C语言函数大全-l开头-完整版
C语言常用函数
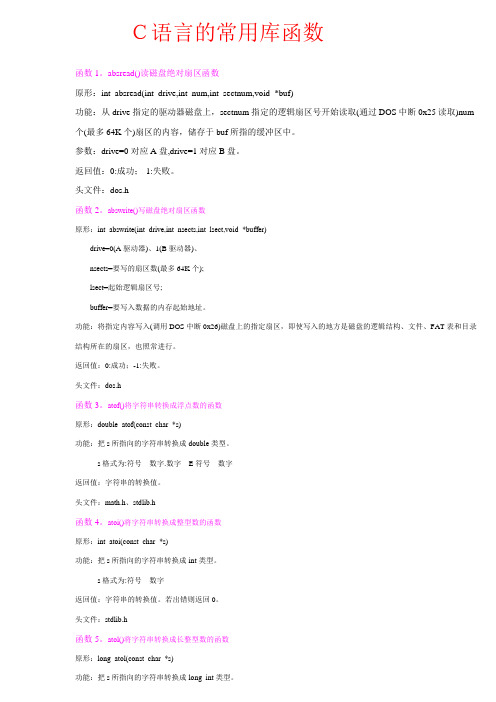
C语言的常用库函数函数1。
absread()读磁盘绝对扇区函数原形:int absread(int drive,int num,int sectnum,void *buf)功能:从drive指定的驱动器磁盘上,sectnum指定的逻辑扇区号开始读取(通过DOS中断0x25读取)num 个(最多64K个)扇区的内容,储存于buf所指的缓冲区中。
参数:drive=0对应A盘,drive=1对应B盘。
返回值:0:成功;-1:失败。
头文件:dos.h函数2。
abswrite()写磁盘绝对扇区函数原形:int abswrite(int drive,int nsects,int lsect,void *buffer)drive=0(A驱动器)、1(B驱动器)、nsects=要写的扇区数(最多64K个);lsect=起始逻辑扇区号;buffer=要写入数据的内存起始地址。
功能:将指定内容写入(调用DOS中断0x26)磁盘上的指定扇区,即使写入的地方是磁盘的逻辑结构、文件、FAT表和目录结构所在的扇区,也照常进行。
返回值:0:成功;-1:失败。
头文件:dos.h函数3。
atof()将字符串转换成浮点数的函数原形:double atof(const char *s)功能:把s所指向的字符串转换成double类型。
s格式为:符号数字.数字E符号数字返回值:字符串的转换值。
头文件:math.h、stdlib.h函数4。
atoi()将字符串转换成整型数的函数原形:int atoi(const char *s)功能:把s所指向的字符串转换成int类型。
s格式为:符号数字返回值:字符串的转换值。
若出错则返回0。
头文件:stdlib.h函数5。
atol()将字符串转换成长整型数的函数原形:long atol(const char *s)功能:把s所指向的字符串转换成long int类型。
s格式为:符号数字返回值:字符串的转换值。
C语言函数大全

C语言函数大全C语言作为一种广泛应用的计算机编程语言,其函数是程序设计中不可或缺的部分。
C语言函数大全涵盖了C语言中常用的各种函数,包括数学函数、字符串函数、输入输出函数等,本文将对这些函数进行详细介绍。
一、数学函数。
1. abs函数。
abs函数用于返回一个整数的绝对值,其原型为int abs(int x)。
2. pow函数。
pow函数用于计算一个数的幂,其原型为double pow(double x, double y)。
3. sqrt函数。
sqrt函数用于计算一个数的平方根,其原型为double sqrt(double x)。
4. sin函数。
sin函数用于计算一个角度的正弦值,其原型为double sin(double x)。
5. cos函数。
cos函数用于计算一个角度的余弦值,其原型为double cos(double x)。
6. tan函数。
tan函数用于计算一个角度的正切值,其原型为double tan(double x)。
二、字符串函数。
1. strlen函数。
strlen函数用于返回一个字符串的长度,其原型为size_t strlen(const char s)。
2. strcpy函数。
strcpy函数用于将一个字符串复制到另一个字符串中,其原型为charstrcpy(char dest, const char src)。
3. strcat函数。
strcat函数用于将一个字符串追加到另一个字符串的末尾,其原型为char strcat(char dest, const char src)。
4. strcmp函数。
strcmp函数用于比较两个字符串,其原型为int strcmp(const char s1, const char s2)。
5. strchr函数。
strchr函数用于在一个字符串中查找指定字符的位置,其原型为charstrchr(const char s, int c)。
c#函数大全

1到9的平方和static void Main(string[] args){int h=0;for(int i=1;i<=9;i++){h=h+i*i;}Console.WriteLine (h);}1到100的累加static void Main(string[] args){ int sum = 0;for (int i = 1;i<=100;i++)sum = sum +i;Console.WriteLine("1到的累加总合为:{0}",sum);}}}10个行输出被7整除的数static void Main(string[] args){for(int i=100;i<=200;i++)for(int j=1;j<=10;j++) {if(i%7==0){ Console.Write ("{0}",i);break;}}7行倒三角for(int a=1;a<=7;a++){for(int b=1;b<=a;b++)Console.Write (" ");for(int c=1;c<=15-2*a;c++) {Console.Write ("*");}Console.Write ("\n");}乘法口诀表static void Main(string[] args){ int i,j; Console.WriteLine ("成法口决表");for(i=1;i<=9;i++){ for(j=1;j<=i;j++){ Console.Write("{0}*{1}={2};",j,i,(i*j));}Console.WriteLine ();}}}10的阶乘static void Main(string[] args){ int i=1; int n=1;do{ n=n*i;i++;}while (i<=10);Console.WriteLine ("10的阶乘结果为;{0}",n);}}For 循环求i的值static void Main(string[] args){ double s=0,x=1; //初始化for (int n=1;Math.Abs (x)>1e-8;n++,x*=(-1.0)*(2*n-3)/(2*n-1))s=s+x; s=s*4;Console.WriteLine ("pi的值是:{0}",s);}While循环求pi的值static void Main(string[] args){ double result =0,initial=1;//初始值 long deno = 1;int flag =1; while (Math.Abs (initial)>1e-8) //循环条件{ result =result+initial; //循环体,计算(Math.PI/4)deno=deno+2;flag=flag*(-1);initial=flag/Convert.ToDouble (deno);} result=result*4; //Math.PI值Console.WriteLine ("pi值是:{0}",result); //输出Math.PI值}}While 循环输出10的阶乘static void Main(string[] args){ int i=1; int n=1; while(i<=10){ n=n*i;i++;}Console.WriteLine ("10的阶乘结果为:{0}",n);}}百钱买百鸡static void Main(string[] args) {for(int g=0;g<20;g++) for(int m=0;m<35;m++) for(intx=0;x<100;x++){ if((g+m+x==100)&&(5*g+3*m+1/(3*x)==100))Console.WriteLine("值为;{0}\n 值为:{1}\n 值:{2}",g,m,x); }} }水仙花static void Main(string[] args) {int x=Convert.ToInt32 (Console.ReadLine ());int y=Convert.ToInt32 (Console.ReadLine ());int z=Convert.ToInt32 (Console.ReadLine ());if(x*100+y*10+z==x*x*x+y*y*y+z*z*z) Console.WriteLine ("水仙花");else Console.WriteLine ("不是水仙花");}}找6ji42能被两个数整除static void Main(string[] args){for(int j=0;j<=9;j++){ for(int i=0;i<=9;i++) {intx=60000+j*1000+i*100+42;if(x%32==0&&x%42==0) Console.WriteLine(x);}} }}正三角形的星using System;class Music{ static void Main(string[] args) { int i,j,b;捉司机using System;namespace ConsoleApplication1{class Class1 {static void Main(string[] args){int x=0;int y=0;for(x=0;x<=9;x++)for(y=0;y<=9;y++){double m=1000*x+100*x+10*y+y;for(int i=10;i<=100;i++)if(m==i*i)Console.WriteLine (m);}}}输出100到200中所有的素数static void Main(string[] args){ for(int i=100;i<=200;i++){ for(int j=2;j<=i;j++){if(i%j==0) break;if(j==i-1)Console.Write(i+"\t"); }}}}求2到10000间的完数static void Main(string[] args){ for (int j=2;j<=10000;j++){ int s=0;for(int i=1;i<j;i++){ if(j%i==0)s=s+i;}if(s==j) Console.WriteLine (j);} }}求两个数的最大公约数static void Main(string[] args){while(true){int a=Convert.ToInt32 (Console.ReadLine ());int b=Convert.ToInt32 (Console.ReadLine ()); if(a>b){ for(int c=a;c>0;c--){if(a%c==0&&b%c==0){ Console.WriteLine (c); break;}}}}}}判断输入的数是否为素数static void Main(string[] args){ while(true){int i; int x=Convert.ToInt32 (Console.ReadLine ()); for( i=2;i<x;i++){ if(x%i==0)Console.WriteLine ("x不是素数");break;}if( i==(x-1)) Console.WriteLine ("x是素数");}}}二原方程根的情况static void Main(string[] args){ double x1,x2; int a=Convert.ToInt32(Console.ReadLine()); int b=Convert.ToInt32(Console.ReadLine());int c=Convert.ToInt32(Console.ReadLine());doubled=b*b-4*a*c;if(a==0) Console.WriteLine("不是二元方程");if(d>0){ x1=(-b+System.Math.Sqrt(d))/(2*a);x2=(-b-System.Math.Sqrt(d))/(2*a);Console.WriteLine("第一个根:{0}\t第二个根:{1}\t",x1,x2);}if(d==0){ x1=-b/(2*a); x2=-b/(2*a); Console.WriteLine("第一个根:{0}\t第二个根:{1}\t",x1,x2);}} 歌德巴赫猜想static void Main(string[] args){ int m=Convert.ToInt32 (Console.ReadLine ());for(int x=6;x<m;x+=2){ for(int y=3;y<x/2;y++) if(prime(y)){int z=x-y;if(prime(z))Console.WriteLine ("结果为:x={0},y={1},z={2}",x,y,z); }}}static bool prime (int n){int i; for(i=2;i<=System.Math.Sqrt (n);i++) if(n%i==0)return false;return true;}}递归函数算任意数的阶乘static void Main(string[] args) { Console.WriteLine ("输入任意的数");int n=Convert.ToInt32 (Console.ReadLine ());double s=f(n);Console.WriteLine (f(n)); }static double f(int n){ double d=0;if(n==0||n==1) d=1; else d=f(n-1)*n;return d;}}函数比较三个数的大小static void Main(string[] args){int