DOCINFO structure
MFC打印与打印预览(1)

MFC打印与打印预览(1)Programming Windows 95 with MFC, Part VIII: Printing and Print PreviewingJeff ProsiseJeff Prosise writes extensively about programming in Windows and is a contributing editor of several computer magazines. He is currently working on a book, Programming Windows 95 with MFC, tobe published this spring by Microsoft Press.Click to open or copy the EZPRNT project files.Click to open or copy the HEXDUMP project files.I demonstrated previously ("Programming Windows® 95 with MFC, Part VII: The Document/View Architecture," MSJ, February 1996) how the document/view architecture simplifies the development of SDI and MDI applications by letting the framework take over key aspects of a program's operation. Now I'll show you how the same document/view architecture simplifies printing and print previewing. Even MFC-style printing isn't something to be taken lightly, but thanks to the support lent by the framework, the tedium of writing and testing code that renders documents on printers and other hardcopy devices is sharply reduced. And once you've implemented Print, Print Preview comes almost for free.Documents and ViewsThe MFC print architecture is built around core API print functions and protocols built into the Windows GDI. To understand what's on the outside, it helps to first understand what's on the inside. Inthis article I will:∙Discuss the Windows printing model and examine the steps an SDK-style application goes through toprint a document.∙Describe the relationship between the MFC print architecture and the Windows print architecture, and the mechanics of printing from MFC applications, including key virtual functions you can override in yourview class.∙Develop a bare-bones printing program that demonstrates how the same code can be used to sendoutput to the screen or printer.∙Develop a more ambitious program with print and preview capabilities on par with those ofcommercial applications. (Sounds pretty good, huh?)The Windows Print ArchitecturePrinting a document from a Windows-based application without the benefit of the framework involves a number of grueling steps. The application normally begins by obtaining a device context for the printer. Just as you need a screen DC to send output to the screen, you need a printer DC to send output to a printer. If you know the device name of the printer you want, you can create a device context with the ::CreateDC function in Windows or CDC::CreateDC in MFC.CDC dc;dc.CreateDC (NULL, "HP LaserJet IIP", NULL, NULL);The device name specified in the second parameter is the same one that appears in the control panel and Print Setup dialog. By the way, this syntax is simpler than Windows 3.1, which requires the driver name and other information. If you don't know the device name but would like to print to the default printer (the one whose context menu has a check mark by the "Set As Default" menu item), you can use the handy MFC CPrintDialog::GetDefaults and CPrintDialog::GetPrinterDC functions.CDC dc;CPrintDialog dlg (FALSE);dlg.GetDefaults ();dc.Attach (dlg.GetPrinterDC ());If you'd like to let the user select a printer, you can display a Print dialog (one of the commondialogs supplied for you by the operating system) with CPrintDialog::DoModal, then callCPrintDialog::GetPrinterDC after the dialog is dismissed.CDC dc;CPrintDialog dlg (FALSE);if (dlg.DoModal () == IDOK)dc.Attach (dlg.GetPrinterDC ());A printer device context obtained by any of these methods should be deleted when it's no longerneeded. If you create a CDC object on the stack, deletion is automatic.Once you have a printer DC in hand, you're ready to print. The next step is to call ::StartDoc or its MFC equivalent, CDC::StartDoc, to mark the beginning of the print job. CDC::StartDoc accepts just one parameter: a pointer to a DOCINFO structure containing a string with a descriptive name for the document that's about to be printed, the name of the file that output will go to (if you're printing to a file), and other information about the print job. The statementsDOCINFO di;::ZeroMemory (&di, sizeof (DOCINFO));di.cbSize = sizeof (DOCINFO);di.lpszDocName = "Budget Figures for the Current FiscalYear";dc.StartDoc (&di);start a print job on the printer associated with the CDC object dc. If the user examines the print queue while the document is printing, the string "Budget Figures for the Current Fiscal Year" will appear in the Document Name column. If StartDoc fails, it returns a zero or less-than-zero value. If it succeeds, it returns a positive integer that identifies the print job. The print job ID can be used in conjunction with Win32® print-control functions such as ::GetJob and ::SetJob.Next comes output to the printed page. Text and graphics are rendered on a printer with GDI functions. If dc refers to a screen device context, the statementdc.Ellipse (0, 0, 100, 100);draws an ellipse 100 logical units wide and 100 logical units high on the screen (that is, a circle). If dc refers to a printer device context, the circle is drawn on the printer instead. Pages of output are framed between calls to CDC::StartPage and CDC::EndPage that mark the beginning and end of each page. A document that contains nPageCount pages of output could be printed as follows:dc.StartDoc (&di);for (int i=1; i<=nPageCount; i++) {dc.StartPage ();// Print page idc.EndPage ();}In a very simplified sense, calling EndPage is like sending a form-feed character to the printer. In between StartPage and EndPage, you print the page by calling CDC member functions. Even if the document contains only one page, you must call StartPage and EndPage.One mistake programmers often make the first time they write printing code is that they fail to initialize the printer DC for each page. In Windows 95, the device context's default attributes are restored each time you call StartPage. You can't select a font or set the mapping mode right after the DC is created and expect those attributes to remain in effect indefinitely the way you can for a screen DC. Instead, you must reinitialize the printer DC for each page. (In Windows NTÔ 3.5 and later, a printer DC retains its settings across calls to StartPage and EndPage—but even in a Windows NT-based application, you should reinitialize the device context at the beginning of each page if you want your code to work under Windows 95 too.) If you print using the MM_LOENGLISH mapping mode, for example, you must call CDC::SetMapMode at the beginning of each new page.for (int i=1; i<=nPageCount; i++) {dc.StartPage ();dc.SetMapMode (MM_LOENGLISH);// Print page idc.EndPage ();}If you do it this way instead,// Don't do this!dc.SetMapMode (MM_LOENGLISH);for (int i=1; i<=nPageCount; i++) {dc.StartPage ();// Print page idc.EndPage ();}printing will be performed in the default MM_TEXT mapping mode.After the final page is printed, you terminate the print job by calling CDC::EndDoc—unless a previous call to EndPage returned a code indicating that the print job was already terminated by GDI. EndPage returns a signed integer value that is greater than zero if the page was successfully printed. A zero or negative return value indicates that either an error occurred or the user canceled the print job while the page was being printed. In either event, the return value is one of the codes shown in Figure 1.Figure 1 Printing Return CodesReturn Code DescriptionSP_ERROR The print job was aborted for an unspecified reasonSP_APPABORT The print job was aborted because the user clicked the Cancel button in the dialog box that displays the status of the print jobSP_USERABORT The print job was aborted because the user canceled it through the operatingsystem shellSP_OUTOFDISK The system is out of disk space, so no further printer data can be spooledSP_OUTOFMEMORY The system is out of memory, so no further printer data can be spooledThe following loop demonstrates the correct use of EndDoc.if (dc.StartDoc (&di) > 0) {BOOL bContinue = TRUE;for (int i=1; i<=nPageCount && bContinue; i++) {dc.StartPage ();// Initialize the device context// Print page iif (dc.EndPage () <= 0)bContinue = FALSE;}if (bContinue)dc.EndDoc ();elsedc.AbortDoc ();}CDC::AbortDoc aborts an uncompleted print job just as EndDoc ends a successful print job. You can also call AbortDoc at any time to terminate a print job before the job is done.The Abort Procedure and Abort DialogIf that's all there were to printing, it wouldn't be such a big deal. But there's more; because a large print job can take minutes or even hours to complete, the user should be able to terminate a print job before it is finished. Windows-based applications traditionally let the user cancel a print job by clicking a Cancel button in a dialog box. The Cancel button cancels printing by forcing EndPage to returnSP_APPABORT.The mechanism that links the Cancel button to the printing code in your application is a function that Windows calls an "abort procedure." An abort procedure is an exported callback function that Windows calls repeatedly as it processes printed output. It's declared like this:BOOL CALLBACK AbortProc (HDC hDC, int nCode)hDC holds the handle of the printer device context. nCode is zero if printing is proceeding smoothly or SP_OUTOFDISK if the print spooler is temporarily out of disk space. nCode is usually ignored because the print spooler responds to an SP_OUTOFDISK condition by waiting for more disk space tofree up. The abort procedure's job is twofold:∙To check the message queue with ::PeekMessage and retrieve and dispatch any messages waitingfor the application.∙To tell Windows whether printing should continue by returning TRUE (to continue printing) or FALSE(to abort).A very simple abort procedure looks like this:BOOL CALLBACK AbortProc (HDC hDC, int nCode)MSG msg;while (::PeekMessage (&msg, NULL, 0, 0,PM_NOREMOVE))AfxGetThread ()->PumpMessage ();return TRUE;}The message loop inside AbortProc allows the WM_COMMAND message (generated when the print-status dialog's Cancel button is clicked) to make it to your window procedure even though the application is busy printing. In 16-bit Windows, the message loop plays an important role in multitasking by yielding so the print spooler and other processes running in the system can get CPU time. In Windows 95, yielding in the abort procedure enhances multitasking performance when 32-bit applications print to 16-bit printer drivers by reducing contention for the Win16Mutex—the flag inside Windows that locks 32-bit applications out of the 16-bit kernel while a 16-bit application executes code in the kernel.Before calling StartDoc, the application calls SetAbortProc to set the abort procedure, then disables its own window by calling CWnd::EnableWindow (FALSE), and displays the print-status or "abort" dialog—a modeless dialog containing a Cancel button and usually one or more static controls to show the document name and number of pages printed. Disabling the main window ensures that no other input will interrupt the printing process. The window is reenabled when printing is finished and the dialog box is destroyed. The dialog, meanwhile, sets a flag—call it bUserAbort—from FALSE to TRUE if the Cancel button is clicked, and the abort procedure returns FALSE to abort printing if bUserAbort isTRUE.BOOL CALLBACK AbortProc (HDC hDC, int nCode){MSG msg;while (!bUserAbort &&::PeekMessage (&msg, NULL, 0, 0, PM_NOREMOVE))AfxGetThread ()->PumpMessage ();return !bUserAbort;Printing proceeds unimpeded until/unless the user cancels, whereupon bUserAbort changes from FALSE to TRUE, the next call to AbortProc returns zero, and Windows terminates the print job. EndPage returns SP_APPABORT and the call to EndDoc is subsequently bypassed.Print SpoolingEverything I've described up to this point constitutes the "front end" of the printing process—the portion that the application is responsible for. Windows handles the back end, which is a joint effort on the part of GDI, the print spooler, the printer driver, and other components of the 32-bit print subsystem. If enhanced metafile (EMF) print spooling is enabled, GDI calls executed through the printer DC are written to an enhanced metafile on the hard disk and stored there until the print spooler, which runs in a separate thread, unspools the commands and "plays" them into the printer driver. If "raw" print spooling (the only option on PostScript printers) is enabled instead, output is processed through the printer driver and spooled to disk in raw form. If spooling is disabled, GDI commands are transmitted directly to the printer driver each time EndPage is called. Print spooling speeds the return-to-application time by letting a program do all its printing to a metafile so the user doesn't have to wait for the printer to physically print each page. Metafiles further speed things up by decoupling the performance of the application from the performance of the printer driver. That's one reason why Windows 95 seems to print so much faster than Windows 3.1. In Windows 3.1, GDI commands are processed by the printer driver before they're spooled to disk. In Windows 95, output is spooled first and played through the printer driver later, so the translation of GDI commands into device-dependent printer data is performed entirely in the background.Fortunately, you can safely ignore what happens on the back end of the printing process and concentrate on printing your document. Still, there's a host of details to fret before you can get down to the real business of printing—paginating the output and executing GDI calls between StartPage and EndPage to render each page on the printer. With this background in mind, let's see how MFC makesprinting easier.The MFC Print ArchitectureThe simplified print architecture in MFC is just one more reason that programmers are migrating away from the SDK and toward object-oriented development environments such as Visual C++®. When you print from a document/view application, you can forget about most of the code samples in the previous section. The framework creates a printer DC for you and deletes the DC when printing is finished. The framework also calls StartDoc and EndDoc to begin and end the print job, and StartPage and EndPage to bracket GDI calls for each page. It even supplies a Cancel dialog and abort procedure.In some cases, the very same OnDraw function you use to render your document on screen can doprinting and Print Preview too.The key to printing in document/view is a set of virtual CView functions that the framework calls at various stages during the printing process. These functions are summarized in Figure 2. Which CView functions you override and what you do in the overrides is highly dependent upon the content of yourprinted output. At the very least, you must override OnPreparePrinting to call DoPreparePrinting so the framework will display a Print dialog and create a printer DC.BOOL CMyView::OnPreparePrinting (CPrintInfo* pInfo){return DoPreparePrinting (pInfo);}Figure 2 CView Print OverridablesFunction DescriptionCView::OnPreparePrinting Called at the very onset of a print job. Override to callCView::DoPreparePrinting and provide the framework with the page count (ifknown) and other information about the print job.CView::OnBeginPrinting Called just before printing begins. Override to allocate fonts and otherresources required for printing.CView::OnPrepareDC Called before each page is printed. Override to set the viewport origin orclipping region to print the current page if OnDraw will be used to produce theprinted output.CView::OnPrint Called to print one page of the document. A page number and printer DC aresupplied to you by the framework. Override to print headers, footers, andother page elements that aren't drawn by the view's OnDraw function or toprint each page without relying on OnDraw.CView::OnEndPrinting Called when printing is finished. Override to deallocate resources allocated inOnBeginPrinting.A nonzero return from OnPreparePrinting begins the printing process, while a zero return cancelsthe pending print job. DoPreparePrinting returns zero if the user cancels the print job by clicking the Cancel button in the Print dialog, if there are no printers installed, or if the framework is unable to create aprinter DC.More on OnPreparePrintingThe CPrintInfo object passed to OnPreparePrinting contains information describing the parameters of the print job, including the minimum and maximum page numbers. The minimum and maximum page numbers default to 1 and 0xFFFF, with the latter value signaling to the framework that the maximum page number is unknown. If you know how many pages your document has, you should callCPrintInfo::SetMaxPage before calling DoPreparePrinting.BOOL CMyView::OnPreparePrinting (CPrintInfo* pInfo){pInfo->SetMaxPage (nMaxPage);return DoPreparePrinting (pInfo);}The framework, in turn, will display the maximum page number in the "to" box of the Print dialog.SetMaxPage is one of several CPrintInfo member functions you can call to set printing parameters or query the framework about print options entered by the user. SetMinPage lets you specify the minimum page number. GetMinPage and GetMaxPage return the minimum and maximum page numbers, while GetFromPage and GetToPage return the starting and ending page numbers entered in the Print dialog's "from" and "to" boxes. CPrintInfo also includes several public data members, including m_pPD, which points to the initialized CPrintDialog object. You can use this pointer to customize the Print dialog before it's displayed, or get information from it. I'll show you an example of this later in the article.OnBeginPrinting and OnEndPrintingOften the maximum page number depends on the size of the printable area of each page. Unfortunately, you don't know what that is until after the user has selected a printer and the framework has created a printer DC. If you don't set the maximum page number in OnPreparePrinting, you should set it in OnBeginPrinting if at all possible. OnBeginPrinting receives a pointer to an initialized CPrintInfo structure and a pointer to a CDC object representing the printer DC. You can determine the dimensions of the printable page area by calling CDC::GetDeviceCaps twice—once with a HORZRES parameter,and once with a VERTRES parameter.void CMyView::OnBeginPrinting (CDC* pDC,CPrintInfo* pInfo){int m_nPageHeight = pDC->GetDeviceCaps (VERTRES);int nDocLength = GetDocument ()->GetDocLength ();int nMaxPage = max (1, (nDocLength +(m_nPageHeight - 1)) /m_nPageHeight);pInfo->SetMaxPage (nMaxPage);}In this example, GetDocLength is a document function that returns the length of the document in pixels. CPrintInfo contains a data member, m_rectDraw, that describes the printable page area in logical coordinates—but don't try to use it in OnBeginPrinting because it isn't initialized until shortly beforeOnPrint is called.Calling SetMaxPage in either OnPreparePrinting or OnBeginPrinting lets the framework know how many times it should call OnPrint to print a page. If it's impossible (or simply not convenient) to determine the document length before printing begins, you can perform "print-time pagination" by overriding OnPrepareDC and setting CPrintInfo::m_bContinuePrinting to TRUE or FALSE each time OnPrepareDC is called. FALSE terminates the print job. If you don't call SetMaxPage, the framework assumes the document is only one page long. Therefore, you must override OnPrepareDC and setm_bContinuePrinting to print additional pages.OnBeginPrinting is also the best place to create fonts and other GDI resources that you need to print. Suppose your view's OnCreate function creates a font that OnDraw uses to draw text on the screen, and the font height is based on screen metrics returned by GetDeviceCaps. To print a WYSIWYG version of that font on the printer, you must create a separate font that's scaled to printer metrics rather than screen metrics. One solution is to let OnDraw create the font each time it's used so the font is scaled for whatever device the CDC corresponds to—printer or screen. An alternative solution is to create the printer font in OnBeginPrinting and delete it in OnEndPrinting. Then you avoid the extra overhead ofcreating and deleting a font each time OnDraw is called.OnEndPrinting is the counterpart to OnBeginPrinting. It's a great place to free fonts and other resources allocated in OnBeginPrinting. If there are no resources to free, or if you didn't override OnBeginPrinting to begin with, then you probably don't need to override OnEndPrinting either.OnPrepareDCMFC calls OnPrepareDC once for each page of your document. One reason for overriding OnPrepareDC is to perform print-time pagination as described in the previous section. Another reason is to calculate a new viewport origin from the current page number so OnDraw will render the appropriate page in your document. Like OnBeginPrinting, OnPrepareDC receives a pointer to a device context and a pointer to a CPrintInfo object. Unlike OnBeginPrinting, OnPrepareDC is called prior to screen repaints as well as in preparation for outputting a page to the printer. In the case of screen rendering, the CDC pointer refers to a screen DC and the CPrintInfo pointer is NULL. For printing, the CDC pointer is a printer DC and the CPrintInfo pointer is non-NULL. In the latter case, the number of the page that's about to beprinted can be obtained from CPrintInfo::m_nCurPage. You can determine whether OnPrepareDC was called for the screen or printer by calling CDC::IsPrinting.The following implementation of OnPrepareDC moves the viewport origin in the y direction so the device point (0, 0)—the pixel in the upper-left corner of the printed page—corresponds to the upper-left corner of the current page. m_nPageHeight is a CMyView data member that holds the printable pageheight.void CMyView::OnPrepareDC (CDC* pDC, CPrintInfo* pInfo){CView::OnPrepareDC (pDC, pInfo);if (pDC->IsPrinting ()) { // If printing...int y = (pInfo->m_nCurPage- 1) * m_nPageHeight;pDC->SetViewportOrg (0, -y);}}Setting the viewport origin this way ensures that an OnDraw function attempting to draw the entire document will actually only draw the portion that corresponds to the current page. This is a simple example of OnPrepareDC that assumes you want to use the entire printable area of the page. Sometimes it's also necessary to set a clipping region to restrict the printed portion of the document to something less than the page's full printable area. Rectangular regions are created with CRgn::CreateRectRgn and selected into DCs to serve as clipping regions with CDC::SelectClipRgn.As a rule, you only need to override OnPrepareDC to print multipage documents for which OnDraw does the printing. If you do all of your printing from OnPrint, as one of my sample programs does, then there's no need to override OnPrepareDC. When you do override OnPrepareDC, you should call the base class before doing anything else so the default implementation will get a chance to do its thing. This is especially important when your view class is derived from CScrollView, because CScrollView::OnPrepareDC sets the viewport origin for screen DCs to match the current scroll position. When CScrollView::OnPrepareDC returns, the DC's mapping mode is set to the mapping mode specified when you called SetScrollSizes. If your view class is not derived from CScrollView, OnPrepareDC is agood place to call SetMapMode.OnPrintAfter calling OnPrepareDC, but prior to physically printing each page, the framework calls CView::OnPrint. The default implementation in Viewcore.cpp verifies the validity of pDC and passes thebuck to OnDraw.void CView::OnPrint(CDC* pDC, CPrintInfo*){ASSERT_VALID(pDC);// Override and set printing variables based on// page numberOnDraw(pDC); // Call Draw}If you prepared the printer DC for printing in OnPrepareDC, or if the document contains only one page, you don't have to override OnPrint; you can let the default implementation call OnDraw to do theprinting.In practice, OnPrint is frequently overridden to perform page-specific printing tasks. Probably the most common reason to override OnPrint is to print headers, footers, page numbers and other visual elements that appear on the printed page but not on the screen (with the exception of print previews). The following OnPrint function calls local member functions PrintHeader and PrintPageNumber to print a header and page number before calling OnDraw to print the page:void CMyView::OnPrint (CDC* pDC, CPrintInfo* pInfo){PrintHeader (pDC);PrintPageNumber (pDC, pInfo->m_nCurPage);// Set the viewport origin and/or clipping// region before calling OnDraw...OnDraw (pDC);}Note that any adjustments made to the printer DC with SetViewportOrg or SelectClipRgn should now be made in OnPrint rather than OnPrepareDC so headers and page numbers won't be affected. An alternative approach is to forego the call to OnDraw and do all your printing in OnPrint. If your drawing and printing code are substantially different, doing the printing in OnPrint draws a clearer boundary between how you print a document and how you render it on screen.CView::OnFilePrint and Other Command HandlersPrinting usually begins when the user selects the Print command from the File menu. MFC provides a CView::OnFilePrint that you can connect to the ID_FILE_PRINT menu item through the view's message map. Figure 3 summarizes what happens when the user prints. It also shows how the MFC print architecture meshes with the Windows print architecture because, if you take away the round rectangles representing the virtual CView functions that the framework calls, you're left with a pretty good schematic of the Windows printing model. Note that OnPrepareDC is called twice per page when your code executes under Windows 95. The first call to OnPrepareDC is made to preserve compatibility with 16-bit versions of MFC, which called OnPrepareDC before StartPage (and got away with it because in 16-bit Windows, it's EndPage, not StartPage, that resets the device context). The second call is made because, in Windows 95, changes made to the device context in the first call to OnPrepareDC arenullified when StartDoc is called.Figure 3 Overwiew of the MFC Print ArchitectureMFC also provides predefined command IDs and default command handlers for the File menu's Print Preview and Print Setup commands. The Print Preview command (ID=ID_FILE_PRINT_PREVIEW) is handled by CView::OnFilePrintPreview, and Print Setup (ID=ID_FILE_PRINT_SETUP) is handled by CWinApp::OnFilePrintSetup. Like OnFilePrint, these command handlers are not prewired into the message maps of the classes to which they belong. To enable them, you must do the message mapping yourself. Of course, if you use AppWizard to generate the skeleton of an application that prints, the message mapping is done for you. AppWizard also maps ID_FILE_PRINT_DIRECT to CView::OnFilePrint to enable "direct" printing—printing performed not by selecting Print from the Filemenu, but by dropping a document icon onto a printer.Print PreviewOnce a document/view application can print, adding print preview is as simple as adding a Print Preview command and hooking it up to CView::OnFilePrintPreview. There's a lot of code in the class library to support OnFilePrintPreview (see Viewprev.cpp for details), but what happens is pretty simple.OnFilePrintPreview takes over the frame window and fills it with a view created from a special CScrollView-derived class named CPreviewView. It adds a toolbar with buttons for going to the next or previous page, switching between one-page and two-page views, zooming in and out, and so on.CPreviewView::OnDraw draws a white rectangle representing a printed page (or two rectangles if。
英语作文的structure

