酒店管理系统实现代码
酒店管理系统代码(C语言版)
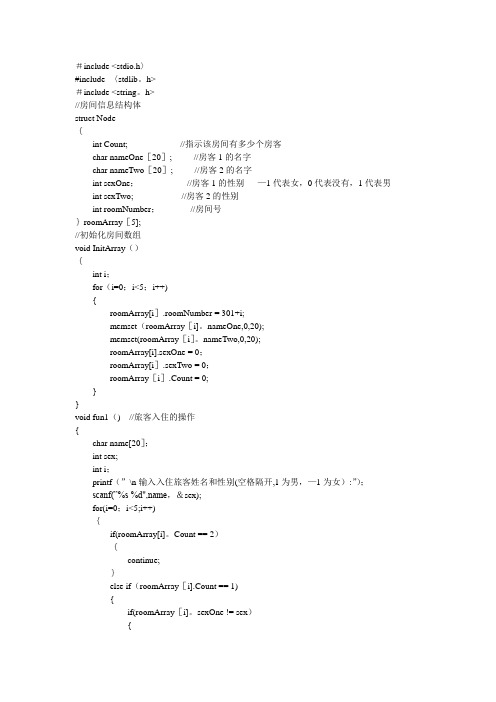
#include <stdio.h〉#include 〈stdlib。
h>#include <string。
h>//房间信息结构体struct Node{int Count; //指示该房间有多少个房客char nameOne[20]; //房客1的名字char nameTwo[20]; //房客2的名字int sexOne;//房客1的性别—1代表女,0代表没有,1代表男int sexTwo; //房客2的性别int roomNumber;//房间号}roomArray[5];//初始化房间数组void InitArray(){int i;for(i=0;i<5;i++){roomArray[i].roomNumber = 301+i;memset(roomArray[i]。
nameOne,0,20);memset(roomArray[i]。
nameTwo,0,20);roomArray[i].sexOne = 0;roomArray[i].sexTwo = 0;roomArray[i].Count = 0;}}void fun1() //旅客入住的操作{char name[20];int sex;int i;printf(”\n输入入住旅客姓名和性别(空格隔开,1为男,—1为女):”);scanf(”%s %d",name,&sex);for(i=0;i<5;i++){if(roomArray[i]。
Count == 2){continue;}else if(roomArray[i].Count == 1){if(roomArray[i]。
sexOne != sex){continue;}strcpy(roomArray[i].nameTwo,name);roomArray[i].sexTwo = sex;roomArray[i]。
Count++;system("cls”);printf("客人已经成功入住,在房间%d”,roomArray[i].roomNumber);return;;}else{strcpy(roomArray[i]。
酒店管理系统c语言程序设计
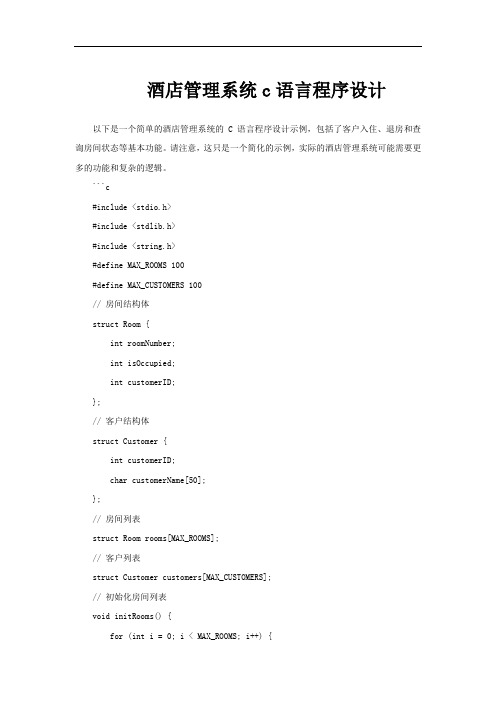
酒店管理系统c语言程序设计以下是一个简单的酒店管理系统的 C 语言程序设计示例,包括了客户入住、退房和查询房间状态等基本功能。
请注意,这只是一个简化的示例,实际的酒店管理系统可能需要更多的功能和复杂的逻辑。
```c#include <stdio.h>#include <stdlib.h>#include <string.h>#define MAX_ROOMS 100#define MAX_CUSTOMERS 100// 房间结构体struct Room {int roomNumber;int isOccupied;int customerID;};// 客户结构体struct Customer {int customerID;char customerName[50];};// 房间列表struct Room rooms[MAX_ROOMS];// 客户列表struct Customer customers[MAX_CUSTOMERS];// 初始化房间列表void initRooms() {for (int i = 0; i < MAX_ROOMS; i++) {rooms[i].roomNumber = i + 1;rooms[i].isOccupied = 0;rooms[i].customerID = 0;}}// 初始化客户列表void initCustomers() {for (int i = 0; i < MAX_CUSTOMERS; i++) {customers[i].customerID = i + 1;strcpy(customers[i].customerName, "Customer");}}// 查找空闲房间int findFreeRoom() {for (int i = 0; i < MAX_ROOMS; i++) {if (rooms[i].isOccupied == 0) {return i;}}return -1;}// 客户入住void checkIn(int customerID, int roomNumber) {int freeRoom = findFreeRoom();if (freeRoom != -1) {rooms[freeRoom].isOccupied = 1;rooms[freeRoom].customerID = customerID;printf("Customer %d checked in to Room %d\n", customerID, roomNumber); } else {printf("No free rooms available\n");}}// 客户退房void checkOut(int roomNumber) {for (int i = 0; i < MAX_ROOMS; i++) {if (rooms[i].roomNumber == roomNumber && rooms[i].isOccupied == 1) { rooms[i].isOccupied = 0;rooms[i].customerID = 0;printf("Customer checked out of Room %d\n", roomNumber);return;}}printf("Room not found\n");}// 查询房间状态void viewRoomStatus(int roomNumber) {for (int i = 0; i < MAX_ROOMS; i++) {if (rooms[i].roomNumber == roomNumber) {if (rooms[i].isOccupied == 1) {printf("Room %d is occupied by Customer %d\n", roomNumber, rooms[i].customerID);} else {printf("Room %d is vacant\n", roomNumber);}return;}}printf("Room not found\n");}// 主函数int main() {initRooms();initCustomers();int choice;while (1) {printf("1. Check In\n2. Check Out\n3. View Room Status\n4. Exit\n"); scanf("%d", &choice);switch (choice) {case 1:int customerID, roomNumber;printf("Enter customer ID: ");scanf("%d", &customerID);printf("Enter room number: ");scanf("%d", &roomNumber);checkIn(customerID, roomNumber);break;case 2:printf("Enter room number: ");scanf("%d", &roomNumber);checkOut(roomNumber);break;case 3:printf("Enter room number: ");scanf("%d", &roomNumber);viewRoomStatus(roomNumber);break;case 4:exit(0);break;default:printf("Invalid choice\n");}}return 0;}```上述代码实现了一个简单的酒店管理系统,包括客户入住、退房和查询房间状态等功能。
酒店管理系统代码

#include <windows.h〉#include <dos。
h>#include〈stdio.h〉#include〈stdlib。
h〉#include<time。
h〉//—-—-—-—-——--———---------————--—---—---—--——-———-——结构定义-————-—-——-----—--———-—-—-—--—-—--—————-—-typedef struct CheckinInformation{char name[10];//姓名int id;//证件号int roomType;//房型int countType;//计费方式}CheckinInfo;typedef struct HotelRoom{int roomType;//房型int roomNum;//房号int checked; //入住情况int price;//房价}Room;typedef struct RoomOrder{CheckinInfo *checkinInfo;//入住信息long date; //入住时间Room *room; //房间信息}Order;typedef struct HotelInfomation{int checkinAmount; //已入住房数int singleRemainAmount;//单人房剩余房数int doubleRemainAmount; //双人房剩余房数int bigRemainAmount; //大床房剩余房数}HotelInfo;//-—-—-——---————----—--——--——-—-——枚举类型——-—-——------—-———-—-—-——--enum {MainUI,HotelInfoUI,CheckinUI,CheckinResultUI,OrderUI,CheckOutUI,Exit};//GUI enum {Single,Double,Big};//Room Typeenum {Hour,Day};//countType//--——---—————--————-—--—---——-———全局变量-----—--———-—-—-—-——-—-——-int GUI = MainUI;Order*orderList[100];//订单数组Room* roomList[100];//房间数组HotelInfo * hotelInfo = NULL;//酒店房间信息//—————--—-—--——---——-—--—--——-——函数声明———--—-—--——--—————-——---——-void initiallizeRoomList();void insertToOrderList(Order * order);Room* getRoomByType(int roomType);Order*getOrderByRoomNum(int roomNum);void showMainUI();void showHotelInfoUI();void showCheckinUI();void showCheckinResultUI();void showOrderUI();void showCheckOutUI();//-——-—-——-—————--——---—-—————-——Main函数—--———-————-—-——-——---—--—--void main() //主函数{//初始化酒店房间信息hotelInfo = (HotelInfo *)malloc(sizeof(HotelInfo));hotelInfo -〉singleRemainAmount = 20;hotelInfo -〉doubleRemainAmount=40;hotelInfo -〉bigRemainAmount=40;hotelInfo -〉checkinAmount=0;//初始化房间列表initiallizeRoomList();//界面显示while(GUI != Exit){switch(GUI){case MainUI:showMainUI();break;case HotelInfoUI:showHotelInfoUI();break;case CheckinUI:showCheckinUI();break;case CheckinResultUI:showCheckinResultUI();break;case OrderUI:showOrderUI();break;case CheckOutUI:showCheckOutUI();break;default:break;}}}//-——---———-—--—-————-—--——-—————函数定义-——---——-—-—--—---—-———---——void initiallizeRoomList(){//房间数组初始化,初始化的结果是让roomList的数组有100个room指针,而且设置了相应的值int i;Room*newRoom=NULL;for(i=0;i<20;i++) //单人房房间信息初始化{newRoom = ( Room* )malloc(sizeof(Room));roomList[i]= newRoom;roomList[i]-〉checked=0;roomList[i]—〉price=110;roomList[i]—>roomNum=i+1;roomList[i]—>roomType=Single;}for(i=20;i<60;i++) //双人房房间信息初始化{newRoom = ( Room* )malloc(sizeof(Room));roomList[i] = newRoom;roomList[i]->checked=0;roomList[i]->price=180;roomList[i]—>roomNum=i+1;roomList[i]->roomType=Double;}for(i=60;i〈100;i++)//大床房房间信息初始化{newRoom = ( Room* )malloc(sizeof(Room));roomList[i] = newRoom;roomList[i]-〉checked=0;roomList[i]-〉price=180;roomList[i]-〉roomNum=i+1;roomList[i]—>roomType=Big;}}//通过所选择的房型获取空房间,获取房间后将房间信息改为已入住,并减少相应房型的剩余房间数Room*getRoomByType(int roomType){int i;switch(roomType){case Single:for(i=0;i〈20;i++){if(roomList[i]-〉checked == 0){roomList[i]->checked=1;hotelInfo—>singleRemainAmount -- ;hotelInfo-〉checkinAmount++;return roomList[i];}}break;case Double:for(i=20;i<60;i++){if( roomList[i]-〉checked == 0){roomList[i]—〉checked=1;hotelInfo—〉doubleRemainAmount —— ;hotelInfo—〉checkinAmount++;return roomList[i];}}break;case Big:for(i=60;i<100;i++){if(roomList[i]—>checked == 0){roomList[i]—〉checked=1;hotelInfo-〉bigRemainAmount --;hotelInfo—〉checkinAmount++;return roomList[i];}}break;}}//将订单放入订单列表void insertToOrderList(Order * order){int i;for( i = 0;i<100;i++){if( orderList[i] ==NULL ){orderList[i] = order;break;}}}//通过房号查询订单Order*getOrderByRoomNum(int roomNum){int i;for(i=0;i<100;i++){if(orderList[i]->room->roomNum == roomNum){return orderList[i];}}}void showMainUI(){//显示主界面,并接受输入int chooseNum;system(”cls");printf(”\n\n==========================酒店房间登记与计费管理管理系统=======================\t\n\n\n”);printf(”*\t\t\t\t1。
酒店管理系统实现代码

//入住import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A1 extends JFrame implements WindowListener {//定义各个组件JFrame frame = new JFrame ("入住系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("入住房号");JLabel moneyLabel = new JLabel("应付金额");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField moneyField= new JTextField();JButton button1,button2;/*public static void main(String args[]){A1 a1 = new A1();a1.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);//frame.addWindowListener(this);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认支付");button2=new JButton("取消支付");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "付款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认支付"){ JOptionPane.showMessageDialog(p1,"支付成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消支付"){ JOptionPane.showMessageDialog(p1,"支付失败","failure",RMA TION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String room= roomField.getText();String roomword= new String(room);}});moneyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= moneyField.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(moneyLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( moneyField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setSize(200,250);frame.setVisible(true);}public void windowClosing(WindowEvent e1){System.exit(0);}public void windowOpened(WindowEvent e2){}public void windowIconified(WindowEvent e3){}public void windowDeiconified(WindowEvent e4){}public void windowClosed(WindowEvent e5){}public void windowActivated(WindowEvent e6){}public void windowDeactivated(WindowEvent e7){ }}class UpperCaseDocument extends PlainDocument { public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第二个://退房import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A2 extends JFrame {//定义各个组件JFrame frame = new JFrame ("退房系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("退房号");JLabel timeLabel = new JLabel("退房时间");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField timeField= new JTextField();JButton button1,button2;//JTextArea ta = new JTextArea(5,20);/*public static void main(String args[]){A2 a2 = new A2();a2.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(2,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "是否结清消费账单");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"谢谢您的大力支持!","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"请结清消费账单","failure",RMA TION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e){ String room= roomField.getText();String roomword= new String(room);}});timeField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1)); labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1)); fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( timeField);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2)); northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);// frame.pack();frame.setSize(200,230);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第三个://客房预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A3 extends JFrame {//定义各个组件JFrame frame = new JFrame ("客房预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel numberLabel = new JLabel("手机号");JTextField nameField = new JTextField();JTextField numberField= new JTextField();JRadioButton rb1 = new JRadioButton("来电预订");JRadioButton rb2 = new JRadioButton("电话预订");JRadioButton rb3 = new JRadioButton("电传预订");JRadioButton rb4 = new JRadioButton("传真预订");JRadioButton rb5 = new JRadioButton("信函预订");JButton button1,button2;/** public static void main(String args[]){A3 a3 = new A3();a3.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "总统套房", "双人间","单人间" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "预订房类型"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"客人预订成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"客人预订失败","failure",RMA TION_MESSAGE);}}});JPanel p2 = new JPanel();p2.add(rb1);p2.add(rb3);p2.add(rb4);p2.add(rb5);p2.setLayout(new FlowLayout());border = BorderFactory.createTitledBorder(etched, "预订方式"); p2.setBorder(border);//创建ButtonGroup按钮组,并在组中添加按钮ButtonGroup group1 = new ButtonGroup();group1.add(rb1);group1.add(rb2);group1.add(rb3);group1.add(rb4);group1.add(rb5);numberField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String number= numberField.getText();String numberword= new String(number);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(2,1));labelPanel.add(nameLabel);labelPanel.add(numberLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(2,1));fieldPanel.add(nameField);fieldPanel.add(numberField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,330);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet) ;}}第四个//餐饮预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A4 extends JFrame {//定义各个组件JFrame frame = new JFrame ("餐饮预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel timeLabel = new JLabel("预订消费时间");JTextField nameField = new JTextField();JTextField timeField= new JTextField();JCheckBox cb1 = new JCheckBox("中餐");JCheckBox cb2 = new JCheckBox("西餐");JCheckBox cb3 = new JCheckBox("红酒");JCheckBox cb4 = new JCheckBox("饮料");JCheckBox cb5 = new JCheckBox("甜点");JCheckBox cb6 = new JCheckBox("水果");JButton button1,button2;/*public static void main(String args[]){A4 a4 = new A4();a4.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();//ta.append("\nUser Name : "+username);}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "确认订餐"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"订餐成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"订餐失败","failure",RMA TION_MESSAGE);}}});timeField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});JPanel p2 = new JPanel();p2.add(cb1);p2.add(cb2);p2.add(cb3);p2.add(cb4);p2.add(cb5);p2.add(cb6);Border etched1 = BorderFactory.createEtchedBorder();Border border1 = BorderFactory.createTitledBorder(etched1, "预订内容"); p2.setBorder(border1);//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,350);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第五个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A5 extends JFrame {//定义各个组件JFrame frame = new JFrame ("订单管理");JLabel a1 = new JLabel("订单号");JLabel a2 = new JLabel("订货名称");JLabel a3 = new JLabel("收货地址");JLabel a4 = new JLabel("应收金额");JLabel a5 = new JLabel("客户名称");JLabel a6 = new JLabel("结算方式");JLabel a7 = new JLabel("经办人");JTextField b1 = new JTextField();JTextField b2= new JTextField();JTextField b3= new JTextField();JTextField b4= new JTextField();JTextField b5 = new JTextField();JTextField b6 = new JTextField();JTextField b7 = new JTextField();JButton button1,button2;public static void main(String args[]){A5 a1 = new A5();a1.go();}public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();b1.setDocument(ucDocument);b1.setForeground(Color.black);b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = b1.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认收款");button2=new JButton("取消收款");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "收款方式"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认收款"){ JOptionPane.showMessageDialog(p1,"收款成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消收款"){ JOptionPane.showMessageDialog(p1,"收款失败","failure",RMA TION_MESSAGE);}}});b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String idtify= a1.getText();String idtifyword= new String(idtify);}});b2.