24C64 EEPROM读写的C语言程序
PIC读写24LCxx系列的EEPROM的实例C语言程序

PIC:读写24LCxx系列的EEPROM的实例C语言程序//********************************************************* //* Using I2C Master Mode for access Slave (EEPRM)//*//* Written by: Richard Yang//* Sr. Corporate Application Engineer//* Microchip Technology Inc.//* Date: Oct. 3nd '2002//* Revision: 1.00//* Language tools : MPLAB-C18 v2.09.13//* MPLINK v3.10//* MPLAB-IDE v6.00.17 & ICD2 //***********************************************************/* Include Header files */#i nclude <p18f452.h>#i nclude <i2c.h> // Load I2C Header file from defult direct#i nclude <timers.h>#i nclude "P18LCD.h" // Load P18LCD Header file form current working direct/* Declare the Function Prototype */void Initialize_I2C_Master(void);void EE_Page_Write(unsigned char,unsigned char,unsigned char,unsigned char *); void EE_SEQU_Read(unsigned char,unsigned char,unsigned char,unsigned char *);void EEPROM_Write(unsigned char,unsigned char,unsigned char);void EEPROM_ACK(unsigned char);unsigned char EEPROM_Read(unsigned char,unsigned char);void I2C_Done(void);void Initialize_Timer2(void);void isr_high_direct(void);void isr_high(void);#pragma romdata My_romdata=0x1000const rom far unsigned char LCD_MSG1[]="SW2: Byte Write ";const rom far unsigned char LCD_MSG2[]="SW6: Random Read";const rom far unsigned char LCD_MSG3[]="Byte Write Mode ";const rom far unsigned char LCD_MSG4[]="Random Read Mode";const rom far unsigned char LCD_MSG5[]="Sended: ";const rom far unsigned char LCD_MSG6[]="Send: ";const rom unsigned char I2C_Write_Buffer[]="Microchip Technology";#pragma romdata/* Define following array in data memory */unsigned char I2C_Read_Buffer [32];/* define following variable in data memory at Access Bank */#pragma udata access My_RAMnear unsigned char Debounce;near unsigned char Send_Addr;near unsigned char Send_Data;near unsigned char Send_Length;near unsigned char Read_Data;near unsigned char P_SW2;near unsigned char P_SW6;#pragma udata#define Page_Length 8#define SW2 PORTAbits.RA4#define SW6 PORTEbits.RE1#define Bounce_Time 6#define EE_CMD 0xA0//***********************************************************/* *//* Main Program *//* *///***********************************************************void main(void){ADCON1=0b00000110; // Disable A/D FunctionTRISAbits.TRISA4=1; // Set SW2 for inputTRISEbits.TRISE1=1; // Set SW6 for InputInitialize_Timer2( );Initialize_I2C_Master( );OpenLCD( );if (SW2 & SW6)Debounce=0;else Debounce = Bounce_Time;while(1){LCD_Set_Cursor(0,0); // Put LCD Cursor on (0,0)putrsLCD(LCD_MSG1);LCD_Set_Cursor(1,0); // Put LCD Cursor on (1,0)putrsLCD(LCD_MSG2);P_SW2=P_SW6=0;Send_Addr=0;while(1){if (P_SW2){P_SW2=0;Debounce = Bounce_Time;LCD_Set_Cursor(0,0); // Put LCD Cursor on (0,0)putrsLCD(LCD_MSG3);LCD_Set_Cursor(1,0); // Put LCD Cursor on (0,0)putrsLCD(LCD_MSG5);do{while (!P_SW2);P_SW2=0;LCD_Set_Cursor(1,8);Send_Data=I2C_Write_Buffer[Send_Addr];EEPROM_Write(EE_CMD,Send_Addr,Send_Data);puthexLCD(EE_CMD);putcLCD(' ');puthexLCD(Send_Addr);putcLCD(' ');puthexLCD(Send_Data);EEPROM_ACK(EE_CMD);Send_Addr++;} while (I2C_Write_Buffer[Send_Addr]!=0x00);break;}if (P_SW6){P_SW6=0;Debounce = Bounce_Time;LCD_Set_Cursor(0,0); // Put LCD Cursor on (0,0)putrsLCD(LCD_MSG4);LCD_Set_Cursor(1,0); // Put LCD Cursor on (0,0)putrsLCD(LCD_MSG6);while(1){if (P_SW6){P_SW6=0;LCD_Set_Cursor(1,5);Read_Data = EEPROM_Read(EE_CMD,Send_Addr);puthexLCD(EE_CMD);putcLCD(' ');puthexLCD(Send_Addr);putcLCD(' ');puthexLCD(EE_CMD);putcLCD(' ');puthexLCD(Read_Data);Send_Addr++;}if (P_SW2) break;}if (P_SW2) break;}if (P_SW2){P_SW2=0;break;}}}}//************************************************ //* #pragma Interrupt Declarations *//* *//* Function: isr_high_direct *//* - Direct execution to the actual *//* high-priority interrupt code. *//************************************************ #pragma code isrhighcode = 0x0008void isr_high_direct(void){_asm //begin in-line assemblygoto isr_high //go to isr_high function_endasm //end in-line assembly}#pragma code//************************************************ //* Function: isr_high(void) *//* High priority interrupt for Timer2 * //************************************************#pragma interrupt isr_highvoid isr_high(void){PIR1bits.TMR2IF=0; // Clear Timer2 interrupt Flagif (Debounce==0){if (!SW2){ P_SW2=1; Debounce =Bounce_Time; }if (!SW6){ P_SW6=1; Debounce =Bounce_Time; }}else if (SW2 & SW6)Debounce--;else Debounce =Bounce_Time;}#pragma code//*********************************************** //* Write a Byte to EEPROM//* - ctrl : Control Byte of EEPROM//* - addr : Location of EEPROM//* - data : Data Byte of EEPROM//***********************************************void Initialize_Timer2(void){RCONbits.IPEN=1; // Enable Interrupt Priority bitIPR1bits.TMR2IP=1; // Set Timer2 for High PriorityINTCONbits.GIEH=1; // Enable High Priority InterruptOpenTimer2 (TIMER_INT_ON // Turn On the Timer2 with Interrupt & T2_PS_1_4 // (4Mhz/4) [4*10*(99+1)] = 4mS */& T2_POST_1_10);PR2 = 99;}//*********************************************** //* Write a Byte to EEPROM *//* - ctrl : Control Byte of EEPROM *//* - addr : Location of EEPROM *//* - data : Data Byte of EEPROM *//*********************************************** void EEPROM_Write(unsigned char ctrl,unsigned char addr,unsigned char data){IdleI2C(); // ensure module is idleStartI2C(); // Start conditionI2C_Done(); // Wait Start condition completed and clear SSPIF flagWriteI2C(ctrl); // Write Control+Write to EEPROM & Check BF flagwhile(SSPCON2bits.ACKSTAT); // wait until received the Acknowledge from EEPROMI2C_Done(); // Clear SSPIF flagWriteI2C(addr); // Write Address to EEPROMwhile(SSPCON2bits.ACKSTAT); // wait until received the Acknowledge from EEPROMI2C_Done();WriteI2C(data); // Write Data to EEPROMwhile(SSPCON2bits.ACKSTAT); // wait until received the Acknowledge from EEPROMI2C_Done();StopI2C(); // Stop conditionI2C_Done(); // Wait the Stop condition completed}//***********************************************//* Pae Write to EEPROM//*//* - ctrl : Control Byte of EEPROM//* - addr : Location of EEPROM//* - length : Write counter//* - *dptr : RAM point --> EEPROM//*//***********************************************void EE_Page_Write(unsigned char ctrl,unsigned char addr,unsigned char length,unsigned char *dptr){IdleI2C(); // ensure module is idleStartI2C(); // Start conditionI2C_Done(); // Wait Start condition completedWriteI2C(ctrl); // Write Control+Write to EEPROM & Check BF flagwhile(SSPCON2bits.ACKSTAT); // wait until received the Acknowledge from EEPROMI2C_Done(); // Clear SSPIF flagWriteI2C(addr); // Write Address to EEPROMwhile(SSPCON2bits.ACKSTAT); // wait until received the Acknowledge from EEPROMI2C_Done();while (length!