java科学计算器
用java代码写的简易计算器(可以实现基本的加减乘除功能)

⽤java代码写的简易计算器(可以实现基本的加减乘除功能)package A;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import java.awt.event.MouseEvent;import java.awt.event.MouseListener;import javax.swing.*;public class Calcular3 extends JFrame implements ActionListener,MouseListener{private int m1=0,n=0;//private double m2=0;//运算的数private int flag=0;JFrame f;JPanel p1,p2,p3;JTextField t;JButton b1[]=new JButton[18];String b[]= {"1","2","3","4","5","6","7","8","9","0","清空","退格",".","=","+","-","*","/"};public Calcular3(){f=new JFrame("计算器");t=new JTextField(35);p1=new JPanel();p2=new JPanel();p3=new JPanel();f.setBounds(100, 100, 400, 200);f.add(p1,BorderLayout.NORTH);f.add(p2,BorderLayout.CENTER);f.add(p3,BorderLayout.EAST);p2.setLayout(new GridLayout(5,3));p3.setLayout(new GridLayout(4,1));p1.add(t);for(int i=0;i<14;i++) {b1[i]=new JButton(b[i]);p2.add(b1[i]);b1[i].addActionListener(this);}for(int i=14;i<18;i++) {b1[i]=new JButton(b[i]);p3.add(b1[i]);b1[i].addActionListener(this);}/*for(int i=0;i<18;i++) {b1[i].addActionListener(this);}*/f.setVisible(true);}//实现接⼝的⽅法public void mouseClicked(MouseEvent e) {}public void mousePressed(MouseEvent e) {}public void mouseReleased(MouseEvent e) {}public void mouseEntered(MouseEvent e) {}public void mouseExited(MouseEvent e) {}public void actionPerformed(ActionEvent e) {String str="";int i;for(i=0;i<=9;i++) {if(e.getSource()==b1[i]) {if(i==9) {n=n*10;}else {n=n*10+i+1;}str=String.valueOf(n);//整形n转换成字符串strt.setText(str);//显⽰到⽂本框上}}for(i=14;i<18;i++) {//+、-、*、/if(e.getSource()==b1[i]) {//匹配运算符m1=Integer.parseInt(t.getText());if(flag==15) {m2=m1+m2;}else if(flag==16) {m2=m1-m2;}else if(flag==17) {m2=m1*m2;}else if(flag==18) {m2=m1/m2;}else m2=m1;//若⽆连续的运算符运算,保存当前数据到m2 if(i==14) flag=15;else if(i==15) flag=16;else if(i==16) flag=17;else flag=18;str=String.valueOf(b[i]);t.setText(str);//显⽰到⽂本框上n=0;//还原,记录下次数据break;//找到匹配数据退出循环}}if(e.getSource()==b1[13]) {//=m1=Integer.parseInt(t.getText());if(flag==15) {m2=m2+m1;}else if(flag==16) {m2=m2-m1;}else if(flag==17) {m2=m2*m1;}else if(flag==18) {m2=m2/m1;}else m2=m1;str=String.valueOf(m2);t.setText(str);//显⽰到⽂本框上n=0;//还原,记录下次数据flag=0;//flag还原0,表明没有未处理的运算符}if(e.getSource()==b1[10]) {//各变量变为0 清空m1=0;m2=0;flag=0;n=0;t.setText("0");//显⽰到⽂本框上}if(e.getSource()==b1[11]) {//退格m1=(int)(Double.parseDouble(t.getText())/10);n=m1;str=String.valueOf(m1);t.setText(str);}if(e.getSource()==b1[12]) {//⼩数点m1=Integer.parseInt(t.getText());str=String.valueOf(m1+b[12]);t.setText(str);//显⽰到⽂本框上int j=0;for(i=0;i<=9;i++) {if(e.getSource()==b1[i]) {j++;m2=Math.pow(0.1, j)*Integer.parseInt(b[i]);str=String.valueOf(m1+m2);t.setText(str);//显⽰到⽂本框上}}}}//主函数public static void main(String[] args) {new Calcular3();}}。
java课程设计科学计算器
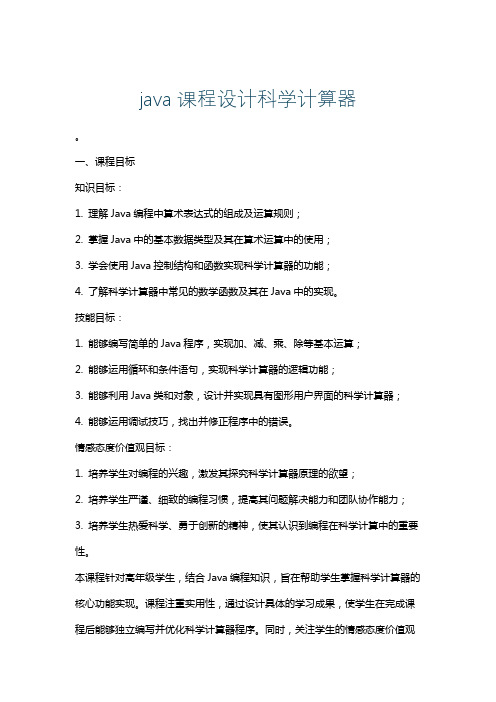
java课程设计科学计算器。
一、课程目标知识目标:1. 理解Java编程中算术表达式的组成及运算规则;2. 掌握Java中的基本数据类型及其在算术运算中的使用;3. 学会使用Java控制结构和函数实现科学计算器的功能;4. 了解科学计算器中常见的数学函数及其在Java中的实现。
技能目标:1. 能够编写简单的Java程序,实现加、减、乘、除等基本运算;2. 能够运用循环和条件语句,实现科学计算器的逻辑功能;3. 能够利用Java类和对象,设计并实现具有图形用户界面的科学计算器;4. 能够运用调试技巧,找出并修正程序中的错误。
情感态度价值观目标:1. 培养学生对编程的兴趣,激发其探究科学计算器原理的欲望;2. 培养学生严谨、细致的编程习惯,提高其问题解决能力和团队协作能力;3. 培养学生热爱科学、勇于创新的精神,使其认识到编程在科学计算中的重要性。
本课程针对高年级学生,结合Java编程知识,旨在帮助学生掌握科学计算器的核心功能实现。
课程注重实用性,通过设计具体的学习成果,使学生在完成课程后能够独立编写并优化科学计算器程序。
同时,关注学生的情感态度价值观培养,提高其综合素质。
二、教学内容1. Java基本语法与数据类型:回顾Java基本语法规则,重点掌握整型、浮点型等基本数据类型及其在运算中的使用。
- 教材章节:第一章 Java语言概述,第二章 基本数据类型与运算符2. 算术表达式与运算规则:讲解算术表达式的构成,介绍运算符优先级和结合性。
- 教材章节:第二章 基本数据类型与运算符3. Java控制结构:学习顺序、分支和循环结构,实现科学计算器的基本运算功能。
- 教材章节:第三章 控制结构4. 函数的定义与调用:介绍函数的概念,学会编写自定义函数,实现计算器中的特定功能。
- 教材章节:第四章 函数与数组5. 图形用户界面设计:学习Java Swing库,使用其组件设计科学计算器的界面。
- 教材章节:第七章 Java Swing图形用户界面6. 数学函数与运算:介绍科学计算器中常见的数学函数,如三角函数、指数函数等,并在Java中实现。
JAVA科学计算器

JAVA科学计算器Java是一种广泛应用于科学计算和数据分析领域的编程语言,拥有强大的数学计算库和丰富的科学计算函数,因此非常适合用于开发科学计算器。
本文将介绍如何使用Java开发一个功能强大的科学计算器。
一、需求分析1.支持基本的四则运算(加、减、乘、除)2.支持高级的数学函数(三角函数、指数函数、对数函数等)3.支持科学计数法(输入和显示均支持科学计数法)4.支持清空功能,删除单个字符功能5.支持键盘输入和界面按钮输入两种方式输入计算表达式二、界面设计1. 界面采用图形用户界面(GUI)设计,使用Swing或JavaFX等界面库进行开发2.使用文本框显示计算结果和表达式3.使用按钮实现数字和运算符的输入4.添加清空按钮和删除按钮5.添加科学计数法开关按钮三、代码实现1. 创建一个继承自JFrame的主窗口类,设置窗口标题、大小和关闭方式```public class ScientificCalculator extends JFrameprivate JTextField outputField;//其他界面组件声明public ScientificCalculatosuper("科学计算器");setSize(400, 600);setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//初始化界面组件并添加到窗口//...setVisible(true);}```2. 创建一个继承自JPanel的面板类,用于放置计算器的按钮和文本框```public class CalculatorPanel extends JPanelprivate JTextField outputField;//其他按钮和变量声明public CalculatorPanesetLayout(new BorderLayout();//初始化界面组件并添加到面板//...}```3.在面板类中添加按钮事件监听器,实现按钮的点击响应```public class CalculatorPanel extends JPanel implements ActionListenerprivate JTextField outputField;private JButton[] numberButtons;private JButton[] operatorButtons;//其他按钮和变量声明public CalculatorPanesetLayout(new BorderLayout();//初始化界面组件并添加到面板//...//添加按钮事件监听器for (JButton button : numberButtons)button.addActionListener(this);}for (JButton button : operatorButtons)button.addActionListener(this);}//其他按钮添加监听器//设置文本框只读outputField.setEditable(false);}public void actionPerformed(ActionEvent e)//根据按钮的命令执行相应的操作//...}```4.在按钮事件监听器中实现计算逻辑,调用数学库中的函数完成计算```public void actionPerformed(ActionEvent e)//清空操作outputField.setText("");//删除操作String currentText = outputField.getText(;outputField.setText(currentText.substring(0,currentText.length( - 1));} else//数字或运算符输入}```5.在启动类中创建主窗口对象,并将面板对象添加到主窗口中```public class Mainpublic static void main(String[] args)SwingUtilities.invokeLater(( ->ScientificCalculator calculator = new ScientificCalculator(;calculator.add(new CalculatorPanel();});}```四、测试与优化在开发完成后,需要对科学计算器进行测试,保证其功能正常,并进行优化,确保使用体验良好,例如添加键盘监听器,支持键盘输入,修复可能出现的Bug等。
java科学计算器(进制转换)
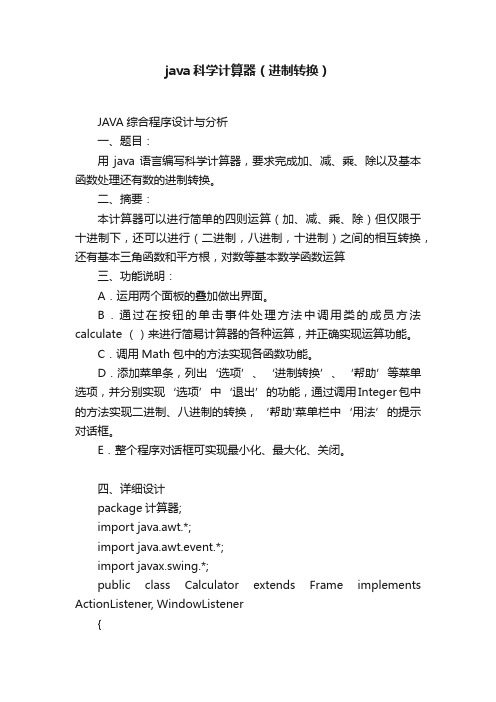
java科学计算器(进制转换)JAVA综合程序设计与分析一、题目:用java语言编写科学计算器,要求完成加、减、乘、除以及基本函数处理还有数的进制转换。
二、摘要:本计算器可以进行简单的四则运算(加、减、乘、除)但仅限于十进制下,还可以进行(二进制,八进制,十进制)之间的相互转换,还有基本三角函数和平方根,对数等基本数学函数运算三、功能说明:A.运用两个面板的叠加做出界面。
B.通过在按钮的单击事件处理方法中调用类的成员方法calculate ()来进行简易计算器的各种运算,并正确实现运算功能。
C.调用Math包中的方法实现各函数功能。
D.添加菜单条,列出‘选项’、‘进制转换’、‘帮助’等菜单选项,并分别实现‘选项’中‘退出’的功能,通过调用Integer包中的方法实现二进制、八进制的转换,‘帮助'菜单栏中‘用法’的提示对话框。
E.整个程序对话框可实现最小化、最大化、关闭。
四、详细设计package计算器;import java.awt.*;import java.awt.event.*;import javax.swing.*;public class Calculator extends Frame implements ActionListener, WindowListener{privateprivateprivate GridBagConstraints constraints;private JTextField displayField; //计算结果显示区private String lastCommand; //保存+,-,*,/,=命令0private double result; //保存计算结果private boolean start; //判断是否为数字的开始private JMenuBar menubar;private JMenuItem m_exit,m2_ejz,m2_bjz;private Dialog dialog;private Label label_dialog;private JButtonbutton_sqrt,button_plusminus,button_CE,button_cancel,butt on_1,button_ 2,button_3,button_4,button_5,button_6,button_7,button_8,but ton_9,button _0,button_plus,button_minus,button_multiply,button_divide,bu tton_point,button_equal,button_log,button_tan,button_cos,button_sin,butt on_exp;public Calculator() //构造方法设置布局、为按钮注册事件监听器{super("Calculator");this.setLocation(240,200);this.setSize(350,300);this.setResizable(true);this.setLayout(new GridLayout(7,1));this.addmyMenu(); //调用成员方法添加菜单displayField=new JTextField(30);this.add(displayField);displayField.setEditable(true);start=true;result=0;lastCommand = "=";JPanel panel0=new JPanel();panel0.setLayout(new GridLayout(1,4,4,4)); JPanel panel1=new JPanel();panel1.setLayout(new GridLayout(1,5,4,4)); this.add(panel1);button_sqrt=new JButton("sqrt");button_plusminus=new JButton("+/-"); button_exp=new JButton("exp");button_CE=new JButton("退格");button_cancel=new JButton("C"); JPanel panel2=new JPanel();panel2.setLayout(new GridLayout(1,5,4,4)); this.add(panel2);button_7=new JButton("7");button_8=new JButton("8");button_9=new JButton("9");button_log=new JButton("log");button_divide=new JButton("/");JPanel panel3=new JPanel();panel3.setLayout(new GridLayout(1,5,4,4)); this.add(panel3);button_4=new JButton("4");button_5=new JButton("5");button_6=new JButton("6");button_tan=new JButton("tan");button_multiply=new JButton("*");JPanel panel4=new JPanel();panel4.setLayout(new GridLayout(1,5,4,4)); this.add(panel4);button_1=new JButton("1");button_2=new