《ACM算法与程序设计》期末问题集
算法与程序设计试题带答案
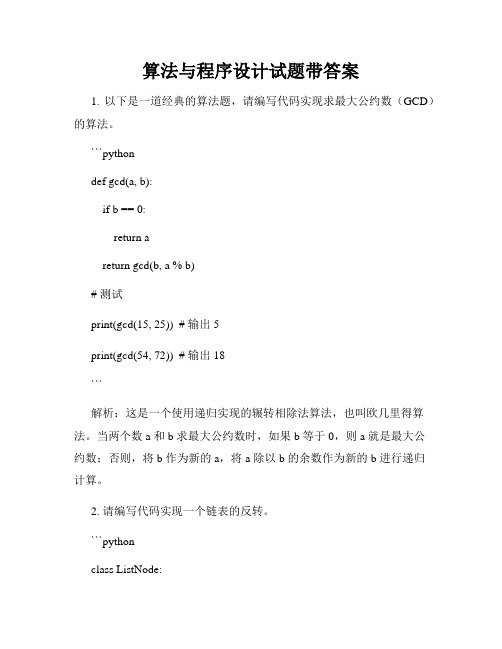
算法与程序设计试题带答案1. 以下是一道经典的算法题,请编写代码实现求最大公约数(GCD)的算法。
```pythondef gcd(a, b):if b == 0:return areturn gcd(b, a % b)# 测试print(gcd(15, 25)) # 输出 5print(gcd(54, 72)) # 输出 18```解析:这是一个使用递归实现的辗转相除法算法,也叫欧几里得算法。
当两个数 a 和 b 求最大公约数时,如果 b 等于 0,则 a 就是最大公约数;否则,将 b 作为新的 a,将 a 除以 b 的余数作为新的 b 进行递归计算。
2. 请编写代码实现一个链表的反转。
```pythonclass ListNode:def __init__(self, val=0, next=None):self.val = valself.next = nextdef reverse_linked_list(head):prev = Nonecurr = headwhile curr:next_node = curr.nextcurr.next = prevprev = currcurr = next_nodereturn prev# 测试node1 = ListNode(1)node2 = ListNode(2)node3 = ListNode(3)node1.next = node2node2.next = node3reversed_head = reverse_linked_list(node1)while reversed_head:print(reversed_head.val)reversed_head = reversed_head.next```解析:这是一个经典的链表反转算法。
使用 prev、curr、next_node 三个指针来实现,其中 prev 用于保存上一个节点,curr 用于保存当前节点,next_node 用于保存下一个节点。
大学ACM考试题目及作业答案整理

ACM作业与答案整理1、平面分割方法:设有n条封闭曲线画在平面上,而任何两条封闭曲线恰好相交于两点,且任何三条封闭曲线不相交于同一点,问这些封闭曲线把平面分割成的区域个数。
#include <iostream.h>int f(int n){if(n==1) return 2;else return f(n-1)+2*(n-1);}void main(){int n;while(1){cin>>n;cout<<f(n)<<endl;}}2、LELE的RPG难题:有排成一行的n个方格,用红(Red)、粉(Pink)、绿(Green)三色涂每个格子,每格涂一色,要求任何相邻的方格不能同色,且首尾两格也不同色.编程全部的满足要求的涂法.#include<iostream.h>int f(int n){if(n==1) return 3;else if(n==2) return 6;else return f(n-1)+f(n-2)*2;}void main(){int n;while(1){cin>>n;cout<<f(n)<<endl;}}3、北大ACM(1942)Paths on a GridTime Limit: 1000MS Memory Limit: 30000K DescriptionImagine you are attending your math lesson at school. Once again, you are bored because your teacher tells things that you already mastered years ago (this time he's explaining that (a+b)2=a2+2ab+b2). So you decide to waste your time with drawing modern art instead.Fortunately you have a piece of squared paper and you choose a rectangle of size n*m on the paper. Let's call this rectangle together with the lines it contains a grid. Starting at the lower left corner of the grid, you move your pencil to the upper right corner, taking care that it stays on the lines and moves only to the right or up. The result is shown on the left:Really a masterpiece, isn't it? Repeating the procedure one more time, you arrive with the picture shown on the right. Now you wonder: how many different works of art can you produce?InputThe input contains several testcases. Each is specified by two unsigned 32-bit integers n and m, denoting the size of the rectangle. As you can observe, the number of lines of the corresponding grid is one more in each dimension. Input is terminated by n=m=0.OutputFor each test case output on a line the number of different art works that can be generated using the procedure described above. That is, how many paths are there on a grid where each step of the path consists of moving one unit to the right orone unit up? You may safely assume that this number fits into a 32-bit unsigned integer.Sample Input5 41 10 0Sample Output1262#include<iostream>using namespace std;longlong f(long long m, long long n){if(n==0) return 1;else return f(m-1,n-1)*m/n;}int main(){longlongm,n;while(scanf("%I64d %I64d",&n,&m) &&n+m){printf("%I64d\n",f(m+n,min(m,n)));}return 0;}1、(并查集)若某个家族人员过于庞大,要判断两个是否是亲戚,确实还很不容易,现在给出某个亲戚关系图,求任意给出的两个人是否具有亲戚关系。
acm试题及答案2
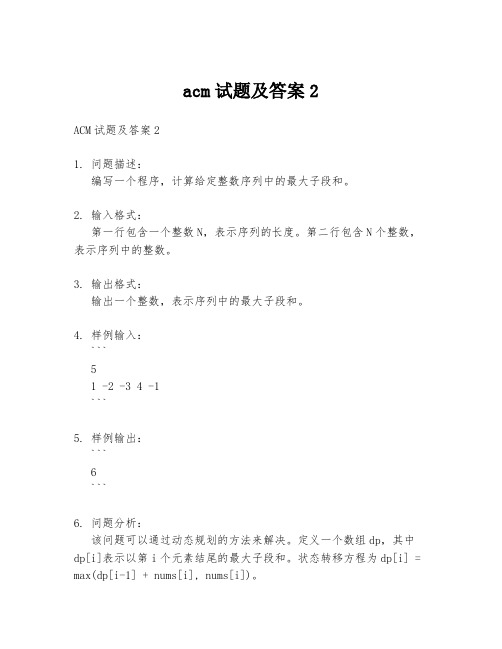
acm试题及答案2ACM试题及答案21. 问题描述:编写一个程序,计算给定整数序列中的最大子段和。
2. 输入格式:第一行包含一个整数N,表示序列的长度。
第二行包含N个整数,表示序列中的整数。
3. 输出格式:输出一个整数,表示序列中的最大子段和。
4. 样例输入:```51 -2 -34 -1```5. 样例输出:```6```6. 问题分析:该问题可以通过动态规划的方法来解决。
定义一个数组dp,其中dp[i]表示以第i个元素结尾的最大子段和。
状态转移方程为dp[i] = max(dp[i-1] + nums[i], nums[i])。
7. 算法实现:```pythondef maxSubArray(nums):n = len(nums)dp = [0] * ndp[0] = nums[0]max_sum = nums[0]for i in range(1, n):dp[i] = max(dp[i-1] + nums[i], nums[i])max_sum = max(max_sum, dp[i])return max_sum```8. 复杂度分析:时间复杂度为O(n),其中n为序列的长度。
空间复杂度为O(n)。
9. 测试用例:- 输入:`[3, -2, 4]`输出:`5`- 输入:`[-2, 1, -3, 4, -1, 2, 1, -5, 4]`输出:`6`10. 注意事项:- 确保输入的序列长度N大于等于1。
- 序列中的整数可以是负数或正数。
- 输出结果应该是一个整数。
11. 扩展思考:- 如何优化算法以减少空间复杂度?- 如果序列中的整数是浮点数,算法是否仍然有效?12. 参考答案:- 可以通过只使用一个变量来存储最大子段和,以及一个变量来存储当前子段和,从而将空间复杂度优化到O(1)。
- 如果序列中的整数是浮点数,算法仍然有效,但需要注意浮点数运算的精度问题。
ACM试题及参考答案

1. 给定一个矩阵M(X, Y),列集为X ,行集为Y 。
如果存在对其列的一个排序,使得每一行的元素都严格递增,称M 是一个次序保持矩阵。
例如下图中存在一个排序x 4,x 1,x 2,x 3,x 5I ⊆X ,满足:子矩阵M(I,Y)是次序保持矩阵。
