2011信息安全数据结构实验1
2011数据结构

2011数据结构《2011 数据结构》在当今数字化的时代,数据结构成为了计算机科学中至关重要的一部分。
它不仅影响着程序的运行效率,还决定了我们如何有效地组织和管理数据。
2011 年,数据结构领域也有着不少值得关注和探讨的发展。
数据结构,简单来说,就是指数据元素之间的关系和组织方式。
它就像是一个仓库的布局,决定了货物(数据)的存放和取出方式。
常见的数据结构包括数组、链表、栈、队列、树和图等等。
数组是一种最简单的数据结构,它就像一排整齐排列的盒子,每个盒子都有一个固定的位置。
通过索引可以快速地访问到特定位置的数据。
但数组的大小是固定的,在需要添加或删除元素时可能会比较麻烦。
链表则不同,它像是一串珠子,每个珠子(节点)都包含数据和指向下一个节点的指针。
链表的长度可以动态变化,添加和删除元素相对比较容易,但访问特定位置的元素就需要逐个节点遍历,效率相对较低。
栈和队列是两种特殊的线性结构。
栈遵循“后进先出”的原则,就像一个堆满盘子的洗碗机,最后放进去的盘子会最先被拿出来。
队列则遵循“先进先出”的原则,好比在银行排队办理业务,先排队的人先得到服务。
树是一种层次结构的数据结构,比如二叉树、二叉搜索树等。
二叉树每个节点最多有两个子节点,而二叉搜索树则具有特定的排序规则,使得查找、插入和删除操作的效率较高。
图则是更加复杂的数据结构,用于表示多个对象之间的复杂关系。
它由顶点和边组成,可以用于解决很多实际问题,比如地图导航、网络拓扑等。
在 2011 年,随着计算机技术的不断发展和应用需求的增加,数据结构的研究和应用也在不断深入。
在算法设计中,数据结构的选择往往起着关键作用。
例如,在需要频繁进行查找操作的情况下,选择二叉搜索树可能会比链表更高效。
而在需要快速添加和删除元素的场景中,栈和队列可能会更适用。
同时,数据结构也在数据库管理系统中扮演着重要角色。
数据库中的索引结构通常基于某种特定的数据结构来实现,以提高数据的查询效率。
信管数据结构实验
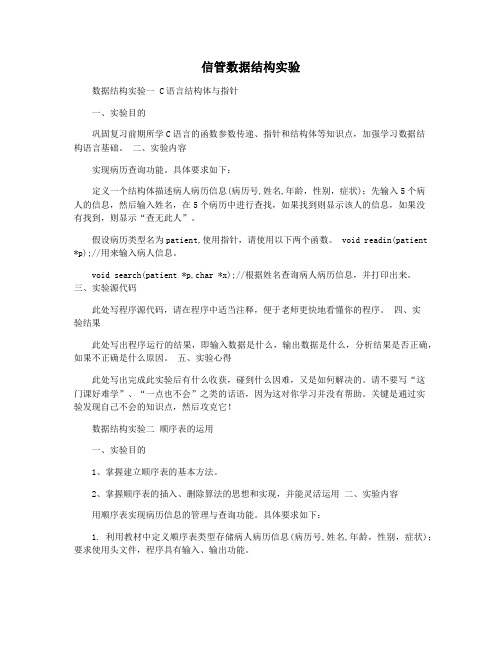
信管数据结构实验数据结构实验一 C语言结构体与指针一、实验目的巩固复习前期所学C语言的函数参数传递、指针和结构体等知识点,加强学习数据结构语言基础。
二、实验内容实现病历查询功能。
具体要求如下:定义一个结构体描述病人病历信息(病历号,姓名,年龄,性别,症状);先输入5个病人的信息,然后输入姓名,在5个病历中进行查找,如果找到则显示该人的信息,如果没有找到,则显示“查无此人”。
假设病历类型名为patient,使用指针,请使用以下两个函数。
void readin(patient *p);//用来输入病人信息。
void search(patient *p,char *x);//根据姓名查询病人病历信息,并打印出来。
三、实验源代码此处写程序源代码,请在程序中适当注释,便于老师更快地看懂你的程序。
四、实验结果此处写出程序运行的结果,即输入数据是什么,输出数据是什么,分析结果是否正确,如果不正确是什么原因。
五、实验心得此处写出完成此实验后有什么收获,碰到什么因难,又是如何解决的。
请不要写“这门课好难学”、“一点也不会”之类的话语,因为这对你学习并没有帮助。
关键是通过实验发现自己不会的知识点,然后攻克它!数据结构实验二顺序表的运用一、实验目的1、掌握建立顺序表的基本方法。
2、掌握顺序表的插入、删除算法的思想和实现,并能灵活运用二、实验内容用顺序表实现病历信息的管理与查询功能。
具体要求如下:1. 利用教材中定义顺序表类型存储病人病历信息(病历号,姓名,年龄,性别,症状);要求使用头文件,程序具有输入、输出功能。
2.设计顺序表定位查找算法,完成的功能为:在线性表L中查找数据元素x,如果存在则返回线性表中和x值相等的第1个数据元素的序号;如果不存在,则返回-1。
函数定义为 int ListFind(SeqList L,char *x)请在主函数中测试查找是否存在姓名为x的病人,并根据返回的序号打印出病人信息。
数据结构实验三有序单链表一、【实验目的】1、掌握建立单链表的基本方法。
信息安全实验报告(一)
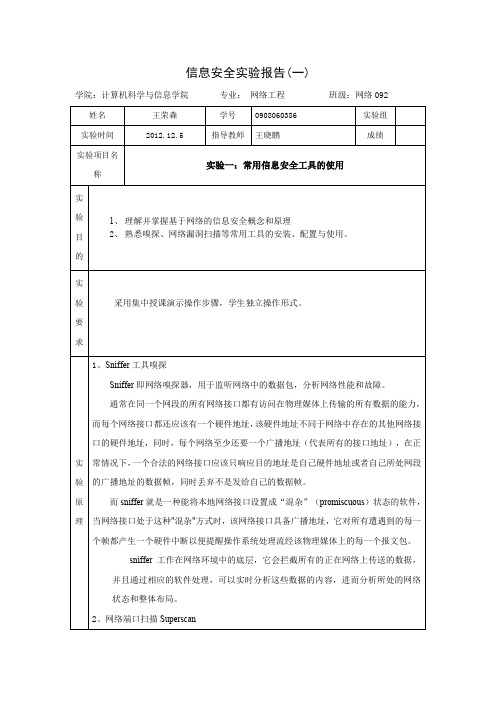
1、关闭计算机的防病毒软件。
2、安装Sniffer Pro。
3、安装Superscan
4、安装Fluxay5。
5、将同一实验组的计算机设为同一网段。
6、利用Sniffer Pro观察对方的网络数据包,分析网络性能和故障。
7、用Superscan扫描对方的计算机,记录扫描的结果和发现的漏洞。
8、用Fluxay5扫描系统的漏洞并破解出另一台机器的帐号与口令
而sniffer就是一种能将本地网络接口设置成“混杂”(promiscuous)状态的软件,当网络接口处于这种"混杂"方式时,该网络接口具备广播地址,它对所有遭遇到的每一个帧都产生一个硬件中断以便提醒操作系统处理流经该物理媒体上的每一个报文包。
sniffer工作在网络环境中的底层,它会拦截所有的正在网络上传送的数据,并且通过相应的软件处理,可以实时分析这些数据的内容,进而分析所处的网络状态和整体布局。
信息安全实验报告(一)
学院:计算机科学与信息学院 专业: 网络工程 班级:网络092
姓名
王荣森
学号
0908060386
实验组
实验时间
2012.12.5
指导教师
王晓鹏
成绩
实验项目名称
实验一:常用信息安全工具的使用
实验目的
1、理解并掌握基于网络的信息安全概念和原理
2、熟悉嗅探、网络漏洞扫描等常用工具的安装、配置与使用。
(2)TCP SYN扫描
本地主机向目标主机发送SYN数据段,如果远端目标主机端口开放,则回应SYN=1,ACK=1,此时本地主机发送RST给目标主机,拒绝连接。如果远端主机端口未开放,则会回应RST给本地主机。由此可知,根据回应的数据段可以判断目标主机的端口是否开放。由于TCP SYN扫描并没有建立TCP正常连接,所以降低了被发现的可能,同时提高了扫描性能。