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String room= a2.getText();String roomword= new String(room);}});b3.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a3.getText();String idtifyword= new String(money);}});b4.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a4.getText();String idtifyword= new String(money);}});b5.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a5.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(7,1));labelPanel.add(a1);labelPanel.add(a2);labelPanel.add(a3);labelPanel.add(a4);labelPanel.add(a5);labelPanel.add(a6);labelPanel.add(a7);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(7,1));fieldPanel.add(b1);fieldPanel.add(b2);fieldPanel.add(b3);fieldPanel.add( b4);fieldPanel.add( b5);fieldPanel.add( b6);fieldPanel.add( b7);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setSize(200,250);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第六个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.text.*;import javax.swing.border.*;public class F{private JFrame frame;private JButton b1,b2,b3,b4,b5,b6,b7,b8,b9,b10,b11,b12,b13;JTextArea ta = new JTextArea(20,20);JTextArea tb = new JTextArea(20,20);public static void main(String args[]){F that=new F();that.go();}void go(){frame=new JFrame("客房检查");Container contentPane=frame.getContentPane();JPanel P1=new JPanel();P1.setLayout(new GridLayout());b1=new JButton("101 \n单人间");b2=new JButton("102 \n单人间");b3=new JButton("103 \n单人间");b4=new JButton("104 \n单人间");b5=new JButton("105 \n单人间");b6=new JButton("201 \n双人间");b7=new JButton("202 \n双人间");b8=new JButton("203 \n双人间");b9=new JButton("204 \n双人间");b10=new JButton("205 \n双人间");b11=new JButton("301 \n总统套房");b12=new JButton("302 \n总统套房");b13=new JButton("303 \n总统套房");b1.setBackground(Color.cyan);b2.setBackground(Color.cyan);b3.setBackground(Color.cyan);b4.setBackground(Color.cyan);b5.setBackground(Color.cyan);b6.setBackground(Color.magenta);b7.setBackground(Color.magenta);b8.setBackground(Color.magenta);b9.setBackground(Color.magenta);b10.setBackground(Color.magenta);b11.setBackground(Color.pink);b12.setBackground(Color.pink);b13.setBackground(Color.pink);P1.add(b1);P1.add(b2);P1.add(b3);P1.add(b4);P1.add(b5);P1.add(b6);P1.add(b7);P1.add(b8);P1.add(b9);P1.add(b10);P1.add(b11);P1.add(b12);P1.add(b13);tb.append("房间总数:13");tb.append("\n当前占用:");tb.append("\n当前可供:");P1.add(tb);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="101 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n101房间是否打扫:");ta.append("\n101房间里是否有家具损坏:");ta.append("\n101房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="102 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n102房间是否打扫:");ta.append("\n102房间里是否有家具损坏:");ta.append("\n102房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="103 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n103房间是否打扫:");ta.append("\n103房间里是否有家具损坏:");ta.append("\n103房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="104 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n104房间是否打扫:");ta.append("\n104房间里是否有家具损坏:");ta.append("\n104房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="105 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n105房间是否打扫:");ta.append("\n105房间里是否有家具损坏:");ta.append("\n105房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="201 \n双人间"){ta.setText("");JFrame frame1=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n201房间是否打扫:");ta.append("\n201房间里是否有家具损坏:");ta.append("\n201房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame1.setContentPane(p2);frame1.pack();frame1.setVisible(true);}else if(e.getActionCommand()=="202 \n双人间"){ta.setText("");JFrame frame2=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n202房间是否打扫:");ta.append("\n202房间里是否有家具损坏:");ta.append("\n202房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame2.setContentPane(p2);frame2.pack();frame2.setVisible(true);}else if(e.getActionCommand()=="203 \n双人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n203房间是否打扫:");ta.append("\n203房间里是否有家具损坏:");ta.append("\n203房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="204 \n双人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n204房间是否打扫:");ta.append("\n204房间里是否有家具损坏:");。
酒店管理系统实现代码
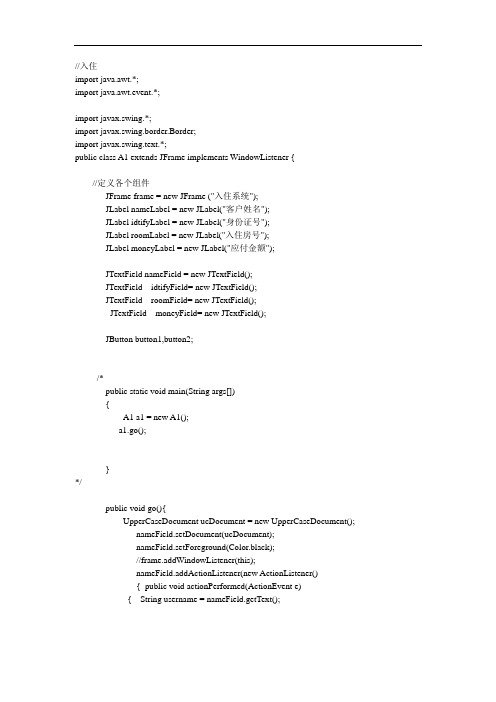
//入住import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A1 extends JFrame implements WindowListener {//定义各个组件JFrame frame = new JFrame ("入住系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("入住房号");JLabel moneyLabel = new JLabel("应付金额");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField moneyField= new JTextField();JButton button1,button2;/*public static void main(String args[]){A1 a1 = new A1();a1.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);//frame.addWindowListener(this);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认支付");button2=new JButton("取消支付");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "付款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认支付"){ JOptionPane.showMessageDialog(p1,"支付成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消支付"){ JOptionPane.showMessageDialog(p1,"支付失败","failure",RMA TION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String room= roomField.getText();String roomword= new String(room);}});moneyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= moneyField.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(moneyLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( moneyField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setSize(200,250);frame.setVisible(true);}public void windowClosing(WindowEvent e1){System.exit(0);}public void windowOpened(WindowEvent e2){}public void windowIconified(WindowEvent e3){}public void windowDeiconified(WindowEvent e4){}public void windowClosed(WindowEvent e5){}public void windowActivated(WindowEvent e6){}public void windowDeactivated(WindowEvent e7){ }}class UpperCaseDocument extends PlainDocument { public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第二个://退房import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A2 extends JFrame {//定义各个组件JFrame frame = new JFrame ("退房系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("退房号");JLabel timeLabel = new JLabel("退房时间");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField timeField= new JTextField();JButton button1,button2;//JTextArea ta = new JTextArea(5,20);/*public static void main(String args[]){A2 a2 = new A2();a2.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(2,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "是否结清消费账单");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"谢谢您的大力支持!","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"请结清消费账单","failure",RMA TION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e){ String room= roomField.getText();String roomword= new String(room);}});timeField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1)); labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1)); fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( timeField);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2)); northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);// frame.pack();frame.setSize(200,230);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第三个://客房预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A3 extends JFrame {//定义各个组件JFrame frame = new JFrame ("客房预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel numberLabel = new JLabel("手机号");JTextField nameField = new JTextField();JTextField numberField= new JTextField();JRadioButton rb1 = new JRadioButton("来电预订");JRadioButton rb2 = new JRadioButton("电话预订");JRadioButton rb3 = new JRadioButton("电传预订");JRadioButton rb4 = new JRadioButton("传真预订");JRadioButton rb5 = new JRadioButton("信函预订");JButton button1,button2;/** public static void main(String args[]){A3 a3 = new A3();a3.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "总统套房", "双人间","单人间" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "预订房类型"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"客人预订成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"客人预订失败","failure",RMA TION_MESSAGE);}}});JPanel p2 = new JPanel();p2.add(rb1);p2.add(rb3);p2.add(rb4);p2.add(rb5);p2.setLayout(new FlowLayout());border = BorderFactory.createTitledBorder(etched, "预订方式"); p2.setBorder(border);//创建ButtonGroup按钮组,并在组中添加按钮ButtonGroup group1 = new ButtonGroup();group1.add(rb1);group1.add(rb2);group1.add(rb3);group1.add(rb4);group1.add(rb5);numberField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String number= numberField.getText();String numberword= new String(number);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(2,1));labelPanel.add(nameLabel);labelPanel.add(numberLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(2,1));fieldPanel.add(nameField);fieldPanel.add(numberField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,330);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第四个//餐饮预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A4 extends JFrame {//定义各个组件JFrame frame = new JFrame ("餐饮预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel timeLabel = new JLabel("预订消费时间");JTextField nameField = new JTextField();JTextField timeField= new JTextField();JCheckBox cb1 = new JCheckBox("中餐");JCheckBox cb2 = new JCheckBox("西餐");JCheckBox cb3 = new JCheckBox("红酒");JCheckBox cb4 = new JCheckBox("饮料");JCheckBox cb5 = new JCheckBox("甜点");JCheckBox cb6 = new JCheckBox("水果");JButton button1,button2;/*public static void main(String args[]){A4 a4 = new A4();a4.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();//ta.append("\nUser Name : "+username);}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "确认订餐"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"订餐成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"订餐失败","failure",RMA TION_MESSAGE);}}});timeField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});JPanel p2 = new JPanel();p2.add(cb1);p2.add(cb2);p2.add(cb3);p2.add(cb4);p2.add(cb5);p2.add(cb6);Border etched1 = BorderFactory.createEtchedBorder();Border border1 = BorderFactory.createTitledBorder(etched1, "预订内容"); p2.setBorder(border1);//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,350);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第五个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A5 extends JFrame {//定义各个组件JFrame frame = new JFrame ("订单管理");JLabel a1 = new JLabel("订单号");JLabel a2 = new JLabel("订货名称");JLabel a3 = new JLabel("收货地址");JLabel a4 = new JLabel("应收金额");JLabel a5 = new JLabel("客户名称");JLabel a6 = new JLabel("结算方式");JLabel a7 = new JLabel("经办人");JTextField b1 = new JTextField();JTextField b2= new JTextField();JTextField b3= new JTextField();JTextField b4= new JTextField();JTextField b5 = new JTextField();JTextField b6 = new JTextField();JTextField b7 = new JTextField();JButton button1,button2;public static void main(String args[]){A5 a1 = new A5();a1.go();}public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();b1.setDocument(ucDocument);b1.setForeground(Color.black);b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = b1.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认收款");button2=new JButton("取消收款");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "收款方式"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认收款"){ JOptionPane.showMessageDialog(p1,"收款成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消收款"){ JOptionPane.showMessageDialog(p1,"收款失败","failure",RMA TION_MESSAGE);}}});b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String idtify= a1.getText();String idtifyword= new String(idtify);}});b2.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String room= a2.getText();String roomword= new String(room);}});b3.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a3.getText();String idtifyword= new String(money);}});b4.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a4.getText();String idtifyword= new String(money);}});b5.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a5.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(7,1));labelPanel.add(a1);labelPanel.add(a2);labelPanel.add(a3);labelPanel.add(a4);labelPanel.add(a5);labelPanel.add(a6);labelPanel.add(a7);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(7,1));fieldPanel.add(b1);fieldPanel.add(b2);fieldPanel.add(b3);fieldPanel.add( b4);fieldPanel.add( b5);fieldPanel.add( b6);fieldPanel.add( b7);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setSize(200,250);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第六个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.text.*;import javax.swing.border.*;public class F{private JFrame frame;private JButton b1,b2,b3,b4,b5,b6,b7,b8,b9,b10,b11,b12,b13;JTextArea ta = new JTextArea(20,20);JTextArea tb = new JTextArea(20,20);public static void main(String args[]){F that=new F();that.go();}void go(){frame=new JFrame("客房检查");Container contentPane=frame.getContentPane();JPanel P1=new JPanel();P1.setLayout(new GridLayout());b1=new JButton("101 \n单人间");b2=new JButton("102 \n单人间");b3=new JButton("103 \n单人间");b4=new JButton("104 \n单人间");b5=new JButton("105 \n单人间");b6=new JButton("201 \n双人间");b7=new JButton("202 \n双人间");b8=new JButton("203 \n双人间");b9=new JButton("204 \n双人间");b10=new JButton("205 \n双人间");b11=new JButton("301 \n总统套房");b12=new JButton("302 \n总统套房");b13=new JButton("303 \n总统套房");b1.setBackground(Color.cyan);b2.setBackground(Color.cyan);b3.setBackground(Color.cyan);b4.setBackground(Color.cyan);b5.setBackground(Color.cyan);b6.setBackground(Color.magenta);b7.setBackground(Color.magenta);b8.setBackground(Color.magenta);b9.setBackground(Color.magenta);b10.setBackground(Color.magenta);b11.setBackground(Color.pink);b12.setBackground(Color.pink);b13.setBackground(Color.pink);P1.add(b1);P1.add(b2);P1.add(b3);P1.add(b4);P1.add(b5);P1.add(b6);P1.add(b7);P1.add(b8);P1.add(b9);P1.add(b10);P1.add(b11);P1.add(b12);P1.add(b13);tb.append("房间总数:13");tb.append("\n当前占用:");tb.append("\n当前可供:");P1.add(tb);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="101 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n101房间是否打扫:");ta.append("\n101房间里是否有家具损坏:");ta.append("\n101房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="102 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n102房间是否打扫:");ta.append("\n102房间里是否有家具损坏:");ta.append("\n102房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="103 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n103房间是否打扫:");ta.append("\n103房间里是否有家具损坏:");ta.append("\n103房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="104 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n104房间是否打扫:");ta.append("\n104房间里是否有家具损坏:");ta.append("\n104房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="105 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n105房间是否打扫:");ta.append("\n105房间里是否有家具损坏:");ta.append("\n105房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="201 \n双人间"){ta.setText("");JFrame frame1=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n201房间是否打扫:");ta.append("\n201房间里是否有家具损坏:");ta.append("\n201房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame1.setContentPane(p2);frame1.pack();frame1.setVisible(true);}else if(e.getActionCommand()=="202 \n双人间"){ta.setText("");JFrame frame2=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n202房间是否打扫:");ta.append("\n202房间里是否有家具损坏:");ta.append("\n202房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame2.setContentPane(p2);frame2.pack();frame2.setVisible(true);}else if(e.getActionCommand()=="203 \n双人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n203房间是否打扫:");ta.append("\n203房间里是否有家具损坏:");ta.append("\n203房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="204 \n双人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n204房间是否打扫:");ta.append("\n204房间里是否有家具损坏:");。