[测试数据] 矩阵M :[测试数据结果] I={ x 1,x 3,x 4,x 7,x 8}[解题思路] 将该问题归约为在一个有向图中找一条最长路径的问题。
给定矩阵M=(a ij ),行集Y ,列集X ,行子集J ⊆Y ,定义有向图D A =(V A ,E A ),其中V A 含有|X|个顶点,每个顶点代表X 中的一列,如果顶点u ,v 对应的列x u ,x v 满足,对于任意的j ∈J ,u v ij ij a a <,则有一条从u 到v 的弧(u ,v )∈E 。
显然,D A 是个无环图,可以在O(|X|2)时间内构造完毕。
对于任意的条件子集J ,A(I,J)是次序保持的当且仅当对应于J 中条件的顶点在D A 中构成一条有向路径。
从而我们只需在有向图D A 中找一条最长路径,该问题可在O(|V A |+| E A |)时间内完成。
按上面的方法构造有向图如下:有向图中找最长路径的线性时间算法。
一些表示方法如下:d out (u )为顶点u 的出度,d in (u )为顶点u 的入度,source 为入度为0的顶点,sink 为出度为0的顶点,N out (u )为u 指向的邻接点集合,P uv 为从u 到v 的最长路,显然应从source 到sink 。
在每一步为每个顶点关联一个永久的或临时的标签。
v被赋了一个临时标签(v’,i v)表明在当前步,算法找出的最长的从source到v的有向路长度为i v,且经由v’而来。
v被赋了一个永久标签[v’,i v]表明从source到v的最长有向路长度为i v,且经由v’而来,通过回溯每个顶点的永久标签就可以找出最长有向路。
杭电acm练习题100例(删减版)
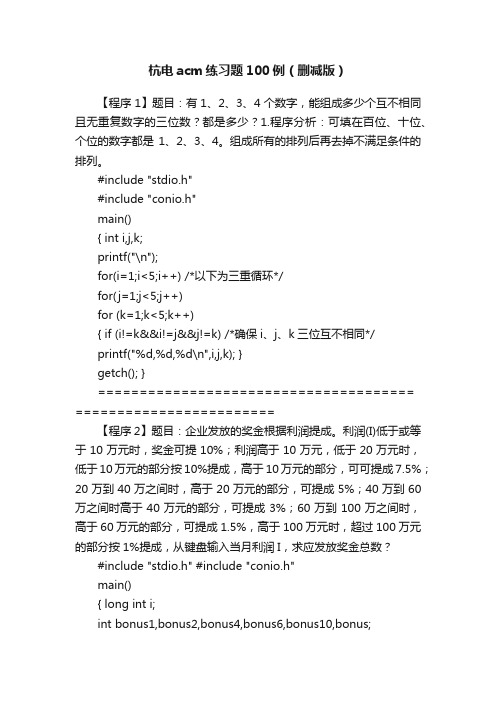
杭电acm练习题100例(删减版)【程序1】题目:有1、2、3、4个数字,能组成多少个互不相同且无重复数字的三位数?都是多少?1.程序分析:可填在百位、十位、个位的数字都是1、2、3、4。
组成所有的排列后再去掉不满足条件的排列。
#include "stdio.h"#include "conio.h"main(){ int i,j,k;printf("\n");for(i=1;i<5;i++) /*以下为三重循环*/for(j=1;j<5;j++)for (k=1;k<5;k++){ if (i!=k&&i!=j&&j!=k) /*确保i、j、k三位互不相同*/printf("%d,%d,%d\n",i,j,k); }getch(); }==============================================================【程序2】题目:企业发放的奖金根据利润提成。
利润(I)低于或等于10万元时,奖金可提10%;利润高于10万元,低于20万元时,低于10万元的部分按10%提成,高于10万元的部分,可可提成7.5%;20万到40万之间时,高于20万元的部分,可提成5%;40万到60万之间时高于40万元的部分,可提成3%;60万到100万之间时,高于60万元的部分,可提成1.5%,高于100万元时,超过100万元的部分按1%提成,从键盘输入当月利润I,求应发放奖金总数?#include "stdio.h" #include "conio.h"main(){ long int i;int bonus1,bonus2,bonus4,bonus6,bonus10,bonus;scanf("%ld",&i);bonus1=100000*0. 1;bonus2=bonus1+100000*0.75;bonus4=bonus2+200000*0.5;bonus6=bonus4+200000*0.3;bonus10=bonus6+400000*0.15;if(i<=100000)bonus=i*0.1;else if(i<=200000)bonus=bonus1+(i-100000)*0.075;else if(i<=400000)bonus=bonus2+(i-200000)*0.05;else if(i<=600000)bonus=bonus4+(i-400000)*0.03;else if(i<=1000000)bonus=bonus6+(i-600000)*0.015;elsebonus=bonus10+(i-1000000)*0.01;printf("bonus=%d",bonus);getch(); }====================================== ========================【程序3】题目:一个整数,它加上100后是一个完全平方数,再加上168又是一个完全平方数,请问该数是多少?#include "math.h"#include "stdio.h"#include "conio.h"main(){ long int i,x,y,z;for (i=1;i<100000;i++){ x=sqrt(i+100); /*x为加上100后开方后的结果*/y=sqrt(i+268); /*y为再加上168后开方后的结果*/if(x*x==i+100&&y*y==i+268)printf("\n%ld\n",i); }getch(); }====================================== ========================【程序4】题目:输入某年某月某日,判断这一天是这一年的第几天?#include "stdio.h" #include "conio.h"main(){ int day,month,year,sum,leap;printf("\nplease input year,month,day\n");scanf("%d,%d,%d",&year,&month,&day);switch(month) /*先计算某月以前月份的总天数*/{ case 1:sum=0;break;case 2:sum=31;break;case 3:sum=59;break;case 4:sum=90;break;case 5:sum=120;break;case 6:sum=151;break;case 7:sum=181;break;case 8:sum=212;break;case 9:sum=243;break;case 10:sum=273;break;case 11:sum=304;break;case 12:sum=334;break;default:printf("data error");break; }sum=sum+day; /*再加上某天的天数*/if(year%400==0||(year%4==0&&year%100!=0)) /*判断是不是闰年*/leap=1;elseleap=0;if(leap==1&&month>2) /*如果是闰年且月份大于2,总天数应该加一天*/sum++;printf("It is the %dth day.",sum);getch(); }====================================== ======================== 【程序5】题目:输入三个整数x,y,z,请把这三个数由小到大输出。
高中信息技术《算法与程序设计》期末测试题概要
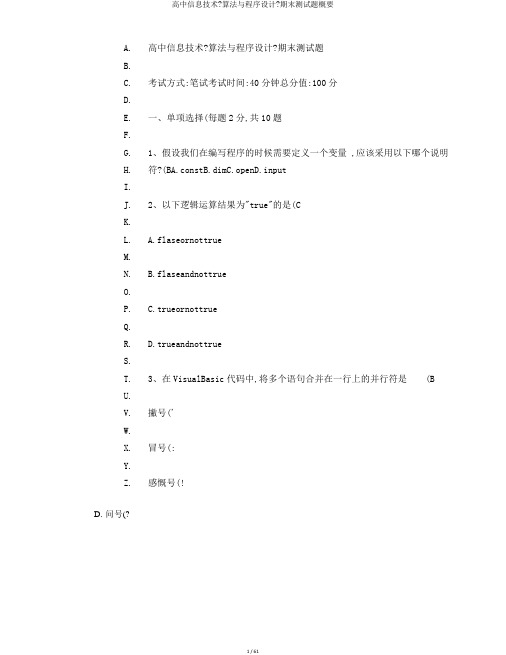
A.高中信息技术?算法与程序设计?期末测试题B.C.考试方式:笔试考试时间:40分钟总分值:100分D.E.一、单项选择(每题2分,共10题F.G.1、假设我们在编写程序的时候需要定义一个变量 ,应该采用以下哪个说明H.符?(BA.constB.dimC.openD.inputI.J.2、以下逻辑运算结果为"true"的是(CK.L. A.flaseornottrueM.N. B.flaseandnottrueO.P. C.trueornottrueQ.R. D.trueandnottrueS.T.3、在VisualBasic代码中,将多个语句合并在一行上的并行符是(BU.V.撇号('W.X.冒号(:Y.Z.感慨号(!D.问号(?4、以下运算结果中,值最大的是(C〖/表示除表示整除,mod表示求余数〗A.3\45、穷举法的适用范围是(CA.一切问题B.解的个数极多的问题C.解的个数有限且可一一列举D.不适合设计算法6、编程求1+2+3++1000的和,最适宜使用的控制结构为(CA.顺序结构B.分支结构C.循环结构D.选择结构7、以下关于算法的特征描述不正确的选项是 (CA.有穷性:算法必须在有限步之内结束B.确定性:算法的每一步必须有确切的含义C.输入:算法必须至少有一个输入D.输出:算法必须至少有一个输出8、在VB中,要想单击按钮“结束〞时结束程序,可在该按钮的(D事件过程中输入代码“End。
〞9、在VB编程中,我们使用函数与过程是为了 (AA.使程序模块化B.使程序易于阅读C.提高程序运行速度D.便于系统的编译10、以下关于人类和计算机解决实际问题说法错误的选项是(DA.人类计算速度慢而计算机快。
B.人类大脑存贮的信息量小而计算机大。
C.人类精确度一般而计算机很精确。
D.人类可以完成任务、得出结果而计算机不能。
二、判断正误(每题2分,共10题1、算法有五大特征,其中包括输入和输出这两种,意思就是说一个算法必须要有输入,也必须要有输出。
(完整版)算法设计与分析期末考试卷及答案a
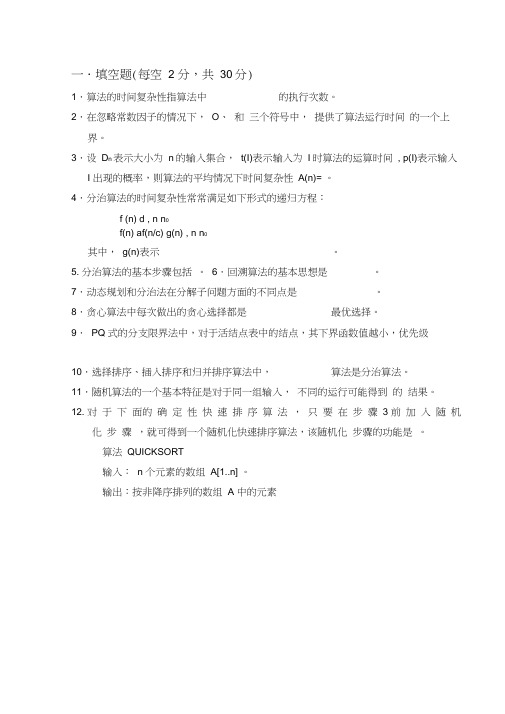
flag=false
_
_
end if
_
__
end for
A[i] A[1]
w =i
return w, A end SPLIT
二.计算题和简答题(每小题
1.用O、、 表示函数f与g之间阶的关系,并分别指出下列函数中阶最低和最高 的函数:
(1)f (n)=100g(n)=100n