信息安全实验报告
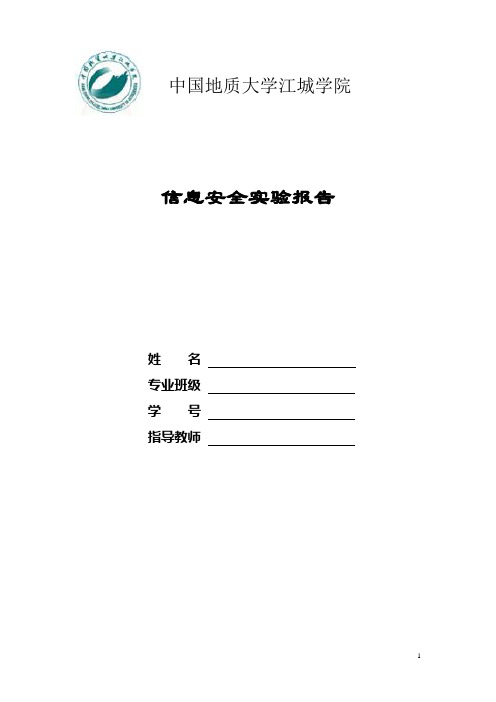
中国地质大学江城学院信息安全实验报告姓名专业班级学号指导教师目录实验1 RAR文件密码破解 (3)实验2 用C语言编程实现凯撒密码加密 (4)实验3 扫描器X—Scan 的使用 (5)实验4 宏病毒分析及清除实验 (6)实验5 CHM木马的制作 (7)实验6 Web恶意代码实验 (8)实验7 T-mouse病毒的实验及分析 (9)实验10 RSA加解密算法的实现 (10)实验12 使用sniffer捕获加密包和非加密包........................错误!未定义书签。
实验15 灰鸽子病毒原理.........................................................错误!未定义书签。
实验1 RAR文件密码破解实验目的:掌握RAR文件密码暴力破解工具的使用方法。
实验设备:计算机一台实验步骤:1.新建一个RAR文件并对其加密。
2.从网上下载任一款RAR文件密码暴力破解软件,查看其使用方法。
(注明所用软件名称)3.利用你所搜索的软件对你的RAR文件进行解密。
(解密过程请详细记录)4.顺利打开你所破解后的RAR文件,实验成功。
实验2 用C语言编程实现凯撒密码加密实验目的:通过C语言编程掌握凯撒密码加密原理。
所用软件:TC2.0实验内容:为了使电报保密,往往按一定规律将其转换成密码。
根据凯撒密码加密原理,已知明文电报,编写一段程序,按照凯撒加密规律将明文电报变为密文。
其中,密钥k=4。
实验3 扫描器X—Scan 的使用实验目的:掌握扫描器X-Scan的使用方法。
利用X-Scan发现系统漏洞。
实验设备:连入Internet的计算机一台所用软件:扫描器 X-Scan实验4 宏病毒分析及清除实验实验目的:1.演示宏的编写2.说明宏的原理及其安全漏洞和缺陷3.理解宏病毒的作用机制实验5 CHM木马的制作CHM是“Compiled Help Manual”的简写,即“已编译的帮助文件”,是微软新一代的帮助文件格式,利用HTML作源文,把帮助内容以类似数据库的形式编译储存。
信息安全实验报告
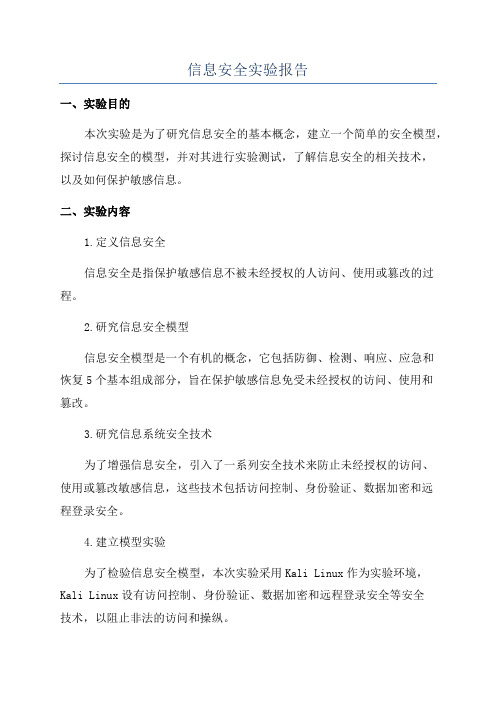
信息安全实验报告
一、实验目的
本次实验是为了研究信息安全的基本概念,建立一个简单的安全模型,探讨信息安全的模型,并对其进行实验测试,了解信息安全的相关技术,
以及如何保护敏感信息。
二、实验内容
1.定义信息安全
信息安全是指保护敏感信息不被未经授权的人访问、使用或篡改的过程。
2.研究信息安全模型
信息安全模型是一个有机的概念,它包括防御、检测、响应、应急和
恢复5个基本组成部分,旨在保护敏感信息免受未经授权的访问、使用和
篡改。
3.研究信息系统安全技术
为了增强信息安全,引入了一系列安全技术来防止未经授权的访问、
使用或篡改敏感信息,这些技术包括访问控制、身份验证、数据加密和远
程登录安全。
4.建立模型实验
为了检验信息安全模型,本次实验采用Kali Linux作为实验环境,Kali Linux设有访问控制、身份验证、数据加密和远程登录安全等安全
技术,以阻止非法的访问和操纵。
三、实验结果
1.安全技术实施完毕
在实验中,实施了访问控制、身份验证、数据加密和远程登录安全等安全技术,保证了正常的服务器运行。
2.平台安全性测试
采用Metasploit框架进行安全测试。
信息安全实验报告

信息安全实验报告一、实验目的随着信息技术的飞速发展,信息安全问题日益凸显。
本次实验的目的在于深入了解信息安全的重要性,掌握常见的信息安全攻击与防御手段,并通过实际操作提高对信息安全问题的应对能力。
二、实验环境本次实验在以下环境中进行:1、操作系统:Windows 10 专业版2、应用软件:Wireshark 网络协议分析工具、Metasploit 渗透测试框架、Nmap 网络扫描工具等3、网络环境:实验室内部局域网三、实验内容1、网络扫描与漏洞检测使用Nmap 工具对目标网络进行扫描,获取网络中主机的存活状态、开放端口等信息。
通过分析扫描结果,发现了一些潜在的安全漏洞,如某些主机开放了不必要的端口,可能导致未经授权的访问。
2、密码破解利用常见的密码破解工具,对预设的密码哈希值进行破解。
实验中采用了字典攻击和暴力攻击两种方法。
通过字典攻击,成功破解了一些简单的密码;而暴力攻击由于计算资源消耗较大,在有限的时间内未能取得明显效果。
3、网络监听与数据包分析使用 Wireshark 工具对网络中的数据包进行捕获和分析。
通过对捕获的数据包进行深入研究,发现了一些明文传输的敏感信息,如用户名和密码,这暴露了网络通信中的安全隐患。
4、漏洞利用与渗透测试借助 Metasploit 框架,对存在漏洞的目标系统进行渗透测试。
成功利用了一个已知的漏洞获取了系统的控制权,这充分说明了及时修复系统漏洞的重要性。
四、实验结果与分析1、网络扫描结果显示,部分主机存在安全配置不当的问题,开放了过多的端口,增加了遭受攻击的风险。
建议加强网络设备的安全配置,只开放必要的端口。
2、密码破解实验表明,简单的密码容易被破解,用户应采用复杂且包含多种字符类型的密码,并定期更换密码,以提高账户的安全性。
3、网络监听和数据包分析揭示了明文传输敏感信息的严重性。
应推广使用加密技术,确保数据在网络中的传输安全。
4、漏洞利用和渗透测试结果强调了及时更新系统补丁和加强安全防护的必要性,以防止黑客利用已知漏洞入侵系统。
信息安全实验内容2011
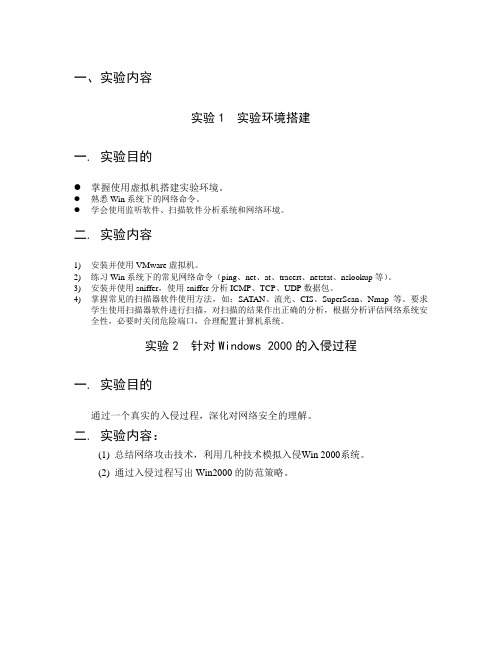
一、实验内容实验1 实验环境搭建一. 实验目的●掌握使用虚拟机搭建实验环境。
