纯C语言写的一个小型游戏源代码
纯C语言写地一个小型游戏源代码
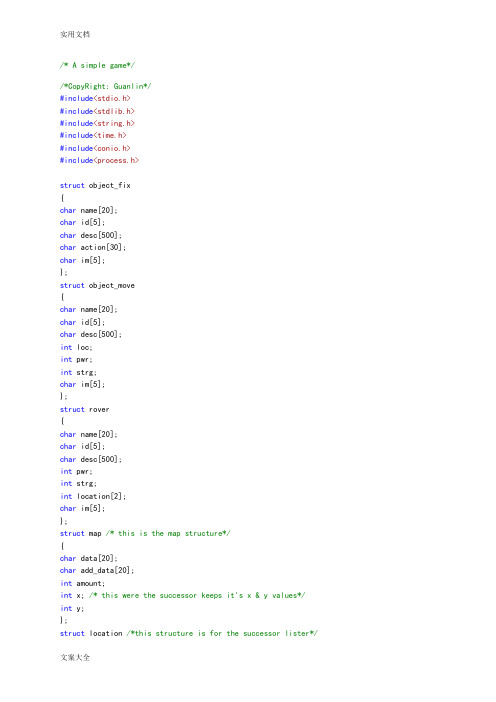
/* A simple game*//*CopyRight: Guanlin*/#include<stdio.h>#include<stdlib.h>#include<string.h>#include<time.h>#include<conio.h>#include<process.h>struct object_fix{char name[20];char id[5];char desc[500];char action[30];char im[5];};struct object_move{char name[20];char id[5];char desc[500];int loc;int pwr;int strg;char im[5];};struct rover{char name[20];char id[5];char desc[500];int pwr;int strg;int location[2];char im[5];};struct map /* this is the map structure*/{char data[20];char add_data[20];int amount;int x; /* this were the successor keeps it's x & y values*/int y;};struct location /*this structure is for the successor lister*/{float height;char obj;};void stats_update(int selected, struct rover *p_rover){switch (selected){case 1:if(p_rover->pwr < 7)printf("\n\nYou do not have enough power to perform this action!\n\n");else{(p_rover->pwr) -= 7;printf("You have destroyed the object!\n\n");}break;case 2:if(p_rover->pwr < 3)printf("\n\nYou do not have enough power to perform this action!\n\n");else if(p_rover->strg > 90)printf("\n\nYou do not have enough storage space for this object!\n\n");else{(p_rover->pwr) -= 3;(p_rover->strg) += 10;printf("You have collected a sample of the object!\n\n");}break;case 3:p_rover->pwr -= 10; /*Distance around object- value gained from mapper module. 1 square = -1 power*/printf("You have avoided the object!\n\n");break;case 4:p_rover->pwr -= 2;printf("You have driven through the obstacle!\n\n");break;case 5:if(p_rover->pwr == 100)printf("\n\nYou do not need to charge up!\n\n");else{p_rover->pwr = 100;printf("You have charged up your rover!\n\n");}break;default:printf("\n\n*****ERROR*****\nInvalid Selection\n\n");break;}}void action(char object, struct rover *p_rover){int selection;switch(object){case 1:printf("\nYou have encountered: A Sandy Rock\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\nPlease choose action 1 or2:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 2:printf("\nYou have encountered: A Solid Rock\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 3:printf("\nYou have encountered: A Mountain\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 4:printf("\nYou have encountered: Dust\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 5:printf("\nYou have encountered: A Sheer Valley\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 6:printf("\nYou have encountered: A Gentle Valley\n\n");printf("This object can be:\n1.\tDriven through\n2.\tAvoided\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;case 7:printf("\nYou have encountered: A 'Martian' Tree\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 8:printf("\nYou have encountered: Shallow Water\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 9:printf("\nYou have encountered: Deep Water\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 10:printf("\nYou have encountered: An Aggressive Alien\n\n");printf("This object can be:\n1.\tDestroyed\nPlease enter 1:\t");scanf("%d", &selection);selection = 1;stats_update(selection, p_rover);break;case 11:printf("\nYou have encountered: A Non-Aggressive Alien\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 12:printf("\nYou have encountered: Another Rover\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 13:printf("\nYou have encountered: A Power Station\n\n");printf("You can:\n1.\tCharge up\n2.\tAvoid\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 5;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;default:printf("\n\n*****ERROR*****\n\n");break;}}void show_map(struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /*the show map function calling number and number_2 from the map structure to see if moveable objects are needed*/{struct map f_map[8][8]; /*8 by 8 map*/int i,j, rx, ry, object; /*this is your x and y value in your map (f_map)*/system("cls"); /* this is your x and y value in your map (f_map)*///srand(time(NULL)); /*calling the time from include to gather random variables*/for(i=0;i<8;i++) /*for loop to copy all your fixed object lists into f_map so they can be displayed*/{for(j=0;j<8;j++)strcpy(f_map[i][j].data,"");if (number->amount>0) /*this is looking at the add function to see weather or not there are aliens in f_map*/{for(i=0;i<number->amount;i++) /*allocating the number of aliens in f_map*/strcpy(f_map[rand()%8][rand()%8].data, anon->im); /*randomizing their position*/}if(number_2->amount>0) /*repeat of above just for rovers instead*/{for(i=0;i<number_2->amount;i++)strcpy(f_map[rand()%8][rand()%8].data, rvr->im);}rx= p_rover->location[0];ry= p_rover->location[1];strcpy(f_map[1][0].data, wdp->im);strcpy(f_map[4][0].data, mnt->im);strcpy(f_map[5][0].data, mnt->im);strcpy(f_map[3][1].data, dst->im);strcpy(f_map[4][1].data, dst->im);strcpy(f_map[3][2].data, dst->im);strcpy(f_map[4][2].data, dst->im);strcpy(f_map[0][3].data, mnt->im);strcpy(f_map[1][3].data, mnt->im);strcpy(f_map[3][3].data, vshr->im);strcpy(f_map[4][3].data, dst->im);strcpy(f_map[5][3].data, vgnt->im);strcpy(f_map[3][4].data, vshr->im);strcpy(f_map[4][4].data, dst->im);strcpy(f_map[5][4].data, vgnt->im);strcpy(f_map[2][5].data, wshl->im);strcpy(f_map[3][5].data, wshl->im);strcpy(f_map[4][5].data, wshl->im);strcpy(f_map[1][6].data, pstn->im);strcpy(f_map[2][6].data, wdp->im);strcpy(f_map[3][6].data, wdp->im);strcpy(f_map[4][6].data, wshl->im);strcpy(f_map[7][6].data, mnt->im);strcpy(f_map[0][7].data, mnt->im);strcpy(f_map[1][7].data, wdp->im);strcpy(f_map[2][7].data, wshl->im);strcpy(f_map[3][7].data, wshl->im);strcpy(f_map[6][7].data, mnt->im);strcpy(f_map[rx][ry].data, p_rover->im);if((rx == 1 && ry == 0) || (rx == 2 && ry == 6) ||(rx == 3 && ry == 6) ||(rx == 1 && ry =={object = 9;action(object, p_rover);}else if((rx == 4 && ry == 0) || (rx == 5 && ry == 0) || (rx == 0 && ry == 3) || (rx == 1 && ry == 3) || (rx == 7 && ry == 6) || (rx == 0 && ry == 7) || (rx == 6 && ry == 7)){object = 3;action(object, p_rover);}else if((rx == 3 && ry== 1) || (rx == 4 && ry == 1) || (rx == 3 && ry== 2) || (rx == 4 && ry == 2) || (rx == 4 && ry == 3) || (rx == 4 && ry == 4)){object = 4;action(object, p_rover);}else if((rx == 3 && ry == 3) || (rx == 3 && ry == 4)){object = 5;action(object, p_rover);}else if((rx == 5 && ry == 3) || (rx == 5 && ry == 4)){object = 6;action(object, p_rover);}else if((rx == 2 && ry == 5) || (rx == 3 && ry == 5 ) || (rx == 4 && ry == 5) || (rx == 4 && ry == 6) ||(rx == 2 && ry == 7) || (rx == 3 && ry == 7)){object = 8;action(object, p_rover);}else if(rx == 1 && ry == 6){object = 13;action(object, p_rover);}i=0; /*re-allocate i to 0 so map is printed from start*/for(i=0;i<8;i++) /*8 by 8 map*/{printf("+----+----+----+----+----+----+----+----+\n");for(j=0;j<8;j++) /*8 by 8 map*/{if(strlen(f_map[i][j].data)!=0) /*if function to print nothing but 4 spaces if there innothing allocated in [i][j]*/printf("|%4s",f_map[i][j].data);elseprintf("| "); /*end of coloumn visible map*/}printf("|\n"); /*end of last coloumn visible map*/}printf("+----+----+----+----+----+----+----+----+\n"); /*bottom of map*/printf("\n");printf("\270");printf("Group B\n");}void add_obj(struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /* add movable object function, *number=aliens, *number_2=rovers*/{int object, t, f;char alien;char rover;printf("This is the add movable object function\n");printf("how many aliens would you like?\n");scanf("%d",&t); /*user input of amount of aliens*/number->amount=t; /*saving the number in structure map-amount*/printf("how many rovers would you like?\n");scanf("%d",&f); /*user input of amount of rovers*/number_2->amount=f; /*saving the number in structure map-amount*/show_map(number, number_2, rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl,wdp, aagr, anon, rvr, pstn, p_rover); /*go to show map function with the number of aliens and number of rovers*/}void successor() /*this functions askes the user for the location and then were they want to go from there, printing out that location*/{struct map location;int menu;int ncol,nrow; /*we can change the n value depends how large the map you need.*/ncol=8;nrow=8;printf("This is the successor function\n");printf("Enter the \nx=\ty=\n");scanf("%d%d",&location.x,&location.y); /*saving location in map location x and y*/printf("Enter operator choice\n");printf("1=left\n");printf("2=right\n");printf("3=upwards\n");printf("4=down\n");printf("5=quit\n");scanf("%d",&menu);switch(menu) /*menu switch to show the position above, underneith, and next to the rover*/ {case 1:location.x=location.x-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 2:location.x=location.x+1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 3:location.y=location.y-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 4:location.y=location.y+1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 5:printf("*****back to program*****\n"); /* Quits the program and prints out the message */ break;default:printf("*****Please enter an integer from 1-5*****\n"); /* A default option if the user enters an incorrect value */break;}printf("\n");}void help(struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover*p_rover) /*help function*/{int i;char mov_obj;printf("++This is the help function++\n");printf("| 1-successor |\n");printf("| 2-add movable obj |\n");printf("| 3-remove moveabla obj |\n\n");printf("\t* * *\n");printf("\t Index: \n\n");printf("\t Sandy Rock: *\n");printf("\t Solid Rock: o\n");printf("\t Water:\262\262\262\262 \n");printf("\t Shallow water: \260\260\260\260\n");printf("\t Mountain: ^^^^\n");printf("\t Dust: ....\n");printf("\t* sher valley: VVVV *\n");printf("\t shallow valey: vvvv\n");printf("\t power station: \025\n");printf("\t alien: *_*\n");printf("\t other rovers: #\n");printf("\t* * *\n");scanf("%d",&i);switch(i) /*switch menu to go to the successor function, or the add/remove function*/ {case 1:successor();break;case 2:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);break;case 3:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);break;default:printf("choose a number from the list\n");}}void direction(int input, struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover){switch(input){case 119: /*w = up*/if(p_rover->location[0] > 0){p_rover->location[0]--;p_rover->pwr--;}else p_rover->location[0]=7;break;case 115: /*s = down*/if(p_rover->location[0] < 7){p_rover->location[0]++;p_rover->pwr--;}else p_rover->location[0]=0;break;case 97: /*a = left*/if(p_rover->location[1] > 0){p_rover->location[1]--;p_rover->pwr--;}else p_rover->location[1]=7;break;case 100: /*d = right*/if(p_rover->location[1] < 7){p_rover->location[1]++;p_rover->pwr--;}else p_rover->location[1]=0;break;default:printf("Invalid operator!\n\n");break;}}int control(int input){input = _getch();return input;}void main(void){int menu;int quit = 0;int input = 0;struct object_fix rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl, wdp;struct object_move aagr, anon, rvr, pstn;struct rover p_rover;struct map number, number_2;number.amount = 0; /* setting the initial amount of aliens as 0*/number_2.amount = 0;strcpy(,"Sandy Rock");strcpy(rsny.id,"RSNY");strcpy(rsny.desc,"A rock made of softer material that can be destroyed or collected"); strcpy(rsny.im,"*");strcpy(,"Solid Rock");strcpy(rsld.id,"RSLD");strcpy(rsld.desc,"A rock made of hard material that cannot be destroyed, but can be collected or avoided");strcpy(rsld.im,"O");strcpy(,"Mountain");strcpy(mnt.id,"MNT");strcpy(mnt.desc,"A large obstacle made of different materials that can only be avoided"); strcpy(mnt.im,"^^^^");strcpy(,"Dust");strcpy(dst.id,"DST");strcpy(dst.desc,"Small particles of sand, rock, etc. that may reduce visibility or slow down the vehicle, can be driven through but has extra power consumption");strcpy(dst.im,"....");strcpy(,"Sheer Valley");strcpy(vshr.id,"VSHR");strcpy(vshr.desc,"A depression in the land with sheer sides, it cannot be driven through and must be avoided");strcpy(vshr.im,"VVVV");strcpy(,"Gentle Valley");strcpy(vgnt.id,"VGNT");strcpy(vgnt.desc,"A depression in the land with gentle sides, it can be driven through with extra power consumption or can be avoided");strcpy(vgnt.im,"vvvv");strcpy(,"'Martian' Tree");strcpy(mtre.id,"MTRE");strcpy(mtre.desc,"A woody plant native to Mars, it may have the same characteristics as an 'Earth' tree");strcpy(mtre.im,"\330");strcpy(,"Shallow Water");strcpy(wshl.id,"WSHL");strcpy(wshl.desc,"A shallow body of water that can be driven through, can be in the formof a small puddle or a large lake");strcpy(wshl.im,"\260\260\260\260");strcpy(,"Deeper Water");strcpy(wdp.id,"WDP");strcpy(wdp.desc,"A deeper body of water that cannot be driven through, commonly in the form of a large lake or river");strcpy(wdp.im,"\262\262\262\262");strcpy(,"Aggressive Alien");strcpy(aagr.id,"AAGR");strcpy(aagr.desc,"An alien creature that will move upon its own accord, may become aggressive on contact");strcpy(aagr.im,"*_*");strcpy(,"Non-Aggressive Alien");strcpy(anon.id,"ANON");strcpy(anon.desc,"An alien creature that will move upon its own accord, will not become aggressive on contact");strcpy(anon.im,"*_*");strcpy(,"Rover");strcpy(rvr.id,"RVR");strcpy(rvr.desc,"A Mars Rover vehicle to transport around the map, it can destroy, collect or avoid objects. It has a power and object storage");strcpy(rvr.im,"#");strcpy(,"Power Station");strcpy(pstn.id,"PSTN");strcpy(pstn.desc,"A movable power station that will restore power to the Mars Rovers"); strcpy(pstn.im,"\025");strcpy(p_,"User Mars Rover");strcpy(p_rover.id,"URVR");strcpy(p_rover.desc,"A Mars vehicle that is controlled by the user. Explores the map collecting, destroying and avoiding objects");strcpy(p_rover.im,"<^^>");p_rover.pwr = 100;p_rover.strg = 0;p_rover.location[0] = 7;p_rover.location[1] = 0; /****************************************************/printf("*******************************START********************************\n\n");do{ /* Object will be gained from mapper */printf("+++++++++++++++++++++++++++++++MENU+++++++++++++++++++++++++++++++++\n");printf("Please choose one of the following option:\n");printf("1 - Start\n");printf("2 - Controls\n");printf("3 - Show Map\n");printf("4 - Help Menu\n");printf("5 - Quit\n\n");printf("--------------->");scanf("%d", &menu);switch (menu){case 1:show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);while (quit != 1 ){input = control(input);if (input == 27)quit = 1;else{if(p_rover.pwr>0){direction(input, &number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);printf("Rover stats: \nPower: %d%% \nStorage: %d%%\n", p_rover.pwr, p_rover.strg);}else{printf("*******************END GAME********************\n\n"); // ends game when charge = 0.break;}}}break;case 2:quit = 0;printf("\n");printf("* * *\n");printf(" Controls \n"); // menu for the keys used on the keyboard to move the rover in different directions.printf(" Up : W \n");printf(" Down : S \n");printf("* Left : A * \n");printf("* Right : D * \n");printf(" choose : Enter \n");printf("* * *\n");break;case 3:quit = 0;show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr, &vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover); break;case 4:quit = 0;help(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover); break;case 5:printf("*********************END***********************\n"); break;}}while(menu!=5);printf("\n -----Please press any key to exit game-----\n"); getch();}。
