17-BonjourAndStreams
CFNetwork

CFNetwork Programming Guide Networking&Internet2011-06-06Apple Inc.©2011Apple Inc.All rights reserved.No part of this publication may be reproduced, stored in a retrieval system,or transmitted,in any form or by any means,mechanical, electronic,photocopying,recording,or otherwise,without prior written permission of Apple Inc.,with the following exceptions:Any person is hereby authorized to store documentation on a single computer for personal use only and to print copies of documentation for personal use provided that the documentation contains Apple’s copyright notice.The Apple logo is a trademark of Apple Inc. No licenses,express or implied,are granted with respect to any of the technology described in this document.Apple retains all intellectual property rights associated with the technology described in this document.This document is intended to assist application developers to develop applications only for Apple-labeled computers.Apple Inc.1Infinite LoopCupertino,CA95014408-996-1010Apple,the Apple logo,Bonjour,Cocoa,iChat, Keychain,Mac,Mac OS,Objective-C,and Safari are trademarks of Apple Inc.,registered in the United States and other countries.IOS is a trademark or registered trademark of Cisco in the U.S.and other countries and is used under license.UNIX is a registered trademark of The Open GroupEven though Apple has reviewed this document, APPLE MAKES NO WARRANTY OR REPRESENTATION, EITHER EXPRESS OR IMPLIED,WITH RESPECT TO THIS DOCUMENT,ITS QUALITY,ACCURACY, MERCHANTABILITY,OR FITNESS FOR A PARTICULAR PURPOSE.AS A RESULT,THIS DOCUMENT IS PROVIDED“AS IS,”AND YOU,THE READER,ARE ASSUMING THE ENTIRE RISK AS TO ITS QUALITY AND ACCURACY.IN NO EVENT WILL APPLE BE LIABLE FOR DIRECT, INDIRECT,SPECIAL,INCIDENTAL,OR CONSEQUENTIAL DAMAGES RESULTING FROM ANY DEFECT OR INACCURACY IN THIS DOCUMENT,even if advised of the possibility of such damages. THE WARRANTY AND REMEDIES SET FORTH ABOVE ARE EXCLUSIVE AND IN LIEU OF ALL OTHERS,ORAL OR WRITTEN,EXPRESS OR IMPLIED.No Apple dealer,agent,or employee is authorized to make any modification,extension,or addition to this warranty.Some states do not allow the exclusion or limitation of implied warranties or liability for incidental or consequential damages,so the above limitation or exclusion may not apply to you.This warranty gives you specific legal rights,and you may also have other rights which vary from state to state.ContentsIntroduction Introduction to CFNetwork Programming Guide7Organization of This Document7See Also8Chapter1CFNetwork Concepts9When to Use CFNetwork9CFNetwork Infrastructure10CFSocket API10CFStream API10CFNetwork API Concepts11CFFTP API11CFHTTP API12CFHTTPAuthentication API13CFHost API13CFNetServices API14CFNetDiagnostics API14Chapter2Working with Streams17Working with Read Streams17Creating a Read Stream17Reading from a Read Stream18Tearing Down a Read Stream18When all data has been read,you should call the CFReadStreamClose function to close the stream,thereby releasing system resources associated with it.Then release the stream reference bycalling the function CFRelease.You may also want to invalidate the reference by setting it toNULL.See Listing2-4for an example.18Working with Write Streams19Preventing Blocking When Working with Streams20Using a Run Loop to Prevent Blocking20Polling a Network Stream22Navigating Firewalls24Chapter3Communicating with HTTP Servers27Creating a CFHTTP Request27Creating a CFHTTP Response28Deserializing an Incoming HTTP Request28Deserializing an Incoming HTTP Response29Using a Read Stream to Serialize and Send HTTP Requests293CONTENTSSerializing and Sending an HTTP Request30Checking the Response30Handling Authentication Errors31Handling Redirection Errors31Canceling a Pending Request31Chapter4Communicating with Authenticating HTTP Servers33Handling Authentication33Keeping Credentials in Memory37Keeping Credentials in a Persistent Store38Authenticating Firewalls42Chapter5Working with FTP Servers43Downloading a File43Setting Up the FTP Streams43Implementing the Callback Function45Uploading a File46Creating a Remote Directory47Downloading a Directory Listing47Chapter6Using Network Diagnostics51Document Revision History534Figures and ListingsChapter1CFNetwork Concepts9Figure1-1CFNetwork and other software layers on Mac OS X9Figure1-2CFStream API structure11Figure1-3Network diagnostics assistant15Chapter2Working with Streams17Listing2-1Creating a read stream from a file17Listing2-2Opening a read stream17Listing2-3Reading from a read stream(blocking)18Listing2-4Releasing a read stream18Listing2-5Creating,opening,writing to,and releasing a write stream19Listing2-6Scheduling a stream on a run loop21Listing2-7Opening a nonblocking read stream21Listing2-8Network events callback function21Listing2-9Polling a read stream23Listing2-10Polling a write stream23Listing2-11Navigating a stream through a proxy server24Listing2-12Creating a handle to a dynamic store session24Listing2-13Adding a dynamic store reference to the run loop24Listing2-14Loading the proxy dictionary25Listing2-15Proxy callback function25Listing2-16Adding proxy information to a stream25Listing2-17Cleaning up proxy information25Chapter3Communicating with HTTP Servers27Listing3-1Creating an HTTP request27Listing3-2Releasing an HTTP request28Listing3-3Deserializing a message29Listing3-4Serializing an HTTP request with a read stream30Listing3-5Redirecting an HTTP stream31Chapter4Communicating with Authenticating HTTP Servers33Figure4-1Handling authentication34Figure4-2Finding an authentication object34Listing4-1Creating an authentication object35Listing4-2Finding a valid authentication object35Listing4-3Finding credentials(if necessary)and applying them365FIGURES AND LISTINGSListing4-4Applying the authentication object to a request37Listing4-5Looking for a matching authentication object38Listing4-6Searching the credentials store38Listing4-7Searching the keychain40Listing4-8Loading server credentials from the keychain40Listing4-9Modifying the keychain entry41Listing4-10Storing a new keychain entry41Chapter5Working with FTP Servers43Listing5-1A stream structure43Listing5-2Writing data to a write stream from the read stream45Listing5-3Writing data to the write stream46Listing5-4Loading data for a directory listing47Listing5-5Loading the directory listing and parsing it48Chapter6Using Network Diagnostics51Listing6-1Using the CFNetDiagnostics API when a stream error occurs51 6CFNetwork is a framework in the Core Services framework that provides a library of abstractions for network protocols.These abstractions make it easy to perform a variety of network tasks,such as:●Working with BSD sockets ●Creating encrypted connections using SSL or TLS ●Resolving DNS hosts ●Working with HTTP ,authenticating HTTP and HTTPS servers ●Working with FTP servers ●Publishing,resolving and browsing Bonjour services (described in NSNetServices and CFNetServicesProgramming Guide )This book is intended for developers who want to use network protocols in their applications.In order to fully understand this book,the reader should have a good understanding of network programming concepts such as BSD sockets,streams and HTTP protocols.Additionally,the reader should be familiar Mac OS X programming concepts including run loops.For more information about Mac OS X please read Mac OS X Technology Overview .Organization of This DocumentThis book contains the following chapters:●"CFNetwork Concepts" (page 9)describes each of the CFNetwork APIs and how they interact. ●"Working with Streams" (page 17)describes how to use the CFStream API to send and receive networkdata.●"Communicating with HTTP Servers" (page 27)describes how to send and receive HTTP messages. ●"Communicating with Authenticating HTTP Servers" (page 33)describes how to communicate with secure HTTP servers.●"Working with FTP Servers" (page 43)describes how to upload and download files from an FTP server,and how to download directory listings.●"Using Network Diagnostics" (page 51)describes how to add network diagnostics to your anization of This Document7Introduction to CFNetwork Programming GuideIntroduction to CFNetwork Programming GuideSee AlsoFor more information about the networking APIs in Mac OS X,read:●Getting Started With NetworkingRefer to the following reference documents for CFNetwork:●CFFTPStream Reference is the reference documentation for the CFFTPStream API.●CFHTTPMessage Reference is the reference documentation for the CFHTTPMessage API.●CFHTTPStream Reference is the reference documentation for the CFHTTPStream API.●CFHTTPAuthentication Reference is the reference documentation for the CFHTTPAuthentication API.●CFHost Reference is the reference documentation for the CFHost API.●CFNetServices Reference is the reference documentation for the CFNetServices API.●CFNetDiagnostics Reference is the reference documentation for the CFNetDiagnostics API.In addition to the documentation provided by Apple,the following is the reference book for socket-levelprogramming:●UNIX Network Programming,Volume1(Stevens,Fenner and Rudoff)8See AlsoCFNetwork ConceptsCFNetwork is a low-level,high-performance framework that gives you the ability to have detailed controlover the protocol stack.It is an extension to BSD sockets,the standard socket abstraction API that providesobjects to simplify tasks such as communicating with FTP and HTTP servers or resolving DNS hosts.CFNetworkis based,both physically and theoretically,on BSD sockets.Just as CFNetwork relies on BSD sockets,there are a number of Cocoa classes that rely on CFNetwork(NSURL,for example).In addition,the Web Kit is a set of Cocoa classes to display web content in windows.Both ofthese classes are very high level and implement most of the details of the networking protocols by themselves.Thus,the structure of the software layers looks like the image in Figure1-1.Figure1-1CFNetwork and other software layers on Mac OSXWhen to Use CFNetworkCFNetwork has a number of advantages over BSD sockets.It provides run-loop integration,so if yourapplication is run loop based you can use network protocols without implementing threads.CFNetwork alsocontains a number of objects to help you use network protocols without having to implement the detailsyourself.For example,you can use FTP protocols without having to implement all of the details with theCFFTP API.If you understand the networking protocols and need the low-level control they provide but don'twant to implement them yourself,then CFNetwork is probably the right choice.There are a number of advantages of using CFNetwork instead of Foundation-level networking APIs.CFNetworkis focused more on the network protocols,whereas the Foundation-level APIs are focused more on dataaccess,such as transferring data over HTTP or FTP.Although Foundation APIs do provide some configurability,CFNetwork provides a lot more.For more information on Foundation networking classes,read URL LoadingSystem Programming Guide.Now that you understand how CFNetwork interacts with the other Mac OS X networking APIs,you're readyto become familiar with the CFNetwork APIs along with two APIs that form the infrastructure for CFNetwork.When to Use CFNetwork9CFNetwork ConceptsCFNetwork InfrastructureBefore learning about the CFNetwork APIs,you must first understand the APIs which are the foundation forthe majority of CFNetwork.CFNetwork relies on two APIs that are part of the Core Foundation framework,CFSocket and CFStream.Understanding these APIs is essential to using CFNetwork.CFSocket APISockets are the most basic level of network communications.A socket acts in a similar manner to a telephonejack.It allows you to connect to another socket(either locally or over a network)and send data to that socket.The most common socket abstraction is BSD sockets.CFSocket is an abstraction for BSD sockets.With verylittle overhead,CFSocket provides almost all the functionality of BSD sockets,and it integrates the socketinto a run loop.CFSocket is not limited to stream-based sockets(for example,TCP),it can handle any typeof socket.You could create a CFSocket object from scratch using the CFSocketCreate function,or from a BSD socketusing the CFSocketCreateWithNative function.Then,you could create a run-loop source using thefunction CFSocketCreateRunLoopSource and add it to a run loop with the function CFRunLoopAddSource.This would allow your CFSocket callback function to be run whenever the CFSocket object receives a message.Read CFSocket Reference for more information about the CFSocket API.CFStream APIRead and write streams provide an easy way to exchange data to and from a variety of media in adevice-independent way.You can create streams for data located in memory,in a file,or on a network(usingsockets),and you can use streams without loading all of the data into memory at once.A stream is a sequence of bytes transmitted serially over a communications path.Streams are one-way paths,so to communicate bidirectionally an input(read)stream and output(write)stream are necessary.Exceptfor file-based streams,you cannot seek within a stream;once stream data has been provided or consumed,it cannot be retrieved again from the stream.CFStream is an API that provides an abstraction for these streams with two new CFType objects:CFReadStreamand CFWriteStream.Both types of stream follow all of the usual Core Foundation API conventions.For moreinformation about Core Foundation types,read Core Foundation Design Concepts.CFStream is built on top of CFSocket and is the foundation for CFHTTP and CFFTP.As you can see in Figure1-2,even though CFStream is not officially part of CFNetwork,it is the basis for almost all of CFNetwork. 10CFNetwork InfrastructureFigure1-2CFStream API structureFrameworkCFNetworkCore FoundationYou can use read and write streams in much the same way as you do UNIX file descriptors.First,you instantiatethe stream by specifying the stream type(memory,file,or socket)and set any options.Next,you open thestream and read or write any number of times.While the stream exists,you can get information about thestream by asking for its properties.A stream property is any information about the stream,such as its sourceor destination,that is not part of the actual data being read or written.When you no longer need the stream,close and dispose of it.CFStream functions that read or write a stream will suspend,or block,the current process until at least onebyte of the data can be read or written.To avoid trying to read from or write to a stream when the streamwould block,use the asynchronous version of the functions and schedule the stream on a run loop.Yourcallback function is called when it is possible to read and write without blocking.In addition,CFStream has built-in support for the Secure Sockets Layer(SSL)protocol.You can set up adictionary containing the stream's SSL information,such as the security level desired or self-signed certificates.Then pass it to your stream as the kCFStreamPropertySSLSettings property to make the stream an SSLstream.The chapter"Working with Streams" (page 17)describes how to use read and write streams. CFNetwork API ConceptsTo understand the CFNetwork framework,you need to be familiar with the building blocks that compose it.The CFNetwork framework is broken up into separate APIs,each covering a specific network protocol.TheseAPIs can be used in combination,or separately,depending on your application.Most of the programmingconventions are common among the APIs,so it's important to comprehend each of them.CFFTP APICommunicating with an FTP server is made easier with ing the CFFTP API,you can create FTP readstreams(for downloading)and FTP write streams(for uploading).Using FTP read and write streams you canperform functions such as:●Download a file from an FTP server●Upload a file to an FTP serverCFNetwork API Concepts112011-06-06 | © 2011 Apple Inc. All Rights Reserved.●Download a directory listing from an FTP server●Create directories on an FTP serverAn FTP stream works like all other CFNetwork streams.For example,you can create an FTP read stream bycalling the function CFReadStreamCreateWithFTPURL function.Then,you can call the functionCFReadStreamGetError at any time to check the status of the stream.By setting properties on FTP streams,you can adapt your stream for its particular application.For example,if the server that the stream is connecting to requires a user name and password,you need to set theappropriate properties so the stream can work properly.For more information about the different propertiesavailable to FTP streams read"Setting up the Streams" (page 43).A CFFTP stream can be used synchronously or asynchronously.To open the connection with the FTP serverthat was specified when the FTP read stream was created,call the function CFReadStreamOpen.To readfrom the stream,use the CFReadStreamRead function and provide the read stream reference,CFReadStreamRef,that was returned when the FTP read stream was created.The CFReadStreamReadfunction fills a buffer with the output from the FTP server.For more information on using CFFTP,see"Working with FTP Servers" (page 43).CFHTTP APITo send and receive HTTP messages,use the CFHTTP API.Just as CFFTP is an abstraction for FTP protocols,CFHTTP is an abstraction for HTTP protocols.Hypertext Transfer Protocol(HTTP)is a request/response protocol between a client and a server.The clientcreates a request message.This message is then serialized,a process that converts the message into a rawbyte stream.Messages cannot be transmitted until they are serialized first.Then the request message is sentto the server.The request typically asks for a file,such as a webpage.The server responds,sending back astring followed by a message.This process is repeated as many times as is necessary.To create an HTTP request message,you specify the following:●The request method,which can be one of the request methods defined by the Hypertext TransferProtocol,such as OPTIONS,GET,HEAD,POST,PUT,DELETE,TRACE,and CONNECT●The URL,such as ●The HTTP version,such as version1.0or1.1●The message’s headers,by specifying the header name,such as User-Agent,and its value,such asMyUserAgent●The message’s bodyAfter the message has been constructed,you serialize it.Following serialization,a request might look likethis:GET / HTTP/1.0\r\nUser-Agent: UserAgent\r\nContent-Length: 0\r\n\r\n12CFNetwork API Concepts2011-06-06 | © 2011 Apple Inc. All Rights Reserved.Deserialization is the opposite of serialization.With deserialization,a raw byte stream received from a client or server is restored to its native representation.CFNetwork provides all of the functions needed to get the message type(request or response),HTTP version,URL,headers,and body from an incoming,serialized message.More examples of using CFHTTP are available in"Communicating with HTTP Servers" (page 27). CFHTTPAuthentication APIIf you send an HTTP request to an authentication server without credentials(or with incorrect credentials), the server returns an authorization challenge(more commonly known as a401or407response).The CFHTTPAuthentication API applies authentication credentials to challenged HTTP messages. CFHTTPAuthentication supports the following authentication schemes:●Basic●Digest●NT LAN Manager(NTLM)●Simple and Protected GSS-API Negotiation Mechanism(SPNEGO)New in Mac OS X v10.4is the ability to carry persistency across requests.In Mac OS X v10.3each time a request was challenged,you had to start the authentication dialog from scratch.Now,you maintain a set of CFHTTPAuthentication objects for each server.When you receive a401or407response,you find the correct object and credentials for that server and apply them.CFNetwork uses the information stored in that object to process the request as efficiently as possible.By carrying persistency across request,this new version of CFHTTPAuthentication provides much better performance.More information about how to use CFHTTPAuthentication is available in"Communicating with Authenticating HTTP Servers" (page 33).CFHost APIYou use the CFHost API to acquire host information,including names,addresses,and reachability information. The process of acquiring information about a host is known as resolution.CFHost is used just like CFStream:●Create a CFHost object.●Start resolving the CFHost object.●Retrieve either the addresses,host names,or reachability information.●Destroy the CFHost object when you are done with it.Like all of CFNetwork,CFHost is IPv4and ing CFHost,you could write code that handles IPv4and IPv6completely transparently.CFNetwork API Concepts13 2011-06-06 | © 2011 Apple Inc. All Rights Reserved.CFHost is integrated closely with the rest of CFNetwork.For example,there is a CFStream function calledCFStreamCreatePairWithSocketToCFHost that will create a CFStream object directly from a CFHostobject.For more information about the CFHost object functions,see CFHost Reference.CFNetServices APIIf you want your application to use Bonjour to register a service or to discover services,use the CFNetServicesAPI.Bonjour is Apple's implementation of zero-configuration networking(ZEROCONF),which allows you topublish,discover,and resolve network services.To implement Bonjour the CFNetServices API defines three object types:CFNetService,CFNetServiceBrowser,and CFNetServiceMonitor.A CFNetService object represents a single network service,such as a printer or afile server.It contains all the information needed for another computer to resolve that server,such as name,type,domain and port number.A CFNetServiceBrowser is an object used to discover domains and networkservices within domains.And a CFNetServiceMonitor object is used to monitor a CFNetService object forchanges,such as a status message in iChat.For a full description of Bonjour,see Bonjour Overview.For more information about using CFNetServices andimplementing Bonjour,see NSNetServices and CFNetServices Programming Guide.CFNetDiagnostics APIApplications that connect to networks depend on a stable connection.If the network goes down,this causesproblems with the application.By adopting the CFNetDiagnostics API,the user can self-diagnose networkissues such as:●Physical connection failures(for example,a cable is unplugged)●Network failures(for example,DNS or DHCP server no longer responds)●Configuration failures(for example,the proxy configuration is incorrect)Once the network failure has been diagnosed,CFNetDiagnostics guides the user to fix the problem.You mayhave seen CFNetDiagnostics in action if Safari failed to connect to a website.The CFNetDiagnostics assistantcan be seen in Figure1-3.14CFNetwork API Concepts2011-06-06 | © 2011 Apple Inc. All Rights Reserved.Figure1-3Network diagnostics assistantBy providing CFNetDiagnostics with the context of the network failure,you can call the CFNetDiagnosticDiagnoseProblemInteractively function to lead the user through the prompts to find a solution.Additionally,you can use CFNetDiagnostics to query for connectivity status and provide uniform error messages to the user.To see how to integrate CFNetDiagnotics into your application read"Using Network Diagnostics" (page 51). CFNetDiagnostics is a new API in Mac OS X v10.4.CFNetwork API Concepts15 2011-06-06 | © 2011 Apple Inc. All Rights Reserved.16CFNetwork API Concepts2011-06-06 | © 2011 Apple Inc. All Rights Reserved.Working with StreamsThis chapter discusses how to create,open,and check for errors on read and write streams.It also describeshow to read from a read stream,how to write to a write stream,how to prevent blocking when reading fromor writing to a stream,and how to navigate a stream through a proxy server.Working with Read StreamsCore Foundation streams can be used for reading or writing files or working with network sockets.With theexception of the process of creating those streams,they behave similarly.Creating a Read StreamStart by creating a read stream.Listing2-1creates a read stream for a file.Listing2-1Creating a read stream from a fileCFReadStreamRef myReadStream = CFReadStreamCreateWithFile(kCFAllocatorDefault,fileURL);In this listing,the kCFAllocatorDefault parameter specifies that the current default system allocator beused to allocate memory for the stream and the fileURL parameter specifies the name of the file for whichthis read stream is being created,such as file:///Users/joeuser/Downloads/MyApp.sit.Similarly,you can create a pair of streams based on a network service by callingCFStreamCreatePairWithSocketToCFHost(described in“Using a Run Loop to Prevent Blocking” (page20))or CFStreamCreatePairWithSocketToNetService(described in NSNetServices and CFNetServicesProgramming Guide).Now that you have created the stream,you can open it.Opening a stream causes the stream to reserve anysystem resources that it requires,such as the file descriptor needed to open the file.Listing2-2is an exampleof opening the read stream.Listing2-2Opening a read streamif (!CFReadStreamOpen(myReadStream)) {CFStreamError myErr = CFReadStreamGetError(myReadStream);// An error has occurred.if (myErr.domain == kCFStreamErrorDomainPOSIX) {// Interpret myErr.error as a UNIX errno.} else if (myErr.domain == kCFStreamErrorDomainMacOSStatus) {// Interpret myErr.error as a MacOS error code.OSStatus macError = (OSStatus)myErr.error;// Check other error domains.}Working with Read Streams172011-06-06 | © 2011 Apple Inc. All Rights Reserved.}The CFReadStreamOpen function returns TRUE to indicate success and FALSE if the open fails for any reason.If CFReadStreamOpen returns FALSE,the example calls the CFReadStreamGetError function,whichreturns a structure of type CFStreamError consisting of two values:a domain code and an error code.Thedomain code indicates how the error code should be interpreted.For example,if the domain code iskCFStreamErrorDomainPOSIX,the error code is a UNIX errno value.The other error domains arekCFStreamErrorDomainMacOSStatus,which indicates that the error code is an OSStatus value definedin MacErrors.h,and kCFStreamErrorDomainHTTP,which indicates that the error code is the one of thevalues defined by the CFStreamErrorHTTP enumeration.Opening a stream can be a lengthy process,so the CFReadStreamOpen and CFWriteStreamOpen functionsavoid blocking by returning TRUE to indicate that the process of opening the stream has begun.To checkthe status of the open,call the functions CFReadStreamGetStatus and CFWriteStreamGetStatus,whichreturn kCFStreamStatusOpening if the open is still in progress,kCFStreamStatusOpen if the open iscomplete,or kCFStreamStatusErrorOccurred if the open has completed but failed.In most cases,itdoesn’t matter whether the open is complete because the CFStream functions that read and write will blockuntil the stream is open.Reading from a Read StreamTo read from a read stream,call the function CFReadStreamRead,which is similar to the UNIX read()system call.Both take buffer and buffer length parameters.Both return the number of bytes read,0if at theend of stream or file,or-1if an error occurred.Both block until at least one byte can be read,and bothcontinue reading as long as they can do so without blocking.Listing2-3is an example of reading from theread stream.Listing2-3Reading from a read stream(blocking)CFIndex numBytesRead;do {UInt8 buf[myReadBufferSize]; // define myReadBufferSize as desirednumBytesRead = CFReadStreamRead(myReadStream, buf, sizeof(buf));if( numBytesRead > 0 ) {handleBytes(buf, numBytesRead);} else if( numBytesRead < 0 ) {CFStreamError error = CFReadStreamGetError(myReadStream);reportError(error);}} while( numBytesRead > 0 );Tearing Down a Read StreamWhen all data has been read,you should call the CFReadStreamClose function to close the stream,therebyreleasing system resources associated with it.Then release the stream reference by calling the functionCFRelease.You may also want to invalidate the reference by setting it to NULL.See Listing2-4for anexample.Listing2-4Releasing a read streamCFReadStreamClose(myReadStream);CFRelease(myReadStream);18Working with Read Streams2011-06-06 | © 2011 Apple Inc. All Rights Reserved.。
达士 DS-2CD2746G1-IZ(S) 4K IR 变焦球形网络摄像头说明书