●熟悉Win系统下的网络命令。
●学会使用监听软件、扫描软件分析系统和网络环境。
二. 实验内容1)安装并使用VMware虚拟机。
2)练习Win系统下的常见网络命令(ping、net、at、tracert、netstat、nslookup等)。
3)安装并使用sniffer,使用sniffer分析ICMP、TCP、UDP数据包。
4)掌握常见的扫描器软件使用方法,如:SATAN、流光、CIS、SuperScan、Nmap等。
要求学生使用扫描器软件进行扫描,对扫描的结果作出正确的分析,根据分析评估网络系统安全性,必要时关闭危险端口,合理配置计算机系统。
实验2 针对Windows 2000的入侵过程一. 实验目的通过一个真实的入侵过程,深化对网络安全的理解。
二. 实验内容:(1) 总结网络攻击技术,利用几种技术模拟入侵Win 2000系统。
(2) 通过入侵过程写出Win2000的防范策略。
实验3 Windows2000的安全配置一. 实验目的了解Windows2000的系统安全原理,掌握其系统安全配置。
二.实验内容以报告的形式编写Windows 2000 Server/Advanced Server的安全配置方案。
参考内容:详见第7章ppt。
1、帐户和口令的安全设置⏹停止Guest帐号、限制用户数量⏹创建多个管理员帐号、管理员帐号改名、陷阱帐号⏹不显示上次登录名⏹开启密码策略、开启帐户策略⏹帐户锁定策略,例如限制用户错误登录次数为3次且锁定机器后30分钟后才复位。
⏹启用密码策略,例如:a)启用密码必须符合复杂性要求;b)设置密码长度最小值为7位;c)设置密码最长寸留期为42天;d)密码最短存留期为7天;e)强制密码历史为3个。
2、启用审核和日志查看3、启用安全策略和安全模板⏹启用安全摸板a)开始-运行-输入“mmc”,打开控制台b)单击“控制台”选择“添加/删除管理单元”选择“安全摸板”和“安全配置分析”,单击“添加”按钮c)打开“安全摸板”文件夹,右击摸板名称,选择“设置描述”,可以看到该摸板的相关信息。
信息安全实验报告
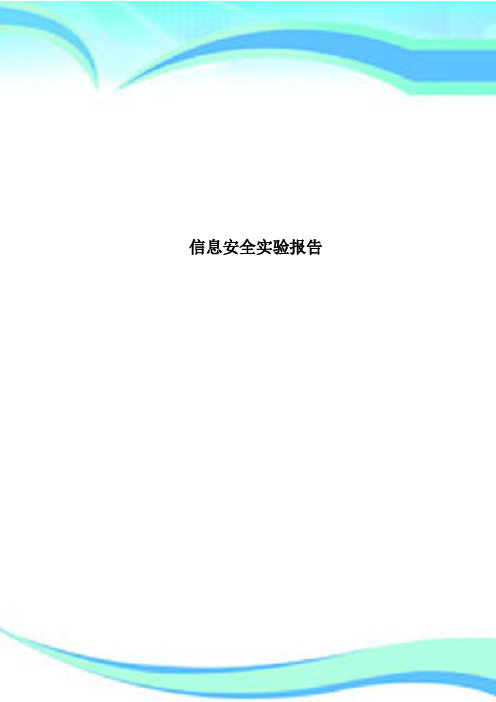
信息安全实验报告————————————————————————————————作者:————————————————————————————————日期:ﻩ信息安全基础实验报告姓名:田廷魁学号:2 班级:网工1201班ARP欺骗工具及原理分析(Sniffer网络嗅探器)一.实验目的和要求实验目的:1.熟悉ARP欺骗攻击有哪些方法。
2.了解ARP欺骗防范工具的使用。
3.掌握ARP欺骗攻击的实验原理。
实验要求:下载相关工具和软件包(ARP攻击检测工具,局域网终结者,网络执法官,ARPsniffer嗅探工具)。
二.实验环境(实验所用的软硬件)ARP攻击检测工具局域网终结者ﻫ网络执法官ARPsniffer嗅探工具三.实验原理ARP(AddressResolutionProtocol)即地址解析协议,是一种将IP地址转化成物理地址的协议。
不管网络层使用什么协议,在网络链路上传送数据帧时,最终还是必须使用硬件地址的。
而每台机器的MAC地址都是不一样的,具有全球唯一性,因此可以作为一台主机或网络设备的标识。
目标主机的MAC地址就是通过ARP协议获得的。
ARP欺骗原理则是通过发送欺骗性的ARP数据包致使接收者收到数据包后更新其ARP缓存表,从而建立错误的IP与MAC对应关系,源主机发送数据时数据便不能被正确地址接收。
四.实验内容与步骤1、利用ARPsniffer嗅探数据实验须先安装winpcap.exe它是arpsniffer.exe运行的条件,接着在arp sniffer.exe同一文件夹下新建记事本,输入Start cmd.exe ,保存为cmd.bat。
ARPsniffer有很多种欺骗方式,下面的例子是其中的一种。
安装截图:步骤一:运行cmd.exe,依次输入以下命令:"arpsf.exe-sniffall-o f:\sniffer.txt -g192.168.137.1 -t 192.168.137.5"(其中IP地址:192.168.137.1是局域网网关的地址,192.168.137.5是被欺骗主机的IP地址,试验获取的数据将会被输入到F盘的sniff er.txt文件中。
信息安全的实验报告(3篇)

第1篇一、实验目的本次实验旨在通过实际操作,加深对信息安全理论知识的理解,提高实际操作能力,培养信息安全意识。
实验内容包括:1. 熟悉常用的信息安全工具和软件;2. 学习基本的加密和解密方法;3. 掌握常见的信息安全攻击和防范措施;4. 了解网络安全的防护策略。
二、实验原理信息安全是指保护信息在存储、传输和处理过程中的保密性、完整性和可用性。
本实验涉及以下原理:1. 加密技术:通过对信息进行加密,使未授权者无法获取原始信息;2. 解密技术:使用密钥对加密信息进行解密,恢复原始信息;3. 安全协议:确保信息在传输过程中的安全;4. 入侵检测:实时监控网络和系统,发现并阻止恶意攻击。
三、实验内容1. 加密与解密实验(1)使用RSA算法对文件进行加密和解密;(2)使用AES算法对文件进行加密和解密;(3)使用对称密钥和非对称密钥进行加密和解密。
2. 信息安全工具使用实验(1)使用Wireshark抓取网络数据包,分析网络通信过程;(2)使用Nmap进行网络扫描,发现目标主机的开放端口;(3)使用XSSTest进行跨站脚本攻击实验;(4)使用SQL注入攻击实验。
3. 信息安全防护实验(1)使用防火墙设置访问控制策略;(2)使用入侵检测系统(IDS)监控网络流量;(3)使用安全审计工具对系统进行安全审计。
