Java进程并发演示模型
2024版年度Java程序设计全套课件完整版

•课程介绍与预备知识•基础语法与程序结构•面向对象编程基础目•常用类库与工具使用•图形用户界面开发录•网络编程与数据库连接•多线程编程技术•设计模式与架构思想目•课程总结与展望录Java语言概述及发展历程Java语言的起源与特点01Java的发展历程02Java的应用领域03编程环境搭建与工具选择JDK的安装与配置开发工具的选择Maven的使用编写HelloWorld 程序带领学员编写并运行第一个Java 程序,了解Java 程序的基本结构。
程序解析详细讲解HelloWorld 程序的每一行代码,让学员了解Java 程序的执行流程。
常见问题与解决方法针对初学者在编写和运行Java 程序时可能遇到的问题,提供解决方案。
第一个Java 程序示例030201介绍Java 中的基本数据类型(如int 、float 、char 等)和引用数据类型(如类、接口等)。
Java 中的数据类型变量的声明与赋值运算符的使用类型转换讲解如何在Java 中声明变量、为变量赋值以及变量的作用域。
介绍Java 中的算术运算符、关系运算符、逻辑运算符等,以及运算符的优先级和结合性。
详细讲解Java 中的自动类型转换和强制类型转换,以及转换过程中可能遇到的问题。
数据类型、变量和运算符根据特定条件执行不同代码块。
if 条件语句根据表达式的值选择执行多个代码块中的一个。
switch 语句简洁的if-else 结构,用于条件判断并返回结果。
三目运算符分支结构循环结构for循环while循环do-while循环一维数组存储表格形式数据,可通过多个下标访问元素。
多维数组数组排序数组查找01020403在数组中查找指定元素,并返回其下标或位置信息。
存储相同类型数据的线性结构,可通过下标访问元素。
使用排序算法对数组元素进行排序,如冒泡排序、选择排序等。
数组及其应用方法定义指定方法名、参数列表和返回类型,编写方法体实现特定功能。
方法调用通过方法名和参数列表调用已定义的方法,执行其功能并获取返回值。
java aio原理
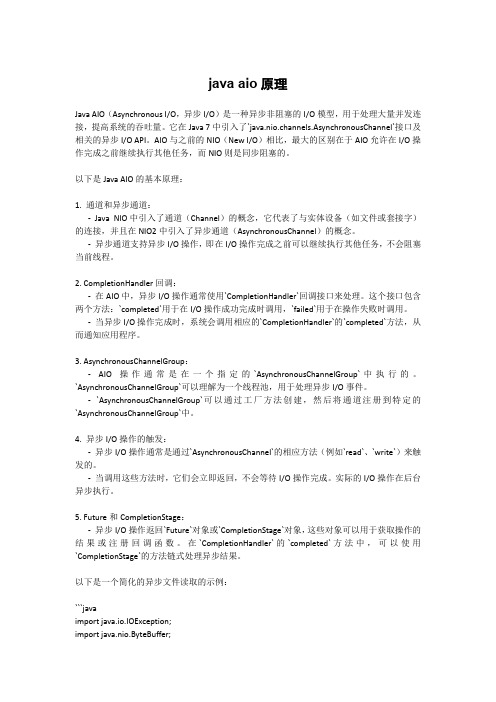
java aio原理Java AIO(Asynchronous I/O,异步I/O)是一种异步非阻塞的I/O模型,用于处理大量并发连接,提高系统的吞吐量。
它在Java 7中引入了`java.nio.channels.AsynchronousChannel`接口及相关的异步I/O API。
AIO与之前的NIO(New I/O)相比,最大的区别在于AIO允许在I/O操作完成之前继续执行其他任务,而NIO则是同步阻塞的。
以下是Java AIO的基本原理:1. 通道和异步通道:-Java NIO中引入了通道(Channel)的概念,它代表了与实体设备(如文件或套接字)的连接,并且在NIO2中引入了异步通道(AsynchronousChannel)的概念。
-异步通道支持异步I/O操作,即在I/O操作完成之前可以继续执行其他任务,不会阻塞当前线程。
2. CompletionHandler回调:-在AIO中,异步I/O操作通常使用`CompletionHandler`回调接口来处理。
这个接口包含两个方法:`completed`用于在I/O操作成功完成时调用,`failed`用于在操作失败时调用。
-当异步I/O操作完成时,系统会调用相应的`CompletionHandler`的`completed`方法,从而通知应用程序。
3. AsynchronousChannelGroup:-AIO操作通常是在一个指定的`AsynchronousChannelGroup`中执行的。
`AsynchronousChannelGroup`可以理解为一个线程池,用于处理异步I/O事件。
-`AsynchronousChannelGroup`可以通过工厂方法创建,然后将通道注册到特定的`AsynchronousChannelGroup`中。
4. 异步I/O操作的触发:-异步I/O操作通常是通过`AsynchronousChannel`的相应方法(例如`read`、`write`)来触发的。
java中实现并发的方法
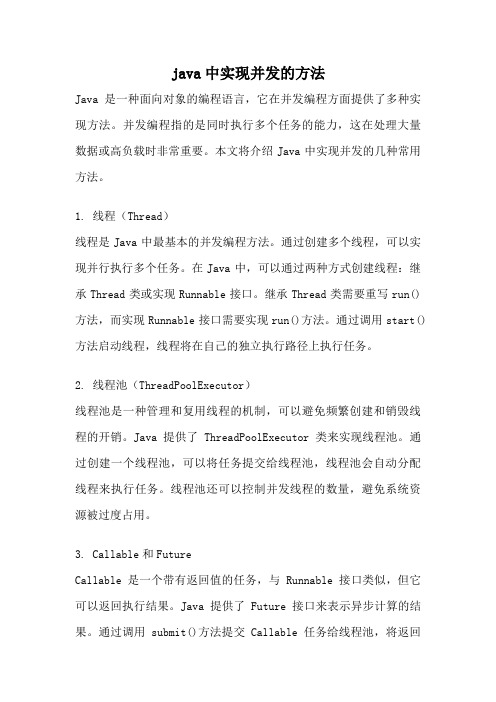
java中实现并发的方法Java是一种面向对象的编程语言,它在并发编程方面提供了多种实现方法。
并发编程指的是同时执行多个任务的能力,这在处理大量数据或高负载时非常重要。
本文将介绍Java中实现并发的几种常用方法。
1. 线程(Thread)线程是Java中最基本的并发编程方法。
通过创建多个线程,可以实现并行执行多个任务。
在Java中,可以通过两种方式创建线程:继承Thread类或实现Runnable接口。
继承Thread类需要重写run()方法,而实现Runnable接口需要实现run()方法。
通过调用start()方法启动线程,线程将在自己的独立执行路径上执行任务。
2. 线程池(ThreadPoolExecutor)线程池是一种管理和复用线程的机制,可以避免频繁创建和销毁线程的开销。
Java提供了ThreadPoolExecutor类来实现线程池。
通过创建一个线程池,可以将任务提交给线程池,线程池会自动分配线程来执行任务。
线程池还可以控制并发线程的数量,避免系统资源被过度占用。
3. Callable和FutureCallable是一个带有返回值的任务,与Runnable接口类似,但它可以返回执行结果。
Java提供了Future接口来表示异步计算的结果。
通过调用submit()方法提交Callable任务给线程池,将返回一个Future对象,可以使用该对象获取任务的执行结果。
4. 并发集合(Concurrent Collections)Java提供了一些并发安全的集合类,例如ConcurrentHashMap、ConcurrentLinkedQueue等。
这些集合类在多线程环境下使用时,可以避免出现线程安全问题。
并发集合类采用了一些特殊的数据结构和算法来保证线程安全性,能够高效地处理并发访问。
5. 锁(Lock)锁是一种同步机制,可以保证多个线程对共享资源的互斥访问。
