JAVA第一阶段测试题及答案
Java1试题加答案()
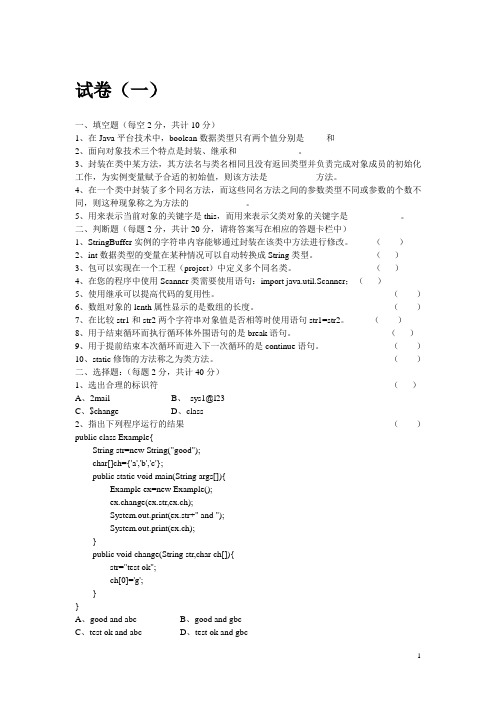
试卷(一)一、填空题(每空2分,共计10分)1、在Java平台技术中,boolean数据类型只有两个值分别是和2、面向对象技术三个特点是封装、继承和。
3、封装在类中某方法,其方法名与类名相同且没有返回类型并负责完成对象成员的初始化工作,为实例变量赋予合适的初始值,则该方法是方法。
4、在一个类中封装了多个同名方法,而这些同名方法之间的参数类型不同或参数的个数不同,则这种现象称之为方法的。
5、用来表示当前对象的关键字是this,而用来表示父类对象的关键字是。
二、判断题(每题2分,共计20分,请将答案写在相应的答题卡栏中)1、StringBuffer实例的字符串内容能够通过封装在该类中方法进行修改。
()2、int数据类型的变量在某种情况可以自动转换成String类型。
()3、包可以实现在一个工程(project)中定义多个同名类。
()4、在您的程序中使用Scanner类需要使用语句:import java.util.Scanner;()5、使用继承可以提高代码的复用性。
()6、数组对象的lenth属性显示的是数组的长度。
()7、在比较str1和str2两个字符串对象值是否相等时使用语句str1=str2。
()8、用于结束循环而执行循环体外围语句的是break语句。
()9、用于提前结束本次循环而进入下一次循环的是continue语句。
()10、static修饰的方法称之为类方法。
()二、选择题:(每题2分,共计40分)1、选出合理的标识符()A、2mailB、_sys1@l23C、$changeD、class2、指出下列程序运行的结果()public class Example{String str=new String("good");char[]ch={'a','b','c'};public static void main(String args[]){Example ex=new Example();ex.change(ex.str,ex.ch);System.out.print(ex.str+" and ");System.out.print(ex.ch);}public void change(String str,char ch[]){str="test ok";ch[0]='g';}}A、good and abcB、good and gbcC、test ok and abcD、test ok and gbc3、Java技术平台共分为3种类型:()A、Java ME、Java SE、Java EEB、Java SE、Java DE、Java EEC、Java UE、Java SE、Java EED、Java FE、Java SE、Java EE4、在控制台显示消息的语句正确的是:()A、system.out.println(“hello world ”);B、System.Out.println(“hello world ”);C、System.out.println(“hello world ”);D、System.Out.Println(“hello world ”);5、下列说法正确的是:()A、Java程序的main方法必须都写在类里面B、Java程序中可以有多个main方法C、Java程序的以public修饰的类名不必与所在的文件的文件名一样。
使用Java实现面向对象编程 阶段测试 机试试卷与参考答案
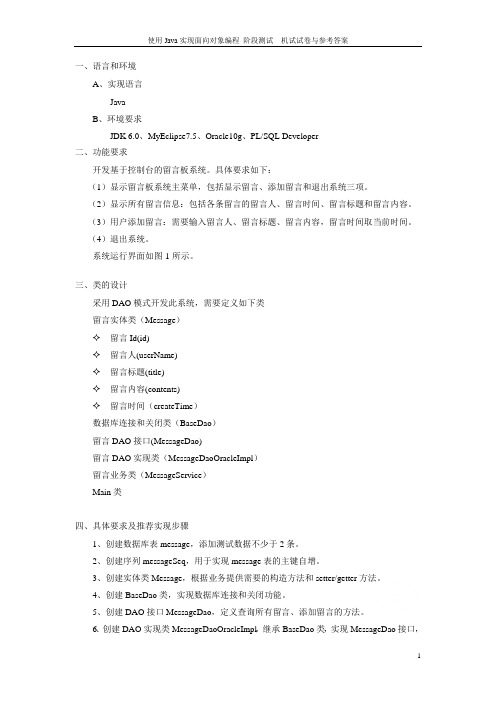
一、语言和环境A、实现语言JavaB、环境要求JDK 6.0、MyEclipse7.5、Oracle10g、PL/SQL Developer二、功能要求开发基于控制台的留言板系统。
具体要求如下:(1)显示留言板系统主菜单,包括显示留言、添加留言和退出系统三项。
(2)显示所有留言信息:包括各条留言的留言人、留言时间、留言标题和留言内容。
(3)用户添加留言:需要输入留言人、留言标题、留言内容,留言时间取当前时间。
(4)退出系统。
系统运行界面如图-1所示。
三、类的设计采用DAO模式开发此系统,需要定义如下类留言实体类(Message)✧留言Id(id)✧留言人(userName)✧留言标题(title)✧留言内容(contents)✧留言时间(createTime)数据库连接和关闭类(BaseDao)留言DAO接口(MessageDao)留言DAO实现类(MessageDaoOracleImpl)留言业务类(MessageService)Main类四、具体要求及推荐实现步骤1、创建数据库表message,添加测试数据不少于2条。
2、创建序列messageSeq,用于实现message表的主键自增。
3、创建实体类Message,根据业务提供需要的构造方法和setter/getter方法。
4、创建BaseDao类,实现数据库连接和关闭功能。
5、创建DAO接口MessageDao,定义查询所有留言、添加留言的方法。
6、创建DAO实现类MessageDaoOracleImpl,继承BaseDao类,实现MessageDao接口,使用JDBC完成相应数据库操作。
7、创建业务类MessageService,完成在控制台显示留言信息和用户添加留言操作。
8、创建Main类,启动和运行系统。
图-1 系统运行界面一、选择题(2分/题)1. char a[3],b[] = "China"; a=b; System.out.print (""+a); 在java 语言中,运行上面的程序段后将输出(d )。
java考试编程题库及答案

java考试编程题库及答案Java考试编程题库及答案1. 基础语法题- 题目:编写一个Java程序,实现两个整数的加法运算,并打印结果。
- 答案:```javapublic class Addition {public static void main(String[] args) {int num1 = 5;int num2 = 10;int sum = num1 + num2;System.out.println("The sum is: " + sum);}}```2. 控制结构题- 题目:编写一个Java程序,判断一个整数是否为素数,并打印结果。
- 答案:```javapublic class PrimeCheck {public static void main(String[] args) {int number = 29;if (isPrime(number)) {System.out.println(number + " is a primenumber.");} else {System.out.println(number + " is not a prime number.");}}public static boolean isPrime(int n) {if (n <= 1) return false;for (int i = 2; i <= Math.sqrt(n); i++) {if (n % i == 0) return false;}return true;}}```3. 数组与循环题- 题目:编写一个Java程序,打印数组中所有元素的平方。
