java练习题
Java日常练习题,每天进步一点点(11)

Java⽇常练习题,每天进步⼀点点(11)⽬录1、对接⼝的描述正确的是()2、类中的数据域使⽤private修饰为私有变量,所以任何⽅法均不能访问它。
3、已知如下类定义:4、在java的⼀个异常处理中,可以包含多个的语句块是()。
5、关于Java语⾔中的final关键字的使⽤,下列说法正确的是()6、在 main() ⽅法中给出的整型数组,如果将其写到⼀个⽂件中,需要()。
7、下列有关Servlet的⽣命周期,说法不正确的是?8、What is displayed when the following is executed?9、假定Base b = new Derived(); 调⽤执⾏b.methodOne()后,输出结果是什么?10、下⾯关于变量及其范围的陈述哪些是不正确的()答案汇总:总结承蒙各位厚爱,我们⼀起每天进步⼀点点!(⿏标选中空⽩处查看答案)1、对接⼝的描述正确的是()正确答案: A⼀个类可以实现多个接⼝接⼝可以有⾮静态的成员变量在jdk8之前,接⼝可以实现⽅法实现接⼝的任何类,都需要实现接⼝的⽅法题解:A,⼀个类只能有⼀个直接⽗类,但是继承是有传递性的。
⼀个类可以实现多的接⼝。
⼀个接⼝可以继承多个类。
B,接⼝中没有普通变量(普通成员变量),接⼝中都是常量,默认修饰符:public static finalC,JDK8之前,接⼝中的⽅法都是默认public abstract的,JDK8之后,接⼝中可以有static、default的修饰的⽅法,⼀旦被修饰,⽅法必须有⽅法体(抽象⽅法可是没有⽅法体的),接⼝中的⽅法都不能被private和protected 修饰,同时外部接⼝、类只能被public修饰或者不写,但是内部接⼝、类可以被四个访问修饰符修饰。
D,实现接⼝,其实就是需要重写接⼝中的abstract⽅法,⼀旦实现的类没有重写完,那么这个类必须是个抽象类(抽象类中可以没有抽象⽅法,但是有抽象⽅法的类必须是抽象类)。
java基础50道经典练习题及答案
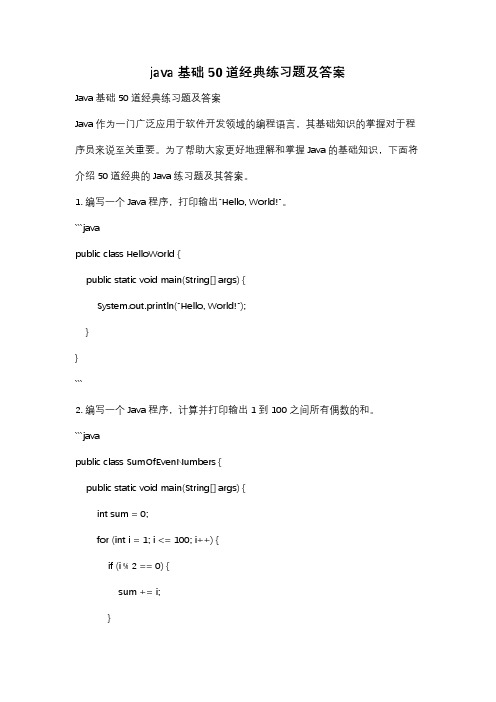
java基础50道经典练习题及答案Java基础50道经典练习题及答案Java作为一门广泛应用于软件开发领域的编程语言,其基础知识的掌握对于程序员来说至关重要。
为了帮助大家更好地理解和掌握Java的基础知识,下面将介绍50道经典的Java练习题及其答案。
1. 编写一个Java程序,打印输出"Hello, World!"。
```javapublic class HelloWorld {public static void main(String[] args) {System.out.println("Hello, World!");}}```2. 编写一个Java程序,计算并打印输出1到100之间所有偶数的和。
```javapublic class SumOfEvenNumbers {public static void main(String[] args) {int sum = 0;for (int i = 1; i <= 100; i++) {if (i % 2 == 0) {sum += i;}System.out.println("Sum of even numbers: " + sum);}}```3. 编写一个Java程序,判断一个整数是否为素数。
```javapublic class PrimeNumber {public static void main(String[] args) {int number = 17;boolean isPrime = true;for (int i = 2; i <= Math.sqrt(number); i++) {if (number % i == 0) {isPrime = false;break;}}if (isPrime) {System.out.println(number + " is a prime number.");} else {System.out.println(number + " is not a prime number."); }}```4. 编写一个Java程序,将一个字符串反转并输出。
JAVA练习题
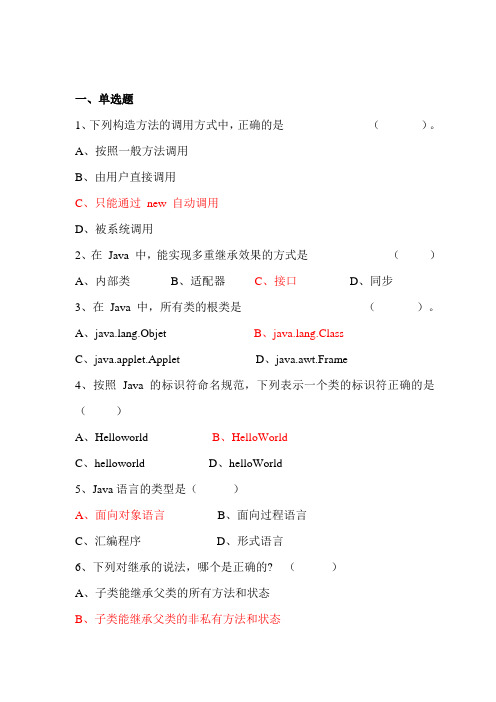
一、单选题1、下列构造方法的调用方式中,正确的是()。
A、按照一般方法调用B、由用户直接调用C、只能通过new 自动调用D、被系统调用2、在Java 中,能实现多重继承效果的方式是()A、内部类B、适配器C、接口D、同步3、在Java 中,所有类的根类是()。
A、ng.ObjetB、ng.ClassC、java.applet.AppletD、java.awt.Frame4、按照Java 的标识符命名规范,下列表示一个类的标识符正确的是()A、HelloworldB、HelloWorldC、helloworldD、helloWorld5、Java语言的类型是()A、面向对象语言B、面向过程语言C、汇编程序D、形式语言6、下列对继承的说法,哪个是正确的? ()A、子类能继承父类的所有方法和状态B、子类能继承父类的非私有方法和状态C、子类只能继承父类public方法和状态D、子类只能继承父类的方法,而不继承状态7、下列哪些内容是异常的含义?()异常是一个运行时错误7、()是不能被当前类的子类重新定义的方法。
