学生信息管理系统C++代码
c语言学生管理系统代码

C语言学生管理系统代码
简介
学生管理系统是一个用于管理学生信息的简单程序,它可以实现添加学生信息、显示学生信息、修改学生信息和删除学生信息等功能。
通过这个系统,学校或机构可以更方便地管理和维护学生的相关数据。
功能
1.添加学生信息:通过输入学生的姓名、学号、年龄和性别等信息,将
学生信息存储在系统中。
每个学生信息包括学号、姓名、年龄和性别等关键信息。
2.显示学生信息:可以显示系统中所有学生的信息,包括学号、姓名、
年龄和性别。
3.修改学生信息:通过输入学生的学号,可以修改该学生的姓名、年龄
和性别等信息。
4.删除学生信息:通过输入学生的学号,可以删除该学生的信息。
实现
下面是一个简单的C语言学生管理系统代码示例:
```c #include <stdio.h> #include <string.h>
#define MAX_STUDENTS 100
// 定义学生结构体 struct Student { int id; char name[100]; int age; char
gender[10]; };
// 定义全局变量,用于存储学生信息和统计学生数量 struct Student
students[MAX_STUDENTS]; int num_students = 0;
// 添加学生 void addStudent() { if (num_students >= MAX_STUDENTS) { printf(。
学生管理系统c语言源代码

int main()
{
initLinkTable(&head);//初始化表头
readStu();//读入源文件
while (1)
{
menu();
system("cls");
}
}
void initLinkTable(studentLinkPoint *p)
int sum(studentLinkPoint);//求和
void avg(studentLinkPoint);//求平均分
void disAvgSum(studentLinkPoint);//显示总分和平均分
char *inputNumber();//专门用来输入一个学生的学号,返回该字符串的指针
char name[20];
char number[18];
int i;
if(temp==0)
{
error("input");
return 0;
}
else
{
temp->student=(studentPint)malloc(sizeof(studentNod));
break;
case 0:
del(head,end);
break;
}
if(c==0)
exit(1);
}
void del(studentLinkPoint p,studentLinkPoint End)
{
studentLinkPoint q;
if(end==head)
printf(" %3.1f %3.1f\n",p->student->sum,p->student->avg);
学生信息管理系统C语言源代码
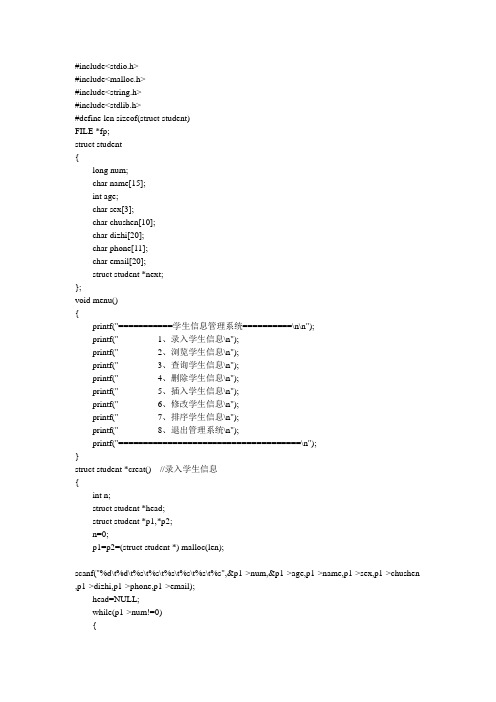
#include<stdio.h>#include<malloc.h>#include<string.h>#include<stdlib.h>#define len sizeof(struct student)FILE *fp;struct student{long num;char name[15];int age;char sex[3];char chushen[10];char dizhi[20];char phone[11];char email[20];struct student *next;};void menu(){printf("===========学生信息管理系统==========\n\n");printf(" 1、录入学生信息\n");printf(" 2、浏览学生信息\n");printf(" 3、查询学生信息\n");printf(" 4、删除学生信息\n");printf(" 5、插入学生信息\n");printf(" 6、修改学生信息\n");printf(" 7、排序学生信息\n");printf(" 8、退出管理系统\n");printf("=====================================\n");}struct student *creat() //录入学生信息{int n;struct student *head;struct student *p1,*p2;n=0;p1=p2=(struct student *) malloc(len);scanf("%d\t%d\t%s\t%s\t%s\t%s\t%s\t%s",&p1->num,&p1->age,p1->name,p1->sex,p1->chushen ,p1->dizhi,p1->phone,p1->email);head=NULL;while(p1->num!=0){n=n+1;if(n==1) head=p1;else p2->next=p1;p2=p1;p1=(struct student *)malloc(len);scanf("%d\t%d\t%s\t%s\t%s\t%s\t%s\t%s",&p1->num,&p1->age,p1->name,p1->sex,p1->chu shen,p1->dizhi,p1->phone,p1->email);}p2->next=NULL;return(head);}void insert(struct student *head) //插入学生信息{int search_num;struct student *p,*q,*s;p=head;printf("在哪个学生前插入请输入学号:\n");scanf("%d",&search_num);while((p!=NULL)&&(p->num!=search_num)){q=p;p=p->next;}s=(struct student *)malloc(len);q->next=s;system("cls");printf("请输入学生信息:\n");printf("学号\t年龄\t姓名\t性别\t出生\t地址\t电话\te-mail\n");scanf("%d\t%d\t%s\t%s\t%s\t%s\t%s\t%s",&s->num,&s->age,s->name,s->sex,s->chushen,s->diz hi,s->phone,s->email);s->next=p;}void printList(struct student *head) //浏览全部学生信息{struct student *p;p=head;if(head==NULL)printf("没有学生信息!!\n");else{do{fread(p,len,1,fp);printf("%d\t%d\t%s\t%s\t%s\t%s\t%s\t%s\n",p->num,p->age,p->name,p->sex,p->chushen,p->diz hi,p->phone,p->email);p=p->next;}while(p!=NULL);}}void findList_num(struct student *head,long search_num) //按学号查找{struct student *p;p=head;while((p!=NULL)&&(p->num!=search_num))p=p->next;if(p!=NULL)printf("%d\t%d\t%s\t%s\t%s\t%s\t%s\t%s\n",p->num,p->age,p->name,p->sex,p->chushen,p->diz hi,p->phone,p->email);elseprintf("没有该学生信息!!\n");}void findList_name(struct student *head,char *search_name) //按姓名查找{struct student *p;int cmp1=0,cmp=0;p=head;while(p!=NULL)if(strcmp(p->name,search_name)!=0){p=p->next;cmp++;}else{printf("%d\t%d\t%s\t%s\t%s\t%s\t%s\t%s\n",p->num,p->age,p->name,p->sex,p->chushen,p->dizhi,p->phone,p->email);p=p->next;cmp1=1;}if(cmp!=0&&cmp1==0)printf("没有该学生信息!!\n");}void xiugai(struct student *p1,long xiu_num) //修改学生信息{struct student *p2;p2=p1;while((p2!=NULL)&&(p2->num!=xiu_num))p2=p2->next;if(p2!=NULL){scanf("%d\t%d\t%s\t%s\t%s\t%s\t%s\t%s",&p2->num,&p2->age,p2->name,p2->sex,p2->chu shen,p2->dizhi,p2->phone,p2->email);}elseprintf("没有该学生信息!!\n");}struct student *delList(struct student *head,long del_num) // 删除学生信息{struct student *p,*q;p=head;q=head;while(p &&(p->num != del_num)){q=p;p=p->next;}if(p==NULL)printf("无此学号!\n");else{if(p == head){head = p->next;free(p);}else{q->next = p->next;free(p);}}return head;}void paixu(struct student *head) //按学号排序{struct student *p,*f,*t;char ch[100];int i;t=f=p=head;for(p=head;p->next!=NULL;p=p->next){for(t=head,f=t->next;t->next!=NULL;t=t->next,f=f->next){if(t->num>f->num>0){i=t->num;t->num=f->num;f->num=i;i=t->age;t->age=f->age;f->age=i;strcpy(ch,t->name);strcpy(t->name,f->name);strcpy(f->name,ch);strcpy(ch,t->sex);strcpy(t->sex,f->sex);strcpy(f->sex,ch);strcpy(ch,t->chushen);strcpy(t->chushen,f->chushen);strcpy(f->chushen,ch);strcpy(ch,t->dizhi);strcpy(t->dizhi,f->dizhi);strcpy(f->dizhi,ch);strcpy(ch,t->phone);strcpy(t->phone,f->phone);strcpy(f->phone,ch);strcpy(ch,t->email);strcpy(t->email,f->email);strcpy(f->email,ch);}}}// return head;}void save(struct student *head) //保存为磁盘文件{struct student *p;if((fp=fopen("keshe","w"))==NULL){printf("cannot open this file\n");exit(0);}p=head;while(p!=NULL){fprintf(fp,"%d\n",p->num);fprintf(fp,"%d\n",p->age);fprintf(fp,"%s\n",p->name);fprintf(fp,"%s\n",p->sex);fprintf(fp,"%s\n",p->chushen);fprintf(fp,"%s\n",p->dizhi);fprintf(fp,"%s\n",p->phone);fprintf(fp,"%s\n",p->email);p=p->next;}fclose(fp);}struct student *read() //从磁盘读取文件{struct student *head=NULL;struct student *p=NULL;struct student *t=NULL;int a;// fp=fopen("keshe","r");if((fp=fopen("keshe","r"))==NULL){printf("cannot open this file\n");exit(0);}while(1){t=(struct student *)malloc(len);a=fscanf(fp,"%d\t%d\t%s\t%s\t%s\t%s\t%s\t%s",&t->num,&t->age,t->name,t->sex,t->chush en,t->dizhi,t->phone,t->email);if(a==0||a==-1)return head;t->next=NULL;if(p==NULL){p=t;head=t;}else{p->next=t;p=p->next;p->next=NULL;}}fclose(fp);}void main(){int code=0;struct student *pt = NULL;while(code!=8){menu();printf("请输入上述序号进行操作:\n");scanf("%d",&code);system("cls");switch(code){case 1:{system("cls");printf("每个学生的信息之间用Tab键分隔\n");printf("===========================录入学生信息==============================\n");printf("---------------------------------------------------------------------\n");printf("学号\t年龄\t姓名\t性别\t出生\t地址\t电话\te-mail\n");pt=creat();save(pt);system("cls");printf("===========================录入学生信息==============================\n");printf("---------------------------------------------------------------------\n");printf("************录入学生信息成功***********!!\n");printf("按回车键返回主菜单\n");getchar();getchar();system("cls");};break;case 2:{system("cls");printf("===========================学生信息表================================\n");printf("---------------------------------------------------------------------\n");printf("学号\t年龄\t姓名\t性别\t出生\t地址\t电话\te-mail\n");pt=read();printList(pt);printf("================================================================ =====\n");printf("---------------------------------------------------------------------\n");printf("\n按回车键返回主菜单\n");getchar();getchar();system("cls");};break;case 3:{int search=0;system("cls");printf("===========================查询学生信息==============================\n");printf("---------------------------------------------------------------------\n");while(search!=3){printf("1、按学号查询\n2、按姓名查询\n3、退出查询\n");scanf("%d",&search);switch(search){case 1:{long search_num;system("cls");printf("请输入学生学号\n");scanf("%d",&search_num);system("cls");printf("===========================查询结果==================================\n");printf("---------------------------------------------------------------------\n");printf("学号\t年龄\t姓名\t性别\t出生\t地址\t电话\te-mail\n");findList_num(read(),search_num);printf("================================================================ =====\n");printf("---------------------------------------------------------------------\n");printf("\n按回车键返回查询菜单\n");getchar();getchar();system("cls");};break;case 2:{char search_name[15];system("cls");printf("请输入学生姓名\n");scanf("%s",search_name);system("cls");printf("===========================查询结果==================================\n");printf("---------------------------------------------------------------------\n");printf("学号\t年龄\t姓名\t性别\t出生\t地址\t电话\te-mail\n");findList_name(read(),search_name);printf("================================================================ =====\n");printf("---------------------------------------------------------------------\n");printf("\n按回车键返回查询菜单\n");getchar();getchar();system("cls");};}}system("cls");};break;case 4:{long del_num;system("cls");printf("===========================删除学生信息==============================\n");printf("---------------------------------------------------------------------\n");printf("请输入要删除学生信息的学号:\n");scanf("%d",&del_num);system("cls");pt=delList(read(),del_num);save(pt);printf("===========================删除结果================================\n");printf("-------------------------------------------------------------------\n");printf("学号为%d的学生信息成功删除\n",del_num);printf("\n按回车键返回主菜单\n");getchar();getchar();system("cls");};break;case 5:{system("cls");printf("每个学生的信息之间用Tab键分隔\n");printf("===========================插入学生信息==============================\n");printf("---------------------------------------------------------------------\n");insert(pt);save(pt);system("cls");printf("===========================插入学生信息==============================\n");printf("---------------------------------------------------------------------\n");printf("****插入学生信息成功***!!