JAVA游戏代码
用Java实现一个简单的打飞机游戏

用Java实现一个简单的打飞机游戏打飞机游戏是一类经典的游戏,具有简单、刺激和容易上手的特点。
在本文中,我们将使用Java编程语言来实现一个简单的打飞机游戏。
要实现这个游戏,我们可以分为三个主要的步骤:游戏初始化、游戏逻辑和游戏界面。
第一步是游戏初始化。
我们需要设置游戏窗口的大小和标题,以及创建游戏界面所需的元素,如玩家飞机、敌机和子弹。
我们可以使用Java提供的图形库,如AWT或JavaFX来创建游戏窗口和界面元素。
第二步是游戏逻辑。
我们需要定义游戏的规则和行为。
首先,我们需要让玩家飞机可以根据用户的输入(如键盘按键)进行移动。
然后,我们需要创建敌机,并使其在游戏界面上自动移动。
接下来,我们需要创建子弹,并使其可以击中敌机。
当玩家的飞机与敌机相撞或者子弹击中敌机时,游戏将结束。
最后一步是游戏界面。
我们需要定义游戏界面的显示方式。
可以在游戏界面上显示玩家的飞机和敌机,并实时更新它们的位置。
还可以显示分数和游戏状态,以提供更好的游戏体验。
下面是一个简单的示例代码,演示了如何使用Java实现一个简单的打飞机游戏:```javaimport javax.swing.*;import java.awt.*;import java.awt.event.KeyEvent;import java.awt.event.KeyListener;public class SimplePlaneGame extends JFrame implements KeyListener {private final int WINDOW_WIDTH = 800;private final int WINDOW_HEIGHT = 600;private int playerX = 400;private int playerY = 500;private boolean gameRunning = true;public SimplePlaneGame() {setTitle("Simple Plane Game");setSize(WINDOW_WIDTH, WINDOW_HEIGHT);setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);setResizable(false);setLocationRelativeTo(null);setLayout(null);setVisible(true);addKeyListener(this);}public void paint(Graphics g) {super.paint(g);g.setColor(Color.BLUE);g.fillRect(playerX, playerY, 50, 50);}public void keyPressed(KeyEvent e) {if (e.getKeyCode() == KeyEvent.VK_LEFT) {playerX -= 5;repaint();} else if (e.getKeyCode() == KeyEvent.VK_RIGHT) { playerX += 5;repaint();}}public void keyReleased(KeyEvent e) {}public void keyTyped(KeyEvent e) {}public static void main(String[] args) {SimplePlaneGame game = new SimplePlaneGame();while (game.gameRunning) {try {Thread.sleep(10);} catch (InterruptedException e) {e.printStackTrace();}}}}```在这个示例中,我们使用了一个继承自JFrame的类来创建游戏窗口。
Java猜拳小游戏源代码

第一个文件:public class Computer {String name;int score;public int showfist(){int quan;quan=(int)(Math.random()*10);if(quan<=2){quan=1;}else if(quan<=5){quan=2;}else{quan=3;}switch(quan){case 1:System.out.println(name+"出拳:剪刀");break;case 2:System.out.println(name+"出拳:石头");break;case 3:System.out.println(name+"出拳:布");break;}return quan;}}第二个文件:import java.util.Scanner;public class Game {int count=0;int countP=0;Person person=new Person();Computer computer=new Computer();Scanner input=new Scanner(System.in);public void initial(){System.out.print("请选择你的角色(1.刘备 2.孙权 3.曹操):");int juese=input.nextInt();switch(juese){case 1:="刘备";break;case 2:="孙权";break;case 3:="曹操";break;}System.out.print("请选择对手角色(1.关羽 2.张飞 3.赵云):");int JueSe=input.nextInt();switch(JueSe){case 1:="关羽";break;case 2:="张飞";break;case 3:="赵云";break;}}public void begin(){System.out.print("\n要开始吗? (y/n)");String ans=input.next();if(ans.equals("y")){String answ;do{int a=person.showFist();int b=computer.showfist();if(a==1&&b==3||a==2&&b==1||a==3&&b==2){System.out.println("结果:你赢了!");person.score++;}else if(a==1&&b==1||a==2&&b==2||a==3&&b==3){System.out.println("结果:平局,真衰!嘿嘿,等着瞧吧!");countP++;}else{System.out.println("结果:你输了!");computer.score++;}count++;System.out.print("\n是否开始下一轮? (y/n)");answ=input.next();}while(answ.equals("y"));}}public String calcResult(){String a;if(person.score>computer.score){a="最终结果:恭喜恭喜!你赢了!";}else if(person.score==computer.score){a="最终结果:打成平手,下次再和你一决高下!";}else{a="最终结果:呵呵,你输了!笨笨,下次加油啊!";}return a;}public void showResult(){System.out.println("---------------------------------------------------");System.out.println("\t\t"++" VS"++"\n");System.out.println("对战次数:"+count+"次");System.out.println("平局:"+countP+"次");System.out.println(+"得:"+person.score+"分");System.out.println(+"得:"+computer.score+"分\n");System.out.println(calcResult());System.out.println("---------------------------------------------------");}}第三个文件:import java.util.Scanner;public class Person {String name;int score;Scanner input=new Scanner(System.in);public int showFist(){System.out.print("\n请出拳:1.剪刀2.石头3.布");int quan=input.nextInt();switch(quan){case 1:System.out.println("你出拳:剪刀");break;case 2:System.out.println("你出拳:石头");break;case 3:System.out.println("你出拳:布");break;}return quan;}}第四个文件:public class Test {public static void main(String[]args){Game g=new Game();System.out.println("-----------------欢迎进入游戏世界--------------------\n\n");System.out.println("\t\t******************");System.out.println("\t\t** 猜拳开始 **");System.out.println("\t\t******************\n\n");System.out.println("出拳规则:1.剪刀2.石头3.布");g.initial();g.begin();g.showResult();}}。
JAVA小游戏代码(剪刀石头布)

JAVA⼩游戏代码(剪⼑⽯头布) /** 创建⼀个类Game,⽯头,剪⼑,布的游戏。
*/public class Game {/*** @param args*/String[] s ={"⽯头","剪⼑","布"};//获取电脑出拳String getComputer(int i){String computerGuess = s[i];return computerGuess;}//判断⼈出拳是否为⽯头,剪⼑,布boolean isOrder(String guess){boolean b = false;for(int x = 0;x < s.length; x++){if(guess.equals(s[x])){b = true;break;}}return b;}//⽐较void winOrLose(String guess1,String guess2){if(guess1.equals(guess2)){System.out.println("你出:" + guess1 + ",电脑出:" + guess2 + "。
平了");}else if(guess1.equals("⽯头")){if(guess2.equals("剪⼑"))System.out.println("你出:" + guess1 + ",电脑出:" + guess2 + "。
You Win!");}else{System.out.println("你出:" + guess1 + ",电脑出:" + guess2 + "。
You Lose!");}}else if(guess1.equals("剪⼑")){if(guess2.equals("布")){System.out.println("你出:" + guess1 + ",电脑出:" + guess2 + "。
初学JAVA好玩的小游戏代码
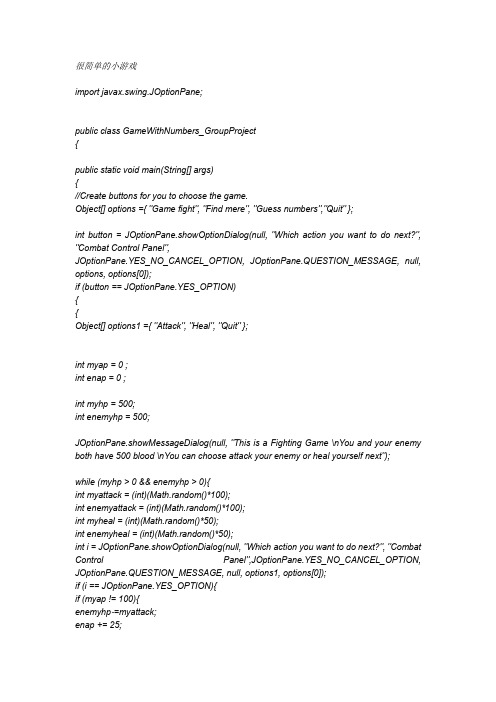
很简单的小游戏import javax.swing.JOptionPane;public class GameWithNumbers_GroupProject{public static void main(String[] args){//Create buttons for you to choose the game.Object[] options ={ "Game fight", "Find mere", "Guess numbers","Quit" };int button = JOptionPane.showOptionDialog(null, "Which action you want to do next?", "Combat Control Panel",JOptionPane.YES_NO_CANCEL_OPTION, JOptionPane.QUESTION_MESSAGE, null, options, options[0]);if (button == JOptionPane.YES_OPTION){{Object[] options1 ={ "Attack", "Heal", "Quit" };int myap = 0 ;int enap = 0 ;int myhp = 500;int enemyhp = 500;JOptionPane.showMessageDialog(null, "This is a Fighting Game \nYou and your enemy both have 500 blood \nYou can choose attack your enemy or heal yourself next");while (myhp > 0 && enemyhp > 0){int myattack = (int)(Math.random()*100);int enemyattack = (int)(Math.random()*100);int myheal = (int)(Math.random()*50);int enemyheal = (int)(Math.random()*50);int i = JOptionPane.showOptionDialog(null, "Which action you want to do next?", "Combat Control Panel",JOptionPane.YES_NO_CANCEL_OPTION, JOptionPane.QUESTION_MESSAGE, null, options1, options[0]);if (i == JOptionPane.YES_OPTION){if (myap != 100){enemyhp-=myattack;enap += 25;if(enemyhp <= 0){JOptionPane.showMessageDialog(null,"You have attacked your enemy this turn \nYour HP: " + myhp + ", \nYour enemy's HP:" + "0" + " \nGet into your enemy's turn?");break;}else{JOptionPane.showMessageDialog(null,"You have attacked your enemy this turn \nYour HP: " + myhp + ", \nYour enemy's HP:" + enemyhp + " \nGet into your enemy's turn?");}}else{enemyhp-=myattack*2;enap += 25;myap = 0 ;if(enemyhp <= 0){JOptionPane.showMessageDialog(null,"You have attacked your enemy"+ " critically this turn \nYour HP: " + myhp + ", \nYour enemy's HP:" + "0" +" \nGet into your enemy's turn?");break;}else{JOptionPane.showMessageDialog(null,"You have attacked your enemy critially this "+ "turn \nYour HP: " + myhp + ", \nYour enemy's HP:" + enemyhp +" \nGet into your enemy's turn?");}}if (i == JOptionPane.NO_OPTION){myhp+=myheal;if (myhp >= 1000){myhp = 1000;}}JOptionPane.showMessageDialog(null,"You have healed yourself this turn \nYour HP: " + myhp + ", \nYour enemy's HP:" + enemyhp + " \nGet into your enemy's turn?");}if (i == JOptionPane.CANCEL_OPTION){break;}int enemyif = (int)(Math.random()*2);if (enemyif == 0){if(enap !=100){myhp-=enemyattack;myap+=25;很简单的小游戏if(myhp>=0){JOptionPane.showMessageDialog(null,"Your enemy has attacked you this turn \nYour HP: " + myhp + ", \nYour enemy's HP: " + enemyhp + " \nDo you want to get into your turn?");}else{JOptionPane.showMessageDialog(null,"Your enemy has attacked you this turn \nYour HP: " + "0" + ", \nYour enemy's HP: " + enemyhp + " \nDo you want to get into your turn?"); break;}}else{myhp-=enemyattack*2;myap+=25;enap = 0;if(myhp>=0){JOptionPane.showMessageDialog(null,"Your enemy has attacked you "+ "critically this turn \nYour HP: " + myhp +", \nYour enemy's HP: " + enemyhp + " \nDo you want to get "+ "into your turn?");}else{JOptionPane.showMessageDialog(null,"Your enemy has attacked you "+ "critically this turn \nYour HP: " + "0" +", \nYour enemy's HP: " + enemyhp + " \nDo you want to get "+ "into your turn?");break;}}}if (enemyif == 1){enemyhp+=enemyheal;if(enemyhp >= 1000){enemyhp = 1000;}JOptionPane.showMessageDialog(null,"Your enemy has healed himself this turn \nyour HP: " + myhp + ", \nYour enemy's HP:" + enemyhp + " \nDo you want to get into your turn?");}}if (myhp <= 0){JOptionPane.showMessageDialog(null,"You die, Game Over");}if (enemyhp <= 0){JOptionPane.showMessageDialog(null,"Your enemy die, You are the winner!");}else{JOptionPane.showMessageDialog(null,"The Player Quit The Game");}}}if (button == JOptionPane.NO_OPTION){//Prompt user enter his or her birthday.int birthmonth=Integer.parseInt(JOptionPane.showInputDialog(null,"Enter the month "+ "you were born in(1 to 12):"));int birthdate=Integer.parseInt(JOptionPane.showInputDialog(null,"Enter the day "+ "you were born in:"));Constellation1(birthmonth,birthdate);}if (button == JOptionPane.CANCEL_OPTION){//Create the number and tell reader whether it is prime number:int guess=(int)(Math.random()*99+2);int