Key Features●Max. 2688 × 1520 @ 30fps●H.265+, H.264+, H.265, H.264●120dB WDR●Powered by DarkFighter●Color: 0.007 Lux @ (F1.2, AGC ON), 0.01 Lux@ (F1.4, AGC ON), 0 Lux with IR●False alarm reduction by human and vehicletarget classification based on deep learning ●12 VDC & PoE (802.3af, class 3)●IR range: Up to 30 m●Support on-board storage, up to 128 GB ●IP67, IK10●BLC/3D DNR/ROISpecificationCameraImage Sensor1/2.7" Progressive Scan CMOSMin. Illumination Color: 0.007 Lux @ (F1.2, AGC ON), 0.01 Lux @ (F1.4, AGC ON), 0 Lux with IR Shutter Speed1/3 s to 1/100,000 sSlow Shutter YesDay &Night IR cut filterDigital Noise Reduction 3D DNRWide Dynamic Range120dB3-Axis Adjustment (with bracket)Pan: 0° to 355°, tilt: 0° to 75°, rotate: 0° to 355°LensFocal length 2.8 mm to 12 mmAperture F1.4Focus AutoFOV Horizontal FOV: 101° to 29°, vertical FOV: 54° to 16°, diagonal FOV: 121° to 34°Lens MountΦ14IRIR Range Up to 30 mWavelength850 nmCompression StandardVideo Compression Main stream: H.265/H.264Sub stream: H.265/H.264/MJPEGH.264 Type Main Profile/High Profile H.264+Main stream supports H.265 Type Main ProfileH.265+Main stream supports Video Bit Rate32 Kbps to 16 Mbps Smart Feature-setBehavior Analysis Line crossing detection, intrusion detection, region entrance detection, region exit detection, false alarm reduction by human and vehicle target classification based on deep learning When H265+, three streams or motion detection is enabled, the behavior analyses (including line crossing detection, intrusion detection, region entrance detection and region exiting detection) are not supported.ROI (Region of Interest)Support 1 fixed region for main stream and sub stream separately ImageMax. Resolution2688 × 1520Main Stream 50Hz: 25fps (2688 × 1520, 1920 × 1080, 1280 × 720) 60Hz: 30fps (2688 × 1520, 1920 × 1080, 1280 × 720)Sub-Stream 50Hz: 25fps (640 × 480, 640 × 360, 320 × 240) 60Hz: 30fps (640 × 480, 640 × 360, 320 × 240)Image Enhancement BLC/3D DNRImage Settings Saturation, brightness, contrast, sharpness, AGC, white balance adjustable by client software or web browserDay/Night Switch Day/Night/Auto/Schedule/Triggered by Alarm In(-S) Audio (-S)Environment Noise Filtering YesSampling Rate8kHz/16kHzAudio Compression G.711/G.722.1/G.726/MP2L2/PCMAudio Bit Rate64Kbps(G.711)/16Kbps(G.722.1)/16Kbps(G.726)/32-160Kbps(MP2L2)NetworkNetwork Storage Support micro SD/SDHC/SDXC card (128G) local storage, NAS (NFS,SMB/CIFS), ANRAlarm Trigger Tampering alarm, network disconnected, IP address conflict, illegal login, HDD full, HDD errorProtocols TCP/IP, ICMP, HTTP, HTTPS, FTP, DHCP, DNS, DDNS, RTP, RTSP, RTCP, PPPoE, NTP, UPnP, SMTP,SNMP, IGMP, 802.1X, QoS, IPv6, UDP, BonjourGeneral Function One-key reset, anti-flicker, heartbeat, mirror, password protection, privacy mask, watermark, IP address filteringFirmware Version V5.5.72API ONVIF (PROFILE S, PROFILE G, PROFILE T), ISAPI Simultaneous Live View Up to 6 channelsUser/Host Up to 32 users3 levels: Administrator, Operator and UserClient iVMS-4200, Hik-Connect, iVMS-5200, iVMS-4500Web Browser Plug-in required live view:IE8+, Chrome 31.0-44, Firefox 30.0-51, Safari 8.0+ Plug-in free live view:Chrome 45.0+, Firefox 52.0+InterfaceCommunication Interface 1 RJ45 10M/100M self-adaptive Ethernet portOn-board Storage Built-in micro SD/SDHC/SDXC slot, up to 128 GBSVC H.265 encoding and H.264 encoding supportReset Button YesAudio (-S) 1 audio input (line in), 1 audio output (line out), mono soundAlarm (-S) 1 alarm input, 1 alarm outputGeneralOperating Conditions-30 °C to +60 °C (-22 °F to +140 °F), humidity 95% or less (non-condensing)Power Supply 12 VDC ± 25%, 5.5 mm coaxial power plug PoE (802.3af, class 3)Power Consumption and Current 12 VDC, 0.9 A, max. 11 WPoE: (802.3af, 36 VDC to 57 VDC), 0.4 A to 0.2 A, max. 12.9 WProtection Level IK10, IP67 (If the camera is installed outdoor, the bracket DS-1253ZJ-L should be used together with other pendant mounting bracket or wall mounting bracket to reduce IR reflective in the rainy day)Material Front cover: metal, bottom base: metal, bubble: plastic Dimensions Camera: Φ 153.3 × 111.6 mm (Φ 6" × 4.4")Weight Camera: 890 g (2 lb.)With package: 1300 g (2.9 lb.)Available ModelDS-2CD2746G1-IZ (2.8 mm to 12 mm), DS-2CD2746G1-IZS (2.8 mm to 12 mm)Camera:153.3(6")111.6(4.4")100.4(4")Φ4.6(0.2")83.5(3.3")120˚46(1.8")Wall Mounting with the Bracket (DS -1473ZJ -155 and DS -1253ZJ -L):230.6(9.1")161.5(6.4")85.7(3.4")158(6.2")66.3(2.6")164.3(6.5")DimensionPendant Mounting with the Bracket (DS -1471ZJ -155 and DS -1253ZJ -L):431.8(17")164.3(6.5")66.3(2.6")Unit: mm(inch)DS -1471ZJ-155Pendant MountDS -1473ZJ -155 Wall MountDS -1475ZJ -SUS Vertical Pole MountDS -1476ZJ -SUS Corner MountDS -1280ZJ -DM55 Junction BoxDS -1253ZJ -L Rain ShadeAccessory05057220200320。
Microsoft Teams 基本版、标准版和高级版说明书

Microsoft Teams Essentials 1Standard Premium1Reflects Microsoft Teams Essentials (AAD Identity) licenses purchased through a Microsoft Partner. For important differences w ith Microsoft Teams Essentials licenses purchased directly from the Microsoft website or customer admin portal, please refer to https:///Micros oftTeams/get-started-with-teams-essentials .Desktop client apps 1●●Microsoft 365 for mobile 2●●●●Install apps on up to 5 PCs/Mac + 5 tablets + 5 smartphones●3●3●●Microsoft 365 for the web●●●●Visio for the web●●●Microsoft Editor premium features●●Multilingual user interface for Microsoft 365 apps●●1 Includes Word, Excel, PowerPoint, OneNote, Outlook, Access (PC only), and Publisher (PC only).2Includes Word, Excel, PowerPoint, Outlook, and OneNote mobile Apps.3 Mobile apps only.Email, calendar, and schedulingExchange Kiosk 2 GB mailbox ●Exchange Plan 1 50 GB mailbox●●●Calendar●●●●Outlook desktop client●●Auto-expanding email archive ●Exchange Online Protection●●●Public folder mailboxes ●●●Resource mailboxes●●●Microsoft Shifts●●●Microsoft Bookings●●●1The non-ADD Identity version of Microsoft Teams Essentials available for online purchase directly form Microsoft includes the Exchange Kiosk service plan to enable Teams calendar only. It does not include a mailbox or voicemail.Microsoft Teams ●●●●Unlimited chat ●●●●Online meetings●●●●Webinars●●Screen sharing and custom backgrounds●●●●Record meetings●●●●Avatars for Teams ●●●●Priority notifications●●●●Audio Conferencing 1●●●●1Check country and region availability. Available via the no-cost Audio Conferencing with Dial-out to US and Canada supplemental license.Microsoft 365 appsEmail, calendar, and scheduling Meetings, calling, and chatIntranet and storage Knowledge and content Project and task managementAnalyticsMicrosoft VivaAutomation, app building, and chatbotsThreat protectionEndpoint and app management Identity and access managementCloud access security broker Information protectioneDiscovery and auditing Add-on licensesMicrosoft Teams Essentials 11Reflects Microsoft Teams Essentials (AAD Identity) licenses purchased through a Microsoft Partner. For important differences w ith Microsoft Teams Essentials licenses purchased directly from the Microsoft website or customer admin portal, please refer to https:///Micros oftTeams/get-started-with-teams-essentials.Productivity Score●●●Secure Score●●●Compliance Management●●●SharePoint Plan 1●●●10 GB additional storage per license 1●●●OneDrive personal storage● (10 GB)● (1 TB)● (1 TB)● (1 TB)1In addition to 1TB storage provided per organization.Microsoft Planner ●●●Microsoft To-Do●●●Microsoft Graph API ●●●Microsoft Search ●●●●Microsoft Lists●●●Microsoft Forms 1●●●Microsoft Stream (on SharePoint)●●●Delve●●●1Licensed users can create/share/manage forms. Completing/responding does not require a Forms license.Viva Insights app in Teams ●●●Personal insights and experiences●●●1Requires Exchange Online. Analyst workbench tools and accelerators, Manager and leader insights and experiences, and Premium personal insights and experiences are available with the Viva Insights and Viva Suite add-ons.Communities, Conversations, and Storylines●●●1Leadership Corner, AMAs, Storyline Delegate Posting, Advanced Analytics, and Answers in Viva available with the Viva Suite ad d-on. Answers in Viva also available with the Viva Topics add-on.Dashboard, Feed, Resources, and Teams app ●●●Microsoft Teams Essentials 11Reflects Microsoft Teams Essentials (AAD Identity) licenses purchased through a Microsoft Partner. For important differences w ith Microsoft Teams Essentials licenses purchased directly from the Microsoft website or customer admin portal, please refer to https:///Micros oftTeams/get-started-with-teams-essentials.1Power Apps for Microsoft 365●●●Power Automate for Microsoft 365●●●Power Virtual Agent for Teams●●●Dataverse for Teams●●●1Refer to the licensing FAQs and Licensing Guide at for details including functionality limits.Microsoft Intune Plan 1●Mobile Device Management ●●Mobile application management●Windows Autopilot ●Group Policy support●1●1Office cloud policy support●1●1Shared computer activation for Microsoft 365 Apps●Endpoint Analytics ●Cortana management●1Limited to policies for web apps and privacy policies for client apps.Microsoft Defender for Business ●Microsoft Defender Exploit Guard ●Microsoft Defender Credential Guard●BitLocker and BitLocker To Go ●Windows Information Protection●Microsoft Defender for Office 365 Plan 1●1Viva Learning in Teams ●●●Create learning tabs in Teams channels●●●Search, share, and chat about learning content●●●Microsoft Learn and Microsoft 365 Training libraries + 125 top LinkedInLearning courses●●●Organization-generated content with SharePoint and Viva Learning●●●1Course recommendations and progress tracking, integration with 3rd party content providers and Learning Management Systems, and learning content surfaced across Microsoft 365 suite available with the Viva Learning or Viva suite add-on license.Microsoft Teams Essentials 11Reflects MicrosoftTeams Essentials (AAD Identity) licenses purchased through a Microsoft Partner. For important differences w ith Microsoft Teams Essentials licenses purchased directly from theMicrosoft website or customer admin portal, please refer to https:///Micros oftTeams/get-started-with-teams-essentials.Content Search ●●●●Litigation Hold ●Audit (Standard)●●●●Cloud access security brokerMicrosoft Defender for Cloud Apps Discovery●Manual retention labels ●Basic org-wide or location-wide retention policies●Teams message retention policies 1●●●●130-day minimum retention period (no maximum period).Azure Information ProtectionPlan 1Manual, default, and mandatory sensitivity labeling in Office 365●Manual labeling with the AIP app and plugin●Data Loss Prevention (DLP) for emails and files●Basic Message Encryption ●Windows 11 Edition BusinessAzure Virtual Desktop●Universal Print (5 jobs/user/month pooled)●Windows Update for Business deployment service●Microsoft Entra ID 1 Plan 1●User Provisioning●Cloud user self-service password change ●●●Cloud user self-service password reset●●Hybrid user self-service password change/reset with on-premises write-back●Conditional Access●On-premises Active Directory sync for SSO●Windows Hello for Business●1Formerly Azure Active Directory Premium .Microsoft Teams Essentials1Basic Standard1Reflects Microsoft Teams Essentials (AAD Identity) licenses purchased through a Microsoft Partner. For important differences w ith Microsoft Teams Essentials licenses purchased directly from theMicrosoft website or customer admin portal, please refer to https:///Micros oftTeams/get-started-with-teams-essentials.Security and ComplianceMicrosoft 365 E5 Info Protection and Governance N/A+1+1+ Microsoft 365 E5 Insider Risk Management N/A+++ Microsoft 365 E5 eDiscovery and Audit N/A+++Microsoft Defender for Business N/A++●Microsoft Defender for Business servers add-on for Microsoft Defender for Business N/A+5+5+6Microsoft Defender for Identity N/A+++ Microsoft Defender for Office 365 Plan 1N/A++●Microsoft Defender for Office 365 Plan 2N/A+++ Microsoft Defender for Cloud Apps N/A+++ App governance add-on for Microsoft Defender for Cloud Apps N/A+2+2+2 Microsoft Defender for Endpoint Plan 1N/A+++Microsoft Defender for Endpoint Plan 2N/A+++ Microsoft Defender for Endpoint for servers3N/A+++ Premium Assessments add-on for Compliance Manager4N/A+++Microsoft Entra7 ID Plan 1N/A++●Microsoft Entra7 ID Plan 2N/A+++Microsoft Intune Plan 1N/A++●Microsoft Intune Plan 2N/A+5+5+Microsoft Intune Suite N/A+5+5+Microsoft Intune Remote Help N/A+5+5+ Microsoft Purview Data Loss Prevention (for email and files)N/A++●Exchange Archiving N/A++●1 Requires EMS E3 or Azure Information Protection Plan 1 standalone required.2 Requires Microsoft Defender for Cloud Apps.3 Separate license required for each OSE (e.g., servers or virtual machines). See OSE definition in the Product Terms glossary.4Get details on available assessments.5 Requires Microsoft Intune Plan 16 Requires Microsoft Defender for Business or Microsoft 365 Business Premium. Maximum quantity/seat cap is 60 licenses per cust omer.7 Formerly Azure Active Directory Premium.Microsoft Teams EssentialsBasic Standard1Reflects Microsoft Teams Essentials (AAD Identity) licenses purchased through a Microsoft Partner. For important differences w ith Microsoft Teams Essentials licenses purchased directly from theMicrosoft website or customer admin portal, please refer to https:///Micros oftTeams/get-started-with-teams-essentials.Teams Services1Teams Premium++++ Audio Conferencing with Dial-out to US and Canada2++++ Extended Dial-out Minutes to US and Canada++2+2+2Teams Phone Standard3++++Domestic Calling Plan4++++ Domestic Calling 120 Minute Plan4++++International Calling Plan4,5++++ Teams Phone with Domestic Calling Plan++++ Teams Phone with International Calling Plan5++++Teams Phone with Pay-as-you-go Calling Plan ++++1 Check country and region availability.2 Available to add at no cost. Includes unlimited toll dial-out, 60 minutes/user/month dial-out to phone numbers in the US and Canada, and Operator Connect Conferencing.3 Audio Conferencing, Audio Conferencing with Dial-out to US and Canada, or Business Voice required.4 Teams Phone Standard required.5 Includes full Domestic Calling Plan.Power PlatformPower BI Pro N/A+++Power BI Premium N/A+++ Power Apps per-app (1 app/user/month)N/A+++ Power Apps per-user (unlimited apps/user/month)N/A+++Power Automate per-user (unlimited flows + 5K AI Builder+++credits/user/month)N/APower Automate per-user w/ Unattended RPA (unlimited flows + 5K AI+++Builder credits/user/month) N/APower Automate per-flow (5 flows/month for unlimited users)1N/A+++ Power Apps per app pay-as-you-go N/A+++ Dataverse-Database Capacity-Data Stored-1 GB N/A+++ Dataverse-File Capacity-Data Stored-10 GB N/A+++Dataverse-Log Capacity-Data Stored-1 GB N/A+++1Initial minimum purchase requirement of 7 licenses.Microsoft Teams EssentialsBasic Standard1Reflects Microsoft Teams Essentials (AAD Identity) licenses purchased through a Microsoft Partner. For important differences w ith Microsoft Teams Essentials licenses purchased directly from the Microsoft website or customer admin portal, please refer to https:///Micros oftTeams/get-started-with-teams-essentials .Universal Print Volume Add-on 1N/A N/A N/A +High Efficiency Video Codec (HEVC)1N/AN/AN/A+1Universal Print and HEVC require Windows. Plans that do not include Windows licenses are marked as “N/A”.SharePoint Advanced ManagementN/A+++Extra Graph Connector Capacity N/A N/AN/AN/ACross-tenant user data migrationN/A +++Office 365 Extra File StorageN/A+++eDiscovery Storage N/A +++Advanced Data ResidencyN/A+++Microsoft VivaViva SuiteN/A +++Viva Workplace Analytics and Employee Feedback N/A +++Viva Employee Communications and CommunitiesN/A +++Viva Topics N/A +++Viva Insights N/A +++Viva Glint N/A +++Viva LearningN/A+++Viva GoalsN/A+++。
ADVANTEST U3741 U3751 U3771 U3772 说明书

