计算器VC源代码
C语言实现计算器功能

C语言实现计算器功能C语言计算器实现是一种基本的编程练习,可以结合简单的数学表达式解析和计算功能来实现一个基本的计算器。
以下是一个简单的示例,它可以接受用户输入的数学表达式并返回计算结果。
首先,我们需要包含标准输入/输出库(stdio.h)和字符串处理库(string.h)。
我们还需要定义一些函数来处理数学表达式的解析和计算。
```c#include <stdio.h>#include <string.h>//返回运算符的优先级int precedence(char op)if (op == '+' , op == '-')return 1;if (op == '*' , op == '/')return 2;return 0;//执行四则运算int calculate(int a, int b, char op)switch (op)case '+':return a + b;case '-':return a - b;case '*':return a * b;case '/':return a / b;}return 0;//解析数学表达式并计算结果int evaluate(char *expression)int i;//创建两个空栈,用于操作数和运算符int values[100];int top_values = -1;char ops[100];int top_ops = -1;//遍历表达式的每个字符for (i = 0; i < strlen(expression); i++)//如果字符是一个数字,将其解析为整数,并将其推到操作数栈中if (expression[i] >= '0' && expression[i] <= '9')int value = 0;while (i < strlen(expression) && expression[i] >= '0' && expression[i] <= '9')value = value * 10 + (expression[i] - '0');i++;}values[++top_values] = value;}//如果字符是一个左括号,将其推到运算符栈中else if (expression[i] == '(')ops[++top_ops] = expression[i];}//如果字符是一个右括号,执行所有高优先级的运算else if (expression[i] == ')')while (top_ops > -1 && ops[top_ops] != '(')int b = values[top_values--];int a = values[top_values--];char op = ops[top_ops--];values[++top_values] = calculate(a, b, op);}top_ops--;}//如果字符是一个运算符,执行所有高优先级的运算elsewhile (top_ops > -1 && precedence(ops[top_ops]) >= precedence(expression[i]))int b = values[top_values--];int a = values[top_values--];char op = ops[top_ops--];values[++top_values] = calculate(a, b, op);}ops[++top_ops] = expression[i];}}//执行剩余的运算while (top_ops > -1)int b = values[top_values--];int a = values[top_values--];char op = ops[top_ops--];values[++top_values] = calculate(a, b, op);}//返回最终结果return values[top_values];int mainchar expression[100];printf("请输入数学表达式:");scanf("%s", expression);int result = evaluate(expression);printf("结果:%d\n", result);return 0;```上面的代码使用了栈来解析和计算数学表达式。
简单计算器c语言源码

#include <stdio.h>#include <string.h>#include <ctype.h>#include <math.h>//expression evaluate#define iMUL 0#define iDIV 1#define iADD 2#define iSUB 3#define iCap 4//#define LtKH 5//#define RtKH 6#define MaxSize 100void iPush(float);float iPop();float StaOperand[MaxSize];int iTop=-1;//char Srcexp[MaxSize];char Capaexp[MaxSize];char RevPolishexp[MaxSize];float NumCapaTab[26];char validexp[]="*/+-()";char NumSets[]="0123456789";char StackSymb[MaxSize];int operands;//void NumsToCapas(char [], int , char [], float []);int CheckExpress(char);int PriorChar(char,char);int GetOperator(char [], char);void counterPolishexp(char INexp[], int slen, char Outexp[]); float CalcRevPolishexp(char [], float [], char [], int);void main(){char ch;char s;int bl=1;while(bl==1){int ilen;float iResult=0.0;printf("输入计算表达式(最后以=号结束):\n");memset(StackSymb,0,MaxSize);memset(NumCapaTab,0,26); //A--NO.1, B--NO.2, etc.gets(Srcexp);ilen=strlen(Srcexp);NumsToCapas(Srcexp,ilen,Capaexp,NumCapaTab);ilen=strlen(Capaexp);counterPolishexp(Capaexp,ilen,RevPolishexp);ilen=strlen(RevPolishexp);iResult=CalcRevPolishexp(validexp, NumCapaTab, RevPolishexp,ilen);printf("\n计算结果:\n%.6f\n",iResult);printf("是否继续运算?(Y/N)\n");ch=getchar();s=getchar();//接受回车if(ch=='y'||ch=='Y'){bl=1;}else{bl=0;}}}void iPush(float value){if(iTop<MaxSize) StaOperand[++iTop]=value;}float iPop(){if(iTop>-1)return StaOperand[iTop--];return -1.0;}void NumsToCapas(char Srcexp[], int slen, char Capaexp[], float NumCapaTab[]) {char ch;int i, j, k, flg=0;int sign;float val=0.0,power=10.0;i=0; j=0; k=0;while (i<slen){ch=Srcexp[i];if (i==0){sign=(ch=='-')?-1:1;if(ch=='+'||ch=='-'){ch=Srcexp[++i];flg=1;}}if (isdigit(ch)){val=ch-'0';while (isdigit(ch=Srcexp[++i])) {val=val*10.0+ch-'0';}if (ch=='.'){while(isdigit(ch=Srcexp[++i])) {val=val+(ch-'0')/power; power*=10;}} //end ifif(flg){val*=sign;flg=0;}} //end if//write Capaexp array// write NO.j to arrayif(val){Capaexp[k++]='A'+j; Capaexp[k++]=ch;NumCapaTab[j++]=val; //A--0, B--1,and C, etc.}else{Capaexp[k++]=ch;}val=0.0;power=10.0;//i++;}Capaexp[k]='\0';operands=j;}float CalcRevPolishexp(char validexp[], float NumCapaTab[], char RevPolishexp[], int slen) {float sval=0.0, op1,op2;int i, rt;char ch;//recursive stacki=0;while((ch=RevPolishexp[i]) && i<slen){switch(rt=GetOperator(validexp, ch)){case iMUL: op2=iPop(); op1=iPop();sval=op1*op2;iPush(sval);break;case iDIV: op2=iPop(); op1=iPop();if(!fabs(op2)){printf("overflow\n");iPush(0);break;}sval=op1/op2;iPush(sval);break;case iADD: op2=iPop(); op1=iPop();sval=op1+op2;iPush(sval);break;case iSUB: op2=iPop(); op1=iPop();sval=op1-op2;iPush(sval);break;case iCap: iPush(NumCapaTab[ch-'A']); break;default: ;}++i;}while(iTop>-1){sval=iPop();}return sval;}int GetOperator(char validexp[],char oper) {int oplen,i=0;oplen=strlen(validexp);if (!oplen) return -1;if(isalpha(oper)) return 4;while(i<oplen && validexp[i]!=oper) ++i;if(i==oplen || i>=4) return -1;return i;}int CheckExpress(char ch){int i=0;char cc;while((cc=validexp[i]) && ch!=cc) ++i;if (!cc)return 0;return 1;}int PriorChar(char curch, char stach){//栈外优先级高于(>)栈顶优先级时,才入栈//否则(<=),一律出栈if (curch==stach) return 0; //等于时应该出栈else if (curch=='*' || curch=='/'){if(stach!='*' && stach!='/')return 1;}else if (curch=='+' || curch=='-'){if (stach=='(' || stach==')')return 1;}else if (curch=='('){if (stach==')')return 1;}return 0;}void counterPolishexp(char INexp[], int slen, char Outexp[]) {int i, j, k,pr;char t;i=0;j=k=0;while (INexp[i]!='=' && i<slen){if (INexp[i]=='(')StackSymb[k++]=INexp[i];//iPush(*(INexp+i));else if(INexp[i]==')'){//if((t=iPop())!=-1)while((t=StackSymb[k-1])!='('){Outexp[j++]=t;k--;}k--;}else if (CheckExpress(INexp[i])) // is oparator{// printf("operator %c k=%d\n",INexp[i],k);while (k){// iPush(*(INexp+i));if(pr=PriorChar(INexp[i],StackSymb[k-1])) break;else{//if ((t=iPop())!=-1)t=StackSymb[k-1]; k--;Outexp[j++]=t;}} //end whileStackSymb[k++]=INexp[i]; //common process }else //if() 变量名{// printf("operand %c k=%d\n",INexp[i],k); Outexp[j++]=INexp[i];}i++; //}while (k){t=StackSymb[k-1]; k--;Outexp[j++]=t;}Outexp[j]='\0';}。
C语言计算器源代码

C语言计算器源代码下面是一个简单的C语言计算器源代码:```c#include<stdio.h>int mainfloat num1, num2, result;char operator;printf("请输入两个数字:");scanf("%f %f", &num1, &num2);printf("请选择运算符(+,-,*,/):"); scanf(" %c", &operator);switch(operator)case '+':result = num1 + num2;printf("结果: %.2f\n", result);break;case '-':result = num1 - num2;printf("结果: %.2f\n", result);break;case '*':result = num1 * num2;printf("结果: %.2f\n", result); break;case '/':if(num2 != 0)result = num1 / num2;printf("结果: %.2f\n", result); }elseprintf("除数不能为0!\n");}break;default:printf("无效的运算符!\n"); break;}return 0;```这个计算器程序可以接受两个数字和一个运算符作为输入,并根据运算符的类型进行相应的计算。
程序使用了`switch`语句来根据不同的运算符执行不同的操作。
输入数字之后,输出计算结果或者错误提示信息。
VC++编写计算器

VC++写的计算器程序源代码课设了,用vc++写了一个计算器小程序,一个系差不多都用的我的代码,最高兴的是自己可以想到用bool变量来区分整数和小数,还有就是在连续运算的时候我没有用大家都用的复制代码的方法,而是用数组实现了。
有点小兴奋,把代码贴上来,呵呵,望大家多多提意见……严格意义上说这是我的第一个像样的MFC小程序。
(对话框控件变量,消息处理函数的关联以及变量的声明和初始化省略)// jisuanqiDlg.cpp : implementation file//#include "stdafx.h"#include "jisuanqi.h"#include "jisuanqiDlg.h"#include "math.h"#ifdef _DEBUG#define new DEBUG_NEW#undef THIS_FILEstatic char THIS_FILE[] = __FILE__;#endif///////////////////////////////////////////////////////////////////////////// // CAboutDlg dialog used for App Aboutclass CAboutDlg : public CDialog{public:CAboutDlg();// Dialog Data//{{AFX_DATA(CAboutDlg)enum { IDD = IDD_ABOUTBOX };//}}AFX_DATA// ClassWizard generated virtual function overrides//{{AFX_VIRTUAL(CAboutDlg)protected:virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support//}}AFX_VIRTUAL// Implementationprotected://{{AFX_MSG(CAboutDlg)//}}AFX_MSGDECLARE_MESSAGE_MAP()};CAboutDlg::CAboutDlg() : CDialog(CAboutDlg::IDD){//{{AFX_DATA_INIT(CAboutDlg)//}}AFX_DATA_INIT}void CAboutDlg::DoDataExchange(CDataExchange* pDX){CDialog::DoDataExchange(pDX);//{{AFX_DATA_MAP(CAboutDlg)//}}AFX_DATA_MAP}BEGIN_MESSAGE_MAP(CAboutDlg, CDialog)//{{AFX_MSG_MAP(CAboutDlg)// No message handlers//}}AFX_MSG_MAPEND_MESSAGE_MAP()///////////////////////////////////////////////////////////////////////////// // CJisuanqiDlg dialogCJisuanqiDlg::CJisuanqiDlg(CWnd* pParent ): CDialog(CJisuanqiDlg::IDD, pParent){//{{AFX_DATA_INIT(CJisuanqiDlg)m_num = 0.0;//}}AFX_DATA_INIT// Note that LoadIcon does not require a subsequent DestroyIcon in Win32m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);}void CJisuanqiDlg::DoDataExchange(CDataExchange* pDX){CDialog::DoDataExchange(pDX);//{{AFX_DATA_MAP(CJisuanqiDlg)DDX_Text(pDX, IDC_EDIT1, m_num);//}}AFX_DATA_MAP}BEGIN_MESSAGE_MAP(CJisuanqiDlg, CDialog)//{{AFX_MSG_MAP(CJisuanqiDlg)ON_WM_SYSCOMMAND()ON_WM_PAINT()ON_WM_QUERYDRAGICON()ON_BN_CLICKED(IDC_BUTTON1, OnButton1)ON_BN_CLICKED(IDC_BUTTON2, OnButton2)ON_BN_CLICKED(IDC_BUTTON3, OnButton3)ON_BN_CLICKED(IDC_BUTTON4, OnButton4)ON_BN_CLICKED(IDC_BUTTON5, OnButton5)ON_BN_CLICKED(IDC_BUTTON6, OnButton6)ON_BN_CLICKED(IDC_BUTTON7, OnButton7)ON_BN_CLICKED(IDC_BUTTON8, OnButton8)ON_BN_CLICKED(IDC_BUTTON9, OnButton9)ON_BN_CLICKED(IDC_BUTTON14, OnButton0)ON_BN_CLICKED(IDC_BUTTON15, OnButtonPoint)ON_BN_CLICKED(IDC_BUTTON16, OnButtonEqual)ON_BN_CLICKED(IDC_BUTTON13, OnButtonChu)ON_BN_CLICKED(IDC_BUTTON12, OnButtonMul)ON_BN_CLICKED(IDC_BUTTON11, OnButtonSub)ON_BN_CLICKED(IDC_BUTTON10, OnButtonAdd)ON_BN_CLICKED(IDC_BUTTON17, OnButtondelet)ON_BN_CLICKED(IDC_BUTTON18, OnButtonclear)ON_BN_CLICKED(IDC_BUTTON19, OnButtonkaifang)ON_BN_CLICKED(IDC_BUTTON20, OnButtonziranduishu)ON_BN_CLICKED(IDC_BUTTON21, OnButtonchangyongduishu)//}}AFX_MSG_MAPEND_MESSAGE_MAP()///////////////////////////////////////////////////////////////////////////// // CJisuanqiDlg message handlersBOOL CJisuanqiDlg::OnInitDialog()//初始化变量{CDialog::OnInitDialog();// Add "About..." menu item to system menu.