guess1=(int)(Math.pow(guess,0.5));String judge="";for (int check=2; check<=guess1;check++){if(guess % check == 0)judge="The number you will guess is not a prime number";elsejudge="The number you will guess is a prime number";}JOptionPane.showMessageDialog(null,judge);//Prompt user to input the numberint user=Integer.parseInt(JOptionPane.showInputDialog(null,"Guess the number(2 to 100): "));do{if(user<guess)JOptionPane.showMessageDialog(null,"The number you guessed is too low, please try again!");if(user>guess)JOptionPane.showMessageDialog(null,"The number you guessed is too large, please try again!");u很简单的小游戏if(myhp>=0){JOptionPane.showMessageDialog(null,"Your enemy has attacked you this turn \nYour HP: " + myhp + ", \nYour enemy's HP: " + enemyhp + " \nDo you want to get into your turn?");}else{JOptionPane.showMessageDialog(null,"Your enemy has attacked you this turn \nYourHP: " + "0" + ", \nYour enemy's HP: " + enemyhp + " \nDo you want to get into your turn?"); break;}}else{myhp-=enemyattack*2;myap+=25;enap = 0;if(myhp>=0){JOptionPane.showMessageDialog(null,"Your enemy has attacked you "+ "critically this turn \nYour HP: " + myhp +", \nYour enemy's HP: " + enemyhp + " \nDo you want to get "+ "into your turn?");}else{JOptionPane.showMessageDialog(null,"Your enemy has attacked you "+ "critically this turn \nYour HP: " + "0" +", \nYour enemy's HP: " + enemyhp + " \nDo you want to get "+ "into your turn?");break;}}}if (enemyif == 1){enemyhp+=enemyheal;if(enemyhp >= 1000){enemyhp = 1000;}JOptionPane.showMessageDialog(null,"Your enemy has healed himself this turn \nyour HP: " + myhp + ", \nYour enemy's HP:" + enemyhp + " \nDo you want to get into your turn?");}}if (myhp <= 0){JOptionPane.showMessageDialog(null,"You die, Game Over");}if (enemyhp <= 0){JOptionPane.showMessageDialog(null,"Your enemy die, You are the winner!");}else{JOptionPane.showMessageDialog(null,"The Player Quit The Game");}}}if (button == JOptionPane.NO_OPTION){//Prompt user enter his or her birthday.int birthmonth=Integer.parseInt(JOptionPane.showInputDialog(null,"Enter the month "+ "you were born in(1 to 12):"));int birthdate=Integer.parseInt(JOptionPane.showInputDialog(null,"Enter the day "+ "you were born in:"));Constellation1(birthmonth,birthdate);}if (button == JOptionPane.CANCEL_OPTION){//Create the number and tell reader whether it is prime number:int guess=(int)(Math.random()*99+2);int guess1=(int)(Math.pow(guess,0.5));String judge="";for (int check=2; check<=guess1;check++){if(guess % check == 0)judge="The number you will guess is not a prime number";elsejudge="The number you will guess is a prime number";}JOptionPane.showMessageDialog(null,judge);//Prompt user to input the numberint user=Integer.parseInt(JOptionPane.showInputDialog(null,"Guess the number(2 to 100): "));do{if(user<guess)JOptionPane.showMessageDialog(null,"The number you guessed is too low, please try again!");if(user>guess)JOptionPane.showMessageDialog(null,"The number you guessed is too large, please try again!");U很简单的小游戏ser=Integer.parseInt(JOptionPane.showInputDialog(null,"Guess the number(2 to 100): "));}while(user != guess);JOptionPane.showMessageDialog(null,"You got the right answer!","Congratulations!",2);}}public static int Constellation1(int month, int date){if((month==3 && date>=21 && date<=31)||(month==4 && date>=1 && date<=19)) {JOptionPane.showMessageDialog(null,"You are Aries!");}else if((month==4 && date>=20 && date<=31)||(month==5 && date>=1 && date<=20)) {JOptionPane.showMessageDialog(null,"You are Taurus!");}else if((month==5 && date>=21 && date<=31)||(month==6 && date>=1 && date<=21)) {JOptionPane.showMessageDialog(null,"You are Gemini!");}else if((month==6 && date>=22 && date<=30)||(month==7 && date>=1 && date<=22)) {JOptionPane.showMessageDialog(null,"You are Cancer!");}else if((month==7 && date>=23 && date<=31)||(month==8 && date>=1 && date<=22)) {JOptionPane.showMessageDialog(null,"You are Leo!");}else if((month==9 && date>=1 && date<=22)||(month==8 && date>=23 && date<=31)) {JOptionPane.showMessageDialog(null,"You are Virgo!");}else if((month==9 && date>=23 && date<=30)||(month==10 && date>=1 && date<=22)) {JOptionPane.showMessageDialog(null,"You are Libra!");}else if((month==11 && date>=1 && date<=21)||(month==10 && date>=23 && date<=31)) {JOptionPane.showMessageDialog(null,"You are Scorpio!");}else if((month==11 && date>=22 && date<=30)||(month==12 && date>=1 && date<=21)) {JOptionPane.showMessageDialog(null,"You are Sagittarius!");}else if((month==1 && date>=1 && date<=19)||(month==12 && date>=22 && date<=31)) {JOptionPane.showMessageDialog(null,"You are Capricorn!");}else if((month==1 && date>=20 && date<=31)||(month==2 && date>=1 && date<=18)) {JOptionPane.showMessageDialog(null,"You are Aquarius!");}else{JOptionPane.showMessageDialog(null,"You are Pisces!");}return Constellation2(month,date);}public static int Constellation2(int month, int date){month=(int)(Math.random()*12+1);if(month==4 || month==6 || month==9 || month==11)date=(int)(Math.random()*31+1);else if(month==2)date=(int)(Math.random()*29+1);elsedate=(int)(Math.random()*30+1);String monthname="";switch(month){case 1:monthname="January";break;case 2:monthname="Feburary";break;case 3:monthname="March";break;case 4:monthname="April";break;case 5:monthname="May";break;case 6:monthname="June";break;case 7:monthname="July";break;case 8:monthname="August";break;case 9:monthname="September";break;case 10:monthname="October";break;case 11:monthname="November";break;default:monthname="December";break;}JOptionP很简单的小游戏ser=Integer.parseInt(JOptionPane.showInputDialog(null,"Guess the number(2 to 100): ")); }while(user != guess);JOptionPane.showMessageDialog(null,"You got the right answer!","Congratulations!",2);}}public static int Constellation1(int month, int date){if((month==3 && date>=21 && date<=31)||(month==4 && date>=1 && date<=19)) {JOptionPane.showMessageDialog(null,"You are Aries!");}else if((month==4 && date>=20 && date<=31)||(month==5 && date>=1 && date<=20)) {JOptionPane.showMessageDialog(null,"You are Taurus!");}else if((month==5 && date>=21 && date<=31)||(month==6 && date>=1 && date<=21)) {JOptionPane.showMessageDialog(null,"You are Gemini!");}else if((month==6 && date>=22 && date<=30)||(month==7 && date>=1 && date<=22)) {JOptionPane.showMessageDialog(null,"You are Cancer!");}else if((month==7 && date>=23 && date<=31)||(month==8 && date>=1 && date<=22)) {JOptionPane.showMessageDialog(null,"You are Leo!");}else if((month==9 && date>=1 && date<=22)||(month==8 && date>=23 && date<=31)) {JOptionPane.showMessageDialog(null,"You are Virgo!");}else if((month==9 && date>=23 && date<=30)||(month==10 && date>=1 && date<=22)) {JOptionPane.showMessageDialog(null,"You are Libra!");}else if((month==11 && date>=1 && date<=21)||(month==10 && date>=23 && date<=31)) {JOptionPane.showMessageDialog(null,"You are Scorpio!");}else if((month==11 && date>=22 && date<=30)||(month==12 && date>=1 && date<=21)) {JOptionPane.showMessageDialog(null,"You are Sagittarius!");}else if((month==1 && date>=1 && date<=19)||(month==12 && date>=22 && date<=31)) {JOptionPane.showMessageDialog(null,"You are Capricorn!");}else if((month==1 && date>=20 && date<=31)||(month==2 && date>=1 && date<=18)) {JOptionPane.showMessageDialog(null,"You are Aquarius!");}else{JOptionPane.showMessageDialog(null,"You are Pisces!");}return Constellation2(month,date);}public static int Constellation2(int month, int date){month=(int)(Math.random()*12+1);if(month==4 || month==6 || month==9 || month==11)date=(int)(Math.random()*31+1);else if(month==2)date=(int)(Math.random()*29+1);elsedate=(int)(Math.random()*30+1);String monthname="";switch(month){case 1:monthname="January"; break;case 2:monthname="Feburary"; break;case 3:monthname="March"; break;case 4:monthname="April"; break;case 5:monthname="May"; break;case 6:monthname="June"; break;case 7:monthname="July"; break;case 8:monthname="August"; break;case 9:monthname="September"; break;case 10:monthname="October";break;case 11:monthname="November"; break;default:monthname="December"; break;}JOptionPane.showMessageDialog(null, "You should find a fere whose birthday is "+date+ "th of "+monthname);System.exit(0);return Constellation1(month,date);}}。
java小游戏源代码

j a v a小游戏源代码 Document number:NOCG-YUNOO-BUYTT-UU986-1986UTJava小游戏第一个Java文件:import class GameA_B {public static void main(String[] args) {Scanner reader=new Scanner;int area;"Game Start…………Please enter the area:(1-9)" +'\n'+"1,2,3 means easy"+'\n'+"4,5,6 means middle"+'\n'+"7,8,9 means hard"+'\n'+"Please choose:");area=();switch((area-1)/3){case 0:"You choose easy! ");break;case 1:"You choose middle! ");break;case 2:"You choose hard! ");break;}"Good Luck!");GameProcess game1=new GameProcess(area);();}}第二个Java文件:import class GameProcess {int area,i,arrcount,right,midright,t;int base[]=new int[arrcount],userNum[]=newint[area],sysNum[]=new int[area];Random random=new Random();Scanner reader=new Scanner;GameProcess(int a){area=a;arrcount=10;right=0;midright=0;t=0;base=new int[arrcount];userNum=new int[area];sysNum=new int[area];for(int i=0;i<arrcount;i++){base[i]=i;// }}void process(){rand();while(right!=area){scanf();compare();print();check();}}void rand(){for(i=0;i<area;i++){t=(arrcount);//sysNum[i]=base[t];delarr(t);}}void delarr(int t){for(int j=t;j<arrcount-1;j++)base[j]=base[j+1];arrcount--;}void scanf(){"The system number has created!"+"\n"+"Please enter "+area+" Numbers");for(int i=0;i<area;i++){userNum[i]=();}}void check(){if(right==area)"You win…………!");}boolean check(int i){return true;}void compare(){int i=0,j=0;right=midright=0;for(i=0;i<area;i++){for(j=0;j<area;j++){if(userNum[i]==sysNum[j]){if(i==j)right++;elsemidright++;}}}}void print(){" A "+right+" B "+midright);}}。