四、实验步骤1. 安装实验所需软件,如Wireshark、Nmap、XSSTest等;2. 按照实验指导书的要求,进行加密和解密实验;3. 使用信息安全工具进行网络扫描、漏洞扫描和攻击实验;4. 设置防火墙和入侵检测系统,对网络进行安全防护;5. 使用安全审计工具对系统进行安全审计。
五、实验结果与分析1. 加密与解密实验:成功使用RSA和AES算法对文件进行加密和解密,验证了加密技术的有效性;2. 信息安全工具使用实验:成功使用Wireshark抓取网络数据包,分析网络通信过程;使用Nmap进行网络扫描,发现目标主机的开放端口;使用XSSTest和SQL 注入攻击实验,验证了信息安全工具的功能;3. 信息安全防护实验:成功设置防火墙和入侵检测系统,对网络进行安全防护;使用安全审计工具对系统进行安全审计,发现潜在的安全隐患。
信息安全实验报告
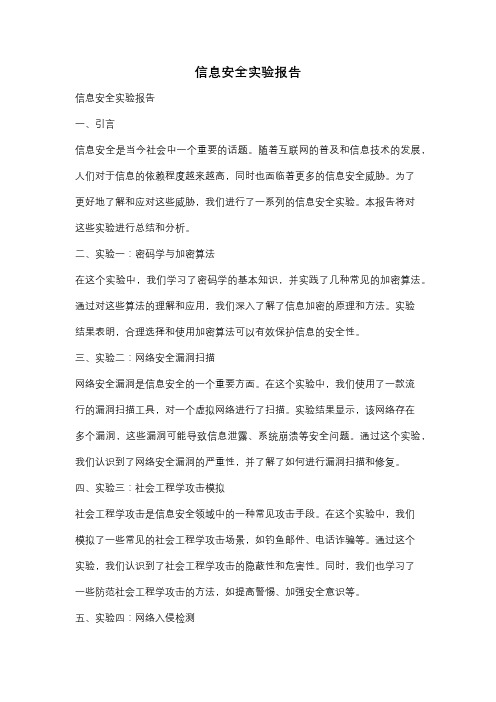
信息安全实验报告信息安全实验报告一、引言信息安全是当今社会中一个重要的话题。
随着互联网的普及和信息技术的发展,人们对于信息的依赖程度越来越高,同时也面临着更多的信息安全威胁。
为了更好地了解和应对这些威胁,我们进行了一系列的信息安全实验。
本报告将对这些实验进行总结和分析。
二、实验一:密码学与加密算法在这个实验中,我们学习了密码学的基本知识,并实践了几种常见的加密算法。
通过对这些算法的理解和应用,我们深入了解了信息加密的原理和方法。
实验结果表明,合理选择和使用加密算法可以有效保护信息的安全性。
三、实验二:网络安全漏洞扫描网络安全漏洞是信息安全的一个重要方面。
在这个实验中,我们使用了一款流行的漏洞扫描工具,对一个虚拟网络进行了扫描。
实验结果显示,该网络存在多个漏洞,这些漏洞可能导致信息泄露、系统崩溃等安全问题。
通过这个实验,我们认识到了网络安全漏洞的严重性,并了解了如何进行漏洞扫描和修复。
四、实验三:社会工程学攻击模拟社会工程学攻击是信息安全领域中的一种常见攻击手段。
在这个实验中,我们模拟了一些常见的社会工程学攻击场景,如钓鱼邮件、电话诈骗等。
通过这个实验,我们认识到了社会工程学攻击的隐蔽性和危害性。
同时,我们也学习了一些防范社会工程学攻击的方法,如提高警惕、加强安全意识等。
五、实验四:网络入侵检测网络入侵是信息安全领域中的一个重要问题。
在这个实验中,我们使用了一款网络入侵检测系统,对一个虚拟网络进行了入侵检测。
实验结果显示,该网络存在多个入侵行为,如端口扫描、暴力破解等。
通过这个实验,我们认识到了网络入侵的危害性和复杂性,并学习了一些网络入侵检测的方法和技巧。
六、实验五:应急响应与恢复在信息安全领域,及时的应急响应和恢复是非常重要的。
在这个实验中,我们模拟了一次网络攻击事件,并进行了应急响应和恢复工作。
通过这个实验,我们了解了应急响应的流程和方法,并学习了一些数据恢复的技巧。
实验结果表明,及时的应急响应和恢复可以最大程度地减少信息安全事件的损失。
信息安全实验报告(一)

DONGFANG COLLEGE,FUJIAN AGRICULTURE AND FORESTRY UNIVERSITY课程名称:信息安全概论系别:计算机系年级专业:计算机系学号:1450301045姓名:陈城任课教师:陈丽娟2016 年 2 月 24 日实验一:PGP软件的安装与使用一、实验目的:1.了解加密工具PGP的原理2.熟悉PGP简单配置方法3.掌握PGP软件公钥与私钥的生成方法,PGPKeys管理密钥的方法。
4.学会使用PGP软件收发加密邮件。
二、实验准备:1.要求实验室内网络是连通的,两个人为一组,组内计算机可以相互访问。
2.安装PGP加密软件;主机操作系统为Windows7或Windows XP;三、实验涉及到的相关软件下载:四、实验原理PGP—Pretty Good Privacy,是一个基于RSA公匙加密体系的邮件加密软件。
可以用它对你的邮件保密以防止非授权者阅读,它还能对你的邮件加上数字签名从而使收信人可以确信邮件是你发来的。
它让你可以安全地和你从未见过的人们通讯,事先并不需要任何保密的渠道用来传递密匙。
它采用了:审慎的密匙管理,一种RSA和传统加密的杂合算法,用于数字签名的邮件文摘算法,加密前压缩等,还有一个良好的人机工程设计。
它的功能强大有很快的速度。
而且它的源代码是免费的。
五、实验步骤【实验分析】(1) 实验拓扑图(2) 导出密钥: 启动PGPkeys, 单击显示刚才创建的用户那里,再在上面点右键,选“Export…(导出)”,在出现的保存对话框中,确认是只选中了“Include 6.0 Extensions”(包含6.0公钥),然后选择一个目录,再点“保存”按钮,即可导出你的公钥,扩展名为.asc导入密钥:点击对方发给自己的扩展名为.asc的公钥(或者直接将pkr公钥文件拖入窗口),将会出现选择公钥的窗口,在这里你能看到该公钥的基本属性,如有效性、创建时间,信任度等,便于了解是否应该导入此公钥。
信息安全实验报告概要
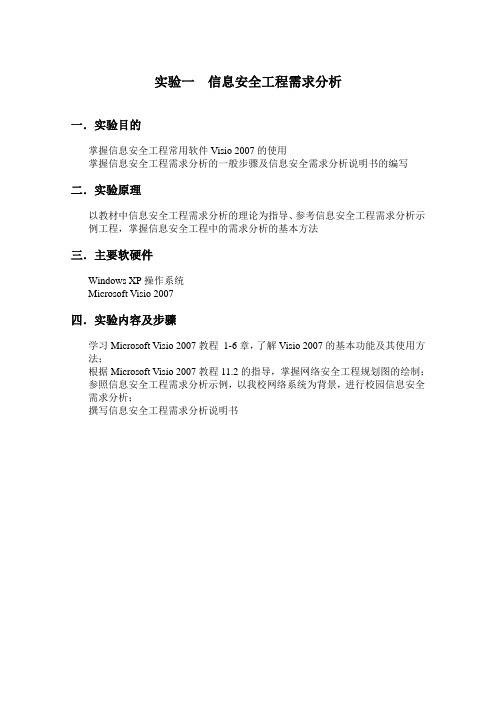
实验一信息安全工程需求分析一.实验目的掌握信息安全工程常用软件Visio 2007的使用掌握信息安全工程需求分析的一般步骤及信息安全需求分析说明书的编写二.实验原理以教材中信息安全工程需求分析的理论为指导、参考信息安全工程需求分析示例工程,掌握信息安全工程中的需求分析的基本方法三.主要软硬件Windows XP操作系统Microsoft Visio 2007四.实验内容及步骤学习Microsoft Visio 2007教程1-6章,了解Visio 2007的基本功能及其使用方法;根据Microsoft Visio 2007教程11.2的指导,掌握网络安全工程规划图的绘制;参照信息安全工程需求分析示例,以我校网络系统为背景,进行校园信息安全需求分析;撰写信息安全工程需求分析说明书{ 重庆邮电大学网络建设} 产品需求规格说明书版本历史文档介绍0.1 文档目的通过编写关于重庆邮电大学网络建设的需求报告,分析重庆邮电大学网络安全隐患,同时提出一些有效的建议和解决方法,以达到对我校网络安全的安全需求分析。