=0) // Check write completed ?{WriteI2C(*dptr); // Write data to EEPROMwhile(SSPCON2bits.ACKSTAT); // wait until received the Acknowledge from EEPROMI2C_Done();dptr++; // Point to next bytelength--;}StopI2C(); // Stop conditionI2C_Done(); // Wait the Stop condition completed}//***********************************************//* EEPROM Acknowledge Polling *//* -- The routine will polling the ACK *//* response from EEPROM *//* -- ACK=0 return *//* -- ACK=1 send Restart & loop check *//***********************************************void EEPROM_ACK(unsigned char ctrl){unsigned char i;IdleI2C(); // ensure module is idleStartI2C(); // Start conditionI2C_Done(); // Wait Start condition completedWriteI2C(ctrl); // Write Control to EEPROM (WRITE)I2C_Done(); // Clear SSPIF flagwhile (SSPCON2bits.ACKSTAT) // test for Acknowledge from EEPROM{for (i=0;i<100;i++); // Delay for next Repet-StartRestartI2C(); // initiate Repet-Start conditionI2C_Done(); // Wait Repet-Start condition completedWriteI2C(ctrl); // Write Control to EEPROM (WRITE)I2C_Done(); // Clear SSPIF flag}StopI2C(); // send STOP conditionI2C_Done(); // wait until stop condition is over}//*********************************************** //* Random Read a Byte from EEPROM *//* - ctrl : Control Byte of EEPROM (Write) *//* (Ctrl +1 ) : Read Command *//* - addr : Address Byte of EEPROM *//* - Return : Read Data from EEPROM *//*********************************************** unsigned char EEPROM_Read(unsigned char ctrl,unsigned char addr){unsigned char f;IdleI2C(); // ensure module is idleStartI2C(); // Start conditionI2C_Done(); // Wait Start condition completedWriteI2C(ctrl); // Write Control to EEPROM while(SSPCON2bits.ACKSTAT); // test for ACK condition, if receivedI2C_Done(); // Clear SSPIF flagWriteI2C(addr); // Write Address to EEPROM while(SSPCON2bits.ACKSTAT); // test for ACK condition, if receivedI2C_Done(); // Clear SSPIF flagRestartI2C(); // initiate Restart conditionI2C_Done();WriteI2C(ctrl+1); // Write Control to EEPROMwhile(SSPCON2bits.ACKSTAT); // test for ACK condition, if receivedI2C_Done(); // Clear SSPIF flagf=ReadI2C(); // Enable I2C Receiver & wait BF=1 until received dataI2C_Done(); // Clear SSPIF flagNotAckI2C(); // Genarate Non_Acknowledge to EEPROMI2C_Done();StopI2C(); // send STOP conditionI2C_Done(); // wait until stop condition is overreturn(f); // Return Data from EEPROM}//***********************************************//* Sequential Read from EEPROM//*//* - ctrl : Control Byte of EEPROM//* - addr : Location of EEPROM//* - length : Read counter//* - *dptr : Store EEPROM data to RAM//*//***********************************************void EE_SEQU_Read(unsigned char ctrl,unsigned char addr,unsigned char length,unsigned char *dptr){IdleI2C(); // ensure module is idleStartI2C(); // Start conditionI2C_Done(); // Wait Start condition completedWriteI2C(ctrl); // Write Control to EEPROMwhile(SSPCON2bits.ACKSTAT); // test for ACK condition, if receivedI2C_Done(); // Clear SSPIF flagWriteI2C(addr); // Write Address to EEPROMwhile(SSPCON2bits.ACKSTAT); // test for ACK condition, if receivedI2C_Done(); // Clear SSPIF flagRestartI2C(); // initiate Restart conditionI2C_Done();WriteI2C(ctrl+1); // Write Control to EEPROMwhile(SSPCON2bits.ACKSTAT); // Test for ACK condition, if receivedI2C_Done(); // Clear SSPIF flagwhile (length!=0){*dptr=ReadI2C(); // Enable I2C Receiver & Store EEPROM data to Point bufferI2C_Done();dptr++;length--;if (length==0) NotAckI2C();else AckI2C(); // Continue read next data, send a acknowledge to EEPROMI2C_Done();}StopI2C(); // send STOP conditionI2C_Done(); // wait until stop condition is over}//***********************************************//* Check I2C action that is completed *//***********************************************void I2C_Done(void){while (!PIR1bits.SSPIF); // Completed the action when the SSPIF is Hi.PIR1bits.SSPIF=0; // Clear SSPIF}//************************************************ //* Initial I2C Master Mode with 7 bits Address *//* Clock Speed : 100KHz @4MHz *//************************************************void Initialize_I2C_Master(void){OpenI2C(MASTER,SLEW_ON);SSPADD= 9;}。
24c64数据写入全攻略(AT24C64)

○1硬件:STC89C52,AT24C64首先是AT24c64的硬件电路:其中scl与单片机的P3.6口相连Sda与单片机的P3.7口相连10k上拉电阻是必须要加的。
连接好单片机与at24c64后,开始程序部分。
○2我用这个eeprom是为了存储LCD5110液晶显示模块的字库,LCD5110不带字库,因此只能把生成的字库存入at24c64中。
我的LCD5110显示程序可参考:/393275398/blog/item/c7562e5e30162250fbf2c0a5.html字模提取软件下载(配套软件的使用说明)/c0z0c9l9q7○3当我们提取了我们需要的字的字模后如:{0x00,0x00,0x00,0x00,0x00,0x80,0xC0,0x40,0x40,0x40,0xC0,0x80,0x00,0x00,0x00,0x00},{0x00,0x00,0xF0,0xFE,0x0F,0x01,0x00,0x00,0x00,0x00,0x00,0x01,0x07,0xFE,0xF0,0x00},{0x00,0x00,0x1F,0xFF,0xE0,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xC0,0xFF,0x1F,0x00},{0x00,0x00,0x00,0x00,0x01,0x03,0x06,0x04,0x04,0x04,0x06,0x03,0x01,0x00,0x00,0x00},//"0"{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x80,0xC0,0x00,0x00,0x00,0x00,0x00,0x00,0x00},{0x00,0x00,0x00,0x01,0x01,0x01,0x01,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00},{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF,0xFF,0x00,0x00,0x00,0x00,0x00,0x00,0x00},{0x00,0x00,0x00,0x04,0x04,0x04,0x06,0x07,0x07,0x06,0x04,0x04,0x04,0x00,0x00,0x00},//"1"0和1的字模,我们要将它写入到at24c64中。
CAT24C64 D64 64Kb I2C CMOS 序列 EEPROM 数据手册说明书