JAVA小游戏源代码

Java 小游戏
public class GameA_B { public static void main(String[] args) { Scanner reader=new Scanner(System.in); int area; System.out.println("Game Start…………Please enter the area:(1-9)" + '\n'+"1,2,3 means easy"+'\n'+"4,5,6 means middle"+'\n'+ "7,8,9 means hard"+'\n'+"Please choose:"); area=reader.nextInt(); switch((area-1)/3) { case 0:System.out.println("You choose easy! ");break; case 1:System.out.println("You choose middle! ");break; case 2:System.out.println("You choose hard! ");break; } System.out.println("Good Luck!"); GameProcess game1=new GameProcess(area); game1.process(); }
Байду номын сангаас
新人Eclipse编写的Java小游戏

Eclipse编写的Java小游戏初学java时编写的简单小游戏,非界面版,纯java基础组成,新人都能看得懂可以做的来,主要是其中的逻辑思想。
使用eclipse编程,直接建立一个java 复制代码进去就可以了。
希望对大家学习java编程有点帮助游戏一:随即取5个数排列,要求猜出这5个数package day;import java.util.Random;import java.util.Scanner;import java.util.regex.Matcher;import java.util.regex.Pattern;public class Game {public static void main(String[] args) {Random r = new Random();int a1 = r.nextInt(10);int a2 = r.nextInt(10);int a3 = r.nextInt(10);int a4 = r.nextInt(10);int a5 = r.nextInt(10);System.out.println("游戏规则:随即取5个数,请输入5个数,数字顺序要求一致,会告诉你答对几个,看你能在几次猜对");Scanner scan = new Scanner(System.in);for(int i=1;;i++){int c=0;System.out.println("请输入5个数:");String dir = scan.nextLine();Pattern pattern = pile("^[0-9]{5}$");Matcher matcher = pattern.matcher(dir);if(matcher.find()){int b1 = Integer.parseInt(dir.substring(0,1));int b2 = Integer.parseInt(dir.substring(1,2));int b3 = Integer.parseInt(dir.substring(2,3));int b4 = Integer.parseInt(dir.substring(3,4));int b5 = Integer.parseInt(dir.substring(4,5));if(a1==b1){c++;}if(a2==b2){c++;}if(a3==b3){c++;}if(a4==b4){c++;}if(a5==b5){c++;}if(c==5){if(i<=5){System.out.println("恭喜你猜对了,猜对次数"+i+",妖才级别");break;}else if(i<=15){System.out.println("恭喜你猜对了,猜对次数"+i+",天才级别");break;}else if(i<=25){System.out.println("恭喜你猜对了,猜对次数"+i+",聪明人级别");break;}else if(i<=40){System.out.println("恭喜你猜对了,猜对次数"+i+",常人级别");break;}else if(i<=60){System.out.println("恭喜你猜对了,猜对次数"+i+",迟钝级别");break;}else{System.out.println("恭喜你猜对了,猜对次数"+i+",傻瓜级别");break;}}else{System.out.println("猜对了"+c+"个,请继续努力");}}else{System.out.println("只能输入5位数字,请重试");}}}}游戏二:package day;import java.util.Arrays;import java.util.Random;import java.util.Scanner;import java.util.regex.Matcher;import java.util.regex.Pattern;public class Game1 {public static void main(String[] args) {String[] str = {"鼠","牛","虎","兔","龙","蛇","马","羊","猴","鸡","狗","猪"};System.out.println("玩个小游戏,现在你手上有100分,从十二生肖中选择押注,只能压一个数目不限,随机选取5次");System.out.println("中1个翻1倍,2个翻3倍,3个翻6倍,4个翻10倍,5个翻15倍(输入如:龙5)。
用Java编程语言编写石头剪刀布游戏示例

用Java编程语言编写石头剪刀布游戏示例文章标题:用Java编程语言编写石头剪刀布游戏示例介绍内容:石头剪刀布游戏是一种简单而受欢迎的游戏,编程语言可以帮助我们实现一个可以和计算机进行对战的石头剪刀布游戏程序。
本文将介绍如何使用Java编程语言编写一个简单而有趣的石头剪刀布游戏示例。
首先,我们需要创建一个Java类,作为我们的游戏程序的主类。
接下来,我们可以使用Java的输入输出和随机数生成的类来实现游戏的逻辑。
下面是一个示例代码,用于实现一个石头剪刀布游戏:```javaimport java.util.Scanner;import java.util.Random;public class RockPaperScissorsGame {public static void main(String[] args) {Scanner scanner = new Scanner(System.in);Random random = new Random();String[] gestures = {"石头", "剪刀", "布"};System.out.println("欢迎来到石头剪刀布游戏!");while (true) {System.out.print("请输入你的选择(石头、剪刀、布):");String playerGesture = scanner.nextLine();if (!isValidGesture(playerGesture)) {System.out.println("无效的手势,请重新输入。
");continue;}int computerChoice = random.nextInt(3);String computerGesture = gestures[computerChoice];System.out.println("你的选择:" + playerGesture);System.out.println("电脑的选择:" + computerGesture);String result = calculateResult(playerGesture, computerGesture);System.out.println(result);System.out.print("是否要继续游戏?(是/否):");String continueChoice = scanner.nextLine();if (continueChoice.equalsIgnoreCase("否")) {break;}}System.out.println("谢谢你玩石头剪刀布游戏!");}private static boolean isValidGesture(String gesture) {return gesture.equals("石头") || gesture.equals("剪刀") || gesture.equals("布");}private static String calculateResult(String playerGesture, String computerGesture) {if (playerGesture.equals(computerGesture)) {return "平局!";} else if ((playerGesture.equals("石头") && computerGesture.equals("剪刀")) ||(playerGesture.equals("剪刀") && computerGesture.equals("布")) ||(playerGesture.equals("布") && computerGesture.equals("石头"))) {return "你赢了!";} else {return "你输了!";}}}```在上述Java代码中,我们首先使用Scanner类来接收玩家输入,使用Random类来生成电脑的随机选择。
java游戏代码

java游戏代码import java.awt.*;import java.awt.event.*;import javax.swing.*;public class JTetrix extends JFrame implements Runnable {private ImageIcon iconLogo;private JPanel nextPanel, scorePanel, opPanel, gamePanel, hidedOpPanel; private JLabel labLevel, labLine, labScore;private final JTextArea keyfocus; // 键盘的操作焦点,隐藏于游戏画面之后private JButton btnNew, btnPause, btnQuit;private showNextPiece nextPieceArea; // 预览下一方块private final gameOpBoard gameOpArea; // 游戏画面private Container c;private int Level, Score, LineRemoved; // 等级、得分、移除总行数private double interval; // 暂停时间,用于控制速度private boolean pause; // 是否暂停private Thread loopThread; // 游戏回圈执行绪static final long serialVersionUID = 1;public JTetrix() {c = getContentPane();//iconLogo = new ImageIcon(JTetrix.class.getResource("logo.jpg"));Level = 1;Score = 0;LineRemoved = 0;// 预览下一个方块的面版配置nextPanel = new JPanel();nextPanel.setLayout(null);nextPanel.setBorder(BorderFactory.createTitledBorder("下一个"));nextPanel.setLocation(10, 10);nextPanel.setSize(150, 120);nextPieceArea = new showNextPiece(5, 6, 15, 15);nextPieceArea.generateNextPiece(); // 先产生第一片待取nextPieceArea.setLocation(40, 20);nextPanel.add(nextPieceArea);// 等级、得分面版配置scorePanel = new JPanel();scorePanel.setBorder(BorderFactory.createTitledBorder("等级/ 得分")); scorePanel.setLocation(10, 140);scorePanel.setSize(150, 160);scorePanel.add(new JLabel(" 速度等级")); scorePanel.add(labLevel = new JLabel("1", SwingConstants.CENTER)); scorePanel.add(new JLabel(" 移除行数")); scorePanel.add(labLine = new JLabel("0", SwingConstants.CENTER)); scorePanel.add(new JLabel(" 目前得分")); scorePanel.add(labScore = new JLabel("0", SwingConstants.CENTER));// 功能面版配置opPanel = new JPanel();opPanel.setBorder(BorderFactory.createTitledBorder(""));opPanel.setLocation(10, 320);opPanel.setSize(150, 130);opPanel.add(btnNew = new JButton("开新游戏"));opPanel.add(btnPause = new JButton("暂停游戏"));opPanel.add(btnQuit = new JButton("关闭游戏"));// 游戏操作画面面版配置gameOpArea = new gameOpBoard(10, 22, 18, 18);gameOpArea.setLocation(20, 20);gameOpArea.setCurrentPiece(nextPieceArea.getNextTetrix()); // 先取出一个方块nextPieceArea.generateNextPiece(); // 预览窗格产生下一个方块预览gamePanel = new JPanel();gamePanel.setLayout(null);gamePanel.setLocation(180, 20);gamePanel.setSize(12 * 18, 24 * 18);gamePanel.setBorder(BorderFactory.createTitledBorder(""));gamePanel.add(gameOpArea);// 隐藏的操作面版配置hidedOpPanel = new JPanel(); hidedOpPanel.setLayout(new BorderLayout()); hidedOpPanel.setLocation(200, 40); hidedOpPanel.setSize(10 * 18, 22 * 18); keyfocus = new JTextArea();keyfocus.setEditable(false);hidedOpPanel.add(keyfocus);// 加入面版至视窗c.setLayout(null);c.add(nextPanel);c.add(scorePanel);c.add(opPanel);c.add(gamePanel);c.add(hidedOpPanel);// 键盘事件处理keyfocus.addKeyListener(new KeyAdapter() { public void keyPressed(KeyEvent e) {int key = e.getKeyCode();if (key == KeyEvent.VK_RIGHT) gameOpArea.moveRight();else if (key == KeyEvent.VK_LEFT) gameOpArea.moveLeft();else if (key == KeyEvent.VK_UP) gameOpArea.RotateRL(1);else if (key == KeyEvent.VK_DOWN) gameOpArea.RotateRL(0);else if (key == KeyEvent.VK_SPACE) { gameOpArea.soonMoveDown();}}});// 开新游戏btnNew.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { newGame();keyfocus.requestFocus();}});// 暂停游戏btnPause.addActionListener(new ActionListener() {public void actionPerformed(ActionEvent e) {pause = true;JOptionPane.showOptionDialog(null, "程式名称:\n JTetrix v0.1\n" + "程式设计:\n younganne\n"+ "简介:\n 一个用Java写的俄罗斯方块游戏\n", "关于JTetrix", JOptionPane.DEFAULT_OPTION,RMATION_MESSAGE, null, null, null);pause = false;interval = 2000 / Level;loopThread.interrupt();keyfocus.requestFocus();}});// 关闭游戏btnQuit.addActionListener(new ActionListener() {public void actionPerformed(ActionEvent e) {System.exit(0);}});// 启动游戏回圈执行绪loopThread = new Thread(this);loopThread.start();setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);setTitle("JTetrix v0.1");setSize(420, 500);setVisible(true);}// 游戏回圈的执行绪执行对象public void run() {while (true) { // 游戏回圈try {// 是否暂停if (pause)interval = 999999999;elseinterval = 2000 / Level;if (gameOpArea.isUpdated()) {// 由游戏画面的阵列是否更新来判断是否取出下一个gameOpArea.setCurrentPiece(nextPieceArea.getNextTetrix());nextPieceArea.generateNextPiece();// 更新等级、得分等资讯Score = gameOpArea.getScore();labScore.setText("" + Score);LineRemoved = gameOpArea.getLineRemoved();labLine.setText("" + LineRemoved);Level = (int) ((Score + 100) / 100); // 每一百分升级一次labLevel.setText("" + Level);}Thread.sleep((int) interval);gameOpArea.MoveDown(); // 不断下移} catch (InterruptedException e) {}}}// 开新游戏public void newGame() {Level = 1;Score = 0;LineRemoved = 0;labScore.setText("" + Score);labLine.setText("" + LineRemoved);labLevel.setText("" + Level);gameOpArea.setCurrentPiece(nextPieceArea.getNextTetrix()); // 先取出一个方块gameOpArea.newState();nextPieceArea.generateNextPiece(); // 预览窗格产生下一个方块预览}public static void main(String[] args) {JTetrix frm = new JTetrix();}}// 方块的资料结构与操作方法class TetrixPiece {private int pieceType; // 方块样式private int[][] coordinates = new int[4][2]; // 四个方块,记录X与Ypublic TetrixPiece() {initialize((int) (Math.random() * 7 + 1));}public TetrixPiece(int type) {initialize(type);}// 向左转动public void rotateLeft() {if (pieceType == 5) // 不转动正方形方块return;int tmp;for (int i = 0; i < 4; i++) {tmp = getXCoord(i);setXCoord(i, getYCoord(i));setYCoord(i, -tmp);}}// 向右转动public void rotateRight() {if (pieceType == 5) // 不转动正方形方块return;int tmp;for (int i = 0; i < 4; i++) {tmp = getXCoord(i);setXCoord(i, -getYCoord(i));setYCoord(i, tmp);}}import java.awt.