英语作文的Structure: Keys to Crafting a Cohesive and Persuasive EssayIn the realm of academic writing, the structure of an essay is paramount. It serves as the backbone that supports the ideas, arguments, and evidence presented within the text. Just as a building requires a solid foundation to stand tall, an essay requires a robust structure to convey its message effectively. Let's delve into the essential elements of an English essay's structure and how they contribute to its overall coherence and persuasiveness.The introduction, often the first paragraph, sets the tone and direction for the entire essay. It should briefly introduce the topic, provide background information, and end with a clear thesis statement that outlines the main argument or point of view the essay will explore. A strong introduction captures the reader's attention and prepares them for the journey ahead.Following the introduction, the body paragraphs develop and expand upon the ideas introduced in the introduction. Each paragraph should have a clear topic sentence that introduces the main idea being discussed, followed bysupporting sentences that provide evidence, examples, or analysis to back up the topic sentence. Transition wordsand phrases are crucial in the body paragraphs to create a smooth flow of ideas and maintain the reader's engagement.The conclusion, the final paragraph of the essay, ties everything together and leaves a lasting impression on the reader. It should restate the main ideas and arguments from the body paragraphs, provide a summary of the evidence presented, and end with a concluding statement that leaves the reader with a final thought or takeaway. The conclusion should leave the reader feeling satisfied and well-informed. Throughout the essay, effective use of language is essential. This includes the use of vocabulary that is both precise and appropriate to the topic, as well as sentence structure that varies to maintain interest and engagement. Clear and concise language helps the reader follow the argument and understand the points being made.In addition to these basic elements, it's important to consider the overall flow and pacing of the essay. The introduction should lead smoothly into the body paragraphs, and the conclusion should bring the argument to asatisfying close. Avoiding冗余信息 and staying focused on the main argument helps maintain the coherence of the essay. In conclusion, the structure of an English essay is crucial to its success. A clear and logical structure that includes an engaging introduction, well-developed body paragraphs, and a satisfying conclusion, combined with effective language use and pacing, creates an essay that is both cohesive and persuasive. With these keys in mind, any writer can craft a powerful essay that captivates thereader and conveys its message effectively.**英语作文结构:构建连贯且有说服力的文章的关键** 在学术写作领域,作文的结构至关重要。
structure 使用说明