\n\n");printf("按回车键返回主菜单\n");getchar();getchar();system("cls");}break;case 6:{long search_num;system("cls");printf("请输入要修改的学生学号:\n");scanf("%d",&search_num);system("cls");printf("每个学生的信息之间用Tab键分隔\n");printf("===========================修改学生信息==================================\n");printf("-------------------------------------------------------------------------\n");printf("学号\t年龄\t姓名\t性别\t出生\t地址\t电话\te-mail\n");findList_num(read(),search_num);printf("\n");printf("请输入修改信息:\n");printf("学号\t年龄\t姓名\t性别\t出生\t地址\t电话\te-mail\n");pt=read();xiugai(pt,search_num);save(pt);printf("================================================================ =====\n");printf("---------------------------------------------------------------------\n");printf("****修改学生信息成功***!!\n\n");printf("\n按回车键返回查询菜单\n");getchar();getchar();system("cls");};break;case 7:{system("cls");printf(" 按学号从小到大排序\n\n");printf("===========================学生信息表================================\n");printf("---------------------------------------------------------------------\n");printf("学号\t年龄\t姓名\t性别\t出生\t地址\t电话\te-mail\n");pt=read();paixu(pt);printList(pt);save(pt);printf("================================================================ =====\n");printf("---------------------------------------------------------------------\n");printf("\n按回车键返回主菜单\n");getchar();getchar();system("cls");};break;case 8:read();break;}}}。
学生管理系统c语言简单版
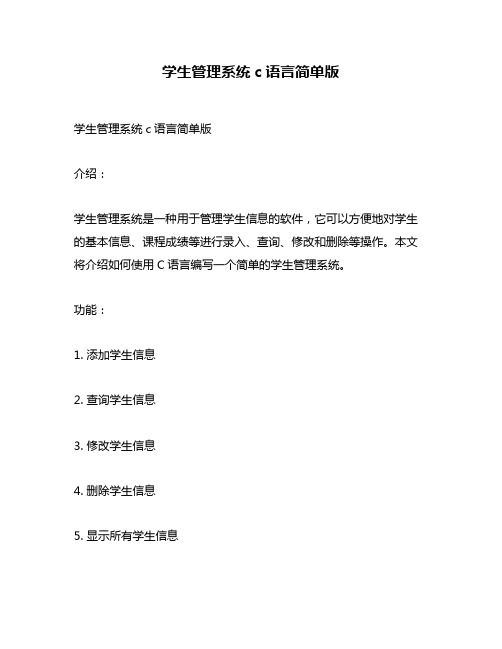
学生管理系统c语言简单版学生管理系统c语言简单版介绍:学生管理系统是一种用于管理学生信息的软件,它可以方便地对学生的基本信息、课程成绩等进行录入、查询、修改和删除等操作。
本文将介绍如何使用C语言编写一个简单的学生管理系统。
功能:1. 添加学生信息2. 查询学生信息3. 修改学生信息4. 删除学生信息5. 显示所有学生信息实现方法:1. 添加学生信息添加学生信息需要输入以下内容:姓名、性别、年龄、班级和电话号码。
我们可以定义一个结构体来存储这些信息,代码如下:```struct Student {char name[20];char sex[10];int age;char class[20];char phone[20];};```然后定义一个数组来存储多个学生的信息:```struct Student students[100];int count = 0; // 学生数量```接下来,我们可以编写一个函数来添加新的学生信息:```void addStudent() {struct Student student;printf("请输入姓名:");scanf("%s", );printf("请输入性别:");scanf("%s", student.sex);printf("请输入年龄:");scanf("%d", &student.age);printf("请输入班级:");scanf("%s", student.class);printf("请输入电话号码:");scanf("%s", student.phone);students[count++] = student; // 将新的学生信息存储到数组中 printf("添加成功!\n");}```2. 查询学生信息查询学生信息可以按照姓名或电话号码进行查询。
学生信息管理系统源代码

#include<stdio.h>#include <stdlib.h>#include <string.h>typedef struct{long class_1; //班级long number; //学号char name[20]; //姓名float math; //数学float c_program; //C语言float physics; //大学物理float english; //大学英语float polity; //政治float sport; //体育float summary; //总分float average; //平均分}Student;Student stud[100]; //定义结构体数组变量的大小int i=0; //i用于记录输入的学生的个数int menu() //菜单函数{int a;printf("***********************学生信息管理系统*************************\n");//菜单选择printf("\t\t【1】输入学生信息\n");printf("\t\t【2】显示所有学生的信息\n");printf("\t\t【3】按平均分升降排序\n");printf("\t\t【4】根据学生的学号查找学生的信息\n");printf("\t\t【5】插入学生的信息\n");printf("\t\t【6】删除学生的信息\n");printf("\t\t【7】修改学生的信息\n");printf("\t\t【8】从文件中读入数据\n");printf("\t\t【9】将所有记录写入文件\n");printf("\t\t【0】退出本系统\n");printf("***********************学生信息管理系统*************************\n");printf("请选择你要的操作【0-9】:");scanf("%d",&a); //读入一个数while(a<0 || a>9){printf("输入错误!请重新输入。
C语言 学生信息查询系统
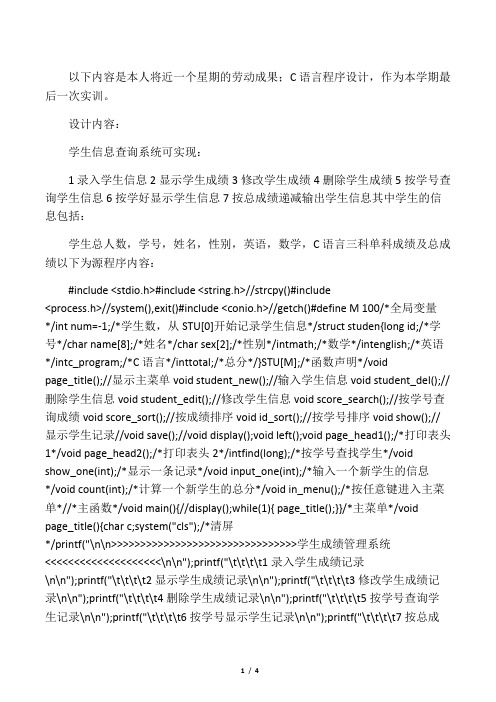
以下内容是本人将近一个星期的劳动成果;C语言程序设计,作为本学期最后一次实训。
设计内容:学生信息查询系统可实现:1录入学生信息2显示学生成绩3修改学生成绩4删除学生成绩5按学号查询学生信息6按学好显示学生信息7按总成绩递减输出学生信息其中学生的信息包括:学生总人数,学号,姓名,性别,英语,数学,C语言三科单科成绩及总成绩以下为源程序内容:#include <stdio.h>#include <string.h>//strcpy()#include<process.h>//system(),exit()#include <conio.h>//getch()#define M 100/*全局变量*/int num=-1;/*学生数,从STU[0]开始记录学生信息*/struct studen{long id;/*学号*/char name[8];/*姓名*/char sex[2];/*性别*/intmath;/*数学*/intenglish;/*英语*/intc_program;/*C语言*/inttotal;/*总分*/}STU[M];/*函数声明*/voidpage_title();//显示主菜单void student_new();//输入学生信息void student_del();//删除学生信息void student_edit();//修改学生信息void score_search();//按学号查询成绩void score_sort();//按成绩排序void id_sort();//按学号排序void show();//显示学生记录//void save();//void display();void left();void page_head1();/*打印表头1*/void page_head2();/*打印表头2*/intfind(long);/*按学号查找学生*/voidshow_one(int);/*显示一条记录*/void input_one(int);/*输入一个新学生的信息*/void count(int);/*计算一个新学生的总分*/void in_menu();/*按任意键进入主菜单*//*主函数*/void main(){//display();while(1){ page_title();}}/*主菜单*/void page_title(){char c;system("cls");/*清屏*/printf("\n\n>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>学生成绩管理系统<<<<<<<<<<<<<<<<<<<<\n\n");printf("\t\t\t\t1录入学生成绩记录\n\n");printf("\t\t\t\t2显示学生成绩记录\n\n");printf("\t\t\t\t3修改学生成绩记录\n\n");printf("\t\t\t\t4删除学生成绩记录\n\n");printf("\t\t\t\t5按学号查询学生记录\n\n");printf("\t\t\t\t6按学号显示学生记录\n\n");printf("\t\t\t\t7按总成绩递减输出\n\n");printf("\t\t\t\t8保存\n\n");printf("\t\t\t\t0退出\n\n");printf("********************************************************** ****************\n");printf("请用数字键0-8选择操作:\n");/*填充程序,完成主菜单显示*/c=getchar();system("cls");switch(c){case'1':student_new();break;case'2':show();break;case'3':student_edit();break;case'4':student_del();break;case'5':score_search();break;case'6':id_sort();break;case'7':score_sort();break;//case'8':save();break;case'0':left();}}/*录入学生基本信息*/void student_new(){int i,n;printf("请输入学生的个数(1-%d)",M);scanf("%d",&n);while(!(n>0 && n<=M)){printf("\n输入的学生人数太多,请重新输入学生人数:");scanf ("%d",&n);}page_head1();while(n--){num++;input_one(num);count(num);}}/*删除学生基本信息*/voidstudent_del(){long id;char c;int n;while(1){printf("请输入你要删除学生的学号:");scanf("%ld",&id);printf("\n");n=find(id);if(n!=-1)break;elsesystem("cls");}page_head2();show_one(n);printf("\n请确认删除吗?请输入Y或者N");c=getch();if('Y'==c || 'y'==c){STU[n]=STU[num];num--;}}/*按学号查找学生*/int find(long id){int n;for(n=0;n<=num;n++)if(STU[n].id==id) return n;return -1;}/*输入一个新学生的信息*/void input_one(intn){scanf("%ld%s%s%d%d%d",&STU[n].id,STU[n].name,STU[n].sex,&STU[n].math,&STU[n].english,&STU[n].c_program);printf("---------------------------------------------------------------------\n");}/*显示所有记录*/void show(){inti,j;page_head2();for(i=0;i<num+1;i++){//if(-1==n)//j=num-i;//else//j=i;show_one(i);}in_menu();}/*显示一条记录*/void show_one(intn){if(strlen(STU[n].name)>=10){printf("%ld\t\t%s\t\t%s\t\t",STU[n].id,STU[n].name,S TU[n].sex);}else{printf("%ld\t\t%s\t\t%s\t",STU[n].id,STU[n].name,STU[n].sex);}printf ("%3d\t%3d\t%3d\t%3d\t\n",STU[n].math,STU[n].english,STU[n].c_program,STU[n].t otal);printf("----------------------------------------------------------------------\n");}/*计算一个新学生的总分*/void count(intn){STU[n].total=STU[n].english+STU[n].math+STU[n].c_program;}/*退出*/voidleft(){exit(0);}/*修改信息*/void student_edit(){long id;int n;while(1){printf("请输入你要修改学生的学号:");scanf("%ld",&id);printf("\n");n=find(id);if(n!=-1)break;elsesystem("cls");}page_head2();show_one(n);printf("\n请输入新的信息:\n");page_head1();scanf("%ld%s%s%d%d%d",&STU[n].id,STU[n].name,STU[n].se x,&STU[n].math,&STU[n].english,&STU[n].c_program);count(n);}/*进入主菜单*/void in_menu(){printf("\n请按任意键进入主菜单");getch();}/*按学号查询成绩*/void score_search(){long id;int n;printf("请输入你要修改学生的学号:");scanf("%ld",&id);printf("\n");n=find(id);if(n!=-1){page_head2();show_one(n);}elseprintf("不存在该学号学生信息!!");in_menu();}/*按学号排序显示*/void id_sort(){int i=0;int n;longmin=STU[0].id;for(i=1;i<=num;i++){if(STU[i].id<min)min=STU[i].id;}page_head2();for(i=0;i<=num;){n=find(min++);if(n!=-1){i++;show_one(n);}}in_menu();}/*按总成绩冒泡降序显示*/voidscore_sort(){struct student t;int i,j;system("cls");for(i=0;i<num;i++){for(j=0;j<num-i;j++){if(STU[j].total<STU[j+1].total){t=STU[j];STU[j]=STU[j+1];STU[j+1]=t;}}}show();}/*打印表头2*/voidpage_head2(){printf("**************************************************** ******************\n");printf("学号\t\t姓名\t\t性别\t数学\t英语\tC语言\t总成绩\n");printf("**********************************************************************\n");}/*打印表头1*/voidpage_head1(){printf("**************************************************** **********\n");printf("学号\t\t姓名\t\t性别\t数学\t英语\tC语言\n");printf("************************************************************ **\n");}。
学生信息管理系统c语言版源代码