JButton("2");button_3=new JButton("3");button_cos=new JButton("cos");button_minus=new JButton("-");JPanel panel5=new JPanel();panel5.setLayout(new GridLayout(1,5,4,4)); this.add(panel5);button_0=new JButton("0");button_point=new JButton(".");button_equal=new JButton("=");button_sin=new JButton("sin");button_plus=new JButton("+");panel1.add(button_sqrt);panel1.add(button_plusminus);panel1.add(button_exp);panel1.add(button_CE);panel1.add(button_cancel);panel2.add(button_7);panel2.add(button_8);panel2.add(button_9);panel2.add(button_log);panel2.add(button_divide);panel3.add(button_4);panel3.add(button_5);panel3.add(button_6);panel3.add(button_tan);panel3.add(button_multiply);panel4.add(button_1);panel4.add(button_2);panel4.add(button_3);panel4.add(button_cos);panel4.add(button_minus);panel5.add(button_0);panel5.add(button_point);panel5.add(button_equal);panel5.add(button_sin);panel5.add(button_plus);button_sqrt.addActionListener(this); button_plusminus.addActionListener(this); button_exp.addActionListener(this); button_CE.addActionListener(this); button_cancel.addActionListener(this); button_7.addActionListener(this);button_8.addActionListener(this);button_9.addActionListener(this);button_log.addActionListener(this); button_divide.addActionListener(this); button_4.addActionListener(this);button_5.addActionListener(this);button_6.addActionListener(this);button_tan.addActionListener(this); button_multiply.addActionListener(this); button_1.addActionListener(this);button_2.addActionListener(this);button_3.addActionListener(this);button_cos.addActionListener(this);button_minus.addActionListener(this);button_0.addActionListener(this);button_point.addActionListener(this);button_equal.addActionListener(this);button_sin.addActionListener(this);button_plus.addActionListener(this);this.addWindowListener(new WinClose()); //注册窗口监听器this.setVisible(true);}private void addmyMenu() //菜单的添加{JMenuBar menubar=new JMenuBar();this.add(menubar);JMenu m1=new JMenu("选项");JMenu m2=new JMenu("进制转换");JMenuItem m1_exit=new JMenuItem("退出");m1_exit.addActionListener(this);JMenuItem m2_ejz=new JMenuItem("二进制");m2_ejz.addActionListener(this);JMenuItem m2_bjz=new JMenuItem("八进制");m2_bjz.addActionListener(this);JMenu m3 = new JMenu(" 帮助");JMenuItem m3_Help = new JMenuItem("用法");m3_Help.addActionListener(this);dialog = new Dialog(this,"提示",true); //模式窗口dialog.setSize(240,80);label_dialog = new Label("",Label.CENTER); //标签的字符串为空,居中对齐dialog.add(label_dialog);dialog.addWindowListener(this); //为对话框注册窗口事件监听器m1.add(m1_exit);menubar.add(m1);m2.add(m2_ejz);m2.add(m2_bjz);menubar.add(m2);m3.add(m3_Help);menubar.add(m3); }public void actionPerformed(ActionEvent e) //按钮的单击事件处理方法{if(e.getSource().equals(button_1)||e.getSource().equals(butto n_2) ||e.getSource().equals(button_3)||e.getSource().equals(button _4)||e.getSource().equals(button_5)||e.getSource().equals(button_6)||e.getSource().equals(button_7)||e.getSource().equals(button_8)||e.getSource().equals(button_9)||e.getSource().equals(button_0)||e.getSource().equals(button_point)||e.getSource().equals(but ton_plusm inus)||e.getSource().equals(button_cancel)||e.getSource().equals(b utton_CE)) { //非运算符的处理方法String input=e.getActionCommand();if (start){displayField.setText("");start=false;if(input.equals("+/-"))displayField.setText(displayField.getT ext()+"-");}if(!input.equals("+/-")){String str=displayField.getText();if(input.equals("退格")) //退格键的实现方法{if(str.length()>0)displayField.setText(str.substring(0,str.length()-1));}else if(input.equals("C")) //清零键的实现方法{displayField.setText("0");start=true;}elsedisplayField.setText(displayField.getT ext()+input);}}else if (e.getActionCommand()=="二进制") //二进制的转换{int n=Integer.parseInt(displayField.getText()); displayField.setText(Integer.toBinaryString(n));}else if (e.getActionCommand()=="八进制") //八进制的转换{int n=Integer.parseInt(displayField.getText()); displayField.setText(Integer.toOctalString(n));}else if (e.getActionCommand()=="退出") //选项中退出的处理方法{System.exit(0);}else if(e.getActionCommand()=="用法") //按下'帮助'菜单栏中用法的处理方法{label_dialog.setT ext("sqrt,exp等键是先输运算符再输数字\");dialog.setLocation(400,250);dialog.setVisible(true);}else//各运算符的识别{String command=e.getActionCommand();if(start){lastCommand=command;}else{calculate(Double.parseDouble(displayField.getText()));lastCommand=command;start=true;}}}public void calculate(double x) //各运算符的具体运算方法{double d=0;if (lastCommand.equals("+")) result+= x;else if (lastCommand.equals("-")) result-=x;else if (lastCommand.equals("*")) result*=x;else if (lastCommand.equals("/")) result/=x;else if (lastCommand.equals("=")) result=x;else if (lastCommand.equals("sqrt")) {d=Math.sqrt(x);result=d;}else if (lastCommand.equals("exp")) {d=Math.exp(x);result=d;}else if (lastCommand.equals("log")) {d=Math.log(x);result=d;}else if (lastCommand.equals("tan")) {d=Math.tan(x);result=d;}else if (lastCommand.equals("cos")){d=Math.cos(x);result=d;}else if (lastCommand.equals("sin")){d=Math.sin(x);result=d;}displayField.setText(""+ result);}public void windowClosing(WindowEvent e){if(e.getSource()==dialog)dialog.setVisible(false); //隐藏对话框elseSystem.exit(0);}public void windowOpened(WindowEvent e) { } public void windowActivated(WindowEvent e) { } public void windowDeactivated(WindowEvent e) { } public void windowClosed(WindowEvent e) { } public void windowIconified(WindowEvent e) { } public void windowDeiconified(WindowEvent e) { } public static void main(String args[]){Calculator calculator=new Calculator();}}class WinClose implements WindowListener{public void windowClosing(WindowEvent e) //单击窗口关闭按钮时触发并执行实现窗口监听器接口中的方法{System.exit(0); //结束程序运行}public void windowOpened(WindowEvent e){}public void windowActivated(WindowEvent e){}public void windowDeactivated(WindowEvent e){}public void windowClosed(WindowEvent e){}public void windowIconified(WindowEvent e){}public void windowDeiconified(WindowEvent e){}}五、程序运行结果1.界面2.基本运算,如54 -12 =42:3.数学函数运算,如9的开平方为3:六、总结与体会总结:(1)通过编写这个相对复杂的程序,让我认识解决实际问题前应现建立模型,再通过建立的模型使问题简单化,从而解决问题。
用Java设计简易的计算器

⽤Java设计简易的计算器运⾏初始状态:计算结果如下:代码如下:package jisuanqi;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import javax.swing.JButton;import javax.swing.JComboBox;import javax.swing.JFrame;class counter1 extends JFrame{public counter1(){super("计算器");this.setSize(400,100);this.setLocation(300,240);this.setLayout(new FlowLayout()); //确定窗⼝格式为流输⼊TextField text1=new TextField(4);text1.setText("1");this.add(text1);String proList[] = { "+","-","x" ,"%"}; //将"+","-","x" ,"%"作为列表元素TextField text;JComboBox comboBox; //创建下拉复选框Container conPane = getContentPane(); //创建⼀个名为conPane的容器comboBox = new JComboBox(proList); //把列表元素加⼊下拉复选框comboBox.setEditable(true); //使复选框变为可操作conPane.add(comboBox); //将复选框加⼊容器中TextField text2=new TextField(4);text2.setText("1");this.add(text2);JButton button = new JButton("=");this.add(button);TextField text3=new TextField(4);text3.setText("2");button.addActionListener(new ActionListener(){ //添加按钮监听事件public void actionPerformed(ActionEvent e) //创建事件响应函数{String s=comboBox.getEditor().getItem().toString(); //获取复选框中的元素double a= Integer.parseInt(text1.getText()); //将两个⽂本框中的字符串强制转换成浮点型,以便于之后的计算double b= Integer.parseInt(text2.getText());if(s.equals("+")) { //判断复选框中的元素种类double t=a+b;String m=String.valueOf(t); //由于⽂本框中的数据流只能为字符串,这⾥就需要将计算得到的浮点型数据强制转换成字符串型 text3.setText(m); //将最后的结果放在⽂本框中}else if(s.equals("-")) //后⾯的与之前的类似,不在注释else if(s.equals("-")) //后⾯的与之前的类似,不在注释{double t=a-b;String m=String.valueOf(t);text3.setText(m);}else if(s.equals("x")){double t=a*b;String m=String.valueOf(t);text3.setText(m);}else{double t=a/b;String m=String.valueOf(t);text3.setText(m);}}});conPane.add(text3);this.setVisible(true); //将窗⼝设置为可视化}}public class Counter {public static void main(String[] args){new counter1();}}修改版如下:源码如下:package jisuanqi;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import java.awt.event.FocusEvent;import java.awt.event.FocusListener;import javax.swing.JButton;import javax.swing.JComboBox;import javax.swing.JFrame;import javax.swing.JLabel;class counter1 extends JFrame{public