加密c破解简单办法
C#字符串加密与解密

string EncryptKey = "yeah";//定义密钥/// <summary>/// 字符串加密/// </summary>/// <param name="str">要加密的字符串</param>/// <returns></returns>private string Encrypt(string str){DESCryptoServiceProvider desc = new DESCryptoServiceProvider();//实例化加密解密对象byte[] key = Encoding.Unicode.GetBytes(EncryptKey);//定义字节数组、用来存储密钥byte[] data = Encoding.Unicode.GetBytes(str);//定义字节数组、用来存储要解密的字符串MemoryStream mstream = new MemoryStream();//实例化内存流对象//使用内存流实例化加密流对象CryptoStream cstream = new CryptoStream(mstream, desc.CreateEncryptor(key, key), CryptoStreamMode.Write);cstream.Write(data, 0, data.Length);//想加密流中写入数据cstream.FlushFinalBlock();//释放加密流return Convert.ToBase64String(mstream.ToArray());//返回加密后的字符串}/// <summary>/// 字符串解密/// </summary>/// <param name="str">要解密的字符串</param>/// <returns></returns>private string Decrypt(string str){DESCryptoServiceProvider desc = new DESCryptoServiceProvider();//实例化加密、解密对象byte[] key = Encoding.Unicode.GetBytes(EncryptKey);//定义字节数组用来存储密钥byte[] data = Convert.ToBase64String(str);//定义字节数组用来存储密钥MemoryStream mstream = new MemoryStream();//实例化内存流对象//使用内存流实例化解密对象CryptoStream cstream = new CryptoStream(mstream, desc.CreateDecryptor(key, key), CryptoStreamMode.Write);cstream.Write(data, 0, data.Length);//向解密流中写入数据cstream.FlushFinalBlock();//释放解密流return Encoding.Unicode.GetString(mstream.ToArray());}。
c++位运算加解密方法

c 位运算加解密方法C语言中的位运算可以用于实现简单的加密和解密方法。
其中,一种常见的方法是使用位运算中的异或(XOR)操作来进行加密和解密。
异或操作是一个二进制操作,它比较两个相邻的二进制位。
如果两个位相同,则结果为0;如果两个位不同,则结果为1。
这个操作可以用于将数据加密和解密,因为对于任何数字,重复进行异或操作会得到同样的结果。
下面是一个使用异或操作进行加密和解密的示例代码:#include <stdio.h>int main() {int message = 0x0A; // 原始数据int key = 0x3A; // 密钥int encrypted_message = message ^ key; // 加密int decrypted_message = encrypted_message ^ key; // 解密printf("Original message: %X\n", message);printf("Encrypted message: %X\n", encrypted_message);printf("Decrypted message: %X\n", decrypted_message);return 0;}在这个示例中,我们将原始数据和密钥存储在变量message和key中。
然后,我们使用异或操作将message和key进行异或操作,得到加密后的数据encrypted_message。
接着,我们将encrypted_message和key再次进行异或操作,得到解密后的数据decrypted_message。
最后,我们使用printf函数将原始数据、加密后的数据和解密后的数据输出到控制台。
需要注意的是,这种加密方法非常简单,容易被破解。
因此,在实际应用中,应该使用更加安全的加密算法来进行数据加密和解密。
C#常用的加密解密方法

byte[] data = puteHash(inputBytes);
StringBuilder sBuilder = new StringBuilder();
//将data中的每个字符都转换为16进制的
using System.Text;
namespace SHA1Test
{
class Program
{
static void Main(string[] args)
{
string source = "Happy Birthday!";
string hash = GetSha1Hash(source);
①不可以从消息摘要中复原信息
②两个不同的消息不会产生同样的消息摘要,(但会有1x10 ^ 48分之一的机率出现相同的消息摘要,一用来验证数据。
测试代码:
演示通过sha1获取一个字符串的hash并做验证:
using System;
using System.Security.Cryptography;
StringComparer comparer = StringComparer.OrdinalIgnoreCase;
if (0 == pare(hashOfInput, hash))
{
return true;
}
else
{
return false;
}
}
}
}
SHA1
安全哈希算法(Secure Hash Algorithm)主要适用于数字签名标准(Digital Signature Standard DSS)里面定义的数字签名算法(Digital Signature Algorithm DSA)。对于长度小于2^64位的消息,SHA1会产生一个160位的消息摘要。当接收到消息的时候,这个消息摘要可以用来验证数据的完整性。在传输的过程中,数据很可能会发生变化,这时候就会产生不同的消息摘要。SHA1有如下特性:
C语言中的数据加密与解密技术

C语言中的数据加密与解密技术数据加密与解密技术在现代信息安全领域中起着至关重要的作用,能够保护数据的隐私性和完整性,防止数据被非法窃取和篡改。
在C语言中,我们可以利用各种加密算法实现数据加密和解密操作,确保数据在传输和存储过程中的安全性。
在C语言中,常用的数据加密算法包括对称加密算法和非对称加密算法。
对称加密算法使用相同的密钥进行加密和解密操作,简单高效,适用于小规模数据加密。
常见的对称加密算法有DES、AES等。
非对称加密算法需要一对公钥和私钥,公钥用于加密数据,私钥用于解密数据,安全性更高,适用于加密大规模数据。
常见的非对称加密算法有RSA、ECC等。
在C语言中,我们可以使用openssl库或者自行实现上述加密算法来实现数据加密和解密。
下面以AES对称加密算法为例进行介绍。
首先,我们需要在C语言中引入openssl库,通过以下方式进行安装和引入:```sudo apt-get install libssl-dev``````c#include <openssl/aes.h>#include <openssl/rand.h>```接下来,我们可以定义一个函数来实现数据的加密和解密操作:```cvoid encrypt_decrypt_data(unsigned char *data, int data_len, unsigned char *key, unsigned char *iv, int encrypt) {AES_KEY aes_key;AES_set_encrypt_key(key, 128, &aes_key);if (encrypt) {AES_cbc_encrypt(data, data, data_len, &aes_key, iv, AES_ENCRYPT);} else {AES_cbc_encrypt(data, data, data_len, &aes_key, iv, AES_DECRYPT);}}```在上面的函数中,我们使用AES算法对数据进行加密或解密操作,其中data 为待加密或解密的数据,data_len为数据长度,key为密钥,iv为初始化向量,encrypt为加密标志。