学生信息管理系统c语言版源代码#include <stdio.h> #include <string.h> #include <stdlib.h> #include <conio.h> #define N 1000typedef struct student {int number;char name[20];int grade;int gaoshu;int yingyu;int jisuanji;int sum;}STUDENT;STUDENT student[N]; int shuliang=0;void menu();void fhzjm(){char biaozhi[20];printf("\n");printf("还需要操作么,如果需要操作请输入:yes,否则请输入:no\n");scanf("%s",biaozhi);if(strcmp(biaozhi,"yes")==0){menu();}else if(strcmp(biaozhi,"no")==0)exit(0);else{printf("请输入正确的字符,谢谢~\n"); fhzjm();}}void DengJi(){int rs;int i,k=1;system("CLS");printf("请输入需要输入几个学生信息:"); scanf("%d",&rs);for(i=shuliang;i<shuliang+rs;i++,k++) {printf("请输入第%d个学生的学号:",k); scanf("%d",&student[i].number);printf("请输入学生的姓名:");scanf("%s",student[i].name);printf("请输入学生3门课的成绩:"); printf("请输入第1门课的成绩:");scanf("%d",&student[i].gaoshu);printf("请输入第2门课的成绩:");scanf("%d",&student[i].yingyu);printf("请输入第3门课的成绩:");scanf("%d",&student[i].jisuanji);}shuliang=shuliang+rs;fhzjm();}void ShanChu(){char shanchuinfo[10];system("CLS");printf("删除全部学生信息请输入\"all\",删除指定学号的学生信息请输入\"one\"\n");scanf("%s",shanchuinfo);if(strcmp(shanchuinfo,"all")==0){int j;printf("你删除的学生信息如下:\n");printf("-----------学号-------------姓名-------------高数--------------英语--------------计算机\t\n");for(j=0;j<shuliang;j++)printf("----%d-------%s-------%d-------%d-------%d\t\n",student[j].number,student[j].name,student[j].gaoshu,student[j].jisuanji);shuliang=0;printf("删除成功\n\n");}else if(strcmp(shanchuinfo,"one")==0){struct student *p=NULL;int choice;int i,j,k=0;printf("请输入你要删除的人的学号:");scanf("%d",&choice);for(i=0;i<shuliang;i++){if(choice==student[i].number){k=1;j=i;break;}}if(k){if(shuliang==1){p=&student[0];free(p);shuliang=0;}else{for(i=j;i<shuliang;i++) {student[i]=student[i+1];}shuliang=shuliang-1;}printf("删除成功\n\n");}else{printf("输入数据错误~\n"); }}fhzjm();}void LiuLan(){int i;system("CLS");if(shuliang==0){printf("系统里面没有任何学生的信息~\n");}else{for(i=0;i<shuliang;i++){printf("第%d个学生的学号为:%d\n",i+1,student[i].number);printf("第%d个学生的姓名为:%s\n",i+1,student[i].name);printf("第%d个学生的第一门课的成绩为:%d\n",i+1,student[i].gaoshu);printf("第%d个学生的第二门课的成绩为:%d\n",i+1,student[i].yingyu);printf("第%d个学生的第三门课的成绩为:%d\n",i+1,student[i].jisuanji);student[i].sum=student[i].gaoshu+student[i].yingyu+student[i].jisuan ji;printf("第%d个学生的总成绩为:%d\n",i+1,student[i].sum);}}fhzjm();}void ChaZhao(){int xx;char choice,yy[20];int i,j,k=0;system("CLS");if(shuliang==0){printf("系统里面没有任何学生的信息~\n");fhzjm();}printf("三种查找方式:学号,姓名,成绩\n");printf("如果按学号查找请输1,如果按姓名查找请输2,如果按成绩查找请输3\n");printf("请输入您查找的方式:");scanf("%s",&choice);if(choice=='1'){printf("请输入需要查找学生的学号:");scanf("%d",&xx);printf("您所查找的学生的信息为:\n");printf("----学号----姓名----高数成绩----英语成绩----计算机成绩----\t\n");for(i=0;i<shuliang;i++){if(xx==student[i].number){j=i;k=1;printf("----%d-------%s-------%d-------%d-------%d----\t\n",student[j].number,student[j].name,student[j].gaoshu,student[j].yingyu,student[i].jis uanji);}}if(k==0)printf("输入信息有误:\n");}else if(choice=='2'){printf("请输入需要查找学生的姓名:\n");scanf("%s",yy);printf("您所查找的学生的信息为:\n");printf("----学号----姓名----高数成绩----英语成绩----计算机成绩----\t\n");for(i=0;i<shuliang;i++){if(strcmp(yy,student[i].name)==0){j=i;k=1;printf("----%d-------%s-------%d-------%d-------%d----\t\n",student[j].number,student[j].name,student[j].gaoshu,student[j].yingyu,student[j].jis uanji);}}if(k==0)printf("输入信息有误:\n");}else if(choice=='3'){printf("请输入需要查找学生的成绩:\n");scanf("%d",&xx);printf("您所查找的学生的信息为:\n");printf("----学号----姓名----高数----英语----计算机----\t\n");for(i=0;i<shuliang;i++){if(xx==student[i].grade){j=i;k=1;printf("----%d-------%s-------%d-------%d-------%d----\t\n",student[j].number,student[j].name,student[j].gaoshu,student[j].yingyu,student[i].jis uanji);}}if(k==0)printf("输入信息有误:\n");}fhzjm();}void PaiXu(){struct student *p1[N],**p2,*temp;int i,j;system("CLS");p2=p1;for( i=0;i<shuliang;i++){p1[i]=student+i;}for( i=0;i<shuliang;i++){for( j=i+1;j<shuliang;j++){if((*(p2+i))->sum<(*(p2+j))->sum){temp=*(p2+i);*(p2+i)=*(p2+j);*(p2+j)=temp;} }}printf("按照总成绩排序之后的信息为:\n");printf("----学号----姓名----总成绩----\t\n");for( i=0;i<shuliang;i++){student[i].sum=student[i].gaoshu+student[i].yingyu+student[i].jisuan ji;printf("----%d-----%s----%d-----\n",(*(p2+i))->number,(*(p2+i))->name,(*(p2+i))->sum);}fhzjm();}void CunChu(){int i;FILE *rs;if((rs=fopen("student.txt","w"))==NULL){printf("not open");exit(0);}for(i=0;i<shuliang;i++){fwrite(&student[i], sizeof(student[i]), 1, rs); }if(ferror(rs)){fclose(rs);perror("写文件失败~\n");return;}printf("存储文件成功~\n");fclose(rs);fhzjm();}void DaoChu(){struct student t;int i=0;FILE* fp = fopen("student.txt", "r");shuliang=0;if(NULL==fp){perror("读取文件打开失败~\n");return;}memset(student,0x0,sizeof(student));while(1){fread(&t,sizeof(t),1,fp);if(ferror(fp)){fclose(fp);perror("读文件过程失败~\n");return;}if(feof(fp)){break;}student[i]=t;i++;}fclose(fp);shuliang=i; printf("导出文件成功~\n"); fhzjm();}void menu(){int n=0;system("CLS");printf(" 学生信息管理系统\n");printf(" 作者:陈椿\n");printf("-------------------MENU-----------------\n"); printf(" 1.登记学生信息\n");printf(" 2.删除学生信息\n");printf(" 3.浏览所有已经登记的学生\n");printf(" 4.查找\n");printf(" 4.1按学号查找\n");printf(" 4.2按姓名查找\n");printf(" 4.3按成绩查找\n");printf(" 5.根据总成绩排序\n");printf(" 6.存储到文件\n");printf(" 7.从文件导出\n");printf(" 8.退出系统\n");a: printf(" 请选择:");scanf("%d",&n);switch (n){case 1:DengJi();break;case 2:ShanChu();break;case 3:LiuLan();break;case 4:ChaZhao();break;case 5:PaiXu();break;case 6:CunChu();break;case 7:DaoChu();break;case 8:exit(0);break;default:{printf("请输入1-8之间的数字,谢谢~\n"); goto a;}}}main() {menu();}。
学生管理系统c语言源代码

学生管理系统c语言源代码学生管理系统c语言源代码#include stdio.h#include dos.h#include string.h#include stdlib.h#include malloc.h#define SIZE 8struct student{char name;char num;int score;float ave;struct student *next;}stu[SIZE],temp,s;void shuru(){int i,j,sum,length,flag=1,a;FILE *fp;while(flag==1){printf(“Define a rangeclass number:");scanf("%d",printf("Input the total number of the class(a):"); scanf("%d",length);if(lengtha)flag=0;}for(i=0;ilength;i++){printf("\n请输入学生的信息:");printf("\n输入姓名:");scanf("%s",stu[i].name);printf("\n输入序号.:");scanf("%s",stu[i].num);printf("\n输入成绩:\n");sum=0;for(j=0;jj++){printf("score %d:",j+1);scanf("%d",stu[i].score[j]);sum+=stu[i].score[j];}stu[i].ave=sum/3.0;}学生管理系统c语言源代码fp=fopen("stu1.txt","w");for(i=0;ilength;i++)if(fwrite(stu[i],sizeof(struct student),1,fp)!=1)printf("File write error\n");fclose(fp);fp=fopen("stu1.txt","r");printf("\name\ NO. score1 score2 score3 sum ave\n");for(i=0;ilength;i++){fread(stu[i],sizeof(struct student),1,fp);printf("%3s%5s%7d%7d%7d%7d%10.2f\n",stu[i].name,stu[i].num,stu[i ].score,stu[i].score,stu[i].score,sum=stu[i].score+stu[i].score+stu[i].score,stu[i].ave);}}void chaxun(){ FILE *fp, *fp1;char n,name;int i,j,k,t,m,flag=1;if((fp=fopen("stu1.txt","r"))==NULL){printf("Can not open the file.");exit(0);}printf("\noriginal data:\n");k=i;printf("\nPlease select the menu(1.number ):"); scanf("%d",switch(m){case 1:printf("\nchaxun number:");scanf("%s",n);for(flag=1,i=0;ii++){if(strcmp(n,stu[i].num)==0){j=i;flag=0;break;}}break;case 2:printf("\nchaxun name:");scanf("%s",name);for(flag=1,i=0;ii++){if(strcmp(name,stu[i].name)==0){j=i;flag=0;break;学生管理系统c语言源代码}}}if(!flag){printf("\nYou can find:\n");fp1=fopen("stu2.txt","w");printf(" name NO. score1 score2 score3ave\n");fwrite(stu[j],sizeof(struct student),1,fp1);printf("%-15s%11s%7d%7d%7d%10.2f",stu[j].name,stu[j].num,stu[j].score,stu[j].score,stu[j].score,stu[j].ave);}else printf("\nNot found!");fclose(fp);fclose(fp1);}xiugai(){ int a;printf("\nplease select the menu(1.CHARU 2.__ ):");scanf("%d",switch(a){case 1:Insert(); break;case 2:Delete(); break;}}Insert(){ FILE *fp;int i,j,t,n;printf("\nNO.:");scanf("%s",s.num);printf("name:");scanf("%s",);printf("score1,score2,score3:");scanf("%d,%d,%d",s.score,s.score,s.score);s.ave=(s.score+s.score+s.score)/3.0;if((fp=fopen("stu1.txt","r"))==NULL){printf("Can not open the file.");exit(0);}printf("\noriginal data:\n");for(i=0;fread(stu[i],sizeof(struct student),1,fp)!=0;i++) {printf("\n%-15s%11s",stu[i].name,stu[i].num);for(j=0;jj++)学生管理系统c语言源代码printf("%7d",stu[i].score[j]);printf("%10.2f",stu[i].ave);}fclose(fp);n=i;for(t=0;stu[t].aves.avett++);printf("\nnow:\n");fp=fopen("stu1.txt","w");for(i=0;ii++){fwrite(stu[i],sizeof(struct student),1,fp);printf("\n%-15s%11s",stu[i].name,stu[i].num);for(j=0;jj++)printf("%7d",stu[i].score[j]);printf("%10.2f",stu[i].ave);}fwrite(s,sizeof(struct student),1,fp);printf("\n%-15s%11s%7d%7d%7d%10.2f",,s.num,s.score,s.score, s.score,s.ave);for(i=t;ii++){fwrite(stu[i],sizeof(struct student),1,fp);printf("\n%-15s%11s",stu[i].name,stu[i].num);for(j=0;jj++)printf("%7d",stu[i].score[j]);printf("%10.2f",stu[i].ave);}fclose(fp);}Delete(){ FILE *fp;int i,j,t,n,flag;char number;if((fp=fopen("stu1.txt","rb"))==NULL){printf("Can not open the file.");exit(0);}printf("\noriginal data:");for(i=0;fread(stu[i],sizeof(struct student),1,fp)!=0;i++) {printf("\n%-15s%11s",stu[i].name,stu[i].num);for(j=0;jj++)printf("%7d",stu[i].score[j]);printf("%10.2f",stu[i].ave);}fclose(fp);n=i;学生管理系统c语言源代码printf("\nInput number deleted:");scanf("%s",number);for(flag=1,i=0;flagii++){if(strcmp(number,stu[i].num)==0){for(t=i;tt++){strcpy(stu[t].num,stu[t+1].num);strcpy(stu[t].name,stu[t+1].name);for(j=0;jj++)stu[t].score[j]=stu[t+1].score[j];stu[t].ave=stu[t+1].ave;}n=n-1;elseprintf("\n Not found!");printf("\nNow,the content of file:\n");fp=fopen("stu1.txt","wb");for(i=0;ii++)fwrite(stu[i],sizeof(struct student),1,fp);fclose(fp);fp=fopen("stu1.txt","r");for(i=0;fread(stu[i],sizeof(struct student),1,fp)!=0;i++)printf("%-15s%11s%7d%7d%7d%10.2f\n",stu[i].name,stu[i].num,stu[i].score, stu[i].score,stu[i].score,stu[i].ave);fclose(fp);}paixu(){FILE *fp;int i,j,n;if((fp=fopen("stu1.txt","r"))==NULL){printf("Can not open the file.");exit(0);}printf("\nfile'stu1.txt':");for(i=0;fread(stu[i],sizeof(struct student),1,fp)!=0;i++) {printf("\n%-15s%11s",stu[i].name,stu[i].num);for(j=0;jj++)printf("%7d",stu[i].score[j]);printf("%10.2f",stu[i].ave);}fclose(fp);n=i;for(i=0;ii++)for(j=i+1;jj++)学生管理系统c语言源代码if(stu[i].avestu[j].ave){temp=stu[i];stu[i]=stu[j];stu[j]=temp;}printf("\nnow:");fp=fopen("stu1.txt","w");for(i=0;ii++){fwrite(stu[i],sizeof(struct student),1,fp);printf("\n%-15s%11s",stu[i].name,stu[i].num);tongji(){ FILE *fp;int i,j,k,labe1,b;int a5=0;int a6=0;int a7=0;int a8=0;int a9=0; int a10=0; float t;if((fp=fopen("stu1.txt","r"))==NULL){printf("Can not open the file.");exit(0);}printf("\nfile'stu1.txt':");for(i=0;fread(stu[i],sizeof(struct student),1,fp)!=0;i++){printf("\n%-15s%11s",stu[i].name,stu[i].num); for(j=0;jj++)printf("%7d",stu[i].score[j]);printf("%10.2f",stu[i].ave);}fclose(fp);k=i;for(i=0;ii++){labe1=0;if(stu[i].ave60){labe1++;t=labe1/(float)k*100;}}printf("\nbujigelv:");printf("%f%",t);printf("\n");for(j=0;jj++){a5=0;a6=0;a7=0;a8=0;a9=0;a10=0;k=i;printf("kemu is %d:\n",j);for(i=0;ii++)学生管理系统c语言源代码{b=stu[i].score[j]/10;if(b6)a5++;elseif(b=6b7)a6++;elseif(b=7b8)a7++;elseif(b=8b9)a8++;if(b=9b10)a9++;elseif(b==10)a10++;}printf(" 不及格is %d\n",a5);printf(" 60--69 is %d\n",a6);printf(" 70--79 is %d\n",a7);printf(" 80--89 is %d\n",a8);printf(" 90--99 is %d\n",a9);printf(" 100 is %d\n",a10);}}main(){int a;printf(" ____\n"); printf(" 欢迎进入学生成绩管理系统\n");printf(" ____\n"); while(1){printf("\n选择菜单:\n");printf("\n");printf(" 1.输入 2.查询 3.排序 4.修改 5.统计 6.退出\n"); scanf("%d",switch(a){case 1: shuru();break;case 2: chaxun(); break;case 3: paixu(); break;case 4: xiugai(); break;学生管理系统c语言源代码case 5: tongji();break; case 6: exit(0); }。