A、抽象方法B、私有方法C、最终方法D、构造方法8、下列命令中,哪个命令是Java的编译命令?()A、javacB、javaC、javadocD、appletviewer9、下列有关Java语言的叙述中,正确的是()A、Java是不区分大小写的B、源文件名与public类型的类名必须相同C、源文件名其扩展名为.jarD、源文件中public类的数目不限10、若数组a定义为int[][]a=new int[3][4],则a是()A、一维数组B、二维数组C、三维数组D、四维数组11、下列关于Java语言特点的叙述中,错误的是( )A、Java支持分布式计算B、Java是面向过程的编程语言C、Java是跨平台的编程语言D、Java支持多线程12、用来导入已定义好的类或包的语句是()A、importB、mainC、public classD、class13、如要抛出异常,应用下列哪种子句?()A、catchB、throwC、tryD、finally14、下列的哪个选项可以正确用以表示十六进制值16?()A、0x8B、0x10C、08D、01015、要想定义一个不能被实例化的抽象类,在类定义中必须加上修饰符( )。
50道java经典练习题
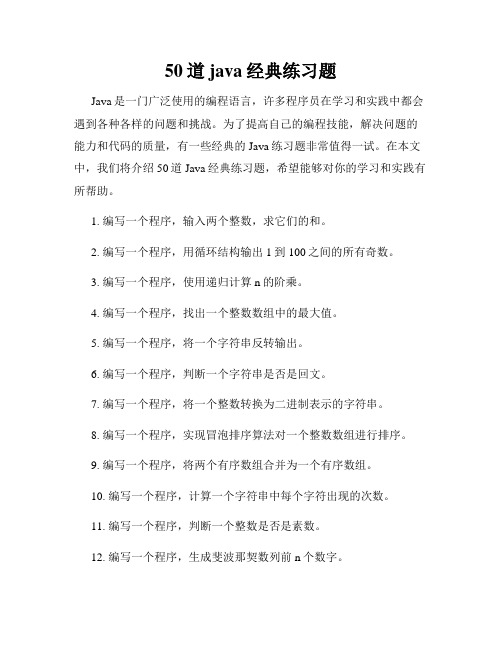
50道java经典练习题Java是一门广泛使用的编程语言,许多程序员在学习和实践中都会遇到各种各样的问题和挑战。
为了提高自己的编程技能,解决问题的能力和代码的质量,有一些经典的Java练习题非常值得一试。
在本文中,我们将介绍50道Java经典练习题,希望能够对你的学习和实践有所帮助。
1. 编写一个程序,输入两个整数,求它们的和。
2. 编写一个程序,用循环结构输出1到100之间的所有奇数。
3. 编写一个程序,使用递归计算n的阶乘。
4. 编写一个程序,找出一个整数数组中的最大值。
5. 编写一个程序,将一个字符串反转输出。
6. 编写一个程序,判断一个字符串是否是回文。
7. 编写一个程序,将一个整数转换为二进制表示的字符串。
8. 编写一个程序,实现冒泡排序算法对一个整数数组进行排序。
9. 编写一个程序,将两个有序数组合并为一个有序数组。
10. 编写一个程序,计算一个字符串中每个字符出现的次数。
11. 编写一个程序,判断一个整数是否是素数。
12. 编写一个程序,生成斐波那契数列前n个数字。
13. 编写一个程序,找出一个整数数组中的前k个最大值。
14. 编写一个程序,计算一个整数数组的平均值。
15. 编写一个程序,判断一个字符串是否是有效的括号序列。
16. 编写一个程序,将一个整数翻转输出。
17. 编写一个程序,计算两个整数的最大公约数。
18. 编写一个程序,找出一个字符串中最长的连续子串。
19. 编写一个程序,找出一个字符串中出现次数最多的字符。
20. 编写一个程序,将一个整数数组循环右移k位。
21. 编写一个程序,计算一个浮点数的平方根。
22. 编写一个程序,将一个字符串按单词逆序输出。
23. 编写一个程序,判断一个字符串是否是有效的回文字符串。
24. 编写一个程序,计算两个浮点数的最大公约数。
25. 编写一个程序,找出一个整数数组中的前k个最小值。
26. 编写一个程序,判断两个字符串是否互为变位词。
27. 编写一个程序,将一个整数数组按奇偶分成两个数组。
java试题练习题(第9套)

—— 学年第 学期 《 Java 程序设计 》课程试题 课程号: √ 考试 □ A 卷 √ 闭卷 □ 考查 □ B 卷 □ 开卷一、单项选择题(20题;每题2分,共40分) 1、下面选项中,___不可以用作变量名的首字符。
A )字母 B )下划线(_) C )数字 D )美元符号(¥) 答案:C (难度系数C )知识点:变量 2、下面语句中,____不会出现编译警告或错误。
A )float f=1.3; B )char c=”a”; C )byte b=25; D )boolean b=null; 答案:C (难度系数B )知识点:赋值相容 3、下列叙述正确的是___。
A )final 类可以有子类 B )abstract 类中只可以有 abstract 方法 C )abstract 类上可以有非abstract 方法,但该方法不可以用final 修饰 D )不可以同时用final 和abstract 修饰一个方法 答案:D (难度系数B ) 知识点:抽象类,抽象方法 4、创建一个标识有“关闭”按钮的语句是___。
A ) TextField b = new TextField(“关闭”);B ) Label b = new Label(“关闭”);C ) Checkbox b = new Checkbox(“关闭”);D ) Button b = new Button(“关闭”);答案:D (难度系数C ) 知识点:GUI 编程5、在编写异常处理的Java 程序中,每个catch 语句块都应该与___语句块对应,使得用该语句块来启动Java 的异常处理机制。
班级:姓名: 学号:试题共页加白纸张密封线A)if – else B)switch C)try D)throw答案:C(难度系数B)知识点:异常6、paint()方法使用_____类型的参数。