MANUAL NUMBER C Printed in JapanADVANTEST CORPORATIONAll rights reserved .U3700 SeriesUser’s GuideFOE-8440185H00First printing November 20, 2004Applicable ModelsU3741U3751U3771U37722004Certificate of ConformityThis is to certify, thatcomplies with the provisions of the EMC Directive 89/336/EEC (All of these factors arerevised by 91/263/EEC,92/31/EEC,93/68/EEC) in accordance with EN61326and Low V oltage Directive 73/23/EEC (All of these factors are revised by 93/68/EEC)in accordance with EN61010.ADVANTEST Corp.ROHDE&SCHWARZ Tokyo, Japan Europe GmbHMunich, Germanyinstrument, type, designation3700.05Spectrum AnalyzerU3700 SeriesNo. CR B00CR-1有毒有害物质含量信息说明书•本有毒有害含量含量说明内容是为了贯彻[电子信息产品污染控制管理办法]而编制的。
This document is made for Chinese Administration on the Control of Pollution Caused by Electronic In-formation Products, unofficially called "China-RoHS".この文書は、中国の「電子情報製品汚染防止管理弁法」のための文書です。
Eaton Oxalis XP40 PTZ摄像头站点数据表说明书

EatonUnit B, Sutton Parkway Oddicroft Lane Sutton in Ashfield United Kingdom NG17 5FBT: +44 (0) 1623 444 /hac *******************XP40 HD IP series - UL rangePTZ camera station,hazardous locationOverviewThe Oxalis XP40 is an explosion protected PTZ camera station for use in hazardous areas in onshore, offshore, marine and heavy industrial environments.The camera housings are designed specifically for the Americas markets or where UL standards on Class and Division have been specified.The base unit carries dual NPT cable entries with easy access for cable termination during installation as standard, maximisingcompatibility and ease of use with existing fixed conduit installations.Our camera stations are designed and manufactured for longevity in harshenvironments, require minimal maintenance and are fully certified to UL standards as required by OSHA in both safe and hazardous areas.See separate datasheet for ATEX/IECEx & other zone certification ranges.Features• Class 1 Division 1 and Zone 1 certified•Electro-polished 316L stainless steel on all welded assemblies• Camera station window in toughened glass • Wall mounting options (see separate datasheet)• NPT entries as standard • Various camera module options• Supply voltage options (24 VAC, 110 or 230 VAC, 50/60Hz)•Certified temperature from -76˚F to +158˚F* (ranging from T4A - T6) •IP66/67*Model dependentAll specifications, dimensions, weights and tolerances are nominal (typical) and Eaton reserve the right to vary all data without prior notice.© 2022 EatonAll Rights Reserved Printed in UKPublication No.DSOU0090/A March 2022Eaton is a registered trademark.All other trademarks are propertyof their respective owners.Sun shield Standard stainless steel 316L mirror finishSupply voltage options 24 VAC, 110 or 230 VAC, 50/60HzIntegral wiper Optional (Silicone wiper blades that are resistant and do not perishafter long exposure to ozone, UV , ice, snow, heat or cold) Power consumption 80W maximum (143W with low temperature operation)Integral demister Standard Electrical connections Terminal block for power, data and video specific to camera configuration Integral washer pump Not applicableCable entry 2 x ¾” NPT entries located in baseWasher systems Compatible with Oxalis XW or XWP washer tanks (see datasheets)Mechanical Pan speed (maximum)45° per second Body material Electro-polished 316L stainless steel on all welded assemblies Tilt speed (maximum)24° per secondFixings material A4 stainless steel Pre-set positional accuracy 64 presets: positional accuracy±0.1°Camera station window Toughened glassTelemetry receiver Integral - Pelco D standard protocol (others to specification)Mounting options Wall (see separate datasheet)RotationContinuous pan or 350° rotation (+/- 175° from straight ahead)Operating temperature From -76°F to +158°F (model dependent)IP direct fibre out options Optional integrated media converter, simplex/duplex singlemode 9/125μm or multimode 50/125μm, 10/100Mb Ethernet, IEEE 802.3Weight (lb)Up to 73lb depending on configuration IP over coax Optional integrated IP Ethernet-over-coax converter (must be used with compatible Rx equipment)Ingress Protection RatingIP66/67Camera options32x XNZ-6320 HP IP camera 22x zoom 3MP HD IP camera Image sensor Progressive scan CMOS 1/2.8"Image sensor Progressive scan CMOS 1/2.8”Resolution Resolution: 1920x1080 @60fps to 320x240Resolution 2304 x 1296 @ 30fpsLens32x optical 32x digital zoom 4.44-142.6 mm F1.6 to F4.4, horizontal angle of view 61.8° - 2.19°Lens22x optical zoom 5.2~114.4mm F1.5~F3.8, horizontal angle of view 53.74°- 2.96°Min. illumination Colour : 0.05Lux (1/30sec, F1.6, 50IRE), B/W : 0.005Lux (1/30sec, F1.6, 50IRE)Min. illumination Colour : 0.002Lux (F1.5, AGC ON), B/W 0.001Lux (F1.5, AGC ON)Streaming H.264, H.265 MJPEG dual codec, multiple streaming, VBR/CBR Streaming Triple streams in H.264, H.265FeaturesIntelligent video analytics, motion detection, day & night (ICR), WDR (150dB), auto focus, auto Iris, AGC, SSDR, ATW, SSNRIII, BLC, DIS, DefogFeaturesAGC, AE,AWB,TDN,DNR,BLC,EIS,WDR,Defog,OSD,Day & Night Auto Colour/BW (IR-cut with auto switch)Standards protocols ONVIF Profile S, TCP/IP , UDP/IP , RTP(UDP), RTP(TCP), RTCP , RTSP , NTP , HTTP , HTTPS, SSL, DHCP , FTP , SMTP , ICMP , IGMP , SNMPv1/v2c/v3(MIB-2), ARP , DNS, DDNS, QoS, PIM-SM, UPnP , BonjourStandards protocolsL2TP , IPv4, IGMP , ICMP , ARP , TCP , UDP , DHCP , PPPoE, RTP , RTSP , DNS, DDNS, NTP , FTP , UPnP , HTTP , SNMP , SIP .33x zoom 3MP HD IP camera 22x zoom 5MP HD IP camera Image Sensor Progressive scan CMOS 1/2.8” Image Sensor Progressive scan CMOS 1/2.7”Resolution 2304 x 1296 @ 60fpsResolution 2880 x 1620 @ 30fpsLens33x optical zoom 4.5~148.5mm F1.5~F4.0, horizontal angle of view 62.93° - 3.67°Lens22x optical zoom 5.2~114.4mm F1.5~F3.8, horizontal angle of view 55.46°- 3.09°Min. Illumination Colour : 0.001Lux (F1.5, AGC ON), B/W 0.0005Lux (F1.5, AGC ON)Min. Illumination Colour : 0.003Lux (F1.5, AGC ON), B/W 0.001Lux (F1.5, AGC ON)Streaming Five streams in H.264, H.265Streaming Triple streams in H.264, H.265FeaturesAGC, AE,AWB,TDN,DNR,BLC,EIS,WDR,Defog,OSD,Day & Night Auto Colour/BW (IR-cut with auto switch)FeaturesAGC, AE,AWB,TDN,DNR,BLC,EIS,WDR,Defog,OSD,Day & Night Auto Colour/BW (IR-cut with auto switch)Standards ProtocolsL2TP , IPv4, IGMP , ICMP , ARP , TCP , UDP , DHCP , PPPoE, RTP , RTSP , DNS, DDNS, NTP , FTP , UPnP , HTTP , SNMP , SIP .Standards ProtocolsL2TP , IPv4, IGMP , ICMP , ARP , TCP , UDP , DHCP , PPPoE, RTP , RTSP , DNS, DDNS, NTP , FTP , UPnP , HTTP , SNMP , SIP .C1/D1+104°F)Class II, Division 1, Groups E, F , G IP67.Class 1 Zone 1 A Ex d IIB + Hydrogen T4 (T5 On Request)On Request: T5 -50°C to +70°C (-58°F to +158°F), T6 -50°C to +50°C (-58°F to +122°F)UL Listing: E477542Ordering requirementsThe following code is designed to help in selection of the correct unit. Build up the reference number by inserting the code for each component into the appropriate box XP40Supply voltage24 VAC ±10% 50/60 Hz 110 VAC ±10% 50/60 Hz 230 VAC ±10% 50/60 Hz Special - price on application Code 1 2 3 SVideo type HD IP systemCodeIThermal core moduleNo thermal coreCodeNThermal core lensNo thermal imaging lensCodeNWiper options Integral wiper with switched 24VAC for external washer pump No wiper CodeENCamera rotationContinuous rotationPan rotation restricted to +/- 175°Code12Video systemIPCodeIHousing type Visual camera housing CodeBVCertificationcULus Class I Div1 (Groups BCD)CodeLT emperature typeT4A -4˚F to +158˚FT4A -58˚F to +158˚FT4A -76˚F to +104˚FT6 -4˚F to +122˚F*T6 -58˚F to +122˚F*T6 -76˚F to +104˚FCodeEFJGHKT ransmission type CodeStandard electrical 0Simplex singlemode9/125μm ethernet3Simplex multimode50/125μm ethernet4IP over coax5Duplex singlemode9/125µm6Duplex Multimode50/125µm7Day/night module32x zoom 2MP HD IP camera 22x zoom 3MP HD IP camera 33x zoom 3MP HD IP camera 22x zoom 5MP HD IP camera CodePUVWProtocol requirementsPelco D protocol, baud rate 2400bpsSpecial - price on applicationCodeDS。
DELL EMC OpenManage 服务器更新实用程序版本 17.00.00 注、小心和警告说明
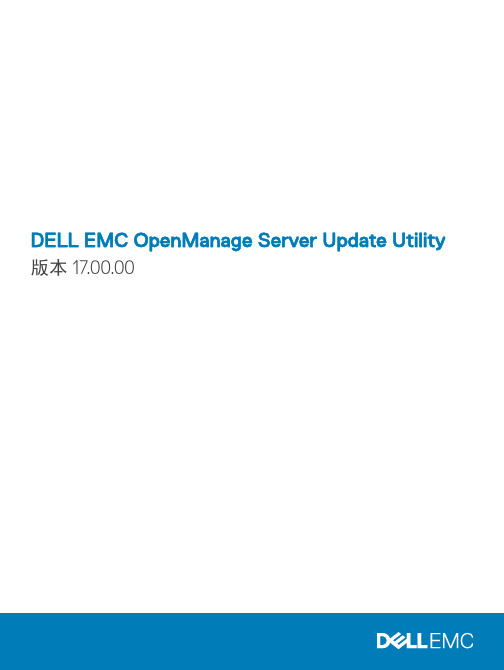
DELL EMC OpenManage Server Update Utility 版本 17.00.00注、小心和警告注: “注”表示帮助您更好地使用该产品的重要信息。
小心: “小心”表示可能会损坏硬件或导致数据丢失,并说明如何避免此类问题。
警告: “警告”表示可能会造成财产损失、人身伤害甚至死亡。
版权所有© 2017 Dell Inc. 或其附属公司。
保留所有权利。
Dell、EMC 和其他商标均为 Dell Inc. 或其附属公司的商标。
其他商标均为其各自所有者的商标。
2017 - 10Rev. A001 关于 OpenManage 服务器更新实用程序 (5)主要功能和功能特性 (5)此版本中的新功能 (6)支持的操作系统 (6)访问 Dell EMC 支持站点上的文档 (6)2 关于图形用户界面模式 (8)在 GUI 模式下启动 SUU (8)前提条件检查失败消息 (8)修复软件前提条件检查失败问题 (9)解决硬件前提条件检查失败问题 (9)创建前提条件介质 (9)比较报告 (10)严重程度级别 (10)比较报告功能 (10)升级系统组件 (11)系统组件降级 (11)更新系统组件 (12)更新失败 (12)3 关于命令行界面模式 (13)在 CLI 模式下启动 SUU (13)CLI 命令列表 (13)4 将服务器更新实用程序与其他组件集成 (15)将 SUU 与 OpenManage Essentials 集成 (15)使用 SUU 选择目录源 (15)使用 Repository Manager 创建自定义 SUU (15)以 SUU 的形式导出 (16)5 已知问题和常见问题 (18)已知问题 (18)SUU GUI 无法在 Red Hat Enterprise Linux 7.2 操作系统中打开 (18)Microsoft Windows Small Business Server 2008 操作系统上的资源清册故障 (18)DUP 在 64 位 Red Hat Enterprise Linux 操作系统上运行失败 (19)启用操作系统设备 (19)Linux 系统库 (19)运行 Linux 更新 (19)用进度选项运行 SUU (19)更新多个设备 (20)目录3更新 PERC 固件 (20)在同一会话中重新启动系统资源清册 (20)通过网络运行 SUU (20)删除临时文件夹 (20)更新 H661 固件 (20)使用 RAC 虚拟介质 (21)在 SUU 目录名称中使用特殊字符 (21)运行 RAC DUP 软件包 (21)常见问题 (21)4目录关于 OpenManage 服务器更新实用程序OpenManage 服务器更新实用程序是一个在 ISO 中可用的应用程序,用于识别和应用更新系统。
DS-2XE6845G0-I(L)Z(H)(S) 4 MP 爆破防护网络弹头摄像头说明书