0.2 文档范围通过文档来了解重庆邮电大学的网络拓扑图和网络概况,让读者能够掌握一些必要的网络问题及解决方法。
0.3 读者对象全体师生及开发者。
0.4 参考文档唐成华信息安全工程与管理西安电子科技大学2012年12月第一版赵俊阁信息安全工程武汉大学出版社,20080.5 术语与缩写解释1.安全风险分析1.1网络体系结构概述重庆邮电大学建有自己的网络体系结构,它包含网络部,信息部等,主要负责网络的维护以及加强涉密计算机和信息系统保密管理。
学校建成万兆双冗余骨干网,有线网络和无线网络覆盖全校所有区域,全网支持IPv4/IPv6双栈,楼宇互联达到千兆,桌面接入达到百兆/千兆,校园网带宽能够满足教育、教学、科研、管理及服务的需要。
学校网络属于高等规模网络体系网,包括了所有的学生宿舍,所有的教学楼以及办公楼以及学校的教师公寓和培训中心、校医院以及核心楼信科大楼等,网络体系结构如下:1.2安全现状当前,学校在安全方面主要采取的措施有:1.在网络边界安装防火墙2.使用核心交换机华为9312-1作为局域网组网设备,使用服务器群作为学校数据中心3.通过各个区域的路由器对数据进行过滤及访问控制4.子网划分采取核心交换机划分,有自己的私网IP规划5.部分设备存在一些不安全因素,如木马病毒,恶意插件6.可以利用操作系统、数据库等方式对学校网络进行权限控制1.3、出现的安全问题学校网络与外界之间只有一个防火墙,虽然可以过滤掉部分数据包,但仍然存在很多安全性问题。
(完整word版)数据结构实验一-通讯录

数据结构实验报告实验名称: 实验一——线性表学生姓名: 大学霸班级: xxxxxxxxxx班内序号: xx学号: xxxxxxxxxx1.日期: 2012年11月1日2.实验要求1.实验目的:2.学习指针, 模板类, 异常处理的使用;3.掌握线性表的操作实现方法;4.培养使用线性表解决实际问题的能力。
实验内容:利用线性表实现一个通讯录管理, 通信录的数据格式如下:struct DataType{int ID; //编号char name[10]; //姓名char ch; //性别char phone[13]; //电话char addr[31]; //地址};1.具体要求:2.实现通信录的建立、增加、删除、修改、查询等功能3.能够实现简单的菜单交互, 即可以根据用户输入的命令, 选择不同的操作4.能够保存每次更新的数据5.编写main()函数测试操作的正确性2.程序分析编程完成通讯录的一般性管理工作如通讯录中记录的增加、修改、查找、删除、输出等功能。
每个记录包含姓名、电话号码、住址等个人基本信息。
用《数据结构》中的链表做数据结构结合c语言基本知识编写一个通讯录管理系统。
本程序为使用方便, 几乎不用特殊的命令, 只需按提示输入即可, 适合更多的用户使用。
对于建立通讯录管理系统, 则需了解并掌握数据结构与算法的设计方法,提高综合运用所学的理论知识和方法独立分析和解决问题的能力。
2.1 存储结构节点结构:存储结构: 带头结点和尾结点的单链表front2.2 关键算法分析本实验从整体上分为七大模块: (1)输入联系人信息;(2)添加联系人信息;(3)查找联系人信息;(4)查看联系人信息;(5)删除联系人信息;(6)修改联系人信息;(7)退出通讯录管理。
通讯录系统图2.2.1通讯录的建立伪代码:1.在堆中申请新的结点;2.新节点的数据域为a[i];3.将新节点加入到链表中;4.修改尾指针;5.全部结点插入后需要将终结结点的指针域设为空。
信息安全专业实验报告

信息安全专业实验报告1. 实验目的本次实验的主要目的是对信息安全领域中常见的攻击手段进行实践操作,并通过实验来加深对信息安全的理解,提高解决问题的能力。
2. 实验环境与工具实验环境:使用一台运行Windows操作系统的计算机。
实验工具:使用Kali Linux、Wireshark、Metasploit等常见的信息安全工具。
3. 实验步骤与结果分析3.1 钓鱼攻击实验3.1.1 实验步骤1. 在Kali Linux上安装并配置Social Engineering Toolkit (SET)。
2. 利用SET工具创建一个钓鱼网页,模拟发送一个伪造的登录页面给目标用户。
3. 将钓鱼网页部署至一个虚拟的Web服务器上。
4. 向目标用户发送包含钓鱼网页链接的欺骗邮件。
5. 目标用户点击链接,进入钓鱼网页并输入账号密码。
6. 分析并记录目标用户的输入数据。
7. 分析并评估钓鱼攻击的成功率。
3.1.2 实验结果分析通过上述实验步骤,成功模拟了一次钓鱼攻击过程。
目标用户在受到欺骗邮件后,点击了链接并输入了账号密码。
我们成功获取到了目标用户的输入数据,并在实验结束后将其销毁,以免造成进一步的安全威胁。
钓鱼攻击是一种常见的社交工程攻击手段,通过伪造可信的网页来诱骗用户输入敏感信息,并达到窃取账号密码等目的。
钓鱼攻击的成功率很大程度上取决于欺骗邮件和钓鱼网页的真实性,因此在实际应用中需要遵守法律法规,并在合法的授权范围内进行实验。
3.2 无线网络攻击实验3.2.1 实验步骤1. 使用Kali Linux将无线网卡设置为监听模式。
2. 扫描周围的无线网络,并获取相关信息。
3. 对目标无线网络进行入侵测试,尝试破解其密码。
4. 分析并评估无线网络的安全性。
3.2.2 实验结果分析通过上述实验步骤,成功获取了周围无线网络的信息,并尝试破解其中一个网络的密码。
在实验过程中,我们使用了常见的密码破解工具,并分析了目标网络的安全性。
中南大学数据结构实验报告
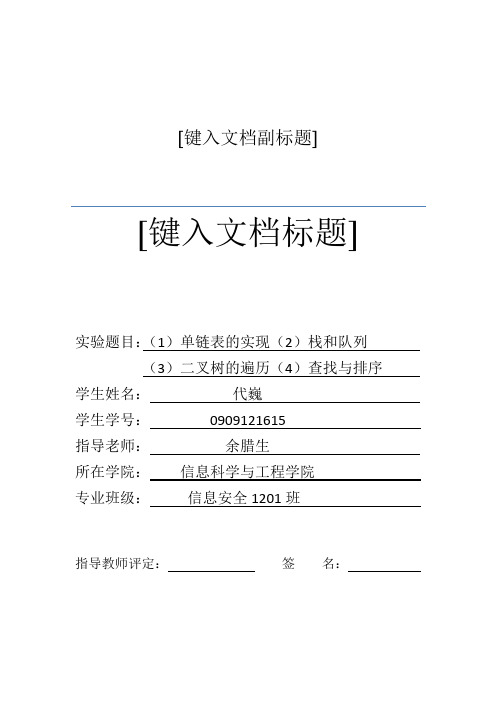
