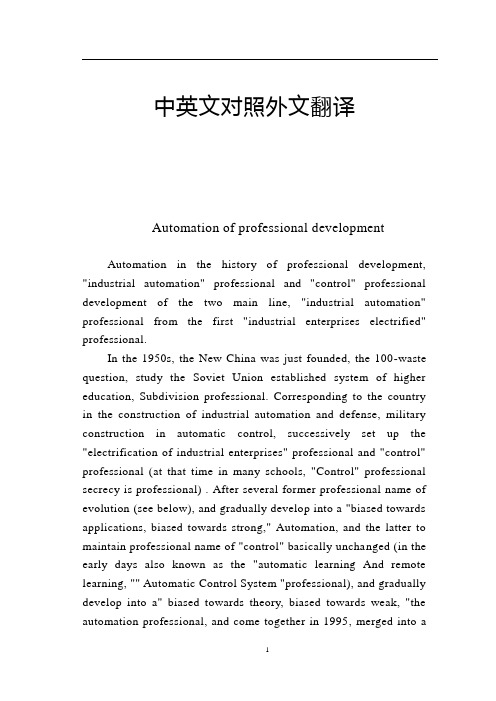
中英文对照外文翻译Automation of professional developmentAutomation in the history of professional development, "industrial automation" professional and "control" professional development of the two main line, "industrial automation" professional from the first "industrial enterprises electrified" professional.In the 1950s, the New China was just founded, the 100-waste question, study the Soviet Union established system of higher education, Subdivision professional. Corresponding to the country in the construction of industrial automation and defense, military construction in automatic control, successively set up the "electrification of industrial enterprises" professional and "control" professional (at that time in many schools, "Control" professional secrecy is professional) . After several former professional name of evolution (see below), and gradually develop into a "biased towards applications, biased towards strong," Automation, and the latter to maintain professional name of "control" basically unchanged (in the early days also known as the "automatic learning And remote learning, "" Automatic Control System "professional), and gradually develop into a" biased towards theory, biased towards weak, "the automation professional, and come together in 1995, merged into aunified" automatic "professional . In 1998, according to the Ministry of Education announced the latest professional undergraduate colleges and universities directory, adjusted, the merger of the new "automated" professional include not only the original "automatic" professional (including "industrial automation" professional and "control" professional ), Also increased the "hydraulic transmission and control of" professional (part), "electrical technology" professional (part) and "aircraft guidance and control of" professional (part).Clearly, one of China's automation professional history of the development of China's higher education actually is a new development of the cause of a microcosm of the history, but also the history of New China industrial development of a miniature. Below "industrial automation" professional development of the main line of this example, a detailed review of its development process in the many professional name change (in real terms in the professional content changes) and its industrial building at the time of the close relationship.First a brief look at the world and China's professional division history. We know that now use the professional division is largely from the 19th century to the beginning of the second half of the first half of the 20th century stereotypes of the engineering, is basically industry (products) for the objects to the division, they have been the image of people Known as the "industry professionals" or "trade associations." At present the international education system in two categories, with Britain and the United States as the representative of the education system not yet out of "industry professionals" system, but has taken the "generalist" the road of education and the former Soviet Union for Europe (close to the Soviet Union) as the representative The education system, at the beginning of theimplementation of "professionals" education, professional-very small, although reforms repeatedly, but to the current "industry professionals" are still very obvious characteristics.In the 1950s, just after the founding of New China, a comprehensive study and the Soviet Union and sub-professional very small; Since reform and opening up, only to Britain and the United States to gradually as the representative of the education system to move closer, and gradually reduce the professional, the implementation of "generalist" education through a number of professional Restructuring and merger (the total number of professionals from the maximum of 1,343 kinds of gradually reducing the current 249 kinds), although not out of "industry professionals" and "Mei Ming," but many of the colleges and universities, mostly only one of a Professional, rather than the past more than a professional.Before that, China's first professional automation from the National University in 1952 when the first major readjustment of the establishment of professional - electrified professional industrial enterprises. At that time, the Soviet Union assistance to the construction of China's 156 large industrial enterprises, automation of much-needed electrical engineering and technical personnel, and such professional and technical personnel training, and then was very consistent with China's industrial construction. By the 1960s, professional name changed to "industrial electric and automation," the late 1970s when to resume enrollment "Electric Industrial Automation" professional. This is not only professional name changes, but has its profound meaning, it reflects China's industries from "electrified" step by step to the "automatic" into the real history and that part of the development trend of China's automation professional reflects how urgent countries Urgent for the country'seconomic construction services that period of history and development of real direction.1993, after four years of the third revision of the undergraduate professional directories, the State Education Commission issued a call "system integrity, more scientific and reasonable, the harmonization of norms," the "ordinary professional directory of undergraduate colleges and universities." "Electric Industrial Automation" and "production process automation" merger of the two professional electrician to set up a kind of "industrial automation" professional, by the then Ministry of Industry Machinery centralized management colleges and universities to set up industrial automation teaching guide at the Commission, responsible for the "Industrial Automation "professional teaching and guiding work at the same time," Control "was attributable to the professional category of electronic information, the then Ministry of Industry of electronic centralized management control to set up colleges and universities teaching guide at the Commission, responsible for the" control " Professional teaching guide our work. After the professional adjustment, further defined the "industrial automation" professional and "control" professional "- both strong and weak, hardware and software into consideration and control theory and practical system integration, and the movement control, process control and other targets of control "The common characteristics with the training objectives, but also the basic set of" industrial automation "biased towards strong, professional, biased towards applications," Control "professional biased towards weak, biased towards the theory of professional characteristics and pattern of division of labor. 1995, the State Education Commission promulgated the "(University) undergraduate engineering leading professional directory", the electrical category "industrialautomation" professional and the original electronic information such as "control" of professional electronic information into a new category of "automatic" professional . As this is the leading professional directory, are not enforced, coupled with general "industrial automation" strong or weak, both professional "into" a weak professional category of electronic information is not conducive to professional development and thus many Schools remain "industrial automation" professional and "control" the situation of professional co-exist. Since 1996 more, again commissioned by the Ministry of National Education Ministry of Industry and electronic machinery industries of other parts of the establishment of the new session (second session) centralized management guidance at the University Teaching Commission, making the leading professionals have not been effective Implemented.1998, to meet the country's economic construction of Kuan Koujing personnel training needs, further consolidation of professional and international "generalist" education track by the Ministry of Education announced a fourth revision of the latest "Universities Undergraduate Catalog." So far in the use of the directory, the total number of professionals from the third amendments to the 504 kinds of substantially reduced to 249 species, the original directory is strong, professional electrician and a weak professional category such as electronics and information into categories Electric power, the unity of Information, a former electrician at the same time kind of "industrial automation" professional and the type of electronic information "control" professional formal merger, together with the "hydraulic transmission and control of" professional (part) , "Electric technology" professional (part) and "aircraft guidance and controlof" professional (part), the composition of the new (enforcement) are electrical information such as "automatic" professional. According to statistics, so far the country has more than 200 colleges and universities set up this kind of "automatic" professional. If the name of automation as part of their professional expertise (such as "electrical engineering and automation," "mechanical design and manufacturing automation," "agricultural mechanization and automation" and other professionals) included Automation has undoubtedly is the largest in China A professional.Of the characteristics of China's automation professional:Recalling China's professional history of the development of automation, combined with the corresponding period of the construction of China's national economy to the demand for automation and automated the development of the cause, it is not difficult to sum up following professional characteristics:(1) China's automation professional is not only a relatively long history (since 1952 have been more than 50 years), and from the first day of the establishment of professional automation, has been a professional one of the countries in urgent need, therefore the number of students has also been The largest and most employers welcome the allocation of the professional one.