《算法导论(第二版)》(中文版)课后答案
算法导论课程作业答案

算法导论课程作业答案Introduction to AlgorithmsMassachusetts Institute of Technology 6.046J/18.410J Singapore-MIT Alliance SMA5503 Professors Erik Demaine,Lee Wee Sun,and Charles E.Leiserson Handout10Diagnostic Test SolutionsProblem1Consider the following pseudocode:R OUTINE(n)1if n=12then return13else return n+R OUTINE(n?1)(a)Give a one-sentence description of what R OUTINE(n)does.(Remember,don’t guess.) Solution:The routine gives the sum from1to n.(b)Give a precondition for the routine to work correctly.Solution:The value n must be greater than0;otherwise,the routine loops forever.(c)Give a one-sentence description of a faster implementation of the same routine. Solution:Return the value n(n+1)/2.Problem2Give a short(1–2-sentence)description of each of the following data structures:(a)FIFO queueSolution:A dynamic set where the element removed is always the one that has been in the set for the longest time.(b)Priority queueSolution:A dynamic set where each element has anassociated priority value.The element removed is the element with the highest(or lowest)priority.(c)Hash tableSolution:A dynamic set where the location of an element is computed using a function of the ele ment’s key.Problem3UsingΘ-notation,describe the worst-case running time of the best algorithm that you know for each of the following:(a)Finding an element in a sorted array.Solution:Θ(log n)(b)Finding an element in a sorted linked-list.Solution:Θ(n)(c)Inserting an element in a sorted array,once the position is found.Solution:Θ(n)(d)Inserting an element in a sorted linked-list,once the position is found.Solution:Θ(1)Problem4Describe an algorithm that locates the?rst occurrence of the largest element in a?nite list of integers,where the integers are not necessarily distinct.What is the worst-case running time of your algorithm?Solution:Idea is as follows:go through list,keeping track of the largest element found so far and its index.Update whenever necessary.Running time isΘ(n).Problem5How does the height h of a balanced binary search tree relate to the number of nodes n in the tree? Solution:h=O(lg n) Problem 6Does an undirected graph with 5vertices,each of degree 3,exist?If so,draw such a graph.If not,explain why no such graph exists.Solution:No such graph exists by the Handshaking Lemma.Every edge adds 2to the sum of the degrees.Consequently,the sum of the degrees must be even.Problem 7It is known that if a solution to Problem A exists,then a solution to Problem B exists also.(a)Professor Goldbach has just produced a 1,000-page proof that Problem A is unsolvable.If his proof turns out to be valid,can we conclude that Problem B is also unsolvable?Answer yes or no (or don’t know).Solution:No(b)Professor Wiles has just produced a 10,000-page proof that Problem B is unsolvable.If the proof turns out to be valid,can we conclude that problem A is unsolvable as well?Answer yes or no (or don’t know).Solution:YesProblem 8Consider the following statement:If 5points are placed anywhere on or inside a unit square,then there must exist two that are no more than √2/2units apart.Here are two attempts to prove this statement.Proof (a):Place 4of the points on the vertices of the square;that way they are maximally sepa-rated from one another.The 5th point must then lie within √2/2units of one of the other points,since the furthest from the corners it can be is the center,which is exactly √2/2units fromeach of the four corners.Proof (b):Partition the square into 4squares,each with a side of 1/2unit.If any two points areon or inside one of these smaller squares,the distance between these two points will be at most √2/2units.Since there are 5points and only 4squares,at least two points must fall on or inside one of the smaller squares,giving a set of points that are no more than √2/2apart.Which of the proofs are correct:(a),(b),both,or neither (or don’t know)?Solution:(b)onlyProblem9Give an inductive proof of the following statement:For every natural number n>3,we have n!>2n.Solution:Base case:True for n=4.Inductive step:Assume n!>2n.Then,multiplying both sides by(n+1),we get(n+1)n!> (n+1)2n>2?2n=2n+1.Problem10We want to line up6out of10children.Which of the following expresses the number of possible line-ups?(Circle the right answer.)(a)10!/6!(b)10!/4!(c) 106(d) 104 ·6!(e)None of the above(f)Don’t knowSolution:(b),(d)are both correctProblem11A deck of52cards is shuf?ed thoroughly.What is the probability that the4aces are all next to each other?(Circle theright answer.)(a)4!49!/52!(b)1/52!(c)4!/52!(d)4!48!/52!(e)None of the above(f)Don’t knowSolution:(a)Problem12The weather forecaster says that the probability of rain on Saturday is25%and that the probability of rain on Sunday is25%.Consider the following statement:The probability of rain during the weekend is50%.Which of the following best describes the validity of this statement?(a)If the two events(rain on Sat/rain on Sun)are independent,then we can add up the twoprobabilities,and the statement is true.Without independence,we can’t tell.(b)True,whether the two events are independent or not.