(2)f(n)=6n+nlog ng(n)=3n
算法EX1
输入:正整数n,n=2k。输出:⋯
ex1(n)
end EX1过程ex1(n) if n=1 then pro1(n)
else
栏
名姓
级年
_
_系
_院学
pro2(n)
ex1(n/2) end if
return
end ex1
3.用Floyd算法求下图每一对顶点之间的最短路径长度, 计算矩阵D0,D1,D2和D3,其中Dk[i, j]表示从顶点i到顶点j的不经过编号大于
i=find ( (1) )
if i>0 then output i
else output“no solution”
end SEARCH
过程find (low, high)
//求A[low..high]中使得A[i]=i的一个下标并返回,若不存在,
//则返回0。
if (2) then return 0
生专
_
订
马的周游问题:给出一个nxn棋盘,已知一个中国象棋马在
_
_
棋盘上的某个起点位置(x0, y0),求一条访问每个棋盘格点恰好
_
_
一次,最后回到起点的周游路线。 (设马走日字。)
整理出ACM所有题目及答案

1000 A + B ProblemProblem DescriptionCalculate A + B .InputEach line will contain two integers A and B. Process to end of file.OutputFor each case, output A + B in one line.Sample Input1 1Sample Output2AuthorHDOJ代码:#include<stdio.h>int main(){int a , b;while( scanf ( "%d %d" ,& a,& b)!= EOF)printf( "%d\n" , a+b);}1001 Sum ProblemProblem DescriptionHey, welcome to HDOJ(Hangzhou Dianzi University Online Judge).In this problem, your task is to calculate SUM(n) = 1 + 2 + 3 + ... + n.InputThe input will consist of a series of integers n, one integer per line.OutputFor each case, output SUM(n) in one line, followed by a blank line. You may assume the result will be in the range of 32-bit signed integer.Sample Input1100Sample Output15050AuthorDOOM III解答:#include<stdio.h>main (){int n , i , sum;sum =0;while (( scanf ( "%d" ,& n)!=- 1)) {sum for =0;( i =0; i <= n; i ++)sum +=i ;printf( "%d\n\n" , sum);}}1002 A + B Problem IIProblem DescriptionI have a very simple problem for you. Given two integers A and B, your job is to calculate the Sum of A + B. InputThe first line of the input contains an integer T(1<=T<=20) which means the number of test cases. Then T lines follow, each line consists of two positive integers, A and B. Notice that the integers are very large, that means you should not process them by using 32-bit integer. You may assume the length of each integer will not exceed 1000.OutputFor each test case, you should output two lines. The first line is "Case #:", # means the number of the test case. The second line is the an equation "A + B = Sum", Sum means the result of A + B. Note there are some spaces int the equation. Output a blank line between two test cases.Sample Input21 2Sample OutputCase 1:1+2=3Case 2:Author代码:#include <stdio.h>#include <string.h>int main (){char str1 [ 1001 ], str2 [ 1001 ];int t , i , len_str1 , len_str2 , len_max , num = 1 , k ; scanf ( "%d" , & t );getchar ();while ( t --){int a [ 1001 ] = { 0}, b [1001]={ 0}, c [1001]={ 0};scanf ( "%s" , str1 );len_str1 = strlen ( str1 );for ( i = 0 ; i <= len_str1 - 1;++ i )a [ i ] = str1 [ len_str1 - 1 - i ] - '0' ;scanf ( "%s" , str2 );len_str2 = strlen ( str2 );for ( i = 0 ; i <= len_str2 - 1;++ i )b [ i ] = str2 [ len_str2 - 1 - i ] - '0' ;if ( len_str1 > len_str2 )len_max = len_str1 ;elselen_max = len_str2 ;k = 0 ;for ( i = 0 ; i <= len_max - 1 ;++ i ){c [ i ] = ( a[ i ] + b [ i ] + k ) % 10 ;k = ( a[ i ] + b [ i ] + k ) / 10 ;}if ( k != 0 )c [ len_max ] = 1 ;printf ( "Case %d:\n" , num );num ++;printf ( "%s + %s = " , str1 , str2 );if ( c[ len_max ] == 1 )printf ( "1" );for ( i = len_max - 1 ; i >= 0 ;-- i ){printf ( "%d" , c [ i ]);}printf ( "\n" );if ( t >= 1 )printf ( "\n" );}return 0 ;}1005 Number Sequence Problem DescriptionA number sequence is defined as follows:f(1) = 1, f(2) = 1, f(n) = (A * f(n - 1) + B * f(n - 2)) mod 7.Given A, B, and n, you are to calculate the value of f(n).InputThe input consists of multiple test cases. Each test case contains 3 integers A, B and n on a single line (1 <= A, B <= 1000, 1 <= n <= 100,000,000). Three zeros signal the end of input and this test case is not to be processed.OutputFor each test case, print the value of f(n) on a single line.Sample Input1 1 312100 0 0Sample Output25AuthorCHEN, ShunbaoSourceRecommendJGShining代码:#include<stdio.h>int f [ 200 ];int main (){int a , b, n, i ;while ( scanf ( "%d%d%d" ,& a,& b,& n)&& a&&b&&n){if ( n>= 3){f [ 1]= 1; f [ 2]= 1;for ( i =3; i <= 200 ; i ++){f [ i ]=( a* f [ i - 1]+ b* f [ i - 2])% 7;if ( f [ i - 1]== 1&&f [ i ]== 1)break ;}i -= 2;n =n%i ;if ( n== 0)printf ( "%d\n" , f [ i ]);elseprintf ( "%d\n" , f [ n]);}elseprintf ( "1\n" );}return 0 ;}1008 ElevatorProblem DescriptionThe highest building in our city has