C#加密解密方式

3、方法三 (可逆加密)
const string KEY_64 = "VavicApp";//注意了,是 8 个字符,64 位
const string IV_64 = "VavicApp"; public string Encode(string data)
for ( int i = 0; i < str.Length; i++)
对全部高中资料试卷电气设备,在安装过程中以及安装结束后进行高中资料试卷调整试验;通电检查所有设备高中资料电试力卷保相护互装作置用调与试相技互术关,通系电1,力过根保管据护线生高0不产中仅工资2艺料22高试2可中卷以资配解料置决试技吊卷术顶要是层求指配,机置对组不电在规气进范设行高备继中进电资行保料空护试载高卷与中问带资题负料2荷试2,下卷而高总且中体可资配保料置障试时2卷,32调需3各控要类试在管验最路;大习对限题设度到备内位进来。行确在调保管整机路使组敷其高设在中过正资程常料1工试中况卷,下安要与全加过,强度并看工且25作尽52下可22都能护可地1关以缩于正小管常故路工障高作高中;中资对资料于料试继试卷电卷连保破接护坏管进范口行围处整,理核或高对者中定对资值某料,些试审异卷核常弯与高扁校中度对资固图料定纸试盒,卷位编工置写况.复进保杂行护设自层备动防与处腐装理跨置,接高尤地中其线资要弯料避曲试免半卷错径调误标试高方中等案资,,料要编试求5写、卷技重电保术要气护交设设装底备备置。4高调、动管中试电作线资高气,敷料中课并设3试资件且、技卷料中拒管术试试调绝路中验卷试动敷包方技作设含案术,技线以来术槽及避、系免管统不架启必等动要多方高项案中方;资式对料,整试为套卷解启突决动然高过停中程机语中。文高因电中此气资,课料电件试力中卷高管电中壁气资薄设料、备试接进卷口行保不调护严试装等工置问作调题并试,且技合进术理行,利过要用关求管运电线行力敷高保设中护技资装术料置。试做线卷到缆技准敷术确设指灵原导活则。。:对对在于于分调差线试动盒过保处程护,中装当高置不中高同资中电料资压试料回卷试路技卷交术调叉问试时题技,,术应作是采为指用调发金试电属人机隔员一板,变进需压行要器隔在组开事在处前发理掌生;握内同图部一纸故线资障槽料时内、,设需强备要电制进回造行路厂外须家部同出电时具源切高高断中中习资资题料料电试试源卷卷,试切线验除缆报从敷告而设与采完相用毕关高,技中要术资进资料行料试检,卷查并主和且要检了保测解护处现装理场置。设。备高中资料试卷布置情况与有关高中资料试卷电气系统接线等情况,然后根据规范与规程规定,制定设备调试高中资料试卷方案。
C语言实现加密解密功能

C语⾔实现加密解密功能加密主要是通过⼀种算法对原内容进⾏处理,使原来内容不直观可见。
解密过程通常要知道加密的算法,然后对加密后的内容进⾏逆处理,从⽽实现解密功能。
当然解密也有⼀些暴⼒破解的⽅法。
接下来以 c 语⾔为例讲解⼀种简单的加密解密以及暴⼒破解字符串的⽅法,带您⾛进加密解密技术的⼤门。
先讲⼀下凯撒加密,凯撒密码相传是古罗马凯撒⼤帝⽤来保护重要军情的加密系统。
它是⼀种置换密码,通过将字母顺序推后起到加密作⽤。
如字母顺序推后 3 位,字母 A 将被推作字母 D,字母 B 将被推作字母 E。
本实例类似于凯撒加密。
加密算法:⼤(⼩)写字母加密后还为⼤(⼩)写字母。
⼤(⼩)写字母向后推 n 位,n 由⽤户输⼊,如果超出⼤(⼩)写字母的 ASCII 范围,则返回⾄第⼀个⼤(⼩)写字母继续循环。
解密算法(与加密算法正好相反):⼤(⼩)写字母解密后还为⼤(⼩)写字母。
⼤(⼩)写字母向前推 n 位,n 由⽤户输⼊,如果超出⼤(⼩)写字母的 ASCII 范围,则返回⾄最后⼀个⼤(⼩)写字母继续循环。
代码如下:/*字符串加密解密程序凯撒加密*/#include <stdio.h>#include <stdlib.h>#include <string.h>//函数encode()将字母顺序推后n位,实现⽂件加密功能void encode(char str[],int n){char c;int i;for(i=0;i<strlen(str);++i){ //遍历字符串c=str[i];if(c>='a' && c<='z'){ //c是⼩写字母if(c+n%26<='z'){ //若加密后不超出⼩写字母范围str[i]=(char)(c+n%26); //加密函数}else{ //加密后超出⼩写字母范围,从头开始循环⼩写字母str[i]=(char)(c+n%26-26);}}else if(c>='A' && c<='Z'){ //c为⼤写字母if(c + n%26 <= 'Z'){ //加密后不超出⼤写字母范围str[i]=(char)(c+n%26);}else{ //加密后超出⼤写字母范围,从头开始循环⼤写字母str[i]=(char)(c+n%26-26);}}else{ //不是字母,不加密str[i]=c;}}printf("\nAfter encode: \n");puts(str); //输出加密后的字符串}//decode()实现解密功能,将字母顺序前移n位void decode(char str[],int n){char c;int i;//遍历字符串for(i=0;i<strlen(str);++i){c=str[i];//c为⼩写字母if(c>='a' && c<='z'){//解密后还为⼩写字母,直接解密if(c-n%26>='a'){str[i]=(char)(c-n%26);}else{//解密后不为⼩写字母了,通过循环⼩写字母处理为⼩写字母str[i]=(char)(c-n%26+26);}}else if(c >= 'A' && c<='Z'){ //c为⼤写字母if(c-n%26>='A'){ //解密后还为⼤写字母str[i]=(char)(c-n%26);}else{ //解密后不为⼤写字母了,循环⼤写字母,处理为⼤写字母str[i]=(char)(c-n%26+26);}}else{ //⾮字母不处理str[i]=c;}}printf("\nAfter decode: \n");puts(str); //输出解密后的字符串}//该函数代码有冗余,读者可改进int main(){char str[50];int k=0,n=0,i=1;printf("\nPlease input strings: ");scanf("%s",str); //输⼊加密解密字符串//打印菜单printf("-----------------\n");printf("1: Encryption\n");printf("2: Decryption\n");printf("3: Violent Crack\n"); //暴⼒破解printf("-----------------\n");printf("\nPlease choose: ");scanf("%d",&k);if(k==1){ //加密printf("\nPlease input number: ");scanf("%d",&n);encode(str,n);}else if(k==2){ //解密printf("\nPlease input number: ");scanf("%d",&n);decode(str,n);}else{for(i=1;i<=25;++i){ //尝试所有可能的n值进⾏暴⼒破解printf("%d ",i);decode(str,i);}}return 0;}测试运⾏如下:成功实现加密解密功能,读者可以稍加改造完成对⽂件的加密解密以上就是本⽂的全部内容,希望对⼤家的学习有所帮助,也希望⼤家多多⽀持。
加密文件文件破解技巧

方法如下:
在c盘新建一个文件夹, 取名为uu 即: c:\uu 这个
文件夹,然后工具——》文件夹选项——》"查看”里的"高级设置"下面选择“显示所有文件和文件夹”
和去掉"隐藏受保护的操作系统文件"前的对号, 会
出来一个对话框, 选择"是";
从你忘记密码的文件夹里copy 这个加密程序 (一般
叫加密什么的吧) , 到 c:\uu, 运行程序, 设置一个密码为 1
打开你的解压缩程序(winrar) , 然后在上面的地址
栏输入: c:\uu, 找到一个Thumbs开头的文件夹, 双击打开--> 将里面的desktop.ini 删除掉!