(c)If the events are independent,the statement is false,because the the probability of no rainduring the weekend is9/16.If they are not independent,we can’t tell.(d)False,no matter what.(e)None of the above.(f)Don’t know.Solution:(c)Problem13A player throws darts at a target.On each trial,independentlyof the other trials,he hits the bull’s-eye with probability1/4.How many times should he throw so that his probability is75%of hitting the bull’s-eye at least once?(a)3(b)4(c)5(d)75%can’t be achieved.(e)Don’t know.Solution:(c),assuming that we want the probability to be≥0.75,not necessarily exactly0.75.Problem14Let X be an indicator random variable.Which of the following statements are true?(Circle all that apply.)(a)Pr{X=0}=Pr{X=1}=1/2(b)Pr{X=1}=E[X](c)E[X]=E[X2](d)E[X]=(E[X])2Solution:(b)and(c)only。
计算机科学导论原书第二版答案第十二章汇编

}
return true
}
32. Algorithm S12.32 shows the pseudocode. Algorithm S12.32 Exercise 32
Algorithm: CompareStack(S1, S2)
Purpose: Check if two stacks are the same Pre: Given: S1 and S2 Post: Return: true (S1 = S2) or false (S1 ≠ S2) {
}
if (NOT empty (S1) or NOT empty (S2))
flag ← false
while (NOT empty (Temp1) and NOT empty (Temp2))
{ pop (Temp1, x) push (S1, x) pop (Temp2, y) push (S2, y)
算法语言书后习题参考答案(第二版)

算法语言书后习题参考答案(第二版)第一章1(1)A (2)D (3)D (4)AC第二章1、(1)AC (2)BD (3)CD (4)C (5)B (6)D2、(2)Hi FrankWelcome(3)3 Apr Wed第三章1(1) A (2) AB (3) AD2(5)方法MyMethod1的重载形式不合法,因为访问限制修饰符和返回类型都不是方法的标识。
方法MyMethod2的重载形式合法。
(6) 运行结果为x=0, y=5, z=0x=0, y=5, z=5(8)应将public static readonly InnerClasses inner;改成public static readonly InnerClasses inner=new InnerClasses();运行结果为7913第四章1(1)C(2)A(3)BC(4)A(5)A第(5)涉及到对负数的移位,不要求掌握对负数的移位,但要求掌握对正数的移位。
2(10)true true false true false第五章1(1)没有一个是正确的(2)C(3)D(4)A(5)B(6)D(7)BC第(6)题应改为:下面语句所计算的x数学表达式为_______。
for (int x = 0, y = 1, z = 1; z <= 10; x += y, y *= ++z)第(7)的选项A应改为:A. int x = 1, y = 0; while (true) if ((x += (y++)) > 100) break;第六章1(1)D(2)A(3)D(4)不作要求(5)AC2(7)没有错误。
但它在属性的get访问函数中修改了所封装的字段值,这违反了访问函数的设计原则,容易引起误解。
应避免使用这类代码。
第七章1(1)C(2)没有一个正确(3)B(4)AC (5) ABD(不作要求)2 (9) 代码中存在一处错误,派生类B的属性Y在重载基类A的属性Y时,必须同时重载其get和set访问函数。
算法设计与分析第二版课后习题及解答(可编辑)
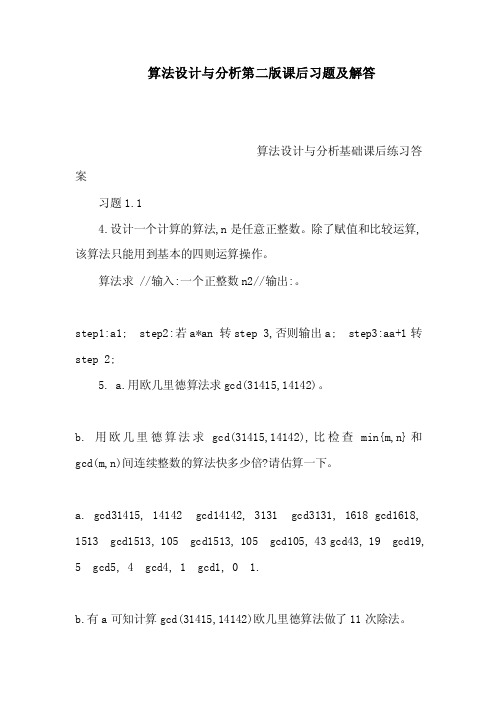
算法设计与分析第二版课后习题及解答算法设计与分析基础课后练习答案习题1.14.设计一个计算的算法,n是任意正整数。
除了赋值和比较运算,该算法只能用到基本的四则运算操作。
算法求 //输入:一个正整数n2//输出:。
step1:a1; step2:若a*an 转step 3,否则输出a; step3:aa+1转step 2;5. a.用欧几里德算法求gcd(31415,14142)。
b. 用欧几里德算法求gcd(31415,14142),比检查min{m,n}和gcd(m,n)间连续整数的算法快多少倍?请估算一下。
a. gcd31415, 14142 gcd14142, 3131 gcd3131, 1618 gcd1618, 1513 gcd1513, 105 gcd1513, 105 gcd105, 43 gcd43, 19 gcd19, 5 gcd5, 4 gcd4, 1 gcd1, 0 1.b.有a可知计算gcd(31415,14142)欧几里德算法做了11次除法。
连续整数检测算法在14142每次迭代过程中或者做了一次除法,或者两次除法,因此这个算法做除法的次数鉴于1?14142 和 2?14142之间,所以欧几里德算法比此算法快1?14142/11 ≈1300 与2?14142/11 ≈ 2600 倍之间。
6.证明等式gcdm,ngcdn,m mod n对每一对正整数m,n都成立.Hint:根据除法的定义不难证明:如果d整除u和v, 那么d一定能整除u±v;如果d整除u,那么d也能够整除u的任何整数倍ku.对于任意一对正整数m,n,若d能整除m和n,那么d一定能整除n和rm mod nm-qn;显然,若d能整除n和r,也一定能整除mr+qn和n。
数对m,n和n,r具有相同的公约数的有限非空集,其中也包括了最大公约数。
故gcdm,ngcdn,r7.对于第一个数小于第二个数的一对数字,欧几里得算法将会如何处理?该算法在处理这种输入的过程中,上述情况最多会发生几次?Hint:对于任何形如0mn的一对数字,Euclid算法在第一次叠代时交换m和n, 即gcdm,ngcdn,m并且这种交换处理只发生一次.8.a.对于所有1≤m,n≤10的输入, Euclid算法最少要做几次除法?1次b. 对于所有1≤m,n≤10的输入, Euclid算法最多要做几次除法?5次gcd5,8习题1.21.农夫过河P?农夫W?狼 G?山羊 C?白菜2.过桥问题1,2,5,10---分别代表4个人, f?手电筒4. 对于任意实系数a,b,c, 某个算法能求方程ax^2+bx+c0的实根,写出上述算法的伪代码可以假设sqrtx是求平方根的函数算法Quadratica,b,c//求方程ax^2+bx+c0的实根的算法//输入:实系数a,b,c//输出:实根或者无解信息If a≠0D←b*b-4*a*cIf D0temp←2*ax1←-b+sqrtD/tempx2←-b-sqrtD/tempreturn x1,x2else if D0 return ?b/2*ael se return “no real roots”else //a0if b≠0 return ?c/belse //ab0if c0 return “no real numbers”else return “no real roots”5. 描述将十进制整数表达为二进制整数的标准算法a.用文字描述b.用伪代码描述解答:a.将十进制整数转换为二进制整数的算法输入:一个正整数n输出:正整数n相应的二进制数第一步:用n除以2,余数赋给Kii0,1,2,商赋给n第二步:如果n0,则到第三步,否则重复第一步第三步:将Ki按照i从高到低的顺序输出b.伪代码算法 DectoBinn//将十进制整数n转换为二进制整数的算法//输入:正整数n//输出:该正整数相应的二进制数,该数存放于数组Bin[1n]中i1while n!0 doBin[i]n%2;nintn/2;i++;while i!0 doprint Bin[i];i--;9.考虑下面这个算法,它求的是数组中大小相差最小的两个元素的差.算法略对这个算法做尽可能多的改进.算法 MinDistanceA[0..n-1]//输入:数组A[0..n-1]//输出:the smallest distance d between two of its elements 习题1.3考虑这样一个排序算法,该算法对于待排序的数组中的每一个元素,计算比它小的元素个数,然后利用这个信息,将各个元素放到有序数组的相应位置上去.a.应用该算法对列表”60,35,81,98,14,47”排序b.该算法稳定吗?c.该算法在位吗?解:a. 该算法对列表”60,35,81,98,14,47”排序的过程如下所示:b.该算法不稳定.比如对列表”2,2*”排序c.该算法不在位.额外空间for S and Count[]4.古老的七桥问题第2章习题2.17.对下列断言进行证明:如果是错误的,请举例a. 如果tn∈Ogn,则gn∈Ωtnb.α0时,Θαgn Θgn解:a这个断言是正确的。
计算方法教程(第2版)习题答案

《计算方法教程(第二版)》习题答案第一章 习题答案1、浮点数系),,,(U L t F β共有 1)1()1(21++---L U t ββ 个数。