64 Kb I2C CMOS Serial EEPROMCAT24C64DescriptionThe CAT24C64 is a 64 Kb CMOS Serial EEPROM device, internally organized as 8192 words of 8 bits each.It features a 32−byte page write buffer and supports the Standard (100 kHz), Fast (400 kHz) and Fast−Plus (1 MHz) I2C protocol. External address pins make it possible to address up to eight CAT24C64 devices on the same bus.Features•Supports Standard, Fast and Fast−Plus I2C Protocol•1.7 V to 5.5 V Supply V oltage Range•32−Byte Page Write Buffer•Hardware Write Protection for Entire Memory•Schmitt Triggers and Noise Suppression Filters on I2C Bus Inputs (SCL and SDA)•Low Power CMOS Technology•1,000,000 Program/Erase Cycles•100 Year Data Retention•Industrial and Extended Temperature Range•SOIC, TSSOP, UDFN 8−pad and Ultra−thin WLCSP 4−bump Packages•This Device is Pb−Free, Halogen Free/BFR Free, and RoHS CompliantFigure 1. Functional Symbol SDASCL WPV CC SSA2, A1, APIN CONFIGURATIONS (Top Views)See detailed ordering and shipping information in the package dimensions section on page 8 of this data sheet.ORDERING INFORMATIONSOIC−8W SUFFIXCASE 751BDSOIC (W), TSSOP (Y),UDFN (HU4)Y SUFFIXCASE 948ALDevice AddressA0, A1, A2Serial DataSDASerial ClockSCLWrite ProtectWPPower SupplyV CCGroundV SSFunctionPin NamePIN FUNCTIONFor the location of Pin 1, please consult thecorresponding package drawing.UDFN−8HU4 SUFFIXCASE 517AZWLCSP−4C4C SUFFIXCASE 567JYSDAWPV CCV SSA2A1A01SCLWLCSPA1A2B1B2SDAV SSSCLV CC1X= Specific Device Code(see ordering information)Y= Production Year (Last Digit)M= Production Month (1−9, O, N, D)W= Production Week CodeMARKINGDIAGRAMS(WLCSP−4)WLCSP−4C4U SUFFIXCASE 567PBXYM(C4C)For serial EEPROM in a US8 package, pleaseTable 1. ABSOLUTE MAXIMUM RATINGSParameters Ratings Units Storage Temperature–65 to +150°C Voltage on Any Pin with Respect to Ground (Note 1)–0.5 to +6.5V Stresses exceeding those listed in the Maximum Ratings table may damage the device. If any of these limits are exceeded, device functionality should not be assumed, damage may occur and reliability may be affected.1.The DC input voltage on any pin should not be lower than −0.5 V or higher than V CC + 0.5 V. During transitions, the voltage on any pin mayundershoot to no less than −1.5 V or overshoot to no more than V CC + 1.5 V, for periods of less than 20 ns.Table 2. RELIABILITY CHARACTERISTICS (Note 2)Symbol Parameter Min UnitsN END (Note 3)Endurance1,000,000Program/Erase Cycles T DR Data Retention100Years2.These parameters are tested initially and after a design or process change that affects the parameter according to appropriate AEC−Q100and JEDEC test methods.3.Page Mode, V CC = 5 V, 25°C.Table 3. D.C. OPERATING CHARACTERISTICS(V CC = 1.8 V to 5.5 V, T A = −40°C to +125°C and V CC = 1.7 V to 5.5 V, T A = −40°C to +85°C, unless otherwise specified.)Symbol Parameter Test Conditions Min Max UnitsI CCR Read Current Read, f SCL = 400 kHz1mAI CCW Write Current Write, f SCL = 400 kHz2mAI SB Standby Current All I/O Pins at GND or V CC T A = −40°C to +85°CV CC≤ 3.3 V1m AT A = −40°C to +85°CV CC > 3.3 V3T A = −40°C to +125°C5I L I/O Pin Leakage Pin at GND or V CC2m AV IL Input Low Voltage−0.5V CC x 0.3V V IH Input High Voltage V CC x 0.7V CC + 0.5V V OL1Output Low Voltage V CC≥ 2.5 V, I OL = 3.0 mA0.4V V OL2Output Low Voltage V CC < 2.5 V, I OL = 1.0 mA0.2V Table 4. PIN IMPEDANCE CHARACTERISTICS(V CC = 1.8 V to 5.5 V, T A = −40°C to +125°C and V CC = 1.7 V to 5.5 V, T A = −40°C to +85°C, unless otherwise specified.) Symbol Parameter Conditions Max UnitsC IN (Note 4)SDA I/O Pin Capacitance V IN = 0 V8pFC IN (Note 4)Input Capacitance (other pins)V IN = 0 V6pFI WP (Note 5)WP Input Current V IN < V IH, V CC = 5.5 V130m AV IN < V IH, V CC = 3.3 V120V IN < V IH, V CC = 1.8 V80V IN> V IH2I A (Note 5)Address Input Current(A0, A1, A2)Product Rev F V IN < V IH, V CC = 5.5 V50m A V IN < V IH, V CC = 3.3 V35V IN < V IH, V CC = 1.8 V25V IN> V IH24.These parameters are tested initially and after a design or process change that affects the parameter according to appropriate AEC−Q100and JEDEC test methods.5.When not driven, the WP, A0, A1 and A2 pins are pulled down to GND internally. For improved noise immunity, the internal pull−down is relativelystrong; therefore the external driver must be able to supply the pull−down current when attempting to drive the input HIGH. T o conserve power, as the input level exceeds the trip point of the CMOS input buffer (~ 0.5 x V CC), the strong pull−down reverts to a weak current source.Table 5. A.C. CHARACTERISTICS(V CC = 1.8 V to 5.5 V, T A = −40°C to +125°C and V CC = 1.7 V to 5.5 V, T A = −40°C to +85°C.) (Note 6)Symbol ParameterStandardV CC = 1.7 V − 5.5 VFastV CC = 1.7 V − 5.5 VFast−PlusV CC = 1.7 V − 5.5 VT A = −405C to +855CUnits Min Max Min Max Min MaxF SCL Clock Frequency1004001,000kHzt HD:STA START Condition Hold Time40.60.25m s t LOW Low Period of SCL Clock 4.7 1.30.45m s t HIGH High Period of SCL Clock40.60.40m s t SU:STA START Condition Setup Time 4.70.60.25m s t HD:DAT Data In Hold Time000m s t SU:DAT Data In Setup Time25010050ns t R (Note 7)SDA and SCL Rise Time1,000300100ns t F (Note 7)SDA and SCL Fall Time300300100ns t SU:STO STOP Condition Setup Time40.60.25m s t BUF Bus Free Time BetweenSTOP and START4.7 1.30.5m st AA SCL Low to Data Out Valid 3.50.90.40m s t DH Data Out Hold Time10010050ns T i (Note 7)Noise Pulse Filtered at SCLand SDA Inputs100100100ns t SU:WP WP Setup Time000m s t HD:WP WP Hold Time 2.5 2.51m s t WR Write Cycle Time555mst PU (Notes 7, 8)Power−up to Ready Mode110.11ms Product parametric performance is indicated in the Electrical Characteristics for the listed test conditions, unless otherwise noted. Product performance may not be indicated by the Electrical Characteristics if operated under different conditions.6.Test conditions according to “A.C. Test Conditions” table.7.Tested initially and after a design or process change that affects this parameter.8.t PU is the delay between the time V CC is stable and the device is ready to accept commands.Table 6. A.C. TEST CONDITIONSInput Levels0.2 