重复第步, 只是文件夹地址改为你忘记密码的文件
地址就行了, 这里先将这个文件说成d:\cc这个文件吧
打开c:\uu里的Thumbs开头的文件夹,将里面的"117789687"这个文件,右键复制一下, 然后打开
d:\cc 文件夹, 找到里的Thumbs开头的文件夹并打开, 右键-->粘贴--> 覆盖 117789687 这个文件,
回到d:\cc 文件夹下面, 运行加密程序, 输入密码1 你所有的文件是不是又重见光明了
注意:
这里的c:\uu是新建的一个文件夹d:\cc 是你忘记了密码的文件。
C加密解密算法

1、方法一 (不可逆)public string EncryptPassword(string PasswordString,string PasswordFormat ){string encryptPassword = null;if (PasswordFormat="SHA1"){encryptPassword=FormsAuthortication.HashPasswordForStoringInConfigFile(PasswordS tring,"SHA1");}elseif (PasswordFormat="MD5"){ encryptPassword=FormsAuthortication.HashPasswordForStoringInConfigFile(Passwor dString,"MD5");}return encryptPassword ;}2、方法二 (可逆)public interface IBindesh{string encode(string str);string decode(string str);}public class EncryptionDecryption : IBindesh{public string encode(string str){string htext = "";for ( int i = 0; i < str.Length; i++){htext = htext + (char) (str[i] + 10 - 1 * 2);}return htext;public string decode(string str){string dtext = "";for ( int i=0; i < str.Length; i++){dtext = dtext + (char) (str[i] - 10 + 1*2);}return dtext;}3、方法三 (可逆)const string KEY_64 = "VavicApp";//注意了,是8个字符,64位const string IV_64 = "VavicApp";public string Encode(string data){byte[] byKey = System.Text.ASCIIEncoding.ASCII.GetBytes(KEY_64);byte[] byIV = System.Text.ASCIIEncoding.ASCII.GetBytes(IV_64);DESCryptoServiceProvider cryptoProvider = new DESCryptoServiceProvider();int i = cryptoProvider.KeySize;MemoryStream ms = new MemoryStream();CryptoStream cst = new CryptoStream(ms, cryptoProvider.CreateEncryptor(byKey,byIV), CryptoStreamMode.Write);StreamWriter sw = new StreamWriter(cst);sw.Write(data);sw.Flush();cst.FlushFinalBlock();sw.Flush();return Convert.ToBase64String(ms.GetBuffer(), 0, (int)ms.Length);public string Decode(string data){byte[] byKey = System.Text.ASCIIEncoding.ASCII.GetBytes(KEY_64);byte[] byIV = System.Text.ASCIIEncoding.ASCII.GetBytes(IV_64);byte[] byEnc;try{byEnc = Convert.FromBase64String(data);}catch{return null;}DESCryptoServiceProvider cryptoProvider = new DESCryptoServiceProvider();MemoryStream ms = new MemoryStream(byEnc);CryptoStream cst = new CryptoStream(ms, cryptoProvider.CreateDecryptor(byKey,byIV), CryptoStreamMode.Read);StreamReader sr = new StreamReader(cst);return sr.ReadToEnd();}4、MD5不可逆加密(32位加密)public string GetMD5(string s, string _input_charset){/**//**//**//// <summary>/// 与ASP兼容的MD5加密算法/// </summary>MD5 md5 = new MD5CryptoServiceProvider();byte[] t = puteHash(Encoding.GetEncoding(_input_charset).GetBytes(s));StringBuilder sb = new StringBuilder(32);for (int i = 0; i < t.Length; i++){sb.Append(t[i].ToString("x").PadLeft(2, '0'));}return sb.ToString();}(16位加密)public static string GetMd5Str(string ConvertString){MD5CryptoServiceProvider md5 = new MD5CryptoServiceProvider();string t2 =BitConverter.ToString(puteHash(UTF8Encoding.Default.GetBytes(ConvertStrin g)), 4, 8);t2 = t2.Replace("-", "");return t2;}5、加解文本文件//加密文件private static void EncryptData(String inName, String outName, byte[] desKey, byte[]desIV){//Create the file streams to handle the input and output files.FileStream fin = new FileStream(inName, FileMode.Open, FileAccess.Read); FileStream fout = new FileStream(outName, FileMode.OpenOrCreate, FileAccess.Write);fout.SetLength(0);//Create variables to help with read and write.byte[] bin = new byte[100]; //This is intermediate storage for the encryption.long rdlen = 0; //This is the total number of bytes written. long totlen = fin.Length; //This is the total length of the input file. int len; //This is the number of bytes to be written ata time.DES des = new DESCryptoServiceProvider();CryptoStream encStream = new CryptoStream(fout, des.CreateEncryptor(desKey, desIV),CryptoStreamMode.Write);//Read from the