Documentation for structure software:Version2.3Jonathan K.Pritchard aXiaoquan Wen aDaniel Falush b123a Department of Human GeneticsUniversity of Chicagob Department of StatisticsUniversity of OxfordSoftware from/structure.htmlApril21,20091Our other colleagues in the structure project are Peter Donnelly,Matthew Stephens and Melissa Hubisz.2Thefirst version of this program was developed while the authors(JP,MS,PD)were in the Department of Statistics,University of Oxford.3Discussion and questions about structure should be addressed to the online forum at structure-software@.Please check this document and search the previous discus-sion before posting questions.Contents1Introduction31.1Overview (3)1.2What’s new in Version2.3? (3)2Format for the datafile42.1Components of the datafile: (4)2.2Rows (5)2.3Individual/genotype data (6)2.4Missing genotype data (7)2.5Formatting errors (7)3Modelling decisions for the user73.1Ancestry Models (7)3.2Allele frequency models (12)3.3How long to run the program (13)4Missing data,null alleles and dominant markers144.1Dominant markers,null alleles,and polyploid genotypes (14)5Estimation of K(the number of populations)155.1Steps in estimating K (15)5.2Mild departures from the model can lead to overestimating K (16)5.3Informal pointers for choosing K;is the structure real? (16)5.4Isolation by distance data (17)6Background LD and other miscellania176.1Sequence data,tightly linked SNPs and haplotype data (17)6.2Multimodality (18)6.3Estimating admixture proportions when most individuals are admixed (18)7Running structure from the command line197.1Program parameters (19)7.2Parameters infile mainparams (19)7.3Parameters infile extraparams (21)7.4Command-line changes to parameter values (25)8Front End268.1Download and installation (26)8.2Overview (27)8.3Building a project (27)8.4Configuring a parameter set (28)8.5Running simulations (30)8.6Batch runs (30)8.7Exporting parameterfiles from the front end (30)8.8Importing results from the command-line program (31)8.9Analyzing the results (32)9Interpreting the text output339.1Output to screen during run (34)9.2Printout of Q (34)9.3Printout of Q when using prior population information (35)9.4Printout of allele-frequency divergence (35)9.5Printout of estimated allele frequencies(P) (35)9.6Site by site output for linkage model (36)10Other resources for use with structure3710.1Plotting structure results (37)10.2Importing bacterial MLST data into structure format (37)11How to cite this program37 12Bibliography371IntroductionThe program structure implements a model-based clustering method for inferring population struc-ture using genotype data consisting of unlinked markers.The method was introduced in a paper by Pritchard,Stephens and Donnelly(2000a)and extended in sequels by Falush,Stephens and Pritchard(2003a,2007).Applications of our method include demonstrating the presence of popu-lation structure,identifying distinct genetic populations,assigning individuals to populations,and identifying migrants and admixed individuals.Briefly,we assume a model in which there are K populations(where K may be unknown), each of which is characterized by a set of allele frequencies at each locus.Individuals in the sample are assigned(probabilistically)to populations,or jointly to two or more populations if their genotypes indicate that they are admixed.It is assumed that within populations,the loci are at Hardy-Weinberg equilibrium,and linkage equilibrium.Loosely speaking,individuals are assigned to populations in such a way as to achieve this.Our model does not assume a particular mutation process,and it can be applied to most of the commonly used genetic markers including microsatellites,SNPs and RFLPs.The model assumes that markers are not in linkage disequilibrium(LD)within subpopulations,so we can’t handle markers that are extremely close together.Starting with version2.0,we can now deal with weakly linked markers.While the computational approaches implemented here are fairly powerful,some care is needed in running the program in order to ensure sensible answers.For example,it is not possible to determine suitable run-lengths theoretically,and this requires some experimentation on the part of the user.This document describes the use and interpretation of the software and supplements the published papers,which provide more formal descriptions and evaluations of the methods.1.1OverviewThe software package structure consists of several parts.The computational part of the program was written in C.We distribute source code as well as executables for various platforms(currently Mac,Windows,Linux,Sun).The C executable reads a datafile supplied by the user.There is also a Java front end that provides various helpful features for the user including simple processing of the output.You can also invoke structure from the command line instead of using the front end.This document includes information about how to format the datafile,how to choose appropriate models,and how to interpret the results.It also has details on using the two interfaces(command line and front end)and a summary of the various user-defined parameters.1.2What’s new in Version2.3?The2.3release(April2009)introduces new models for improving structure inference for data sets where(1)the data are not informative enough for the usual structure models to provide accurate in-ference,but(2)the sampling locations are correlated with population membership.In this situation, by making explicit use of sampling location information,we give structure a boost,often allowing much improved performance(Hubisz et al.,2009).We hope to release further improvements in the coming months.loc a loc b loc c loc d loc eGeorge1-914566092George1-9-964094Paula110614268192Paula110614864094Matthew2110145-9092Matthew2110148661-9Bob210814264194Bob2-9142-9094Anja1112142-91-9Anja111414266194Peter1-9145660-9Peter1110145-91-9Carsten2108145620-9Carsten211014564192Table1:Sample datafile.Here MARKERNAMES=1,LABEL=1,POPDATA=1,NUMINDS=7, NUMLOCI=5,and MISSING=-9.Also,POPFLAG=0,LOCDATA=0,PHENOTYPE=0,EX-TRACOLS=0.The second column shows the geographic sampling location of individuals.We can also store the data with one row per individual(ONEROWPERIND=1),in which case thefirst row would read“George1-9-9145-96664009294”.2Format for the datafileThe format for the genotype data is shown in Table2(and Table1shows an example).Essentially, the entire data set is arranged as a matrix in a singlefile,in which the data for individuals are in rows,and the loci are in columns.The user can make several choices about format,and most of these data(apart from the genotypes!)are optional.For a diploid organism,data for each individual can be stored either as2consecutive rows, where each locus is in one column,or in one row,where each locus is in two consecutive columns. Unless you plan to use the linkage model(see below)the order of the alleles for a single individual does not matter.The pre-genotype data columns(see below)are recorded twice for each individual. (More generally,for n-ploid organisms,data for each individual are stored in n consecutive rows unless the ONEROWPERIND option is used.)2.1Components of the datafile:The elements of the inputfile are as listed below.If present,they must be in the following order, however most are optional(as indicated)and may be deleted completely.The user specifies which data are present,either in the front end,or(when running structure from the command line),in a separatefile,mainparams.At the same time,the user also specifies the number of individuals and the number of loci.2.2Rows1.Marker Names(Optional;string)Thefirst row in thefile can contain a list of identifiersfor each of the markers in the data set.This row contains L strings of integers or characters, where L is the number of loci.2.Recessive Alleles(Data with dominant markers only;integer)Data sets of SNPs or mi-crosatellites would generally not include this line.However if the option RECESSIVEALLE-LES is set to1,then the program requires this row to indicate which allele(if any)is recessive at each marker.See Section4.1for more information.The option is used for data such as AFLPs and for polyploids where genotypes may be ambiguous.3.Inter-Marker Distances(Optional;real)the next row in thefile is a set of inter-markerdistances,for use with linked loci.These should be genetic distances(e.g.,centiMorgans),or some proxy for this based,for example,on physical distances.The actual units of distance do not matter too much,provided that the marker distances are(roughly)proportional to recombination rate.The front end estimates an appropriate scaling from the data,but users of the command line version must set LOG10RMIN,LOG10RMAX and LOG10RSTART in thefile extraparams.The markers must be in map order within linkage groups.When consecutive markers are from different linkage groups(e.g.,different chromosomes),this should be indicated by the value-1.Thefirst marker is also assigned the value-1.All other distances are non-negative.This row contains L real numbers.4.Phase Information(Optional;diploid data only;real number in the range[0,1]).This isfor use with the linkage model only.This is a single row of L probabilities that appears after the genotype data for each individual.If phase is known completely,or no phase information is available,these rows are unnecessary.They may be useful when there is partial phase information from family data or when haploid X chromosome data from males and diploid autosomal data are input together.There are two alternative representations for the phase information:(1)the two rows of data for an individual are assumed to correspond to the paternal and maternal contributions,respectively.The phase line indicates the probability that the ordering is correct at the current marker(set MARKOVPHASE=0);(2)the phase line indicates the probability that the phase of one allele relative to the previous allele is correct(set MARKOVPHASE=1).Thefirst entry should befilled in with0.5tofill out the line to L entries.For example the following data input would represent the information from an male with5unphased autosomal microsatellite loci followed by three X chromosome loci, using the maternal/paternal phase model:102156165101143105104101100148163101143-9-9-90.50.50.50.50.5 1.0 1.0 1.0where-9indicates”missing data”,here missing due to the absence of a second X chromo-some,the0.5indicates that the autosomal loci are unphased,and the1.0s indicate that the X chromosome loci are have been maternally inherited with probability1.0,and hence are phased.The same information can be represented with the markovphase model.In this case the inputfile would read:102156165101143105104101100148163101143-9-9-90.50.50.50.50.50.5 1.0 1.0Here,the two1.0s indicate that thefirst and second,and second and third X chromosome loci are perfectly in phase with each other.Note that the site by site output under these two models will be different.In thefirst case,structure would output the assignment probabilities for maternal and paternal chromosomes.In the second case,it would output the probabilities for each allele listed in the inputfile.5.Individual/Genotype data(Required)Data for each sampled individual are arranged intoone or more rows as described below.2.3Individual/genotype dataEach row of individual data contains the following elements.These form columns in the datafile.bel(Optional;string)A string of integers or characters used to designate each individualin the sample.2.PopData(Optional;integer)An integer designating a user-defined population from which theindividual was obtained(for instance these might designate the geographic sampling locations of individuals).In the default models,this information is not used by the clustering algorithm, but can be used to help organize the output(for example,plotting individuals from the same pre-defined population next to each other).3.PopFlag(Optional;0or1)A Booleanflag which indicates whether to use the PopDatawhen using learning samples(see USEPOPINFO,below).(Note:A Boolean variable(flag)isa variable which takes the values TRUE or FALSE,which are designated here by the integers1(use PopData)and0(don’t use PopData),respectively.)4.LocData(Optional;integer)An integer designating a user-defined sampling location(orother characteristic,such as a shared phenotype)for each individual.This information is used to assist the clustering when the LOCPRIOR model is turned on.If you simply wish to use the PopData for the LOCPRIOR model,then you can omit the LocData column and set LOCISPOP=1(this tells the program to use PopData to set the locations).5.Phenotype(Optional;integer)An integer designating the value of a phenotype of interest,foreach individual.(φ(i)in table.)(The phenotype information is not actually used in structure.It is here to permit a smooth interface with the program STRAT which is used for association mapping.)6.Extra Columns(Optional;string)It may be convenient for the user to include additionaldata in the inputfile which are ignored by the program.These go here,and may be strings of integers or characters.7.Genotype Data(Required;integer)Each allele at a given locus should be coded by a uniqueinteger(eg microsatellite repeat score).2.4Missing genotype dataMissing data should be indicated by a number that doesn’t occur elsewhere in the data(often-9 by convention).This number can also be used where there is a mixture of haploid and diploid data (eg X and autosomal loci in males).The missing-data value is set along with the other parameters describing the characteristics of the data set.2.5Formatting errors.We have implemented reasonably careful error checking to make sure that the data set is in the correct format,and the program will attempt to provide some indication about the nature of any problems that exist.The front end requires returns at the ends of each row,and does not allow returns within rows;the command-line version of structure treats returns in the same way as spaces or tabs.One problem that can arise is that editing programs used to assemble the data prior to importing them into structure can introduce hidden formatting characters,often at the ends of lines,or at the end of thefile.The front end can remove many of these automatically,but this type of problem may be responsible for errors when the datafile seems to be in the right format.If you are importing data to a UNIX system,the dos2unix function can be helpful for cleaning these up.3Modelling decisions for the user3.1Ancestry ModelsThere are four main models for the ancestry of individuals:(1)no admixture model(individuals are discretely from one population or another);(2)the admixture model(each individual draws some fraction of his/her genome from each of the K populations;(3)the linkage model(like the admixture model,but linked loci are more likely to come from the same population);(4)models with informative priors(allow structure to use information about sampling locations:either to assist clustering with weak data,to detect migrants,or to pre-define some populations).See Pritchard et al.