酒店管理系统实现代码
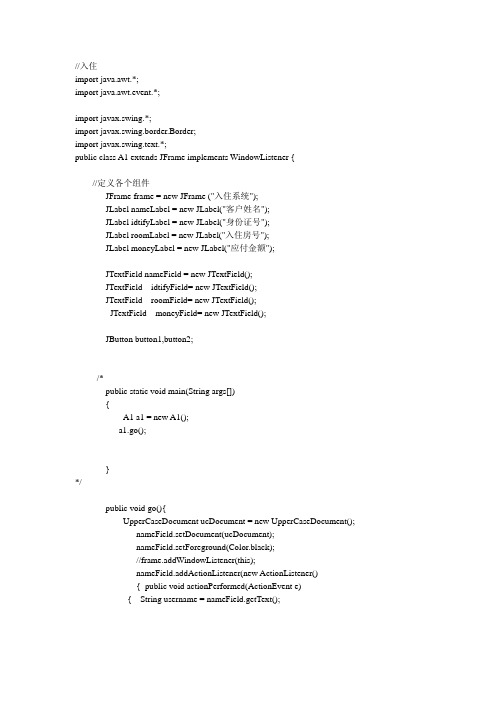
//入住import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A1 extends JFrame implements WindowListener {//定义各个组件JFrame frame = new JFrame ("入住系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("入住房号");JLabel moneyLabel = new JLabel("应付金额");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField moneyField= new JTextField();JButton button1,button2;/*public static void main(String args[]){A1 a1 = new A1();a1.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);//frame.addWindowListener(this);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认支付");button2=new JButton("取消支付");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "付款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认支付"){ JOptionPane.showMessageDialog(p1,"支付成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消支付"){ JOptionPane.showMessageDialog(p1,"支付失败","failure",RMA TION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String room= roomField.getText();String roomword= new String(room);}});moneyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= moneyField.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(moneyLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( moneyField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setSize(200,250);frame.setVisible(true);}public void windowClosing(WindowEvent e1){System.exit(0);}public void windowOpened(WindowEvent e2){}public void windowIconified(WindowEvent e3){}public void windowDeiconified(WindowEvent e4){}public void windowClosed(WindowEvent e5){}public void windowActivated(WindowEvent e6){}public void windowDeactivated(WindowEvent e7){ }}class UpperCaseDocument extends PlainDocument { public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第二个://退房import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A2 extends JFrame {//定义各个组件JFrame frame = new JFrame ("退房系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("退房号");JLabel timeLabel = new JLabel("退房时间");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField timeField= new JTextField();JButton button1,button2;//JTextArea ta = new JTextArea(5,20);/*public static void main(String args[]){A2 a2 = new A2();a2.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(2,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "是否结清消费账单");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"谢谢您的大力支持!","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"请结清消费账单","failure",RMA TION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e){ String room= roomField.getText();String roomword= new String(room);}});timeField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1)); labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1)); fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( timeField);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2)); northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);// frame.pack();frame.setSize(200,230);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第三个://客房预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A3 extends JFrame {//定义各个组件JFrame frame = new JFrame ("客房预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel numberLabel = new JLabel("手机号");JTextField nameField = new JTextField();JTextField numberField= new JTextField();JRadioButton rb1 = new JRadioButton("来电预订");JRadioButton rb2 = new JRadioButton("电话预订");JRadioButton rb3 = new JRadioButton("电传预订");JRadioButton rb4 = new JRadioButton("传真预订");JRadioButton rb5 = new JRadioButton("信函预订");JButton button1,button2;/** public static void main(String args[]){A3 a3 = new A3();a3.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "总统套房", "双人间","单人间" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "预订房类型"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"客人预订成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"客人预订失败","failure",RMA TION_MESSAGE);}}});JPanel p2 = new JPanel();p2.add(rb1);p2.add(rb3);p2.add(rb4);p2.add(rb5);p2.setLayout(new FlowLayout());border = BorderFactory.createTitledBorder(etched, "预订方式"); p2.setBorder(border);//创建ButtonGroup按钮组,并在组中添加按钮ButtonGroup group1 = new ButtonGroup();group1.add(rb1);group1.add(rb2);group1.add(rb3);group1.add(rb4);group1.add(rb5);numberField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String number= numberField.getText();String numberword= new String(number);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(2,1));labelPanel.add(nameLabel);labelPanel.add(numberLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(2,1));fieldPanel.add(nameField);fieldPanel.add(numberField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,330);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第四个//餐饮预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A4 extends JFrame {//定义各个组件JFrame frame = new JFrame ("餐饮预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel timeLabel = new JLabel("预订消费时间");JTextField nameField = new JTextField();JTextField timeField= new JTextField();JCheckBox cb1 = new JCheckBox("中餐");JCheckBox cb2 = new JCheckBox("西餐");JCheckBox cb3 = new JCheckBox("红酒");JCheckBox cb4 = new JCheckBox("饮料");JCheckBox cb5 = new JCheckBox("甜点");JCheckBox cb6 = new JCheckBox("水果");JButton button1,button2;/*public static void main(String args[]){A4 a4 = new A4();a4.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();//ta.append("\nUser Name : "+username);}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "确认订餐"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"订餐成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"订餐失败","failure",RMA TION_MESSAGE);}}});timeField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});JPanel p2 = new JPanel();p2.add(cb1);p2.add(cb2);p2.add(cb3);p2.add(cb4);p2.add(cb5);p2.add(cb6);Border etched1 = BorderFactory.createEtchedBorder();Border border1 = BorderFactory.createTitledBorder(etched1, "预订内容"); p2.setBorder(border1);//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,350);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第五个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A5 extends JFrame {//定义各个组件JFrame frame = new JFrame ("订单管理");JLabel a1 = new JLabel("订单号");JLabel a2 = new JLabel("订货名称");JLabel a3 = new JLabel("收货地址");JLabel a4 = new JLabel("应收金额");JLabel a5 = new JLabel("客户名称");JLabel a6 = new JLabel("结算方式");JLabel a7 = new JLabel("经办人");JTextField b1 = new JTextField();JTextField b2= new JTextField();JTextField b3= new JTextField();JTextField b4= new JTextField();JTextField b5 = new JTextField();JTextField b6 = new JTextField();JTextField b7 = new JTextField();JButton button1,button2;public static void main(String args[]){A5 a1 = new A5();a1.go();}public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();b1.setDocument(ucDocument);b1.setForeground(Color.black);b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = b1.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认收款");button2=new JButton("取消收款");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "收款方式"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认收款"){ JOptionPane.showMessageDialog(p1,"收款成功","success",RMA TION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消收款"){ JOptionPane.showMessageDialog(p1,"收款失败","failure",RMA TION_MESSAGE);}}});b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String idtify= a1.getText();String idtifyword= new String(idtify);}});b2.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String room= a2.getText();String roomword= new String(room);}});b3.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a3.getText();String idtifyword= new String(money);}});b4.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a4.getText();String idtifyword= new String(money);}});b5.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a5.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(7,1));labelPanel.add(a1);labelPanel.add(a2);labelPanel.add(a3);labelPanel.add(a4);labelPanel.add(a5);labelPanel.add(a6);labelPanel.add(a7);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(7,1));fieldPanel.add(b1);fieldPanel.add(b2);fieldPanel.add(b3);fieldPanel.add( b4);fieldPanel.add( b5);fieldPanel.add( b6);fieldPanel.add( b7);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setSize(200,250);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第六个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.text.*;import javax.swing.border.*;public class F{private JFrame frame;private JButton b1,b2,b3,b4,b5,b6,b7,b8,b9,b10,b11,b12,b13;JTextArea ta = new JTextArea(20,20);JTextArea tb = new JTextArea(20,20);public static void main(String args[]){F that=new F();that.go();}void go(){frame=new JFrame("客房检查");Container contentPane=frame.getContentPane();JPanel P1=new JPanel();P1.setLayout(new GridLayout());b1=new JButton("101 \n单人间");b2=new JButton("102 \n单人间");b3=new JButton("103 \n单人间");b4=new JButton("104 \n单人间");b5=new JButton("105 \n单人间");b6=new JButton("201 \n双人间");b7=new JButton("202 \n双人间");b8=new JButton("203 \n双人间");b9=new JButton("204 \n双人间");b10=new JButton("205 \n双人间");b11=new JButton("301 \n总统套房");b12=new JButton("302 \n总统套房");b13=new JButton("303 \n总统套房");b1.setBackground(Color.cyan);b2.setBackground(Color.cyan);b3.setBackground(Color.cyan);b4.setBackground(Color.cyan);b5.setBackground(Color.cyan);b6.setBackground(Color.magenta);b7.setBackground(Color.magenta);b8.setBackground(Color.magenta);b9.setBackground(Color.magenta);b10.setBackground(Color.magenta);b11.setBackground(Color.pink);b12.setBackground(Color.pink);b13.setBackground(Color.pink);P1.add(b1);P1.add(b2);P1.add(b3);P1.add(b4);P1.add(b5);P1.add(b6);P1.add(b7);P1.add(b8);P1.add(b9);P1.add(b10);P1.add(b11);P1.add(b12);P1.add(b13);tb.append("房间总数:13");tb.append("\n当前占用:");tb.append("\n当前可供:");P1.add(tb);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="101 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n101房间是否打扫:");ta.append("\n101房间里是否有家具损坏:");ta.append("\n101房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="102 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n102房间是否打扫:");ta.append("\n102房间里是否有家具损坏:");ta.append("\n102房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="103 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n103房间是否打扫:");ta.append("\n103房间里是否有家具损坏:");ta.append("\n103房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="104 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n104房间是否打扫:");ta.append("\n104房间里是否有家具损坏:");ta.append("\n104房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="105 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n105房间是否打扫:");ta.append("\n105房间里是否有家具损坏:");ta.append("\n105房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="201 \n双人间"){ta.setText("");JFrame frame1=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n201房间是否打扫:");ta.append("\n201房间里是否有家具损坏:");ta.append("\n201房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame1.setContentPane(p2);frame1.pack();frame1.setVisible(true);}else if(e.getActionCommand()=="202 \n双人间"){ta.setText("");JFrame frame2=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n202房间是否打扫:");ta.append("\n202房间里是否有家具损坏:");ta.append("\n202房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame2.setContentPane(p2);frame2.pack();frame2.setVisible(true);}else if(e.getActionCommand()=="203 \n双人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n203房间是否打扫:");ta.append("\n203房间里是否有家具损坏:");ta.append("\n203房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMA TION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="204 \n双人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n204房间是否打扫:");ta.append("\n204房间里是否有家具损坏:");。
简易酒店管理系统代码
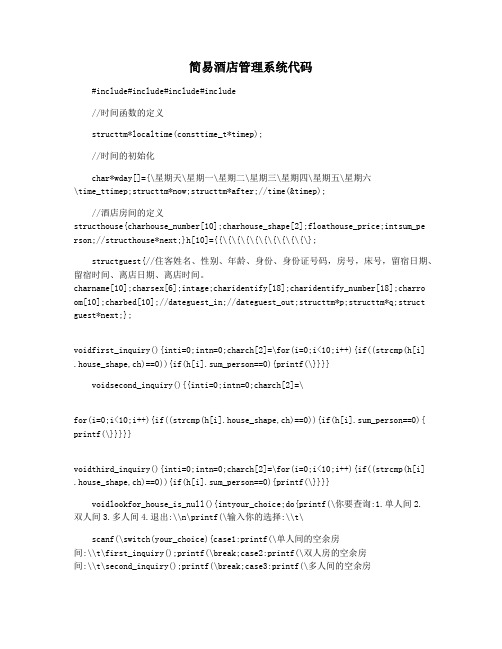
简易酒店管理系统代码#include#include#include#include//时间函数的定义structtm*localtime(consttime_t*timep);//时间的初始化char*wday[]={\星期天\星期一\星期二\星期三\星期四\星期五\星期六\time_ttimep;structtm*now;structtm*after;//time(&timep);//酒店房间的定义structhouse{charhouse_number[10];charhouse_shape[2];floathouse_price;intsum_pe rson;//structhouse*next;}h[10]={{\{\{\{\{\{\{\{\{\{\};structguest{//住客姓名、性别、年龄、身份、身份证号码,房号,床号,留宿日期、留宿时间、离店日期、离店时间。