result=MaxThree(2,7,4); Console.WriteLine (result); }static int MaxTwo(int x,int y){ if(x>y) return x;else return y; }static int MaxThree(int x,int y,int z){ int temp;temp=MaxTwo(x,y);if(z>temp)return z;else return temp;}}}调用编成世界using System;namespace ConsoleApplication11{class Class1{static void Main(string[] args){string name =Convert.ToString(Console.ReadLine()); welcome( name); }static void welcome(string name){ for(int i=1;i<=5;i++)Console.WriteLine("欢迎你,{0}同志,这里是C#编程世界",name);}}大小写字母转换char c=Convert.ToChar(Console.ReadLine());if(c>='a'&&c<='z'){int b=(int)c-32;char ch=(char)b;Console.WriteLine(ch);}if(c>='A'&&c<='Z'){int d=(int)c+32;char ch=(char)d;Console.WriteLine(ch);}判断瑞年int year=Convert.ToInt32(Console.ReadLine());if(year%4==0&&year%100==0)//[if(year%400==0) ]Console.WriteLine("瑞年");elseConsole.WriteLine("不是瑞年");求最小公倍数int a=Convert.ToInt32 (Console.ReadLine());int b=Convert.ToInt32 (Console.ReadLine ());int d=Convert.ToInt32 (Console.ReadLine ());if(a>b&&a>d){for(int c=a;c>0;c--){if(c%a==0&&c%b==0&&c%d==0){Console.WriteLine (c);break;}正三角形的星using System;class Music{ static void Main(string[] args){ int i,j,b; for( i=1;i<=4;i++){ for(j=0;j<=4-i;j++) Console.Write(" ");for(b=1;b<=2*i-1;b++) Console.Write("*"); Console.Write("\n"); }}namespace ConsoleApplication1{ class Program { static void Main(string[] args){实验五第一题输入月号,输出月份的英文名称do {Console.WriteLine("请输入您需要的月份:");Console.WriteLine ("下面的程序将对它进行英文翻译."); int a=Convert.ToInt16 (Console.ReadLine ());switch(a){case(1): Console.WriteLine ("Jan");break; case(2):Console.WriteLine ("Feb");break;case(3): Console.WriteLine ("Mar");break;case(4): Console.WriteLine ("Apr");break;case(5):Console.WriteLine("May");break;case(6):Console.WriteLine ("Jun");break;case(7): Console.WriteLine ("Jul");break;case(8):Console.WriteLine ("Aug");break;case(9): Console.WriteLine ("Sep");break;case(10): Console.WriteLine ("Oct");break;case(11):Console.WriteLine ("Nov");break;case(12):Console.WriteLine ("Der");break;}}while(true); Console.ReadLine ();实验五第二题托儿所问题Console.WriteLine("请输入你的年龄大小:");int a =Convert.ToInt16(Console .ReadLine ());switch (a){ case (2): Console.WriteLine("please enter lower class"); break;case(3):Console.WriteLine("please enter lower class"); break;case(4): Console .WriteLine ("please enter middle class"); break;case(5): Console.WriteLine("please enter higher class"); break;case(6): Console .WriteLine ("please enter higher class"); break;}Console .ReadLine ();实验五第三题开发一个数学工具do{Console.WriteLine("欢迎使用本数学工具,本数学工具具有以下功能");Console.WriteLine("图形面积计算按1");Console.WriteLine("算术计算按2");Console.WriteLine("三角函数计算按3");Console.WriteLine("请输入数字选择您此次计算的类型");int x = int.Parse(Console.ReadLine());double area, v;switch (x){case (1):{Console.WriteLine("请输入字母选择您此次计算的图形面积的类型");Console.WriteLine("三角形的面积按1:"); Console.WriteLine("长方形的面积按2:");Console.WriteLine("圆形的面积按3:"); Console.WriteLine("梯形的面积按4:");int y = int.Parse(Console.ReadLine());switch (y){ case (1):{Console.WriteLine("请输入三角形的边q:");Console.WriteLine("请输入三角形的边p:");Console.WriteLine("请输入三角形的边l:");double q = Convert.ToInt64(Console.ReadLine());double p = double.Parse(Console.ReadLine());double l = double.Parse(Console.ReadLine());double h;if ((q + p) > l && (q + l) > p && (l + p) > q){ h = (1 / 2) * (q + p + l);rea = Math.Sqrt(h * (h - q) * (h - p) * (h - l));Console.WriteLine("面积="+area); }else{ Console.WriteLine("输入三边错误不能够成三角形");}break; }case (2):{Console.WriteLine("请输入长方形的短边q:"); Console.WriteLine("请输入长方形的长边p:");double q = Convert.ToInt64(Console.ReadLine()); double p = double.Parse(Console.ReadLine());area = q * p;Console.WriteLine("面积:"+area); break;}case (3):{Console.WriteLine("请输入园的半径q:");double q = Convert.ToInt64(Console.ReadLine()); area = q * q * Math.PI;Console.WriteLine("面积:"+area);break;}case (4):{Console.WriteLine("请输入上底的长度q:");Console.WriteLine("请输入下底的长度p:");Console.WriteLine("请输入高的长度h:");double q = Convert.ToInt64(Console.ReadLine());double p = double.Parse(Console.ReadLine());double h = double.Parse(Console.ReadLine());area = ((q + p) * h) / 2;Console.WriteLine("面积:"+area);break;}}break;}case (2):{Console.WriteLine("要算加法请输入1:");Console.WriteLine("要算减法请输入2:");Console.WriteLine("要算乘法请输入3:");Console.WriteLine("要算除法请输入4:");int k = Convert.ToInt16(Console.ReadLine());switch (k){ case (1):{ Console.WriteLine("请输入q:"); Console.WriteLine("请输入p:");double q = Convert.ToInt64(Console.ReadLine());double p = double.Parse(Console.ReadLine());v = q + p;Console.WriteLine("两数和:"+v); break; }case (2):{Console.WriteLine("请输入较大数q:");Console.WriteLine("请输入较小数p:");double q = Convert.ToInt64(Console.ReadLine());double p = double.Parse(Console.ReadLine());v = q - p;Console.WriteLine("两数差:"+v); break;}case (3):{Console.WriteLine("请输入被除数q:");Console.WriteLine("请输入除数p:");double q = Convert.ToInt64(Console.ReadLine());double p = double.Parse(Console.ReadLine());v = q / p;Console.WriteLine("两数商:" + v);break;}case (4):{Console.WriteLine("请输入其中要乘的数一q:");Console.WriteLine("请输入另一个乘数二p:"); double q = Convert.ToDouble(Console.ReadLine());double p = double.Parse(Console.ReadLine());v = q * p;Console.WriteLine("两数积:"+v); break;} }break;}case (3):{ Console.WriteLine("要想求sin请输入1:");Console.WriteLine("要想求cos请输入2:");Console.WriteLine("要想求tan请输入3:"); int m = Convert.ToInt16(Console.ReadLine());switch (m){case (1):{Console.WriteLine("请输入您的度数:");double q= double .Parse (Console .ReadLine ());q=Math.Sin ();Console.WriteLine(m);break;}case (2):{Console.WriteLine("请输入您的度数:");double q = Convert .ToInt64 (Console.ReadLine());q = Math.Cos();Console.WriteLine(q); break; }case (3):{Console.WriteLine("请输入您的度数:");double q = Convert .ToDouble (Console.ReadLine()); q = Math.Tan();Console.WriteLine(q);break; }}break;} while(true); Console.ReadLine();}}}试验七第一题求e的值double i, n=1;double sum=0;for (i = 1; i <= 10; i++){ n = n*i;sum = sum + 1/n ; Console.WriteLine(sum); }Console.ReadLine();实验七第二题方法一m和n的最大公约数int i,temp=0;Console.WriteLine("请输入您的数a:");int a = Convert.ToInt32(Console.ReadLine()); Console.WriteLine("请输入您的数b");int b = Convert.ToInt32(Console.ReadLine());for (i = 1; i <=a && i<= b; i++){ if ((a % i == 0) && (b % i == 0)) temp = i;} Console .WriteLine (temp); //Console.ReadLine();/ 实验七第三题人口增长率Console.WriteLine("该程序有三种%数,分别是%5,%2,%1。