(2000a)and(Hubisz et al.,2009)for more on models1,2,and4and Falush et al.(2003a)for model3.1.No admixture model.Each individual comes purely from one of the K populations.The output reports the posterior probability that individual i is from population k.The prior probability for each population is1/K.This model is appropriate for studying fully discrete populations and is often more powerful than the admixture model at detecting subtle structure.2.Admixture model.Individuals may have mixed ancestry.This is modelled by saying that individual i has inherited some fraction of his/her genome from ancestors in population k.The output records the posterior mean estimates of these proportions.Conditional on the ancestry vector,q(i),the origin of each allele is independent.We recommend this model as a starting point for most analyses.It is a reasonablyflexible model for dealing with many of the complexities of real populations.Admixture is a common feature of real data,and you probably won’tfind it if you use the no-admixture model.The admixture model can also deal with hybrid zones in a natural way.Label Pop Flag Location Phen ExtraCols Loc1Loc2Loc3....Loc LM1M2M3....M Lr1r2r3....r L-1D1,2D2,3....D L−1,LID(1)g(1)f(1)l(1)φ(1)y(1)1,...,y(1)n x(1,1)1x(1,1)2x(1,1)3....x(1,1)LID(1)g(1)f(1)l(1)φ(1)y(1)1,...,y(1)n x(1,2)1x(1,2)2x(1,2)3....x(1,2)Lp(1)1p(1)2p(1)3....p(1)LID(2)g(2)f(2)l(2)φ(2)y(2)1,...,y(2)n x(2,1)1x(2,1)2x(2,1)3....x(2,1)LID(2)g(2)f(2)l(2)φ(2)y(2)1,...,y(2)n x(2,2)1x(2,2)2x(2,2)3....x(2,2)Lp(2)1p(2)2p(2)3....p(2)L ....ID(i)g(i)f(i)l(i)φ(i)y(i)1,...,y(i)n x(i,1)1x(i,1)2x(i,1)3....x(i,1)LID(i)g(i)f(i)l(i)φ(i)y(i)1,...,y(i)n x(i,2)1x(i,2)2x(i,2)3....x(i,2)Lp(3)1p(3)2p(3)3....p(3)L ....ID(N)g(N)f(N)l(N)φ(N)y(N)1,...,y(N)n x(N,1)1x(N,1)2x(N,1)3....x(N,1)LID(N)g(N)f(N)l(N)φ(N)y(N)1,...,y(N)n x(N,2)1x(N,2)2x(N,2)3....x(N,2)Lp(L)1p(L)2p(L)3....p(1)LTable2:Format of the datafile,in two-row format.Most of these components are optional(see text for details).M l is an identifier for marker l.r l indicates which allele,if any,is recessive at each marker(dominant genotype data only).D i,i+1is the distance between markers i and i+1.ID(i) is the label for individual i,g(i)is a predefined population index for individual i(PopData);f(i)is aflag used to incorporate learning samples(PopFlag);l(i)is the sampling location of individual i (LocData);φ(i)can store a phenotype for individual i;y(i)1,...,y(i)n are for storing extra data(ignoredby the program);(x i,1l ,x i,2l)stores the genotype of individual i at locus l.p(l)i is the phase informationfor marker l in individual i.3.Linkage model.This is essentially a generalization of the admixture model to deal with“ad-mixture linkage disequilibrium”–i.e.,the correlations that arise between linked markers in recently admixed populations.Falush et al.(2003a)describes the model,and computations in more detail.The basic model is that,t generations in the past,there was an admixture event that mixed the K populations.If you consider an individual chromosome,it is composed of a series of“chunks”that are inherited as discrete units from ancestors at the time of the admixture.Admixture LD arises because linked alleles are often on the same chunk,and therefore come from the same ancestral population.The sizes of the chunks are assumed to be independent exponential random variables with mean length1/t(in Morgans).In practice we estimate a“recombination rate”r from the datathat corresponds to the rate of switching from the present chunk to a new chunk.1Each chunkin individual i is derived independently from population k with probability q(i)k ,where q(i)kis theproportion of that individual’s ancestry from population k.Overall,the new model retains the main elements of the admixture model,but all the alleles that are on a single chunk have to come from the same population.The new MCMC algorithm integrates over the possible chunk sizes and break points.It reports the overall ancestry for each individual,taking account of the linkage,and can also report the probability of origin of each bit of chromosome,if desired by the user.This new model performs better than the original admixture model when using linked loci to study admixed populations.It achieves more accurate estimates of the ancestry vector,and can extract more information from the data.It should be useful for admixture mapping.The model is not designed to deal with background LD between very tightly linked markers.Clearly,this model is a big simplification of the complex realities of most real admixed popu-lations.However,the major effect of admixture is to create long-range correlation among linked markers,and so our aim here is to encapsulate that feature within a fairly simple model.The computations are a bit slower than for the admixture model,especially with large K and unphased data.Nonetheless,they are practical for thousands of sites and individuals and multiple populations.The model can only be used if there is information about the relative positions of the markers(usually a genetic map).ing prior population information.The default mode for structure uses only genetic information to learn about population structure.However,there is often additional information that might be relevant to the clustering(e.g.,physical characteristics of sampled individuals or geographic sampling locations).At present,structure can use this information in three ways:•LOCPRIOR models:use sampling locations as prior information to assist the clustering–for use with data sets where the signal of structure is relatively weak2.There are some data sets where there is genuine population structure(e.g.,significant F ST between sampling locations),but the signal is too weak for the standard structure models to detect.This is often the case for data sets with few markers,few individuals,or very weak structure.To improve performance in this situation,Hubisz et al.(2009)developed new models that make use of the location information to assist clustering.The new models can often provide accurate inference of population structure and individual ancestry in data sets where the signal of structure is too weak to be found using the standard structure models.Briefly,the rationale for the LOCPRIOR models is as ually,structure assumes that all partitions of individuals are approximately equally likely a priori.Since there is an immense number of possible partitions,it takes highly informative data for structure to 1Because of the way that this is parameterized,the map distances in the inputfile can be in arbitrary units–e.g.,genetic distances,or physical distances(under the assumption that these are roughly proportional to genetic distances).Then the estimated value of r represents the rate of switching from one chunks to the next,per unit of whatever distance was assumed in the inputfile.E.g.,if an admixture event took place ten generations ago,then r should be estimated as0.1when the map distances are measured in cM(this is10∗0.01,where0.01is the probability of recombination per centiMorgan),or as10−4=10∗10−5when the map distances are measured in KB(assuming a constant crossing-over rate of1cM/MB).The prior for r is log-uniform.The front end tries to make some guesses about sensible upper and lower bounds for r,but the user should adjust these to match the biology of the situation.2Daniel refers to this as“Better priors for worse data.”conclude that any particular partition of individuals into clusters has compelling statistical support.In contrast,the LOCPRIOR models take the view that in practice,individuals from the same sampling location often come from the same population.Therefore,the LOCPRIOR models are set up to expect that the sampling locations may be informative about ancestry. If the data suggest that the locations are informative,then the LOCPRIOR models allow structure to use this information.Hubisz et al.(2009)developed a pair of LOCPRIOR models:for no-admixture and for admix-ture.In both cases,the underlying model(and the likelihood)is the same as for the standard versions.The key difference is that structure is allowed to use the location information to assist the clustering(i.e.,by modifying the prior to prefer clustering solutions that correlate with the locations).The LOCPRIOR models have the desirable properties that(i)they do not tend tofind struc-ture when none is present;(ii)they are able to ignore the sampling information when the ancestry of individuals is uncorrelated with sampling locations;and(iii)the old and new models give essentially the same answers when the signal of population structure is very strong.Hence,we recommend using the new models in most situations where the amount of available data is very limited,especially when the standard structure models do not provide a clear signal of structure.However,since there is now a great deal of accumulated experience with the standard structure models,we recommend that the basic models remain the default for highly informative data sets(Hubisz et al.,2009).To run the LOCPRIOR model,the user mustfirst specify a“sampling location”for each individual,coded as an integer.That is,we assume the samples were collected at a set of discrete locations,and we do not use any spatial information about the locations.(We recognize that in some studies,every individual may be collected at a different location,and so clumping individuals into a smaller set of discrete locations may not be an ideal representation of the data.)The“locations”could also represent a phenotype,ecotype,or ethnic group. The locations are entered into the inputfile either in the PopData column(set LOCISPOP=1), or as a separate LocData column(see Section2.3).To use the LOCPRIOR model you must first specify either the admixture or no-admixture models.If you are using the Graphical User Interface version,tick the“use sampling locations as prior”box.If you are using the command-line version,set LOCPRIOR=1.(Note that LOCPRIOR is incompatible with the linkage model.)Our experience so far is that the LOCPRIOR model does not bias towards detecting structure spuriously when none is present.You can use the same diagnostics for whether there is genuine structure as when you are not using a LOCPRIOR.Additionally it may be helpful to look at the value of r,which parameterizes the amount of information carried by the locations. Values of r near1,or<1indicate that the locations are rger values of r indicate that either there is no population structure,or that the structure is independent of the locations.•USEPOPINFO model:use sampling locations to test for migrants or hybrids–for use with data sets where the data are very informative.In some data sets,the user mightfind that pre-defined groups(eg sampling locations)correspond almost exactly to structure clusters,except for a handful of individuals who seem to be misclassified.Pritchard et al.(2000a)developed a formal Bayesian test for evaluating whether any individuals in the sample are immigrants to their supposed populations,or have recent immigrant ancestors.Note that this model assumes that the predefined populations are usually correct.It takes quite strong data to overcome the prior against misclassification.Before using the USEPOPINFO model,you should also run the program without population information to ensure that the pre-defined populations are in rough agreement with the genetic information.To use this model set USEPOPINFO to1,and choose a value of MIGRPRIOR(which isνin Pritchard et al.(2000a)).You might choose something in the range0.001to0.1forν.The pre-defined population for each individual is set in the input datafile(see PopData).In this mode,individuals assigned to population k in the inputfile will be assigned to cluster k in the structure algorithm.Therefore,the predefined populations should be integers between 1and MAXPOPS(K),inclusive.If PopData for any individual is outside this range,their q will be updated in the normal way(ie without prior population information,according to the model that would be used if USEPOPINFO was turned off.3).•USEPOPINFO model:pre-specify the population of origin of some individuals to assist ancestry estimation for individuals of unknown origin.A second way to use the USEPOPINFO model is to define“learning samples”that are pre-defined as coming from particular clusters.structure is then used to cluster the remaining individuals.Note:In the Front End,this option is switched on using the option“Update allele frequencies using only individuals with POPFLAG=1”,located under the“Advanced Tab”.Learning samples are implemented using the PopFlag column in the datafile.The pre-defined population is used for those individuals for whom PopFlag=1(and whose PopData is in(1...K)).The PopData value is ignored for individuals for whom PopFlag=0.If there is no PopFlag column in the datafile,then when USEPOPINFO is turned on,PopFlag is set to1 for all individuals.Ancestry of individuals with PopFlag=0,or with PopData not in(1...K) are updated according to the admixture or no-admixture model,as specified by the user.As noted above,it may be helpful to setαto a sensible value if there are few individuals without predefined populations.This application of USEPOPINFO can be helpful in several contexts.For example,there may be some individuals of known origin,and the goal is to classify additional individuals of unknown origin.For example,we might collect data from a set of dogs of known breeds (numbered1...K),and then use structure to estimate the ancestry for additional dogs of unknown(possibly hybrid)origin.By pre-setting the population numbers,we can ensure that the structure clusters correspond to pre-defined breeds,which makes the output more interpretable,and can improve the accuracy of the inference.(Of course,if two pre-defined breeds are genetically identical,then the dogs of unknown origin may be inferred to have mixed ancestry.Another use of USEPOPINFO is for cases where the user wants to update allele frequen-cies using only a subset of the individuals.Ordinarily,structure analyses update the allele frequency estimates using all available individuals.However there are some settings where you might want to estimate ancestry for some individuals,without those individuals affecting the allele frequency estimates.For example you may have a standard collection of learning samples,and then periodically you want to estimate ancestry for new batches of genotyped 3If the admixture model is used to estimate q for those individuals without prior population information,αis updated on the basis of those individuals only.If there are very few such individuals,you may need tofixαat a sensible value.。
组织结构(英文)

