Linux实验报告_大三上
linux实验报告
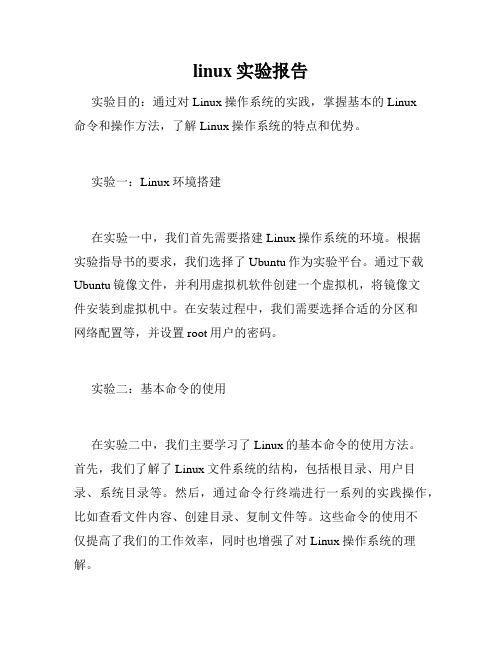
linux实验报告实验目的:通过对Linux操作系统的实践,掌握基本的Linux命令和操作方法,了解Linux操作系统的特点和优势。
实验一:Linux环境搭建在实验一中,我们首先需要搭建Linux操作系统的环境。
根据实验指导书的要求,我们选择了Ubuntu作为实验平台。
通过下载Ubuntu镜像文件,并利用虚拟机软件创建一个虚拟机,将镜像文件安装到虚拟机中。
在安装过程中,我们需要选择合适的分区和网络配置等,并设置root用户的密码。
实验二:基本命令的使用在实验二中,我们主要学习了Linux的基本命令的使用方法。
首先,我们了解了Linux文件系统的结构,包括根目录、用户目录、系统目录等。
然后,通过命令行终端进行一系列的实践操作,比如查看文件内容、创建目录、复制文件等。
这些命令的使用不仅提高了我们的工作效率,同时也增强了对Linux操作系统的理解。
实验三:软件安装与卸载实验三主要涉及到Linux的软件安装与卸载。
我们首先学习了使用APT工具进行软件包管理,通过安装命令行界面的方式安装了一些常用的软件,比如文本编辑器、终端工具等。
此外,我们还学习了如何卸载已安装的软件包,清理不需要的文件,以保持系统的整洁性。
实验四:权限管理在实验四中,我们学习了Linux的权限管理机制。
Linux操作系统采用了基于用户和组的权限模型,通过设置文件和目录的权限,实现对文件的读、写、执行的控制。
我们通过实际操作,创建了新的用户和组,并为不同的用户和组设置了不同的权限。
这样,可以有效地保护系统的文件和数据的安全性。
实验五:网络配置与服务搭建在实验五中,我们主要学习了Linux的网络配置和服务搭建。
通过设置网络接口、IP地址和网关等参数,实现了网络的正常连接。
同时,我们还学习了一些常用的网络命令,比如ping、ssh等。
此外,我们尝试搭建了一个简单的Web服务器,通过浏览器访问,可以查看服务器上的网页。
实验六:系统监控和故障恢复在实验六中,我们学习了Linux的系统监控和故障恢复方法。
linux的实验报告

linux的实验报告Linux的实验报告引言:Linux作为一种开源操作系统,具有广泛的应用领域和强大的稳定性,已经成为计算机科学领域中不可或缺的一部分。
在本次实验中,我们将对Linux进行深入探索和实践,以了解其基本原理和功能。
一、Linux的起源与发展Linux诞生于1991年,由芬兰大学生林纳斯·托瓦兹(Linus Torvalds)开发而成。
起初,Linux只是一个小型的个人项目,但随着时间的推移,越来越多的程序员加入其中,使得Linux逐渐成为一个强大的操作系统。
二、Linux的核心特性1. 开源性:Linux的源代码对所有人开放,任何人都可以对其进行修改和改进。
这使得Linux具有强大的灵活性和可定制性。
2. 多用户和多任务:Linux支持多用户同时登录,并能够同时处理多个任务,大大提高了工作效率。
3. 稳定性和安全性:Linux具有出色的稳定性和安全性,很少出现崩溃和漏洞。
这使得Linux成为服务器和网络设备的首选操作系统。
4. 强大的命令行界面:Linux提供了强大的命令行界面,使得用户可以通过命令行操作来完成各种任务,提高了操作的灵活性和效率。
三、Linux的实验应用在本次实验中,我们通过以下几个方面对Linux进行了实践应用。
1. 安装和配置Linux操作系统首先,我们需要选择适合的Linux发行版,并进行安装和配置。
在安装过程中,我们需要选择合适的分区方案、安装软件包和设置用户账户等。
通过这一步骤,我们熟悉了Linux的安装过程和基本配置。
2. 熟悉Linux的文件系统Linux的文件系统与Windows有所不同,我们需要了解Linux的文件结构和文件路径。
在实验中,我们通过命令行界面进入不同的目录,创建和删除文件,以及修改文件权限等操作,深入了解了Linux的文件系统。
3. 学习Linux的基本命令Linux的命令行界面是其最大的特点之一,我们需要掌握一些基本的命令来完成各种任务。
linux实验报告
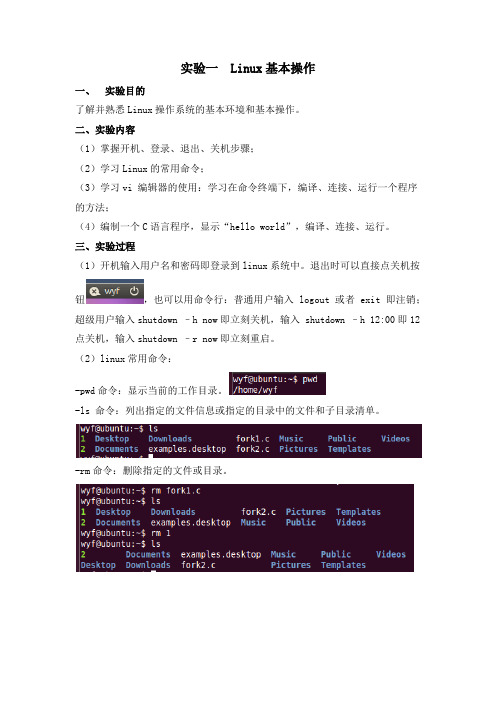
实验一 Linux基本操作一、实验目的了解并熟悉Linux操作系统的基本环境和基本操作。
二、实验内容(1)掌握开机、登录、退出、关机步骤;(2)学习Linux的常用命令;(3)学习vi 编辑器的使用:学习在命令终端下,编译、连接、运行一个程序的方法;(4)编制一个C语言程序,显示“hello world”,编译、连接、运行。
三、实验过程(1)开机输入用户名和密码即登录到linux系统中。
退出时可以直接点关机按钮,也可以用命令行:普通用户输入 logout 或者 exit 即注销;超级用户输入shutdown –h now即立刻关机,输入 shutdown –h 12:00即12点关机,输入shutdown –r now即立刻重启。
(2)linux常用命令:-pwd命令:显示当前的工作目录。
-ls 命令:列出指定的文件信息或指定的目录中的文件和子目录清单。
-rm命令:删除指定的文件或目录。
-cp命令:复制文件或目录。
(3)编译:对于c 文件:cc -o name xxx.c;对于c++ 文件:c++ -o name xxx.cpp (其中name是可执行文件名,xxx是编写时候的文件名)。
运行:./name四、调试程序过程命名程序:编写程序:编译程序:运行程序:五、总结1、调试过程中遇到的主要问题及解决过程命名的时候如果不加扩展名 .c或者 .cpp 执行的时候会提示错误,找不到文件。
2、体会和收获通过这次实验我初步认识了虚拟机环境下的linux系统的界面和一些基本的命令行操作;也学会了用vi编辑器编写c和c++文件;同时知道了一些常用的命令,比如查看文件、查看当前目录、复制、删除等等,觉得很有意思,引起了我学习linux的兴趣。
六、附录:源程序代码(另附)。
linux操作系统实验报告
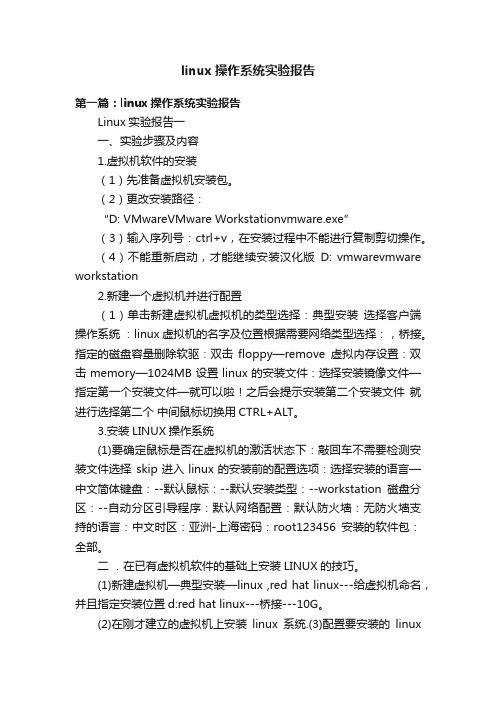
linux操作系统实验报告第一篇:linux操作系统实验报告Linux实验报告一一、实验步骤及内容1.虚拟机软件的安装(1)先准备虚拟机安装包。
(2)更改安装路径:“D: VMwareVMware Workstationvmware.exe”(3)输入序列号:ctrl+v,在安装过程中不能进行复制剪切操作。
(4)不能重新启动,才能继续安装汉化版D: vmwarevmware workstation2.新建一个虚拟机并进行配置(1)单击新建虚拟机虚拟机的类型选择:典型安装选择客户端操作系统:linux虚拟机的名字及位置根据需要网络类型选择:,桥接。
指定的磁盘容量删除软驱:双击floppy—remove虚拟内存设置:双击memory—1024MB设置linux的安装文件:选择安装镜像文件—指定第一个安装文件—就可以啦!之后会提示安装第二个安装文件就进行选择第二个中间鼠标切换用CTRL+ALT。
3.安装LINUX操作系统(1)要确定鼠标是否在虚拟机的激活状态下:敲回车不需要检测安装文件选择skip进入linux的安装前的配置选项:选择安装的语言—中文简体键盘:--默认鼠标:--默认安装类型:--workstation磁盘分区:--自动分区引导程序:默认网络配置:默认防火墙:无防火墙支持的语言:中文时区:亚洲-上海密码:root123456安装的软件包:全部。
二.在已有虚拟机软件的基础上安装LINUX的技巧。
(1)新建虚拟机—典型安装—linux ,red hat linux---给虚拟机命名,并且指定安装位置d:red hat linux---桥接---10G。