*;import java.awt.event.*;import javax.swing.*;public class JTetrix extends JFrame implements Runnable {private ImageIcon iconLogo;private JPanel nextPanel, scorePanel, opPanel, gamePanel, hidedOpPanel; private JLabel labLevel, labLine, labScore;private final JTextArea keyfocus; // 键盘的操作焦点,隐藏于游戏画面之后private JButton btnNew, btnPause, btnQuit;private showNextPiece nextPieceArea; // 预览下一方块private final gameOpBoard gameOpArea; // 游戏画面private Container c;private int Level, Score, LineRemoved; // 等级、得分、移除总行数private double interval; // 暂停时间,用于控制速度private boolean pause; // 是否暂停private Thread loopThread; // 游戏回圈执行绪static final long serialVersionUID = 1;public JTetrix() {c = getContentPane();//iconLogo = new ImageIcon(JTetrix.class.getResource("logo.jpg"));Level = 1;Score = 0;LineRemoved = 0;// 预览下一个方块的面版配置nextPanel = new JPanel();nextPanel.setLayout(null);nextPanel.setBorder(BorderFactory.createTitledBorder("下一个"));nextPanel.setLocation(10, 10);nextPanel.setSize(150, 120);nextPieceArea = new showNextPiece(5, 6, 15, 15);nextPieceArea.generateNextPiece(); // 先产生第一片待取nextPieceArea.setLocation(40, 20);nextPanel.add(nextPieceArea);// 等级、得分面版配置scorePanel = new JPanel();scorePanel.setBorder(BorderFactory.createTitledBorder("等级/ 得分")); scorePanel.setLocation(10, 140);scorePanel.setSize(150, 160);scorePanel.add(new JLabel(" 速度等级")); scorePanel.add(labLevel = new JLabel("1", SwingConstants.CENTER)); scorePanel.add(new JLabel(" 移除行数")); scorePanel.add(labLine = new JLabel("0", SwingConstants.CENTER)); scorePanel.add(new JLabel(" 目前得分")); scorePanel.add(labScore = new JLabel("0", SwingConstants.CENTER));// 功能面版配置opPanel = new JPanel();opPanel.setBorder(BorderFactory.createTitledBorder(""));opPanel.setLocation(10, 320);opPanel.setSize(150, 130);opPanel.add(btnNew = new JButton("开新游戏"));opPanel.add(btnPause = new JButton("暂停游戏"));opPanel.add(btnQuit = new JButton("关闭游戏"));// 游戏操作画面面版配置gameOpArea = new gameOpBoard(10, 22, 18, 18);gameOpArea.setLocation(20, 20);gameOpArea.setCurrentPiece(nextPieceArea.getNextTetrix()); // 先取出一个方块nextPieceArea.generateNextPiece(); // 预览窗格产生下一个方块预览gamePanel = new JPanel();gamePanel.setLayout(null);gamePanel.setLocation(180, 20);gamePanel.setSize(12 * 18, 24 * 18);gamePanel.setBorder(BorderFactory.createTitledBorder(""));gamePanel.add(gameOpArea);// 隐藏的操作面版配置hidedOpPanel = new JPanel();hidedOpPanel.setLayout(new BorderLayout());hidedOpPanel.setLocation(200, 40);hidedOpPanel.setSize(10 * 18, 22 * 18);keyfocus = new JTextArea();keyfocus.setEditable(false);hidedOpPanel.add(keyfocus);// 加入面版至视窗c.setLayout(null);c.add(nextPanel);c.add(scorePanel);c.add(opPanel);c.add(gamePanel);c.add(hidedOpPanel);// 键盘事件处理keyfocus.addKeyListener(new KeyAdapter() { public void keyPressed(KeyEvent e) {int key = e.getKeyCode();if (key == KeyEvent.VK_RIGHT) gameOpArea.moveRight();else if (key == KeyEvent.VK_LEFT) gameOpArea.moveLeft();else if (key == KeyEvent.VK_UP) gameOpArea.RotateRL(1);else if (key == KeyEvent.VK_DOWN) gameOpArea.RotateRL(0);else if (key == KeyEvent.VK_SPACE) { gameOpArea.soonMoveDown();}}});// 开新游戏btnNew.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { newGame();keyfocus.requestFocus();}});// 暂停游戏btnPause.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { pause = true;JOptionPane.showOptionDialog(null, "程式名称:\n JTetrix v0.1\n"+ "程式设计:\n younganne\n"+ "简介:\n 一个用Java写的俄罗斯方块游戏\n", "关于JTetrix", JOptionPane.DEFAULT_OPTION,RMATION_MESSAGE, null, null, null);pause = false;interval = 2000 / Level;loopThread.interrupt();keyfocus.requestFocus();}});// 关闭游戏btnQuit.addActionListener(new ActionListener() {public void actionPerformed(ActionEvent e) {System.exit(0);}});// 启动游戏回圈执行绪loopThread = new Thread(this);loopThread.start();setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);setTitle("JTetrix v0.1");setSize(420, 500);setVisible(true);}// 游戏回圈的执行绪执行对象public void run() {while (true) { // 游戏回圈try {// 是否暂停if (pause)interval = 999999999;elseinterval = 2000 / Level;if (gameOpArea.isUpdated()) {// 由游戏画面的阵列是否更新来判断是否取出下一个gameOpArea.setCurrentPiece(nextPieceArea.getNextTetrix());nextPieceArea.generateNextPiece();// 更新等级、得分等资讯Score = gameOpArea.getScore();labScore.setText("" + Score);LineRemoved = gameOpArea.getLineRemoved();labLine.setText("" + LineRemoved);Level = (int) ((Score + 100) / 100); // 每一百分升级一次labLevel.setText("" + Level);}Thread.sleep((int) interval);gameOpArea.MoveDown(); // 不断下移} catch (InterruptedException e) {}}}// 开新游戏public void newGame() {Level = 1;Score = 0;LineRemoved = 0;labScore.setText("" + Score);labLine.setText("" + LineRemoved);labLevel.setText("" + Level);gameOpArea.setCurrentPiece(nextPieceArea.getNextTetrix()); // 先取出一个方块gameOpArea.newState();nextPieceArea.generateNextPiece(); // 预览窗格产生下一个方块预览}public static void main(String[] args) {JTetrix frm = new JTetrix();}}// 方块的资料结构与操作方法class TetrixPiece {private int pieceType; // 方块样式private int[][] coordinates = new int[4][2]; // 四个方块,记录X与Y public TetrixPiece() {initialize((int) (Math.random() * 7 + 1));}public TetrixPiece(int type) {initialize(type);}// 向左转动public void rotateLeft() {if (pieceType == 5) // 不转动正方形方块return;int tmp;for (int i = 0; i < 4; i++) {tmp = getXCoord(i);setXCoord(i, getYCoord(i));setYCoord(i, -tmp);}}// 向右转动public void rotateRight() {if (pieceType == 5) // 不转动正方形方块return;int tmp;for (int i = 0; i < 4; i++) {tmp = getXCoord(i);setXCoord(i, -getYCoord(i));setYCoord(i, tmp);}}// 可否移动指定位移?// xStep: 1 表向右xStep: -1 表向左xStep: 0 不移动X方向// yStep: 1 表向下yStep: 0 不移动Y方向public boolean canMoveTo(int xStep, int yStep) {int x, y;for (int i = 0; i < 4; i++) {x = currentPiece.getXCoord(i) + xOffset + xStep;y = currentPiece.getYCoord(i) + yOffset + yStep;// 是否超出边界if (x < 0 || x >= boardWidth || y >= boardHeight)return false;// 是否已经有方块if (gameBoard[x][y] != 0)return false;}return true;}// 向左移public void moveLeft() {if (canMoveTo(-1, 0))xOffset--;repaint();}// 向右移public void moveRight() {if (canMoveTo(1, 0))xOffset++;repaint();}// 向下移public void MoveDown() {if (canMoveTo(0, 1))yOffset++;repaint();}// 瞬间下移public void soonMoveDown() {while (canMoveTo(0, 1))yOffset++;score += 3;repaint();}// 转动方块// r: 0 表向左转r: 1 表示向右转public void RotateRL(int r) {TetrixPiece tmp = new TetrixPiece(currentPiece.getType());for (int i = 0; i < 4; i++) {tmp.setCoord(i, currentPiece.getXCoord(i), currentPiece.getYCoord(i));}if (r == 0)tmp.rotateLeft();elsetmp.rotateRight();// 测试是否可转动int x, y;for (int i = 0; i < 4; i++) {x = tmp.getXCoord(i) + xOffset;y = tmp.getYCoord(i) + yOffset;// 超出边界?if (x < 0 || x >= boardWidth || y >= boardHeight || y < 0) return;// 已经有方块?if (gameBoard[x][y] != 0)return;}currentPiece = tmp;repaint();}// 是否更新游戏画面阵列public boolean isUpdated() {if (gameover)return false;if (canMoveTo(0, 1))return false;int x, y;for (int i = 0; i < 4; i++) {x = currentPiece.getXCoord(i) + xOffset;y = currentPiece.getYCoord(i) + yOffset;gameBoard[x][y] = currentPiece.getType();if (y < nClearLines) {nClearLines = y;}}// 移除完整的行while (removeFullLines() != 0);repaint();return true;}// 移除完整的行public int removeFullLines() {int i, j, k;int nFullLines = 0; // 完整行的数目for (j = boardHeight - 1; j >= nClearLines; j--) {for (i = 0; i < boardWidth; i++) {if (gameBoard[i][j] == 0) // 不是完整的一行break;}if (i == boardWidth) { // 找到完整的一行nFullLines = 1;for (k = j - 1; k >= nClearLines; k--) { // 继续找完整行for (i = 0; i < boardWidth; i++)if (gameBoard[i][k] == 0) // 不是完整行break;if (i == boardWidth) { // 找到完整行nFullLines++;} else {for (i = 0; i < boardWidth; i++) { // 下移完整行上面的一行if (gameBoard[i][k + nFullLines] != gameBoard[i][k]) { gameBoard[i][k + nFullLines] = gameBoard[i][k];}}}}// 更新相关数据nClearLines = nClearLines + nFullLines;nLinesRemoved = nLinesRemoved + nFullLines; score = score + 10 * nFullLines;}}// 清除游戏区域阵列移动后的行资料for (j = 0; j < nClearLines; j++)for (i = 0; i < boardWidth; i++) gameBoard[i][j] = 0;return nFullLines;}// 目前得分public int getScore() {return score;}// 移除总行数public int getLineRemoved() {return nLinesRemoved;}// 绘图// 取得格子颜色public Color getGridColor(int type) {switch (type) {case 1:return Color.red;case 2:return Color.orange;case 3:return Color.yellow;case 4:return Color.green;case 5:return Color.blue;case 6:return Color.magenta;case 7:return Color.gray;}return Color.black;}// 画格子// isPiece: true 画格子isPiece: false 画堆积方块区public void drawGrid(Graphics g, int x, int y, boolean isPiece) {if (isPiece) // 这是方块g.setColor(getGridColor(currentPiece.getType()));else// 堆积的区域g.setColor(getGridColor(gameBoard[x][y]));g.fillRect(x * gridWidth, y * gridHeight, gridWidth, gridHeight);g.setColor(Color.lightGray);g.drawRect(x * gridWidth, y * gridHeight, gridWidth, gridHeight);}public void paint(Graphics g) {// 清除前一个画面g.setColor(Color.black);g.fillRect(0, 0, boardWidth * gridWidth, boardHeight * gridHeight);// 画方块for (int i = 0; i < 4; i++) {drawGrid(g, currentPiece.getXCoord(i) + xOffset, currentPiece.getYCoord(i)+ yOffset, true);}// 画堆积方块区for (int i = 0; i < boardWidth; i++) {for (int j = 0; j < boardHeight; j++) {if (gameBoard[i][j] == 0)continue;drawGrid(g, i, j, false);}}// 是否画出Game Over字样if (gameover) {g.setFont(new Font("细明体", Font.ITALIC | Font.BOLD, 26));g.setColor(Color.white);g.drawString("Game Over", 30, 50);g.setColor(Color.red);g.drawString("Game Over", 32, 52);}}}//old 998 lines//new 318 lines第一个类,服务器主线程类。