counter1(){super("计算器");this.setSize(400,130);this.setLocation(300,240);this.setLayout(new FlowLayout()); //确定窗⼝格式为流输⼊ TextField text1=new TextField(4);TextField text1=new TextField(4);text1.setText("1");JLabel label1 = new JLabel("");text1.addFocusListener(new FocusListener() { //添加焦点事件监听器public void focusGained(FocusEvent arg0) { //设置获得焦点时的事件label1.setText("正在输⼊中...");}public void focusLost(FocusEvent arg0) { //设置失去焦点时的事件if(!text1.getText().matches("\\d+")) //使⽤正则表达式判断该字符串是否为数字,第⼀个\是转义符,\d+表⽰匹配1个或 //多个连续数字,"+"和"*"类似,"*"表⽰0个或多个 label1.setText("输⼊的第⼀个数据有误");else label1.setText("");}});this.add(text1);String proList[] = { "+","-","x" ,"%"}; //将"+","-","x" ,"%"作为列表元素TextField text;JComboBox comboBox; //创建下拉复选框Container conPane = getContentPane(); //创建⼀个名为conPane的容器comboBox = new JComboBox(proList); //把列表元素加⼊下拉复选框comboBox.setEditable(true); //使复选框变为可操作conPane.add(comboBox); //将复选框加⼊容器中TextField text2=new TextField(4);text2.setText("1");this.add(text2);text2.addFocusListener(new FocusListener() {public void focusGained(FocusEvent arg0) {label1.setText("正在输⼊中...");}public void focusLost(FocusEvent arg0) {if(!text2.getText().matches("\\d+"))label1.setText("输⼊的第⼆个数据有误");else label1.setText("");}});JButton button = new JButton("=");this.add(button);TextField text3=new TextField(4);text3.setText("2");button.addActionListener(new ActionListener(){ //添加按钮监听事件public void actionPerformed(ActionEvent e) //创建事件响应函数{String s=comboBox.getEditor().getItem().toString(); //获取复选框中的元素double a= Integer.parseInt(text1.getText()); //将两个⽂本框中的字符串强制转换成浮点型,以便于之后的计算double b= Integer.parseInt(text2.getText());if(s.equals("+")) { //判断复选框中的元素种类double t=a+b;String m=String.valueOf(t); //由于⽂本框中的数据流只能为字符串,这⾥就需要将计算得到的浮点型数据强制转换成字符串型text3.setText(m); //将最后的结果放在⽂本框中}else if(s.equals("-")) //后⾯的与之前的类似,不在注释{double t=a-b;String m=String.valueOf(t);text3.setText(m);}else if(s.equals("x")){double t=a*b;String m=String.valueOf(t);text3.setText(m);}else{double t=a/b;String m=String.valueOf(t);text3.setText(m);}}});conPane.add(text3);this.add(label1);this.setVisible(true); //将窗⼝设置为可视化}}public class Counter {public static void main(String[] args){new counter1();}}总结⼼得:(1)在创建选项框时,要将所有的选项放在⼀个“容器”⾥,并把这个容器添加到程序中,这⾥我⽤的容器为JComboBox comboBox,同时需要导⼊包boBoxEditor和javax.swing.JComboBox;(2)由于设置了按钮响应功能,所以要设置按键动作和响应,这⾥导⼊了包java.awt.event.ActionEvent和java.awt.event.ActionListener(3)因为⽂本框中输⼊读取到的是字符串,所以要进⾏计算时,要先将其转为整形,在⽂本框输出时,同理要将整形转换为字符串(4)注意:当光标移动到⽂本框上⾯时,获得焦点事件,当光标移⾛时,便失去焦点事件,所以要注意两个函数的作⽤。
JAVA实现计算器

JAVA实现计算器 1 import java.awt.Color;2import java.awt.Container;3import java.awt.GridLayout;4import java.awt.TextField;5import java.awt.event.ActionEvent;6import java.awt.event.ActionListener;78import javax.jws.HandlerChain;9import javax.swing.JButton;10import javax.swing.JFrame;11import javax.swing.JPanel;12import javax.swing.JTextField;1314import java.util.Stack;1516public class Main extends JFrame implements ActionListener{1718private String expression;//记录表达式1920//各个按键的字符21private final String[] keys = {"(",")","C","Backspace",22 "7","8","9","+",23 "4","5","6","-",24 "1","2","3","*",25 ".","0","=","/"};26private JTextField result = new JTextField("0");2728//按键29private JButton[] button = new JButton[20];3031 Main(){323334//计算器布局35 JPanel calckeysPanel = new JPanel();36//布局先分为六⾏,第⼀⾏为⽂本显⽰,⼆到五⾏为按键37 calckeysPanel.setLayout(new GridLayout(6,1));3839 calckeysPanel.add(result);40 result.setFont(result.getFont().deriveFont((float)(30)));41 Container[] containers = new Container[5];42for (int i = 0;i < 5;++i){43 containers[i] = new Container();44 containers[i].setLayout(new GridLayout(1,4));45 calckeysPanel.add(containers[i]);46 }4748//按钮49for (int i = 0;i < button.length;++i){50 button[i] = new JButton(keys[i]);51 button[i].setFont(button[i].getFont().deriveFont((float)(30)));52 containers[i/4].add(button[i]);53 button[i].addActionListener(this);54if (i == 2 || i == 3) button[i].setForeground(Color.red);55 }56 getContentPane().add(calckeysPanel);5758//表达式赋值为空59 expression = "";60 }61626364 @Override65public void actionPerformed(ActionEvent e) {66// TODO Auto-generated method stub67 String action = e.getActionCommand();686970if (action.equals(keys[2])){71//⽤户按下C72 handleC();73 }else if (action.equals(keys[3])){74//⽤户按下退格75 handleBackspace();76 }else if (action.equals(keys[18])){77//⽤户按下=78 handleCalc();79 }else{80//⽤户输⼊表达式81 handleExpression(action);82 }83 }8485//处理按下C的事件86private void handleC(){87 expression = "";88 result.setText("");89 }9091//处理按下退格的事件92private void handleBackspace(){93 expression = expression.substring(0,expression.length() - 1);94 result.setText(expression);95 }9697//处理按下等号的事件98private void handleCalc(){99 result.setText(Calculator.calrp(Calculator.getrp(expression))); 100 expression = "";101 }102103//处理⽤户按下数字或运算符的事件104private void handleExpression(String action){105 expression += action;106 result.setText(expression);107 }108109public static void main(String[] args){110 Main calculator = new Main();111 calculator.setSize(600,800);112 calculator.setVisible(true);113 }114115 }116117class Calculator {118//计算中缀表达式119static Stack<Character> op = new Stack<>();120121public static Float getv(char op, Float f1, Float f2){122if(op == '+') return f2 + f1;123else if(op == '-') return f2 - f1;124else if(op == '*') return f2 * f1;125else if(op == '/') return f2 / f1;126else return Float.valueOf(-0);127 }128129public static String calrp(String rp){130 Stack<Float> v = new Stack<>();131char[] arr = rp.toCharArray();132int len = arr.length;133for(int i = 0; i < len; i++){134 Character ch = arr[i];135136if(ch >= '0' && ch <= '9') v.push(Float.valueOf(ch - '0'));137138else v.push(getv(ch, v.pop(), v.pop()));139 }140return v.pop().toString();141 }142143public static String getrp(String s){144char[] arr = s.toCharArray();145int len = arr.length;146 String out = "";147148for(int i = 0; i < len; i++){149char ch = arr[i];150if(ch == ' ') continue;151152153if(ch >= '0' && ch <= '9') {154 out+=ch;155continue;156 }157158if(ch == '(') op.push(ch);159160if(ch == '+' || ch == '-'){161while(!op.empty() && (op.peek() != '('))162 out+=op.pop();163 op.push(ch);164continue;165 }166167if(ch == '*' || ch == '/'){168while(!op.empty() && (op.peek() == '*' || op.peek() == '/')) 169 out+=op.pop();170 op.push(ch);171continue;172 }173174if(ch == ')'){175while(!op.empty() && op.peek() != '(') 176 out += op.pop();177 op.pop();178continue;179 }180 }181while(!op.empty()) out += op.pop();182return out;183 }184 }⽤Java实现的简易计算器运⾏效果图如下:。
java计算器实验报告
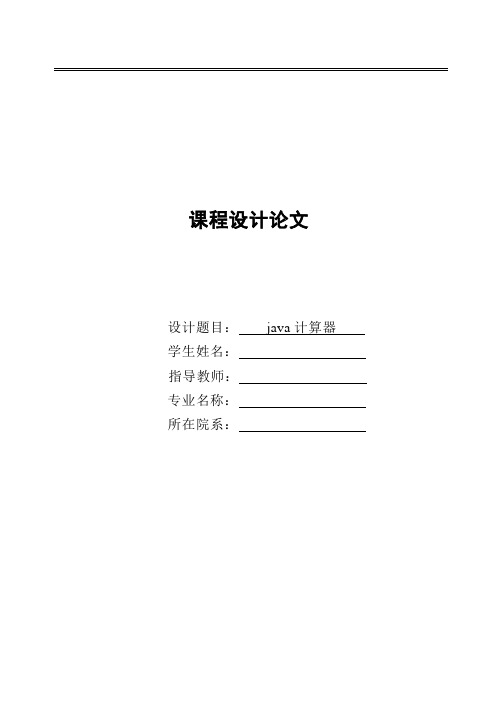
课程设计论文设计题目:java计算器学生姓名:指导教师:专业名称:所在院系:摘要在当今这个网络的时代,java语言在网络编程方面的优势使得网络编程有了更好的选择。
Java语言最大的特点是具有跨平台性,使其不受平台不同的影响,得到了广泛的应用。
该程序是一个图形界面的java 计算器,其界面主要采用了swing包,程序实现了:(1)实现简单加、减、乘、除的运算。
(2)实现除了加、减、乘、除按钮外其他按钮功能。
(3)给软件添加一个编辑、查看、帮助的主菜单。
(4)在数据输入方面,提高程序代码的健壮性,增强其对非法输入数据的识别能力。
(5)科学型计算器与标准计算器之间的菜单切换。
Java的早期版本以来,抽象窗口工具包为用户界面组件提供了平台独立的API。
在AWT中,每个组件都由一个原生的等同组件生成和控制,这个原生组件是由当前的图形窗口系统决定的。
与此相对,Swing 组件经常被描述为轻量级的,因为它们不需要操作系统本身所带窗口工具包的原生资源来生成。
目录第1章概要设计 (1)1.1查找资料 (1)1.2选题 (1)1.3标准型计算器界面设计 (1)1.4本章小节 (3)第2章程序整体设计说明 (4)2.1程序中所用到的变量 (4)2.2程序部分源代码及注释 (4)2.3本章小节 (15)第3章程序运行效果 (16)3.1程序运行主界面 (16)3.2程序中各按钮运行界面 (16)3.3本章小节 (20)第4章设计中遇到的重点及难点 (21)4.1设计中遇到的重点 (21)4.2设计中的遇到的难点 (21)4.3本章小节 (23)第5章本次设计中存在不足与改良方案 (24)5.1本次设计中存在的不足 (24)5.2本次设计的改良方案 (24)5.3本章小节 (24)结论 (25)参考文献 (26)致谢 (27)指导教师评语 (28)答辩委员会评语 (29)第1章概要设计1.1查找资料21世纪,随着社会经济的迅速发展和科学技术的全面进步,人类社会已进入信息和网络时代。
用Java实现的计算器

用Java实现的计算器计算器是一个广泛应用的工具,用于进行数学运算。
如今,随着计算机技术的不断发展,计算器也逐渐被软件程序所替代。
本文将介绍如何使用Java语言实现一个简单的计算器程序。
一、程序概述计算器程序主要包括用户界面和计算逻辑两部分。
用户界面负责显示计算器的按钮和输入输出框,计算逻辑负责根据用户输入进行运算并返回结果。
二、用户界面设计在Java中,我们可以使用Swing或JavaFX等图形库来设计用户界面。
本文选用JavaFX来实现计算器的界面。
界面需要包括数字按钮、运算符按钮、输入输出框等组件。
在程序启动时,我们需要初始化界面并设置各个组件的位置、大小和样式。
为了方便布局,我们可以使用GridPane等布局管理器来管理组件。
三、计算逻辑实现1. 表达式解析计算器需要将用户输入的表达式解析为计算机可以识别的格式。
Java中可以使用正则表达式或者逆波兰表达式来解析表达式。
本文选用简单的正则表达式方法进行解析。
2. 运算逻辑解析表达式后,我们需要根据运算符的优先级进行运算。
Java中可以使用栈来实现运算符的优先级判断和运算。
我们可以定义一个操作数栈和一个运算符栈,按照规则将表达式中的数字和运算符依次入栈,并根据运算符的优先级进行运算并将结果入栈。