A)Graphics B)Graphics2D C)String D)Color 答案:A (难度系数B,知识点applet)7、下列语句正确的是________。
Java语言练习题库(含答案)

Java语⾔练习题库(含答案)单选题1. 为了保证⽅法的线程安全,声明⽅法的时候必须⽤哪个修饰符?(A) new(B) transient(C) void(D) synchronized2. 编译Java源⽂件使⽤哪个?(A) javac(B) jdb(C) javadoc(D) junit3. 哪⼀种类的对象中包含有Internet地址。
(A) Applet(B) Datagramsocket(C) InetAddress(D) AppletContext4. 有关GUI容器叙述,不正确的是?(A) 容器是⼀种特殊的组件,它可⽤来放置其它组件(B) 容器是组成GUI所必需的元素(C) 容器是⼀种特殊的组件,它可被放置在其它容器中(D) 容器是⼀种特殊的组件,它可被放置在任何组件中5. 使⽤javadoc⽣成的⽂档的⽂件格式是?(A) XML格式(B) ⾃定义格式(C) ⼆进制格式(D) HTML格式6. 下列有关类、对象和实例的叙述,正确的是哪⼀项?(A) 类就是对象,对象就是类,实例是对象的另⼀个名称,三者没有差别(B) 对象是类的抽象,类是对象的具体化,实例是对象的另⼀个名称(C) 类是对象的抽象,对象是类的具体化,实例是类的另⼀个名称(D) 类是对象的抽象,对象是类的具体化,实例是对象的另⼀个名称7. 在事件委托类的继承体系中,最⾼层次的类是哪项?(A) java.util.EventListener(B) java.util.EventObject(C) java.awt.AWTEvent(D) java.awt.event.AWTEvent8. Java语⾔中异常的分类是哪项?(A) 运⾏时异常和异常(B) 受检异常和⾮受检异常(C) 错误和异常(D) 错误和运⾏时异常9. 使⽤下列哪些关键字可以判定实参的具体类型?(A) as(B) is(C) instanceof(D) extends10. 在⽅法的声明中,要求该⽅法必须抛出异常时使⽤哪个关键字?(A) Throw(B) catch(C) finally(D) throws11. Applet的布局设置默认是FlowLayout,下列中哪项代码可以改变Applet的布局⽅式?(A) setLayoutManager(new GridLayout());(B) setLayout (new GridLayout(2,2));(C) setGridLayout (2,2);(D) setBorderLayout();12. 下列哪项正确?(A) JDK中包含JRE,JVM中包含JRE(B) JRE中包含JDK,JDK中包含JVM(C) JRE中包含JDK,JVM中包含JRE(D) JDK中包含JRE,JRE中包含JVM13. 在MyThread类的main⽅法中,为⽤Thread实例化。
java练习

一、单选题(每题2分,共20分)1、Java 属于以下哪种语言?A 、机器语言B 、 汇编语言C 、高级语言D 、以上都不对 2、下面哪种类型的文件可以在Java 虚拟机中运行?A 、.javaB 、.jreC 、.exeD 、.class3、安装好JDK 后,在其bin 目录下有许多exe 可执行文件,其中java.exe 命令的作用是以下哪一种?A 、Java 文档制作工具B 、Java 解释器C 、Java 编译器D 、Java 启动器4、以下关于变量的说法错误的是?A 、变量名必须是一个有效的标识符B 、变量在定义时可以没有初始值C 、变量一旦被定义,在程序中的任何位置都可以被访问D 、在程序中,可以将一个byte 类型的值赋给一个int 类型的变量,不需要特殊声明5、请先阅读下面的代码。
int x = 1; int y = 2;if (x % 2 == 0) { y++; } else { y--; }System.out.println("y=" + y);上面一段程序运行结束时,变量y 的值为下列哪一项?A 、1B 、2C 、3D 、switch 语句6、以下哪个选项可以正确创建一个长度为3的二维数组?A 、 new int [2][3];B 、 new int[3][];C 、 new int[][3];D 、 以上答案皆不对7、下面哪一个是正确的类的声明? DA 、 public void HH {…}B 、 public class Move(){…}C 、 public class void number{}D 、 public class Car {…}8、在以下什么情况下,构造方法会被调用?A 、 类定义时B 、 创建对象时C 、 调用对象方法时D 、 使用对象的变量时9、在Java 中,针对类、成员方法和属性提供了4种访问级别,以下控制级别由小到大依次是( )。
第1章 Java入门练习题

10.设有如下程序:
public class Hello{
public void main(String args[])
{ System.out.println("Hello World!");}
}
该程序有如下哪种可能的结果?()
(A)执行时产生异常(B)程序不能编译
四、简答题
1.为什么说Java的运行与计算机硬件平台无关?