// IDM_ABOUTBOX must be in the system command range.ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);ASSERT(IDM_ABOUTBOX < 0xF000);CMenu* pSysMenu = GetSystemMenu(FALSE);if (pSysMenu != NULL){CString strAboutMenu;strAboutMenu.LoadString(IDS_ABOUTBOX);if (!strAboutMenu.IsEmpty()){pSysMenu->AppendMenu(MF_SEPARATOR);pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);}}// Set the icon for this dialog. The framework does this automatically // when the application's main window is not a dialogSetIcon(m_hIcon, TRUE); // Set big iconSetIcon(m_hIcon, FALSE); // Set small icon// TODO: Add extra initialization heret=true;j=true;i=10;p=0;q=0;m_num=0;m_lnum=0;return TRUE; // return TRUE unless you set the focus to a control}void CJisuanqiDlg::OnSysCommand(UINT nID, LPARAM lParam){if ((nID & 0xFFF0) == IDM_ABOUTBOX){CAboutDlg dlgAbout;dlgAbout.DoModal();}else{CDialog::OnSysCommand(nID, lParam);}}// If you add a minimize button to your dialog, you will need the code below // to draw the icon. For MFC applications using the document/view model, // this is automatically done for you by the framework.void CJisuanqiDlg::OnPaint(){if (IsIconic()){CPaintDC dc(this); // device context for paintingSendMessage(WM_ICONERASEBKGND, (WPARAM) dc.GetSafeHdc(), 0);// Center icon in client rectangleint cxIcon = GetSystemMetrics(SM_CXICON);int cyIcon = GetSystemMetrics(SM_CYICON);CRect rect;GetClientRect(&rect);int x = (rect.Width() - cxIcon + 1) / 2;int y = (rect.Height() - cyIcon + 1) / 2;// Draw the icondc.DrawIcon(x, y, m_hIcon);}else{CDialog::OnPaint();}// The system calls this to obtain the cursor to display while the user drags // the minimized window.HCURSOR CJisuanqiDlg::OnQueryDragIcon(){return (HCURSOR) m_hIcon;}void CJisuanqiDlg::OnButton1(){// TODO: Add your control notification handler code hereif(t){m_num=m_num*10+1;UpdateData(FALSE);}else{m_num=m_num+1.0/i;i*=10;UpdateData(FALSE);}}void CJisuanqiDlg::OnButton2(){// TODO: Add your control notification handler code hereif(t){m_num=m_num*10+2;UpdateData(FALSE);else{m_num=m_num+2.0/i;i*=10;UpdateData(FALSE);}}void CJisuanqiDlg::OnButton3(){// TODO: Add your control notification handler code hereif(t){m_num=m_num*10+3;UpdateData(FALSE);}else{m_num=m_num+3.0/i;i*=10;UpdateData(FALSE);}}void CJisuanqiDlg::OnButton4(){// TODO: Add your control notification handler code hereif(t){m_num=m_num*10+4;UpdateData(FALSE);else{m_num=m_num+4.0/i;i*=10;UpdateData(FALSE);}}void CJisuanqiDlg::OnButton5(){// TODO: Add your control notification handler code hereif(t){m_num=m_num*10+5;UpdateData(FALSE);}else{m_num=m_num+5.0/i;i*=10;UpdateData(FALSE);}}void CJisuanqiDlg::OnButton6(){// TODO: Add your control notification handler code hereif(t){m_num=m_num*10+6;UpdateData(FALSE);else{m_num=m_num+6.0/i;i*=10;UpdateData(FALSE);}}void CJisuanqiDlg::OnButton7(){// TODO: Add your control notification handler code hereif(t){m_num=m_num*10+7;UpdateData(FALSE);}else{m_num=m_num+7.0/i;i*=10;UpdateData(FALSE);}}void CJisuanqiDlg::OnButton8(){// TODO: Add your control notification handler code hereif(t){m_num=m_num*10+8;UpdateData(FALSE);else{m_num=m_num+8.0/i;i*=10;UpdateData(FALSE);}}void CJisuanqiDlg::OnButton9(){// TODO: Add your control notification handler code hereif(t){m_num=m_num*10+9;UpdateData(FALSE);}else{m_num=m_num+9.0/i;i*=10;UpdateData(FALSE);}}void CJisuanqiDlg::OnButton0(){// TODO: Add your control notification handler code here //UpdateData();if(t){m_num=m_num*10+0;UpdateData(FALSE);}else{m_num=m_num+0.0/i;i*=10;UpdateData(FALSE);}}void CJisuanqiDlg::OnButtonPoint(){// TODO: Add your control notification handler code here int i=10;t=false;}void CJisuanqiDlg::OnButtonEqual(){// TODO: Add your control notification handler code hereswitch(r){case '+':{m_num=m_num+m_lnum;UpdateData(FALSE);break;}case '-':{m_num=m_snum-m_num;UpdateData(FALSE);break;}case '*':{m_num=m_mnum*m_num;UpdateData(FALSE);break;}case '/':{if(m_num==0){MessageBox("除数不能是0!");}else{m_num=m_cnum/m_num;UpdateData(FALSE);break;}}}t=true;}void CJisuanqiDlg::OnButtonMul(){// TODO: Add your control notification handler code here r='*';t=true;m_mnum=m_num;m_num=0;UpdateData(FALSE);}void CJisuanqiDlg::OnButtonChu(){// TODO: Add your control notification handler code here r='/';t=true;i=10;m_cnum=m_num;m_num=0;UpdateData(FALSE);}void CJisuanqiDlg::OnButtonSub(){// TODO: Add your control notification handler code here r='-';i=10;t=true;if(j){m_snum=m_num;}else{p=0;adda[p]=m_num;p++;for(q=0;q<=p;q++){m_lnum=m_lnum+adda[q];q++;}m_num=m_lnum;UpdateData(FALSE);m_num=0;m_snum=m_lnum;}m_num=0;}void CJisuanqiDlg::OnButtonAdd(){// TODO: Add your control notification handler code here r='+';t=true;j=false;i=10;p=0;adda[p]=m_num;p++;for(q=0;q<=p;q++){m_lnum=m_lnum+adda[q];q++;}m_num=m_lnum;UpdateData(FALSE);m_num=0;}void CJisuanqiDlg::OnButtondelet(){// TODO: Add your control notification handler code here int p;p=m_num/10;m_num=p;UpdateData(FALSE);}void CJisuanqiDlg::OnButtonclear(){// TODO: Add your control notification handler code here t=true;i=10;j=true;m_num=0;m_lnum=0;UpdateData(FALSE);}void CJisuanqiDlg::OnButtonkaifang(){// TODO: Add your control notification handler code herem_num=sqrt(m_num);UpdateData(FALSE);}void CJisuanqiDlg::OnButtonziranduishu(){// TODO: Add your control notification handler code here m_num=log(m_num);UpdateData(FALSE);}void CJisuanqiDlg::OnButtonchangyongduishu(){// TODO: Add your control notification handler code here m_num=log10(m_num);UpdateData(FALSE);}。
VC 计算器程序 代码及设计思想
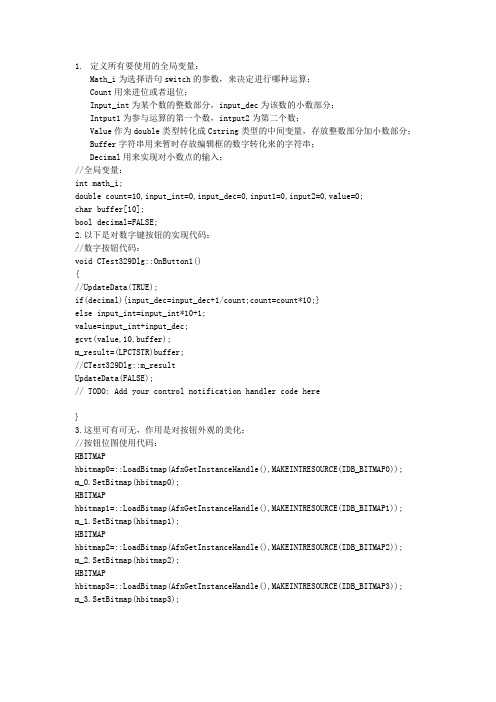
1.定义所有要使用的全局变量:Math_i为选择语句switch的参数,来决定进行哪种运算;Count用来进位或者退位;Input_int为某个数的整数部分,input_dec为该数的小数部分;Intput1为参与运算的第一个数,intput2为第二个数;Value作为double类型转化成Cstring类型的中间变量,存放整数部分加小数部分;Buffer字符串用来暂时存放编辑框的数字转化来的字符串;Decimal用来实现对小数点的输入;//全局变量:int math_i;double count=10,input_int=0,input_dec=0,input1=0,input2=0,value=0;char buffer[10];bool decimal=FALSE;2.以下是对数字键按钮的实现代码://数字按钮代码:void CTest329Dlg::OnButton1(){//UpdateData(TRUE);if(decimal){input_dec=input_dec+1/count;count=count*10;}else input_int=input_int*10+1;value=input_int+input_dec;gcvt(value,10,buffer);m_result=(LPCTSTR)buffer;//CTest329Dlg::m_resultUpdateData(FALSE);// TODO: Add your control notification handler code here}3.这里可有可无,作用是对按钮外观的美化://按钮位图使用代码:HBITMAPhbitmap0=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAP0)); m_0.SetBitmap(hbitmap0);HBITMAPhbitmap1=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAP1)); m_1.SetBitmap(hbitmap1);HBITMAPhbitmap2=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAP2)); m_2.SetBitmap(hbitmap2);HBITMAPhbitmap3=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAP3)); m_3.SetBitmap(hbitmap3);HBITMAPhbitmap4=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAP4));m_4.SetBitmap(hbitmap4);HBITMAPhbitmap5=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAP5));m_5.SetBitmap(hbitmap5);HBITMAPhbitmap6=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAP6));m_6.SetBitmap(hbitmap6);HBITMAPhbitmap7=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAP7));m_7.SetBitmap(hbitmap7);HBITMAPhbitmap8=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAP8));m_8.SetBitmap(hbitmap8);HBITMAPhbitmap9=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAP9));m_9.SetBitmap(hbitmap9);HBITMAPhbitmapjia=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAPjia)); m_jia.SetBitmap(hbitmapjia);HBITMAPhbitmapjian=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAPjian) );m_jian.SetBitmap(hbitmapjian);HBITMAPhbitmapcheng=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAPchen g));m_cheng.SetBitmap(hbitmapcheng);HBITMAPhbitmapchu=::LoadBitmap(AfxGetInstanceHandle(),MAKEINTRESOURCE(IDB_BITMAPchu)); m_chu.SetBitmap(hbitmapchu);4.等号键的实现代码。
计算器编程c语言
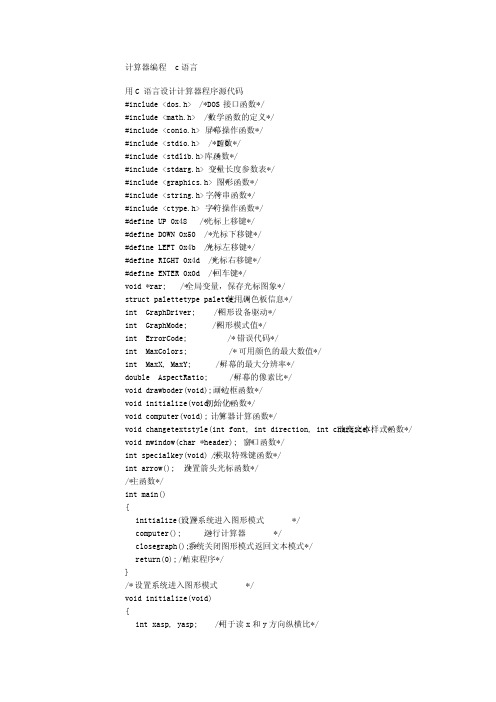