DS-2XE6845G0-(I)(L)Z(H)(S)4 MP Explosion-Proof Network Bullet Camera⏹ Explosion-proof design: the camera has passedinternational ATEX & IECEx certifications, and can be usedin gas and dust hazardous scenarios⏹ 316L stainless steel enclosure, protecting the camera fromdamage caused by sparks or corrosive materials⏹ IP68 water resistant, preventing water ingress for outdooruse⏹H.265+/H.265/H.264+/H.264 encoding: efficientcompression technology saves storage space and reducesbandwidth requirements ⏹ AC 100 V to 240 V wide range power supply: easy todeploy cables and safer compared to a power adaptersolutionSpecificationCameraImage Sensor 1/2.8″ Progressive Scan CMOSMax. Resolution 2688 × 1520Min. Illumination Color: 0.009 Lux @ (F1.2, AGC ON), B/W: 0.0009 Lux @ (F1.2, AGC ON), 0 Lux with IR Shutter Time 1 s to 1/100,000 sDay & Night IR cut filterLensLens Type Varifocal lens, 2.8 to 12 mm and 8 to 32 mm lensFocal Length & FOV 2.8 to 12 mm, horizontal FOV 115° to 42°, vertical FOV 59° to 24°, diagonal FOV 141° to 48°8 to 32 mm, horizontal FOV 43° to 15°, vertical FOV 23° to 9°, diagonal FOV 50° to 17°Lens Mount IntegratedFocus Auto, semi-auto, manual Iris Type Auto-iris, DC driveAperture 2.8 to 12 mm: F1.48 to 32 mm: F1.7, constant F1.7 throughout the zoom rangeDORIDORI 2.8 to 12 mm: D: 150.3 m, O: 59.7 m, R: 30.1 m, I: 15 m 8 to 32 mm: D: 400 m, O: 158.7 m, R: 80 m, I: 40 mIlluminatorSupplement Light Type -I model: IR-L model: white lightSupplement Light Range -I model: up to 100 m IR range-L model: up to 30 m white light rangeIR Wavelength -I model: 850 nm VideoMain Stream 50 Hz: 25 fps (2688 × 1520, 2560 × 1440, 1920 × 1080, 1280 × 720) 60 Hz: 30 fps (2688 × 1520, 2560 × 1440, 1920 × 1080, 1280 × 720)Sub-Stream 50 Hz: 25 fps (704 × 576, 640 × 480) 60 Hz: 30 fps (704 × 480, 640 × 480)Third Stream 50 Hz: 25 fps (1920 × 1080, 1280 × 720, 704 × 576, 640 × 480) 60 Hz: 30 fps (1920 × 1080, 1280 × 720, 704 × 480, 640 × 480)Fourth Stream 50 Hz: 25 fps (704 × 576, 640 × 480) 60 Hz: 30 fps (704 × 480, 640 × 480)Fifth Stream 50 Hz: 25 fps (704 × 576, 640 × 480) 60 Hz: 30 fps (704 × 480, 640 × 480)Video Compression Main stream: H.265+/H.265/H.264+/H.264Sub-stream/Fourth stream/Fifth stream: H.265/H.264/MJPEG Third stream: H.265/H.264Video Bit Rate 32 Kbps to 8 MbpsH.264 Type Baseline Profile/Main Profile/High Profile H.265 Type Main ProfileBit Rate Control CBR, VBRScalable Video Coding (SVC) H.265 and H.264 supportRegion of Interest (ROI) 5 fixed regions for each streamTarget Cropping YesAudioAudio Type Mono soundAudio Compression G.711/G.722.1/G.726/MP2L2/PCM/AAC-LC/MP3Audio Bit Rate 64 Kbps (G.711)/16 Kbps (G.722.1)/16 Kbps (G.726)/32 to 192 Kbps (MP2L2)/16 to 64 Kbps (AAC-LC)/8 to 320 Kbps (MP3)Audio Sampling Rate 8 kHz/16 kHz/32 kHz/44.1 kHz/48 kHz Environment Noise Filtering YesNetworkProtocols TCP/IP, ICMP, HTTP, HTTPS, FTP, SFTP, SRTP, DHCP, DNS, DDNS, RTP, RTSP, RTCP, PPPoE, NTP, UPnP, SMTP, SNMP, IGMP, 802.1X, QoS, IPv6, UDP, Bonjour, SSL/TLS, WebSocket, WebSocketsSimultaneous Live View Up to 20 channelsAPI Open Network Video Interface (Profile S, Profile G, Profile T), ISAPI, SDK, ISUP User/Host Up to 32 users. 3 user levels: administrator, operator and userSecurity Password protection, complicated password, HTTPS encryption, 802.1X authentication (EAP-MD5), watermark, IP address filter, basic and digest authentication forHTTP/HTTPS, WSSE and digest authentication for ONVIF, TLS1.2Network Storage Support microSD/SDHC/SDXC card (256 GB), local storage and NAS (NFS, SMB/CIFS), ANRTogether with high-end Hikvision memory card, memory card encryption and health detection are supported.Client iVMS-4200, Hik-Connect, Hik-CentralWeb Browser Plug-in required live view: IE10, IE11Plug-in free live view: Chrome 57.0+, Firefox 52.0+, Safari12+ Local service: Chrome 57.0+, Firefox 52.0+ImageImage Parameters Switch YesImage Settings Rotate mode, saturation, brightness, contrast, sharpness, gain, white balance, adjustable by client software or web browserDay/Night Switch Day, Night, Auto, Schedule, Alarm Trigger (-S), Video TriggerWide Dynamic Range (WDR) 120 dBSNR ≥ 52 dBImage Stabilization EISImage Enhancement BLC, HLC, Defog, 3D DNRPicture Overlay LOGO picture can be overlaid on video with 128 × 128 24bit bmp format InterfaceVideo Output 1Vp-p Composite Output (75 Ω/BNC)Ethernet Interface 1 RJ45 10 M/100 M self-adaptive Ethernet port, optical interface FC -S: RS-485 (half duplex, HIKVISION, Pelco-P, Pelco-D, self-adaptive)On-Board Storage Built-in microSD/SDHC/SDXC slot, up to 256 GB*You are recommended to purchase memory card together with the product if needed. After ordering, the memory card will be installed to product during manufacturing.Audio -S: 1 line in/outAlarm -S: 1 input, 1 output (max. 24 VDC, 1 A or 110 VAC 500 mA)EventBasic Event Motion detection, video tampering alarm, exception (network disconnected, IP address conflict, illegal login, abnormal reboot, HDD full, HDD error), video quality diagnosis, vibration detectionSmart Event Line crossing detection, intrusion detection, region entrance detection, region exiting detection (support alarm triggered by specified target types (human and vehicle)) Scene change detection, audio exception detection (-S model), defocus detectionLinkage Upload to FTP/NAS/memory card, notify surveillance center, send email, trigger alarm output (-S model), trigger recording, trigger capture, audible warningDeep Learning FunctionHard Hat Detection Detects up to 30 human targets simultaneously Supports up to 4 shield regionsMetadata Metadata of road traffic is supportedRoad Traffic and Vehicle Detection Blocklist and allowlist: up to 10,000 recordsCaptures vehicle that has no license plateSupport license plate recognition of motorcycles (only in checkpoint scenario) Support vehicle attribute detection, including vehicle type, color, brand, etc. (City Street mode is recommended.)GeneralPower -L:100 to 240 VAC, 0.4 A, max. 22 W, terminal block PoE: 802.3at, 42.5 to 57 V, 0.55 to 0.45 A, max. 22 W -I:100 to 240 VAC, 0.4 A, max. 18 W, terminal block PoE: 802.3at, 42.5 to 57 V, 0.55 to 0.45 A, max. 18 WDimension 352 × 200 × 214 mm (13.6" × 7.9" × 8.4")Package Dimension 438 × 283 × 303 mm (17.2" × 11.1" × 11.9")Weight 14 kg (37.5 lb.)With Package Weight 15 kg (40.2 lb.)Storage Conditions -30 °C to 60 °C (-22 °F to 140 °F), humidity 95% or less (non-condensing)Startup and Operating Conditions -H model: -40 °C to 60 °C (-40 °F to 140 °F), humidity 95% or less (non-condensing) Without -H model: -30 °C to 60 °C (-22 °F to 140 °F), humidity 95% or less (non-condensing)Language 32 languages. English, Russian, Estonian, Bulgarian, Hungarian, Greek, German, Italian, Czech, Slovak, French, Polish, Dutch, Portuguese, Spanish, Romanian, Danish, Swedish, Norwegian, Finnish, Croatian, Slovenian, Serbian, Turkish, Korean, Traditional Chinese, Thai, Vietnamese, Japanese, Latvian, Lithuanian, Portuguese (Brazil)General Function Anti-flicker, 5 streams and 5 custom streams, heartbeat, mirror, privacy mask, pixel counterHeater -H: Yes ApprovalEMC FCC: FCC-SDoC(ANSI C63.4, FCC Part15 sub B)CE: CE-EMC(EN 55024: 2010 + A1: 2015, EN 55032: 2015, EN 61000-3-2: 2014, EN 61000-3-3: 2013)RCM: AS/NZS 60950.1: 2003 + Am1, Am2 and Am3, AS/NZS CISPR 32: 2015IC: IC-VoC(ICES-003 Issue 7: 2020)Safety UL: UL 60950-1: 2014, CAN/CSA C22.2 No. 60950-1-07: 2014UL: Class I, Zone 1, AEx db IIC T6 Zone 21, AEx tb IIIC T80°C Ex db IIC T6 Gb X Ex tb IIIC T80°C Db XCB: IEC IEC 60079-0CE: CE-LVD(EN 60950-1: 2006 + A11: 2009 + A1: 2010 + A12: 2011 + A2: 2013)BIS: IS 13252(Part 1)2010 + A1: 2013 + A2: 2015Protection IP68: IEC 60529-2013Explosion-Proof Protection ATEX: Ex II 2 G D Ex db IIC T6 Gb/Ex tb IIIC T80°C Db IP68IECEx: Ex db IIC T6 Gb/Ex tb IIIC T80°C Db IP68For explosive gas atmospheres, the maximum surface temperature is 85°C For flammable dust atmospheres, the maximum surface temperature is 80°C IIC: for explosive gas atmospheres other than mines susceptible to firedamp For explosive gas mixture atmospheres: Zone 1, Zone 2, Zone 21, Zone 22.Anti-Corrosion Protection NEMA 4X (NEMA 250-2014) C5 (ISO 6270-1, ISO 9227)*You are recommended to purchase memory card together with the product if needed. After ordering, the memory card will be installed to product during manufacturing.Available ModelDS-2XE6845G0-LZHS (2.8 to 12 mm)DS-2XE6845G0-LZHS (8 to 32 mm)DS-2XE6845G0-IZHS (2.8 to 12 mm)DS-2XE6845G0-IZHS (8 to 32 mm)DS-2XE6845G0-LZ (2.8 to 12 mm)DS-2XE6845G0-LZ (8 to 32 mm)DS-2XE6845G0-IZ (2.8 to 12 mm)DS-2XE6845G0-IZ (8 to 32 mm)⏹Accessory⏹OptionalDS-1707ZJ-Y-AC(OS)Wall Mount050704020220905。
iDS-2CD7586G0 S-IZHSY 8 MP IR Varifocal Dome网络摄像头说

iDS-2CD7586G0/S-IZHSY8 MP IR Varifocal Dome Network Camera⏹⏹High quality imaging with 8 MP resolution⏹Clear imaging against strong back light due to 120 dB trueWDR technology⏹Efficient H.265+ compression technology to savebandwidth and storage⏹Advanced streaming technology that enables smooth liveview and data self-correcting in poor network⏹ 5 streams and up to 5 custom streams to meet a widevariety of applications⏹Water and dust resistant (IP67) and vandal proof (IK10)⏹Anti-IR reflection bubble guarantees image qualityFunctionHard Hat DetectionWith the embedded deep learning algorithms, the camera detects the persons in the specified region. It detects whether the person is wearing a hard hat, and captures the head of the person and reports an alarm if not.Queue ManagementWith embedded deep learning based algorithms, the camera detects queuing-up people number and waiting time of each person. The body feature detection algorithm helps filter out wrong targets and increase the accuracy of detection. MetadataMetadata uses individual instances of application data or the data content. Metadata can be used for third-party application development.Smooth StreamingSmooth streaming offers solutions to improve the video quality in different network conditions. For example, in poor network conditions, adapting to the detected real-time network condition, streaming bit rate and resolution are automatically adjusted to avoid mosaic and lower latency in live view; in multiplayer network conditions, the camera transmits the redundant data for the self-error correcting in back-end device, so that to solve the mosaic problem because of the packet loss and error rate.Anti-Corrosion CoatingThe camera adopts high weatherproof and wearproof molding powder that confirms to AAMA2604-02 and Qualicoat (Class2) specifications, and uses two-layer special spraying technology, so its surface has excellent long-term anti-corrosion performance and physical protection function. Besides, all the exposed screws use SUS316 material and features highanti-corrosion performance. Therefore, the whole camera meets anti-corrosion requirements NEMA 250-2018.SpecificationCameraImage Sensor 1/2″ Progressive Scan CMOSMin. Illumination Color: 0.009 Lux @ (F1.2, AGC ON); B/W: 0.0009 Lux @ (F1.2, AGC ON) Shutter Speed 1 s to 1/100,000 sSlow Shutter YesP/N P/NWide Dynamic Range 120 dBDay & Night IR cut filterAngle Adjustment Pan: 0° to 355°, tilt: 0° to 75°, rotate: 0° to 355°Power-off Memory YesLensFocus Auto, semi-auto, manual.Lens Type & FOV 2.8 to 12 mm, horizontal FOV 112.3° to 41.2°, vertical FOV 58° to 23.1°, diagonal FOV 137.3° to 47.3°8 to 32 mm, horizontal FOV 41.8° to 14.9°, vertical FOV 22.9° to 8.5°, diagonal FOV 48.7° to 17°Aperture 2.8 to 12mm: F1.2 to F2.5 8 to 32 mm: F1.7 to F1.73Lens Mount IntegratedBlue Glass Module Blue glass module to reduce ghost phenomenon. P-Iris YesIlluminatorIR Range 2.8 to 12 mm: 30 m 8 to 32 mm: 50 mWavelength 850 nm Smart Supplement Light YesVideoMax. Resolution 3840 × 2160Main Stream 50Hz: 25fps (3840 × 2160, 3200 × 1800, 2560 × 1440, 1920 × 1080, 1280 × 720) 60Hz: 30fps (3840 × 2160, 3200 × 1800, 2560 × 1440, 1920 × 1080, 1280 × 720)Sub-Stream 50Hz: 25fps (704 × 576, 640 × 480) 60Hz: 30fps (704 × 480, 640 × 480)Third Stream 50Hz: 25fps (1920 × 1080, 1280 × 720, 704 × 576, 640 × 480) 60Hz: 30fps (1920 × 1080, 1280 × 720, 704 × 480, 640 × 480)Fourth Stream 50Hz: 25fps (1920 × 1080, 1280 × 720, 704 × 576, 640 × 480) 60Hz: 30fps (1920 × 1080, 1280 × 720, 704 × 480, 640 × 480)Fifth Stream 50Hz: 25fps (704 × 576, 640 × 480) 60Hz: 30fps (704 × 480, 640 × 480)Custom Stream 50Hz: 25fps (1920 × 1080, 1280 × 720, 704 × 576, 640 × 480) 60Hz: 30fps (1920 × 1080, 1280 × 720, 704 × 480, 640 × 480)Video Compression Main stream: H.265+/H.265/H.264+/ H.264Sub-stream/Third stream/Fourth stream/Fifth stream/custom stream:H.265/H.264/MJPEGVideo Bit Rate 32 Kbps to 16 MbpsH.264 Type Baseline Profile/Main Profile/High ProfileCBR/VBRStream Type Main stream/Sub-stream/third stream/fourth stream/fifth stream/custom stream Scalable Video Coding (SVC) H.265 and H.264 supportRegion of Interest (ROI) 4 fixed regions for each streamAudioEnvironment Noise Filtering YesAudio Sampling Rate 8 kHz/16 kHz/32 kHz/44.1 kHz/48 kHzAudio Compression G.711/G.722.1/G.726/MP2L2/PCM/MP3Audio Bit Rate 64Kbps(G.711)/16Kbps(G.722.1)/16Kbps(G.726)/32-192Kbps(MP2L2)/32Kbps(PCM)/8-320Kbps(MP3)Audio Type mono soundNetworkSimultaneous Live View Up to 20 channelsAPI Open Network Video Interface (PROFILE S, PROFILE G, PROFILE T), ISAPI, SDK, ISUPProtocols TCP/IP, ICMP, HTTP, HTTPS, FTP, SFTP, DHCP, DNS, DDNS, RTP, RTSP, RTCP, PPPoE, NTP,UPnP, SMTP, SNMP, IGMP, 802.1X, QoS, IPv6, UDP, Bonjour, SSL/TLSSmooth Streaming YesUser/Host Up to 32 users. 3 user levels: administrator, operator and userSecurity Password protection, complicated password, HTTPS encryption, 802.1X authentication (EAP-TLS, EAP-LEAP, EAP-MD5), watermark, IP address filter, basic and digest authentication for HTTP/HTTPS, WSSE and digest authentication for Open Network Video Interface, RTP/RTSP OVER HTTPS, Control Timeout Settings, Security Audit Log, TLS 1.2Network Storage microSD/SDHC/SDXC card (256 GB) local storage, and NAS (NFS, SMB/CIFS), auto network replenishment (ANR)Together with high-end Hikvision memory card, memory card encryption and health detection are supported.Client iVMS-4200, Hik-Connect, Hik-CentralWeb Browser Plug-in required live view: IE8+Plug-in free live view: Chrome 57.0+, Firefox 52.0+, Safari 11+ Local service: Chrome 41.0+, Firefox 30.0+ImageSmart IR The IR LEDs on camera should support Smart IR function to automatically adjust power to avoid image overexposure.Day/Night Switch Day, Night, Auto, Schedule, Triggered by Alarm InTarget Cropping YesDistortion Correction Distortion YesPicture Overlay LOGO picture can be overlaid on video with 128 × 128 24bit bmp format Image Enhancement BLC, HLC, 3D DNR, Defog, EIS, Distortion CorrectionImage Parameters Switch YesImage Settings Saturation, brightness, contrast, sharpness, gain, white balance adjustable by client software or web browserSNR ≥52dBInterfaceAlarm 1 input, 1 output (max. 24 VDC, 1 A)1 input (line in), 3.5 mm connector, max. input amplitude: 3.3 vpp, input impedance:4.7 KΩ, interface type: non-equilibrium;-equilibrium, mono sound; 2 built-inRS-485 1 RS-485(half duplex, HIKVISION, Pelco-P, Pelco-D, self-adaptive)Video Output 1 Vp-p Composite Output(75 Ω/CVBS) (For debugging only) On-board Storage Built-in micro SD/SDHC/SDXC slot, up to 256 GB Hardware Reset YesCommunication Interface 1 RJ45 10 M/100 M/1000 M self-adaptive Ethernet port Power Output 12 VDC, max. 100 mA (supported by all power supply types) Heater YesSmart Feature-SetBasic Event Motion detection, video tampering alarm, vibration detection, exception (network disconnected, IP address conflict, illegal login, HDD full, HDD error)Smart Event Line crossing detection, up to 4 lines configurableIntrusion detection, up to 4 regions configurableRegion entrance detection, up to 4 regions configurableRegion exiting detection, up to 4 regions configurableUnattended baggage detection, up to 4 regions configurableObject removal detection, up to 4 regions configurable,Scene change detection, audio exception detection, defocus detectionCounting Yes Intelligent (Deep Learning Algorithm)Hard Hat Detection Detects up to 30 human targets simultaneously; Supports up to 4 shield regionsPremier Protection Line crossing, intrusion, region entrance, region exitingSupport alarm triggering by specified target types (human and vehicle)Filtering out mistaken alarm caused by target types such as leaf, light, animal, and flag, etc.Queue Management Detects queuing-up people number, and waiting time of each personGenerates reports to compare the efficiency of different queuing-ups and display the changing status of one queueSupports raw data export for further analysisGeneralLinkage Method Upload to FTP/NAS/memory card, notify surveillance center, send email, trigger alarm output, trigger recording, trigger captureTrigger recording: memory card, network storage, pre-record and post-record Trigger captured pictures uploading: FTP, SFTP, HTTP, NAS, EmailTrigger notification: HTTP, ISAPI, alarm output, EmailOnline Upgrade Yes Dual Backup Yes Firmware Version V5.5.121Web Client Language 33 languagesEnglish, Russian, Estonian, Bulgarian, Hungarian, Greek, German, Italian, Czech, Slovak, French, Polish, Dutch, Portuguese, Spanish, Romanian, Danish, Swedish, Norwegian, Finnish, Croatian, Slovenian, Serbian, Turkish, Korean, Traditional Chinese, Thai, Vietnamese, Japanese, Latvian, Lithuanian, Portuguese (Brazil),UkrainianGeneral Function Anti-flicker, 5 streams and up to 5 custom streams, EPTZ, heartbeat, mirror, privacy masks,flash log, password reset via e-mail, pixel counterSoftware Reset YesStorage Conditions -30 °C to 60 °C (-22 °F to 140 °F).Humidity 95% or less (non-condensing) Startup and Operating-40 ° C (-40 °F). Humidity 95% or less (non-condensing)Power Supply12 VDC ± 20%, three-core terminal block, reverse polarity protection; 24 VAC ± 20%, three-core terminal block, reverse polarity protection; PoE: 802.3at, Type 2, Class 4Power Consumption and CurrentWith extra load: 12 VDC, 1.16 A, max. 13.9 W; PoE (802.3at, 42.5 V to 57 V), 0.33 A to 0.25 A, class 4;Without extra load: 12 VDC, 0.98 A, max. 12.5 W; PoE (802.3at, 42.5 V to 57 V), 0.3 A to 0.22 A, class 4;"with extra load" means that an extra device is connected to and powered by the camera.Power Interface Three-core terminal block Camera Material Aluminum alloy bodyCamera Dimension Ø162 × 141.8 mm (Ø6.4″ × 5.6″)Package Dimension 251 × 215 × 189 mm (9.9" × 8.5" × 7.4") Camera Weight1550 g (3.4 lb.) With Package Weight 2206 g (4.9 lb.) Anti-IR reflection bubble YesMetadata Metadata Metadata of intrusion detection, line crossing detection, region entrance detection, region exiting detection, unattended baggage detection, object removal, and queue management are supported.Approval Class Class BEMCFCC (47 CFR Part 15, Subpart B); CE-EMC (EN 55032: 2015, EN 61000-3-2: 2014, EN 61000-3-3: 2013, EN 50130-4: 2011 +A1: 2014); RCM (AS/NZS CISPR 32: 2015); IC (ICES-003: Issue 6, 2016); KC (KN 32: 2015, KN 35: 2015)SafetyUL (UL 60950-1); CB (IEC 60950-1:2005 + Am 1:2009 + Am 2:2013); CE-LVD (EN 60950-1:2005 + Am 1:2009 + Am 2:2013); BIS (IS 13252(Part 1):2010+A1:2013+A2:2015); LOA (IEC/EN 60950-1)Environment CE-RoHS (2011/65/EU); WEEE (2012/19/EU); Reach (Regulation (EC) No 1907/2006) ProtectionIP67 (IEC 60529-2013), IK10 (IEC 62262:2002) Anti-Corrosion Protection NEMA 4X (NEMA 250-2018) OtherPVC free⏹Dimension⏹Available ModeliDS-2CD7586G0/S-IZHSY(2.8-12mm), iDS-2CD7586G0/S-IZHSY(8-32mm)AccessoryDS-1475ZJ-Y Vertical Mount DS-1471ZJ-155-YPendant MountDS-1473ZJ-155-YWall MountDS-1476ZJ-YCorner Mount。
高分辨率爆破防护网络直视相机系列(HS-2XE68x5G0-IZHS)说明书