(2) China's automation is accompanied by a professional from the electrification of China's industrial automation step by step to the development of stable development, professional direction and the main content from the first prominent electrified "the electrification of industrial enterprises" step by step for the development of both the electric and automation " Industrial electric and automation ", highlighting the electrical automation" Electric Industrial Automation "and prominent automation" industrial automation ", then the merger of professional education reform in1995 and" control "of professional content into a broader" automated " Professional. From which we can see that China's automation professional Although the initial study in the Soviet education system established under the general environment, but in their development and the Soviet Union or the United States and Britain did not copy the mode, but with China's national conditions (to meet national needs for The main goal) from the innovation and development of "cross-industry professionals," features the professional.自动化专业的发展自动化专业的发展历史中,有“工业自动化”专业与“自动控制”专业两条发展主线,其中“工业自动化”专业最早源于“工业企业电气化”专业。
自动化专业英语翻译

Control Engineering and TechnologySome Advances in TechnologyControl engineering is driven by available technology and the pace of the relevant technology advances is now rapid . In this section we mention a few of the advances in technology that currently have ,or will have ,an impact on control engineering . More specific details can be found in the Notes and References at the end of this chapter.1.3 控制工程和技术1.3.1 一些技术上的进步控制工程是由可用的技术和相关技术快速进步的步伐所推动的。
在本节中,我们提到的一些目前拥有或将要拥有的技术进步以及它在控制工程上的影响。
更具体的细节可以在本章末尾的注释和参考中被找到。
note n. 注释,说明;[金融] 票据reference n. [图情] 参考文献;参照;推荐信Integrated and Intelligent SensorsOver the past decade the technology of integrated sensors has been developed . Integrated sensors are built using the techniques of microfabrication originally developed for integrated circuits ; they often include the signal conditioning and interface circuitry on the same chip, in which case they are called intelligent sensors. This signal conditioning might include, for example, temperature compensation. Integrated sensors promise greater reliability and linearity than many conventional sensors, and because they are typically cheaper and smaller than conventional sensors, it will be possible to incorporate many more sensors in the design of control systems than is currently done.集成智能传感器在过去的十年中集成传感器的技术已经得到了发展。
信息自动化专业外文翻译
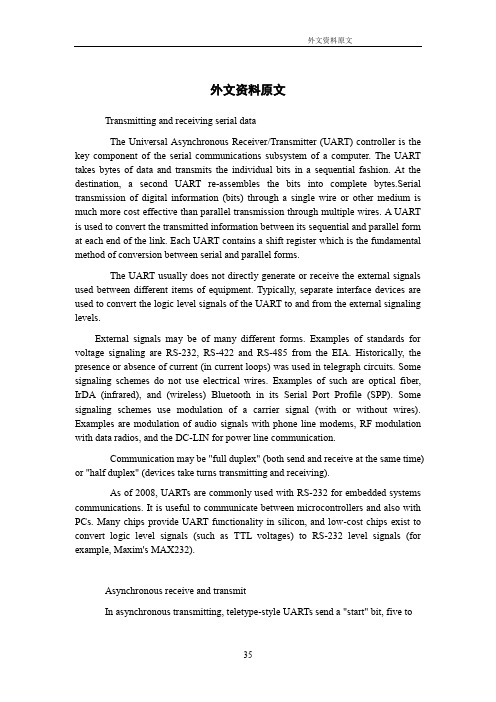
外文资料原文外文资料原文Transmitting and receiving serial dataThe Universal Asynchronous Receiver/Transmitter (UART) controller is the key component of the serial communications subsystem of a computer. The UART takes bytes of data and transmits the individual bits in a sequential fashion. At the destination, a second UART re-assembles the bits into complete bytes.Serial transmission of digital information (bits) through a single wire or other medium is much more cost effective than parallel transmission through multiple wires. A UART is used to convert the transmitted information between its sequential and parallel form at each end of the link. Each UART contains a shift register which is the fundamental method of conversion between serial and parallel forms.The UART usually does not directly generate or receive the external signals used between different items of equipment. Typically, separate interface devices are used to convert the logic level signals of the UART to and from the external signaling levels.External signals may be of many different forms. Examples of standards for voltage signaling are RS-232, RS-422 and RS-485 from the EIA. Historically, the presence or absence of current (in current loops) was used in telegraph circuits. Some signaling schemes do not use electrical wires. Examples of such are optical fiber, IrDA (infrared), and (wireless) Bluetooth in its Serial Port Profile (SPP). Some signaling schemes use modulation of a carrier signal (with or without wires). Examples are modulation of audio signals with phone line modems, RF modulation with data radios, and the DC-LIN for power line communication.Communication may be "full duplex" (both send and receive at the same time) or "half duplex" (devices take turns transmitting and receiving).As of 2008, UARTs are commonly used with RS-232 for embedded systems communications. It is useful to communicate between microcontrollers and also with PCs. Many chips provide UART functionality in silicon, and low-cost chips exist to convert logic level signals (such as TTL voltages) to RS-232 level signals (for example, Maxim'sMAX232).Asynchronous receive and transmitIn asynchronous transmitting, teletype-style UARTs send a "start" bit, five to电子科技大学成都学院毕业设计论文eight data bits, least-significant-bit first, an optional "parity" bit, and then one, one and a half, or two "stop" bits. The start bit is the opposite polarity of the data-line's idle state. The stop bit is the data-line's idle state, and provides a delay before the next character can start. (This is called asynchronous start-stop transmission). In mechanical teletypes, the "stop" bit was often stretched to two bit times to give the mechanism more time to finish printing a character. A stretched "stop" bit also helps resynchronization.The parity bit can either make the number of "one" bits between any start/stop pair odd, or even, or it can be omitted. Odd parity is more reliable because it assures that there will always be at least one data transition, and this permits many UARTs to resynchronize.In synchronous transmission, the clock data is recovered separately from the data stream and no start/stop bits are used. This improves the efficiency of transmission on suitable channels since more of the bits sent are usable data and not character framing. An asynchronous transmission sends no characters over the interconnection when the transmitting device has nothing to send -- only idle stop bits; but a synchronous interface must send "pad" characters to maintain synchronism between the receiver and transmitter. The usual filler is the ASCII "SYN" character. This may be done automatically by the transmitting device.USART chips have both synchronous and asynchronous modes.Serial to Parallel AlgorithmAsynchronous transmission allows data to be transmitted without the sender having to send a clock signal to the receiver. Instead, the sender and receiver must agree on timing parameters in advance and special bits are added to each word which are used to synchronize the sending and receiving units.When a word is given to the UART for Asynchronous transmissions, a bit called the "Start Bit" is added to the beginning of each word that is to be transmitted. The Start Bit is used to alert the receiver that a word of data is about to be sent, and to force the clock in the receiver into synchronization with the clock in the transmitter. These two clocks must be accurate enough to not have the frequency drift by more than 10% during the transmission of the remaining bits in the word. (This requirement was set in the days of mechanical teleprinters and is easily met by modern electronic equipment.)After the Start Bit, the individual bits of the word of data are sent, with the Least Significant Bit (LSB) being sent first. Each bit in the transmission is transmitted外文资料for exactly the same amount of time as all of the other bits, and the receiver “looks” at the wire at approximately halfway through the period assigned to each bit to determine if the bit is a 1 or a 0. For example, if it takes two seconds to send each bit, the receiver will examine the signal to determine if it is a 1 or a 0 after one second has passed, then it will wait two seconds and then examine the value of the next bit, and so on.The sender does not know when the receiver has “looked” at the value of the bit. The sender only knows when the clock says to begin transmitting the next bit of the word.When the entire data word has been sent, the transmitter may add a Parity Bit that the transmitter generates. The Parity Bit may be