(2)在刚才建立的虚拟机上安装linux系统.(3)配置要安装的linux系统的安装文件iso镜像文件(4)选择配置好linux镜像文件刚才建立的虚拟机,启动虚拟机,开始安装。
三.实验总结充分了解linux系统的安装,学会新建虚拟机,在虚拟机上安装linux系统.和一些简单的技巧并对此门课程有了一定的兴趣。
Linux操作系统实验实验报告
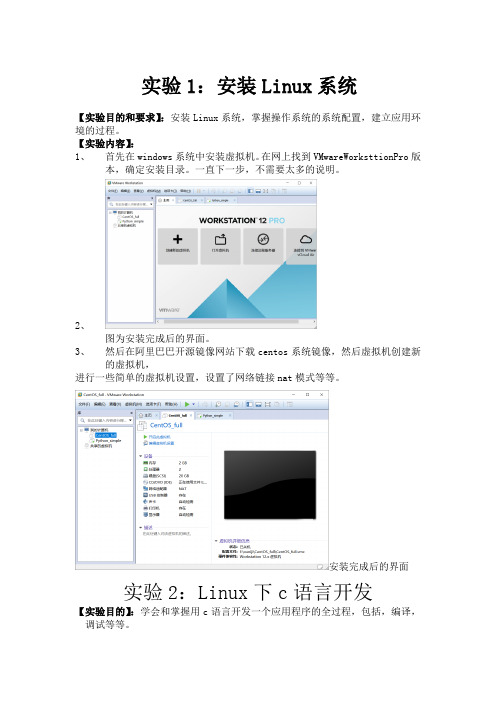
实验1:安装Linux系统【实验目的和要求】:安装Linux系统,掌握操作系统的系统配置,建立应用环境的过程。
【实验内容】:1、首先在windows系统中安装虚拟机。
在网上找到VMwareWorksttionPro版本,确定安装目录。
一直下一步,不需要太多的说明。
2、图为安装完成后的界面。
3、然后在阿里巴巴开源镜像网站下载centos系统镜像,然后虚拟机创建新的虚拟机,进行一些简单的虚拟机设置,设置了网络链接nat模式等等。
安装完成后的界面实验2:Linux下c语言开发【实验目的】:学会和掌握用c语言开发一个应用程序的全过程,包括,编译,调试等等。
【实验步骤】:首先在系统中查看是否已经安装有gcc,输入查看命令发现没有,于是需要安装gcc。
在centos系统中可以使用比较简便的yum命令。
在之前已经配置好了yum源。
直接输入yuminstallgcc。
回车自动安装程序和需要的依赖包。
因为虚拟机中和电脑很多地方切换使用不方便,所以安装了xshell软件。
图为xshell中的截图。
安装完毕。
然后使用vi或者vim编写hello.c运行,在屏幕上打印出hello,world。
实验3:进程创建【实验目的和要求】1.了解进程的概念及意义;2.了解子进程和父进程3.掌握创建进程的方法。
【实验内容】1.子进程和父进程的创建;2.编写附件中的程序实例【实验步骤】一1、打开终端,输入命令gedit1_fork.c,在1_fork.c文件中输入1_fork.bmp中的代码;2、输入命令gcc1_fork.c-o1_fork,回车后显示无错误;3、输入命令:./1_fork运行程序。
二、1、打开终端,输入命令gedit2_vfork.c,在2_vfork.c文件中输入2_vfork.bmp中的代码;2、输入命令gcc2_vfork.c-o2_vfork,回车后显示无错误:3、输入命令:./2_vfork运行程序。
从上面可以看到两次的运行结果不一样。
linux实验报告

linux实验报告Linux 实验报告一、实验目的本次 Linux 实验的主要目的是熟悉 Linux 操作系统的基本命令和操作,了解其文件系统、进程管理、用户权限等核心概念,并通过实际操作加深对这些知识的理解和应用能力。
二、实验环境本次实验使用的是虚拟机软件 VirtualBox 安装的 Ubuntu 2004 LTS 操作系统。
三、实验内容及步骤(一)用户和权限管理1、使用`sudo adduser` 命令创建新用户`user1` 和`user2`。
2、使用`sudo passwd user1` 和`sudo passwd user2` 为新用户设置密码。
3、使用`sudo usermod aG sudo user1` 将`user1` 添加到`sudo` 组,使其具有管理员权限。
4、以`user1` 身份登录系统,创建一个文件`file1txt`,尝试修改其权限为 777,观察权限变化。
(二)文件和目录操作1、使用`mkdir` 命令创建目录`directory1` 和`directory2`。
2、使用`touch` 命令在当前目录下创建文件`file2txt` 和`file3txt`。
3、使用`cp` 命令将`file2txt` 复制到`directory1` 目录下。
4、使用`mv` 命令将`file3txt` 移动到`directory2` 目录下。
5、使用`rm` 命令删除`file2txt` 和`directory2` 目录及其下的所有文件。
(三)进程管理1、使用`ps` 命令查看当前系统中的进程信息。
2、使用`top` 命令实时监控系统的进程状态。
3、使用`kill` 命令结束指定进程(例如,通过进程 ID 结束一个占用大量资源的进程)。
(四)文件系统管理1、使用`df` 命令查看磁盘空间使用情况。
2、使用`du` 命令查看目录或文件的磁盘使用量。
3、使用`mount` 命令挂载一个新的磁盘分区(假设已经在虚拟机中添加了新的磁盘分区)。
linux实验系统实验报告

linux实验系统实验报告Linux实验系统实验报告一、引言Linux实验系统是一个基于Linux操作系统的实验平台,旨在提供一个实践学习的环境,帮助学生深入了解Linux操作系统的原理和应用。
本实验报告旨在总结和分析我在使用Linux实验系统进行实验时的经验和收获。
二、实验环境1. 硬件环境:我使用的是一台配备Intel Core i5处理器和8GB内存的个人电脑。
2. 软件环境:我下载并安装了Linux实验系统的最新版本,该版本基于Ubuntu操作系统,并预装了一系列常用的开发工具和软件包。
三、实验内容1. 实验一:Linux基础命令的使用在这个实验中,我通过终端窗口使用了一些常用的Linux命令,如ls、cd、mkdir、rm等。
通过实际操作,我熟悉了Linux文件系统的结构和基本操作,掌握了如何在Linux中创建、删除和移动文件夹,以及如何查看文件和文件夹的属性。
2. 实验二:Shell脚本编程这个实验要求我们使用Shell脚本编写一个简单的程序,实现对指定文件夹中所有文件进行备份的功能。
通过这个实验,我学会了如何使用Shell编程语言,掌握了一些基本的语法和命令,比如if语句、for循环和cp命令。
我还学会了如何将Shell脚本保存为可执行文件,并在终端中运行。
3. 实验三:网络配置与管理这个实验主要涉及Linux系统的网络配置和管理。
我学会了如何配置网络接口,包括设置IP地址、子网掩码和网关。
我还学会了如何使用ping命令测试网络连接,以及如何使用ifconfig命令查看和管理网络接口的状态。
通过这个实验,我对Linux系统的网络配置有了更深入的了解。
四、实验收获通过使用Linux实验系统进行实验,我获得了以下收获:1. 对Linux操作系统有了更深入的了解:通过实际操作,我对Linux操作系统的基本原理和文件系统有了更深入的了解。
我学会了如何在Linux中进行文件和文件夹的管理,以及如何使用命令行界面进行各种操作。
linux实验报告总结-共10篇
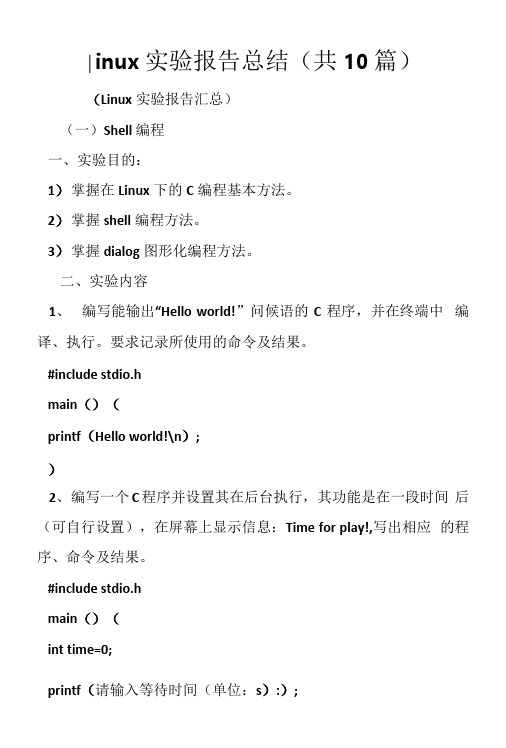
∣inux实验报告总结(共10篇)(Linux实验报告汇总)(一)Shell 编程一、实验目的:1)掌握在Linux下的C编程基本方法。
2)掌握shell编程方法。
3)掌握dialog图形化编程方法。
二、实验内容1、编写能输出“Hello world!”问候语的C程序,并在终端中编译、执行。
要求记录所使用的命令及结果。
#include stdio.hmain()(printf(Hello world!\n);)2、编写一个C程序并设置其在后台执行,其功能是在一段时间后(可自行设置),在屏幕上显示信息:Time for play!,写出相应的程序、命令及结果。
#include stdio.hmain()(int time=0;printf(请输入等待时间(单位:s):);scanf(%d/&time);sleep(time);printf(Time for play!\n);)3、编写C程序,求1到100之间整数的阶乘和,并对程序进行优化。