DS-2XE68x5G0-IZHSHigh Resolution Explosion-Proof Network Bullet Camera Series● 2 MP or 8 MP High Resolution Options ● 2.8 mm to 12 mm or 8 mm to 32 mm Lens Option ● 120 dB Wide Dynamic Range ● H.265, H.265+, H.264, H.264+ ● On-Board Storage up to 256 GB● ATEX: Ex II 2 G D Ex db IIC T6 Gb/Ex tb IIIC T80°C Db IP68 ● IECEx: Ex db IIC T6 Gb/Ex tb IIIC T80°C Db IP68 ● UL(Zone): Class I, Zone 1, AEx db IIC T6 Zone 21, AEx tb IIIC T80°C ● cUL(Zone): Ex db IIC T6 Gb X Ex tb IIIC T80°C Db X ● UL/cUL (Division): Class I, Division 2, Groups A,B,C and D,T6; Class II, Division 2, Groups F and G,T80°CHikvision’s DS-2XE68x5G0-IZHS Explosion-ProofNetwork Bullet Camera Series feature 2 MP or8 MP resolution and 2.8 mm to 12 mm or 8 mm to32 mm Lens options. On-board storage of up to256 GB via a microSD card offers addedfunctionality. H.265, H.265+, H.264, and H.264+video compression options saves storage space and bandwidth.Available ModelsDS-2XE6825G0-IZHS (2.8 mm to 12 mm): 2 MP DS-2XE6825G0-IZHS (8 mm to 32 mm): 2 MP DS-2XE6885G0-IZHS (2.8 mm to 12 mm): 8 MP DS-2XE6885G0-IZHS (8 mm to 32 mm): 8 MPSpecificationsDS-2XE6825G0-IZHSDS-2XE6885G0-IZHSCamera Image Sensor1/2.8" progressive scan CMOS 1/2" progressive scan CMOS Minimum Illumination 2.8 to 12 mm Color: 0.012 lux @ (ƒ/1.4, AGC on), B/W: 0.0012 lux @ (ƒ/1.4, AGC on), 0 lux with IR 8 to 32 mmColor: 0.018 lux @ (ƒ/1.7, AGC on), B/W: 0.0018 lux @ (ƒ/1.7, AGC on), 0 lux with IR Shutter Speed 1s to 1/100,000 s Slow Shutter YesDay/NightIR cut filter WDR 120 dBLens Lens Type Varifocal lens, 2.8 mm to 12 mm or 8 mm to 32 mm lens Auto-IrisDC drive Aperture2.8 mm to 12 mmƒ/1.4ƒ/1.7, constant ƒ/1.7 throughout the zoom range 8 mm to 32 mmFocusAuto, semi-auto, manualFOV2.8 mm to 12 mm Horizontal FOV 115° to 42°, vertical FOV 59° to 24°, diagonal FOV 141° to 48° Horizontal FOV 112° to 41°, vertical FOV 58° to 23°,diagonal FOV 137° to 47°8 mm to 32 mm Horizontal FOV 43° to 15°, vertical FOV 23° to 9°, diagonal FOV 50° to 17° Horizontal FOV 42° to 15°, vertical FOV 23° to 8°,diagonal FOV 49° to 17°Lens MountIntegrated IlluminatorIR Range Up to 330 ft (100 m) Wavelength 850 nmVideoMaximum Resolution 1920 × 10803840 × 2160 Video Streams 5 defined streamsMain Stream 50 Hz 50 fps (1920 × 1080, 1280 × 720) 25 fps (3840 × 2160, 3072 × 1728, 2560 × 1440,1920 × 1080, 1280 × 720)60 Hz 60 fps (1920 × 1080, 1280 × 720) 30 fps (3840 × 2160, 3072 × 1728, 2560 × 1440,1920 × 1080, 1280 × 720) Sub-Stream50 Hz 25 fps (704 × 576, 640 × 480)60 Hz 30 fps (704 × 480, 640 × 480)Third Stream50 Hz 25 fps (1920 × 1080, 1280 × 720, 704 × 576, 640 × 480)60 Hz 30 fps (1920 × 1080, 1280 ×720, 704 × 480, 640 × 480) Fourth Stream50 Hz 25 fps (704 × 576, 640 × 480)60 Hz 30 fps (704 × 480, 640 × 480) Fifth Stream50 Hz 25 fps (704 × 576, 640 × 480)60 Hz 30 fps (704 × 480, 640 × 480) Video CompressionMain stream H.265+/H.265/H.264+/H.264 Sub stream H.265/H.264/MJPEGThird stream H.265/H.264/MJPEGFourth stream H.265/H.264/MJPEG Fifth stream H.265/H.264/MJPEGH.264 Type Baseline Profile/Main Profile/High ProfileH.264+ Main stream supports H.265 Type Main profileH.265+ Main stream supports Video Bit Rate 32 Kbps to 16 MbpsSVC H.265 and H.264 support AudioAudio Compression G.711/G.722.1/G.726/MP2L2/PCMAudio Bit Rate 64 Kbps (G.711)/16 Kbps (G.722.1)/16 Kbps (G.726)/32–192 Kbps (MP2L2)Environment Noise Filtering YesAudio Sampling Rate 8 kHz/16 kHz/32 kHz/44.1 kHz/48 kHzSmart FeaturesSmart Event Line crossing detection, up to 4 lines configurable Intrusion detection, up to 4 regions configurableRegion entrance detection, up to 4 regions configurableRegion exiting detection, up to 4 regions configurableUnattended baggage detection, up to 4 regions configurable Object removal detection, up to 4 regions configurableScene change detection, audio exception detection, defocus detectionBasic Event Motion detection, video tampering alarm, exception (network disconnected, IP address conflict,illegal login, HDD full, HDD error)Face Capture Detects up to 40 faces simultaneouslyHard Hat Detection Detects up to 30 human targets simultaneouslySupports up to 4 shield regionsLinkage Method Upload to FTP/NAS/memory card, notify surveillance center, send e-mail, trigger alarm output,trigger recording, trigger captureRegion of Interest 4 fixed regions for main stream, sub stream, and third stream, and dynamic face trackingSpecifications (continued)DS-2XE6825G0-IZHSDS-2XE6885G0-IZHSImageImage EnhancementBLC, HLC, defog, EIS, distortion correction, 3D DNRImage Settings Saturation, brightness, contrast, sharpness, AGC, and white balance are adjustable by client software or Web browser Target Cropping YesDay/Night Switch Day/Night/Auto/Schedule/Triggered by alarm inPicture OverlayLogo picture can be overlaid on video with 128 × 128 24-bit bmp formatNetwork Network StorageSupports microSD/SDHC/SDXC card (256 GB), local storage, and NAS (NFS, SMB/CIFS), ANRIf used with a Hikvision high-end class memory card 1, memory card encryption and health detection are supportedProtocolsTCP/IP, ICMP, HTTP, HTTPS, FTP, DHCP, DNS, DDNS, RTP, RTSP, RTCP, PPPoE, NTP, UPnP, SMTP, SNMP, IGMP, 802.1x, QoS, IPv6, UDP, Bonjour, SSL/TLS API ONVIF (PROFILE S, PROFILE G), ISAPI, SDKSecurityPassword protection, complicated password, HTTPS encryption, 802.1x authentication (EAP-MD5), watermark, IP address filter, basic and digest authentication for HTTP/HTTPS, WSSE and digest authentication for ONVIF, TLS1.2 Simultaneous Live ViewUp to 20 channelsUser/HostUp to 32 users. 3 user levels: administrator, operator, and user ClientiVMS-4200, Hik-Connect, HikCentral Web BrowserPlug-in required live view: IE8–11Plug-in free live view: Mozilla Firefox 52+InterfaceCommunication Interface1 RJ-45 10M/100M self-adaptive Ethernet port, optical interface FC RS-485 (half duplex, HIKVISION, Pelco-P, Pelco-D, self-adaptive) Audio 1 line in/outAlarm1 input, 1 output (maximum 24 VDC, 1 A or 110 VAC 500 mA) Interface StyleConnector panelVideo Out1Vp-p composite output (75 Ω/CVBS)On-Board StorageBuilt-in microSD/SDHC/SDXC slot, up to 256 GBGeneralFirmware VersionV5.5.96Web Client Language 32 languages: English, Russian, Estonian, Bulgarian, Hungarian, Greek, German, Italian, Czech, Slovak, French, Polish, Dutch, Portuguese, Spanish, Romanian, Danish, Swedish, Norwegian, Finnish, Croatian,Slovenian, Serbian, Turkish, Korean, Traditional Chinese, Thai, Vietnamese, Japanese, Latvian, Lithuanian,Portuguese (Brazil)General Function Anti-flicker, heartbeat, mirror, privacy mask, pixel counterReset Reset via reset button on camera body, Web browser, and client softwareStartup and Operating Conditions -40° to +60° C (-40° to +140° F), humidity 95% or less (non-condensing)Storage Conditions -30° to +60° C (-22° to +140° F), humidity 95% or less (non-condensing)Power Supply 100 to 240 VAC, PoE (42.5 to 57 V, 802.3 at), terminal blockPower Consumption and Current 100 to 240 VAC, 0.4 A, maximum 18 W; PoE (802.3at, 42.5 to 57 V), 0.55 to 0.45 A, maximum 18 WMaterial Stainless steel 316L and anti-corrosion coating Heater YesDimensions Camera: 352 mm × 200 mm × 214 mm (13.6" × 7.9" × 8.4")With package: 438 mm × 283 mm × 303 mm (17.2" × 11.1" × 11.9")Weight Camera: 14 kg (37.5 lb)With package: 15 kg (40.2 lb) ApprovalsEMC 47 CFR Part 15, Subpart B; EN 55032: 2015, EN 61000-3-2: 2014, EN 61000-3-3: 2013, EN 50130-4: 2011+A1: 2014; AS/NZS CISPR 32: 2015; ICES-003: Issue 6, 2016; KN 32: 2015, KN 35: 2015Safety UL 60950-1, IEC 60950-1:2005 + Am 1:2009 + Am 2:2013, EN 60950-1:2005 + Am 1:2009 + Am 2:2013, IS13252(Part 1):2010+A1:2013+A2:2015Environment 2011/65/EU, 2012/19/EU, Regulation (EC) No 1907/2006Protection IP68 (IEC 60529-2013) Explosion-proof:ATEX: Ex II 2 G D Ex db IIC T6 Gb/Ex tb IIIC T80°C Db IP68IECEx: Ex db IIC T6 Gb/Ex tb IIIC T80°C Db IP68 UL(Zone): Class I, Zone 1, AEx db IIC T6 Zone 21, AEx tb IIIC T80°CcUL(Zone): Ex db IIC T6 Gb X Ex tb IIIC T80°C Db XUL/cUL (Division): Class I, Division 2, Groups A,B,C and D,T6; Class II, Division 2, Groups F and G,T80°C Anti-Corrosion: NEMA 4X (NEMA 250-2014), C5 (ISO 6270-1, ISO 9227) Explosion-Proof Certificate ATEX: Ex II 2 G D Ex db IIC T6 Gb/Ex tb IIIC T80°C Db IP68 IECEx: Ex db IIC T6 Gb/Ex tb IIIC T80°C Db IP68For explosive gas atmospheres, the maximum surface temperature is 85° CFor flammable dust atmospheres, the maximum surface temperature is 80° CIIC: for explosive gas atmospheres other than mines susceptible to firedamp For explosive gas mixture atmospheres: Zone 1, Zone 2, Zone 21, Zone 221You are recommended to purchase the memory card together with the product if needed. After ordering, the memory card will be installed to product during manufacturing.DimensionsAccessoriesNOT INCLUDEDDS-1707ZJ-Y-AC (OS)Wall Mount。
金属年代项目PRE-573 PREMIER微型微电压放大器说明说明书