input file, then encrypt and write to the output file.while (rdlen < totlen){len = fin.Read(bin, 0, 100);encStream.Write(bin, 0, len);rdlen = rdlen + len;}encStream.Close();fout.Close();fin.Close();}//解密文件private static void DecryptData(String inName, String outName, byte[] desKey, byte[]desIV){//Create the file streams to handle the input and output files.FileStream fin = new FileStream(inName, FileMode.Open, FileAccess.Read); FileStream fout = new FileStream(outName, FileMode.OpenOrCreate, FileAccess.Write);fout.SetLength(0);//Create variables to help with read and write.byte[] bin = new byte[100]; //This is intermediate storage for the encryption.long rdlen = 0; //This is the total number of bytes written. long totlen = fin.Length; //This is the total length of the input file. int len; //This is the number of bytes to be written at a time.DES des = new DESCryptoServiceProvider();CryptoStream encStream = new CryptoStream(fout, des.CreateDecryptor(desKey, desIV),CryptoStreamMode.Write);//Read from the input file, then encrypt and write to the output file. while (rdlen < totlen){len = fin.Read(bin, 0, 100);encStream.Write(bin, 0, len);rdlen = rdlen + len;}encStream.Close();fout.Close();fin.Close();}6、using System;using System.Collections.Generic;using System.Text;using System.Security.Cryptography;using System.IO;namespace Component{public class Security{public Security(){}//默认密钥向量private static byte[] Keys = { 0x12, 0x34, 0x56, 0x78, 0x90, 0xAB, 0xCD, 0xEF };/**//**//**//**//**//**//**//// <summary>/// DES加密字符串/// </summary>/// <param name="encryptString">待加密的字符串</param>/// <param name="encryptKey">加密密钥,要求为8位</param>/// <returns>加密成功返回加密后的字符串,失败返回源串</returns>public static string EncryptDES(string encryptString, string encryptKey){try{byte[] rgbKey = Encoding.UTF8.GetBytes(encryptKey.Substring(0, 8));byte[] rgbIV = Keys;byte[] inputByteArray = Encoding.UTF8.GetBytes(encryptString); DESCryptoServiceProvider dCSP = new DESCryptoServiceProvider(); MemoryStream mStream = new MemoryStream();CryptoStream cStream = new CryptoStream(mStream, dCSP.CreateEncryptor(rgbKey,rgbIV), CryptoStreamMode.Write);cStream.Write(inputByteArray, 0, inputByteArray.Length);cStream.FlushFinalBlock();return Convert.ToBase64String(mStream.ToArray());}catch{return encryptString;}}/**//**//**//**//**//**//**//// <summary>/// DES解密字符串/// </summary>/// <param name="decryptString">待解密的字符串</param>/// <param name="decryptKey">解密密钥,要求为8位,和加密密钥相同</param> /// <returns>解密成功返回解密后的字符串,失败返源串</returns>public static string DecryptDES(string decryptString, string decryptKey) {try{byte[] rgbKey = Encoding.UTF8.GetBytes(decryptKey);byte[] rgbIV = Keys;byte[] inputByteArray = Convert.FromBase64String(decryptString); DESCryptoServiceProvider DCSP = new DESCryptoServiceProvider(); MemoryStream mStream = new MemoryStream();CryptoStream cStream = new CryptoStream(mStream, DCSP.CreateDecryptor(rgbKey,rgbIV), CryptoStreamMode.Write);cStream.Write(inputByteArray, 0, inputByteArray.Length);cStream.FlushFinalBlock();return Encoding.UTF8.GetString(mStream.ToArray()); }catch{return decryptString;}}}}。
c语言解密代码
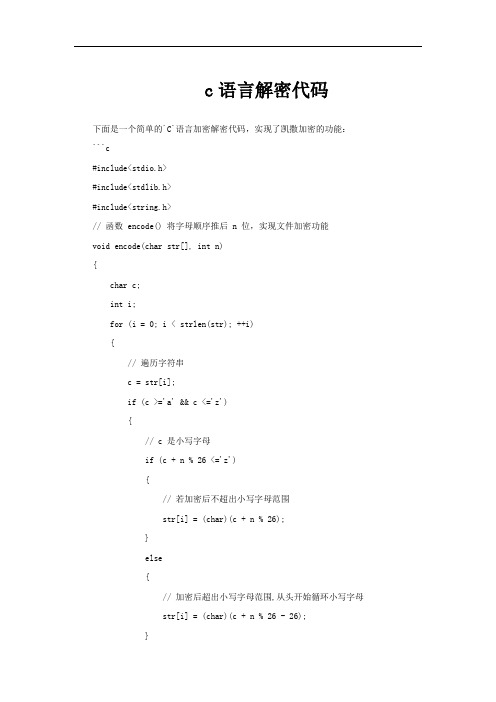
c语言解密代码下面是一个简单的`C`语言加密解密代码,实现了凯撒加密的功能:```c#include<stdio.h>#include<stdlib.h>#include<string.h>// 函数 encode() 将字母顺序推后 n 位,实现文件加密功能void encode(char str[], int n){char c;int i;for (i = 0; i < strlen(str); ++i){// 遍历字符串c = str[i];if (c >='a' && c <='z'){// c 是小写字母if (c + n % 26 <='z'){// 若加密后不超出小写字母范围str[i] = (char)(c + n % 26);}else{// 加密后超出小写字母范围,从头开始循环小写字母 str[i] = (char)(c + n % 26 - 26);}elseif (c >='A' && c <='Z'){// c 为大写字母if (c + n % 26 <= 'Z'){// 加密后不超出大写字母范围str[i] = (char)(c + n % 26);}else{// 加密后超出大写字母范围,从头开始循环大写字母 str[i] = (char)(c + n % 26 - 26);}}else{// 