Java提供了synchronized关键字来实现锁机制,也提供了Lock接口及其实现类来实现更加灵活的锁。
Java定时任务Quartz(三)——并发

Java定时任务Quartz(三)——并发1 前⾔根据 Quartz 的设计,⼀个 Job 可以绑定多个 Trigger,必然会遇到并发的问题。
2 并发2.1 复现让我们编写⼀个并发的例⼦:1/**2 * @author pancc3 * @version 1.04*/5public class AcceptConcurrentDemo {67public static void main(String[] args) throws SchedulerException, InterruptedException {8 JobDetail detail = JobBuilder.newJob(AcceptConcurrentJob.class)9 .withIdentity("detail", "group0")10 .build();111213 Trigger trigger = TriggerBuilder.newTrigger()14 .withIdentity("ben_trigger")15 .usingJobData("name", "ben")16 .startNow()17 .build();1819 Trigger triggers = TriggerBuilder.newTrigger()20 .withIdentity("mike_trigger")21 .usingJobData("name", "mike")22 .forJob("detail", "group0")23 .startNow()24 .build();252627 Scheduler scheduler = new StdSchedulerFactory().getScheduler();2829 scheduler.start();30 scheduler.scheduleJob(detail, trigger);31 scheduler.scheduleJob(triggers);32/*33 * 6 秒钟后关闭34*/35 Thread.sleep(6_000);36 scheduler.shutdown();37 }3839 @Data40public static class AcceptConcurrentJob implements Job {41private String name;4243 @Override44public void execute(JobExecutionContext context) {45try {46 System.out.printf("i am %s \n", name);47 Thread.sleep(2_000);48 } catch (InterruptedException e) {49 e.printStackTrace();50 }51 }52 }53 }请注意上边的 Details 的 Identity ,设置为 group0.detail,同时我们创建了两个 Trigger,第⼆个 trigger 在创建的时候通过指定 Identity 绑定到了⽬标 Job,接着提交这个 Job,与两个 Trigger ,可以看到两个触发器同时出发了 Job 的 execute ⽅法上边的代码也可以简化为以下形式:1/**2 * @author pancc3 * @version 1.04*/5public class AcceptConcurrentDemo {67public static void main(String[] args) throws SchedulerException, InterruptedException {8 JobDetail detail = JobBuilder.newJob(AcceptConcurrentJob.class)9 .withIdentity("detail", "group0")10 .build();111213 Trigger trigger = TriggerBuilder.newTrigger()14 .withIdentity("ben_trigger")15 .usingJobData("name", "ben")16 .startNow()17 .build();1819 Trigger triggers = TriggerBuilder.newTrigger()20 .withIdentity("mike_trigger")21 .usingJobData("name", "mike")22 .startNow()23 .build();242526 Scheduler scheduler = new StdSchedulerFactory().getScheduler();2728 scheduler.start();29 scheduler.scheduleJob(detail, Sets.newHashSet(trigger,triggers),true);30/*31 * 6 秒钟后关闭32*/33 Thread.sleep(6_000);34 scheduler.shutdown();35 }3637 @Data38public static class AcceptConcurrentJob implements Job {39private String name;4041 @Override42public void execute(JobExecutionContext context) {43try {44 System.out.printf("i am %s \n", name);45 Thread.sleep(2_000);46 } catch (InterruptedException e) {47 e.printStackTrace();48 }49 }50 }51 }2.2 避免并发为了避免并发,我们可以使⽤官⽅提供的注解 @DisallowConcurrentExecution,通过在类上增加这个注解,我们可以观察到第⼆个 trigger 进⾏了排队处理: 1/**2 * @author pancc3 * @version 1.04*/5public class RejectConcurrentDemo {67public static void main(String[] args) throws SchedulerException, InterruptedException {8 JobDetail detail = JobBuilder.newJob(RejectConcurrentJob.class)9 .withIdentity("detail", "group0")10 .build();111213 Trigger trigger = TriggerBuilder.newTrigger()14 .withIdentity("ben_trigger")15 .usingJobData("name", "ben")16 .startNow()17 .build();1819 Trigger triggers = TriggerBuilder.newTrigger()20 .withIdentity("mike_trigger")21 .usingJobData("name", "mike")22 .forJob("detail", "group0")23 .startNow()24 .build();252627 Scheduler scheduler = new StdSchedulerFactory().getScheduler();2829 scheduler.start();30 scheduler.scheduleJob(detail, trigger);31 scheduler.scheduleJob(triggers);32/*33 * 6 秒钟后关闭34*/35 Thread.sleep(6_000);36 scheduler.shutdown();37 }383940 @DisallowConcurrentExecution41 @Data42public static class RejectConcurrentJob implements Job {43private String name;4445 @Override46public void execute(JobExecutionContext context) {47try {48 System.out.printf("i am %s \n", name);49 Thread.sleep(2_000);50 } catch (InterruptedException e) {51 e.printStackTrace();52 }53 }54 }55 }3 避免并发的原理探索让我们找到 JobStore 的实现类,在这⾥是 RAMJobStore,点进去⽅法 org.quartz.simpl.RAMJobStore#acquireNextTriggers,可以看到这个⽅法的某个块:通过对 Job 类上的是否存在 DisallowConcurrentExecution 注解,如果存在,表⽰拒绝并发执⾏ execute ⽅法。
java面试题库java面试题目及答案(3篇)
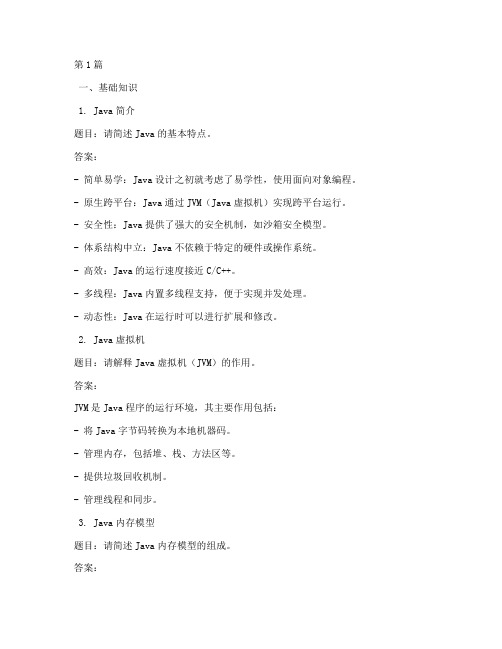
第1篇一、基础知识1. Java简介题目:请简述Java的基本特点。
答案:- 简单易学:Java设计之初就考虑了易学性,使用面向对象编程。
- 原生跨平台:Java通过JVM(Java虚拟机)实现跨平台运行。
- 安全性:Java提供了强大的安全机制,如沙箱安全模型。
- 体系结构中立:Java不依赖于特定的硬件或操作系统。
- 高效:Java的运行速度接近C/C++。
- 多线程:Java内置多线程支持,便于实现并发处理。
- 动态性:Java在运行时可以进行扩展和修改。
2. Java虚拟机题目:请解释Java虚拟机(JVM)的作用。
答案:JVM是Java程序的运行环境,其主要作用包括:- 将Java字节码转换为本地机器码。
- 管理内存,包括堆、栈、方法区等。
- 提供垃圾回收机制。
- 管理线程和同步。
3. Java内存模型题目:请简述Java内存模型的组成。
答案:Java内存模型主要由以下部分组成:- 堆(Heap):存储对象实例和数组。
- 栈(Stack):存储局部变量和方法调用。
- 方法区(Method Area):存储类信息、常量、静态变量等。
- 本地方法栈(Native Method Stack):存储本地方法调用的相关数据。
- 程序计数器(Program Counter Register):存储线程的当前指令地址。
4. Java关键字题目:请列举并解释Java中的几个关键字。
答案:- `public`:表示访问权限为公开。
- `private`:表示访问权限为私有。
- `protected`:表示访问权限为受保护。
- `static`:表示属于类本身,而非对象实例。
- `final`:表示常量或方法不能被修改。
- `synchronized`:表示线程同步。
- `transient`:表示数据在序列化时不会被持久化。
二、面向对象编程5. 类和对象题目:请解释类和对象之间的关系。
答案:类是对象的模板,对象是类的实例。
process model15解读

1. 简介进程模型是操作系统中的核心概念之一,它描述了程序如何在计算机中执行,如何进行通信和同步等重要内容。
在计算机科学中,有许多不同的进程模型,每种模型都有其特定的特点和适用场景。
本文将对进程模型进行深入解读,包括其基本概念、分类、特点、应用等方面的内容。
2. 进程模型的基本概念进程是指在计算机系统中运行的程序的实例。
它是操作系统资源分配的基本单位,具有独立的位置区域空间、独立的内存空间、独立的文件系统等特点。
进程模型则是描述进程如何被创建、管理、调度、通信和同步的理论模型。
它包括了进程的状态转换、进程间的通信机制、进程的调度算法等内容。
3. 进程模型的分类根据进程的调度方式,进程模型可以分为多种类型。