C语言常用的库函数表
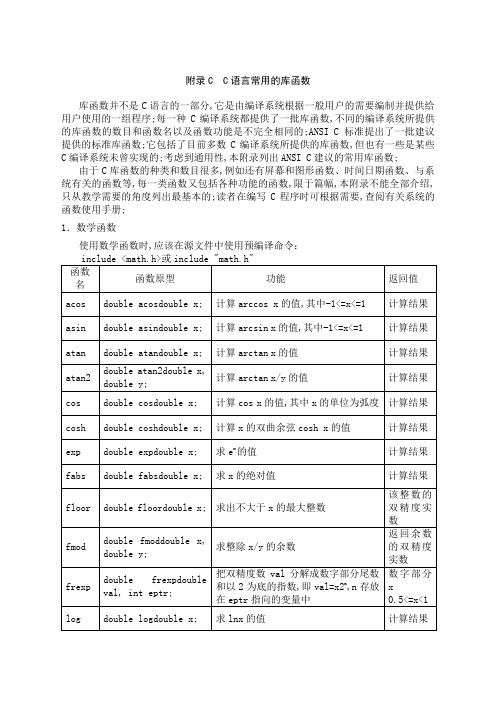
附录C C语言常用的库函数
库函数并不是C语言的一部分,它是由编译系统根据一般用户的需要编制并提供给用户使用的一组程序;每一种C编译系统都提供了一批库函数,不同的编译系统所提供的库函数的数目和函数名以及函数功能是不完全相同的;ANSI C标准提出了一批建议提供的标准库函数;它包括了目前多数C编译系统所提供的库函数,但也有一些是某些C编译系统未曾实现的;考虑到通用性,本附录列出ANSI C建议的常用库函数;
由于C库函数的种类和数目很多,例如还有屏幕和图形函数、时间日期函数、与系统有关的函数等,每一类函数又包括各种功能的函数,限于篇幅,本附录不能全部介绍,只从教学需要的角度列出最基本的;读者在编写C程序时可根据需要,查阅有关系统的函数使用手册;
1.数学函数
使用数学函数时,应该在源文件中使用预编译命令:
2.字符函数
在使用字符函数时,应该在源文件中使用预编译命令:
3.字符串函数
使用字符串中函数时,应该在源文件中使用预编译命令:
4.输入输出函数
在使用输入输出函数时,应该在源文件中使用预编译命令:
5.动态存储分配函数
在使用动态存储分配函数时,应该在源文件中使用预编译命令:include <stdlib.h>或include "stdlib.h"
6.其他函数
有些函数由于不便归入某一类,所以单独列出;使用这些函数时,应该在源文件中使用预编译命令:。
C语言常用数学函数
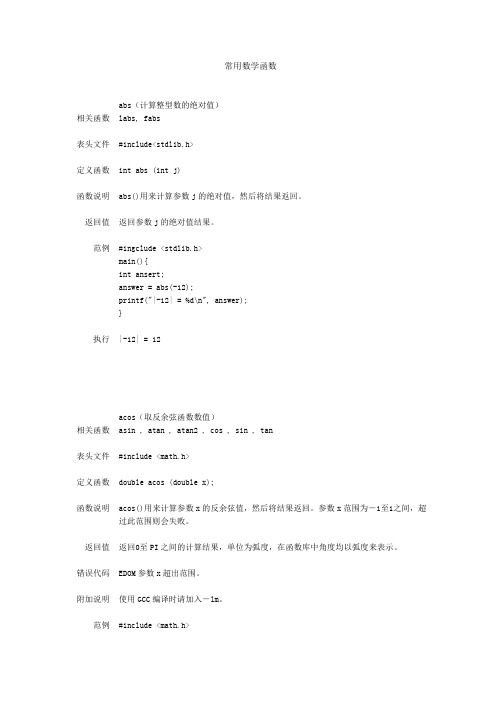
常用数学函数abs(计算整型数的绝对值)相关函数labs, fabs表头文件#include<stdlib.h>定义函数int abs (int j)函数说明abs()用来计算参数j的绝对值,然后将结果返回。
返回值返回参数j的绝对值结果。
范例#ingclude <stdlib.h>main(){int ansert;answer = abs(-12);printf("|-12| = %d\n", answer);}执行|-12| = 12acos(取反余弦函数数值)相关函数asin , atan , atan2 , cos , sin , tan表头文件#include <math.h>定义函数double acos (double x);函数说明acos()用来计算参数x的反余弦值,然后将结果返回。
参数x范围为-1至1之间,超过此范围则会失败。
返回值返回0至PI之间的计算结果,单位为弧度,在函数库中角度均以弧度来表示。
错误代码EDOM参数x超出范围。
附加说明使用GCC编译时请加入-lm。
范例#include <math.h>main (){double angle;angle = acos(0.5);printf("angle = %f\n", angle);}执行angle = 1.047198asin(取反正弦函数值)相关函数acos , atan , atan2 , cos , sin , tan表头文件#include <math.h>定义函数double asin (double x)函数说明asin()用来计算参数x的反正弦值,然后将结果返回。
参数x范围为-1至1之间,超过此范围则会失败。
返回值返回-PI/2之PI/2之间的计算结果。
错误代码EDOM参数x超出范围附加说明使用GCC编译时请加入-lm范例#include<math.h>main(){double angle;angle = asin (0.5);printf("angle = %f\n",angle);}执行angle = 0.523599atan(取反正切函数值)相关函数acos,asin,atan2,cos,sin,tan表头文件#include<math.h>定义函数double atan(double x);函数说明atan()用来计算参数x的反正切值,然后将结果返回。
C语言~所有的字符串操作函数

7.函数名: strcspn 功 能: 在串中查找第一个给定字符集内容的段 用 法: int strcspn(char *str1, char *str2); 程序例: #i nclude <stdio.h> #i nclude <string.h> #i nclude <alloc.h> int main(void) { char *string1 = "1234567890"; char *string2 = "747DC8"; int length;
printf("buffer 2 is greater than buffer 1\n"); if (ptr < 0)
printf("buffer 2 is less than buffer 1\n"); if (ptr == 0)
printf("buffer 2 equals buffer 1\n"); return 0; }
printf("buffer 2 is less than buffer 1\n"); if (ptr == 0)
printf("buffer 2 equals buffer 1\n"); return 0; }
14.函数名: strncpy 功 能: 串拷贝 用 法: char *strncpy(char *destin, char *source, int maxlen); 程序例: #i nclude <stdio.h> #i nclude <string.h> int main(void) {
i--的用法c语言

i--的用法c语言i--是C语言中的一个操作符,被称为递减操作符。
它用于将一个变量的值减1、在C语言中,它可以用作前缀和后缀操作符,有不同的使用方式和语义。
1.前缀i--:先减后使用语法:--i使用前,先将i的值减1,然后再使用减少后的值。
例如:```cint i = 5;printf("%d\n", --i); //输出:4printf("%d\n", i); //输出:4```在这个例子中,i的值先被减1变成4,然后输出4、再次打印i的值还是42.后缀i--:先使用后减语法:i--使用i的当前值,然后再将i的值减1、例如:```cint i = 5;printf("%d\n", i--); //输出:5printf("%d\n", i); //输出:4```在这个例子中,打印出来的结果是5,然后才将i的值减1变成4、再次打印i的值就是4i--可以用于内存分配和循环控制等多种情况中,下面是一些常见的用法:1.内存分配```cint size = 10;int *ptr = (int*)malloc(size * sizeof(int));for (int i = size-1; i >= 0; i--)ptr[i] = 0;}```这个例子中,通过递减操作符可以逆序初始化一段内存空间。
2.数组遍历```cint arr[] = {1, 2, 3, 4, 5};int length = sizeof(arr) / sizeof(arr[0]);for (int i = length-1; i >= 0; i--)printf("%d ", arr[i]);}```这个例子中,i--被用于逆序遍历数组的索引,将数组元素从后往前依次打印出来。
3.循环控制```cfor (int i = 10; i > 0; i--)printf("%d\n", i);}```这个例子中,通过递减操作符将循环变量i的值从10递减到1,实现了倒计时的效果。
c语言常用函数大全及详解
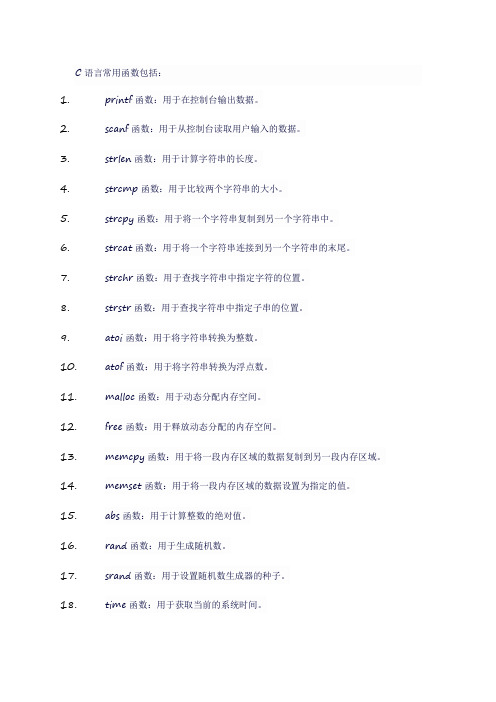
C语言常用函数包括:1.printf函数:用于在控制台输出数据。
2.scanf函数:用于从控制台读取用户输入的数据。
3.strlen函数:用于计算字符串的长度。
4.strcmp函数:用于比较两个字符串的大小。
5.strcpy函数:用于将一个字符串复制到另一个字符串中。
6.strcat函数:用于将一个字符串连接到另一个字符串的末尾。
7.strchr函数:用于查找字符串中指定字符的位置。
8.strstr函数:用于查找字符串中指定子串的位置。
9.atoi函数:用于将字符串转换为整数。
10.atof函数:用于将字符串转换为浮点数。
11.malloc函数:用于动态分配内存空间。
12.free函数:用于释放动态分配的内存空间。
13.memcpy函数:用于将一段内存区域的数据复制到另一段内存区域。
14.memset函数:用于将一段内存区域的数据设置为指定的值。
15.abs函数:用于计算整数的绝对值。
16.rand函数:用于生成随机数。
17.srand函数:用于设置随机数生成器的种子。
18.time函数:用于获取当前的系统时间。
19.localtime函数:用于将时间戳转换为本地时间。
20.strtol函数:用于将字符串转换为长整型数。
21.strtod函数:用于将字符串转换为双精度浮点数。
22.fprintf函数:用于将数据格式化输出到文件中。
23.fscanf函数:用于从文件中读取格式化的数据。
24.fgets函数:用于从文件中读取一行数据。
25.fputs函数:用于将数据写入文件中。
26.fopen函数:用于打开文件。
27.fclose函数:用于关闭文件。
28.feof函数:用于判断文件是否已经到达文件末尾。
29.ferror函数:用于判断文件操作是否发生错误。
30.fprintf函数:用于将数据格式化输出到文件中。
c语言常用函数大全及详解

c语言常用函数大全及详解C语言是一种通用的、面向过程的编程语言,被广泛应用于系统软件、嵌入式开发以及科学计算领域。
在C语言中,函数是一种模块化编程的基本方法,通过函数可以将一段代码进行封装和复用,提高了代码的可读性和可维护性。
本文将介绍一些C语言中常用的函数,并详细解释其用法及重要参数。
一、数学函数1. abs()函数函数原型:int abs(int x);函数功能:返回x的绝对值。
参数说明:x为一个整数。
2. pow()函数函数原型:double pow(double x, double y);函数功能:计算x的y次方。
参数说明:x和y为两个double类型的实数。
3. sqrt()函数函数原型:double sqrt(double x);函数功能:计算x的平方根。
参数说明:x为一个double类型的实数。
二、字符串函数1. strcpy()函数函数原型:char* strcpy(char* destination, const char* source);函数功能:将source字符串复制到destination字符串。
参数说明:destination为目标字符串,source为源字符串。
2. strlen()函数函数原型:size_t strlen(const char* str);函数功能:计算str字符串的长度。
参数说明:str为一个以'\0'结尾的字符串。
3. strcat()函数函数原型:char* strcat(char* destination, const char* source);函数功能:将source字符串拼接到destination字符串的末尾。
参数说明:destination为目标字符串,source为源字符串。
三、文件操作函数1. fopen()函数函数原型:FILE* fopen(const char* filename, const char* mode);函数功能:打开一个文件,并返回文件指针。
C语言中的数学函数

C语言中的数学函数数学函数在计算机编程中起着重要的作用,尤其是在C语言中。
C语言提供了丰富的数学函数库,方便开发者进行各种数学计算和操作。
本文将介绍C语言中常用的数学函数,包括数值运算、三角函数、指数函数等。
一、数值运算函数1.1 绝对值函数(fabs)绝对值函数fabs(x)返回x的绝对值。
例如,fabs(-5)的返回值是5。
1.2 向上取整函数(ceil)向上取整函数ceil(x)返回大于或等于x的最小整数。
例如,ceil(4.2)的返回值是5。
1.3 向下取整函数(floor)向下取整函数floor(x)返回小于或等于x的最大整数。
例如,floor(4.8)的返回值是4。
1.4 平方根函数(sqrt)平方根函数sqrt(x)返回x的平方根。
例如,sqrt(16)的返回值是4。
1.5 幂运算函数(pow)幂运算函数pow(x, y)返回x的y次方。
例如,pow(2, 3)的返回值是8。
二、三角函数2.1 正弦函数(sin)正弦函数sin(x)返回以弧度为单位的角x的正弦值。
例如,sin(0)的返回值是0。
2.2 余弦函数(cos)余弦函数cos(x)返回以弧度为单位的角x的余弦值。
例如,cos(3.14)的返回值是-1。
2.3 正切函数(tan)正切函数tan(x)返回以弧度为单位的角x的正切值。
例如,tan(0.8)的返回值是0.999.2.4 反正弦函数(asin)反正弦函数asin(x)返回x的反正弦值,以弧度为单位。
例如,asin(1)的返回值是1.57.2.5 反余弦函数(acos)反余弦函数acos(x)返回x的反余弦值,以弧度为单位。
例如,acos(0)的返回值是1.57.三、指数函数3.1 自然指数函数(exp)自然指数函数exp(x)返回e的x次方。
其中e的值约为2.71828。
例如,exp(1)的返回值是2.71828.3.2 对数函数(log)对数函数log(x)返回以e为底,x的对数值。