2. Java对源程序文件的命名规则有什么要求?源程序文件编译后生成的是什么文件?
五、编程题
1.编写一个Java Application,利用JDK软件包中的工具编译并运行这个程序,在屏幕上输出“Welcome to Java World!”。
(A)13个字节码文件,扩展名为.class(B)1个字节码文件,扩展名为.class
(C)3个字节码文件,扩展名为.java(D)3个字节码文件,扩展名为.class
4.阅读下列代码,选出该代码段正确的文件名()。
class A{
void method1(){
System.out.println("Method1 inclass A");
2.编写一个程序,在屏幕上打印出以下的图形:
*************************************
********* Java程序设计*********
*************************************
(B)原因是没有安装JDK开发环境。
(C)原因是java源文件名后缀一定是以.txt结尾。
(D)原因是JDK安装后没有正确设置环境变量PATH和Classpath。
(完整版)java练习题(含答案)

1.编写程序,用数组实现乘法小九九的存储和输出。
【提示:采用多个一维数组。
】public class Multipation {public static void main(String[] args) {// TODO Auto-generated method stubint x[][]=new int[9][9];for(int i=0;i<9;i++){for(int j=0;j<9;j++){if(i>=j){int m=i+1;int n=j+1;x[i][j]=m*n;System.out.print(m+"*"+n+"="+x[i][j]);}}System.out.println();}}}2. 定义一个类Student,属性为学号、姓名和成绩;方法为增加记录SetRecord和得到记录GetRecord。
SetRecord给出学号、姓名和成绩的赋值,GetRecord通过学号得到考生的成绩。
public class Student {/***@param args*/private int ID;private String name;private float score;public void SetRecord(int ID,String name,float score){this.ID=ID;=name;this.score=score;}public float getRecord(int ID){if(ID==this.ID)return this.score;elsereturn -1;}public static void main(String[] args) {// TODO Auto-generated method stubStudent s=new Student();s.SetRecord(0,"alex",100);float Sco=s.getRecord(0);System.out.print(Sco);}}3.给出上题中设计类的构造函数,要求初始化一条记录(学号、姓名、成绩)。
Java练习题库(含答案及解析)100题
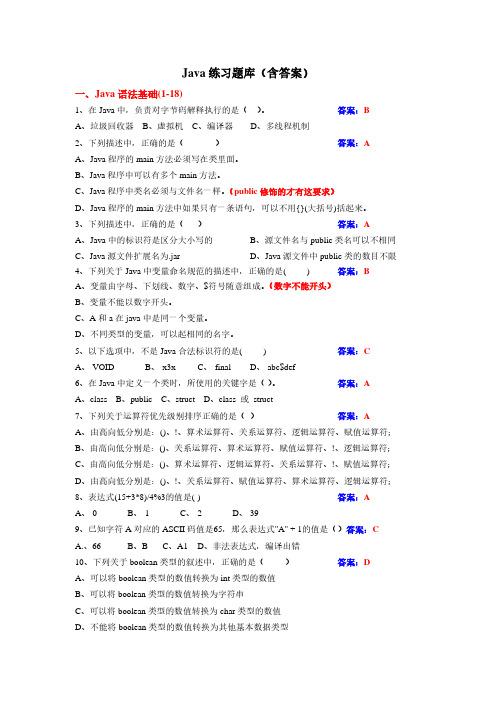
Java练习题库(含答案)一、Java语法基础(1-18)1、在Java中,负责对字节码解释执行的是()。
答案:BA、垃圾回收器B、虚拟机C、编译器D、多线程机制2、下列描述中,正确的是()答案:AA、Java程序的main方法必须写在类里面。
B、Java程序中可以有多个main方法。
C、Java程序中类名必须与文件名一样。
(public修饰的才有这要求)D、Java程序的main方法中如果只有一条语句,可以不用{}(大括号)括起来。
3、下列描述中,正确的是()答案:AA、Java中的标识符是区分大小写的B、源文件名与public类名可以不相同C、Java源文件扩展名为.jarD、Java源文件中public类的数目不限4、下列关于Java中变量命名规范的描述中,正确的是( ) 答案:BA、变量由字母、下划线、数字、$符号随意组成。
(数字不能开头)B、变量不能以数字开头。
C、A和a在java中是同一个变量。
D、不同类型的变量,可以起相同的名字。
5、以下选项中,不是Java合法标识符的是( ) 答案:CA、 VOIDB、 x3xC、 finalD、 abc$def6、在Java中定义一个类时,所使用的关键字是()。
答案:AA、classB、publicC、structD、class 或struct7、下列关于运算符优先级别排序正确的是()答案:AA、由高向低分别是:()、!、算术运算符、关系运算符、逻辑运算符、赋值运算符;B、由高向低分别是:()、关系运算符、算术运算符、赋值运算符、!、逻辑运算符;C、由高向低分别是:()、算术运算符、逻辑运算符、关系运算符、!、赋值运算符;D、由高向低分别是:()、!、关系运算符、赋值运算符、算术运算符、逻辑运算符;8、表达式(15+3*8)/4%3的值是( )答案:AA、 0B、 1C、 2D、 399、已知字符A对应的ASCII码值是65,那么表达式"A" + 1的值是()答案:CA.、66 B、B C、A1 D、非法表达式,编译出错10、下列关于boolean类型的叙述中,正确的是()答案:DA、可以将boolean类型的数值转换为int类型的数值B、可以将boolean类型的数值转换为字符串C、可以将boolean类型的数值转换为char类型的数值D、不能将boolean类型的数值转换为其他基本数据类型11、下面关于for循环的描述正确的是( ) 答案:AA、 for循环体语句中,可以包含多条语句,但要用大括号括起来。
java基础50道经典练习题及答案
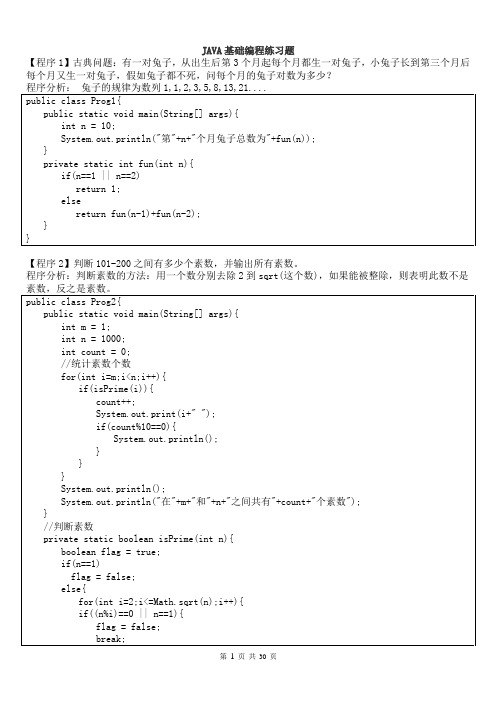
JAVA基础编程练习题【程序1】古典问题:有一对兔子,从出生后第3个月起每个月都生一对兔子,小兔子长到第三个月后每个月又生一对兔子,假如兔子都不死,问每个月的兔子对数为多少?【程序2】判断101-200之间有多少个素数,并输出所有素数。
程序分析:判断素数的方法:用一个数分别去除2到sqrt(这个数),如果能被整除,则表明此数不是【程序3】打印出所有的"水仙花数",所谓"水仙花数"是指一个三位数,其各位数字立方和等于该数本身。