- 答案:```javapublic class SquareElements {public static void main(String[] args) {int[] numbers = {1, 2, 3, 4, 5};for (int i = 0; i < numbers.length; i++) {int square = numbers[i] * numbers[i];System.out.println("Square of " + numbers[i] + " is " + square);}}}4. 面向对象题- 题目:定义一个名为`Car`的类,包含属性`color`和`speed`,以及一个方法`increaseSpeed(int increment)`来增加速度。
Java编程基础智慧树知到课后章节答案2023年下潍坊学院

Java编程基础智慧树知到课后章节答案2023年下潍坊学院潍坊学院第一章测试1.下列关于JDK、JRE和JVM关系的描述中,正确的是()。
A:JRE中包含了JDK,JVM中包含了JRE。
B:JDK中包含了JRE,JRE中包含了JVM。
C:JDK中包含了JRE,JVM中包含了JRE。
D:JRE中包含了JDK,JDK中包含了JVM。
答案:JDK中包含了JRE,JRE中包含了JVM。
2.下面哪种类型的文件可以在Java虚拟机中运行()A:.javaB:.jreC:.exeD:.class答案:.class3.下面关于javac命令作用的描述中,正确的是()。
A:可以将编写好的Java文件编译成.class文件B:可以把文件压缩C:可以把数据打包D:可以执行java程序答案:可以将编写好的Java文件编译成.class文件4.如果jdk的安装路径为:c:\jdk,若想在命令窗口中任何当前路径下,都可以直接使用javac和java命令,需要将环境变量path设置为以下哪个选项()A:c:jreB:c:jdkinC:c:jdkD:c:jrein答案:c:\jdk\bin5.下列Java命令中,哪一个可以编译HelloWorld.java文件()A:javac HelloWorld.javaB:javac HelloWorldC:java HelloWorldD:java HelloWorld.java答案:javac HelloWorld.java6.下列选项中,哪些是Java语言的特性()A:面向对象B:支持多线程C:跨平台性D:简单性答案:面向对象;支持多线程;跨平台性;简单性7.下列关于JRE目录的描述中,正确的是()?A:JRE是一个小型的数据库 B:JRE是Java运行时环境的根目录 C:JRE用于存放一些可执行程序 D:JRE是Java Runtime Environment的缩写答案:JRE是Java运行时环境的根目录;JRE是Java Runtime Environment的缩写8.在Eclipse集成开发环境中,选择【new】->【class】可以创建一个java类。
java模拟试题及答案

java模拟试题及答案Java模拟试题及答案一、选择题(每题2分,共20分)1. 下列哪个关键字是Java中用于定义类的方法的?A. classB. publicC. staticD. void答案: B2. Java中的哪个类是所有Java类的根类?A. ObjectB. StringC. SystemD. Main答案: A3. 在Java中,以下哪个是正确的条件语句?A. if (x > y)B. if x > yC. if (x > y) {D. if (x > y)答案: A4. Java中的哪个关键字用于定义一个接口?A. classB. interfaceC. abstractD. package答案: B5. 下列哪个不是Java的访问修饰符?A. publicB. privateC. protectedD. global答案: D6. Java中,哪个关键字用于实现多态?A. extendsB. implementsC. newD. abstract答案: A7. 在Java中,哪个关键字用于定义一个类为抽象类?A. abstractB. finalC. staticD. void答案: A8. Java中,哪个关键字用于定义一个类为最终类,不能被继承?A. abstractB. finalC. staticD. strictfp答案: B9. 下列哪个是Java中的集合框架?A. AWTB. SwingC. JDBCD. Collections答案: D10. Java中,哪个关键字用于实现异常处理?A. tryB. catchC. finallyD. All of the above答案: D二、简答题(每题5分,共20分)1. 简述Java的垃圾回收机制。
答案: Java的垃圾回收机制是一种自动内存管理功能,用于识别和回收不再使用的对象,从而释放内存。
javaee培训第一阶段基础考试题第一套(含答案)

第一阶段Java培训基础考试题姓名:得分:一、问答题(每题3分, 共27分)1. 垃圾回收的优点和原理。
并考虑2种回收机制。
2. Error与Exception有什么区别?3.谈谈final, finally, finalize的区别。
4.&和&&的区别5.Collection 和Collections的区别。
19,String s = new String("xyz");创建了几个String Object?6. short s1 = 1; s1 = s1 + 1;有什么错? short s1 = 1; s1 += 1;有什么错?7. sleep() 和wait() 有什么区别?8. 数组有没有length()这个方法? String有没有length()这个方法?9. Overload和Override的区别。
Overloaded的方法是否可以改变返回值的类型?二、选择(每题1分,共10分)1.欲构造ArrayList类的一个实例,此类继承了List接口,下列哪个方法是正确的?A ArrayList myList=new Object();B List myList=new ArrayList();C ArrayList myList=new List();D List myList=new List();2.指出正确的表达式A byte=128;B Boolean=null;C long l=0xfffL;D double=0.9239d;3.指出下列程序运行的结果public class Example{String str=new String("good");char[]ch={'a','b','c'};public static void main(String args[]){Example ex=new Example();ex.change(ex.str,ex.ch);System.out.print(ex.str+" and ");Sytem.out.print(ex.ch);}public void change(String str,char ch[]){str="test ok";ch[0]='g';}}A good and abcB good and gbcC test ok and abcD test ok and gbc4.运行下列程序, 会产生什么结果public class X extends Thread implements Runable{public void run(){System.out.println("this is run()");}public static void main(String args[]){Thread t=new Thread(new X());t.start();}}A 第一行会产生编译错误B 第六行会产生编译错误C 第六行会产生运行错误D 程序会运行和启动5.给出下面代码:public class Person{static int arr[] = new int[10];public static void main(String a[]){System.out.println(arr[1]);}}那个语句是正确的?A 编译时将产生错误;B 编译时正确,运行时将产生错误;C 输出零;D 输出空。