学生管理系统 C语言代码

#include"stdio.h"#include"stdlib.h"#include"string.h"struct stu_info1{char num[13];//学号char name[10];//姓名char sex[5];//性别char cls[20];//班级}stu1[6];struct stu_info2{char counum[6];//课程号char counam[20];//课程名称int credit;//学分}stu2[6];struct stu_info3{char num[13];//学号char counum[6];//课程号float results;//分数}stu3[12];struct stu_info4{char num[13];//学号char counum[6];//课程号float results;//分数}stu4[12];int n=11;void main(){void gengxin();void input1();void input2();void input3();void output();void xianshi();void chaxun();void printf1();void printf2();input1();input2();output();int i;loop: ;printf("*************欢迎使用分数查询系统*************\n");printf("** 请选择**\n");printf("** 1.录入2.删除无用信息(管理员功能) **\n");printf("** 3.显示4.查询(学生功能) **\n");printf("** 5.显示学生信息6.显示课程信息**\n");printf("** 7.退出**\n");printf("**********************************************\n");scanf("%d",&i);switch(i){case 1: input3();break;case 2:gengxin();break;case 3:xianshi();goto loop;case 4: chaxun();goto loop;case 5: printf1();goto loop;case 6: printf2();goto loop;case 7:break;default:printf("error");break;}}void input1()//录入结构体stu1[]{int i;FILE *fp;if((fp=fopen("A.txt","r"))==NULL){printf("can not open file\n");exit(0);}/* printf(" 学号姓名性别班级\n");*/for(i=0;i<=5;i++){fscanf(fp,"%s%s%s%s",&stu1[i].num,&stu1[i].name,&stu1[i].sex,&stu1[i].cls);/*printf("%-13s %-10s %-5s %-20s\n",stu1[i].num,stu1[i].name,stu1[i].sex,stu1[i] .cls);*/}fclose(fp);}void input2()//录入结构体stu2[]{int i;FILE *fp;if((fp=fopen("B.txt","r"))==NULL){printf("can not open file\n");exit(0);}for(i=0;i<=5;i++){fscanf(fp,"%s%s%d",&stu2[i].counum,&stu2[i].counam,&stu2[i].credit);}fclose(fp);}void input3()//录入成绩{FILE *fp;fp=fopen("C.txt","w");int a,i,j,k;float cetss;char number[13],cnum[6],mima[10];printf("请输入管理员密码\n");scanf("%s",mima);if(strcmp(mima,"abc111")==0){printf("请输入要录入学生成绩的个数\n");scanf("%d",&a);for(i=1;i<=a;i++){printf("请输入要录入的第%d同学的学号:",i);scanf("%s",number);printf("请输入要录入的第%d同学的课程号:",i);scanf("%s",cnum);for(j=0;j<=5;j++)//学号{if((strcmp(number,stu1[j].num)==0))break;}if(j<=5){for(k=0;k<=5;k++)//课程号{if(strcmp(cnum,stu2[k].counum)==0){printf("请输入要录入同学的成绩:");scanf("%f",&cetss);fprintf(fp,"%s %s %f\n",number,cnum,cetss);break;}}}if(j>5||k>5){printf("Error,please input again");i=i-1;}printf("录入成功\n");}}else{printf("密码错误\n");}}void output()// 录入结构体stu3[]{int i;FILE *fp=fopen("C.txt","r");for(i=0;i<n;i++){fscanf(fp,"%s%s%f",&stu3[i].num,&stu3[i].counum,&stu3[i].results);/*printf("%s%s%f\n",stu3[i].num,stu3[i].counum,stu3[i].results);*/ }fclose(fp);}void xianshi()// 显示成绩{int i,j;for(i=0;i<n;i++){for(j=0;j<6;j++){if((strcmp(stu3[i].num,stu1[j].num))==0)printf("%s\t",stu1[j].name);}for(j=0;j<6;j++){if(strcmp(stu3[i].counum,stu2[j].counum)==0)printf("%s\t",stu2[j].counam);}printf("%3.1f\n",stu3[i].results);}}void chaxun()//查询功能{char number[13],c;int i,j,k,a,b,d;while((c=getchar())!='Q'){a=0,b=0;//a记录学分b记录学科printf("请输入要查询同学的学号\n");scanf("%s",number);for(i=0;i<n;i++){if(strcmp(number,stu3[i].num)==0){printf("学号:%s\t",stu3[i].num);for(j=0;j<6;j++){if(strcmp(stu3[i].num,stu1[j].num)==0){printf("姓名:%s\n",stu1[j].name);}}break;}}d=i;for(i=0;i<n;i++)if(strcmp(number,stu3[i].num)==0){b=b+1;for(j=0;j<6;j++){if(strcmp(stu3[i].num,stu1[j].num)==0){for(k=0;k<6;k++){if(strcmp(stu3[i].counum,stu2[k].counum)==0)break;}break;}}if(stu3[i].results>=60){a=a+stu2[k].credit;printf("课程号:%s\t课程名称:%s\t成绩:%3.1f\t实得学分:%d\n",stu3[i].counum,stu2[k].counam,stu3[i].results,stu2[k].credit);}elseprintf("课程号:%s\t课程名称:%s\t成绩:%3.1f\t实得学分:%d\n",stu3[i].counum,stu2[k].counam,stu3[i].results,0);}if(d<n){printf("共修%d科\t\t实得总学分:%d\n",b,a);}else{printf("学号输入错误\n");}getchar();printf("退出请按Q+回车,继续查询请按回车键");}}void gengxin()//更新信息,删除C.txt中无用信息{FILE *fp;int i,j,k;char mima[10];printf("请输入管理员密码\n");scanf("%s",mima);if(strcmp(mima,"abc111")==0){fp=fopen("C.txt","w");for(i=0;i<n;i++){for(j=0;j<6;j++)if(strcmp(stu3[i].num,stu1[j].num)==0){for(k=0;k<=5;k++)if(strcmp(stu3[i].counum,stu2[k].counum)==0)break;if(k<=5){fprintf(fp,"%s %s %f\n",stu3[i].num,stu3[i].counum,stu3[i].results);}}}printf("更新C.txt成功\n");fclose(fp);}else{printf("密码错误\n");}}void printf1()//显示学生信息{int i;printf(" 学号姓名性别班级\n");for(i=0;i<=5;i++){printf("%-13s%-10s%-5s %-20s\n\n",stu1[i].num,stu1[i].name,stu1[i].sex,stu1[i].cls);}}void printf2()//显示课程信息{int i;printf(" 课程编号课程名称学分\n");for(i=0;i<=5;i++){printf("%-6s%-20s %-4d\n\n",stu2[i].counum,stu2[i].counam,stu2[i].credit);}}。
学生信息管理系统c语言代码
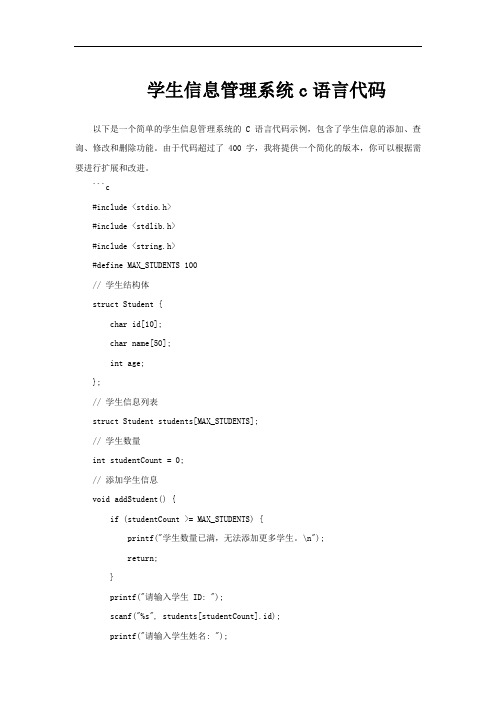
学生信息管理系统c语言代码以下是一个简单的学生信息管理系统的 C 语言代码示例,包含了学生信息的添加、查询、修改和删除功能。
由于代码超过了 400 字,我将提供一个简化的版本,你可以根据需要进行扩展和改进。
```c#include <stdio.h>#include <stdlib.h>#include <string.h>#define MAX_STUDENTS 100// 学生结构体struct Student {char id[10];char name[50];int age;};// 学生信息列表struct Student students[MAX_STUDENTS];// 学生数量int studentCount = 0;// 添加学生信息void addStudent() {if (studentCount >= MAX_STUDENTS) {printf("学生数量已满,无法添加更多学生。
\n");return;}printf("请输入学生 ID: ");scanf("%s", students[studentCount].id);printf("请输入学生姓名: ");scanf("%s", students[studentCount].name);printf("请输入学生年龄: ");scanf("%d", &students[studentCount].age);studentCount++;printf("学生信息添加成功。
\n");}// 查询学生信息void queryStudent() {if (studentCount == 0) {printf("没有学生信息可供查询。
学生信息管理系统(vc)
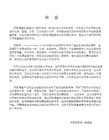
void SaveToFile();/* 保存到文件 */
void LoadFile();/* 从文件中读入记录 */
void CopyFile(); /* 复制文件 */
void InsertToFile();/* 追加记录到文件中 */
OutList();
break;
/* 查找记录 */
case 5:
SearchPrintNode();
SWITCH[6] = 0;
printf("追加完毕!\n");
break;
/* 索引 */
case 13:
{
switch (n)
{
/* 执行初始化 */
case 1:
head = Init();
void InputList(); /*读取多个学生信息*/
void OutputNodeInfo(Student*);/*显示学生信息*/
void OutList();/*显示多个学生信息*/
Student* SearchFrontNode(Student*);/*查找前一结点*/
void SearchPrintNode();/* 按姓名查找记录并打印 */
if (SWITCH[8])
{
head = Sort(CmpID, 0);
system("cls");
void Compute();/* 计算总分和均分 */
int CmpID(Student*, Student*, int);/*比较两个id*/
C语言 学生信息管理系统(完整版)
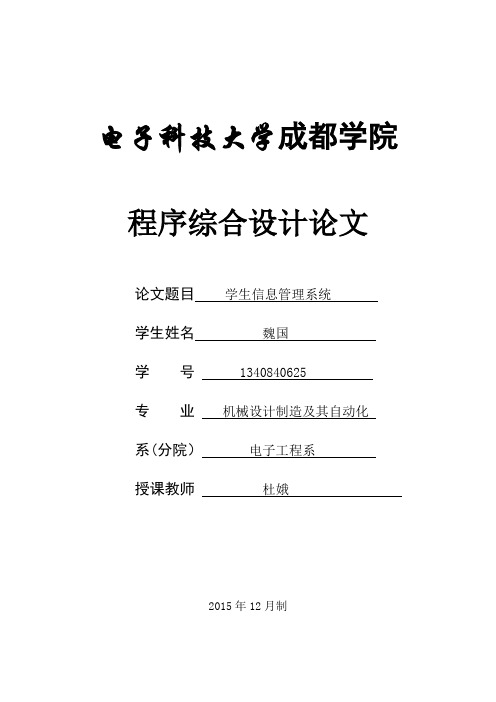
电子科技大学成都学院程序综合设计论文论文题目学生信息管理系统学生姓名魏国学号1340840625专业机械设计制造及其自动化系(分院)电子工程系授课教师杜娥2015年12月制摘要随着经济的发展,社会的进步,计算机越来越深入到我们日常的工作学习及生活中,成为我们日常生活不可或缺的辅助工具。
随着科学技术的不断提高,计算机科学日渐成熟,其强大的功能已成为人们深刻认识,它已为人们深刻认识,它已进入人类社会的各个领域并发挥着越来越重要的作用。
现在由于学校规模进一步扩大,学生人数逐渐上升,在学校的学生信息管理中,虽然已经存在许多学生信息管理系统,但由于学校之间的管理差异很信息的不同,各个学校的学生信息管理的要求不一致,这样我们需要根据具体学习的具体要求来开发学生信息管理系统以方便学生管理。
本系统主要对学生各种信息进行处理。
本系统采用C语言编写,设计从实用性出发,设计开发出一个操作简单且符合实际需要的学生信息管理系统。
本文设计出一个可以添加、修改、查询、删除、统计的学生信息管理系统;最后,通过测试分析,力求将学到的只是在学生信息管理系统的得到全面运用,并使系统在实际的操作中能按照设计的要求安全有效的正确运行。
学生信息管理系统是为了实现学校对学生信息管理的系统化、规范化和自动化,从而提高学校管理效率而设计的。
它完全取代了原来一直用人工管理的工作方式,避免了由于管理人员的工作疏忽以及管理质量问题所造成的各种错误,为及时、准确、高效的完成学生信息管理提供了强有力的工具和管理手段。
学生信息管理系统是一个中小型数据库管理系统,它界面美观、操作简单、安全性高,基本满足了学生信息管理的要求。
学生信息管理系统在运行阶段,效果好,数据准确性高,提高了工作效率,同时也实现了学生信息管理计算机化。
关键字:学生信息,管理系统,数据库,C语言编写第一章系统功能和组成模块1.1系统功能学生信息管理系统存放了每个学生的学号,姓名,性别,年龄,出生年月,家庭住址,政治面貌等信息的数据库。
C语言学生管理系统代码

struct scores *snext;
}stscores;
//宏定义学生个人信息
#define stmes (stmessage *)malloc(sizeof(stmessage)) //为学生个人信息结构体开辟动态内存空间
fflush(stdin);
s--;
}
switch(a)
{
case '1': mainmessage(); continue;//学生基本信息
case '2': mainresult();continue;//学生的成绩信息
case '3': exit(1);//退出系统
void xiugairesult();//修改学生成绩信息
void delatemessag1();//删除学生个人基本信息
void delatemessag2();//删除班级学生基本信息
void delatemessag3();//删除系基本信息
void delatemessag4();//删除全校基本信息
void ziresult4();//学生成绩信息修改菜单
void addmessage();//添加学生基本信息
void judgefile();//判断文件是否存在
void addresult();//添加学生成绩信息
void searchresult();//查询学生成绩信息
void readresult();//加载学生成绩信息到程序
//全局变量
stmessage *head=NULL;
stscores *shead=NULL;
学生管理系统源代码