C语言小游戏源代码《贪吃蛇》

void init(void){/*构建图形驱动函数*/ int gd=DETECT,gm; initgraph(&gd,&gm,""); cleardevice(); }
欢迎您阅读该资料希望该资料能给您的学习和生活带来帮助如果您还了解更多的相关知识也欢迎您分享出来让我们大家能共同进步共同成长
C 语言小游戏源代码《贪吃பைடு நூலகம்》
#define N 200/*定义全局常量*/ #define m 25 #include <graphics.h> #include <math.h> #include <stdlib.h> #include <dos.h> #define LEFT 0x4b00 #define RIGHT 0x4d00 #define DOWN 0x5000 #define UP 0x4800 #define Esc 0x011b int i,j,key,k; struct Food/*构造食物结构体*/ { int x; int y; int yes; }food; struct Goods/*构造宝贝结构体*/ { int x; int y; int yes; }goods; struct Block/*构造障碍物结构体*/ { int x[m]; int y[m]; int yes; }block; struct Snake{/*构造蛇结构体*/ int x[N]; int y[N]; int node; int direction; int life; }snake; struct Game/*构建游戏级别参数体*/ { int score; int level; int speed;
(完整word版)纯C语言写的一个小型游戏源代码
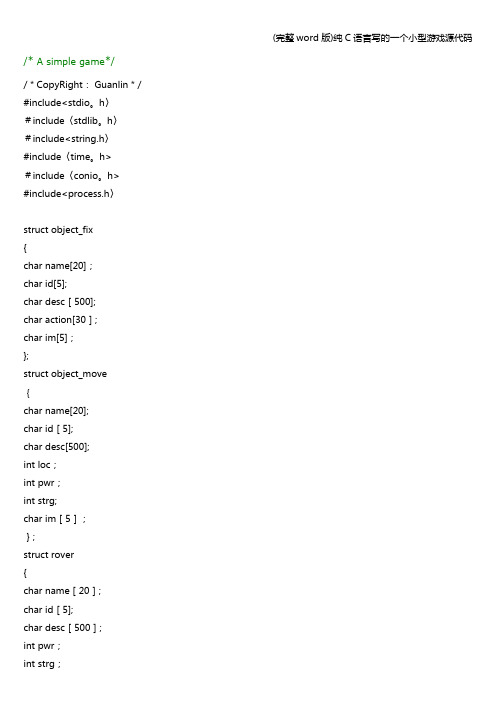
/* A simple game*//*CopyRight: Guanlin*/ #include<stdio。
h〉#include〈stdlib。
h〉#include<string.h〉#include〈time。
h>#include〈conio。
h>#include<process.h〉struct object_fix{char name[20];char id[5];char desc[500];char action[30];char im[5];};struct object_move{char name[20];char id[5];char desc[500];int loc;int pwr;int strg;char im[5];};struct rover{char name[20];char id[5];char desc[500];int pwr;int strg;int location[2];char im[5];};struct map /* this is the map structure*/{char data[20];char add_data[20];int amount;int x; /* this were the successor keeps it's x & y values*/int y;};struct location /*this structure is for the successor lister*/{float height;char obj;};void stats_update(int selected, struct rover *p_rover){switch (selected){case 1:if(p_rover->pwr 〈 7)printf("\n\nYou do not have enough power to perform this action!\n\n”);else{(p_rover—〉pwr)—= 7;printf("You have destroyed the object!\n\n");}break;case 2:if(p_rover->pwr 〈 3)printf(”\n\nYou do not have enough power to perform this action!\n\n"); else if(p_rover—〉strg > 90)printf("\n\nYou do not have enough storage space for this object!\n\n");{(p_rover-〉pwr) —= 3;(p_rover—>strg) += 10;printf("You have collected a sample of the object!\n\n");}break;case 3:p_rover->pwr -= 10; /*Distance around object— value gained from mapper module. 1 square = -1 power*/printf(”You have avoided the object!\n\n”);break;case 4:p_rover—>pwr —= 2;printf("You have driven through the obstacle!\n\n”);break;case 5:if(p_rover—〉pwr == 100)printf(”\n\nYou do not need to charge up!\n\n");else{p_rover—〉pwr = 100;printf(”You have charged up your rover!\n\n”);}break;default:printf("\n\n*****ERROR*****\nInvalid Selection\n\n”);break;}}void action(char object, struct rover *p_rover){int selection;switch(object)case 1:printf(”\nYou have encountered: A Sandy Rock\n\n");printf(”This object can be:\n1.\tDestroyed\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d”, &selection);stats_update(selection, p_rover);break;case 2:printf(”\nYou have encountered: A Solid Rock\n\n");printf(”This object can be:\n1。
C语言小游戏源代码《打砖块》
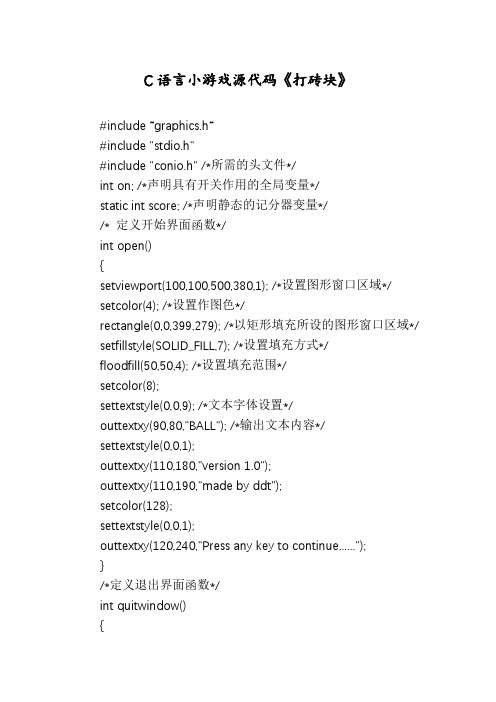
C语言小游戏源代码《打砖块》#include “graphics.h“#include "stdio.h"#include "conio.h" /*所需的头文件*/int on; /*声明具有开关作用的全局变量*/static int score; /*声明静态的记分器变量*//* 定义开始界面函数*/int open(){setviewport(100,100,500,380,1); /*设置图形窗口区域*/ setcolor(4); /*设置作图色*/rectangle(0,0,399,279); /*以矩形填充所设的图形窗口区域*/ setfillstyle(SOLID_FILL,7); /*设置填充方式*/floodfill(50,50,4); /*设置填充范围*/setcolor(8);settextstyle(0,0,9); /*文本字体设置*/outtextxy(90,80,"BALL"); /*输出文本内容*/settextstyle(0,0,1);outtextxy(110,180,"version 1.0");outtextxy(110,190,"made by ddt");setcolor(128);settextstyle(0,0,1);outtextxy(120,240,"Press any key to continue......");}/*定义退出界面函数*/int quitwindow(){char s; /*声明用于存放字符串的数组*/setviewport(100,150,540,420,1);setcolor(YELLOW);rectangle(0,0,439,279);setfillstyle(SOLID_FILL,7);floodfill(50,50,14);setcolor(12);settextstyle(0,0,8);outtextxy(120,80,"End");settextstyle(0,0,2);outtextxy(120,200,"quit? Y/N");sprintf(s,"Your score is:%d",score);/*格式化输出记分器的值*/ outtextxy(120,180,s);on=1; /*初始化开关变量*/}/*主函数*/main(){int gdriver,gmode;gdriver=DETECT; /*设置图形适配器*/gmode=VGA; /*设置图形模式*/registerbgidriver(EGAVGA_driver); /*建立独立图形运行程序*/initgraph(gdriver,gmode,""); /*图形系统初试化*/setbkcolor(14);open(); /*调用开始界面函数*/getch(); /*暂停*/while(1) /*此大循环体控制游戏的反复重新进行*/{intdriver,mode,l=320,t=400,r,a,b,dl=5,n,x=200,y=400,r1=10,dx=-2,dy=-2;/*初始化小球相关参数*/int left,top,right,bottom,i,j,k,off=1,m,num;/*方砖阵列相关参数*/static int pp;static int phrase; /*一系列起开关作用的变量*/int oop=15;pp=1;score=0;driver=DETECT;mode=VGA;registerbgidriver(EGAVGA_driver);initgraph(driver,mode,"");setbkcolor(10);cleardevice(); /*图形状态下清屏*/clearviewport(); /*清除现行图形窗口内容*/b=t+6;r=l+60;setcolor(1);rectangle(0,0,639,479);setcolor(4);rectangle(l,t,r,b);setfillstyle(SOLID_FILL,1);floodfill(l+2,t+2,4);for(i=0,k=0;ii++) /*此循环绘制方砖阵列*/{top[i]=k;bottom[i]=top[i]+20;k=k+21;oop--;for(j=0,m=0;jj++){left[j]=m;right[j]=left[j]+80;m=m+81;setcolor(4);rectangle(left[j],top[i],right[j],bottom[i]); setfillstyle(SOLID_FILL,j+oop);floodfill(left[j]+1,top[i]+1,4);num[i][j]=pp++;}}while(1) /*此循环控制整个动画*/{while(!kbhit()){x=x+dx; /*小球运动的圆心变量控制*/ y=y+dy;if(x+r1r||x+r1r){ phrase=0;}if((x-r1=r||x+r1=r)x+r1=l){if(yt)phrase=1;if(y+r1=tphrase==1){dy=-dy;y=t-1-r1;}}if(off==0)continue;for(i=0;ii++) /*此循环用于判断、控制方砖阵列的撞击、擦除*/for(j=0;jj++){if((x+r1=right[j]x+r1=left[j])||(x-r1=right[j]x-r1=left[j])){if(( y-r1top[i]y-r1=bottom[i])||(y+r1=top[i]y+r1=bottom[i] )) {if(num[i][j]==0){continue; }setcolor(10);rectangle(left[j],top[i],right[j],bottom[i]);setfillstyle(SOLID_FILL,10);floodfill(left[j]+1,top[i]+1,10);dy=-dy;num[i][j]=0;score=score+10;printf(“%d\b\b\b",score);}}if((y+r1=top[i]y+r1=bottom[i])||(y-r1=top[i]y-r1=bottom[i])) {if((x+r1=left[j]x+r1right[j])||(x-r1=right[j]x-r1left[j])){if(num[i][j]==0){ continue;}setcolor(10);rectangle(left[j],top[i],right[j],bottom[i]); setfillstyle(SOLID_FILL,10);floodfill(left[j]+1,top[i]+1,10);dx=-dx;num[i][j]=0;score=score+10;printf("%d\b\b\b",score);}}}if(x+r1639) /*控制小球的弹射范围*/ {dx=-dx;x=638-r1;}if(x=r1){dx=-dx;x=r1+1;}if(y+r1=479){off=0;quitwindow();break;}if(y=r1){dy=-dy;y=r1+1;}if(score==560){off=0;quitwindow();break;}setcolor(6);circle(x,y,r1);setfillstyle(SOLID_FILL,14);floodfill(x,y,6);delay(1000);setcolor(10);circle(x,y,r1);setfillstyle(SOLID_FILL,10);floodfill(x,y,10);}a=getch();setcolor(10);rectangle(l,t,r,b);setfillstyle(SOLID_FILL,10);floodfill(l+2,t+2,10);if(a==77l=565)/*键盘控制设定*/{dl=20;l=l+dl;}if(a==75l=15){dl=-20;l=l+dl;}if(a=='y'on==1)break;if(a=='n'on==1)break;if(a==27){quitwindow();off=0;}r=l+60;setcolor(4);rectangle(l,t,r,b);setfillstyle(SOLID_FILL,1);floodfill(l+5,t+5,4);delay(100);}if(a=='y'on==1) /*是否退出游戏*/ {break;}if(a=='n'on==1){ continue;} } closegraph(); }。
C语言游戏源代码

C语言游戏源代码1、简单的开机密码程序#include "conio.h"#include "string.h"#include "stdio.h"void error(){window(12,10,68,10);textbackground(15);textcolor(132);clrscr();cprintf("file or system error! you can't enter the system!!!");while(1); /*若有错误不能通过程序*/}void look(){FILE *fauto,*fbak;char *pass="c:\\windows\\password.exe"; /*本程序的位置*/char a[25],ch;char *au="autoexec.bat",*bname="hecfback.^^^"; /*bname 是autoexec.bat 的备份*/setdisk(2); /*set currently disk c:*/chdir("\\"); /*set currently directory \*/fauto=fopen(au,"r+");if (fauto==NULL){fauto=fopen(au,"w+");if (fauto==NULL) error();}fread(a,23,1,fauto); /*读取autoexec.bat前23各字符*/a[23]='\0';if (strcmp(a,pass)==0) /*若读取的和pass指针一样就关闭文件,不然就添加*/fclose(fauto);else{fbak=fopen(bname,"w+");if (fbak==NULL) error();fwrite(pass,23,1,fbak);fputc('\n',fbak);rewind(fauto);while(!feof(fauto)){ch=fgetc(fauto);fputc(ch,fbak);}rewind(fauto);rewind(fbak);while(!feof(fbak)){ch=fgetc(fbak);fputc(ch,fauto);}fclose(fauto);fclose(fbak);remove(bname); /*del bname file*/}}void pass(){char *password="";char input[60];int n;while(1){window(1,1,80,25);textbackground(0);textcolor(15);clrscr();n=0;window(20,12,60,12);textbackground(1);textcolor(15);clrscr();cprintf("password:");while(1){input[n]=getch();if (n>58) {putchar(7); break;} /*若字符多于58个字符就结束本次输入*/ if (input[n]==13) break;if (input[n]>=32 && input[n]<=122) /*若字符是数字或字母才算数*/ {putchar('*');n++;}if (input[n]==8) /*删除键*/if (n>0){cprintf("\b \b");input[n]='\0';n--;}}input[n]='\0';if (strcmp(password,input)==0)break;else{putchar(7);window(30,14,50,14);textbackground(15);textcolor(132);clrscr();cprintf("password error!");getch();}}}main(){look();pass();}2、彩色贪吃蛇#include <graphics.h>#include <stdlib.h>#define N 200#define up 0x4800#define down 0x5000#define left 0x4b00#define right 0x4d00#define esc 0x011b#define Y 0x1579#define n 0x316eint gamespeed; /* 游戏速度*/int i, key, color;int score = 0; /* 游戏分数*/char cai48H[] ={0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x04, 0x00, 0x18, 0x00, 0x00, 0x00, 0x0E, 0x00,0x1C, 0x00, 0x00, 0x00, 0x1C, 0x00, 0x1C, 0x00,0x00, 0x00, 0x20, 0x00, 0x38, 0x00, 0x00, 0x00,0x40, 0x00, 0x78, 0x00, 0x00, 0x01, 0x80, 0x40,0x70, 0x00, 0x00, 0x03, 0x80, 0xC0, 0xE0, 0x00,0x00, 0x07, 0x80, 0x80, 0xC0, 0x00, 0x00, 0x0E,0x11, 0x81, 0xC0, 0x00, 0x00, 0x08, 0x61, 0x01,0x80, 0x00, 0x00, 0x00, 0x23, 0x03, 0x04, 0x00,0x00, 0x02, 0x02, 0x00, 0x06, 0x00, 0x00, 0x1E,0x04, 0x00, 0x0F, 0x00, 0x00, 0x1C, 0x1F, 0x80,0x1E, 0x00, 0x00, 0x08, 0x3F, 0x80, 0x3C, 0x00,0x00, 0x00, 0xFF, 0x80, 0x38, 0x00, 0x00, 0x03,0xFF, 0x80, 0x78, 0x00, 0x00, 0x0F, 0xF8, 0x00,0xF0, 0x00, 0x00, 0x7F, 0xF0, 0x00, 0xE0, 0x00,0x03, 0xFF, 0xFC, 0x01, 0x80, 0x00, 0x03, 0xC0,0x03, 0x80, 0x00, 0x01, 0x3F, 0x00, 0x07, 0x80, 0x00, 0x02, 0x11, 0x00, 0x07, 0x00, 0x00, 0x00, 0x10, 0x00, 0x07, 0x00, 0x00, 0x00, 0x10, 0x00, 0x0E, 0x00, 0x00, 0x08, 0x10, 0x00, 0x1C, 0x00, 0x00, 0x30, 0x10, 0x00, 0x18, 0x00, 0x00, 0x70, 0x10, 0x00, 0x30, 0x00, 0x01, 0xE0, 0x10, 0x00, 0x70, 0x00, 0x03, 0x80, 0x10, 0x00, 0x60, 0x00, 0x00, 0x00, 0x30, 0x00, 0xE0, 0x00, 0x00, 0x00, 0xF0, 0x01, 0xC0, 0x00, 0x00, 0x00, 0x70, 0x03, 0xC0, 0x00, 0x00, 0x00, 0x10, 0x07, 0x80, 0x00, 0x00, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1E, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3C, 0x00, 0x00, 0x00, 0x00, 0x00, 0x70, 0x00, 0x00, 0x00, 0x00, 0x01, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };char she48H[] ={0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x00, 0x03, 0x00, 0x07, 0x00, 0x00, 0x00, 0x02, 0x00, 0x03, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x02, 0x00, 0x07, 0x86, 0x00, 0x00, 0x02, 0x00, 0x18, 0x03, 0x00, 0x00, 0x02, 0x00, 0x00, 0x07, 0x80, 0x00, 0x03, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x0F, 0xFC, 0x00, 0x0C, 0x00, 0x00, 0x7E, 0x3F, 0x80, 0x00, 0x00, 0x01, 0xFE, 0x1F, 0x80, 0x00, 0x00, 0x01, 0xE2, 0x39, 0x8C, 0x00, 0x00, 0x00, 0xC2, 0x30, 0x08, 0x00, 0x00, 0x00, 0xC2, 0x60, 0x08, 0x00, 0x00, 0x00, 0xC3, 0xE0, 0x08, 0x60, 0x00, 0x00, 0x7F, 0xE0, 0x01, 0xE0, 0x00, 0x00, 0x3F, 0x80, 0x1F, 0xE0, 0x00, 0x00, 0x1E, 0x00, 0x1F, 0x80, 0x00, 0x00, 0x1E, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x02, 0x38, 0x1E, 0x00, 0x00, 0x00, 0x07, 0xFC, 0x1C,0x00, 0x1F, 0x0C, 0x10, 0x00, 0x20, 0x00, 0x7C, 0x04, 0x10, 0x00, 0x60, 0x01, 0xF0, 0x00, 0x10, 0x00, 0x60, 0x01, 0xE0, 0x00, 0x08, 0x00, 0xF0, 0x00, 0x80, 0x00, 0x08, 0x03, 0xF0, 0x00, 0x00, 0x00, 0x07, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x07, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };char tun48H[] ={0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3E, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x00, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x00, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x40, 0x00, 0x00, 0x00, 0x06, 0x07, 0xC0, 0x00, 0x00, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x0F, 0xFF, 0x80, 0x00, 0x00, 0x00, 0x7F, 0xF8, 0x00, 0x00, 0x00, 0x1F, 0xFF, 0xF8, 0x00, 0x00, 0x00, 0x1F, 0xFF, 0xF8, 0x00, 0x00, 0x00, 0x1F, 0xFC, 0x3C, 0x00, 0x00, 0x00, 0x0F, 0xF8, 0x0E, 0x00, 0x00, 0x00, 0x04, 0x70, 0x07, 0x00, 0x00, 0x00, 0x00, 0x60, 0x03, 0x80, 0x00, 0x00, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0x00, 0x01, 0x80, 0x00, 0x30, 0x00, 0x00, 0x01, 0x00, 0x3C, 0x18, 0x00, 0x00, 0x02, 0x03, 0xFF, 0x0C, 0x00, 0x00, 0x0C, 0x7F, 0xFF, 0x8E, 0x00, 0x00, 0x18, 0xFF, 0xFF, 0xC7, 0x80, 0x00, 0x78, 0xFE, 0x07, 0x87, 0xE0, 0x01, 0xF0, 0x70, 0x07, 0x03, 0xF8, 0x07, 0xE0, 0x70, 0x0E, 0x03, 0xFE, 0x00, 0x00, 0x38, 0x1E, 0x01, 0xFE, 0x00, 0x00, 0x3F, 0xFE, 0x00, 0x0C, 0x00, 0x00, 0x1F, 0xFE, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };char dan48H[] ={0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFC, 0x00, 0x00, 0x00, 0x00, 0x07, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xC0, 0x80, 0x00, 0x00, 0x03, 0xFF, 0x80, 0x40, 0x00, 0x00, 0x01, 0xF1, 0x80, 0x40, 0x00, 0x00, 0x01, 0x81, 0x80, 0xE0, 0x00, 0x00, 0x00, 0x01, 0x93, 0xF0, 0x00, 0x00, 0x00, 0x01, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x21, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x21, 0xF8, 0x00, 0x00, 0x00, 0x00, 0x61, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x61, 0x80, 0x00, 0x00, 0x00, 0x00, 0xF3, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x01, 0xFF, 0xC0, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xF8, 0x00, 0x00, 0x00, 0x02, 0x00, 0xFC, 0x00, 0x00, 0x00, 0x04, 0x02, 0x1F, 0x00, 0x00, 0x00, 0x08, 0x03, 0x01, 0xC0, 0x00, 0x00, 0x38, 0x03, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x07, 0xF8, 0x3F, 0xC0, 0x01, 0xF0, 0x3F, 0xFE, 0x3F, 0xF8, 0x03, 0xC1, 0xFF, 0x0F, 0x1F, 0xF8, 0x00, 0x01, 0xE3, 0x0F, 0x0F, 0xF0, 0x00, 0x01, 0xC3, 0x0E, 0x00, 0x00, 0x00, 0x01, 0x83, 0xFC, 0x00, 0x00, 0x00, 0x00, 0xC7, 0xF8, 0x00, 0x00, 0x00, 0x00, 0xFF, 0xF8, 0x00, 0x00, 0x00, 0x00, 0x7F, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x3F, 0x03, 0x80, 0x00, 0x00, 0x00, 0x03, 0x04, 0x00, 0x00, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x00, 0x00, 0x1F, 0xF8, 0x20, 0x00, 0x00, 0x00, 0xFF, 0xFF, 0xE0, 0x00, 0x00, 0x07, 0xFF, 0x81, 0xE0, 0x00, 0x00, 0x07, 0xE0, 0x00, 0xE0, 0x00, 0x00, 0x03, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };char zuo16H[] ={0x18, 0xC0, 0x18, 0xC0, 0x19, 0x80, 0x31, 0xFE, 0x33, 0xFE, 0x76, 0xC0, 0xF0, 0xFC, 0xB0, 0xFC, 0x30, 0xC0, 0x30, 0xC0, 0x30, 0xFE, 0x30, 0xFE, 0x30, 0xC0, 0x30, 0xC0, 0x30, 0xC0, 0x00, 0x00, };char zhe16H[] ={0x03, 0x00, 0x03, 0x0C, 0x1F, 0xCC, 0x1F, 0xD8, 0x03, 0x30, 0xFF, 0xFE, 0xFF, 0xFE, 0x03, 0x00, 0x0F, 0xF8, 0x3F, 0xF8, 0xEC, 0x18, 0xCF, 0xF8, 0x0C, 0x18, 0x0F, 0xF8, 0x0F, 0xF8, 0x00, 