写出程序、命令和结果。
#include stdio.hmain()int i;double s = l,sum = 0;for( i= l;i= 100;i++)sum+=s*=i;printf( 1到100之间整数的阶乘和:%f\n,sum);printf( 1到100之间整数的阶乘和:%e\n,sum);}4、编写C程序,根据键盘输入的半径求圆面积,要求在命令行周率(P∣=3∙14,PI=3∙14159,PI=3.14159626 等)进行编使用不同的译,写出程序、命令和结果。
#include stdio.hint main()double r = 0.0 , Area = 0.0;printf(请输入半径:);scanf(%lf, &r);Area = PI * r * r;printf(圆面积:%f∖n, Area);)5、编写shell程序sh.l,完成向用户输出“你好!”的问候语。
Linux实验报告,,
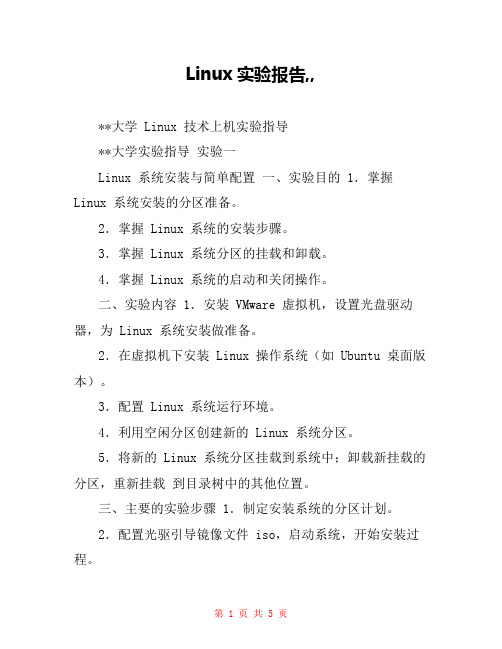
Linux实验报告,,**大学 Linux 技术上机实验指导**大学实验指导实验一Linux 系统安装与简单配置一、实验目的 1.掌握Linux 系统安装的分区准备。
2.掌握 Linux 系统的安装步骤。
3.掌握 Linux 系统分区的挂载和卸载。
4.掌握 Linux 系统的启动和关闭操作。
二、实验内容 1.安装 VMware 虚拟机,设置光盘驱动器,为 Linux 系统安装做准备。
2.在虚拟机下安装 Linux 操作系统(如 Ubuntu 桌面版本)。
3.配置 Linux 系统运行环境。
4.利用空闲分区创建新的 Linux 系统分区。
5.将新的 Linux 系统分区挂载到系统中;卸载新挂载的分区,重新挂载到目录树中的其他位置。
三、主要的实验步骤 1.制定安装系统的分区计划。
2.配置光驱引导镜像文件 iso,启动系统,开始安装过程。
3.根据安装计划,对磁盘空间进行分区设置。
4.根据系统安装指导,完成 Linux 系统的安装过程。
5.安装成功后,退出系统,重新启动虚拟机,登陆Linux 操作系统。
6.对 Linux 系统进行配置,如网络设备等。
7.利用磁盘使用工具和 mount,将新创建的 Linux 系统分区挂载到系统中。
将新挂载的分区卸载,并重新挂载到目录树的其他位置。
1为作者的博览群书赞一个。
切合实际,有生活。
嵌入式Linux学习心得1、Linux命令ls:查看目录-l以列表方式查看;ls –l 与ll的功能一样 pwd: 查看当前的目录cd:改变当前操作目录cd /直接跳到根目录 cd ..回到上一级目录 cat: 打印显示当前文件的内容信息mkdir:创建目录fdisk: 查看硬盘分区信息,-l以列表方式查看->代表是链接文件,类似window下的快捷方式。
cp: 复制命令,例子cp 文件名 /home/dir/mv: 移动或改名,如mv sonf.confsonf.txt (改名)移动:mv sonf.conf / rm:删除命令,如rm –f test.c ; 如删除目录rm –fr dman:查看某个命令的帮助,man 命令2、各系统目录的功能drw—r—w-- :d代表是目录,drw代表当前用户的权限,r代表组用户的权限,w代表其它用户的权限。
linux系统使用实验报告

linux系统使用实验报告Linux 系统使用实验报告一、实验目的本次实验旨在深入了解和熟悉 Linux 操作系统的基本操作、命令行使用以及系统配置,掌握常见的文件管理、进程管理、用户权限管理等功能,提高对 Linux 系统的实际应用能力。
二、实验环境1、操作系统:Ubuntu 2004 LTS2、实验工具:终端模拟器(Terminal)三、实验内容与步骤(一)系统登录与基本命令1、启动计算机,选择 Ubuntu 操作系统,输入用户名和密码登录系统。
2、打开终端模拟器,熟悉常用的基本命令,如`ls` (列出当前目录下的文件和文件夹)、`cd` (切换目录)、`mkdir` (创建新目录)、`rmdir` (删除空目录)等。
(二)文件管理1、在用户主目录下创建一个名为`experiment` 的文件夹,使用`mkdir experiment` 命令。
2、进入该文件夹,使用`cd experiment` 命令。
3、在`experiment` 文件夹中创建一个文本文件`filetxt` ,使用`touch filetxt` 命令。
4、使用`vi` 或`nano` 编辑器打开`filetxt` 文件,输入一些文本内容,并保存退出。
5、查看文件的内容,使用`cat filetxt` 命令。
6、复制文件,使用`cp filetxt file_copytxt` 命令。
7、移动文件,使用`mv filetxt/`命令将文件移动到上级目录。
8、删除文件,使用`rm file_copytxt` 命令。
(三)进程管理1、运行一个后台进程,例如`ping &`,然后使用`jobs` 命令查看后台进程。
2、将后台进程切换到前台,使用`fg %1` (其中%1 为后台进程的编号)命令。
3、终止进程,使用`Ctrl + C` 组合键终止正在运行的进程。
4、查看系统当前运行的进程,使用`ps aux` 命令。
(四)用户权限管理1、创建一个新用户,使用`sudo adduser username` 命令,其中`username` 为新用户的用户名。
Linux实验报告

Linux实验报告Linux 实验报告实验[ 一]:Linux操作系统的基本操作学生:学号:班级:实验时间:报告时间:系别:学院:实验一:Linux操作系统的基本操作一、实验目的:1、熟悉Linux的桌面环境;2、了解Linux所安装的软件包3、了解Linux的文件目录结构;4、熟悉Linux的终端方式或文本方式下文件目录操作命令。
5、了解Linux的命令及使用格式。
6、熟悉Linux系统的文件和目录二、实验要求1、Linux的桌面环境GNOME、KDE;2、Linux的终端方式和文本方式下的命令操作。
3、练习并掌握常用的Linux操作命令,如ls、cat、ps、who、echo、cd、more、cp、rm、ps等;4、学习使用Linux的在线求助系统,如man和help命令等。
三、实验内容1、系统的使用进入终端、文本模式,分别以用户身份登录,在窗口模式中打开终端(Termainal)仿真程序:点击:系统工具/终端(SystemTools/Terminal);在窗口模式中按:Ctrl+Alt+F1,进入文本模式,Ctrl+Alt+F7,反回GUI模式。
第一次进入文本模式时需登录(login),输入用户名及口令。
进入后提示:[root@ylinux root]# _提示符#:超级用户,提示符$:普通用户。