例如:153是一个"水仙花数",因为153=1的三次方+5的三次方+3的三次方。
【程序4】将一个正整数分解质因数。
例如:输入90,打印出90=2*3*3*5。
程序分析:对n进行分解质因数,应先找到一个最小的质数k,然后按下述步骤完成:(1)如果这个质数恰等于n,则说明分解质因数的过程已经结束,打印出即可。
(2)如果n<>k,但n能被k整除,则应打印出k的值,并用n除以k的商,作为新的正整数n,重复执行第一步。
【程序5】利用条件运算符的嵌套来完成此题:学习成绩>=90分的同学用A表示,60-89分之间的用B 表示,60分以下的用C表示。
【程序6】输入两个正整数m和n,求其最大公约数和最小公倍数。
【程序8】求s=a+aa+aaa+aaaa+aa...a的值,其中a是一个数字。
例如2+22+222+2222+22222(此时共有5个数相加),几个数相加有键盘控制。
【程序9】一个数如果恰好等于它的因子之和,这个数就称为"完数"。
例如6=1+2+3.编程找出1000【程序10】一球从100米高度自由落下,每次落地后反跳回原高度的一半;再落下,求它在第10次【程序11】有1、2、3、4个数字,能组成多少个互不相同且无重复数字的三位数?都是多少?程序分析:可填在百位、十位、个位的数字都是1、2、3、4。
JAVA练习题及答案

name=s; age=a; step=0; } public void run(Dog fast) { fast.step++; } } public static void main (String args[]) { A a=new A(); Dog d=a.new Dog("Tom",3); d.step=25; d.run(d); System.out.println(d.step); } } 四、 编程题
4) while ( I > 0 ) { 5) j = I * 2; 6) System.out.println (" The value of j is " + j ); 7) k = k + 1; 8) I--; 9) } 10) } A line 4 B line 6 C line 7 D line 8 二、多项选择
D sleep() E.yield() F.synchronized(this) 7.构造 BufferedInputStream 的合适参数是哪个? CA A BufferedInputStream B BufferedOutputStream C FileInputStream D FileOuterStream E. File 8.下列说法正确的是 DBC A ng.Clonable 是类 B ng.Runnable 是接口 C Double 对象在 ng 包中 D Double a=1.0 是正确的 java 语句 9.指出正确的表达式 AB A double a=1.0; B Double a=new Double(1.0); C byte a = 340; D Byte a = 120; 10.定义一个类名为"MyClass.java"的类,并且该类可被一个工程中的所有类访问,那么该类的正确声明应为: CD A private class MyClass extends Object B class MyClass extends Object C public class MyClass D public class MyClass extends Object 11.指出下列哪个方法与方法 public void add(int a){}为合理的重载方法。 CD A public int add(int a) B public void add(long a) C public void add(int a,int b) D public void add(float a) 12.如果下列的方法能够正常运行,在控制台上将显示什么? ACD public void example(){
java考试题及答案
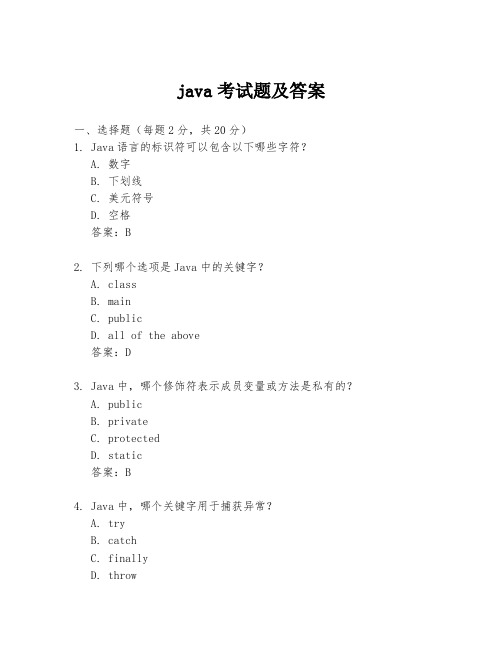
java考试题及答案一、选择题(每题2分,共20分)1. Java语言的标识符可以包含以下哪些字符?A. 数字B. 下划线C. 美元符号D. 空格答案:B2. 下列哪个选项是Java中的关键字?A. classB. mainC. publicD. all of the above答案:D3. Java中,哪个修饰符表示成员变量或方法是私有的?A. publicB. privateC. protectedD. static答案:B4. Java中,哪个关键字用于捕获异常?A. tryB. catchC. finallyD. throw答案:B5. 在Java中,下列哪个数据类型是基本数据类型?A. StringB. intC. ArrayListD. HashMap答案:B6. 下列哪个选项不是Java集合框架的一部分?A. ListB. MapC. SetD. String答案:D7. Java中,哪个关键字用于定义接口?A. classB. interfaceC. abstractD. final答案:B8. 在Java中,下列哪个选项不是线程安全的?A. VectorB. ArrayListC. LinkedListD. HashMap答案:B9. Java中,下列哪个选项是正确的继承关系?A. Object is a subclass of StringB. String is a subclass of ObjectC. Object is a superclass of StringD. String is a superclass of Object答案:B10. 下列哪个选项不是Java中的访问修饰符?A. publicB. privateC. protectedD. global答案:D二、填空题(每题2分,共20分)1. Java中,用于定义类的关键字是______。
答案:class2. Java中,用于定义接口的关键字是______。
java练习题
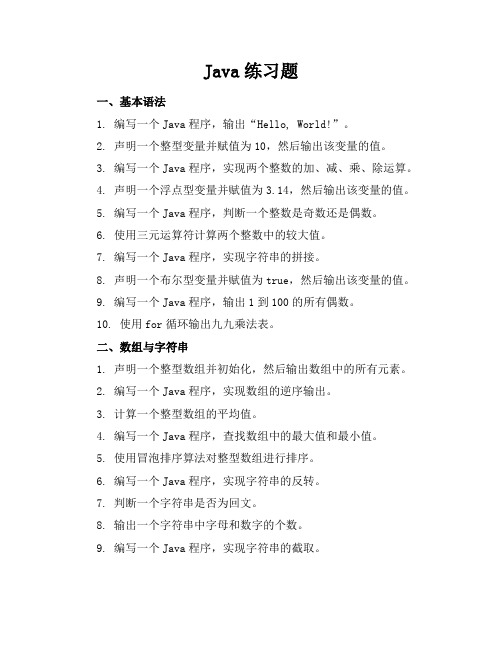
Java练习题一、基本语法1. 编写一个Java程序,输出“Hello, World!”。
2. 声明一个整型变量并赋值为10,然后输出该变量的值。
3. 编写一个Java程序,实现两个整数的加、减、乘、除运算。
4. 声明一个浮点型变量并赋值为3.14,然后输出该变量的值。
5. 编写一个Java程序,判断一个整数是奇数还是偶数。