计算器编程 c语言用C语言设计计算器程序源代码#include <dos.h> /*DOS接口函数*/#include <math.h> /*数学函数的定义*/#include <conio.h> /*屏幕操作函数*/函数*/#include <stdio.h> /*I/O#include <stdlib.h> /*库函数*/变量长度参数表*/#include <stdarg.h> /*图形函数*/#include <graphics.h> /*字符串函数*/#include <string.h> /*字符操作函数*/#include <ctype.h> /*#define UP 0x48 /*光标上移键*/#define DOWN 0x50 /*光标下移键*/#define LEFT 0x4b /*光标左移键*/#define RIGHT 0x4d /*光标右移键*/#define ENTER 0x0d /*回车键*/void *rar; /*全局变量,保存光标图象*/使用调色板信息*/struct palettetype palette; /*int GraphDriver; /* 图形设备驱动*/int GraphMode; /* 图形模式值*/int ErrorCode; /* 错误代码*/int MaxColors; /* 可用颜色的最大数值*/int MaxX, MaxY; /* 屏幕的最大分辨率*/double AspectRatio; /* 屏幕的像素比*/void drawboder(void); /*画边框函数*/初始化函数*/void initialize(void); /*计算器计算函数*/void computer(void); /*改变文本样式函数*/ void changetextstyle(int font, int direction, int charsize); /*窗口函数*/void mwindow(char *header); /*/*获取特殊键函数*/int specialkey(void) ;设置箭头光标函数*//*int arrow();/*主函数*/int main(){设置系统进入图形模式 */initialize();/*运行计算器 */computer(); /*系统关闭图形模式返回文本模式*/closegraph();/*/*结束程序*/return(0);}/* 设置系统进入图形模式 */void initialize(void){int xasp, yasp; /* 用于读x和y方向纵横比*/GraphDriver = DETECT; /* 自动检测显示器*/initgraph( &GraphDriver, &GraphMode, "" );/*初始化图形系统*/ErrorCode = graphresult(); /*读初始化结果*/如果初始化时出现错误*/if( ErrorCode != grOk ) /*{printf("Graphics System Error: %s\n",显示错误代码*/grapherrormsg( ErrorCode ) ); /*退出*/exit( 1 ); /*}getpalette( &palette ); /* 读面板信息*/MaxColors = getmaxcolor() + 1; /* 读取颜色的最大值*/MaxX = getmaxx(); /* 读屏幕尺寸 */MaxY = getmaxy(); /* 读屏幕尺寸 */getaspectratio( &xasp, &yasp ); /* 拷贝纵横比到变量中*/计算纵横比值*/ AspectRatio = (double)xasp/(double)yasp;/*}/*计算器函数*/void computer(void){定义视口类型变量*/struct viewporttype vp; /*int color, height, width;int x, y,x0,y0, i, j,v,m,n,act,flag=1;操作数和计算结果变量*/float num1=0,num2=0,result; /*char cnum[5],str2[20]={""},c,temp[20]={""};定义字符串在按钮图形上显示的符号 char str1[]="1230.456+-789*/Qc=^%";/**/mwindow( "Calculator" ); /*显示主窗口 */设置灰颜色值*//*color = 7;getviewsettings( &vp ); /* 读取当前窗口的大小*/width=(vp.right+1)/10; /* 设置按钮宽度 */设置按钮高度 */height=(vp.bottom-10)/10 ; /*/*设置x的坐标值*/x = width /2;设置y的坐标值*/y = height/2; /*setfillstyle(SOLID_FILL, color+3);bar( x+width*2, y, x+7*width, y+height );/*画一个二维矩形条显示运算数和结果*/setcolor( color+3 ); /*设置淡绿颜色边框线*/rectangle( x+width*2, y, x+7*width, y+height );/*画一个矩形边框线*/设置颜色为红色*/setcolor(RED); /*输出字符串"0."*/outtextxy(x+3*width,y+height/2,"0."); /*/*设置x的坐标值*/x =2*width-width/2;设置y的坐标值*/y =2*height+height/2; /*画按钮*/for( j=0 ; j<4 ; ++j ) /*{for( i=0 ; i<5 ; ++i ){setfillstyle(SOLID_FILL, color);setcolor(RED);bar( x, y, x+width, y+height ); /*画一个矩形条*/rectangle( x, y, x+width, y+height );sprintf(str2,"%c",str1[j*5+i]);/*将字符保存到str2中*/outtextxy( x+(width/2), y+height/2, str2);移动列坐标*/x =x+width+ (width / 2) ;/*}y +=(height/2)*3; /* 移动行坐标*/x =2*width-width/2; /*复位列坐标*/}x0=2*width;y0=3*height;x=x0;y=y0;gotoxy(x,y); /*移动光标到x,y位置*/显示光标*/arrow(); /*putimage(x,y,rar,XOR_PUT);m=0;n=0;设置str2为空串*/strcpy(str2,""); /*当压下Alt+x键结束程序,否则执行下面的循环while((v=specialkey())!=45) /**/{当压下键不是回车时*/while((v=specialkey())!=ENTER) /*{putimage(x,y,rar,XOR_PUT); /*显示光标图象*/if(v==RIGHT) /*右移箭头时新位置计算*/if(x>=x0+6*width)如果右移,移到尾,则移动到最左边字符位置*//*{x=x0;m=0;}else{x=x+width+width/2;m++;否则,右移到下一个字符位置*/} /*if(v==LEFT) /*左移箭头时新位置计算*/if(x<=x0){x=x0+6*width;m=4;} /*如果移到头,再左移,则移动到最右边字符位置*/else{x=x-width-width/2;m--;} /*否则,左移到前一个字符位置*/if(v==UP) /*上移箭头时新位置计算*/if(y<=y0){y=y0+4*height+height/2;n=3;} /*如果移到头,再上移,则移动到最下边字符位置*/else{y=y-height-height/2;n--;} /*否则,移到上边一个字符位置*/if(v==DOWN) /*下移箭头时新位置计算*/if(y>=7*height){ y=y0;n=0;} /*如果移到尾,再下移,则移动到最上边字符位置*/else{y=y+height+height/2;n++;} /*否则,移到下边一个字符位置*/putimage(x,y,rar,XOR_PUT); /*在新的位置显示光标箭头*/ }将字符保存到变量c中*/c=str1[n*5+m]; /*判断是否是数字或小数点*/if(isdigit(c)||c=='.') /*{如果标志为-1,表明为负数*/if(flag==-1) /*{将负号连接到字符串中*/strcpy(str2,"-"); /*flag=1;} /*将标志值恢复为1*/将字符保存到字符串变量temp中*/ sprintf(temp,"%c",c); /*将temp中的字符串连接到str2中*/strcat(str2,temp); /*setfillstyle(SOLID_FILL,color+3);bar(2*width+width/2,height/2,15*width/2,3*height/2);显示字符串*/outtextxy(5*width,height,str2); /*}if(c=='+'){将第一个操作数转换为浮点数*/num1=atof(str2); /*将str2清空*/strcpy(str2,""); /*做计算加法标志值*/act=1; /*setfillstyle(SOLID_FILL,color+3);bar(2*width+width/2,height/2,15*width/2,3*height/2);显示字符串*/outtextxy(5*width,height,"0."); /*}if(c=='-'){如果str2为空,说明是负号,而不是减号*/ if(strcmp(str2,"")==0) /*设置负数标志*/flag=-1; /*else{将第二个操作数转换为浮点数*/num1=atof(str2); /*将str2清空*/strcpy(str2,""); /*act=2; /*做计算减法标志值*/setfillstyle(SOLID_FILL,color+3);画矩形*/ bar(2*width+width/2,height/2,15*width/2,3*height/2); /*显示字符串*/outtextxy(5*width,height,"0."); /*}}if(c=='*'){将第二个操作数转换为浮点数*/num1=atof(str2); /*strcpy(str2,""); /*将str2清空*/做计算乘法标志值*/act=3; /*setfillstyle(SOLID_FILL,color+3); bar(2*width+width/2,height/2,15*width /2,3*height/2);显示字符串*/outtextxy(5*width,height,"0."); /*}if(c=='/'){将第二个操作数转换为浮点数*/num1=atof(str2); /*strcpy(str2,""); /*将str2清空*/做计算除法标志值*/act=4; /*setfillstyle(SOLID_FILL,color+3);bar(2*width+width/2,height/2,15*width/2,3*height/2);outtextxy(5*width,height,"0."); /*显示字符串*/}if(c=='^'){将第二个操作数转换为浮点数*/num1=atof(str2); /*将str2清空*/strcpy(str2,""); /*做计算乘方标志值*/act=5; /*设置用淡绿色实体填充*/ setfillstyle(SOLID_FILL,color+3); /*画矩形*/ bar(2*width+width/2,height/2,15*width/2,3*height/2); /*显示字符串*/outtextxy(5*width,height,"0."); /*}if(c=='%'){将第二个操作数转换为浮点数*/num1=atof(str2); /*strcpy(str2,""); /*将str2清空*/做计算模运算乘方标志值*/act=6; /*setfillstyle(SOLID_FILL,color+3); /*设置用淡绿色实体填充*/画矩形*/ bar(2*width+width/2,height/2,15*width/2,3*height/2); /*显示字符串*/outtextxy(5*width,height,"0."); /*}if(c=='='){将第二个操作数转换为浮点数*/num2=atof(str2); /*根据运算符号计算*/switch(act) /*{case 1:result=num1+num2;break; /*做加法*/case 2:result=num1-num2;break; /*做减法*/case 3:result=num1*num2;break; /*做乘法*/case 4:result=num1/num2;break; /*做除法*/case 5:result=pow(num1,num2);break; /*做x的y次方*/case 6:result=fmod(num1,num2);break; /*做模运算*/ }设置用淡绿色实体填充*/ setfillstyle(SOLID_FILL,color+3); /*覆盖结果区*/ bar(2*width+width/2,height/2,15*width/2,3*height/2); /*将结果保存到temp中*/sprintf(temp,"%f",result); /*outtextxy(5*width,height,temp); /*显示结果*/}if(c=='c'){num1=0; /*将两个操作数复位0,符号标志为1*/num2=0;flag=1;strcpy(str2,""); /*将str2清空*/设置用淡绿色实体填充*/ setfillstyle(SOLID_FILL,color+3); /*覆盖结果区*/ bar(2*width+width/2,height/2,15*width/2,3*height/2); /*显示字符串*/outtextxy(5*width,height,"0."); /*}如果选择了q回车,结束计算程序*/if(c=='Q')exit(0); /*}putimage(x,y,rar,XOR_PUT); /*在退出之前消去光标箭头*/返回*/return; /*}/*窗口函数*/void mwindow( char *header ){int height;cleardevice(); /* 清除图形屏幕 */setcolor( MaxColors - 1 ); /* 设置当前颜色为白色*//* 设置视口大小 */ setviewport( 20, 20, MaxX/2, MaxY/2, 1 );height = textheight( "H" ); /* 读取基本文本大小 */settextstyle( DEFAULT_FONT, HORIZ_DIR, 1 );/*设置文本样式*/settextjustify( CENTER_TEXT, TOP_TEXT );/*设置字符排列方式*/输出标题*/outtextxy( MaxX/4, 2, header ); /*setviewport( 20,20+height+4, MaxX/2+4, MaxY/2+20, 1 ); /*设置视口大小*/ 画边框*/drawboder(); /*}画边框*/void drawboder(void) /*{定义视口类型变量*/struct viewporttype vp; /*setcolor( MaxColors - 1 ); /*设置当前颜色为白色 */setlinestyle( SOLID_LINE, 0, NORM_WIDTH );/*设置画线方式*/将当前视口信息装入vp所指的结构中*/getviewsettings( &vp );/*画矩形边框*/rectangle( 0, 0, vp.right-vp.left, vp.bottom-vp.top ); /*}/*设计鼠标图形函数*/int arrow(){int size;定义多边形坐标*/int raw[]={4,4,4,8,6,8,14,16,16,16,8,6,8,4,4,4}; /*设置填充模式*/setfillstyle(SOLID_FILL,2); /*/*画出一光标箭头*/fillpoly(8,raw);测试图象大小*/size=imagesize(4,4,16,16); /*分配内存区域*/rar=malloc(size); /*存放光标箭头图象*/getimage(4,4,16,16,rar); /*putimage(4,4,rar,XOR_PUT); /*消去光标箭头图象*/return 0;}/*按键函数*/int specialkey(void){int key;等待键盘输入*/while(bioskey(1)==0); /*key=bioskey(0); /*键盘输入*/只取特殊键的扫描值,其余为0*/ key=key&0xff? key&0xff:key>>8; /*return(key); /*返回键值*/}。
c语言计算器加减乘除开方混合计算和清零代码
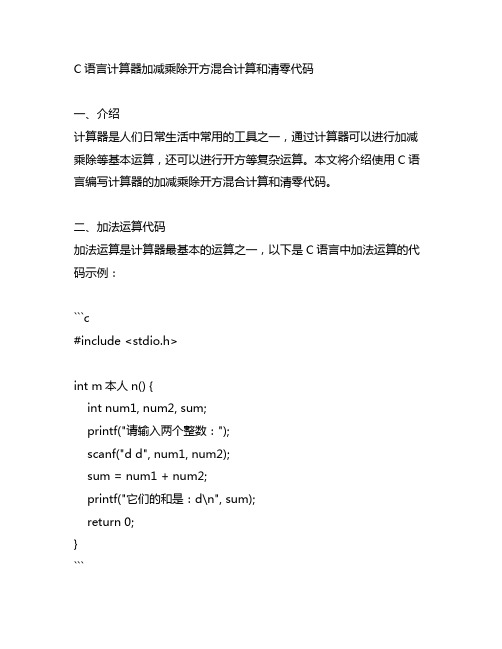
C语言计算器加减乘除开方混合计算和清零代码一、介绍计算器是人们日常生活中常用的工具之一,通过计算器可以进行加减乘除等基本运算,还可以进行开方等复杂运算。
本文将介绍使用C语言编写计算器的加减乘除开方混合计算和清零代码。
二、加法运算代码加法运算是计算器最基本的运算之一,以下是C语言中加法运算的代码示例:```c#include <stdio.h>int m本人n() {int num1, num2, sum;printf("请输入两个整数:");scanf("d d", num1, num2);sum = num1 + num2;printf("它们的和是:d\n", sum);return 0;}```三、减法运算代码接下来是减法运算的C语言代码示例:```c#include <stdio.h>int m本人n() {int num1, num2, diff;printf("请输入两个整数:");scanf("d d", num1, num2);diff = num1 - num2;printf("它们的差是:d\n", diff);return 0;}```四、乘法运算代码乘法运算是计算器中常用的运算之一,以下是C语言中乘法运算的代码示例:```c#include <stdio.h>int m本人n() {int num1, num2, product;printf("请输入两个整数:");scanf("d d", num1, num2);product = num1 * num2;printf("它们的积是:d\n", product);return 0;}```五、除法运算代码除法运算涉及到除数不能为0的情况,以下是C语言中除法运算的代码示例:```c#include <stdio.h>int m本人n() {float num1, num2, quotient;printf("请输入两个数:");scanf("f f", num1, num2);if(num2 != 0) {quotient = num1 / num2;printf("它们的商是:.2f\n", quotient);} else {printf("除数不能为0!\n");}return 0;}```六、开方运算代码开方运算是比较复杂的运算之一,以下是C语言中开方运算的代码示例:```c#include <stdio.h>#include <math.h>int m本人n() {float num, sqrt_num;printf("请输入一个数:");scanf("f", num);if(num >= 0) {sqrt_num = sqrt(num);printf("它的开方是:.2f\n", sqrt_num);} else {printf("输入的数不能为负数!\n");}return 0;}```七、混合计算代码有时候计算器需要进行混合运算,即在一个表达式中包含多种运算,以下是C语言中混合计算的代码示例:```c#include <stdio.h>int m本人n() {float num1, num2, result;char operator;printf("请输入一个表达式(如:2+3*4):");scanf("f c f", num1, operator, num2);switch(operator) {case '+':result = num1 + num2;break;case '-':result = num1 - num2;break;case '*':result = num1 * num2;break;case '/':if(num2 != 0) {result = num1 / num2;} else {printf("除数不能为0!\n"); return 1;}break;default:printf("无效的运算符!\n"); return 1;}printf("结果是:.2f\n", result);return 0;}```八、清零代码最后一个功能是清零,即将计算器上的结果归零,以下是C语言中清零的代码示例:```c#include <stdio.h>int m本人n() {float result = 0;printf("当前结果为:.2f\n", result);char choice;printf("是否清零?(Y/N):");scanf(" c", choice);if(choice == 'Y' || choice == 'y') {result = 0;printf("已清零,当前结果为:.2f\n", result);} else if(choice == 'N' || choice == 'n') {printf("未清零,当前结果为:.2f\n", result);} else {printf("无效的输入!\n");}return 0;}```九、结论在本文中,我们以C语言的形式介绍了计算器的加减乘除开方混合计算和清零代码。
用c语言编写的计算器源代码
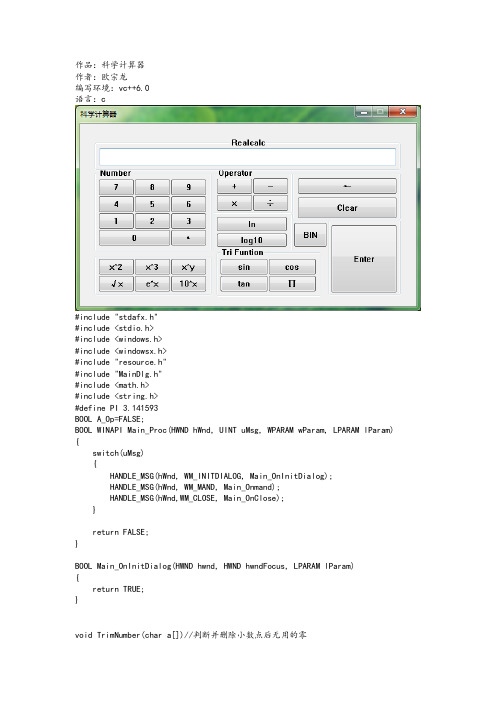