2、命令的使用(1)显示目录文件ls执行格式:ls [-atFlgR] [name] (name可为文件或目录名称)例:ls 显示出当前目录下的文件ls -a 显示出包含隐藏文件的所有文件ls -t 按照文件最后修改时间显示文件ls -F 显示出当前目录下的文件及其类型ls -l 显示目录下所有文件的许可权、拥有者、文件大小、修改时间及名称ls -lg 同上ls -R 显示出该目录及其子目录下的文件注:ls与其它命令搭配使用可以生出很多技巧(最简单的如"ls -l | more"),更多用法请输入ls --help查看,其它命令的更多用法请输入命令名--help 查看.(2)建新目录mkdir执行格式:mkdir directory-name例:mkdir dir1(新建一名为dir1的目录)(3)删除目录rmdir执行格式:rmdir directory-name 或rm directory-name例:rmdir dir1 删除目录dir1,但它必须是空目录,否则无法删除rm -r dir1 删除目录dir1及其下所有文件及子目录rm -rf dir1 不管是否空目录,统统删除,而且不给出提示,使用时要小心(4)改变工作目录位置cd执行格式:cd [name]例:cd 改变目录位置至用户login时的working directorycd dir1 改变目录位置,至dir1目录cd ~user 改变目录位置,至用户的working directorycd .. 改变目录位置,至当前目录的上层目录cd ../user 改变目录位置,至上一级目录下的user目录cd /dir-name1/dir-name2 改变目录位置,至绝对路径(Full path)cd - 回到进入当前目录前的上一个目录(5)显示当前所在目录pwd执行格式:pwd(6)查看目录大小du执行格式:du [-s] directory例:du dir1 显示目录dir1及其子目录容量(以kb为单位)du -s dir1 显示目录dir1的总容量(7)显示环境变量echo $HOME 显示家目录echo $PATH 显示可执行文件搜索路径env 显示所有环境变量(可能很多,最好用"env | more","env | grep PATH"等)(8)修改环境变量,在bash下用export,如:export PATH=$PATH:/usr/local/bin想知道export的具体用法,可以用shell的help命令:help export2、文件操作(1)查看文件的内容cat执行格式:cat filename或more filename 或cat filename|more 例:cat file1 以连续显示方式,查看文件file1的内容more file1或cat file1|more 以分页方式查看文件的内容(2)删除文件rm执行格式:rm filename例:rm file?rm f*(3)复制文件cp执行格式:cp [-r] source destination例:cp file1 file2 将file1复制成file2cp file1 dir1 将file1复制到目录dir1cp /tmp/file1 将file1复制到当前目录cp /tmp/file1 file2 将file1 复制到当前目录名为file2cp –r dir1 dir2 (recursive copy)复制整个目录。
Linux实验报告
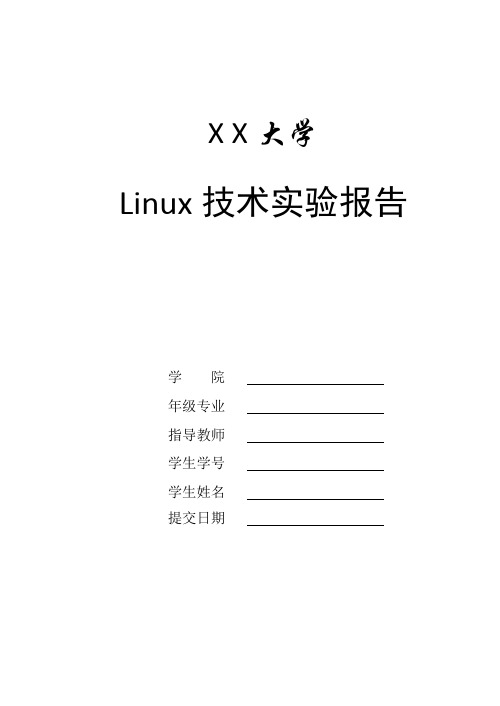
X X大学Linux技术实验报告
学院
年级专业
指导教师
学生学号
学生姓名
提交日期
实验一Linux常用命令使用
一、实验目的
1.掌握Linux一般命令格式。
2.掌握有关文件和目录操作的常用命令。
3.熟练使用man命令。
二、实验内容
1.熟悉ls、cd、date、pwd、cal、who、echo、clear、passwd等常用命令及常用选项。
Ls命令:
Cd命令:
Date命令:Cal命令:
Who命令:
echo命令:
Passwd命令:
2.在用户主目录下对文件进行操作:创建文件、复制文件、显示文件内容、查找文件中指定内容、移动文件、删除文件、修改文件时间属性等。
显示文件:
复制文件:
删除文件:
3.对目录进行管理:创建和删除子目录、改变和显示工作目录、列出和更改文件权限等。
创建目录:
删除目录:
改变工作目录:
更改文件权限:
4.利用man命令显示date、echo等命令的手册页。
Man命令:
5.显示系统中的进程信息。
实验三vi编辑器的使用
一、实验目的
1.学习使用vi编辑器建立、编辑、显示及加工处理文本文件。
二、实验内容
1.进入和退出vi编辑器,并掌握保存文件的命令。
进入vi命令:
退出并保存文件:
2.利用文本插入方式建立一个文件。
3.在新建的文本文件上移动光标位置。
4.对该文件执行删除、复原、修改、替换等操作。
燕山大学实验报告
燕山大学实验报告。
linux操作系统实验报告书
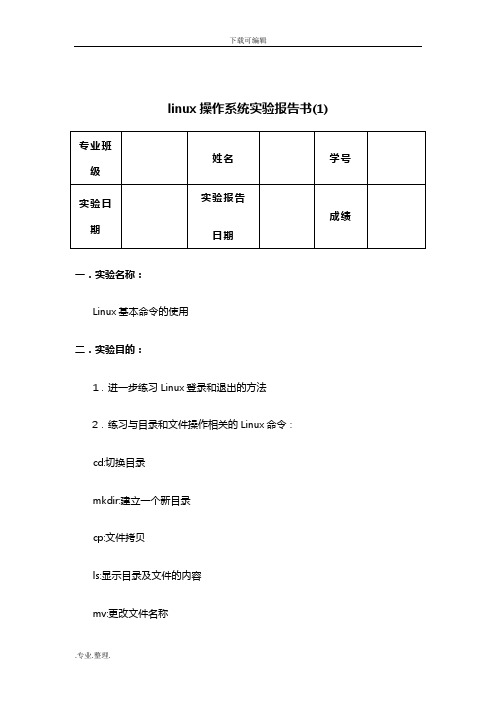
linux操作系统实验报告书(1)一.实验名称:Linux基本命令的使用二.实验目的:1.进一步练习Linux登录和退出的方法2.练习与目录和文件操作相关的Linux命令:cd:切换目录mkdir:建立一个新目录cp:文件拷贝ls:显示目录及文件的内容mv:更改文件名称cat、more、less:浏览文件内容chmod:更改文件或目录的访问权限rm:删除文件rmdir:删除目录三.实验内容:1.登录进入Linux 系统。
2.将工作目录切换到根目录,用ls命令查看根目录下的内容,尝试使用-a,-l,-F,-A,-lF等不同选项并比较不同之处。
3.在家目录下建立一个名为test的新目录,将工作目录切换到test下,然后将/tmp下的文件lesson.log拷贝到该目录下,并将lesson.log用mv命令改名为:TestRenName.txt。
4.用cat命令浏览文件TestRenName.txt的内容,用more命令进行浏览翻页操作。
再用less命令浏览文件TestRenName.txt的内容。
5.用ls命令查看test下的文件的权限,并更改文件TestRenName.txt的权限为:只允许自己读写,不允许其他用户访问。
用ls命令查看更改后的结果后再将文件TestRenName.txt的权限更改为系统默认的权限:-rw-r--r--。
6.用rm命令删除test目录下的所有文件,再用rmdir命令删除test目录。
7.用logout命令退出系统。
四.结果分析:五.实验心得*********************************************************************linux操作系统实验报告书(2)一.实验名称:Linux基本命令的使用二.实验目的:1. 掌握监视系统的几个Linux基本命令:PS--查看系统的进程tty--查看当前使用的终端df--查看系统的文件系统的空间使用情况du--查看具体某个文件和目录的磁盘空间的占用情况free--查看使用的内存资源的情况2. 掌握Linux下的联机帮助手册的使用3. 掌握Linux命令的简要帮助的获取方法4. 掌握一些其他常用的Linux命令:cal--显示日历date--显示系统的日期和时间clear--清屏find--查找文件uname--查看系统信息who--查看其他登录的用户which--查看命令的全路径tar--Linux下的压缩与解压缩命令三.实验内容:1.使用ps查看系统的进程运行情况,使用不同的命令选项并比较显示结果的差别;查看当前系统已安装的文件系统的空间使用情况;查看用户的家目录占用了多少空间;查看使用的内存资源的情况.2.查看ls命令的详细使用方法,查看ps命令的详细使用方法.3.获取ls命令的简要帮助信息,获取ps命令的简要帮助信息.4.用cal命令显示日历,用date命令显示系统的日期和时间,用clear清除屏幕,用find命令在系统中查找文件ch1.doc,用uname命令查看系统的信息,用who 命令查看其他登录的用户,用which命令查看一些命令的全路径,用tar命令来压缩test目录下的所有后缀为doc的文件到文件doc.tar.gz中,将doc.tar.gz 复制到用户的家目录并展开压缩文件.四.结果分析:1. 要查看系统的进程运行情况可使用ps命令:[stu@cs-linux stu]$ psPID TTY TIME CMD4442 pts/0 00:00:00 bash11683 pts/0 00:00:00 ps第一列中的PID表示进程编号,第二列中的TTY表示提交该进程的终端号,第三列中的TIME表示该进程已运行的时间,第四列中的CMD表示该进程所对应的命令.