(完整版)C语言函数大全
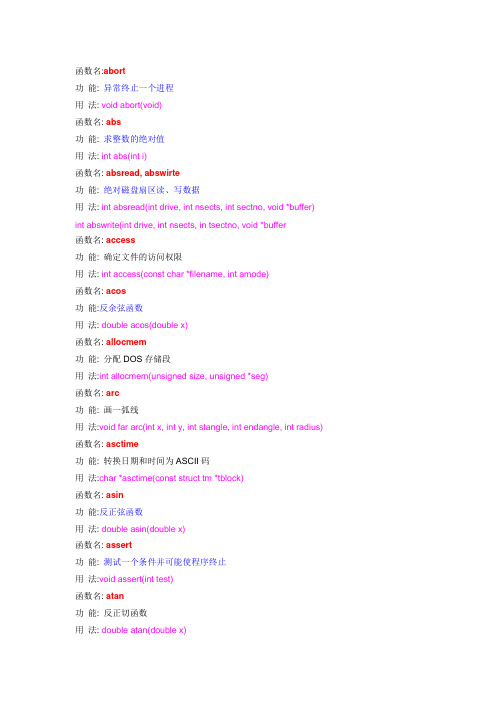
功能: 异常终止一个进程用法: void abort(void)函数名: abs功能: 求整数的绝对值用法: int abs(int i)函数名: absread, abswirte功能: 绝对磁盘扇区读、写数据用法: int absread(int drive, int nsects, int sectno, void *buffer) int abswrite(int drive, int nsects, in tsectno, void *buffer函数名: access功能: 确定文件的访问权限用法: int access(const char *filename, int amode)函数名: acos功能:反余弦函数用法: double acos(double x)函数名: allocmem功能: 分配DOS存储段用法:int allocmem(unsigned size, unsigned *seg)函数名: arc功能: 画一弧线用法:void far arc(int x, int y, int stangle, int endangle, int radius)函数名: asctime功能: 转换日期和时间为ASCII码用法:char *asctime(const struct tm *tblock)函数名: asin功能:反正弦函数用法: double asin(double x)函数名: assert功能: 测试一个条件并可能使程序终止用法:void assert(int test)函数名: atan功能: 反正切函数用法: double atan(double x)功能: 计算Y/X的反正切值用法: double atan2(double y, double x)函数名:atexit功能: 注册终止函数用法: int atexit(atexit_t func)函数名: atof功能: 把字符串转换成浮点数用法:double atof(const char *nptr)函数名: atoi功能: 把字符串转换成长整型数用法: int atoi(const char *nptr)函数名: atol功能: 把字符串转换成长整型数用法: long atol(const char *nptr)函数名: bar功能: 画一个二维条形图用法: void far bar(int left, int top, int right, int bottom)函数名: bar3d功能: 画一个三维条形图用法:void far bar3d(int left, int top, int right, int bottom,int depth, int topflag)函数名: bdos功能: DOS系统调用用法: int bdos(int dosfun, unsigned dosdx, unsigned dosal)函数名:bdosptr功能:DOS系统调用用法: int bdosptr(int dosfun, void *argument, unsigned dosal)函数名:bioscom功能: 串行I/O通信用法:int bioscom(int cmd, char abyte, int port)函数名:biosdisk功能: 软硬盘I/O用法:int biosdisk(int cmd, int drive, int head, int track, int sectorint nsects, void *buffer)函数名:biosequip功能: 检查设备用法:int biosequip(void)函数名:bioskey功能: 直接使用BIOS服务的键盘接口用法:int bioskey(int cmd)函数名:biosmemory功能: 返回存储块大小用法:int biosmemory(void)函数名:biosprint功能: 直接使用BIOS服务的打印机I/O用法:int biosprint(int cmd, int byte, int port)函数名:biostime功能: 读取或设置BIOS时间用法: long biostime(int cmd, long newtime)函数名: brk功能: 改变数据段空间分配用法:int brk(void *endds)函数名:bsearch功能: 二分法搜索用法:void *bsearch(const void *key, const void *base, size_t *nelem, size_t width, int(*fcmp)(const void *, const *))函数名: cabs功能: 计算复数的绝对值用法: double cabs(struct complex z);函数名:calloc功能:分配主存储器用法:void *calloc(size_t nelem, size_t elsize);函数名: ceil功能: 向上舍入用法: double ceil(double x);函数名: cgets功能: 从控制台读字符串用法: char *cgets(char *str)函数名:chdir功能: 改变工作目录用法: int chdir(const char *path);函数名:_chmod, chmod功能: 改变文件的访问方式用法: int chmod(const char *filename, int permiss);函数名:chsize功能: 改变文件大小用法: int chsize(int handle, long size);函数名: circle功能: 在给定半径以(x, y)为圆心画圆用法: void far circle(int x, int y, int radius);函数名: cleardevice功能: 清除图形屏幕用法: void far cleardevice(void);函数名:clearerr功能: 复位错误标志用法:void clearerr(FILE *stream);函数名: clearviewport功能: 清除图形视区用法: void far clearviewport(void);函数名:_close, close功能: 关闭文件句柄用法:int close(int handle);函数名: clock功能:确定处理器时间用法: clock_t clock(void);函数名:closegraph功能: 关闭图形系统用法: void far closegraph(void);函数名:clreol功能: 在文本窗口中清除字符到行末用法:void clreol(void)函数名:clrscr功能: 清除文本模式窗口用法:void clrscr(void);函数名: coreleft功能: 返回未使用内存的大小用法:unsigned coreleft(void);函数名: cos功能: 余弦函数用法:double cos(double x);函数名:cosh功能: 双曲余弦函数用法: dluble cosh(double x);函数名: country功能: 返回与国家有关的信息用法: struct COUNTRY *country(int countrycode, struct country *country); 函数名: cprintf功能: 送格式化输出至屏幕用法:int cprintf(const char *format[, argument, ...]);函数名: cputs功能: 写字符到屏幕用法: void cputs(const char *string);函数名: _creat creat功能: 创建一个新文件或重写一个已存在的文件用法: int creat (const char *filename, int permiss)函数名:creatnew功能: 创建一个新文件用法:int creatnew(const char *filename, int attrib);函数名: cscanf功能: 从控制台执行格式化输入用法:int cscanf(char *format[,argument, ...]);函数名: ctime功能: 把日期和时间转换为字符串用法:char *ctime(const time_t *time);功能: 设置Ctrl-Break处理程序用法: void ctrlbrk(*fptr)(void);函数名: delay功能: 将程序的执行暂停一段时间(毫秒)用法: void delay(unsigned milliseconds);函数名: delline功能: 在文本窗口中删去一行用法: void delline(void);函数名:detectgraph功能: 通过检测硬件确定图形驱动程序和模式用法: void far detectgraph(int far *graphdriver, int far *graphmode); 函数名: difftime功能: 计算两个时刻之间的时间差用法: double difftime(time_t time2, time_t time1);函数名: disable功能: 屏蔽中断用法:void disable(void);函数名: div功能: 将两个整数相除, 返回商和余数用法:div_t (int number, int denom);函数名: dosexterr功能: 获取扩展DOS错误信息用法:int dosexterr(struct DOSERR *dblkp);函数名: dostounix功能: 转换日期和时间为UNIX时间格式用法: long dostounix(struct date *dateptr, struct time *timeptr);函数名: drawpoly功能: 画多边形用法: void far drawpoly(int numpoints, int far *polypoints);函数名:dup功能: 复制一个文件句柄用法: int dup(int handle);函数名:dup2功能: 复制文件句柄用法: int dup2(int oldhandle, int newhandle);功能: 把一个浮点数转换为字符串用法: char ecvt(double value, int ndigit, int *decpt, int *sign);函数名: ellipse功能: 画一椭圆用法:void far ellipse(int x, int y, int stangle, int endangle,int xradius, int yradius);函数名: enable功能: 开放硬件中断用法: void enable(void);函数名: eof功能: 检测文件结束用法: int eof(int *handle);函数名: exec...功能: 装入并运行其它程序的函数用法: int execl(char *pathname, char *arg0, arg1, ..., argn, NULL); int execle(char *pathname, char *arg0, arg1, ..., argn, NULL,char *envp[]);int execlp(char *pathname, char *arg0, arg1, .., NULL);int execple(char *pathname, char *arg0, arg1, ..., NULL,char *envp[]);int execv(char *pathname, char *argv[]);int execve(char *pathname, char *argv[], char *envp[]);int execvp(char *pathname, char *argv[]);int execvpe(char *pathname, char *argv[], char *envp[]);函数名:exit功能: 终止程序用法: void exit(int status);函数名: exp功能: 指数函数用法: double exp(double x);函数名: gcvt功能: 把浮点数转换成字符串用法: char *gcvt(double value, int ndigit, char *buf);函数名: geninterrupt功能: 产生一个软中断函数名: getarccoords功能: 取得最后一次调用arc的坐标用法: void far getarccoords(struct arccoordstype far *arccoords); 函数名: getaspectratio功能: 返回当前图形模式的纵横比用法: void far getaspectratio(int far *xasp, int far *yasp);函数名: getbkcolor功能: 返回当前背景颜色用法: int far getbkcolor(void);函数名: getc功能: 从流中取字符用法: int getc(FILE *stream);函数名: getcbrk功能: 获取Control_break设置用法: int getcbrk(void);函数名: getch功能: 从控制台无回显地取一个字符用法: int getch(void);函数名: getchar功能: 从stdin流中读字符用法: int getchar(void);函数名: getche功能: 从控制台取字符(带回显)用法: int getche(void);函数名: getcolor功能: 返回当前画线颜色用法: int far getcolor(void);函数名: getcurdir功能: 取指定驱动器的当前目录用法: int getcurdir(int drive, char *direc);函数名: getcwd功能: 取当前工作目录用法: char *getcwd(char *buf, int n);函数名: getdate功能: 取DOS日期函数名: getdefaultpalette功能: 返回调色板定义结构用法: struct palettetype *far getdefaultpalette(void);函数名: getdisk功能: 取当前磁盘驱动器号用法: int getdisk(void);函数名: getdrivername功能: 返回指向包含当前图形驱动程序名字的字符串指针用法: char *getdrivename(void);函数名: getdta功能: 取磁盘传输地址用法: char far *getdta(void);函数名: getenv功能: 从环境中取字符串用法: char *getenv(char *envvar);函数名: getfat, getfatd功能: 取文件分配表信息用法: void getfat(int drive, struct fatinfo *fatblkp);函数名: getfillpattern功能: 将用户定义的填充模式拷贝到内存中用法: void far getfillpattern(char far *upattern);函数名: getfillsettings功能: 取得有关当前填充模式和填充颜色的信息用法: void far getfillsettings(struct fillsettingstype far *fillinfo); 函数名: getftime功能: 取文件日期和时间用法: int getftime(int handle, struct ftime *ftimep);函数名: getgraphmode功能: 返回当前图形模式用法: int far getgraphmode(void);函数名: getftime功能: 取文件日期和时间用法: int getftime(int handle, struct ftime *ftimep);函数名: getgraphmode功能: 返回当前图形模式用法: int far getgraphmode(void);函数名: getimage功能: 将指定区域的一个位图存到主存中用法: void far getimage(int left, int top, int right, int bottom,void far *bitmap);函数名: getlinesettings功能: 取当前线型、模式和宽度用法: void far getlinesettings(struct linesettingstype far *lininfo): 函数名: getmaxx功能: 返回屏幕的最大x坐标用法: int far getmaxx(void);函数名: getmaxy功能: 返回屏幕的最大y坐标用法: int far getmaxy(void);函数名: getmodename功能: 返回含有指定图形模式名的字符串指针用法: char *far getmodename(int mode_name);函数名: getmoderange功能: 取给定图形驱动程序的模式范围用法: void far getmoderange(int graphdriver, int far *lomode,int far *himode);函数名: getpalette功能: 返回有关当前调色板的信息用法: void far getpalette(struct palettetype far *palette);函数名: getpass功能: 读一个口令用法: char *getpass(char *prompt);函数名: getpixel功能: 取得指定像素的颜色用法: int far getpixel(int x, int y);函数名: gets功能: 从流中取一字符串用法: char *gets(char *string);函数名: gettext功能: 将文本方式屏幕上的文本拷贝到存储区用法: int gettext(int left, int top, int right, int bottom, void *destin);函数名: gettextinfo功能: 取得文本模式的显示信息用法: void gettextinfo(struct text_info *inforec);函数名: gettextsettings功能: 返回有关当前图形文本字体的信息用法: void far gettextsettings(struct textsettingstype far *textinfo); 函数名: gettime功能: 取得系统时间用法: void gettime(struct time *timep);函数名: getvect功能: 取得中断向量入口用法: void interrupt(*getvect(int intr_num));函数名: getverify功能: 返回DOS校验标志状态用法: int getverify(void);函数名: getviewsetting功能: 返回有关当前视区的信息用法: void far getviewsettings(struct viewporttype far *viewport); 函数名: getw功能: 从流中取一整数用法: int getw(FILE *strem);函数名: getx功能: 返回当前图形位置的x坐标用法: int far getx(void);函数名: gety功能: 返回当前图形位置的y坐标用法: int far gety(void);函数名: gmtime功能: 把日期和时间转换为格林尼治标准时间(GMT)用法: struct tm *gmtime(long *clock);函数名: gotoxy功能: 在文本窗口中设置光标用法: void gotoxy(int x, int y);函数名: gotoxy功能: 在文本窗口中设置光标用法: void gotoxy(int x, int y);函数名: graphdefaults功能: 将所有图形设置复位为它们的缺省值用法: void far graphdefaults(void);函数名: grapherrormsg功能: 返回一个错误信息串的指针用法: char *far grapherrormsg(int errorcode);函数名: graphresult功能: 返回最后一次不成功的图形操作的错误代码用法: int far graphresult(void);函数名: _graphfreemem功能: 用户可修改的图形存储区释放函数用法: void far _graphfreemem(void far *ptr, unsigned size);函数名: _graphgetmem功能: 用户可修改的图形存储区分配函数用法: void far *far _graphgetmem(unsigned size);函数名: harderr功能: 建立一个硬件错误处理程序用法: void harderr(int (*fptr)());函数名: hardresume功能: 硬件错误处理函数用法: void hardresume(int rescode);函数名: highvideo功能: 选择高亮度文本字符用法: void highvideo(void);函数名: hypot功能: 计算直角三角形的斜边长用法: double hypot(double x, double y);函数名: imagesize功能: 返回保存位图像所需的字节数用法: unsigned far imagesize(int left, int top, int right, int bottom); 函数名: initgraph功能: 初始化图形系统用法: void far initgraph(int far *graphdriver, int far *graphmode函数名: inport功能: 从硬件端口中输入用法: int inp(int protid);函数名: insline功能: 在文本窗口中插入一个空行用法: void insline(void);函数名: installuserdriver功能: 安装设备驱动程序到BGI设备驱动程序表中用法: int far installuserdriver(char far *name, int (*detect)(void));函数名: installuserfont功能: 安装未嵌入BGI系统的字体文件(CHR)用法: int far installuserfont(char far *name);函数名: int86功能: 通用8086软中断接口用法: int int86(int intr_num, union REGS *inregs, union REGS *outregs) 函数名: int86x功能: 通用8086软中断接口用法: int int86x(int intr_num, union REGS *insegs, union REGS *outregs, 函数名: intdos功能: 通用DOS接口用法: int intdos(union REGS *inregs, union REGS *outregs);函数名: intdosx功能: 通用DOS中断接口用法: int intdosx(union REGS *inregs, union REGS *outregs,struct SREGS *segregs);函数名: intr功能: 改变软中断接口用法: void intr(int intr_num, struct REGPACK *preg);函数名: ioctl功能: 控制I/O设备用法: int ioctl(int handle, int cmd[,int *argdx, int argcx]);函数名: isatty功能: 检查设备类型用法: int isatty(int handle);函数名: itoa功能: 把一整数转换为字符串用法: char *itoa(int value, char *string, int radix);函数名: kbhit功能: 检查当前按下的键用法: int kbhit(void);函数名: keep功能: 退出并继续驻留用法: void keep(int status, int size);函数名: kbhit功能: 检查当前按下的键用法: int kbhit(void);函数名: keep功能: 退出并继续驻留用法: void keep(int status, int size);函数名: labs用法: long labs(long n);函数名: ldexp功能: 计算value*2的幂用法: double ldexp(double value, int exp);函数名: ldiv功能: 两个长整型数相除, 返回商和余数用法: ldiv_t ldiv(long lnumer, long ldenom);函数名: lfind功能: 执行线性搜索用法: void *lfind(void *key, void *base, int *nelem, int width,int (*fcmp)());函数名: line功能: 在指定两点间画一直线用法: void far line(int x0, int y0, int x1, int y1);函数名: linerel功能: 从当前位置点(CP)到与CP有一给定相对距离的点画一直线用法: void far linerel(int dx, int dy);函数名: localtime功能: 把日期和时间转变为结构用法: struct tm *localtime(long *clock);函数名: lock功能: 设置文件共享锁用法: int lock(int handle, long offset, long length);函数名: log功能: 对数函数ln(x)用法: double log(double x);函数名: log10功能: 对数函数log用法: double log10(double x);函数名: longjump功能: 执行非局部转移用法: void longjump(jmp_buf env, int val);函数名: lowvideo功能: 选择低亮度字符用法: void lowvideo(void);函数名: lrotl, _lrotl功能: 将无符号长整型数向左循环移位用法: unsigned long lrotl(unsigned long lvalue, int count);unsigned long _lrotl(unsigned long lvalue, int count);函数名: lsearch功能: 线性搜索用法: void *lsearch(const void *key, void *base, size_t *nelem,size_t width, int (*fcmp)(const void *, const void *));函数名: lseek功能: 移动文件读/写指针用法: long lseek(int handle, long offset, int fromwhere);main()主函数每一C 程序都必须有一main() 函数, 可以根据自己的爱好把它放在程序的某个地方。
c语言数学函数大全及详解

c语言数学函数大全及详解C语言提供了一系列的数学函数,这些函数包含在`math.h` 头文件中。
以下是一些常见的C 语言数学函数及其简要说明:1. fabs:-函数原型:`double fabs(double x);`-描述:返回`x` 的绝对值。
2. sqrt:-函数原型:`double sqrt(double x);`-描述:返回`x` 的平方根。
3. pow:-函数原型:`double pow(double x, double y);`-描述:返回`x` 的`y` 次方。