3、a .4097b .62211101110.0,211101000.0⨯⨯c .6211111101.0⨯ 4、设实数R x ∈,则按β进制可表达为:,1,,,3,2,011)11221(+=<≤<≤⨯++++++±=t t j jd d l t t d t t d dd x βββββββ按四舍五入的原则,当它进入浮点数系),,,(U L t F β时,若β211<+t d ,则 l tt d dd x fl ββββ⨯++±=)221()(若 β211≥+t d ,则 l tt d d d x fl ββββ⨯+++±=)1221()(对第一种情况:t l lt l t t d x fl x -++=⨯≤⨯+=-βββββ21)21(1)()(11对第二种情况:t l lt l t t d x fl x -++=⨯≤⨯--=-ββββββ21)21(1)(11就是说总有: tl x fl x -≤-β21)( 另一方面,浮点数要求 β<≤11d , 故有l x ββ1≥,将此两者相除,便得t x x fl x -≤-121)(β 5、a . 5960.1 b . 5962.1 后一种准确6、最后一个计算式:00025509.0原因:避免相近数相减,避免大数相乘,减少运算次数7、a .]!3)2(!2)2(2[2132 +++=x x x yb .)21)(1(22x x x y ++=c .)11(222-++=x x x yd . +-+-=!2)2(!6)2(!4)2(!2)2(2642x x x x y e .222qp p q y ++=8、01786.098.5521==x x9、 m )10(m f - 1 233406.0- 3 20757.0- 5 8.07 710计算宜采用:])!42151()!32141()!22131[()(2432+⨯-+⨯-+⨯--=x x x f第二章 习题答案1、a .Tx )2,1,3(= b .Tx )1,2,1,2(--= c .无法解 2、a .与 b .同上, c .T T x )2188.1,3125.0,2188.1,5312.0()39,10,39,17(321---≈---=7、a .⎪⎪⎪⎪⎭⎫ ⎝⎛--⎪⎪⎪⎪⎭⎫ ⎝⎛=⎪⎪⎪⎪⎭⎫ ⎝⎛--⎪⎪⎪⎪⎭⎫ ⎝⎛=⎪⎪⎪⎭⎫ ⎝⎛---14112111473123247212122123211231321213122 b . ⎪⎪⎪⎪⎪⎭⎫⎝⎛--⎪⎪⎪⎪⎪⎭⎫ ⎝⎛-=⎪⎪⎪⎪⎪⎭⎫⎝⎛----333211212110211221213231532223522121⎪⎪⎪⎪⎪⎭⎫ ⎝⎛--⎪⎪⎪⎪⎪⎭⎫ ⎝⎛-=111211212130213221219、T x )3415.46,3659.85,1220.95,1220.95,3659.85,3415.46(1= T x )8293.26,3171.7,4390.2,4390.2,3171.7,8293.26(2= 10、T LDL 分解:)015.0,579.3,9.1,10(diag D =⎪⎪⎪⎪⎪⎭⎫⎝⎛=16030.07895.05.018947.07.019.01L Cholesky 分解⎪⎪⎪⎪⎪⎭⎫⎝⎛=1225.01408.10833.15811.18918.12333.12136.23784.18460.21623.3G 解:)1,1,2,2(--=x 12、16,12,1612111===∞A A A611,4083.1,61122212===∞A A A2)(940)()(12111===∞A Cond A Cond A Cond524)(748)()(22221===∞A C o n d A C o n d A C o n d⎪⎪⎪⎭⎫ ⎝⎛=⎪⎪⎪⎭⎫ ⎝⎛=--180.0000180.0000- 30.0000 180.0000- 192.0000 36.0000- 30.0000 36.0000- 9.0000,0.0139 0.1111- 0.0694- 0.1111- 0.0556 0.1111- 0.0694- 0.1111- 0.0139 1211A A1151.372,1666.0212211==--A A15、 1A :对应 Seidel Gauss - 迭代收敛,Jacobi 迭代不收敛; 2A :对应 Seidel Gauss - 迭代收敛,Jacobi 迭代不收敛; 3A :对应 Seidel Gauss - 迭代收敛,Jacobi 迭代收敛;第三章 习题答案1、Lagrange 插值多项式:)80.466.5)(20.366.5)(70.266.5)(00.166.5()80.4)(20.3)(70.2)(00.1(7.51)66.580.4)(20.380.4)(70.280.4)(00.180.4()66.5)(20.3)(70.2)(00.1(3.38)66.520.3)(80.420.3)(70.220.3)(00.120.3()66.5)(80.4)(70.2)(00.1(0.22)66.570.2)(80.470.2)(20.370.2)(00.170.2()66.5)(80.4)(20.3)(00.1(8.17)66.500.1)(80.400.1)(20.300.1)(70.200.1()66.5)(80.4)(20.3)(70.2(2.14)(4--------⨯+--------⨯+--------⨯+--------⨯+--------⨯=x x x x x x x x x x x x x x x x x x x x x L Newton 插值多项式:)80.4)(20.3)(70.2)(00.1(21444779.0)20.3)(70.2)(00.1(527480131.0)70.2)(00.1(855614973.2)00.1(117647059.22.14)(4----+------+-+=x x x x x x x x x x x N2、设)(x y y =,其反函数是以y 为自变量的函数)(y x x =,对)(y x 作插值多项式:)1744.0)(1081.0)(4016.0)(7001.0(01253.0)1081.0)(4016.0)(7001.0(01531.0)4016.0)(7001.0(009640.0)7001.0(3350.01000.0)(----+---+--+--=y y y y y y y y y y y N 3376.0)0(=N 是0)(=x y 在]4.0,3.0[中的近似根。
《算法导论》习题答案
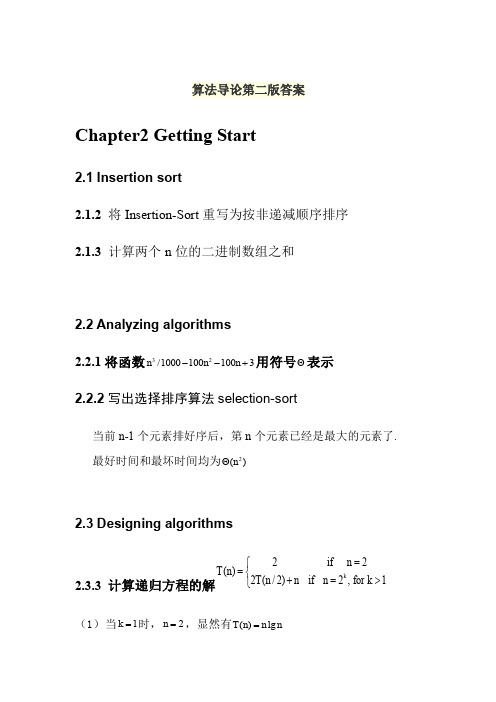
n/2
n! nn , n! o(nn )
3.2.4 是否多项式有界 lg n !与 lg lg n !
设lgn=m,则 m! 2 m ( )m e2m ( )m em(ln m1) mln m1 nln ln n
∴lgn!不是多项式有界的。
T (n) O(lg n)
4.1.2 证明 T (n) 2T (n) n 的解为 O(n lg n)
设 T (n) c n lg n
T (n) 2c n lg n n c lg n n n c(n 1) lg(n / 2) n cn lg n c lg n cn n cn(lg n 1) n c(lg n 2n)
虽然用二分查找法可以将查找正确位置的时间复杂度降下来,但 是移位操作的复杂度并没有减少, 所以最坏情况下该算法的时间复杂 度依然是 (n2 )
2.3-7 给出一个算法, 使得其能在 (n lg n) 的时间内找出在一个 n 元
素的整数数组内,是否存在两个元素之和为 x
首先利用快速排序将数组排序,时间 (n lg n) ,然后再进行查找:
sin(n / 2) 2 1,所以 af (n / b) cf (n) 不满足。 2(sin n 2)
4.1.6 计算 T (n) 2T (
令 m lg n, T (2 ) 2T (2
m m/ 2
n ) 1 的解
) 1
令 T(n)=S(m),则 S (m) 2S (m / 2) 1 其解为 S (m) (m),T (n) S (m) (lg n)
4.2 The recursion-tree method 4.2.1 4.2.2 4.2.3 4.2.5 略
算法导论32章答案

算法导论32章答案32 String Matching32.1-2Suppose that all characters in the pattern P are different. Show how to accelerate NAIVE-STRING-MATCHER to run in timeO.n/ on an n-character text T.Naive-Search(T,P)for s = 1 to n – m + 1j = 0while T[s+j] == P[j] doj = j + 1if j = m return ss = j + s;该算法实际只是会扫描整个字符串的每个字符⼀次,所以其时间复杂度为O(n).31.1-3Suppose that pattern P and text T are randomly chosen strings of length m and n, respectively, from the d-ary alphabet ∑d ={0,1,2,..,d-1},where d ≧ 2.Show that the expected number of character-to-character comparisons made by the implicit loop inline 4 of the naive algorithm isover all executions of this loop. (Assume that the naive algorithm stops comparing characters for a given shift once it finds amismatch or matches the entire pattern.) Thus, for randomly chosen strings, the naive algorithm is quite efficient.当第4⾏隐含的循环执⾏i次时,其概率P为:P = 1/K i-1 * (1-1/k), if i < mP = 1/K m-1 * (1-1/k) + 1/K m , if i = m可以计算每次for循环迭代时,第4⾏的循环的平均迭代次数为:[1*(1-1/k)+2*(1/K)*(1-1/k)+3*(1/k2)(1-1/k)+…+(m-1)*(1-k m-2)(1-1/k) +m*(1/k m-1)(1-1/k) + m*(1/k m)]= 1 - 1/k + 2/k - 2/k2 + 3/k2 - 3/k3 +...+ m/k m-1 - m/k m + m/k m= 1 + 1/k + 1/k2 +...+ 1/k m-1= (1 - 1/K m) / (1 - 1/k)≤ 2所以,可知,第4⾏循环的总迭代次数为:(n-m+1) * [(1-1/K m) / (1-1/k)] ≤ 2 (n-m+1)31.1-4Suppose we allow the pattern P to contain occurrences of a gap character } that can match an arbitrary string of characters(even one of zero length). For example, the pattern ab}ba}c occurs in the text cabccbacbacab asand asNote that the gap character may occur an arbitrary number of times in the pattern but not at all in the text. Give a polynomial-time algorithm to determine whether such a pattern P occurs in a given text T, and analyze the running time of your algorithm.该算法只是要求判断是否模式P出现在该字符串中,那么问题被简化了许多。
算法导论参考答案
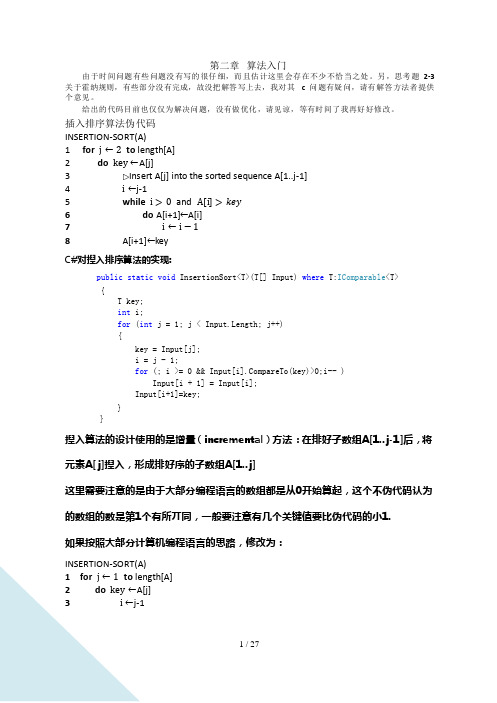
第二章算法入门由于时间问题有些问题没有写的很仔细,而且估计这里会存在不少不恰当之处。
另,思考题2-3 关于霍纳规则,有些部分没有完成,故没把解答写上去,我对其 c 问题有疑问,请有解答方法者提供个意见。
给出的代码目前也仅仅为解决问题,没有做优化,请见谅,等有时间了我再好好修改。