作品:科学计算器作者:欧宗龙编写环境:vc++6.0语言:c#include "stdafx.h"#include <stdio.h>#include <windows.h>#include <windowsx.h>#include "resource.h"#include "MainDlg.h"#include <math.h>#include <string.h>#define PI 3.141593BOOL A_Op=FALSE;BOOL WINAPI Main_Proc(HWND hWnd, UINT uMsg, WPARAM wParam, LPARAM lParam) {switch(uMsg){HANDLE_MSG(hWnd, WM_INITDIALOG, Main_OnInitDialog);HANDLE_MSG(hWnd, WM_MAND, Main_Onmand);HANDLE_MSG(hWnd,WM_CLOSE, Main_OnClose);}return FALSE;}BOOL Main_OnInitDialog(HWND hwnd, HWND hwndFocus, LPARAM lParam){return TRUE;}void TrimNumber(char a[])//判断并删除小数点后无用的零for(unsigned i=0;i<strlen(a);i++){if(a[i]=='.'){for(unsigned j=strlen(a)-1;j>=i;j--){if(a[j]=='0'){a[j]='\0';}else if(a[j]=='.'){a[j]='\0';}else break;}}}}double Operate(char Operator,double n1,double n2) //判断符号,进行相应的运算{if(Operator=='0'){}if(Operator=='+'){n2+=n1;}if(Operator=='-'){n2=n1-n2;}if(Operator=='*'){n2*=n1;}if(Operator=='/'){n2=n1/n2;}if(Operator=='^'){n2=pow(n1,n2);}return n2;}////////////////////////////////////////////////void IntBinary(char a[],int n){if(n>1)IntBinary(a,n/2);sprintf(a,"%s%i",a,n%2);}void decimal(char a[],double m){if(m>0.000001){m=m*2;sprintf(a,"%s%d",a,(long)m);decimal(a,m-(long)m);}}void Binary(char a[],double Num){char DecP[256]="";double x,y;double *iptr=&y;x=modf(Num,iptr);decimal(DecP,x);IntBinary(a,(int)y);strcat(a,".");strcat(a,DecP);}////////////////////////////////////void Main_Onmand(HWND hwnd, int id, HWND hwndCtl, UINT codeNotify) {static DELTIMES=0;static char str[256];static char Operator='0';static double RNum[3];switch(id){case IDC_BUTTONN1://数字1{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));strcat(str,"1");SetDlgItemText(hwnd,IDC_EDIT,str);RNum[1]=atof(str);A_Op=FALSE;}break;case IDC_BUTTONN2://数字2{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));strcat(str,"2");SetDlgItemText(hwnd,IDC_EDIT,str);RNum[1]=atof(str);A_Op=FALSE;}break;case IDC_BUTTONN3://数字3{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));strcat(str,"3");SetDlgItemText(hwnd,IDC_EDIT,str);RNum[1]=atof(str);A_Op=FALSE;}break;case IDC_BUTTONN4://数字4{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));strcat(str,"4");SetDlgItemText(hwnd,IDC_EDIT,str);RNum[1]=atof(str);A_Op=FALSE;}break;case IDC_BUTTONN5://数字5{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));strcat(str,"5");SetDlgItemText(hwnd,IDC_EDIT,str);RNum[1]=atof(str);A_Op=FALSE;}break;case IDC_BUTTONN6://数字6{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));strcat(str,"6");SetDlgItemText(hwnd,IDC_EDIT,str);RNum[1]=atof(str);A_Op=FALSE;}break;case IDC_BUTTONN7://数字7{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));strcat(str,"7");SetDlgItemText(hwnd,IDC_EDIT,str);RNum[1]=atof(str);A_Op=FALSE;}break;case IDC_BUTTONN8://数字8{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));strcat(str,"8");SetDlgItemText(hwnd,IDC_EDIT,str);RNum[1]=atof(str);A_Op=FALSE;}break;case IDC_BUTTONN9://数字9{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));strcat(str,"9");SetDlgItemText(hwnd,IDC_EDIT,str);RNum[1]=atof(str);A_Op=FALSE;}break;case IDC_BUTTONN0://数字0{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));strcat(str,"0");SetDlgItemText(hwnd,IDC_EDIT,str);RNum[1]=atof(str);A_Op=FALSE;}break;case IDC_BUTTONDEL://小数点.del{if(A_Op){SetDlgItemText(hwnd,IDC_EDIT,NULL);}GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));if(DELTIMES==0){strcat(str,".");}DELTIMES++;SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=FALSE;}break;case IDC_BUTTONADD: //加法运算{RNum[1]=atof(str);RNum[0]=RNum[1];RNum[1]=RNum[2];RNum[2]=Operate(Operator,RNum[1],RNum[0]);sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);Operator='+';DELTIMES=0;A_Op=TRUE;}break;case IDC_BUTTONSUB: //减法运算{RNum[1]=atof(str);RNum[0]=RNum[1];RNum[1]=RNum[2];RNum[2]=Operate(Operator,RNum[1],RNum[0]);sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);DELTIMES=0;A_Op=TRUE;Operator='-';}break;case IDC_BUTTONMUL: //乘法运算{RNum[1]=atof(str);RNum[0]=RNum[1];RNum[1]=RNum[2];RNum[2]=Operate(Operator,RNum[1],RNum[0]);sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);Operator='*';DELTIMES=0;A_Op=TRUE;}break;case IDC_BUTTONDIV: //除法运算{RNum[1]=atof(str);RNum[0]=RNum[1];RNum[1]=RNum[2];RNum[2]=Operate(Operator,RNum[1],RNum[0]);sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);Operator='/';DELTIMES=0;A_Op=TRUE;}break;case IDC_BUTTONXY://x的y次方{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[1]=atof(str);RNum[0]=RNum[1];RNum[1]=RNum[2];RNum[2]=Operate(Operator,RNum[1],RNum[0]);sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);Operator='^';DELTIMES=0;}break;case IDC_BUTTONPI: //圆周率PI,弧度{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));if(atof(str)!=0){RNum[2]=atof(str)*PI;sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);}else{sprintf(str,"%f",PI);SetDlgItemText(hwnd,IDC_EDIT,str);}A_Op=TRUE;}break;case IDC_BUTTONSQRT: //开根号{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=sqrt(atof(str));sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTONSIN: //三角函数sin函数{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=sin(atof(str));sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTONCOS://三角函数cos函数{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=cos(atof(str));sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTONTAN://三角函数tan函数{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=tan(atof(str));sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTONSQ: //平方{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=atof(str)*atof(str);sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTONCUBE://三次方{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=atof(str)*atof(str)*atof(str);sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTONEX://e的x次方{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=exp(atof(str));sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTON10X://10的x次方{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=pow(10,atof(str));sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTONLN: //ln x{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=log(atof(str));sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTONLOG10: //log10{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=log10(atof(str));sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTONBINARY: //十进制转换为二进制{char a[256]="";GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[2]=atof(str);Binary(a,RNum[2]);strcpy(str,a);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);A_Op=TRUE;}break;case IDC_BUTTONCLEAR://清除数据{DELTIMES=0;Operator='0';RNum[0]=RNum[1]=RNum[2]=0;memset(str,0,sizeof(str));SetDlgItemText(hwnd,IDC_EDIT,NULL);A_Op=FALSE;}break;case IDC_BUTTONBACKSPACE://退格键{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));int i=strlen(str);str[i-1]='\0';SetDlgItemText(hwnd,IDC_EDIT,str);}break;case IDC_ENTER://Enter键{GetDlgItemText(hwnd,IDC_EDIT,str,sizeof(str));RNum[1]=atof(str);RNum[0]=RNum[1];RNum[1]=RNum[2];RNum[2]=Operate(Operator,RNum[1],RNum[0]);sprintf(str,"%f",RNum[2]);TrimNumber(str);SetDlgItemText(hwnd,IDC_EDIT,str);Operator='0';DELTIMES=0;}break;default:break;}}void Main_OnClose(HWND hwnd){EndDialog(hwnd, 0);}本人拙作,如有不足之处请谅解。
C语言简易计算器的实现

C语言简易计算器的实现C语言简易计算器是一种用于进行基本数学运算的程序。
实现一个简易计算器的关键是要能够解析用户输入的数学表达式,并将其转化为计算机可以理解的形式,然后进行计算,并输出结果。
下面是一个大约1200字以上的示例实现。
```c#include <stdio.h>#include <stdlib.h>#include <stdbool.h>#include <ctype.h>#define MAX_SIZE 100//定义操作符的优先级int getPriority(char op)if (op == '+' , op == '-')return 1;else if (op == '*' , op == '/')return 2;elsereturn 0;//进行四则运算int calculate(int a, int b, char op)switch (op)case '+': return a + b;case '-': return a - b;case '*': return a * b;case '/': return a / b;default: return 0;}//将中缀表达式转换为后缀表达式void infixToPostfix(char* infixExp, char* postfixExp) char stack[MAX_SIZE];int top = -1;int j = 0;for (int i = 0; infixExp[i] != '\0'; i++)if (isdigit(infixExp[i])) { // 数字直接输出到后缀表达式while (isdigit(infixExp[i]))postfixExp[j++] = infixExp[i++];}postfixExp[j++] = ' ';i--;}else if (infixExp[i] == '(') { // 左括号压入栈stack[++top] = infixExp[i];}else if (infixExp[i] == ')') { // 右括号弹出栈内所有操作符并输出到后缀表达式,直到遇到左括号while (top != -1 && stack[top] != '(')postfixExp[j++] = stack[top--];postfixExp[j++] = ' ';}top--; // 弹出栈顶的左括号}else { // 操作符while (top != -1 && getPriority(stack[top]) >=getPriority(infixExp[i]))postfixExp[j++] = stack[top--];postfixExp[j++] = ' ';stack[++top] = infixExp[i];}}while (top != -1) { // 将栈内剩余操作符弹出并输出到后缀表达式postfixExp[j++] = stack[top--];postfixExp[j++] = ' ';}postfixExp[j] = '\0';//计算后缀表达式的值int evaluatePostfix(char* postfixExp)char stack[MAX_SIZE];int top = -1;for (int i = 0; postfixExp[i] != '\0'; i++)if (isdigit(postfixExp[i])) { // 数字压入栈int num = 0;while (isdigit(postfixExp[i]))num = num * 10 + (postfixExp[i++] - '0');stack[++top] = num;i--;}else if (postfixExp[i] == ' ')continue;}else { // 操作符,弹出栈顶的两个数进行计算,并将结果压入栈int b = stack[top--];int a = stack[top--];int result = calculate(a, b, postfixExp[i]);stack[++top] = result;}}return stack[top];int maichar infixExp[MAX_SIZE];printf("请输入中缀表达式:");fgets(infixExp, sizeof(infixExp), stdin); // 读取用户输入//将中缀表达式转换为后缀表达式char postfixExp[MAX_SIZE];infixToPostfix(infixExp, postfixExp);printf("后缀表达式为:%s\n", postfixExp);//计算后缀表达式的值并输出int result = evaluatePostfix(postfixExp);printf("计算结果为:%d\n", result);return 0;```这个简易计算器的实现基于栈的数据结构。
计算器VC源代码
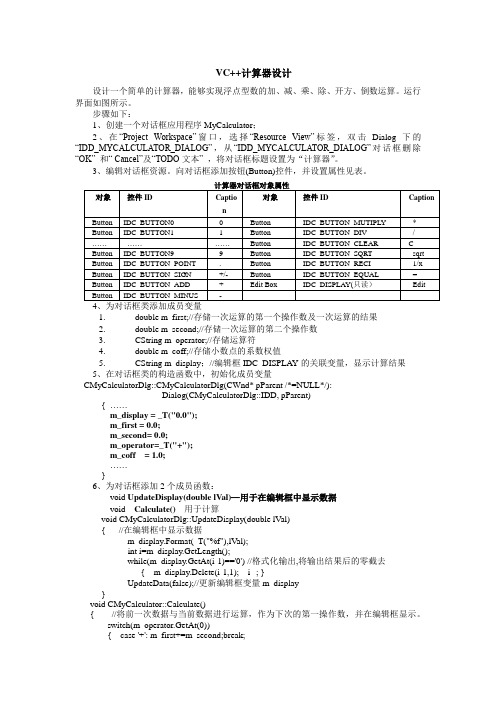
{ //在编辑框中显示数据
m_display.Format(_T("%f"),lVal);
int i=m_display.GetLength();
while(m_display.GetAt(i-1)=='0') //格式化输出,将输出结果后的零截去
/
……
……
……
Button
IDC_BUTTON_CLEAR
C
Button
IDC_BUTTON9
9
Button
IDC_BUTTON_SQRT
sqrt
Button
IDC_BUTTON_POINT
.