x V CC to 0.8 x V CCInput Rise and Fall Times≤ 50 nsInput Reference Levels0.3 x V CC, 0.7 x V CCOutput Reference Levels0.5 x V CCOutput Load Current Source: I OL = 3 mA (V CC≥ 2.5 V); I OL = 1 mA (V CC< 2.5 V); C L = 100 pFPower −On Reset (POR)Each CAT24C64 incorporates Power −On Reset (POR)circuitry which protects the internal logic against powering up in the wrong state. The device will power up into Standby mode after V CC exceeds the POR trigger level and will power down into Reset mode when V CC drops below the POR trigger level. This bi −directional POR behavior protects the device against ‘brown −out’ failure following a temporary loss of power.Pin DescriptionSCL: The Serial Clock input pin accepts the clock signal generated by the Master.SDA: The Serial Data I/O pin accepts input data and delivers output data. In transmit mode, this pin is open drain. Data is acquired on the positive edge, and is delivered on the negative edge of SCL.A 0, A 1 and A 2: The Address inputs set the device address that must be matched by the corresponding Slave address bits. The Address inputs are hard −wired HIGH or LOW allowing for up to eight devices to be used (cascaded) on the same bus. When left floating, these pins are pulled LOW internally. The Address inputs are not available for use with WLCSP 4−bumps.WP: When pulled HIGH, the Write Protect input pin inhibits all write operations. When left floating, this pin is pulled LOW internally. The WP input is not available for the WLCSP 4−bumps, therefore all write operations are allowed for the device in this package.Functional DescriptionThe CAT24C64 supports the Inter −Integrated Circuit (I 2C) Bus protocol. The protocol relies on the use of a Master device, which provides the clock and directs bus traffic, and Slave devices which execute requests. The CAT24C64operates as a Slave device. Both Master and Slave cantransmit or receive, but only the Master can assign those roles.I 2C Bus ProtocolThe 2−wire I 2C bus consists of two lines, SCL and SDA,connected to the V CC supply via pull −up resistors. The Master provides the clock to the SCL line, and either the Master or the Slaves drive the SDA line. A ‘0’ is transmitted by pulling a line LOW and a ‘1’ by letting it stay HIGH. Data transfer may be initiated only when the bus is not busy (see A.C. Characteristics). During data transfer, SDA must remain stable while SCL is HIGH.START/STOP Condition An SDA transition while SCL is HIGH creates a START or STOP condition (Figure 2). The START consists of a HIGH to LOW SDA transition, while SCL is HIGH. Absent the START, a Slave will not respond to the Master. The STOP completes all commands, and consists of a LOW to HIGH SDA transition, while SCL is HIGH.Device AddressingThe Master addresses a Slave by creating a START condition and then broadcasting an 8−bit Slave address. For the CA T24C64, the first four bits of the Slave address are set to 1010 (Ah); the next three bits, A 2, A 1 and A 0, must match the logic state of the similarly named input pins. The devices in WLCSP 4−bumps respond only to the Slave Address with A2 A1 A0 = 000 (CA T24C64C4xTR). The R/W bit tells the Slave whether the Master intends to read (1) or write (0) data (Figure 3).AcknowledgeDuring the 9th clock cycle following every byte sent to the bus, the transmitter releases the SDA line, allowing the receiver to respond. The receiver then either acknowledges (ACK) by pulling SDA LOW, or does not acknowledge (NoACK) by letting SDA stay HIGH (Figure 4). Bus timing is illustrated in Figure 5.START CONDITIONSTOP CONDITIONSDASCLFigure 2. Start/Stop TimingFigure 3. Slave Address BitsDEVICE ADDRESS** The devices in WLCSP 4−bumps respond only to the Slave Address with: A2 A1 A0 = 000, CAT24C64C4xTRFigure 4. Acknowledge TimingSCL FROM MASTERDATA OUTPUTFROM TRANSMITTERDATA OUTPUT FROM RECEIVER≥ t SU:DAT )Figure 5. Bus TimingSCLSDA INSDA OUTWRITE OPERATIONSByte WriteTo write data to memory, the Master creates a START condition on the bus and then broadcasts a Slave address with the R/W bit set to ‘0’. The Master then sends two address bytes and a data byte and concludes the session by creating a STOP condition on the bus. The Slave responds with ACK after every byte sent by the Master (Figure 6). The STOP starts the internal Write cycle, and while this operation is in progress (t WR ), the SDA output is tri −stated and the Slave does not acknowledge the Master (Figure 7).Page WriteThe Byte Write operation can be expanded to Page Write,by sending more than one data byte to the Slave before issuing the STOP condition (Figure 8). Up to 32 distinct data bytes can be loaded into the internal Page Write Buffer starting at the address provided by the Master. The page address is latched, and as long as the Master keeps sending data, the internal byte address is incremented up to the end of page, where it then wraps around (within the page). New data can therefore replace data loaded earlier. Following the STOP, data loaded during the Page Write session will be written to memory in a single internal Write cycle (t WR ).Acknowledge PollingAs soon (and as long) as internal Write is in progress, the Slave will not acknowledge the Master. This feature enables the Master to immediately follow −up with a new Read or Write request, rather than wait for the maximum specified Write time (t WR ) to elapse. Upon receiving a NoACK response from the Slave, the Master simply repeats the request until the Slave responds with ACK.Hardware Write ProtectionWith the WP pin held HIGH, the entire memory is protected against Write operations. If the WP pin is left floating or is grounded, it has no impact on the Write operation. The state of the WP pin is strobed on the last falling edge of SCL immediately preceding the 1st data byte (Figure 9). If the WP pin is HIGH during the strobe interval,the Slave will not acknowledge the data byte and the Write request will be rejected.Delivery StateThe CAT24C64 is shipped erased, i.e., all