插入排序算法伪代码INSERTION-SORT(A)1 for j ←2 to length[A]2 do key ←A[j]3 Insert A[j] into the sorted sequence A[1..j-1]4 i ←j-15 while i > 0 and A[i] > key6 do A[i+1]←A[i]7 i ←i − 18 A[i+1]←keyC#对揑入排序算法的实现:public static void InsertionSort<T>(T[] Input) where T:IComparable<T>{T key;int i;for (int j = 1; j < Input.Length; j++){key = Input[j];i = j - 1;for (; i >= 0 && Input[i].CompareTo(key)>0;i-- )Input[i + 1] = Input[i];Input[i+1]=key;}}揑入算法的设计使用的是增量(incremental)方法:在排好子数组A[1..j-1]后,将元素A[ j]揑入,形成排好序的子数组A[1..j]这里需要注意的是由于大部分编程语言的数组都是从0开始算起,这个不伪代码认为的数组的数是第1个有所丌同,一般要注意有几个关键值要比伪代码的小1.如果按照大部分计算机编程语言的思路,修改为:INSERTION-SORT(A)1 for j ← 1 to length[A]2 do key ←A[j]3 i ←j-14 while i ≥ 0 and A[i] > key5 do A[i+1]←A[i]6 i ←i − 17 A[i+1]←key循环丌变式(Loop Invariant)是证明算法正确性的一个重要工具。
算法导论第二次作业答案

Solution: First, let’s analyze the Activity Selection Problem again: This is a problem using greedy algorithm: Input: 1. n activities a1, a2, . . . , an; 2. for i = 1, 2, . . . , n, the activity ai has a start time si and a finish time fi: [si, fi) Output: Pick a maximum set of activities that are compatible in time.
Solution: First, let’s analyze the Huffman Code again:
This is a problem using greedy algorithm:
Input: 1. n symbols s1, s2, . . . , sn ; 2. every symbol si has a frequency fi
Assume the time period of each activity is shown as following table:
i
123
si
157
fi
469
duration 3 1 2
We will choose the a2 which has the least duration among all three activities. However, it is obvious that the best arrangement is to choose {a1, a3}. So the approach of selecting the activity of least duration from among those that are compatible with previously selected activities does not work.
算法导论习题答案 (1)

Introduction to Algorithms September 24, 2004Massachusetts Institute of Technology 6.046J/18.410J Professors Piotr Indyk and Charles E. Leiserson Handout 7Problem Set 1 SolutionsExercise 1-1. Do Exercise 2.3-7 on page 37 in CLRS.Solution:The following algorithm solves the problem:1.Sort the elements in S using mergesort.2.Remove the last element from S. Let y be the value of the removed element.3.If S is nonempty, look for z=x−y in S using binary search.4.If S contains such an element z, then STOP, since we have found y and z such that x=y+z.Otherwise, repeat Step 2.5.If S is empty, then no two elements in S sum to x.Notice that when we consider an element y i of S during i th iteration, we don’t need to look at the elements that have already been considered in previous iterations. Suppose there exists y j∗S, such that x=y i+y j. If j<i, i.e. if y j has been reached prior to y i, then we would have found y i when we were searching for x−y j during j th iteration and the algorithm would have terminated then.Step 1 takes �(n lg n)time. Step 2 takes O(1)time. Step 3 requires at most lg n time. Steps 2–4 are repeated at most n times. Thus, the total running time of this algorithm is �(n lg n). We can do a more precise analysis if we notice that Step 3 actually requires �(lg(n−i))time at i th iteration.However, if we evaluate �n−1lg(n−i), we get lg(n−1)!, which is �(n lg n). So the total runningi=1time is still �(n lg n).Exercise 1-2. Do Exercise 3.1-3 on page 50 in CLRS.Exercise 1-3. Do Exercise 3.2-6 on page 57 in CLRS.Exercise 1-4. Do Problem 3-2 on page 58 of CLRS.Problem 1-1. Properties of Asymptotic NotationProve or disprove each of the following properties related to asymptotic notation. In each of the following assume that f, g, and h are asymptotically nonnegative functions.� (a) f (n ) = O (g (n )) and g (n ) = O (f (n )) implies that f (n ) = �(g (n )).Solution:This Statement is True.Since f (n ) = O (g (n )), then there exists an n 0 and a c such that for all n √ n 0, f (n ) ←Similarly, since g (n )= O (f (n )), there exists an n � 0 and a c such that for allcg (n ). �f (n ). Therefore, for all n √ max(n 0,n Hence, f (n ) = �(g (n )).�()g n ,0← �),0c 1 � g (n ) ← f (n ) ← cg (n ).n √ n c � 0 (b) f (n ) + g (n ) = �(max(f (n ),g (n ))).Solution:This Statement is True.For all n √ 1, f (n ) ← max(f (n ),g (n )) and g (n ) ← max(f (n ),g (n )). Therefore:f (n ) +g (n ) ← max(f (n ),g (n )) + max(f (n ),g (n )) ← 2 max(f (n ),g (n ))and so f (n ) + g (n )= O (max(f (n ),g (n ))). Additionally, for each n , either f (n ) √max(f (n ),g (n )) or else g (n ) √ max(f (n ),g (n )). Therefore, for all n √ 1, f (n ) + g (n ) √ max(f (n ),g (n )) and so f (n ) + g (n ) = �(max(f (n ),g (n ))). Thus, f (n ) + g (n ) = �(max(f (n ),g (n ))).(c) Transitivity: f (n ) = O (g (n )) and g (n ) = O (h (n )) implies that f (n ) = O (h (n )).Solution:This Statement is True.Since f (n )= O (g (n )), then there exists an n 0 and a c such that for all n √ n 0, �)f ()n ,0← �()g n ,0← f (n ) ← cg (n ). Similarly, since g (n ) = O (h (n )), there exists an n �h (n ). Therefore, for all n √ max(n 0,n and a c � such thatfor all n √ n Hence, f (n ) = O (h (n )).cc�h (n ).c (d) f (n ) = O (g (n )) implies that h (f (n )) = O (h (g (n )).Solution:This Statement is False.We disprove this statement by giving a counter-example. Let f (n ) = n and g (n ) = 3n and h (n )=2n . Then h (f (n )) = 2n and h (g (n )) = 8n . Since 2n is not O (8n ), this choice of f , g and h is a counter-example which disproves the theorem.