0x00, };char tian16H[] ={0x00, 0x00, 0x3F, 0xFC, 0x3F, 0xFC, 0x31, 0x8C, 0x31, 0x8C, 0x31, 0x8C, 0x3F, 0xFC, 0x3F, 0xFC, 0x31, 0x8C, 0x31, 0x8C, 0x31, 0x8C, 0x3F, 0xFC, 0x3F, 0xFC, 0x30, 0x0C, 0x00, 0x00, 0x00, 0x00, };char xue16H[] ={0x33, 0x18, 0x19, 0x98, 0x08, 0xB0, 0x7F, 0xFC, 0x7F, 0xFC, 0x60, 0x0C, 0x1F, 0xF0, 0x1F, 0xF0, 0x00, 0xC0, 0x7F, 0xFC, 0x7F, 0xFC, 0x01, 0x80, 0x01, 0x80, 0x07, 0x80, 0x03, 0x00, 0x00, 0x00, };char ke16H[] ={0x00, 0x00, 0x0C, 0x18, 0xFD, 0x98, 0xF8, 0xD8, 0x18, 0x58, 0xFE, 0x18, 0xFE, 0x98, 0x18, 0xD8, 0x3C, 0x58, 0x7E, 0x1E, 0xDB, 0xFE, 0x9B, 0xF8, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x00, 0x00, };struct Food/*定义结构体存储食物的属性*/{int x; /* 食物的坐标*/int y;int yes; /* 值为0表示屏幕上没有食物,值为1表示屏幕上有食物*/ int color; /* 食物颜色*/} food;struct Snake/*定义结构体存储蛇的属性*/{int x[N]; /* 每一节蛇的坐标*/int y[N];int color[N];/*存储每一节蛇的颜色*/int node; /* 蛇的节数*/int direction; /* 蛇移动的方向*/int life; /* 蛇的生命,如果为1,蛇死,游戏结束*/} snake;void init(void)/*图形驱动*/{int driver = DETECT, mode = 0;registerbgidriver(EGAVGA_driver);initgraph(&driver, &mode, "");}void drawmat(char *mat, int matsize, int x, int y, int color) /*汉字点阵*/ {int i, j, k, m;m = (matsize - 1) / 8 + 1;for(j = 0; j < matsize; j++)for(i = 0; i < m; i++)for(k = 0; k < 8; k++)if(mat[j*m+i]&(0x80 >> k))putpixel(x + i * 8 + k, y + j, color);}void showword(void){/* 调用汉字点阵输出程序,显示标题和作者信息*/drawmat(cai48H, 48, 249, -4, 7);drawmat(she48H, 48, 329, -4, 7);drawmat(tun48H, 48, 409, -4, 7);drawmat(dan48H, 48, 489, -4, 7);drawmat(cai48H, 48, 250, -5, 4);drawmat(she48H, 48, 330, -5, 4);drawmat(tun48H, 48, 410, -5, 4);drawmat(dan48H, 48, 490, -5, 4);/*作者田学科*/drawmat(zuo16H, 16, 515, 465, 7);drawmat(zhe16H, 16, 530, 465, 7);drawmat(tian16H, 16, 550, 465, 7);drawmat(xue16H, 16, 565, 465, 7);drawmat(ke16H, 16, 580, 465, 7); }void draw(void)/*画出四周的墙*/ {if(color == 15)color = 0;setcolor(++color);setlinestyle(SOLID_LINE, 0, 1);for(i = 30; i <= 600; i += 10){rectangle(i, 40, i + 10, 49);rectangle(i, 451, i + 10, 460);}for(i = 40; i < 450; i += 10){rectangle(30, i, 39, i + 10);rectangle(601, i, 610, i + 10);}}void prscore(void){/* 打印游戏分数*/char str[10];setfillstyle(SOLID_FILL, YELLOW);bar(50, 10, 200, 30);setcolor(6);settextstyle(0, 0, 2);sprintf(str, "score:%d", score);outtextxy(55, 15, str);}void gameover(void){cleardevice(); /* 清屏函数*/for(i = 0; i < snake.node; i++) /* 画出蛇死时的位置*/{setcolor(snake.color[i]);rectangle(snake.x[i], snake.y[i], snake.x[i] + 10, snake.y[i] + 10);}prscore(); /* 显示分数*/draw();showword();settextstyle(0, 0, 6);setcolor(7);outtextxy(103, 203, "GAME OVER");setcolor(RED);outtextxy(100, 200, "GAME OVER");}void gameplay(void)/* 玩游戏的具体过程*/{int flag, flag1;randomize();prscore();gamespeed = 50000;food.yes = 0; /* food.yes=0表示屏幕上没有食物*/snake.life = 1; /* snake.life=1表示蛇是活着的*/snake.direction = 4; /* 表示蛇的初始方向为向右*/snake.node = 2; /* 蛇的初始化为两节*/snake.color[0] = 2; /*两节蛇头初始化为绿色*/snake.color[1] = 2;snake.x[0] = 100;snake.y[0] = 100;snake.x[1] = 110;snake.y[1] = 100;food.color = random(15) + 1;while(1){while(1){if(food.yes == 0) /* 如果蛇活着*/{while(1){flag = 1;food.x = random(56) * 10 + 40;food.y = random(40) * 10 + 50;for(i = 0; i < snake.node; i++){if(food.x == snake.x[i] && food.y == snake.y[i])flag = 0;}if(flag) break;}}if(food.yes){setcolor(food.color);rectangle(food.x, food.y, food.x + 10, food.y + 10);}for(i = snake.node - 1; i > 0; i--){snake.x[i] = snake.x[i-1];snake.y[i] = snake.y[i-1];}switch(snake.direction){case 1:snake.y[0] -= 10;break;case 2:snake.y[0] += 10;break;case 3:snake.x[0] -= 10;break;case 4:snake.x[0] += 10;break;}for(i = 3; i < snake.node; i++){if(snake.x[i] == snake.x[0] && snake.y[i] == snake.y[0]) {gameover();break;}}if(snake.x[0] < 40 || snake.x[0] > 590 || snake.y[0] < 50 || snake.y[0] > 440) {gameover();snake.life = 0;}if(snake.life == 0)break;if(snake.x[0] == food.x && snake.y[0] == food.y) /*蛇吃掉食物*/{setcolor(0);rectangle(food.x, food.y, food.x + 10, food.y + 10);snake.x[snake.node] = -20;snake.y[snake.node] = -20;snake.color[snake.node] = food.color;snake.node++;food.yes = 0;food.color = random(15) + 1;score += 10;prscore();if(score % 100 == 0 && score != 0){for(i = 0; i < snake.node; i++) /* 画出蛇*/{setcolor(snake.color[i]);rectangle(snake.x[i], snake.y[i], snake.x[i] + 10, snake.y[i] + 10);}sound(200);delay(50000);delay(50000);delay(50000);delay(50000);delay(50000);delay(50000);nosound();gamespeed -= 5000;draw();}else{sound(500);delay(500);nosound();}}for(i = 0; i < snake.node; i++) /* 画出蛇*/{setcolor(snake.color[i]);rectangle(snake.x[i], snake.y[i], snake.x[i] + 10, snake.y[i] + 10);}delay(gamespeed);delay(gamespeed);flag1 = 1;setcolor(0);rectangle(snake.x[snake.node-1], snake.y[snake.node-1],snake.x[snake.node-1] + 10, snake.y[snake.node-1] + 10);if(kbhit() && flag1 == 1) /*如果没按有效键就重新开始循环*/{flag1 = 0;key = bioskey(0);if(key == esc)exit(0);else if(key == up && snake.direction != 2)snake.direction = 1;else if(key == down && snake.direction != 1)snake.direction = 2;else if(key == left && snake.direction != 4)snake.direction = 3;else if(key == right && snake.direction != 3)snake.direction = 4;}}if(snake.life == 0) /*如果蛇死了就退出循环*/break;}}void main(void){while(1){color = 0;init();cleardevice();showword();draw();gameplay();setcolor(15);settextstyle(0, 0, 2);outtextxy(200, 400, "CONTINUE(Y/N)?");while(1){key = bioskey(0);if(key == Y || key == n || key == esc)break;}if(key == n || key == esc)break;}closegraph();}3、c语言实现移动电话系统#include <stdio.h>#define GRID-SIZE 5#define SELECTED -1 /*低于矩阵中所有元素*/#define TRAFFIC-FILE “traffic.dat”/*关于交通数据的文件*/#define NUM-TRANSMITTERS 10 /*可用的发射器数量*/void get-traffic-data(int commuters[GRID-SIZE][GRID-SIZE],int salesforce[GRID-SIZE][GRID-SIZE],int weekends [GRID-SIZE][GRID-SIZE];voide print-matrix[GRID-SIZE][GRID-SIZE];intmain(void){int commuters[GRID-SIZE][GRID-SIZE];/*上午8:30的交通数据*/int salesforce[GRID-SIZE][GRID-SIZE]; /*上午11:00的交通数据*/int weekend[GRID-SIZE][GRID-SIZE];/*周末交通数据*/int commuter-weight, /*通勤人员数据的权重因子*/sale-weight,/*营销人员数据的权重因子*/weekend-weight;/*周末数据的权重因子*/int location-i,/*每个发射器的位置*/location-j;int current-max;/*和数据中当前的最大值*/int i,j,/*矩阵的循环计数器*/tr;/*发射器的循环计数器*//*填入并显示交通数据*/Get-traffic-data (commuters,salesforce,weekend);Printf(“8:30 TRAFFIC DATA 、\n\n”)print-matrix(commuters);printf(“\n\n WEEKEND TRAFFIC DATA\n\n”);print-matrix(salesforce);printf(“\n\n WEEKEND TRAFFIC DATA\n\n”);printf_matrix(weekeng);/*请用户输入权重因子*/printf(“\n\nPlease input the following value:\n”);printf(“Weight (an interger>=0) for the 8:30 data>”)scanf(“%d”,&commuter_weight);printf(“weight(an integer>=0) for the weekeng data>”);scanf(“%d”,&weekend_weight);scanf(“%d”,&weekend_weight);/*计算并显示加权后求和的数据*/for (i=0;i<GRID_SIZE;++i)for (j=0;j<GRID_SIZE;++j)summed_data[i][j]=commuter_weight*commuter[i][j]+salesforce_weight*salesforce[i][j]+weekend_weight*weekend[i][j];printf(“\n\nThe weighted,summed data is :\n\n”);printf_matrix(summed_data);/*在summed_data矩阵中找出NUM_TRANSMITTERS个最大值,将坐标临时存储在location_i和location_j中,然后把最后的结果坐标输出*/printf(“\n\nLocations of the %d transmitters:\n\n”,NUM_TRANSMITTERS);for (tr=1;tr<=NUM_TRANSMITTERS;++tr){current_max=SELECTED;/*以一个过低的值为起点开始查找*/for (i=0;i<GRID_SIZE;++i){for(j=0;j<GRID_SIZE;++j){if(current_max<summed_data[i][j]){current_max=summed_data[i][j]){location_i=i;location_j=j;}}}/*将选中的单元赋一较低的值以避免下次再选中这一元素,显示查找结果*/ summed_data[location_i][location_j]=SELECTED;printf(“Transmitter %3d:at location %3d %3d\n”,tr,location_i,location_j);}return (0);}/**把TRAFFIC_FILE中的交通数据填充到3个GRID_SIZE×GRID_SIZE数组中*/voidget_traffic_data(int commuters[GRID_SIZE],/*输出*/int salesforce[GRID_SIZE][GRID_SIZE],/*输出*/int weekend[GRID_SIZE][GRID_SIZE],/*输出*/{int i,j; /*循环计数器*/FILE *fp; /*文件指针*/fq=fopen(TRAFFIC_FILE,“r”);for(i=0;i<GRID_SIZE;++i)for(j=0;j<GRID_SIZE;++j)fscanf(fp,“%d”,&commnters[i][j];for(i=0;i<GRID_SIZE;++j)for(j=0;j<GRID_SIZE;++j)fscanf(fq,“%d”,&weekend[i][j]);fclose(fq);}/**显示一个GRID_SIZE×GRID_SIZE整数矩阵的内容*/voidprint_matrix(int matrix[GRID_SIZE][GRID_SIZE]){int i,j; /*循环计数器*/for(i=0;i<GRID_SIZE;++j){ for(j=0;j<GRID_SIZE;++J)printf(“%3d”,matrix[i][j]);printf(“\n”);}}4、扑克牌游戏/*************************************Copyright(C) 2004-2005 vision,math,NJU.File Name: guess_card.cppAuthor: vision Version: 1.0 Data: 23-2-2004Description: 给你9张牌,然后让你在心中记住那张牌,然后电脑分组让你猜你记住的牌在第几组,然后猜出你记住的那张牌.Other: 出自儿童时的一个小魔术History:修改历史**************************************/#include <stdio.h>#include <stdlib.h>#include <string.h>#include <time.h>#include <assert.h>#define CARDSIZE 52 /*牌的总张数*/#define SUITSIZE 13 /*一色牌的张数*//*扑克牌结构*/typedef struct Card{char val;/*扑克牌面上的大小*/int kind :4; /*扑克牌的花色*/}Card;/*************************************************Function: // riffleDescription: // 洗牌,然后随机的得到9张牌,要求九张牌不能有重复. Calls: //Called By: // main()Table Accessed: //被修改的表(此项仅对于牵扯到数据库操作的程序)Table Updated: // 被修改的表(此项仅对于牵扯到数据库操作的程序)Input: //Card card[] 牌结构, int size 结构数组的大小Output: //Return: // voidOthers: // 此函数修改card[]的值,希望得到九张随机牌Bug: //此函数有bug,有时会产生两个相同的牌,有待修订*************************************************/void riffle(Card *cards, int size);/*************************************************Function: // showDescription: // 显示数组的内容Calls: //Called By: // main()Table Accessed: //被修改的表(此项仅对于牵扯到数据库操作的程序)Table Updated: // 被修改的表(此项仅对于牵扯到数据库操作的程序)Input: //Card *card 牌结构指针, int size 结构数组的大小Output: //Return: // voidOthers: //*************************************************/void show(const Card *cards, int size);/*************************************************Function: // groupingDescription: //把9张牌分别放到3个数组中,每组3张,a.e分组Calls: //Called By: // main()Table Accessed: //被修改的表(此项仅对于牵扯到数据库操作的程序)Table Updated: // 被修改的表(此项仅对于牵扯到数据库操作的程序)Input: //Card *card 牌结构指针, int size 结构数组的大小Output: //Return: // voidOthers: // 此函数修改*carr1,*carr2,* carr3的值*************************************************/void grouping(const Card *cards, Card *carr1, Card *carr2, Card *carr3);/*************************************************Function: // result_processDescription: //用递归计算,所选的牌Calls: // rshiftCalled By: // main()Table Accessed: //被修改的表(此项仅对于牵扯到数据库操作的程序)Table Updated: // 被修改的表(此项仅对于牵扯到数据库操作的程序)Input: //Card *carr1, Card *carr2, Card *carr3Output: //Return: // voidOthers: // 此函数修改*carr1,*carr2,* carr3的值*************************************************/Card* result_process(Card *carr1, Card *carr2, Card *carr3, int counter);/*************************************************Function: // rshiftDescription: //右移操作Calls: //Called By: // result_processTable Accessed: //被修改的表(此项仅对于牵扯到数据库操作的程序)Table Updated: // 被修改的表(此项仅对于牵扯到数据库操作的程序)Input: //Card *carr1, Card *carr2, Card *carr3 ,int counterOutput: //Return: // Card*Others: // 此函数修改*carr1,*carr2,* carr3的值*************************************************/void rshift(Card *carr1, Card *carr2, Card *carr3, int counter);void main(){Card cards[9]; /*存放九张牌*/Card carr1[3]; /*第一组牌,cards array 1*/Card carr2[3]; /*第二组牌,cards array 2*/Card carr3[3]; /*第三组牌,cards array 3*/int select = 0; /*玩家的选择*/Card *selected_card;/*存放玩家所记住(选)的牌*/int counter = 0;riffle(cards, 9); /*洗牌,得到九张牌*/puts("请记住一张牌千万别告诉我!最多经过下面三次我与你的对话,我就会知道你所记的那张牌!");puts("如果想继续玩,请准确的回答我问你的问题,根据提示回答!");puts("请放心,我不会问你你选了哪张牌的!");grouping(cards, carr1, carr2, carr3); /*把9张牌分别放到3个数组中,每组3张,a.e分组*/show(carr1, 3);show(carr2, 3);show(carr3, 3);puts("请告诉我你记住的那张牌所在行数");select = getchar();switch(select)/*分支猜你玩家记住的牌*/{case '1':selected_card = result_process(carr1, carr2, carr3, counter);break;case '2':selected_card = result_process(carr2, carr3, carr1, counter);break;case '3':selected_card = result_process(carr3, carr1, carr2, counter);break;default:puts("你在撒谎!不和你玩了!");fflush(stdin);getchar();exit(0);}if( selected_card ==NULL){fflush(stdin);getchar();exit(0);}puts("你猜的牌为:");show(selected_card, 1);puts("我猜的对吧,哈哈~~~~");fflush(stdin);getchar();}/*riffle的原代码*/void riffle(Card *cards, int size){char deck[CARDSIZE];/*临时数组,用于存储牌*/ unsigned int seed;/*最为产生随机数的种的*/int deckp = 0; /*在牌的产生中起着指示作用*/seed = (unsigned int)time(NULL);srand(seed);/*洗牌*/while (deckp < CARDSIZE){char num = rand() % CARDSIZE;if ((memchr(deck, num, deckp)) == 0){assert(!memchr(deck,num,deckp));deck[deckp] = num;deckp++;}}/*找9张牌给card*/for (deckp = 0; deckp < size; deckp++){div_t card = div(deck[deckp], SUITSIZE);cards[deckp].val = "ATJQK"[card.rem]; /*把余数给card.val*/ cards[deckp].kind = "3456"[card.quot]; /*把商给card.kind*/ }}/*show的原代码,将会自动换行*/void show(const Card *cards, int size){for(int i = 0; i < size; i++){printf("%c%c ",cards[i].kind,cards[i].val);if( (i !=0) && (((i+1 ) % 3) == 0))puts("");}puts(""); /*自动换行*/}/*grouping 的原代码*/void grouping(const Card *cards, Card *carr1, Card *carr2, Card *carr3) {int i = 0;/*循环参数*//*分给carr1三个数*/while (i < 3){carr1[i].val = cards[i].val;carr1[i].kind = cards[i].kind;i++;}/*分给carr2接下来的三个数*/while (i < 6){carr2[i-3].val = cards[i].val;carr2[i-3].kind = cards[i].kind;i++;}/*分给carr3接下来的三个数*/while (i < 9){carr3[i-6].val = cards[i].val;carr3[i-6].kind = cards[i].kind;i++;}}/*rshift的实现*/void rshift(Card *carr1, Card *carr2, Card *carr3, int counter){Card temp2;/*用于存放carr2[counter]*/Card temp3;/*用于存放carr3[counter]*//*temp = carr2*/temp2.val = carr2[counter].val;temp2.kind = carr2[counter].kind;/*carr2 = carr1*/carr2[counter].val = carr1[counter].val;carr2[counter].kind = carr1[counter].kind;/*temp3 = carr3*/temp3.val = carr3[counter].val;temp3.kind = carr3[counter].kind;/*carr3 = carr2*/carr3[counter].val = temp2.val;carr3[counter].kind = temp2.kind;/*carr1 = carr3*/carr1[counter].val = temp3.val;carr1[counter].kind = temp3.kind;}Card* result_process(Card *carr1, Card *carr2, Card *carr3, int counter){rshift(carr1, carr2, carr3, counter); /* 把数组的第一个元素依次右移*/if(counter == 2){return(&carr2[2]);}show(carr1, 3);show(carr2, 3);show(carr3, 3);puts("请给出你记住的牌所在行数:");fflush(stdin);int input = 1;input = getchar(); /*获取你选的组*/switch(input){case '1':return(result_process(carr1, carr2, carr3, ++counter));break;case '2':return(&carr2[counter]);break;default:puts("你在撒谎!不和你玩了!");return NULL;}}5、C语言实现打字游戏#include "stdio.h"#include "time.h"#include "stdlib.h"#include "conio.h"#include "dos.h"#define xLine 70#define yLine 20#define full 100#define true 1#define false 0/*---------------------------------------------------------------------*/void printScreen(int level,int right,int sum,char p[yLine][xLine])/* 刷新屏幕的输出图像 */{int i,j;clrscr();printf("level:%d Press 0 to exit;1 topause score:%d/%d\n",level,right,sum);/* 输出现在的等级,击中数和现在已下落总数 */printf("----------------------------------------------------------------------\n");for (i=0;i<yLine;i++){for(j=0;j<xLine;j++)printf ("%c",p[i][j]);printf("\n");}/* for (i) */printf("----------------------------------------------------------------------\n");}/* printScreen *//*---------------------------------------------------------------------*/void leave()/* 离开程序时,调用该函数结束程序。
C语言小游戏源代码
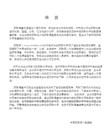
不管怎么样,九格游戏最后的结果只有两个:
123 | 123
456 | 456
78 | 87 (证明从略)
而要两两交换而始终有解的话,(从原序列开始)必须:相邻交换的次数为偶
但我们用一种更有效的方法:
每一个总与其下第二个交换.