常见的进程模型包括批处理系统、交互式系统、实时系统等。
批处理系统是指按照程序提交的顺序进行执行的系统,其中每个程序都需要等待前一个程序执行完毕才能开始执行。
交互式系统是指用户可以直接与系统进行交互的系统,用户可以随时输入指令并得到相应的结果。
实时系统是指对时间要求非常严格的系统,能够在严格的时间限制内完成任务的系统。
4. 进程模型的特点不同的进程模型具有不同的特点。
批处理系统具有高效、稳定的特点,但用户体验较差;交互式系统可以提供良好的用户体验,但需要保证系统响应速度和并发执行能力;实时系统需要满足时间要求非常严格的特点,能够在规定的时间内完成任务。
5. 进程模型的应用进程模型的应用非常广泛。
在操作系统中,不同类型的进程模型可以应用于不同的场景。
批处理系统常用于需要进行大量计算的场景,如科学计算、数据分析等;交互式系统常用于普通用户使用的计算机系统,能够提供良好的用户体验;实时系统常用于对时间要求非常严格的场景,如航空航天、工业控制等领域。
6. 结语进程模型是操作系统中非常重要的概念,对于理解计算机系统的运行原理和优化程序设计具有重要意义。
不同的进程模型具有不同的特点和适用场景,合理地选择和使用进程模型能够提高系统的性能和可靠性。
Java课程24_多线程

1.3 创建线程的方式
• • • • • • • • • • • • } } } public void run() { System.out.println("子线程是:"+this); public static void main(String args[]) { Thread t= Thread.currentThread(); System.out.println("主线程是: "+t); MyThread1 ex = new MyThread1(); ex.start(); class MyThread1 extends Thread { 或者使用 implements Runnable
21
2.1 线程类常用方法
• • • • • • • resume() 恢复挂起的线程,使其处于可运行状态(Runnable)。 yield() 将CPU控制权主动移交到下一个可运行线程。 setPriority() 设置线程优先级。 getPriority() 返回线程优先级。 setName() 设置线程的名字。 getName() 返回该线程的名字。 isAlive( ) 如果线程已被启动并且未被终止,那么isAlive( )返回true。如果返 回false,则该线程是新创建或是已被终止的。
IT教育系列
JAVA多线程
版权声明
• • 本课件由浙江浙大网新集团有限公司(以下简称:网新集团)编制,仅 供网新集团培训机构的学员学习使用; 网新集团享有本课件中的文字叙述、文档格式、插图、照片等所有信息 资料的版权,受知识产权法及版权法等法律、法规的保护。任何个人或 组织未经网新集团的书面授权许可,均不得以任何形式使用本课件的任 何内容,否则将视为不法侵害,网新集团保留追究侵权人相关法律责任 的权利 如您不接受上述声明,请勿使用本课件,并尽快销毁或删除本课件任何 形式的备份;如您使用本课件,将被视为您接受并同意遵守上述声明。
JAVA自学教程(完整版)PPT课件(2024)
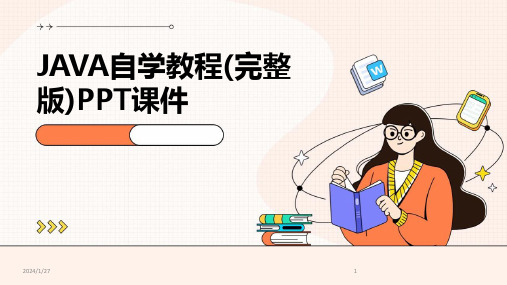
二分查找
针对有序数组,每次取中间元 素与目标元素比较,缩小查找 范围
12
03 面向对象编程基础
2024/1/27
13
类与对象的概念
类的定义
类是对象的模板,它定 义了对象的属性和方法 。
2024/1/27
对象的概念
对象是类的实例,具有 类定义的属性和行为。
类与对象的关系
类是对象的抽象描述, 而对象是类的具体实现 。
2024/1/27
32
Socket通信原理及示例
Socket通信原理
理解Socket通信的基本原理,掌握Socket 类和ServerSocket类的使用。
TCP编程
学习基于TCP协议的Socket通信,实现客户 端与服务器之间的数据传输。
多线程处理
掌握多线程在Socket通信中的应用,提高服 务器的并发处理能力。
TreeSet类的特点和使用
TreeSet是Set接口的另一个常用实现类,它基于红黑树实 现。TreeSet会对元素进行排序,因此它适用于需要排序的 场景。
26
Map接口及其实现类
01
Map接口的定义和特 点
Map接口表示一种键值对的映射关系 。Map中的每个元素都包含一个键和 一个值,键在Map中是唯一的。
学习ReentrantLock锁的使用,了解 公平锁与非公平锁的区别。
2024/1/27
等待/通知机制
掌握Object类的wait()、notify()和 notifyAll()方法的使用,实现线程间 的通信。
死锁与避免
了解死锁的概念及产生条件,学习如 何避免死锁的发生。
31
网络编程基础
网络编程概述
ArrayList类的特点和使用
第2章Java多线程应用ppt课件全

2
• 2. join( ) • join( )方法使当前正在执行的线程进入等待状态(挂起),直至方法join( )所调用
• 2.1 线程和多线程 • 2.2 实例1 Java程序的多线程机制 • 2.3 实例2 Java程序中的多线程实现 • 2.4 实例3 基于Java语言的多线程同步机制 • 2.5实例4 用Java语言实• 线程(thread)是指计算机正在执行的程序中的一个控制流程。线程本 身不是完整程序,没有执行的入口,也没有出口,因此其自身不能自 动运行,而必须栖身于某一进程之中,由进程触发执行。
•
try //睡眠一随机时间,让出处理器
•
{Thread.sleep((int)(Math.random()*50));}