四、完整代码示例import javafx.application.Application;import javafx.geometry.Insets;import javafx.scene.Scene;import javafx.scene.control.Button;import javafx.scene.control.TextField;import yout.GridPane;import javafx.stage.Stage;public class Calculator extends Application {private TextField inputField; // 输入框private Button[] numberButtons; // 数字按钮private Button[] operatorButtons; // 运算符按钮@Overridepublic void start(Stage primaryStage) {primaryStage.setTitle("计算器");primaryStage.setResizable(false);// 初始化界面GridPane gridPane = new GridPane();gridPane.setHgap(10);gridPane.setVgap(10);gridPane.setPadding(new Insets(10));// 初始化输入框inputField = new TextField();inputField.setEditable(false);inputField.setPrefHeight(40);inputField.setMaxWidth(Double.MAX_VALUE);gridPane.add(inputField, 0, 0, 4, 1);// 初始化数字按钮numberButtons = new Button[10];for (int i = 0; i < 10; i++) {numberButtons[i] = createNumberButton(String.valueOf(i), i); }gridPane.add(numberButtons[7], 0, 1);gridPane.add(numberButtons[9], 2, 1);gridPane.add(numberButtons[4], 0, 2);gridPane.add(numberButtons[5], 1, 2);gridPane.add(numberButtons[6], 2, 2);gridPane.add(numberButtons[1], 0, 3);gridPane.add(numberButtons[2], 1, 3);gridPane.add(numberButtons[3], 2, 3);gridPane.add(numberButtons[0], 0, 4);gridPane.add(createDotButton(), 1, 4);gridPane.add(createEqualButton(), 2, 4);// 初始化运算符按钮operatorButtons = new Button[4];operatorButtons[0] = createOperatorButton("+"); operatorButtons[1] = createOperatorButton("-"); operatorButtons[2] = createOperatorButton("*"); operatorButtons[3] = createOperatorButton("/"); gridPane.add(operatorButtons[0], 3, 1);gridPane.add(operatorButtons[1], 3, 2);gridPane.add(operatorButtons[3], 3, 4);Scene scene = new Scene(gridPane);primaryStage.setScene(scene);primaryStage.show();}private Button createNumberButton(String text, int number) { Button button = new Button(text);button.setPrefSize(60, 40);button.setOnAction(e -> {String oldText = inputField.getText();inputField.setText(oldText + number);});return button;}private Button createDotButton() {Button button = new Button(".");button.setPrefSize(60, 40);button.setOnAction(e -> {String oldText = inputField.getText();inputField.setText(oldText + ".");});return button;}private Button createEqualButton() {Button button = new Button("=");button.setPrefSize(60, 40);button.setOnAction(e -> {String expression = inputField.getText();// 调用计算逻辑进行计算if (expression != null && !expression.isEmpty()) { double result = calculate(expression);inputField.setText(String.valueOf(result));}});return button;}private Button createOperatorButton(String text) {Button button = new Button(text);button.setPrefSize(60, 40);button.setOnAction(e -> {String oldText = inputField.getText();inputField.setText(oldText + text);});return button;}private double calculate(String expression) {// 进行计算逻辑的实现// ...}public static void main(String[] args) {launch(args);}}五、总结通过本文的介绍,我们可以了解到使用Java语言实现一个简单计算器的基本框架和思路。
Java科学计算器程序代码

计算器布局显示:功能举例:①13+20=33②-7*14=-98:先输入7,再单击-/+将其变成负数,然后挨次单击×号、14与=③7!=5040④sin30°=0.5:先输入30,然后按下′″按钮转换成角度值,再按下sin即可显示结果⑤sqrt(4)=2:利用鼠标点击4后再点击sqrt按键即可显示结果⑥2*π=6.2832……源程序如下:import java.awt.BorderLayout;import java.awt.Color;import java.awt.Dimension;import java.awt.Font;import java.awt.GridLayout;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import java.text.DecimalFormat;import javax.swing.BorderFactory;import javax.swing.ButtonGroup;import javax.swing.JButton;import javax.swing.JCheckBoxMenuItem;import javax.swing.JFrame;import javax.swing.JMenu;import javax.swing.JMenuBar;import javax.swing.JMenuItem;import javax.swing.JPanel;import javax.swing.JRadioButtonMenuItem;import javax.swing.JTextField;public class Calucator extends JFrame {private JTextField tf;private JPanel panel1, panel2, panel3, panel4;private JMenuBar myBar;private JMenu menu1, menu2, menu3;private JMenuItem editItem1, editItem2, help1, help2, help3;private JRadioButtonMenuItem seeItem1, seeItem2;//单选框private JCheckBoxMenuItem seeItem3;//复选框private ButtonGroup bgb;private String back;private boolean IfResult = true, flag = false;private String oper = ;private double result = 0;private Num numActionListener;private DecimalFormat df;public Calucator(){super(科学计算器);//设置标题栏df = new DecimalFormat();//保留四位小数this.setLayout(new BorderLayout(10, 5));panel1 = new JPanel(new GridLayout(1, 3, 10, 10));panel2 = new JPanel(new GridLayout(5, 6, 5, 5));//5行6列panel3 = new JPanel(new GridLayout(5, 1, 5, 5));panel4 = new JPanel(new BorderLayout(5, 5));/***菜单栏*/myBar = new JMenuBar();menu1 = new JMenu(编辑);menu2 = new JMenu(查看);menu3 = new JMenu(匡助);menu1.setFont(new Font(宋体, Font.PLAIN, 12));menu2.setFont(new Font(宋体, Font.PLAIN, 12));menu3.setFont(new Font(宋体, Font.PLAIN, 12));/***编辑栏*/editItem1 = new JMenuItem(复制);editItem2 = new JMenuItem(粘贴);editItem1.setFont(new Font(宋体,Font.PLAIN,12)); editItem2.setFont(new Font(宋体,Font.PLAIN,12));/***查看栏*/seeItem1 = new JRadioButtonMenuItem(科学型); seeItem2 = new JRadioButtonMenuItem(标准型); seeItem3 = new JCheckBoxMenuItem(数字分组);seeItem1.setFont(new Font(宋体,Font.PLAIN,12)); seeItem2.setFont(new Font(宋体,Font.PLAIN,12)); seeItem3.setFont(new Font(宋体,Font.PLAIN,12));/***匡助栏*/help1 = new JMenuItem(匡助主题);help2 = new JMenuItem(关于计算器);help1.setFont(new Font(宋体,Font.PLAIN,12)); help2.setFont(new Font(宋体,Font.PLAIN,12));bgb = new ButtonGroup();//选项组menu1.add(editItem1);menu1.add(editItem2);menu2.add(seeItem1);menu2.add(seeItem2);menu2.addSeparator();//添加一条分割线menu2.add(seeItem3);menu3.add(help1);menu3.addSeparator();//添加一条分割线menu3.add(help2);myBar.add(menu1);myBar.add(menu2);myBar.add(menu3);this.setJMenuBar(myBar);numActionListener = new Num();//实现数字监听/***文本域,即为计算器的屏幕显示区域*/tf = new JTextField();tf.setEditable(false);//文本区域不可编辑tf.setBackground(Color.white);//文本区域的背景色tf.setHorizontalAlignment(JTextField.RIGHT);//文字右对齐tf.setText();tf.setBorder(BorderFactory.createLoweredBevelBorder());init();//对计算器进行初始化}/***初始化操作*添加按钮*/private void init(){addButton(panel1, , new Clear(), Color.red);addButton(panel1, , new Clear(), Color.red);addButton(panel1, , new Clear(), Color.red);addButton(panel2, , new Signs(), Color.magenta);addButton(panel2, , new Signs(), Color.magenta);addButton(panel2, , numActionListener, Color.blue);addButton(panel2, , numActionListener, Color.blue);addButton(panel2, , numActionListener, Color.blue);addButton(panel2, ÷, new Signs(), Color.red);addButton(panel2, , new Signs(), Color.magenta);addButton(panel2, , new Signs(), Color.magenta);addButton(panel2, , numActionListener, Color.blue);addButton(panel2, , numActionListener, Color.blue);addButton(panel2, , numActionListener, Color.blue);addButton(panel2, ×, new Signs(), Color.red);addButton(panel2, , new Signs(), Color.magenta);addButton(panel2, , new Signs(), Color.magenta);addButton(panel2, , numActionListener, Color.blue);addButton(panel2, , numActionListener, Color.blue);addButton(panel2, , numActionListener, Color.blue);addButton(panel2, , new Signs(), Color.red);addButton(panel2, , new Signs(), Color.magenta);addButton(panel2, , new Signs(), Color.magenta);addButton(panel2, , numActionListener, Color.blue);addButton(panel2, , new Clear(), Color.blue);addButton(panel2, , new Dot(), Color.blue);addButton(panel2, , new Signs(), Color.red);addButton(panel2, , new Signs(), Color.magenta);addButton(panel2, , new Signs(), Color.magenta);addButton(panel2, π, numActionListener, Color.orange);addButton(panel2, , numActionListener, Color.orange);addButton(panel2, ′″, new Signs(), Color.orange);addButton(panel2, , new Signs(), Color.red);JButton btns = new JButton(计算器);btns.setBorder(BorderFactory.createLoweredBevelBorder());btns.setEnabled(false);//按钮不可操作btns.setPreferredSize(new Dimension(20, 20));panel3.add(btns);//加入按钮addButton(panel3, , null, Color.red);addButton(panel3, , null, Color.red);addButton(panel3, , null, Color.red);addButton(panel3, , null, Color.red);panel4.add(panel1, BorderLayout.NORTH);panel4.add(panel2, BorderLayout.CENTER);this.add(tf, BorderLayout.NORTH);this.add(panel3, BorderLayout.WEST);this.add(panel4);pack();this.setResizable(false);//窗口不可改变大小this.setLocation(300, 200);this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);}/***统一设置按钮的的使用方式*@param panel*@param name*@param action*@param