Button
IDC_BUTTON_RECI
1/x
Button
IDC_BUTTON_SIGN
+/-
Button
3.CString m_operator;//存储运算符
4.double m_coff;//存储小数点的系数权值
5.CString m_display;//编辑框IDC_DISPLAY的关联变量,显示计算结果
5、在对话框类的构造函数中,初始化成员变量
CMyCalculatorDlg::CMyCalculatorDlg(CWnd* pParent /*=NULL*/): Dialog(CMyCalculatorDlg::IDD, pParent)
{……
m_display = _T("0.0");
m_first = 0.0;
m_second= 0.0;
m_operator=_T("+");
C编写简易计算器附源代码超详细
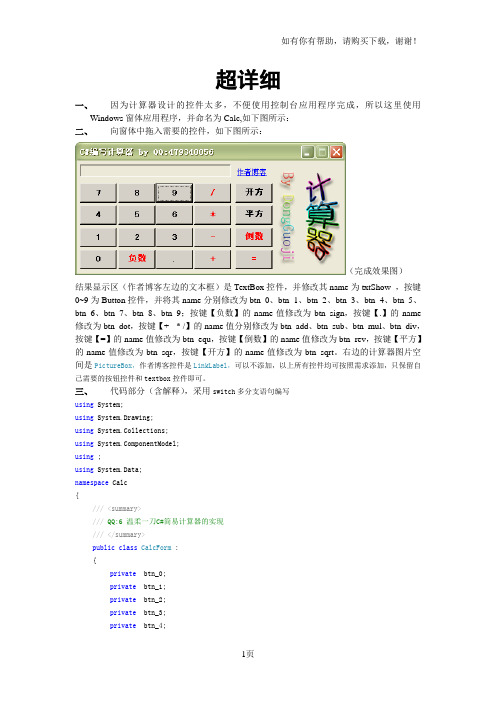
超详细一、因为计算器设计的控件太多,不便使用控制台应用程序完成,所以这里使用Windows窗体应用程序,并命名为Calc,如下图所示:二、向窗体中拖入需要的控件,如下图所示:(完成效果图)结果显示区(作者博客左边的文本框)是TextBox控件,并修改其name为txtShow ,按键0~9为Button控件,并将其name分别修改为btn_0、btn_1、btn_2、btn_3、btn_4、btn_5、btn_6、btn_7、btn_8、btn_9;按键【负数】的name值修改为btn_sign,按键【.】的name 修改为btn_dot,按键【+ - * /】的name值分别修改为btn_add、btn_sub、btn_mul、btn_div,按键【=】的name值修改为btn_equ,按键【倒数】的name值修改为btn_rev,按键【平方】的name值修改为btn_sqr,按键【开方】的name值修改为btn_sqrt。
右边的计算器图片空间是PictureBox,作者博客控件是LinkLabel,可以不添加,以上所有控件均可按照需求添加,只保留自己需要的按钮控件和textbox控件即可。
三、代码部分(含解释),采用switch多分支语句编写using System;using System.Drawing;using System.Collections;using ponentModel;using ;using System.Data;namespace Calc{///<summary>/// QQ:6 温柔一刀C#简易计算器的实现///</summary>public class CalcForm :{private btn_0;private btn_1;private btn_2;private btn_3;private btn_4;private btn_5;private btn_6;private btn_7;private btn_8;private btn_9;private btn_add;private btn_sub;private btn_mul;private btn_div;private btn_sqrt;private btn_sign;private btn_equ;private btn_dot;private btn_rev;private txtShow;private btn_sqr;private PictureBox pictureBox1;private LinkLabel linkLabel1;///<summary>///必需的设计器变量。
VC++设计简易计算器

VC++设计简易计算器// CalculatorDlg.cpp : implementation file//#include "stdafx.h"#include "Calculator.h"#include "CalculatorDlg.h"#ifdef _DEBUG#define new DEBUG_NEW#undef THIS_FILEstatic char THIS_FILE[] = __FILE__;#endifextern MyOperator optrs[14];extern Compare op[14][14];/////////////////////////////////////////////////////////////////// //////////// CAboutDlg dialog used for App Aboutclass CAboutDlg : public CDialog{public:CAboutDlg();// Dialog Data//{{AFX_DATA(CAboutDlg)enum { IDD = IDD_ABOUTBOX };//}}AFX_DATA// ClassWizard generated virtual function overrides//{{AFX_VIRTUAL(CAboutDlg)protected:virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support//}}AFX_VIRTUAL// Implementationprotected://{{AFX_MSG(CAboutDlg)//}}AFX_MSGDECLARE_MESSAGE_MAP()};CAboutDlg::CAboutDlg() : CDialog(CAboutDlg::IDD){//{{AFX_DATA_INIT(CAboutDlg)//}}AFX_DATA_INIT}void CAboutDlg::DoDataExchange(CDataExchange* pDX){CDialog::DoDataExchange(pDX);//{{AFX_DATA_MAP(CAboutDlg)//}}AFX_DATA_MAP}BEGIN_MESSAGE_MAP(CAboutDlg, CDialog)//{{AFX_MSG_MAP(CAboutDlg)// No message handlers//}}AFX_MSG_MAPEND_MESSAGE_MAP()/////////////////////////////////////////////////////////////////// //////////// CCalculatorDlg dialogCCalculatorDlg::CCalculatorDlg(CWnd* pParent /*=NULL*/) : CDialog(CCalculatorDlg::IDD, pParent){//{{AFX_DATA_INIT(CCalculatorDlg)m_strResult = _T("");//}}AFX_DATA_INIT// Note that LoadIcon does not require a subsequent DestroyIcon in Win32m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);}void CCalculatorDlg::DoDataExchange(CDataExchange* pDX) {CDialog::DoDataExchange(pDX);//{{AFX_DATA_MAP(CCalculatorDlg)DDX_Control(pDX, IDC_EDIT_RESULT, m_editResult);DDX_Text(pDX, IDC_EDIT_RESULT, m_strResult);//}}AFX_DATA_MAP}BEGIN_MESSAGE_MAP(CCalculatorDlg, CDialog)//{{AFX_MSG_MAP(CCalculatorDlg)ON_WM_SYSCOMMAND()ON_WM_PAINT()ON_WM_QUERYDRAGICON()ON_BN_CLICKED(IDC_EQUAL, OnEqual)ON_BN_CLICKED(IDC_NUM_1, OnNum1)ON_BN_CLICKED(IDC_NUM_0, OnNum0)ON_BN_CLICKED(IDC_NUM_2, OnNum2)ON_BN_CLICKED(IDC_NUM_3, OnNum3)ON_BN_CLICKED(IDC_NUM_4, OnNum4)ON_BN_CLICKED(IDC_NUM_5, OnNum5)ON_BN_CLICKED(IDC_NUM_6, OnNum6)ON_BN_CLICKED(IDC_NUM_7, OnNum7)ON_BN_CLICKED(IDC_NUM_8, OnNum8)ON_BN_CLICKED(IDC_NUM_9, OnNum9)ON_BN_CLICKED(IDC_POW, OnPow)ON_BN_CLICKED(IDC_SIN, OnSin)ON_BN_CLICKED(IDC_TAN, OnTan)ON_BN_CLICKED(IDC_NEGATIVE, OnNegative) ON_BN_CLICKED(IDC_MUT, OnMut)ON_BN_CLICKED(IDC_LOG, OnLog)ON_BN_CLICKED(IDC_LN, OnLn)ON_BN_CLICKED(IDC_DIV, OnDiv)ON_BN_CLICKED(IDC_DOT, OnDot)ON_BN_CLICKED(IDC_DEC, OnDec)ON_BN_CLICKED(IDC_COT, OnCot)ON_BN_CLICKED(IDC_ADD, OnAdd)ON_BN_CLICKED(IDC_COS, OnCos)ON_BN_CLICKED(IDC_AC, OnAc)ON_BN_CLICKED(IDC_BACK, OnBack)//}}AFX_MSG_MAPEND_MESSAGE_MAP()/////////////////////////////////////////////////////////////////////////////// CCalculatorDlg message handlersBOOL CCalculatorDlg::OnInitDialog(){CDialog::OnInitDialog();// Add "About..." menu item to system menu.// IDM_ABOUTBOX must be in the system command range.ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);ASSERT(IDM_ABOUTBOX < 0xF000);CMenu* pSysMenu = GetSystemMenu(FALSE);if (pSysMenu != NULL){CString strAboutMenu;strAboutMenu.LoadString(IDS_ABOUTBOX);if (!strAboutMenu.IsEmpty()){pSysMenu->AppendMenu(MF_SEPARATOR);pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);}}// Set the icon for this dialog. The framework does this automatically// when the application's main window is not a dialogSetIcon(m_hIcon, TRUE); // Set big iconSetIcon(m_hIcon, FALSE); // Set small icon// TODO: Add extra initialization heremc.InitStack();OperatorPressed=false;m_strResult="0.0";UpdateData(FALSE);Restarted=true;return TRUE; // return TRUE unless you set the focus to a control}void CCalculatorDlg::OnSysCommand(UINT nID, LPARAM lParam){if ((nID & 0xFFF0) == IDM_ABOUTBOX){CAboutDlg dlgAbout;dlgAbout.DoModal();}else{CDialog::OnSysCommand(nID, lParam);}}// If you add a minimize button to your dialog, you will need the code below// to draw the icon. For MFC applications using the document/view model,// this is automatically done for you by the framework.void CCalculatorDlg::OnPaint(){if (IsIconic()){CPaintDC dc(this); // device context for paintingSendMessage(WM_ICONERASEBKGND, (WPARAM) dc.GetSafeHdc(), 0);// Center icon in client rectangleint cxIcon = GetSystemMetrics(SM_CXICON);int cyIcon = GetSystemMetrics(SM_CYICON);CRect rect;GetClientRect(&rect);int x = (rect.Width() - cxIcon + 1) / 2;int y = (rect.Height() - cyIcon + 1) / 2;// Draw the icondc.DrawIcon(x, y, m_hIcon);}else{CDialog::OnPaint();}// The system calls this to obtain the cursor to display while the user drags// the minimized window.HCURSOR CCalculatorDlg::OnQueryDragIcon(){return (HCURSOR) m_hIcon;}void CCalculatorDlg::OnEqual(){UpdateData(TRUE);if(OperatorPressed==false)mc.PushOpnd(mc.StringToDouble(m_strResult));mc.CalculateAll();m_strResult=mc.GetResult();UpdateData(FALSE);}void CCalculatorDlg::OnNum1(){UpdateData(TRUE);if(OperatorPressed==true||Restarted==true)m_strResult="1";elsem_strResult+="1";OperatorPressed=false;Restarted=false;UpdateData(FALSE);void CCalculatorDlg::OnNum0(){UpdateData(TRUE);if(OperatorPressed==true||Restarted==true) m_strResult="0";elsem_strResult+="0";OperatorPressed=false;Restarted=false;UpdateData(FALSE);}void CCalculatorDlg::OnNum2(){UpdateData(TRUE);if(OperatorPressed==true||Restarted==true) m_strResult="2";elsem_strResult+="2";OperatorPressed=false;Restarted=false;UpdateData(FALSE);}void CCalculatorDlg::OnNum3(){UpdateData(TRUE);if(OperatorPressed==true||Restarted==true) m_strResult="3";elsem_strResult+="3";OperatorPressed=false;Restarted=false;UpdateData(FALSE);}void CCalculatorDlg::OnNum4(){UpdateData(TRUE);if(OperatorPressed==true||Restarted==true) m_strResult="4";elsem_strResult+="4";OperatorPressed=false;Restarted=false;UpdateData(FALSE);}void CCalculatorDlg::OnNum5(){UpdateData(TRUE);if(OperatorPressed==true||Restarted==true) m_strResult="5";elsem_strResult+="5";OperatorPressed=false;Restarted=false;UpdateData(FALSE);}void CCalculatorDlg::OnNum6(){UpdateData(TRUE);if(OperatorPressed==true||Restarted==true) m_strResult="6";elsem_strResult+="6";OperatorPressed=false;Restarted=false;UpdateData(FALSE);}void CCalculatorDlg::OnNum7(){UpdateData(TRUE);if(OperatorPressed==true||Restarted==true) m_strResult="7";elsem_strResult+="7";OperatorPressed=false;Restarted=false;UpdateData(FALSE);}void