for the 500 Series rack formatPRE-573 PREMIERINTRODUCTIONCongratulations on choosing the Golden Age Project PRE-573 PREMIER microphone preamplifier!The PRE-573 PREMIER is a one-channel vintage style microphone, line and instrument preamplifier. Thesignal path uses only discrete components like resistors, capacitors and transistors. The microphone andline input and the line output are transformer balanced, using two different transformers, each one opti-Array mized for its purpose.This is the way audio components were built before integrated circuits became available. The subjectivesound quality delivered by vintage equipment is often prefered to the one delivered by modern units, a situa-tion that is even more obvious now when music is recorded with clean sounding digital audio equipment.The circuit used in the PRE-573 PREMIER is similar to the preamp section in the classical 1073 module witha corresponding sound character that is warm, punchy, sweet and musical. These classic characteristicshave been heard on countless recordings through the years and it is a versatile sound that works very wellon most sound sources and in most genres. The essence of this sound is now available at a surprisingly lowcost, making it available to nearly everyone.FEATURES- Vintage Style electronics. No integrated circuits in the signal path.- UK-made Carnhill in- and output transformer. The output is balanced and fully floating and can drive a 600ohm load up to level of about 28 dBu.- Maximum gain in MIC mode is 80 dB, enough to handle passive ribbon mics with quiet sound sources.- The total gain range goes from -10 to +80 dB. The 20 to 70 dB range (in MIC mode) is handled by a turnswitch and a toggle switch selects an additional 5 or 10 dB gain.- Switchable impedance on the mic input, 1200 or 300 ohms, will change the tone of many mics.- The input can be configured to accept line level signals by another switch, the level is then lowered byabout 30 dB and the input impedance increased to about 10 kohm.- A selectable two-position 6 dB / octave highpass filter with fres of about 45 or 180 Hz.- A flexible front panel instrument input for electric guitar or bass that can be switched between a highimpedance active or a mid-Z passive mode. These two DI-options makes it possible to get different tonesfrom instruments. Mains connected sound modules or other signal sources should be connected to the MIC/LINE input of the 500-rack, unless they have a fully floating, transformer balanced output stage.- The output level control makes it possible to make fine gain adjustments and also to overload the maingain stage(s) for more character and then lower the signal to a suitable level before the output stage.- Insert jack for inserting Golden Age Project and Premier 500 Series eq and effect units. The insert is acti-vated by removing a jumper at the back of the module.- A soft start circuit that ramps up the supply voltage slowly (~10 sec.) to avoid a power-on current surge.- Phase switch and switchable phantom power with a LED indicator.- Selectable AIR equalizer adds a boost of about 6 dB centered at 30 kHz.- A simple but effective signal and overload indicator is offered by a single LED that starts glowing red atabout +4 dBu and then increases its intensity up to the maximum output level.- Tantalum and polystyrene capacitors used in the traditional positions.- The 6.8 kohm phantom power resistors are matched to within 0.1% to maintain good common-mode rejec-tion in the circuit.- Selectable 600 ohm output termination at the back of the module.- Circuit board star grounding scheme.- A solid build quality that will last many years of normal use.W W W.G O L D E N A G E P R E M I E R.C O M ICIRCUIT DESCRIPTIONThe signal first enters an input transformer.The primary of the input transformer hastwo windings that are either connected inseries or in parallell which results in aninput impedance of either 1200 Ohm or 300Ohm (in MIC mode).The transformer are followed by two inputgain stages. For gains up to 40 dB, only oneof them is being used. For gains from 45 dB and up, the second gain stage is inserted in the signal path. Both gain stages uses only three transistors each.The signal then goes to the Insert connector with its bypass jumper, to the output level potentiometer and from there on to the output stage. This stage again only uses three transistors, the last one in the chain is a hefty 2N3055 power transistor run in class-A mode, driving the output transformer.So, all in all, the complete signal chain only contains a maximum of nine active elements. Compare that to the big number of transistors that are usu-ally used in one single integrated circuit!USING THE PRE-573 PREMIERUsing a preamplifier is not rocket science. Here are some points though to help you getting the maximum out of the PRE-573 PREMIER:As a start, mount the module in a 500 series rack unit. There are several alternatives available from different manufacturers, the PRE-573 PREMIER should work fine in most of them. Make sure that the rack unit power supply is turned off when you mount or remove the PRE-573 PREMIER.MIC / LINE INPUTConnect your Mic or Line source to the input connector on the 500 rack unit corresponding to the slot where the PRE-573 PREMIER is placed.For Microphone sources:1. Set the MIC - LINE switch in the MIC position.2. Set the MIC/LINE - ACTIVE DI switch in the MIC/LINE position.3. Set the PASSIVE DI switch in the downward off position.4. Engage +48 V if the connected mic needs phantom power. It is good procedure to always disengage the phantom power and wait for about 10 seconds before unplugging a mic.The 300 - 1200 switch will select the input impedance. 1200 ohm is the normal position for most mics. Lowering the mic input impedance to 300 ohm will change the tone of many microphones and will give you one more soundshaping option.For Line level sources:1. Set the MIC - LINE switch in the LINE position.2. Set the MIC/LINE - ACTIVE DI switch in the MIC/LINE position.3. Set the PASSIVE DI switch in the downward off position.The LINE input mode will attenuate the input signal with about 30 dB and also increase the input impedance to about 10 kohm.If you want the smallest amount of coloration, always set the OUTPUT LEVEL potentiometer at or close to maximum, and adjust the output level with the stepped GAIN switch.The +10 - 0 - +5 dB switch should normally be in the 0 position but you can set it to +5 or +10 dB anytime you want to add gain.The +5 and +10 dB positions of this switch corresponds to the -75 and -80 dB positions (with the PRE-573 PREMIER GAIN switch set to 70 dB) and the -45 and -50 dB positions (with the PRE-573 PREMIER GAIN switch set to 40 dB ) in the classical 1073 unit.This setup makes it possible to use both gain stages also for gains of 45 and 50 dB (with the +10 - 0 - +5 switch set to 0) for some added character.If you want more character, turn the OUTPUT LEVEL potentiometer counter-clockwise and increase the gain with the GAIN switch. This will drive the input gain stage(s) harder and provoke more character from them.DI INSTRUMENT INPUTInstruments that are not connected to the mains AC power line can be con-nected to the DI instrument TRS input on the front panel.The DI input has two selectable modes, Passive and Active. The Active mode uses a FET-buffer and has an input impedance of about 1,5 Mohm. The signal is fed through the input transformer and then onwards to the gain stage(s).The Passive mode feeds the signal from the TRS jack directly to the gain stage(s). It has an input impedance of about 100 kohm.To use Active mode:1. Set the MIC/LINE - ACTIVE DI switch to the ACTIVE DI position.2. Set the PASSIVE DI switch in the off position, ie, the downward one.To use Passive mode:Set the PASSIVE DI switch in the On position, ie, the upward one.PLEASE NOTE:- DO NOT use a higher GAIN than about 70 dB in Passive mode and 50 dB in Active mode (you can try it if needed but self oscillation might occur). GAIN might have to be decreased a bit further when Air EQ is engaged to avoid self oscillation.- The DI input in Active mode has a much higher sensitivity than in Passive mode so GAIN must be set lower than in Passive mode for the same output level. The input impedance is also much higher so the DI input will load the connected instrument less compared to the DI in Passive mode.- The tone of most instrument will differ in the two modes giving you several sound options.- Some instruments will sound and work better in one of these modes, the best way of finding out is to experiment.- Signal sources connected to the 500 rack input can remain connected when you use the DI input.- DO NOT connect equipment to the DI input (unless they have a fully float-ing, transformer balanced output). It is only intended for instruments like guitars and basses. For all other signal sources, do use the MIC/LINE input on the 500-rack. The reason is that the internal ground of the module and hence the DI-input jack IS NOT the same as the 500 rack ground.OTHER FUNCTIONSThe three position HP1 - OFF - HP2 highpass filter switch is located close to the OUTPUT LEVEL control. The HP1 position has a -3dB frequency of about 45 Hz. The HP2 position has a -3dB frequency of about 180 Hz,The three position AIR - OFF - PHASE switch works like this:1. The Phase position simply reverses the phase by reversing the wires from the secondary winding of the output transformer. Reversing the phase of the signal is useful on a number of occasions, one is phase reversing the the lower mic on a snare drum to make it sum in phase with the upper mic.2. In the middle position of the switch, phase is not reversed.3. Putting the switch in the upper AIR position adds a boost of about 6 dB centered at about 30 Khz. It will affect the range from about 5 kHz and up. There is an unbalanced insert connector located at the back of the unit where you can insert Golden Age Project/Premier 500 Series effect mod-ules. The operating level is about -18 dBu. To activate the insert, the jumper located close to the connector must be removed.The output transformer used in the PRE-573 PREMIER is designed for having an ideal load of about 600 ohm. The input impedance of most modern units is 10 kohm or more. The PRE-573 PREMIER has a jumper selectable 600 ohm output termination resistor. The jumper, which is located at the back of the unit, is connected from factory to set the load to the ideal 600 ohm when modern units are connected after the PRE-573 PREMIER. Disengaging the termination resistor by removing the TERM jumper will result in a slightly higher output level. The jumper should always be discon-nected if an old-style unit with a low input impenace like 600 ohms is con-nected after the PRE-573 PREMIER.WARRANTYThe PRE-573 PREMIER is built to last. But as in any electronic device, com-ponents can break down. If the unit has a problem and will need repair, you should contact the reseller where you bought the unit.The warranty period is decided by the Distributor for your country. The Distributor will support Golden Age Premier resellers and end users with repairs and spare parts.REGISTRATIONYou are welcome to register your unit at: ---------------------------I would like to thank you for chosing the PRE-573 PREMIER!I hope it will serve you well and that it will help you in makingmany great sounding recordings.Bo MedinHandmade high end pro audio for a lot less than you would expect!W W W.G O L D E N A G E P R E M I E R.C O M。
AXIS XPQ1785 爆炸保护PTZ摄像头商品说明书