charname[10];charsex[6];intage;charidentify[18];charidentify_number[18];charro om[10];charbed[10];//dateguest_in;//dateguest_out;structtm*p;structtm*q;struct guest*next;};voidfirst_inquiry(){inti=0;intn=0;charch[2]=\for(i=0;i<10;i++){if((strcmp(h[i].house_shape,ch)==0)){if(h[i].sum_person==0){printf(\}}}}voidsecond_inquiry(){{inti=0;intn=0;charch[2]=\for(i=0;i<10;i++){if((strcmp(h[i].house_shape,ch)==0)){if(h[i].sum_person==0){ printf(\}}}}}voidthird_inquiry(){inti=0;intn=0;charch[2]=\for(i=0;i<10;i++){if((strcmp(h[i].house_shape,ch)==0)){if(h[i].sum_person==0){printf(\}}}}voidlookfor_house_is_null(){intyour_choice;do{printf(\你要查询:1.单人间2.双人间3.多人间4.退出:\\n\printf(\输入你的选择:\\t\scanf(\switch(your_choice){case1:printf(\单人间的空余房间:\\t\first_inquiry();printf(\break;case2:printf(\双人房的空余房间:\\t\second_inquiry();printf(\break;case3:printf(\多人间的空余房间:\\t\third_inquiry();printf(\break;case4://exit(1);break;}}while(your_choice !=4);printf(\printf(\}voidadd_house_sum_number(charstr[10]){inti=0;for(i=0;i<10;i++){if((strcmp(h[i] .house_number,str)==0)){h[i].sum_person=1;}else{continue;}}}typedefstructguest*link_guest;//建立link_guestcreate_guest(void){link_guesthead=(link_guest)malloc(sizeof(structgu est));if(head!=null){head->next=null;}else{printf(\}returnhead;}boolis_null_guest(link_guesthead){if(head->next==null){returntrue;}returnfalse;}//写个函数判断一下当输入住客的房号时,是否还有个这个房间boolis_null_this_house(charstr[10]){inti=0;。
酒店管理系统实现代码
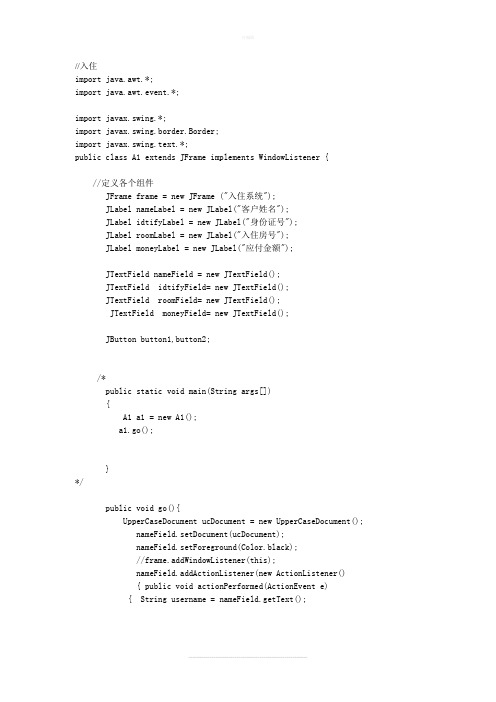
//入住import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A1 extends JFrame implements WindowListener {//定义各个组件JFrame frame = new JFrame ("入住系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("入住房号");JLabel moneyLabel = new JLabel("应付金额");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField moneyField= new JTextField();JButton button1,button2;/*public static void main(String args[]){A1 a1 = new A1();a1.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);//frame.addWindowListener(this);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认支付");button2=new JButton("取消支付");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "付款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认支付"){ JOptionPane.showMessageDialog(p1,"支付成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消支付"){ JOptionPane.showMessageDialog(p1,"支付失败","failure",RMATION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String room= roomField.getText();String roomword= new String(room);}});moneyField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String money= moneyField.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(moneyLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( moneyField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setSize(200,250);frame.setVisible(true);}public void windowClosing(WindowEvent e1){System.exit(0);}public void windowOpened(WindowEvent e2){}public void windowIconified(WindowEvent e3){}public void windowDeiconified(WindowEvent e4){}public void windowClosed(WindowEvent e5){}public void windowActivated(WindowEvent e6){}public void windowDeactivated(WindowEvent e7){}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet); }}第二个://退房import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A2 extends JFrame {//定义各个组件JFrame frame = new JFrame ("退房系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("退房号");JLabel timeLabel = new JLabel("退房时间");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField timeField= new JTextField();JButton button1,button2;//JTextArea ta = new JTextArea(5,20);/*public static void main(String args[]){A2 a2 = new A2();a2.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(2,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "是否结清消费账单");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"谢谢您的大力支持!","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"请结清消费账单","failure",RMATION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String room= roomField.getText();String roomword= new String(room);}});timeField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String time= timeField.getText();String timeword= new String(time);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( timeField);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);// frame.pack();frame.setSize(200,230);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第三个://客房预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A3 extends JFrame {//定义各个组件JFrame frame = new JFrame ("客房预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel numberLabel = new JLabel("手机号");JTextField nameField = new JTextField();JTextField numberField= new JTextField();JRadioButton rb1 = new JRadioButton("来电预订");JRadioButton rb2 = new JRadioButton("电话预订");JRadioButton rb3 = new JRadioButton("电传预订");JRadioButton rb4 = new JRadioButton("传真预订");JRadioButton rb5 = new JRadioButton("信函预订");JButton button1,button2;/** public static void main(String args[]){A3 a3 = new A3();a3.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "总统套房", "双人间","单人间" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "预订房类型");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"客人预订成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"客人预订失败","failure",RMATION_MESSAGE);}}});JPanel p2 = new JPanel();p2.add(rb1);p2.add(rb2);p2.add(rb3);p2.add(rb4);p2.add(rb5);p2.setLayout(new FlowLayout());border = BorderFactory.createTitledBorder(etched, "预订方式"); p2.setBorder(border);//创建ButtonGroup按钮组,并在组中添加按钮ButtonGroup group1 = new ButtonGroup();group1.add(rb1);group1.add(rb2);group1.add(rb3);group1.add(rb4);group1.add(rb5);numberField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String number= numberField.getText();String numberword= new String(number);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(2,1));labelPanel.add(nameLabel);labelPanel.add(numberLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(2,1));fieldPanel.add(nameField);fieldPanel.add(numberField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,330);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第四个//餐饮预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A4 extends JFrame {//定义各个组件JFrame frame = new JFrame ("餐饮预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel timeLabel = new JLabel("预订消费时间");JTextField nameField = new JTextField();JTextField timeField= new JTextField();JCheckBox cb1 = new JCheckBox("中餐");JCheckBox cb2 = new JCheckBox("西餐");JCheckBox cb3 = new JCheckBox("红酒");JCheckBox cb4 = new JCheckBox("饮料");JCheckBox cb5 = new JCheckBox("甜点");JCheckBox cb6 = new JCheckBox("水果");JButton button1,button2;/*public static void main(String args[]){A4 a4 = new A4();a4.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();//ta.append("\nUser Name : "+username);}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "确认订餐");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"订餐成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"订餐失败","failure",RMATION_MESSAGE);}}});timeField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});JPanel p2 = new JPanel();p2.add(cb1);p2.add(cb2);p2.add(cb3);p2.add(cb4);p2.add(cb5);p2.add(cb6);Border etched1 = BorderFactory.createEtchedBorder();Border border1 = BorderFactory.createTitledBorder(etched1, "预订内容");p2.setBorder(border1);//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,350);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第五个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A5 extends JFrame {//定义各个组件JFrame frame = new JFrame ("订单管理");JLabel a1 = new JLabel("订单号");JLabel a2 = new JLabel("订货名称");JLabel a3 = new JLabel("收货地址");JLabel a4 = new JLabel("应收金额");JLabel a5 = new JLabel("客户名称");JLabel a6 = new JLabel("结算方式");JLabel a7 = new JLabel("经办人");JTextField b1 = new JTextField();JTextField b2= new JTextField();JTextField b3= new JTextField();JTextField b4= new JTextField();JTextField b5 = new JTextField();JTextField b6 = new JTextField();JTextField b7 = new JTextField();JButton button1,button2;public static void main(String args[]){A5 a1 = new A5();a1.go();}public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();b1.setDocument(ucDocument);b1.setForeground(Color.black);b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = b1.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认收款");button2=new JButton("取消收款");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "收款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认收款"){ JOptionPane.showMessageDialog(p1,"收款成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消收款"){ JOptionPane.showMessageDialog(p1,"收款失败","failure",RMATION_MESSAGE);}}});b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= a1.getText();String idtifyword= new String(idtify);}});b2.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String room= a2.getText();String roomword= new String(room);}});b3.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= a3.getText();String idtifyword= new String(money);}});b4.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= a4.getText();String idtifyword= new String(money);}});b5.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= a5.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(7,1));labelPanel.add(a1);labelPanel.add(a2);labelPanel.add(a3);labelPanel.add(a4);labelPanel.add(a5);labelPanel.add(a6);labelPanel.add(a7);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(7,1));fieldPanel.add(b1);fieldPanel.add(b2);fieldPanel.add(b3);fieldPanel.add( b4);fieldPanel.add( b5);fieldPanel.add( b6);fieldPanel.add( b7);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setSize(200,250);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第六个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.text.*;import javax.swing.border.*;public class F{private JFrame frame;private JButton b1,b2,b3,b4,b5,b6,b7,b8,b9,b10,b11,b12,b13;JTextArea ta = new JTextArea(20,20);JTextArea tb = new JTextArea(20,20);public static void main(String args[]){F that=new F();that.go();}void go(){frame=new JFrame("客房检查");Container contentPane=frame.getContentPane();JPanel P1=new JPanel();P1.setLayout(new GridLayout());b1=new JButton("101 \n单人间");b2=new JButton("102 \n单人间");b3=new JButton("103 \n单人间");b4=new JButton("104 \n单人间 ");b5=new JButton("105 \n单人间");b6=new JButton("201 \n双人间");b7=new JButton("202 \n双人间");b8=new JButton("203 \n双人间");b9=new JButton("204 \n双人间");b10=new JButton("205 \n双人间");b11=new JButton("301 \n总统套房");b12=new JButton("302 \n总统套房");b13=new JButton("303 \n总统套房");b1.setBackground(Color.cyan);b2.setBackground(Color.cyan);b3.setBackground(Color.cyan);b4.setBackground(Color.cyan);b5.setBackground(Color.cyan);b6.setBackground(Color.magenta);b7.setBackground(Color.magenta);b8.setBackground(Color.magenta);b9.setBackground(Color.magenta);b10.setBackground(Color.magenta);b11.setBackground(Color.pink);b12.setBackground(Color.pink);b13.setBackground(Color.pink);P1.add(b1);P1.add(b2);P1.add(b3);P1.add(b4);P1.add(b5);P1.add(b6);P1.add(b7);P1.add(b8);P1.add(b9);P1.add(b10);P1.add(b11);P1.add(b12);P1.add(b13);tb.append("房间总数:13");tb.append("\n当前占用:");tb.append("\n当前可供:");P1.add(tb);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="101 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n101房间是否打扫: ");ta.append("\n101房间里是否有家具损坏: ");ta.append("\n101房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="102 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n102房间是否打扫: ");ta.append("\n102房间里是否有家具损坏: ");ta.append("\n102房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="103 \n单人间"){ ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n103房间是否打扫: ");ta.append("\n103房间里是否有家具损坏: ");ta.append("\n103房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="104 \n单人间"){ ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n104房间是否打扫: ");ta.append("\n104房间里是否有家具损坏: ");ta.append("\n104房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="105 \n单人间"){ ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n105房间是否打扫: ");ta.append("\n105房间里是否有家具损坏: ");ta.append("\n105房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="201 \n双人间"){ ta.setText("");JFrame frame1=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n201房间是否打扫: ");ta.append("\n201房间里是否有家具损坏: ");ta.append("\n201房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame1.setContentPane(p2);frame1.pack();frame1.setVisible(true);}else if(e.getActionCommand()=="202 \n双人间"){ ta.setText("");JFrame frame2=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n202房间是否打扫: ");ta.append("\n202房间里是否有家具损坏: ");ta.append("\n202房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame2.setContentPane(p2);frame2.pack();frame2.setVisible(true);}else if(e.getActionCommand()=="203 \n双人间"){ ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n203房间是否打扫: ");ta.append("\n203房间里是否有家具损坏: ");ta.append("\n203房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="204 \n双人间"){ ta.setText("");。
酒店管理系统实现代码