only one elevator. A request list is made up with N positive numbers. The numbers denote at which floors the elevator will stop, in specified order. It costs 6 seconds to move the elevatorup one floor, and 4 seconds to move down one floor. The elevator will stay for 5 seconds at each stop.For a given request list, you are to compute the total time spent to fulfill the requests on the list. The elevator is on the 0th floor at the beginning and does not have to return to the ground floor when the requests are fulfilled.InputThere are multiple test cases. Each case contains a positive integer N, followed by N positive numbers. All the numbers in the input are less than 100. A test case with N = 0 denotes the end of input. This test case is not to be processed.OutputPrint the total time on a single line for each test case.Sample Input1 23231Sample Output1741AuthorZHENG, JianqiangSourceRecommendJGShining代码:#include<stdio.h>int a [ 110 ];int main(){int while { sum , i , n;( scanf ( "%d" ,& n)&& n!= 0)forscanf ( i =1; i <= n; i ++)( "%d" ,& a[ i ]);sum a for=0;[ 0]= 0;( i =1; i <= n; i ++){ifsum ( a[ i ]> a[ i - 1])+=6*( a[ i ]- a[ i - 1]);elsesum +=4*( a[ i - 1]- a[ i ]);sum +=5;printf ( "%d\n" , sum);}return 0 ;}1009 FatMouse' TradeProblem DescriptionFatMouse prepared M pounds of cat food, ready to trade with the cats guarding the warehouse containing his favorite food, JavaBean.The warehouse has N rooms. The i-th room contains J[i] pounds of JavaBeans and requires F[i] pounds of cat food. FatMouse does not have to trade for all the JavaBeans in the room, instead, he may get J[i]* a% pounds of JavaBeans if he pays F[i]* a% pounds of cat food. Here a is a real number. Now he is assigning this homework to you: tell him the maximum amount of JavaBeans he can obtain.InputThe input consists of multiple test cases. Each test case begins with a line containing two non-negative integersM and N. Then N lines follow, each contains two non-negative integers J[i] and F[i] respectively. The last test caseis followed by two -1's. All integers are not greater than 1000.OutputFor each test case, print in a single line a real number accurate up to 3 decimal places, which is the maximum amount of JavaBeans that FatMouse can obtain.Sample Input53724352203251824151510-1-1Sample OutputAuthorCHEN, YueSourceRecommendJGShining代码:#include<stdio.h>#include<string.h>#define MAX 1000int main(){int i,j,m,n,temp;int J[MAX],F[MAX];double P[MAX];double sum,temp1;scanf("%d%d",&m,&n);while(m!=-1&&n!=-1){sum=0;memset(J,0,MAX*sizeof(int));memset(F,0,MAX*sizeof(int));memset(P,0,MAX*sizeof(double));for(i=0;i<n;i++){ scanf("%d%d",&J[i],&F[i]); P[i]=J[i]*1.0/((double)F[i]); }for(i=0;i<n;i++){for(j=i+1;j<n;j++){if(P[i]<P[j]){temp1=P[i];P[i]=P[j];P[j]=temp1;temp=J[i]; J[i]=J[j]; J[j]=temp; temp=F[i];F[i]=F[j]; F[j]=temp;} }}for(i=0;i<n;i++) { if(m<F[i]){ else{sum+=m/((double)F[i])*J[i];sum+=J[i];break;m-=F[i];} }}printf("%.3lf\n",sum); scanf("%d%d",&m,&n); }return 0; }1021 Fibonacci AgainProblem DescriptionThere are another kind of Fibonacci numbers: F(0) = 7, F(1) = 11, F(n) = F(n-1) + F(n-2) (n>=2).InputInput consists of a sequence of lines, each containing an integer n. (n < 1,000,000).OutputPrint the word "yes" if 3 divide evenly into F(n). Print the word "no" if not.Sample Input0 1 2 3 4 5Sample Outputno no yes no no noAuthorLeojayRecommendJGShining#include<stdio.h> int main () { long while if printfn ;( scanf ( "%ld" ,& n) !=( n%8==2 || n %8==6) ( "yes\n" ); EOF ) elseprintf ( "no\n");return0 ;}1089 A+B for Input-Output Practice (I)Problem DescriptionYour task is to Calculate a + b.Too easy?! Of course! I specially designed the problem for acm beginners.You must have found that some problems have the same titles with this one, yes, all these problems were designed for the same aim.InputThe input will consist of a series of pairs of integers a and b, separated by a space, one pair of integers per line.OutputFor each pair of input integers a and b you should output the sum of a and b in one line, and with one line of output for each line in input.Sample Input151020Sample Output630AuthorlcyRecommendJGShining解答:#include<stdio.h>main (){int a , b;while( scanf ( "%d%d" ,& a,& b)!