6. 使用三元运算符计算两个整数中的较大值。
7. 编写一个Java程序,实现字符串的拼接。
8. 声明一个布尔型变量并赋值为true,然后输出该变量的值。
9. 编写一个Java程序,输出1到100的所有偶数。
10. 使用for循环输出九九乘法表。
二、数组与字符串1. 声明一个整型数组并初始化,然后输出数组中的所有元素。
2. 编写一个Java程序,实现数组的逆序输出。
3. 计算一个整型数组的平均值。
4. 编写一个Java程序,查找数组中的最大值和最小值。
5. 使用冒泡排序算法对整型数组进行排序。
6. 编写一个Java程序,实现字符串的反转。
7. 判断一个字符串是否为回文。
8. 输出一个字符串中字母和数字的个数。
9. 编写一个Java程序,实现字符串的截取。
10. 使用StringBuilder类拼接一个由100个“Java”组成的字符串。
三、面向对象1. 定义一个学生类(Student),包含姓名、年龄和成绩属性,并实现一个打印学生信息的方法。
2. 编写一个Java程序,创建一个学生对象并设置其属性,然后调用打印学生信息的方法。
3. 定义一个矩形类(Rectangle),包含长和宽属性,并实现计算面积和周长的方法。
4. 编写一个Java程序,创建两个矩形对象,并比较它们的面积大小。
5. 定义一个动物类(Animal),包含吃、睡和叫的方法,然后创建一个狗类(Dog)继承动物类,并重写叫的方法。
6. 编写一个Java程序,演示多态性,创建一个动物数组,包含狗和猫对象,并调用它们的叫方法。
JAVA习题集(含答案)
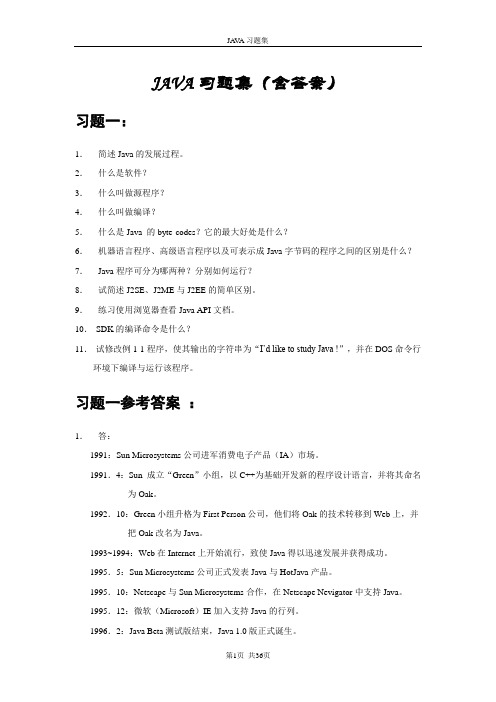
JAVA习题集(含答案)习题一:1.简述Java的发展过程。
2.什么是软件?3.什么叫做源程序?4.什么叫做编译?5.什么是Java 的byte-codes?它的最大好处是什么?6.机器语言程序、高级语言程序以及可表示成Java字节码的程序之间的区别是什么?7.Java程序可分为哪两种?分别如何运行?8.试简述J2SE、J2ME与J2EE的简单区别。
9.练习使用浏览器查看Java API文档。
10.SDK的编译命令是什么?11.试修改例1-1程序,使其输出的字符串为“I’d like to study Java !”,并在DOS命令行环境下编译与运行该程序。
习题一参考答案:1.答:1991:Sun Microsystems公司进军消费电子产品(IA)市场。
1991.4:Sun 成立“Green”小组,以C++为基础开发新的程序设计语言,并将其命名为Oak。
1992.10:Green小组升格为First Person公司,他们将Oak的技术转移到Web上,并把Oak改名为Java。
1993~1994:Web在Internet上开始流行,致使Java得以迅速发展并获得成功。
1995.5:Sun Microsystems公司正式发表Java与HotJava产品。
1995.10:Netscape与Sun Microsystems合作,在Netscape Nevigator中支持Java。
1995.12:微软(Microsoft)IE加入支持Java的行列。
1996.2:Java Beta测试版结束,Java 1.0版正式诞生。
1997.2:Java发展至1.1版。
Java的第一个开发包JDK(Java Development Kit)发布。
1999.7:Java升级至1.2版。
2000.9:Java升级至1.3版。
2001.7:Java升级至1.4版。
2.答:软件可以理解为程序的另一种名称。
3.答:直接使用高级语言书写的程序代码称为源程序4.答:把用高级语言编写的源程序翻译成可执行(目标)程序的过程称为编译。
java考试试题及答案

java考试试题及答案一、选择题(每题2分,共20分)1. 下列哪个是Java的基本数据类型?A. StringB. IntegerC. intD. Object答案:C2. Java中的main方法是程序的入口点,它属于哪个类?A. ng.ObjectB. ng.SystemC. ng.RuntimeD. java.applet.Applet答案:A3. 下列哪个关键字用于定义类?A. classB. publicC. staticD. void答案:A4. Java中的哪个关键字用于实现接口?A. implementsB. extendsC. classD. interface答案:A5. 下列哪个是Java集合框架中的接口?A. ArrayListB. ListC. LinkedListD. HashMap答案:B6. 下列哪个不是Java的控制流语句?A. ifB. forC. switchD. while答案:C7. Java中的哪个类提供了日期和时间的处理功能?A. java.util.DateB. java.util.CalendarC. java.time.LocalDateD. java.time.LocalDateTime答案:B8. 下列哪个是Java的异常处理关键字?A. tryB. catchC. finallyD. all of the above答案:D9. 下列哪个不是Java的访问修饰符?A. publicB. privateC. protectedD. global答案:D10. 下列哪个不是Java的注释方式?A. // 单行注释B. /* 多行注释 */C. / 文档注释 */D. # 预处理指令答案:D二、填空题(每空2分,共20分)1. Java语言是________面向对象的编程语言。
答案:完全2. Java程序的执行流程是:编写源代码,编译成字节码,通过________加载并执行。
java编程练习题及答案
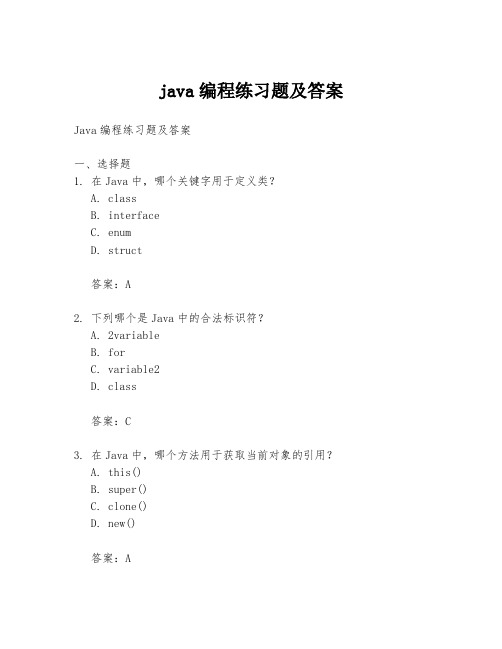
java编程练习题及答案Java编程练习题及答案一、选择题1. 在Java中,哪个关键字用于定义类?A. classB. interfaceC. enumD. struct答案:A2. 下列哪个是Java中的合法标识符?A. 2variableB. forC. variable2D. class答案:C3. 在Java中,哪个方法用于获取当前对象的引用?A. this()B. super()C. clone()D. new()答案:A4. 以下哪个是Java的访问修饰符?A. publicB. staticC. finalD. abstract答案:A5. 在Java中,哪个关键字用于定义接口?A. classB. interfaceC. abstractD. enum答案:B二、简答题1. 请简述Java中的继承是如何工作的?答案:Java中的继承允许一个类(子类)继承另一个类(父类)的属性和方法。