DatasheetAXIS XPQ1785Explosion-Protected PTZ Camera Globally certified explosion-protected positioning cameraAXIS XPQ1785is a robust,stainless steel(316L)PTZ camera globally certified for use in potentially combustible environ-ments according to Class I/II/III Div1and Zone1,21(NEC,CEC,IECEx,ATEX,and more).The built-in smoke alert analytic monitors for signs of smoke or fire.The camera offers total situational awareness with360°continuous pan and+/-90°tilt,both at up to200°/sec.Axis Zipstream provides exceptional savings on bandwidth and storage requirements without compromising image quality.Ease of installation is supported by a compact and low-weight design,with both RJ45and SFP for fiber or coax and100–240V AC power input.>Worldwide hazardous area certifications>Smoke alert analytics>Zipstream and Lightfinder>HDTV1080p with32x optical zoom>-60℃to60℃(-76°F to140°F)AXIS XPQ1785Explosion-Protected PTZ Camera CameraImage sensor1/2.8"progressive scan RGB CMOSLens 4.3–137mm,F1.4–4.0Horizontal field of view:60°–2.3°Vertical field of view:39°–1.3°AutofocusMinimum illumination Color:0.16lux at50IRE F1.4 B/W:0.03lux at50IRE F1.4Shutter speed1/66500s to1sPan/Tilt/Zoom Pan:360°endless,0.6-200°/sTilt:±90°,0.6-200°/s32x optical zoom and12x digital zoom,total384x zoom100preset positions,preset accuracy±0.05°,Guard Tour,ControlqueueSystem on chip(SoC)Model ARTPEC-6Memory1024MB RAM,512MB FlashVideoVideo compression H.264(MPEG-4Part10/AVC)Baseline,and Main profiles Motion JPEGResolution1920x1080HDTV1080p to160x90Maximum pixel density with32x optical zoom:25m(82ft):1912px/m50m(164ft):956px/m250m(820ft):191px/mFrame rate With WDR:Up to25/30fps(50/60Hz)in all resolutionsWithout WDR:Up to50/60fps(50/60Hz)in all resolutions Video streaming Multiple,individually configurable streams in H.264andMotion JPEGAxis Zipstream technology in H.264Controllable frame rate and bandwidthVBR/MBR H.264Image settings Saturation,contrast,brightness,sharpness,Forensic WDR,defogging,white balance,exposure mode,exposure zones,compression,mirroring of images,electronic image stabilization,text and image overlay,dynamic text and image overlayRotation:0°,180°Scene profiles:forensic,vivid,traffic overview,backlit entrance NetworkSecurity Password protection,IP address filtering,HTTPS a encryption,IEEE802.1X a network access control,digest authentication,useraccess log,centralized certificate management,signed firmware,brute force delay protectionNetwork protocols IPv4,IPv6USGv6,ICMPv4/ICMPv6,HTTP,HTTPS a,HTTP/2,TLS a, QoS Layer3DiffServ,FTP,SFTP,CIFS/SMB,SMTP,mDNS(Bonjour), UPnP®,SNMP v1/v2c/v3(MIB-II),DNS/DNSv6,,DDNS,NTP,NTS, RTSP,RTCP,RTP,SRTP,TCP,UDP,IGMPv1/v2/v3,RTCP,ICMP, DHCPv4/v6,ARP,SSH,LLDP,CDP,MQTT v3.1.1,Syslog,Link-Local address(ZeroConf)System integrationApplication Programming Interface Open API for software integration,including VAPIX®and AXIS Camera Application Platform;specifications at One-Click Cloud ConnectionONVIF®Profile G,ONVIF®Profile S,and ONVIF®Profile T, specification at Event conditions Device status:above operating temperature,above or belowoperating temperature,below operating temperature,IP addressremoved,network lost,new IP address,storage failure,systemready,within operating temperatureEdge storage:recording ongoing,storage disruption,storagehealth issues detectedI/O:manual trigger,virtual inputMQTT:subscribePTZ:PTZ malfunctioning,PTZ movement,PTZ preset positionreached,PTZ readyScheduled and recurring:scheduleVideo:average bitrate degradation,day-night mode,live streamopen,tampering Event actions Day-night mode,defog,guard tours,overlay text,presetpositions,SNMP trap messages,status LED,WDR modeMQTT:publishNotification:email,HTTP,HTTPS and TCPRecord video:SD card and network shareUpload of images or video clips:FTP,SFTP,HTTP,HTTPS,networkshare and emailData streaming Event dataBuilt-ininstallation aidsPixel counterAnalyticsApplications IncludedSmoke alert,AXIS Video Motion Detection,AXIS Fence Guard,AXIS Loitering Guard,AXIS Motion Guard,active tamperingalarm,gatekeeperSupportedAXIS Perimeter Defender,AXIS Cross Line DetectionSupport for AXIS Camera Application Platform enablinginstallation of third-party applications,see /acap GeneralCasing IP66-,IP67-and IP68-rated,electropolished ASTM316L(EN1.4404)stainless steel casing for maximum corrosion protectionWiper includedPower Max consumption:150WPower input:100–240V ACConnectors Two M25x1.5cable entries3-pole connector for power,0.2-3.0mm²(24-12AWG)2-pole connector for48V DC(max14.4W)AUX out,0.2-3.0mm²(24-12AWG)RJ4510BASE-T/100BASE-TXSFP cage for alternative network connectionStorage Pre-installed AXIS Surveillance microSDXC Card128GBSupport for SD card encryptionRecording to network-attached storage(NAS)For SD card and NAS recommendations see Operatingconditions-60°C to60°C(-76°F to140°F)Start-up temperature:-40°CHumidity10–100%RH(condensing)Storageconditions-40°C to65°C(-40°F to149°F)Humidity0-98%RH(non-condensing)Approvals EMCEN/IEC55032Class A,EN/IEC61000-3-2,EN/IEC61000-3-3,EN/IEC61000-6-1,EN/IEC61000-6-2,EN/IEC55035,FCC Part15Subpart B Class A,ICES-3(A)/NMB-3(A),RCM AS/NZS CISPR32Class AEnvironmentIEC/EN60529IP66,IP67,IP68IEC60068-2-6,IEC60068-2-27,IEC60068-2-64EN/IEC62262IK10(IK08front glass),UL50E/CSA C22.2No94.2Type4XSafetyEN/IEC62368-1,UL62368-1,CSA C22.2No.62368-1ExplosionIEC/EN60079-0,IEC/EN60079-1,IEC/EN60079-31,UL60079-0,UL60079-1,UL60079-31,CSA C22.2No.60079-0,CSA C22.2No.60079-1,CSA C22.2No.60079-31CSA C22.2No.25,CSA C22.2No.30,CSA C22.2No213,UL1203,UL121201Certifications Type P21ATEX:I M2Ex db I MbII2G Ex db IIC T5GbII2D Ex tb IIIC T100°C DbCertificate:ExVeritas20ATEX0651XIECEx:Ex db I MbEx db IIC T5GbEx tb IIIC T100°C DbCertificate:IECEx EXV20.0017XcMETus:Class I Div1Groups B,C,D T5Class II Div1Groups E,F,G T5Class I Zone1AEx db IIC GbZone21AEx tb IIICCertificate:MET E115198PESO,EAC Ex,KCs,UKCA,INMETRO pending Dimensions240x301x372mm(9.45x11.85x14.63in) Weight23kg(50.7lb)Included accessories Washer nozzle,M25to¾”NPT adapters Connector kit,installation kitOptional accessories AXIS TQ1301-E Pole Mount50-150mm(material:stainless steel,weight:5.3kg,dimensions:190x234x250mm,maximumload:150kg)AXIS TQ1001-E Wall Mount(material:stainless steel,weight:3.8kg,dimensions:170x150x350mm,maximum load:150kg)AXIS TQ1301-E Pole Mount need to be combined with AXISTQ1001-E Wall Mount.For more accessories,see VideomanagementsoftwareAXIS Companion,AXIS Camera Station,video managementsoftware from Axis Application Development Partners availableat /vmsLanguages English,German,French,Spanish,Italian,Russian,SimplifiedChinese,Japanese,Korean,Portuguese,Traditional ChineseWarranty5-year warranty,see /warrantya.This product includes software developed by the OpenSSL Project for use in theOpenSSL Toolkit.(),and cryptographic software written by Eric Young(*****************).Environmental responsibility:/environmental-responsibility©2021-2022Axis Communications AB.AXIS COMMUNICATIONS,AXIS,ARTPEC and VAPIX are registered trademarks ofAxis AB in various jurisdictions.All other trademarks are the property of their respective owners.We reserve the right tointroduce modifications without notice.T10162187/EN/M13.2/2211。
辛普西亚商务端云端门户用户指南说明书
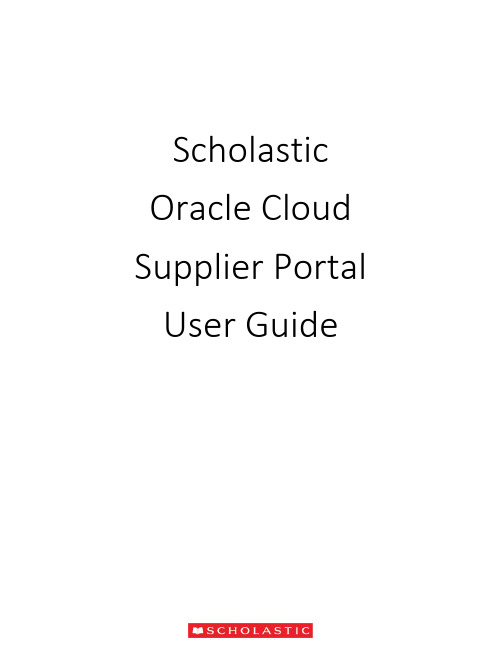
Scholastic Oracle Cloud Supplier Portal User GuideTable of ContentsIntroduction to the Supplier Portal (3)What is the Supplier Portal? (3)Navigating the Supplier portal (3)Logging in (3)Homepage Navigation (4)Notifications (5)Overview Menu (6)Summary Tab (6)Orders Tab (7)Schedules (8)Negotiations Tab (9)Request for Quote (RFQ) (10)Receiving an Invite for a Negotiation (10)Viewing the RFQ (10)Creating a Response (12)Award Decision (18)Purchase Orders (PO) (20)PO Notification (20)Review PO Details (21)Acknowledge a PO (22)Enter/Revise a Promised Ship Date for a Schedule (24)Manage Schedules (26)Order Life Cycle (27)Invoices (29)Create and Submit an Invoice (29)Miscellaneous Charges (33)Create Invoice without PO (34)View Invoices (36)View Payments (37)Supplier Preferences (39)Introduction to the Supplier PortalWhat is the Supplier Portal?The Scholastic Supplier Portal is a secure, web-based workspace that provides our vendors with full visibility to Scholastic transactions, including request for quotations (RFQ), purchase orders, and invoices.The Supplier Portal plays a key role in Scholastic’s Or acle ERP transformation, as this tool helps improve communication with our suppliers by automating and streamlining the source-to settleprocess.Navigating the Supplier portalLogging inOnce your password has been set, click on the “Oracle Fusion Prod” icon to reach the Supplier Portal home page.Once your password has been set, log in on the home pageHomepage NavigationOn the home page, click on the Supplier Portal icon to open the “Overview” page.NotificationsAt the top right corner of the home page, a bell icon will display your pending notifications. This includes new purchase orders pending acknowledgement as well as invitations to a negotiation.Note: Clicking on the notification brings you to the PO or Negotiation in questionOverview MenuThe overview page provides a snapshot into Scholastic’s most recent transact ions as well as anything requiring attention.Summary TabWorklist: A list of all pending notifications sent to the current supplier user. These are the same notifications found in the bell at the top of the page. Please note that most notifications willalso be sent via email.Watch list: Contains a set of saved searches which display counts of urgent or recenttransactions, possibly requiring action, such as Orders Pending Acknowledgment. Clicking awatch list entry navigates direct to the screen so that user can begin working on the transactions immediately.Contains the header detail of our purchase orders. The header contains the PO issued date andthe total $ amount ordered. The item level detail, quantity, and ship-to locations can be foundin “Schedules”.Orders with Recent Activity: A list of orders that have been Opened, Changed, or Canceledwithin the last week. This date can be manually changed to display more or less orders. Indicates an orderhas been cancelledA purchase order “Schedule” contains the quantity, ship-to location, and promised ship date.An order with a ship date in the past is highlighted with an alert. Schedules must be kept up todate. The promised ship dates that you provide are visible for everyone at Scholastic.At the bottom, “Recent Receipts” will list all purchase order schedules received within the lastweek.Indicates a promisedship date is past dueNegotiations TabRequest for Quotation (RFQ) transactions that the supplier is involved in or is invited to by Scholastic. It provides a quick summary to easily monitor the status and responses.Request for Quote (RFQ)Receiving an Invite for a NegotiationThe Scholastic Sourcing group has the ability to invite vendors to bid on projects through theSupplier Portal. If your organization is invited to a negotiation, you will receive an email as well as a notification in the Supplier Portal.The email invitation will include a PDF overview of the project. There is also a link that will take you directly to the RFQ in the Supplier Portal.Viewing the RFQYou can view the RFQ by clicking on the link the email notification. Another option is to go to the Supplier Portal and navigate to the Negotiations tab. Click on the negotiation number inquestion.This will bring up the RFQ cover page with the time remaining to respond displayed. On the left hand side of the RFQ, you will see links to the different components of the RFQ.Click on the Overview link. This will bring up the Overview page, which has key dates tied to the RFQ. On the right hand side, you will find attachments to the RFQ. The attachment will have the requirements for the RFQ along with instructions, quantities, and any other relevant information.Click on the Lines link. This will bring up the line items tied to the RFQ. In some cases, due to complex requirements, there will be one placeholder line visible with the advanced requirements included in an attachment.Creating a ResponseWhen you decide to bid on an RFQ, you must first acknowledge that you will participate. You can do this from the Negotiations dashboard by scrolling to the “Open Invitations” section. Highlight the negotiation, and click on the Acknowledge Participation button.This will bring up the Acknowledge Participation popup. You can select Yes or No, and enter a comment to the Scholastic buyer.At this point, if you refresh the Negotiations dashboard, you will see YES listed in the ‘Will Participate’ column. You can create your response by highlighting the Negotiation and clicking on the Create Response button.Another way to create a response is to open the RFQ and click on the Create Response button on the upper right hand sideThis will bring up the response page. Enter the quote expiration date, an internal quote number if you have one, and any notes to the buyer. You can also attach any correspondence by clicking on the + sign next to Attachments.You can attach more than one file by clicking on the + sign.Once you attach a file, it will show up on the main screen. You can remove the attachment by clicking on the X next to the file name. Hit Save and then Next.This will bring you to the “Lines” screen. Enter unit costs for the lines along with a Promised Ship Date.For most negotiations, the Scholastic buyer will give the supplier an option to create your own line by clicking on the + sign under Create Alternate. This is especially useful if you want to incorporate a unique idea or proposal.On the Alternate Line screen, you are required to enter a description, response price, and response quantity. You can enter a note to the buyer and also attach files to the alternate line.After hitting Save and Close, the alternate line will be added to the RFQ response.Hit Save and Next. This will bring you to the Review screen, where you can view the response as a whole. There are tabs for the Overview and Lines. When you are ready to submit the response to Scholastic, hit Submit.You will receive confirmation that the response was submitted.Award DecisionAfter the Scholastic buyer receives all bids, they will award either the whole job, or part of the job.If you are selected, you will receive an email notification as well as an Oracle notification confirming which lines of the negation were awarded to you. If you are not selected, it’s up to the Scholastic buyer if they want to inform the suppliers systematically that were not selected. You would receive a similar alert, but t he awarded lines amount will be “0”.Clicking on the notification will bring up the award decision. In the screenshot below, one line was awarded.Here is a screenshot of an award decision where nothing was awarded:Another way to view the outcome of the RFQ is to go to the Negotiations home screen. Scroll down to Completed Negotiations. The little green circle with a check mark indicates that the bid was awarded, while the note “No award” in the amount signifies that your bid was not accepted.Purchase Orders (PO)PO NotificationWhen a Scholastic purchase order is issued, you will receive an email notification with a PDFattachmentIf an acknowledgment is required, the email subject will include “Requires Acknowledgment”. A notification alert will also be displayed at the top of Supplier Portal to note an action is required.When a “R evised” PO is received, the email subject will include the “Revision #”Review PO DetailsOpen the attachment in the email to view a PDF of the purchase order. Additionally, on the “Orders” overview tab in the Supplier Portal, all recent PO’s will be listed under “Opened”. You will have the option of viewing a PDF, opening the order for acknowledgment, as well as updating the order with a confirmed promised ship date.Acknowledge a POSome Scholastic POs will require a supplier acknowledgment within the Portal. The PO will not be considered “open” until the Supplier completes the acknowledgment process. If a PO requires acknowl edgment, the email subject will note “Requires Acknowledgement”. You can acknowledge the PO from the link in the email or log into the supplier portal and click on the “Pending Acknowledgment” link in the Watch list.Note: A notification alert will pop-up as well as a task in the “Work list”. While both of these serve as links to the PO, it is suggested to acknowledge an order by using the link in the “Watch List”, as this method is most efficient.A “Manage Orders” screen will be displayed with all orders pending acknowledgement. Click on an order #.The PO header and details will be displayed. At the top right of the screen, select the “Acknowledge PO” button.You may need to acknowledge both the order (under “Terms”) and each Schedule line. You are given the option to “Accept” or “Reject”. Please only “Accept” the PO using this process. If there is something wrong with the order, please reach out to your Scholastic buyer via email or phone.In the top right hand corner, hit “Submit”Then hit “OK” and “Done” to close out of the order.The acknowledgment has been sent back to Scholastic and the order status is now “Open”.Enter/Revise a Promised Ship Date for a ScheduleOn every order, we send a “Requested Ship Date”. We expect that every supplier will respond with a “Promised Ship Date” confirming when you can ship. These dates are loaded into the Scholastic system for reporting purposes, so it’s i mportant that they are populated for every order and kept up to date.From the “Orders” or “Schedules” tab, select an order to edit:This brings you to the PO screen. The top part contains the PO header information with your supplier information. The “Lines” and Schedules” tabs at the bottom contain the PO detail.In the previous screenshot, the requested ship date is 4/26/18. However, the promised ship date is blank. In order to enter a new promised ship date or revise an existing promised ship date, click the “Actions” button on the top right of the screen and select “Edit”.A warning message pops up to confirm that any action will create a change order. Click “Yes”.In the “Schedules” tab, enter a new promised ship date and a change reason if applicable.Enter a description of the change order you made at the top o f the PO and then hit “Submit”.Note: Hitting “Save” will save your work, but will not send the updated date back to Scholastic. You must select “Submit”.After hitting “submit”, a popup message confirms that your changes have been sent to Scholastic. We will reach out to you with any questions.Manage SchedulesTo search and view all orders and schedules, select the task button while in the Overview screen in the supplier portalIt opens up a tab on the right side with a number of options. Under “Orders”, select “Manage Schedules”.The Manage Orders screen allows you to search all PO Schedules, open or closed. The default search is “All”, but you can search using a number of parameters, as well as setup custom searches. You can also easily tell which schedules don’t have a “Promised Ship Date” by sorting the field. In fact, all of the fields are sortable.Order Life CycleWhen you select a PO to view or edit, the “Order Life Cycle” graph can be found on the top right of the screen. It is a graphical view of the dollar amount ordered, received, delivered, and invoiced. Select “View Details” for additional information.The Order Life Cycle now displays a complete order summary including in-transit shipment information (pulled in from our OTM module), receipt dates, and invoice status.InvoicesCreate and Submit an InvoiceThe Scholastic Supplier Portal allows you to submit invoices directly to Scholastic. Processing your invoices through the supplier portal will increase the speed that your payment isprocessed. In the task list, select “Create Invoice”.In the “Create Invoice” section, s elect an order from the “Identifying PO” drop down list. This will populate most of the fields. Then enter y our internal “Invoice Number” and today’s date.In the “Items” section, hit the “Select and add” button to choose the PO lines you’d like to add to the invoice.Select the line(s) and hit “Apply”.Then hit “OK”. The item(s) has been added to the invoice.In the quantity field, enter the shipped quantity.Note: This version of Oracle Cloud does not allow overage to be invoiced. Theinvoiced quantity cannot exceed the order quantity. We are working with Oracle to correct this in a future release. For now, please work with the buyer to revise the PO when the shipped quantity exceeds the ordered amount, or add the overage amount as a miscellaneous cost under “Shipping and Handling” at the bottom of the invoice.In the Location of Final Discharge, enter the tax Province to capture the correct tax code.Once all the line items have been added to the Invoice, click on the Calculate Tax Button. Your taxes should now be calculated on the Invoice.Note: if you calculate tax after only one line item is picked, it will not work on anysubsequent line items.Miscellaneous ChargesHit the “Add” button under “Shipping and Handling” and select “Miscellaneous”. Enter an amount and descriptionNote: Freight is not a viable option as all of our shipments should route through the Scholastic Logistics group via the OTM system.Hit “Submit” to send the invoice to ScholasticA pop-up message will confirm that the invoice has been submittedCreate Invoice without POFor services completed where a Scholastic PO wasn’t issued, please submit an invoice using the “Create Invoice without PO”.On the invoice header, enter your invoice number, today’s date, and attach any pertinent documents. You must also enter the email address of the buyer at Scholastic who will receive and approve the invoice.Must be TODAYS DATEFor the invoice details, hit the + icon to add a line. Select a ship to and Location of Final Discharge, enter an amount and a description of the services provided.To add taxes, hit the “Calculate Tax” button.Note: Location of Final Discharge much be populated in order to calculate taxes on the invoice.Add miscellaneous charges at the bottom under “Shipping and Handling”.Hit “Submit” at the top of the screen and you’ll receive a confirmation message that your invoice has been submitted.To search for all submitted invoices, o n the Task Menu, select “View Invoices”Select your “Supplier” name, and hit search. You can use the fields to narrow your search as well as create custom searches. You can see the Invoice Status, as well as a Payment Number to confirm that a payment has been made against your invoice. Please note that all of these columns can be sorted.To search for invoice payment status, on the Task Menu, select “View Payments”Select your “Supplier” name, and hit search. You can use the fields to narrow your search as well as create custom searches. You can also see the payment status to confirm that a payment has been made. Select a “Payment Number” to see the complete detai ls of the payment.Complete payment detail will be displayedSupplier PreferencesOn the home page, select “Set Preferences”Select “Regional” in the General Preferences list to review the option to update your date and time format, preferred number format, primary currency, and time zone.Select “Save and Close” when finished.Select “Language” to change the primary language of the website. The default is “American English”。
活力摄像头 B87 3MP 室外放大墩相机 D N、自适应 IR、超越 WDR、3 倍放大镜头说明书

PHOTO INDICATION
DIMENSION DIAGRAM
3
1
4
5 2
6
O <>
Cube
PTZ
Speed Dome Video Encoder Video Decoder
150922
* All specifications are subject to change without notice. * All brand names and registered trademarks are the property of their respective owners.
1.26 kg (2.78 lb) 152 mm x 114.5 mm (6.0'' x 4.5'') Weatherproof (IP67 rated); Vandal proof (IK10); Transparent dome cover Surface, Pendant, Wall, Corner, Pole, Flush, Gang box -40°C ~ 50°C (-40°F ~ 122°F) within 30 minutes
Fully compatible with ACTi software Software Development Kit (SDK) available ; ONVIF compliant
Manual setting Microsoft Internet Explorer 8.0 or newer (full functionality) Safari with QuickTime installed, and other browsers with VLC installed (partial functionality)
爱迪思x 版本 10.32.8750 发布说明说明书