=EOF)printf( "%d\n" , a+b);}1090 A+B for Input-Output Practice (II)Problem DescriptionYour task is to Calculate a + b.InputInput contains an integer N in the first line, and then N lines follow. Each line consists of a pair of integers a and b, separated by a space, one pair of integers per line.OutputFor each pair of input integers a and b you should output the sum of a and b in one line, and with one line of output for each line in input.Sample Input2151020Sample Output630AuthorlcyRecommendJGShining解答:#include<stdio.h>#define M 1000void main (){int a , b, n, j [ M], i ;//printf("please input n:\n");scanf( "%d" ,& n);for( i =0; i <n; i ++){scanf( "%d%d" ,& a,& b);//printf("%d %d",a,b);j[ i ]= a+b;}i=0;while( i <n){printf( "%d" , j [ i ]);i++;printf( "\n" );}}1091 A+B for Input-Output Practice(III) Problem DescriptionYour task is to Calculate a + b.InputInput contains multiple test cases. Each test case contains a pair of integers a and b, one pair of integers per line.A test case containing 0 0 terminates the input and this test case is not to be processed.OutputFor each pair of input integers a and b you should output the sum of a and b in one line, and with one line ofoutput for each line in input.Sample Input15102000Sample Output630AuthorlcyRecommendJGShining解答:#include<stdio.h>main (){int a , b;scanf( "%d %d" ,& a,& b);while(!( a== 0&&b==0)){printf( "%d\n" , a+b);scanf( "%d %d" ,& a,& b);}}1092 A+B for Input-Output Practice(IV) Problem DescriptionYour task is to Calculate the sum of some integers.InputInput contains multiple test cases. Each test case contains a integer N, and then N integers follow in the same line. A test case starting with 0 terminates the input and this test case is not to be processed.OutputFor each group of input integers you should output their sum in one line, and with one line of output for each line in input.。
ACM题库完整版
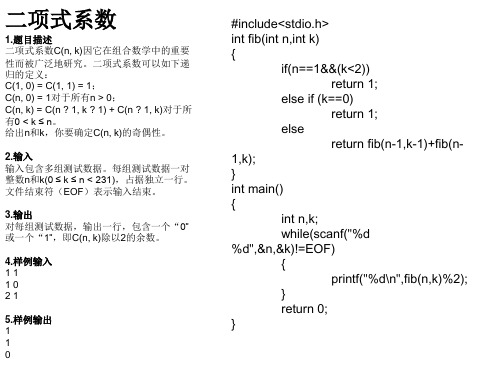
#include<stdio.h> int type(int); char week[7][10]={"saturday","sunday","monday","tuesday","wednesday","thursday","friday"}; int year[2]={365,366}; int month[2][12]={31,28,31,30,31,30,31,31,30,31,30,31,31,29,31,30,31,30,31,31,30,31,30,31}; int main(void) { int days,dayofweek; int i=0,j=0; while(scanf("%d",&days)&&days!=-1) { dayofweek=days%7; for(i=2000;days>=year[type(i)];i++) days-=year[type(i)]; for(j=0;days>=month[type(i)][j];j++) days-=month[type(i)][j]; printf("%d-%02d-%02d%s\n",i,j+1,days+1,week[dayofweek]); } return 0; } int type(int m) { if(m%4!=0||(m%100==0&&m%400!=0)) return 0; else return 1; }
构造新的模运算
1.题目描述 给定整数a,b,n,要求计算(a^b)mod n 2.输入 多组数据;=a<=40,0<=b<=3,1<=n<=500 3.输出 每组数据输出一行,为所求值 4.样例输入 #include<stdio.h> 235 224 #include<math.h> 5.样例输出 int main() 3 { 0
ACM几道练习题及其答案
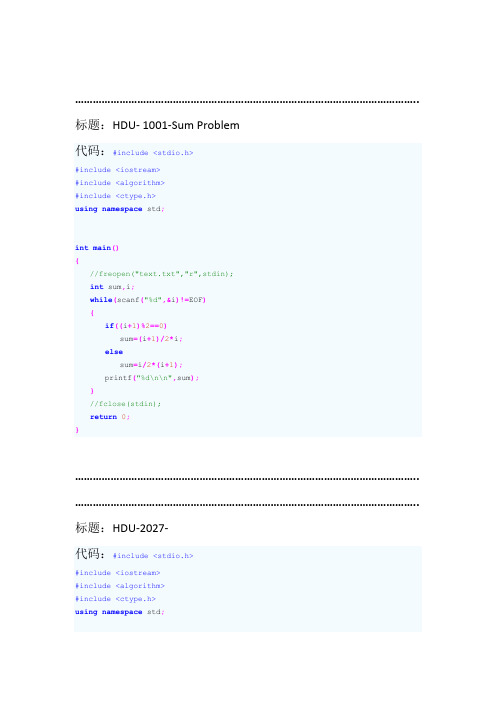
…………………………………………………………………………………………………….. 标题:HDU- 1001-Sum Problem代码:#include <stdio.h>#include <iostream>#include <algorithm>#include <ctype.h>using namespace std;int main(){//freopen("text.txt","r",stdin);int sum,i;while(scanf("%d",&i)!=EOF){if((i+1)%2==0)sum=(i+1)/2*i;elsesum=i/2*(i+1);printf("%d\n\n",sum);}//fclose(stdin);return 0;}…………………………………………………………………………………………………….. …………………………………………………………………………………………………….. 标题:HDU-2027-代码:#include <stdio.h>#include <iostream>#include <algorithm>#include <ctype.h>using namespace std;int main(){//freopen("text.txt","r",stdin);char c[101];int n,j,k,a,e,i,o,u;scanf("%d\n",&n);//注意这里for(j=1;j<=n;j++){a=e=i=o=u=0;gets(c);for(k=0;c[k]!='\0';k++){if(c[k]=='a')a++;if(c[k]=='e')e++;if(c[k]=='i')i++;if(c[k]=='o')o++;if(c[k]=='u')u++;}printf("a:%d\ne:%d\ni:%d\no:%d\nu:%d\n",a,e,i,o,u);if(j<n)printf("\n");}//fclose(stdin);return 0;}…………………………………………………………………………………………………….. …………………………………………………………………………………………………….. 标题:HDU-2025- 查找最大元素代码:#include <stdio.h>#include <iostream>#include <algorithm>#include <ctype.h>using namespace std;int main(){//freopen("text.txt","r",stdin);char a[105],max='A';int i,n;while(scanf("%s",a)!=EOF){n=strlen(a);max='A';for(i=0;i<n;i++){if(a[i]>max) max=a[i];}for(i=0;i<n;i++){printf("%c",a[i]);if(a[i]==max)printf("(max)");}printf("\n");}//fclose(stdin);return 0;}…………………………………………………………………………………………………….. …………………………………………………………………………………………………….. 