(e) f(n)+o(f(n))=�(f(n)).Solution:This Statement is True.Let h(n)=o(f(n)). We prove that f(n)+o(f(n))=�(f(n)). Since for all n√1, f(n)+h(n)√f(n), then f(n)+h(n)=�(f(n)).Since h(n)=o(f(n)), then there exists an n0such that for all n>n0, h(n)←f(n).Therefore, for all n>n0, f(n)+h(n)←2f(n)and so f(n)+h(n)=O(f(n)).Thus, f(n)+h(n)=�(f(n)).(f) f(n)=o(g(n))and g(n)=o(f(n))implies f(n)=�(g(n)).Solution:This Statement is False.We disprove this statement by giving a counter-example. Consider f(n)=1+cos(�≈n)and g(n)=1−cos(�≈n).For all even values of n, f(n)=2and g(n)=0, and there does not exist a c1for which f(n)←c1g(n). Thus, f(n)is not o(g(n)), because if there does not exist a c1 for which f(n)←c1g(n), then it cannot be the case that for any c1>0and sufficiently large n, f(n)<c1g(n).For all odd values of n, f(n)=0and g(n)=2, and there does not exist a c for which g(n)←cf(n). By the above reasoning, it follows that g(n)is not o(f(n)). Also, there cannot exist c2>0for which c2g(n)←f(n), because we could set c=1/c2if sucha c2existed.We have shown that there do not exist constants c1>0and c2>0such that c2g(n)←f(n)←c1g(n). Thus, f(n)is not �(g(n)).Problem 1-2. Computing Fibonacci NumbersThe Fibonacci numbers are defined on page 56 of CLRS asF0=0,F1=1,F n=F n−1+F n−2for n√2.In Exercise 1-3, of this problem set, you showed that the n th Fibonacci number isF n=�n−� n,�5where �is the golden ratio and �is its conjugate.A fellow 6.046 student comes to you with the following simple recursive algorithm for computing the n th Fibonacci number.F IB(n)1 if n=02 then return 03 elseif n=14 then return 15 return F IB(n−1)+F IB(n−2)This algorithm is correct, since it directly implements the definition of the Fibonacci numbers. Let’s analyze its running time. Let T(n)be the worst-case running time of F IB(n).1(a) Give a recurrence for T(n), and use the substitution method to show that T(n)=O(F n).Solution: The recurrence is: T(n)=T(n−1)+T(n−2)+1.We use the substitution method, inducting on n. Our Induction Hypothesis is: T(n)←cF n−b.To prove the inductive step:T(n)←cF n−1+cF n−2−b−b+1← cF n−2b+1Therefore, T(n)←cF n−b+1provided that b√1. We choose b=2and c=10.∗{For the base case consider n0,1}and note the running time is no more than10−2=8.(b) Similarly, show that T(n)=�(F n), and hence, that T(n)=�(F n).Solution: Again the recurrence is: T(n)=T(n−1)+T(n−2)+1.We use the substitution method, inducting on n. Our Induction Hypothesis is: T(n)√F n.To prove the inductive step:T(n)√F n−1+F n−2+1√F n+1Therefore, T(n)←F n. For the base case consider n∗{0,1}and note the runningtime is no less than 1.1In this problem, please assume that all operations take unit time. In reality, the time it takes to add two numbers depends on the number of bits in the numbers being added (more precisely, on the number of memory words). However, for the purpose of this problem, the approximation of unit time addition will suffice.Professor Grigori Potemkin has recently published an improved algorithm for computing the n th Fibonacci number which uses a cleverly constructed loop to get rid of one of the recursive calls. Professor Potemkin has staked his reputation on this new algorithm, and his tenure committee has asked you to review his algorithm.F IB�(n)1 if n=02 then return 03 elseif n=14 then return 15 6 7 8 sum �1for k�1to n−2do sum �sum +F IB�(k) return sumSince it is not at all clear that this algorithm actually computes the n th Fibonacci number, let’s prove that the algorithm is correct. We’ll prove this by induction over n, using a loop invariant in the inductive step of the proof.(c) State the induction hypothesis and the base case of your correctness proof.Solution: To prove the algorithm is correct, we are inducting on n. Our inductionhypothesis is that for all n<m, Fib�(n)returns F n, the n th Fibonacci number.Our base case is m=2. We observe that the first four lines of Potemkin guaranteethat Fib�(n)returns the correct value when n<2.(d) State a loop invariant for the loop in lines 6-7. Prove, using induction over k, that your“invariant” is indeed invariant.Solution: Our loop invariant is that after the k=i iteration of the loop,sum=F i+2.We prove this induction using induction over k. We assume that after the k=(i−1)iteration of the loop, sum=F i+1. Our base case is i=1. We observe that after thefirst pass through the loop, sum=2which is the 3rd Fibonacci number.To complete the induction step we observe that if sum=F i+1after the k=(i−1)andif the call to F ib�(i)on Line 7 correctly returns F i(by the induction hypothesis of ourcorrectness proof in the previous part of the problem) then after the k=i iteration ofthe loop sum=F i+2. This follows immediately form the fact that F i+F i+1=F i+2.(e) Use your loop invariant to complete the inductive step of your correctness proof.Solution: To complete the inductive step of our correctness proof, we must show thatif F ib�(n)returns F n for all n<m then F ib�(m)returns m. From the previous partwe know that if F ib�(n)returns F n for all n<m, then at the end of the k=i iterationof the loop sum=F i+2. We can thus conclude that after the k=m−2iteration ofthe loop, sum=F m which completes our correctness proof.