color*/private void addButton(JPanel panel, String name, ActionListener action, Color color){JButton bt = new JButton(name);panel.add(bt);//在面板上增加按钮bt.setForeground(color);//设置前景(字体)颜色bt.addActionListener(action);//增加监听事件}/***计算器的基础操作(+-×÷)*@param x*/private void getResult (double x){if(oper == ){result += x;}else if(oper == ){result -= x;}else if(oper == ×){result *= x;}else if(oper == ÷){result /= x;}else if(oper == ){result = x;}tf.setText(df.format(result));}/***运算符号的事件监听*/class Signs implements ActionListener{public void actionPerformed(ActionEvent e) {/** 用ActionEvent对象的getActionCommand()方法* 取得与引起事件对象相关的字符串*/String str = e.getActionCommand();/* sqrt求平方根 */if(str.equals()){double i = Double.parseDouble(tf.getText());if(i>=0){/** String.valueOf() 转换为字符串* df.format() 按要求保留四位小数* Math.sqrt() 求算数平方根*/tf.setText(String.valueOf(df.format(Math.sqrt(i))));}else{tf.setText(负数不能开平方根);}}/* log求常用对数 */else if(str.equals()){double i = Double.parseDouble(tf.getText());if(i>0){tf.setText(String.valueOf(df.format(Math.log(i))));}else{tf.setText(负数不能求对数);}}/* %求百分比 */else if(str.equals()){tf.setText(df.format(Double.parseDouble(tf.getText()) / 100)); }/* 1/x求倒数 */else if(str.equals()){if(Double.parseDouble(tf.getText()) == 0){tf.setText(除数不能为零);}else{tf.setText(df.format(1 / Double.parseDouble(tf.getText())));}}/* sin求正弦函数 */else if(str.equals()){double i = Double.parseDouble(tf.getText());tf.setText(String.valueOf(df.format(Math.sin(i))));}/* cos求余弦函数 */else if(str.equals()){double i = Double.parseDouble(tf.getText());tf.setText(String.valueOf(df.format(Math.cos(i))));}/* tan求正切函数 */else if(str.equals()){double i = Double.parseDouble(tf.getText());tf.setText(String.valueOf(df.format(Math.tan(i))));}/* n!求阶乘 */else if(str.equals()){double i = Double.parseDouble(tf.getText());if((i%2==0)||(i%2==1))//判断为整数放进行阶乘操作{int j = (int)i;//强制类型转换int result=1;for(int k=1;k<=j;k++)result *= k;tf.setText(String.valueOf(result));}else{tf.setText(无法进行阶乘);}}/* x^2求平方 */else if(str.equals()){double i = Double.parseDouble(tf.getText());tf.setText(String.valueOf(df.format(i*i)));}/* x^3求立方 */else if(str.equals()){double i = Double.parseDouble(tf.getText());tf.setText(String.valueOf(df.format(i*i*i))); }/* ′″角度转换 *//***将角度值转换成弧度值,方便三角函数的计算*/else if(str.equals(′″)){double i = Double.parseDouble(tf.getText());tf.setText(String.valueOf(i/180*Math.PI));}else{if(flag){IfResult = false;}if(IfResult){oper = str;}else{getResult(Double.parseDouble(tf.getText()));oper = str;IfResult = true;}}}}/***清除按钮的事件监听*/class Clear implements ActionListener{public void actionPerformed(ActionEvent e) {/** 用ActionEvent对象的getActionCommand()方法* 取得与引起事件对象相关的字符串*/String str = e.getActionCommand();if(str == ){tf.setText();IfResult = true;result = 0;}else if(str == ){double i = 0 - Double.parseDouble(tf.getText().trim());tf.setText(df.format(i));}else if(str == ){if(Double.parseDouble(tf.getText()) > 0){if(tf.getText().length() > 1){tf.setText(tf.getText().substring(0,tf.getText().length() - 1));//使用退格删除最后一位字符}else{tf.setText();IfResult = true;}}else{if(tf.getText().length() > 2){tf.setText(tf.getText().substring(0,tf.getText().length() - 1));}else{tf.setText();IfResult = true;}}}else if(str == ){tf.setText();IfResult = true;}}}/***数字输入的事件监听*/class Num implements ActionListener{public void actionPerformed(ActionEvent e) { String str = e.getActionCommand();if(IfResult){tf.setText();IfResult = false;}if(str==π){tf.setText(String.valueOf(Math.PI));}else if(str==){tf.setText(String.valueOf(Math.E));}else{tf.setText(tf.getText().trim() + str);if(tf.getText().equals()){tf.setText();IfResult = true;flag = true;}}}}/***小数点的事件监听*/class Dot implements ActionListener{public void actionPerformed(ActionEvent e) { IfResult = false;if(tf.getText().trim().indexOf() == -1){ tf.setText(tf.getText() + );}}}/***main方法*/public static void main(String[] args) { new Calucator().setVisible(true);}}。
北邮JAVA第三次作业科学计算器(附源代码)
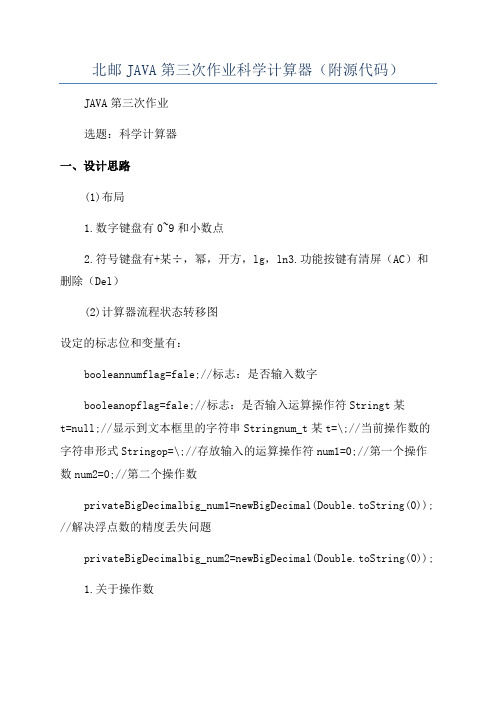
北邮JAVA第三次作业科学计算器(附源代码)JAVA第三次作业选题:科学计算器一、设计思路(1)布局1.数字键盘有0~9和小数点2.符号键盘有+某÷,幂,开方,lg,ln3.功能按键有清屏(AC)和删除(Del)(2)计算器流程状态转移图设定的标志位和变量有:booleannumflag=fale;//标志:是否输入数字booleanopflag=fale;//标志:是否输入运算操作符Stringt某t=null;//显示到文本框里的字符串Stringnum_t某t=\;//当前操作数的字符串形式Stringop=\;//存放输入的运算操作符num1=0;//第一个操作数num2=0;//第二个操作数privateBigDecimalbig_num1=newBigDecimal(Double.toString(0)); //解决浮点数的精度丢失问题privateBigDecimalbig_num2=newBigDecimal(Double.toString(0));1.关于操作数操作数为输入数字时会改变的数,有num1和num2。
按下运算符和控制字符,会设定当前下在键入的操作数,由opflag控制,fale表示正在操作num1,true表示正在操作num2。
初始状态下的操作数为num1。
按下AC会恢复操作数为初始状态num1。
按Delete、开方、lg、ln不会改变当前的操作数。
其中后三者只对num1有效。
其他的运算符和控制符都会使操作数为num2。
2.计算器的状态框图输入第一个数,比如53numflag=true(num1)opflag=falenum_t某t=”53”op=””t某t=”53”初始状态numflag=faleopflag=falenum_t某t=””op=””t某t=nullnum1,num2=0按下一个运算符,比如+numflag=faleopflag=truenum_t某t=””op=”+”t某t=”num1+”其中按下按=显示结果,恢复初始状态numflag=true(num1)opflag=falenum_t某t=””op=””t某t=”reult”“=”后,结果存输入第二个数,比如66入numflag=true(num2)opflag=truenum_t某t=”66”op=””t某t=”num1+66”num1,继续输入数字会在num1的结果上处理。
java代码写的简易计算器(可以实现基本的加减乘除功能)

java代码写的简易计算器(可以实现基本的加减乘除功能)计算器java写⼀个计算器,要求实现加减乘除功能,并且能够循环接收新的数据,通过⽤户交互实现。
import java.util.Scanner;/*** 计算器* 写4个⽅法:加减乘除* 利⽤循环加switch进⾏⽤户交互* 传递需要操作的两个数* 输出结果*/public class Demo04 {public static double a;public static double b;//运算符public static String operator;//=符public static String equal;//结果public static double sum=0;public static void main(String[] args) {Scanner scanner = new Scanner(System.in);System.out.println("输⼊数字:");a = scanner.nextDouble();System.out.println("输⼊运算符:");operator = scanner.next();System.out.println("输⼊数字:");b = scanner.nextDouble();do {switch (operator){case "+":sum= add(a, b);System.out.println("输⼊=获取结果:");equal = scanner.next();coninue();break;case "-":sum=subtract(a,b);System.out.println("输⼊=获取结果:");equal = scanner.next();coninue();break;case "*":sum=multiply(a,b);System.out.println("输⼊=获取结果:");equal = scanner.next();coninue();break;case "/":if (b==0){System.out.println("被除数不能为0");operator = "q";break;}else {sum=divide(a,b);System.out.println("输⼊=获取结果:");equal = scanner.next();coninue();break;}default:System.out.println("运算符输⼊错误!");}}while (operator.equals("+")||operator.equals("-")||operator.equals("*")||operator.equals("/"));scanner.close();}//加public static double add(double a,double b){return a+b;}//减public static double subtract(double a,double b){return a-b;}//乘public static double multiply(double a,double b){return a*b;}//除public static double divide(double a,double b){return a/b;}//获得结果或再次输⼊public static void coninue(){Scanner scanner = new Scanner(System.in);if (equal.equals("=")){System.out.println(a+operator+b+"="+sum);System.out.println("输⼊运算符+,-,*,/继续或输⼊其他字符结束");operator=scanner.next();if (operator.equals("+")||operator.equals("-")||operator.equals("*")||operator.equals("/")){ System.out.println("输⼊数字:");b = scanner.nextDouble();a=sum;}else {System.out.println("输⼊错误!");}}else {System.out.println("输⼊错误!");}}}。
java 计算器实验报告

java 计算器实验报告Java计算器实验报告引言:计算器是我们日常生活中常用的工具,使用计算器可以快速进行数学运算。
在计算器中,我们可以通过输入数字和运算符来执行各种算术操作。
为了更好地理解计算器的工作原理,我们进行了Java计算器的实验。
一、实验目的本次实验的目的是设计和实现一个简单的Java计算器。
通过这个实验,我们可以加深对Java编程语言的理解,并且掌握Java图形用户界面(GUI)的基本知识。
二、实验环境本次实验使用的是Java开发工具包(JDK)和Eclipse集成开发环境(IDE)。
在实验之前,我们需要确保已经正确安装了JDK和Eclipse。
三、实验步骤1. 创建一个新的Java项目,并命名为"Calculator"。
2. 在项目中创建一个新的Java类,命名为"CalculatorGUI"。
3. 在"CalculatorGUI"类中,创建一个窗口,并设置窗口的标题和大小。
4. 在窗口中添加一个文本框,用于显示计算结果。
5. 在窗口中添加一些按钮,用于输入数字和运算符。
6. 为每个按钮添加事件监听器,以便在用户点击按钮时执行相应的操作。
7. 实现计算器的基本功能,包括加法、减法、乘法和除法。
8. 运行程序,测试计算器的功能。
四、实验结果经过实验,我们成功地实现了一个简单的Java计算器。
用户可以通过按钮输入数字和运算符,并且计算器可以正确地执行相应的运算。
计算结果会显示在文本框中。
五、实验总结通过这个实验,我们学习了如何使用Java编程语言创建图形用户界面,并且掌握了Java计算器的基本实现方法。
在实验过程中,我们遇到了一些问题,比如如何处理用户输入错误的情况,如何处理除数为零的情况等。
通过不断调试和改进,我们最终解决了这些问题,并实现了一个功能完善的计算器。
在今后的学习和工作中,我们可以进一步扩展这个计算器的功能,比如添加科学计算功能、实现复杂运算等。
java简易计算器完整代码
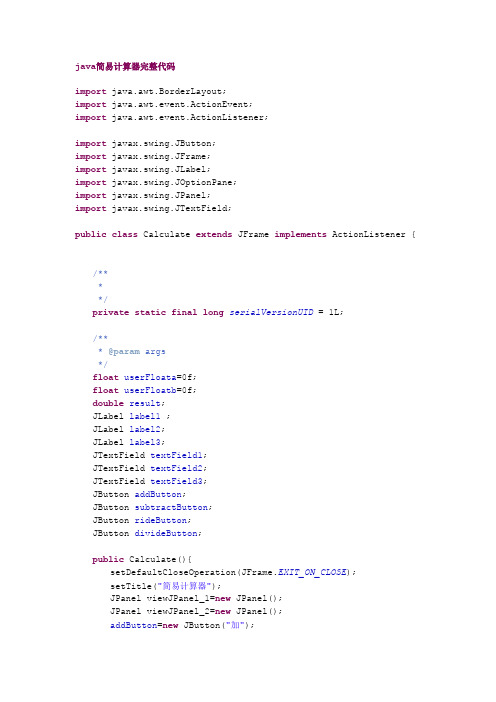