bytes are FFh.SLAVE ADDRESSSA ***C KA C KA C KS T O P PST ARTA CKBUS ACTIVITY:MASTER SLAVEADDRESS BYTE ADDRESS BYTE DAT A BYTE Figure 6. Byte Write Sequence*a 15 − a 13 are don’t care bits.a 15 − a 8a 7 − a 0d 7 − d 0Figure 7. Write Cycle TimingSTOPCONDITIONSTARTCONDITIONADDRESSSCLSDASLAVE ADDRESSSA C K A C K C K ST ARTC K S T O C KC K C K BUSACTIVITY:MASTER SLAVEADDRESS BYTE ADDRESS BYTEDATA BYTE DATA BYTE DATA BYTE Figure 8. Page Write SequenceFigure 9. WP TimingADDRESS BYTE DATA BYTESCLSDA WPREAD OPERATIONSImmediate ReadTo read data from memory, the Master creates a START condition on the bus and then broadcasts a Slave address with the R/W bit set to ‘1’. The Slave responds with ACK and starts shifting out data residing at the current address.After receiving the data, the Master responds with NoACK and terminates the session by creating a STOP condition on the bus (Figure 10). The Slave then returns to Standby mode.Selective ReadTo read data residing at a speci fic address, the selected address must first be loaded into the internal address register.This is done by starting a Byte Write sequence, whereby the Master creates a START condition, then broadcasts a Slave address with the R/W bit set to ‘0’ and then sends two address bytes to the Slave. Rather than completing the ByteWrite sequence by sending data, the Master then creates a START condition and broadcasts a Slave address with the R/W bit set to ‘1’. The Slave responds with ACK after every byte sent by the Master and then sends out data residing at the selected address. After receiving the data, the Master responds with NoACK and then terminates the session by creating a STOP condition on the bus (Figure 11).Sequential ReadIf, after receiving data sent by the Slave, the Master responds with ACK, then the Slave will continue transmitting until the Master responds with NoACK followed by STOP (Figure 12). During Sequential Read the internal byte address is automatically incremented up to the end of memory, where it then wraps around to the beginning of memory.Figure 10. Immediate Read Sequence and TimingSCL SDA 8th Bit STOPNO ACKDATA OUT89SLAVE ADDRESSSA C KDATA BYTEN OA C K S T O P PST ARTBUS ACTIVITY:MASTER SLAVEFigure 11. Selective Read SequenceSLAVE ADDRESS SA C KA C KA C K ST ARTSLAVE SA C KS T A R T PS T O P ADDRESS BYTE ADDRESS BYTE ADDRESSN O A C KDATA BYTEBUS ACTIVITY:MASTER SLAVEFigure 12. Sequential Read SequenceS T O SLAVE C KA C A C N O A C A C BYTE n BYTE n+1BYTE n+2BYTE n+xBUS ACTIVITY:MASTERSLAVEORDERING INFORMATIONDevice Order Number SpecificDeviceMarking Package Type Temperature Range Lead Finish ShippingCAT24C64WI−GT324C64F SOIC−8, JEDEC I = Industrial(−40°C to +85°C)NiPdAu Tape & Reel,3,000 Units / ReelCAT24C64YI−GT3C64F TSSOP−8I = Industrial(−40°C to +85°C)NiPdAu Tape & Reel,3,000 Units / ReelCAT24C64HU4I−GT3C6U UDFN−8I = Industrial(−40°C to +85°C)NiPdAu Tape & Reel,3,000 Units / ReelCAT24C64C4CTR A WLCSP−4with Die CoatIndustrial(−40°C to +85°C)N/A Tape & Reel,5,000 Units / ReelCAT24C64C4UTR A WLCSP−4with Die CoatIndustrial(−40°C to +85°C)N/A Tape & Reel,5,000 Units / Reel9.All packages are RoHS−compliant (Lead−free, Halogen−free).10.The standard lead finish is NiPdAu.11.For information on tape and reel specifications, including part orientation and tape sizes, please refer to our Tape and Reel PackagingSpecifications Brochure, BRD8011/D.12.Caution: The EEPROM devices delivered in WLCSP must never be exposed to ultra violet light. When exposed to ultra violet lightthe EEPROM cells lose their stored data.UDFN8, 2x3 EXTENDED PADCASE 517AZ ISSUE ADATE 23 MAR 2015SCALE 2:1NOTES:1.DIMENSIONING AND TOLERANCING PER ASME Y14.5M, 1994.2.CONTROLLING DIMENSION: MILLIMETERS.3.DIMENSION b APPLIES TO PLATEDTERMINAL AND IS MEASURED BETWEEN 0.15 AND 0.25MM FROM THE TERMINAL TIP .4.COPLANARITY APPLIES TO THE EXPOSEDPAD AS WELL AS THE TERMINALS.DIM MIN MAX MILLIMETERS A 0.450.55A10.000.05b 0.200.30D 2.00 BSC D2 1.35 1.45E 3.00 BSC E2 1.25 1.35e 0.50 BSC L 0.250.35*For additional information on our Pb −Free strategy and soldering details, please download the ON Semiconductor Soldering and Mounting Techniques Reference Manual, SOLDERRM/D.SOLDERING FOOTPRINT*DIMENSIONS: MILLIMETERSDETAIL AA30.13 REF L1DETAIL ALALTERNATE CONSTRUCTIONSLL1−−−0.15RECOMMENDEDGENERICMARKING DIAGRAM*XXXXX = Specific Device Code A = Assembly Location WL = Wafer Lot Y = YearW = Work WeekG= Pb −Free Package*This information is generic. Please refer to device data sheet for actual part marking.Pb −Free indicator, “G” or microdot “ G ”,may or may not be present.XXXXX AWLYW G18XDETAIL BALTERNATE CONSTRUCTIONSWLCSP4, 0.77x0.77CASE 567JY ISSUE CDATE 07 MAR 2017PIN A1REFERENCESCALE 4:1TOP VIEWBOTTOM VIEWDIMENSIONS: MILLIMETERS*For additional information on our Pb −Free strategy and soldering details, please download the ON Semiconductor Soldering and Mounting Techniques Reference Manual, SOLDERRM/D.SOLDERING FOOTPRINT*RECOMMENDEDNOTES:1.DIMENSIONING AND TOLERANCING PER ASME Y14.5M, 1994.2.CONTROLLING DIMENSION: MILLIMETERS.3.DATUM C, THE SEATING PLANE, IS DEFINED BY THE SPHERICAL CROWNS OF THE SOLDER BALLS.4.COPLANARITY APPLIES TO SPHERICAL CROWNS OF THE SOLDER BALLS.5.DIMENSION b IS MEASURED AT THE MAXIMUMCONTACT BALL DIAMETER PARALLEL TO DATUM C.6.BACKSIDE COATING IS OPTIONAL.DIM A MIN NOM −−−MILLIMETERS A1D E b 0.150.155e0.40 BSC−−−0.040.06A20.23 REF A30.025 REF 0.750.770.750.77MAX 0.160.350.080.790.79DETAIL ANOTE 6X = Specific Device Code Y = YearW= Work Week*This information is generic. Please refer to device data sheet for actual part marking.Pb −Free indicator, “G” or microdot “ G ”,may or may not be present.GENERICMARKING DIAGRAM*X YWWLCSP4, 0.77x0.77CASE 567PBISSUE ADATE 09 NOV 2016PIN A1REFERENCESCALE 4:1TOP VIEWBOTTOM VIEWDIMENSIONS: MILLIMETERS*For additional information on our Pb−Free strategy and solderingdetails, please download the ON Semiconductor Soldering andMounting Techniques Reference Manual, SOLDERRM/D.SOLDERING FOOTPRINT*RECOMMENDEDNOTES:1.DIMENSIONING AND TOLERANCING PER ASMEY14.5M, 1994.2.CONTROLLING DIMENSION: MILLIMETERS.3.DATUM C, THE SEATING PLANE, IS DEFINED BY THESPHERICAL CROWNS OF THE SOLDER BALLS.4.COPLANARITY APPLIES TO SPHERICAL CROWNS OFTHE SOLDER BALLS.5.DIMENSION b IS MEASURED AT THE MAXIMUMCONTACT BALL DIAMETER PARALLEL TO DATUM C.6.BACKSIDE COATING IS OPTIONAL.DIMAMIN NOM−−−MILLIMETERSA1DEb0.150.155e0.40 BSC−−−0.040.055A20.19 REFA30.025 REF0.750.770.750.77MAX0.160.300.070.790.79DETAIL ANOTE 6X= Specific Device CodeY= YearW= Work Week*This information is generic. Please refer todevice data sheet for actual part marking.Pb−Free indicator, “G” or microdot “ G”,may or may not be present.GENERICMARKING DIAGRAM*XYWSOIC −8, 150 mils CASE 751BD ISSUE ODATE 19 DEC 2008IDENTIFICATIONTOP VIEWSIDE VIEWEND VIEWNotes:(1) All dimensions are in millimeters. Angles in degrees.