CCalculatorDlg::OnNum8(){UpdateData(TRUE);if(OperatorPressed==true||Restarted==true)m_strResult="8";elsem_strResult+="8";OperatorPressed=false;Restarted=false;UpdateData(FALSE);}void CCalculatorDlg::OnNum9(){UpdateData(TRUE);if(OperatorPressed==true||Restarted==true)m_strResult="9";elsem_strResult+="9";OperatorPressed=false;Restarted=false;UpdateData(FALSE);}void CCalculatorDlg::OnPow(){UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[9]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;}void CCalculatorDlg::OnSin(){UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[4]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;}void CCalculatorDlg::OnTan(){UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[6]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;}void CCalculatorDlg::OnNegative(){UpdateData(TRUE);if(m_strResult.GetAt(0)=='-')m_strResult=m_strResult.Mid(1);elsem_strResult="-"+m_strResult;UpdateData(FALSE);}void CCalculatorDlg::OnMut(){UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[2]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;}void CCalculatorDlg::OnLog(){UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[11]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;}void CCalculatorDlg::OnLn(){UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[10]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;void CCalculatorDlg::OnDiv(){UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[3]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;}void CCalculatorDlg::OnDot(){UpdateData(TRUE);m_strResult+=".";UpdateData(FALSE);}void CCalculatorDlg::OnDec(){UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[1]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;}void CCalculatorDlg::OnCot()UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[7]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;}void CCalculatorDlg::OnAdd(){UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[0]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;}void CCalculatorDlg::OnCos(){UpdateData(TRUE);mc.PushOpnd(mc.StringToDouble(m_strResult)); mc.PushOptr(optrs[5]);m_strResult=mc.GetResult();UpdateData(FALSE);OperatorPressed=true;}void CCalculatorDlg::OnAc(){Restarted=true;mc.InitStack();m_strResult="0.0";UpdateData(FALSE);}void CCalculatorDlg::OnBack(){UpdateData(TRUE);if(m_strResult!="0"&&m_strResult!="0.0"&&m_strResult.Lef t(m_strResult.GetLength()-1)!="")m_strResult=m_strResult.Left(m_strResult.GetLength()-1);elsem_strResult="0.0";UpdateData(FALSE);}。
VC计算器源文件
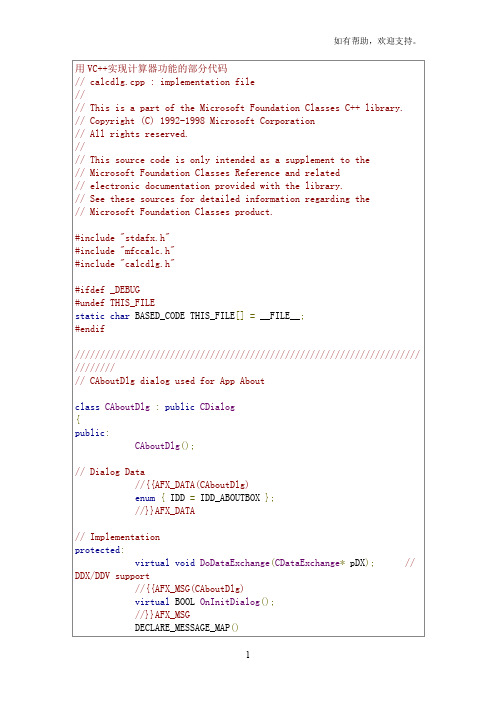
// Microsoft Foundation Classes product.
#include "stdafx.h"
#include "mfccalc.h"
#include "calcdlg.h"
// Implementation
protected:
virtualvoidDoDataExchange(CDataExchange*pDX);// DDX/DDV support
//{{AFX_MSG(CAboutDlg)
virtualBOOLOnInitDialog();
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
用VC++实现计算器功能的部分代码
// calcdlg.cpp : implementation file
//
// This is a part of the Microsoft Foundation Classes C++ library.
// Copyright (C) 1992-1998 Microsoft Corporation
};
CAboutDlg::CAboutDlg():CDialog(CAboutDlg::IDD)
{
//{{AFX_DATA_INIT(CAboutDlg)
//}}AFX_DATA_INIT
}
voidCAboutDlg::DoDataExchange(CDataExchange*pDX)
{
CDialog::DoDataExchange(pDX);
C语言计算器程序源代码

C语⾔计算器程序源代码//strcmp(s1,s2) 当s1⼤于s2时,返回1 ,s1⼩于s2时,返回-1,相等时,返回0 #include "stdio.h"#include "ctype.h"#include "string.h"#include "math.h"#define MAX 256#define STACK_SIZE 128#define WORD_LEN 8#define POP 1#define PUSH 0#define ERR -1#define END 2#define OPER 0#define NUM 1#define WORD 2#define ADD 1#define SUB 2#define MUL 3#define DIV 4#define POW 5#define FAC 6#define BRA_L 7#define BRA_R 8#define SIN 9#define COS 10#define TAN 11#define CTG 12#define LG 13 //以10为底的常⽤对数//#define LN 14//#define LOG 15//⾏标为当前操作符代号,列标为栈顶元素代号//2表⽰计算结束,0表⽰当前操作符进栈,1表⽰栈顶操作符出栈// \0 + - * / ^ ! ( ) sin cos tg ctg lgint Priority[14][14]={2, 1, 1, 1, 1, 1, 1,-1,-1, 1, 1, 1, 1, 1, /* \0 */0, 1, 1, 1, 1, 1, 1, 0,-1, 1, 1, 1, 1, 1, /* + */0, 1, 1, 1, 1, 1, 1, 0,-1, 1, 1, 1, 1, 1, /* - */0, 0, 0, 1, 1, 1, 1, 0,-1, 1, 1, 1, 1, 1, /* * */0, 0, 0, 1, 1, 1, 1, 0,-1, 1, 1, 1, 1, 1, /* / */0, 0, 0, 1, 1, 1, 1, 0,-1, 0, 0, 0, 0, 0, /* ^ */0, 0, 0, 0, 0, 0, 1, 0,-1, 0, 0, 0, 0, 0, /* ! */0, 0, 0, 0, 0, 0,-1, 0,-1, 0, 0, 0, 0, 0, /* ( */-1,1, 1, 1, 1, 1, 1, 1,-1, 1, 1, 1, 1, 1, /* ) */0, 0, 0, 0, 0, 0,-1, 0,-1, 0, 0, 0, 0, 0, /* sin */0, 0, 0, 0, 0, 0,-1, 0,-1, 0, 0, 0, 0, 0, /* cos */0, 0, 0, 0, 0, 0,-1, 0,-1, 0, 0, 0, 0, 0, /* tg */0, 0, 0, 0, 0, 0,-1, 0,-1, 0, 0, 0, 0, 0, /* ctg */0, 0, 0, 0, 0, 0,-1, 0,-1, 0, 0, 0, 0, 0}; /* lg */char KeyWord[36][WORD_LEN+1]={"sin", //前12个为函数,多余的⽤于扩展"cos","tan","tg","ctg","lg","","","","","","","","","","help", // 后⾯为命令,多余的为扩展"version","set","digit", //精度,⼩数点后的位数"color","radian", //弧度"degree", //⾓度"file","clr","clear","window", //窗⼝模式"fullscr", //全屏模式"","","","","","","","",""};int OperCode(char c){int code;switch(c){case '\0':code=0;break;case '+':code=1;break;case '-':code=2;break;case '*':code=3;break;case '/':code=4;break;case '^':code=5;break;case '!':code=6;break;case '(':code=7;break;case ')':code=8;break;case 's':code=9;break; //sincase 'c':code=10;break; //coscase 't':code=11;break; //tgcase 'C':code=12;break; //ctgcase 'l':code=13;break; //log default:code=-1;break;};return code;}int WordCode(char* word){int i;for(i=0;i<25;i++)if(strcmp(KeyWord[i],word)==0) break;if(i>=25)return -1;else}void help(){printf("显⽰帮助信息!\n");return;}void version(){printf("显⽰版本信息!\n");return;}void Err(int errcode,int position,char *p){printf("\n ERR:%d Position:%d %s",errcode,position,p); return;}double long factorial(int i){if(i==1 || i==0)return(1.0);elsereturn(i*factorial(i-1));}main(){char Expression[MAX+1];int Operator[STACK_SIZE];int OperStackTop;double long Number[STACK_SIZE];int NumStackTop;double long NumList[STACK_SIZE];int NumCursor,NumListSize;int OperList[STACK_SIZE];int OperCursor,OperListSize;int WordList[STACK_SIZE];int WordCursor,WordListSize;int Index[MAX+1];int IndexCursor,IndexSize;char Word[WORD_LEN+1];double long num,num1,num2,weight,tempnum;int Oper;int isDecimal,isErr,isNumber,isEnd;char CurrentOper;int i,j,k,m,n;char ch;num=0.0;num2=0.0;tempnum=0.0;Oper=-1;while(1){for(i=0;i<=MAX;i++) //表达式初始化,中间表索引初始化{Expression[i]='\0';Index[i]=-1;}for(i=0;i{Operator[i]='0';Number[i]=0.0;NumList[i]=0.0;OperList[i]=-1;WordList[i]=-1;}NumStackTop=-1; //栈顶指针初始化OperStackTop=0; //操作符栈压⼊\0Operator[OperStackTop]=OperCode('\0');NumCursor=0; //各种中间表指针初始化,各种中间表的长度初始化NumListSize=0;OperCursor=0;OperListSize=0; //操作符表中先写⼊第⼀个操作符'\0'WordCursor=0;WordListSize=0;IndexCursor=0;IndexSize=0;// Index[0]=OPER;printf("Cal>"); //初始化完成,输出提⽰符i=0;while((ch=getchar())!='\n'){if(i>MAX) /*输⼊超长,则出错*/{Err(0,i,"输⼊的表达式长度超过规定值!\n");isErr=1;break;}if(isupper(ch))ch=tolower(ch);Expression[i]=ch;i++;}if(isErr==1){isErr=0;continue;}if(strlen(Expression)==0) //直接回车continue;if(strcmp("end",Expression)==0 ||strcmp("exit",Expression)==0 || strcmp("quit",Expr ession)==0)break;//⼀下代码为编译预处理,主要处理负号,并检查括号是否配对k=0;for(i=0;Expression[i]!='\0';i++){if((i==0&&Expression[i]=='-') || (i>0&&Expression[i]=='-'&&Expression[i-1]=='(')){for(j=strlen(Expression);j>i;j--)Expression[j]=Expression[j-1];Expression[i]='0';}if(Expression[i]=='(') //检查括号k++;if(Expression[i]==')')k--;}if(k>0) //如果括号不配对{Err(1,-1,"缺少右括号 )\n");continue;}if(k<0){Err(1,-1,"缺少左括号 (\n");continue;}//编译预处理结束i=0; //词法分析while(1){if(Expression[i]=='\0'){OperList[OperListSize]=OperCode(Expression[i]);OperListSize++;Index[IndexSize]=OPER;IndexSize++;// printf("IndexSize=%d,Index[IndexSize]=%d,Expression[i]=%c\n",IndexSize,Index[IndexSize],Expression[i]); break;}isDecimal=0;isNumber=0;while(isdigit(Expression[i])||Expression[i]=='.') //读取数字{isNumber=1;if(Expression[i]=='.'){if((i<(MAX-1) && !isdigit(Expression[i+1])) || (i+1)==MAX) //不正确的⼩数点位置{Err(2,i,"⼩数点位置不正确!