如果要显示进程更详细的信息,可以使用参数ps u:[stu@cs-linux stu]$ ps uUSER PID %CPU %MEM VSZ RSSTTY STAT START TIME COMMAND501 4442 0.0 0.3 5560 784 pts/0 S 09:45 0:00 bash501 11684 0.0 0.2 2592 644 pts/0 R 10:16 0:00 ps -uUSER表示进程拥有者,%CPU表示CPU时间的占用比例,%MEM表示内存的占用比例,VSZ表示进程的大小,RSS表示常驻内存部分大小,START表示进程的启动时间.如果要用长格式显示系统进程的信息,可以使用参数ps l:[stu@cs-linux stu]$ ps lFS UID PID PPID C PRI NI ADDR SZ WCHAN TTY TIME C MD4 S 501 4174 4173 0 75 0 - 1389 wait4 pts/0 00:00:00 bash0 R 501 4201 4174 0 80 0 - 776 - pts/0 00:00:00 ps要查看当前系统已安装的文件系统的空间使用情况,使用命令df:[stu@cs-linux stu]$ df文件系统1K-块已用可用已用% 挂载点/dev/hda7 9574520 2833764 6254392 32% /none 111472 0 111472 0% /dev/shm/dev/hda5 30701232 13538096 17163136 45% /mnt/hdd要查看用户的家目录占用了多少空间,先将工作目录切换到家目录,再使用命令du:[stu@cs-linux stu]$ du12 ./.kde/Autostart16 ./.kde8 ./.xemacs4 ./test64 .以上结果表示家目录公占用64KB的空间.要查看使用的内存资源的情况,应使用命令free:[stu@cs-linux stu]$ freetotal used free shared buffers cached Mem: 222948 216688 6260 0 17832 92792 -/+ buffers/cache: 106064 116884Swap: 514040 0 514040以上结果表示系统内存及交换空间使用情况.2. 查看ls命令的详细使用方法,使用命令man ls:[stu@cs-linux stu]$ man ls(.......显示内容省略)查看ps命令的详细使用方法,使用命令man ps:[stu@cs-linux stu]$ man ps(.......显示内容省略)3. 获取ls命令的简要帮助信息,可使用命令ls --help:[stu@cs-linux stu]$ ls --help(.......显示内容省略)获取ps命令的简要帮助信息,可使用命令ps --help:[stu@cs-linux stu]$ ps --help(.......显示内容省略)4. 要显示日历可使用命令cal:[stu@cs-linux stu]$ cal六月2004日一二三四五六1 2 3 4 56 7 8 9 10 11 1213 14 15 16 17 18 1920 21 22 23 24 25 2627 28 29 30以上用命令cal不加任何参数时显示当月日历,如果要指定查看某年某月的日历,可以加[月][年]参数,如要查看2004年8月:[stu@cs-linux stu]$ cal 08 2004八月2004日一二三四五六1 2 3 4 5 6 78 9 10 11 12 13 1415 16 17 18 19 20 2122 23 24 25 26 27 2829 30 31要显示系统的日期和时间,可使用命令date:[stu@cs-linux stu]$ date五6月18 11:23:23 CST 2004表示当前时间为2004年6月18日星期五11:23:23.用clear命令清除屏幕,之后将把已前显示的结果清除:[stu@cs-linux stu]$ clear用find命令在系统中查找文件ch1.doc,应首先退出到根目录下,在使用命令find ch1.doc:[stu@cs-linux /]$ cd /[stu@cs-linux /]$ find / -name "ch1.doc"find: /home/wb: 权限不够/home/stu/test/ch1.doc结果表示找到的文件在/home/stu/test/目录下.用uname命令查看系统的信息:[stu@cs-linux /]$ unameLinux用who命令查看其他登录的用户:[stu@cs-linux /]$ whostu tty1 Jun 18 14:22root pts/0 Jun 18 14:23 (:0.0)用which命令查看一些命令的全路径,如查看命令ls和ps的全路径:[stu@cs-linux /]$ which lsalias ls='ls --color=tty'/bin/ls[stu@cs-linux /]$ which ps/bin/ps用tar命令来压缩test目录下的所有后缀为doc的文件到文件doc.tar.gz中,如下:[stu@cs-linux test]$ tar cvf doc.tar.gz *.docch1.docch2.doc[stu@cs-linux test]$ lsch1.doc ch2.doc doc.tar.gz sesson.txt使用命令加参数cvf来打包所有后缀为doc的文件,参数c表示建立新文档,参数v表示在处理过程中显示相关信息,参数f表示以文件方式打包.现test目录下共有doc文件2个,所以显示ch1.doc,ch2.doc.打包后用ls命令查看,发现多了一个doc.tar.gz文件,表明操作成功.将doc.tar.gz复制到用户的家目录并展开压缩文件:[stu@cs-linux test]$ cd ..[stu@cs-linux stu]$ cp test/doc.tar.gz doc.tar.gz[stu@cs-linux stu]$ tar xvf doc.tar.gz *.docch1.docch2.doc[stu@cs-linux stu]$ lsch1.doc ch2.doc doc.tar.gz test第三行的参数x表示解包文件.五.实验心得这一章主要学习了Linux下的一些关于监视系统的命令以及一些常用辅助命令,对于一个Linux的系统管理员,掌握这些命令的使用是非常重要的,因为这些命令不仅可以让系统管理员了解到系统的运行情况,还可以了解到磁盘及内存的占用情况,以便系统管理员根据情况作出相应调整。
linux实习报告

linux实习报告Linux 实习报告一、实习背景本次实习是在某互联网科技公司的系统运维部门进行的,实习时间为两个月。
作为一名计算机科学专业学生,我选择了 Linux 实习,旨在深入了解和熟悉 Linux 系统的运维和管理。
二、实习目标1. 熟悉 Linux 操作系统的基本概念和原理;2. 掌握 Linux 系统的安装、配置和管理技巧;3. 学习常用的 Linux 命令及其应用;4. 理解 Linux 网络配置和安全管理。
三、实习内容1. Linux 系统的安装和配置在实习的第一周,我首先进行了 Linux 操作系统的安装。
选择了最新版本的 CentOS,并按照给定的步骤完成了安装过程。
之后,我学习了如何对系统进行基本的配置,包括网络设置、用户管理等。