//入住import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A1 extends JFrame implements WindowListener { //定义各个组件JFrame frame = new JFrame ("入住系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("入住房号");JLabel moneyLabel = new JLabel("应付金额");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField moneyField= new JTextField();JButton button1,button2;/*public static void main(String args[]){A1 a1 = new A1();a1.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);//frame.addWindowListener(this);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认支付");button2=new JButton("取消支付");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "付款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认支付"){ JOptionPane.showMessageDialog(p1,"支付成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消支付"){ JOptionPane.showMessageDialog(p1,"支付失败","failure",RMATION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String room= roomField.getText();String roomword= new String(room);}});moneyField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String money= moneyField.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(moneyLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( moneyField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在north cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,250);frame.setVisible(true);}public void windowClosing(WindowEvent e1){ System.exit(0);}public void windowOpened(WindowEvent e2){}public void windowIconified(WindowEvent e3){}public void windowDeiconified(WindowEvent e4){ }public void windowClosed(WindowEvent e5){}public void windowActivated(WindowEvent e6){}public void windowDeactivated(WindowEvent e7){ }}class UpperCaseDocument extends PlainDocument { public void insertString(int offset,String string, AttributeSet attributeSet) throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet); }}第二个://退房import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A2 extends JFrame {//定义各个组件JFrame frame = new JFrame ("退房系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("退房号");JLabel timeLabel = new JLabel("退房时间");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField timeField= new JTextField();JButton button1,button2;//JTextArea ta = new JTextArea(5,20);/*public static void main(String args[]){A2 a2 = new A2();a2.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String username = nameField.getText();}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(2,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "是否结清消费账单");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"谢谢您的大力支持!","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"请结清消费账单","failure",RMATION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String room= roomField.getText();String roomword= new String(room);}});timeField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String time= timeField.getText();String timeword= new String(time);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在north cp.add(p1,BorderLayout.SOUTH);// frame.pack();frame.setSize(200,230);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet); }}第三个://客房预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A3 extends JFrame {//定义各个组件JFrame frame = new JFrame ("客房预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel numberLabel = new JLabel("手机号");JTextField nameField = new JTextField();JTextField numberField= new JTextField();JRadioButton rb1 = new JRadioButton("来电预订");JRadioButton rb2 = new JRadioButton("电话预订");JRadioButton rb3 = new JRadioButton("电传预订");JRadioButton rb4 = new JRadioButton("传真预订");JRadioButton rb5 = new JRadioButton("信函预订");JButton button1,button2;/** public static void main(String args[]){A3 a3 = new A3();a3.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "总统套房", "双人间","单人间" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "预订房类型");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"客人预订成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"客人预订失败","failure",RMATION_MESSAGE);}}});JPanel p2 = new JPanel();p2.add(rb1);p2.add(rb2);p2.add(rb3);p2.add(rb4);p2.add(rb5);p2.setLayout(new FlowLayout());border = BorderFactory.createTitledBorder(etched, "预订方式");p2.setBorder(border);//创建ButtonGroup按钮组,并在组中添加按钮ButtonGroup group1 = new ButtonGroup();group1.add(rb1);group1.add(rb2);group1.add(rb3);group1.add(rb4);group1.add(rb5);numberField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String number= numberField.getText();String numberword= new String(number);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(2,1));labelPanel.add(nameLabel);labelPanel.add(numberLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(2,1));fieldPanel.add(nameField);fieldPanel.add(numberField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在north cp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,330);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第四个//餐饮预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A4 extends JFrame {//定义各个组件JFrame frame = new JFrame ("餐饮预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel timeLabel = new JLabel("预订消费时间");JTextField nameField = new JTextField();JTextField timeField= new JTextField();JCheckBox cb1 = new JCheckBox("中餐");JCheckBox cb2 = new JCheckBox("西餐");JCheckBox cb3 = new JCheckBox("红酒");JCheckBox cb4 = new JCheckBox("饮料");JCheckBox cb5 = new JCheckBox("甜点");JCheckBox cb6 = new JCheckBox("水果");JButton button1,button2;/*public static void main(String args[]){A4 a4 = new A4();a4.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String username = nameField.getText();//ta.append("\nUser Name : "+username);}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "确认订餐");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"订餐成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"订餐失败","failure",RMATION_MESSAGE);}}});timeField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});JPanel p2 = new JPanel();p2.add(cb1);p2.add(cb2);p2.add(cb3);p2.add(cb4);p2.add(cb5);p2.add(cb6);Border etched1 = BorderFactory.createEtchedBorder();Border border1 = BorderFactory.createTitledBorder(etched1, "预订内容");p2.setBorder(border1);//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在north cp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,350);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet); }}第五个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A5 extends JFrame {//定义各个组件JFrame frame = new JFrame ("订单管理");JLabel a1 = new JLabel("订单号");JLabel a2 = new JLabel("订货名称");JLabel a3 = new JLabel("收货地址");JLabel a4 = new JLabel("应收金额");JLabel a5 = new JLabel("客户名称"); JLabel a6 = new JLabel("结算方式"); JLabel a7 = new JLabel("经办人");JTextField b1 = new JTextField();JTextField b2= new JTextField();JTextField b3= new JTextField();JTextField b4= new JTextField();JTextField b5 = new JTextField();JTextField b6 = new JTextField();JTextField b7 = new JTextField();JButton button1,button2;public static void main(String args[]){A5 a1 = new A5();a1.go();}public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();b1.setDocument(ucDocument);b1.setForeground(Color.black);b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = b1.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认收款");button2=new JButton("取消收款");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "收款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认收款"){ JOptionPane.showMessageDialog(p1,"收款成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消收款"){ JOptionPane.showMessageDialog(p1,"收款失败","failure",RMATION_MESSAGE);}}});b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String idtify= a1.getText();String idtifyword= new String(idtify);}});b2.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String room= a2.getText();String roomword= new String(room);}});b3.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a3.getText();String idtifyword= new String(money);}});b4.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a4.getText();String idtifyword= new String(money);}});b5.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a5.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(7,1));labelPanel.add(a1);labelPanel.add(a2);labelPanel.add(a3);labelPanel.add(a4);labelPanel.add(a5);labelPanel.add(a6);labelPanel.add(a7);//面板fieldPanel放文本框 JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(7,1));fieldPanel.add(b1);fieldPanel.add(b2);fieldPanel.add(b3);fieldPanel.add( b4);fieldPanel.add( b5);fieldPanel.add( b6);fieldPanel.add( b7);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在north cp.add(p1,BorderLayout.SOUTH);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setSize(200,250);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第六个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.text.*;import javax.swing.border.*;public class F{private JFrame frame;private JButton b1,b2,b3,b4,b5,b6,b7,b8,b9,b10,b11,b12,b13; JTextArea ta = new JTextArea(20,20);JTextArea tb = new JTextArea(20,20);public static void main(String args[]){F that=new F();that.go();}void go(){frame=new JFrame("客房检查");Container contentPane=frame.getContentPane();JPanel P1=new JPanel();P1.setLayout(new GridLayout());b1=new JButton("101 \n单人间");b2=new JButton("102 \n单人间");b3=new JButton("103 \n单人间");b4=new JButton("104 \n单人间 ");b5=new JButton("105 \n单人间");b6=new JButton("201 \n双人间");b7=new JButton("202 \n双人间");b8=new JButton("203 \n双人间");b9=new JButton("204 \n双人间");b10=new JButton("205 \n双人间"); b11=new JButton("301 \n总统套房"); b12=new JButton("302 \n总统套房"); b13=new JButton("303 \n总统套房"); b1.setBackground(Color.cyan);b2.setBackground(Color.cyan);b3.setBackground(Color.cyan);b4.setBackground(Color.cyan);b5.setBackground(Color.cyan);b6.setBackground(Color.magenta);b7.setBackground(Color.magenta);b8.setBackground(Color.magenta);b9.setBackground(Color.magenta);b10.setBackground(Color.magenta); b11.setBackground(Color.pink);b12.setBackground(Color.pink);b13.setBackground(Color.pink);P1.add(b1);P1.add(b2);P1.add(b3);P1.add(b4);P1.add(b5);P1.add(b6);P1.add(b7);P1.add(b8);P1.add(b9);P1.add(b10);P1.add(b11);P1.add(b12);P1.add(b13);tb.append("房间总数:13");tb.append("\n当前占用:");tb.append("\n当前可供:");P1.add(tb);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="101 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n101房间是否打扫: ");ta.append("\n101房间里是否有家具损坏: ");ta.append("\n101房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="102 \n单人间"){ ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n102房间是否打扫: ");ta.append("\n102房间里是否有家具损坏: ");ta.append("\n102房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="103 \n单人间"){ ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n103房间是否打扫: ");ta.append("\n103房间里是否有家具损坏: ");ta.append("\n103房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="104 \n单人间"){ ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n104房间是否打扫: ");ta.append("\n104房间里是否有家具损坏: ");ta.append("\n104房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.setVisible(true);}else if(e.getActionCommand()=="105 \n单人间"){ ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n105房间是否打扫: ");ta.append("\n105房间里是否有家具损坏: ");ta.append("\n105房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="201 \n双人间"){ ta.setText("");JFrame frame1=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n201房间是否打扫: ");ta.append("\n201房间里是否有家具损坏: ");ta.append("\n201房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame1.setContentPane(p2);frame1.pack();frame1.setVisible(true);}else if(e.getActionCommand()=="202 \n双人间"){ ta.setText("");JFrame frame2=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n202房间是否打扫: ");ta.append("\n202房间里是否有家具损坏: ");ta.append("\n202房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame2.setContentPane(p2);frame2.pack();frame2.setVisible(true);}else if(e.getActionCommand()=="203 \n双人间"){ ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n203房间是否打扫: ");ta.append("\n203房间里是否有家具损坏: ");ta.append("\n203房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){ if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="204 \n双人间"){ ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n204房间是否打扫: ");ta.append("\n204房间里是否有家具损坏: ");ta.append("\n204房间是否交足余额: ");。
酒店管理系统实现代码---精品管理资料
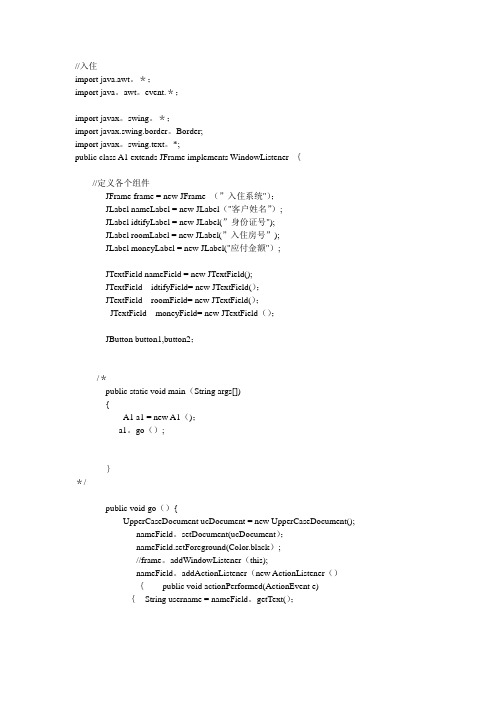
//入住import java.awt。
*;import java。
awt。
event.*;import javax。
swing。
*;import javax.swing.border。
Border;import javax。
swing.text。
*;public class A1 extends JFrame implements WindowListener {//定义各个组件JFrame frame = new JFrame (”入住系统");JLabel nameLabel = new JLabel("客户姓名”);JLabel idtifyLabel = new JLabel(”身份证号");JLabel roomLabel = new JLabel(”入住房号”);JLabel moneyLabel = new JLabel("应付金额");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField moneyField= new JTextField();JButton button1,button2;/*public static void main(String args[]){A1 a1 = new A1();a1。
go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField。
setDocument(ucDocument);nameField.setForeground(Color.black);//frame。
酒店管理系统实现代码【范本模板】
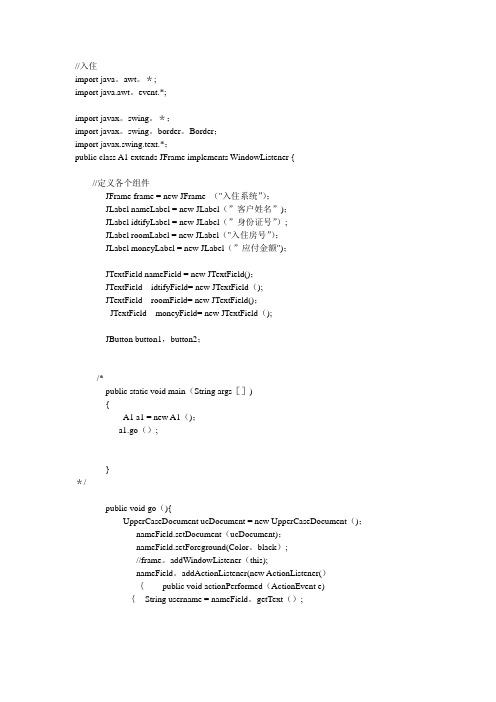
//入住import java。
awt。
*;import java.awt。
event.*;import javax。
swing。
*;import javax。
swing。
border。
Border;import javax.swing.text.*;public class A1 extends JFrame implements WindowListener {//定义各个组件JFrame frame = new JFrame ("入住系统”);JLabel nameLabel = new JLabel(”客户姓名”);JLabel idtifyLabel = new JLabel(”身份证号”);JLabel roomLabel = new JLabel("入住房号”);JLabel moneyLabel = new JLabel(”应付金额");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField moneyField= new JTextField();JButton button1,button2;/*public static void main(String args[]){A1 a1 = new A1();a1.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color。
black);//frame。
addWindowListener(this);nameField。
酒店管理系统代码
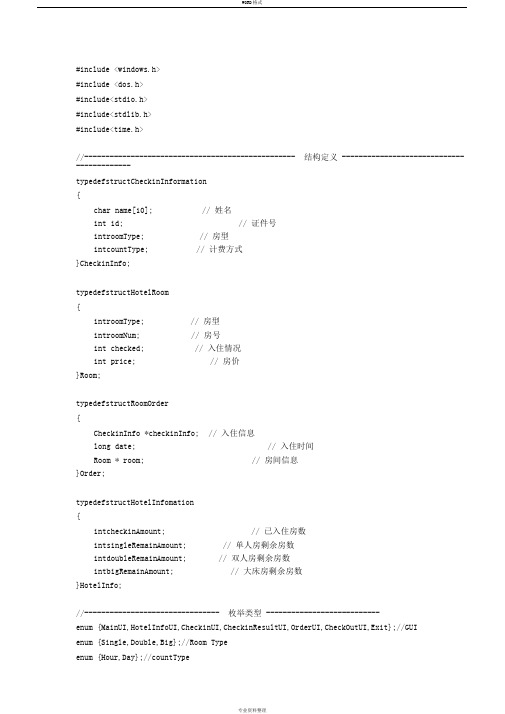
#include <windows.h>#include <dos.h>#include<stdio.h>#include<stdlib.h>#include<time.h>//-------------------------------------------------- 结构定义 ------------------------------------------typedefstructCheckinInformation{char name[10]; // 姓名int id; // 证件号introomType; // 房型intcountType; // 计费方式}CheckinInfo;typedefstructHotelRoom{introomType; // 房型introomNum; // 房号int checked; // 入住情况int price; // 房价}Room;typedefstructRoomOrder{CheckinInfo *checkinInfo; // 入住信息long date; // 入住时间Room * room; // 房间信息}Order;typedefstructHotelInfomation{intcheckinAmount; // 已入住房数intsingleRemainAmount; // 单人房剩余房数intdoubleRemainAmount; // 双人房剩余房数intbigRemainAmount; // 大床房剩余房数}HotelInfo;//-------------------------------- 枚举类型 ---------------------------enum {MainUI,HotelInfoUI,CheckinUI,CheckinResultUI,OrderUI,CheckOutUI,Exit};//GUIenum {Single,Double,Big};//Room Typeenum {Hour,Day};//countType//-------------------------------- 全局变量 --------------------------int GUI = MainUI;Order*orderList[100]; Room*roomList[100]; // 订单数组// 房间数组HotelInfo * hotelInfo = NULL;// 酒店房间信息//------------------------------- 函数声明 ---------------------------- voidinitiallizeRoomList();voidinsertToOrderList(Order * order);Room* getRoomByType(introomType);Order* getOrderByRoomNum(introomNum);voidshowMainUI();voidshowHotelInfoUI();voidshowCheckinUI();voidshowCheckinResultUI();voidshowOrderUI();voidshowCheckOutUI();//-------------------------------Main函数 ----------------------------void main() // 主函数{//初始化酒店房间信息hotelInfo = (HotelInfo *)malloc(sizeof(HotelInfo));hotelInfo ->singleRemainAmount = 20;hotelInfo ->doubleRemainAmount=40;hotelInfo ->bigRemainAmount=40;hotelInfo ->checkinAmount=0;//初始化房间列表initiallizeRoomList();//界面显示while(GUI != Exit){switch(GUI){caseMainUI:showMainUI();break; caseHotelInfoUI:showHotelInfoUI();break;caseCheckinUI:showCheckinUI();break;caseCheckinResultUI:showCheckinResultUI();break;caseOrderUI:showOrderUI();break;caseCheckOutUI:showCheckOutUI();break;default:break;}}}//------------------------------- 函数定义----------------------------voidinitiallizeRoomList(){//房间数组初始化,初始化的结果是让 roomList 的数组有 100 个 room 指针,而且设置了相应的值inti;Room*newRoom=NULL;for(i=0;i<20;i++){// 单人房房间信息初始化newRoom = ( Room* )malloc(sizeof(Room));roomList[i] = newRoom;roomList[i]->checked=0;roomList[i]->price=110;roomList[i]->roomNum=i+1;roomList[i]->roomType=Single;}for(i=20;i<60;i++){// 双人房房间信息初始化newRoom = ( Room* )malloc(sizeof(Room));roomList[i] = newRoom; roomList[i]->checked=0; roomList[i]->price=180; roomList[i]->roomNum=i+1;roomList[i]->roomType=Double;}// 大床房房间信息初始化for(i=60;i<100;i++){newRoom = ( Room* )malloc(sizeof(Room));roomList[i] = newRoom;roomList[i]->checked=0;roomList[i]->price=180;roomList[i]->roomNum=i+1;roomList[i]->roomType=Big;}}// 通过所选择的房型获取空房间,获取房间后将房间信息改为已入住,并减少相应房型的剩余房间数Room* getRoomByType(introomType){inti;switch(roomType){case Single:for(i=0;i<20;i++){if(roomList[i]->checked == 0){roomList[i]->checked=1;hotelInfo->singleRemainAmount -- ;hotelInfo->checkinAmount++;returnroomList[i];}}break;case Double:for(i=20;i<60;i++){if(roomList[i]->checked == 0){roomList[i]->checked=1;hotelInfo->doubleRemainAmount -- ;hotelInfo->checkinAmount++;returnroomList[i];}}break;case Big:for(i=60;i<100;i++){if(roomList[i]->checked == 0){roomList[i]->checked=1;hotelInfo->bigRemainAmount --;hotelInfo->checkinAmount++;returnroomList[i];}}break;}}//将订单放入订单列表voidinsertToOrderList(Order * order){inti;for(i = 0;i<100;i++){if(orderList[i] ==NULL ){orderList[i] = order;break;}}}//通过房号查询订单Order* getOrderByRoomNum(introomNum){inti;for(i=0;i<100;i++){if(orderList[i]->room->roomNum == roomNum){returnorderList[i]; }}}voidshowMainUI(){//显示主界面,并接受输入intchooseNum;system("cls");printf("\n\n========================== 酒店房间登记与计费管理管理系统=======================\t\n\n\n");printf("*\t\t\ t\t1.