及在这段时间内线程能完成的任务,在线程的生命周期中有四种状态,通过对线程进 行操作来改变其状态。 • 1.创建状态 • 创建了一个线程而还没有启动它,则处于创建状态,此时仅是一个空的线程对象,并 不获得应有资源,只有启动后,系统才为它分配资源。处于创建状态的线程可以进行 两种操作:一是通过调用start()方法启动,使其进入可运行状态;二是调用stop()方法, 使其进入消亡状态。 • 2.可运行状态 • 在线程的创建状态中进行启动操作,则此线程进入可运行状态。可运行状态只说明该 线程具备了运行的条件,但并不一定是运行状态,因为在单处理器系统中运行多线程 程序,实际上在每个“时刻”至多有一个线程在运行,而系统中可能有多个线程都处 于运行状态,系统通过快速切换和调度使所有可运行的线程共享处理器,造成宏观上 的多线程并发运行。在可运行状态,线程运行的是线程体,线程体由run()方法规定, 在自己定义的线程类中重写。 • 在可运行状态下可进行多种操作:调用suspend()方法,使线程挂起,从而进入不可运 行状态;调用sleep()方法,使线侱睡眠,从而进入不可运行状态;调用wait()方法,使线 程等待,从而进入不可运行状态;调用yield()方法,使线程退让,使线程把CPU控制权 提前交给同级优先权的其他线程;调用stop()方法,使线程终止,从而进入消亡状态。正 常的情况下是执行完run()方法,使线程结束,进入消亡状态。
实战Java高并发程序设计

4.2 Java 虚拟机对锁优化所做的努力 146 4.2.1 锁偏向 146 4.2.2 轻量级锁 146 4.2.3 自旋锁 146 4.2.4 锁消除 146
3.1 多线程的团队协作:同步控制 70 3.1.1 synchronized 的功能扩展:重入锁 71 3.1.2 重入锁的好搭档:Condition 条件 80 3.1.3 允许多个线程同时访问:信号量(Semaphore) 83 3.1.4 ReadWriteLock 读写锁 85 3.1.5 倒计时器:CountDownLatch 87 3.1.6 循环栅栏:CyclicBarrier 89 3.1.7 线程阻塞工具类:LockSupport 92
3.2 线程复用:线程池 95 3.2.1 什么是线程池 96 3.2.2 不要重复发明轮子:JDK 对线程池的支持 97 3.2.3 刨根究底:核心线程池的内部实现 102 3.2.4 超负载了怎么办:拒绝策略 106 3.2.5 自定义线程创建:ThreadFactory 109 3.2.6 我的应用我做主:扩展线程池 110 3.2.7 合理的选择:优化线程池线程数量 112 3.2.8 堆栈去哪里了:在线程池中寻找堆栈 113 3.2.9 分而治之:Fork/Join 框架 117
V
1.3.5 无等待(Wait-Free) 13 1.4 有关并行的两个重要定律 13
1.4.1 Amdahl 定律 13 1.4.2 Gustafson 定律 16 1.4.3 Amdahl 定律和 Gustafson 定律是否相互矛盾 16 1.5 回到 Java:JMM 17 1.5.1 原子性(Atomicity) 18 1.5.2 可见性(Visibility) 20 1.5.3 有序性(Ordering) 22 1.5.4 哪些指令不能重排:Happen-Before 规则 27 1.6 参考文献 27 第 2 章 Java 并行程序基础 ..............................................................................................................................29 2.1 有关线程你必须知道的事 29 2.2 初始线程:线程的基本操作 32 2.2.1 新建线程 32 2.2.2 终止线程 34 2.2.3 线程中断 38 2.2.4 等待(wait)和通知(notify) 41 2.2.5 挂起(suspend)和继续执行(resume)线程 44 2.2.6 等待线程结束(join)和谦让(yield) 48 2.3 volatile 与 Java 内存模型(JMM)50 2.4 分门别类的管理:线程组 52 2.5 驻守后台:守护线程(Daemon) 54 2.6 先干重要的事:线程优先级 55 2.7 线程安全的概念与 synchronized 57
如何在Java中进行并发计算和分布式系统的优化设计

如何在Java中进行并发计算和分布式系统的优化设计并发计算是指多个任务在同一时间段内同时执行的计算方式。
而分布式系统是指将一个计算机系统分布在不同的物理位置上,通过网络互联,形成一个整体的计算系统。
在Java中,可以使用多线程技术来实现并发计算,同时也可以使用分布式框架来优化分布式系统的设计。
1.并发计算的优化设计:在Java中,可以通过以下几种方式来优化并发计算的设计:1.1使用线程池:线程池是一个管理线程的工具,可以重用已创建的线程,有效地管理线程的创建和销毁。
通过使用线程池,可以避免频繁地创建和销毁线程所带来的开销,并且可以控制同时执行的线程数量,避免系统资源被过度占用。
1.2使用锁机制:Java提供了synchronized关键字和Lock接口来实现锁机制,可以保证多个线程访问共享资源的互斥性,避免数据竞争和不一致性。
在多线程环境下,通过合理的锁机制设计,可以提高并发计算的效率和准确性。
1.3使用并发容器:Java提供了一系列的并发容器,如ConcurrentHashMap、ConcurrentLinkedQueue等,这些容器在多线程环境下具有较高的并发性能。
通过使用并发容器,可以避免手动实现线程安全的数据结构,减少错误和并发问题的发生。
1.4使用无锁算法:无锁算法是一种高效的并发计算方式,通过使用原子操作或CAS(Compare and Swap)指令来实现多个线程对共享资源的并发操作,避免了锁机制带来的性能损耗。
在Java中,可以使用Atomic类或java.util.concurrent.atomic包下的原子类来实现无锁算法。
1.5使用并行流和并行算法:Java 8引入了Stream API和并行流(Parallel Stream),通过将计算任务分解为多个子任务,然后并行执行,可以利用多核处理器的性能优势,提高计算速度。
同时,还可以使用Java 8提供的并行算法,如并行排序、并行归约等,进一步提高并发计算的效率。
基于Java的高并发服务器设计与实现

基于Java的高并发服务器设计与实现在当今互联网时代,高并发服务器已经成为许多互联网企业的核心需求之一。