第7,8个与0,1交换,只要交换次数多,仍可获得相同的效果.
}
}
int isSuccess(){ /*判断是否游戏*/
int i,ret=1;
for(i=0;i<8;i++)
ret=ret&&(num[i]==(i+1));
return ret;
}
int GetTheNull(){ /*获得空格的位置*/
if (num[j+i*3]!=0)
printf("\324\315\315\315\315\274");
else
printf(" ");
}
printf("\n");
把光标移动到屏幕的x(1~80),y(1~25/50)处*/
/*和clrscr():清屏*/
int num[]={1,2,3,4,5,6,7,8,0}; /*方块的数字*/
main(){
char key=0; /*键盘码*/
int pos; /*九格中,空格的位置*/
a=random(8); /*产生随机数*/
b=(a+2)%8; /*得到下第二个的数组下标*/
change(a,b); /*交换*/
}
纯C语言写的一个小型游戏-源代码
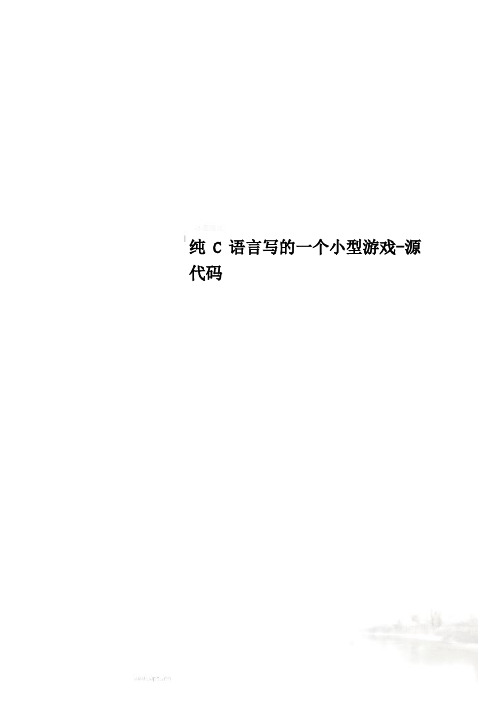
纯C语言写的一个小型游戏-源代码/* A simple game*//*CopyRight: Guanlin*/#include<stdio.h>#include<stdlib.h>#include<string.h>#include<time.h>#include<conio.h>#include<process.h>struct object_fix{char name[20];char id[5];char desc[500];char action[30];char im[5];};struct object_move{char name[20];char id[5];char desc[500];int loc;int pwr;int strg;char im[5];};struct rover{char name[20];char id[5];char desc[500];int pwr;int strg;int location[2];char im[5];};struct map /* this is the map structure*/{char data[20];char add_data[20];int amount;int x; /* this were the successor keeps it's x & y values*/ int y;};struct location /*this structure is for the successor lister*/ {float height;char obj;{int selection;switch(object){case 1:printf("\nYou have encountered: A Sandy Rock\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\nPlease choose action 1 or 2:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 2:printf("\nYou have encountered: A Solid Rock\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 3:printf("\nYou have encountered: A Mountain\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 4:printf("\nYou have encountered: Dust\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 5:printf("\nYou have encountered: A Sheer Valley\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 6:printf("\nYou have encountered: A Gentle Valley\n\n");printf("This object can be:\n1.\tDriven through\n2.\tAvoided\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;case 7:printf("\nYou have encountered: A 'Martian' Tree\n\n");printf("This object canbe:\n1.\tDestroyed\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 8:printf("\nYou have encountered: Shallow Water\n\n");printf("This object can be:\n1.\tDriventhrough\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t"); scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 9:printf("\nYou have encountered: Deep Water\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 10:printf("\nYou have encountered: An Aggressive Alien\n\n");printf("This object can be:\n1.\tDestroyed\nPlease enter 1:\t");scanf("%d", &selection);selection = 1;stats_update(selection, p_rover);break;case 11:printf("\nYou have encountered: A Non-Aggressive Alien\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 12:printf("\nYou have encountered: Another Rover\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 13:printf("\nYou have encountered: A Power Station\n\n");printf("You can:\n1.\tCharge up\n2.\tAvoid\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 5;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;default:printf("\n\n*****ERROR*****\n\n");break;}}void show_map(struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr, struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /*the show map function calling number and number_2 from the map structure to see if moveable objects are needed*/{struct map f_map[8][8]; /*8 by 8 map*/int i,j, rx, ry, object; /*this is your x and y value in your map (f_map)*/system("cls"); /* this is your x and y value in your map (f_map)*///srand(time(NULL)); /*calling the time from include to gather random variables*/for(i=0;i<8;i++) /*for loop to copy all your fixed object lists into f_map so they can be displayed*/{for(j=0;j<8;j++)strcpy(f_map[i][j].data,"");}if (number->amount>0) /*this is looking at the add function to see weather or not there are aliens in f_map*/{for(i=0;i<number->amount;i++) /*allocating the number of aliens in f_map*/ strcpy(f_map[rand()%8][rand()%8].data, anon->im); /*randomizing their position*/}if(number_2->amount>0) /*repeat of above just for rovers instead*/{for(i=0;i<number_2->amount;i++)strcpy(f_map[rand()%8][rand()%8].data, rvr->im);}rx= p_rover->location[0];ry= p_rover->location[1];strcpy(f_map[1][0].data, wdp->im);strcpy(f_map[4][0].data, mnt->im);strcpy(f_map[5][0].data, mnt->im);strcpy(f_map[3][1].data, dst->im);strcpy(f_map[4][1].data, dst->im);strcpy(f_map[3][2].data, dst->im);strcpy(f_map[4][2].data, dst->im);strcpy(f_map[0][3].data, mnt->im);strcpy(f_map[1][3].data, mnt->im);strcpy(f_map[3][3].data, vshr->im);strcpy(f_map[4][3].data, dst->im);strcpy(f_map[5][3].data, vgnt->im);strcpy(f_map[3][4].data, vshr->im);strcpy(f_map[4][4].data, dst->im);strcpy(f_map[5][4].data, vgnt->im);strcpy(f_map[2][5].data, wshl->im);strcpy(f_map[3][5].data, wshl->im);strcpy(f_map[4][5].data, wshl->im);strcpy(f_map[1][6].data, pstn->im);strcpy(f_map[2][6].data, wdp->im);strcpy(f_map[3][6].data, wdp->im);strcpy(f_map[4][6].data, wshl->im);strcpy(f_map[7][6].data, mnt->im);strcpy(f_map[0][7].data, mnt->im);strcpy(f_map[1][7].data, wdp->im);strcpy(f_map[2][7].data, wshl->im);strcpy(f_map[3][7].data, wshl->im);strcpy(f_map[6][7].data, mnt->im);strcpy(f_map[rx][ry].data, p_rover->im);if((rx == 1 && ry == 0) || (rx == 2 && ry == 6) ||(rx == 3 && ry == 6) ||(rx == 1 && ry == 7)){object = 9;action(object, p_rover);}else if((rx == 4 && ry == 0) || (rx == 5 && ry == 0) || (rx == 0 && ry == 3) || (rx == 1 && ry == 3) || (rx == 7 && ry == 6) || (rx == 0 && ry == 7) || (rx == 6 && ry == 7)){object = 3;action(object, p_rover);}else if((rx == 3 && ry== 1) || (rx == 4 && ry == 1) || (rx == 3 && ry== 2) || (rx == 4 && ry == 2) || (rx == 4 && ry == 3) || (rx == 4 && ry == 4)){object = 4;action(object, p_rover);}else if((rx == 3 && ry == 3) || (rx == 3 && ry == 4)){object = 5;action(object, p_rover);}else if((rx == 5 && ry == 3) || (rx == 5 && ry == 4)){object = 6;action(object, p_rover);}else if((rx == 2 && ry == 5) || (rx == 3 && ry == 5 ) || (rx == 4 && ry == 5) || (rx == 4 && ry == 6) ||(rx == 2 && ry == 7) || (rx == 3 && ry == 7)){object = 8;action(object, p_rover);}else if(rx == 1 && ry == 6){object = 13;action(object, p_rover);}i=0; /*re-allocate i to 0 so map is printed from start*/for(i=0;i<8;i++) /*8 by 8 map*/{printf("+----+----+----+----+----+----+----+----+\n");for(j=0;j<8;j++) /*8 by 8 map*/{if(strlen(f_map[i][j].data)!=0) /*if function to print nothing but 4 spaces if there in nothing allocated in [i][j]*/printf("|%4s",f_map[i][j].data);elseprintf("| "); /*end of coloumn visible map*/}printf("|\n"); /*end of last coloumn visible map*/}printf("+----+----+----+----+----+----+----+----+\n"); /*bottom of map*/printf("\n");printf("\270");printf("Group B\n");}void add_obj(struct map *number, struct map *number_2, struct object_fix*rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /* add movable object function, *number=aliens,*number_2=rovers*/{int object, t, f;char alien;char rover;printf("This is the add movable object function\n");printf("how many aliens would you like?\n");scanf("%d",&t); /*user input of amount of aliens*/number->amount=t; /*saving the number in structure map-amount*/printf("how many rovers would you like?\n");scanf("%d",&f); /*user input of amount of rovers*/number_2->amount=f; /*saving the number in structure map-amount*/show_map(number, number_2, rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover); /*go to show map function with the number of aliens and number of rovers*/}void successor() /*this functions askes the user for the location and then were they want to go from there, printing out that location*/{struct map location;int menu;int ncol,nrow; /*we can change the n value depends how large the map you need.*/ncol=8;nrow=8;printf("This is the successor function\n");printf("Enter the \nx=\ty=\n");scanf("%d%d",&location.x,&location.y); /*saving location in map location x and y*/printf("Enter operator choice\n");printf("1=left\n");printf("2=right\n");printf("3=upwards\n");printf("4=down\n");printf("5=quit\n");scanf("%d",&menu);switch(menu) /*menu switch to show the position above, underneith, and next to the rover*/{case 1:location.x=location.x-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 2:location.x=location.x+1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 3:location.y=location.y-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 4:location.y=location.y+1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 5:printf("*****back to program*****\n"); /* Quits the program and prints out the message */break;default:printf("*****Please enter an integer from 1-5*****\n"); /* A default option if the user enters an incorrect value */break;}printf("\n");}void help(struct map *number, struct map *number_2, struct object_fix *rsny, struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr, struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /*help function*/{int i;char mov_obj;printf("++This is the help function++\n");printf("| 1-successor |\n");printf("| 2-add movable obj |\n");printf("| 3-remove moveabla obj |\n\n");printf("\t* * *\n");printf("\t Index: \n\n");printf("\t Sandy Rock: *\n");printf("\t Solid Rock: o\n");printf("\t Water:\262\262\262\262 \n");printf("\t Shallow water: \260\260\260\260\n");printf("\t Mountain: ^^^^\n");printf("\t Dust: ....\n");printf("\t* sher valley: VVVV *\n");printf("\t shallow valey: vvvv\n");printf("\t power station: \025\n");printf("\t alien: *_*\n");printf("\t other rovers: #\n");printf("\t* * *\n");scanf("%d",&i);switch(i) /*switch menu to go to the successor function, or the add/remove function*/{case 1:successor();break;case 2:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);break;case 3:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);break;default:printf("choose a number from the list\n");}}void direction(int input, struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr, struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover){switch(input){case 119: /*w = up*/if(p_rover->location[0] > 0){p_rover->location[0]--;p_rover->pwr--;}else p_rover->location[0]=7;break;case 115: /*s = down*/if(p_rover->location[0] < 7){p_rover->location[0]++;p_rover->pwr--;}else p_rover->location[0]=0;break;case 97: /*a = left*/if(p_rover->location[1] > 0){p_rover->location[1]--;p_rover->pwr--;}else p_rover->location[1]=7;break;case 100: /*d = right*/if(p_rover->location[1] < 7){p_rover->location[1]++;p_rover->pwr--;}else p_rover->location[1]=0;break;default:printf("Invalid operator!\n\n");break;}}int control(int input){input = _getch();return input;}void main(void){int menu;int quit = 0;int input = 0;struct object_fix rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl, wdp;struct object_move aagr, anon, rvr, pstn;struct rover p_rover;struct map number, number_2;number.amount = 0; /* setting the initial amount of aliens as 0*/number_2.amount = 0;strcpy(,"Sandy Rock");strcpy(rsny.id,"RSNY");strcpy(rsny.desc,"A rock made of softer material that can be destroyed or collected");strcpy(rsny.im,"*");strcpy(,"Solid Rock");strcpy(rsld.id,"RSLD");strcpy(rsld.desc,"A rock made of hard material that cannot be destroyed, but can be collected or avoided");strcpy(rsld.im,"O");strcpy(,"Mountain");strcpy(mnt.id,"MNT");strcpy(mnt.desc,"A large obstacle made of different materials that can only be avoided");strcpy(mnt.im,"^^^^");strcpy(,"Dust");strcpy(dst.id,"DST");strcpy(dst.desc,"Small particles of sand, rock, etc. that may reduce visibility or slow down the vehicle, can be driven through but has extra power consumption");strcpy(dst.im,"....");strcpy(,"Sheer Valley");strcpy(vshr.id,"VSHR");strcpy(vshr.desc,"A depression in the land with sheer sides, it cannot be driven through and must be avoided");strcpy(vshr.im,"VVVV");strcpy(,"Gentle Valley");strcpy(vgnt.id,"VGNT");strcpy(vgnt.desc,"A depression in the land with gentle sides, it can be driven through with extra power consumption or can be avoided");strcpy(vgnt.im,"vvvv");strcpy(,"'Martian' Tree");strcpy(mtre.id,"MTRE");strcpy(mtre.desc,"A woody plant native to Mars, it may have the same characteristics as an 'Earth' tree");strcpy(mtre.im,"\330");strcpy(,"Shallow Water");strcpy(wshl.id,"WSHL");strcpy(wshl.desc,"A shallow body of water that can be driven through, can be in the form of a small puddle or a large lake");strcpy(wshl.im,"\260\260\260\260");strcpy(,"Deeper Water");strcpy(wdp.id,"WDP");strcpy(wdp.desc,"A deeper body of water that cannot be driven through, commonly in the form of a large lake or river");strcpy(wdp.im,"\262\262\262\262");strcpy(,"Aggressive Alien");strcpy(aagr.id,"AAGR");strcpy(aagr.desc,"An alien creature that will move upon its own accord, may become aggressive on contact");strcpy(aagr.im,"*_*");strcpy(,"Non-Aggressive Alien");strcpy(anon.id,"ANON");strcpy(anon.desc,"An alien creature that will move upon its own accord, will not become aggressive on contact");strcpy(anon.im,"*_*");strcpy(,"Rover");strcpy(rvr.id,"RVR");strcpy(rvr.desc,"A Mars Rover vehicle to transport around the map, it can destroy, collect or avoid objects. It has a power and object storage");strcpy(rvr.im,"#");strcpy(,"Power Station");strcpy(pstn.id,"PSTN");strcpy(pstn.desc,"A movable power station that will restore power to the Mars Rovers");strcpy(pstn.im,"\025");strcpy(p_,"User Mars Rover");strcpy(p_rover.id,"URVR");strcpy(p_rover.desc,"A Mars vehicle that is controlled by the user. Explores the map collecting, destroying and avoiding objects");strcpy(p_rover.im,"<^^>");p_rover.pwr = 100;p_rover.strg = 0;p_rover.location[0] = 7;p_rover.location[1] = 0;/****************************************************/printf("*******************************START********************* ***********\n\n");do{ /* Object will be gained from mapper */printf("+++++++++++++++++++++++++++++++MENU++++++++++++ +++++++++++++++++++++\n");printf("Please choose one of the following option:\n");printf("1 - Start\n");printf("2 - Controls\n");printf("3 - Show Map\n");printf("4 - Help Menu\n");printf("5 - Quit\n\n");printf("--------------->");scanf("%d", &menu);switch (menu){case 1:show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);while (quit != 1 ){input = control(input);if (input == 27)quit = 1;else{if(p_rover.pwr>0){direction(input, &number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);printf("Rover stats: \nPower: %d%% \nStorage: %d%%\n", p_rover.pwr,p_rover.strg);}else{printf("*******************END GAME********************\n\n"); // ends game when charge = 0.break;}}}break;case 2:quit = 0;printf("\n");printf("* * *\n");printf(" Controls \n"); // menu for the keys used on the keyboard to move the rover in different directions.printf(" Up : W \n");printf(" Down : S \n");printf("* Left : A * \n");printf("* Right : D * \n");printf(" choose : Enter \n");printf("* * *\n");break;case 3:quit = 0;show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);break;case 4:quit = 0;help(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);break;case 5:printf("*********************END***********************\n"); break;}}while(menu!=5);printf("\n -----Please press any key to exit game-----\n");getch();}。