[键入文档副标题][键入文档标题]实验题目:(1)单链表的实现(2)栈和队列(3)二叉树的遍历(4)查找与排序学生姓名:代巍学生学号:0909121615指导老师:余腊生所在学院:信息科学与工程学院专业班级:信息安全1201班指导教师评定:签名:实验一单链表的实现一、实验目的了解线性表的逻辑结构和各种存储表示方法,以及定义在逻辑结构上的各种基本运算及其在某种存储结构上如何实现这些基本运算。
在熟悉上述内容的基础上,能够针对具体应用问题的要求和性质,选择合适的存储结构设计出相应的有效算法,解决与线性表相关的实际问题二、实验内容用C/C++语言编写程序,完成以下功能:(1)运行时输入数据,创建一个单链表(2)可在单链表的任意位置插入新结点(3)可删除单链表的任意一个结点(4)在单链表中查找结点(5)输出单链表三、程序设计的基本思想,原理和算法描述:(包括程序的结构,数据结构,输入/输出设计,符号名说明等)用一组地址任意的存储单元存放线性表中的数据元素。
以元素(数据元素的映象) + 指针(指示后继元素存储位置) = 结点(表示数据元素或数据元素的映象)以“结点的序列”表示线性表称作线性链表(单链表)单链表是指数据接点是单向排列的。
一个单链表结点,其结构类型分为两部分:(1)、数据域:用来存储本身数据。
(2)、链域或称为指针域:用来存储下一个结点地址或者说指向其直接后继的指针。
1、单链表的查找对单链表进行查找的思路为:对单链表的结点依次扫描,检测其数据域是否是我们所要查好的值,若是返回该结点的指针,否则返回NULL。
2、单链表的插入因为在单链表的链域中包含了后继结点的存储地址,所以当我们实现的时候,只要知道该单链表的头指针,即可依次对每个结点的数据域进行检测。
假设在一个单链表中存在2个连续结点p、q(其中p为q的直接前驱),若我们需要在p、q之间插入一个新结点s,那么我们必须先为s分配空间并赋值,然后使p的链域存储s的地址,s的链域存储q的地址即可。
信息安全学实验一免费范文精选

《信息安全学》实验云南大学软件学院实验报告课程:信息安全学实验任课教师:专业:学号:姓名:成绩:实验1 古典密码算法一、实验目的通过编程实现替代密码算法和置换密码算法,加深对古典密码体系的了解,为以后深入学习密码学奠定基础。
二、实验原理古典密码算法曾被广泛应用,大都比较简单。
它的主要应用对象是文字信息,利用密码算法实现文字信息的加密和解密。
其中替代密码和置换密码是具有代表性的两种古典密码算法。
三、实验环境PC机,C或C++编译环境四、实验内容和步骤1、根据实验原理部分对替代密码算法的介绍,同时查找和学习相关知识,自己创建明文信息,并选择一个密钥,编写替代密码算法的实现程序,实现加密和解密操作。
对于替换密码,在本实验分别实现了移位密码和维吉尼亚密码,其中维吉尼亚算法中能够对文本文件进行加密和解密操作。
《信息安全学》实验移位密码程序流程图:《信息安全学》实验算法设计:实现加密的过程中,先输入明文m,明文以字符串的形式储存在数组中,再输入密钥k,密钥范围为1-25,加密函数encrypt对明文m中的字符进行逐个加密E(m)=(m+k)mod26。
解密时先输入需要解密的密文c,密文以字符串的形式储存在数组中,再输入相对应的密钥k,解密函数decrypt对密文c中的字符进行逐个解密D(c)=(c-k)mod26。
该算法中时间复杂度为O(n)。
测试结果截图:《信息安全学》实验维吉尼亚密码程序流程图:《信息安全学》实验m,明文以字符串的形式储存在数组中,再输入密钥k,维吉尼亚密码在移位密码的基础上增大了密钥空间,密钥以字符串的形式储存于数组中,加密函数encrypt按照密钥的字符循环对明文m中的字符进行逐个加密。
解密时先输入需要解密的密文c,密文以字符串的形式储存在数组中,再输入相对应的密钥k并储存在数组中,解密函数decrypt对密文c中的字符进行逐个解密。
该算法中时间复杂度为O(n)。
测试结果截图:对字符串加密:对文本文件加密:。
信息安全实验报告一课案
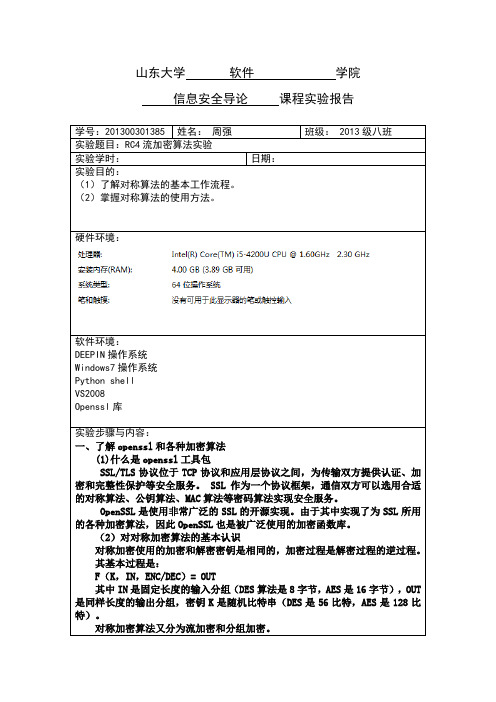
self.public_key = public_key or 'none_public_key'
key = hashlib.md5(self.public_key).hexdigest()
self.keya = hashlib.md5(key[0:16]).hexdigest()
计算文件的MD5值:>openssl md5 < 1.key
计算文件的SHA1值:>openssl sha1 < 1.key
3、使用openssl进行AES 256位密钥cbc模式对称加密
使用OpenSSL对文件进行加密,其实就跟对消息进行加密一样简单。我们使用-in选项,后面跟以我们想进行加密的实际文件,并使用-out选项,这会指令OpenSSL将经过加密的文件存储到某个名称的文件中:
山东大学软件学院
信息安全导论课程实验报告
学号:201300301385
姓名:周强
班级:2013级八班
实
(1)了解对称算法的基本工作流程。
(2)掌握对称算法的使用方法。
硬件环境:
软件环境:
DEEPIN操作系统
Windows7操作系统
Python shell
$ openssl enc -aes-256-cbc -in××××××-out×××××××
1、加密前需要输入口令密钥
2、加密前文件
3、加密后文件
4、解密
5、解密后文件
四、Python实现RC4加密
import sys,os,hashlib,time,base64
class rc4:
def __init__(self,public_key = None,ckey_lenth = 16):
信息安全概论实验一(修改)

实验一网络信息检测实验(使用Sniffer工具嗅探)一、实验目的通过使用Sniffer Pro软件掌握sniffer(嗅探器)工具的使用方法,实现捕捉FTP、HTTP 等协议的数据包,以理解TCP/IP协议中多种协议的数据结构、会话连接建立和终止的过程、TCP序列号、应答序号的变化规律。