随着用户量的不断增加,服务器需要能够同时处理大量的请求,确保系统的稳定性和性能。
本文将介绍基于Java的高并发服务器设计与实现,包括服务器架构设计、并发编程模型、性能优化等方面的内容。
服务器架构设计在设计高并发服务器时,首先需要考虑服务器的架构设计。
一个典型的高并发服务器通常包括以下几个组件:网络通信模块:负责接收客户端请求,并将请求分发给后端处理模块。
请求处理模块:负责处理客户端请求,执行相应的业务逻辑。
数据库访问模块:负责与数据库进行交互,读取或写入数据。
缓存模块:用于缓存热点数据,提高系统响应速度。
在Java中,可以使用NIO(New Input/Output)或者Netty等框架来实现高效的网络通信模块,提升服务器的并发处理能力。
并发编程模型在高并发服务器中,并发编程是至关重要的。
Java提供了多种并发编程模型,如线程、线程池、锁等机制。
合理地利用这些机制可以提高服务器的并发处理能力。
线程池:通过线程池可以有效地管理线程资源,避免频繁地创建和销毁线程带来的开销。
锁机制:使用锁机制可以保护共享资源,避免多个线程同时访问导致的数据竞争问题。
并发集合:Java提供了诸如ConcurrentHashMap、ConcurrentLinkedQueue等并发集合类,可以在多线程环境下安全地操作数据。
性能优化除了良好的架构设计和并发编程模型外,性能优化也是设计高并发服务器不可或缺的一部分。
以下是一些常见的性能优化策略:减少锁竞争:尽量减少锁粒度,避免长时间持有锁。
异步处理:将一些耗时操作改为异步执行,提高系统吞吐量。
内存管理:合理管理内存资源,避免内存泄漏和频繁GC (Garbage Collection)带来的性能损耗。
横向扩展:通过横向扩展增加服务器节点数量,提高系统整体处理能力。
实现示例下面是一个简单的基于Java的高并发服务器实现示例:示例代码star:编程语言:javapublic class HighConcurrencyServer {public static void main(String[] args) {ServerSocket serverSocket = new ServerSocket(8888);ExecutorService executorService = Executors.newFixedThreadPool(10);while (true) {Socket socket = serverSocket.accept();executorService.execute(() -> {// 业务逻辑处理handleRequest(socket);});}}private static void handleRequest(Socket socket) {// 处理客户端请求}}示例代码end在上面的示例中,通过ServerSocket监听端口,并使用线程池处理客户端请求,实现了一个简单的高并发服务器。
java并发控制的处理机制

Java并发控制的处理机制1. 引言在多线程编程中,为了保证数据的一致性和避免竞态条件等问题,需要使用并发控制的处理机制。
Java提供了多种机制来实现并发控制,包括锁、原子变量、线程安全的集合类等。
本文将详细介绍Java并发控制的处理机制。
2. 锁机制锁是最常用的并发控制机制之一。
Java提供了两种锁机制:synchronized关键字和Lock接口。
2.1 synchronized关键字synchronized关键字可以修饰方法或代码块,实现对临界资源的互斥访问。
当一个线程获得了对象的锁后,其他线程将被阻塞,直到该线程释放锁。
public synchronized void synchronizedMethod() {// 临界区代码}public void method() {synchronized (this) {// 临界区代码}}2.2 Lock接口Lock接口提供了更加灵活的锁机制,可以实现更复杂的并发控制。
Lock接口的常用实现类有ReentrantLock和ReadWriteLock。
Lock lock = new ReentrantLock();lock.lock();try {// 临界区代码} finally {lock.unlock();}3. 原子变量原子变量是一种特殊的变量类型,可以保证多个线程对其操作的原子性。
Java提供了一系列原子变量类,如AtomicInteger、AtomicLong和AtomicReference等。
AtomicInteger count = new AtomicInteger(0);count.incrementAndGet(); // 原子性地增加计数器的值4. 线程安全的集合类Java提供了一些线程安全的集合类,如ConcurrentHashMap和CopyOnWriteArrayList等。
这些集合类通过内部的并发控制机制,保证了多线程环境下的安全访问。
java中接口并发的一般处理方案
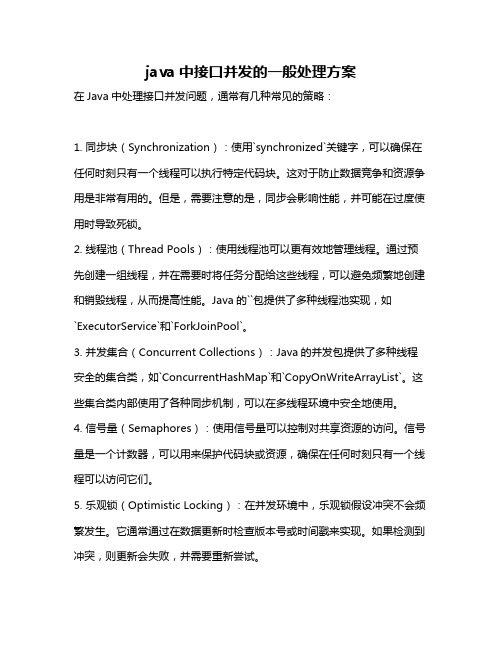
java中接口并发的一般处理方案在Java中处理接口并发问题,通常有几种常见的策略:1. 同步块(Synchronization):使用`synchronized`关键字,可以确保在任何时刻只有一个线程可以执行特定代码块。
这对于防止数据竞争和资源争用是非常有用的。
但是,需要注意的是,同步会影响性能,并可能在过度使用时导致死锁。
2. 线程池(Thread Pools):使用线程池可以更有效地管理线程。
通过预先创建一组线程,并在需要时将任务分配给这些线程,可以避免频繁地创建和销毁线程,从而提高性能。
Java的``包提供了多种线程池实现,如`ExecutorService`和`ForkJoinPool`。
3. 并发集合(Concurrent Collections):Java的并发包提供了多种线程安全的集合类,如`ConcurrentHashMap`和`CopyOnWriteArrayList`。
这些集合类内部使用了各种同步机制,可以在多线程环境中安全地使用。