java好玩的简单代码

Java好玩的简单代码一、介绍Java作为一门广泛应用于软件开发的编程语言,拥有着丰富的功能和强大的生态系统。
除了应用于复杂的企业级应用开发,Java也可以用来编写一些好玩的简单代码,让我们在编程的过程中感受到乐趣和创造力的发挥。
本文将介绍一些有趣的Java代码示例,帮助读者了解Java的一些有趣特性和编程技巧。
二、Java代码示例2.1 Hello Worldpublic class HelloWorld {public static void main(String[] args) {System.out.println("Hello World!");}}这是Java程序员入门必学的第一个示例代码。
通过这段代码,我们可以看到Java 的基本结构和语法。
运行这段代码后,控制台将输出”Hello World!“。
这简单的一行代码,展示了Java的输出功能。
2.2 计算器import java.util.Scanner;public class Calculator {public static void main(String[] args) {Scanner scanner = new Scanner(System.in);System.out.print("请输入第一个数字: ");int num1 = scanner.nextInt();System.out.print("请输入第二个数字: ");int num2 = scanner.nextInt();System.out.println("请选择操作符 (+, -, *, /): ");String operator = scanner.next();int result = 0;switch (operator) {case "+":result = num1 + num2;break;case "-":result = num1 - num2;break;case "*":result = num1 * num2;break;case "/":result = num1 / num2;break;default:System.out.println("无效的操作符!");return;}System.out.println("计算结果: " + result);}}这是一个简单的计算器示例代码。
java简易小游戏制作代码

java简易⼩游戏制作代码java简易⼩游戏制作游戏思路:设置⼈物移动,游戏规则,积分系统,随机移动的怪物,游戏胜负判定,定时器。
游戏内容部分package 代码部分;import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import java.awt.event.KeyEvent;import java.awt.event.KeyListener;import java.util.Random;public class TestGamePanel extends JPanel implements KeyListener, ActionListener {//初始化⼈物坐标int p1X;int p1Y;int p2X;int p2Y;boolean isStart = false; //游戏是否开始boolean p1isFail = false; //游戏是否失败boolean p2isFail = false;String fx1; //左:L,右:R,上:U,下:DString fx2;Timer timer = new Timer(50,this);//定时器//积分int p1score = 0;int p2score = 0;//苹果int AppleX;int AppleY;//怪物int monster1X;int monster1Y;int monster2X;int monster2Y;int monster3X;int monster3Y;int monster4X;int monster4Y;int monster5X;int monster5Y;//随机积分Random random = new Random();public TestGamePanel() {init();this.setFocusable(true);this.addKeyListener(this);timer.start();}//初始化public void init() {p1X = 25;p1Y = 150;p2X = 700;p2Y = 550;fx1 = "L";fx2 = "R";monster1X = 25*random.nextInt(28);monster1Y = 100 + 25*random.nextInt(18);monster2X = 25*random.nextInt(28);monster2Y = 100 + 25*random.nextInt(18);monster3X = 25*random.nextInt(28);monster3Y = 100 + 25*random.nextInt(18);monster4X = 25*random.nextInt(28);monster4Y = 100 + 25*random.nextInt(18);monster5X = 25*random.nextInt(28);monster5Y = 100 + 25*random.nextInt(18);AppleX = 25*random.nextInt(28);AppleY = 100 + 25*random.nextInt(18);add(kaishi);add(chongkai);guize.addActionListener(new ActionListener() {@Overridepublic void actionPerformed(ActionEvent e) {new TestGameRule();}});}//游戏功能按钮JButton kaishi = new JButton("开始");JButton chongkai = new JButton("重新开始");JButton guize = new JButton("游戏规则");//画板@Overrideprotected void paintComponent(Graphics g) {super.paintComponent(g);TestGameData.header.paintIcon(this,g,0,0);g.setColor(Color.CYAN);g.fillRect(0,100,780,520);//画⼈物TestGameData.p1player1.paintIcon(this,g,p1X,p1Y);TestGameData.p2player1.paintIcon(this,g,p2X,p2Y);//画得分g.setFont(new Font("华⽂彩云",Font.BOLD,18)); //设置字体g.setColor(Color.RED);g.drawString("玩家1:" + p1score,20,20 );g.drawString("玩家2:" + p2score,680,20);//画苹果TestGameData.apple.paintIcon(this,g,AppleX,AppleY);//画静态怪物TestGameData.monster.paintIcon(this,g,monster1X,monster1Y);TestGameData.monster.paintIcon(this,g,monster2X,monster2Y);TestGameData.monster.paintIcon(this,g,monster3X,monster3Y);TestGameData.monster.paintIcon(this,g,monster4X,monster4Y);TestGameData.monster.paintIcon(this,g,monster5X,monster5Y);//游戏提⽰,是否开始if(!isStart) {g.setColor(Color.BLACK);g.setFont(new Font("华⽂彩云",Font.BOLD,30));g.drawString("请点击开始游戏",300,300);}//游戏结束提⽰,是否重新开始if(p2isFail || p1score == 15) {g.setColor(Color.RED);g.setFont(new Font("华⽂彩云",Font.BOLD,30));g.drawString("玩家⼀获胜,请点击重新开始游戏",200,300);if(p1isFail || p2score == 15) {g.setColor(Color.RED);g.setFont(new Font("华⽂彩云",Font.BOLD,30));g.drawString("玩家⼆获胜,请点击重新开始游戏",200,300); }}//键盘监听事件@Overridepublic void keyPressed(KeyEvent e) {//控制⼈物⾛动//玩家1if(isStart == true && (p1isFail == false && p2isFail == false)) { if(e.getKeyCode() == KeyEvent.VK_D) {fx1 = "R";p1X += 25;if(p1X >= 750) {p1X = 750;}}else if(e.getKeyCode() == KeyEvent.VK_A) {fx1 = "L";p1X -= 25;if(p1X <= 0) {p1X = 0;}}else if(e.getKeyCode() == KeyEvent.VK_W) {fx1 = "U";p1Y -= 25;if(p1Y <= 100) {p1Y = 100;}}else if(e.getKeyCode() == KeyEvent.VK_S) {fx1 = "D";p1Y += 25;if(p1Y >= 600) {p1Y = 600;}}//玩家2if(e.getKeyCode() == KeyEvent.VK_RIGHT) {fx2 = "R";p2X += 25;if(p2X >= 750) {p2X = 750;}}else if(e.getKeyCode() == KeyEvent.VK_LEFT) {fx2 = "L";p2X -= 25;if(p2X <= 0) {p2X = 0;}}else if(e.getKeyCode() == KeyEvent.VK_UP) {fx2 = "U";p2Y -= 25;if(p2Y <= 100) {p2Y = 100;}}else if(e.getKeyCode() == KeyEvent.VK_DOWN) {fx2 = "D";p2Y += 25;if(p2Y >= 600) {p2Y = 600;}}}repaint();}@Overridepublic void actionPerformed(ActionEvent e) {kaishi.addActionListener(new ActionListener() {@Overridepublic void actionPerformed(ActionEvent e) {isStart = true;}});chongkai.addActionListener(new ActionListener() {@Overridepublic void actionPerformed(ActionEvent e) {if(p1isFail) { p1isFail = !p1isFail; init(); }if(p2isFail) { p2isFail = !p2isFail; init(); }}});add(kaishi);add(chongkai);add(guize);if(isStart == true && (p1isFail == false && p2isFail == false)) { //让⼈动起来if(fx1.equals("R")) {p1X += 25;if(p1X >= 750) { p1X = 750; }}if(fx1.equals("L")) {p1X -= 25;if(p1X <= 0) { p1X = 0; }}if(fx1.equals("U")) {p1Y -= 25;if(p1Y <= 100) { p1Y = 100; }}if(fx1.equals("D")) {p1Y += 25;if(p1Y >= 600) { p1Y = 600; }}if(fx2.equals("R")) {p2X += 25;if(p2X >= 750) { p2X = 750; }}if(fx2.equals("L")) {p2X -= 25;if(p2X <= 0) { p2X = 0; }}if(fx2.equals("U")) {p2Y -= 25;if(p2Y <= 100) { p2Y = 100; }}if(fx2.equals("D")) {p2Y += 25;if(p2Y >= 600) { p2Y = 600; }}//让怪物动起来//怪物1int i = random.nextInt(4) + 1;if(i == 1) {monster1X += 5;if(monster1X >= 750) {monster1X = 750;}}if(i == 2) {monster1X -= 5;if(monster1X <= 0) {monster1X = 0;}}if(i == 3) {monster1Y += 5;if(monster1Y >= 600) {monster1Y = 600;}if(i == 4) {monster1Y -= 5;if(monster1Y <= 100) {monster1Y = 100;}}//怪物2int j = random.nextInt(4) + 1;if(j == 1) {monster2X += 5;if(monster2X >= 750) {monster2X = 750;}}if(j == 2) {monster2X -= 5;if(monster2X <= 0) {monster2X = 0;}}if(j == 3) {monster2Y += 5;if(monster2Y >= 600) {monster2Y = 600;}}if(j == 4) {monster2Y -= 5;if(monster2Y <= 100) {monster2Y = 100;}}//怪物3int k = random.nextInt(4) + 1;if(k == 1) {monster3X += 5;if(monster3X >= 750) {monster3X = 750;}}if(k == 2) {monster3X -= 5;if(monster3X <= 0) {monster3X = 0;}}if(k == 3) {monster3Y += 5;if(monster3Y >= 600) {monster3Y = 600;}}if(k == 4) {monster3Y -= 5;if(monster3Y <= 100) {monster3Y = 100;}}//怪物4int n= random.nextInt(4) + 1;if(n == 1) {monster4X += 5;if(monster4X >= 750) {monster4X = 750;}}if(n == 2) {monster4X -= 5;if(monster4X <= 0) {monster4X = 0;}}if(n == 3) {monster4Y += 5;if(monster4Y >= 600) {monster4Y = 600;}}if(n == 4) {monster4Y -= 5;if(monster4Y <= 100) {monster4Y = 100;}}//怪物5int m = random.nextInt(4) + 1;if(m == 1) {monster5X += 5;if(monster5X >= 750) {monster5X = 750;}}if(m == 2) {monster5X -= 5;if(monster5X <= 0) {monster5X = 0;}}if(m == 3) {monster5Y += 5;if(monster5Y >= 600) {monster5Y = 600;}}if(m == 4) {monster5Y -= 5;if(monster5Y <= 100) {monster5Y = 100;}}//如果有玩家吃到⾷物if(p1X == AppleX && p1Y == AppleY) {p1score++;AppleX = 25*random.nextInt(28);AppleY = 100 + 25*random.nextInt(18);} else if(p2X == AppleX && p2Y == AppleY) {p2score++;AppleX = 25*random.nextInt(28);AppleY = 100 + 25*random.nextInt(18);}//如果有玩家碰到怪物,判定死亡,游戏结束后续有修改,暂⽤ //怪物1死亡if(p1X >= monster1X -25 && p1X <= monster1X +25) {if(p1Y == monster1Y) { p1isFail = !p1isFail; p1score = p2score = 0;} }if(p1Y >= monster1Y -25 && p1Y <= monster1Y +25) {if(p1X == monster1X) { p1isFail = !p1isFail; p1score = p2score = 0;} }if(p2X >= monster1X -25 && p2X <= monster1X +25) {if(p2Y == monster1Y) { p2isFail = !p2isFail; p1score = p2score = 0;} }if(p2Y >= monster1Y -25 && p2Y <= monster1Y +25) {if(p2X == monster1X) { p2isFail = !p2isFail; p1score = p2score = 0;} }//怪物2死亡if(p1X >= monster2X -25 && p1X <= monster2X +25) {if(p1Y == monster2Y) { p1isFail = !p1isFail; p1score = p2score = 0;} }if(p1Y >= monster2Y -25 && p1Y <= monster2Y +25) {if(p1X == monster2X) { p1isFail = !p1isFail; p1score = p2score = 0;} }if(p2X >= monster2X -25 && p2X <= monster2X +25) {if(p2Y == monster2Y) { p2isFail = !p2isFail; p1score = p2score = 0;} }if(p2Y >= monster2Y -25 && p2Y <= monster2Y +25) {if(p2X == monster2X) { p2isFail = !p2isFail; p1score = p2score = 0;} }//怪物3死亡if(p1X >= monster3X -25 && p1X <= monster3X +25) {if(p1Y == monster3Y) { p1isFail = !p1isFail; p1score = p2score = 0;} }if(p1Y >= monster3Y -25 && p1Y <= monster3Y +25) {if(p1X == monster3X) { p1isFail = !p1isFail; p1score = p2score = 0;} }if(p2X >= monster3X -25 && p2X <= monster3X +25) {if(p2Y == monster3Y) { p2isFail = !p2isFail; p1score = p2score = 0;}if(p2Y >= monster3Y -25 && p2Y <= monster3Y +25) {if(p2X == monster3X) { p2isFail = !p2isFail; p1score = p2score = 0;}}//怪物4死亡if(p1X >= monster4X -25 && p1X <= monster4X +25) {if(p1Y == monster4Y) { p1isFail = !p1isFail; p1score = p2score = 0;}}if(p1Y >= monster4Y -25 && p1Y <= monster4Y +25) {if(p1X == monster1X) { p1isFail = !p1isFail; p1score = p2score = 0;}}if(p2X >= monster4X -25 && p2X <= monster4X +25) {if(p2Y == monster4Y) { p2isFail = !p2isFail; p1score = p2score = 0;}}if(p2Y >= monster4Y -25 && p2Y <= monster4Y +25) {if(p2X == monster4X) { p2isFail = !p2isFail; p1score = p2score = 0;}}//怪物5死亡if(p1X >= monster5X -25 && p1X <= monster5X +25) {if(p1Y == monster5Y) { p1isFail = !p1isFail; p1score = p2score = 0;}}if(p1Y >= monster5Y -25 && p1Y <= monster5Y +25) {if(p1X == monster5X) { p1isFail = !p1isFail; p1score = p2score = 0;}}if(p2X >= monster5X -25 && p2X <= monster5X +25) {if(p2Y == monster5Y) { p2isFail = !p2isFail; p1score = p2score = 0;}}if(p2Y >= monster5Y -25 && p2Y <= monster5Y+25) {if(p2X == monster5X) { p2isFail = !p2isFail; p1score = p2score = 0;}}//如果有玩家达到指定积分,判定获胜,游戏结束if(p1score == 15) { p2isFail = !p2isFail; }if(p2score == 15) { p1isFail = !p1isFail; }repaint();}timer.start();}@Overridepublic void keyTyped(KeyEvent e) {}@Overridepublic void keyReleased(KeyEvent e) {}}游戏规则(使⽤弹窗)部分package 代码部分;import javax.swing.*;import java.awt.*;public class TestGameRule extends JDialog {private int num = 1;public TestGameRule() {TextArea textArea = new TextArea(20,10);textArea.setText("游戏中有五个怪物随机移动,碰到怪物算死亡\\\n游戏中有随机出现的苹果,碰到⼀个苹果加⼀分,\\\n先达到⼗五分或者对⼿死亡算游戏胜利!"); JScrollPane jScrollPane = new JScrollPane(textArea);this.add(jScrollPane);this.setBounds(200,200,400,400);this.setVisible(true);textArea.setEditable(false);setResizable(false);textArea.setBackground(Color.PINK);}}图⽚素材package 代码部分;import javax.swing.*;import .URL;public class TestGameData {public static URL headerurl = TestGameData.class.getResource("/图⽚素材/header.jpg");public static URL p1player1url = TestGameData.class.getResource("/图⽚素材/1.jpg");public static URL p2player2url = TestGameData.class.getResource("/图⽚素材/2.jpg");public static URL appleurl = TestGameData.class.getResource("/图⽚素材/apple.jpg");public static URL monsterurl = TestGameData.class.getResource("/图⽚素材/monster.jpg");public static ImageIcon p1player1 = new ImageIcon(p1player1url);public static ImageIcon p2player1 = new ImageIcon(p2player2url);public static ImageIcon header = new ImageIcon(headerurl);public static ImageIcon apple = new ImageIcon(appleurl);public static ImageIcon monster = new ImageIcon(monsterurl);}主函数package 代码部分;import javax.swing.*;public class TestStartGame {public static void main(String[] args) {//制作窗⼝JFrame jFrame = new JFrame("2D对战⼩游戏");jFrame.setBounds(10,10,790,660);jFrame.setResizable(false);jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//添加游戏⾯板jFrame.add(new TestGamePanel());//设置可见jFrame.setVisible(true);}}实现效果到此这篇关于java简易⼩游戏制作代码的⽂章就介绍到这了,更多相关java简易⼩游戏内容请搜索以前的⽂章或继续浏览下⾯的相关⽂章希望⼤家以后多多⽀持!。
java小游戏源代码
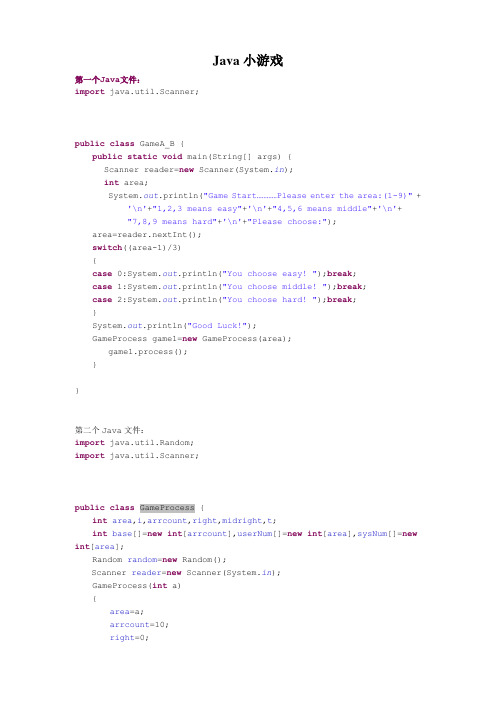
Java小游戏第一个Java文件:import java.util.Scanner;public class GameA_B {public static void main(String[] args) {Scanner reader=new Scanner(System.in);int area;System.out.println("Game Start…………Please enter the area:(1-9)"+ '\n'+"1,2,3 means easy"+'\n'+"4,5,6 means middle"+'\n'+"7,8,9 means hard"+'\n'+"Please choose:");a rea=reader.nextInt();s witch((area-1)/3){c ase 0:System.out.println("You choose easy! ");break;c ase 1:System.out.println("You choose middle! ");break;c ase 2:System.out.println("You choose hard! ");break;}S ystem.out.println("Good Luck!");G ameProcess game1=new GameProcess(area);game1.process();}}第二个Java文件:import java.util.Random;import java.util.Scanner;public class GameProcess {int area,i,arrcount,right,midright,t;int base[]=new int[arrcount],userNum[]=new int[area],sysNum[]=new int[area];Random random=new Random();Scanner reader=new Scanner(System.in);GameProcess(int a){area=a;arrcount=10;midright=0;t=0;base=new int[arrcount];userNum=new int[area];sysNum=new int[area];for(int i=0;i<arrcount;i++){base[i]=i;//System.out.println(base[i]);}}void process(){r and();w hile(right!=area){s canf();c ompare();p rint();c heck();}}void rand(){f or(i=0;i<area;i++){t=random.nextInt(arrcount);//System.out.println(t);sysNum[i]=base[t];System.out.println(base[t]);delarr(t);}}void delarr(int t){f or(int j=t;j<arrcount-1;j++)base[j]=base[j+1];a rrcount--;}void scanf(){S ystem.out.println("The system number has created!"+"\n"+"Please enter "+area+" Numbers");{userNum[i]=reader.nextShort();}}void check(){if(right==area)S ystem.out.println("You win…………!");}boolean check(int i){r eturn true;}void compare(){i nt i=0,j=0;r ight=midright=0;f or(i=0;i<area;i++){for(j=0;j<area;j++){if(userNum[i]==sysNum[j]){if(i==j)right++;elsemidright++;}}}}void print(){S ystem.out.println(" A "+right+" B "+midright); }}import java.awt.*;import java.awt.event.*;import javax.swing.*;class TestGame {public static void main(String[] args) {App ap = new App(); //调用App()开始运行程序ap.show();}}class App extends JFrame {MainPanel mp;public App() {mp = new MainPanel();this.getContentPane().add(mp);this.setSize(400, 450);this.setTitle("小游戏");}}/*** 主面板* 显示格子* @author Administrator**/class MainPanel extends JPanel {ButtonPanel bp = new ButtonPanel();CtrlPanel rp = new CtrlPanel();public MainPanel() {this.setLayout(new BorderLayout());rp.btnstart.addActionListener(new StartListener()); this.add(bp, "Center");this.add(rp, "South");class StartListener implements ActionListener {/*** 重新开始按钮的事件* 调用按钮面板里面的颜色初始化方法*/public void actionPerformed(ActionEvent e) {if (e.getActionCommand() == "重新开始") {bp.ColorInit();}}}}class ButtonPanel extends JPanel {JButton[][] b = new JButton[5][5];/*** 按钮界面的构造器* 设置布局方式为Grid布局,并生成5*5的格子,* 在每个格子生成一个按钮,* 为每个按钮添加一个监听事件*/public ButtonPanel() {this.setLayout(new GridLayout(5, 5));for (int i = 0; i < 5; i++) {for (int j = 0; j < 5; j++) {b[i][j] = new JButton();b[i][j].setActionCommand("" + (i + 1) + (j + 1));b[i][j].addActionListener(new MyButtonListener());this.add(b[i][j]);}}this.ColorInit();}* 面板初始化时候给所有的格子都绘上深灰色* i.j分别是行和列*/public void ColorInit() {for (int i = 0; i < 5; i++) {for (int j = 0; j < 5; j++) {b[i][j].setBackground(Color.DARK_GRAY);}}}/*** 按钮上监听的时事件,监听点击* @author Administrator**/class MyButtonListener implements ActionListener { int r, c;/*** 需要改变颜色的行和列* r row* c colunm* 调用change()来改变颜色*/public void actionPerformed(ActionEvent e) {int i = Integer.parseInt(e.getActionCommand()); r = i / 10 - 1;c = i % 10 - 1;this.changer();}/*** 传一个按钮控件进去* 判断颜色,如果是深灰则变为粉红* 否则义相反* @param b*/if (b.getBackground() == Color.DARK_GRAY) {b.setBackground(Color.pink);} else {b.setBackground(Color.DARK_GRAY);}}/*** 这个方法是根据点击的按钮判断周围需要* 不能超越数组的下标*/public void changer() {this.btnChange(b[r][c]);if (r > 0) //行号大于0this.btnChange(b[r - 1][c]);if (r < 4)this.btnChange(b[r + 1][c]);if (c > 0)//列号大于0this.btnChange(b[r][c - 1]);if (c < 4)//列好小余0this.btnChange(b[r][c + 1]);}}}/*** 控制面板* @author Administrator*下面的开始按钮*/class CtrlPanel extends JPanel {JButton btnstart;public CtrlPanel() {btnstart = new JButton("重新开始");this.add(btnstart);}}import java.util.*;public class Cai {enum Res{SHITOU, JIANZI, BU};Res res;public static void main(String[] args) throws Exception { // TODO Auto-generated method stubCai cai = new Cai();System.out.println("请输入你的选择:");System.out.println("0表示石头,1表示剪子,2表示布"); char yourResultOfChar =(char) System.in.read();int yourResultOfInt = yourResultOfChar - '0';int computerResult = pb();cai.getYourResult(yourResultOfInt);switch (computerResult){case 0:System.out.println("电脑选择石头");break;case 1:System.out.println("电脑选择剪子");break;case 2:System.out.println("电脑选择布");break;}cai.pa(computerResult);}public void getYourResult(int count){res = result[count];}void pa(int computer){Res[] result = Res.values();if(this.res == Res.SHITOU){System.out.println("我选择石头"); switch(result[computer]){case SHITOU:System.out.println("平局,再来!");break;case JIANZI:System.out.println("我赢了!");break;case BU:System.out.println("我输了!");break;}} else if(this.res == Res.JIANZI) {System.out.println("我选择剪子"); switch(result[computer]){case JIANZI:System.out.println("平局,再来!");break;case BU:System.out.println("我赢了!");break;case SHITOU:System.out.println("我输了!");break;}} else if(this.res == Res.BU){System.out.println("我选择布");switch(result[computer]){case BU:System.out.println("平局,再来!");break;case SHITOU:System.out.println("我赢了!");break;case JIANZI:System.out.println("我输了!");break;}}}static int pb(){Random ran = new Random();int res = ran.nextInt(3);return res;//输出0-2的整数,0表示石头,1表示剪子,2表示布,和enum Res中的顺序相对应}}import java.util.*; //导入实用包util下所有的类import javax.swing.*;import java.awt.*;import java.awt.event.*;public class CaiShu {public static void main(String[] args) {Win f = new Win();f.setVisible(true);}}class Win extends JFrame implements ActionListener {JLabel labe;JButton butt;JButton button;Random a = new Random();private int i = 0;private int num;JTextField text1, text2;JPanel p;public Win() {super("猜数游戏");labe = new JLabel("我心里有个数,它是1---100之间的,你能猜出来吗?"); butt = new JButton("确认");button = new JButton("重开");text1 = new JTextField(5);text2 = new JTextField(20);p = new JPanel();Container con = getContentPane();// 调用JFrame的getContentPane得到容器text2.setEditable(false);// 使输出结果文本域不可编辑butt.addActionListener(this);// 执行结果动作con.setLayout(new GridLayout(4, 1));// 设置整个界面的长宽比p.add(text1);// 添加输入数字文本域p.add(butt);p.add(button);button.addActionListener(new ActionListener(){ public void actionPerformed(ActionEvent e) { text1.setText("");text2.setText("");i=0;}});con.add(labe);// 添加游戏标签con.add(p);con.add(text2);// 添加输出结果信息文本域setSize(300, 300);// 设置窗口尺寸setVisible(true);// 设置窗口可视pack();addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent e) { setVisible(false);System.exit(0);}});}public void actionPerformed(ActionEvent e) {int shu;while (true) {shu = Integer.parseInt(text1.getText());if (i == 0) {num = a.nextInt(100);}i++;if (i == 10) {text2.setText("结束吧,你没有希望了!!");i = 0;break;}if (e.getSource() == butt) {if (shu > num) {text2.setText("输入的数大了,输小点的!");} else if (shu < num) {text2.setText("输入的数小了,输大点的!");} else if (shu == num) {text2.setText("恭喜你,猜对了!");if (i <= 2)text2.setText("你真是个天才!");else if (i <= 6)text2.setText("还将就,你过关了!");else if (i <= 8)text2.setText("但是你还……真笨!");elsetext2.setText("你实在是太笨了!");}break;}}}}。