used by the receiver to perform simple error checking. Then at least one Stop Bit is sent by the transmitter.When the receiver has received all of the bits in the data word, it may check for the Parity Bits (both sender and receiver must agree on whether a Parity Bit is to be used), and then the receiver looks for a Stop Bit. If the Stop Bit does not appear when it is supposed to, the UART considers the entire word to be garbled and will report a Framing Error to the host processor when the data word is read. The usual cause of a Framing Error is that the sender and receiver clocks were not running at the same speed, or that the signal was interrupted.Regardless of whether the data was received correctly or not, the UART automatically discards the Start, Parity and Stop bits. If the sender and receiver are configured identically, these bits are not passed to the host. If another word is ready for transmission, the Start Bit for the new word can be sent as soon as the Stop Bit for the previous word has been sent. Because asynchronous data is “self synchronizing”, if there is no data to transmit, the transmission line can be idle. A data communicationpulse can only be in one of two states but there are many names for the two states. When on, circuit closed, low voltage, current flowing, or a logical zero, the pulse is said to be in the "space" condition. When off, circuit open, high voltage, current stopped, or a logical one, the pulse is said to be in the "mark" condition. A character code begins with the data communication circuit in the space condition. If the mark condition appears, a logical one is recorded otherwise a logical zero.Figure 1 shows this format.The start bit is always a 0 (logic low), which is also called a space. The start bit signals the receiving DTE that a character code is coming. The next five to eight bits, depending on the code set employed, represent the character. In the ASCII code set the eighth data bit may be a parity bit. The next one or two bits are always in the电子科技大学成都学院毕业设计论文mark (logic high, i.e., '1') condition and called the stop bit(s). They provide a "rest" interval for the receiving DTE so that it may prepare for the next character which may be after the stop bit(s). The rest interval was required by mechanical Teletypes which used a motor driven camshaft to decode each character. At the end of each character the motor needed time to strike the character bail (print the character) and reset the camshaft.All operations of the UART hardware are controlled by a clock signal which runs at a multiple (say, 16) of the data rate - each data bit is as long as 16 clock pulses. The receiver tests the state of the incoming signal on each clock pulse, looking for the beginning of the start bit. If the apparent start bit lasts at least one-half of the bit time, it is valid and signals the start of a new character. If not, the spurious pulse is ignored. After waiting a further bit time, the state of the line is again sampled and the resulting level clocked into a shift register. After the required number of bit periods for the character length (5 to 8 bits, typically) have elapsed, the contents of the shift register is made available (in parallel fashion) to the receiving system. The UART will set a flag indicating new data is available, and may also generate a processor interrupt to request that the host processor transfers the received data. In some common types of UART, a small first-in, first-out (FIFO) buffer memory is inserted between the receiver shift register and the host system interface. This allows the host processor more time to handle an interrupt from the UART and prevents loss of received data at high rates.Transmission operation is simpler since it is under the control of the transmitting system. As soon as data is deposited in the shift register, the UART hardware generates a start bit, shifts the required number of data bits out to the line,generates and appends the parity bit (if used), and appends the stop bits. Since transmission of a single character may take a long time relative to CPU speeds, the UART will maintain a flag showing busy status so that the host system does not deposit a new character for transmission until the previous one has been completed; this may also be done with an interrupt. Since full-duplex operation requires characters to be sent and received at the same time, practical UARTs use two different shift registers for transmitted characters and received characters.Transmitting and receiving UARTs must be set for the same bit speed, character length, parity, and stop bits for proper operation. The receiving UART may detect some mismatched settings and set a "framing error" flag bit for the host system; in exceptional cases the receiving UART will produce an erratic stream of mutilated characters and transfer them to the host system.Typical serial ports used with personal computers connected to modems use eight data bits, no parity, and one stop bit; for this configuration the number of ASCII外文原文character per seconds equals the bit rate divided by 10.HistorySome early telegraph schemes used variable-length pulses (as in Morse code) and rotating clockwork mechanisms to transmit alphabetic characters. The first UART-like devices (with fixed-length pulses) were rotating mechanical switches (commutators). These sent 5-bit Baudot codes for mechanical teletypewriters, and replaced morse code. Later, ASCII required a seven bit code. When IBM built computers in the early 1960s with 8-bit characters, it became customary to store the ASCII code in 8 bits.Gordon Bell designed the UART for the PDP series of computers. Western Digital made the first single-chip UART WD1402A around 1971; this was an early example of a medium scale integrated circuit.An example of an early 1980s UART was the National Semiconductor8250. In the 1990s, newer UARTs were developed with on-chip buffers. This allowed higher transmission speed without data loss and without requiring such frequent attention from the computer. For example, the popular National Semiconductor 16550 has a 16 byte FIFO, and spawned many variants, including the 16C550, 16C650, 16C750, and 16C850.Depending on the manufacturer, different terms are used to identify devices that perform the UART functions. Intel called their 8251 device a "Programmable Communication Interface". MOS Technology6551 was known under the name "Asynchronous Communications Interface Adapter" (ACIA). The term "Serial Communications Interface" (SCI) was first used at Motorola around 1975 to refer to their start-stop asynchronous serial interface device, which others were calling a UART.Some very low-cost home computers or embedded systems dispensed with a UART and used the CPU to sample the state of an input port or directly manipulate an output port for data transmission. While very CPU-intensive, since the CPU timing was critical, these schemes avoided the purchase of a costly UART chip. The technique was known as a bit-banging serial port.UART models8250, 16450, early 16550: Obsolete with 1-byte buffers16550, 16550A, 16C552: 16-byte buffers, TL=1,4,8,14; 115.2 kbps standard, many support 230.4 or 460.8 kbps16650: 32-byte buffers. 460.8 kbps电子科技大学成都学院毕业设计论文16750: 64-byte buffer for send, 56-byte for receive. 921.6 kbps16850, 16C850: 128-byte buffers. 460.8 kbps or 1.5 mbps16950Hayes ESP: 1k-byte buffers.StructureA UART usually contains the following components:a clock generator, usually a multiple of the bit rate to allow sampling in the middle of a bit period.input and output shift registerstransmit/receive controlread/write control logictransmit/receive buffers (optional)parallel data bus buffer (optional)First-in, first-out (FIFO) buffer memory (optional)Special Receiver ConditionsOverrun ErrorAn "overrun error" occurs when the UART receiver cannot process the character that just came in before the next one arrives. Various UART devices have differing amounts of buffer space to hold received characters. The CPU must service the UART in order to remove characters from the input buffer. If the CPU does not service the UART quickly enough and the buffer becomes full, an Overrun Error will occur.Underrun ErrorAn "underrun error" occurs when the UART transmitter has completed sending a character and the transmit buffer is empty. In asynchronous modes this is treated as an indication that no data remains to be transmitted, rather than an error, since additional stop bits can be appended. This error indication is commonly found in USARTs, since an underrun is more serious in synchronous systems.Framing Error外文原文A "framing error" occurs when the designated "start" and "stop" bits are not valid. As the "start" bit is used to identify the beginning of an incoming character, itacts as a reference for the remaining bits. If the data line is not in the expected idle state when the "stop" bit is expected, a Framing Error will occur.Parity ErrorA "parity error" occurs when the number of "active" bits does not agree with the specified parity configuration of the UART, producing a Parity Error. Because