2. Linux 系统的命令操作通过实习的学习和积累,我熟练掌握了 Linux 下的常用命令,比如文件和目录操作、文件权限管理、文本编辑等。
特别是在文件操作和权限管理方面,我深入了解了 Linux 的文件系统和权限机制,并学会了如何正确地操作和管理文件。
3. Linux 系统的网络配置在实习的第三周,我开始学习 Linux 网络配置,包括 IP 地址配置、网关设置、DNS 解析等。
通过实际操作和网络参数的调试,我逐渐掌握了网络配置的技巧和原理。
此外,还学习了如何使用 Linux 服务器搭建 Web 服务和 FTP 服务器。
4. Linux 系统的安全管理在实习的最后两周,我重点学习了 Linux 系统的安全管理。
学习了如何防范常见的网络攻击,如 DoS/DDoS 攻击、SQL 注入等。
通过实际操作和防护措施的设置,我掌握了一些基本的网络安全技巧,并加深了对系统安全的认识。
四、实习总结通过两个月的实习,我对 Linux 操作系统有了更深入的了解。
我不仅学到了很多知识,还锻炼了解决问题和快速学习的能力。
实习期间,我与同事们一起合作完成了一些实际的项目,这让我更好地理解了Linux 在实际工作中的应用。
Linux 实验报告
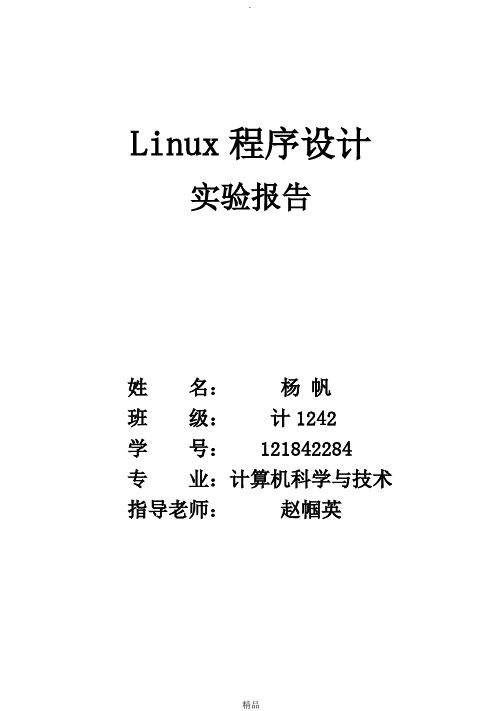
Linux程序设计实验报告姓名:杨帆班级:计1242学号: 121842284专业:计算机科学与技术指导老师:赵帼英实验一 Linux系统安装与启动(选做实验)一、实验目的(通过实践及查阅资料完成如下实验)1.掌握虚拟机的配置过程。
2.掌握Linux系统的安装过程和简单配置方法。
3.掌握与Linux相关的多操作系统的安装方法。
4.掌握Linux系统的启动、关闭步骤。
5.了解Linux文件系统和目录结构。
二、实验内容1.安装虚拟机软件(如VMware、Virtualbox)。
2.在虚拟机软件中安装Linux操作系统(如Fedora、Ubuntu、redhat等)。
3.配置Linux系统运行环境。
4.正确地启动、关闭系统。
三、实验步骤1 .安装虚拟机软件(VMware Workstation 7.0) 。
2.在虚拟机软件中新建Linux虚拟机2.1设置内存大小(建议大于256MB)2.2设置虚拟硬盘大小(建议大于8GB)2.3设置虚拟光驱(虚拟方式,镜像文件)2.4设置网络连接模式(建议采用桥接方式)2.5设置共享目录(建议英文目录名)1.在Linux虚拟机中安装Linux操作系统(fedora10 )。
3.1选择安装界面3.2检测安装截介质(建议跳过)3.3安装过程中的语言、键盘选择3.4磁盘分区(采用自动分区,总大小与2.2相匹配)3.5引导程序选择(采用GRUB)3.6注意事项(禁用SELinux)2.通过相关命令实现对操作系统的登录、退出与关机3.使用文件浏览器査看Linux操作系统目录结构四、实验思考题1.举例说明Linux操作系统不同目录下文件的主要作用。
2.列出你发现的Linux与Windows操作系统区别。
3.列出决定文本界面及图形界面显示的关键文件,并说明设置的关键。
4.列出你所能想到的虚拟机软件的用途。
实验结论:实验二:linux基本命令(必做实验)一、实验目的、要求(请在操作后附实验结果)1.熟悉Linux操作环境。
Linux实验报告_大三上

实验三普通文件和目录编程1.编写程序mycp.c,实现从命令行读入文件的复制功能,用原始文件系统调用。
实验流程图:实验程序代码://mycp.c#include <stdio.h>#include <sys/types.h>#include <sys/stat.h>#include <fcntl.h>#define bufsize 5int main(int argc,char * argv[]){int fd1,fd2;int i;char buf[bufsize];if(argc!=3){printf("argument error\n");exit(1);}fd1=open(argv[1],O_RDONLY);if(fd1==-1){printf("file %s can not opened\n",argv[1]);exit(1);}fd2=open(argv[2],O_RDWR|O_CREAT);if(fd2==-1){printf("Can not open file %s\n",argv[2]);exit(1);}while(1){i=read(fd1,buf,bufsize);write(fd2,buf,i);if(i!=bufsize) break;}close(fd1);close(fd2);return 0;}Linux环境下运行情况如下:road@ubuntu:~/桌面/work$ gcc -o cp cp.croad@ubuntu:~/桌面/work$ ./cpargument errorPlease use:cp file1 file2road@ubuntu:~/桌面/work$ ./cp a b其中,a为一文件,b为空文件。
2.编写程序mycat.c,实现文件内容的显示,用原始文件系统调用实现。
linux操作系统实验报告
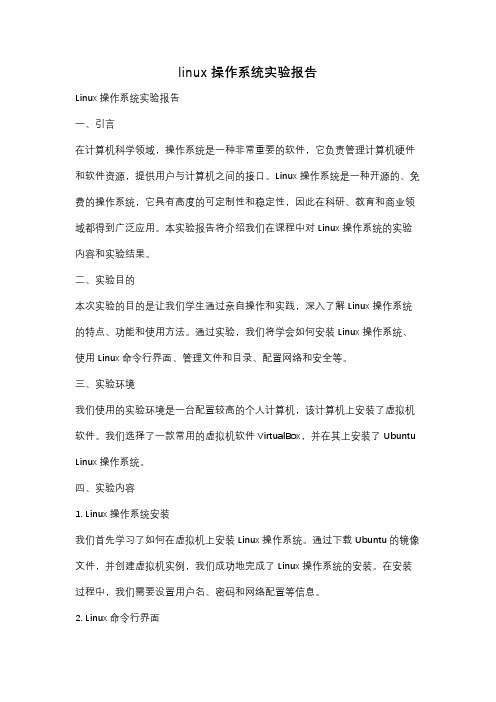
linux操作系统实验报告Linux操作系统实验报告一、引言在计算机科学领域,操作系统是一种非常重要的软件,它负责管理计算机硬件和软件资源,提供用户与计算机之间的接口。
Linux操作系统是一种开源的、免费的操作系统,它具有高度的可定制性和稳定性,因此在科研、教育和商业领域都得到广泛应用。
本实验报告将介绍我们在课程中对Linux操作系统的实验内容和实验结果。
二、实验目的本次实验的目的是让我们学生通过亲自操作和实践,深入了解Linux操作系统的特点、功能和使用方法。
通过实验,我们将学会如何安装Linux操作系统、使用Linux命令行界面、管理文件和目录、配置网络和安全等。
三、实验环境我们使用的实验环境是一台配置较高的个人计算机,该计算机上安装了虚拟机软件。
我们选择了一款常用的虚拟机软件VirtualBox,并在其上安装了Ubuntu Linux操作系统。
四、实验内容1. Linux操作系统安装我们首先学习了如何在虚拟机上安装Linux操作系统。
通过下载Ubuntu的镜像文件,并创建虚拟机实例,我们成功地完成了Linux操作系统的安装。
在安装过程中,我们需要设置用户名、密码和网络配置等信息。
2. Linux命令行界面Linux操作系统的命令行界面是其最基本的用户接口。
我们学习了一些常用的Linux命令,如cd、ls、mkdir、rm等,用于管理文件和目录。
我们还学习了如何使用管道和重定向符号来处理命令的输入和输出。
3. 文件和目录管理Linux操作系统以文件和目录的形式来组织和管理数据。
我们学习了如何创建、复制、移动和删除文件和目录。
我们还学习了如何修改文件和目录的权限和所有权。
4. 网络配置在现代计算机网络中,网络配置是非常重要的一部分。
我们学习了如何配置Linux操作系统的网络设置,包括IP地址、子网掩码、网关等。
我们还学习了如何使用ping命令测试网络连通性。
5. 安全配置在网络环境中,安全性是一个重要的考虑因素。
操作系统Linux系统使用实验报告

操作系统Linux系统使用实验报告电大教师评语教师签字日期成绩学生姓名学号实验名称 Linux 系统使用实验实验报告一、实验目的学会 Linux 系统的基本操作和常用命令,学会使用 vi 编辑器建立、编辑、显示及加工处理文本文件。
二、实验要求:(1)基本操作和常用命令①能够正确地登录和退出 Linux 系统。
②熟悉使用 Linux 常用命令。
(2) vi 编辑器①能够正确地进入和退出 vi。