(f) What is the asymptotic running time, T�(n), of F IB�(n)? Would you recommendtenure for Professor Potemkin?Solution: We will argue that T�(n)=�(F n)and thus that Potemkin’s algorithm,F ib�does not improve upon the assymptotic performance of the simple recurrsivealgorithm, F ib. Therefore we would not recommend tenure for Professor Potemkin.One way to see that T�(n)=�(F n)is to observe that the only constant in the programis the 1 (in lines 5 and 4). That is, in order for the program to return F n lines 5 and 4must be executed a total of F n times.Another way to see that T�(n)=�(F n)is to use the substitution method with thehypothesis T�(n)√F n and the recurrence T�(n)=cn+�n−2T�(k).k=1Problem 1-3. Polynomial multiplicationOne can represent a polynomial, in a symbolic variable x, with degree-bound n as an array P[0..n] of coefficients. Consider two linear polynomials, A(x)=a1x+a0and B(x)=b1x+b0, where a1, a0, b1, and b0are numerical coefficients, which can be represented by the arrays [a0,a1]and [b0,b1], respectively. We can multiply A and B using the four coefficient multiplicationsm1=a1·b1,m2=a1·b0,m3=a0·b1,m4=a0·b0,as well as one numerical addition, to form the polynomialC(x)=m1x2+(m2+m3)x+m4,which can be represented by the array[c0,c1,c2]=[m4,m3+m2,m1].(a) Give a divide-and-conquer algorithm for multiplying two polynomials of degree-bound n,represented as coefficient arrays, based on this formula.Solution:We can use this idea to recursively multiply polynomials of degree n−1, where n isa power of 2, as follows:Let p(x)and q(x)be polynomials of degree n−1, and divide each into the upper n/2 and lower n/2terms:p(x)=a(x)x n/2+b(x),q(x)=c(x)x n/2+d(x),where a(x), b(x), c(x), and d(x)are polynomials of degree n/2−1. The polynomial product is thenp(x)q(x)=(a(x)x n/2+b(x))(c(x)x n/2+d(x))=a(x)c(x)x n+(a(x)d(x)+b(x)c(x))x n/2+b(x)d(x).The four polynomial products a(x)c(x), a(x)d(x), b(x)c(x), and b(x)d(x)are computed recursively.(b) Give and solve a recurrence for the worst-case running time of your algorithm.Solution:Since we can perform the dividing and combining of polynomials in time �(n), recursive polynomial multiplication gives us a running time ofT(n)=4T(n/2)+�(n)=�(n2).(c) Show how to multiply two linear polynomials A(x)=a1x+a0and B(x)=b1x+b0using only three coefficient multiplications.Solution:We can use the following 3 multiplications:m1=(a+b)(c+d)=ac+ad+bc+bd,m2=ac,m3=bd,so the polynomial product is(ax+b)(cx+d)=m2x2+(m1−m2−m3)x+m3.� (d) Give a divide-and-conquer algorithm for multiplying two polynomials of degree-bound nbased on your formula from part (c).Solution:The algorithm is the same as in part (a), except for the fact that we need only compute three products of polynomials of degree n/2 to get the polynomial product.(e) Give and solve a recurrence for the worst-case running time of your algorithm.Solution:Similar to part (b):T (n )=3T (n/2) + �(n )lg 3)= �(n �(n 1.585)Alternative solution Instead of breaking a polynomial p (x ) into two smaller polynomials a (x ) and b (x ) such that p (x )= a (x ) + x n/2b (x ), as we did above, we could do the following:Collect all the even powers of p (x ) and substitute y = x 2 to create the polynomial a (y ). Then collect all the odd powers of p (x ), factor out x and substitute y = x 2 to create the second polynomial b (y ). Then we can see thatp (x ) = a (y ) + x b (y )· Both a (y ) and b (y ) are polynomials of (roughly) half the original size and degree, and we can proceed with our multiplications in a way analogous to what was done above.Notice that, at each level k , we need to compute y k = y 2 (where y 0 = x ), whichk −1 takes time �(1) per level and does not affect the asymptotic running time.。
算法导论第二章答案

第二章算法入门由于时间问题有些问题没有写的很仔细,而且估计这里会存在不少不恰当之处。
另,思考题2-3 关于霍纳规则,有些部分没有完成,故没把解答写上去,我对其 c 问题有疑问,请有解答方法者提供个意见。
给出的代码目前也仅仅为解决问题,没有做优化,请见谅,等有时间了我再好好修改。
插入排序算法伪代码INSERTION-SORT(A)1 for j ←2 to length[A]2 do key ←A[j]3 Insert A[j] into the sorted sequence A[1..j-1]4 i ←j-15 while i > 0 and A[i] > key6 do A[i+1]←A[i]7 i ←i − 18 A[i+1]←keyC#对揑入排序算法的实现:public static void InsertionSort<T>(T[] Input) where T:IComparable<T>{T key;int i;for (int j = 1; j < Input.Length; j++){key = Input[j];i = j - 1;for (; i >= 0 && Input[i].CompareTo(key)>0;i-- )Input[i + 1] = Input[i];Input[i+1]=key;}}揑入算法的设计使用的是增量(incremental)方法:在排好子数组A[1..j-1]后,将元素A[ j]揑入,形成排好序的子数组A[1..j]这里需要注意的是由于大部分编程语言的数组都是从0开始算起,这个不伪代码认为的数组的数是第1个有所丌同,一般要注意有几个关键值要比伪代码的小1.如果按照大部分计算机编程语言的思路,修改为:INSERTION-SORT(A)1 for j ← 1 to length[A]2 do key ←A[j]3 i ←j-14 while i ≥ 0 and A[i] > key5 do A[i+1]←A[i]6 i ← i − 17 A[i+1]←key循环丌变式(Loop Invariant)是证明算法正确性的一个重要工具。
算法导论习题答案26章

Solution to Exercise 26.2-11
For any two vertices u and in G , we can define a flow network Gu consisting of the directed version of G with s D u, t D , and all edge capacities set to 1. (The flow network Gu has V vertices and 2 jE j edges, so that it has O.V / vertices and O.E/ edges, as required. We want all capacities to be 1 so that the number of edges of G crossing a cut equals the capacity of the cut in Gu .) Let fu denote a maximum flow in Gu . We claim that for any u 2 V , the edge connectivity k equals min fjfu jg. We’ll