the "parity" bit is optional, this error will not occur if parity has been disabled. Parity error is set when the parity of an incoming data character does not match the expected value.Break ConditionA "break condition" occurs when the receiver input is at the "space" level for longer than some duration of time, typically, for more than a character time. This is not necessarily an error, but appears to the receiver as a character of all zero bits with a framing error.Some equipment will deliberately transmit the "break" level for longer than a character as an out-of-band signal. When signaling rates are mismatched, no meaningful characters can be sent, but a long "break" signal can be a useful way to get the attention of a mismatched receiver to do something (such as resetting itself). UNIX systems and UNIX-like systems such as Linux can use the long "break" level as a request to change the signaling rate.BaudrateIn embedded designs, it is necessary to choose proper oscillator to get correct baud rate with small or no error. Some examples of common crystal frequencies and baud rates with no error:18.432 MHz: 300, 600, 1200, 2400, 4800, 9600, 19200 Bd22.118400 MHz: 300, 600, 1200, 1800, 2400, 4800, 7200, 9600, 14400, 19200, 38400, 57600, 115200 Bd16 MHz: 125000, 500000 BdSee also电子科技大学成都学院毕业设计论文Asynchronous serial communicationBaudbit rateModemMorse codeSerial communicationSerial portUSB16550 UART8250 UARTExternal linksA tutorial on the RS-232 standard, describing the definition of mark, space and their relationship with negative and positive voltagesFreebsd Tutorials (includes standard signal definitions, history of UART ICs, and pinout for commonly used DB25 connector)UART Tutorial for Robotics (contains many practical examples)译文通用异步接收/发送器是一种“异步接收器/发射器” ,一块电脑硬件的转换之间的数据并行和串行的形式。
自动化专业英语原文和翻译

自动化专业英语原文和翻译英文原文:Automation is the technology by which a process or procedure is performed with minimal human assistance. Automation or automatic control is the use of various control systems for operating equipment such as machinery, processes in factories, boilers, and heat treating ovens, switching on telephone networks, steering, and stabilization of ships, aircraft, and other applications and vehicles with minimal or reduced human intervention. Some processes have been completely automated.自动化是一种通过至少的人力辅助来执行过程或者程序的技术。
自动化或者自动控制是使用各种控制系统来操作设备,例如机械、工厂中的工艺流程、锅炉和热处理炉、电话网络的开关、船舶、飞机和其他应用和车辆的控制和稳定,从而实现最小化或者减少人类干预。
一些过程已经彻底自动化。
Automation plays a crucial role in various industries and sectors, including manufacturing, transportation, healthcare, and many others. It involves the use of advanced technologies and control systems to streamline processes, improve efficiency, and reduce human error.In the manufacturing industry, automation is used extensively to carry out repetitive tasks, such as assembly line operations. This not only speeds up production but also ensures consistent quality and reduces the risk of accidents. Robots and robotic systems are commonly employed in manufacturing plants to handle tasks that are dangerous or require high precision.在创造业中,自动化被广泛应用于执行重复性任务,例如流水线操作。
自动化控制工程外文翻译外文文献英文文献
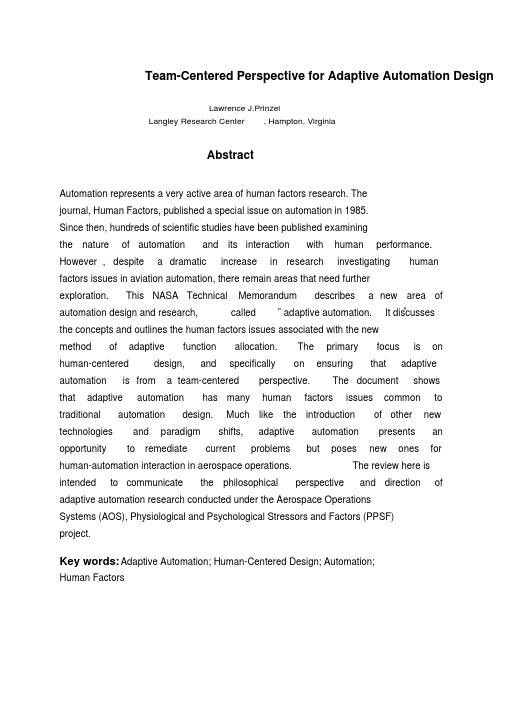
Team-Centered Perspective for Adaptive Automation DesignLawrence J.PrinzelLangley Research Center, Hampton, VirginiaAbstractAutomation represents a very active area of human factors research. Thejournal, Human Factors, published a special issue on automation in 1985.Since then, hundreds of scientific studies have been published examiningthe nature of automation and its interaction with human performance.However, despite a dramatic increase in research investigating humanfactors issues in aviation automation, there remain areas that need furtherexploration. This NASA Technical Memorandum describes a new area ofIt discussesautomation design and research, called “adaptive automation.” the concepts and outlines the human factors issues associated with the newmethod of adaptive function allocation. The primary focus is onhuman-centered design, and specifically on ensuring that adaptiveautomation is from a team-centered perspective. The document showsthat adaptive automation has many human factors issues common totraditional automation design. Much like the introduction of other new technologies and paradigm shifts, adaptive automation presents an opportunity to remediate current problems but poses new ones forhuman-automation interaction in aerospace operations. The review here isintended to communicate the philosophical perspective and direction ofadaptive automation research conducted under the Aerospace OperationsSystems (AOS), Physiological and Psychological Stressors and Factors (PPSF)project.Key words:Adaptive Automation; Human-Centered Design; Automation;Human FactorsIntroduction"During the 1970s and early 1980s...the concept of automating as much as possible was considered appropriate. The expected benefit was a reduction inpilot workload and increased safety...Although many of these benefits have beenrealized, serious questions have arisen and incidents/accidents that have occurredwhich question the underlying assumptions that a maximum availableautomation is ALWAYS appropriate or that we understand how to designautomated systems so that they are fully compatible with the capabilities andlimitations of the humans in the system."---- ATA, 1989The Air Transport Association of America (ATA) Flight Systems Integration Committee(1989) made the above statement in response to the proliferation of automation in aviation. They noted that technology improvements, such as the ground proximity warning system, have had dramatic benefits; others, such as the electronic library system, offer marginal benefits at best. Such observations have led many in the human factors community, most notably Charles Billings (1991; 1997) of NASA, to assert that automation should be approached from a "human-centered design" perspective.The period from 1970 to the present was marked by an increase in the use of electronic display units (EDUs); a period that Billings (1997) calls "information" and “management automation." The increased use of altitude, heading, power, and navigation displays; alerting and warning systems, such as the traffic alert and collision avoidance system (TCAS) and ground proximity warning system (GPWS; E-GPWS; TAWS); flight management systems (FMS) and flight guidance (e.g., autopilots; autothrottles) have "been accompanied by certain costs, including an increased cognitive burden on pilots, new information requirements that have required additional training, and more complex, tightly coupled, less observable systems" (Billings, 1997). As a result, human factors research in aviation has focused on the effects of information and management automation. The issues of interest include over-reliance on automation, "clumsy" automation (e.g., Wiener, 1989), digital versus analog control, skill degradation, crew coordination, and data overload (e.g., Billings, 1997). Furthermore, research has also been directed toward situational awareness (mode & state awareness; Endsley, 1994; Woods & Sarter, 1991) associated with complexity, coupling, autonomy, and inadequate feedback. Finally, human factors research has introduced new automation concepts that will need to be integrated into the existing suite of aviationautomation.Clearly, the human factors issues of automation have significant implications for safetyin aviation. However, what exactly do we mean by automation? The way we choose to define automation has considerable meaning for how we see the human role in modern aerospace s ystems. The next section considers the concept of automation, followed by an examination of human factors issues of human-automation interaction in aviation. Next, a potential remedy to the problems raised is described, called adaptive automation. Finally, the human-centered design philosophy is discussed and proposals are made for how the philosophy can be applied to this advanced form of automation. The perspective is considered in terms of the Physiological /Psychological Stressors & Factors project and directions for research on adaptive automation.Automation in Modern AviationDefinition.Automation refers to "...systems or methods in which many of the processes of production are automatically performed or controlled by autonomous machines or electronic devices" (Parsons, 1985). Automation is a tool, or resource, that the human operator can use to perform some task that would be difficult or impossible without machine aiding (Billings, 1997). Therefore, automation can be thought of as a process of substituting the activity of some device or machine for some human activity; or it can be thought of as a state of technological development (Parsons, 1985). However, some people (e.g., Woods, 1996) have questioned whether automation should be viewed as a substitution of one agent for another (see "apparent simplicity, real complexity" below). Nevertheless, the presence of automation has pervaded almost every aspect of modern lives. From the wheel to the modern jet aircraft, humans