学习Java编程使用示例代码制作饥慌控制台游戏

学习Java编程使用示例代码制作饥慌控制台游戏import java.util.Scanner;public class HungerGame {public static void main(String[] args) {System.out.println("Welcome to the Hunger Game! Are you ready to start? (yes/no)");Scanner scanner = new Scanner(System.in);String response = scanner.nextLine();if(response.equalsIgnoreCase("yes")) {System.out.println("Let's begin the game!\n");startGame();} else {System.out.println("Come back when you are ready to play!");}}public static void startGame() {System.out.println("You wake up in a dark forest, feeling hungry and disoriented. You have no idea how you got here.");System.out.println("You see a sign that says 'Find food before hunger takes over!'");System.out.println("You have three options: \n1. Look for berries in the bushes \n2. Hunt for animals \n3. Go fishing in the nearby river");System.out.println("Enter the number corresponding to your choice:");Scanner scanner = new Scanner(System.in);int choice = scanner.nextInt();switch(choice) {case 1:System.out.println("You decide to look for berries in the bushes.");findBerries();break;case 2:System.out.println("You decide to hunt for animals.");huntAnimals();break;case 3:System.out.println("You decide to go fishing in the nearby river.");goFishing();break;default:System.out.println("Invalid choice. Please try again.");startGame();break;}}public static void findBerries() {System.out.println("You start searching for berries in the bushes. After a while, you find a bush full of ripe berries.");System.out.println("You carefully pick the berries and eat them. They taste delicious!");System.out.println("Your hunger level decreases, but you need more food to survive.");System.out.println("Do you want to continue searching for more berries? (yes/no)");Scanner scanner = new Scanner(System.in);String response = scanner.nextLine();if(response.equalsIgnoreCase("yes")) {System.out.println("You continue searching for more berries.");findBerries();} else {System.out.println("You decide to stop searching for berries and look for other sources of food.");startGame();}}public static void huntAnimals() {System.out.println("You set off into the forest to hunt for animals. After a while, you come across a wild boar.");System.out.println("You take aim with your bow and arrow, and manage to hit the boar.");System.out.println("You successfully kill the boar and gather the meat.");System.out.println("Your hunger level decreases significantly, but you need more food to sustain yourself.");System.out.println("Do you want to continue hunting for more animals? (yes/no)");Scanner scanner = new Scanner(System.in);String response = scanner.nextLine();if(response.equalsIgnoreCase("yes")) {System.out.println("You continue hunting for more animals.");huntAnimals();} else {System.out.println("You decide to stop hunting and look for other sources of food.");startGame();}}public static void goFishing() {System.out.println("You make your way to the nearby river to go fishing. You find a suitable spot and cast your fishing line.");System.out.println("After a while, you feel a tug on your line. You reel it in and find a big, fresh fish.");System.out.println("You cook the fish over a campfire and enjoy a satisfying meal.");System.out.println("Your hunger level decreases significantly, but you need more food to survive.");System.out.println("Do you want to continue fishing? (yes/no)");Scanner scanner = new Scanner(System.in);String response = scanner.nextLine();if(response.equalsIgnoreCase("yes")) {System.out.println("You continue fishing.");goFishing();} else {System.out.println("You decide to stop fishing and look for other sources of food.");startGame();}}}通过这个示例代码,你可以了解到如何使用Java编写一个简单的控制台游戏。
Java示例代码创建一个简单的饥慌控制台游戏
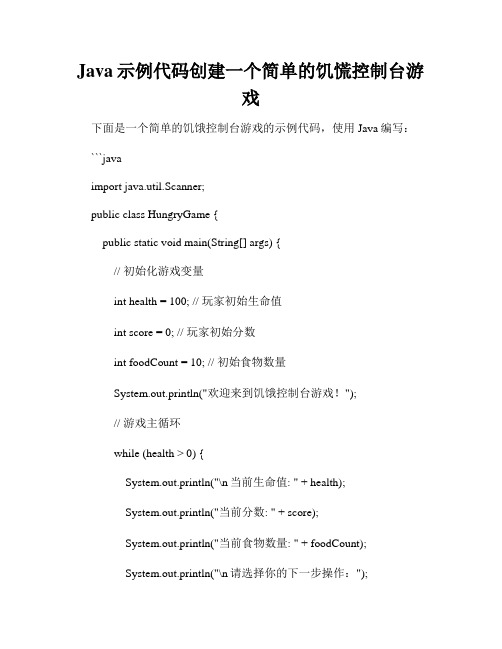
Java示例代码创建一个简单的饥慌控制台游戏下面是一个简单的饥饿控制台游戏的示例代码,使用Java编写:```javaimport java.util.Scanner;public class HungryGame {public static void main(String[] args) {// 初始化游戏变量int health = 100; // 玩家初始生命值int score = 0; // 玩家初始分数int foodCount = 10; // 初始食物数量System.out.println("欢迎来到饥饿控制台游戏!");// 游戏主循环while (health > 0) {System.out.println("\n当前生命值: " + health);System.out.println("当前分数: " + score);System.out.println("当前食物数量: " + foodCount);System.out.println("\n请选择你的下一步操作:");System.out.println("1. 进食");System.out.println("2. 锻炼");System.out.println("3. 休息");System.out.println("4. 退出游戏");// 获取玩家输入Scanner scanner = new Scanner(System.in);int choice = scanner.nextInt();// 根据玩家选择进行相应操作switch (choice) {case 1:if (foodCount > 0) {health += 10;score += 5;foodCount--;System.out.println("进食成功!生命值+10,分数+5"); } else {System.out.println("食物不足,请选择其他操作。
Java模板游戏代码

魔板游戏代码public class PuzzleFrame extends JFrame implements ActionListener {PuzzlePad puzzlePad;JButton 开始;JMenuBar bar;JMenu fileMenu;JMenuItem 初级,高级;JRadioButton 数字玩法,图像玩法;ButtonGroup group=null;Container con=null;public PuzzleFrame(){bar=new JMenuBar();fileMenu=new JMenu("魔板游戏");初级=new JMenuItem("初级");高级=new JMenuItem("高级");fileMenu.add(初级);fileMenu.add(高级);bar.add(fileMenu);setJMenuBar(bar);初级.addActionListener(this);高级.addActionListener(this);开始=new JButton("开始玩");开始.addActionListener(this);group=new ButtonGroup();数字玩法=new JRadioButton("数字玩法",true);图像玩法=new JRadioButton("图像玩法",false);group.add(数字玩法);group.add(图像玩法);puzzlePad=new PuzzlePad(3,3,50,50);con=getContentPane();con.add(puzzlePad,BorderLayout.CENTER);JPanel pNorth=new JPanel();pNorth.add(数字玩法);pNorth.add(图像玩法);pNorth.add(开始);con.add(pNorth,BorderLayout.NORTH);con.validate();addWindowListener(new WindowAdapter(){ public void windowClosing(WindowEvent e){System.exit(0);}});setVisible(true);setBounds(100,50,440,360);validate();}public void actionPerformed(ActionEvent e){ if(e.getSource()==开始){if(数字玩法.isSelected()){puzzlePad.随机排列数字();}else if(图像玩法.isSelected()){puzzlePad.随机排列图像();}}else if(e.getSource()==初级){con.remove(puzzlePad);puzzlePad=new PuzzlePad(3,3,50,50);con.add(puzzlePad,BorderLayout.CENTER); con.validate();this.validate();}else if(e.getSource()==高级){con.remove(puzzlePad);puzzlePad=new PuzzlePad(4,4,50,50);con.add(puzzlePad,BorderLayout.CENTER); con.validate();this.validate();}}public static void main(String args[]){new PuzzleFrame();}}MobanFrame类:import java.awt.*;import java.awt.event.*;import .*;import java.applet.Applet;import javax.imageio.ImageIO;import java.io.File;import java.awt.image.*;import javax.swing.JOptionPane;public class MobanFrame extends Frame implements ActionListener,MouseListener,ItemListener{MobanSquare[][] position;Point startPoint=new Point(100,130);Point rightStartPoint=new Point(400,90);int[] num;Point[] pointMove;int totalStep=0;String selectedImage="狗";String gamingImage=" ";boolean startGame=false;int squareNumber=3;int level=1;int squareSize=80;Image sourceImage;Image spaceSourceImage;Image spaceImage;Image[] myImage;Graphics2D ggg ;/*--------------------*//*显示在界面上的一些组件*//*--------------------*/TextField tfx;TextField tfy;MenuBar mnbMyMenuBar;Menu mnChooseLevel;MenuItem mniLevel1, mniLevel2;Label lbStep;Label lbSuccess=new Label("");Button btnStartGame;Choice chChoiceImage;public MobanFrame(){super("魔板游戏");pointMove=new Point[4];pointMove[0]=new Point(-1,0);pointMove[1]=new Point(1,0);pointMove[2]=new Point(0,-1);pointMove[3]=new Point(0,1);setLayout(new FlowLayout());chChoiceImage=new Choice();chChoiceImage.add("狗");chChoiceImage.add("鸡");chChoiceImage.add("鱼");chChoiceImage.add("熊猫");chChoiceImage.add("大象");chChoiceImage.add("长颈鹿");chChoiceImage.add("牛");chChoiceImage.add("小猫");chChoiceImage.add("小猫2");chChoiceImage.add("羊");chChoiceImage.add("猴 ");chChoiceImage.add("数字");mniLevel1=new MenuItem("简单");mniLevel2=new MenuItem("困难");mnChooseLevel=new Menu("难度");mnbMyMenuBar=new MenuBar();tfx=new TextField(8);tfy=new TextField(8);tfx.setText("0");tfy.setText("1");tfx.setVisible(false);tfy.setVisible(false);lbStep=new Label("已走步数: "+Integer.toString(totalStep));btnStartGame=new Button("开始游戏");mnChooseLevel.add(mniLevel1);mnChooseLevel.add(mniLevel2);mnbMyMenuBar.add(mnChooseLevel);this.setMenuBar(mnbMyMenuBar);add(tfx);add(tfy);add(lbSuccess);add(lbStep);add(chChoiceImage);add(btnStartGame);mniLevel1.addActionListener(this);mniLevel2.addActionListener(this);btnStartGame.addActionListener(this);chChoiceImage.addItemListener(this);this.addMouseListener(this);addWindowListener(new WindowAdapter(){public void windowClosing(WindowEvent