#include<iostream>#include<stdlib.h>#include<string.h>#include<malloc.h>#define INIT_SIZE 10#define INCRE_SIZE 10#define SUBJECT_NUM 3#define LEN 3void show_Start();void show_Table();void addRecord();void Info_delete();void deleteRecord();void delete_Num(int);void delete_Name(char tarName[]);void Info_modify();void modifyRecord();void modify_Num(int);void modify_Name(char[]);void Info_query();void queryRecord();void query_Num(int);void query_Name(char[]);void display();void quit();void menu_CMD();char *subject[SUBJECT_NUM] =struct STUDENT{int num;char name[20];char sex;float score[SUBJECT_NUM];};//struct STUDENT stu[LEN + 1];//STUDENT *record = (STUDENT*)malloc(sizeof(STUDENT)*INIT_SIZE);int static stuNum = 0;//STUDENT *record = (STUDENT*)malloc(sizeof(STUDENT)*INIT_SIZE);;int main(){//record = (STUDENT*)malloc(sizeof(STUDENT)*INIT_SIZE);//STUDENT *record = (STUDENT*)malloc(sizeof(STUDENT)*INIT_SIZE);/*record[1].num = 1001;strcpy(record[1].name,"Jason");record[1].sex = 'M';record[1].score[0] = 85.0;record[1].score[1] = 90.0;record[1].score[2] = 95.0;record[2].num = 1002;strcpy(record[2].name,"Jerry");record[2].sex = 'M';record[2].score[0] = 85.0;record[2].score[1] = 90.0;record[2].score[2] = 95.0;record[3].num = 1003;strcpy(record[3].name,"Jessie");record[3].sex = 'F';record[3].score[0] = 85.0;record[3].score[1] = 90.0;record[3].score[2] = 95.0;*//*Info_modify();int key;cout<cin>>key;if(key == 1){int targetNum;coutcin>>targetNum;modify_Num(targetNum);cout<<endl;display();}if(key == 2){char targetName[20];cout<cin>>targetName;modify_Name(targetName);cout<<endl;display();}if(key == 3){exit(0);}*/show_Start();menu_CMD();return 0;}void show_Start(){//cout<<endl;cout<<" **************************************** "<<endl;cout<<" "<<endl;cout<<" "<<endl;cout<<" "<<endl;cout<<" "<<endl;cout<<" Made by Jason "<<endl; cout<<" **************************************** "<<endl;}//.void show_Table(){cout<<" <<"\t"<<" "<<"\t"<<"cout<<"\t"<<subject[0]<<"\t"<<subject[1]<<"\t"<<subject[2];cout<<endl;}void menu_CMD(){int key;while(1){cout<<"1. <<endl; cout<<"2. <<endl; cout<<"3. <<endl; cout<<"4. <<endl; cout<<"5. <<endl; cout<<"6. <<endl; cout<<"cin>>key;while(1){if((key < 1)||(key > 6)){int key;cout<< "<<endl; cout<< (1 - 5) : "; cin>>key;}else{break;}}switch(key){case 1:addRecord();break;case 2:deleteRecord();break;case 3:modifyRecord();break;case 4:queryRecord();break;case 5:display();break;case 6:quit();break;}}}/////////////////////////////////////////////////////////////////////////////// /////////////////////////////////////////////////////////////////////////////////void addRecord(){if(stuNum == 0){cout<< <<endl;stuNum++;}else{cout<<" <<endl;stuNum++;}//if(stuNum > INIT_SIZE){cout<< "<<endl;record = (STUDENT*)realloc(record,(INIT_SIZE + INCRE_SIZE)*sizeof(STUDENT));cout<<" !"<<endl;}cout<<" <<endl;cout<<" (Y/N) : ";char choi;cin>>choi;if((choi == 'Y')||(choi == 'y')){cout<<" : ";cin>>record[stuNum].num;cout<<" : ";cin>>record[stuNum].name;cout<<"(M , F) : ";cin>>record[stuNum].sex;int i;for(i = 0;i < SUBJECT_NUM;i++){cout<<" "<<subject[i]<< : ";cin>>record[stuNum].score[i];}}if((choi == 'N')||(choi == 'n')){cout<<" <<endl;cout<<endl;}cout<< <<stuNum<<" !"<<endl; cout<<endl;}////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// \//deleteRecordvoid Info_delete(){cout<<" : "<<endl;cout<<"1. "<<endl;cout<<"2. <<endl;cout<<"3. "<<endl; }//void deleteRecord(){int key;cout<<endl;Info_delete();cout<<" : "; cin>>key;if(key == 1){int targetNum;cout<<" : ";cin>>targetNum;//delete_Num(targetNum);cout<<endl;}if(key == 2){char targetName[20];cout<<" : "; cin>>targetName;delete_Name(targetName); cout<<endl;}if(key == 3){while(1){menu_CMD();}}}//void delete_Num(int tarNum){int i;for(i = 1;i <= stuNum;i++){if(record[i].num == tarNum){////1.//2.if(i = stuNum){cout<<" : "<<endl;show_Table();cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex<<"\t"<<record[i].score[0]<<record[i].score[1]<<"\t"<<record[i].score[2];cout<<endl;cout<<endl<<" : "<<endl;show_Table();for(int i = 1;i <= stuNum - 1;i++){cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex;for(int j = 0;j < SUBJECT_NUM;j++){cout<<"\t"<<record[i].score[j];}cout<<endl;}stuNum--;cout<<" <<stuNum<<";cout<<endl;*/}//2.if(i != stuNum){cout<<" : "<<endl;show_Table();cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex<<"\t"<<record[i].score[0]<<"\t"<<record[i].score[1]<<"\t"<<record[i].score[2];for(int j = i+1;j <= stuNum;j++){record[j-1] = record[j];}//cout<<endl;cout<<" : "<<endl;show_Table();for(int i = 1;i <= stuNum-1;i++){cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex;for(int j = 0;j < SUBJECT_NUM;j++){cout<<"\t"<<record[i].score[j];}cout<<endl;}/*stuNum--;cout<<" "<<stuNum<<"cout<<endl;*/}stuNum--;cout<<" "<<stuNum<<cout<<endl;}}}/*//void delete_Name(char tarName[]){int i;for(i = 1;i <= stuNum;i++){if(strcmp(record[i].name,tarName) == 0){////1.//2.//if(i = stuNum){cout<<" : "<<endl;show_Table();cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex<<"\t"<<record[i].score[0]<<record[i].score[1]<<"\t"<<record[i].score[2];cout<<endl;cout<<endl<<" : "<<endl;show_Table();for(int i = 1;i <= stuNum - 1;i++){cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex;for(int j = 0;j < SUBJECT_NUM;j++){cout<<"\t"<<record[i].score[j];}cout<<endl;}}/if(i != stuNum){cout<<" : "<<endl;show_Table();cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex<<"\t"<<record[i].score[0]<<"\t"<<record[i].score[1]<<"\t"<<record[i].score[2];//for(int j = i+1;j <= stuNum;j++){record[j-1] = record[j];}cout<<endl;//cout<<" : "<<endl;show_Table();for(int i = 1;i <= stuNum-1;i++){cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex;for(int j = 0;j < SUBJECT_NUM;j++){cout<<"\t"<<record[i].score[j];}cout<<endl;}cout<<endl;void delete_Name(char tarName[]){int i;for(i = 1;i <= stuNum;i++){////1.//2.// if(strcmp(record[i].name,tarName) == 0){if(i == stuNum){cout<<" : "<<endl;show_Table();cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex<<"\t"<<record[i].score[0]<<"\t"<<record[i].score[1]<<"\t"<<record[i].score[2];cout<<endl;cout<<" : "<<endl;show_Table();for(int i = 1;i <= stuNum-1;i++){cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex;for(int j = 0;j < SUBJECT_NUM;j++){cout<<"\t"<<record[i].score[j];}cout<<endl;}/*stuNum--;cout<<" "<<stuNum<<" ";cout<<endl;}/if(i != stuNum){cout<<" "<<endl;show_Table();cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex<<"\t";cout<<record[i].score[0]<<"\t"<<record[i].score[1]<<"\t"<<record[i].score[2];cout<<endl;for(int j = i+1;j <= stuNum;j++){record[j-1] = record[j];}//. cout<<endl;cout<<" : "<<endl;show_Table();for(int i = 1;i <= stuNum-1;i++){cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex;for(int j = 0;j < SUBJECT_NUM;j++){cout<<"\t"<<record[i].score[j];}cout<<endl;}/*stuNum--;cout<<" "<<stuNum<<cout<<endl;*/}stuNum--;cout<<" <<stuNum<< ";cout<<endl;}}}/***************************************************************************** ******************************************************************************/void Info_modify(){cout<<"\ : "<<endl;cout<<"1. "<<endl;cout<<"2. "<<endl;cout<<"3. "<<endl;}void modifyRecord(){int key;cout<<endl;Info_modify();cout<<" : ";cin>>key;//if(key == 1){int targetNum;cout<< : ";cin>>targetNum;modify_Num(targetNum);cout<<endl;//display();//if(key == 2){char targetName[20];cout<< : ";cin>>targetName;modify_Name(targetName); cout<<endl;//display();}if(key == 3){while(1){menu_CMD();}}}//void modify_Num(int tarNum) {int i;for(i = 1;i <= stuNum;i++) {if(record[i].num == tarNum) {cout<<endl<<" "<<endl;cout<<" : ";cin>>record[i].num;cout<<" : ";cin>>record[i].name;cout<<";cin>>record[i].sex;cout<<" "<<subject[0]<<" ";cin>>record[i].score[0];cout<<" "<<subject[1]<<" : ";cin>>record[i].score[1];cout<<" "<<subject[2]<< ";cin>>record[i].score[2];}}}void modify_Name(char tarName[]){int i;for(i = 1;i <= stuNum;i++){if(strcmp(record[i].name,tarName) == 0) {cout<<endl<<" : "<<endl;cout<<" : ";cin>>record[i].num;cout<<" : ";cin>>record[i].name;cout<<" : ";cin>>record[i].sex;cout<<" "<<subject[0]<<" : ";cin>>record[i].score[0];cout<<" "<<subject[1]<<" : ";cin>>record[i].score[1];cout<<" "<<subject[2]<<" : ";cin>>record[i].score[2];}}}void Info_query(){cout<<" : "<<endl; cout<<"1. "<<endl; cout<<"2. "<<endl; cout<<"3. "<<endl; }//queryRecordvoid queryRecord(){int key;cout<<endl;Info_query();cout<<" ";cin>>key;if(key == 1){int targetNum;cout<<" : ";cin>>targetNum;query_Num(targetNum);cout<<endl;}if(key == 2){char targetName[20];cout<<" : ";cin>>targetName;query_Name(targetName);cout<<endl;}// .if(key == 3){while(1){menu_CMD();}void query_Num(int tarNum){int i;for(i = 1;i <= stuNum;i++){if(record[i].num == tarNum){// cout<<" : "<<endl;//show_Table();// cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex;cout<<"\t"<<record[i].score[0]<<"\t"<<record[i].score[1]<<"\t"<<record[i].score[ 2];cout<<endl;}}}//void query_Name(char tarName[]){int i;for(i = 1;i <= stuNum;i++){if(strcmp(record[i].name,tarName) == 0){cout<<" : "<<endl;show_Table();cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex;cout<<"\t"<<record[i].score[0]<<"\t"<<record[i].score[1]<<"\t"<<record[i].score[ 2];cout<<endl;}}}//void display(){show_Table();int i,j;for(i = 1;i <= stuNum;i++){//cout<<"学号"<<"\t"<<"姓名"<<"\t"<<"性别";cout<<record[i].num<<"\t"<<record[i].name<<"\t"<<record[i].sex;for(j = 0;j < SUBJECT_NUM;j++){cout<<"\t"<<record[i].score[j];}cout<<endl;}cout<<endl;}//void quit(){char choi;cout<<" "<<endl;cout<<" (Y/N) : ";cin>>choi;if((choi == 'Y')||(choi == 'y')){cout<< "<<endl;exit(0);}/ else{cout<<endl; menu_CMD();。
学生信息管理系统C语言报告

学生信息管理系统C语言报告简介学生信息管理系统是一种可以对学生信息进行管理的软件系统。
通过该系统,可以方便地添加、修改、查询和删除学生信息,提高学生信息管理的效率和准确性。
本报告将介绍学生信息管理系统的设计和实现过程,包括系统功能、技术选型和代码结构等方面。
通过该报告的阅读,读者可以了解到该系统的开发背景和主要功能,以及查看源代码和运行效果。
系统功能学生信息管理系统的主要功能如下:1. 添加学生信息:可以输入学生的姓名、年龄、性别、学号等基本信息,并保存到系统中。
2. 修改学生信息:可以根据学生的学号或姓名,修改学生的基本信息。
3. 查询学生信息:可以根据学生的学号、姓名、年龄、性别等条件,查询学生的基本信息。
4. 删除学生信息:可以根据学生的学号或姓名,将学生的基本信息从系统中删除。
技术选型为了实现学生信息管理系统的需求,我们选择使用C语言进行开发。
C语言是一种高效、可靠的编程语言,有着丰富的开发资源和庞大的用户群体。
在开发过程中,我们采用了以下技术选型:- 编程语言:C语言C语言作为一种结构化的编程语言,具有强大的计算能力和灵活的控制语句,非常适合开发类似学生信息管理系统这样的小规模应用。