have sought to improve the quality of life. We have built machines and systems that not only make work easier, more efficient, and safe, but also give us more leisure time. The advent of automation has further enabled us to achieve this end. With automation, machines can now perform many of the activities that we once had to do. Our automobile transmission will shift gears for us. Our airplanes will fly themselves for us. All we have to dois turn the machine on and off. It has even been suggested that one day there may not be aaccidents resulting from need for us to do even that. However, the increase in “cognitive” faulty human-automation interaction have led many in the human factors community to conclude that such a statement may be premature.Automation Accidents. A number of aviation accidents and incidents have been directly attributed to automation. Examples of such in aviation mishaps include (from Billings, 1997):DC-10 landing in control wheel steering A330 accident at ToulouseB-747 upset over Pacific DC-10 overrun at JFK, New YorkB-747 uncommandedroll,Nakina,Ont. A320 accident at Mulhouse-HabsheimA320 accident at Strasbourg A300 accident at NagoyaB-757 accident at Cali, Columbia A320 accident at BangaloreA320 landing at Hong Kong B-737 wet runway overrunsA320 overrun at Warsaw B-757 climbout at ManchesterA310 approach at Orly DC-9 wind shear at CharlotteBillings (1997) notes that each of these accidents has a different etiology, and that human factors investigation of causes show the matter to be complex. However, what is clear is that the percentage of accident causes has fundamentally shifted from machine-caused to human-caused (estimations of 60-80% due to human error) etiologies, and the shift is attributable to the change in types of automation that have evolved in aviation.Types of AutomationThere are a number of different types of automation and the descriptions of them vary considerably. Billings (1997) offers the following types of automation:?Open-Loop Mechanical or Electronic Control.Automation is controlled by gravity or spring motors driving gears and cams that allow continous and repetitive motion. Positioning, forcing, and timing were dictated by the mechanism and environmental factors (e.g., wind). The automation of factories during the Industrial Revolution would represent this type of automation.?Classic Linear Feedback Control.Automation is controlled as a function of differences between a reference setting of desired output and the actual output. Changes a re made to system parameters to re-set the automation to conformance. An example of this type of automation would be flyball governor on the steam engine. What engineers call conventional proportional-integral-derivative (PID) control would also fit in this category of automation.?Optimal Control. A computer-based model of controlled processes i s driven by the same control inputs as that used to control the automated process. T he model output is used to project future states and is thus used to determine the next control input. A "Kalman filtering" approach is used to estimate the system state to determine what the best control input should be.?Adaptive Control. This type of automation actually represents a number of approaches to controlling automation, but usually stands for automation that changes dynamically in response to a change in state. Examples include the use of "crisp" and "fuzzy" controllers, neural networks, dynamic control, and many other nonlinear methods.Levels of AutomationIn addition to “types ” of automation, we can also conceptualize different “levels ” of automation control that the operator can have. A number of taxonomies have been put forth, but perhaps the best known is the one proposed by Tom Sheridan of Massachusetts Institute of Technology (MIT). Sheridan (1987) listed 10 levels of automation control:1. The computer offers no assistance, the human must do it all2. The computer offers a complete set of action alternatives3. The computer narrows the selection down to a few4. The computer suggests a selection, and5. Executes that suggestion if the human approves, or6. Allows the human a restricted time to veto before automatic execution, or7. Executes automatically, then necessarily informs the human, or8. Informs the human after execution only if he asks, or9. Informs the human after execution if it, the computer, decides to10. The computer decides everything and acts autonomously, ignoring the humanThe list covers the automation gamut from fully manual to fully automatic. Although different researchers define adaptive automation differently across these levels, the consensus is that adaptive automation can represent anything from Level 3 to Level 9. However, what makes adaptive automation different is the philosophy of the approach taken to initiate adaptive function allocation and how such an approach may address t he impact of current automation technology.Impact of Automation TechnologyAdvantages of Automation . Wiener (1980; 1989) noted a number of advantages to automating human-machine systems. These include increased capacity and productivity, reduction of small errors, reduction of manual workload and mental fatigue, relief from routine operations, more precise handling of routine operations, economical use of machines, and decrease of performance variation due to individual differences. Wiener and Curry (1980) listed eight reasons for the increase in flight-deck automation: (a) Increase in available technology, such as FMS, Ground Proximity Warning System (GPWS), Traffic Alert andCollision Avoidance System (TCAS), etc.; (b) concern for safety; (c) economy, maintenance, and reliability; (d) workload reduction and two-pilot transport aircraft certification; (e) flight maneuvers and navigation precision; (f) display flexibility; (g) economy of cockpit space; and (h) special requirements for military missions.Disadvantages o f Automation. Automation also has a number of disadvantages that have been noted. Automation increases the burdens and complexities for those responsible for operating, troubleshooting, and managing systems. Woods (1996) stated that automation is "...a wrapped package -- a package that consists of many different dimensions bundled together as a hardware/software system. When new automated systems are introduced into a field of practice, change is precipitated along multiple dimensions." As Woods (1996) noted, some of these changes include: ( a) adds to or changes the task, such as device setup and initialization, configuration control, and operating sequences; (b) changes cognitive demands, such as requirements for increased situational awareness; (c) changes the roles of people in the system, often relegating people to supervisory controllers; (d) automation increases coupling and integration among parts of a system often resulting in data overload and "transparency"; and (e) the adverse impacts of automation is often not appreciated by those who advocate the technology. These changes can result in lower job satisfaction (automation seen as dehumanizing human roles), lowered vigilance, fault-intolerant systems, silent failures, an increase in cognitive workload, automation-induced failures, over-reliance, complacency, decreased trust, manual skill erosion, false alarms, and a decrease in mode awareness (Wiener, 1989).Adaptive AutomationDisadvantages of automation have resulted in increased interest in advanced automation concepts. One of these concepts is automation that is dynamic or adaptive in nature (Hancock & Chignell, 1987; Morrison, Gluckman, & Deaton, 1991; Rouse, 1977; 1988). In an aviation context, adaptive automation control of tasks can be passed back and forth between the pilot and automated systems in response to the changing task demands of modern aircraft. Consequently, this allows for the restructuring of the task environment based upon (a) what is automated, (b) when it should be automated, and (c) how it is automated (Rouse, 1988; Scerbo, 1996). Rouse(1988) described criteria for adaptive aiding systems:The level of aiding, as well as the ways in which human and aidinteract, should change as task demands vary. More specifically,the level of aiding should increase as task demands become suchthat human performance will unacceptably degrade withoutaiding. Further, the ways in which human and aid interact shouldbecome increasingly streamlined as task demands increase.Finally, it is quite likely that variations in level of aiding andmodes of interaction will have to be initiated by the aid rather thanby the human whose excess task demands have created a situationrequiring aiding. The term adaptive aiding is used to denote aidingconcepts that meet [these] requirements.Adaptive aiding attempts to optimize the allocation of tasks by creating a mechanism for determining when tasks need to be automated (Morrison, Cohen, & Gluckman, 1993). In adaptive automation, the level or mode of automation can be modified in real time. Further, unlike traditional forms of automation, both the system and the pilot share control