4. exp:-函数原型:`double exp(double x);`-描述:返回自然对数的底`e` 的`x` 次方。
5. log:-函数原型:`double log(double x);`-描述:返回`x` 的自然对数。
6. sin, cos, tan:-函数原型:- `double sin(double x);`- `double cos(double x);`- `double tan(double x);`-描述:分别返回`x` 的正弦、余弦和正切值。
这些函数中`x` 的单位是弧度。
7. asin, acos, atan:-函数原型:- `double asin(double x);`- `double acos(double x);`- `double atan(double x);`-描述:分别返回`x` 的反正弦、反余弦和反正切值。
返回的值是弧度。
8. sinh, cosh, tanh:-函数原型:- `double sinh(double x);`- `double cosh(double x);`- `double tanh(double x);`-描述:分别返回`x` 的双曲正弦、双曲余弦和双曲正切值。
9. ceil:-函数原型:`double ceil(double x);`-描述:返回不小于`x` 的最小整数值。
C语言中常用函数大全
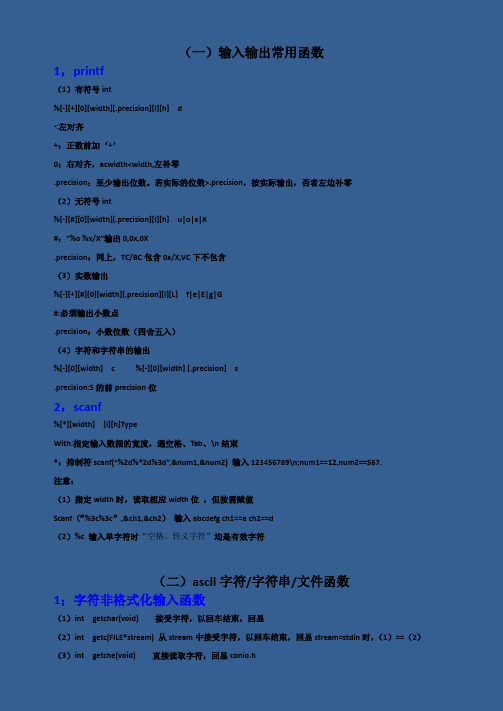
(一)输入输出常用函数1,printf(1)有符号int%[-][+][0][width][.precision][l][h] d-:左对齐+:正数前加‘+’0:右对齐,acwidth<width,左补零.precision:至少输出位数。
若实际的位数>.precision,按实际输出,否者左边补零(2)无符号int%[-][#][0][width][.precision][l][h] u|o|x|X#:”%o %x/X”输出0,0x,0X.precision:同上,TC/BC包含0x/X,VC下不包含(3)实数输出%[-][+][#][0][width][.precision][l][L] f|e|E|g|G#:必须输出小数点.precision:小数位数(四舍五入)(4)字符和字符串的输出%[-][0][width] c %[-][0][width] [.precision] s.precision:S的前precision位2,scanf%[*][width] [l][h]TypeWith:指定输入数据的宽度,遇空格、Tab、\n结束*:抑制符scanf(“%2d%*2d%3d”,&num1,&num2) 输入123456789\n;num1==12,num2==567.注意:(1)指定width时,读取相应width位,但按需赋值Scanf(“%3c%3c”,&ch1,&ch2)输入a bc d efg ch1==a ch2==d(2)%c 输入单字符时“空格、转义字符”均是有效字符(二)ascll字符/字符串/文件函数1;字符非格式化输入函数(1)int getchar(void) 接受字符,以回车结束,回显(2)int getc(FILE*stream) 从stream中接受字符,以回车结束,回显stream=stdin时,(1)==(2)(3)int getche(void) 直接读取字符,回显conio.h(4)int getchar(void) 直接读取字符,不回显conio.h注意:(1,2)对于回车键返回‘\n’(3,4)对于回车键返回‘\r’2;字符/串非格式化输出函数(1)int putchar(int c) 正常返回字符代码值,出错返回EOF(2)int putc(int c,FILE*stream) 正常返回字符代码值,出错返回EOF stream==stdout(1)=(2)(3)int puts(char*stream) 自动回车换行1;字符串的赋值#include< string.h memory.h >Void *memset (void *s, char ch, unsigned n)将以S为首地址的,一片连续的N个字节内存单元赋值为CH.Void *memcpy ( void *d, void*s, unsigned n)将以S为首地址的一片连续的N个字节内存单元的值拷贝到以D为首地址的一片连续的内存单元中。
C语言常用库函数
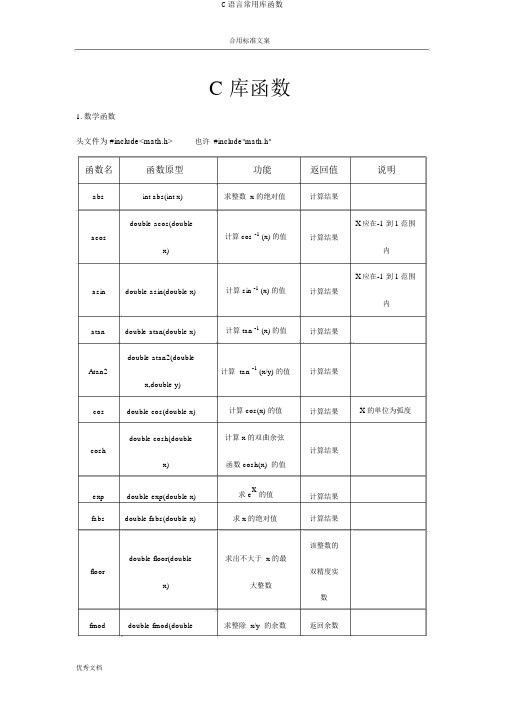
合用标准文案C 库函数1. 数学函数头文件为 #include<math.h>也许 #include"math.h"函数名函数原型功能返回值说明absint abs(int x)求整数 x 的绝对值计算结果double acos(doubleX 应在-1 到1范围acos计算 cos -1 (x) 的值计算结果x)内X 应在-1 到1范围asindouble asin(double x)计算 sin -1 (x) 的值计算结果内atan double atan(double x) 计算 tan -1 (x) 的值计算结果double atan2(doubleAtan2计算 tan -1 (x/y) 的值计算结果x,double y)cos double cos(double x) 计算 cos(x) 的值 计算结果 X 的单位为弧度double cosh(double计算 x 的双曲余弦cosh计算结果x)函数 cosh(x) 的值exp double exp(double x) 求 e x的值 计算结果 fabsdouble fabs(double x)求 x 的绝对值计算结果该整数的double floor(double求出不大于 x 的最floor双精度实x)大整数数fmod double fmod(double 求整除 x/y 的余数 返回余数合用标准文案x,double y) double frexp(double的双精度实数把双精度数val 分解为数字局部(尾数 )x返回数字和以 2 为底的指数frexpval, int *eptr)n ,即 val=x*2局部 xn0.5 ≤x< 1log double log(double x)double log10(double log10x)Double modf(double modfval, double *iptr)double pow(double powx,double y) rand Int rand(void)n 存放在eptr指向的变量中求 log ex,即 ln x计算结果求 log10x计算结果把双精度数val 分解为整数局部和小数Val 的小数局部,把整数局部存局部到 iptr计算xy的值计算结果产生随机 -90 到32767间的随机整随机整数数sin Double sin(double x)计算sin x的值计算结果X 单位为弧度sinh double sinh(double x)计算x的双曲正弦计算结果函数 sinh(x) 的值sqrt Double sqrt(double x)计算x计算结果X 应≥0 tan Double tan(double x)计算tan(x)的值计算结果X 单位为弧度Double tanh(double计算x的双曲正切tanh计算结果x)函数 tanh(x) 的值2.字符函数和字符串函数函数名函数原型功能返回值包括文件检查 ch 是否是字母isalnu Int isalnum (int是字母或数字返(alpha) 或数字m ch);回 1 ;否那么返回 0(numeric)是,返回 1 ;不是,isalpha Int isalpha(int ch);检查 ch 可否字母那么返回 0检查 ch 可否控制字符是,返回 1 ;不是,iscntrl Int iscntrl (int ch);〔其 ASCII 码在 0 和那么返回 00x1F 之间〕检查 ch 可否为数字是,返回 1 ;不是,isdigit Int isdigit (int ch);〔0~9 〕那么返回 0检查 ch 可否可打印字符Int isgraph (int是,返回 1 ;不是,isgraph〔其 ASCII 码在 0x21 和ch);那么返回 00x7E 之间〕,不包括空格Int islower (int检查 ch 可否小写字母是,返回 1 ;不是,islowerch);〔 a~z 〕那么返回 0检查 ch 可否可打印字符,〔包括空格〕,其是,返回 1 ;不是,isprint Intisprint (int ch);ASCII 码在 0x20 和 0x7E那么返回 0之间,ispunct Int ispunct (int检查 ch 可否标点字符是,返回 1 ;不是,ch);〔不包括空格〕,即除字那么返回0母、数字和空格以外的所有可打印字符Int isspace (int检查ch可否空格符、跳是,返回1;不是,isspacech);格符〔制表符〕或换行符那么返回0Int isupper (int检查ch可否大写字母是,返回1;不是,isupperch);〔A~Z 〕那么返回0检查 ch 可否一个十六进Intisxdigit (int是,返回1;不是,isxdigit制数字字符〔即0~9 ,ch);那么返回 0或 A~F ,或 a~f 〕把字符串str2 接到 str1char *strcat(charstrcat后边,str1 最后边的’ \0 ’Str1 *str1,char *str2);被取消找出 str 指向的字符串中返回指向该地址char *strchr(charstrchr第一次出现字符ch 的位的指针,如找不*str,int ch);置到,那么返回空指针Str1 <str2 ,返回负数;char *strcmp(char比较两个字符串str1 、Str1 =str2 ,返回strcmp*str1,char *str2);str20 ;str1 > str2 ,返回正数。
C语言曲线函数

像素函数putpix el() 画像素点函数getpix el()返回像素色函数直线和线型函数line() 画线函数lineto() 画线函数linere l() 相对画线函数setlin estyl e() 设置线型函数getlin esett ings() 获取线型设置函数setwri temod e() 设置画线模式函数多边形函数rectan gle()画矩形函数bar() 画条函数bar3d() 画条块函数drawpo ly() 画多边形函数圆、弧和曲线函数getasp ectra tio()获取纵横比函数circle()画圆函数arc() 画圆弧函数ellips e()画椭圆弧函数fillel lipse() 画椭圆区函数piesli ce() 画扇区函数sector() 画椭圆扇区函数getarc coord s()获取圆弧坐标函数填充函数setfil lstyl e() 设置填充图样和颜色函数setfil lpatt ern() 设置用户图样函数floodf ill() 填充闭域函数fillpo ly() 填充多边形函数getfil lsett ings() 获取填充设置函数getfil lpatt ern() 获取用户图样设置函数图像函数images ize() 图像存储大小函数getima ge() 保存图像函数putima ge() 输出图像函数图形和图像函数对许多图形应用程序,直线和曲线是非常有用的。
但对有些图形只能靠操作单个像素才能画出。
当然如果没有画像素的功能,就无法操作直线和曲线的函数。
而且通过大规模使用像素功能,整个图形就可以保存、写、擦除和与屏幕上的原有图形进行叠加。
(一) 像素函数putpix el() 画像素点函数功能:函数putp ixel() 在图形模式下屏幕上画一个像素点。
c语言头函数(Clanguageheadfunction)

c语言头函数(C language head function)Graphics and image functions(1) pixel function56. putpiel () draw pixel function57. GetPixel () returns the pixel color function(two) linear and linear functions58. line () draw line function59. lineto () draw line function(five) filled function76. setfillstyle () sets the fill pattern and the color function77. setfillpattern () sets the user pattern function78. floodfill () fills the closed field function(1) pixel function56. putpixel () draw pixel functionFunction: the function putpixel () draws a pixel on the screen in graphic mode.Usage: the function call method is void putpixel (int, x, int,y, int, color);Explanation: the parameter x, y is the coordinates of pixels, and color is the color of the pixel. It can be either a color name or an integer color value.The corresponding header file for this function is graphics.hReturn value: NoneCases:#include<graphics.h>Const