标题:HDU-1037-Keep on Truckin'代码:#include <stdio.h>#include <iostream>#include <algorithm>#include <ctype.h>using namespace std;int main(){//freopen("text.txt","r",stdin);int a,b,c,min;while(cin >> a >> b >> c){min = a>b?b:a;min = min>c?c:min;if(min<=168)printf("CRASH %d\n",min);elseprintf("NO CRASH\n");}//fclose(stdin);return 0;}…………………………………………………………………………………………………….. …………………………………………………………………………………………………….. 标题:HDU-2052-Picture代码:#include <stdio.h>#include <iostream>#include <algorithm>#include <ctype.h>using namespace std;int main(){//freopen("text.txt","r",stdin);int n,m,i;while(scanf("%d%d",&n,&m)!=EOF){printf("+");for(i=0;i<n;++i){printf("-");}printf("+\n");for(i=0;i<m;++i){printf("|");for(int j=0;j<n;++j){printf(" ");}printf("|\n");}printf("+");for(i=0;i<n;++i){printf("-");}printf("+\n\n");}//fclose(stdin);return 0;}…………………………………………………………………………………………………….. …………………………………………………………………………………………………….. 标题:HDU-1034-Candy Sharing Game代码:#include <stdio.h>#include <iostream>#include <algorithm>#include <ctype.h>using namespace std;const int MAXN=1000;int a[MAXN];int main(){//freopen("text.txt","r",stdin);int n;int i;while(scanf("%d",&n),n){for(i=0;i<n;i++) scanf("%d",&a[i]);int res=0;while(1){for(i=1;i<n;i++)if(a[i-1]!=a[i]) break;if(i>=n) break;res++;int temp=a[n-1]/2;for(i=n-1;i>0;i--){a[i]/=2;a[i]+=a[i-1]/2;}a[0]/=2;a[0]+=temp;for(i=0;i<n;i++)if(a[i]&1) a[i]++;}printf("%d %d\n",res,a[0]);}//fclose(stdin);return 0;}……………………………………………………………………………………………………..。
acm大学生程序设计试题

acm大学生程序设计试题题目一:最大公约数(GCD)题目描述:给定两个正整数,求它们的最大公约数(GCD)。
输入两个正整数a和b(1 <= a, b <= 10^9),求它们的最大公约数。
输入格式:两个正整数,以空格分隔。
输出格式:输出一个整数,表示输入两个正整数的最大公约数。
示例:输入:14 21输出:7思路和分析:最大公约数(GCD)可以使用欧几里得算法来求解,即辗转相除法。
具体的步骤如下:1. 用较大的数除以较小的数,将得到的余数作为新的较大数。
2. 再用新的较大数除以较小数,将得到的余数作为新的较大数。
3. 如此重复,直到两个数可以整除,此时较小的数就是最大公约数。
代码实现:```cpp#include <iostream>using namespace std;int gcd(int a, int b) {if (b == 0)return a;return gcd(b, a % b);}int main() {int a, b;cin >> a >> b;int result = gcd(a, b);cout << result << endl;return 0;}```题目二:字符串反转题目描述:给定一个字符串,要求将其反转并输出。
输入一个字符串s(1 <= |s| <= 1000),输出该字符串的反转结果。
输入格式:一个字符串s,只包含大小写字母和数字。
输出格式:一个字符串,表示输入字符串的反转结果。
示例:输入:HelloWorld123输出:321dlroWolleH思路和分析:字符串反转可以使用双指针的方法来实现。
初始时,左指针指向字符串的开头,右指针指向字符串的末尾,然后交换左右指针所指向的字符,并向中间移动,直到左指针不小于右指针。
代码实现:```cpp#include <iostream>using namespace std;string reverseString(string s) {int left = 0, right = s.length() - 1; while (left < right) {swap(s[left], s[right]);left++;right--;}return s;}int main() {string s;cin >> s;string result = reverseString(s); cout << result << endl;return 0;}```题目三:字符串匹配题目描述:给定一个字符串s和一个模式串p,判断s中是否存在与p相匹配的子串。
ACM算法题以及答案

在做这些题目之前必须了解vector(数组),list(链表)、deque(双端队列)、queue(队列),priority_queue(优先队列)Stack(栈)、set(集合)、map(键值对),mutilset、mutilmap。
stack堆栈,没有迭代器,支持push()方法。后进先出,top()返回最顶端的元素,pop()剔除最顶元素
定义queue对象的示例代码如下:
queue<int> q1;
queue<double> q2;
queue的基本操作有:
入队,如例:q.push(x);将x接到队列的末端。
出队,如例:q.pop();弹出队列的第一个元素,注意,并不会返回被弹出元素的值。
访问队首元素,如例:q.front(),即最早被压入队列的元素。
#include <vector>
vector属于std命名域的,因此需要通过命名限定,如下完成你的代码:
using std::vector; vector<int> v;
或者连在一起,使用全名:
std::vector<int> v;
建议使用全局的命名域方式:
using namespace std;
list不支持随机访问。所以没有 at(pos)和operator[]。
list对象list1, list2 分别有元素list1(1,2,3),list2(4,5,6) 。list< int>::iterator it;
list成员
说明
constructor
构造函数
destructor
析构函数
acm大学生程序试题及答案

acm大学生程序试题及答案1. 题目:字符串反转描述:给定一个字符串,编写一个函数来将字符串中的字符按相反的顺序重新排列。
输入:一个字符串输出:反转后的字符串答案:```pythondef reverse_string(s):return s[::-1]```2. 题目:寻找最大数描述:给定一个整数数组,找出数组中的最大数。
输入:一个整数数组输出:数组中的最大数答案:```pythondef find_max(nums):return max(nums)```3. 题目:两数之和描述:给定一个整数数组和一个目标值,找出数组中和为目标值的两个数的索引(从1开始计数)。
输入:一个整数数组和一个目标值输出:两个数的索引,如果没有则返回空数组答案:```pythondef two_sum(nums, target):num_to_index = {}for i, num in enumerate(nums):complement = target - numif complement in num_to_index:return [num_to_index[complement] + 1, i + 1] num_to_index[num] = ireturn []```4. 题目:无重复字符的最长子串描述:给定一个字符串,请你找出其中不含有重复字符的最长子串的长度。
输入:一个字符串输出:最长子串的长度答案:```pythondef length_of_longest_substring(s):char_map = {}start = max_length = 0for end in range(len(s)):if s[end] in char_map:start = max(start, char_map[s[end]] + 1)char_map[s[end]] = endmax_length = max(max_length, end - start + 1)return max_length```5. 题目:整数转罗马数字描述:将一个整数转换为罗马数字。
ACM题库
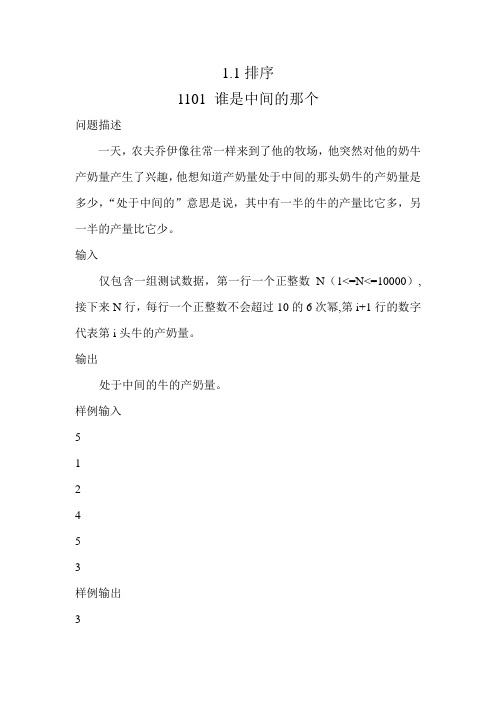
1.1排序1101 谁是中间的那个问题描述一天,农夫乔伊像往常一样来到了他的牧场,他突然对他的奶牛产奶量产生了兴趣,他想知道产奶量处于中间的那头奶牛的产奶量是多少,“处于中间的”意思是说,其中有一半的牛的产量比它多,另一半的产量比它少。