c语言代码小游源代码

c语言代码小游源代码C语言代码小游 - 用代码玩转游戏世界在计算机编程领域中,C语言是一门广泛应用于系统开发和游戏开发的编程语言。
它的特点是简洁高效,同时也因为其强大的功能而受到广大开发者的喜爱。
本文将以C语言代码小游为主题,介绍一些有趣的小游戏,并通过代码实例展示它们的实现过程。
1. 猜数字游戏猜数字游戏是一款简单而又经典的小游戏。
在游戏开始时,程序会生成一个随机数,玩家需要通过输入来猜测这个数是多少。
程序会根据玩家的猜测给出相应的提示,直到玩家猜中为止。
以下是一个简单的猜数字游戏的C语言代码实现:```c#include <stdio.h>#include <stdlib.h>#include <time.h>int main() {int number, guess, count = 0;srand(time(0));number = rand() % 100 + 1;printf("猜数字游戏开始!\n");do {printf("请输入一个数:");scanf("%d", &guess);count++;if (guess > number) {printf("太大了!\n");} else if (guess < number) {printf("太小了!\n");} else {printf("恭喜你,猜对了!你用了%d次猜中了答案%d。
\n", count, number);}} while (guess != number);return 0;}```2. 井字棋游戏井字棋游戏是一款经典的二人对战游戏。
在游戏开始时,程序会绘制一个3x3的棋盘,玩家轮流在棋盘上放置自己的棋子,先连成一条线的玩家获胜。
以下是一个简单的井字棋游戏的C语言代码实现:```c#include <stdio.h>char board[3][3] = {' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' '};void drawBoard() {printf(" %c | %c | %c \n", board[0][0], board[0][1], board[0][2]);printf("---+---+---\n");printf(" %c | %c | %c \n", board[1][0], board[1][1], board[1][2]);printf("---+---+---\n");printf(" %c | %c | %c \n", board[2][0], board[2][1], board[2][2]);}int checkWin() {for (int i = 0; i < 3; i++) {if (board[i][0] == board[i][1] && board[i][1] ==board[i][2] && board[i][0] != ' ') {return 1;}if (board[0][i] == board[1][i] && board[1][i] == board[2][i] && board[0][i] != ' ') {return 1;}}if (board[0][0] == board[1][1] && board[1][1] == board[2][2] && board[0][0] != ' ') {return 1;}if (board[0][2] == board[1][1] && board[1][1] == board[2][0] && board[0][2] != ' ') {return 1;}return 0;}int main() {int row, col, player = 1;printf("井字棋游戏开始!\n");do {drawBoard();if (player % 2 == 1) {printf("轮到玩家1(X)下棋:");} else {printf("轮到玩家2(O)下棋:");}scanf("%d %d", &row, &col);if (board[row][col] == ' ') {if (player % 2 == 1) {board[row][col] = 'X';} else {board[row][col] = 'O';}player++;} else {printf("该位置已经被占用,请重新选择!\n"); }} while (!checkWin() && player <= 9);drawBoard();if (checkWin()) {if (player % 2 == 1) {printf("恭喜玩家2(O)获胜!\n");} else {printf("恭喜玩家1(X)获胜!\n");}} else {printf("游戏结束,平局!\n");}return 0;}```通过以上两个简单的C语言代码小游戏,我们可以看到C语言的强大之处。
简单的迷宫小游戏C语言程序源代码

简单的迷宫小游戏C语言程序源代码#include <stdio.h>#include <conio.h>#include <windows.h> #include <time.h>#define Height 31 //迷宫的高度,必须为奇数 #define Width 25 //迷宫的宽度,必须为奇数 #define Wall 1#define Road 0#define Start 2#define End 3#define Esc 5#define Up 1#define Down 2#define Left 3#define Right 4int map[Height+2][Width+2]; void gotoxy(int x,int y) //移动坐标{COORD coord;coord.X=x;coord.Y=y;SetConsoleCursorPosition( GetStdHandle( STD_OUTPUT_HANDLE ), coord );}void hidden()//隐藏光标{HANDLE hOut = GetStdHandle(STD_OUTPUT_HANDLE);CONSOLE_CURSOR_INFO cci; GetConsoleCursorInfo(hOut,&cci);cci.bVisible=0;//赋1为显示,赋0为隐藏SetConsoleCursorInfo(hOut,&cci);}void create(int x,int y) //随机生成迷宫 {int c[4][2]={0,1,1,0,0,-1,-1,0}; //四个方向 int i,j,t;//将方向打乱for(i=0;i<4;i++){j=rand()%4;t=c[i][0];c[i][0]=c[j][0];c[j][0]=t;t=c[i][1];c[i][1]=c[j][1];c[j][1]=t;}map[x][y]=Road;for(i=0;i<4;i++)if(map[x+2*c[i][0]][y+2*c[i][1]]==Wall){map[x+c[i][0]][y+c[i][1]]=Road;create(x+2*c[i][0],y+2*c[i][1]);}}int get_key() //接收按键{char c;while(c=getch()) {if(c==27) return Esc; //Escif(c!=-32)continue; c=getch();if(c==72) return Up; //上if(c==80) return Down; //下if(c==75) return Left; //左if(c==77) return Right; //右}return 0;}void paint(int x,int y) //画迷宫 { gotoxy(2*y-2,x-1); switch(map[x][y]) { case Start:printf("入");break; //画入口case End:printf("出");break; //画出口case Wall:printf("※");break; //画墙case Road:printf(" ");break; //画路}}void game(){int x=2,y=1; //玩家当前位置,刚开始在入口处 int c; //用来接收按键while(1){gotoxy(2*y-2,x-1); printf("?"); //画出玩家当前位置if(map[x][y]==End) //判断是否到达出口{gotoxy(30,24); printf("到达终点,按任意键结束");getch();break;}c=get_key(); if(c==Esc){gotoxy(0,24); break;}switch(c){case Up: //向上走if(map[x-1][y]!=Wall){paint(x,y); x--;}break;case Down: //向下走if(map[x+1][y]!=Wall){paint(x,y); x++;}break;case Left: //向左走if(map[x][y-1]!=Wall){paint(x,y); y--;}break;case Right: //向右走if(map[x][y+1]!=Wall){paint(x,y); y++;}break;}}}int main(){int i,j;srand((unsigned)time(NULL)); //初始化随即种子 hidden(); //隐藏光标for(i=0;i<=Height+1;i++) for(j=0;j<=Width+1;j++)if(i==0||i==Height+1||j==0||j==Width+1) //初始化迷宫 map[i][j]=Road;else map[i][j]=Wall;create(2*(rand()%(Height/2)+1),2*(rand()%(Width/2)+1)); //从随机一个点开始生成迷宫,该点行列都为偶数for(i=0;i<=Height+1;i++) //边界处理 {map[i][0]=Wall;map[i][Width+1]=Wall; }for(j=0;j<=Width+1;j++) //边界处理{map[0][j]=Wall;map[Height+1][j]=Wall; }map[2][1]=Start; //给定入口map[Height-1][Width]=End; //给定出口 for(i=1;i<=Height;i++)for(j=1;j<=Width;j++) //画出迷宫paint(i,j);game(); //开始游戏getch();return 0;}。
C语言游戏源代码

C语言游戏源代码 Document number【980KGB-6898YT-769T8CB-246UT-18GG08】C语言游戏源代码1、简单的开机密码程序#include ""#include ""#include ""void error(){window(12,10,68,10);textbackground(15);textcolor(132);clrscr();cprintf("file or system error! you can't enter the system!!!");while(1); /*若有错误不能通过程序*/}void look(){FILE *fauto,*fbak;char *pass="c:\\windows\\"; /*本程序的位置*/char a[25],ch;char *au="",*bname="hecfback.^^^"; /*bname 是的备份*/setdisk(2); /*set currently disk c:*/chdir("\\"); /*set currently directory \*/fauto=fopen(au,"r+");if (fauto==NULL){fauto=fopen(au,"w+");if (fauto==NULL) error();}fread(a,23,1,fauto); /*读取前23各字符*/a[23]='\0';if (strcmp(a,pass)==0) /*若读取的和pass指针一样就关闭文件,不然就添加*/fclose(fauto);else{fbak=fopen(bname,"w+");if (fbak==NULL) error();fwrite(pass,23,1,fbak);fputc('\n',fbak);rewind(fauto);while(!feof(fauto)){ch=fgetc(fauto);fputc(ch,fbak);}rewind(fauto);rewind(fbak);while(!feof(fbak)){ch=fgetc(fbak);fputc(ch,fauto);}fclose(fauto);fclose(fba k);remove(bname); /*del bname file*/}}void pass()char input[60];int n;while(1){window(1,1,80,25);textbackground(0);textcolor(15);clrscr();n= 0;window(20,12,60,12);textbackground(1);textcolor(15);clrscr();cprintf(" password:");while(1){input[n]=getch();if (n>58) {putchar(7); break;} /*若字符多于58个字符就结束本次输入*/if (input[n]==13) break;if (input[n]>=32 && input[n]<=122) /*若字符是数字或字母才算数*/{putchar('*');n++;}if (input[n]==8) /*删除键*/if (n>0){cprintf("\b \b");input[n]='\0';n--;}}input[n]='\0';if(strcmp(password,input)==0)break;else{putchar(7);window(30,14,50,14);tex tbackground(15);textcolor(132);clrscr();cprintf("passworderror!");getch();}}}main(){look();pass();}2、彩色贪吃蛇#include <>#include <>#define N 200#define up 0x4800#define down 0x5000#define left 0x4b00#define right 0x4d00#define esc 0x011b#define Y 0x1579#define n 0x316eint gamespeed; /* 游戏速度 */int i, key, color;int score = 0; /* 游戏分数 */char cai48H[] ={0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x04, 0x00, 0x18, 0x00, 0x00, 0x00, 0x0E, 0x00,0x1C, 0x00, 0x00, 0x00, 0x1C, 0x00, 0x1C, 0x00,0x00, 0x00, 0x20, 0x00, 0x38, 0x00, 0x00, 0x00,0x40, 0x00, 0x78, 0x00, 0x00, 0x01, 0x80, 0x40,0x70, 0x00, 0x00, 0x03, 0x80, 0xC0, 0xE0, 0x00,0x00, 0x07, 0x80, 0x80, 0xC0, 0x00, 0x00, 0x0E,0x11, 0x81, 0xC0, 0x00, 0x00, 0x08, 0x61, 0x01,0x80, 0x00, 0x00, 0x00, 0x23, 0x03, 0x04, 0x00,0x00, 0x02, 0x02, 0x00, 0x06, 0x00, 0x00, 0x1E,0x04, 0x00, 0x0F, 0x00, 0x00, 0x1C, 0x1F, 0x80, 0x1E, 0x00, 0x00, 0x08, 0x3F, 0x80, 0x3C, 0x00, 0x00, 0x00, 0xFF, 0x80, 0x38, 0x00, 0x00, 0x03, 0xFF, 0x80, 0x78, 0x00, 0x00, 0x0F, 0xF8, 0x00, 0xF0, 0x00, 0x00, 0x7F, 0xF0, 0x00, 0xE0, 0x00, 0x03, 0xFF, 0xFC, 0x01, 0x80, 0x00, 0x03, 0xC0, 0xFF, 0x01, 0x03, 0x80, 0x01, 0x01, 0xFF, 0x00, 0x03, 0x80, 0x00, 0x01, 0x3F, 0x00, 0x07, 0x80, 0x00, 0x02, 0x11, 0x00, 0x07, 0x00, 0x00, 0x00, 0x10, 0x00, 0x07, 0x00, 0x00, 0x00, 0x10, 0x00, 0x0E, 0x00, 0x00, 0x08, 0x10, 0x00, 0x1C, 0x00, 0x00, 0x30, 0x10, 0x00, 0x18, 0x00, 0x00, 0x70, 0x10, 0x00, 0x30, 0x00, 0x01, 0xE0, 0x10, 0x00, 0x70, 0x00, 0x03, 0x80, 0x10, 0x00, 0x60, 0x00, 0x00, 0x00, 0x30, 0x00, 0xE0, 0x00, 0x00, 0x00, 0xF0, 0x01, 0xC0, 0x00, 0x00, 0x00, 0x70, 0x03,0xC0, 0x00, 0x00, 0x00, 0x10, 0x07, 0x80, 0x00,0x00, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x00,0x00, 0x1E, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3C,0x00, 0x00, 0x00, 0x00, 0x00, 0x70, 0x00, 0x00,0x00, 0x00, 0x01, 0xC0, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };char she48H[] ={0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04,0x00, 0x00, 0x00, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x00, 0x00, 0x03, 0x00, 0x07, 0x00, 0x00, 0x00, 0x02, 0x00, 0x03, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x02, 0x00, 0x07, 0x86, 0x00, 0x00, 0x02, 0x00, 0x18, 0x03, 0x00, 0x00, 0x02, 0x00, 0x00, 0x07, 0x80, 0x00, 0x03, 0xF0, 0x00, 0x07, 0x80, 0x00, 0x0F, 0xFC, 0x00, 0x0C, 0x00, 0x00, 0x7E, 0x3F, 0x80, 0x00, 0x00, 0x01, 0xFE, 0x1F, 0x80, 0x00, 0x00, 0x01, 0xE2, 0x39, 0x8C, 0x00, 0x00, 0x00, 0xC2, 0x30, 0x08, 0x00, 0x00, 0x00, 0xC2, 0x60, 0x08, 0x00, 0x00, 0x00, 0xC3, 0xE0, 0x08, 0x60, 0x00, 0x00, 0x7F, 0xE0, 0x01, 0xE0, 0x00, 0x00, 0x3F, 0x80, 0x1F, 0xE0, 0x00, 0x00, 0x1E, 0x00, 0x1F, 0x80, 0x00,0x00, 0x1E, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x02,0x38, 0x1E, 0x00, 0x00, 0x00, 0x07, 0xFC, 0x1C,0x00, 0x20, 0x00, 0x07, 0xFC, 0x18, 0x00, 0x20,0x00, 0x1F, 0x0C, 0x10, 0x00, 0x20, 0x00, 0x7C,0x04, 0x10, 0x00, 0x60, 0x01, 0xF0, 0x00, 0x10,0x00, 0x60, 0x01, 0xE0, 0x00, 0x08, 0x00, 0xF0,0x00, 0x80, 0x00, 0x08, 0x03, 0xF0, 0x00, 0x00,0x00, 0x07, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x07,0xFF, 0xF0, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xE0,0x00, 0x00, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };char tun48H[] ={0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x0E, 0x00, 0x00, 0x00, 0x00, 0x00, 0x3E,0x00, 0x00, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x00,0x00, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x00, 0x00,0x03, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x00,0x00, 0x00, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x00,0x00, 0x01, 0xF8, 0x00, 0x00, 0x00, 0x00, 0x03,0xF8, 0x00, 0x40, 0x00, 0x00, 0x00, 0x06, 0x07,0xC0, 0x00, 0x00, 0x00, 0x07, 0xFF, 0xE0, 0x00,0x00, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x00, 0x00,0x0F, 0xFF, 0x80, 0x00, 0x00, 0x00, 0x7F, 0xF8,0x00, 0x1F, 0xFF, 0xF8, 0x00, 0x00, 0x00, 0x1F, 0xFC, 0x3C, 0x00, 0x00, 0x00, 0x0F, 0xF8, 0x0E, 0x00, 0x00, 0x00, 0x04, 0x70, 0x07, 0x00, 0x00, 0x00, 0x00, 0x60, 0x03, 0x80, 0x00, 0x00, 0x00, 0xC0, 0x00, 0xC0, 0x00, 0x00, 0x01, 0x80, 0x00, 0x30, 0x00, 0x00, 0x01, 0x00, 0x3C, 0x18, 0x00, 0x00, 0x02, 0x03, 0xFF, 0x0C, 0x00, 0x00, 0x0C, 0x7F, 0xFF, 0x8E, 0x00, 0x00, 0x18, 0xFF, 0xFF, 0xC7, 0x80, 0x00, 0x78, 0xFE, 0x07, 0x87, 0xE0, 0x01, 0xF0, 0x70, 0x07, 0x03, 0xF8, 0x07, 0xE0, 0x70, 0x0E, 0x03, 0xFE, 0x00, 0x00, 0x38, 0x1E, 0x01, 0xFE, 0x00, 0x00, 0x3F, 0xFE, 0x00, 0x0C, 0x00, 0x00, 0x1F, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x1F, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x0F, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };char dan48H[] ={0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0xFC, 0x00, 0x00, 0x00, 0x00, 0x07, 0xFF,0x00, 0x00, 0x00, 0x00, 0x7F, 0xC0, 0x80, 0x00,0x00, 0x03, 0xFF, 0x80, 0x40, 0x00, 0x00, 0x01,0xE0, 0x00, 0x00, 0x00, 0x01, 0x93, 0xF0, 0x00, 0x00, 0x00, 0x01, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x21, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x21, 0xF8, 0x00, 0x00, 0x00, 0x00, 0x61, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x61, 0x80, 0x00, 0x00, 0x00, 0x00, 0xF3, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x01, 0xFF, 0xC0, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xF8, 0x00, 0x00, 0x00, 0x02, 0x00, 0xFC, 0x00, 0x00, 0x00, 0x04, 0x02, 0x1F, 0x00, 0x00, 0x00, 0x08, 0x03, 0x01, 0xC0, 0x00, 0x00, 0x38, 0x03, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x07, 0xF8, 0x3F, 0xC0, 0x01, 0xF0, 0x3F, 0xFE, 0x3F, 0xF8, 0x03, 0xC1, 0xFF, 0x0F, 0x1F, 0xF8, 0x00, 0x01, 0xE3, 0x0F, 0x0F, 0xF0, 0x00, 0x01, 0xC3, 0x0E, 0x00, 0x00, 0x00, 0x01, 0x83, 0xFC,0x00, 0x00, 0xFF, 0xF8, 0x00, 0x00, 0x00, 0x00,0x7F, 0xF0, 0x00, 0x00, 0x00, 0x00, 0x3F, 0x03,0x80, 0x00, 0x00, 0x00, 0x03, 0x04, 0x00, 0x00,0x00, 0x00, 0x03, 0xF8, 0x00, 0x00, 0x00, 0x00,0x1F, 0xF8, 0x20, 0x00, 0x00, 0x00, 0xFF, 0xFF,0xE0, 0x00, 0x00, 0x07, 0xFF, 0x81, 0xE0, 0x00,0x00, 0x07, 0xE0, 0x00, 0xE0, 0x00, 0x00, 0x03,0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };char zuo16H[] =0x18, 0xC0, 0x18, 0xC0, 0x19, 0x80, 0x31, 0xFE,0x33, 0xFE, 0x76, 0xC0, 0xF0, 0xFC, 0xB0, 0xFC,0x30, 0xC0, 0x30, 0xC0, 0x30, 0xFE, 0x30, 0xFE,0x30, 0xC0, 0x30, 0xC0, 0x30, 0xC0, 0x00, 0x00, };char zhe16H[] ={0x03, 0x00, 0x03, 0x0C, 0x1F, 0xCC, 0x1F, 0xD8,0x03, 0x30, 0xFF, 0xFE, 0xFF, 0xFE, 0x03, 0x00,0x0F, 