Java软件开发工程师入职测试题及参考解答(第1部分)
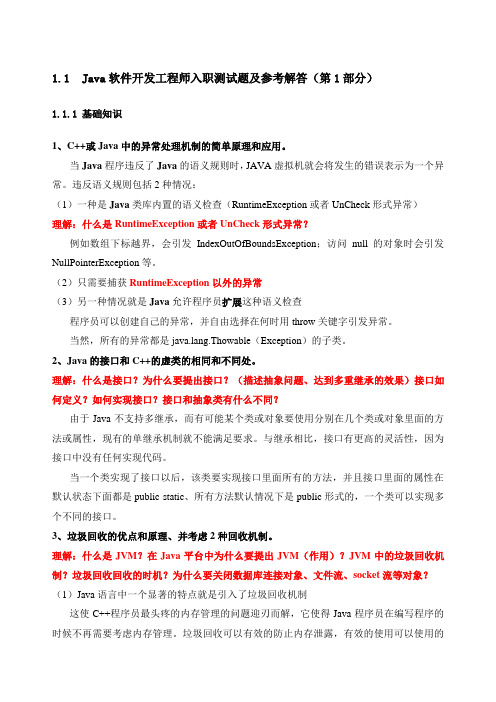
1.1Java软件开发工程师入职测试题及参考解答(第1部分)1.1.1基础知识1、C++或Java中的异常处理机制的简单原理和应用。
当Java程序违反了Java的语义规则时,JAVA虚拟机就会将发生的错误表示为一个异常。
违反语义规则包括2种情况:(1)一种是Java类库内置的语义检查(RuntimeException或者UnCheck形式异常)理解:什么是RuntimeException或者UnCheck形式异常?例如数组下标越界,会引发IndexOutOfBoundsException;访问null的对象时会引发NullPointerException等。
(2)只需要捕获RuntimeException以外的异常(3)另一种情况就是Java允许程序员扩展这种语义检查程序员可以创建自己的异常,并自由选择在何时用throw关键字引发异常。
当然,所有的异常都是ng.Thowable(Exception)的子类。
2、Java的接口和C++的虚类的相同和不同处。
理解:什么是接口?为什么要提出接口?(描述抽象问题、达到多重继承的效果)接口如何定义?如何实现接口?接口和抽象类有什么不同?由于Java不支持多继承,而有可能某个类或对象要使用分别在几个类或对象里面的方法或属性,现有的单继承机制就不能满足要求。
与继承相比,接口有更高的灵活性,因为接口中没有任何实现代码。
当一个类实现了接口以后,该类要实现接口里面所有的方法,并且接口里面的属性在默认状态下面都是public static、所有方法默认情况下是public形式的,一个类可以实现多个不同的接口。
3、垃圾回收的优点和原理、并考虑2种回收机制。
理解:什么是JVM?在Java平台中为什么要提出JVM(作用)?JVM中的垃圾回收机制?垃圾回收回收的时机?为什么要关闭数据库连接对象、文件流、socket流等对象?(1)Java语言中一个显著的特点就是引入了垃圾回收机制这使C++程序员最头疼的内存管理的问题迎刃而解,它使得Java程序员在编写程序的时候不再需要考虑内存管理。
JAVA第一阶段测试题及答案
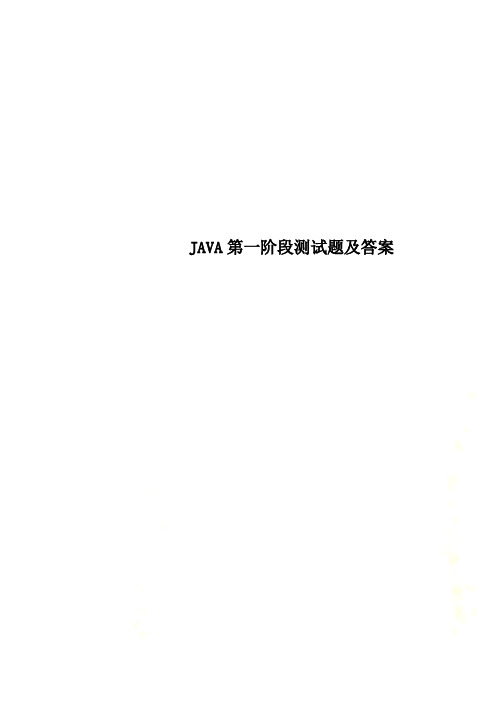
JAVA第一阶段测试题及答案初级部分阶段测试卷选择题1) 在Java类中,使用以下()声明语句来定义公有的int型常量MAX。
A. p ublic int MAX = 100;B. final int MAX = 100;C. p ublic static int MAX = 100;D. p ublic static final int MAX = 100;2) 给定Java代码如下所示,在横线处新增下列()方法,是对cal方法的重载。
(选二项)public class Test{public void cal(int x, int y, int z) {}}A. p ublic int cal(int x, int y, float z){ return 0; }B. public int cal(int x, int y, int z){ return 0; }C. p ublic void cal(int x, int z){ }D. p ublic void cal(int z, int y, int x){ }3) 下面Java代码的运行结果是()。
class Penguin {private String name=null; // 名字private int health=0; // 健康值private String sex=null; // 性别public void Penguin() {health = 10;sex = "雄";System.out.println("执行构造方法。
");}public void print() {System.out.println("企鹅的名字是" + name +",健康值是" + health + ",性别是" + sex+ "。
公司java基础机试题及答案

公司java基础机试题及答案1. 以下哪个选项是Java中的关键字?A. classB. interfaceC. publicD. All of the above答案:D2. Java中,哪个类是所有Java类的根类?A. ObjectB. StringC. SystemD. Thread答案:A3. 在Java中,以下哪种类型的变量不能被声明为final?A. intB. doubleC. StringD. None of the above答案:D4. 下列哪个是Java中的合法标识符?A. 2variableB. variable2C. variable-2D. $variable答案:B5. Java中,哪个关键字用于抛出异常?A. throwB. throwsC. catchD. try答案:A6. 在Java中,以下哪个选项正确地声明了一个字符串数组?A. String[] array;B. String array[];C. Both A and BD. None of the above答案:C7. Java中,哪个关键字用于定义一个接口?A. classB. interfaceC. abstractD. final答案:B8. 在Java中,以下哪个选项是正确的方法重载示例?A. public void display(int number);public void display(double number);B. public int add(int a, int b);public double add(double a, double b);C. Both A and BD. None of the above答案:C9. Java中,哪个关键字用于定义一个抽象方法?A. abstractB. finalC. staticD. synchronized答案:A10. 在Java中,以下哪个选项是正确的内部类声明?A. class Outer {class Inner {}}B. class Outer {public class Inner {}}C. Both A and BD. None of the above答案:C11. Java中,哪个关键字用于实现多态?A. extendsB. implementsC. Both A and BD. None of the above答案:C12. 在Java中,以下哪个选项是正确的泛型类声明?A. public class Box<T> { T t; }B. public class Box { T t; }C. Both A and BD. None of the above答案:A13. Java中,哪个关键字用于声明一个枚举类型?A. enumB. finalC. classD. interface答案:A14. 在Java中,以下哪个选项是正确的异常处理语句?A. try { } catch (Exception e) { }B. catch (Exception e) { } try { }C. Both A and BD. None of the above答案:A15. Java中,哪个关键字用于声明一个同步方法?A. synchronizedB. volatileC. transientD. strictfp 答案:A。