输入仅包含一组测试数据,第一行一个正整数N(1<=N<=10000),接下来N行,每行一个正整数不会超过10的6次幂,第i+1行的数字代表第i头牛的产奶量。
输出处于中间的牛的产奶量。
样例输入512453样例输出3#include<iostream>#include<algorithm>#include<cstdio>#include<cstdlib>u sing namespace std;typedef struct{int MakeMilk;int num;}COW;COW cow[10005];bool cmp(COW A,COW B)int main(){int n;while(scanf(“%d”,&n)!=EOF) {for(int i=1;i<=n;i++){scanf(“%d”,&cow[i].MakeMilk); cow[i].num=i;}sort(cow+1,cow+1+n,cmp);printf(“%d\n”,cow[(n+1)/2].MakeMilk);}return 0;}bool cmp(COW A,COW B){if(A.MakeMilk<B.MakeMilk)return true;if(A.MakeMilk==B.MakeMilk&&A.num>B.num)return true;return false;}1102一问一答问题描述现在输入一个序列,这个序列中有N个数字,输入时它们是无序的,而后它们会被写到数据库中,在数据库中,它们将被按照从小到大的顺序排列,当有人在外部向数据库输入一个数字N时,数据库会返回当中的第N小的数输入包括两部分,第一部分为输入部分,第一行为一个正整数N,代表数据库中共存有N个数,接下来N行,每行一个正整数,代表依次代表数据库中存储的数字,接下来一行是3个“#”,下面是询问部分,询问部分第一行为一个正整数K,接下来K行每一行一个正整数ki,代表要询问第ki小的数(1<=K,N<=5000),每个插入的数字不超过10000输出对于每个询问输出一行,代表第ki小的数样例输入571211237121###43325样例输出1217123#include<iostream>#include<algorithm>using namespace std;int num[120000];int main(){int n,m;char str[10];scanf(“%d”,&n);for(int i=1;i<=n;i++)scanf(“%d”,&num[i]); scanf(“%s”,str);sort(num+1,num+1+n);scanf(“%d”,&m);for(int j,i=1;i<=m;i++) {scanf(“%d”,&j);printf(“%d\n”,num[j]);return 0;}1103 478-3279问题描述商业上一般愿意采用易记的电话号码,把电话号码映射成一个容易记住的单词是其中一种方法,比如可以把滑铁卢大学的电话号码几位TUT-GLOP,有些时候,只有一部分数字能用来进行这种字母与数字能用来进行这种字母与数字间的映射,另外一种使电话号码容易被记住的方法,就是将电话号分割成有规律的几块,然后进行记忆,例如,订购比萨的电话为310-1010,可以记为一个3,三个10,即3-10-10-10 电话号码的标准形式为前三后四(例如,888-1200),下面是一些字母所映射的数字:(1)A,B和C映射到2;(2)D,E和F映射到3;(3)G,H和I映射到4;(4)J,K和L映射到5;(5)M,N和O映射到6;(6)P,R和S映射到7;(7)T,U和V映射到8;(8)W,X和Y映射到9;这里没有数字对应Q或者是Z。
ACM程序设计试题及参考答案

ACM程序设计试题及参考答案猪的安家Andy和Mary养了很多猪。
他们想要给猪安家。
但是Andy没有足够的猪圈,很多猪只能够在一个猪圈安家。
举个例子,假如有16头猪,Andy建了3个猪圈,为了保证公平,剩下1头猪就没有地方安家了。
Mary生气了,骂Andy没有脑子,并让他重新建立猪圈。
这回Andy建造了5个猪圈,但是仍然有1头猪没有地方去,然后Andy又建造了7个猪圈,但是还有2头没有地方去。
Andy都快疯了。
你对这个事情感兴趣起来,你想通过Andy建造猪圈的过程,知道Andy家至少养了多少头猪。
输入输入包含多组测试数据。
每组数据第一行包含一个整数n (n <= 10) – Andy 建立猪圈的次数,解下来n行,每行两个整数ai, bi( bi <= ai <= 1000), 表示Andy建立了ai个猪圈,有bi头猪没有去处。
你可以假定(ai, aj) = 1.输出输出包含一个正整数,即为Andy家至少养猪的数目。
样例输入33 15 17 2样例输出16答案:// 猪的安家.cpp : Defines the entry point for the console application.//#include "stdafx.h"#include "iostream.h"void main(){int n;int s[10][2];bool r[10];char ch;cout<<"请输入次数:"<<endl;cin>>n;for (int i=0;i<n;i++){cout<<"请输入第"<<i+1<<"次的猪圈个数和剩下的猪:,用--分开,"<<endl;cin>>s[i][0]>>ch>>ch>>s[i][1];}for (i=0;i<10;i++)r[i]=true;for (int sum=1;;sum++){for (i=0;i<n;i++)r[i]=(sum%s[i][0]==s[i][1]);for (i=0;i<n;i++){if (r[i]==0)break;}if (i==n)break;}cout<<"猪至少有"<<sum<<"只。
算法期末考试试题及答案

算法期末考试试题及答案一、选择题(每题2分,共20分)1. 在排序算法中,时间复杂度为O(n^2)的算法是:A. 快速排序B. 归并排序C. 冒泡排序D. 堆排序2. 哈希表的冲突解决方法不包括:A. 开放寻址法B. 链接法C. 链表法D. 排序法3. 以下哪个不是二叉树的性质:A. 每个节点最多有两个子节点B. 没有兄弟节点C. 左子树的所有节点的值小于根节点的值D. 右子树的所有节点的值大于根节点的值4. 在图的遍历算法中,深度优先搜索(DFS)使用的是:A. 栈B. 队列C. 链表D. 哈希表5. 动态规划与分治法的区别在于:A. 动态规划使用贪心选择,分治法不使用B. 分治法使用贪心选择,动态规划不使用C. 动态规划使用递归,分治法不使用D. 动态规划使用迭代,分治法使用递归...二、简答题(每题10分,共30分)1. 简述快速排序算法的基本思想。
2. 解释什么是贪心算法,并给出一个实际应用的例子。
3. 描述图的广度优先搜索(BFS)算法的步骤。
三、计算题(每题15分,共30分)1. 给定一个数组A=[3, 1, 4, 1, 5, 9, 2, 6, 5, 3],请使用归并排序算法对其进行排序,并给出排序过程中的每一步。
2. 假设有一个无向图,顶点集为V={A, B, C, D},边集为E={(A, B), (B, C), (C, D), (D, A)},请使用Kruskal算法找到该图的最小生成树。
四、编程题(每题20分,共20分)1. 编写一个函数,实现单源最短路径的Dijkstra算法。
函数输入为图的邻接矩阵和起始顶点,输出为从起始顶点到所有其他顶点的最短路径长度。
答案一、选择题1. C2. D3. B4. A5. D二、简答题1. 快速排序算法的基本思想是通过一个划分操作,将数组分为两部分,一部分比另一部分的所有元素都要小,然后再递归地对这两部分进行快速排序,最后将两部分合并。
2. 贪心算法是一种在每一步选择中都采取在当前状态下最好或最优的选择,从而希望导致结果是全局最好或最优的算法。
acm试题及答案

acm试题及答案ACM试题及答案试题 1: 给定一个整数数组,请找出数组中第二大的数。
答案:1. 对数组进行排序。
2. 数组排序后,倒数第二个元素即为第二大的数。
试题 2: 编写一个函数,计算给定字符串中字符出现的次数。
答案:```pythondef count_characters(s):count_dict = {}for char in s:if char in count_dict:count_dict[char] += 1else:count_dict[char] = 1return count_dict```试题 3: 判断一个数是否为素数。
答案:1. 如果数小于2,则不是素数。
2. 从2开始到该数的平方根,检查是否有因数。
3. 如果没有因数,则该数是素数。
试题 4: 实现一个算法,将一个整数数组按照奇数在前,偶数在后的顺序重新排列。
答案:```pythondef rearrange_array(arr):odd = []even = []for num in arr:if num % 2 == 0:even.append(num)else:odd.append(num)return odd + even```试题 5: 给定一个链表,删除链表的倒数第n个节点。
答案:1. 遍历链表,找到链表的长度。
2. 再次遍历链表,找到倒数第n个节点的前一个节点。
3. 将前一个节点的next指针指向当前节点的下一个节点。
4. 如果当前节点是头节点,则更新头节点。
试题 6: 编写一个函数,实现字符串反转。
答案:```pythondef reverse_string(s):return s[::-1]```试题 7: 给定一个整数数组,找出数组中没有出现的最小正整数。
答案:1. 遍历数组,使用哈希表记录出现的数字。
2. 从1开始,检查每个数字是否在哈希表中。
3. 第一个不在哈希表中的数字即为答案。