0xF8, 0x3F, 0xF8, 0xEC, 0x18, 0xCF, 0xF8,0x0C, 0x18, 0x0F, 0xF8, 0x0F, 0xF8, 0x00, 0x00, };char tian16H[] =0x00, 0x00, 0x3F, 0xFC, 0x3F, 0xFC, 0x31, 0x8C,0x31, 0x8C, 0x31, 0x8C, 0x3F, 0xFC, 0x3F, 0xFC,0x31, 0x8C, 0x31, 0x8C, 0x31, 0x8C, 0x3F, 0xFC,0x3F, 0xFC, 0x30, 0x0C, 0x00, 0x00, 0x00, 0x00, };char xue16H[] ={0x33, 0x18, 0x19, 0x98, 0x08, 0xB0, 0x7F, 0xFC,0x7F, 0xFC, 0x60, 0x0C, 0x1F, 0xF0, 0x1F, 0xF0,0x00, 0xC0, 0x7F, 0xFC, 0x7F, 0xFC, 0x01, 0x80,0x01, 0x80, 0x07, 0x80, 0x03, 0x00, 0x00, 0x00, };char ke16H[] ={0x00, 0x00, 0x0C, 0x18, 0xFD, 0x98, 0xF8, 0xD8,0x18, 0x58, 0xFE, 0x18, 0xFE, 0x98, 0x18, 0xD8,0x3C, 0x58, 0x7E, 0x1E, 0xDB, 0xFE, 0x9B, 0xF8,0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x00, 0x00,};struct Food/*定义结构体存储食物的属性*/{int x; /* 食物的坐标 */int y;int yes; /* 值为0表示屏幕上没有食物,值为1表示屏幕上有食物 */ int color; /* 食物颜色 */} food;struct Snake/*定义结构体存储蛇的属性*/{int x[N]; /* 每一节蛇的坐标 */int y[N];int color[N];/*存储每一节蛇的颜色*/int node; /* 蛇的节数 */int direction; /* 蛇移动的方向 */int life; /* 蛇的生命,如果为1,蛇死,游戏结束 */} snake;void init(void)/*图形驱动*/{int driver = DETECT, mode = 0;registerbgidriver(EGAVGA_driver);initgraph(&driver, &mode, "");}void drawmat(char *mat, int matsize, int x, int y, int color) /*汉字点阵*/{int i, j, k, m;m = (matsize - 1) / 8 + 1;for(j = 0; j < matsize; j++)for(i = 0; i < m; i++)for(k = 0; k < 8; k++)if(mat[j*m+i]&(0x80 >> k))putpixel(x + i * 8 + k, y + j, color);}void showword(void){/* 调用汉字点阵输出程序,显示标题和作者信息 */drawmat(cai48H, 48, 249, -4, 7);drawmat(she48H, 48, 329, -4, 7);drawmat(tun48H, 48, 409, -4, 7);drawmat(dan48H, 48, 489, -4, 7);drawmat(cai48H, 48, 250, -5, 4);drawmat(she48H, 48, 330, -5, 4);drawmat(tun48H, 48, 410, -5, 4);drawmat(dan48H, 48, 490, -5, 4);/*作者田学科*/drawmat(zuo16H, 16, 515, 465, 7);drawmat(zhe16H, 16, 530, 465, 7);drawmat(tian16H, 16, 550, 465, 7);drawmat(xue16H, 16, 565, 465, 7);drawmat(ke16H, 16, 580, 465, 7); }void draw(void)/*画出四周的墙*/if(color == 15)color = 0;setcolor(++color);setlinestyle(SOLID_LINE, 0, 1);for(i = 30; i <= 600; i += 10){rectangle(i, 40, i + 10, 49);rectangle(i, 451, i + 10, 460); }for(i = 40; i < 450; i += 10){rectangle(30, i, 39, i + 10);rectangle(601, i, 610, i + 10); }void prscore(void){/* 打印游戏分数 */char str[10];setfillstyle(SOLID_FILL, YELLOW);bar(50, 10, 200, 30);setcolor(6);settextstyle(0, 0, 2);sprintf(str, "score:%d", score);outtextxy(55, 15, str);}void gameover(void){cleardevice(); /* 清屏函数 */for(i = 0; i < ; i++) /* 画出蛇死时的位置 */ {setcolor[i]);rectangle[i], [i], [i] + 10, [i] + 10);}prscore(); /* 显示分数 */draw();showword();settextstyle(0, 0, 6);setcolor(7);outtextxy(103, 203, "GAME OVER");setcolor(RED);outtextxy(100, 200, "GAME OVER");}void gameplay(void)/* 玩游戏的具体过程 */ {int flag, flag1;randomize();prscore();gamespeed = 50000;= 0; /* =0表示屏幕上没有食物 */= 1; /* =1表示蛇是活着的 */= 4; /* 表示蛇的初始方向为向右 */= 2; /* 蛇的初始化为两节 */[0] = 2; /*两节蛇头初始化为绿色*/[1] = 2;[0] = 100;[0] = 100;[1] = 110;[1] = 100;= random(15) + 1; while(1){while(1){if == 0) /* 如果蛇活着 */ {while(1){flag = 1;= 1;= random(56) * 10 + 40; = random(40) * 10 + 50;for(i = 0; i < ; i++){if == [i] && == [i])flag = 0;}if(flag) break;}}if{setcolor;rectangle, , + 10, + 10); }for(i = - 1; i > 0; i--) {[i] = [i-1];[i] = [i-1];}switch{case 1:[0] -= 10; break;case 2:[0] += 10; break;case 3:[0] -= 10; break;case 4:[0] += 10; break; }for(i = 3; i < ; i++){if[i] == [0] && [i] == [0]){gameover();= 0;break;}}if[0] < 40 || [0] > 590 || [0] < 50 || [0] > 440) {gameover();= 0;}if == 0)break;if[0] == && [0] == /*蛇吃掉食物*/ {setcolor(0);rectangle, , + 10, + 10);[] = -20;[] = -20;[] = ;++;= 0;= random(15) + 1;score += 10;prscore();if(score % 100 == 0 && score != 0){for(i = 0; i < ; i++) /* 画出蛇 */{setcolor[i]);rectangle[i], [i], [i] + 10, [i] + 10); }sound(200);delay(50000);delay(50000);delay(50000);delay(50000);delay(50000);delay(50000);nosound();gamespeed -= 5000; draw();}else{sound(500);delay(500);nosound();}}for(i = 0; i < ; i++) /* 画出蛇 */{setcolor[i]);rectangle[i], [i], [i] + 10, [i] + 10); }delay(gamespeed);delay(gamespeed);flag1 = 1;setcolor(0);rectangle[], [],[] + 10, [] + 10);if(kbhit() && flag1 == 1) /*如果没按有效键就重新开始循环*/ {flag1 = 0;key = bioskey(0);if(key == esc)exit(0);else if(key == up && != 2)= 1;else if(key == down && != 1)= 2;else if(key == left && != 4)= 3;else if(key == right && != 3)= 4;}}if == 0) /*如果蛇死了就退出循环*/ break;}}void main(void){while(1){color = 0;init();cleardevice();showword();draw();gameplay();setcolor(15);settextstyle(0, 0, 2);outtextxy(200, 400, "CONTINUE(Y/N)"); while(1) { key = bioskey(0);if(key == Y || key == n || key == esc) break; } if(key == n || key == esc) break; } closegraph();}3、c语言实现移动电话系统#include <>#define GRID-SIZE 5#define SELECTED -1 /*低于矩阵中所有元素*/#define TRAFFIC-FILE “”/*关于交通数据的文件*/#define NUM-TRANSMITTERS 10 /*可用的发射器数量*/void get-traffic-data(int commuters[GRID-SIZE][GRID-SIZE],int salesforce[GRID-SIZE][GRID-SIZE],int weekends [GRID-SIZE][GRID-SIZE];voide print-matrix[GRID-SIZE][GRID-SIZE];intmain(void){int commuters[GRID-SIZE][GRID-SIZE];/*上午8:30的交通数据*/int salesforce[GRID-SIZE][GRID-SIZE]; /*上午11:00的交通数据*/ int weekend[GRID-SIZE][GRID-SIZE];/*周末交通数据*/int commuter-weight, /*通勤人员数据的权重因子*/sale-weight,/*营销人员数据的权重因子*/weekend-weight; /*周末数据的权重因子*/int location-i, /*每个发射器的位置*/location-j;int current-max; /*和数据中当前的最大值*/int i,j, /*矩阵的循环计数器*/tr; /*发射器的循环计数器*//*填入并显示交通数据*/Get-traffic-data (commuters,salesforce,weekend);Printf(“8:、\n\n”)print-matrix(commuters);printf(“\n\n WEEKEND TRAFFIC DATA\n\n”);print-matrix(salesforce);printf(“\n\n WEEKEND TRAFFIC DATA\n\n”);printf_matrix(weekeng);/*请用户输入权重因子*/printf(“\n\nPlease input the following value:\n”); printf(“Weight (an interger>=0) for the 8:30 data>”) scanf(“%d”,&commuter_weight);printf(“weight(an integer>=0) for the weekeng data>”);scanf(“%d”,&weekend_weight);scanf(“%d”,&weekend_weight);/*计算并显示加权后求和的数据*/for (i=0;i<GRID_SIZE;++i)for (j=0;j<GRID_SIZE;++j)summed_data[i][j]=commuter_weight*commuter[i][j]+salesforce_weight*salesforce[i][j]+weekend_weight*weekend[i][j];printf(“\n\nThe weighted,summed data is :\n\n”);printf_matrix(summed_data);/*在summed_data矩阵中找出NUM_TRANSMITTERS个最大值,将坐标临时存储在location_i和location_j中,然后把最后的结果坐标输出*/printf(“\n\nLocations of the %d transmitters:\n\n”,NUM_TRANSM ITTERS); for (tr=1;tr<=NUM_TRANSMITTERS;++tr){current_max=SELECTED;/*以一个过低的值为起点开始查找*/for (i=0;i<GRID_SIZE;++i){for(j=0;j<GRID_SIZE;++j){if(current_max<summed_data[i][j]){current_max=summed_data[i][j]){location_i=i;location_j=j;}}}/*将选中的单元赋一较低的值以避免下次再选中这一元素,显示查找结果*/ summed_data[location_i][location_j]=SELECTED;printf(“Transmitter %3d:at location %3d %3d\n”,tr,location_i,location_j);}return (0);/**把 TRAFFIC_FILE中的交通数据填充到3个GRID_SIZE×GRID_SIZE数组中*/voidget_traffic_data(int commuters[GRID_SIZE],/*输出*/int salesforce[GRID_SIZE][GRID_SIZE],/*输出*/int weekend[GRID_SIZE][GRID_SIZE],/*输出*/{int i,j; /*循环计数器*/FILE *fp; /*文件指针*/fq=fopen(TRAFFIC_FILE,“r”);for(i=0;i<GRID_SIZE;++i)for(j=0;j<GRID_SIZE;++j)fscanf(fp,“%d”,&commnt ers[i][j];for(i=0;i<GRID_SIZE;++j)for(j=0;j<GRID_SIZE;++j)fscanf(fq,“%d”,&weekend[i][j]);fclose(fq);}/**显示一个GRID_SIZE×GRID_SIZE整数矩阵的内容*/voidprint_matrix(int matrix[GRID_SIZE][GRID_SIZE]) {int i,j; /*循环计数器*/for(i=0;i<GRID_SIZE;++j){ for(j=0;j<GRID_SIZE;++J)printf(“%3d”,matrix[i][j]);printf(“\n”);}}4、扑克牌游戏/*************************************Copyright(C) 2004-2005 vision,math,NJU.File Name:Author: vision Version: Data: 23-2-2004Description: 给你9张牌,然后让你在心中记住那张牌,然后电脑分组让你猜你记住的牌在第几组,然后猜出你记住的那张牌.Other: 出自儿童时的一个小魔术History:修改历史**************************************/#include <>#include <>#include <>#include <>#include <>#define CARDSIZE 52 /*牌的总张数*/#define SUITSIZE 13 /*一色牌的张数*//*扑克牌结构*/typedef struct Card{char val;/*扑克牌面上的大小*/int kind :4; /*扑克牌的花色*/}Card;/************************************************* Function:Calls: al把余数给*/cards[deckp].kind = "3456"[]; /*把商给*/}}/*show的原代码,将会自动换行*/void show(const Card *cards, int size){for(int i = 0; i < size; i++){printf("%c%c ",cards[i].kind,cards[i].val);if( (i !=0) && (((i+1 ) % 3) == 0))puts("");}puts(""); /*自动换行*/}/*grouping 的原代码*/void grouping(const Card *cards, Card *carr1, Card *carr2, Card *carr3) {int i = 0;/*循环参数*//*分给carr1三个数*/while (i < 3){carr1[i].val = cards[i].val;carr1[i].kind = cards[i].kind; i++;}/*分给carr2接下来的三个数*/ while (i < 6){carr2[i-3].val = cards[i].val; carr2[i-3].kind = cards[i].kind; i++;}/*分给carr3接下来的三个数*/ while (i < 9){carr3[i-6].val = cards[i].val;carr3[i-6].kind = cards[i].kind;i++;}}/*rshift的实现*/void rshift(Card *carr1, Card *carr2, Card *carr3, int counter) {Card temp2;/*用于存放carr2[counter]*/Card temp3;/*用于存放carr3[counter]*//*temp = carr2*/= carr2[counter].val;= carr2[counter].kind;/*carr2 = carr1*/carr2[counter].val = carr1[counter].val;carr2[counter].kind = carr1[counter].kind;/*temp3 = carr3*/= carr3[counter].val;= carr3[counter].kind;/*carr3 = carr2*/carr3[counter].val = ;carr3[counter].kind = ;/*carr1 = carr3*/carr1[counter].val = ;carr1[counter].kind = ;}Card* result_process(Card *carr1, Card *carr2, Card *carr3, int counter) {rshift(carr1, carr2, carr3, counter); /* 把数组的第一个元素依次右移*/if(counter == 2){return(&carr2[2]);}show(carr1, 3);show(carr2, 3);show(carr3, 3);puts("请给出你记住的牌所在行数:");fflush(stdin);int input = 1;input = getchar(); /*获取你选的组*/switch(input){case '1':return(result_process(carr1, carr2, carr3, ++counter)); break;case '2':return(&carr2[counter]);break;default:puts("你在撒谎!不和你玩了!");return NULL;}}5、C语言实现打字游戏#include ""#include ""#include ""#include ""#include ""#define xLine 70#define yLine 20#define full 100#define true 1#define false 0。
一种C语言小游戏程序设计

一种C语言小游戏程序设计以下是一种基于C语言的小游戏程序设计示例,该示例为一个简单的猜数字游戏。
该游戏程序的主要功能是生成一个随机的数字,然后提示玩家根据提示进行猜测,直到猜对为止。
```c#include <stdio.h>#include <stdlib.h>int maiint secretNumber, guess, attempts = 0;secretNumber = rand( % 100 + 1; // 生成1-100之间的随机数printf("欢迎来到猜数字游戏!\n");doprintf("请输入一个1-100之间的整数: ");scanf("%d", &guess);attempts++;if (guess > secretNumber)printf("太大了!请再试一次。
\n");} else if (guess < secretNumber)printf("太小了!请再试一次。
\n");} elseprintf("恭喜你猜对了!\n");printf("你猜了%d次。
\n", attempts);}} while (guess != secretNumber);return 0;```以上是一个简单的猜数字游戏程序,下面我将详细解释每个部分的功能。
接下来,我们使用 `printf` 输出欢迎信息,并使用 `do-while` 循环来进行游戏的主要逻辑。
在每次循环中,我们使用 `printf` 提示玩家输入一个1到100之间的整数,并使用 `scanf` 将输入的值存储在变量`guess` 中。
然后,我们使用 `if-else` 条件语句来判断玩家的猜测结果。
如果玩家猜测的数字大于秘密数字,我们输出 "太大了!请再试一次。
c语言小游戏代码

c语言小游戏代码#include <stdio.h>#include <stdlib.h>#include <windows.h>// 定义元素类型#define ELEMENT char// 游戏行数#define ROW 10// 游戏显示延迟#define SLEEPTIME 100int main(int argc, char *argv[]){// 定义游戏的棋盘,用数组存放ELEMENT array[ROW][ROW];// 定义获胜条件int winCondition = 5;// 初始化,把棋盘清空system("cls");int i,j;for(i = 0; i < ROW; i++){for(j = 0; j < ROW; j++){array[i][j] = ' ';}}// 循环游戏,当有一方满足胜利条件时终止int tmp;int count = 0; // 存放棋子数while(1){// 依次取出玩家记录的棋子int x, y;// 如果已经有子落下,则计算是第几步if(count > 0){printf("第%d步:\n", count);}// 显示游戏棋盘for(i = 0; i < ROW; i++){printf(" ");for(j = 0; j < ROW; j++){printf("---");}printf("\n|");for(j = 0; j < ROW; j++){printf("%c |", array[i][j]);}printf("\n");}printf(" ");for(j = 0; j < ROW; j++){printf("---");}printf("\n");// 要求玩家输入放下棋子的位置printf("请玩家输入要放弃棋子的位置(1-%d)\n", ROW); printf("横坐标:");scanf("%d", &x);printf("纵坐标:");scanf("%d", &y);// 判断棋子位置是否有效if(x < 1 || x > ROW || y < 1 || y > ROW || array[x-1][y-1] != ' '){printf("输入错误!\n");system("pause");system("cls");continue;}// 把棋子记录,并计数if(count % 2 == 0){array[x-1][y-1] = 'X';}else{array[x-1][y-1] = 'O';}count++;// 判断是否有获胜者int i, j, k;int tempx, tempy;for(i = 0; i < ROW; i++){for(j = 0; j < ROW; j++){if(array[i][j] == 'X' || array[i][j] == 'O') {// 判断横向是否有获胜者tmp = 1;for(k = 1; k < winCondition; k++){// 注意边界,必须验证范围有效if(j + k > ROW - 1) break;// 如果和前一个位置的棋子相同,则计数加1,否则跳出if(array[i][j+k] == array[i][j])tmp++;else break;}// 如果计数满足获胜条件,则显示获胜者if(tmp >= winCondition){printf("玩家 %c 获胜!\n", array[i][j]);system("pause");return 0;}// 判断纵向是否有获胜者tmp = 1;for(k。
C语言游戏源代码
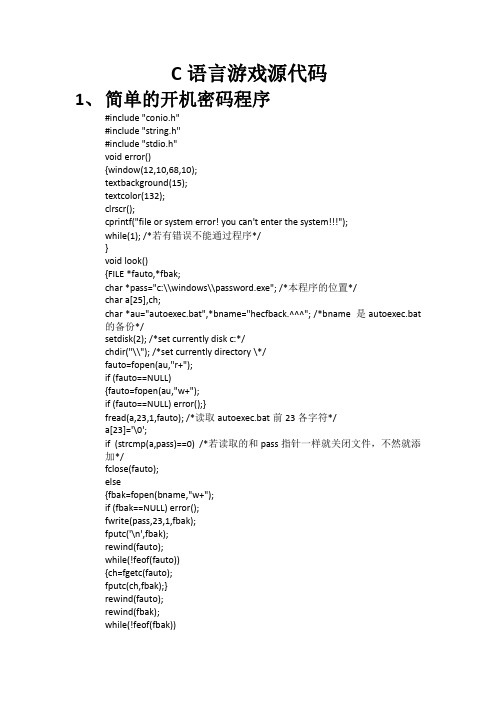