over changes in the state of automation (Scerbo, 1994; 1996). Parasuraman, Bahri, Deaton, Morrison, and Barnes (1992) have argued that adaptive automation represents the optimal coupling of the level of pilot workload to the level of automation in the tasks. Thus, adaptive automation invokes automation only when task demands exceed the pilot's capabilities. Otherwise, the pilot retains manual control of the system functions. Although concerns have been raised about the dangers of adaptive automation (Billings & Woods, 1994; Wiener, 1989), it promises to regulate workload, bolster situational awareness, enhance vigilance, maintain manual skill levels, increase task involvement, and generally improve pilot performance.Strategies for Invoking AutomationPerhaps the most critical challenge facing system designers seeking to implement automation concerns how changes among modes or levels of automation will be accomplished (Parasuraman e t al., 1992; Scerbo, 1996). Traditional forms of automation usually start with some task or functional analysis and attempt to fit the operational tasks necessary to the abilities of the human or the system. The approach often takes the form of a functional allocation analysis (e.g., Fitt's List) in which an attempt is made to determine whether the human or the system is better suited to do each task. However, many in the field have pointed out the problem with trying to equate the two in automated systems, as each have special characteristics that impede simple classification taxonomies. Such ideas as these have led some to suggest other ways of determining human-automation mixes. Although certainly not exhaustive, some of these ideas are presented below.Dynamic Workload Assessment.One approach involves the dynamic assessment o fmeasures t hat index the operators' state of mental engagement. (Parasuraman e t al., 1992; Rouse,1988). The question, however, is what the "trigger" should be for the allocation of functions between the pilot and the automation system. Numerous researchers have suggested that adaptive systems respond to variations in operator workload (Hancock & Chignell, 1987; 1988; Hancock, Chignell & Lowenthal, 1985; Humphrey & Kramer, 1994; Reising, 1985; Riley, 1985; Rouse, 1977), and that measures o f workload be used to initiate changes in automation modes. Such measures include primary and secondary-task measures, subjective workload measures, a nd physiological measures. T he question, however, is what adaptive mechanism should be used to determine operator mental workload (Scerbo, 1996).Performance Measures. One criterion would be to monitor the performance of the operator (Hancock & Chignel, 1987). Some criteria for performance would be specified in the system parameters, and the degree to which the operator deviates from the criteria (i.e., errors), the system would invoke levels of adaptive automation. For example, Kaber, Prinzel, Clammann, & Wright (2002) used secondary task measures to invoke adaptive automation to help with information processing of air traffic controllers. As Scerbo (1996) noted, however,"...such an approach would be of limited utility because the system would be entirely reactive."Psychophysiological M easures.Another criterion would be the cognitive and attentional state of the operator as measured by psychophysiological measures (Byrne & Parasuraman, 1996). An example of such an approach is that by Pope, Bogart, and Bartolome (1996) and Prinzel, Freeman, Scerbo, Mikulka, and Pope (2000) who used a closed-loop system to dynamically regulate the level of "engagement" that the subject had with a tracking task. The system indexes engagement on the basis of EEG brainwave patterns.Human Performance Modeling.Another approach would be to model the performance of the operator. The approach would allow the system to develop a number of standards for operator performance that are derived from models of the operator. An example is Card, Moran, and Newell (1987) discussion of a "model human processor." They discussed aspects of the human processor that could be used to model various levels of human performance. Another example is Geddes (1985) and his colleagues (Rouse, Geddes, & Curry, 1987-1988) who provided a model to invoke automation based upon system information, the environment, and expected operator behaviors (Scerbo, 1996).Mission Analysis. A final strategy would be to monitor the activities of the mission or task (Morrison & Gluckman, 1994). Although this method of adaptive automation may be themost accessible at the current state of technology, Bahri et al. (1992) stated that such monitoring systems lack sophistication and are not well integrated and coupled to monitor operator workload or performance (Scerbo, 1996). An example of a mission analysis approach to adaptive automation is Barnes and Grossman (1985) who developed a system that uses critical events to allocate among automation modes. In this system, the detection of critical events, such as emergency situations or high workload periods, invoked automation.Adaptive Automation Human Factors IssuesA number of issues, however, have been raised by the use of adaptive automation, and many of these issues are the same as those raised almost 20 years ago by Curry and Wiener (1980). Therefore, these issues are applicable not only to advanced automation concepts, such as adaptive automation, but to traditional forms of automation already in place in complex systems (e.g., airplanes, trains, process control).Although certainly one can make the case that adaptive automation is "dressed up" automation and therefore has many of the same problems, it is also important to note that the trend towards such forms of automation does have unique issues that accompany it. As Billings & Woods (1994) stated, "[i]n high-risk, dynamic environments...technology-centered automation has tended to decrease human involvement in system tasks, and has thus impaired human situation awareness; both are unwanted consequences of today's system designs, but both are dangerous in high-risk systems. [At its present state of development,] adaptive ("self-adapting") automation represents a potentially serious threat ... to the authority that the human pilot must have to fulfill his or her responsibility for flight safety."The Need for Human Factors Research.Nevertheless, such concerns should not preclude us from researching the impact that such forms of advanced automation are sure to have on human performance. Consider Hancock’s (1996; 1997) examination of the "teleology for technology." He suggests that automation shall continue to impact our lives requiring humans to co-evolve with the technology; Hancock called this "techneology."What Peter Hancock attempts to communicate to the human factors community is that automation will continue to evolve whether or not human factors chooses to be part of it. As Wiener and Curry (1980) conclude: "The rapid pace of automation is outstripping one's ability to comprehend all the implications for crew performance. It is unrealistic to call for a halt to cockpit automation until the manifestations are completely understood. We do, however, call for those designing, analyzing, and installing automatic systems in the cockpit to do so carefully; to recognize the behavioral effects of automation; to avail themselves of present andfuture guidelines; and to be watchful for symptoms that might appear in training andoperational settings." The concerns they raised are as valid today as they were 23 years ago.However, this should not be taken to mean that we should capitulate. Instead, becauseobservation suggests that it may be impossible to fully research any new Wiener and Curry’stechnology before implementation, we need to form a taxonomy and research plan tomaximize human factors input for concurrent engineering of adaptive automation.Classification of Human Factors Issues. Kantowitz and Campbell (1996)identified some of the key human factors issues to be considered in the design of advancedautomated systems. These include allocation of function, stimulus-response compatibility, andmental models. Scerbo (1996) further suggested the need for research on teams,communication, and training and practice in adaptive automated systems design. The impactof adaptive automation systems on monitoring behavior, situational awareness, skilldegradation, and social dynamics also needs to be investigated. Generally however, Billings(1997) stated that the problems of automation share one or more of the followingcharacteristics: Brittleness, opacity, literalism, clumsiness, monitoring requirement, and dataoverload. These characteristics should inform design guidelines for the development, analysis,and implementation of adaptive automation technologies. The characteristics are defined as: ?Brittleness refers to "...an attribute of a system that works well under normal or usual conditions but that does not have desired behavior at or close to some margin of its operating envelope."