int X=200;Const int Y=300;Const int N=10;Void main (void){Int, driver=DETECT, mode, i;Initgraph (&driver, &mode, "" ");Setbkcolor (WHITE);For (i=0; i<N; i+=100)Putpixel (X+i, Y+i, GREEN);}In this way!Don't link urls!Question: OK, the website address is OKQuestioner: 3214999 - probation one level best answer Classification function, where the function library is ctype.hInt isalpha (int CH), if ch is the letter ('A'-'Z','a'-'z'), returns no 0 value, otherwise returns 0Int isalnum (int, CH), if ch is the letter ('A'-'Z','a'-'z') or number ('0'-'9')Returns no 0 value, otherwise returns 0Int isascii (int CH), if ch is a character (0-127 of the ASCII code), returns no 0 value, otherwise returns 0Int iscntrl (int CH) if ch is an invalid character (0x7F) or an ordinary control character (0x00-0x1F)Returns no 0 value, otherwise returns 0Int isDigit (int CH), if ch is numeric ('0'-'9'), returns no 0 value, otherwise returns 0Int isgraph (int CH), if ch is printable character (without spaces) (0x21-0x7E), returns no 0 value, otherwise returns 0Int islower (int CH), if ch is a lowercase letter ('a'-'z'), returns no 0 value, otherwise returns 0Int isprint (int CH), if ch is printable characters (containing spaces) (0x20-0x7E), returns no 0 value, otherwise returns 0Int ispunct (int CH) returns no 0 value if ch is a punctuation character (0x00-0x1F), otherwise returns 0Int isspace (int CH) if ch is a space (` '), horizontal tab ('\t'), carriage return ('\r'),Take a paper feed line ('\f'), a vertical tab ('\v'), and a line break ('\n')Returns no 0 value, otherwise returns 0Int isupper (int CH), if ch is uppercase ('A'-'Z'), returns no 0 value, otherwise returns 0Int isxdigit (int CH), if ch is the 16 hexadecimal number ('0'-'9','A'-'F','a'-'f'), returns the non 0 value,Otherwise, return 0Int tolower (int CH), if ch is uppercase ('A'-'Z'), returns the corresponding lower case letter ('a'-'z')Int toupper (int CH), if ch is a lowercase letter ('a'-'z'), returns the corresponding uppercase letter ('A'-'Z')Mathematical function, where the function library for math.h, stdlib.h, string.h, float.hInt ABS (int i) returns the absolute value of the integer parameter IDouble cabs (struct, complex, znum) returns the absolute value of the complex znumDouble Fabs (double x) returns the absolute value of the double precision parameter xLong labs (long n) returns the absolute value of the long integer parameter nDouble exp (double, x) returns the value of the exponential function exDouble frexp (double, value, int, *eptr) returns the value of value=x*2n in X, and N is stored in eptrDouble ldexp (double, value, int, exp); returns the value of value*2expdouble log (double x) 返回logex的值返回log10x的值 double log10 (double x)double pow (double x, double y) 返回xy的值double pow10 (int p) 返回10p的值double sqrt (double x) 返回+ √x的值double acos (double x) 返回x的反余弦cos - 1 (x) 值, x为弧度double asin (double x) 返回x的反正弦sin - 1 (x) 值, x为弧度double atan (double x) 返回x的反正切tan - 1 (x) 值, x为弧度double atan2 (double x, double y) 返回y / x的反正切tan - 1 (x) 值, y的x为弧度double cos (double x) (x) 值, x为弧度返回x的余弦cosdouble sin (double x) (x) 值, x为弧度返回x的正弦sindouble tan (double x) (x) 值, x为弧度返回x的正切tandouble (double x) 返回x的双曲余弦cosh cosh (x) 值, x为弧度double sinh (double x) (x) 值, x为弧度返回x的双曲正弦sinhdouble tanh (double x) (x) 值, x为弧度返回x的双曲正切tanhdouble (double x, double y hypot) 返回直角三角形斜边的长度(z).x和y为直角边的长度 = x2 + y2, z2double ceil (double x) 返回不小于x的最小整数double floor (double x) 返回不大于x的最大整数for 初始化随机数发生器 srand (unsigned seed)in 产生一个随机数并返回这个数 rand ()double poly (int n, double x, double 从参数产生一个多项式 ([])double modf (double value, double * iptr) 将双精度数value分解成尾数和阶double (double x, double y fmod 返回x / y的余数)double frexp (double value, int * eptr) 将双精度数value分成尾数和阶double atof (char * nptr) 将字符串nptr转换成浮点数并返回这个浮点数double (char * nptr) have 将字符串nptr转换成整数并返回这个整数double (char * nptr) 将字符串nptr转换成长整数并返回这个整数atolchar * vtec (double value, int ndigit, int * decpt, int * sign)将浮点数value转换成字符串并返回该字符串char * fcvt (double value, int ndigit, int * decpt, int * sign)将浮点数value转换成字符串并返回该字符串char * gcvt (double value, int ndigit, char * buf)将数value转换成字符串并存于buf中, 并返回buf的指针ultoa (unsigned char * char * string length value, int radix)将无符号整型数value转换成字符串并返回该字符串, radix为转换时所用基数char * * * (long value, char * ltoa string, int radix)将长整型数value转换成字符串并返回该字符串, radix为转换时所用基数char * itoa (int value, char * string, int radix)将整数value转换成字符串存入string, radix为转换时所用基数double atof (char * nptr) 将字符串nptr转换成双精度数, 并返回这个数, 错误返回0int (char * nptr) have 将字符串nptr转换成整型数, 并返回这个数, 错误返回0long atol (char * nptr) 将字符串nptr转换成长整型数, 并返回这个数, 错误返回0strtod (char * str, double char * * endptr) 将字符串str转换成双精度数, 并返回这个数.strtol (char * str, long endptr char * *, int) 将字符串str 转换成长整型数.并返回这个数,int matherr(struct例外* E)用户修改数学错误返回信息函数(没有必要使用)双_matherr(_mexcep为什么,字符串,双arg1p,双arg2p,双用)用户修改数学错误返回信息函数(没有必要使用)unsigned int _clear87()清除浮点状态字并返回原来的浮点状态_fpreset()重新初使化浮点数学程序包无效unsigned int _status87()返回浮点状态字int chdir(char *path)使指定的目录路径(如:“C:\ WPS”)变成当前的工作目录,成功返回0int FindFirst(char *的路径名,结构ffblk * ffblk,int属性)查找指定的文件,成功返回0路径为指定的目录名和文件名,如“C:\软件\“ffblk为指定的保存文件信息的一个结构,定义如下:┏━━━━━━━━━━━━━━━━━━━━┓┃结构ffblk┃┃{┃┃焦ff_reserved [ 21 ];/ * * /┃DOS保留字┃焦ff_attrib;/ * * /┃文件属性┃int ff_ftime;/ * * /┃文件时间┃int ff_fdate;/ * * /┃文件日期┃长ff_fsize;/ * * /┃文件长度┃焦ff_name [ 13 ];/ * * /┃文件名┃}┃┗━━━━━━━━━━━━━━━━━━━━━┛属性为文件属性,由以下字符代表┏━━━━━━━━━┳━━━━━━━━━┓┃fa_rdonly只读文件┃fa_label卷标号┃┃fa_hidden隐藏文件┃fa_direc目录┃┃fa_system系统文件┃fa_arch档案┃┗━━━━━━━━━┻━━━━━━━━━┛例:结构ffblk FF;FindFirst(”*。
C语言中函数名

写文件函数(将数据流写入文件中)
ftell()
取得文件流的读取位置
fseek()
移动文件流的读写位置
freopen()
打开文件函数,并获得文件句柄
fread()
读文件函数(从文件流读取数据)
fputs()
写文件函数(将一指定的字符串写入文件内)
fputc()
写文件函数(将一指定字符写入文件流中)
设置程序正常结束前调用的函数
八、文件权限控制函数
utimes()
修改文件的存取时间和更改时间
utime()
修改文件的存取时间和更改时间
unlink()
删除文件
umask()
设置建立新文件时的权限遮罩
truncate()
改变文件大小
telldir()
取得目录流的读取位置
symlink()
建立文件符号连接
link()
建立文件连接
getcwd()
取得当前的工作目录
ftruncate()
改变文件大小
fstat()
由文件描述词取得文件状态
fchown()
改变文件的所有者
fchmod()
改变文件的权限
fchmod()
修改文件的权限
fchdir()
改变当前工作目录
closedir()
关闭目录
chroot()
改变文件根目录