• in large organization,complement bureaucracy, achieve the efficiency of bureaucracy’s standardization while gaining team’s flexibility
• lean,flat;2 or 3 vertical levels,a loose body of employees,& 1 individual in whom the D-M authority is centralized
• strength--fast,flexible,inexpensive to maintain,& accountability is clear
training.
2. Employee’s skills at performing a tasks
successfully increase through repetition---
• by the 1960s:the human diseconomies from specialization--boredom,fatigue,stress,low productivity,
effectively;the advancement of IT
Business Process Reengineering
1.The team structure
• The use of teams as the central device to coordinate work activities
英语五种建筑构造简介PPT

and asymmetric according to elevation; in terms of material into the steel frame and concrete frame, wood frame or steel frame and reinforced concrete mixture. One of the most commonly used is the concrete frame (cast as a whole, assembly, assembly as a whole, can also be applied in accordance with the need to stress, mainly on the beam or plate), steel frame. Assembling and assembling concrete frame and steel frame are suitable for large scale industrial construction, with high efficiency and good quality. 框架结构又称构架式结构。房屋的框架按跨数分有单跨、多跨;按层数分有单层、多层;按立面构成分为对称 、不对称;按所用材料分为钢框架、混凝土框架、胶合木结构框架或钢与钢筋混凝土混合框架等。其中最常用的 是混凝土框架(现浇整体式、装配式、装配整体式,也可根据需要施加预应力,主要是对梁或板)、钢框架。装 配式、装配整体式混凝土框架和钢框架适合大规模工业化施工,效率较高,工程质量较好。
排架结构特点: 传力明确,构造简单,有利于实现设计标准化、构件生产工业化以及施工机械化 ,提高建筑工业化水平。
04
Shear wall structure 剪力墙结构
建筑专业英语词汇整理大全模板

建筑专业英语词汇整理大全常用景观英文1.主入口大门/岗亭(车行 & 人行) MAIN ENTRANCE GATE/GUARDHOUSE (FOR VEHICLE& PEDESTRIAN )2.次入口/岗亭(车行 & 人行 ) 2ND ENTRANCE GATE/GUARDHOUSE (FOR VEHICLE& PEDESTRIAN )3.商业中心入口 ENTRANCE TO SHOPPING CTR.4.水景 WATER FEATURE5.小型露天剧场 MINI AMPHI-THEATRE6.迎宾景观-1 WELCOMING FEATURE-17.观景木台 TIMBER DECK (VIEWING)8.竹园 BAMBOO GARDEN9.漫步广场 WALKWAY PLAZA10.露天咖啡廊 OUT DOOR CAFE11.巨大迎宾水景-2 GRAND WELCOMING FEATURE-212.木桥 TIMBER BRIDGE13.石景、水瀑、洞穴、观景台 ROCK'SCAPE WATERFALL'S GROTTO/VIEWING TERRACE14.吊桥 HANGING BRIDGE15.休憩台地(低处) LOUNGING TERRACE (LOWER )16.休憩台地(高处) LOUNGING TERRACE (UPPER )17.特色踏步 FEATURE STEPPING STONE18.野趣小溪 RIVER WILD19.儿童乐园 CHILDREN'S PLAYGROUND20.旱冰道 SLIDE21.羽毛球场 BADMINTON COURT22.旱景 DRY LANDSCAPE23.日艺园 JAPANESE GARDEN24.旱喷泉 DRY FOUNTAIN25.观景台 VIEWING DECK26.游泳池 SWIMMING POOL27.极可意 JACUZZI28.嬉水池 WADING POOL29.儿童泳池 CHILDREN'S POOL30.蜿蜒水墙 WINDING WALL31.石景雕塑 ROCK SCULPTURE32.中心广场 CENTRAL PLAZA33.健身广场 EXERCISE PLAZA34.桥 BRIDGE35.交流广场 MEDITATING PLAZA36.趣味树阵 TREE BATTLE FORMATION37.停车场 PARING AREA38.特色花架 TRELLIS39.雕塑小道 SCULPTURE TRAIL40.(高尔夫)轻击区 PUTTING GREEN41.高尔夫球会所 GOLF CLUBHOUSE42.每栋建筑入口 ENTRANCE PAVING TO UNIT43.篮球场 BASKETBALL COURT44.网球场 TENNIS COURT45.阶梯坐台/种植槽 TERRACING SEATWALL/PLANTER46.广场 MAIN PLAZA47.森林、瀑布 FOREST GARDEN WATERFALL48.石景园 ROCKERY GARDEN49.旱溪 DRY STREAM50.凉亭 PAVILION51.户外淋浴 OUTDOOR SHOWER52.拉膜构造 TENSILE STRUCTURE53.台阶 STAIR54.高尔夫球车停车场 PARKING ( GOLF CAR )55.健身站 EXERCISE STATION56.晨跑小路 JOGGING FOOTPATH57.车道/人行道 DRIVEWAY /SIDEWALK58.人行漫步道 PROMENADE59.瀑布及跳舞喷泉(入口广场) WATER FALL AND DANCINGFOUNTAIN ( ENTRY PLAZA )60.特色入口 ENTRY FEATURE 61.石景广场 ROCKERY PLAZA常用造价英语词汇常用造价英语词汇估算/费用估算:estimate/cost estimate;估算类型:types of estimate;详细估算:是偏差幅度最小估算,defined estimate;设备估算:equipment estimate;分析估算:analysis estimate;报价估算:proposal estimate;控制估算:control estimate;初期控制估算:interim control estimate/initial controlestimate批准控制估算:initial approved cost核定估算:check estimate首次核定估算:first check estimate二次核定估算:production check estimate人工时估算:man hour estimate材料费用/直接材料费用:material cost/direct materialcost设备费用/设备购置费用:equipment cost/purchased cost ofequipment散装材料费用/散装材料购置费用:bulk materialcost/purchased cost of bulk material施工费用:construction cost施工人工费用:labor cost/construction force cost设备安装人工费用:labor cost associated with equipment 散装材料施工安装人工费用:labor cost associated with bulkmaterials人工时估算定额:standard manhours施工人工时估算定额:standard labor manhours标准工时定额:standard hours劳动生产率:labor productivity/productivityfactor/productivity ratio修正人工时估算值:adjusted manhours人工时单价:manhours rate施工监视费用:cost of construction supervision施工间接费用:cost of contruction indirects分包合同费用/现场施工分包合同费用:subcontractcost/field subcontract cost公司本部费用:home office cost公司管理费用:overhead非工资费用:non payroll开车效劳费用:cost of start-up services其他费用:other cost利润/预期利润:profit/expected profit效劳酬金:service gains风险:risk风险分析:risk analysis风险备忘录:risk memorandum未可预见费:contingency根本未可预见费:average contingency 最大风险未可预见费:maximum risk contingency用户变更/合同变更:cilent change/contract change 认可用户变更:approved client change待定用户变更:pending client change工程变更:project change内部变更:internalchange批准变更:authoried change强制性变更:mandatory change选择性变更:optional change内部费用转换:internal transfer认可预计费用:anticipated approved cost涨价值:escalation工程费用汇总报告:project cost summary report工程实施费用状态报告:project operation cost statusreport总价合同:lump sum contract偿付合同:reimbursible contract预算:budget规划专业英语规划词典环境、根底设施、交通运输环境设计( Environmental design ) 以物质环境质量为根本点,以优良环境是人根本权利与需要为前提土地利用规划。
Unit 3 Company structure教学教材

Unit 3 Company structureOne of the most important tasks for the management of any organization employing more than a few people is to determine its organizational structure, and to change this when and where necessary. This unit contains a text which outlines the most common organizational systems and exercise which focuses on the potential conflicts among the different departments of a manufacturing organization, an example of an organization chart, and a critical look at the flexible organizational structure of an American computer company.1a DiscussionThis discussion activity follows on naturally from activity 3d in the previous unit, about managing companies or having more limited responsibilities in a particular department.1b Vocabulary1. Autonomous: C independent, able to take decisions without consulting a higher authority2. Decentralization: E dividing an organization into decision-making units that are not centrally controlled.3. Function: B a specific activity in a company, e.g. production, marketing, finance4. Hierarchy: A system of authority with different levels, one above the other.5. Line authority: F the power to give instructions to people at the level below in the chain of command6. Report to: G to be responsible to someone and to take instructions from him or her7. Subordinates: D people working under someone else in a hierarchy1c ReadingThe text summarizes the most common ways in which companies and other organizations are structured, and mentions the people usually credited with inventing functional organization and decentralization. It mentions the more recent development of matrix management, and a well-known objection to it.How arte most organizations structured?Most companies are too large to be organized as a single hierarchy. The hierarchy is usually divided up. In what way?What are the obvious disadvantages of functional structure?(Discuss briefly in pairs) give some examples of standard conflicts in companies between departments with different objectivesAre there any other ways of organizing companies that might solve these problems?A.F unctional structureB.M atrix structureC.L ine structureD.Staff structureBritish: personnel department = American: staff department or human resources department1d ComprehensionThe only adequate summary is the second. The first stresses the disadvantages of hierarchies much more strongly than the text, and disregards the criticisms of matrix management and decentralization. The third is simply misleading: matrix management and teams are designed to facilitate communication among functional departments rather than among autonomous divisions.Second summaryMost business organizations have a hierarchy consisting of several levels and a clear line of command. There may also be staff positions that are not integrated into the hierarchy. The organization might also be divided into functional departments, such as production, finance, marketing, sales and personnel.Larger organizations are often further divided into autonomous divisions, each with its own functional sections. More recent organizational systems include matrix management and teams, both of which combine people from different functions and keep decision-making at lower levels.1e discussionThe text mentions the often incompatible goals of the finance, marketing and production (or operations) department. Classify the following strategies according to which departments would probably favor them.Production managers: 1.a factory working at full capacity 4.a standard product without optional features 11.machines that give the possibility of making various different products. (1, 4 and 11 would logically satisfy production managers, although 11 should also satisfy other departments.)Marketing managers: 2.a large advertising budget 3.a large sales force earning high commission 6.a strong market share for new products 7.generous credit facilities for customers rge inventories to make sure that products are available (2, 3, 6, 7, 9, would logically be the demands of marketing managers)Finance managers: 5.a strong cash balance 8.high profit margins 10.low research and development spending 12.self-financing (using retained earnings rather than borrowing) (5, 8, 10, and 12 would logically keep finance managers happy.)1f Describing company structureNow write a description of either the organization chart above, or a company you know, in about 100-150 words.Here is a short description of the organization chart illustrated.The Chief Executive Officer reports to the President and the Board of Directors. The company is divided into five major departments: Production, Marketing, Finance, Research & Development, and Personnel. The Marketing Department is subdivided into Market Research, Sales, and Advertising & Promotions. The Finance Department contains both Financial Management and Accounting. Sales consists of two sections, the Northern and Southern Regions, whose heads report to the Sales Manager, who is accountable to the Marketing Manager.2a VocabularyMatch up the words on the left with the definitions on the right.1.industrial belt: C an area with lots of industrial companies, around the edge ofa city2.wealth: F the products of economic activity3.productivity: E the amount of output produced (in a certain period, using acertain number of inputs)4.corporate ethos: A a company’s ways of working and thinking5.collaboration: G working together and sharing ideas6.insulated or isolated: B alone, placed in a position away from others7.fragmentation: D breaking something up into pieces2b ListeningListen to Jared Diamond, and then answer question 1. Listen a second time to check your answers, and then do question 2.1 Which of these do the part-sentences 1-8 refer to?A Route 128 (the industrial belt around Boston, Massachusetts)B Silicon Valley (the high-tech companies in the area between San Francisco and San Jose, California)C IBMD Microsoft1 has lots of companies that are secretive, and don’t communicate or collaborate with each other. (A)2 has lots of companies that compete with each other but communicate ideas and information. (B)3 has always had lots of semi-independent units competing within the same company, while communicating with each other. (D)4 is organized in an unusual but very effective way (D)5 is currently the center of innovation (B)6 used to have insulated groups that did not communicate with each other (C)7 used to lead the industrial world in scientific creativity and imagination (A)8 was very successful, then less successful and is now innovative again because it changed the way it was organized (C)2 Working in pairs, rearrange the following part-sentences to make up a short paragraph summarizing Diamond’s ideas about the best form of business organization.A and regularly engage staff who have worked for your competitors.B are at a disadvantage,C because most groups of people getD but also communicate with each other quite freely.E creativity, innovation, and wealth,F into a number of groups which competeG Isolated companies or groupsH most of their ideas and innovations from the outside.I So order to maximize productivity,J You should also exchange ideas and information with other companies,K you should break up your businessIsolated companies or groups are at a disadvantage, because most groups of people get most of their ideas and innovations from the outside. So order to maximize productivity, creativity, innovation, and wealth, you should break up your business into a number of groups which compete but also communicate with each other quite freely. You should also exchange ideas and information with other companies, and regularly engage staff who have worked for your competitors.3a DiscussionRead the following statements, and decide whether they are about the advantages of working in a big or small company.Advantages of working in a small company: 2, 3, 4, 7, 9, 11, 13.Advantages of working in a big company: 1, 5, 6, 7, 8, 10, 12, 14.Some of these answers are open to discussion. For example, number 8: some people might argue that you have a better possibility of realizing your potential in a small company in which you are required to take on a number of different tasks.New words in this unit 03Autonomous, boss, chain of command, Chief Executive Officer (CEO), collaboration, competitor, corporate ethos, decentralization, department, division, downsizing, downturn, finance, fragmentation, functional organization, hierarchy, input, insulated, isolated, level, line authority, Managing Director, marketing, output, personnel, position, President, production, productivity, reorganization, report to, responsibility, salary, sales, subsidiary, wealth.。
structure-2.3---中文使用手册