不是字母,不加密str[i] = c;printf("\nAfter encode: \n");puts(str);}}// 输出加密后的字符串printf("\nAfter encode: \n");puts(str);}// 实现解密功能,将字母顺序前移 n 位void decode(char str[], int n){int i;for (i = 0; i < strlen(str); ++i){c = str[i];if (c >='a' && c <='z'){// 解密后还为小写字母,直接解密if (c - n % 26 >='a'){str[i] = (char)(c - n % 26);}else{// 解密后不为小写字母了,通过循环小写字母处理为小写字母 str[i] = (char)(c - n % 26 + 26);}}elseif (c >= 'A' && c <='Z'){// c 为大写字母if (c - n % 26 >='A'){// 解密后还为大写字母str[i] = (char)(c - n % 26);}else{// 解密后不为大写字母了,循环大写字母,处理为大写字母str[i] = (char)(c - n % 26 + 26);}}else{// 不是字母,不加密str[i] = c;}}// 输出解密后的字符串printf("\nAfter decode: \n");puts(str);}int main(){char str[20];int n;printf("请输入字符串(以空格结束输入):\n");gets(str);printf("请输入密钥(1-25的整数):\n");scanf("%d", &n);printf("加密前的字符串为:%s\n", str);encode(str, n);printf("加密后的字符串为:%s\n", str);decode(str, n);printf("解密后的字符串为:%s\n", str);return 0;}```在上述代码中,加密函数`encode()`通过将字符串中的每个字符循环向后移动`n`位实现加密,解密函数`decode()`通过将字符串中的每个字符循环向前移动`n`位实现解密。
c语言实现加密解密
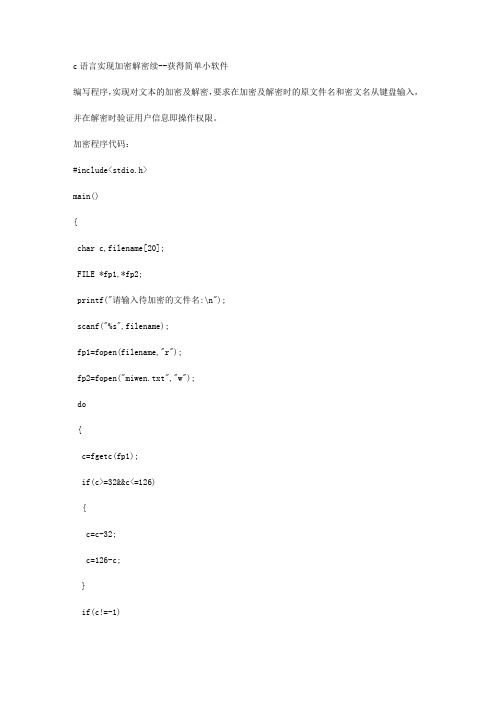
c语言实现加密解密续--获得简单小软件编写程序,实现对文本的加密及解密,要求在加密及解密时的原文件名和密文名从键盘输入,并在解密时验证用户信息即操作权限。
加密程序代码:#include<stdio.h>main(){char c,filename[20];FILE *fp1,*fp2;printf("请输入待加密的文件名:\n");scanf("%s",filename);fp1=fopen(filename,"r");fp2=fopen("miwen.txt","w");do{c=fgetc(fp1);if(c>=32&&c<=126){c=c-32;c=126-c;}if(c!=-1)fprintf(fp2,"%c",c);}while(c!=-1);}解密程序代码:#include<stdio.h>#include<string.h>main(){char c,filename[20];char yanzhengma[20];FILE *fp1,*fp2;printf("请输入待解密文件名:\n");scanf("%s",filename);printf("请输入验证码:\n");scanf("%s",yanzhengma);if(strcmp(yanzhengma,"shan")==0){fp1=fopen(filename,"r"); fp2=fopen("yuanwen.txt","w"); do{c=fgetc(fp1);if(c>=32&&c<=126){c=126-c;c=32+c;}if(c!=-1)fprintf(fp2,"%c",c);}while(c!=-1);}else{printf("验证码错误!请重新输入:\n");scanf("%s",filename);}}运行结果:文件加密:如需要加密的文件名为yusnwen.txt,则屏幕显示为:如yuanwen.txt内容为:qing dao li gong da xue tong xin yu dian zi gong cheng xue yuan 2006 ji dain zi xin xi gong cheng zhuan ye2009 05 17加密后得到的密文miwen.txt为:-507~:=/~25~7/07~:=~&)9~*/07~&50~%)~:5=0~$5~7/07~;6907~&)9~%)=0~lnnh~45~:=50~$5 ~&50~&5~7/07~;6907~$6)=0~%9~~~lnne~ni~mg文件解密:如需解密的文件即为上面加密后的文件miwen.txt,则:当验证码正确时,屏幕显示:得到的原文如加密时的原文。
C语言加密与解密算法的实现与应用

C语言加密与解密算法的实现与应用密码学是信息安全领域的重要分支之一,加密与解密算法是密码学中的核心概念。
在本文中,我们将讨论C语言中加密与解密算法的实现与应用,介绍几种常见的算法,并为读者提供实用的示例代码。
1. 对称加密算法对称加密算法是指加密和解密使用相同密钥的算法。
C语言中常用的对称加密算法有DES(Data Encryption Standard)和AES(Advanced Encryption Standard)。
下面以AES算法为例进行介绍。
AES算法是一种高级加密标准,广泛应用于各种领域的数据保护中。
C语言中可以使用openssl库来实现AES算法的加密和解密操作。
以下为一个简单的AES加密和解密的示例代码:```c#include <openssl/aes.h>#include <string.h>int aes_encrypt(const unsigned char *plaintext, int plaintext_len, unsigned char *key, unsigned char *ciphertext) {AES_KEY aesKey;AES_set_encrypt_key(key, 128, &aesKey);AES_encrypt(plaintext, ciphertext, &aesKey);return 0;int aes_decrypt(const unsigned char *ciphertext, int ciphertext_len, unsigned char *key, unsigned char *plaintext) {AES_KEY aesKey;AES_set_decrypt_key(key, 128, &aesKey);AES_decrypt(ciphertext, plaintext, &aesKey);return 0;}int main() {unsigned char key[] = "0123456789012345";unsigned char plaintext[] = "Hello, World!";unsigned char ciphertext[128];unsigned char decryptedtext[128];aes_encrypt(plaintext, strlen((char *)plaintext), key, ciphertext);aes_decrypt(ciphertext, strlen((char *)ciphertext), key, decryptedtext);printf("Plaintext: %s\n", plaintext);printf("Ciphertext: %s\n", ciphertext);printf("Decrypted text: %s\n", decryptedtext);return 0;```2. 非对称加密算法非对称加密算法使用一对密钥,分别为公钥和私钥。
C语言加解密算法详解
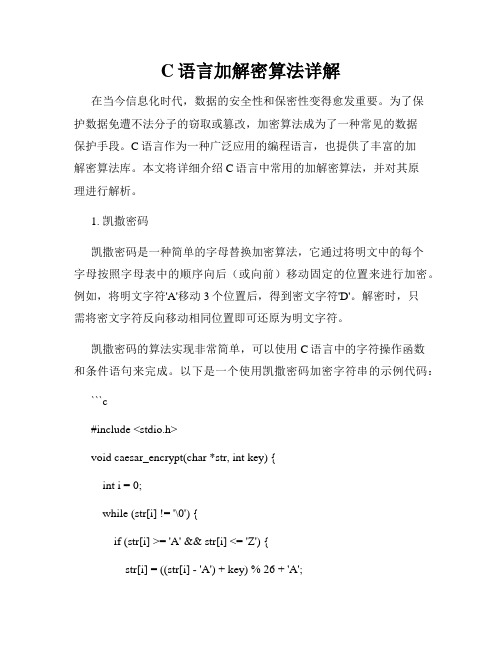
C语言加解密算法详解在当今信息化时代,数据的安全性和保密性变得愈发重要。
为了保护数据免遭不法分子的窃取或篡改,加密算法成为了一种常见的数据保护手段。
C语言作为一种广泛应用的编程语言,也提供了丰富的加解密算法库。
本文将详细介绍C语言中常用的加解密算法,并对其原理进行解析。
1. 凯撒密码凯撒密码是一种简单的字母替换加密算法,它通过将明文中的每个字母按照字母表中的顺序向后(或向前)移动固定的位置来进行加密。
例如,将明文字符'A'移动3个位置后,得到密文字符'D'。