二、字符串操作函数(#include<string.h>)
strtok()
字符串分割函数
strstr()
字符串查找函数
strspn()
字符查找函数
strrchr()
定位字符串中最后出现的指定字符
C语言标准库函数大全.pdf
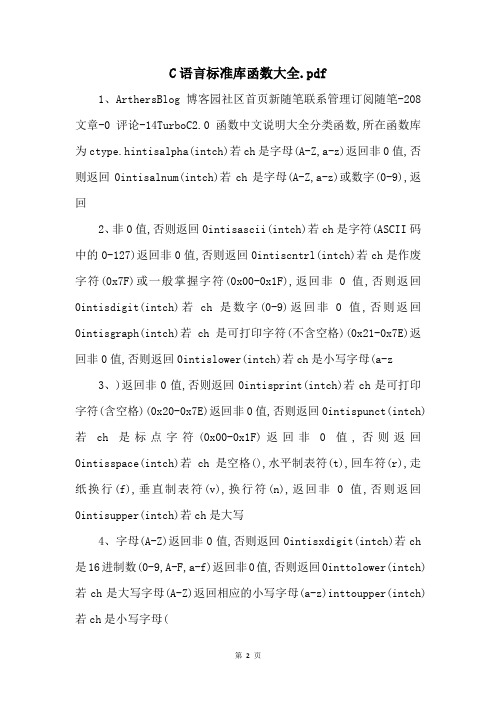
C语言标准库函数大全.pdf1、ArthersBlog博客园社区首页新随笔联系管理订阅随笔-208文章-0评论-14TurboC2.0函数中文说明大全分类函数,所在函数库为ctype.hintisalpha(intch)若ch是字母(A-Z,a-z)返回非0值,否则返回0intisalnum(intch)若ch是字母(A-Z,a-z)或数字(0-9),返回2、非0值,否则返回0intisascii(intch)若ch是字符(ASCII码中的0-127)返回非0值,否则返回0intiscntrl(intch)若ch是作废字符(0x7F)或一般掌握字符(0x00-0x1F),返回非0值,否则返回0intisdigit(intch)若ch是数字(0-9)返回非0值,否则返回0intisgraph(intch)若ch是可打印字符(不含空格)(0x21-0x7E)返回非0值,否则返回0intislower(intch)若ch是小写字母(a-z3、)返回非0值,否则返回0intisprint(intch)若ch是可打印字符(含空格)(0x20-0x7E)返回非0值,否则返回0intispunct(intch)若ch是标点字符(0x00-0x1F)返回非0值,否则返回0intisspace(intch)若ch是空格(),水平制表符(t),回车符(r),走纸换行(f),垂直制表符(v),换行符(n),返回非0值,否则返回0intisupper(intch)若ch是大写4、字母(A-Z)返回非0值,否则返回0intisxdigit(intch)若ch 是16进制数(0-9,A-F,a-f)返回非0值,否则返回0inttolower(intch)若ch是大写字母(A-Z)返回相应的小写字母(a-z)inttoupper(intch)若ch是小写字母(5、a-z)返回相应的大写字母(A-Z)数学函数,所在函数库为math.h、stdlib.h、string.h、float.hintabs(inti)返回整型参数i的肯定值doublecabs(structcomplexznum)返回复数znum的肯定值doublefabs(doublex)返回双精度参数x的肯定值longlabs(longn)返回长整型参数n的肯定值doubleexp(doublex)返回指数函数ex的值doublefrexp(doublevalue,int*eptr)返回v6、alue=x*2n中x的值,n存贮在eptr中doubleldexp(doublevalue,intexp);返回value*2exp的值doublelog(doublex)返回logex的值doublelog10(doublex)返回log10x的值doublepow(doublex,doubley)返回xy的值doublepow10(intp)返回10p的值doublesqrt(doublex)返回x的开方doubleacos(doublex)返回x的反余弦cos-1(x)值,x为弧度c语言库函数大全--资料收集预备是胜利的首要前提与我联系发短消息搜寻常用链接我的随笔7、我的空间我的短信我的评论更多链接留言簿给我留言查看留言随笔分类(174).Net(rss)Algorithm(15)(rss)Basic(10)(rss)CC++(33)(rss) DBase(2)(rss)Essay(112)(rss)Java(2)(rss)随笔档案(208)2021年8月(1)2021年5月(5)2021年4月(4)2021年3月(1)2021年1月(21)2021年12月(48)2021年11月(121)2021年10月(7)找找看Page1of222021-4-3file://F:DOCUME~1lichaoLOCALS~1Tem8、pKABU69VF.htm。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
函数名: labs
用法: long labs(long n);
程序例:
#include #include int main(void) { long result; long x = -12345678L; result= labs(x); printf("number: %ld abs value: %ld\n", x, result); return 0; }
函数名: log10
功能: 对数函数 log
用法: double log10(double x);
程序例:
#include #include int main(void) { double result; double x = 800.6872; result = log10(x); printf("The common log of %lf is %lf\n", x, result); return 0; }
函数名: lock
功能: 设置文件共享锁
用法: int lock(int handle, long offset, long length);
程序例:
#include #include #include #include #include #include int main(void) { int handle, status; long length; /* Must have DOS Share.exe loaded for */
函数名: ldexp
功能: 计算 value*2的幂
用法: double ldexp(double value, int exp);
程序例:
#include #include int main(void) { double value; double x = 2; /* ldexp raises 2 by a power of 3 then multiplies the result by 2 */ value = ldexp(x,3); printf("The ldexp value is: %lf\n", value); return 0; }
函数名: lfind
功能: 执行线性搜索
用法: void *lfind(void *key, void *base, int *nelem, int width,
int (*fcmp)());
程序例:
#include #include int compare(int *x, int *y) { return( *x - *y ); } int main(void) { int array[5] = {35, 87, 46, 99, 12}; size_t nelem = 5; int key; int *result; key = 99; result = lfind(&key, array, &nelem, sizeof(int), (int(*)(const void *,const void *))compare); if (result) printf("Number %d found\n",key); else
函数名: log
功能: 对数函数 ln(x)
用法: double log(double x);
程序例:
#include #include int main(void) { double result; double x = 8.6872; result = log(x); printf("The natural log of %lf is %lf\n", x, result); return 0; }
/* lrotl example */ #include #include int main(void) { unsigned long result; unsigned long value = 100; result = _lrotl(value,1); printf("The value %lu rotated left one bit is: %lu\n", value, result); return 0; }
函数名: lowvideo
功能: 选择低亮度字符
用法: void lowvideo(void);
程序例:
#include int main(void) { clrscr(); highvideo(); cprintf("High Intesity Text\r\n"); lowvideo(); gotoxy(1,2); cprintf("Low Intensity Text\r\n"); return 0; }
函数名: longjump
功能: 执行非局部转移
用法: void longjump(jmp_buf env, int val);
程序例:
#include #include #include void subroutine(jmp_buf); int main(void) { int value; jmp_buf jumper; value = setjmp(jumper); if (value != 0) { printf("Longjmp with value %d\n", value); exit(value); }QQ291911320 printf("About to call subroutine ... \n"); subroutine(jumper); return 0; } void subroutine(jmp_buf jumper) { longjmp(jumper,1); }
/* file locking to function properly */ handle = sopen("c:\\autoexec.bat", O_RDONLY,SH_DENYNO,S_IREAD); if (handle < 0) { printf("sopen failed\n"); exit(1); } length = filelength(handle); status = lock(handle,0L,length/2); if (status == 0) printf("lock succeeded\n"); else printf("lock failed\n"); status = unlock(handle,0L,length/2); if (status == 0) printf("unlock succeeded\n"); else printf("unlock failed\n"); close(handle); return 0; }
函数名: lrotl, _lrotl
功能: 将无符号长整型数向左循环移位
用法: unsigned long lrotl(unsigned long lvalue, int count);
unsigned long _lrotl(unsigned long lvalue, int count);
程序例:
函数名: linerel
功能: 从当前位置点(CP)到与 CP 有一给定相对距离的点画一直线
用法: void far linerel(int dx, int dy);
程序例:
#include #include #include #include int main(void) { /* request auto detection */ int gdriver = DETECT, gmode, errorcode; char msg[80]; /* initialize graphics and local variables */ initgraph(&gdriver, &gmode, ""); /* read result of initialization */ errorcode = graphresult(); if (errorcode != grOk) { printf("Graphics error: %s\n", grapherrormsg(errorcode)); printf("Press any key to halt:"); getch(); exit(1); } /* move the C.P. to location (20, 30) */ moveto(20, 30); /* create and output a message at (20, 30) */ sprintf(msg, " (%d, %d)", getx(), gety()); outtextxy(20, 30, msg); /* draw a line to a point a relative distance away from the current value of C.P. */ linerel(100, 100); /* create and output a message at C.P. */ sprintf(msg, " (%d, %d)", getx(), gety()); outtext(msg); /* clean up */ getch(); closegraph();
函数名: ldiv
功能: 两个长整型数相除, 返回商和余数
用法: ldiv_t ldiv(long lnumer, long ldenom);
程序例:
/* ldiv example */ #include #include int main(void) { ldiv_t lx; lx = ldiv(100000L, 30000L); printf("100000 div 30000 = %ld remainder %ld\n", lx.quot, lx.rem); return 0caltime