Structure 2.3中文使用手册Jonathan K. Pritchard aXiaoquan Wen aDaniel Falush b 1 2 3a芝加哥大学人类遗传学系b牛津大学统计学系软件来自/structure.html2010年2月2日1我们在Structure项目中的其他的同事有Peter Donnelly、Matthew Stephens和Melissa Hubisz。
2开发这个程序的第一版时作者(JP、MS、PD)在牛津大学统计系。
3关于Structure的讨论和问题请发给在线的论坛上:***********************************。
在邮递问题之前请查对这个文档并搜索以前的讨论。
1 引言程序Structure使用由不连锁的标记组成的基因型数据实施基于模型的聚类方法来推断群体结构。
这种方法由普里查德(Pritchard)、斯蒂芬斯(Stephens)和唐纳利(Donnelly)(2000a)在一篇文章中引入,由Falush、斯蒂芬斯(Stephens)和普里查德(Pritchard)(2003a,2007)在续篇中进行了扩展。
我们的方法的应用包括证明群体结构的存在,鉴定不同的遗传群体,把个体归到群体,以及鉴定移居者和掺和的个体。
简言之,我们假定有K个群体(这里K可能是未知的)的一个模型,每个群体在每个位点上由一组等位基因频率来刻画。
样本内的个体被(按照概率)分配到群体,或共同分配到两个或更多个群体,如果它们的基因型表明它们是混和的。
假定在群体内,位点处于哈迪-温伯格平衡和连锁平衡。
不精确地讲,个体被按达到这一点那样的方法指定到群体。
我们的模型不假定一个特别的突变过程,并且它可以应用于大多数通常使用的遗传标记,包括微卫星(microsatellites)、SNP和RFLP。
模型假定在亚群体内标记不处于连锁不平衡(LD),因此我们不能处理极其靠近的标记。
从2.0版开始,我们现在能够处理弱连锁的标记。
coordinate structure语言学 -回复
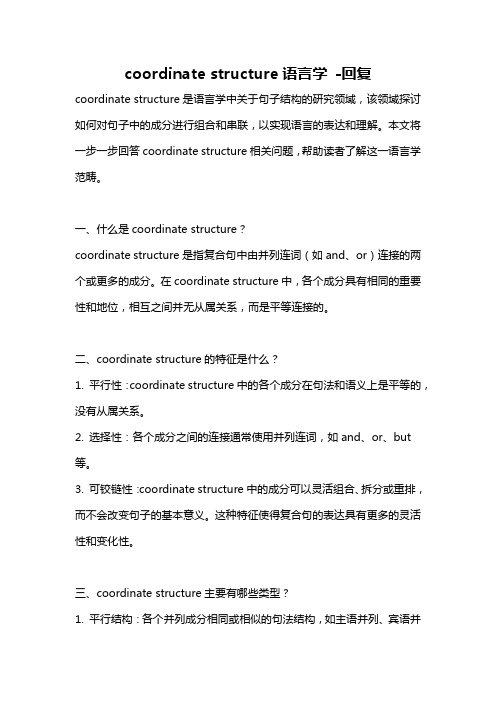
coordinate structure语言学-回复coordinate structure是语言学中关于句子结构的研究领域,该领域探讨如何对句子中的成分进行组合和串联,以实现语言的表达和理解。
本文将一步一步回答coordinate structure相关问题,帮助读者了解这一语言学范畴。
一、什么是coordinate structure?coordinate structure是指复合句中由并列连词(如and、or)连接的两个或更多的成分。
在coordinate structure中,各个成分具有相同的重要性和地位,相互之间并无从属关系,而是平等连接的。
二、coordinate structure的特征是什么?1. 平行性:coordinate structure中的各个成分在句法和语义上是平等的,没有从属关系。
2. 选择性:各个成分之间的连接通常使用并列连词,如and、or、but 等。
3. 可铰链性:coordinate structure中的成分可以灵活组合、拆分或重排,而不会改变句子的基本意义。
这种特征使得复合句的表达具有更多的灵活性和变化性。
三、coordinate structure主要有哪些类型?1. 平行结构:各个并列成分相同或相似的句法结构,如主语并列、宾语并列、谓语并列等。
例如:“David reads books and plays basketball.”2. 不对称结构:并列成分之间在句法结构或语义上存在差异,但仍然保持并列关系。
例如:“She likes swimming but doesn't like running.”3. 多元结构:一个并列连接词连接三个或更多成分,构成复杂的coordinate structure。
例如:“He is tall, handsome, and intelligent.”四、coordinate structure的句法分析方法是什么?在句法分析中,coordinate structure可以使用结构分析法或标记法进行分析。
MFC对话框使用CPrintDialog实现打印,指定打印机、后台打印

MFC对话框使用CPrintDialog实现打印,指定打印机、后台打印对话框打印,网上一搜一大堆,基本分2类:A类:CPrintDialog.DoModal,然后在模态对话框里选打印机、打印配置;B类:GetPrinterDeviceDefaults,调用默认打印机。
我的工作内容是理解以上2类后,再根据MSDN,实现MDF对话框后台指定打印机打印。
废话不多说,上菜~功能:基于对话框的MFC打印(非文档视图结构),指定打印机,后台打印(不弹出对话框)思路:1、枚举打印机,并选择其中一个;2、CPrintDialog实例指定到选中的打印机;3、CPrintDialog后台打印具体实现:1、变量(控件)。
在对话框上添加一个combobox (IDC_COMBO1,对应变量m_cboPrint)、一个edit (IDC_EDIT1),edit允许回车,多行(代码就不贴了,知道MFC应该就懂);2、在OnInitDialog里枚举打印机设备,如果报函数未定义,加入头文件#include <winspool.h>需要调用两次EnumPrinters函数,第一次的到结构体的大小,第二次得到打印机列表// TODO: 在此添加额外的初始化代码DWORD dwNeeded;DWORD dwReturn;DWORD dwFlag = PRINTER_ENUM_CONNECTIONS | PRINTER_ENUM_LOCAL;EnumPrinters(dwFlag, NULL, 4, NULL, 0, &dwNeeded, &dwReturn);PRINTER_INFO_4* p4;p4 = new PRINTER_INFO_4[dwNeeded];EnumPrinters(dwFlag, NULL, 4, (PBYTE)p4, dwNeeded, &dwNeeded, &dwReturn);for (int i = 0; i<(int)dwReturn; i++)this->m_cboPrint.AddString(p4[i].pPrinterName);delete []p4;3、操作对话框,在IDC_EDIT1里输入打印的内容,在IDC_COMBO1里选中打印机;4、打印(我是用OK按钮打印的,大家随便)// TODO: 在此添加控件通知处理程序代码// CDialogEx::OnOK();this->UpdateData();CString strMessage;CString strPrintDevice;this->GetDlgItem(IDC_EDIT1)->GetWindowT extW(strMessa ge);strMessage += _T("\r\n"); //添加结尾,方便后面循环读取打印数据this->GetDlgItem(IDC_COMBO1)->GetWindowTextW(strPri ntDevice);DWORD dwFlag = PD_ALLPAGES | PD_NOPAGENUMS | PD_USEDEVMODECOPIES | PD_HIDEPRINTTOFILE; //打印配置界面的按钮可用性,因为后台打印,其实这个配置没什么意义CPrintDialog pPrintdlg(FALSE, dwFlag, this);//CPrintDialog实例化,因为MFC的打印设备无关性,可以理解为这就是一台打印机HGLOBAL hDevMode = NULL;HGLOBAL hDevNames = NULL;if (GetPrinterDevice(strPrintDevice.GetBuffer(0), &hDevNames, &hDevMode)) //获得指定打印机的配置、名字AfxGetApp()->SelectPrinter(hDevNames, hDevMode);elseAfxMessageBox(_T("Failed to select custom printer"));strPrintDevice.ReleaseBuffer();pPrintdlg.m_pd.hDevMode = hDevMode;//让pPrintdlg使用我们指定的打印机pPrintdlg.m_pd.hDevNames = hDevNames;CDC dc;dc.Attach(pPrintdlg.CreatePrinterDC());//后台打印创建法,如果需要弹出打印对话框,请用DoModalDOCINFO di;//下面的内容网上很多,就不解释了di.cbSize = sizeof(DOCINFO);di.lpszDocName = _T("有驱打印测试");di.lpszDatatype = NULL;di.lpszOutput = NULL;di.fwType = 0;dc.StartDocW(&di);dc.StartPage();dc.SetMapMode(MM_TEXT);CRect recPrint(0, 0, dc.GetDeviceCaps(LOGPIXELSX), dc.GetDeviceCaps(LOGPIXELSY));dc.DPtoLP(&recPrint);dc.SetWindowOrg(0, 0);CFont newFont;VERIFY(newFont.CreatePointFont(120, _T("宋体"), &dc));CFont* oldFont = dc.SelectObject(&newFont);dc.SetTextAlign(TA_TOP | TA_LEFT);CString strPrint;int nIndex = 0;int x = 50;int y = 50;CSize textSize;textSize = dc.GetT extExtent(_T("00"), 2);//根据当前字体的宽、高,后面以此高度为行高while ((nIndex = strMessage.Find(_T("\r\n"))) > -1)//将IDC_EDIT1编辑框中内容打印,支持换行,一次换行等于'\r\n',所以在开头strMessage += _T("\r\n") {strPrint = strMessage.Left(nIndex);strMessage = strMessage.Mid(nIndex+2);dc.TextOutW(x, y, strPrint);y += textSize.cy;//下移一行,行高为字体高度}dc.SelectObject(oldFont);newFont.DeleteObject();dc.EndPage();dc.EndDoc();DeleteDC(dc.Detach());关于GetPrinterDevice,来自微软的一篇文章,点我跳转代码也贴出来BOOL CMFCApplication2Dlg::GetPrinterDevice(LPTSTR pszPrinterName, HGLOBAL* phDevNames, HGLOBAL* phDevMode){// if NULL is passed, then assume we are setting app object's// devmode and devnamesif (phDevMode == NULL || phDevNames == NULL)return FALSE;// Open printerHANDLE hPrinter;if (OpenPrinter(pszPrinterName, &hPrinter, NULL) == FALSE) return FALSE;// obtain PRINTER_INFO_2 structure and close printerDWORD dwBytesReturned, dwBytesNeeded;GetPrinter(hPrinter, 2, NULL, 0, &dwBytesNeeded);PRINTER_INFO_2* p2 = (PRINTER_INFO_2*)GlobalAlloc(GPTR, dwBytesNeeded);if (GetPrinter(hPrinter, 2, (LPBYTE)p2, dwBytesNeeded,&dwBytesReturned) == 0) {GlobalFree(p2);ClosePrinter(hPrinter);return FALSE;}ClosePrinter(hPrinter);// Allocate a global handle for DEVMODEHGLOBAL hDevMode = GlobalAlloc(GHND, sizeof(*p2->pDevMode) +p2->pDevMode->dmDriverExtra);ASSERT(hDevMode);DEVMODE* pDevMode = (DEVMODE*)GlobalLock(hDevMode);ASSERT(pDevMode);// copy DEVMODE data from PRINTER_INFO_2::pDevMode memcpy(pDevMode, p2->pDevMode, sizeof(*p2->pDevMode) +p2->pDevMode->dmDriverExtra);GlobalUnlock(hDevMode);// Compute size of DEVNAMES structure from PRINTER_INFO_2's dataDWORD drvNameLen = lstrlen(p2->pDriverName)+1; // driver nameDWORD ptrNameLen = lstrlen(p2->pPrinterName)+1; // printer nameDWORD porNameLen = lstrlen(p2->pPortName)+1; // port name// Allocate a global handle big enough to hold DEVNAMES.HGLOBAL hDevNames = GlobalAlloc(GHND,sizeof(DEVNAMES) +(drvNameLen + ptrNameLen + porNameLen)*sizeof(TCHAR));ASSERT(hDevNames);DEVNAMES* pDevNames = (DEVNAMES*)GlobalLock(hDevNames);ASSERT(pDevNames);// Copy the DEVNAMES information from PRINTER_INFO_2 // tcOffset = TCHAR Offset into structureint tcOffset = sizeof(DEVNAMES)/sizeof(TCHAR);ASSERT(sizeof(DEVNAMES) == tcOffset*sizeof(TCHAR));pDevNames->wDriverOffset = tcOffset;memcpy((LPTSTR)pDevNames + tcOffset, p2->pDriverName, drvNameLen*sizeof(TCHAR));tcOffset += drvNameLen;pDevNames->wDeviceOffset = tcOffset;memcpy((LPTSTR)pDevNames + tcOffset, p2->pPrinterName,ptrNameLen*sizeof(TCHAR));tcOffset += ptrNameLen;pDevNames->wOutputOffset = tcOffset;memcpy((LPTSTR)pDevNames + tcOffset, p2->pPortName, porNameLen*sizeof(TCHAR));pDevNames->wDefault = 0;GlobalUnlock(hDevNames);GlobalFree(p2); // free PRINTER_INFO_2// set the new hDevMode and hDevNames*phDevMode = hDevMode;*phDevNames = hDevNames;return TRUE;}基本上是完整代码了,如果有内存错误,请联系我。
structure中文说明