解密时,只需将密文字符反向移动相同位置即可还原为明文字符。
凯撒密码的算法实现非常简单,可以使用C语言中的字符操作函数和条件语句来完成。
以下是一个使用凯撒密码加密字符串的示例代码:```c#include <stdio.h>void caesar_encrypt(char *str, int key) {int i = 0;while (str[i] != '\0') {if (str[i] >= 'A' && str[i] <= 'Z') {str[i] = ((str[i] - 'A') + key) % 26 + 'A';}else if (str[i] >= 'a' && str[i] <= 'z') {str[i] = ((str[i] - 'a') + key) % 26 + 'a';}i++;}}int main() {char str[100] = "Hello, World!";int key = 3;caesar_encrypt(str, key);printf("Encrypted string: %s\n", str);return 0;}```2. DES算法DES(Data Encryption Standard)是一种对称分组密码算法,使用56位的密钥对64位的数据进行加密和解密。
C语言中的加密与解密算法实现

C语言中的加密与解密算法实现在计算机编程领域中,加密与解密算法的实现是非常重要的。
通过加密算法,可以将敏感数据进行保护,以防止未经授权的访问。
同时,解密算法则用于将加密过的数据恢复为原始数据。
本文将介绍C语言中加密与解密算法的实现方法,并探讨一些常用的加密算法。
一、加密算法的实现方法加密算法的实现可以采用C语言中的各种方法和技术。
下面列举了几种常用的加密算法实现方法:1. 移位加密算法移位加密算法是一种简单的加密算法,它通过将字符的ASCII码值向右移动若干位来实现。
例如,将字符'A'的ASCII码值向右移动1位,即可得到字符'B'的ASCII码值。
移位加密算法的实现如下:```cvoid encryptShift(char* message, int key) {int i = 0;while (message[i] != '\0') {message[i] = message[i] + key; // 向右移动key位i++;}}```2. 替换加密算法替换加密算法是通过将字符替换为其他字符来实现加密的。
替换加密算法可以使用预定义的映射表或通过自定义映射关系来实现。
例如,将字符'A'替换为字符'Z',将字符'B'替换为字符'Y',以此类推。
替换加密算法的实现如下:```cvoid encryptSubstitution(char* message) {int i = 0;while (message[i] != '\0') {if (message[i] >= 'A' && message[i] <= 'Z') {message[i] = 'Z' - (message[i] - 'A'); // 替换为对应的字符}i++;}}```3. 数字加密算法数字加密算法主要用于加密数字,例如将手机号码、银行账号等敏感数字进行保护。
C语言中的信息加密与解密

C语言中的信息加密与解密信息加密与解密在现代通信技术中起着非常重要的作用。
在信息传输过程中,为了保护数据的隐私和安全,我们常常需要对敏感信息进行加密,然后再通过网络传输。
而在接收方,我们又需要解密这些加密过的数据,以便正常使用。
在C语言中,我们可以利用一些算法和函数来实现信息的加密与解密。
本文将介绍C语言中常用的几种信息加密与解密的方法。
一、凯撒密码凯撒密码是一种古老且简单的加密方法,它是通过将字母按照一定的偏移量进行替换来加密文本。
在C语言中,我们可以通过 ASCII 码来实现凯撒密码的加密和解密。
加密函数示例代码:```c#include <stdio.h>void caesarCipherEncrypt(char *text, int shift) {int i = 0;while (text[i] != '\0') {if (text[i] >= 'A' && text[i] <= 'Z') {text[i] = ((text[i] - 'A') + shift) % 26 + 'A';}else if (text[i] >= 'a' && text[i] <= 'z') {text[i] = ((text[i] - 'a') + shift) % 26 + 'a'; }i++;}}int main() {char message[100];int shift;printf("请输入要加密的消息: ");gets(message); //输入待加密的消息printf("请输入偏移量: ");scanf("%d", &shift);caesarCipherEncrypt(message, shift);printf("加密后的消息: %s\n", message);return 0;}```解密函数示例代码:```c#include <stdio.h>void caesarCipherDecrypt(char *text, int shift) {int i = 0;while (text[i] != '\0') {if (text[i] >= 'A' && text[i] <= 'Z') {text[i] = ((text[i] - 'A') - shift + 26) % 26 + 'A'; }else if (text[i] >= 'a' && text[i] <= 'z') {text[i] = ((text[i] - 'a') - shift + 26) % 26 + 'a'; }i++;}}int main() {char message[100];int shift;printf("请输入要解密的消息: ");gets(message); //输入待解密的消息printf("请输入偏移量: ");scanf("%d", &shift);caesarCipherDecrypt(message, shift);printf("解密后的消息: %s\n", message);return 0;}```以上代码演示了如何使用凯撒密码进行信息的加密和解密。
C++简单的文件加密和解密实例
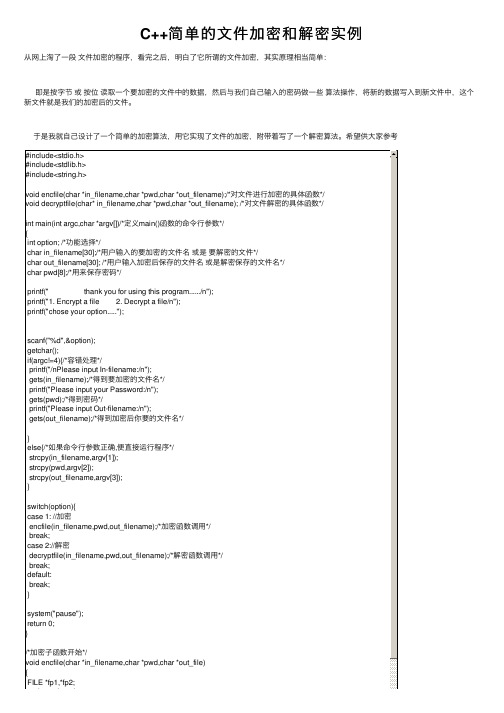
C++简单的⽂件加密和解密实例从⽹上淘了⼀段⽂件加密的程序,看完之后,明⽩了它所谓的⽂件加密,其实原理相当简单:即是按字节或按位读取⼀个要加密的⽂件中的数据,然后与我们⾃⼰输⼊的密码做⼀些算法操作,将新的数据写⼊到新⽂件中,这个新⽂件就是我们的加密后的⽂件。
于是我就⾃⼰设计了⼀个简单的加密算法,⽤它实现了⽂件的加密,附带着写了⼀个解密算法。
希望供⼤家参考fclose(fp1);/*关闭源⽂件*/fclose(fp2);/*关闭⽬标⽂件*/}/*解密⼦函数开始*/void decryptfile(char *in_filename,char *pwd,char *out_file) {FILE *fp1,*fp2;register char ch;int j=0;int j0=0;fp1=fopen(in_filename,"r");/*打开要解密的⽂件*/if(fp1==NULL){printf("cannot open in-file./n");exit(1);/*如果不能打开要解密的⽂件,便退出程序*/}fp2=fopen(out_file,"w");if(fp2==NULL){printf("cannot open or create out-file./n");exit(1);/*如果不能建⽴解密后的⽂件,便退出*/}while(pwd[++j0]);ch=fgetc(fp1);/*解密算法开始*/while(!feof(fp1)){if(j0 >7)j0 = 0;ch -= pwd[j0++];fputc(ch,fp2);/*我的解密算法*/ch=fgetc(fp1);}fclose(fp1);/*关闭源⽂件*/fclose(fp2);/*关闭⽬标⽂件*/}。
C#-文件加密解密

程序的主要功能是:用户通过文件选择框选择要加密的文件-》输入密码进行加密;选择加密后的文件,输入密码进行解密。