(2) Complies with JEDEC MS-012.SYMBOLMIN NOM MAX θA A1b cD E E1e h 0º8º0.100.330.190.254.805.803.801.27 BSC1.750.250.510.250.505.006.204.00L0.401.271.35TSSOP8, 4.4x3.0, 0.65PCASE 948AL ISSUE ADATE 20 MAY 2022qXXX = Specific Device Code Y = YearWW = Work WeekA = Assembly Location G= Pb −Free Package*This information is generic. Please refer to device data sheet for actual part marking.Pb −Free indicator, “G” or microdot “G ”, may or may not be present. Some products may not follow the Generic Marking.GENERICMARKING DIAGRAM*XXX YWW A GPUBLICATION ORDERING INFORMATIONTECHNICAL SUPPORTLITERATURE FULFILLMENT:。
单片机模拟I2C总线读写EEPROM(24CXX)程序一

单片机模拟I2C总线读写EEPROM(24CXX)程序一下面是一个最简单的读写程序,可以用来检测线路状况。
先附上程序和电路,后面附有说明。
电路:说明:P2 口的LED 都是我用来检测电路执行到哪一步的,个人觉得一目了然。
程序:#include #define unit unsigned int#define uchar unsigned charint ok;sbit scl=P0;sbit sda=P0;sb it led0=P2;sbit led1=P2;sb it led2=P2 ;sbit led3=P2;sb it led4=P2;sb it led5=P2 ;sbit led6=P2;sb it led7=P2;delay(void) //delay{ int i; led1=1; for(i=0;istart(void) //start{ sda=1; scl=1; delay(); sda=0; delay(); scl=0; led0=0;}stop(void) //stop{ sda=0; scl=1; delay(); sda=1; delay(); scl=0;}checkanswer(void) //check answer{ sda=1; scl=1; if(sda==1) { F0=1; led7=0; } scl=0; led3=0;}sendabyte(int temps) //send a byte{ uchar n=8; while(n--) { led2=1; if((temps&0x80)==0x80){ sda=1; scl=1; delay(); scl=0;}else{ sda=0; scl=1; delay(); scl=0;}temps=tempsreciveabyte() //recive a byte{ uchar n=8,tempr; while(n--) {//uchar idata *abyte scl=1;tempr=temprmain(void) //MAIN{start();sendabyte(0xa0);checkanswer();if(F0==1) return;sendabyte(0x00);checkanswer();if(F0==1) return;sendabyte(0x11);checkanswer();if(F0==1) return;/*-----------------------*/start(); sendabyte(0xa0);checkanswer();if(F0==1) return;。
24C01-24C256共9种EEPROM的字节读写操作程序

24C01-24C256共9种EEPROM的字节读写操作程序一个通用的24C01-24C256共9种EEPROM的字节读写操作程序,此程序有五个入口条件,分别为读写数据缓冲区指针,进行读写的字节数,EEPROM首址,EEPROM控制字节,以及EEPROM类型。
此程序结构性良好,具有极好的容错性,程序机器码也不多:#pragma ot(6,SIZE)#include#include#define ERRORCOUNT 10sbit SDA=P0^0;sbit SCL=P0^1;enum eepromtype {M2401,M2402,M2404,M2408,M2416,M2432,M2464,M24128,M 24256};enum eepromtype EepromType;//DataBuff为读写数据输入/输出缓冲区的首址//ByteQuantity 为要读写数据的字节数量//Address 为EEPROM的片内地址//ControlByte 为EEPROM的控制字节,具体形式为(1)(0)(1)(0)(A2)(A1)(A0)(R/W),其中R/W=1,//表示读操作,R/W=0为写操作,A2,A1,A0为EEPROM的页选或片选地址;//EepromType为枚举变量,需为M2401至M24256中的一种,分别对应24C01至24C256;//函数返回值为一个位变量,若返回1表示此次操作失效,0表示操作成功;//ERRORCOUNT为允许最大次数,若出现ERRORCOUNT次操作失效后,则函数中止操作,并返回1//SDA和SCL由用户自定义,这里暂定义为P0^0和P0^1;//其余的用户不用管,只要把只子程序放在你的程序中并调用它就可以了;/************************************************************** *********************/bit RW24XX(unsigned char *DataBuff,unsigned char ByteQuantity,unsigned int Address,unsigned char ControlByte,enum eepromtype EepromType) {void Delay(unsigned char DelayCount);void IICStart(void);void IICStop(void);bit IICRecAck(void);void IICNoAck(void);void IICAck(void);unsigned char IICReceiveByte(void);void IICSendByte(unsigned char sendbyte);unsigned char data j,i=ERRORCOUNT;bit errorflag=1;while(i--){IICStart();IICSendByte(ControlByte&0xfe);if(IICRecAck())continue;if(EepromType>M2416){IICSendByte((unsigned char)(Address>>8)); if(IICRecAck())continue;}IICSendByte((unsigned char)Address);if(IICRecAck())continue;if(!(ControlByte&0x01)){j=ByteQuantity;errorflag=0; //********clr errorflagwhile(j--){IICSendByte(*DataBuff++);if(!IICRecAck())continue;errorflag=1;break;}if(errorflag==1)continue;break;}else{IICStart();IICSendByte(ControlByte);if(IICRecAck())continue;while(--ByteQuantity){*DataBuff++=IICReceiveByte();IICAck();}*DataBuff=IICReceiveByte(); //read last byte data IICNoAck();errorflag=0;break;}}IICStop();if(!(ControlByte&0x01)){Delay(255);Delay(255);Delay(255);Delay(255);}return(errorflag);}/*****************以下是对IIC总线的操作子程序***//*****************启动总线**********************/ void IICStart(void){SCL=0; //SDA=1;SCL=1;_nop_();_nop_();_nop_();SDA=0;_nop_();_nop_();_nop_();_nop_();SCL=0;SDA=1; //}/*****************停止IIC总线****************/ void IICStop(void){SCL=0;SDA=0;SCL=1;_nop_();_nop_();_nop_();SDA=1;_nop_();_nop_();SCL=0;}/**************检查应答位*******************/bit IICRecAck(void){SCL=0;SDA=1;SCL=1;_nop_();_nop_();_nop_();_nop_();CY=SDA; //因为返回值总是放在CY中的SCL=0;return(CY);}/***************对IIC总线产生应答*******************/ void IICACK(void){SDA=0;SCL=1;_nop_();_nop_();_nop_();_nop_();SCL=0;_nop_();}/*****************不对IIC总线产生应答***************/void IICNoAck(void){SDA=1;SCL=1;_nop_();_nop_();_nop_();_nop_();SCL=0;}/*******************向IIC总线写数据*********************/void IICSendByte(unsigned char sendbyte){unsigned char data j=8;for(;j>0;j--){SCL=0;sendbyte<<=1; //无论C51怎样实现这个操作,始终会使CY=sendbyte^7;SDA=CY;SCL=1;}SCL=0;}/**********************从IIC总线上读数据子程序**********/ unsigned char IICReceiveByte(void){register receivebyte,i=8;SCL=0;while(i--){SCL=1;receivebyte=(receivebyte<<1)|SDA;SCL=0;}return(receivebyte);}/***************一个简单延时程序************************/ void Delay(unsigned char DelayCount){while(DelayCount--);}。
EEPROM---AT24Cxx应用介绍
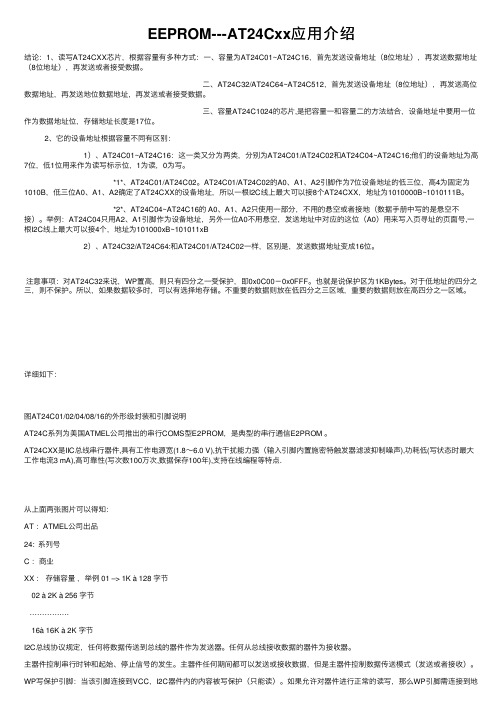
EEPROM---AT24Cxx应⽤介绍结论:1、读写AT24CXX芯⽚,根据容量有多种⽅式:⼀、容量为AT24C01~AT24C16,⾸先发送设备地址(8位地址),再发送数据地址(8位地址),再发送或者接受数据。
⼆、AT24C32/AT24C64~AT24C512,⾸先发送设备地址(8位地址),再发送⾼位数据地址,再发送地位数据地址,再发送或者接受数据。