4. 信号量(Semaphores):使用信号量可以控制对共享资源的访问。
信号量是一个计数器,可以用来保护代码块或资源,确保在任何时刻只有一个线程可以访问它们。
5. 乐观锁(Optimistic Locking):在并发环境中,乐观锁假设冲突不会频繁发生。
它通常通过在数据更新时检查版本号或时间戳来实现。
如果检测到冲突,则更新会失败,并需要重新尝试。
6. 悲观锁(Pessimistic Locking):与乐观锁相反,悲观锁假设冲突会频繁发生,因此在访问数据时会立即锁定数据。
其他线程必须等待锁释放后才能访问数据。
以上是处理Java接口并发问题的一些常见策略。
具体使用哪种方案取决于具体的应用场景和需求。
Java并发程序设计教程
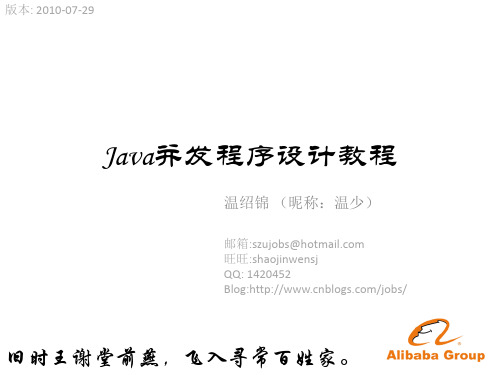
使用阻塞队列
Queue<E>
add(E) : boolean offer() : boolean remove() : E poll() : E element() : E peek() : E
使用BlockingQueue的时候,尽量不要使用从Queue继承下来的 方法,否则就失去了Blocking的特性了。
initialValue() : T get() : T set(T value) remove()
顾名思义它是local variable(线程局部变量)。它的功用非 常简单,就是为每一个使用该变量的线程都提供一个变量值 的副本,是每一个线程都可以独立地改变自己的副本,而不 会和其它线程的副本冲突。从线程的角度看,就好像每一个 线程都完全拥有该变量。
Task Executor
Callable<Object> task = new Callable<Object>() { public Object call() throws Exception { Object result = …; return result; } };
Task Submitter把任务提交给Executor执行,他们之间需要一种 通讯手段,这种手段的具体实现,通常叫做Future。Future通常 包括get(阻塞至任务完成), cancel,get(timeout)(等待一 段时间)等等。Future也用于异步变同步的场景。
在BlockingQueue中,要使用put和take,而非offer和poll。如果 要使用offer和poll,也是要使用带等待时间参数的offer和poll。
使用drainTo批量获得其中的内容,能够减少锁的次数。
Java高并发测试框架JCStress
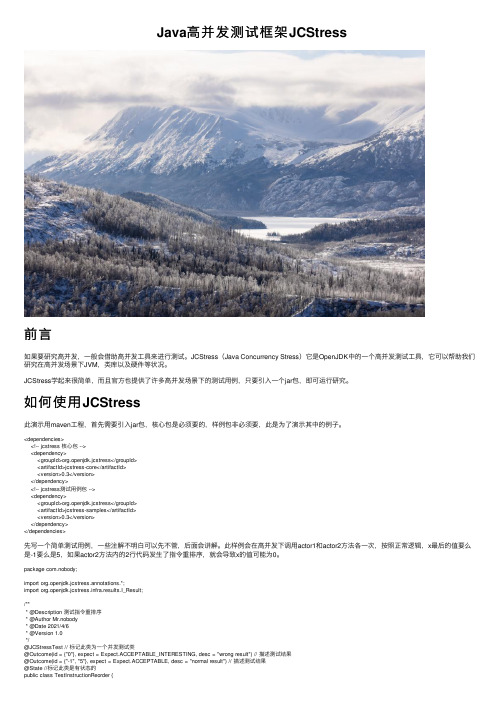
Java⾼并发测试框架JCStress前⾔如果要研究⾼并发,⼀般会借助⾼并发⼯具来进⾏测试。
JCStress(Java Concurrency Stress)它是OpenJDK中的⼀个⾼并发测试⼯具,它可以帮助我们研究在⾼并发场景下JVM,类库以及硬件等状况。
JCStress学起来很简单,⽽且官⽅也提供了许多⾼并发场景下的测试⽤例,只要引⼊⼀个jar包,即可运⾏研究。
如何使⽤JCStress此演⽰⽤maven⼯程,⾸先需要引⼊jar包,核⼼包是必须要的,样例包⾮必须要,此是为了演⽰其中的例⼦。
<dependencies><!-- jcstress 核⼼包 --><dependency><groupId>org.openjdk.jcstress</groupId><artifactId>jcstress-core</artifactId><version>0.3</version></dependency><!-- jcstress测试⽤例包 --><dependency><groupId>org.openjdk.jcstress</groupId><artifactId>jcstress-samples</artifactId><version>0.3</version></dependency></dependencies>先写⼀个简单测试⽤例,⼀些注解不明⽩可以先不管,后⾯会讲解。
此样例会在⾼并发下调⽤actor1和actor2⽅法各⼀次,按照正常逻辑,x最后的值要么是-1要么是5,如果actor2⽅法内的2⾏代码发⽣了指令重排序,就会导致x的值可能为0。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
进程并发演示模型
一实验截图
二代码
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class CZFrame extends JFrame implements ActionListener
{
CZThread thread1,thread2,thread3;
JButton wait1=new JButton("等待"),wait2=new JButton("等待"),wait3=new JButton("等待");
JButton exit=new JButton("退出");
JLabel name=new JLabel("作者:田毛毛");
public CZFrame(int n1,int n2,int n3)
{
setTitle("进程并发演示模型");
setSize(500,550);
setVisible(true);
thread1=new CZThread(n1);
thread2=new CZThread(n2);
thread3=new CZThread(n3);
Container container=getContentPane();
container.setBackground(Color.pink);
container.setLayout(null);
container.add(thread1.panel);
container.add(thread2.panel);
container.add(thread3.panel);
wait1.setBounds(400,150,70,30);
wait2.setBounds(400,250,70,30);