程序的主界面如下:三个按钮的Click事件处理程序如下:private void btnSelectFile_Click(object sender, EventArgs e){if (openFileDialog1.ShowDialog() ==System.Windows.Forms.DialogResult.OK){txtFileName.Text = openFileDialog1.FileName ;}}private void btnEncryptFile_Click(object sender, EventArgs e){string inFile=txtFileName.Text;string outFile = inFile + ".dat";string password=txtPassword.Text ;DESFile.DESFileClass.EncryptFile(inFile, outFile, password);//加密文件//删除加密前的文件File.Delete(inFile);txtFileName.Text = string.Empty;MessageBox.Show("加密成功");}private void btnDecryptFile_Click(object sender, EventArgs e){string inFile = txtFileName.Text;string outFile = inFile.Substring(0,inFile.Length - 4);string password = txtPassword.Text;DESFile.DESFileClass.DecryptFile (inFile, outFile, password);//解密文件//删除解密前的文件File.Delete(inFile);txtFileName.Text = string.Empty;MessageBox.Show("解密成功");}加密解密的Help文件源码如下:using System;using System.Collections.Generic;using System.Text;using System.Security.Cryptography;using System.IO;namespace DESFile{/// <summary>/// 异常处理类/// </summary>public class CryptoHelpException : ApplicationException{public CryptoHelpException(string msg) : base(msg) { }}/// <summary>/// CryptHelp/// </summary>public class DESFileClass{private const ulong FC_TAG = 0xFC010*********CF;private const int BUFFER_SIZE = 128 * 1024;/// <summary>/// 检验两个Byte数组是否相同/// <param name="b1">Byte数组</param>/// <param name="b2">Byte数组</param>/// <returns>true-相等</returns>private static bool CheckByteArrays(byte[] b1, byte[] b2){if (b1.Length == b2.Length){for (int i = 0; i < b1.Length; ++i){if (b1[i] != b2[i])return false;}return true;}return false;}/// <summary>/// 创建DebugLZQ ,/DebugLZQ/// </summary>/// <param name="password">密码</param>/// <param name="salt"></param>/// <returns>加密对象</returns>private static SymmetricAlgorithm CreateRijndael(string password, byte[] salt){PasswordDeriveBytes pdb = new PasswordDeriveBytes(password, salt, "SHA256", 1000);SymmetricAlgorithm sma = Rijndael.Create();sma.KeySize = 256;sma.Key = pdb.GetBytes(32);sma.Padding = PaddingMode.PKCS7;return sma;}/// 加密文件随机数生成/// </summary>private static RandomNumberGenerator rand = new RNGCryptoServiceProvider();/// <summary>/// 生成指定长度的随机Byte数组/// </summary>/// <param name="count">Byte数组长度</param>/// <returns>随机Byte数组</returns>private static byte[] GenerateRandomBytes(int count){byte[] bytes = new byte[count];rand.GetBytes(bytes);return bytes;}/// <summary>/// 加密文件/// </summary>/// <param name="inFile">待加密文件</param>/// <param name="outFile">加密后输入文件</param>/// <param name="password">加密密码</param>public static void EncryptFile(string inFile, string outFile, string password){using (FileStream fin = File.OpenRead(inFile),fout = File.OpenWrite(outFile)){long lSize = fin.Length; // 输入文件长度int size = (int)lSize;byte[] bytes = new byte[BUFFER_SIZE]; // 缓存int read = -1; // 输入文件读取数量int value = 0;// 获取IV和saltbyte[] IV = GenerateRandomBytes(16);byte[] salt = GenerateRandomBytes(16);// 创建加密对象SymmetricAlgorithm sma = DESFileClass.CreateRijndael(password, salt);sma.IV = IV;// 在输出文件开始部分写入IV和saltfout.Write(IV, 0, IV.Length);fout.Write(salt, 0, salt.Length);// 创建散列加密HashAlgorithm hasher = SHA256.Create();using (CryptoStream cout = new CryptoStream(fout,sma.CreateEncryptor(), CryptoStreamMode.Write),chash = new CryptoStream(Stream.Null, hasher, CryptoStreamMode.Write)){BinaryWriter bw = new BinaryWriter(cout);bw.Write(lSize);bw.Write(FC_TAG);// 读写字节块到加密流缓冲区while ((read = fin.Read(bytes, 0, bytes.Length)) != 0){cout.Write(bytes, 0, read);chash.Write(bytes, 0, read);value += read;}// 关闭加密流chash.Flush();chash.Close();// 读取散列byte[] hash = hasher.Hash;// 输入文件写入散列cout.Write(hash, 0, hash.Length);// 关闭文件流cout.Flush();cout.Close();}}}/// <summary>/// 解密文件/// </summary>/// <param name="inFile">待解密文件</param>/// <param name="outFile">解密后输出文件</param>/// <param name="password">解密密码</param>public static void DecryptFile(string inFile, string