入住登记\t\t\t\t*\n");printf("*\t\t\t\t2. 查询入住情况\t\t\t*\n");printf("*\t\t\t\t3. 查询当前费用\t\t\t*\n");printf("*\t\t\t\t4. 结账退房\t\t\t\t*\n");printf("*\t\t\t\t5. 退出程序\t\t\t\t*\n\n\n");printf("\n\n========================== 酒店房间登记与计费管理管理系统 =======================\t\n\n\n");printf(" 请输入相应编号进入菜单 \t");//接受输入scanf("%d",&chooseNum);switch(chooseNum){case 1:GUI = HotelInfoUI;break;case 2:GUI = HotelInfoUI;break;case 3:GUI = OrderUI;break;case 4:GUI = OrderUI;break;case 5:专业资料整理Sleep(3000);GUI = Exit;break;default:break;专业资料整理}}voidshowHotelInfoUI(){intchooseNum;system("cls");printf("\n\n========================= 酒店入住情况查询菜单=======================\t\n\n\n\n");printf("*\t\t\t 入住房间数 : %d\t\t\t\t*\n", hotelInfo->checkinAmount);printf("*\t\t\t 剩余房间数 : \t");printf(" 单人房: %d\t\t*\n",hotelInfo->singleRemainAmount);printf("*\t\t\t\t\t 双人房: %d\t\t*\n",hotelInfo->doubleRemainAmount);printf("*\t\t\t\t\t 大床房: %d\t\t*\n\n",hotelInfo->bigRemainAmount);printf("\n\n========================= 酒店入住情况查询菜单=======================\t\n\n\n");printf(" 按 0 :返回\n");printf(" 按 1 : 登记入住 \n");scanf("%d",&chooseNum);switch(chooseNum){case 0:GUI = MainUI;break;case 1:GUI = CheckinUI;break;default:GUI = HotelInfoUI;break;}}voidshowCheckinUI(){Order * newOrder;Room* newRoom = NULL;//填写一个新的入住信息CheckinInfo * newCheckinInfo = NULL;专业资料整理introomTypeNum;intcountTypeNum;专业资料整理time_ttimep;system("cls");printf("\n\n=========================== 酒店入住登记菜单=========================\t\n\n\n");newCheckinInfo = ( CheckinInfo * )malloc(sizeof(CheckinInfo));printf("*\t\t 请输入姓名 :");scanf("%s", &(newCheckinInfo->name) );printf("*\t\t 请输入证件号 :");scanf("%d", &(newCheckinInfo->id) );printf("*\t\t 请选择入住房型 :\n");printf("\t\t\t1. 单人房 \n\t\t\t2. 双人房\n\t\t\t3. 大床房 \n"); scanf("%d",&(roomTypeNum));switch(roomTypeNum) // 通过输入的数字对应房型{case 1:newCheckinInfo->roomType = Single;break;case 2:newCheckinInfo->roomType = Double;break;case 3:newCheckinInfo->roomType = Big;break;default:newCheckinInfo->roomType = Single;break;}printf("*\ t\tprintf("\t \t\t1. 请选择计费方式:\n");按小时计费 ;\n\t\t\t2.按天数计费\n"); // 通过输入的数字对应计费方式scanf("%d",&countTypeNum);switch(countTypeNum){case 1:专业资料整理newCheckinInfo->countType = Hour;break;case 2:专业资料整理newCheckinInfo->countType = Day;break;}printf("\n\n=========================== 酒店入住登记菜单=========================\t\n\n\n");//生成一个新的订单newOrder = ( Order* )malloc(sizeof(Order));newOrder ->checkinInfo = newCheckinInfo;newOrder -> date = time(0);switch(newCheckinInfo->roomType){case Single:newRoom = getRoomByType(Single);break;case Double:newRoom=getRoomByType(Double);break;case Big :newRoom=getRoomByType(Big);break; // 通过房型获取房间}newOrder->room = newRoom; insertToOrderList(newOrder);print f(" 房间号为:%d\n",newOrder->room->roomNum);GUI = CheckinResultUI;}voidshowCheckinResultUI(){intchooseNum;printf("\n\n========================= 酒店入住登记确认菜单=======================\t\n\n\n");printf("\t\t\t************\t\t\t\t\n");printf("\t\t\t* 登记成功*\t\t\t\t\n");printf("\t\t\t************\t\t\t\t\n\n");printf("\n\n========================= 酒店入住登记确认菜单=======================\t\n\n\n");专业资料整理printf(" 按 0 :返回\n");scanf("%d",&chooseNum);专业资料整理switch(chooseNum){case 0:GUI = MainUI;break;default:GUI = CheckinResultUI;break;}}voidshowOrderUI(){introomNum;intchooseNum;int amount;Order * theOrder = NULL;system("cls");printf("\n\n========================= 酒店房间信息查询菜单=======================\t\n\n\n");printf(" 请输入房间号 :");scanf("%d",&roomNum);if (roomNum<0 || roomNum>100){printf("\n 输入有误请重新输入") ;GUI = OrderUI;}else{theOrder = getOrderByRoomNum(roomNum);printf(" 房型: ");switch(theOrder->room->roomType){case Single:printf(" 单人房 \n");break;case Double:printf(" 双人房 \n");break;case Big:printf(" 大床房 \n");break;}printf(" 计费方式: ");专业资料整理switch(theOrder->checkinInfo->countType ) {专业资料整理case Hour:printf(" 小时计费 \n");amount = (time(0) - theOrder->date) / 3600 +1;printf(" 已入住时间: %d 小时 \n",amount);break;case Day:printf(" 天计费 \n");amount = (time(0) - theOrder->date) / (3600*24) +1;printf(" 已入住时间: %d 天\n",amount);break;}printf(" 房价: %d\n",theOrder->room->price);printf(" 应支付: %d\n\n",amount * theOrder->room->price);printf("\n\n========================= 酒店房间信息查询菜单=======================\t\n\n\n");printf(" 按 0:返回 \n");printf(" 按 1:结账退房 \n");scanf("%d",&chooseNum);switch(chooseNum){case 0:GUI = MainUI;break;case 1:GUI = CheckOutUI;break;default:break;}}}voidshowCheckOutUI(){intchooseNum;printf("\n\n========================= 酒店结账退房确认菜单=======================\t\n\n\n");printf("\t\t\t\t************\t\t\t\t\n");printf("\t\t\t\t* 结账成功*\t\t\t\t\n");printf("\t\t\t\t************\t\t\t\t\n\n");printf("\n\n========================= 酒店结账退房确认菜单=======================\t\n\n\n");printf(" 按 0 :返回 "); scanf("%d",&chooseNum);switch(chooseNum) {case 0:GUI = MainUI;break;default:GUI = CheckOutUI;break;}}。
简易酒店管理系统代码
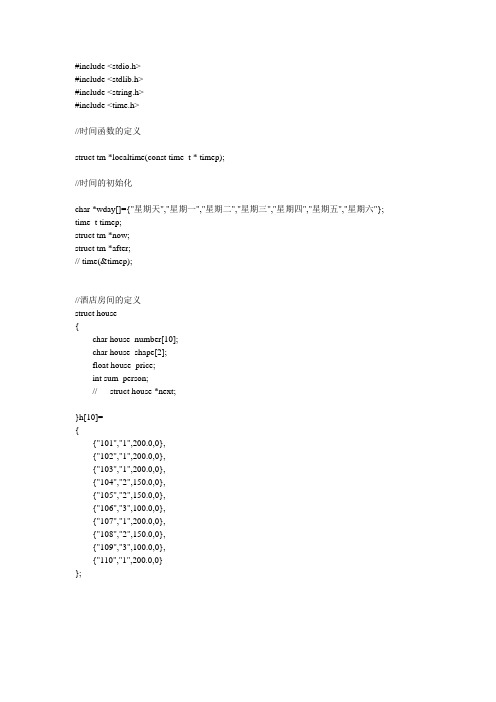
#include <stdio.h>#include <stdlib.h>#include <string.h>#include <time.h>//时间函数的定义struct tm *localtime(const time_t * timep);//时间的初始化char *wday[]={"星期天","星期一","星期二","星期三","星期四","星期五","星期六"}; time_t timep;struct tm *now;struct tm *after;// time(&timep);//酒店房间的定义struct house{char house_number[10];char house_shape[2];float house_price;int sum_person;// struct house *next;}h[10]={{"101","1",200.0,0},{"102","1",200.0,0},{"103","1",200.0,0},{"104","2",150.0,0},{"105","2",150.0,0},{"106","3",100.0,0},{"107","1",200.0,0},{"108","2",150.0,0},{"109","3",100.0,0},{"110","1",200.0,0}};{//住客姓名、性别、年龄、身份、身份证号码,房号,床号,入住日期、入住时间、离店日期、离店时间。
酒店管理系统实现代码

//入住import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A1 extends JFrame implements WindowListener {//定义各个组件JFrame frame = new JFrame ("入住系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("入住房号");JLabel moneyLabel = new JLabel("应付金额");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField moneyField= new JTextField();JButton button1,button2;/*public static void main(String args[]){A1 a1 = new A1();a1.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);//frame.addWindowListener(this);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认支付");button2=new JButton("取消支付");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "付款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认支付"){ JOptionPane.showMessageDialog(p1,"支付成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消支付"){ JOptionPane.showMessageDialog(p1,"支付失败","failure",RMATION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String room= roomField.getText();String roomword= new String(room);}});moneyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= moneyField.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(moneyLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( moneyField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setSize(200,250);frame.setVisible(true);}public void windowClosing(WindowEvent e1){System.exit(0);}public void windowOpened(WindowEvent e2){}public void windowIconified(WindowEvent e3){}public void windowDeiconified(WindowEvent e4){}public void windowClosed(WindowEvent e5){}public void windowActivated(WindowEvent e6){}public void windowDeactivated(WindowEvent e7){}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第二个://退房import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A2 extends JFrame {//定义各个组件JFrame frame = new JFrame ("退房系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("退房号");JLabel timeLabel = new JLabel("退房时间");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField timeField= new JTextField();JButton button1,button2;//JTextArea ta = new JTextArea(5,20);/*public static void main(String args[]){A2 a2 = new A2();a2.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(2,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "是否结清消费账单");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"谢谢您的大力支持!","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"请结清消费账单","failure",RMATION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String room= roomField.getText();String roomword= new String(room);}});timeField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( timeField);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2)); northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);// frame.pack();frame.setSize(200,230);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第三个://客房预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A3 extends JFrame {//定义各个组件JFrame frame = new JFrame ("客房预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel numberLabel = new JLabel("手机号");JTextField nameField = new JTextField();JTextField numberField= new JTextField();JRadioButton rb1 = new JRadioButton("来电预订");JRadioButton rb2 = new JRadioButton("电话预订"); JRadioButton rb3 = new JRadioButton("电传预订"); JRadioButton rb4 = new JRadioButton("传真预订"); JRadioButton rb5 = new JRadioButton("信函预订");JButton button1,button2;/** public static void main(String args[]){A3 a3 = new A3();a3.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "总统套房", "双人间","单人间" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "预订房类型"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"客人预订成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"客人预订失败","failure",RMATION_MESSAGE);}}});JPanel p2 = new JPanel();p2.add(rb1);p2.add(rb3);p2.add(rb4);p2.add(rb5);p2.setLayout(new FlowLayout());border = BorderFactory.createTitledBorder(etched, "预订方式"); p2.setBorder(border);//创建ButtonGroup按钮组,并在组中添加按钮ButtonGroup group1 = new ButtonGroup();group1.add(rb1);group1.add(rb2);group1.add(rb3);group1.add(rb4);group1.add(rb5);numberField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String number= numberField.getText();String numberword= new String(number);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(2,1));labelPanel.add(nameLabel);labelPanel.add(numberLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(2,1));fieldPanel.add(nameField);fieldPanel.add(numberField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,330);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第四个//餐饮预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A4 extends JFrame {//定义各个组件JFrame frame = new JFrame ("餐饮预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel timeLabel = new JLabel("预订消费时间");JTextField nameField = new JTextField();JTextField timeField= new JTextField();JCheckBox cb1 = new JCheckBox("中餐");JCheckBox cb2 = new JCheckBox("西餐");JCheckBox cb3 = new JCheckBox("红酒");JCheckBox cb4 = new JCheckBox("饮料");JCheckBox cb5 = new JCheckBox("甜点");JCheckBox cb6 = new JCheckBox("水果");JButton button1,button2;/*public static void main(String args[]){A4 a4 = new A4();a4.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();//ta.append("\nUser Name : "+username);}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "确认订餐"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"订餐成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){ JOptionPane.showMessageDialog(p1,"订餐失败","failure",RMATION_MESSAGE);}}});timeField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});JPanel p2 = new JPanel();p2.add(cb1);p2.add(cb2);p2.add(cb3);p2.add(cb4);p2.add(cb5);p2.add(cb6);Border etched1 = BorderFactory.createEtchedBorder();Border border1 = BorderFactory.createTitledBorder(etched1, "预订内容"); p2.setBorder(border1);//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,350);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第五个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A5 extends JFrame {//定义各个组件JFrame frame = new JFrame ("订单管理");JLabel a1 = new JLabel("订单号");JLabel a2 = new JLabel("订货名称");JLabel a3 = new JLabel("收货地址");JLabel a4 = new JLabel("应收金额");JLabel a5 = new JLabel("客户名称");JLabel a6 = new JLabel("结算方式");JLabel a7 = new JLabel("经办人");JTextField b1 = new JTextField();JTextField b2= new JTextField();JTextField b3= new JTextField();JTextField b4= new JTextField();JTextField b5 = new JTextField();JTextField b6 = new JTextField();JTextField b7 = new JTextField();JButton button1,button2;public static void main(String args[]){A5 a1 = new A5();a1.go();}public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();b1.setDocument(ucDocument);b1.setForeground(Color.black);b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = b1.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认收款");button2=new JButton("取消收款");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "收款方式"); p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认收款"){ JOptionPane.showMessageDialog(p1,"收款成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消收款"){ JOptionPane.showMessageDialog(p1,"收款失败","failure",RMATION_MESSAGE);}}});b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String idtify= a1.getText();String idtifyword= new String(idtify);}});b2.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String room= a2.getText();String roomword= new String(room);}});b3.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a3.getText();String idtifyword= new String(money);}});b4.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a4.getText();String idtifyword= new String(money);}});b5.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a5.