. ②利用文本插入方式建立-一个文件。
③在新建的文本文件上移动光标位置。
④对该文件执行删除、复原、修改、替换等操作。
三、实验步骤 (1) 登录和退出 Linux 系统①登录。
不论是 GUI 方式或命令行方式的 Linux,在登录系统时,用户都需要输入注册名和密码。
密码验证正确后,用户登录成功。
Linux 系统提供的命令需要在 shell 环境下运行。
为此,要从图形界面进入 shell 界面(即命令行界面)。
在桌面环境下,可以利用终端程序进入传统的命令行操作界面,进入方式有多种,如在“开始”菜单中选择“实用工具”rarr;“终端程序”命令。
②修改用户密码。
用户在使用 Linux 系统的过程中,如果需要,可以使用 passwd 命令修改自己的用户登录密码。
注意:普通用户只能修改自己的密码,管理员 root 用户可以设置任何用户的密码。
③退出系统。
要退出终端程序,可以单击窗口右上角的“关闭”按钮,或在 shell 提示符下执行 exit 命令或logout 命令,也可按快捷键 Ctrl+D。
(2)常用命令命令功能命令功能 cal 显示日历 grep 搜索指定的模式 cat 显示文件内容 ls 列出所有目录 cd 改变目录 more 分页显示文件内容 cp 复制文件 rm 删除文件或目录 date 显示日期 wc 统计文件 echo 显示输入参数 who 显示登录的用户信息(3)vi 编辑器①进入 vi。
②建立一个文件,如 file.c。
linux系统基本操作实验报告
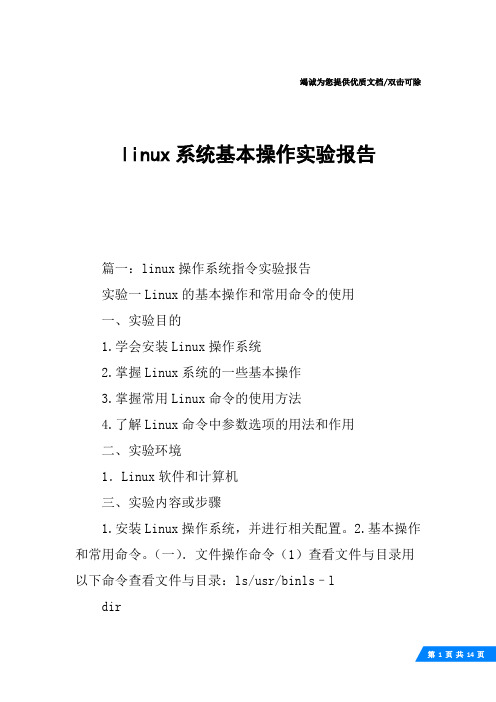
竭诚为您提供优质文档/双击可除linux系统基本操作实验报告篇一:linux操作系统指令实验报告实验一Linux的基本操作和常用命令的使用一、实验目的1.学会安装Linux操作系统2.掌握Linux系统的一些基本操作3.掌握常用Linux命令的使用方法4.了解Linux命令中参数选项的用法和作用二、实验环境1.Linux软件和计算机三、实验内容或步骤1.安装Linux操作系统,并进行相关配置。
2.基本操作和常用命令。
(一).文件操作命令(1)查看文件与目录用以下命令查看文件与目录:ls/usr/binls–ldir(2)显示文件内容命令(cat)设当前目录下包括两个文件text1、text2,用以下列命令了解cat命令的使用:cattext1cattext1text2>text3cattext3|more(3)文件复制命令(cp)了解cp命令的功能和使用技巧,并注意它们的区别:cp/root/*/tempcpreadmetext4cp–r/root/*/temp(带目录复制)cp/root/.[a-z]*/temp(复制所有小写字母开头的隐藏文件)(4)文件改名命令(mv)了解mv命令的功能和使用方法,并注意各命令的区别:mvtext4newtextmvnewtext/home(5)删除文件命令(rm)了解rm命令的功能和使用方法:rmnewtext(二).目录操作命令的使用(1)改变当前目录命令(cd)和显示当前目录命令(pwd)掌握cd命令的功能和使用,并了解以下各命令的区别:cd/rootcd..(返回上一级目录)cd(返回到用户目录内)-1-pwd(显示当前目录在文件系统层次中的位置)(2)建立子目录命令(mkdir)在用户目录下创建如图5-4所示的目录结构。
(3)删除子目录命令(rmdir)在图5-4所建立的目录结构中,删除a1和b1目录。
命令如下:rmdir/home/x/b1rm–r/home/x/a1rm–rf/home/x/a1注意:可选项-r和-f的作用。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
实验三普通文件和目录编程1.编写程序mycp.c,实现从命令行读入文件的复制功能,用原始文件系统调用。
实验流程图:实验程序代码://mycp.c#include <stdio.h>#include <sys/types.h>#include <sys/stat.h>#include <fcntl.h>#define bufsize 5int main(int argc,char * argv[]){int fd1,fd2;int i;char buf[bufsize];if(argc!=3){printf("argument error\n");exit(1);}fd1=open(argv[1],O_RDONLY);if(fd1==-1){printf("file %s can not opened\n",argv[1]);exit(1);}fd2=open(argv[2],O_RDWR|O_CREAT);if(fd2==-1){printf("Can not open file %s\n",argv[2]);exit(1);}while(1){i=read(fd1,buf,bufsize);write(fd2,buf,i);if(i!=bufsize) break;}close(fd1);close(fd2);return 0;}Linux环境下运行情况如下:road@ubuntu:~/桌面/work$ gcc -o cp cp.croad@ubuntu:~/桌面/work$ ./cpargument errorPlease use:cp file1 file2road@ubuntu:~/桌面/work$ ./cp a b其中,a为一文件,b为空文件。
2.编写程序mycat.c,实现文件内容的显示,用原始文件系统调用实现。
//mycat.c#include<unistd.h>#include<sys/types.h>#include<sys/stat.h>#include<fcntl.h>int main(int argc,char* argv[]){int fd;char buffer[100];int num;if(argc!=2){printf("usage : %s filename\n",argv[0]);return 1;}if((fd=open(argv[1],O_RDONLY))==-1){perror("cannot open the file");return 1;}while((num=read(fd,buffer,99))>0){buffer[num]='\0';printf("%s",buffer);}close(fd);return 0;}Linux环境下运行情况如下:road@ubuntu:~/桌面/work$ gcc -o mycat mycat.c road@ubuntu:~/桌面/work$ ./mycatusage : ./mycat filenameroad@ubuntu:~/桌面/work$ ./mycat aiamroadroad@ubuntu:~/桌面/work$其中,a为一个文件名。
3.1用流文件系统函数重新编写上面的程序。