子类可以扩展或修改父类的行为,实现代码复用。
继承是面向对象编程的核心概念之一。
2. 请解释Java中接口和抽象类的区别?答案:接口定义了一组方法规范,但不提供实现。
任何实现接口的类都必须提供接口中所有方法的具体实现。
抽象类可以包含抽象方法和具体方法,并且可以有成员变量。
抽象类可以作为其他类的基类,但不能被实例化。
三、编程题1. 编写一个Java程序,实现一个简单的计算器,可以进行加、减、乘、除运算。
```javaimport java.util.Scanner;public class SimpleCalculator {public static void main(String[] args) {Scanner scanner = new Scanner(System.in);System.out.print("请输入第一个数字: ");double num1 = scanner.nextDouble();System.out.print("请选择运算符(+, -, *, /): "); char operator = scanner.next().charAt(0);System.out.print("请输入第二个数字: ");double num2 = scanner.nextDouble();double result = 0;switch (operator) {case '+':result = num1 + num2;break;case '-':result = num1 - num2;break;case '*':result = num1 * num2;break;case '/':if (num2 != 0) {result = num1 / num2;} else {System.out.println("除数不能为0"); }break;default:System.out.println("无效的运算符");}if (result != 0) {System.out.println("结果是: " + result);}scanner.close();}}```2. 编写一个Java程序,实现一个简单的学生管理系统,可以添加学生信息、显示所有学生信息。
java练习题+答案

1、在下列说法中,选出最正确的一项是( )。
1.Java语言是以类为程序的基本单位的2.Java语言是不区分大小写的3.多行注释语句必须以//开始4.在Java语言中,类的源文件名和该类名可以不相同2、下列选项中不属于Java虚拟机的执行特点的一项是( )。
1.异常处理2.多线程3.动态链接4.简单易学3、下列选项中属于Java语言的垃圾回收机制的一项是( )。
1.语法检查2.堆栈溢出检查3.跨平台4.内存跟踪4、下列选项中属于 Java语言的安全性的一项是( )。
1.动态链接2.高性能3.访问权限4.内存跟踪5、下列选项中,属丁JVM执行过程中的特点的一项是( )。
1.编译执行2.多进程3.异常处理4.静态链接6、在Java语言中,那一个是最基本的元素?( )1.方法2.包3.对象4.接口7、如果有2个类A和B,A类基于 B类,则下列描述中正确的一个是( )。
1.这2个类都是子类或者超类2.A是B超类的子类3.B是A超类的子类4.这2个类郡是对方的子类8、使用如下哪个保留字可以使只有在定义该类的包中的其他类才能访问该类?( )1.abstract2.private3.protected4.不使用保留字9、编译一个定义了3个类和10个办法的Java源文件后,会产生多少个字符码文件,扩展名是什么?( )1.13个字节码文件,扩展名是.class2.1个字节码文件,扩展名是.class3.3个字节码文件,扩展名是.java4.3个字节码文件,扩展名是.class10、下列属于Java语言的特点的一项是( )。
1.运算符重载2.类间多重继承3.指针操作4.垃圾回收11、在创建Applet应用程序时,需要用户考虑的问题是( )。
1.窗口如何创建2.绘制的图形在窗口中的位置3.程序的框架4.事件处理12、于Java语言的内存回收机制,下列选项中最正确的一项是( )。
1.Java程序要求用户必须手工创建一个线程来释放内存2.Java程序允许用户使用指针来释放内存3.内存回收线程负责释放无用内存4.内存回收线程不能释放内存对象13、下列关于Java程序结构的描述中,不正确的一项是( )。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
1 When you compile a java program you should use command:
A java *.java
B javab *.java
C javac *.java
D javad *.java
2 Which of the following lines will compile without error.
A float f=1.3; double
B char c="a"; string
C boolean b=null;
D double d=1.3;
3 Which statement will compile wrong:
A if(3>5)System.out.println("hello");
B if (3)System.out.println("hello");
C if(true)System.out.println("hello");
D if(false)System.out.println("hello");
4 Consider the following code:
Integer s = new Integer(9);
Integer t = new Integer(9);
Long u = new Long(9);
Which test would return true?
A (s.equals(new Integer(9))
B (s.equals(9))
C (s==u)
D (s==t)
5 All of the following are wrapper classes except:
A String
B Integer
C Character
D Double
6 When you use the assignment operator with variables of a class type, you are assigning a:
A value
B primitive type
C local variable
D reference
7 A class design requires that a particular member variable must be accesible for direct access by any subclasses of this class, otherwise not by classes which are not members of the same package. What should be done to achieve this?
A. The variable should be marked public
B. The variable should be marked private
C. The variable should be marked protected
D. The variable should have no special access modifier
8 Given:
class A {
A() { }
}
class B extends A {
}
Which statement is true?
A. Class B's constructor is public.
B. Class B's constructor has arguments.
C. Class B's constructor includes a call to this().
D. Class B's constructor includes a call to super().
9 class super {
public int getLength() {return 4;}
}
public class Sub extends Super {
public long getLength() {return 5;}
public static void main (String[]args) {
super sooper = new Super ();
Sub sub = new Sub();
System.out.printIn(sooper.getLength()+ "," + sub.getLength() };
}
What is the output?
A.4, 4
B.4, 5
C.5, 4
D.the code will not compile
10 in java which one is not a Layout Management class:
A. BorderLayout
B. FlowLayout
C. CardLayout
D. LayoutManage
11 which one can construct a BufferedOutputStream object:
A. new BufferedOutputStream();
B. new BufferedOutputStream("o.txt");
C. new BufferedOutputStream(new Writer("o.txt"));
D. new BufferedOutputStream(new FileOutputStream("o.txt"));
12 Which one is valid declaration within an interface definition?
A. public void methoda();
B. protected void methoda(double d1);
C. public final double methoda();
D. private void methoda(double d1);
13 Given:
1. public class Foo {
2. public static void main(String[] args) {
3. try {
4. return;
5. } finally {
6. System.out.println( "Finally" );
7. }
8. }
9. }
What is the result?
A. Finally
B. Compilation fails.
C. The code runs with no output.
D. An exception is thrown at runtime.
14 Given:
public class A {
public int add(int a, int b){
return a+b
}
}
Which one overload the add method?
A. double add (int a, int b){ return a+b; }
B. public double add(double ax, double by) { return ax+by; }
C. public double add(int ax, int by) { return ax+by; }
D. public int add(int ax, int by){ return ax+by;}
15 Given:
1. public interface Foo {
2. int k = 4;
3. }
Which one is equivalent to line 2?
A.final int k = 4;
B abstract int;
C.private int k = 4;
D.protected int k=4;
16 Given:
1. class BaseClass {
2. Private float x = 1.0f ;
3. protected float getVar ( ) ( return x;)
4. }
5. class Subclass extends BaseClass (
6. private float x = 2.0f;
7. //insert code here
8. )
Which one is valid examples of method overriding?
A float getVar ( ) { return x;}
B public float getVar ( ) { return x;}
C double getVar ( ) { return x;}
D public float getVar (float f ) { return f;}。