1、待分析数据文件的编辑
可新建文本文件并命名为project_data,以文本编辑的方式编辑数列:
第一列:样品代码,每一样品占两行,每一行为其一个基因型,如样品1的基因型为AA,样品2的基因型为AT,样品3的基因型为TT,则编辑为:
1 1
1 1
2 1
2 2
柱形图绘制软件Distruct使用指南
当Structure软件运行完毕获得结果后,往往需要以CLUMPP或distruct软件绘制柱形图。distruct用法如下:
1、从/distruct.html下载并解压缩Distruct 1.1压缩包,可以看到含7个以casia为名的文件及5个名字字首为distruct的文件,还有一个drawparams的文件和一个颜色文件夹;
4、STEP 2-->填入待分析样品数量,如220-->Ploidy of data即选择单倍体或二倍体,选2-->Number of loci,选位点个数-->MiMissing data value,一般选-9;
5、STEP 3-->依次选择row of marker names, row of recessive alleles, map distance between loci, phase information等,没有就不选;最下面,如果没有data file stores data for individuals in a single line就不选;
54 Hazara
629 Uygur
699 Yakut
56 Kalash"
替换为现内容
"1 CEU
2 CHB
3 YRI"
coordinate structure语言学

coordinate structure语言学Coordinate Structure语言学,又称协调结构语言学,是一种研究句子结构的语言学理论。
它关注的是句子中并列的成分(如词语、短语、从句等)的组合方式和语义关系。
Coordinate Structure语言学通过研究并列结构的构成、功能和语义等方面,揭示了语言表达中并列关系的重要性,并对句子的结构和语义进行了深入探讨。
在Coordinate Structure语言学中,最基本的概念是并列关系(coordination)。
并列是指两个或多个成分在语法上平等地连接在一起,且彼此之间具有对等的语义关系。
在句子中,这些成分可以是词语、短语、从句等,它们通过连词(如“和”、“或”、“而”等)或标点符号(如逗号、分号等)进行连接。
并列结构可以出现在主语、谓语、宾语、状语、定语等句子成分中,表达出并列关系的同时保持句子结构的平衡和对称。
通过并列结构,人们可以在句子中传达出多个成分的相互关系,增强句子的表达力和信息量。
在Coordinate Structure语言学中,还有一些重要的概念需要理解。
首先是对等连接(parataxis),指的是成分之间没有层级关系,只是通过连词或标点符号连接在一起;而非对等连接(hypotaxis)则指的是成分之间存在层级关系,其中的一部分成分作为主要成分,而其他成分则作为从属成分。
其次,是并列句(coordinate clause)和并列成分(coordinate constituent)的区分。
并列句是完整的、可以独立成句的句子,它具有主语、谓语和其他必要的成分;而并列成分则是句子中的独立于句子类型的附属成分,它可以是独立的词语、短语、从句等,并列成分之间的关系相对自由。
Coordinate Structure语言学主要关注句子的结构和语义,通过对并列结构的分析和研究,可以揭示出句子中成分之间的关系和作用。
例如,在主语并列结构中,两个或多个主语共同表示出动作的执行者,这体现了人们将不同的实体并列起来在句子中表达的需求。
三维工业产品数据可视化应用格式规范-最新国标

目次1范围 (1)2规范性引用文件 (1)3术语和定义 (1)4缩略语 (2)5 概述 (3)5.1 格式内容 (3)5.2 格式特性 (3)5.3 扩展名 (3)6 文件结构 (3)6.1 结构 (4)6.2 文件头 (4)6.3 索引表 (5)6.4 数据块 (6)6.5 场景图 (6)7 数据对象 (7)7.1 场景对象 (7)7.2 产品结构树对象 (8)7.3 模型对象 (9)7.4 网格对象 (9)7.5 几何拓扑对象 (12)7.6 属性对象 (17)7.7 材质对象 (18)7.8 摄像机对象 (21)7.9 灯光对象 (22)7.10 标注对象 (25)7.11 审阅对象 (27)7.12 场景视图对象 (27)7.13 动画对象 (28)7.14 资源文件对象 (31)7.15 安全控制对象 (31)7.16 外部链接对象 (31)7.17 用户自定义对象 (32)附录A(规范性)基本类型说明 (33)附录B(规范性)扩展类型说明 (34)附录C(规范性)数据来源类型说明 (37)附录D(规范性)曲面类型定义 (39)三维产品数据可视化应用格式规范1 范围本文件规定了三维产品数据的文件结构,以及场景、产品结构树、模型、网格、几何拓扑、属性、材质、摄像机、标注、审阅、场景视图、动画、资源文件、安全控制、外部链接等数据对象的数据格式。
本文件适用于三维产品数据的存储、交换和利用。
2 规范性引用文件下列文件中的内容通过文中的规范性引用而构成本文件必不可少的条款。
其中,注日期的引用文件,仅该日期对应的版本适用于本文件;不注日期的引用文件,其最新版本(包括所有的修改单)适用于本文件。
GB/T 131—2009 产品几何技术规范(GPS)技术产品文件中表面结构的表示法GB/T 1988—1998 信息技术信息交换用七位编码字符集GB/T 24734.9—2009 技术产品文件数字化产品定义数据通则第9部分:基准的应用GB∕T 24734.10—2009 技术产品文件数字化产品定义数据通则第10部分:几何公差的应用3 术语和定义下列术语和定义适用于本文件。
coordinate structure 的例子

coordinate structure 的例子Coordinate structure例子在语法学中,coordinate structure(协同结构)是一种由两个或多个相同类型的词、短语或从句组成的结构。
这些成分通过连接词(如”and”、“or”、“but”等)进行连接,构成一个整体的句子成分。
以下是一些常见的coordinate structure例子,并对其进行详细的讲解:1. 词的coordinate structure•例子1:I like swimming and running.•讲解:这个例子中,词”swimming”和”running”是两个并列的动词,它们都在句子中作谓语。
它们通过连接词”and”来形成coordinate structure。
2. 短语的coordinate structure•例子2:She bought a dress and a pair of shoes.•讲解:这个例子中,短语”a dress”和”a pair of shoes”都是并列的宾语短语,它们通过连接词”and”来形成coordinate structure。
3. 从句的coordinate structure•例子3:She said that she loves reading and that she loves writing.•讲解:这个例子中,两个从句”she loves reading”和”she loves writing”都是并列的宾语从句,它们通过连接词”and”来形成coordinate structure。
4. 句子的coordinate structure•例子4:He went to the store, bought some groceries, and went back home.•讲解:这个例子中,三个句子”he went to the store”、“bought some groceries”和”went back home”都是并列的简单句,它们通过连接词”and”来形成coordinate structure。
英文合同中structure

英文合同中structureA contract is a legally binding agreement between two or more parties that outlines the terms and conditions of their relationship. It is essential to have a well-structured contract to ensure clarity, enforceability, and the protection of the parties involved. In this article, we will discuss the key elements and structure of an English contract.1. Introduction:The contract should begin with an introduction that clearly identifies the parties involved and their roles. It should state the purpose of the contract and provide a brief overview of its contents. This section sets the context for the entire agreement.2. Definitions:To avoid any ambiguity, it is important to define key terms used throughout the contract. This section should provide clear definitions of specific words or phrases that may have different interpretations. Definitions ensure that all parties have a shared understanding of the contract's language.3. Scope of Work:The scope of work section outlines the specific tasks, responsibilities, and deliverables of each party. It should clearly define what is expected from each party, including timelines, milestones, and any relevant specifications. This section helps to manage expectations and avoid misunderstandings.4. Payment Terms:The payment terms section specifies the agreed-upon payment amount, schedule, and method. It should include details such as the currency, due dates, and any applicable penalties or late fees. Clear payment terms ensure transparency and help prevent disputes related to financial obligations.5. Intellectual Property:If the contract involves the creation or use of intellectual property, this section should address ownership rights, licensing, and any restrictions. It should clearly define who owns the intellectual property and how it can be used or transferred. This protects the parties' rights and avoids future disputes.6. Confidentiality:Confidentiality provisions protect sensitive information shared between the parties. This section should outline what information is considered confidential, how it should be handled, and any limitations on its disclosure. Confidentiality clauses help maintain trust and prevent unauthorized disclosure of sensitive information.7. Termination:The termination section specifies the conditions under which either party can end the contract. It should outline the notice period required, any penalties or consequences, and any obligations that continue after termination. Clear termination provisions provide a framework for ending the contract if necessary.8. Dispute Resolution:In the event of a dispute, the contract should include a dispute resolution clause. This section outlines the preferred method of resolving conflicts, such as negotiation, mediation, or arbitration. It may also specify the jurisdiction or governing law applicable to the contract. A well-defined dispute resolution process helps avoid costly litigation.9. Governing Law and Jurisdiction:This section determines which laws and jurisdiction will govern the contract. It may specify the state or country whose laws will apply to the agreement. This ensures consistency and provides a legal framework for resolving any disputes that may arise.10. Miscellaneous:The miscellaneous section includes any additional provisions that are not covered in the previous sections but are relevant to the contract. This may include force majeureclauses, amendments, waivers, or any other terms that the parties deem necessary to include.In conclusion, a well-structured contract is crucial for establishing clear expectations, protecting the rights of the parties involved, and minimizing potential disputes. By following a logical structure and including the necessary elements, an English contract can effectively outline the terms and conditions of a business relationship.。
paragraphstructure