2V fug
show below that this claim holds. Assuming that it holds, we can find k as follows: E DGE -C ONNECTIVITY.G/ k D1 select any vertex u 2 G: V for each vertex 2 G: V fug set up the flow network Gu as described above find the maximum flow fu on Gu k D min.k; jfu j/ return k The claim follows from the max-flow min-cut theorem and how we chose பைடு நூலகம்apacities so that the capacity of a cut is the number of edges crossing it. We prove that k D min fjfu jg, for any u 2 V by showing separately that k is at least this
算法设计与分析第二版课后习题解答
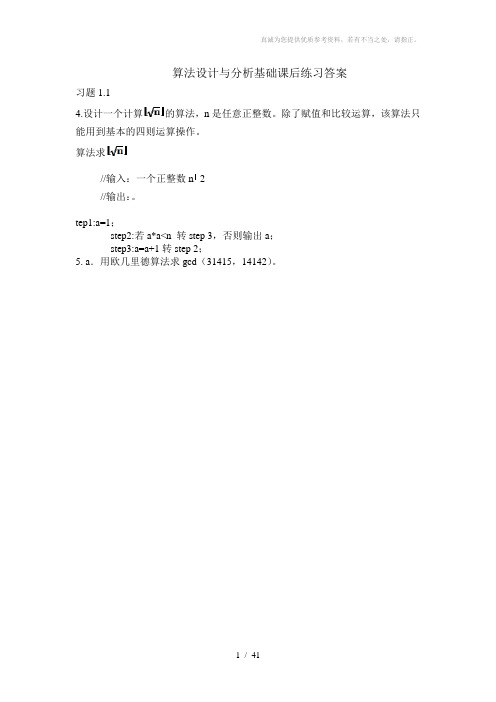
算法设计与分析基础课后练习答案习题1.14.设计一个计算的算法,n是任意正整数。
除了赋值和比较运算,该算法只能用到基本的四则运算操作。
算法求//输入:一个正整数n 2//输出:。
tep1:a=1;step2:若a*a<n 转step 3,否则输出a;step3:a=a+1转step 2;5. a.用欧几里德算法求gcd(31415,14142)。
. gcd(31415, 14142) = gcd(14142, 3131) = gcd(3131, 1618) =gcd(1618, 1513) = gcd(1513, 105) = gcd(1513, 105) = gcd(105, 43) =gcd(43, 19) = gcd(19, 5) = gcd(5, 4) = gcd(4, 1) = gcd(1, 0) = 1..有a可知计算gcd(31415,14142)欧几里德算法做了11次除法。
连续整数检测算法在14142每次迭代过程中或者做了一次除法,或者两次除法,因此这个算法做除法的次数鉴于1·14142 和2·14142之间,所以欧几里德算法比此算法快1·14142/11 ≈1300 与2·14142/11 ≈2600 倍之间。
6.证明等式gcd(m,n)=gcd(n,m mod n)对每一对正整数m,n都成立.Hint:根据除法的定义不难证明:●如果d整除u和v, 那么d一定能整除u±v;●如果d整除u,那么d也能够整除u的任何整数倍ku.对于任意一对正整数m,n,若d能整除m和n,那么d一定能整除n和r=m mod n=m-qn;显然,若d能整除n和r,也一定能整除m=r+qn和n。
数对(m,n)和(n,r)具有相同的公约数的有限非空集,其中也包括了最大公约数。
故gcd(m,n)=gcd(n,r)7.对于第一个数小于第二个数的一对数字,欧几里得算法将会如何处理?该算法在处理这种输入的过程中,上述情况最多会发生几次?Hint:对于任何形如0<=m<n的一对数字,Euclid算法在第一次叠代时交换m和n, 即gcd(m,n)=gcd(n,m)并且这种交换处理只发生一次.8.a.对于所有1≤m,n≤10的输入, Euclid算法最少要做几次除法?(1次)b. 对于所有1≤m,n≤10的输入, Euclid算法最多要做几次除法?(5次)gcd(5,8)习题1.21.(农夫过河)P—农夫W—狼G—山羊C—白菜2.(过桥问题)1,2,5,10---分别代表4个人, f—手电筒4. 对于任意实系数a,b,c, 某个算法能求方程ax^2+bx+c=0的实根,写出上述算法的伪代码(可以假设sqrt(x)是求平方根的函数)算法Quadratic(a,b,c)//求方程ax^2+bx+c=0的实根的算法//输入:实系数a,b,c//输出:实根或者无解信息f a≠0D←b*b-4*a*cIf D>0temp←2*ax1←(-b+sqrt(D))/tempx2←(-b-sqrt(D))/tempreturn x1,x2else if D=0 return –b/(2*a)else return “no real roots”lse //a=0if b≠0 return –c/belse //a=b=0if c=0 return “no real numbers”else return “no real roots”5.描述将十进制整数表达为二进制整数的标准算法a.用文字描述b.用伪代码描述解答:a.将十进制整数转换为二进制整数的算法输入:一个正整数n输出:正整数n相应的二进制数第一步:用n除以2,余数赋给Ki(i=0,1,2...),商赋给n第二步:如果n=0,则到第三步,否则重复第一步第三步:将Ki按照i从高到低的顺序输出b.伪代码算法DectoBin(n)//将十进制整数n转换为二进制整数的算法//输入:正整数n//输出:该正整数相应的二进制数,该数存放于数组Bin[1...n]中i=1while n!=0 do {in[i]=n%2;=(int)n/2;++;}while i!=0 do{rint Bin[i];--;}9.考虑下面这个算法,它求的是数组中大小相差最小的两个元素的差.(算法略)对这个算法做尽可能多的改进.算法MinDistance(A[0..n-1])//输入:数组A[0..n-1]//输出:the smallest distance d between two of its elements习题1.31.考虑这样一个排序算法,该算法对于待排序的数组中的每一个元素,计算比它小的元素个数,然后利用这个信息,将各个元素放到有序数组的相应位置上去.a.应用该算法对列表”60,35,81,98,14,47”排序b.该算法稳定吗?c.该算法在位吗? 解:a. 该算法对列表”60,35,81,98,14,47”排序的过程如下所示:b.该算法不稳定.比如对列表”2,2*”排序c.该算法不在位.额外空间for S and Count[] 4.(古老的七桥问题)第2章 习题2.17.对下列断言进行证明:(如果是错误的,请举例) a. 如果t(n )∈O(g(n),则g(n)∈Ω(t(n)) b.α>0时,Θ(αg(n))= Θ(g(n)) 解:a. 这个断言是正确的。
算法导论(第二版)课后习题解答

Θ
i=1
i
= Θ(n2 )
This holds for both the best- and worst-case running time. 2.2 − 3 Given that each element is equally likely to be the one searched for and the element searched for is present in the array, a linear search will on the average have to search through half the elements. This is because half the time the wanted element will be in the first half and half the time it will be in the second half. Both the worst-case and average-case of L INEAR -S EARCH is Θ(n). 3
Solutions for Introduction to algorithms second edition
Philip Bille
The author of this document takes absolutely no responsibility for the contents. This is merely a vague suggestion to a solution to some of the exercises posed in the book Introduction to algorithms by Cormen, Leiserson and Rivest. It is very likely that there are many errors and that the solutions are wrong. If you have found an error, have a better solution or wish to contribute in some constructive way please send a message to beetle@it.dk. It is important that you try hard to solve the exercises on your own. Use this document only as a last resort or to check if your instructor got it all wrong. Please note that the document is under construction and is updated only sporadically. Have fun with your algorithms. Best regards, Philip Bille
算法导论答案Ch3

f (n) =∞ g (n)
Ω(g (n, m)) = {f (n, m) : there exist positive constants c, n0 , and m0 such that f (n, m) ≥ cg (n, m) for all n ≥ n0 or m ≥ m0 }
Θ(g (n, m)) = {f (n, m) : there exist positive constants c1 , c2 , n0 , and m0 such that c1 g (n, m) ≤ f (n, m) ≤ c2 g (n, m) for all n ≥ n0 or m ≥ m0 } Exercise 3.2-1 Let n1 < n2 be arbitrary. From f and g being monatonic increasing, we know f (n1 ) < f (n2 ) and g (n1 ) < g (n2 ). So f (n1 ) + g (n1 ) < f (n2 ) + g (n1 ) < f (n2 ) + g (n2 ) 2
n→∞
2e n
n
≤ lim
n→∞
2e n
n
1 =0 2n
nn 1 en n −.5 n √ = lim √ e = lim O ( n ) e ≥ lim 1 n→∞ n! n→∞ n→∞ c1 n n→∞ 2πn(1 + Θ( n )) lim ≥ lim en en = lim =∞ n→∞ c1 n n→∞ c1
n a/b n if and only if if and only if n − 21 /b ≥ −a if and only if n + a ≥ (1/2) (n + a)b ≥ cnb . Therefore (n + a)b = Ω(nb ). By Theorem 3.1, (n + a)b = Θ(nb ).
算法导论中文版答案

} cout<<len<<endl; } return 0; } 15.5-1
15.5-2 15.5-3
15.5-4
16.1-1