三、容量AT24C1024的芯⽚,是把容量⼀和容量⼆的⽅法结合,设备地址中要⽤⼀位作为数据地址位,存储地址长度是17位。
2、它的设备地址根据容量不同有区别: 1)、AT24C01~AT24C16:这⼀类⼜分为两类,分别为AT24C01/AT24C02和AT24C04~AT24C16;他们的设备地址为⾼7位,低1位⽤来作为读写标⽰位,1为读,0为写。
*1*、AT24C01/AT24C02。
AT24C01/AT24C02的A0、A1、A2引脚作为7位设备地址的低三位,⾼4为固定为1010B,低三位A0、A1、A2确定了AT24CXX的设备地址,所以⼀根I2C线上最⼤可以接8个AT24CXX,地址为1010000B~1010111B。
*2*、AT24C04~AT24C16的 A0、A1、A2只使⽤⼀部分,不⽤的悬空或者接地(数据⼿册中写的是悬空不接)。
举例:AT24C04只⽤A2、A1引脚作为设备地址,另外⼀位A0不⽤悬空,发送地址中对应的这位(A0)⽤来写⼊页寻址的页⾯号,⼀根I2C线上最⼤可以接4个,地址为101000xB~101011xB 2)、AT24C32/AT24C64:和AT24C01/AT24C02⼀样,区别是,发送数据地址变成16位。
注意事项:对AT24C32来说,WP置⾼,则只有四分之⼀受保护,即0x0C00-0x0FFF。
也就是说保护区为1KBytes。
对于低地址的四分之三,则不保护。
所以,如果数据较多时,可以有选择地存储。
24C64中文资料

EEPROM是"Electrically Erasable Programmable Read-only"(电可擦写可编程只读存储器)的缩写,EEPROM在正常情况下和EPROM一样,可以在掉电的情况下保存数据,所不同的是它可以在特定引脚上施加特定电压或使用特定的总线擦写命令就可以在在线的情况下方便完成数据的擦除和写入,这使EEPROM被用于广阔的的消费者范围,如:汽车、电信、医疗、工业和个人计算机相关的市场,主要用于存储个人数据和配置/调整数据。
EEPROM又分并行EEPROM和串行EEPROM,并行EEPROM器件虽然有很快的读写的速度,但要使用很多的电路引脚。
串行EEPROM器件功能上和并行EEPROM基本相同,提供更少的引脚数、更小的封装、更低的电压和更低的功耗,是现在使用的非易失性存储器中灵活性最高的类型。
串行EEPROM按总线分,常用的有I2C,SPI,Microwire总线。
本文将介绍这三种总线连接单片机的编程方法。
I2C总线I2C总线(Inter Integrated Circuit内部集成电路总线)是两线式串行总线,仅需要时钟和数据两根线就可以进行数据传输,仅需要占用微处理器的2个IO引脚,使用时十分方便。
I2C总线还可以在同一总线上挂多个器件,每个器件可以有自己的器件地址,读写操作时需要先发送器件地址,该地址的器件得到确认后便执行相应的操作,而在同一总线上的其它器件不做响应,称之为器件寻址,这个原理就像我们打电话的原理相当。
I2C总线产生80年代,由PHLIPS公司开发,早期多用于音频和视频设备,如今I2C总线的器件和设备已多不胜数。
最常见的采用I2C总线的EEPROM也已被广泛使用于各种家电、工业及通信设备中,主要用于保存设备所需要的配置数据、采集数据及程序等。
生产I2C总线EEPROM的厂商很多,如ATMEL、Microchip公司,它们都是以24来开头命名芯片型号,最常用就是24C系列。
EEPROM读写c代码

UCB0CTL1 |= UCTXSTP; // 在接收最后一个字节之前发送停止位
while(!(IFG2 & UCB0RXIFG)); // 读取最后一个字节内容
*pword_buf = UCB0RXBUF;
while( UCB0CTL1 & UCTXSTP );
unsigned char eeprom_writebyte(unsigned char word_addr,unsigned char word_value){
IE2 &= ~UCB0TXIE; // Disable TX interrupt
while(UCB0CTL1 & UCTXSTP);
while(UCB0CTL1 & UCTXSTT); // 等待 UCTXSTT = 0
// 若无应答 UCNACKIFG = 1
UCB0CTL1 |= UCTXSTP; // 先发送停止位
while(!(IFG2 & UCB0RXIFG)); // 读取字节内容
while(!(IFG2 & UCB0TXIFG)) //等待 UCB0TXIFG = 1 与 UCTXSTT = 0 同时变化 等待一个标志位即可
{
if( UCB0STAT & UCNACKIFG ) // 若无应答 UCNACKIFG = 1
{
while(UCB0CTL1 & UCTXSTP);
UCB0CTL1 |= UCTR; // 写模式
UCB0CTL1 |= UCTXSTT; // 发送启动位
UCB0TXBUF = FirstWordAddr; // 发送第一个字节地址
24C16的EEPROM的读写程序

24C16规范的51读写C文件(开发板程序之一)/*I2C总线是由数据线SDA和时钟SCL构成的串行总线,可发送和接收数据。
在CPU与被控IC之间、IC与IC之间进行双向传送,最高传送速率400kbps。
各种被控制电路均并联在这条总线上,但就像电话机一样只有拨通各自的号码才能工作,所以每个电路和模块都有唯一的地址,在信息的传输过程中,I2C总线上并接的每一模块电路既是主控器(或被控器),又是发送器(或接收器),这取决于它所要完成的功能。
CPU发出的控制信号分为地址码和控制量两部分,地址码用来选址,即接通需要控制的电路,确定控制的种类;控制量决定该调整的类别(如对比度、亮度等)及需要调整的量。
这样,各控制电路虽然挂在同一条总线上,却彼此独立,互不相关。
I2C总线在传送数据过程中共有三种类型信号,它们分别是:开始信号、结束信号和应答信号。
开始信号:SCL为高电平时,SDA由高电平向低电平跳变,开始传送数据。
结束信号:SCL为低电平时,SDA由低电平向高电平跳变,结束传送数据。
应答信号:接收数据的IC在接收到8bit数据后,向发送数据的IC发出特定的低电平脉冲,表示已收到数据。
CPU向受控单元发出一个信号后,等待受控单元发出一个应答信号,CPU接收到应答信号后,根据实际情况作出是否继续传递信号的判断。
若未收到应答信号,由判断为受控单元出现故障。
目前有很多半导体集成电路上都集成了I2C接口。
*//********************************************************************** ******** (C) Copyright 2007,单片机初学者园地* All Rights reserved.***项目名称: 51单片机学习开发系统***本文件名称:IIC.c** 完成作者:单片机初学者* 当前版本: V1.0* 完成日期:* 描述:本程序结构简单、合理,有帮助于初学者养成良好的编* 程风格。
芯片24C64中文资料

EEPROM是"Electrically Erasable Programmable Read-only"(电可擦写可编程只读存储器)的缩写,EEPROM在正常情况下和EPROM一样,可以在掉电的情况下保存数据,所不同的是它可以在特定引脚上施加特定电压或使用特定的总线擦写命令就可以在在线的情况下方便完成数据的擦除和写入,这使EEPROM被用于广阔的的消费者范围,如:汽车、电信、医疗、工业和个人计算机相关的市场,主要用于存储个人数据和配置/调整数据。
EEPROM又分并行EEPROM和串行EEPROM,并行EEPROM器件虽然有很快的读写的速度,但要使用很多的电路引脚。
串行EEPROM器件功能上和并行EEPROM基本相同,提供更少的引脚数、更小的封装、更低的电压和更低的功耗,是现在使用的非易失性存储器中灵活性最高的类型。
串行EEPROM按总线分,常用的有I2C,SPI,Microwire总线。
本文将介绍这三种总线连接单片机的编程方法。
I2C总线I2C总线(Inter Integrated Circuit内部集成电路总线)是两线式串行总线,仅需要时钟和数据两根线就可以进行数据传输,仅需要占用微处理器的2个IO引脚,使用时十分方便。
I2C总线还可以在同一总线上挂多个器件,每个器件可以有自己的器件地址,读写操作时需要先发送器件地址,该地址的器件得到确认后便执行相应的操作,而在同一总线上的其它器件不做响应,称之为器件寻址,这个原理就像我们打电话的原理相当。
I2C总线产生80年代,由PHLIPS公司开发,早期多用于音频和视频设备,如今I2C总线的器件和设备已多不胜数。
最常见的采用I2C总线的EEPROM也已被广泛使用于各种家电、工业及通信设备中,主要用于保存设备所需要的配置数据、采集数据及程序等。
生产I2C总线EEPROM的厂商很多,如ATMEL、Microchip公司,它们都是以24来开头命名芯片型号,最常用就是24C系列。
单片机EEPROM读写数据流程解析
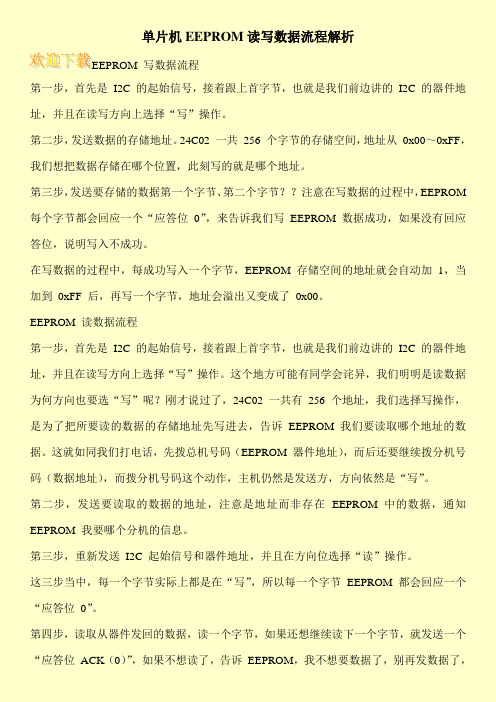
单片机EEPROM读写数据流程解析EEPROM 写数据流程第一步,首先是I2C 的起始信号,接着跟上首字节,也就是我们前边讲的I2C 的器件地址,并且在读写方向上选择“写”操作。
第二步,发送数据的存储地址。
24C02 一共256 个字节的存储空间,地址从0x00~0xFF,我们想把数据存储在哪个位置,此刻写的就是哪个地址。
第三步,发送要存储的数据第一个字节、第二个字节??注意在写数据的过程中,EEPROM 每个字节都会回应一个“应答位0”,来告诉我们写EEPROM 数据成功,如果没有回应答位,说明写入不成功。
在写数据的过程中,每成功写入一个字节,EEPROM 存储空间的地址就会自动加1,当加到0xFF 后,再写一个字节,地址会溢出又变成了0x00。
EEPROM 读数据流程第一步,首先是I2C 的起始信号,接着跟上首字节,也就是我们前边讲的I2C 的器件地址,并且在读写方向上选择“写”操作。
这个地方可能有同学会诧异,我们明明是读数据为何方向也要选“写”呢?刚才说过了,24C02 一共有256 个地址,我们选择写操作,是为了把所要读的数据的存储地址先写进去,告诉EEPROM 我们要读取哪个地址的数据。
这就如同我们打电话,先拨总机号码(EEPROM 器件地址),而后还要继续拨分机号码(数据地址),而拨分机号码这个动作,主机仍然是发送方,方向依然是“写”。
第二步,发送要读取的数据的地址,注意是地址而非存在EEPROM 中的数据,通知EEPROM 我要哪个分机的信息。
第三步,重新发送I2C 起始信号和器件地址,并且在方向位选择“读”操作。
这三步当中,每一个字节实际上都是在“写”,所以每一个字节EEPROM 都会回应一个“应答位0”。
第四步,读取从器件发回的数据,读一个字节,如果还想继续读下一个字节,就发送一个“应答位ACK(0)”,如果不想读了,告诉EEPROM,我不想要数据了,别再发数据了,那就发送一个“非应答位NAK(1)”。
24c64C储存单元使用说明及程序控制

scl_24c64 = 1;
Delay_xus(1);
sda_24c64 = 1; //SDA 上升沿
Delay_xus(1);
scl_24c64 = 0;
}
void ask_24c64() //写数据应答,第九个脉冲 sda_24c64 为低,则 24c64 成功接受数据
{ uchar k=30;
scl_24c64 = 1;
scl_24c64 = 0;
sda_24c64 = 1;
return data_from_24c64;
}
void write_o_data_24c64(uchar addH,uchar addL,uchar data_24c64)//写入单个数据
{ start_24c64();
writebyte_24c64(0xa1);//写 读指令(A0A1A2=000)
for(i=0;i<n;i++) //读 n 个字节数据 { m_data_from_24c64[i] = readbyte_24c64(0); }
stop_24c64(); }*/ void main() { uchar i;
始连续多字节写入
{ uchar i;
start_24c64();
writebyte_24c64(0xa0);//写指令(A0A1A2=000)
writebyte_24c64(addH);//写高位地址
writebyte_24c64(addL);//写低位地址
if(n>32) //每页最多 32 字节
Delay_xus(1);
scl_24c64 = 0;
data_to_24c64<<=1;
I2C接口的EEPROM 24C64芯片的驱动方法

I2C接口的EEPROM24C64芯片的驱动方法与93C46类似的,24C64也是EEPROM,但不同的是24C64是I2C接口的,容量也要更大些,用来存储较大容量的数据,甚至在某些单片机中可以用作程序存储器。
24C64提供65536个位,它们是以字节方式进行组织的。
通过设置不同的地址,可以实现多达8个芯片共享两线总线。
它被广泛应用于工业、化工等需要低功耗与低电压的领域。
同时,它还提供诸如4.5V~5.5V、2.7V~5.5V、2.5V~5.5V与1.8V~5.5V各种工作电压范围的芯片,从而使其应用更加通用。
24C64的引脚定义:引脚功能详细描述:24C64的功能框图:引脚功能描述:串行时钟(SCL):在SCL的上升沿数据写入芯片中,在下降沿从芯片中读出数据。
串行数据(SDA):SDA用作双向数据传输。
这个引脚是漏极开路驱动,需要加上拉电阻。
设备地址(A2,A1,A0):A2~A0是设备地址设置引脚,可以通过接高或接低来设置不同的地址,也可以直接悬空。
设置为不同地址时最多可以在同一总线上存在多达8个芯片。
当这些引脚悬空时,默认地址为0。