\n");isErr=1;isNumber=0;i++;break;}isDecimal=1;weight=0.1;i++;continue;}if(isDecimal==0)num=num*10.0+(double long)(Expression[i]-'0');{num=num+(double long)(Expression[i]-'0')*weight;weight=weight*0.1;}i++;} //数字读完if(isErr==1)break;if(isNumber==1) //如果刚才成功读取了数字,则数字⼊栈{NumList[NumListSize]=num;NumListSize++;isNumber=0;num=0.0;Index[IndexSize]=NUM;// printf("IndexSize=%d,Index[IndexSize]=%d\n",IndexSize,Index[IndexSize]); IndexSize++;}for(k=0;k<=WORD_LEN;k++)Word[k]='\0';j=0;while(isalpha(Expression[i])){if(j>=WORD_LEN) //超过长度仍然未匹配,则出错{Err(3,i,"单词长度超过规定值/未定义的单词:");printf("%s\n",Word);isErr=1;break;}Word[j]=Expression[i];j++;// printf("WORD:%s\n",Word);if(WordCode(Word)==-1) //匹配不成功{if(!isalpha(Expression[i+1]))//匹配不成功,但是下⼀个字符已经不是字母,{Err(4,i,"未定义的单词:"); //则出错,并跳出循环printf("%s\n",Word);isErr=1;break;} //匹配不成功且还能继续读取字符,则继续读取下⼀个字母i++;continue;}else //匹配成功,则单词⼊表,读取下⼀个字符{switch(WordCode(Word)){case 0:ch='s';break;case 1:ch='c';break;case 2:case 3:ch='t';break;case 4:ch='C';case 5:ch='l';break;default:ch='\0';WordList[WordListSize]=WordCode(Word);WordListSize++;Index[IndexSize]=WORD;IndexSize++;break;};if(ch!='\0'){OperList[OperListSize]=OperCode(ch);OperListSize++;Index[IndexSize]=OPER;IndexSize++;}i++;break;}} //单词读完if(isErr==1)break;if(Expression[i]==' ')i++;if(!isdigit(Expression[i]) && !isalpha(Expression[i]) && Expression[i]!='\0') {if(OperCode(Expression[i])==-1){isErr=1;Err(5,i,"未定义的操作符:");printf("%c\n",Expression[i]);break;}else{OperList[OperListSize]=OperCode(Expression[i]);OperListSize++;Index[IndexSize]=OPER;IndexSize++;i++;}} //操作符读完if(isErr==1)break;} //词法分析结束if(isErr==1){isErr=0;continue;}/* for(k=0;kprintf("NumList[%d]=%f\n",k,NumList[k]);for(k=0;kprintf("OperList[%d]=%d\n",k,OperList[k]);for(k=0;kprintf("WordList[%d]=%d\n",k,WordList[k]);for(k=0;kprintf("Index[%d]=%d\n",k,Index[k]);printf("\n\n IndexCursor=%d IndexSize=%d,\n",IndexCursor,IndexSize);printf("OperStacktop=%d,Operator[OperStackTop]=%d,NumStackTop=%d\n",OperStackTop,Operator[OperStackTop],NumStackTop); // continue;*/isEnd=0;IndexCursor=0;while(1){if(Index[IndexCursor]==NUM){if(NumCursor<0 || NumListSize<0){Err(10,-1,"索引列表与操作数列表信息不匹配\n");isErr=1;break;}NumStackTop++;Number[NumStackTop]=NumList[NumCursor];NumCursor++;IndexCursor++;continue;} //数字处理if(Index[IndexCursor]==OPER){m=OperList[OperCursor];n=Operator[OperStackTop];switch(Priority[m][n]){case ERR:Err(20,IndexCursor,"不可预见的错误!\n");isErr=1;break;case PUSH:OperStackTop++;Operator[OperStackTop]=m;OperCursor++;IndexCursor++;break;case END:isEnd=1;break;case POP:Oper=Operator[OperStackTop];OperStackTop--;switch(Oper){case BRA_L: IndexCursor++;OperCursor++;break;case ADD:if(NumStackTop>=1){num2=Number[NumStackTop];NumStackTop--;num1=Number[NumStackTop];NumStackTop--;tempnum=num1+num2;NumStackTop++;Number[NumStackTop]=tempnum;num1=0.0;num2=0.0;tempnum=0.0;}else{Err(11,IndexCursor,"加法运算缺少操作数!\n");isErr=1;}break;case SUB:if(NumStackTop>=1){num2=Number[NumStackTop];NumStackTop--;num1=Number[NumStackTop];NumStackTop--;tempnum=num1-num2;NumStackTop++;Number[NumStackTop]=tempnum;num1=0.0;num2=0.0;tempnum=0.0;}else{Err(12,IndexCursor,"减法运算缺少操作数!\n");isErr=1;}break;case MUL:if(NumStackTop>=1){num2=Number[NumStackTop];NumStackTop--;num1=Number[NumStackTop];NumStackTop--;tempnum=num1*num2;NumStackTop++;Number[NumStackTop]=tempnum;num1=0.0;num2=0.0;tempnum=0.0;}else{Err(13,IndexCursor,"乘法运算缺少操作数!\n");isErr=1;}break;case DIV:if(NumStackTop>=1){num2=Number[NumStackTop];NumStackTop--;if(num2==0.0){Err(14,IndexCursor,"除数为 0 ,不能进⾏除法运算!\n"); isErr=1;break;}num1=Number[NumStackTop];NumStackTop--;tempnum=num1/num2;NumStackTop++;Number[NumStackTop]=tempnum;num1=0.0;num2=0.0;tempnum=0.0;}else{Err(15,IndexCursor,"除法运算缺少操作数!\n"); isErr=1;}break;case POW:if(NumStackTop>=1){num2=Number[NumStackTop]; NumStackTop--;num1=Number[NumStackTop]; NumStackTop--;tempnum=pow(num1,num2); NumStackTop++;Number[NumStackTop]=tempnum;num1=0.0;num2=0.0;tempnum=0.0;}else{Err(16,IndexCursor,"乘⽅运算缺少操作数!\n"); isErr=1;}break;case FAC:if(NumStackTop>=0){num2=Number[NumStackTop]; NumStackTop--;tempnum=factorial(num2); NumStackTop++;Number[NumStackTop]=tempnum;num2=0.0;tempnum=0.0;}else{Err(17,IndexCursor,"阶乘运算缺少操作数!\n"); isErr=1;}break;case SIN:if(NumStackTop>=0){num2=Number[NumStackTop]; NumStackTop--;tempnum=sin(num2);NumStackTop++;Number[NumStackTop]=tempnum;num2=0.0;tempnum=0.0;}{Err(18,IndexCursor,"正弦函数缺少参数!\n"); isErr=1;}break;case COS:if(NumStackTop>=0){num2=Number[NumStackTop]; NumStackTop--;tempnum=cos(num2);NumStackTop++;Number[NumStackTop]=tempnum;num2=0.0;tempnum=0.0;}else{Err(19,IndexCursor,"余弦函数缺少参数!\n"); isErr=1;}break;case TAN:if(NumStackTop>=0){num2=Number[NumStackTop]; NumStackTop--;tempnum=tan(num2);NumStackTop++;Number[NumStackTop]=tempnum;num2=0.0;tempnum=0.0;}else{Err(20,IndexCursor,"正切函数缺少参数!\n");isErr=1;}break;case CTG:if(NumStackTop>=0){num2=Number[NumStackTop]; NumStackTop--;tempnum=1.0/tan(num2); NumStackTop++;Number[NumStackTop]=tempnum;num2=0.0;tempnum=0.0;}else{Err(21,IndexCursor,"余切函数缺少参数!\n"); isErr=1;}break;/* case LN:if(NumStackTop>=0)num2=Number[NumStackTop]; NumStackTop--;if(num2<=0.0){Err(20,IndexCursor,"⾃然对数函数真数:"); printf(" %f ⼩于0!\n",num2);isErr=1;break;}tempnum=log(num2);NumStackTop++;Number[NumStackTop]=tempnum;num2=0.0;tempnum=0.0;}else{Err(17,IndexCursor,"⾃然对数函数缺少参数!\n"); isErr=1;}break; */case LG:if(NumStackTop>=0){num2=Number[NumStackTop]; NumStackTop--;if(num2<=0.0){Err(23,IndexCursor,"常⽤对数函数真数:"); printf(" %f ⼩于0!\n",num2);isErr=1;break;}tempnum=log10(num2);NumStackTop++;Number[NumStackTop]=tempnum;num2=0.0;tempnum=0.0;}else{Err(22,IndexCursor,"常⽤对数函数缺少参数!\n"); isErr=1;}break;default:Err(100,IndexCursor,"运算符(代码:"); printf(" %d )暂不⽀持!\n",Oper);isErr=1;break;/*#define SIN 9#define COS 10#define TAN 11#define CTG 12#define LOG 13 */}; //switch 语句结束break;};//switchif(isErr==1 || isEnd==1)break;continue;} //运算符处理if(Index[IndexCursor]==WORD){printf(" 单词尚未处理!\n");break;}//在这⾥处理单词}//核⼼计算结束if(isErr==1){isErr=0;continue;}if(NumStackTop>0){Err(1000,-1,"多余的操作数:");printf("%f!\n",Number[NumStackTop]);}elseif(NumStackTop==0 && isEnd==1){if(fabs(Number[NumStackTop])>1e20) printf(" %.20e\n",Number[NumStackTop]); elseprintf(" %f\n",Number[NumStackTop]); isEnd=0;}}//主循环结束}//主函数结束。
C语言编写一个科学计算器

C语言编写一个科学计算器科学计算器是一种功能强大的工具,可以进行各种复杂的科学计算。
下面是一个使用C语言编写的科学计算器的示例代码,用于演示其基本功能。
```c#include <stdio.h>#include <math.h>void mainMenprintf("------------------------\n");printf(" 科学计算器 \n");printf("------------------------\n");printf("1. 四则运算\n");printf("2. 求平方根\n");printf("3. 求立方根\n");printf("4. 求幂次方\n");printf("5. 求对数\n");printf("6. 退出\n");void arithmeticOperationfloat num1, num2;printf("请输入两个操作数:\n");scanf("%f %f", &num1, &num2);printf("1. 加法结果:%f\n", num1 + num2); printf("2. 减法结果:%f\n", num1 - num2); printf("3. 乘法结果:%f\n", num1 * num2); if (num2 != 0)printf("4. 除法结果:%f\n", num1 / num2); } elseprintf("操作数2不能为0!\n");}void squareRoofloat num;printf("请输入一个数字:\n");scanf("%f", &num);printf("平方根:%f\n", sqrt(num));void cubeRoofloat num;printf("请输入一个数字:\n");scanf("%f", &num);printf("立方根:%f\n", cbrt(num));void exponentiatiofloat base, exponent;printf("请输入底数和指数:\n");scanf("%f %f", &base, &exponent);printf("幂次方:%f\n", pow(base, exponent)); void logarithfloat num;printf("请输入一个数字:\n");scanf("%f", &num);printf("以e为底的自然对数:%f\n", log(num)); printf("以10为底的常用对数:%f\n", log10(num)); int maiint choice;domainMenu(;printf("请输入选项:\n");scanf("%d", &choice);switch (choice)case 1: arithmeticOperations(; break;case 2: squareRoot(;break;case 3: cubeRoot(;break;case 4: exponentiation(;break;case 5: logarithm(;break;case 6: printf("退出程序!\n"); break;default: printf("无效选项!\n"); break;}} while (choice != 6);return 0;```这个科学计算器的源码中,定义了一个主菜单函数`mainMenu(`,用户可以从菜单中选择执行的操作。
c语言计算器源代码