java基础笔试题及答案
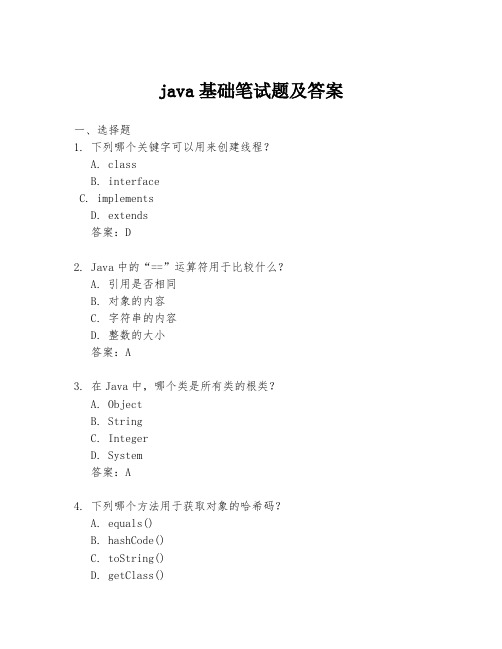
java基础笔试题及答案一、选择题1. 下列哪个关键字可以用来创建线程?A. classB. interfaceC. implementsD. extends答案:D2. Java中的“==”运算符用于比较什么?A. 引用是否相同B. 对象的内容C. 字符串的内容D. 整数的大小答案:A3. 在Java中,哪个类是所有类的根类?A. ObjectB. StringC. IntegerD. System答案:A4. 下列哪个方法用于获取对象的哈希码?A. equals()B. hashCode()C. toString()D. getClass()答案:B5. Java中,哪个关键字用于实现单例模式?A. privateB. publicC. staticD. final答案:C二、简答题1. 解释Java中的垃圾回收机制是什么?答案:Java中的垃圾回收机制是一种自动内存管理功能,它周期性地执行,以识别不再使用的对象,并释放这些对象占用的内存空间。
垃圾回收器会检查内存中的对象,并确定对象是否仍然被应用程序中的其他对象引用。
如果一个对象没有任何引用指向它,那么它就被认为是“垃圾”,垃圾回收器会回收其内存。
2. 什么是Java的异常处理机制?答案:Java的异常处理机制允许程序在发生错误时继续运行,而不是立即崩溃。
它通过使用try、catch、finally和throw关键字来实现。
try块用于捕获可能出现异常的代码,catch块用于处理这些异常,finally块用于执行无论是否发生异常都需要执行的代码,throw关键字用于手动抛出异常。
三、编程题1. 编写一个Java程序,实现一个简单的计算器,能够进行加、减、乘、除操作。
```javaimport java.util.Scanner;public class Calculator {public static void main(String[] args) {Scanner input = new Scanner(System.in);System.out.print("请输入第一个数字:");double num1 = input.nextDouble();System.out.print("请输入第二个数字:");double num2 = input.nextDouble();System.out.print("请选择操作(+、-、*、/):"); char operation = input.next().charAt(0);double result;switch (operation) {case '+':result = num1 + num2;break;case '-':result = num1 - num2;break;case '*':result = num1 * num2;break;case '/':if (num2 != 0) {result = num1 / num2;} else {System.out.println("除数不能为0。
java第一阶段考试题及答案

java第一阶段考试题及答案一、选择题(每题2分,共20分)1. Java中,以下哪个关键字用于声明一个类?A. classB. interfaceC. structD. enum答案:A2. 在Java中,哪个包包含了基本的输入输出类?A. java.utilB. ngC. java.ioD. 答案:C3. 下列哪个数据类型是Java中的原始数据类型?A. StringB. intC. ArrayListD. HashMap答案:B4. Java中的哪个关键字用于捕获异常?A. tryB. catchC. finallyD. throw答案:B5. 在Java中,以下哪个是正确的方法声明?A. public static void main(String args[])B. public static void main(String[] args)C. public static void main(String args)D. public static void main(String args[])答案:B6. Java中,哪个关键字用于定义一个接口?A. classB. interfaceC. structD. enum答案:B7. 在Java中,以下哪个是正确的继承关系?A. 类继承类B. 接口继承接口C. 类继承接口D. 接口继承类答案:C8. Java中,哪个关键字用于实现多态?A. extendsB. implementsC. overrideD. abstract答案:A9. 在Java中,以下哪个是正确的字符串连接操作?A. "Hello" + 5B. "Hello" + '5'C. "Hello" + "World"D. "Hello" + null答案:C10. Java中,哪个关键字用于声明一个抽象方法?A. abstractB. finalC. staticD. synchronized答案:A二、填空题(每题2分,共20分)1. Java中的______关键字用于声明一个方法,该方法没有具体的实现。
java基础笔试题题库及答案

java基础笔试题题库及答案1. Java中,`int`类型变量默认的初始值是多少?答案:Java中,`int`类型变量默认的初始值是0。
2. 在Java中,`String`类型是可变的还是不可变的?答案:在Java中,`String`类型是不可变的。
3. Java中,`==`和`equals()`方法的区别是什么?答案:在Java中,`==`用于比较两个引用是否指向同一对象(对于基本数据类型是比较值),而`equals()`是一个方法,用于比较对象内容是否相等。
4. Java中,`ArrayList`和`LinkedList`的主要区别是什么?答案:`ArrayList`是基于动态数组实现的,支持快速随机访问;而`LinkedList`是基于双向链表实现的,支持快速的插入和删除操作。
5. Java中,`try-catch-finally`语句块的作用是什么?答案:`try-catch-finally`语句块用于异常处理。
`try`块用于捕获异常,`catch`块用于处理异常,`finally`块用于执行清理操作,无论是否发生异常都会执行。
6. Java中,`HashMap`和`Hashtable`的主要区别是什么?答案:`HashMap`允许键和值为null,是非同步的;而`Hashtable`不允许键和值为null,是同步的。
7. Java中,什么是垃圾回收?它是如何工作的?答案:垃圾回收是Java中自动释放不再使用的对象所占内存的过程。
它通过识别不再被任何引用指向的对象来进行回收。
8. Java中,`synchronized`关键字的作用是什么?答案:`synchronized`关键字用于实现多线程同步,确保同一时间只有一个线程可以访问某个特定的资源或代码段。
9. Java中,`final`关键字可以修饰哪些元素?答案:`final`关键字可以修饰变量、方法和类。
被`final`修饰的变量称为常量,其值在初始化后不能被改变;被`final`修饰的方法不能被重写;被`final`修饰的类不能被继承。