写保护(WP):当此引脚接到GND上时,允许正常的写操作。
当WP接到V CC时,所有的写操作都是被禁止的。
如果悬空,则WP在内部被拉到GND。
24C64的组织方式:24C64在内部被组织为256个页,每个页32个字节。
可以按字节来进行操作,地址为13位。
24C64的操作方法:24C64是采用I2C接口来进行数据传输的,在这里不再介绍I2C接口数据传输的相关内容,具体的I2C总线协议在相关章节有详细讲解,敬请翻阅。
下面只针对于24C64的操作方法进行讲解。
1)设备寻址在开始条件使芯片使能后,需要给其写入一个8位的设备地址码,以使某一芯片被命中。
在地址码的开头有两个“10”序列,共4位,然后是3位的地址,最后是1位的读写标识位。
具体的地址码结构如下:24C64使用3个设备地址位A2、A1、A0使多达8个芯片同时存在于一条总线上。
24c64读写程序

24c64读写程序#include //包括一个52标准内核的头文件#define uchar unsigned char //定义一下方便使用#define uint unsigned int#define ulong unsigned long#define WriteDeviceAddress 0xa0 //定义器件在IIC总线中的地址#define ReadDviceAddress 0xa1sbit SCL=P2^7;sbit SDA=P2^6;sbit P20=P2^0;//定时函数void DelayMs(unsigned int number){unsigned char temp;for(;number!=0;number--){for(temp=112;temp!=0;temp--) ;}}//开始总线void Start(){SDA=1;SCL=1;SDA=0;SCL=0;}//结束总线void Stop(){SCL=0;SDA=0;SCL=1;SDA=1;}//发ACK0void NoAck(){SDA=1;SCL=1;SCL=0;}//测试ACKbit TestAck(){bit ErrorBit;SDA=1;SCL=1;ErrorBit=SDA;SCL=0;return(ErrorBit);}//写入8个bit到24c02Write8Bit(unsigned char input) {unsigned char temp;for(temp=8;temp!=0;temp--) {SDA=(bit)(input&0x80);SCL=1;SCL=0;input=input<<1;}}//写入一个字节到24c02中void Write24c64(uchar ch,uchar address) {Start();Write8Bit(WriteDeviceAddress); TestAck();Write8Bit(address);TestAck();Write8Bit(ch);TestAck();Stop();DelayMs(10);}//从24c02中读出8个bituchar Read8Bit(){unsigned char temp,rbyte=0;for(temp=8;temp!=0;temp--){SCL=1;rbyte=rbyte<<1;rbyte=rbyte|((unsigned char)(SDA)); SCL=0;}return(rbyte);}//从24c02中读出1个字节uchar Read24c64(uchar address){uchar ch;Start();Write8Bit(WriteDeviceAddress);TestAck();Write8Bit(address);TestAck();Start();Write8Bit(ReadDviceAddress);TestAck();ch=Read8Bit();NoAck();Stop();return(ch);}//本课试验写入一个字节到24c02并读出来验证void main(void) // 主程序{uchar c1;uchar address = 0x01;for ( address = 0x01;address<0xff;address++){Write24c64(0x00,address);// 将0x88写入到24c02的第2个地址空间c1=Read24c64(address);}P20=0;while(1); //程序挂起}。
用c语言实现24lc256读写

用c语言实现24LC256读写用c语言实现24LC256读写(非软件模拟方式)24LC256工作电压为2.5V~5.5V,容量为32K×8bit,为两线串行接口总线,标准与I2C TM兼容。
SCL为24LC256的时钟输入管脚,SDA为其串行地址/数据输入/数据输出管脚。
24LC256提供读顺序地址内容的操作方式,其内部的地址指针在每次读操作完成以后加1,此地址指针允许在一次读操作期间,持续顺序地读出整个存储器的内容。
#includeunsigned char i=0;unsigned char receive=0x00;void i2c_start(){SEN=1; 1.1.1005-6-15005-6-15005-6-15005-6-15005-6-15005-6-15us_Nop();_Nop();_Nop();_Nop();SCL = 1; //置时钟线为高使数据有敿br> _Nop();_Nop();retc = retc<<1;if(SDA==1){retc = retc + 1; //读数据位,接收的数据放入retc丿br> }_Nop();_Nop();}SCL = 0;_Nop();_Nop();return(retc);}/************************************ I2C_Ackn ************************************函数名:void I2C_Ackn(bit a)入口_房出口_/p>功能描述:主控制器进行应答信号(可以是应答或非应答信号)调用函数_br> 全局变量_/p>创建者:陈曦日期_005-6-15修改者:日期_/p>**********************************************************************************/void I2C_Ackn(bit a){if(a==0) //在此发送应答或非应答信叿br> {SDA = 0;}else{SDA = 1;}_Nop();_Nop();_Nop();SCL = 1;_Nop(); //时钟电平周期大于4 us_Nop();_Nop();_Nop();_Nop();SCL = 0; //清时钟线钳住I2C总线以便继续接收_Nop();_Nop();}/******************************** I2C_ISendB ************************************函数名:bit I2C_ISendB(uchar sla, uchar suba, uchar c)入口:从器件地址sla,子地址suba, 发送字芿c出口_(操作有误)_(操作成功)功能描述:从启动总线到发送地址、数据,结束总线的全进程_br> 若是返回1,表示操作成功,不然操作有误?/p>挪用函数:I2C_Start(),I2C_SendB(uchar c),I2C_Stop()全局变量:I2C_Ack创建者:陈曦日期_005-6-15修改者:日期_/p>**********************************************************************************/bit I2C_ISendB(uchar sla, uchar suba, uchar c){I2C_Start(); //启动总线I2C_SendB(sla); //发送器件地址if(!I2C_Ack){return(0);}I2C_SendB(suba); //发送器件子地址if(!I2C_Ack){return(0);}I2C_SendB(c); //发送数捿br> if(!I2C_Ack){return(0);}I2C_Stop(); //结束总线return(1);}/********************************** I2C_IRcvB ************************************函数名:bit I2C_IRcvB(uchar sla, uchar suba, uchar *c)入口:从器件地址sla, 子地址suba, 收到的数据在c出口_(操作成功)_(操作有误)功能描述:从启动总线到发送地址、读数据,结束总线的全进程?/p>挪用函数_nbsp;I2CS_tart(),I2C_SendB(uchar c),I2C_RcvB(),I2C_Ackn(bit a),I2C_Stop()全局变量:I2C_Ack创建者:陈曦日期_005-5-15修改者:日期_/p>**********************************************************************************/bit I2C_IRcvB(uchar sla, uchar suba, uchar *c){I2C_Start(); //启动总线I2C_SendB(sla);if(!I2C_Ack){return(0);}I2C_SendB(suba); //发送器件子地址if(!I2C_Ack){return(0);}I2C_Start(); //重复起始条件I2C_SendB(sla+1); //发送读操作的地址if(!I2C_Ack){return(0);}*c = I2C_RcvB(); //读取数据I2C_Ackn(1); //发送非应答使br> I2C_Stop(); //结束总线 return(1);}。
芯片24C64中文资料

EEPROM是"Electrically Erasable Programmable Read-only"〔电可擦写可编程只读存储器〕的缩写,EEPROM在正常情况下和EPROM一样,可以在掉电的情况下保存数据,所不同的是它可以在特定引脚上施加特定电压或使用特定的总线擦写命令就可以在在线的情况下方便完成数据的擦除和写入,这使EEPROM被用于广阔的的消费者X围,如:汽车、电信、医疗、工业和个人计算机相关的市场,主要用于存储个人数据和配置/调整数据。
EEPROM又分并行EEPROM和串行EEPROM,并行EEPROM器件虽然有很快的读写的速度,但要使用很多的电路引脚。
串行EEPROM器件功能上和并行EEPROM根本一样,提供更少的引脚数、更小的封装、更低的电压和更低的功耗,是现在使用的非易失性存储器中灵活性最高的类型。
串行EEPROM按总线分,常用的有I2C,SPI,Microwire总线。
本文将介绍这三种总线连接单片机的编程方法。
I2C总线I2C总线〔Inter Integrated Circuit内部集成电路总线〕是两线式串行总线,仅需要时钟和数据两根线就可以进展数据传输,仅需要占用微处理器的2个IO引脚,使用时十分方便。
I2C总线还可以在同一总线上挂多个器件,每个器件可以有自己的器件地址,读写操作时需要先发送器件地址,该地址的器件得到确认后便执行相应的操作,而在同一总线上的其它器件不做响应,称之为器件寻址,这个原理就像我们打的原理相当。
I2C总线产生80年代,由PHLIPS公司开发,早期多用于音频和视频设备,如今I2C总线的器件和设备已多不胜数。
最常见的采用I2C总线的EEPROM也已被广泛使用于各种家电、工业与通信设备中,主要用于保存设备所需要的配置数据、采集数据与程序等。
生产I2C总线EEPROM的厂商很多,如ATMEL、Microchip公司,它们都是以24来开头命名芯片型号,最常用就是24C系列。
K24C系列EEPROM烧写操作流程
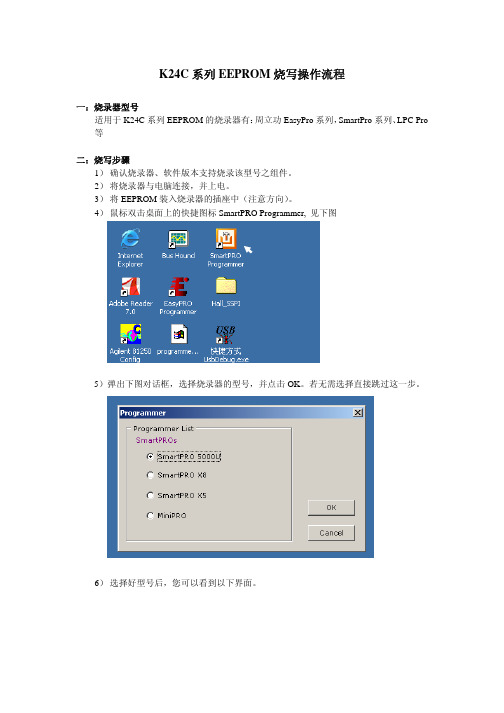
K24C系列EEPROM烧写操作流程一:烧录器型号适用于K24C系列EEPROM的烧录器有:周立功EasyPro系列,SmartPro系列、LPC Pro 等二:烧写步骤1)确认烧录器、软件版本支持烧录该型号之组件。
2)将烧录器与电脑连接,并上电。
3)将EEPROM装入烧录器的插座中(注意方向)。
4)鼠标双击桌面上的快捷图标SmartPRO Programmer, 见下图5)弹出下图对话框,选择烧录器的型号,并点击OK。
若无需选择直接跳过这一步。
6)选择好型号后,您可以看到以下界面。
7)“芯片”菜单,点击“选择芯片”,或在快捷工具栏中点击“选择”。
见下图参见下图红色标记处。
9)打开“选项”菜单,点击“系统设置”,或点击快捷工具栏内的“设置”,参见下图10)在设置对话框内选中所需设置,点击“确定”。
11)点击“编辑”菜单,选择“编辑缓冲区”或“填充缓冲区”,或点击“打开”菜单其中:“编辑缓冲区”您可以进行所有字节编辑,填写您所需数据。
光标停留处可以通过键盘输入。
“填充缓冲区”您可以对所有字节进行编辑,但填充的数据是单一的。
当然您可以通过“打开”文件菜单,导入您所需要的hex文件。
12)若您对每个芯片设置不同的ID,可以点击“芯片”菜单,选择“芯片编号”13)在“芯片编号”对话框中填写您要设定的不同ID的地址段,变化量(自增步长),初始值,自增方式。
例如,我要求每个芯片除了00000040h---00000045h的数据不同,其余地址数据都相同,那么在“自增首址”中填入00000040h,“自增末址”中填入00000045h,自增步长为“1”,初始文件为“00”。
既第一个芯片的00000040h的数据为00,第二个芯片的00000040h的数据为01,其余数据都相同。
14)点击快捷工具栏的“编程”,开始烧录数据。
见下图,若您进行了第12、13步的操作,请在“芯片编号自增”栏打勾。
15)编程结束后,您可以选择“校验”,对烧录好的数据进行对比是否出错。