paragraphstructureParagraph StructureReviewRemoval of potassium perchlorate was achieved by centrifugation of the supernatant liquid at 1400 X g for 10 min.D1-like receptors exert a permissive or enabling regulation of D2-like receptors.The patients were instructed to eat regular meals, that they should drink plenty of water, and they should take adequate exercise. ?The mechanical response of heart muscles depends on both the absolute osmolal increase and on the species studied.Total microsphere losses were greater at 34, 64, and 124 min when compared to 4 min.Losses of apolipoprotein A-I during other isolation methods were smaller in comparison to ultracentrifugation.Title: “Bloo d-Brain Barrier CSF pH Regulation” (CSF=cerebrospinal fluid)Factors influencing primary liver cancer resection survival rateGrammar MistakesThe esophagus, stomach, and duodenum of each rabbit was examined.The tissue was minced and the samples incubated. ?Blood flow was allowed to return to baseline before proceeding with the next occlusion.Paragraph StructureEven if a paper has perfect word choice and perfect sentence structure, it can be difficult to understand if theparagraphs are not clearly constructed.Each paragraph must be constructed to tell a story. ?Readers should be able to recognize themessage and follow the story of eachparagraph whether or not theyunderstand the science.Three AspectsFor a paragraph to tell a clear story,–The ideas in the paragraph must be organized.–The continuity, that is, the relationship between ideas, must be clear.–Important ideas must be emphasized.–Paragraph development–Continuity–EmphasisParagraph Development先概要,后细节Topic sentence first, then supporting sentence. ?Create a expectation, and then fulfill the expectation.Patterns of the organization ?Least to most importanceMost to least importanceAnnounced orderChronological orderProblem-solutionFunnelPro-conContinuityContinuity is the smooth flow of ideas from sentence to sentence (and from paragraph to paragraph).The essence of continuity is a clear relationship between every sentence and the sentence before it.Continuity: five skills1. Repeat the key terms exactly and early2. use transitions3. keep a consistent order4. keep a consistent point of view5. signal subtopics within a paragraphRepeat the key termsKey terms are terms that name important ideas in a paper. ?Key terms can be technical terms, such as “G-protein” or “mitogenesis,” or nontechnical terms such as “increase” or “function.”Repeating key terms from sentence to sentence (and from paragraph to paragraph) is the strongest technique for providing continuity.Digitalis increases the contractility of the mammalian heart. This change in inotropic state is a result of changes in calcium flux through the muscle cell membrane. ?Digitalis increases the contractility of the mammalian heart. Changes in the in calcium flux through the musclecell membrane cause this increased contractility. ?Digitalis increases the contractility of the mammalian heart. This increased contractility is a result of changes in calcium flux through the muscle cell membrane.Q : Compare the three sentences and decide which is the best. PS: Digitalis 洋地黄,用于治疗心力衰竭inotropic 肌肉收缩力的calcium flux 钙通量Repeat Key Terms ExactlyThe best advice for clear continuity is to repeat key terms exactly. If a key term is not repeated exactly and insteadanother term is used, a mental manipulation is needed tosee the relationship between the two terms. Readersfamiliar with the field can usually see the relationship; those less familiar may not.Repeat the Key Terms Early ?Continuity is clearest if the key term is repeated early in the sentence. If the key term is delayed until the end of thesentence, the continuity is broken and the reader is kept in suspense temporarily.The more delayed the repetition of the key term, the less obvious the continuity. The reason is that more and more new key terms are added before the relationship between the two sentences is clear.Repeat Key Terms Exactlyto Avoid “Noise”You are taught not to repeat the same word twice in a sentence, a paragraph, or some other limit. ??(1) In humans, apo-B100, mainly synthesized in the liver, and apo-B48,mainly synthesized in the intestine, are the products of a single apo-B gene (ref). (2) The production of apo-48 in the human intestine is the result of an RNA-editing process that changes a glutamine codon of the mRNA forapo-B100 into a translational stop codon. (3) This apo-B mRNA-editing process does not occur in human livers, so apo-B48 is not synthesized in human livers. (4) However, the mRNA-editing process, and thus apo-B48 formation, occurs in mouse and rat livers.Link the Key Terms ?specific term & category term(A) The v-erbB gene is related to the neu oncogene. Bothoncogenes have…(B) The v-erbB gene, an oncogene of the avianerythroblastosis virus, is related to the neu oncogene. Both oncogenes have…Link the Key TermsHow to link:Place the definition either right after or right before the term to be defined.Set off the item in the “after” position by commas. ?Check that the definition repeats a key term from the previous sentence or prepares for a key term in the nextsentence.The family of TGF-signaling molecules play inductive roles in various developmental contexts. One member of this family, Drosophila Decapentaplegic (Dpp), serves as amorphogen that patterns both the embryo and adult.Use TransitionsUse transitions to indicate relationship ?Transition word (Conjunctions: furthermore, however, nevertheless ……) Transition phraseTransition clauseExerciseDirection:Below are three versions of two sentences from a Methods section. For each version, state (1) what the logical relationship of the second sentence to the first sentence is and (2) how you know what the relationship is.Version 1:The microspheres were prepared for injection as previously described. They were then suspended in 1 ml of dextran solution in a glass injection vial that was connected to the appropriate catheter and to a syringe containing 4 ml ofsaline.Version 2: The microspheres were prepared for injection as previously described. In brief, they were suspended in 1 ml of dextran solution ina glass injection vial that was connected to the appropriate catheterand to a syringe containing 4 ml of saline. ?Relationship:How You Know:Transition phraseTwo types:Prepositional phraseInfinitive phrasePrepositional phrase(1) Our aim was to assess the mechanisms involved in the beneficial effects of hydralazine on ventricular function in patients who have chronic aortic insufficiency. (2) For this assessment, we did a radionuclide study of ventricular function in 15 patients at rest and during supine exercise.Infinitive phrase(1) The effects of intra-arterial pressure gradients onsteady-state circumflex pressure-flow relations derived during long diastoles were examined in five dogs. (2) T o obtain each pressure-flow point, we first set mean circumflex pressure to the desired level and then arrested the heart by turning off the peace-maker.Transition phraseTransition phrase connects the sentences in three ways: ?First, the preposition/ infinitive itself indicates a logical relationship.Second, the object of the preposition/infinitive completes the logic(for what? in what? To do what?)?Finally, the object ofthe preposition/infinitive repeats a key term, thus further connecting the two sentences.Transition clauseTransition clauses, like transition phrases, keep the story ofa paragraph going by stating the logical relationshipbetween two sentences.The only difference is in the form: a transition clause uses a subject and verb to indicate the logical relationshipbetween ideas, whereas a transition phrase uses apreposition or an infinitive and an object. The subject in the transition clause, like the object in a transition phrase, is usually a repeated key term.(1) Our findings demonstrate that in patients with clinically moderate to severe congestive heart failure and left ventricular dysfunction, the arteriolar vasodilator hydralazine produces significant hemodynamic benefits independent of the presence or absence of mitral regurgitation. (2) The benefits we found were significant increase in cardiac index, stroke volume index, and stroke work index, and significant decreases in systemic vascular resistance in all patients.The Placement of Transitions ?Continuity is strongest and the story in a paragraph is clearest when transition words, phrases, and clauses are placed at the beginning of a sentence.Keep a Consistent OrderIf you list two or more items in a topic sentence and then go on to describe or explain them in supporting sentences, keep the same order:if the items in the topic sentence are A, B, C, the supporting sentences should explain first A, then B, and last C. ?Furthermore, the supporting sentences should include all the items mentionedin the topic sentence and should notadd any items not mentioned in the topic sentence.Keep a Consistent Point of View ?Having the same subject in two or more sentences that deal with the same topic is called keeping a consistent point of view.Specifically, the point of view is consistent when the same term, or the same category of term, is the subject ofsuccessive sentences that deal with the same topic. ?The point of view is inconsistent when the topic is the same but the subjects of the sentences are different. Aninconsistent point of view is disorienting to the reader, making similarities and differences difficult to see.Keep a Consistent Point of View ?Point of view: the perspective of the narration or argumentation在论著写作中,视角就是叙述问题的角度,也就是表达句子主题的主语的类型。
structure的用法和短语例句

structure的用法和短语例句structure有结构;构造;建筑物等意思,那么你知道structure的用法吗?下面跟着店铺一起来学习一下,希望对大家的学习有所帮助!structure的用法:structure的用法1:structure用作名词时意思是“结构”,转化为动词后意思是“组织”“安排”“构造”。
structure的用法2:structure只用作及物动词,接名词、代词作宾语,可用于被动结构。
structure的常用短语:用作动词 (v.)structure for (v.+prep.)为…组织structure的用法例句:1. The theatre is a futuristic steel and glass structure.这家剧院是钢筋和玻璃结构的未来派建筑。
2. Torn muscles retract, and lose strength, structure, and tightness.撕裂的肌肉会收缩,丧失原来的力量、结构和紧实度。
3. My grumble is with the structure and organisation of the material.我所不满的是素材的结构和组织。
4. The chronological sequence gives the book an element of structure.时间顺序让这本书有了一定的结构。
5. The chemical structure of this particular molecule is very unusual.这个特殊分子的化学结构很不寻常。
6. The Congress voted down a motion to change the union's structure.国会投票否决了改变工会结构的动议。
7. Air ducts and electrical cables were threaded through the complex structure.通风管和电缆在这幢结构复杂的建筑物中穿过。
英语五种建筑构造简介PPT

2 3 4
5
Bent structure Shear wall structure
Tube structure
01
Frame structure 框架结构
Frame structure
Frame structure
The frame structure refers to the structure of the bearing system, which is formed by the connection of Liang Hezhu with steel bar, which is the horizontal load and vertical load which appear in the course of the use of Liang Hezhu. The housing wall frame structure not only plays the loadbearing, surrounding and separating effect, general use of aerated concrete, prefabricated expanded perlite, porous brick, hollow brick or pumice, vermiculite, ceramsite lightweight masonry or plate assembly. 框架结构是指由梁和柱以钢筋相连接而成,构成承重体系的结构,即由梁和柱组成框架共同抵抗使用过程中 出现的水平荷载和竖向荷载。框架结构的房屋墙体不承重,仅起到围护和分隔作用,一般用预制的加气混凝土、 膨胀珍珠岩、空心砖或多孔砖、浮石、蛭石、陶粒等轻质板材砌筑或装配而成。
Frame structure
Deep Structure and Surface Structure
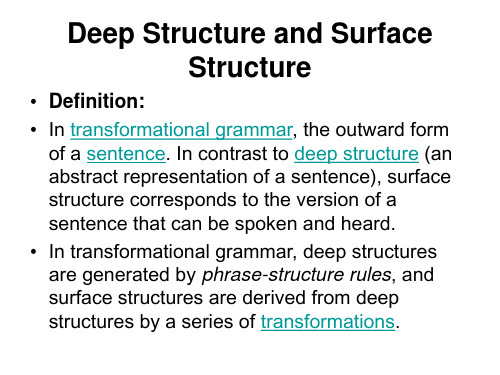
• Definition: • In transformational grammar, the outward form of a sentence. In contrast to deep structure (an abstract representation of a sentence), surface structure corresponds to the version of a sentence that can be spoken and heard. • In transformational grammar, deep structures are generated by phrase-structure rules, and surface structures are derived from deep structures by a series of transformations.
The distinction between ‘deep structure’ and ‘surface structure’ permits us to explain ambiguous sentences such as ‘I have seen eating a rabbit’ that can have two interpretations . • 1) I have seen someone eating a rabbit • 2) I have seen a rabbit eating something.
• Examples: Boy loves Girl (deep structure). The boy kissed the girl (surface structure). The boy was kissing the girl. The girl was kissed by the boy. (surface and deep structure).
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
DOCINFO structure
3 out of 3 rated this helpful - Rate this topic
The DOCINFO structure contains the input and output file names and other information used by the StartDoc function.
Syntax
C++
Copy
typedef struct {
int cbSize;
LPCTSTR lpszDocName;
LPCTSTR lpszOutput;
LPCTSTR lpszDatatype;
DWORD fwType;
} DOCINFO, *LPDOCINFO;
Members
cbSize
The size, in bytes, of the structure.
lpszDocName
Pointer to a null-terminated string that specifies the
name of the document.
lpszOutput
Pointer to a null-terminated string that specifies the
name of an output file. If this pointer is NULL, the
output will be sent to the device identified by the
device context handle that was passed to the
StartDoc function.
lpszDatatype
Pointer to a null-terminated string that specifies the
type of data used to record the print job. The legal
values for this member can be found by calling
EnumPrintProcessorDatatypes and can include
such values as raw, emf, or XPS_PASS. This
member can be NULL. Note that the requested data
type might be ignored.
fwType
Specifies additional information about the print job.
This member must be zero or one of the following
values.
Examples
For a sample program that uses this structure, see How To: Print Using the GDI Print API. Requirements
Minimum supported client Windows 2000 Professional [desktop apps only]
Minimum supported server Windows 2000 Server [desktop apps only]
Header Wingdi.h (include Windows.h)
See also
Printing
Print Spooler API Structures
StartDoc。