e) {System.exit(0);}});ProInit();GetImage();GetRandom();setSize(600,300);setVisible(true);}public void GetImage(){myImage=new Image[squareNumber*squareNumber];ImageFilter cropFilter1;ImageFilter cropFilter2;ImageFilter cropFilter3;Toolkit tool=getToolkit();sourceImage=tool.createImage(selectedImage+".jpg");spaceSourceImage=tool.createImage("space.jpg");sourceImage=sourceImage.getScaledInstance(squareSize*squareNumber ,squareSize*squareNumber,Image.SCALE_DEFAULT);cropFilter1 =new CropImageFilter(0,0,squareSize*squareNumber,squareSize*squareNumber);sourceImage=createImage(newFilteredImageSource(sourceImage.getSource(),cropFilter1));for(int i=0;i<squareNumber*squareNumber;i++){cropFilter2 =new CropImageFilter((i%squareNumber)*squareSize,(i/squareNumber)*squareSi ze,squareSize,squareSize);myImage[i]=createImage(newFilteredImageSource(sourceImage.getSource(),cropFilter2));}cropFilter3=new CropImageFilter(0,0,squareSize,squareSize);spaceImage=createImage(newFilteredImageSource(spaceSourceImage.getSource(),cropFilter3));myImage[squareNumber*squareNumber-1]=spaceImage;}public void ProInit(){num=new int[squareNumber*squareNumber];position=new MobanSquare[squareNumber+2][squareNumber+2];}public void paint(Graphics g){boolean showSuccess=true;lbStep.setText("已走步数: "+Integer.toString(totalStep));if (startGame){for (int i=1;i<squareNumber+1;i++){for (int j=1;j<squareNumber+1;j++){g.drawImage(position[i][j].GetImage(),startPoint.x+(position[i][j ].y-1)*squareSize,startPoint.y+(position[i][j].x-1)*squareSize,this); //绘制左边打乱的方块}}g.setColor(Color.cyan);for (int i=0;i<squareNumber+1;i++){g.drawLine(startPoint.x+squareSize*i,startPoint.y,startPoint.x+sq uareSize*i,startPoint.y+squareNumber*squareSize);g.drawLine(startPoint.x,startPoint.y+squareSize*i,startPoint.x+sq uareNumber*squareSize,startPoint.y+squareSize*i);}}g.drawImage(sourceImage,rightStartPoint.x,rightStartPoint.y,this) ;//g.drawImage(spaceImage,rightStartPoint.x+(squareNumber-1)*squar eSize,rightStartPoint.y+(squareNumber-1)*squareSize,this);for (int i=1;i<squareNumber+1;i++){for (int j=1;j<squareNumber+1;j++){if(position[i][j].GetOrder()!=(i-1)*squareNumber+(j-1)){showSuccess=false;}}}if(showSuccess){showSuccess=false;lbSuccess.setText("恭喜您,成功完成");}}public void GetRandom(){int k=squareNumber*squareNumber;boolean numExist=false;int total=0;for (int j=0;j<squareNumber*squareNumber;j++){num[j]=-1;}while(total<squareNumber*squareNumber){k=((int)(Math.random()*10)+(int)(Math.random()*10))%(squareNumber *squareNumber);for (int j=0;j<total;j++){if (k==num[j]){numExist=true;break;}}if (!numExist){num[total]=k;total++;}numExist=false;}for(int i=0;i<squareNumber+2;i++){for (int j=0;j<squareNumber+2;j++){if(i==0||i==squareNumber+1||j==0||j==squareNumber+1){this.position[i][j]=newMobanSquare(i,j,-1,squareNumber*squareNumber-1);}}}for (int i=1;i<squareNumber+1;i++){for (int j=1;j<squareNumber+1;j++){this.position[i][j]=newMobanSquare(i,j,num[(i-1)*squareNumber+j-1],squareNumber*squareNumber -1);this.position[i][j].SetImage(myImage[this.position[i][j].GetOrder ()]);}}}public void Move(int x,int y){int square_X,square_Y,order_Old,order_New;square_X=(y-1-startPoint.y)/squareSize+1;square_Y=(x-1-startPoint.x)/squareSize+1;for (int i=0;i<4;i++){if(position[square_X+pointMove[i].x][square_Y+pointMove[i].y].arrive) {this.totalStep++;Point newPoint=new Point(square_X+pointMove[i].x,square_Y+pointMove[i].y);order_Old=position[square_X][square_Y].GetOrder();order_New=position[newPoint.x][newPoint.y].GetOrder();position[square_X][square_Y].SetOrder(order_New);position[square_X][square_Y].SetImage(myImage[order_New]);position[newPoint.x][newPoint.y].SetOrder(order_Old);position[newPoint.x][newPoint.y].SetImage(myImage[order_Old]);repaint();break;}}}public void actionPerformed(ActionEvent e){if (e.getSource()==btnStartGame){lbSuccess.setText(" ");totalStep=0;if (level==1){squareNumber=3;}if (level==2){squareNumber=4;}startGame=true;gamingImage=selectedImage;ProInit();GetImage();GetRandom();repaint();}if (e.getSource()==mniLevel1){level=1;startPoint=new Point(110,120);rightStartPoint=new Point(450,120);}if (e.getSource()==mniLevel2){level=2;startPoint=new Point(40,100);rightStartPoint=new Point(440,100);}}public void itemStateChanged(ItemEvent e){if (e.getItemSelectable() instanceof Choice){selectedImage=((Choice)e.getItemSelectable()).getSelectedItem();tfx.setText(selectedImage);tfy.setText(gamingImage);if (selectedImage!=gamingImage){GetImage();startGame=false;repaint();}}}public void mouseClicked(MouseEvent e){int click_X,click_Y;click_X=e.getX();click_Y=e.getY();tfx.setText(Integer.toString((e.getY()-1-startPoint.x)/squareSize +1));tfy.setText(Integer.toString((e.getX()-1-startPoint.y)/squareSize +1));if(click_X>=startPoint.x&&click_X<=startPoint.x+squareNumber*squa reSize&&click_Y>=startPoint.y&&click_Y<=startPoint.y+squareNumber*squ areSize){Move(click_X,click_Y);}}public void mouseExited(MouseEvent e){}public void mouseEntered(MouseEvent e){}public void mousePressed(MouseEvent e){}public void mouseReleased(MouseEvent e) {}public static void main(String[] args) {new MobanFrame();}}MobanSquare类:import java.awt.*;import java.awt.event.*;public class MobanSquare{public int x,y;private int order;private int nowSpaceOrder;private boolean squareHere;public boolean arrive;private Image squareImage;public MobanSquare(int px,int py,int order,int spaceOrder) {this.x=px;this.y=py;this.order=order;this.nowSpaceOrder=spaceOrder;if (this.order==this.nowSpaceOrder)arrive=true;elsearrive=false;if (this.order==this.nowSpaceOrder||this.order==-1) squareHere=false;elsesquareHere=true;}public boolean GetSquareHere(){return this.squareHere;}public void SetSquareHere(boolean b){this.squareHere=b;}public int GetOrder(){return this.order;}public void SetOrder(int order){this.order=order;if (this.order==this.nowSpaceOrder){arrive=true;squareHere=false;}else{arrive=false;squareHere=true;}}public Image GetImage(){return this.squareImage;}public void SetImage(Image ima){this.squareImage=ima;}}。
天黑请闭眼java代码

天黑请闭眼java代码天黑请闭眼,是一款非常经典的游戏,它可以培养人们的观察能力、心理素质和团队合作精神。
而今天,我们将用Java代码的方式来实现这个游戏。
我们需要创建一个玩家类,每个玩家有一个唯一的编号和一个身份。
身份可以是狼人、平民或预言家。
我们可以使用枚举类型来表示这些身份。
```javapublic class Player {private int id;private Identity identity;public Player(int id, Identity identity) {this.id = id;this.identity = identity;}// 省略getter和setter方法}public enum Identity {WOLF, // 狼人CIVILIAN, // 平民SEER // 预言家}```接下来,我们需要创建一个游戏类,用来控制游戏的整个流程。
游戏开始时,玩家们闭上眼睛,然后根据身份执行相应的操作。
为了模拟这个过程,我们可以使用线程的方式来实现。
```javapublic class Game {private List<Player> players;public Game(List<Player> players) {this.players = players;}public void start() {System.out.println("天黑请闭眼...");for (Player player : players) {switch (player.getIdentity()) {case WOLF:new Thread(() -> {System.out.println("狼人" + player.getId() + " 请睁眼...");// 狼人的操作System.out.println("狼人" + player.getId() + " 请闭眼...");}).start();break;case CIVILIAN:new Thread(() -> {System.out.println("平民" + player.getId() + " 请睁眼...");// 平民的操作System.out.println("平民" + player.getId() + " 请闭眼...");}).start();break;case SEER:new Thread(() -> {System.out.println("预言家" + player.getId() + " 请睁眼...");// 预言家的操作System.out.println("预言家" + player.getId() + " 请闭眼...");}).start();break;default:break;}}}}```在游戏开始时,我们创建了多个线程,每个线程代表一个玩家。
天黑请闭眼java代码
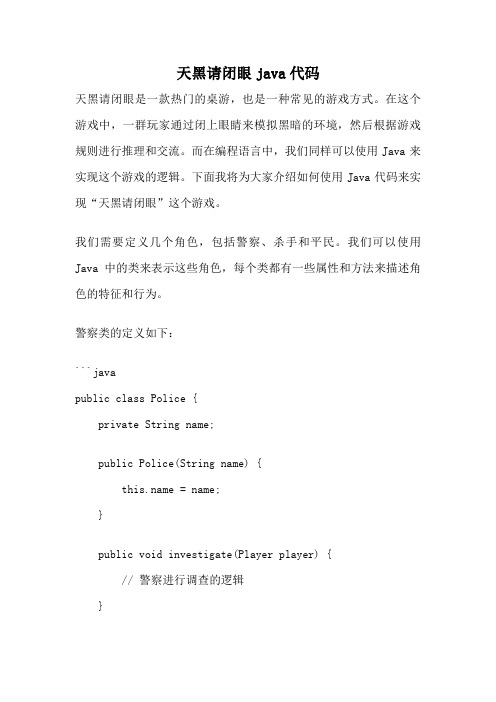
天黑请闭眼java代码天黑请闭眼是一款热门的桌游,也是一种常见的游戏方式。
在这个游戏中,一群玩家通过闭上眼睛来模拟黑暗的环境,然后根据游戏规则进行推理和交流。
而在编程语言中,我们同样可以使用Java来实现这个游戏的逻辑。
下面我将为大家介绍如何使用Java代码来实现“天黑请闭眼”这个游戏。
我们需要定义几个角色,包括警察、杀手和平民。
我们可以使用Java中的类来表示这些角色,每个类都有一些属性和方法来描述角色的特征和行为。
警察类的定义如下:```javapublic class Police {private String name;public Police(String name) { = name;}public void investigate(Player player) {// 警察进行调查的逻辑}public void save(Player player) { // 警察进行救人的逻辑}}```杀手类的定义如下:```javapublic class Killer {private String name;public Killer(String name) { = name;}public void kill(Player player) { // 杀手杀人的逻辑}}```平民类的定义如下:```javapublic class Civilian {private String name;public Civilian(String name) { = name;}public void vote(Player player) {// 平民投票的逻辑}}```接下来,我们需要定义一个玩家类,用来表示游戏中的每个玩家。
玩家类中包含一个角色对象,通过调用角色对象的方法来实现相应的行为。
玩家类的定义如下:```javapublic class Player {private String name;private Role role;public Player(String name, Role role) { = name;this.role = role;}public void action(Player player) {if (role instanceof Police) {((Police) role).investigate(player);} else if (role instanceof Killer) {((Killer) role).kill(player);} else if (role instanceof Civilian) {((Civilian) role).vote(player);}}}```在游戏开始时,我们需要创建一些玩家,并为每个玩家分配一个角色。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
1index.html页面显示主菜单
2addStudent.jsp页面添加学生信息页面
3addStudent_do.jsp页面调用JavaBean处理添加
4deleteStudent.jsp页面列表显示所以学生,选择删除的学生
5deleteStudent_do.jsp页面调.jsp页面列表显示所以学生,选择修改的学生
7modifyStudent_pro.jsp页面修改某一学生信息
8modifyStudent_do.jsp页面调用JavaBean处理修改
9viewStudent.jsp页面列表显示所有学生信息
10searchStudentBySid.jsp页面根据学号进行查询
USE jsp_db;
DROP TABLE IF EXISTS student;
CREATE TABLE student (
sid varchar(20) NOT NULL,
name varchar(30) default NULL,
sex int(1) default NULL,
在src文件新建ch6包;
把三个Java类拷贝到ch6里,更改DataBaseConnection.java的连接MySL字符串; 把mysl-connector-java-5.1.5-bin.jar拷贝到W
phone varchar(16) default NULL,
birth date default NULL,
Constraint primary key pk_student(sid)
) ENGINE=InnoDB DEFAULT CHARSET=gb2312;
二、在Eclipse里新建web工程ch6-3;
11DataBaseConnection.javaJavaBean连接数据库
12Student.javaJavaBean封装学生信息的JavaBean
13StudentUtil.javaJavaBean实现学生信息的增、删、改、查
一、首先建立数据库:
在MySL数据里建立jsp_db数据库-student表