# include <stdio.h># include <malloc.h># include <conio.h># define maxsize 100typedef double datatype1;typedef char datatype2;typedef struct stack1{datatype1 data1[maxsize];int top1; /*栈顶元素*/}seqstack1,*pseqstack1; /*顺序栈*/typedef struct stack2{datatype2 data2[maxsize];int top2; /*栈顶元素*/}seqstack2,*pseqstack2; /*顺序栈*//*栈的初始化*/pseqstack1 init_seqstack1(void){pseqstack1 S;S=(pseqstack1)malloc(sizeof(pseqstack1)); if(S)S->top1=-1;return S;}pseqstack2 init_seqstack2(void){pseqstack2 S;S=(pseqstack2)malloc(sizeof(pseqstack2)); if(S)S->top2=-1;return S;}/*判断栈空*/int empty_seqstack1(pseqstack1 S){if(S->top1==-1)return 1;elsereturn 0;}int empty_seqstack2(pseqstack2 S){if(S->top2==-1)return 1;elsereturn 0;}/*X入栈*/int push_seqstack1(pseqstack1 S,datatype1 X) {if(S->top1==maxsize-1){printf("栈满,无法入栈!\n");return 0;}else{S->top1++;S->data1[S->top1]=X;return 1;}}int push_seqstack2(pseqstack2 S,datatype2 X) {if(S->top2==maxsize-1){printf("栈满,无法入栈!\n");return 0;}else{S->top2++;S->data2[S->top2]=X;return 1;}}/*X出栈*/int pop_seqstack1(pseqstack1 S,datatype1 *X) {if(empty_seqstack1(S))return 0;else{*X=S->data1[S->top1];S->top1--;return 1;}}int pop_seqstack2(pseqstack2 S,datatype2 *X) {if(empty_seqstack2(S))return 0;else{*X=S->data2[S->top2];S->top2--;return 1;}}/*求栈顶元素*/int gettop_seqstack1(pseqstack1 S,datatype1 *X){if(empty_seqstack1(S))return 0;else*X=S->data1[S->top1];return 1;}int gettop_seqstack2(pseqstack2 S,datatype2 *X){if(empty_seqstack2(S))return 0;else*X=S->data2[S->top2];return 1;}/*判断字符是否为操作数。
C语言 计算器 源代码

rectangle(25,25,375,75); /*画屏显的边框*/
setcolor(GREEN); /*设置绿色模式*/
settextstyle(0,0,3); /*改变字符大小方向函数*/
if (error) {strcpy(scr_main,"error"); setcolor(RED); Flag=0; Num_flag=0; clrnum(0,1); }
inregs.x.ax=0x01; /*显示鼠标*/
int86(0x33,&inregs,&outregs); /*通用软中断库函数*/
}
/*鼠标消息*/
int mouse_message()
{
drawmouse(&Mx,&My,&Mkey); /*调用画鼠标函数*/
for (;Mk0==Mkey;)
initgraph( &GraphDriver, &GraphMode, "" ); /*初始化图形系统*/
ErrorCode = graphresult(); /*读初始化结果*/
if( ErrorCode != grOk ) /*如果初始化时出现错误*/
{
void calculator(int fun_code); /*实现计算器功能函数声明*/
void clrnum(int n0,int n1); /*清空Num数组函数声明*/
void adv(void); /*高级函数功能声明*/
double qiu_zhi(char *bds_start,char *bds_end); /*求表达式值函数声明*/
VC++计算器源文件
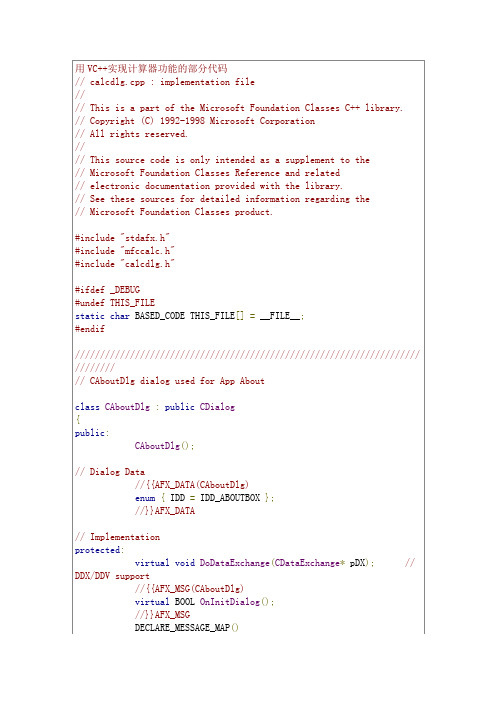
0x62c4dd10,0xf45e,0x11cd,0x8c,0x3d,0x0,0xaa,0x0,0x4b,0xb3,0xb7);
CCalcDlg::CCalcDlg(CWnd*pParent/*=NULL*/)
DISP_FUNCTION(CCalcDlg,"Button",Button,VT_BOOL,VTS_BSTR)
//}}AFX_DISPATCH_MAP
END_DISPATCH_MAP()
#ifndef IMPLEMENT_OLECREATE_SINGLE
// MFC will provide this macro in the future. For now, we define it.
#define IMPLEMENT_OLECREATE_SINGLE(class_name, external_name, \
l,w1,w2,b1,b2,b3,b4,b5,b6,b7,b8)\
AFX_DATADEFCOleObjectFactoryclass_name::factory(class_name::guid,\
// NOTE: the ClassWizard will add member initialization here
//}}AFX_DATA_INIT
// Note that LoadIcon does not require a subsequent DestroyIcon in Win32
m_hIcon=AfxGetApp()->LoadIcon(IDR_MAINFRAME);
C语言实现简单计算器

C语⾔实现简单计算器本⽂实例为⼤家分享了C语⾔实现简单计算器的具体代码,供⼤家参考,具体内容如下实现效果如图:实现代码如下:#include<stdio.h>#include<windows.h>//gotoxy#include<conio.h>#define width 80#define height 30void gotoxy(int x, int y);void GreateFrame(){int i = 0;for (i = 0 ; i < width; i += 2){gotoxy(i, 0); printf("■");gotoxy(i, height); printf("■");}for (i = 0; i < height + 1; i++){gotoxy(0 , i); printf("■");gotoxy(width, i); printf("■");}}void Add(){float i, j;printf("*加法运算*\n");scanf_s("%f %f", &i, &j);printf("%5.2f + %5.2f=%5.2f\n", i, j, i+j); _getch();}void Sub(){float i, j;printf("*减法运算*\n");scanf_s("%f %f", &i, &j);printf("%5.2f - %5.2f=%5.2f\n", i, j, i -j); _getch();}void Mul(){float i, j;printf("*乘法运算*\n");scanf_s("%f %f", &i, &j);printf("%5.2f * %5.2f=%5.2f\n", i, j, i * j); _getch();}void Div(){float i, j;printf("*除法运算*\n");scanf_s("%f %f", &i, &j);printf("%5.2f / %5.2f=%5.2f\n", i, j, i / j); _getch();}int main(int argc, char* argv[]){int choose;while (1){GreateFrame();gotoxy(width / 3, height / 3 + 2);printf("1.加法");gotoxy(width / 3, height / 3 + 4);printf("2.减法");gotoxy(width / 3, height / 3 + 6);printf("3.乘法");gotoxy(width / 3, height / 3 + 8);printf("4.除法");gotoxy(width / 3, height / 3 + 10);printf("5.退出程序");gotoxy(width / 3, height / 3);printf("请选择你要计算的⽅式:( )\b\b"); scanf_s("%d", &choose);switch (choose){case 1:system("cls");Add(); break;case 2:system("cls");Sub(); break;case 3:system("cls");Mul(); break;case 4:system("cls");Div(); break;case 5:exit(0);}system("cls");}}void gotoxy(int x, int y){COORD POS;POS.X = x;POS.Y = y;SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE),POS);}以上就是本⽂的全部内容,希望对⼤家的学习有所帮助,也希望⼤家多多⽀持。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
(10)“fabs”按钮的消息处理函数
void CMyCalculatorDlg::OnButtonFabs()
{
if( m_second==0)
{ m_first=fabs(m_first); UpdateDisplay(m_first); }
else
{ m_second=fabs(m_second); UpdateDisplay(m_second);}
{ m_first=0.0;
m_second=0.0;
m_operator = "+";
m_coff = 1.0;
UpdateDisplay(0.0);
}
(9)“n!”按钮的消息处理函数
void CMyCalculatorDlg::OnButtonJiecheng()
{
if( m_second==0)
void CMyCalculatorDlg::UpdateDisplay(double lVal)
{ //在编辑框中显示数据
m_display.Format(_T("%f"),lVal);
int i=m_display.GetLength();
while(m_display.GetAt(i-1)=='0') //格式化输出,将输出结果后的零截去
{ case '+': m_first+=m_second;break;
case '-': m_first-=m_second;break;
case '*': m_first*=m_second;break;
case '/':
if(fabs(m_second)<=0.000001)
{m_display="除数不能为0";
/
……
……
……
Button
IDC_BUTTON_CLEAR
C
Button
IDC_BUTTON9
9
Button
IDC_BUTTON_SQRT
sqrt
Button
IDC_BUTTON_POINT
.
Button
IDC_BUTTON_RECI
1/x
Button
IDC_BUTTON_SIGN
+/
Button
}
(10)“ln”按钮的消息处理函数
void CMyCalculatorDlg::OnButtonLn()
{
if( m_second==0)
{ m_first=log(m_first); UpdateDisplay(m_first); }
else
{ m_second=log(m_second); UpdateDisplay(m_second);}
}
“cos”按钮的消息处理函数
void CMyCalculatorDlg::OnButtonCos()
{
if( m_second==0)
{ m_first=cos(m_first); UpdateDisplay(m_first); }
else
{ m_second=cos(m_second); UpdateDisplay(m_second);}
{ m_display.Delete(i-1,1); i--; }
UpdateData(false);//更新编辑框变量m_display
}
void CMyCalculator::Calculate()
{ //将前一次数据与当前数据进行运算,作为下次的第一操作数,并在编辑框显示。
switch(m_operator.GetAt(0))
m_coff *= 0.1;}
UpdateDisplay(m_second);//更新编辑框的数字显示
}
void CMyCalculatorDlg::OnButtonPi()
{
m_first=3.14159265;
UpdateDisplay(m_first);
}
(2)运算符按钮的消息响应函数:
“+”按钮的消息处理函数
{m_display = "除数不能为零";
UpdateData(false);
return;}
if( fabs(m_second)<0.000001)
{ m_first=1.0/m_first;
UpdateDisplay(m_first);
}
else
{ m_second=1.0/m_second;
cos
Button
IDC_BUTTON_SIN
sin
Button
IDC_BUTTON_TAN
tan
Button
IDC_BUTTON_LN
Ln
Button
IDC_BUTTON_PI
Pi
4、为对话框类添加成员变量
1.double m_first;//存储一次运算的第一个操作数及一次运算的结果
2.double m_second;//存储一次运算的第二个操作数
UpdateData(false);
return; }
m_first/=m_second;break;
}
m_second=0.0;
m_coff=1.0;
m_operator=_T("+");
UpdateDisplay(m_first);//更新编辑框显示内容
}
7、为Button按钮的BN_CLICKED事件添加响应函数,并编写代码
}
最后在MyCalculatorDlg.cpp文件首加入语句#include <math.h>,编译并运行。
(1)数字”N”的消息响应函数(N=0,1,…9)
void CMyCalculatorDlg::OnButtonN()
{if( m_coff == 1.0)
m_second = m_second*10 + N;//作为整数输入数字时
else
{ m_second = m_second + N*m_coff; //作为小数输入数字
3.CString m_operator;//存储运算符
4.double m_coff;//存储小数点的系数权值
5.CString m_display;//编辑框IDC_DISPLAY的关联变量,显示计算结果
5、在对话框类的构造函数中,初始化成员变量
CMyCalculatorDlg::CMyCalculatorDlg(CWnd* pParent /*=NULL*/): Dialog(CMyCalculatorDlg::IDD, pParent)
void CMyCalculatorDlg::OnButtonAdd() //加、减、乘类似
{ Calculate();
m_operator="+"; //减为“-”、乘为“*”
}
void CMyCalculatorDlg::OnButtonMinus() //加、减、乘类似
{ Calculate();
UpdateDisplay(m_second);}
}
(4)“Sqrt”按钮的消息处理函数
void CMyCalculatorDlg::OnButtonSqrt()
{
if( m_second==0)
{ m_first=sqrt(m_first); UpdateDisplay(m_first); }
else
}
(11)“sin”按钮的消息处理函数
void CMyCalculatorDlg::OnButtonSin()
{
if( m_second==0)
{ m_first=sin(m_first); UpdateDisplay(m_first); }
else
{ m_second=sin(m_second); UpdateDisplay(m_second);}
}
“tan”按钮的消息处理函数
void CMyCalculatorDlg::OnButtonTan()
{
if( m_second==0)
{ m_first=tan(m_first); UpdateDisplay(m_first); }
else
{ m_second=tan(m_second); UpdateDisplay(m_second);}
{……
m_display = _T("0.0");
m_first = 0.0;
m_second= 0.0;
m_operator=_T("+");
m_coff = 1.0;
……
}
6、为对话框添加2个成员函数:
voidUpdateDisplay(double lVal)—用于在编辑框中显示数据
voidCMyCalculator::Calculate()---用于计算
VC++计算器设计
设计一个简单的计算器,能够实现浮点型数的加、减、乘、除、开方、倒数运算。运行界面如图所示。
步骤如下:
1、创建一个对话框应用程序MyCalculator;
2、在“Project Workspace”窗口,选择“Resource View”标签,双击Dialog下的“IDD_MYCALCULATOR_DIALOG”,从“IDD_MYCALCULATOR_DIALOG”对话框删除“OK”和“ Cancel”及“TODO文本”,将对话框标题设置为“计算器”。
{ m_second=sqrt(m_second); UpdateDisplay(m_second);}