java基础笔试题及答案

java基础笔试题及答案1. Java中,下列哪个关键字用于定义一个类?A. classB. interfaceC. abstractD. public答案:A2. 下列哪个选项不是Java语言的基本数据类型?A. intB. floatC. charD. String答案:D3. 在Java中,下列哪个关键字用于抛出一个异常?A. throwB. throwsC. catchD. finally答案:A4. 下列哪个选项是Java中正确的继承关系?A. 类可以继承多个类B. 接口可以继承多个接口C. 类可以实现多个接口D. 接口可以实现类答案:C5. 在Java中,下列哪个关键字用于定义一个接口?A. classB. interfaceC. abstractD. public答案:B6. 下列哪个选项是Java中正确的访问修饰符?A. publicB. privateC. protectedD. all of the above答案:D7. 在Java中,下列哪个关键字用于定义一个方法?A. functionB. methodC. defD. void答案:D8. 下列哪个选项是Java中的集合框架?A. ArrayListB. LinkedListC. HashMapD. all of the above答案:D9. 在Java中,下列哪个关键字用于定义一个抽象类?A. abstractB. finalC. staticD. interface答案:A10. 下列哪个选项是Java中正确的多线程实现方式?A. 实现Runnable接口B. 继承Thread类C. 实现Callable接口D. all of the above答案:D11. 在Java中,下列哪个关键字用于定义一个静态方法?A. staticB. finalC. abstractD. synchronized答案:A12. 下列哪个选项是Java中正确的异常处理关键字?A. tryB. catchC. finallyD. all of the above答案:D13. 在Java中,下列哪个关键字用于定义一个私有成员?A. privateB. protectedC. publicD. static答案:A14. 下列哪个选项是Java中正确的集合初始化方式?A. List<String> list = new ArrayList<>();B. List<String> list = new LinkedList<>();C. List<String> list = new HashMap<>();D. all of the above答案:A15. 在Java中,下列哪个关键字用于定义一个同步方法?A. synchronizedB. volatileC. transientD. strictfp答案:A16. 下列哪个选项是Java中正确的泛型声明方式?A. List list = new ArrayList<String>();B. List<String> list = new ArrayList();C. List<String> list = new ArrayList<String>();D. all of the above答案:B17. 在Java中,下列哪个关键字用于定义一个局部变量?A. finalB. staticC. transientD. volatile答案:A18. 下列哪个选项是Java中正确的异常类?A. ExceptionB. ErrorC. ThrowableD. all of the above答案:D19. 在Java中,下列哪个关键字用于定义一个常量?A. finalB. staticC. transientD. volatile答案:A20. 下列哪个选项是Java中正确的集合遍历方式?A. for loopB. while loopC. for-each loopD. all of the above答案:D。
Java面试笔试试卷及答案2020

JAVA开发试题(卷A)本试题第一、二部分是单项选择,共50题,每小题有且只有一个正确答案,每题2分。
请将答案写在答题纸上。
一、基础知识1、堆栈和队列的相同之处是_(1)__.(1) A.元素的进出满足先进后出 B.元素的进出满足后进先出C.只允许在端点进行插入和删除操作D.无共同点2、十进制数33用十六进制数表示为__(2)__。
A.33H B.21H C.FFH D.12H3、给定一个有n个元素的线性表,若采用顺序存储结构,则在等概率的前提下,向其插入一个元素需要移动的元素个数平均为_(3)__。
A.n + 1B.C.D.n4、判断“链式队列为空”的条件是_(4)_(front为头指针,rear为尾指针)A. front == NULLB. rear == NULLC.front == rearD.front != rear5、在第一趟排序之后,一定能把数据表中最大或最小元素放在其最终位置上的排序算法是__(5)__。
A.冒泡排序B.基数排序C.快速排序D.归并排序6、计算机的总线包含地址总线、数据总线和控制总线。
某计算机CPU有16条地址总线,则该计算机最大的寻址空间为_(6)_字节。
A.32KB. 48KC.64KD.128K7、某页式存储管理系统中的地址结构如下图所示,则_(7)_。
页号页内地址页C.页的大小为4K,最多有1M页D.页的大小为8K,最多有2M页8、接收电子邮件时,通常使用的协议时_(8)_。
A.POP3B.SNMPC.FTPD.WWW9、下列元件中存取速度最快的是_(9)__。
A.CacheB.寄存器C.内存D.外存10、链表不具备的特点是_(10)__。
A.可随机访问任何一个元素B.插入、删除操作不需要移动元素C.无需先估计存储空间大小D.所需存储空间与线性表长度成正比11、在具有100个结点的树中,其边的数目为_(11)_。
A.101B.100C.99D.9812、PUSH 和 POP 命令常用于_(12)__操作。
信思智学Java第一阶段答案-A卷

..............密................封.............线..............学校院系专业Java基础课程试卷 A 卷Array一、选择题(每题1分,共40分)1-5:BACCD 6-10:CBACD11-15:CDBAC 16-20:ABAAC21-25:BDAAC 26-30:D C DBC31-35:DABDC 36-40:BABCB二、填空题(每题2分,共30分)1、*.java ,*.class2、大写3、由字母、数字、下划线、$所组成,其中不能以数字开头,不能是Java的关键字4、wait() 、notify()、notifyAll()5、Java.io6、FileInputStream、FileReader,FileOutputStream、FileWriter7、Interface,implements,static,abstract8、运行时异常,编译时异常,Throwable9、indexof,substring,trim10、SimpleDateFormat11、输入、字节流12、泛型13、Start14、ResultSet15、PreparedStatement三、判断题(每题1分,共10分)1-5:√××××6-10:×√××√四、简答题(每题5分,共10分)1、字节流类抽象父类: InputStream,OutputStream 实现类包括如下几种:缓冲流-过虑流BufferedInputStream、 BufferedOutputStream,字节数组流-节点流ByteArrayInputStream、 ByteArrayOutputStream;字符流抽象父类:Reader, Writer 实现类:BufferedReader、BufferedWriter、FileReader、FileWriter。