并且,通过实验了解FTP、HTTP等协议明文传输的特性,以建立安全意识,防止FTP、HTTP等协议由于传输明文密码造成的泄密。
二、实验原理Sniffer即网络嗅探器,用于监听网络中的数据包,分析网络性能和故障。
Sniffer主要用于网络管理和网络维护,系统管理员通过Sniffer可以诊断出通过常规工具难以解决的网络疑难问题,包括计算机之间的异常通讯、不同网络协议的通讯流量、每个数据包的源地址和目的地址等,它将提供非常详细的信息。
通常每个网络接口都有一个互不相同的硬件地址(MAC),同时,每个网段有一个在此网段中广播数据包的广播地址(代表所有的接口地址)。
一般情况下,一个网络接口只响应目的地址是自己硬件地址或者自己所处网段的广播地址的数据帧,并由操作系统进一步进行处理,而丢弃不是发给自己的数据帧。
而通过Sniffer工具,可以将网络接口设置为“混杂”(promiscuous)模式。
在这种模式下,网络接口就处于一个对网络进行“监听”的状态,而它可以监听此网络中传输的所有数据帧,而不管数据帧的目标地址是广播地址还是自己或者其他网络接口的地址了。
它将对遭遇的每一个数据帧产生硬件中断,交由操作系统对这个帧进行处理,比如截获这个数据帧,进而实现实时分析数据帧中包含的内容。
当然,如果一个数据帧没有发送到目标主机的网络接口,则目标主机将无法监听到该帧。
所以Sniffer所能监听到的信息将仅限于在同一个物理网络内传送的数据帧,就是说和监听的目标中间不能有路由(交换)或其他屏蔽广播包的设备。
因此,当Sniffer工作在由集线器(HUB)构建的广播型局域网时,它可以监听到此物理网络内所有传送的数据;而对于由交换机(switch)和路由器(router)构建的网络中,由于这些网络设备只根据目标地址分发数据帧,所以在这种网络中,sniffer工具就只能监测到目标地址是自己的数据帧再加上针对广播地址的数据帧了。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
实验二栈的应用-括号匹配一、实验目的1、掌握STL中栈的基本使用2、练习使用栈进行程序编写二、实验内容题目一:STL下面代码使用STL中的栈进行序列的反转,请修改好下面代码中的语法错误,编译运行#include <stack>int main( )/* Pre: The user supplies an integer n and n decimal numbers.Post: The numbers are printed in reverse order.Uses: The STL class stack and its methods */{int n;double item;stack<double> numbers; // declares and initializes a stack of numberscout << " Type in an integer n followed by n decimal numbers."<< endl<< " The numbers will be printed in reverse order."<< endl;cin >> n;for (int i = 0; i < n; iCC) {cin >> item;numbers.push(item);}cout << endl << endl;while (!numbers.empty( )) {cout << numbers.top( ) << " ";numbers.pop( );}cout << endl;}提示:(1)由于程序是用了STL(标准模板库,可以简单的看成是一个函数库,在其中有各种有用的类、函数和算法),栈在其中有实现。
栈在STL中的实现用到了类模板,也就是说其栈是独立于类型的,模板提供参数化类型,也就是能将类型名作为参数传递给接收方来建立类或函数。
比如stack<double> numbers;中就是声明了一个栈,这个栈中存放的数据类型为double。
(2)要使用c++的输入输出需要加上几行语句如下,因为cout和cin是在命名空间std中的:#include <iostream>using namespace std;题目二:括号匹配使用STL中的栈编写程序,实现算术表达式中的括号匹配(有能力的同学可以同时进行小括号,大括号,中括号的匹配)注意:(1)配对的算术表达式中左右括号的数目应相等例如:表达式(a+b))和a+b)中的括号不配对(2)对的算术表达式中每一个左括号都一定有一个右括号与之匹配,并且一定是先出现左括号,才能有右括号例如:表达式)a+b(+c和(b+a))+(c+d也属于不配对的情况算法提示:方式是先创建一个存放左括号的空栈,并将“#”作为栈底元素。
顺序扫描表达式,当遇到非括号时,继续扫描,遇到“(”时,进栈,而遇到“)”时,与栈顶元素比较,如果括号匹配,栈顶元素退栈,继续扫描表达式,否则,报错退出。
括号配对检查的原则是:对表达式从左向右扫描。
当遇到左括号时,左括号入栈;而遇到右括号时,首先将栈中的栈顶元素弹出栈,再比较弹出元素是否与右括号匹配,如果两者匹配,则操作继续;否则,检查出错,打印“no”,并停止操作。
当表达式全部扫描完后,如果栈顶元素为“#”,说明括号作用层次嵌套正确,打印“yes”,并停止操作。