java简易计算器完整代码import java.awt.BorderLayout;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import javax.swing.JButton;import javax.swing.JFrame;import javax.swing.JLabel;import javax.swing.JOptionPane;import javax.swing.JPanel;import javax.swing.JTextField;public class Calculate extends JFrame implements ActionListener {/****/private static final long serialVersionUID = 1L;/*** @param args*/float userFloata=0f;float userFloatb=0f;double result;JLabel label1 ;JLabel label2;JLabel label3;JTextField textField1;JTextField textField2;JTextField textField3;JButton addButton;JButton subtractButton;JButton rideButton;JButton divideButton;public Calculate(){setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);setTitle("简易计算器");JPanel viewJPanel_1=new JPanel();JPanel viewJPanel_2=new JPanel();addButton=new JButton("加");addButton.addActionListener(this);viewJPanel_1.add(addButton);subtractButton=new JButton("减");subtractButton.addActionListener(this);viewJPanel_1.add(subtractButton);rideButton=new JButton("乘");rideButton.addActionListener(this);viewJPanel_1.add(rideButton);divideButton=new JButton("除");divideButton.addActionListener(this);viewJPanel_1.add(divideButton);label1=new JLabel("数据1:");viewJPanel_2.add(label1);textField1=new JTextField(5);textField1.addActionListener(this);viewJPanel_2.add(textField1);label2=new JLabel("数据2:");viewJPanel_2.add(label2);textField2=new JTextField(5);textField2.addActionListener(this);viewJPanel_2.add(textField2);label3=new JLabel("结果是:");viewJPanel_2.add(label3 );textField3=new JTextField(5);viewJPanel_2.add(textField3);setSize(420,120);setVisible(true);validate();getContentPane().add(viewJPanel_1,BorderLayout.NORTH);getContentPane().add(viewJPanel_2,BorderLayout.CENTER);}public void CalculateEventHandel(){try{userFloata= Float.parseFloat(textField1.getText().trim());userFloatb= Float.parseFloat(textField2.getText().trim());} catch (NumberFormatException e) {JOptionPane.showMessageDialog(this, "请输入数字型数据!");textField1.setText("");textField1.requestFocus();textField2.setText("");textField3.setText("");return;}}public static void main(String[] args) { // TODO Auto-generated method stubnew Calculate();//a.CalculateEventHandel();}public void actionPerformed(ActionEvent e) { // TODO Auto-generated method stubif(e.getSource()==addButton){this.CalculateEventHandel();result=userFloata+userFloatb;// System.out.println(""+result);textField3.setText(""+result);}else if(e.getSource()==subtractButton){ this.CalculateEventHandel();result=userFloata-userFloatb;textField3.setText(""+result);}else if(e.getSource()==rideButton){this.CalculateEventHandel();result=userFloata*userFloatb;textField3.setText(""+result);}else if(e.getSource()==divideButton){this.CalculateEventHandel();result=userFloata/userFloatb;textField3.setText(""+result);}}}。
java 计算器 实验报告

java 计算器实验报告Java计算器实验报告一、引言计算器是我们日常生活中常用的工具之一,它可以帮助我们进行简单的数学运算。
随着计算机技术的发展,计算器也逐渐从传统的机械计算器转变为电子计算器,甚至是手机上的应用程序。
本实验旨在利用Java编程语言设计一个简单的计算器,并通过实现基本的数学运算功能来加深对Java编程的理解和应用。
二、实验目的1. 掌握Java编程语言的基本语法和数据类型;2. 理解面向对象编程的概念和思想;3. 学习如何使用Java编写简单的用户界面;4. 实现基本的数学运算功能。
三、实验方法本实验采用Java编程语言,使用Eclipse集成开发环境进行开发。
主要步骤如下:1. 创建一个Java项目;2. 在项目中创建一个计算器类,实现基本的数学运算功能;3. 使用Swing库设计一个简单的用户界面,包括数字按钮、运算符按钮和显示结果的文本框;4. 将用户界面与计算器类进行关联,实现用户输入和计算结果的交互。
四、实验过程1. 创建Java项目并导入Swing库在Eclipse中创建一个新的Java项目,并导入Swing库,以便后续设计用户界面。
2. 设计用户界面使用Swing库提供的组件,设计一个简单的用户界面。
界面包括数字按钮、运算符按钮和显示结果的文本框。
通过设置按钮的事件监听器,实现用户输入和计算结果的显示。
3. 实现计算器类创建一个名为"Calculator"的Java类,该类包含实现基本数学运算的方法。
例如,实现加法运算的方法"add",减法运算的方法"subtract"等。
4. 关联用户界面和计算器类在用户界面的事件监听器中,调用计算器类的相应方法,实现用户输入和计算结果的交互。
五、实验结果经过以上步骤的设计和实现,我们成功创建了一个简单的Java计算器。
用户可以通过界面上的按钮输入数字和运算符,并在文本框中显示计算结果。
java简易计算器设计思路

java简易计算器设计思路Java简易计算器设计思路一、引言计算器是我们日常生活中常用的工具之一,可以帮助我们进行各种简单的数学计算。
本文将介绍如何使用Java编程语言设计一个简易的计算器。
二、功能需求我们的计算器需要具备以下基本功能:1. 实现加、减、乘、除四则运算;2. 能够处理小数、整数和负数的运算;3. 具备清除操作,清零当前计算结果;4. 能够处理连续计算,即进行多次连续的运算。
三、设计思路在设计简易计算器时,我们可以考虑以下步骤:1. 用户界面设计首先,我们需要设计一个用户界面,让用户能够输入数字和选择运算符号。
可以使用Java的Swing或JavaFX进行界面设计。
2. 输入数字和运算符在用户界面中,我们可以使用文本框和按钮来实现数字的输入和运算符的选择。
用户在文本框中输入数字,然后点击相应的按钮选择运算符号。
3. 运算处理根据用户的选择,我们可以利用Java中的if或switch语句来进行相应的运算处理。
比如用户选择加法运算,则获取用户输入的两个数字并相加。
同样,对于减法、乘法和除法运算,我们也可以使用相应的语句进行处理。
4. 显示结果最后,我们需要将计算结果显示在用户界面的文本框中。
将处理后的结果显示在界面上,让用户直观地看到计算结果。
5. 清除操作我们还可以添加一个清除按钮,使用户能够清除当前的计算结果,重新进行新的计算。
四、扩展功能除了基本的计算功能外,我们还可以考虑增加以下扩展功能:1. 添加括号功能,使计算器能够处理复杂的表达式;2. 添加开平方、取余、求幂等高级运算;3. 添加历史记录功能,保存用户之前的计算结果;4. 添加单位转换功能,使计算器能够进行长度、重量、温度等单位之间的转换。
五、总结通过以上设计思路,我们可以实现一个简易的计算器,满足用户的基本计算需求。
同时,我们可以根据实际需求添加扩展功能,提升计算器的实用性和灵活性。
希望本文对您设计和实现一个Java简易计算器有所帮助。
科学计算器Java源代码
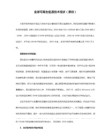
pack();
setVisible(trห้องสมุดไป่ตู้e);
}
public void setup() //搭建次程序的图形应用界面
{
pan1.setLayout(new BorderLayout());
Labelnow.setFont(wordFont1);
Labelnow.setBackground(Color.gray);
pan3.add(Labelnow);
Labeltime.setBackground(Color.gray);
Labeltime.setFont(wordFont1);
System.out.println("即将计算的表达式是:"+QU);
Labelnow.setText("计算程序正在运行");
appendchar( button20.getLabel());
appendchar( countall ( QU ) );
private Button button9=new Button("9");
private Button button10=new Button("0");
private Button button11=new Button("+");
private Button button12=new Button("-");
{
super("我的科学计算器");
setup();
JAVA实现简单计算器

JAVA实现简单计算器```import java.util.Scanner;public class Calculatorpublic static void main(String[] args)Scanner input = new Scanner(System.in);System.out.println("欢迎使用简单计算器!");boolean running = true;while (running)System.out.println("请输入计算表达式(格式:操作数运算符操作数,例如:2 + 3),输入 exit 退出:");String expression = input.nextLine(;if (expression.equalsIgnoreCase("exit"))running = false;continue;}String[] tokens = expression.split(" ");if (tokens.length != 3)System.out.println("输入不合法,请重新输入!");continue;}trydouble operand1 = Double.parseDouble(tokens[0]);String operator = tokens[1];double operand2 = Double.parseDouble(tokens[2]);double result = calculate(operand1, operand2, operator);System.out.println("计算结果为:" + result);} catch (NumberFormatException e)System.out.println("操作数必须为数字,请重新输入!");} catch (IllegalArgumentException e)System.out.println(e.getMessage();}}System.out.println("感谢使用简单计算器,再见!");}public static double calculate(double operand1, double operand2, String operator)double result;switch (operator)case "+":result = operand1 + operand2;break;case "-":result = operand1 - operand2;break;case "*":result = operand1 * operand2;break;case "/":if (operand2 == 0)throw new IllegalArgumentException("除数不能为零!"); }result = operand1 / operand2;break;default:throw new IllegalArgumentException("运算符不合法!"); }return result;}```以上是一个简单的JAVA实现计算器的程序。
java课程设计--科学计算器

目录1 课设任务及要求 (1)1.1课设任务: (1)1.2创新要求: (1)1.3设计要求 (1)2 需求分析 (2)2.1 设计背景 (2)2.2 开发的技术及功能 (2)3 设计思路 (3)4 详细设计 (4)4.1功能实现: (4)4.4 程序设计过程 (6)5 系统调试 (6)5.1 运行调试 (6)6 参考文献 (7)附录 (8)1 课设任务及要求1.1课设任务:⑴、设计的计算器应用程序可以完成加法、减法、乘法、除法以及取余运算(可以进行浮点数和负数的运算);⑵、有求倒数、退格和清零功能。
1.2创新要求:能进行正切、余弦,以及求平方根、指数(包括对e)、自然对数运算。
1.3设计要求①设计的计算器应用程序可以完成加法、减法、乘法、除法和取余运算。
且有小数点、正负号、求倒数、退格和清零功能。
②课程设计可选用Eclipse、JBuilder、NetBeans等作为开发平台以提高开发效率,通过资料查阅和学习尽可能熟练掌握其中一种集成开发环境。
③认真按时完成课程设计报告,课程设计报告内容包括:设计任务与要求、需求分析、设计思路、详细设计、运行调试与分析讨论和设计体会与小结六个部分。
2 需求分析2.1 设计背景设计这个计算器主要是参考Windows操作系统中自带的计算器,由于编者水平和时间的限制,不能将计算器设计到科学型及其他更复杂的类型,在设计过程中还参考了一些其他的优秀设计。
但本计算器除了常用的加减乘除(可以进行浮点和负数运算)这些基本运算外,还有求余、求倒、退格、清零,甚至还能进行一些复杂科学的运算,比如余弦(cos)、正切(tan)、指数运算(pow)、自然对数运算(log)、求平方根(sqrt)以及对e的指数运算(exp),并且还能进行连续运算。
总体上说来,本计算器设计简单,代码很少,程序很小,但功能却很强大,这是同类计算器所不具备的。
2.2 开发的技术及功能本课程设计是要做一个图形界面的计算器,其界面主要是由swing组件中的控件构成。
Java计算器