?Opacity reflects the degree of understanding of how and why automation functions as it does. The term is closely associated with "mode awareness" (Sarter & Woods, 1994), "transparency"; or "virtuality" (Schneiderman, 1992).?Literalism concern the "narrow-mindedness" of the automated system; that is, theflexibility of the system to respond to novel events.?Clumsiness was coined by Wiener (1989) to refer to automation that reduced workload demands when the demands are already low (e.g., transit flight phase), but increases them when attention and resources are needed elsewhere (e.g., descent phase of flight). An example is when the co-pilot needs to re-program the FMS, to change the plane's descent path, at a time when the co-pilot should be scanning for other planes.?Monitoring requirement refers to the behavioral and cognitive costs associated withincreased "supervisory control" (Sheridan, 1987; 1991).?Data overload points to the increase in information in modern automated contexts (Billings, 1997).These characteristics of automation have relevance for defining the scope of humanfactors issues likely to plague adaptive automation design if significant attention is notdirected toward ensuring human-centered design. The human factors research communityhas noted that these characteristics can lead to human factors issues of allocation of function(i.e., when and how should functions be allocated adaptively); stimulus-response compatibility and new error modes; how adaptive automation will affect mental models,situation models, and representational models; concerns about mode unawareness and-of-the-loop” performance problem; situation awareness decay; manual skill decay and the “outclumsy automation and task/workload management; and issues related to the design of automation. This last issue points to the significant concern in the human factors communityof how to design adaptive automation so that it reflects what has been called “team-centered”;that is, successful adaptive automation will l ikely embody the concept of the “electronic team member”. However, past research (e.g., Pilots Associate Program) has shown that designing automation to reflect such a role has significantly different requirements than those arising in traditional automation design. The field is currently focused on answering the questions,does that definition translate into“what is it that defines one as a team member?” and “howUnfortunately, the literature also shows that the designing automation to reflect that role?” answer is not transparent and, therefore, adaptive automation must first tackle its own uniqueand difficult problems before it may be considered a viable prescription to currenthuman-automation interaction problems. The next section describes the concept of the electronic team member and then discusses t he literature with regard to team dynamics, coordination, communication, shared mental models, and the implications of these foradaptive automation design.Adaptive Automation as Electronic Team MemberLayton, Smith, and McCoy (1994) stated that the design of automated systems should befrom a team-centered approach; the design should allow for the coordination betweenmachine agents and human practitioners. However, many researchers have noted that automated systems tend to fail as team players (Billings, 1991; Malin & Schreckenghost,1992; Malin et al., 1991;Sarter & Woods, 1994; Scerbo, 1994; 1996; Woods, 1996). Thereason is what Woods (1996) calls “apparent simplicity, real complexity.”Apparent Simplicity, Real Complexity.Woods (1996) stated that conventional wisdomabout automation makes technology change seem simple. Automation can be seen as simply changing the human agent for a machine agent. Automation further provides for more optionsand methods, frees up operator time to do other things, provides new computer graphics and interfaces, and reduces human error. However, the reality is that technology change has often。
自动化专业英语原文和翻译

自动化专业英语原文和翻译Automated Professional English Original Text and TranslationOriginal Text:Automation plays a crucial role in the field of engineering, particularly in the domain of industrial processes. It involves the use of control systems and information technologies to reduce human intervention, increase efficiency, and improve productivity.In the context of the automation industry, professionals need to have a strong command of English to effectively communicate and collaborate with international partners and clients. Therefore, it is essential for students studying automation to develop their English language skills, especially in technical and professional contexts.The curriculum for automation majors should include courses that focus on English for specific purposes, such as technical writing, presentations, and negotiations. These courses should provide students with the necessary vocabulary, grammar, and communication strategies to effectively convey complex technical information in English.In addition to language skills, automation professionals should also be familiar with industry-specific terminology and concepts. They should have a solid understanding of automation systems, robotics, control systems, and programming languages commonly used in the field. This knowledge will enable them to effectively communicate and work with colleagues and clients from different countries.Furthermore, automation professionals should be aware of the latest advancements and trends in the industry. They should stay updated on new technologies, regulations, and best practices. This can be achieved through continuous professional development, attending conferences, workshops, and participating in online forums and communities.Translation:自动化在工程领域中起着至关重要的作用,特别是在工业过程领域。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
景德镇陶瓷学院毕业设计(论文)有关外文翻译院系:机械电子工程学院专业:自动化姓名:肖骞学号: 201010320116指导教师:万军完成时间: 2014.5.8说明1、将与课题有关的专业外文翻译成中文是毕业设计(论文)中的一个不可缺少的环节。
此环节是培养学生阅读专业外文和检验学生专业外文阅读能力的一个重要环节。
通过此环节进一步提高学生阅读专业外文的能力以及使用外文资料为毕业设计服务,并为今后科研工作打下扎实的基础。
2、要求学生查阅与课题相关的外文文献3篇以上作为课题参考文献,并将其中1篇(不少于3000字)的外文翻译成中文。
中文的排版按后面格式进行填写。
外文内容是否与课题有关由指导教师把关,外文原文附在后面。
3、指导教师应将此外文翻译格式文件电子版拷给所指导的学生,统一按照此排版格式进行填写,完成后打印出来。
4、请将封面、译文与外文原文装订成册。
5、此环节在开题后毕业设计完成前完成。
6、指导教师应从查阅的外文文献与课题紧密相关性、翻译的准确性、是否通顺以及格式是否规范等方面去进行评价。
指导教师评语:签名:年月日TMS320LF2407, TMS320LF2406, TMS320LF2402TMS320LC2406, TMS320LC2404, MS320LC2402DSP CONTROLLERSThe TMS320LF240x and TMS320LC240x devices, new members of the ‘24x family of digital signal processor (DSP) controllers, are part of the C2000 platform of fixed-point DSPs. The ‘240x devices offer the enhanced TMS320 architectural design of the ‘C2xx core CPU for low-cost, low-power, high-performance processing capabilities. Several advanced peripherals, optimized for digital motor and motion control applications, have been integrated to provide a true single chip DSP controller. While code-compatible with the existing ‘24x DSP controller devices, the ‘240x offers increased processing performance (30 MIPS) and a higher level of peripheral integration. See the TMS320x240x device summary section for device-specific features.The ‘240x family offers an array of memory sizes and different peripherals tailored to meet the specific price/performance points required by various applications. Flash-based devices of up to 32K words offer a reprogrammable solution useful for:◆Applications requiring field programmability upgrades.◆Development and initial prototyping of applications that migrate toROM-based devices.Flash devices and corresponding ROM devices are fully pin-to-pin compatible. Note that flash-based devices contain a 256-word boot ROM to facilitate in-circuit programming.All ‘240x devices offer at least one event manager module which has been optimized for digital motor control and power conversion applications. Capabilities of this module include centered- and/or edge-aligned PWM generation, programmable deadband to prevent shoot-through faults, and synchronized analog-to-digital conversion. Devices with dual event managers enable multiple motor and/or convertercontrol with a single ‗240x DSP controller.The high performance, 10-bit analog-to-digital converter (ADC) has a minimum conversion time of 500 ns and offers up to 16 channels of analog input. The auto sequencing capability of the ADC allows a maximum of 16 conversions to take place in a single conversion session without any CPU overhead.A serial communications interface (SCI) is integrated on all devices to provide asynchronous communication to other devices in the system. For systems requiring additional communication interfaces; the ‘2407, ‘2406, and ‘2404 offer a 16-bit synchronous serial peripheral interface (SPI). The ‘2407 and ‘2406 offer a controller area network (CAN) communications module that meets 2.0B specifications. To maximize device flexibility, functional pins are also configurable as general purpose inputs/outputs (GPIO).To streamline development time, JTAG-compliant scan-based emulation has been integrated into all devices. This provides non-intrusive real-time capabilities required to debug digital control systems. A complete suite of code generation tools from C compilers to the industry-standard