代码结构学生信息管理系统的代码结构如下:cinclude <stdio.h>struct Student {char name[50];int age;char gender[10];int student_id;};void add_student(struct Student *students, int *count) {添加学生信息的代码逻辑}void modify_student(struct Student *students, int count) {修改学生信息的代码逻辑}void query_student(struct Student *students, int count) {查询学生信息的代码逻辑}void delete_student(struct Student *students, int *count) {删除学生信息的代码逻辑}int main() {struct Student students[100];int count = 0;主程序逻辑,包括用户界面等}在代码结构中,我们定义了一个`Student`结构体,用于存储学生的基本信息。
C语言学生信息管理系统(完整版)

#define PRINT0 printf("name:%s\nsex:%s\nage:%d\nID_card:%d\naddress:%s\n",st[i].name,st[i].sex,st[i].age,st[i] .ID_card,st[i].addr)#define PRINT1 printf("prefession:%s\nstudent_number:%d\n*****score*****\nwuli:%d\n",st[i].prefession,st[i] .student_number,st[i].score.wuli)#define PRINT2 printf("gaoshu:%d\nyingyu:%d\ntiyu:%d\naverage: %d\n",st[i].score.gaoshu,st[i].score.yingyu,st[i ].score.tiyu,st[i].score.aver)#define print1 printf("________________________________")#define N 2#include "string.h"#include "stdio.h"int sum=0;struct score{int wuli;int gaoshu;int yingyu;int tiyu;int aver;};struct message{ char name[10];int age;char sex[5];int ID_card;char addr[30];char prefession[30];int student_number;struct score score;}st[100];/*************write message*************/write_message(){ int flag;char chioce;do{system("cls");flag=2; sum++;printf("_______________________________");printf("please input student's message:\n");printf("\n");print1;printf("%dth student message:",sum);print1;printf("\nname:");scanf("%s",st[sum].name);printf("\nsex:");scanf("%s",st[sum].sex);printf("\nage:");scanf("%d",&st[sum].age);printf("\nID_card:");scanf("%d",&st[sum].ID_card);printf("\naddress:");scanf("%s",st[sum].addr);printf("\nprefession:");scanf("%s",st[sum].prefession);printf("\nschool number:");scanf("%d",&st[sum].student_number);printf("\n*****score******\n");printf("wuli:");scanf("%d",&st[sum].score.wuli);printf("\ngaoshu:");scanf("%d",&st[sum].score.gaoshu);printf("\nyingyu:");scanf("%d",&st[sum].score.yingyu);printf("\ntiyu:");scanf("%d",&st[sum].score.tiyu);printf("\naverage:");scanf("%d",&st[sum].score.aver);do{printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");getchar();scanf("%c",&chioce);printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}else if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);save_message();}/*************save message****************/save_message(){ FILE *fp;int i;if((fp=fopen("student.txt","wb"))==NULL){printf("read error \n");printf("press any key back to menu\n");getch();exit(1);}for(i=0;i<sum;i++)if(fwrite(&st[i],sizeof(struct message),1,fp)!=1){printf("write error\n");fclose(fp);}fclose(fp);printf("\n********___OK!___**********\n___press any key back___");sum=i;bioskey(0);}/***************add message*****************/add_message(){int i,j,flag; char chioce;i=0;j=sum-1;flag=0;do{ system("cls");i++; j++;print1;printf("add %dth student's meaasge\n",i);print1;printf("\nname:");scanf("%s",st[j].name);printf("\nsex:");scanf("%s",st[j].sex);printf("\nage:");scanf("%d",&st[j].age);printf("\nID_card:");scanf("%d",&st[j].ID_card);printf("\naddress:");scanf("%s",st[j].addr);printf("\nprefession:");scanf("%s",st[j].prefession);printf("\nstudent_number:");scanf("%d",&st[j].student_number);printf("\n*****score******\n");printf("wuli:");scanf("%d",&st[j].score.wuli);printf("\ngaoshu:");scanf("%d",&st[j].score.gaoshu);printf("\nyingyu:");scanf("%d",&st[j].score.yingyu);printf("\ntiyu:");scanf("%d",&st[j].score.tiyu);printf("\naverage:");scanf("%d",&st[j].score.aver);printf("\n\nweather add %dth student's message: \n",i+1);do{printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");getchar();scanf("%c",&chioce);printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}else if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;}while(1);}while(flag==1);sum=j+1;save_message();}/**********inqiure message******/inqiure_message(){int chioce;do{system("cls");printf("**********choose 0-3**********\n\n\n");printf(" 1:name inquire\n\n\n");printf(" 2:IDcard inqiure\n\n\n");printf(" 3:student_number\n \n\n");printf(" 0:back menu\n\n\n");scanf("%d",&chioce);switch(chioce){case 1: name_inqiure();break;case 2: ID_card_inqiure();break;case 3: grade_inqiure();break;case 0:break;}}while(chioce!=0);}/**********name inqiure*********/name_inqiure(){char NAME[30];int i; int flag,k;char chioce;do{ system("cls");k=0;printf("please input the message you inqiure");printf("\nname:");scanf("%s",NAME);getchar();printf("\n");for(i=0;i<sum;i++){if(strcmp(st[i].name,NAME)==0){PRINT0;PRINT1;PRINT2;k=1;}}if(k==0) printf("without message you inqiure\n");do{ printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");scanf("%c",&chioce); getchar();printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);printf("press any key return to menu");bioskey(0);}/**********IDcard inqiure*********/ID_card_inqiure(){int card;int i; int flag,k;char chioce;do{ clrscr(); k=0;printf("please input the message you inqiure");printf("\nIDcard:");scanf("%d",&card);getchar();printf("\n");for(i=0;i<sum;i++){if(st[i].ID_card==card){PRINT0;PRINT1;PRINT2;k=1;}}if(k==0) printf("without message you inqiure\n");do{ printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");scanf("%c",&chioce); getchar();printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);printf("press any key return to menu");bioskey(0);}/*********grade inqiure***********/grade_inqiure(){int GRADE;int i; int flag,k;char chioce;do{ system("cls");k=0;printf("please input the message you inqiure");printf("\nstudent_number:");scanf("%d",&GRADE);getchar();printf("\n");for(i=0;i<sum;i++){if(st[i].student_number==GRADE){PRINT0;PRINT1;PRINT2;k=1;}}if(k==0) printf("without message you inqiure\n");do{ printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");scanf("%c",&chioce); getchar();printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);printf("press any key return to menu");bioskey(0);}/*********change message**********/change_message(){char pre[30],chioce;int i,gra,flag,num,s;s=0;do{system("cls");printf("please input message you want to change\n ");printf("student_number:");scanf("%d",&gra); getchar();for(i=0;i<sum;i++){if(st[i].student_number==gra){PRINT0;PRINT1;PRINT2;printf("\n********input message you want to change********\n");printf("0:name***1:sex***2:age***3:ID_card***4:address***\n5:prefession***6:wuli***7:stud ent_number***8\n:gaoshu***9:yingyu***10:tiyu***11:average***________\n");printf("choose 0-11\n");scanf("%d",&num); getchar();switch(num){case 0: printf("input the name changed\n");scanf("%s",st[i].name); getchar(); break;case 1: printf("input the sex changed\n");scanf("%s",st[i].sex); getchar(); break;case 2: printf("input the age changed\n");scanf("%d",&st[i].age); getchar(); break;case 3: printf("input the ID_card changed\n");scanf("%d",&st[i].ID_card); getchar(); break;case 4: printf("input the address changed\n");scanf("%s",st[i].addr); getchar(); break;case 5: printf("input the prefession changed\n");scanf("%s",st[i].prefession); getchar(); break;case 6: printf("input the wuli_score changed\n");scanf("%d",&st[i].score.wuli); getchar(); break;case7: printf("input the student_number changed\n");scanf("%d",&st[i].student_number); getchar(); break;case 8: printf("input the gaoshu_score changed\n");scanf("%d",&st[i].score.gaoshu); getchar(); break;case 9: printf("input the yingyu_score changed\n");scanf("%d",&st[i].score.yingyu); getchar(); break;case 10: printf("input the tiyu_score changed\n");scanf("%d",&st[i].score.tiyu); getchar(); break;case 11: printf("input the average_score changed\n");scanf("%d",&st[i].score.aver); getchar(); break;default: printf("input error\n"); break;}printf("\n*********the changed message*********\n\n");PRINT0;PRINT1;PRINT2;s=1;}}if(s!=1) printf("without message you want to change\n");do{ printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");scanf("%c",&chioce); getchar();printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);save_message();}/*********delete message**********/delete_message(){ int GRADE;int i,j,flag1,flag;char chioce1,chioce2;flag1=3;flag=3;do{ system("cls");printf("please input student's student_number you want to delete\n");printf("student_number:");scanf("%d",&GRADE); getchar();for(i=0;i<sum;i++)if(st[i].student_number==GRADE){do{PRINT0;PRINT1;PRINT2;printf("\n******************************\n_________________ _________________\n");printf("press y/Y deleted:\npress n/N cancel:\n");scanf("%c",&chioce1);getchar();system("cls");if(chioce1=='y'||chioce1=='Y') flag1=1;else if(chioce1=='n'||chioce1=='N') return;else {printf("***input error***\n___press any ker return___\n"); bioskey(0);}}while(flag1!=1);for(j=i;j<sum;j++){ st[j]=st[j+1];flag=2;printf("message was deleted\n");sum-=1; }}if(flag!