S1Java笔试试卷A卷
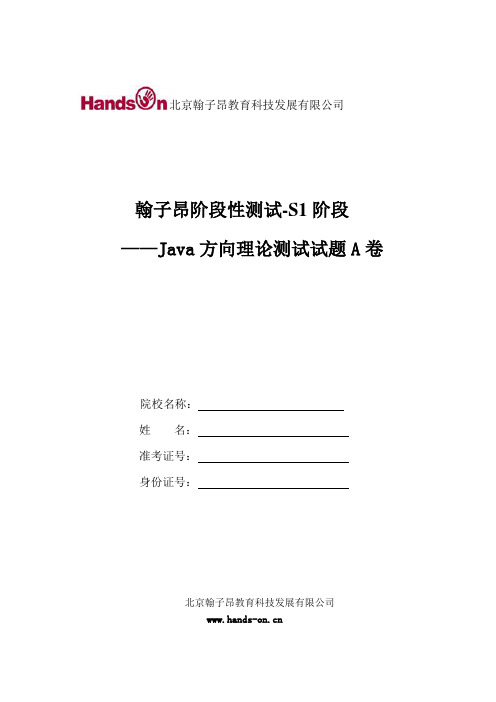
北京翰子昂教育科技发展有限公司翰子昂阶段性测试-S1阶段——Java方向理论测试试题A卷院校名称:姓名:准考证号:身份证号:北京翰子昂教育科技发展有限公司翰子昂阶段性测试_S1阶段——JA V A 方向理论测试试题A卷姓名:准考证号:身份证号:1. short类型的取值范围是:(c )。
(选择一项)a) -27 - 27-1b) 0 – 216-1c) -215– 215-1d) -231– 231-1答:需要记下7种数据类型byte short int long char double float2. 下面哪些是合法的标识符是:(ab)。
(选择两项)a) $personsb) TwoUsersc) *pointd) this答:标示符包含字母数字下划线以及$符号只有数字不可以开头其他的都可以Java标示符大小写敏感长度无限制。
3. 下面哪个是将一个十六进制值赋值给一个long型变量的:(d)。
(选择一项)a) long number = 345L;b) long number = 0345;c) long number = 0345L;d) long number = 0x345L;答:以0x开头的是16进制以0开头的是8进制的4. 下面关于继承的哪些叙述是正确的:(ad)。
(选择两项)a) 在java中只允许单一继承b) 在java中一个类只能实现一个接口c) 在java中一个类不能同时继承一个类和实现一个接口d) java的单一继承使代码更可靠答:一个类可以实现多个接口,但是java中只允许单一继承。
接口与接口之间extends (多继承)接口与类之间implements (可以实现多个接口)类与类extends (只允许单一继承)接口与类不存在接口是一个抽象类比抽象类更特殊5. int的取值范围是:(d)。
(选择一项)a) -27 - 27-1b) 0 – 232-1c) -215– 215-1d) -231– 231-1答:6. main()方法的返回类型是:(b)。
[java]第一阶段测试题-含答案
![[java]第一阶段测试题-含答案](https://img.taocdn.com/s3/m/677e99db49649b6649d74703.png)
第一阶段测试题1、以下是冒泡排序算法,从大到小的排序,请在相应空格地方进行填空:public class BubbleSort{public static void main(String[] args){int score[] = {67, 69, 75, 87, 89, 90, 99, 100};for (int i = 0; i < score.length -1; i++){for(int j = 0 ;j < length-i-1 ; j++){if( score[j] < score[j+1] ){int temp = score[j];score[j] = score[j+1] (4);score[j + 1] = temp;}}}}}2、请问如下代码每个赋值是否正确,请说出错误的编号,并说明原因。
public static void main(String[] args){char v1=16;short v2=16;int v3=32;float v4;char q1=v1+v2; ―――――(1)错。
四则运算至少结果为4字节,所以要强转。
short q2=v1-v2; ―――――(2)错。
同上。
int q3=v1+v2; ――――――(3)正确。
float q4=v4;――――――(4)错,无初始化,不能赋值。
v4=v3;――――――(5)}3、假如有如下代码,请回答输出的结果,并说明原因。
class A{public String value="hi";public String getValue(){return this.value;}public static void test(){System.out.println("hi");}}class B extends A{public String value="hello";public String getValue(){return this.value;}public static void test(){System.out.println("hello");}}public class多态问题{public static void main(String[] args){A obj=new B(); hi 。
java大一期末考试试题及答案

java大一期末考试试题及答案一、选择题(每题2分,共20分)1. Java语言中,哪个关键字用于捕获异常?A. tryB. catchC. throwD. finally答案:B2. 下面哪个选项不是Java语言中的访问修饰符?A. publicB. privateC. protectedD. static答案:D3. Java中,哪个类是所有类的父类?A. ObjectB. StringC. ClassD. System答案:A4. 在Java中,下列哪个方法用于将字符串转换为整型?A. parseInt()B. toInt()C. toInteger()D. Integer.parseInt()5. 下列哪个选项不是Java集合框架中的接口?A. ListB. SetC. MapD. Collection答案:D6. Java中,下列哪个关键字用于定义接口?A. interfaceB. classC. abstractD. extends答案:A7. 下列哪个选项不是Java中的异常类型?A. RuntimeExceptionB. IOExceptionC. SQLExceptionD. Error答案:D8. Java中,下列哪个关键字用于定义抽象方法?A. abstractB. staticC. finalD. synchronized答案:A9. 在Java中,下列哪个关键字用于定义内部类?B. nestedC. innerclassD. class答案:D10. 下列哪个选项不是Java中的集合类?A. ArrayListB. LinkedListC. StackD. Vector答案:C二、填空题(每题2分,共20分)1. Java语言中,用于定义类的关键字是________。
答案:class2. Java中,用于定义方法的关键字是________。
答案:void3. 在Java中,用于定义变量的关键字是________。
java基础知识测试题
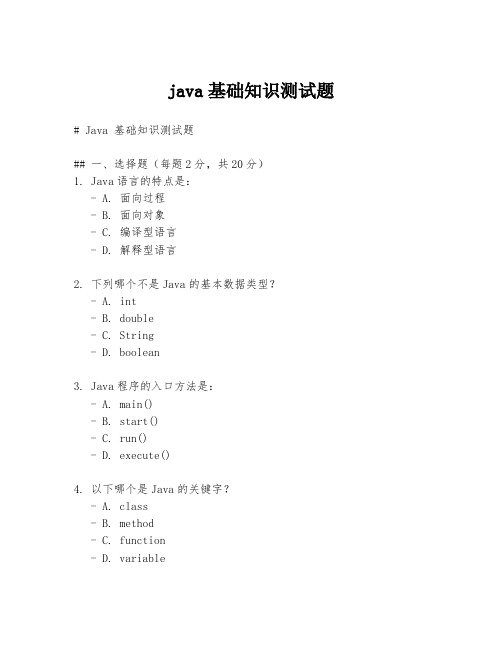