Code Composerdebugger supports this family. Numerous third party developers not only offer device-level development tools, but also system-level design and development support.PERIPHERALSThe integrated peripherals of the TMS320x240x are described in the following subsections:●Two event-manager modules (EV A, EVB)●Enhanced analog-to-digital converter (ADC) module●Controller area network (CAN) module●Serial communications interface (SCI) module●Serial peripheral interface (SPI) module●PLL-based clock module●Digital I/O and shared pin functions●External memory interfaces (‘LF2407 only)Watchdog (WD) timer moduleEvent manager modules (EV A, EVB)The event-manager modules include general-purpose (GP) timers, full-compare/PWM units, capture units, and quadrature-encoder pulse (QEP) circuits. EV A‘s and EVB‘s timers, compare units, and capture units function identically. However, timer/unit names differ for EV A and EVB. Table 1 shows the module and signal names used. Table 1 shows the features and functionality available for the event-manager modules and highlights EV A nomenclature.Event managers A and B have identical peripheral register sets with EV A starting at 7400h and EVB starting at 7500h. The paragraphs in this section describe the function of GP timers, compare units, capture units, and QEPs using EV A nomenclature. These paragraphs are applicable to EVB with regard to function—however, module/signal names would differ.Table 1. Module and Signal Names for EV A and EVBEVENT MANAGER MODULESEV AMODULESIGNALEVBMODULESIGNALGP Timers Timer 1Timer 2T1PWM/T1CMPT2PWM/T2CMPTimer 3Timer 4T3PWM/T3CMPT4PWM/T4CMPCompare Units Compare 1Compare 2Compare 3PWM1/2PWM3/4PWM5/6Compare 4Compare 5Compare 6PWM7/8PWM9/10PWM11/12Capture Units Capture 1Capture 2Capture 3CAP1CAP2CAP3Capture 4Capture 5Capture 6CAP4CAP5CAP6QEP QEP1QEP2QEP1QEP2QEP3QEP4QEP3QEP4External Inputs DirectionExternalClockTDIRATCLKINADirectionExternal ClockTDIRBTCLKINBGeneral-purpose (GP) timersThere are two GP timers: The GP timer x (x = 1 or 2 for EV A; x = 3 or 4 for EVB) includes:● A 16-bit timer, up-/down-counter, TxCNT, for reads or writes● A 16-bit timer-compare register, TxCMPR (double-buffered with shadowregister), for reads or writes● A 16-bit timer-period register, TxPR (double-buffered with shadowregister), for reads or writes● A 16-bit timer-control register,TxCON, for reads or writes●Selectable internal or external input clocks● A programmable prescaler for internal or external clock inputs●Control and interrupt logic, for four maskable interrupts: underflow,overflow, timer compare, and period interrupts● A selectable direction input pin (TDIR) (to count up or down whendirectional up-/down-count mode is selected)The GP timers can be operated independently or synchronized with each other. The compare register associated with each GP timer can be used for compare function and PWM-waveform generation. There are three continuous modes of operations for each GP timer in up- or up/down-counting operations. Internal or external input clocks with programmable prescaler are used for each GP timer. GP timers also provide the time base for the other event-manager submodules: GP timer 1 for all the compares and PWM circuits, GP timer 2/1 for the capture units and the quadrature-pulse counting operations. Double-buffering of the period and compare registers allows programmable change of the timer (PWM) period and the compare/PWM pulse width as needed.Full-compare unitsThere are three full-compare units on each event manager. These compare units use GP timer1 as the time base and generate six outputs for compare and PWM-waveform generation using programmable deadband circuit. The state of each of the six outputs is configured independently. The compare registers of the compare units are double-buffered, allowing programmable change of the compare/PWM pulse widths as needed.Programmable deadband generatorThe deadband generator circuit includes three 8-bit counters and an 8-bit compare register. Desired deadband values (from 0 to 24 µs) can be programmed into the compare register for the outputs of the three compare units. The deadband generation can be enabled/disabled for each compare unit output individually. The deadband-generator circuit produces two outputs (with or without deadband zone) for each compare unit output signal. The output states of the deadband generator are configurable and changeable as needed by way of the double-buffered ACTR register.PWM waveform generationUp to eight PWM waveforms (outputs) can be generated simultaneously by each event manager: three independent pairs (six outputs) by the three full-compare units with programmable deadbands, and two independent PWMs by the GP-timer compares.PWM characteristicsCharacteristics of the PWMs are as follows:●16-bit registers●Programmable deadband for the PWM output pairs, from 0 to 24 µs●Minimum deadband width of 50 ns●Change of the PWM carrier frequency for PWM frequency wobbling asneeded●Change of the PWM pulse widths within and after each PWM period asneeded●External-maskable power and drive-protection interrupts●Pulse-pattern-generator circuit, for programmable generation of asymmetric,symmetric, and four-space vector PWM waveforms●Minimized CPU overhead using auto-reload of the compare and periodregistersCapture unitThe capture unit provides a logging function for different events or transitions. The values of the GP timer 2 counter are captured and stored in the two-level-deep FIFO stacks when selected transitions are detected on capture input pins, CAPx (x = 1, 2, or 3 for EV A; and x = 4, 5, or 6 for EVB). The capture unit consists of three capture circuits.Capture units include the following features:●One 16-bit capture control register, CAPCON (R/W)●One 16-bit capture FIFO status register, CAPFIFO (eight MSBs areread-only, eight LSBs are write-only)●Selection of GP timer 2 as the time base●Three 16-bit 2-level-deep FIFO stacks, one for each capture unit●Three Schmitt-triggered capture input pins (CAP1, CAP2, and CAP3)—oneinput pin per capture unit. [All inputs are synchronized with the device (CPU)clock. In order for a transition to be captured, the input must hold at itscurrent level to meet two rising edges of the device clock. The input pinsCAP1 and CAP2 can also be used as QEP inputs to the QEP circuit.]●User-specified transition (rising edge, falling edge, or both edges) detection●Three maskable interrupt flags, one for each capture unitEnhanced analog-to-digital converter (ADC) moduleA simplified functional block diagram of the ADC module is shown in Figure 1. The ADC module consists of a 10-bit ADC with a built-in sample-and-hold (S/H) circuit. Functions of the ADC module include:●10-bit ADC core with built-in S/H●Fast conversion time (S/H + Conversion) of 500 ns●16-channel, muxed inputs●Autosequencing capability provides up to 16 ―autoconversions‖ in a singlesession. Each conversion can be programmed to select any 1 of 16 inputchannels●Sequencer can be operated as two independent 8-state sequencers or as onelarge 16-state sequencer (i.e., two cascaded 8-state sequencers)●Sixteen result registers (individually addressable) to store conversion values●Multiple triggers as sources for the start-of-conversion (SOC) sequence✧S/W – software immediate start✧EV A – Event manager A (multiple event sources within EV A)✧EVB – Event manager B (multiple event sources within EVB)✧Ext – External pin (ADCSOC)●Flexible interrupt control allows interrupt request on every end of sequence(EOS) or every other EOS●Sequencer can operate in ―start/stop‖ mode, allowing multiple―time-sequenced triggers‖ to synchronize conversions●EV A and EVB triggers can operate independently in dual-sequencer mode●Sample-and-hold (S/H) acquisition time window has separate prescalecontrol●Built-in calibration mode●Built-in self-test modeThe ADC module in the ‘240x has been enhanced to provide flexible interface to event managers A and B. The ADC interface is built around a fast, 10-bit ADC module with total conversion time of 500 ns (S/H + conversion). The ADC module has 16 channels, configurable as two independent 8-channel modules to service event managers A and B. The two independent 8-channel modules can be cascaded to form a 16-channel module. Figure 2 shows the block diagram of the ‘240x ADC module.The two 8-channel modules have the capability to autosequence a series of conversions, each module has the choice of selecting any one of the respective eight channels available through an analog mux. In the cascaded mode, the autosequencer functions as a single 16-channel sequencer. On each sequencer, once the conversion is complete, the selected channel value is stored in its respective RESULT register. Autosequencing allows the system to convert the same channel multiple times, allowing the user to perform oversampling algorithms. This gives increased resolution over traditional single-sampled conversion results.From TMS320LF2407, TMS320LF2406, TMS320LF2402TMS320LC2406, TMS320LC2404, MS320LC2402数字信号处理控制器TMS320LF240x和TMS320LC240x系列芯片作为’24x系列DSP控制器的新成员,是C2000平台下的一种定点DSP芯片。