C语言游戏源代码1、简单的开机密码程序#include "conio.h"#include "string.h"#include "stdio.h"void error(){window(12,10,68,10);textbackground(15);textcolor(132);clrscr();cprintf("file or system error! you can't enter the system!!!");while(1); /*若有错误不能通过程序*/}void look(){FILE *fauto,*fbak;char的备份*/读取autoexec.bat前23各字符*//*若读取的和pass指针一样就关闭文件,不然就添fclose(fauto);else{fbak=fopen(bname,"w+");if (fbak==NULL) error();fwrite(pass,23,1,fbak);fputc('\n',fbak);rewind(fauto);while(!feof(fauto)){ch=fgetc(fauto);fputc(ch,fbak);}rewind(fauto);rewind(fbak);while(!feof(fbak)){ch=fgetc(fbak);fputc(ch,fauto);}fclose(fauto);fclose(fbak);remove(bname); /*del bname file*/}}void pass(){char *password="88888888";char input[60];int n;while(1){window(1,1,80,25);textbackground(0);textcolor(15);clrscr();n=0;clrscr();while(1)*/*/删除键*/{cprintf("\b \b");input[n]='\0';n--;}}input[n]='\0';if (strcmp(password,input)==0)break;else{putchar(7);window(30,14,50,14);textbackground(15);textcolor(132);clrscr();cprintf("password error!");getch();}}}main(){look();pass();}2、彩色贪吃蛇#include <graphics.h>#include <stdlib.h>#define N 200#define up 0x4800游戏速度*/int i, key, color;int score = 0; /* 游戏分数*/char cai48H[] ={0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x18, 0x00, 0x00, 0x00, 0x0E, 0x00, 0x1C, 0x00, 0x00, 0x00, 0x1C, 0x00, 0x1C, 0x00, 0x00, 0x00, 0x20, 0x00, 0x38, 0x00, 0x00, 0x00, 0x40, 0x00, 0x78, 0x00, 0x00, 0x01, 0x80, 0x40, 0x70, 0x00, 0x00, 0x03, 0x80, 0xC0, 0xE0, 0x00,0x03, 0xFF, 0xFC, 0x01, 0x80, 0x00, 0x03, 0xC0, 0xFF, 0x01, 0x03, 0x80, 0x01, 0x01, 0xFF, 0x00, 0x03, 0x80, 0x00, 0x01, 0x3F, 0x00, 0x07, 0x80, 0x00, 0x02, 0x11, 0x00, 0x07, 0x00, 0x00, 0x00, 0x10, 0x00, 0x07, 0x00, 0x00, 0x00, 0x10, 0x00, 0x0E, 0x00, 0x00, 0x08, 0x10, 0x00, 0x1C, 0x00,0x10, 0x00, 0x30, 0x00, 0x01, 0xE0, 0x10, 0x00, 0x70, 0x00, 0x03, 0x80, 0x10, 0x00, 0x60, 0x00, 0x00, 0x00, 0x30, 0x00, 0xE0, 0x00, 0x00, 0x00, 0xF0, 0x01, 0xC0, 0x00, 0x00, 0x00, 0x70, 0x03, 0xC0, 0x00, 0x00, 0x00, 0x10, 0x07, 0x80, 0x00, 0x00, 0x00, 0x00, 0x0F, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0C, 0x00, 0x00,0x00, 0x0E, 0x00, 0x00, 0x00, 0x03, 0x00, 0x07, 0x00, 0x00, 0x00, 0x02, 0x00, 0x03, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0xF8, 0x00, 0x00, 0x02, 0x00, 0x07, 0x86, 0x00, 0x00, 0x02, 0x00, 0x18, 0x03, 0x00, 0x00, 0x02, 0x00, 0x00, 0x07, 0x80, 0x00, 0x03,0x38, 0x1E, 0x00, 0x00, 0x00, 0x07, 0xFC, 0x1C, 0x00, 0x20, 0x00, 0x07, 0xFC, 0x18, 0x00, 0x20, 0x00, 0x1F, 0x0C, 0x10, 0x00, 0x20, 0x00, 0x7C, 0x04, 0x10, 0x00, 0x60, 0x01, 0xF0, 0x00, 0x10, 0x00, 0x60, 0x01, 0xE0, 0x00, 0x08, 0x00, 0xF0, 0x00, 0x80, 0x00, 0x08, 0x03, 0xF0, 0x00, 0x00,0xFF, 0xF0, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x01, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,};{0x00, 0x00, 0x00, 0x00, 0x00, 0x7F, 0x00, 0x00, 0x00, 0x00, 0x00, 0xE0, 0x00, 0x00, 0x00, 0x00, 0x03, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x1F, 0x00, 0x00, 0x00, 0x00, 0x00, 0x7C, 0x00, 0x00, 0x00, 0x00, 0x01, 0xF8, 0x00, 0x00, 0x00, 0x00, 0x03, 0xF8, 0x00, 0x40, 0x00, 0x00, 0x00, 0x06, 0x07,0x00, 0x00, 0x07, 0xFF, 0xE0, 0x00, 0x00, 0x00, 0x0F, 0xFF, 0x80, 0x00, 0x00, 0x00, 0x7F, 0xF8, 0x00, 0x00, 0x00, 0x1F, 0xFF, 0xF8, 0x00, 0x00, 0x00, 0x1F, 0xFF, 0xF8, 0x00, 0x00, 0x00, 0x1F, 0xFC, 0x3C, 0x00, 0x00, 0x00, 0x0F, 0xF8, 0x0E, 0x00, 0x00, 0x00, 0x04, 0x70, 0x07, 0x00, 0x00,0x00, 0x00, 0x1F, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x1F, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x0F, 0xFE, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };char dan48H[] ={0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,0x21, 0xFF, 0xF0, 0x00, 0x00, 0x00, 0x21, 0xF8, 0x00, 0x00, 0x00, 0x00, 0x61, 0xC0, 0x00, 0x00, 0x00, 0x00, 0x61, 0x80, 0x00, 0x00, 0x00, 0x00, 0xF3, 0x00, 0x00, 0x00, 0x00, 0x00, 0xFF, 0x00, 0x00, 0x00, 0x00, 0x01, 0xFF, 0xC0, 0x00, 0x00, 0x00, 0x03, 0xFF, 0xF8, 0x00, 0x00, 0x00, 0x02,0x00, 0x00, 0x00, 0x08, 0x03, 0x01, 0xC0, 0x00, 0x00, 0x38, 0x03, 0x00, 0x7C, 0x00, 0x00, 0xF8, 0x07, 0xF8, 0x3F, 0xC0, 0x01, 0xF0, 0x3F, 0xFE, 0x3F, 0xF8, 0x03, 0xC1, 0xFF, 0x0F, 0x1F, 0xF8, 0x00, 0x01, 0xE3, 0x0F, 0x0F, 0xF0, 0x00, 0x01, 0xC3, 0x0E, 0x00, 0x00, 0x00, 0x01, 0x83, 0xFC,0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };char zuo16H[] ={0x18, 0xC0, 0x18, 0xC0, 0x19, 0x80, 0x31, 0xFE, 0x33, 0xFE, 0x76, 0xC0, 0xF0, 0xFC, 0xB0, 0xFC, 0x30, 0xC0, 0x30, 0xC0, 0x30, 0xFE, 0x30, 0xFE, 0x30, 0xC0, 0x30, 0xC0, 0x30, 0xC0, 0x00, 0x00, };char zhe16H[] ={char tian16H[] ={0x00, 0x00, 0x3F, 0xFC, 0x3F, 0xFC, 0x31, 0x8C, 0x31, 0x8C, 0x31, 0x8C, 0x3F, 0xFC, 0x3F, 0xFC, 0x31, 0x8C, 0x31, 0x8C, 0x31, 0x8C, 0x3F, 0xFC, 0x3F, 0xFC, 0x30, 0x0C, 0x00, 0x00, 0x00, 0x00,};char xue16H[] ={0x33, 0x18, 0x19, 0x98, 0x08, 0xB0, 0x7F, 0xFC,0x7F, 0xFC, 0x60, 0x0C, 0x1F, 0xF0, 0x1F, 0xF0,0x00, 0xC0, 0x7F, 0xFC, 0x7F, 0xFC, 0x01, 0x80,0x01, 0x80, 0x07, 0x80, 0x03, 0x00, 0x00, 0x00,};char ke16H[] ={struct Food/*定义结构体存储食物的属性*/{int x; /* 食物的坐标*/int y;int yes; /* 值为0表示屏幕上没有食物,值为1表示屏幕上有食物*/int color; /* 食物颜色*/} food;struct Snake/*定义结构体存储蛇的属性*/{int x[N]; /* 每一节蛇的坐标*/int y[N];int color[N];/*存储每一节蛇的颜色*/*/图形驱动*/int driver = DETECT, mode = 0;registerbgidriver(EGA VGA_driver);initgraph(&driver, &mode, "");}void drawmat(char *mat, int matsize, int x, int y, int color) /*汉字点阵*/{int i, j, k, m;m = (matsize - 1) / 8 + 1;for(j = 0; j < matsize; j++)for(i = 0; i < m; i++)for(k = 0; k < 8; k++)if(mat[j*m+i]&(0x80 >> k))}*/drawmat(tun48H, 48, 409, -4, 7);drawmat(dan48H, 48, 489, -4, 7);drawmat(cai48H, 48, 250, -5, 4);drawmat(she48H, 48, 330, -5, 4);drawmat(tun48H, 48, 410, -5, 4);drawmat(dan48H, 48, 490, -5, 4);/*作者田学科*/drawmat(zuo16H, 16, 515, 465, 7);drawmat(zhe16H, 16, 530, 465, 7);drawmat(tian16H, 16, 550, 465, 7);drawmat(xue16H, 16, 565, 465, 7);drawmat(ke16H, 16, 580, 465, 7); }{for(i = 30; i <= 600; i += 10){rectangle(i, 40, i + 10, 49);rectangle(i, 451, i + 10, 460);}for(i = 40; i < 450; i += 10){rectangle(30, i, 39, i + 10);rectangle(601, i, 610, i + 10);}}void prscore(void){/*outtextxy(55, 15, str);}void gameover(void){cleardevice(); /* 清屏函数*/for(i = 0; i < snake.node; i++) /* 画出蛇死时的位置*/{setcolor(snake.color[i]);rectangle(snake.x[i], snake.y[i], snake.x[i] + 10, snake.y[i] + 10);}prscore(); /* 显示分数*/draw();showword();void gameplay(void)/* 玩游戏的具体过程*/{int flag, flag1;randomize();prscore();gamespeed = 50000;food.yes = 0; /* food.yes=0表示屏幕上没有食物*/ snake.life = 1; /* snake.life=1表示蛇是活着的*/ snake.direction = 4; /* 表示蛇的初始方向为向右*/ snake.node = 2; /* 蛇的初始化为两节*/snake.color[0] = 2; /*两节蛇头初始化为绿色*/ snake.color[1] = 2;snake.x[0] = 100;snake.y[0] = 100;snake.x[1] = 110;如果蛇活着*/{while(1){flag = 1;food.yes = 1;food.x = random(56) * 10 + 40;food.y = random(40) * 10 + 50;for(i = 0; i < snake.node; i++){if(food.x == snake.x[i] && food.y == snake.y[i])flag = 0;}if(flag) break;}}{{snake.x[i] = snake.x[i-1];snake.y[i] = snake.y[i-1];}switch(snake.direction){case 1:snake.y[0] -= 10;break;case 2:snake.y[0] += 10;break;case 3:snake.x[0] -= 10;{if(snake.x[i] == snake.x[0] && snake.y[i] == snake.y[0]) {gameover();snake.life = 0;break;}}if(snake.x[0] < 40 || snake.x[0] > 590 || snake.y[0] < 50 || snake.y[0] > 440){gameover();snake.life = 0;}蛇吃掉setcolor(0);rectangle(food.x, food.y, food.x + 10, food.y + 10);snake.x[snake.node] = -20;snake.y[snake.node] = -20;snake.color[snake.node] = food.color;snake.node++;food.yes = 0;food.color = random(15) + 1;score += 10;prscore();if(score % 100 == 0 && score != 0){for(i = 0; i < snake.node; i++) /* 画出蛇*/{setcolor(snake.color[i]);delay(50000);delay(50000);delay(50000);delay(50000);delay(50000);nosound();gamespeed -= 5000;draw();}else{sound(500);delay(500);nosound();}}{delay(gamespeed);delay(gamespeed);flag1 = 1;setcolor(0);rectangle(snake.x[snake.node-1], snake.y[snake.node-1], snake.x[snake.node-1] + 10, snake.y[snake.node-1] +10);if(kbhit() && flag1 == 1) /*如果没按有效键就重新开始循环*/{flag1 = 0;key = bioskey(0);if(key == esc)exit(0);snake.direction = 3;snake.direction = 4;}}if(snake.life == 0) /*如果蛇死了就退出循环*/break;}}void main(void){while(1){color = 0;init();cleardevice();{key = bioskey(0);if(key == Y || key == n || key == esc)break;}if(key == n || key == esc)break;}closegraph();}3、c语言实现移动电话系统#include <stdio.h>#define GRID-SIZE 5#define SELECTED -1 /*#define TRAFFIC-FILE “traffic.dat”/*main(void){int commuters[GRID-SIZE][GRID-SIZE];/*上午8:30的交通数据*/int salesforce[GRID-SIZE][GRID-SIZE]; /*上午11:00的交通数据*/int weekend[GRID-SIZE][GRID-SIZE];/*周末交通数据*/ int commuter-weight, /*通勤人员数据的权重因子*/sale-weight,/*营销人员数据的权重因子*/weekend-weight;/*周末数据的权重因子*/int location-i,/*每个发射器的位置*/location-j;int current-max;/*和数据中当前的最大值*/int i,j,/*矩阵的循环计数器tr;/*发射器的循环计数器/*Printf(“8DATA 、\n\n”)n”);n”);printf_matrix(weekeng);/*请用户输入权重因子*/printf(“\n\nPlease input the following value:\n”);printf(“Weight (an interger>=0) for the 8:30 muter data>”) scanf(“%d”,&commuter_weight);printf(“weight(an integer>=0) for the weekeng data>”);scanf(“%d”,&weekend_weight);scanf(“%d”,&weekend_weight);/*计算并显示加权后求和的数据*/for (i=0;i<GRID_SIZE;++i)for (j=0;j<GRID_SIZE;++j)printf(“个最大中,然后把最后*/of the %d transmitters:\n\n”,NUM_TRANSMITTERS);for (tr=1;tr<=NUM_TRANSMITTERS;++tr){current_max=SELECTED;/*以一个过低的值为起点开始查找*/for (i=0;i<GRID_SIZE;++i){for(j=0;j<GRID_SIZE;++j){if(current_max<summed_data[i][j]){current_max=summed_data[i][j]){location_i=i;location_j=j;}}}/*\n”,/**把TRAFFIC_FILE中的交通数据填充到3个GRID_SIZE×GRID_SIZE数组中*/voidget_traffic_data(int commuters[GRID_SIZE],/*输出*/int salesforce[GRID_SIZE][GRID_SIZE],/*输出*/int weekend[GRID_SIZE][GRID_SIZE],/*输出*/ {int i,j; /*循环计数器*/FILE *fp; /*文件指针*/fq=fopen(TRAFFIC_FILE,“r”);for(i=0;i<GRID_SIZE;++i)for(j=0;j<GRID_SIZE;++j)}/**显示一个GRID_SIZE×GRID_SIZE整数矩阵的内容*/voidprint_matrix(int matrix[GRID_SIZE][GRID_SIZE]){int i,j; /*循环计数器*/for(i=0;i<GRID_SIZE;++j){ for(j=0;j<GRID_SIZE;++J)printf(“%3d”,matrix[i][j]);printf(“\n”);}}4、,然后电脑,然后猜出你记住的那张牌.History:修改历史**************************************/#include <stdio.h>#include <stdlib.h>#include <string.h>#include <time.h>#include <assert.h>#define CARDSIZE 52 /*牌的总张数*/#define SUITSIZE 13 /*一色牌的张数*//*扑克牌结构*/typedef struct Card{char val;/*扑克牌面上的大小*/int kind :4; /*扑克牌的花色*/}Card;张牌,要求九张牌不能// main()被修改的表(此项仅对于牵扯到数据库操作的程序)Table Updated: // 被修改的表(此项仅对于牵扯到数据库操作的程序)Input: //Card card[] 牌结构, int size 结构数组的大小Output: //Return: // voidOthers: // 此函数修改card[]的值,希望得到九张随机牌Bug: //此函数有bug,有时会产生两个相同的牌,有待修订*************************************************/ void riffle(Card *cards, int size);/************************************************* Function: // showDescription: // 显示数组的内容Calls: //的程序)//Card *card 牌结构指针, int size 结构数组的大Output: //Return: // voidOthers: //*************************************************/ void show(const Card *cards, int size);/*************************************************Function: // groupingDescription: //把9张牌分别放到3个数组中,每组3张,a.e分组Calls: //Called By: // main()Table Accessed: //被修改的表(此项仅对于牵扯到数据库操作的程序)Table Updated: // 被修改的表的程序)小的值Card *carr1, Card *carr2, Card *carr3);/************************************************* Function: // result_processDescription: //用递归计算,所选的牌Calls: // rshiftCalled By: // main()Table Accessed: //被修改的表(此项仅对于牵扯到数据库操作的程序)Table Updated: // 被修改的表(此项仅对于牵扯到数据库操作的程序)Input: //Card *carr1, Card *carr2, Card *carr3Output: //Return: // voidOthers: // 此函数修改Card*counter);//// result_processTable Accessed: //被修改的表(此项仅对于牵扯到数据库操作的程序)Table Updated: // 被修改的表(此项仅对于牵扯到数据库操作的程序)Input: //Card *carr1, Card *carr2, Card *carr3 ,int counter Output: //Return: // Card*Others: // 此函数修改*carr1,*carr2,* carr3的值*************************************************/ void rshift(Card *carr1, Card *carr2, Card *carr3, int counter); void main(){Card cards[9]; /*存放九张牌*/Card carr1[3]; /*第一组牌,cards array 1*/Card carr2[3]; /*第二组牌,cards array 2*/的牌*/洗牌,得到九张牌*/!最多经过下面三次我与你的对话,我就会知道你所记的那张牌!");puts("如果想继续玩,请准确的回答我问你的问题,根据提示回答!");puts("请放心,我不会问你你选了哪张牌的!");grouping(cards, carr1, carr2, carr3); /*把9张牌分别放到3个数组中,每组3张,a.e分组*/show(carr1, 3);show(carr2, 3);show(carr3, 3);puts("请告诉我你记住的那张牌所在行数"); select = getchar();switch(select)/*分支猜你玩家记住的牌*/ {case '1':break;case '2':default:puts("你在撒谎!不和你玩了!"); fflush(stdin);getchar();exit(0);}if( selected_card ==NULL){fflush(stdin);getchar();exit(0);}puts("你猜的牌为:");show(selected_card, 1);puts("我猜的对吧,哈哈~~~~");getchar();}临时数组,用于存储牌*/ unsigned int seed;/*最为产生随机数的种的*/int deckp = 0; /*在牌的产生中起着指示作用*/seed = (unsigned int)time(NULL);srand(seed);/*洗牌*/while (deckp < CARDSIZE){char num = rand() % CARDSIZE;if ((memchr(deck, num, deckp)) == 0){assert(!memchr(deck,num,deckp));deck[deckp] = num;deckp++;}}cards[deckp].val = "A23456789TJQK"[card.rem]; /*把余数给card.val*/cards[deckp].kind = "3456"[card.quot]; /*把商给card.kind*/}}/*show的原代码,将会自动换行*/void show(const Card *cards, int size){for(int i = 0; i < size; i++){printf("%c%c ",cards[i].kind,cards[i].val);if( (i !=0) && (((i+1 ) % 3) == 0))puts("");}puts(""); /*自动换行*/}void Card *carr2, Card*//*分给carr1三个数*/while (i < 3){carr1[i].val = cards[i].val;carr1[i].kind = cards[i].kind;i++;}/*分给carr2接下来的三个数*/while (i < 6){carr2[i-3].val = cards[i].val;carr2[i-3].kind = cards[i].kind;i++;}/*分给carr3接下来的三个数*/{/*rshift的实现*/void rshift(Card *carr1, Card *carr2, Card *carr3, int counter) {Card temp2;/*用于存放carr2[counter]*/Card temp3;/*用于存放carr3[counter]*//*temp = carr2*/temp2.val = carr2[counter].val;temp2.kind = carr2[counter].kind;/*carr2 = carr1*/carr2[counter].val = carr1[counter].val;carr2[counter].kind = carr1[counter].kind;/*temp3 = carr3*/temp3.val = carr3[counter].val;}Card* result_process(Card *carr1, Card *carr2, Card *carr3, int counter){rshift(carr1, carr2, carr3, counter); /* 把数组的第一个元素依次右移*/if(counter == 2){return(&carr2[2]);}show(carr1, 3);show(carr2, 3);show(carr3, 3);puts("请给出你记住的牌所在行数:");fflush(stdin);case '2':return(&carr2[counter]);break;default:puts("你在撒谎!不和你玩了!"); return NULL;}}5、C语言实现打字游戏#include "stdio.h"#include "time.h"#include "stdlib.h"#include "conio.h"#include "dos.h"#define xLine 70#define yLine 20#define full 100#define true 1#define false 0--*/void新屏幕的输出图像{int i,j;clrscr();输出现在的for(j=0;j<xLine;j++)printf ("%c",p[i][j]);printf("\n");}/* for (i) */printf("----------------------------------------------------------------------\n");}/* printScreen *//*---------------------------------------------------------------------*/void leave()/* 离开程序时,调用该函数结束程序。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