java基础知识测试题# Java 基础知识测试题## 一、选择题(每题2分,共20分)1. Java语言的特点是:- A. 面向过程- B. 面向对象- C. 编译型语言- D. 解释型语言2. 下列哪个不是Java的基本数据类型?- A. int- B. double- C. String- D. boolean3. Java程序的入口方法是:- A. main()- B. start()- C. run()- D. execute()4. 以下哪个是Java的关键字?- A. class- B. method- C. function- D. variable5. 以下哪个不是Java的控制流程语句?- A. if- B. switch- C. for- D. goto6. 哪个是Java的集合框架中最基本的接口? - A. List- B. Set- C. Map- D. Collection7. Java中,哪个类是所有类的父类?- A. Object- B. Class- C. System- D. String8. 以下哪个是Java的异常处理关键字?- A. try- B. catch- C. throw- D. All of the above9. Java中,哪个关键字用于定义接口?- A. class- B. interface- C. package- D. import10. 以下哪个不是Java的访问控制修饰符?- A. public- B. protected- C. private- D. global## 二、填空题(每空2分,共20分)1. Java语言的跨平台特性主要依赖于______。
2. 在Java中,所有的方法都必须在______中定义。
3. Java的异常分为两类:编译时异常和______。
4. Java中的______关键字用于实现多重继承的功能。
Java语言程序设计(一)真题及答案

Java语言程序设计(一)真题及答案-卷面总分:100分答题时间:80分钟试卷题量:35题一、单选题(共25题,共50分)1.以下方法中,不能实现挂起线程的是()。
A.sleep()B.notify()C.wait()D.join()正确答案:B您的答案:本题解析:暂无解析2.以下标识符中,不是Java语言关键字的是()。
A.waitB.newC.longD.switch正确答案:A您的答案:本题解析:暂无解析3.以下数据类型转换中,必须进行强制类型转换的是()。
A.int→charB.short→longC.float→doubleD.byte→int正确答案:A您的答案:本题解析:暂无解析4.以下供选择的概念中,属于面向对象语言重要概念和机制之一的是()。
A.函数调用B.模块C.继承D.结构化正确答案:C您的答案:本题解析:暂无解析5.以下Java程序代码中,能正确创建数组的是()。
A.intmyArray[];myArray[]=newint[5]B.intmyArray[]=newmy(5)C.int[]myArray={1,2,3,4,5}D.intmyArray[5]={1,2,3,4,5}正确答案:C您的答案:本题解析:暂无解析6.某Java程序的类A要利用Swing创建框架窗口,则A需要继承的类是()。
A.JWindowB.JFrameC.JDialogD.JApplet正确答案:B您的答案:本题解析:暂无解析7.MouseMotionListener接口能处理的鼠标事件是()。
A.按下鼠标键B.鼠标点击C.鼠标进入D.鼠标移动正确答案:D您的答案:本题解析:暂无解析8.以下术语中,属于文字字型风格属性的是()。
A.颜色B.宋体C.斜体D.字号正确答案:C您的答案:本题解析:暂无解析9.以下能作为表示线程优先级的数值,并且级别最低的是()。
A.0B.1C.1.5D.1.6正确答案:B您的答案:本题解析:暂无解析10.某Java程序用javax.swing包中的类JFileChooser来实现打开和保存文件对话框。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
初级部分阶段测试卷选择题1) 在Java类中,使用以下()声明语句来定义公有的int型常量MAX。
A. public int MAX = 100;B. final int MAX = 100;C. public static int MAX = 100;D. public static final int MAX = 100;2) 给定Java代码如下所示,在横线处新增下列()方法,是对cal方法的重载。
(选二项)public class Test{public void cal(int x, int y, int z) {}}A. public int cal(int x, int y, float z){ return 0; }B. public int cal(int x, int y, int z){ return 0; }C. public void cal(int x, int z){ }D. public void cal(int z, int y, int x){ }3) 下面Java代码的运行结果是()。
class Penguin {private String name=null; // 名字private int health=0; // 健康值private String sex=null; // 性别public void Penguin() {health = 10;sex = "雄";System.out.println("执行构造方法。
");}public void print() {System.out.println("企鹅的名字是" + name +",健康值是" + health + ",性别是" + sex+ "。
");}public static void main(String[] args) {Penguin pgn = new Penguin();pgn.print();}}A. 企鹅的名字是null,健康值是10,性别是雄。
B. 执行构造方法。
企鹅的名字是null,健康值是0,性别是null。
C. 企鹅的名字是null,健康值是0,性别是null。
D. 执行构造方法。
企鹅的名字是null,健康值是10,性别是雄。
4)在Java中,以下程序编译运行后的输出结果为()。
public class Test {int x, y;Test(int x, int y) {this.x = x;this.y = y;}public static void main(String[] args) {Test pt1, pt2;pt1 = new Test(3, 3);pt2 = new Test(4, 4);System.out.print(pt1.x + pt2.x);}}A. 6B. 3 4C. 8D. 75) Java中,如果类C是类B的子类,类B是类A的子类,那么下面描述正确的是()。
A. C不仅继承了B中的公有成员,同样也继承了A中的公有成员B. C只继承了B中的成员C. C只继承了A中的成员D. C不能继承A或B中的成员6) 给定如下一个Java源文件Child.java,编译并运行Child.java,以下结果正确的是()。
class Parent1 {Parent1(String s){System.out.println(s);}}class Parent2 extends Parent1{Parent2(){System.out.println("parent2");}}public class Child extends Parent2 {public static void main(String[] args) {Child child = new Child();}}A. 编译错误:没有找到构造器Child()B. 编译错误:没有找到构造器Parent1()C. 正确运行,没有输出值D. 正确运行,输出结果为:parent27) 以下关于Object类说法错误的是()。