=2) printf("without message you want to delete\n");do{printf("press y/Y continue:\npress n/N stop:\n");scanf("%c",&chioce2);printf("****************************\n");if(chioce2=='y'||chioce2=='Y') {flag=1; break;}else if(chioce2=='n'||chioce2=='N') {flag=0; break;}else {system("cls");printf("input error\n");}print1;printf("\n");}while(1);}while(flag==1);save_message();}/************insert message*************/insert_message(){ int chioce,flag,i; flag=2;do{system("cls");printf("please input the number of people you insert\n");scanf("%d",&chioce);if(chioce>=sum){printf("xin xi pai zai zui hou ");chioce=sum;}for(i=sum;i>chioce;i--) st[i]=st[i-1];printf("\nplease input message you insert");printf("\nname:");scanf("%s",st[i].name);printf("\nsex:");scanf("%s",st[i].sex);printf("\nage:");scanf("%d",&st[i].age);printf("\nID_card:");scanf("%d",&st[i].ID_card);printf("\naddress:");scanf("%s",st[i].addr);printf("\nprefession:");scanf("%s",st[i].prefession);printf("\ngrade:");scanf("%d",&st[i].student_number);printf("\n*****score******\n");printf("wuli:");scanf("%d",&st[i].score.wuli);printf("\ngaoshu:");scanf("%d",&st[i].score.gaoshu);printf("\nyingyu:");scanf("%d",&st[i].score.yingyu);printf("\ntiyu:");scanf("%d",&st[i].score.tiyu);printf("\naverage:");scanf("%d",&st[i].score.aver);sum+=1;do{printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");getchar();scanf("%c",&chioce);printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}else if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);save_message();}/*********school_number_order***********/grade_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].student_number>st[j].student_number){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("grade order from min to max\n");for(i=0;i<sum;i++){ print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);bioskey(0);}/***********wuli score order***************/wuli_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].score.wuli>st[j].score.wuli){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("wuli score order from min to max\n");for(i=0;i<sum;i++){print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);print1;bioskey(0);}/***********gaoshu score order***************/gaoshu_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].score.gaoshu>st[j].score.gaoshu){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("gaoshu score order from min to max\n");for(i=0;i<sum;i++){print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);print1;bioskey(0);}/***********yingyu score order***************/yingyu_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].score.yingyu>st[j].score.yingyu){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("yingyu score order from min to max\n");for(i=0;i<sum;i++){print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);print1;bioskey(0);}/***********tiyu score order***************/tiyu_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].score.tiyu>st[j].score.tiyu){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("yitu order from min to max\n");for(i=0;i<sum;i++){print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);print1;bioskey(0);}/***********average score order***************/average_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].score.wuli>st[j].score.wuli){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("average score order from min to max\n");for(i=0;i<sum;i++){print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);print1;bioskey(0);}/********order message****************/order_message(){int chioce;do{system("cls");printf("**********choose 0-6**********\n\n");printf(" 1:grade order\n\n");printf(" 2:wuli score order\n\n");printf(" 3:gaoshu score order\n \n");printf(" 4:ying yu score order\n\n");printf(" 5:tiyu score order\n\n");printf(" 6:average score order\n\n");printf(" 0:back menu\n\n");scanf("%d",&chioce);switch(chioce){case 1: grade_order();break;case 2: wuli_order();break;case 3: gaoshu_order();break;case 4: yingyu_order();break;case 5: tiyu_order();break;case 6: average_order();break;case 0:break;}}while(chioce!=0);}/**********answer secretory***********/mima_message(){int flag;char answer[10];char secret[10]="abcd";flag=2;do{system("cls");printf("\n______________________mi ma wei 'abcd'________________\n\n");printf("______________________qing shu ru mi ma:");scanf("%s",answer);getchar();if(strcmp(secret,answer)==0){flag=1;printf("\n\n =====throngh=====\n\n\n");printf("____________________press any key into next:\n");bioskey(0);}else{flag=0;printf("______________________input error:\n");printf("______________________press any key to return:\n");bioskey(0);}}while(flag!=1);}/*********read message****************/read_message(){int i;system("cls");if(sum<=0){ printf("without message\n");getch();return;}for(i=0;i<sum;i++){system("cls");print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;printf("\n********press any key -show the next one********\n ");getch();}printf("________________totle num :%d________________\n",sum); printf("_____________press any key return______________\n"); bioskey(0);}/*************************************/main(){int chioce,flag;mima_message();do{system("cls");chioce=9;printf("**********************************************\n");printf("****welcome to system of managing students****\n");printf("**********************************************\n\n");printf("-------------------choose 0-8-----------------\n\n");printf(" 1:write message\n\n");printf(" 2:add message\n\n");printf(" 3:inqiure name\n\n");printf(" 4:change message\n\n");printf(" 5:insert message\n\n");printf(" 6:order message\n\n");printf(" 7:delete message\n\n");printf(" 8:read messaeg\n\n");printf(" 0:***exit***\n\n");scanf("%d",&chioce);getchar();switch(chioce){case 1: write_message();break;case 2: add_message();break;case 3: inqiure_message();break;case 4: change_message();break;case 5: insert_message();break;case 6: order_message();break;case 7: delete_message();break;case 8: read_message();break;case 0: printf("___sure press y/Y:___\n\n___no sure press n/N:___");scanf("%c",&chioce);getchar();if(chioce=='y'||chioce=='Y') flag=0;else flag=1;break;default : printf("\n ___input error___\n\n");printf("***press any key to go on***\n");getch();break;}}while(flag!=0);save_message();system("cls");printf("\n\n___message was saved___\n\n\n*****file name is student.txt*****\n");bioskey(0);}。
学生信息管理系统C语言编程

学生信息管理系统C语言编程【问题描述】学生信息的管理是每个学校必须具有的管理功能,主要是对学生的基本情况及学习成绩等方面的管理。
该系统模拟一个简单的学生管理系统,要求对文件中所存储的学生数据进行各种常规操作,如:排序、查找、计算、显示等功能。
通过此课题,熟练掌握文件、数组、结构体的各种操作,在程序设计中体现一定的算法思想,实现一个简单的学生信息管理系统。
【基本要求】(1)学生信息包括:学生基本信息文件(student.txt)(注:该文件不需要编程录入数据,可用文本编辑工具直接生成)的内容如下:(2)学生成绩基本信息文件(score.dat)及其内容如下:((注:该文件内容需要编程录入数据,具体做法见下面的要求)学号课程编号课程名称学分平时成绩实验成绩卷面成绩综合成绩实得学分(3)需要实现的功能1)数据录入和计算功能:对score.dat进行数据录入,只录入每个学生的学号、课程编号、课程名称、学分、平时成绩、实验成绩、卷面成绩共7个数据,综合成绩、实得学分由程序根据条件自动运算。
综合成绩的计算:如果本课程的实验成绩为-1,则表示无实验,综合成绩=平时成绩*30%+卷面成绩*70%;如果实验成绩不为-1,表示本课程有实验,综合成绩=平时成绩*15%+实验成绩*15%+卷面成绩*70% 。
实得学分的计算:采用等级学分制,综合成绩在90-100之间,应得学分=学分*100%;综合成绩在80-90之间,应得学分=学分*80%;综合成绩在70-80之间 ,应得学分=学分*75%;综合成绩在60-70之间,应得学分=学分*60%;综合成绩在60以下 ,应得学分=学分*0%。
2)查询功能:分为学生基本情况查询和成绩查询两种(1)学生基本情况查询:①、输入一个学号或姓名(可实现选择),查出此生的基本信息并显示输出,格式如下:②、输入一个宿舍号码,可查询出本室所有的学生的基本信息并显示输出。
(格式如上所示)(2)成绩查询:①、输入一个学号时,查询出此生的所有课程情况,格式如下:学号:xx 姓名:xxxxx课程编号:xxx 课程名称:xxxxx 综合成绩:xxxx 实得学分: xx课程编号:xxx 课程名称:xxxxx 综合成绩:xxxx 实得学分: xx课程编号:xxx 课程名称:xxxxx 综合成绩:xxxx 实得学分: xx ……………………共修:xx科,实得总学分为: xxx(3)删除功能:提供待删除学生的学号,则在student.txt和score.dat中删除所有与该学生有关的信息。
C语言上机实验报告--学生信息管理系统设计__内附源代码
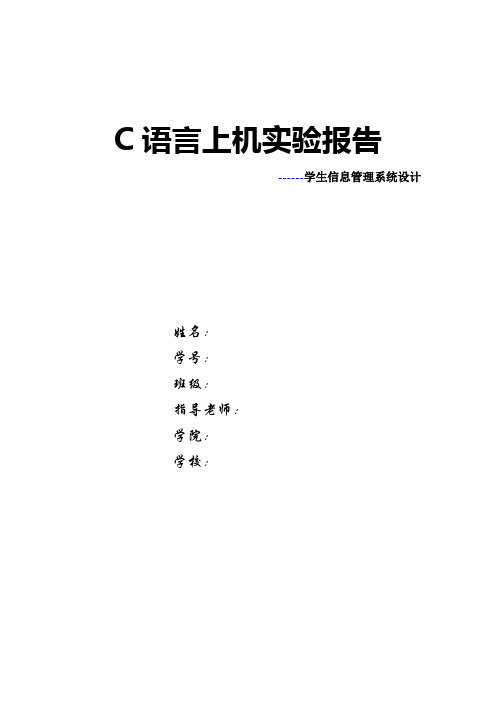
C语言上机实验报告------学生信息管理系统设计姓名:学号:班级:指导老师:学院:学校:C语言上机实验报告------学生信息管理系统设计一、实验题目学生信息管理系统设计学生信息包括:学号,姓名,年龄,性别,出生年月,地址,电话,E-mail 等。
试设计一学生信息管理系统,使之能提供以下功能:(1)系统以菜单方式工作(2)学生信息录入功能(学生信息用文件保存)---输入(3)学生信息浏览功能---输出(4)查询、排序功能---算法(5)按学号查询(6)按姓名查询(7)学生信息的删除与修改(可选项)二、本课程的地位、作用与目的为综合应用C语言程序设计理论知识、进一步提高学生综合解决问题、协调工作的能力和良好的软件开发习惯,特安排本实验内容。
希望通过该实习能够进一步激发学生的学习热情,培养学生初步编程的能力,为后续的学习和发展奠定基础。
三、分析过程1、能够实现对学生基本信息数据的增加和删除2、实现对录入保存后的学生基本信息进行格式化浏览3、提供学号和姓名两种方式对学生基本信息数据的查询4、能够对随机输入的学生基本信息数据按学号进行排序5、学生信息数据保存在文件中,方便数据的多次使用为了实现以上功能,必须设计的函数如下:录入函数、查询函数(分为姓名查询和学号查询)、删除函数、排序函数和几个菜单绘制函数等四、实验源代码:#include <stdio.h>#include<string.h>#include<conio.h>#include<stdlib.h>#include<time.h>#define num 12//定义学生结构体typedef struct student{int id;char name[num];int age;char sex[4];struct birth{int year;int month;int day;}date;char add[30];int phone;}stu;//功能选择菜单void menu(){printf("\n");printf("\n");printf(" 学生信息管理系统\n");printf("\n");printf("********************************************************************* **\n");printf(" ==============1.学生信息录入 2.学生信息浏览===============\n");printf("\n");printf(" ==============3.学号查询信息 4.姓名查询信息===============\n");printf("\n");printf(" ==============5.按学号排序 6.删除学生信息===============\n");printf("\n");printf(" ============== 0.退出系统===============\n");printf("\n");printf("\n");printf("********************************************************************* *\n");printf("\n");printf(" 按编号选择对应功能\n");printf("\n");printf(" +++++++++++++++++++ XXXXXXX制作+++++++++++++++++++++\n");printf("\n");}//修改菜单void printmenu(){printf("********************************************************* **\n");printf("-------1.完全修改 2.学号修改---------");printf("-------3.姓名修改 4.性别修改---------");printf("-------5.生日修改 6.地址修改---------");printf("-------7.电话修改 0.退出修改---------");printf("********************************************************* **\n");}//取当前时间int nowtime(){struct tm *local;time_t t;t=time(NULL);local=localtime(&t);return local->tm_year+1900;//求出当前时间的年份}//输出文件中学生信息void print(char *file){stu temp;FILE *fp=fopen(file,"r");if(fp==NULL){printf("打开文件%s失败!\n",file);return;}printf("文件%s中学生信息如下:\n",file);printf("%8s%8s%6s%6s%8s%4s%4s%16s%12s\n","学号","姓名","年龄","性别","出生年","月","日","地址","电话");fseek(fp,0,SEEK_SET);//从文件开始为之读取while(fread(&temp,sizeof(stu),1,fp))printf("%8d%8s%6d%6s%8d%4d%4d%16s%12d\n",temp.id,,temp.age,t emp.sex,temp.date.year,temp.date.month,temp.date.day,temp.add,temp.ph one);//格式化输出学生信息fclose(fp);//关闭文件}void luru(char *file){int count,sexnum,i=0,m;stu temp,temp1;FILE *fp=fopen(file,"a+");//追加方式打开文件if(fp==NULL){printf("打开文件%s失败!",file);return;}printf("请确定本次需要录入几名学生信息:");scanf("%d",&count);for (i=0;i<count ;i++ ){printf("录入要写入文件%s的第%d名学生信息\n",file,i+1); Repeat:printf("请输入学号:");m=scanf("%d",&temp.id);if (m==0){printf("输入的数据不是整型数据!