/* A simple game*//*CopyRight: Guanlin*/ #include<stdio.h>#include<stdlib.h>#include<string.h>#include<time.h>#include<conio.h>#include<process.h>struct object_fix{char name[20];char id[5];char desc[500];char action[30];char im[5];};struct object_move {char name[20];char id[5];char desc[500];int loc;int pwr;int strg;char im[5];};struct rover{char name[20];char id[5];char desc[500];int pwr;int strg;int location[2];char im[5];};struct map /* this is the map structure*/{char data[20];char add_data[20];int amount;int x; /* this were the successor keeps it's x & y values*/int y;};struct location /*this structure is for the successor lister*/{float height;char obj;};void stats_update(int selected, struct rover *p_rover){switch (selected){case 1:if(p_rover->pwr < 7)printf("\n\nYou do not have enough power to perform this action!\n\n"); else(p_rover->pwr) -= 7;printf("You have destroyed the object!\n\n");}break;case 2:if(p_rover->pwr < 3)printf("\n\nYou do not have enough power to perform this action!\n\n");else if(p_rover->strg > 90)printf("\n\nYou do not have enough storage space for this object!\n\n");else{(p_rover->pwr) -= 3;(p_rover->strg) += 10;printf("You have collected a sample of the object!\n\n");}break;case 3:p_rover->pwr -= 10; /*Distance around object- value gained from mapper module. 1 square = -1 power*/printf("You have avoided the object!\n\n");break;case 4:p_rover->pwr -= 2;printf("You have driven through the obstacle!\n\n");break;case 5:if(p_rover->pwr == 100)printf("\n\nYou do not need to charge up!\n\n");elsep_rover->pwr = 100;printf("You have charged up your rover!\n\n");}break;default:printf("\n\n*****ERROR*****\nInvalid Selection\n\n");break;}}void action(char object, struct rover *p_rover){int selection;switch(object){case 1:printf("\nYou have encountered: A Sandy Rock\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\nPlease choose action 1 or2:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 2:printf("\nYou have encountered: A Solid Rock\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 3:printf("\nYou have encountered: A Mountain\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 4:printf("\nYou have encountered: Dust\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 5:printf("\nYou have encountered: A Sheer Valley\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 6:printf("\nYou have encountered: A Gentle Valley\n\n");printf("This object can be:\n1.\tDriven through\n2.\tAvoided\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;case 7:printf("\nYou have encountered: A 'Martian' Tree\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 8:printf("\nYou have encountered: Shallow Water\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 9:printf("\nYou have encountered: Deep Water\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 10:printf("\nYou have encountered: An Aggressive Alien\n\n");printf("This object can be:\n1.\tDestroyed\nPlease enter 1:\t");scanf("%d", &selection);selection = 1;stats_update(selection, p_rover);break;case 11:printf("\nYou have encountered: A Non-Aggressive Alien\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 12:printf("\nYou have encountered: Another Rover\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 13:printf("\nYou have encountered: A Power Station\n\n");printf("You can:\n1.\tCharge up\n2.\tAvoid\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 5;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;default:printf("\n\n*****ERROR*****\n\n");break;}}void show_map(struct map *number, struct map *number_2, struct object_fix *rsny, struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /*the show map function calling number and number_2 from the map structure to see if moveable objects are needed*/{struct map f_map[8][8]; /*8 by 8 map*/int i,j, rx, ry, object; /*this is your x and y value in your map (f_map)*/system("cls"); /* this is your x and y value in your map (f_map)*///srand(time(NULL)); /*calling the time from include to gather random variables*/for(i=0;i<8;i++) /*for loop to copy all your fixed object lists into f_map so they can be displayed*/{for(j=0;j<8;j++)strcpy(f_map[i][j].data,"");}if (number->amount>0) /*this is looking at the add function to see weather or not there are aliens in f_map*/{for(i=0;i<number->amount;i++) /*allocating the number of aliens in f_map*/strcpy(f_map[rand()%8][rand()%8].data, anon->im); /*randomizing their position*/}if(number_2->amount>0) /*repeat of above just for rovers instead*/{for(i=0;i<number_2->amount;i++)strcpy(f_map[rand()%8][rand()%8].data, rvr->im); }rx= p_rover->location[0];ry= p_rover->location[1];strcpy(f_map[1][0].data, wdp->im);strcpy(f_map[4][0].data, mnt->im);strcpy(f_map[5][0].data, mnt->im);strcpy(f_map[3][1].data, dst->im);strcpy(f_map[4][1].data, dst->im);strcpy(f_map[3][2].data, dst->im);strcpy(f_map[4][2].data, dst->im);strcpy(f_map[0][3].data, mnt->im);strcpy(f_map[1][3].data, mnt->im);strcpy(f_map[3][3].data, vshr->im);strcpy(f_map[4][3].data, dst->im);strcpy(f_map[5][3].data, vgnt->im);strcpy(f_map[3][4].data, vshr->im);strcpy(f_map[4][4].data, dst->im);strcpy(f_map[5][4].data, vgnt->im);strcpy(f_map[2][5].data, wshl->im);strcpy(f_map[3][5].data, wshl->im);strcpy(f_map[4][5].data, wshl->im);strcpy(f_map[1][6].data, pstn->im);strcpy(f_map[2][6].data, wdp->im);strcpy(f_map[3][6].data, wdp->im);strcpy(f_map[4][6].data, wshl->im);strcpy(f_map[7][6].data, mnt->im);strcpy(f_map[0][7].data, mnt->im);strcpy(f_map[1][7].data, wdp->im);strcpy(f_map[2][7].data, wshl->im);strcpy(f_map[3][7].data, wshl->im);strcpy(f_map[6][7].data, mnt->im);strcpy(f_map[rx][ry].data, p_rover->im);if((rx == 1 && ry == 0) || (rx == 2 && ry == 6) ||(rx == 3 && ry == 6) ||(rx == 1 && ry == 7)){object = 9;action(object, p_rover);}else if((rx == 4 && ry == 0) || (rx == 5 && ry == 0) || (rx == 0 && ry == 3) || (rx == 1 && ry == 3) || (rx == 7 && ry == 6) || (rx == 0 && ry == 7) || (rx == 6 && ry == 7)){object = 3;action(object, p_rover);}else if((rx == 3 && ry== 1) || (rx == 4 && ry == 1) || (rx == 3 && ry== 2) || (rx == 4 && ry == 2) || (rx == 4 && ry == 3) || (rx == 4 && ry == 4)){object = 4;action(object, p_rover);}else if((rx == 3 && ry == 3) || (rx == 3 && ry == 4)){object = 5;action(object, p_rover);else if((rx == 5 && ry == 3) || (rx == 5 && ry == 4)){object = 6;action(object, p_rover);}else if((rx == 2 && ry == 5) || (rx == 3 && ry == 5 ) || (rx == 4 && ry == 5) || (rx == 4 && ry == 6) ||(rx == 2 && ry == 7) || (rx == 3 && ry == 7)){object = 8;action(object, p_rover);}else if(rx == 1 && ry == 6){object = 13;action(object, p_rover);}i=0; /*re-allocate i to 0 so map is printed from start*/for(i=0;i<8;i++) /*8 by 8 map*/{printf("+----+----+----+----+----+----+----+----+\n");for(j=0;j<8;j++) /*8 by 8 map*/{if(strlen(f_map[i][j].data)!=0) /*if function to print nothing but 4 spaces if there in nothing allocated in [i][j]*/printf("|%4s",f_map[i][j].data);elseprintf("| "); /*end of coloumn visible map*/printf("|\n"); /*end of last coloumn visible map*/}printf("+----+----+----+----+----+----+----+----+\n"); /*bottom of map*/printf("\n");printf("\270");printf("Group B\n");}void add_obj(struct map *number, struct map *number_2, struct object_fix *rsny, struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /* add movable object function, *number=aliens, *number_2=rovers*/ {int object, t, f;char alien;char rover;printf("This is the add movable object function\n");printf("how many aliens would you like?\n");scanf("%d",&t); /*user input of amount of aliens*/number->amount=t; /*saving the number in structure map-amount*/printf("how many rovers would you like?\n");scanf("%d",&f); /*user input of amount of rovers*/number_2->amount=f; /*saving the number in structure map-amount*/show_map(number, number_2, rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl,wdp, aagr, anon, rvr, pstn, p_rover); /*go to show map function with the number of aliens and number of rovers*/}void successor() /*this functions askes the user for the location and then were they want to go from there, printing out that location*/{struct map location;int menu;int ncol,nrow; /*we can change the n value depends how large the map you need.*/ ncol=8;nrow=8;printf("This is the successor function\n");printf("Enter the \nx=\ty=\n");scanf("%d%d",&location.x,&location.y); /*saving location in map location x and y*/ printf("Enter operator choice\n");printf("1=left\n");printf("2=right\n");printf("3=upwards\n");printf("4=down\n");printf("5=quit\n");scanf("%d",&menu);switch(menu) /*menu switch to show the position above, underneith, and next to the rover*/{case 1:location.x=location.x-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 2:location.x=location.x+1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 3:location.y=location.y-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 4:location.y=location.y+1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 5:printf("*****back to program*****\n"); /* Quits the program and prints out the message */break;default:printf("*****Please enter an integer from 1-5*****\n"); /* A default option if the user enters an incorrect value */break;}printf("\n");}void help(struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /*help function*/int i;char mov_obj;printf("++This is the help function++\n");printf("| 1-successor |\n");printf("| 2-add movable obj |\n");printf("| 3-remove moveabla obj |\n\n");printf("\t* * *\n");printf("\t Index: \n\n");printf("\t Sandy Rock: *\n");printf("\t Solid Rock: o\n");printf("\t Water:\262\262\262\262 \n");printf("\t Shallow water: \260\260\260\260\n");printf("\t Mountain: ^^^^\n");printf("\t Dust: ....\n");printf("\t* sher valley: VVVV *\n");printf("\t shallow valey: vvvv\n");printf("\t power station: \025\n");printf("\t alien: *_*\n");printf("\t other rovers: #\n");printf("\t* * *\n");scanf("%d",&i);switch(i) /*switch menu to go to the successor function, or the add/remove function*/ {case 1:successor();break;case 2:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);break;case 3:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);break;default:printf("choose a number from the list\n");}}void direction(int input, struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover){switch(input){case 119: /*w = up*/if(p_rover->location[0] > 0){p_rover->location[0]--;p_rover->pwr--;}else p_rover->location[0]=7;break;case 115: /*s = down*/if(p_rover->location[0] < 7)p_rover->location[0]++;p_rover->pwr--;}else p_rover->location[0]=0; break;case 97: /*a = left*/if(p_rover->location[1] > 0) {p_rover->location[1]--;p_rover->pwr--;}else p_rover->location[1]=7; break;case 100: /*d = right*/if(p_rover->location[1] < 7) {p_rover->location[1]++;p_rover->pwr--;}else p_rover->location[1]=0; break;default:printf("Invalid operator!\n\n"); break;}}int control(int input){input = _getch();return input;void main(void){int menu;int quit = 0;int input = 0;struct object_fix rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl, wdp;struct object_move aagr, anon, rvr, pstn;struct rover p_rover;struct map number, number_2;number.amount = 0; /* setting the initial amount of aliens as 0*/number_2.amount = 0;strcpy(,"Sandy Rock");strcpy(rsny.id,"RSNY");strcpy(rsny.desc,"A rock made of softer material that can be destroyed or collected"); strcpy(rsny.im,"*");strcpy(,"Solid Rock");strcpy(rsld.id,"RSLD");strcpy(rsld.desc,"A rock made of hard material that cannot be destroyed, but can be collected or avoided");strcpy(rsld.im,"O");strcpy(,"Mountain");strcpy(mnt.id,"MNT");strcpy(mnt.desc,"A large obstacle made of different materials that can only be avoided"); strcpy(mnt.im,"^^^^");strcpy(,"Dust");strcpy(dst.id,"DST");strcpy(dst.desc,"Small particles of sand, rock, etc. that may reduce visibility or slow down the vehicle, can be driven through but has extra power consumption");strcpy(dst.im,"....");strcpy(,"Sheer Valley");strcpy(vshr.id,"VSHR");strcpy(vshr.desc,"A depression in the land with sheer sides, it cannot be driven through and must be avoided");strcpy(vshr.im,"VVVV");strcpy(,"Gentle Valley");strcpy(vgnt.id,"VGNT");strcpy(vgnt.desc,"A depression in the land with gentle sides, it can be driven through with extra power consumption or can be avoided");strcpy(vgnt.im,"vvvv");strcpy(,"'Martian' Tree");strcpy(mtre.id,"MTRE");strcpy(mtre.desc,"A woody plant native to Mars, it may have the same characteristics as an 'Earth' tree");strcpy(mtre.im,"\330");strcpy(,"Shallow Water");strcpy(wshl.id,"WSHL");strcpy(wshl.desc,"A shallow body of water that can be driven through, can be in the form of a small puddle or a large lake");strcpy(wshl.im,"\260\260\260\260");strcpy(,"Deeper Water");strcpy(wdp.id,"WDP");strcpy(wdp.desc,"A deeper body of water that cannot be driven through, commonly in the form of a large lake or river");strcpy(wdp.im,"\262\262\262\262");strcpy(,"Aggressive Alien");strcpy(aagr.id,"AAGR");strcpy(aagr.desc,"An alien creature that will move upon its own accord, may become aggressive on contact");strcpy(aagr.im,"*_*");strcpy(,"Non-Aggressive Alien");strcpy(anon.id,"ANON");strcpy(anon.desc,"An alien creature that will move upon its own accord, will not become aggressive on contact");strcpy(anon.im,"*_*");strcpy(,"Rover");strcpy(rvr.id,"RVR");strcpy(rvr.desc,"A Mars Rover vehicle to transport around the map, it can destroy, collect or avoid objects. It has a power and object storage");strcpy(rvr.im,"#");strcpy(,"Power Station");strcpy(pstn.id,"PSTN");strcpy(pstn.desc,"A movable power station that will restore power to the Mars Rovers"); strcpy(pstn.im,"\025");strcpy(p_,"User Mars Rover");strcpy(p_rover.id,"URVR");strcpy(p_rover.desc,"A Mars vehicle that is controlled by the user. Explores the map collecting, destroying and avoiding objects");strcpy(p_rover.im,"<^^>");p_rover.pwr = 100;p_rover.strg = 0;p_rover.location[0] = 7;p_rover.location[1] = 0;/****************************************************/printf("*******************************START***************************** ***\n\n");do{ /* Object will be gained from mapper */printf("+++++++++++++++++++++++++++++++MENU++++++++++++++++ +++++++++++++++++\n");printf("Please choose one of the following option:\n");printf("1 - Start\n");printf("2 - Controls\n");printf("3 - Show Map\n");printf("4 - Help Menu\n");printf("5 - Quit\n\n");printf("--------------->");scanf("%d", &menu);switch (menu){case 1:show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);while (quit != 1 ){input = control(input);if (input == 27)quit = 1;else{if(p_rover.pwr>0){direction(input, &number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);printf("Rover stats: \nPower: %d%% \nStorage: %d%%\n", p_rover.pwr, p_rover.strg);}else{printf("*******************END GAME********************\n\n"); // ends game when charge = 0.break;}}}break;case 2:quit = 0;printf("\n");printf("* * *\n");printf(" Controls \n"); // menu for the keys used on the keyboard to move the rover in different directions.printf(" Up : W \n");printf(" Down : S \n");printf("* Left : A * \n");printf("* Right : D * \n");printf(" choose : Enter \n");printf("* * *\n");break;case 3:quit = 0;show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);break;case 4:quit = 0;help(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);break;case 5:printf("*********************END***********************\n"); break;}}while(menu!=5);printf("\n -----Please press any key to exit game-----\n");getch();}。