试题 8: 实现一个算法,计算斐波那契数列的第n项。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
一、综合处理题1、两倍- /problem?id=2807Description给定2到15个不同的正整数,你的任务是计算这些数里面有多少个数对满足:数对中一个数是另一个数的两倍。
比如给定1 4 3 2 9 7 18 22,得到的答案是3,因为2是1的两倍,4是2个两倍,18是9的两倍。
Input输入包括多组测试数据。
每组数据包括一行,给出2到15个两两不同且小于100的正整数。
每一行最后一个数是0,表示这一行的结束后,这个数不属于那2到15个给定的正整数。
输入的最后一行只包括一个整数-1,这行表示输入数据的结束,不用进行处理。
Output对每组输入数据,输出一行,给出有多少个数对满足其中一个数是另一个数的两倍。
Sample Input1 4 32 9 7 18 22 02 4 8 10 07 5 11 13 1 3 0-1Sample Output322、谁拿了最多奖学金 - /problem?id=2715Description某校的惯例是在每学期的期末考试之后发放奖学金。
发放的奖学金共有五种,获取的条件各自不同:1) 院士奖学金,每人8000元,期末平均成绩高于80分(>80),并且在本学期内发表1篇或1篇以上论文的学生均可获得;2) 五四奖学金,每人4000元,期末平均成绩高于85分(>85),并且班级评议成绩高于80分(>80)的学生均可获得;3) 成绩优秀奖,每人2000元,期末平均成绩高于90分(>90)的学生均可获得;4) 西部奖学金,每人1000元,期末平均成绩高于85分(>85)的西部省份学生均可获得;5) 班级贡献奖,每人850元,班级评议成绩高于80分(>80)的学生干部均可获得;只要符合条件就可以得奖,每项奖学金的获奖人数没有限制,每名学生也可以同时获得多项奖学金。
例如姚林的期末平均成绩是87分,班级评议成绩82分,同时他还是一位学生干部,那么他可以同时获得五四奖学金和班级贡献奖,奖金总数是4850元。
现在给出若干学生的相关数据,请计算哪些同学获得的奖金总数最高(假设总有同学能满足获得奖学金的条件)。
Input输入的第一行是一个整数N(1 <= N <= 100),表示学生的总数。
接下来的N行每行是一位学生的数据,从左向右依次是姓名,期末平均成绩,班级评议成绩,是否是学生干部,是否是西部省份学生,以及发表的论文数。
姓名是由大小写英文字母组成的长度不超过20的字符串(不含空格);期末平均成绩和班级评议成绩都是0到100之间的整数(包括0和100);是否是学生干部和是否是西部省份学生分别用一个字符表示,Y表示是,N表示不是;发表的论文数是0到10的整数(包括0和10)。
每两个相邻数据项之间用一个空格分隔。
Output输出包括三行,第一行是获得最多奖金的学生的姓名,第二行是这名学生获得的奖金总数。
如果有两位或两位以上的学生获得的奖金最多,输出他们之中在输入文件中出现最早的学生的姓名。
第三行是这N个学生获得的奖学金的总数。
Sample Input4YaoLin 87 82 Y N 0ChenRuiyi 88 78 N Y 1LiXin 92 88 N N 0ZhangQin 83 87 Y N 1Sample OutputChenRuiyi900028700二、字符串处理1、古代密码- /problem?id=2820Description古罗马帝王有一个包括各种部门的强大政府组织。
其中有一个部门就是保密服务部门。
为了保险起见,在省与省之间传递的重要文件中的大写字母是加密的。
当时最流行的加密方法是替换和重新排列。
替换方法是将所有出现的字符替换成其它的字符。
有些字符会碰巧替换成它自己。
例如:替换规则可以是将'A' 到'Y'替换成它的下一个字符,将'Z'替换成 'A',如果原词是"VICTORIOUS" 则它变成"WJDUPSJPVT"。
排列方法改变原来单词中字母的顺序。
例如:将顺序<2, 1, 5, 4, 3, 7, 6, 10, 9, 8> 应用到"VICTORIOUS" 上,则得到"IVOTCIRSUO"。
人们很快意识到单独应用替换方法或排列方法,加密是很不保险的。
但是如果结合这两种方法,在当时就可以得到非常可靠的加密方法。
所以,很多重要信息先使用替换方法加密,再将加密的结果用排列的方法加密。
用两中方法结合就可以将"VICTORIOUS" 加密成"JWPUDJSTVP"。
考古学家最近在一个石台上发现了一些信息。
初看起来它们毫无意义,所以有人设想它们可能是用替换和排列的方法被加密了。
人们试着解读了石台上的密码,现在他们想检查解读的是否正确。
他们需要一个计算机程序来验证她,你的任务就是写这个验证程序。
Input输入有两行。
第一行是石台上的文字。
文字中没有空格,并且只有大写英文字母。
第二行是被解读出来的加密前的文字。
第二行也是由大写英文字母构成的。
两行字符数目的长度都不超过计划100。
Output如果第二行经过某种加密方法后可以产生第一行的信息,输出"YES",否则输出"NO"。
Sample InputJWPUDJSTVPVICTORIOUSSample OutputYES2、词典- /problem?id=2804Description你旅游到了一个国外的城市。
那里的人们说的外国语言你不能理解。
不过幸运的是,你有一本词典可以帮助你。
Input首先输入一个词典,词典中包含不超过100000个词条,每个词条占据一行。
每一个词条包括一个英文单词和一个外语单词,两个单词之间用一个空格隔开。
而且在词典中不会有某个外语单词出现超过两次。
词典之后是一个空行,然后给出一个由外语单词组成的文档,文档不超过100000行,而且每行只包括一个外语单词。
输入中出现单词只包括小写字母,而且长度不会超过10。
Output在输出中,你需要把输入文档翻译成英文,每行输出一个英文单词。
如果某个外语单词不在词典中,就把这个单词翻译成“eh”。
Sample Inputcat atcaypig igpayfroot ootfrayloops oopslayatcayittenkayoopslaySample Outputcatehloops3、最短前缀- /problem?id=2797Description一个字符串的前缀是从该字符串的第一个字符起始的一个子串。
例如"carbon"的字串是: "c", "ca", "car", "carb", "carbo", 和"carbon"。
注意到这里我们不认为空串是字串, 但是每个非空串是它自身的字串. 我们现在希望能用前缀来缩略的表示单词。
例如, "carbohydrate" 通常用"carb"来缩略表示. 现在给你一组单词, 要求你找到唯一标识每个单词的最短前缀在下面的例子中,"carbohydrate" 能被缩略成"carboh", 但是不能被缩略成"carbo" (或其余更短的前缀) 因为已经有一个单词用"carbo"开始一个精确匹配会覆盖一个前缀匹配,例如,前缀"car"精确匹配单词"car". 因此"car" 是"car"的缩略语是没有二义性的, “car”不会被当成"carriage"或者任何在列表中以"car"开始的单词.Input输入包括至少2行,至多1000行. 每行包括一个以小写字母组成的单词,单词长度至少是1,至多是20.Output输出的行数与输入的行数相同。
每行输出由相应行输入的单词开始,后面跟着一个空格接下来是相应单词的没有二义性的最短前缀标识符。
Sample InputcarbohydratecartcarburetorcaramelcariboucarboniccarboncarriagecartoncarcarbonateSample Outputcarbohydrate carbohcart cartcarburetor carbucaramel caracaribou caricarbonic carbonicartilage carticarbon carboncarriage carrcarton cartocar carcarbonate carbona三、模拟1、数根– /problem?id=2764Description数根可以通过把一个数的各个位上的数字加起来得到。
如果得到的数是一位数,那么这个数就是数根。
如果结果是两位数或者包括更多位的数字,那么再把这些数字加起来。
如此进行下去,直到得到是一位数为止。
比如,对于24来说,把2和4相加得到6,由于6是一位数,因此6是24的数根。
再比如39,把3和9加起来得到12,由于12不是一位数,因此还得把1和2加起来,最后得到3,这是一个一位数,因此3是39的数根。
Input输入包括一些正整数(小于101000),每个一行。
输入的最后一行是0,表示输入的结束,这一行不用处理。
Output对每个正整数,输出它的数根。
每个结果占据一行。
Sample Input2439Sample Output632、循环数– /problem?id=2952Descriptionn 位的一个整数是循环数(cyclic)的条件是:当用一个1 到n 之间的整数去乘它时, 会得到一个将原来的数首尾相接循环移动若干数字再在某处断开而得到的数字。
也就是说,如果把原来的数字和新的数字都首尾相接,他们得到的环是相同的。
只是两个数的起始数字不一定相同。
例如,数字142857 是循环数,因为:142857 *1 = 142857142857 *2 = 285714142857 *3 = 428571142857 *4 = 571428142857 *5 = 714285142857 *6 = 857142Input写一个程序确定给定的数是否是循环数。
输入包括多个长度为2 位到60 位的整数。
(注意,先导的0也是合理的输入不应该被忽略,例如"01"是 2 位数,"1" 是 1 位数。