附录:STL中栈的使用#pragma warning(disable:4786)#include <stack>#include <iostream>using namespace std ;typedef stack<int> STACK_INT;int main(){STACK_INT stack1;cout << "stack1.empty() returned " <<(stack1.empty()? "true": "false") << endl; // Function 3cout << "stack1.push(2)" << endl;stack1.push(2);if (!stack1.empty()) // Function 3 cout << "stack1.top() returned " <<stack1.top() << endl; // Function 1cout << "stack1.push(5)" << endl;stack1.push(5);if (!stack1.empty()) // Function 3 cout << "stack1.top() returned " <<stack1.top() << endl; // Function 1cout << "stack1.push(11)" << endl;stack1.push(11);if (!stack1.empty()) // Function 3 cout << "stack1.top() returned " <<stack1.top() << endl; // Function 1// Modify the top item. Set it to 6.if (!stack1.empty()) { // Function 3 cout << "stack1.top()=6;" << endl;stack1.top()=6; // Function 1 }// Repeat until stack is emptywhile (!stack1.empty()) { // Function 3 const int& t=stack1.top(); // Function 2cout << "stack1.top() returned " << t << endl;cout << "stack1.pop()" << endl;stack1.pop();}}运行结果:stack1.empty() returned truestack1.push(2)stack1.top() returned 2stack1.push(5)stack1.top() returned 5stack1.push(11)stack1.top() returned 11stack1.top()=6;stack1.top() returned 6stack1.pop()stack1.top() returned 5 stack1.pop()stack1.top() returned 2 stack1.pop()实验三按层次构造二叉树及二叉树遍历一、实验目的1、设计数据结构和算法,实现按层次构造二叉树的算法2、掌握树的前根序、中根序和后根序遍历算法二、实验内容1、实验题目按层次(从上到下,从左到右的顺序)输入树的结点,如果该结点为空,则用一个特定的值替代(比如0或者.)。
例如下面的图中,输入为e b f a d . g . . c(当然为了方便输入,也可以用#结束字符串的输入)要求构造一棵如下的二叉树,当二叉树构造成功后,需要对其进行先序遍历,后序遍历,中序遍历。
2、按层次构造树的两种方法对于如下的一棵树输入:为了构造上图所示的这样一棵二叉树,键盘上输入的顺序如下:ebfad.g..c#其中可以看到这是根据层次的一种输入,先输入第一层的结点,然后是第二层的结点,第三层….直到把所有的带信息的结点输入完。
也可以看到,在输入的序列中,有.号,这是代表结点为空。
注意在输入的时候,为空的结点也要这样输入进去。
构造二叉树:方法一:用队列queue<Binary_node*> A;queue<Binary_node*> B;假如我们把读入的数据放到一个队列中A,用于方便我们的后续处理,那么,在读入后,这个队列中的数据为ebfad.g..c,其中e为队列头存放的元素值(即该指针所指向的节点空间数据域中的值为e),而点代表空指针NULL把数据读入队列A中的方法如下:Binary_node* tempNode=new Binary_node();cin>>tempNode->data;tempNode->left=NULL;tempNode->right=NULL;A.push(tempNode);为了按层次的构造二叉树,我们还要使用一个队列B,这个队列中保存的是指向要有儿子结点的父亲结点的指针。
下面是这两个队列的变化和树的构造变化情况:(1)第一步很特殊,首先是树根Binary_node* pNode=A.front();A.pop();B.push(pNode);A:bfad.g..cB:e树:(2)后面的每一步都是从A中取出两个队首,放入B队列尾部(如果为NULL则不放)。
从B中取出队首,队列A中取出的元素正好是B中取出元素的小孩子Binary_node* pfather= B.front();B.pop();Binary_node* pLchild= A.front();//先出来的是左孩子A.pop();Binary_node* pRchild= A.front();A.pop();pfather->left=pLchild; pfather->right=pRchild; //先放入左孩子if(pLchild!=NULL) {B.push(pLchild);}if(pRchild!=NULL) {B.push(pLchild);}A:ad.g..cB:bf树:(3)A:.g..cB:fad树:(4)A:..cB:adg树:(5)A:cB:dg树:(6)A:空(当队列A为空的时候整个算法结束,树构造成功)B:g树:第二种方法:给树的结点按层次从上到下,从左到右编号(编号从1开始,空节点也要编号),利用父亲跟小孩的编号的关系来编写代码。