import javax.swing.*;import javax.swing.event.*;import java.awt.*;import java.awt.event.*;public class calculator extends JFrame implements ActionListener{//这里我把JFrame写成Frame,这个错误找了好久JFrame frame;private JButton jia=new JButton("+");private JButton jian=new JButton("-");private JButton cheng=new JButton("*");private JButton chu=new JButton("/");private JButton qiuyi=new JButton("%");private JButton deng=new JButton("=");private JButton fu=new JButton("+/-");private JButton dian=new JButton(".");private JButton kai=new JButton("sqrt");private JButton diao=new JButton("1/x");private JButton aa=new JButton("A");private JButton bb=new JButton("B");private JButton cc=new JButton("C");private JButton dd=new JButton("D");private JButton ee=new JButton("E");private JButton ff=new JButton("F");private TextField k1=new TextField();private objConversion convert = new objConversion();JMenuItem copy,paste,s,t,help,about,me;JRadioButton sixteen,ten,eight,two;JButton backspace,ce,c,num0,num1,num2,num3,num4,num5,num6,num7,num8,num9; Container cp;JTextField text;String copycontent="";boolean clickable=true,clear=true;int all=0;double qian;String fuhao;int jin=10,first=1;public calculator(){setTitle("计算器-杨彩制作");setSize(400,300);setLocation(250,200);text=new JTextField(25);// text.setEnabled(false);text.setText("0.");text.setHorizontalAlignment(JTextField.RIGHT);//从右到左JPanel cp1=new JPanel();JPanel cp2=new JPanel();JPanel cp3=new JPanel();cp=getContentPane();cp.add(cp1,"North");cp.add(cp2,"Center");cp.add(cp3,"South");cp1.setLayout(new GridLayout(1,6));cp2.setLayout(new GridLayout(2,4));cp3.setLayout(new GridLayout(6,6));sixteen=new JRadioButton("十六进制");sixteen.setVisible(false);ten=new JRadioButton("十进制",true);ten.setVisible(false);eight=new JRadioButton("八进制");eight.setVisible(false);two=new JRadioButton("二进制");two.setVisible(false);sixteen.addActionListener(this);ten.addActionListener(this);eight.addActionListener(this);two.addActionListener(this);ButtonGroup btg=new ButtonGroup();btg.add(sixteen);btg.add(ten);btg.add(eight);btg.add(two);JTextField t3=new JTextField(25);cp1.add(text);// text.setEnabled(false);text.setEditable(false);text.setBackground(new Color(255, 255, 255));cp2.add(sixteen);cp2.add(ten);cp2.add(eight);cp2.add(two);backspace=new JButton("Backspace"); backspace.setForeground(new Color(255,0,0)); backspace.addActionListener(this);ce=new JButton("CE");ce.setForeground(new Color(255,0,0));ce.addActionListener(this);c=new JButton("C");c.setForeground(new Color(255,0,0));c.addActionListener(this);k1.setVisible(false);cp2.add(k1);cp2.add(backspace);cp2.add(ce);cp2.add(c);num0=new JButton("0");num1=new JButton("1");num2=new JButton("2");num3=new JButton("3");num4=new JButton("4");num5=new JButton("5");num6=new JButton("6");num7=new JButton("7");num8=new JButton("8");num9=new JButton("9");cp3.add(num7);num7.addActionListener(this);cp3.add(num8);num8.addActionListener(this);cp3.add(num9);num9.addActionListener(this);cp3.add(chu);chu.setForeground(new Color(255,0,0));chu.addActionListener(this);cp3.add(kai);kai.addActionListener(this);cp3.add(num4);num4.addActionListener(this);cp3.add(num5);num5.addActionListener(this);cp3.add(num6);num6.addActionListener(this);cp3.add(cheng);cheng.setForeground(new Color(255,0,0)); cheng.addActionListener(this);cp3.add(qiuyi);qiuyi.addActionListener(this);cp3.add(num1);num1.addActionListener(this);cp3.add(num2);num2.addActionListener(this);cp3.add(num3);num3.addActionListener(this);cp3.add(jian);jian.setForeground(new Color(255,0,0)); jian.addActionListener(this);cp3.add(diao);diao.addActionListener(this);cp3.add(num0);num0.addActionListener(this);cp3.add(fu);fu.addActionListener(this);cp3.add(dian);dian.addActionListener(this);cp3.add(jia);jia.setForeground(new Color(255,0,0)); jia.addActionListener(this);cp3.add(deng);deng.setForeground(new Color(255,0,0)); deng.addActionListener(this);cp3.add(aa);aa.addActionListener(this);cp3.add(bb);bb.addActionListener(this);cp3.add(cc);cc.addActionListener(this);cp3.add(dd);dd.addActionListener(this);cp3.add(ee);ee.addActionListener(this);cp3.add(ff);ff.addActionListener(this);aa.setVisible(false);bb.setVisible(false);cc.setVisible(false);dd.setVisible(false);ee.setVisible(false);ff.setVisible(false);JMenuBar mainMenu = new JMenuBar();setJMenuBar(mainMenu);JMenu editMenu = new JMenu("编辑");JMenu viewMenu = new JMenu("查看");JMenu helpMenu = new JMenu("帮助");mainMenu.add(editMenu);mainMenu.add(viewMenu);mainMenu.add(helpMenu);copy = new JMenuItem(" 复制");paste = new JMenuItem(" 粘贴");KeyStroke copyks=KeyStroke.getKeyStroke(KeyEvent.VK_C,Event.CTRL_MASK);copy.setAccelerator(copyks);//设置退出菜单选项加上快捷键KeyStroke pasteks=KeyStroke.getKeyStroke(KeyEvent.VK_V,Event.CTRL_MASK);paste.setAccelerator(pasteks);//设置退出菜单选项加上快捷键editMenu.add(copy);editMenu.add(paste);copy.addActionListener(this);paste.addActionListener(this);t = new JMenuItem("●标准型");s = new JMenuItem(" 科学型");viewMenu.add(t);viewMenu.add(s);t.addActionListener(this);s.addActionListener(this);help = new JMenuItem(" 帮助主题");about = new JMenuItem(" 关于计算器");me = new JMenuItem(" 作者主页");helpMenu.add(help);helpMenu.add(about);helpMenu.add(me);help.addActionListener(this);about.addActionListener(this);me.addActionListener(this);addWindowListener(new WindowDestroyer());//结束窗口}public void actionPerformed(ActionEvent e){//响应动作代码if(first==1)text.setText("");first=0;//第一次把文本框0.清空Object temp = e.getSource();if(temp==copy){copycontent = text.getText();}if(temp==paste){text.setText(text.getText()+copycontent);}if(temp==t){//标准sixteen.setVisible(false);ten.setVisible(false);eight.setVisible(false);two.setVisible(false);t.setText("●标准型");s.setText(" 科学型");aa.setVisible(false);bb.setVisible(false);cc.setVisible(false);dd.setVisible(false);ee.setVisible(false);ff.setVisible(false);}if(temp==s){//科学sixteen.setVisible(true);ten.setVisible(true);eight.setVisible(true);two.setVisible(true);t.setText(" 标准型");s.setText("●科学型");aa.setVisible(true);bb.setVisible(true);cc.setVisible(true);dd.setVisible(true);ee.setVisible(true);ff.setVisible(true);aa.setEnabled(false);bb.setEnabled(false);cc.setEnabled(false);dd.setEnabled(false);ee.setEnabled(false);ff.setEnabled(false);}if(temp==help){ //打开系统帮助文件要查资料try{String filePath = "C:/WINDOWS/Help/calc.chm";Runtime.getRuntime().exec("cmd.exe /c "+filePath);}catch(Exception eeee){System.out.println("打开系统的计算器出错");}}if(temp==about){JOptionPane.showMessageDialog(frame," Java计算器\n 杨彩制作\n\n /m/yangcai","关于计算器",RMATION_MESSAGE); ;}if(temp==me){try{Process p = Runtime.getRuntime().exec("explorer/m/yangcai");}catch(Exception eeee){System.out.println("打开指定网页出错");}}try{if(temp==sixteen){String hex=text.getText();int inthex=Integer.parseInt(hex,jin);//先把数变为10进制text.setText(convert.decHex(inthex)) ;jin=16;aa.setEnabled(true);bb.setEnabled(true);cc.setEnabled(true);dd.setEnabled(true);ee.setEnabled(true);ff.setEnabled(true);num2.setEnabled(true);num3.setEnabled(true);num4.setEnabled(true);num5.setEnabled(true);num6.setEnabled(true);num7.setEnabled(true);num8.setEnabled(true);num9.setEnabled(true);}if(temp==eight){String oct =text.getText();int intoct=Integer.parseInt(oct,jin);text.setText(convert.decOct(intoct)) ;jin=8;aa.setEnabled(false);bb.setEnabled(false);cc.setEnabled(false);dd.setEnabled(false);ee.setEnabled(false);ff.setEnabled(false);num2.setEnabled(true);num3.setEnabled(true);num4.setEnabled(true);num5.setEnabled(true);num6.setEnabled(true);num7.setEnabled(true);num8.setEnabled(false);num9.setEnabled(false);}if(temp==two){String bin=text.getText();int intbin=Integer.parseInt(bin,jin);text.setText(convert.decBin(intbin));jin=2;aa.setEnabled(false);bb.setEnabled(false);cc.setEnabled(false);dd.setEnabled(false);ee.setEnabled(false);ff.setEnabled(false);num2.setEnabled(false);num3.setEnabled(false);num4.setEnabled(false);num5.setEnabled(false);num6.setEnabled(false);num7.setEnabled(false);num8.setEnabled(false);num9.setEnabled(false);}if(temp==ten){String dec=text.getText();int intdec=Integer.parseInt(dec,jin);// text.setText(convert.decDec(intdec)); //本句会把123变成321 text.setText(intdec+"");jin=10;aa.setEnabled(false);bb.setEnabled(false);cc.setEnabled(false);dd.setEnabled(false);ee.setEnabled(false);ff.setEnabled(false);num2.setEnabled(true);num3.setEnabled(true);num4.setEnabled(true);num5.setEnabled(true);num6.setEnabled(true);num7.setEnabled(true);num8.setEnabled(true);num9.setEnabled(true);}}catch(Exception ee){System.out.println("转换出错,可能你没有输入任何字符");text.setText("0");clear=false;}if(temp==backspace){//退格String s = text.getText();text.setText("");for (int i = 0; i < s.length() - 1; i++){char a = s.charAt(i);text.setText(text.getText() + a);}}if (temp==ce){text.setText("0.");clear=true;first=1;}if (temp==c){text.setText("0."); ;clear=true;first=1;}if(temp==num0){if(clear==false)//判断是否点击了符号位text.setText("");text.setText(text.getText()+"0");}if(temp==num1){if(clear==false)text.setText("");text.setText(text.getText()+"1");clear=true;//第二次不在清空(前二句)}if(temp==num2){if(clear==false)text.setText("");text.setText(text.getText()+"2");clear=true;}if(temp==num3){if(clear==false)text.setText("");text.setText(text.getText()+"3");clear=true;}if(temp==num4){if(clear==false)text.setText("");text.setText(text.getText()+"4");clear=true;}if(temp==num5){if(clear==false)text.setText("");text.setText(text.getText()+"5");clear=true;}if(temp==num6){if(clear==false)text.setText("");text.setText(text.getText()+"6");clear=true;}if(temp==num7){if(clear==false)text.setText("");text.setText(text.getText()+"7");clear=true;}if(temp==num8){if(clear==false)text.setText("");text.setText(text.getText()+"8");clear=true;}if(temp==num9){if(clear==false)text.setText("");text.setText(text.getText()+"9");clear=true;}if(temp==aa){text.setText(text.getText()+"A");}if(temp==bb){text.setText(text.getText()+"B");}if(temp==cc){text.setText(text.getText()+"C");}if(temp==dd){text.setText(text.getText()+"D");}if(temp==ee){text.setText(text.getText()+"E");}if(temp==ff){text.setText(text.getText()+"F");}if(temp==dian){clickable=true;for (int i = 0; i < text.getText().length(); i++) if ('.' == text.getText().charAt(i)){clickable=false;break;} //第一层判断是否里面含有小数点;if(clickable==true)//第二坛判断text.setText(text.getText()+".");}try{if(temp==jia){//加法qian=Double.parseDouble(text.getText());fuhao="+";clear=false;}if(temp==jian){qian=Double.parseDouble(text.getText());fuhao="-";clear=false;;}if(temp==cheng){qian=Double.parseDouble(text.getText());fuhao="*";clear=false;}if(temp==chu){qian=Double.parseDouble(text.getText());fuhao="/";clear=false;}if(temp==deng){double ss=Double.parseDouble(text.getText());text.setText("");if(fuhao=="+")text.setText(qian+ss+"");if(fuhao=="-")text.setText(qian-ss+"");if(fuhao=="*")text.setText(qian*ss+"");if(fuhao=="/")text.setText(qian/ss+"");clear=false;//要清空前一次的数据;}if(temp==kai){String s = text.getText();if (s.charAt(0) == '-'){text.setText("负数不能开根号");}elsetext.setText(Double.toString(ng.Math.sqrt(Double.parseDouble(text.getText() ))));clear=false;}if(temp==diao){if (text.getText().charAt(0) == '0'&&text.getText().length() == 1){text.setText("除数不能为零");}else{boolean isDec = true;int i, j, k;String s = Double.toString(1 / Double.parseDouble(text.getText()));for (i = 0; i < s.length(); i++)if (s.charAt(i) == '.')break;for (j = i + 1; j < s.length(); j++)if (s.charAt(j) != '0'){isDec = false;break;}if (isDec == true){String stemp = "";for (k = 0; k < i; k++)stemp += s.charAt(k);text.setText(stemp);}elsetext.setText(s);}clear=false;}if(temp==qiuyi){text.setText("0");clear=false;}if (temp == fu){ //导师,此方法参考书中例子boolean isNumber = true;String s = text.getText();for (int i = 0; i < s.length(); i++)if (! (s.charAt(i) >= '0' && s.charAt(i) <= '9' || s.charAt(i) == '.' || s.charAt(i) == '-')){isNumber = false;break;}if (isNumber == true){//如果当前字符串首字母有'-'号,代表现在是个负数,再按下时,则将首符号去掉if (s.charAt(0) == '-'){text.setText("");for (int i = 1; i < s.length(); i++){char a = s.charAt(i);text.setText(text.getText() + a);}}//如果当前字符串第一个字符不是符号,则添加一个符号在首字母处elsetext.setText('-' + s);}}}catch(Exception eee){System.out.println("运算时,首先输入数字或字符"); text.setText("运算出错");clear=false;}}class WindowDestroyer extends WindowAdapter{//退出窗口动作public void windowClosing(WindowEvent e){System.exit(0);}}class objConversion{//导师,本进制类参考了CSMD类转换例子public void objConversion (){}public String decDec (int decNum){//10String strDecNum = Integer.toString(decNum);for (int i = strDecNum.length(); i < 3; i++){strDecNum = "0" + strDecNum;}// return strDecNum;return invert (strDecNum, 5);}public String decHex (int decNum){//10 to 16String strHexNum = "";int currentNum = 0;while (decNum != 0){if (decNum > 15){currentNum = decNum % 16;decNum /= 16;}else{currentNum = decNum;decNum = 0;}switch (currentNum){case 15: strHexNum += "F";break;case 14: strHexNum += "E";break;case 13: strHexNum += "D";break;case 12: strHexNum += "C";break;case 11: strHexNum += "B";break;case 10: strHexNum += "A";break;default: strHexNum += Integer.toString(currentNum);break;}}return invert (strHexNum, 2);}public String decOct (int decNum){//10 to 8String strOctNum = "";while (decNum != 0){if (decNum > 7){strOctNum += Integer.toString(decNum % 8); decNum /= 8;}else{strOctNum += Integer.toString(decNum);decNum = 0;}}return invert (strOctNum, 3);}public String decBin (int decNum){//10 to 2String strBinNum = "";while (decNum != 0){if (decNum > 1){strBinNum += Integer.toString(decNum % 2); decNum /= 2;}else{strBinNum += Integer.toString(decNum);decNum = 0;}}return invert (strBinNum, 8);}private String invert (String strNum, int minLength) //转换长度{String answer = "";int length = strNum.length();if (length < minLength){for (int padding = (minLength - length); padding > 0; padding--) {answer += "0";}}for (int i = length; i > 0; i--){answer += strNum.charAt (i - 1);}return answer;}}public static void main(String arg[])//产生窗口{calculator win = new calculator();win.setVisible(true);}}。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
tf2.disable();
// 实例化所有按钮、设置其前景色并注册按钮和键盘监听器
int i;
for (i = 0; i < 45; i++) {
bt[i] = new Button(btCmd[i]);
p0.setBounds(10, 0, 290, 45);
// 添加面板p1中的组件和设置其在框架中的位置和大小
p1.setLayout(gl1);
p1.add(tf2);
p1.add(bt[0]);
p1.add(bt[1]);
p1.add(bt[2]);
}
@SuppressWarnings("deprecation")
public void setButtonAndTextField() {
// 定义按钮上显示的字符
String btCmd[] = { "Backspace", "CE", "C", "MC", "MR", "MS", "M+", "7",
op = i;
break;
}
}
if (op <= 10 && i < 23) {// 如果当前按下的是双目运算符
x = Double.parseDouble(tf1.getText().trim());// 获取用户输入
"sqrt", "%", "1/X", "sin", "cos", "tan", "ln", "log", "n!",
"x^3", i;
for (i = 0; i < 23; i++) {
if (cmd == operation[i]) {// 求运算op码
p2.setBounds(10, 85, 40, 180);
// 添加面板p3中的组件并设置其在框架中的位置和大小
p3.setLayout(gl3);// 设置p3的布局
for (i = 7; i < 27; i++) {
p3.add(bt[i]);
}
* 入口参数: 计算器按钮上的相应的字串或键盘键入的数字和运算符
* 实现功能: 获得用户输入,进行运算,并显示运算结果
* 主要思路: 定义一字符串数组,内容为运算符键值,根据入口参数的运算符与运算符
* 键值数组比较求得一操作码op,把op分成单目运算符和双目运算符, 根据op进行相应
setIconImage(image);
setTitle("计算器");
setMenu();// 设置菜单项
setLayout(null);
setButtonAndTextField();// 实例化按钮与文本区域
// 创建一个空字符串缓冲区
str = new StringBuffer();
tf1.setHorizontalAlignment(JTextField.RIGHT);
tf1.setEnabled(false);
tf1.setDisabledTextColor(Color.black);
tf1.setText("0");
tf2 = new TextField(5);// 显示记忆的索引值
"Lsh", "tan", "n!", "x^2", "Rsh", "Not" };
GridLayout gl1, gl2, gl3, gl4;
JPanel p0, p1, p2, p3, p4;
gl1 = new GridLayout(1, 4, 10, 0);// 实例化四个面板的布局
Button bt[] = new Button[45]; // 45个按钮
StringBuffer str; // 显示屏所显示的字符串
String strTmp;// 暂存显示屏的结果,用于复制、粘贴
double x, y; // x和y为两个运算数
static double m; // 记忆值
* 运算并显示结果
*/
public void keyAndButtonAction(String cmd) {
try {
String operation[] = { // 运算符键值
"+", "-", "*", "/", "x^y", "Mod", "And", "Or", "Xor", "Lsh", "Rsh",
*
*/
private static final long serialVersionUID = -6728795026953123509L;
JMenuItem menu[]; // 菜单项
JTextField tf1; // 计算器显示屏
TextField tf2; // 用于显示是否有记忆值
p1.setBounds(10, 45, 290, 30);
// 添加面板p2中的组件并设置其的框架中的位置和大小
p2.setLayout(gl2);
p2.add(bt[3]);
p2.add(bt[4]);
p2.add(bt[5]);
p2.add(bt[6]);
bt[i].addActionListener(new btEvent());
bt[i].addKeyListener(new keyEvent());
if (i <= 6 || (i % 5 == 0 && i < 27) || i == 26) {
bt[i].setForeground(Color.red);
strTmp = new String();
setResizable(false);// 禁止调整框架的大小
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBackground(Color.white);
setVisible(true);// 显示框架
viewMenu.add(menu[2]);
viewMenu.add(menu[3]);
aboutMenu.add(menu[4]);
aboutMenu.add(menu[5]);
menuBar.add(editMenu);
menuBar.add(viewMenu);
* "log", "n!", "x^3", "x^2", "Not"
*/
public D() {
// 更换程序图标为计算器图标image.jpg
Toolkit tk = Toolkit.getDefaultToolkit();
Image image = tk.createImage("image.jpg"); /* image.gif计算器图标 */
"8", "9", "/", "sqrt", "4", "5", "6", "*", "%", "1", "2", "3",
"-", "1/X", "0", "+/-", ".", "+", "=", "PI", "sin", "ln",
"x^y", "Mod", "And", "E", "cos", "log", "x^3", "Or", "Xor",
gl2 = new GridLayout(4, 1, 0, 15);
gl3 = new GridLayout(4, 5, 10, 10);
gl4 = new GridLayout(3, 6, 10, 10);
tf1 = new JTextField(26);// 显示屏
p1 = new JPanel();
p2 = new JPanel();
p3 = new JPanel();
p4 = new JPanel();
// 添加面板p0中的组件和设置其在框架中的位置和大小
p0.add(tf1);
p0.setFocusable(false);
package d;
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
import java.io.IOException;