实验流程图:不为3,提示用流文件写的文件复制功能函数://fcp.c#include <stdio.h>#include <sys/types.h>#include <sys/stat.h>#include <fcntl.h>int main(int argc,char * argv[]){FILE *fd1,*fd2;char buffer[20];if(argc!=3){printf("argument error\n");printf("usage : %s filename1 filename2\n",argv[0]);return 1;}fd1=fopen(argv[1],"r");if(fd1==NULL){printf("file %s can not opened\n",argv[1]);return 1;}fd2=fopen(argv[2],"w");if(fd2==-1){printf("Can not open file %s\n",argv[2]);return 1;}while(!feof(fd1)){fread(&buffer,sizeof(char),1,fd1);fwrite(&buffer,sizeof(char),1,fd2);}fclose(fd1);fclose(fd2);return 0;}运行情况如下:road@ubuntu:~/桌面/work$ gcc -o fcp fcp.cfcp.c: In function ‘main’:fcp.c:25: warning: comparison between pointer and integer road@ubuntu:~/桌面/work$ ./fcp a b3.2用流文件编写显示函数:流程图:源程序代码://myfcat.c#include <stdio.h>#include <sys/types.h>#include <sys/stat.h>#include <fcntl.h>int main(int argc,char * argv[]){FILE *fd1;char buffer;if(argc!=2){printf("argument error\n");return 1;}fd1=fopen(argv[1],"r");if(fd1==NULL){printf("file %s can not opened\n",argv[1]);return 1;}while(!feof(fd1)){fscanf(fd1,"%c",&buffer);printf("%c",buffer);}fclose(fd1);return 0;}运行情况如下:road@ubuntu:~/桌面/work$ gcc -o myfcat myfcat.c road@ubuntu:~/桌面/work$ ./myfcat aiamroad程序把文件a的内容显示出来了:iamroad4.调用目录函数,编写程序myls.c,实现按下面格式显示当前目录文件列表:文件名文件大小文件创建时间注意研究文件创建时间的转换,注意研究asctime()函数和ctime()函数的用法。
流程图如下:程序代码如下://myls.c#include <sys/stat.h>#include <stddef.h>#include <stdio.h>#include <sys/types.h>#include <dirent.h>int main(void){DIR *dp;struct dirent *ep;struct stat dup;dp=opendir("./");if(dp!=NULL){while(ep = readdir(dp)){ puts(ep->d_name);stat(ep->d_name,&dup);printf("%u ",dup.st_size);printf("%s ",ctime(&dup.st_atime));}closedir(dp);}elseputs("Couldn't open the directory .\n");return 0;}运行结果:列出了当前目录的所有文件的名称、大小和创建时间road@ubuntu:~/桌面/work$ gcc -o myls myls.cmyls.c: In function ‘main’:myls.c:18: warning: format ‘%u’ expects type ‘unsigned int’, but argument 2 has type ‘__off_t’myls.c:19: warning: format ‘%s’ expects type ‘char *’, but argument 2 has type ‘int’road@ubuntu:~/桌面/work$ ./mylsserver2.c~947 Sat Jan 9 17:25:42 2010pipol.c324 Fri Jan 8 22:47:21 2010newls.c498 Fri Jan 8 22:47:21 2010getip.c~635 Fri Jan 8 22:38:40 2010network1.c1552 Sat Jan 9 15:28:09 2010server28493 Sat Jan 9 17:19:13 2010程序打印出了当前目录所有文件的名称、文件大小和创建时间。
实验四进程实验1.编写程序,显示所有环境变量的名称和值。
程序源代码://environ.c#include<stdio.h>#include<unistd.h>#include<stdlib.h>int main(){char **ch;extern char**environ;ch=environ;while(*ch){printf("%s\n",*ch);ch++;}return 0;}2.*编写程序,模仿讲义上的mysystem程序,实现输入命令的执行。
流程图:程序源代码://mysystem.c#include <stddef.h>#include <stdlib.h>#include <unistd.h>#include <sys/types.h>#include <sys/wait.h>#define SHELL "/bin/sh"int mysystem(const char* command){int status;pid_t pid;pid=fork();if(pid==0){execl(SHELL,SHELL,"-c",command,NULL);_exit(EXIT_FAILURE);}else if(pid<0)status=-1;elseif(waitpid(pid,&status,0)!=pid)status=-1;return status;}int main(int argc,char * argv[]){mysystem(argv[1]);}运行结果:其中用了Shell中的ls命令,列出了当前目录的所有文件road@ubuntu:~/桌面/work$ gcc -o mysystem mysystem.croad@ubuntu:~/桌面/work./mysystem lsa fifo_channel4 mycat.c~ myls.c~ netsever.c read.ca~ fmycat.c myfcat my_sg_get netsever.c~ serverb getip.c myfcat.c my_sg_get.c network server2b~ getip.c~ myfcat.c~ my_sg_get.c~ network1 server2.cclose.c ipop.c myfifo.c mysystem network1.c server2.c~cp kill.c~ mykill mysystem.c network1.c~ server.ccp.c ls.c mykill.c mysystem.c~ network.c server.c~cp.c~ my mykill.c~ netclt network.c~ showev.cfcp my.c mylist.c netclt.c newls.c tcp k.txtfcp.c mycat myls netclt.c~ open.c tcp.txtfcp.c~ mycat.c myls.c netsever pipol.c write.croad@ubuntu:~/桌面/work$实验五命名管道实验1.研究mkfifo命令,在当前目录下创建一个myfifo的命名管道。