\n");fflush(stdin);//清除缓存goto Repeat;}fseek(fp,0,SEEK_SET);//从文件开始位置读取while(fread(&temp1,sizeof(stu),1,fp)){if (temp1.id==temp.id)//判断学号是否已存在{printf("学号已存在!\n");goto Repeat;}//若学号已存在,返回继续读取学号}printf("请输入姓名:");scanf("%s",);printf("请输入性别:0为女生,1为男生:");{scanf("%d",&sexnum);if (sexnum==1) strcpy(temp.sex,"男");else strcpy(temp.sex,"女");}printf("请输入出生年月日:");scanf("%d%d%d",&temp.date.year,&temp.date.month,&temp.date.day);temp.age=nowtime()-temp.date.year;printf("请输入地址:");scanf("%s",&temp.add);printf("请输入联系电话11位:");scanf("%d",&temp.phone);if(!fwrite(&temp,sizeof(stu),1,fp)){printf("向文件%s写入信息失败!\n",file);return;}}printf("向文件%s写入信息成功!\n",file);fclose(fp);}int IsEmpty(FILE *fp){int len;fseek(fp,0,SEEK_END);//指针移动到文件尾len=ftell(fp);//取指针的文职获取长度return(len==0)?1:0;//0代表文件为空}void idsearch(char *file){int findid;stu temp;FILE *fp=fopen(file,"r");if (fp==NULL){printf("文件无法打开!");return;}if(IsEmpty(fp)){printf("文件为空,请先录入信息!");return;}printf("输入要查找学生的学号:");scanf("%d",&findid);fseek(fp,0,SEEK_SET);while(fread(&temp,sizeof(stu),1,fp)){if(temp.id==findid){printf("%8s%8s%6s%6s%8s%4s%4s%16s%11s\n","学号","姓名","年龄","性别","出生年","月","日","地址","电话");printf("%8d%8s%6d%6s%8d%4d%4d%16s%11d\n",temp.id,,temp.a ge,temp.sex,temp.date.year,temp.date.month,temp.date.day,temp.add,tem p.phone);//格式化输出学生信息return;}}printf("没有找到学号为%d的学生的信息!",findid);return;fclose(fp);}void namesearch(char *file){char name[num];stu temp;FILE *fp=fopen(file,"r");if (fp==NULL){printf("文件无法打开!");return;}if(IsEmpty(fp)){printf("文件为空,请先输入学生信息!");return;}printf("输入要查找的学生的姓名:");scanf("%s",name);fseek(fp,0,SEEK_SET);while(fread(&temp,sizeof(stu),1,fp)){if (!strcmp(,name)){printf("下面是姓名为%s的学生的信息:\n",name);printf("%8s%8s%6s%6s%8s%4s%4s%16s%11s\n","学号","姓名","年龄","性别","出生年","月","日","地址","电话");printf("%8d%8s%6d%6s%8d%4d%4d%16s%11d\n",temp.id,,temp.a ge,temp.sex,temp.date.year,temp.date.month,temp.date.day,temp.add,tem p.phone);//格式化输出学生信息return;}}printf("没有找到姓名为%s的学生的信息!",name);fclose(fp);}//排序函数void paixu(char *file){int i,j,k,len;stu s[num],temp;FILE *fp=fopen(file,"r");if(fp==NULL){printf("文件无法打开!");return;}if(IsEmpty(fp)){printf("文件为空,请先录入信息!");return;}fseek(fp,0,SEEK_END);//指针移动到文件末尾len=ftell(fp)/sizeof(stu);fseek(fp,0,SEEK_SET);for(i=0;i<len;i++)fread(s+i,sizeof(stu),1,fp);fclose(fp);for(i=0;i<len;i++){k=i;for(j=i+1;j<len;j++){if(s[j].id<s[k].id)k=j;}if(k!=i){temp=s[i];s[i]=s[k];s[k]=temp;}}printf("下面是按学号从小到大顺序排序后的学生的信息:\n");printf("%8s%8s%6s%6s%8s%4s%4s%16s%11s\n","学号","姓名","年龄","性别","出生年","月","日","地址","电话");for(i=0;i<len;i++)printf("%8d%8s%6d%6s%8d%4d%4d%16s%11d\n",s[i].id,s[i].name,s[i].a ge,s[i].sex,s[i].date.year,s[i].date.month,s[i].date.day,s[i].add,s[i ].phone);//格式化输出学生信息remove("学生信息表.txt");//删除以前存放数据的文件fp=fopen(file,"w");for(i=0;i<len;i++)fwrite(s+i,sizeof(stu),1,fp);fclose(fp);}//删除函数void shanchu(char *file){int i=0,len,del;stu s[num];FILE *fp=fopen(file,"r");if(fp==NULL){printf("文件无法打开!");return;}if(IsEmpty(fp)){printf("文件为空,请先录入学生信息!");return;}printf("输入需要删除的学生的学号:");scanf("%d",&del);fseek(fp,0,SEEK_SET);while(fread(s+i,sizeof(stu),1,fp)){if(s[i].id==del){printf("下面是按学号为%d的学生的信息:\n",del);printf("%8s%8s%6s%6s%8s%4s%4s%16s%11s\n","学号","姓名","年龄","性别","出生年","月","日","地址","电话");printf("%8d%8s%6d%6s%8d%4d%4d%16s%11d\n",s[i].id,s[i].name,s[i].a ge,s[i].sex,s[i].date.year,s[i].date.month,s[i].date.day,s[i].add,s[i ].phone);//格式化输出学生信息i=i-1;}i++;}fclose(fp);len=i;fp=fopen(file,"w");fseek(fp,0,SEEK_SET);for(i=0;i<len;i++) //删除后重写文件fwrite(s+i,sizeof(stu),1,fp);fclose(fp);}void main(){int key;char file[]={"学生信息表.txt"};do{menu();printf("选择对应功能操作:");scanf("%d",&key);switch(key){case 1:luru(file);break;case 2:print(file);break;case 3:idsearch(file);break;case 4:namesearch(file);break;case 5:paixu(file);break;case 6:shanchu(file);break;case 0:exit(0);default:printf("选择错误,请重新选择!");}printf("\n");printf("按任意键返回上级菜单!");getch();fflush(stdin);system("cls");}while (key!=0);}五.实验过程及结果:1.程序主菜单运行界面2.学生信息录入运行界面3.学生信息浏览运行界面4.学生学号查询运行界面5.学生姓名查询运行界面6.按学号排序运行界面第一次出现乱码,改正后运行如第二张图片所示7.删除学生信息运行界面六、感想在本次实验的过程中,出现了各种各样的问题。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
学生信息管理系统C++代码-标准化文件发布号:(9556-EUATWK-MWUB-WUNN-INNUL-DDQTY-KII1.程序执行后的部分效果1.1项目主菜单效果图1.2初始化信息,第一次对信息的录入1.3添加学生信息1.4删除某学生信息1.5修改某学生信息1.6查询某学生信息1.7显示全部学生信息源代码:/*把StudentData.cpp(源代码) 和 student.txt(数据存放处)放在同一个文件夹下*/#include <iostream>#include <string>#include <fstream>#include <string>#include <iomanip>using namespace std;//最多提供50个学生的数据,可根据需要进行更改const int MAX = 50;int count = 0; //用来统计学生人数class Student{public:void Set(); //初始化信息,第一次对信息的录入void Add(); //添加学生信息//从磁盘读取数据以便进行数据的操作,方便再重写进磁盘friend void Read(string no[],string name[],string sex[],string special[],string clas[]);int Judge(string num); //判断 num 是否在学生信息数据库中(注意它有一个参数,并且有一个int型的返回值)void Delete(); //删除某学生信息void Change(); //修改某学生信息void Search(); //查询某学生信息void Display(); //显示全部学生信息private:string m_no;string m_name;string m_sex; //m_ 指的是成员变量(member)string m_special;string m_clas;};void Student::Set(){string no, name, sex, special, clas;ofstream outfile("student.txt"); //打开文件if(!outfile){cerr<<" open error"<<endl;exit(1); //退出程序}cout<<"当学号输入为 0 时,停止输入!"<<endl;cout<<"请依次输入学生的学号,姓名,性别,专业,班级:"<<endl;for(int i=0; i<MAX; i++){cout<<"第"<<count+1<<"个学生:"<<endl;cin>>no;if(no == "0") break; //当输入的学号是0 时,停止录入cin>>name>>sex>>special>>clas;count++;m_no = no;outfile<<m_no<<"\t"; //每录入一个学号,写进磁盘保存,以下同理m_name = name;outfile<<m_name<<"\t";m_sex = sex;outfile<<m_sex<<"\t";m_special = special;outfile<<m_special<<"\t";m_clas = clas;outfile<<m_clas<<endl;}outfile.close();}//添加学生信息void Student::Add(){string no, name, sex, special, clas;//以追加的方式录入信息,直接将信息追加到以前文件的末尾ofstream outfile("student.txt",ios::app);if(!outfile){cerr<<" open error"<<endl;exit(1);}count++; //添加一个学生信息,当然 count 要 +1cout<<"请依次输入要添加的学生学号,姓名,性别,专业,班级:"<<endl;cin>>no>>name>>sex>>special>>clas;m_no = no;outfile<<m_no<<"\t";m_name = name;outfile<<m_name<<"\t";m_sex = sex;outfile<<m_sex<<"\t";m_special = special;outfile<<m_special<<"\t";m_clas = clas;outfile<<m_clas<<endl;outfile.close();cout<<"已添加成功!"<<endl;}//从磁盘读取数据void Read(string no[],string name[],string sex[],string special[],string clas[]){ifstream infile("student.txt",ios::in);if(!infile){cerr<<" open error"<<endl;exit(1);}for(int i=0; i<count; i++) //只读取存放在数组中但不对其进行相关操作{infile>>no[i]>>name[i]>>sex[i]>>special[i]>>clas[i];}infile.close();}//判断某学号的学生是否在数据库中int Student::Judge(string num){string no[MAX], name[MAX], sex[MAX], special[MAX], clas[MAX];Read(no, name, sex, special, clas); //调用Read()函数,获取数据,以便等下进行相关数据的判断for(int i=0; i<count; i++){if(num == no[i]){return i; //如果存在,返回其下标break;}}return -1; //否则,返回-1}//删除某学生信息void Student::Delete(){string num, no[MAX], name[MAX], sex[MAX], special[MAX], clas[MAX];Read(no, name, sex, special, clas); //读取学生所有数据,cout<<"请输入你要删除的学生学号:";cin>>num;int k = Judge(num); //定义一个k来接收Judge()的返回值,等下用来判断该num是否存在if(k != -1) //如果k不等于-1,表示要删除的学生存在{ofstream outfile("student.txt");if(!outfile){cerr<<" open error"<<endl;exit(1);}for(int i=0; i<count; i++){if(i != k) //把下标不等于K(即除了要删的学生外)其余的数据重新写入磁盘保存{outfile<<no[i]<<"\t";outfile<<name[i]<<"\t";outfile<<sex[i]<<"\t";outfile<<special[i]<<"\t";outfile<<clas[i]<<endl;}}outfile.close();count--; //删除一个学生,人数 -1cout<<"删除成功!"<<endl;}elsecout<<"该数据库没有此学生!"<<endl;}void Student::Change(){string num, no[MAX], name[MAX], sex[MAX], special[MAX], clas[MAX];cout<<"请输入你要修改的学生学号:";cin>>num;int k = Judge(num);if(k != -1){Read(no, name, sex, special, clas); //读取学生所有数据,cout<<"请依次输入修改后的学生学号,姓名,性别,专业,班级:"<<endl;cin>>no[k]>>name[k]>>sex[k]>>special[k]>>clas[k];//把下标是k的学生进行修改ofstream outfile("student.txt",ios::out);if(!outfile){cerr<<" open error"<<endl;exit(1);}for(int i=0; i<count; i++) //改完后,回写进磁盘保存{outfile<<no[i]<<"\t";outfile<<name[i]<<"\t";outfile<<sex[i]<<"\t";outfile<<special[i]<<"\t";outfile<<clas[i]<<endl;}outfile.close();cout<<"修改成功!"<<endl;}elsecout<<"该数据库没有此学生!"<<endl;}void Student::Search(){string num, no[MAX], name[MAX], sex[MAX], special[MAX], clas[MAX];Read(no, name, sex, special, clas);cout<<"请输入你要查找的学生学号";cin>>num;int k = Judge(num);if(k != -1) //找到该学生就打印出其信息{cout<<"学号\t姓名\t性别\t专业\t班级"<<endl;cout<<no[k]<<"\t";cout<<name[k]<<"\t";cout<<sex[k]<<"\t";cout<<special[k]<<"\t";cout<<clas[k]<<endl;}elsecout<<"该数据库没有此学生!"<<endl;}//显示学生的所有信息void Student::Display(){string no[MAX], name[MAX], sex[MAX], special[MAX], clas[MAX];Read(no, name, sex, special, clas); //先读取cout<<"学号\t姓名\t性别\t专业\t班级"<<endl;for(int i=0; i<count; i++) //后打印{cout<<no[i]<<"\t";cout<<name[i]<<"\t";cout<<sex[i]<<"\t";cout<<special[i]<<"\t";cout<<clas[i]<<endl;}}int main(){int choice = -1;Student s;while(choice != 0) //除非你选择退出,否则一直循环{cout<<"\n\t\t\t--学生信息管理系统--\n\n";cout<<"\t\t\t| 1.录入学生信息 |\n";cout<<"\t\t\t| 2.添加一个信息 |\n";cout<<"\t\t\t| 3.删除一个信息 |\n";cout<<"\t\t\t| 4.修改学生信息 |\n";cout<<"\t\t\t| 5.查询学生信息 |\n";cout<<"\t\t\t| 6.显示学生信息 |\n";cout<<"\t\t\t| 0.退出系统 |\n";cout<<"请选择所需要的操作:";cin>>choice;switch(choice){case 1:s.Set();break;case 2:s.Add();break;case 3:s.Delete();break;case 4:s.Change();break;case 5:s.Search();break;case 6:s.Display();break;case 0:break;default:cout<<"你的选择有误!请重新选择!"<<endl;break;}}return 0;}。