outFile, string password){// 创建打开文件流using (FileStream fin = File.OpenRead(inFile),fout = File.OpenWrite(outFile)){int size = (int)fin.Length;byte[] bytes = new byte[BUFFER_SIZE];int read = -1;int value = 0;int outValue = 0;byte[] IV = new byte[16];fin.Read(IV, 0, 16);byte[] salt = new byte[16];fin.Read(salt, 0, 16);SymmetricAlgorithm sma = DESFileClass.CreateRijndael(password, salt);sma.IV = IV;value = 32;long lSize = -1;// 创建散列对象, 校验文件HashAlgorithm hasher = SHA256.Create();using (CryptoStream cin = new CryptoStream(fin, sma.CreateDecryptor(), CryptoStreamMode.Read),chash = new CryptoStream(Stream.Null, hasher, CryptoStreamMode.Write)){// 读取文件长度BinaryReader br = new BinaryReader(cin);lSize = br.ReadInt64();ulong tag = br.ReadUInt64();if (FC_TAG != tag)throw new CryptoHelpException("文件被破坏"); long numReads = lSize / BUFFER_SIZE;long slack = (long)lSize % BUFFER_SIZE;for (int i = 0; i < numReads; ++i){read = cin.Read(bytes, 0, bytes.Length);fout.Write(bytes, 0, read);chash.Write(bytes, 0, read);value += read;outValue += read;}if (slack > 0){read = cin.Read(bytes, 0, (int)slack);fout.Write(bytes, 0, read);chash.Write(bytes, 0, read);value += read;outValue += read;}chash.Flush();chash.Close();fout.Flush();fout.Close();byte[] curHash = hasher.Hash;// 获取比较和旧的散列对象byte[] oldHash = new byte[hasher.HashSize / 8];read = cin.Read(oldHash, 0, oldHash.Length);if ((oldHash.Length != read) || (!CheckByteArrays(oldHash, curHash)))throw new CryptoHelpException("文件被破坏");}if (outValue != lSize)throw new CryptoHelpException("文件大小不匹配");}}}}。
c加密方法 -回复
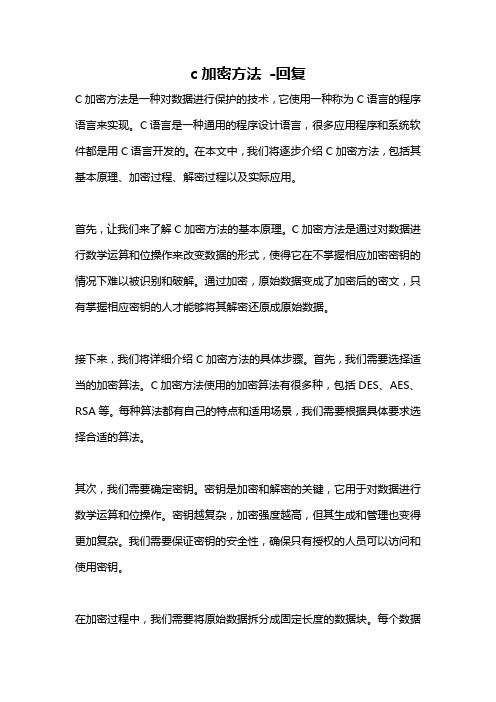
c加密方法-回复C加密方法是一种对数据进行保护的技术,它使用一种称为C语言的程序语言来实现。
C语言是一种通用的程序设计语言,很多应用程序和系统软件都是用C语言开发的。
在本文中,我们将逐步介绍C加密方法,包括其基本原理、加密过程、解密过程以及实际应用。
首先,让我们来了解C加密方法的基本原理。
C加密方法是通过对数据进行数学运算和位操作来改变数据的形式,使得它在不掌握相应加密密钥的情况下难以被识别和破解。
通过加密,原始数据变成了加密后的密文,只有掌握相应密钥的人才能够将其解密还原成原始数据。
接下来,我们将详细介绍C加密方法的具体步骤。
首先,我们需要选择适当的加密算法。
C加密方法使用的加密算法有很多种,包括DES、AES、RSA等。
每种算法都有自己的特点和适用场景,我们需要根据具体要求选择合适的算法。
其次,我们需要确定密钥。
密钥是加密和解密的关键,它用于对数据进行数学运算和位操作。
密钥越复杂,加密强度越高,但其生成和管理也变得更加复杂。
我们需要保证密钥的安全性,确保只有授权的人员可以访问和使用密钥。
在加密过程中,我们需要将原始数据拆分成固定长度的数据块。
每个数据块都会经过一系列的算法和位操作,使其改变形式。
这些操作包括位运算、异或运算、移位运算等。
通过多次的操作,数据块的内容被改变,使其与原始数据之间的关联变得困难。
解密过程与加密过程相反。
我们需要使用相同的密钥和相同的算法,对密文进行逆向操作,将其还原为原始数据。
解密过程需要确保使用正确的密钥,否则无法还原数据。
除了基本的加密和解密操作,C加密方法还可以进一步增强其安全性。
例如,我们可以使用哈希算法对数据进行摘要,将摘要添加到数据中,以确保数据的完整性。
此外,我们还可以使用数字签名来验证数据的来源和真实性。
C加密方法在实际应用中有着广泛的用途。
它可以用于保护敏感信息,如用户密码、银行账户和个人隐私数据等。
它也可以用于保护数据传输过程中的安全,如网络通信、文件传输等。
c语言去除压缩文件密码方法

C语言去除压缩文件密码方法详解在日常工作中,我们经常会遇到需要处理各种类型的压缩文件,其中一些文件可能被设置了密码。
如果忘记了密码,可能会导致无法访问文件中的内容。
那么,如何使用C语言来去除压缩文件的密码呢?本文将详细解析这个问题。
首先,我们需要了解的是,去除压缩文件的密码并不是一件简单的事情。
因为大多数压缩工具(如WinRAR、7-Zip等)都会对加密后的数据进行复杂的变换,以防止未经授权的人访问。
因此,直接通过编程手段破解密码是相当困难的。
然而,如果我们知道压缩文件的密码,就可以通过编程的方式去除它。
下面,我们将以RAR文件为例,讲解如何使用C语言去除压缩文件的密码。
RAR是一种流行的压缩格式,它的密码保护功能非常强大。
然而,RAR库提供了一种称为"SetPassword"的方法,可以让我们设置或取消RAR文件的密码。
以下是一个简单的C语言示例,演示了如何使用RAR库去除RAR文件的密码:```c#include <rar.h>#include <stdio.h>int main(int argc, char argv) {if (argc != 3) {printf("Usage: %s <archive> <password>\n", argv[0]);return 1;}RARHeaderDataEx hd;memset(&hd, 0, sizeof(hd));hd.NewNumber = -1;HANDLE hArcData = OpenArchive(argv[1], false);if (hArcData == NULL) {printf("Failed to open archive\n");return 1;}SetFileAttr(NULL, argv[1], FILE_ATTRIBUTE_NORMAL);if (!LockArchive(hArcData)) {printf("Failed to lock archive\n");CloseArchive(hArcData);return 1;}if (!SetPassword(hArcData, argv[2])) {printf("Invalid password\n");UnlockArchive(hArcData);CloseArchive(hArcData);return 1;}int result = ChangeArchiveExt(hd, hArcData, NULL, argv[1], "tmp.rar"); if (result != E_SUCCESS) {printf("Failed to change archive extension: %d\n", result);}UnlockArchive(hArcData);CloseArchive(hArcData);return 0;}```这个程序接受两个参数:第一个参数是要操作的RAR文件名,第二个参数是要去除的密码。