wait3.setBounds(400,350,70,30);
exit.setBounds(200,450,70,30);
container.add(wait1);
container.add(wait2);
container.add(wait3);
container.add(exit);
wait1.addActionListener(this);
wait2.addActionListener(this);
wait3.addActionListener(this);
exit.addActionListener(this);
JLabel label=new JLabel("进程并发演示模型");
Font font=new Font("宋体",Font.BOLD,16);
label.setFont(font);
label.setBounds(175,20,180,50);
container.add(label);
name.setBounds(400,40,100,50);
container.add(name);
thread1.start();
thread2.start();
thread3.start();
}
public void actionPerformed(ActionEvent e)
{
Object s=e.getSource();
if(s==exit)
{
/*thread1.suspend();
thread2.suspend();
thread3.suspend();*/
int i=JOptionPane.showConfirmDialog(null,"确认退出吗?","退出",JOptionPane.YES_NO_OPTION);
if(i==0) System.exit(0);
/*thread1.resume();
thread2.resume();
thread3.resume();*/
}
else if(s==wait1)
{
try
{
if(thread1.st==0)
{
thread1.suspend();
thread1.st=1;
wait1.setText("执行");
thread1.num--;
}
else
{
thread1.resume();
thread1.st=0;
wait1.setText("等待");
thread1.num++;
}
}
catch(Exception e1)
{}
}
else if(s==wait2)
{
try
{
if(thread2.st==0)
{
thread2.suspend();
thread2.st=1;
wait2.setText("执行");
thread1.num--;
}
else
{
thread2.resume();
thread2.st=0;
wait2.setText("等待");
thread1.num++;
}
}
catch(Exception e1)
{}
}
else
{
try
{
if(thread3.st==0)
{
thread3.suspend();
thread3.st=1;
wait3.setText("执行");
thread1.num--;
}
else
{
thread3.resume();
thread3.st=0;
wait3.setText("等待");
thread1.num++;
}
}
catch(Exception e1)
{}
}
}
public static void main(String args[])
{
int n1=0,n2=1,n3=2;
CZFrame frame=new CZFrame(n1,n2,n3);
frame.setDefaultCloseOperation(EXIT_ON_CLOSE);
frame.show();
}
}
class CZThread extends Thread
{
CZPanel panel;
int st=0;
static int num=3;
public CZThread(int n)
{
panel=new CZPanel(n);
panel.setBounds(0,130+n*100,380,60);
}
public void S()
{
}
public void run()
{
try
{
while(true)
{
while(panel.m<=330&&panel.flag==0)
{
panel.repaint();
sleep(20*num);
}
panel.m=330;
panel.flag=1;
while(panel.m>=20&&panel.flag==1)
{
panel.repaint();
sleep(20*num);
}
panel.m=20;
panel.flag=0;
}
}
catch(Exception e)
{}
}
private class CZPanel extends JPanel
{
public CZPanel(int n)
{
this.n=n;
}
public void paintComponent(Graphics g)
{
super.paintComponent(g);
g.setColor(Color.cyan);
g.fillRect(0,0,20,30);
g.setColor(Color.blue);
g.fillRect(0,30,20,30);
g.setColor(Color.yellow);
g.fillRect(20,0,359,59);
g.setColor(Color.green);
if(m<=330&&flag==0)
{
g.fillRect(m,0,50,60);
g.setColor(Color.red);
g.drawString("进程"+(n+1),m+10,28);
m+=20;
}
else if(m>=20&&flag==1)
{
g.fillRect(m,0,50,60);
g.setColor(Color.red);
g.drawString("进程"+(n+1),m+10,28);
m-=20;
}
}
int m=20,n=0,flag=0;
}
}。