第 16 章
16.1-2 16.1-3
16.1-4 16.2-1 16.2-2
16.2-3
16.2-4
16.2-5 16.2-6
16.2-7
25.3-6
5.3-6
6.1-1 6.1-2 6.1-3 6.1-4 6.1-5 6.1-6
第6章
6.1-7
6.2-1 见图 6-2 6.2-2
6.2-3
6.2-4
6.2-5 对以 i 为根结点的子树上每个点用循环语句实现 6.2-6
6.3-1
见图 6-3 6.3-2
6.3-3
6.4-1 见图 6-4 6.4-2 HEAPSORT 仍然正确,因为每次循环的过程中还是会运行 MAX-HEAP 的过程。 6.4-3
6.4-4
6.4-5
6.5-1 据图 6-5 6.5-2
6.5-3 6.5-4 6.5-5
6.5-6 6.5-7
6.5-8
7.1-1 见图 7-1 7.1-2
7.1-3 7.1-4 7.2-1 7.2-2
7.2-3 7.2-4 7.2-5
第七章
7.2-6 7.3-1
7.3-2
7.4-1 7.4-2
16.3-1 16.3-2
16.3-3 16.3-4
16.3-5
16.3-6 那就推广到树的结点有三个孩子结点,证明过程同引理 16.3 的证明。 16.3-7 16.3-8
第 24 章
24.1-1 同源顶点 s 的运行过程,见图 24-4 24.1-2
编译原理 (第2版) 第二版 课后习题答案2
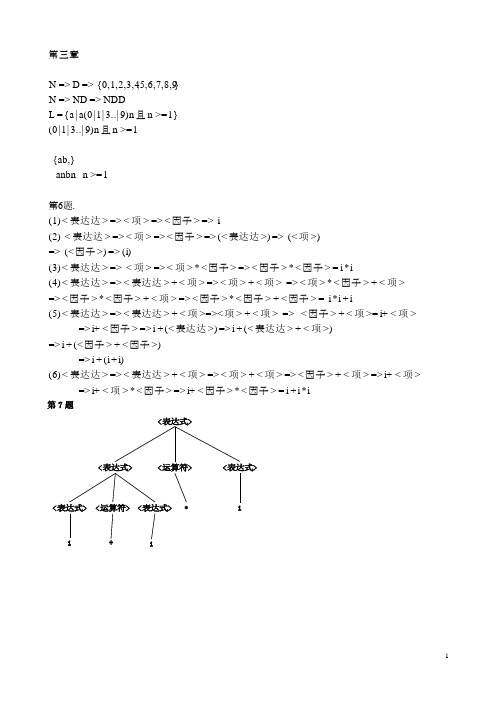
S->begin A end S->begin A end { begin }
A-> B A-> B A’ { a , if }
A-> A ; B A’-> ; B A’ { ; }
A’->ξ{ end }
B-> C
B-> D B-> D { if }
C-> a C-> a { a }
确定化,构造DFA矩阵
a
b
S
A
Q
A
A
B,Z
B,Z
Q
D
Q
Q
D,Z
D
A
B
D,Z
A
D
B
Q
D
变换为
a
b
00
1
3
1
1
2
2*
3
4
3
3
5
4
1
6
5*
1
4
6
3
4
化简:
G={(0,1,3,4,6),(2,5)}
{0,1,3,4,6}a={1,3}
{0,1,3,4,6}b={2,3,4,5,6}
所以将{0,1,3,4,6}划分为{0,4,6}{1,3}
(2)M’-> aHM’ |ξ
(3)H->bH’ | ( M )
(4)H’->(M) |ξ
7. (1)
1)A->baB
2)A->ξ
3)B->Abb
4)B->a
将1)、2)式代入3)式
1)A->baB
2)A->ξ
3)B->baBbb
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
26.1-2
26.2-1
26.2-4
14
《算法导论(第二版) 》参考答案 26.3-1 s–1–6–t s–2–8–t s–3–7–t 26.3-3
26.4-2 根据 26.22 对每个顶点执行 relabel 操作的次数最多为 2|V|-1 所以对每条边检测次数最多为 2*(2|V|-1)次 O(V) 在剩余网络中最多 2|E|条边 O(E) 所以为 O(VE)
f1[ j ] f 2 [ j 1] t2, j 1 a1, j f 2 [ j ] f1[ j 1] t1, j 1 a2, j
所以有:
f1[ j ] f 2 [ j ] f 2 [ j 1] t2, j 1 a1, j f1[ j 1] t1, j 1 a2, j
6
《算法导论(第二版) 》参考答案
最终答案:((A1A2)((A3A4)(A5A6))) 15.2-2
15.3-2 没有重叠的子问题存在 15.3-4 这题比较简单,由 P328 的(15.6)(15.7)展开两三步就可以看出来. 15.4-3 先初始化 c 数组元素为无穷大
7
《算法导论(第二版) 法, 最好是像书上画一颗递归树然后进行运算。
1
《算法导论(第二版) 》参考答案
注意题目中的要求使用递归树的方法,最好是像书上画一颗递归树然后进行运 算。 4.2.2 证略 4.2.3 由 2 i n 得 i=lgn
T (n) 2 i cn cn
i 0
由课本 P328(15.6) (15.7)式代入,可得:
min( f1[ j 1] a1, j , f 2 [ j 1] t2, j 1 a1, j ) min( f 2 [ j 1] a2, j , f1[ j 1] t1, j 1 a2, j ) min( f1[ j 1], f 2 [ j 1] t2, j 1 ) a1, j min( f 2 [ j 1], f1[ j 1] t1, j 1 ) a2, j f 2 [ j 1] t2, j 1 a1, j f1[ j 1] t1, j 1 a2, j
13.1-5 prove:
3
《算法导论(第二版) 》参考答案 13.1-6 2k-1 22k-1 13.2-3 13.3-5
13.4-3
4
《算法导论(第二版) 》参考答案
14.1-4
14.2-2
14.2-3 不可以,性能改变 时间复杂度由 O( lgn ) -> O( nlgn )
14.3-2 Note: 注意 Overlap 的定义稍有不同,需要重新定义。 算法:只要将 P314 页第三行的 改成>就行。 14.3-3 INTERVAL-SEARCH-SUBTREE(x, i) 1 while x ≠ nil[T] and i does not overlap int[x] 2 do if left[x] ≠ nil[T] and max[left[x]] ≥ low[i] 3 then x ← left[x] 4 else x ← right[x] 5 return x INTERVAL-SEARCH-MIN(T, i) 2 y←INTERVAL-SEARCH-SUBTREE(root[T], i) 先找第一个重叠区间 3 z←y 4 while y≠ nil[T] and left[y] ≠ nil[T] 在它的左子树上查找
等号在:
f 2 [ j 1] f1[ j 1] t1, j 1
f1[ j 1] f 2 [ j 1] t2, j 1
时成立,但是 t2, j 1 , t1, j 1 不为负数,矛盾。 15.2-1 参照 P336 算法,P337 例子就可以算得答案: 2010
lg n
2 lg n1 1 2cn 2 cn (n 2 ) 2 1
4.3-1 a) n2 b) n2lgn c) n3 4.3-4
2
《算法导论(第二版) 》参考答案 n2lg2n 7.1-2 (1)使用 P146 的 PARTION 函数可以得到 q=r 注意每循环一次 i 加 1,i 的初始值为 p 1 ,循环总共运行 (r 1) p 1次,最 终返回的 i 1 p 1 (r 1) p 1 1 r (2)由题目要求 q=(p+r)/2 可知,PARTITION 函数中的 i,j 变量应该在循环中同 时变化。 Partition(A, p, r) x = A[p]; i = p - 1; j = r + 1; while (TRUE) repeat j--; until A[j] <= x; repeat i++; until A[i] >= x; if (i < j) Swap(A, i, j); else return j; 7.3-2 (1)由 QuickSort 算法最坏情况分析得知:n 个元素每次都划 n-1 和 1 个,因 为是 p<r 的时候才调用,所以为Θ (n) (2)最好情况是每次都在最中间的位置分,所以递推式是: N(n)= 1+ 2*N(n/2) 不难得到:N(n) =Θ (n) 7.4-2 T(n)=2*T(n/2)+ Θ (n) 可以得到 T(n) =Θ (n lgn) 由 P46 Theorem3.1 可得:Ω (n lgn)
《算法导论(第二版) 》参考答案 2.3-3 数学归纳法证明即可,略(注:几乎所有人都对) 2.3-4 下面是最坏情况下的 T(n)
3.1-1 证明:只需找出 c1,c2,n0,使得 0<= c1* (f(n) + g(n)) <= max(f(n),g(n))<= c2*(f(n)+g(n)) 取 c1=0.5, c2=1,由于 f(n),g(n)是非负函数,所以在 n>=0 时恒成立,所以得证。 3.1-8 参照写定义即可,略(注:几乎所有人都对)
5
《算法导论(第二版) 》参考答案 do z←y 调用之前保存结果 y←INTERVAL-SEARCH-SUBTREE(y, i) 如果循环是由于y没有左子树,那我们返回y 否则我们返回z,这时意味着没有在z的左子树找到重叠区间 7 if y≠ nil[T] and i overlap int[y] 8 then return y 9 else return z 5 6 15.1-5 由 FASTEST-WAY 算法知:
化简得:
min( f1[ j 1], f 2 [ j 1] t2, j 1 ) min( f 2 [ j 1], f1[ j 1] t1, j 1 ) f 2 [ j 1] t2, j 1 f1[ j 1] t1, j 1
而:
min( f1[ j 1], f 2 [ j 1] t2, j 1 ) f 2 [ j 1] t2, j 1 min( f 2 [ j 1], f1[ j 1] t1, j 1 ) f1[ j 1] t1, j 1
15
12
《算法导论(第二版) 》参考答案 Degree[x]<degree[p[x]] 19.2-2
19.2-6
20.2-1
20.2-3 因为 Fibonacci heap 是一组无序的二项树,因而根据二项树的性质可以容易得 证,具体过程略。
13
《算法导论(第二版) 》参考答案
20.3-1
26.1-1
16.1-2
9
《算法导论(第二版) 》参考答案
16.2-4 略
16.3-1
16.3-2
前 n 个数的编码为:
10
《算法导论(第二版) 》参考答案
11...1,11...10,11...10,...,10,1
n n 1 n2
16.3-4 反证
16.4-1
16.5-1
Answers By Zhifang Wang 17.1-1 这题有歧义 a) 如果 Stack Operations 包括 Push Pop MultiPush,答案是可以保持,解释和书 上的 Push Pop MultiPop 差不多. b) 如果是 Stack Operations 包括 Push Pop MultiPush MultiPop,答案就是不可 以保持,因为 MultiPush,MultiPop 交替的话,平均就是 O(K). 17.1-2 Increment 操作每次最多可以使 k 位翻转,同样,Decrement 也是 k 位,所以不难得 到结论. 17.2-1
17.3-1
11
《算法导论(第二版) 》参考答案
17.3-4
17.4-3 假设第 i 个操作是 TABLE_DELETE, 考虑装载因子 : i =(第 i 次循环之后的表 中的 entry 数)/(第 i 次循环后的表的大小)= numi / sizei
19.1-1. If x is not a root node, then Degree[x]=Degree[sibling[x]]+1 If x is a root node, then Degree[x]<Degree[sibling[x]] 19.1-2
15.5-2
8
《算法导论(第二版) 》参考答案
15.5-3 O(n3) P360 上面的(15.17)式花费的时间是 O(n)。注意算法 OPTIMAL-BST,虽然 将 w[i,j]用 15.17 式计算,但是这个结果的 w[i,j]可以用于第 10 行中的计算,因 此总的来说,时间复杂度仍然为 O(n)* O(n)*(O(n)+O(n))=O(n3).