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(7,1));labelPanel.add(a1);labelPanel.add(a2);labelPanel.add(a3);labelPanel.add(a4);labelPanel.add(a5);labelPanel.add(a6);labelPanel.add(a7);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(7,1));fieldPanel.add(b1);fieldPanel.add(b2);fieldPanel.add(b3);fieldPanel.add( b4);fieldPanel.add( b5);fieldPanel.add( b6);fieldPanel.add( b7);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setSize(200,250);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第六个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.text.*;import javax.swing.border.*;public class F{private JFrame frame;private JButton b1,b2,b3,b4,b5,b6,b7,b8,b9,b10,b11,b12,b13;JTextArea ta = new JTextArea(20,20);JTextArea tb = new JTextArea(20,20);public static void main(String args[]){F that=new F();that.go();}void go(){frame=new JFrame("客房检查");Container contentPane=frame.getContentPane();JPanel P1=new JPanel();P1.setLayout(new GridLayout());b1=new JButton("101 \n单人间");b2=new JButton("102 \n单人间");b3=new JButton("103 \n单人间");b4=new JButton("104 \n单人间");b5=new JButton("105 \n单人间");b6=new JButton("201 \n双人间");b7=new JButton("202 \n双人间");b8=new JButton("203 \n双人间");b9=new JButton("204 \n双人间");b10=new JButton("205 \n双人间");b11=new JButton("301 \n总统套房");b12=new JButton("302 \n总统套房");b13=new JButton("303 \n总统套房");b1.setBackground(Color.cyan);b2.setBackground(Color.cyan);b3.setBackground(Color.cyan);b4.setBackground(Color.cyan);b5.setBackground(Color.cyan);b6.setBackground(Color.magenta);b7.setBackground(Color.magenta);b8.setBackground(Color.magenta);b9.setBackground(Color.magenta);b10.setBackground(Color.magenta);b11.setBackground(Color.pink);b12.setBackground(Color.pink);b13.setBackground(Color.pink);P1.add(b1);P1.add(b2);P1.add(b3);P1.add(b4);P1.add(b5);P1.add(b6);P1.add(b7);P1.add(b8);P1.add(b9);P1.add(b10);P1.add(b11);P1.add(b12);P1.add(b13);tb.append("房间总数:13");tb.append("\n当前占用:");tb.append("\n当前可供:");P1.add(tb);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="101 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n101房间是否打扫:");ta.append("\n101房间里是否有家具损坏:");ta.append("\n101房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="102 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n102房间是否打扫:");ta.append("\n102房间里是否有家具损坏:");ta.append("\n102房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="103 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n103房间是否打扫:");ta.append("\n103房间里是否有家具损坏:");ta.append("\n103房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="104 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n104房间是否打扫:");ta.append("\n104房间里是否有家具损坏:");ta.append("\n104房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="105 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n105房间是否打扫:");ta.append("\n105房间里是否有家具损坏:");ta.append("\n105房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="201 \n双人间"){ta.setText("");JFrame frame1=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n201房间是否打扫:");ta.append("\n201房间里是否有家具损坏:");ta.append("\n201房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame1.setContentPane(p2);frame1.pack();frame1.setVisible(true);}else if(e.getActionCommand()=="202 \n双人间"){ta.setText("");JFrame frame2=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n202房间是否打扫:");ta.append("\n202房间里是否有家具损坏:");ta.append("\n202房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame2.setContentPane(p2);frame2.pack();frame2.setVisible(true);}else if(e.getActionCommand()=="203 \n双人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n203房间是否打扫:");ta.append("\n203房间里是否有家具损坏:");ta.append("\n203房间是否交足余额:");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="204 \n双人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n204房间是否打扫:");ta.append("\n204房间里是否有家具损坏:");。
酒店管理系统实现代码之欧阳家百创编
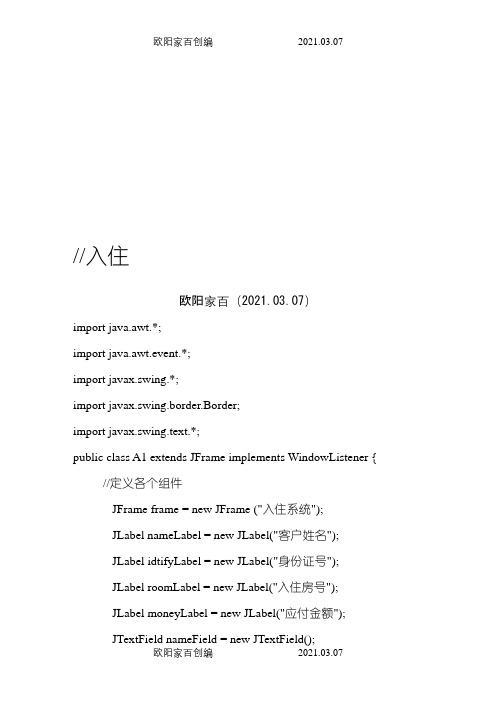
//入住欧阳家百(2021.03.07)import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A1 extends JFrame implements WindowListener { //定义各个组件JFrame frame = new JFrame ("入住系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("入住房号");JLabel moneyLabel = new JLabel("应付金额");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField moneyField= new JTextField();JButton button1,button2;/*public static void main(String args[]){A1 a1 = new A1();a1.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);//frame.addWindowListener(this);nameField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认支付");button2=new JButton("取消支付");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "付款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认支付"){JOptionPane.showMessageDialog(p1,"支付成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消支付"){JOptionPane.showMessageDialog(p1,"支付失败","failure",RMATION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String room= roomField.getText();String roomword= new String(room);}});moneyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= moneyField.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1)); labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(moneyLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1)); fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( moneyField);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel 放在northcp.add(p1,BorderLayout.SOUTH);frame.setSize(200,250);frame.setVisible(true);}public void windowClosing(WindowEvent e1){System.exit(0);}public void windowOpened(WindowEvent e2){ }public void windowIconified(WindowEvent e3){}public void windowDeiconified(WindowEvent e4){}public void windowClosed(WindowEvent e5){}public void windowActivated(WindowEvent e6){}public void windowDeactivated(WindowEvent e7){}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet); }}第二个://退房import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A2 extends JFrame {//定义各个组件JFrame frame = new JFrame ("退房系统");JLabel nameLabel = new JLabel("客户姓名"); JLabel idtifyLabel = new JLabel("身份证号"); JLabel roomLabel = new JLabel("退房号"); JLabel timeLabel = new JLabel("退房时间"); JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField timeField= new JTextField();JButton button1,button2;//JTextArea ta = new JTextArea(5,20);/*public static void main(String args[]){A2 a2 = new A2();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button2);p1.setLayout(new GridLayout(2,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "是否结清消费账单");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){JOptionPane.showMessageDialog(p1,"谢谢您的大力支持!","success",}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){JOptionPane.showMessageDialog(p1,"请结清消费账单","failure",}}});idtifyField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String room= roomField.getText();String roomword= new String(room);}timeField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String time= timeField.getText();String timeword= new String(time);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel 放在northcp.add(p1,BorderLayout.SOUTH);// frame.pack();frame.setSize(200,230);}}class UpperCaseDocument extends PlainDocument { public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第三个://客房预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.text.*;public class A3 extends JFrame {//定义各个组件JFrame frame = new JFrame ("客房预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel numberLabel = new JLabel("手机号");JTextField nameField = new JTextField();JTextField numberField= new JTextField();JRadioButton rb1 = new JRadioButton("来电预订");JRadioButton rb2 = new JRadioButton("电话预订"); JRadioButton rb3 = new JRadioButton("电传预订");JRadioButton rb4 = new JRadioButton("传真预订");JRadioButton rb5 = new JRadioButton("信函预订");JButton button1,button2;/*{A3 a3 = new A3();a3.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "总统套房", "双人间","单人间" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "预订房类型");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){JOptionPane.showMessageDialog(p1,"客人预订成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public voidactionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){JOptionPane.showMessageDialog(p1,"客人预订失败","failure",RMATION_MESSAGE);}}});JPanel p2 = new JPanel();p2.add(rb1);p2.add(rb2);p2.add(rb3);p2.add(rb4);p2.add(rb5);p2.setLayout(new FlowLayout());border = BorderFactory.createTitledBorder(etched, "预订方式");p2.setBorder(border);//创建ButtonGroup按钮组,并在组中添加按钮ButtonGroup group1 = new ButtonGroup();group1.add(rb1);group1.add(rb2);group1.add(rb3);group1.add(rb4);group1.add(rb5);numberField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String number= numberField.getText();String numberword= new String(number);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(2,1));labelPanel.add(nameLabel);labelPanel.add(numberLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(2,1));fieldPanel.add(nameField);fieldPanel.add(numberField);//面板northPanel放面板lanelPanel和面板fieldPanel JPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel 放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,330);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第四个//餐饮预订import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A4 extends JFrame {//定义各个组件JFrame frame = new JFrame ("餐饮预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel timeLabel = new JLabel("预订消费时间"); JTextField nameField = new JTextField();JTextField timeField= new JTextField();JCheckBox cb1 = new JCheckBox("中餐");JCheckBox cb2 = new JCheckBox("西餐");JCheckBox cb3 = new JCheckBox("红酒");JCheckBox cb4 = new JCheckBox("饮料");JCheckBox cb5 = new JCheckBox("甜点");JCheckBox cb6 = new JCheckBox("水果");JButton button1,button2;/*public static void main(String args[]){A4 a4 = new A4();a4.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();//ta.append("\nUser Name : "+username);}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "确认订餐");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){JOptionPane.showMessageDialog(p1,"订餐成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){JOptionPane.showMessageDialog(p1,"订餐失败","failure",RMATION_MESSAGE);}}});timeField.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { String time= timeField.getText();String timeword= new String(time);}});JPanel p2 = new JPanel();p2.add(cb1);p2.add(cb2);p2.add(cb3);p2.add(cb4);p2.add(cb5);p2.add(cb6);Border etched1 = BorderFactory.createEtchedBorder();Border border1 = BorderFactory.createTitledBorder(etched1, "预订内容");p2.setBorder(border1);//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel 放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,350);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第五个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.border.Border;import javax.swing.text.*;public class A5 extends JFrame {//定义各个组件JFrame frame = new JFrame ("订单管理");JLabel a1 = new JLabel("订单号");JLabel a2 = new JLabel("订货名称");JLabel a3 = new JLabel("收货地址");JLabel a4 = new JLabel("应收金额");JLabel a5 = new JLabel("客户名称"); JLabel a6 = new JLabel("结算方式"); JLabel a7 = new JLabel("经办人");JTextField b1 = new JTextField();JTextField b2= new JTextField();JTextField b3= new JTextField();JTextField b4= new JTextField();JTextField b5 = new JTextField();JTextField b6 = new JTextField();JTextField b7 = new JTextField();JButton button1,button2;public static void main(String args[]){A5 a1 = new A5();a1.go();}public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();b1.setDocument(ucDocument);b1.setForeground(Color.black);b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = b1.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认收款");button2=new JButton("取消收款");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "收款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认收款"){JOptionPane.showMessageDialog(p1,"收款成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消收款"){JOptionPane.showMessageDialog(p1,"收款失败","failure",RMATION_MESSAGE);}}});b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= a1.getText();String idtifyword= new String(idtify);}});b2.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String room= a2.getText();String roomword= new String(room);}});b3.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a3.getText();String idtifyword= new String(money);}});b4.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { String money= a4.getText();String idtifyword= new String(money);}});b5.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= a5.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(7,1));labelPanel.add(a1);labelPanel.add(a2);labelPanel.add(a3);labelPanel.add(a4);labelPanel.add(a5);labelPanel.add(a6);labelPanel.add(a7);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(7,1));fieldPanel.add(b1);fieldPanel.add(b2);fieldPanel.add(b3);fieldPanel.add( b4);fieldPanel.add( b5);fieldPanel.add( b6);fieldPanel.add( b7);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel 放在northcp.add(p1,BorderLayout.SOUTH);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setSize(200,250);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第六个:import java.awt.*;import java.awt.event.*;import javax.swing.*;import javax.swing.text.*;import javax.swing.border.*;public class F{private JFrame frame;private JButton b1,b2,b3,b4,b5,b6,b7,b8,b9,b10,b11,b12,b13; JTextArea ta = new JTextArea(20,20);JTextArea tb = new JTextArea(20,20);public static void main(String args[]){F that=new F();that.go();}void go(){frame=new JFrame("客房检查");Container contentPane=frame.getContentPane(); JPanel P1=new JPanel();P1.setLayout(new GridLayout());b1=new JButton("101 \n单人间");b2=new JButton("102 \n单人间");b3=new JButton("103 \n单人间");b4=new JButton("104 \n单人间 ");b5=new JButton("105 \n单人间");b6=new JButton("201 \n双人间");b7=new JButton("202 \n双人间");b8=new JButton("203 \n双人间");b9=new JButton("204 \n双人间");b10=new JButton("205 \n双人间"); b11=new JButton("301 \n总统套房"); b12=new JButton("302 \n总统套房"); b13=new JButton("303 \n总统套房"); b1.setBackground(Color.cyan);b2.setBackground(Color.cyan);b3.setBackground(Color.cyan);b4.setBackground(Color.cyan);b5.setBackground(Color.cyan);b6.setBackground(Color.magenta);b7.setBackground(Color.magenta);b8.setBackground(Color.magenta);b9.setBackground(Color.magenta);b10.setBackground(Color.magenta); b11.setBackground(Color.pink);b12.setBackground(Color.pink);b13.setBackground(Color.pink);P1.add(b1);P1.add(b2);P1.add(b3);P1.add(b4);P1.add(b5);P1.add(b6);P1.add(b7);P1.add(b8);P1.add(b9);P1.add(b10);P1.add(b11);P1.add(b12);P1.add(b13);tb.append("房间总数:13");tb.append("\n当前占用:");tb.append("\n当前可供:");P1.add(tb);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="101 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n101房间是否打扫: ");ta.append("\n101房间里是否有家具损坏:");ta.append("\n101房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