A. 一切类都直接或间接继承自Object类B. 接口亦继承Object类C. Object类中定义了toString()方法D. Object类在ng包中8) 给定Java代码如下所示,则编译运行后,输出结果是()。
class Parent {public void count() {System.out.println(10%3);}}public class Child extends Parent{public void count() {System.out.println(10/3);}public static void main(String args[]) {Parent p = new Child();p.count();}}A. 1B. 1.0C. 3D. 3.33333333333333359) 编译运行如下Java代码,输出结果是()。
class Base {public void method(){System.out.print ("Base method");}}class Child extends Base{public void methodB(){System.out.print ("Child methodB");}}class Sample {public static void main(String[] args) {Base base= new Child();base.methodB();}}A. Base methodB. Child methodBC. Base method Child MethodBD. 编译错误10) 给定如下Java程序代码,在横线处加入()语句,可以使这段代码编译通过。
(选二项)interface Parent{public int count(int i);}public class Test implements Parent {public int count(int i){return i % 9;}public static void main(String[] args){________________int i = p.count(20);}}A. Test p = new Test();B. Parent p = new Test();C. Parent p = new Parent();D. Test p = new Parent();11.import java.util.*;public class TestListSet{public static void main(String args[]){List list = new ArrayList();list.add(“Hello”);list.add(“Learn”);list.add(“Hello”);list.add(“Welcome”);Set set = new HashSet();set.addAll(list);System.out.println(set.size());}}选择正确答案A.编译不通过B.编译通过,运行时异常C.编译运行都正常,输出3D.编译运行都正常,输出412. 下面关于Java接口的说法错误的是()。
A. 一个Java接口是一些方法特征的集合,但没有方法的实现B. Java接口中定义的方法在不同的地方被实现,可以具有完全不同的行为C. Java接口中可以声明私有成员D. Java接口不能被实例化13. 有如下代码class Example {public static void main(String args[]) {Thread.sleep(3000);System.out.println(“sleep”);}}选择正确答案:A. 编译出错B. 运行时异常C. 正常编译运行,输出sleepD. 正常编译运行,但没有内容输出14、下列表达式不能正确判断String对象str以“fr”开头的是()A.str.substring(0,1).equals(“fr”)B.str.startsWith(“fr”)C.str.indexOf(“fr”)==0D.str.charAt(0)==’f’&&str.charAt(1)==’r’15、关于String和StringBuilder的描述说法正确的是()A.String长度不可变,StringBuilder长度可变B.String长度可变,StringBuilder长度不可变C.String和StringBuilder长度都可变D.String和StringBuilder长度都不可变16、面向对象的特征不包括()A. 封装B. 继承C. 多态D. 实现17、以下不全是引用类型的是()A.包装类B.Float、Double、StringC.int、int[]、int[][]D.除了8种基本数据类型的其他类型18、下面关于可见限定修饰符描述正确的是()A.public修饰的成员任何位置均可访问B.private修饰的成员任何位置都不可访问C.protected修饰的成员只在扩展类中可访问D.没有修饰符的成员同private19、下面关于final关键字描述不正确的是()A.final可以修饰成员变量、方法、类B.final修饰的成员变量可以看成常量C.final修饰的方法不能被重载D.final修饰的类不能被继承20、下面异常处理语句结构一定不正确的是()A.try{} catch(……){} finally{}B.try{} catch(……){}C.try{} finally{}D.catch(……){} finally{}21、下面关于Java集合框架说法错误的是()A.Java集合框架主要包括Collection和Map两类B.Collection描述集合,Map描述映射C.Collection主要包括Set和List两类D.Set内元素无序,而List内元素有序,但元素均不可重复22、下面关于流描述不正确的是()A.按流向分为输入流和输出流B.输入流表示将外部数据读入程序C.输出流表示由程序写出到外部D.复制文件是由外部写到外部,和输入输出无关23、描述线程功能和启动线程的方法分别是()A.run、startB.run、runC.start、startD.start、run24、代码String s=new String(“abc”);对其内存结构说法正确的是()A.创建了一个对象,位于栈内存B.创建了一个对象,位于堆内存C.创建了两个对象,分别位于堆内存和和栈内存D.创建了两个对象,分别位于堆内存和常量池中25、下面关于Constructor说法错误的是()A.Constructor不能被继承,因此不能重写和重载B.Constructor不能是native,final,static,synchronized 的,可以是public,private,或什么都没有C.构造方法里可以写return,但后面什么都不许有D.成员变量声明时候赋值,比构造方法执行还早26、下列关于栈的叙述正确的是()A.栈是非线性结构B.栈只允许在两端插入和删除元素C.栈具有先进先出的特征D.栈具有后进先出的特征27、链表不具有的特点是()A.不必事先估计存储空间B.可随机访问任一元素C.插入删除不需要移动元素D.所需空间与线性表长度成正比28、下列哪些语句关于Java垃圾回收机制的说法是正确的是()A.程序员必须创建一个线程来释放内存B.Java垃圾回收机制负责释放无用内存C.Java垃圾回收机制允许程序员直接释放内存D.Java垃圾回收机制可以在指定的时间释放内存对象29、JAVA语言中的套接字(Socket)是一种基于网络进程通信的接口,是网络通信协议的一种应用。