酒店管理系统实现代码集团标准化小组:[VVOPPT-JOPP28-JPPTL98-LOPPNN]//入住import java.awt.*;import javax.swing.*;public class A1 extends JFrame implements WindowListener {//定义各个组件JFrame frame = new JFrame ("入住系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("入住房号");JLabel moneyLabel = new JLabel("应付金额");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField moneyField= new JTextField();JButton button1,button2;/*public static void main(String args[]){A1 a1 = new A1();a1.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);//frame.addWindowListener(this);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认支付");button2=new JButton("取消支付");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "付款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认支付"){ JOptionPane.showMessageDialog(p1,"支付成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消支付"){JOptionPane.showMessageDialog(p1,"支付失败","failure",RMATION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String room= roomField.getText();String roomword= new String(room);}});moneyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= moneyField.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(moneyLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( moneyField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setSize(200,250);frame.setVisible(true);}public void windowClosing(WindowEvent e1){System.exit(0);}public void windowOpened(WindowEvent e2){}public void windowIconified(WindowEvent e3){}public void windowDeiconified(WindowEvent e4){}public void windowClosed(WindowEvent e5){}public void windowActivated(WindowEvent e6){}public void windowDeactivated(WindowEvent e7){}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第二个://退房import java.awt.*;import javax.swing.*;public class A2 extends JFrame {//定义各个组件JFrame frame = new JFrame ("退房系统");JLabel nameLabel = new JLabel("客户姓名");JLabel idtifyLabel = new JLabel("身份证号");JLabel roomLabel = new JLabel("退房号");JLabel timeLabel = new JLabel("退房时间");JTextField nameField = new JTextField();JTextField idtifyField= new JTextField();JTextField roomField= new JTextField();JTextField timeField= new JTextField();JButton button1,button2;//JTextArea ta = new JTextArea(5,20);/*public static void main(String args[]){A2 a2 = new A2();a2.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(2,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "是否结清消费账单");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"谢谢您的大力支持!","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){JOptionPane.showMessageDialog(p1,"请结清消费账单","failure",RMATION_MESSAGE);}}});idtifyField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= idtifyField.getText();String idtifyword= new String(idtify);}});roomField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String room= roomField.getText();String roomword= new String(room);}});timeField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(idtifyLabel);labelPanel.add(roomLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(idtifyField);fieldPanel.add(roomField);fieldPanel.add( timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);// frame.pack();frame.setSize(200,230);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第三个://客房预订import java.awt.*;import javax.swing.*;public class A3 extends JFrame {//定义各个组件JFrame frame = new JFrame ("客房预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel numberLabel = new JLabel("手机号");JTextField nameField = new JTextField();JTextField numberField= new JTextField();JRadioButton rb1 = new JRadioButton("来电预订");JRadioButton rb2 = new JRadioButton("电话预订");JRadioButton rb3 = new JRadioButton("电传预订");JRadioButton rb4 = new JRadioButton("传真预订");JRadioButton rb5 = new JRadioButton("信函预订");JButton button1,button2;/** public static void main(String args[]){A3 a3 = new A3();a3.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();}});JComboBox jcb1;//组合框String[] itemList = { "总统套房", "双人间","单人间" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "预订房类型");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"客人预订成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){JOptionPane.showMessageDialog(p1,"客人预订失败","failure",RMATION_MESSAGE);}}});JPanel p2 = new JPanel();p2.add(rb1);p2.add(rb2);p2.add(rb3);p2.add(rb4);p2.add(rb5);p2.setLayout(new FlowLayout());border = BorderFactory.createTitledBorder(etched, "预订方式");p2.setBorder(border);//创建ButtonGroup按钮组,并在组中添加按钮ButtonGroup group1 = new ButtonGroup();group1.add(rb1);group1.add(rb2);group1.add(rb3);group1.add(rb4);group1.add(rb5);numberField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String number= numberField.getText();String numberword= new String(number);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(2,1));labelPanel.add(nameLabel);labelPanel.add(numberLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(2,1));fieldPanel.add(nameField);fieldPanel.add(numberField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,330);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第四个//餐饮预订import java.awt.*;import javax.swing.*;public class A4 extends JFrame {//定义各个组件JFrame frame = new JFrame ("餐饮预订");JLabel nameLabel = new JLabel("预订人姓名");JLabel timeLabel = new JLabel("预订消费时间");JTextField nameField = new JTextField();JTextField timeField= new JTextField();JCheckBox cb1 = new JCheckBox("中餐");JCheckBox cb2 = new JCheckBox("西餐");JCheckBox cb3 = new JCheckBox("红酒");JCheckBox cb4 = new JCheckBox("饮料");JCheckBox cb5 = new JCheckBox("甜点");JCheckBox cb6 = new JCheckBox("水果");JButton button1,button2;/*public static void main(String args[]){A4 a4 = new A4();a4.go();}*/public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();nameField.setDocument(ucDocument);nameField.setForeground(Color.black);nameField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = nameField.getText();//ta.append("\nUser Name : "+username);}});button1=new JButton("是");button2=new JButton("否");final JPanel p1 = new JPanel();p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "确认订餐");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="是"){ JOptionPane.showMessageDialog(p1,"订餐成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="否"){JOptionPane.showMessageDialog(p1,"订餐失败","failure",RMATION_MESSAGE);}}});timeField.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String time= timeField.getText();String timeword= new String(time);}});JPanel p2 = new JPanel();p2.add(cb1);p2.add(cb2);p2.add(cb3);p2.add(cb4);p2.add(cb5);p2.add(cb6);Border etched1 = BorderFactory.createEtchedBorder();Border border1 = BorderFactory.createTitledBorder(etched1, "预订内容");p2.setBorder(border1);//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(4,1));labelPanel.add(nameLabel);labelPanel.add(timeLabel);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(4,1));fieldPanel.add(nameField);fieldPanel.add(timeField);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p2,BorderLayout.CENTER);cp.add(p1,BorderLayout.SOUTH);frame.setSize(200,350);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第五个:import java.awt.*;import javax.swing.*;public class A5 extends JFrame {//定义各个组件JFrame frame = new JFrame ("订单管理");JLabel a1 = new JLabel("订单号");JLabel a2 = new JLabel("订货名称");JLabel a3 = new JLabel("收货地址");JLabel a4 = new JLabel("应收金额");JLabel a5 = new JLabel("客户名称");JLabel a6 = new JLabel("结算方式");JLabel a7 = new JLabel("经办人");JTextField b1 = new JTextField();JTextField b2= new JTextField();JTextField b3= new JTextField();JTextField b4= new JTextField();JTextField b5 = new JTextField();JTextField b6 = new JTextField();JTextField b7 = new JTextField();JButton button1,button2;public static void main(String args[]){A5 a1 = new A5();a1.go();}public void go(){UpperCaseDocument ucDocument = new UpperCaseDocument();b1.setDocument(ucDocument);b1.setForeground(Color.black);b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String username = b1.getText();}});JComboBox jcb1;//组合框String[] itemList = { "现金", "刷卡" };jcb1 = new JComboBox(itemList);jcb1.setSelectedIndex(0);button1=new JButton("确认收款");button2=new JButton("取消收款");final JPanel p1 = new JPanel();p1.add(jcb1);p1.add(button1);p1.add(button2);p1.setLayout(new GridLayout(3,1));Border etched = BorderFactory.createEtchedBorder();Border border = BorderFactory.createTitledBorder(etched, "收款方式");p1.setBorder(border);button1.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="确认收款"){ JOptionPane.showMessageDialog(p1,"收款成功","success",RMATION_MESSAGE);}}});button2.addActionListener(new ActionListener(){public void actionPerformed(ActionEvent e){if (e.getActionCommand()=="取消收款"){JOptionPane.showMessageDialog(p1,"收款失败","failure",RMATION_MESSAGE);}}});b1.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String idtify= a1.getText();String idtifyword= new String(idtify);}});b2.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String room= a2.getText();String roomword= new String(room);}});b3.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= a3.getText();String idtifyword= new String(money);}});b4.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= a4.getText();String idtifyword= new String(money);}});b5.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e){ String money= a5.getText();String idtifyword= new String(money);}});//面板labelPanel放标签JPanel labelPanel = new JPanel();labelPanel.setLayout(new GridLayout(7,1));labelPanel.add(a1);labelPanel.add(a2);labelPanel.add(a3);labelPanel.add(a4);labelPanel.add(a5);labelPanel.add(a6);labelPanel.add(a7);//面板fieldPanel放文本框JPanel fieldPanel = new JPanel();fieldPanel.setLayout(new GridLayout(7,1));fieldPanel.add(b1);fieldPanel.add(b2);fieldPanel.add(b3);fieldPanel.add( b4);fieldPanel.add( b5);fieldPanel.add( b6);fieldPanel.add( b7);//面板northPanel放面板lanelPanel和面板fieldPanelJPanel northPanel = new JPanel();northPanel.setLayout(new GridLayout(1,2));northPanel.add(labelPanel);northPanel.add(fieldPanel);Container cp = frame.getContentPane();cp.add(northPanel,BorderLayout.NORTH);//northPanel放在northcp.add(p1,BorderLayout.SOUTH);frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frame.setSize(200,250);frame.setVisible(true);}}class UpperCaseDocument extends PlainDocument{ public void insertString(int offset,String string, AttributeSet attributeSet)throws BadLocationException{ string = string.toUpperCase();super.insertString(offset,string, attributeSet);}}第六个:import java.awt.*;import javax.swing.*;public class F{private JFrame frame;private JButton b1,b2,b3,b4,b5,b6,b7,b8,b9,b10,b11,b12,b13;JTextArea ta = new JTextArea(20,20);JTextArea tb = new JTextArea(20,20);public static void main(String args[]){F that=new F();that.go();}void go(){frame=new JFrame("客房检查");Container contentPane=frame.getContentPane(); JPanel P1=new JPanel();P1.setLayout(new GridLayout());b1=new JButton("101 \n单人间");b2=new JButton("102 \n单人间");b3=new JButton("103 \n单人间");b4=new JButton("104 \n单人间 ");b5=new JButton("105 \n单人间");b6=new JButton("201 \n双人间");b7=new JButton("202 \n双人间");b8=new JButton("203 \n双人间");b9=new JButton("204 \n双人间");b10=new JButton("205 \n双人间");b11=new JButton("301 \n总统套房");b12=new JButton("302 \n总统套房");b13=new JButton("303 \n总统套房");b1.setBackground(Color.cyan);b2.setBackground(Color.cyan);b3.setBackground(Color.cyan);b4.setBackground(Color.cyan);b5.setBackground(Color.cyan);b6.setBackground(Color.magenta);b7.setBackground(Color.magenta);b8.setBackground(Color.magenta);b9.setBackground(Color.magenta);b10.setBackground(Color.magenta);b11.setBackground(Color.pink);b12.setBackground(Color.pink);b13.setBackground(Color.pink);P1.add(b1);P1.add(b2);P1.add(b3);P1.add(b4);P1.add(b5);P1.add(b6);P1.add(b7);P1.add(b8);P1.add(b9);P1.add(b10);P1.add(b11);P1.add(b12);P1.add(b13);tb.append("房间总数:13");tb.append("\n当前占用:");tb.append("\n当前可供:");P1.add(tb);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="101 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n101房间是否打扫: ");ta.append("\n101房间里是否有家具损坏: ");ta.append("\n101房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="102 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n102房间是否打扫: ");ta.append("\n102房间里是否有家具损坏: ");ta.append("\n102房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="103 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n103房间是否打扫: ");ta.append("\n103房间里是否有家具损坏: ");ta.append("\n103房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="104 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n104房间是否打扫: ");ta.append("\n104房间里是否有家具损坏: ");ta.append("\n104房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="105 \n单人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n105房间是否打扫: ");ta.append("\n105房间里是否有家具损坏: ");ta.append("\n105房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.yellow);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="201 \n双人间"){ta.setText("");JFrame frame1=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n201房间是否打扫: ");ta.append("\n201房间里是否有家具损坏: ");ta.append("\n201房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame1.setContentPane(p2);frame1.pack();frame1.setVisible(true);}else if(e.getActionCommand()=="202 \n双人间"){ta.setText("");JFrame frame2=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n202房间是否打扫: ");ta.append("\n202房间里是否有家具损坏: ");ta.append("\n202房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame2.setContentPane(p2);frame2.pack();frame2.setVisible(true);}else if(e.getActionCommand()=="203 \n双人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n203房间是否打扫: ");ta.append("\n203房间里是否有家具损坏: ");ta.append("\n203房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame.setContentPane(p2);frame.pack();frame.setVisible(true);}else if(e.getActionCommand()=="204 \n双人间"){ta.setText("");JFrame frame=new JFrame("检查信息");JPanel p2=new JPanel();Container cp=frame.getContentPane();ta.append("\n204房间是否打扫: ");ta.append("\n204房间里是否有家具损坏: ");ta.append("\n204房间是否交足余额: ");JButton a=new JButton("提交");p2.add(a);ActionListener al=new ActionListener(){public void actionPerformed(ActionEvent e){if(e.getActionCommand()=="提交"){JOptionPane.showMessageDialog(ta,"提交成功 ",null,RMATION_MESSAGE);}}};a.addActionListener(al);p2.add(ta);p2.setBackground(Color.cyan);frame.setContentPane(p2);frame.pack();。