EDIUS® X EDIT ANYTHING. FAST Software version 10.32.8750Release NotesMay 2022Copy and Trademark NoticeGrass Valley®, GV® and the Grass Valley logo and / or any of the Grass Valley products listed in this document are trademarks or registered trademarks of GVBB Holdings SARL, Grass Valley USA, LLC, or one of its affiliates or subsidiaries. All third party intellectual property rights (including logos or icons) remain the property of their respective ownersCopyright ©2021 GVBB Holdings SARL and Grass Valley USA, LLC. All rights reserved.Specifications are subject to change without notice.Other product names or related brand names are trademarks or registered trademarks of their respective companies.Terms and ConditionsPlease read the following terms and conditions carefully. By using EDIUS documentation, you agree to the following terms and conditions.Grass Valley hereby grants permission and license to owners of to use their product manuals for their own internal business use. Manuals for Grass Valley products may not be reproduced or transmitted in any form or by any means, electronic or mechanical, including photocopying and recording, for any purpose unless specifically authorized in writing by Grass Valley.A Grass Valley manual may have been revised to reflect changes made to the product during its manufacturing life.Thus, different versions of a manual may exist for any given product. Care should be taken to ensure that one obtains the proper manual version for a specific product serial number.Information in this document is subject to change without notice and does not represent a commitment on the part of Grass Valley.Warranty i nformation is available from the Legal Terms and Conditions section of Grass Valley’s website ().Important NotificationSupported OSWindows 7 OS is no longer supported. You are only able to use EDIUS X on Windows 10 or 11 OS.If Windows Defender SmartScreen prevents the installer from startingIf Windows Defender SmartScreen prevents the installer from starting, please follow the following steps.1) Right-click the installer file then select “Properties”2) Open “Digital Signatures” tab then make sure the file has the digital signature of “GRASS VALLEY K.K.”3) Open “General” tab then check [Unlock] checkbox4) Click [Apply] button, then click [OK] button5) Run the installer againUsing with Floating LicenseIf Floating License Server is being used, its version has to be the same (or upper) as EDIUS X.Precautions when using subscription licensesSubscription licenses have the following restrictions:∙Bonus Contents for EDIUS X including the OFX Bridge are not available*OpenFX plugins cannot be used because the OFX Bridge is not available∙Disc Burner is not available∙H.264/AVC Blu-ray and Blu-ray 3D exporters are not available∙Internet connection is required for regular online validation of the licenses and the eID even for Workgroup∙The same license is not allowed to be installed on two computers at the same time*Some types of perpetual licenses are permitted to be installed on up to two computers per license only for use by asingle user under certain conditionsIf the above restrictions are an issue, please consider purchasing perpetual licenses.About offline usageFrom 10.30, the maximum offline usage period for licenses that require Internet access regularly, such as EDIUS X Pro, is shortened from 60 days to 30 days.Upgrading from an earlier buildIf you update EDIUS X from build 10.20 or earlier, eID log in dialog appears at the first startup.∙You can skip the eID validation only in Workgroup license∙If the eID validation skipped to start Workgroup editor, eID log in dialog doesn’t appear on subsequent running of EDIUS∙Internet connection is required for eID validationIf you upgrade EDIUS X from build 10.21 or earlier, the following settings of GV Job Monitor will be reset to the default settings:- Windows colors- Display settings of jobsUsing with virtual machinesWhen a virtual machine is created by duplicating another virtual machine on which EDIUS has been installed, please follow the following steps to use EDIUS with the created machine:1) Run SelfCertificationInstaller.exe on "C:\Program Files\Grass Valley\EDIUS Hub"2) Restart the OS*Only EDIUS Cloud supports use with virtual machines in cloud environments such as AWSSystem RequirementsThe following are the system requirements of this build*System requirements are subject to change without noticeNew Features & fixed issuesNew Features*No additional / improved featureFixed or improved issuesThe following issues are fixed or improved in this version:EDIUS∙When a partial render job is divided into multiple jobs, the order of these jobs is incorrect∙Export with the option "Export Between In and Out" fails if the timeline has a sequence on which "Remove cut points" is possible∙EDIUS crashes when performing "Consolidate Project" if audio waveform is displayed in the timeline∙External Render Engine crashes if EDIUS Hub Server is not found (EDIUS Hub Server environment only) Mync*No fix providedKnown issuesThis build has below known issues:EDIUS∙Frame number of source timecode is always shown as even number in 50p/60p clips∙There is a security software that detect EDIUS.exe as a malware∙Encoding in Dolby Digital Professional/Plus changes the volume of audio∙Standalone GV Job Monitor requests “EdiusHubPackage.msi” when it is launchedWorkaround: Use EDIUS integrated GV Job Monitor∙Two “EDIUS X” items appear in “App & Features” in Windows settings∙MPEG2 Elementary Stream exporter is unavailable∙Some of third party plug-ins and Bonus Contents cannot be uninstalled or updated properly if GV Render Engine is running in the backgroundWorkaround: Follow the following steps when uninstalling or updating plug-ins:1. Close EDIUS if it is running2. Click GV Render Engine on the Taskbar, then select "Pause"*If GV Render Engine is not found on the Taskbar, sign out all accounts from the OS, then sign in with the accountused to uninstall or update plug-ins3. Uninstall or update plug-ins4. Click GV Render Engine on the Taskbar, then select "Start"∙Updating Floating License Server fails if the installed version is 10.30 or earlierWorkaround: Uninstall the old version first∙If the OS has not been restarted after changing the display scaling, sizes of texts created by QuickTitler will be unexpectedly changed at the exportWorkaround: Restart GV Render Engine before file export (See FAQ for the steps)∙AVCHD 3D writer exporter fails to export files∙When a clip exported by the P2 3D exporter is registered in the bin, it will be handled as a sequence clip instead of a 3D clip∙When "Separate Left and Right" is selected to export a stereoscopic clip, only the L side file is export∙"Add and Transfer to Bin" from Amazon S3 source browser fails if the path contains multibyte characters (EDIUS Cloud only)∙Vorbis exporter fails if the number of audio channels is 7 or 8, even though the exporter's specifications allow up to 8 channels of audio∙When exporting MP3 audio, noise is exported if "Bit Depth" of audio format is set to 20bit∙The color space of the project settings is not reflected to still image files created by "Create a Still Image"function∙File export from a checked out project failsMync∙Updating Floating License Server fails if the installed version is 10.30 or earlierWorkaround: Uninstall the old version firstDesign LimitationsRestrictions by no support of QuickTime for WindowsIn both EDIUS X and Mync, QuickTime modules are no longer used even though installing QuickTime Essentials. As the result, the following file formats are no longer supported:∙Still Image File Formats: Flash Pix; Mac Pict; QuickTime Image∙Video File Formats (Import / Export): M4V or some MOV file formats*MOV files whose video formats are general ones such as MPEG-2, H.264/AVC, ProRes, etc. are able to be imported / exported∙Video File Formats (Export): 3GP (MOV); 3G2 (MOV)∙Audio File Formats: MOV (other than Linear PCM and AAC); QuickTime AudioIMPORTANT NOTEIf loaded project contains type of above clips, they will be off-line in EDIUS X。
签证 行程单中英文
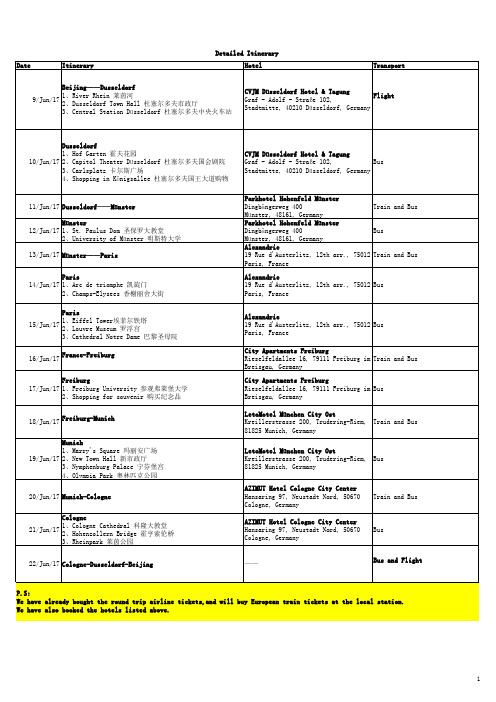
18/Jun/17 Freiburg-Munich
Munich 1、Marry's Square 玛丽安广场 19/Jun/17 2、New Town Hall 新市政厅 3、Nymphenburg Palace 宁芬堡宫 4、Olympia Park 奥林匹克公园
1
20/Jun/17 Munich-Cologne
Cologne
21/Jun/17
1、Cologne Cathedral 科隆大教堂 2、Hohenzollern Bridge 霍亨索伦桥
3、Rheinpark 莱茵公园
22/Jun/17 Cologne-Dusseldorf-Beijing
CVJM Düsseldorf Hotel & Tagung
11/Jun/17 Dusseldorf——Münster
Münster 12/Jun/17 1、St. Paulus Dom 圣保罗大教堂
2、University of Münster 明斯特大学
13/Jun/17 Münster——Paris
Paris 14/Jun/17 1、Arc de triomphe 凯旋门
2、Champs-Elysees 香榭丽舍大街
Paris
15/Jun/17
1、Eiffel 2、Louvre
Tower埃菲尔铁塔 Museum 罗浮宫
3、Cathedral Notre Dame 巴黎圣母院
16/Jun/17 France-Freiburg
Freiburg 17/Jun/17 1、Freiburg University 参观弗莱堡大学
AZIMUT Hotel Cologne City Center Hansaring 97, Neustadt Nord, 50670 Cologne, Germany
霍尼韦尔 视掉网络摄像机 HD55IP 说明书

HD55IPKey Benefits•Outstanding image quality,720p (1280 x 720) resolution•30 fps (25 fps PAL) progressive scan •Day/Night, 3.3-12 mm VFAI lens •Camera tamper detection•Video motion detection•PSIA support •Dual digital video streams,independently configurable,H.264 and/or H.264/MJPEG•Remote firmware updates•Supports both dynamic and staticIP address assignment•Single model for surface mountapplications•Includes advanced IP locator softwareto make system setup easy•Web server for remote setup of cameravideo and network parameters•Choice of 24 V AC or PoE IEEE 802.3afClass 1 power inputs•Rugged polycarbonate dome•Wall and pendant mount kits availableSPECIFICATIONSLike all cameras in the Performance Series, the HD55IP network camera uses Honeywell technology for camera video motion detection, tamper detection, remote firmware updating and for secure storage of all camera settings. Video motion detection recognizes object motion within the field of view; then a simple on-screen notification alerts the user. Camera tamper detection notifies the user when a camera’s field of view is altered, blinded, or blurred. This ensures the video’s integrity and alerts the customer to either a live event or a system problem that is causing disruption to the video stream. Honeywell’s technology also allows users to remotely upload firmware to the camera. Setup is quick and easy and can be customized through a web client or from an NVR or DVR. The built-in web server provides password-protected access to the camera’s video and network setup.System DiagramSPECIFICATIONS*Actual bitrate is scene and motion dependent.SPECIFICATIONSAutomation and Control Solutions Honeywell Systems2700 Blankenbaker Pkwy, Suite 150Louisville, KY NOTE:Honeywell reserves the right, without notification, to make changes in product design or specifications.L/HD55IPD/D December 2012© 2011 Honeywell International Inc.HD4CHIP-PK Pendant MountHD4CHIP-WK Wall Mount。
未成年球员国际转会制度与儿童权益保护——以《儿童权利公约》为视角

心是RSTP第19条,其中第1款是第19条的主要实 质性规定,它确立了严格禁止未成年球员国际转会的 一般规则。依照国际足联的禁止性规定,只有在法定 例外情形下未成年球员才能够进行国际转会。转会 规则最初仅规定了三种例外情形:(1)球员的父母因 与足球无关的原因搬到新俱乐部所在的国家(父母例 外);(2)该转会发生在欧盟或欧洲经济区境内,且该
• 22 •
从上述条文来看,RWI似乎加强了对于未成年球 员代表权的监管力度,但RWI的其他条文似乎又将原 已收紧的监管网络再一次放松了。首先,RWI放弃了 施行近15年的经纪人牌照制,代之以更宽松简便的中 介制,新规之下任何没有犯罪记录的人都可以注册成 为足球中介。同时,伴随着牌照制的废除,RWI将监 督足球中介行为的责任下放给各国的足球协会,进而 实质上放宽了对足球中介的监管。其次,尽管条例禁 止足球中介向未成年球员收取佣金,但是RWI并没有 对球员和中介之间的代表合同的最长期限进行限制, 也没有对球员签署经纪合同的最低年龄作出要求。 因此,在实践中,足球中介可以不受任何限制地与未 成年人签订长期合同,并在经纪合同中设置18周岁以 后激活支付佣金的条款。 1.3《未成年球员国际转会申请指南》
根据《公约》第1条,儿童是指18岁以下的任何 人,这与国际足联对未成年球员的定义相一致。《公 约》作为评价框架可以从两个方面助力于评价未成年 球员保护制度:首先,《公约》概述了贯穿各领域的一 般原则,这些一般原则能够用于解释和适用儿童权 利。其次,在一般原则的基础上,《公约》列举了儿童 在各领域享有的具体权利,这使得它可被用于确定在 足球行业招募球员时可能发生的侵犯儿童权利的 情况。 2.2《儿童权利公约》概述的一般原则
CHERRY STREAM DESKTOP - User 说明书
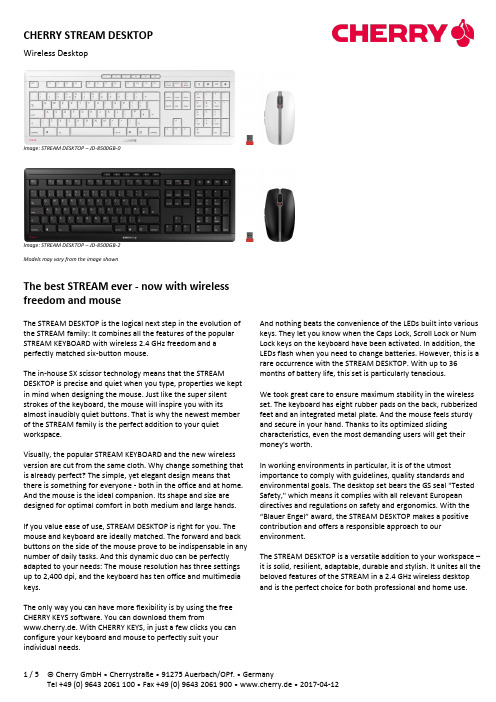
Image: STREAM DESKTOP – JD-8500GB-0Image: STREAM DESKTOP – JD-8500GB-2Models may vary from the image shownThe best STREAM ever - now with wireless freedom and mouseThe STREAM DESKTOP is the logical next step in the evolution of the STREAM family: It combines all the features of the popular STREAM KEYBOARD with wireless 2.4 GHz freedom and a perfectly matched six-button mouse.The in-house SX scissor technology means that the STREAM DESKTOP is precise and quiet when you type, properties we kept in mind when designing the mouse. Just like the super silent strokes of the keyboard, the mouse will inspire you with its almost inaudibly quiet buttons. That is why the newest member of the STREAM family is the perfect addition to your quiet workspace.Visually, the popular STREAM KEYBOARD and the new wireless version are cut from the same cloth. Why change something that is already perfect? The simple, yet elegant design means that there is something for everyone - both in the office and at home. And the mouse is the ideal companion. Its shape and size are designed for optimal comfort in both medium and large hands.If you value ease of use, STREAM DESKTOP is right for you. The mouse and keyboard are ideally matched. The forward and back buttons on the side of the mouse prove to be indispensable in any number of daily tasks. And this dynamic duo can be perfectly adapted to your needs: The mouse resolution has three settings up to 2,400 dpi, and the keyboard has ten office and multimedia keys.The only way you can have more flexibility is by using the free CHERRY KEYS software. You can download them fromwww.cherry.de. With CHERRY KEYS, in just a few clicks you can configure your keyboard and mouse to perfectly suit your individual needs. And nothing beats the convenience of the LEDs built into various keys. They let you know when the Caps Lock, Scroll Lock or Num Lock keys on the keyboard have been activated. In addition, the LEDs flash when you need to change batteries. However, this is a rare occurrence with the STREAM DESKTOP. With up to 36 months of battery life, this set is particularly tenacious.We took great care to ensure maximum stability in the wireless set. The keyboard has eight rubber pads on the back, rubberized feet and an integrated metal plate. And the mouse feels sturdy and secure in your hand. Thanks to its optimized sliding characteristics, even the most demanding users will get their money's worth.In working environments in particular, it is of the utmost importance to comply with guidelines, quality standards and environmental goals. The desktop set bears the GS seal "Tested Safety," which means it complies with all relevant European directives and regulations on safety and ergonomics. With the “Blauer Engel” award, the STREAM DESKTOP makes a positive contribution and offers a responsible approach to our environment.The STREAM DESKTOP is a versatile addition to your workspace –it is solid, resilient, adaptable, durable and stylish. It unites all the beloved features of the STREAM in a 2.4 GHz wireless desktopand is the perfect choice for both professional and home use.Key benefitsMaximum productivity:→Extremely quiet keystrokes→CHERRY SX scissor mechanism→Quiet mouse buttons→3-level adjustable mouse sensor with up to 2400 dpiHighest quality:→Rubberized feet for perfect slip resistance→Integrated metal plate for maximum torsional rigidity →…Blauer Engel“ ecolabel→Durable key lettering→Keyboard with AES-128 encryptionOptimum operating comfort:→Battery life up to 36 months (keyboard: 36 months, mouse: 12 months)→Status LEDs show low battery charge and mouse resolution→Keyboard with integrated status LEDs for the CAPS LOCK, NUM and SCROLL keys→Keyboard with 10 office & multimedia keys→6 mouse buttons and scroll wheelVery latest design:→Flat overall height→GS approval (full size layout)→Perfectly coordinated design Technical DataLayout (country or language):Product dependant, see table "Models"Housing color:Product dependant, see table "ModelsWeight (product):Keyboard: approx. 925 g (with Batterie)Mouse: approx. 115 g (with Batterie) Receiver: approx. 3 gTotal weight (with packaging):approx. 1.360 gTransmission range:approx. 10 mFrequency range:2.400 GHz - 2.4835 GHz (frequency)Effective radiated power: max. 10 mW [EIRP] Storage temperature:--20 °C to 60 °C max 85% humidityOperating temperature:0 °C to 40 °C max 85% humidityCurrent consumption:Keyboard: max. 1,2 mAMouse: max. 4 mAReceiver: typ. 20 mAInterface:USBReliability:MTBF > 80.000 hoursProduct approvals:•CE•FCC•cULus•Blauer Engel•VDE-GS•WHQL Win 10 64-bitSystem requirements:•USB port (Type A)•Windows 7, 8 or 10Delivery volume:•Keyboard•Mouse•Batteries for Keyboard (2 x AA) and Mouse (1 X AA) •Nano-USB-Receiver•Operating instruction•WarrantyTechnical Data (continued)Dimensions product:Keyboard: approx. 462,2 x 162,2 x 23,1 mmapprox. 462,2 x 165,8 x 34,1 mm (with feet up) Mouse: approx. 116 x 64,7 x 37,7 mmReceiver: approx. 19 x 14,5 x 6,5 mmPackaging dimensions:approx. 560 x 186 x 48 mmMouse:•Design: symmetrical•Scanning: Optical Sensor (PixArt)•Resolution: 1000 dpi / 1600 dpi / 2400 dpi (adjustable) •Number of buttons: 6•Model of buttons: Silent click•Function buttons: Right/Left-click, Scroll wheel; Browser forward; Browser back, DPI-switch•Mouse wheel design: Scroll wheel with key function •Status LED: DPI, battery status•Housing color: Product dependant, see table "Models" •Key color: Product dependant, see table "Models" Keyboard:•Fullsize layout with numeric pad•Key technology: CHERRY SX scissor mechanism•Service life, standard key: > 20 million key operations•Anti-slip feet 8 pieces (18 x 4 mm)•Status-LED: Caps Look, scroll- and Num-keyBattery status (via Status LED in the scroll key)•Number of additional keys: 10•Additional keys function: Volume up, Mute, Volume down, Previous title, Next title, Play/pause, Windows Lock, Browser, E-Mail program, Calculator•Housing color: Product dependant, see table "Models" •Key color: Product dependant, see table "Models" Packaging Unit:Number of products in the master carton: 10Number of master packages per pallet: 12 WarrantyWe put a lot of dedication and hard work into the development of our products and we are proud of their quality. This is why, in addition to the statutory guarantee mentioned in the operating instructions, we are granting an expanded manufacturer’s guarantee that is subject to the conditions stated below.For the first two years after delivery, the statutory warranty applies. In the third year after delivery, CHERRY voluntarily grants an additional warranty according to the following conditions ("extended warranty"). In the event of a defect, please contact the seller of your CHERRY product. Do not carry out any repairs on your own and do not open the product. There is no warranty ifunauthorized changes to the product cause a defect. Conditions for the extended warrantyIn the case of a defect after the first 2 years after delivery of the CHERRY product, CHERRY grants its customers for the additional period of one year the right to assert claims for cure, i.e. demand that the defect is remedied or a thing free of defects is supplied. The extended warranty is to be asserted against the seller of the CHERRY product upon presentation of the original invoice, proof of purchase or a comparable proof of the time of purchase. CHERRY, and the seller of the CHERRY product, where applicable, shall remedy the defect in the event the customer has justified claims for cure under the terms of the extended warranty. Excluded from the extended warranty are damages caused by improper use, in particular by the effects of chemical substances, other damages caused by external influences, as well as normal wear and tear and optical changes, in particular discoloration or abrasion of shiny areas. Also excluded from the extended warranty are accessories and other parts which are not an integral part of the purchased item.4 /5 © Cherry GmbH • Cherrystraße • 91275 Auerbach/OPf. • Germany Tel +49 (0) 9643 2061 100 • Fax +49 (0) 9643 2061 900 • www.cherry.de • 2017-04-12Models:(possible country/layout versions, others available on request)Image: STREAM DESKTOP – JD-8500GB-0JD-8500xx-0 (pale grey)* Keyboard layout not in stock. Delivery time/quantity on request.5 / 5 © Cherry GmbH • Cherrystraße • 91275 Auerbach/OPf. • Germany Tel +49 (0) 9643 2061 100 • Fax +49 (0) 9643 2061 900 • www.cherry.de • 2017-04-12Models:(possible country/layout versions, others available on request)Image: STREAM DESKTOP – JD-8500GB-2JD-8500xx-2 (black)* Keyboard layout not in stock. Delivery time/quantity on request.。
Bonjour协议简介

Where Bonjour works?
由于Bonjour协议基于组播的DNS服务,所以其 只能在本地网络中工作(LAN),不可以过WAN。 Bonjour与微软的UPnP比较相似都是在局域网工 作,但功能上各有千秋。
Bonjour的常见应用
Safari:MAC OS上广泛使用的浏览器,类似于 Windows上的IE iTunes:Apple平台上用来共享音乐
使用UDP连接,5353端口。 广播地址:224.0.0.251(IPv4) or ff02::fb(IPv6)
DNS-SD
DNS based Service Discovery,Bonjour协议的另一半, 与MDNS一起形成完美的零配置组网。
使用DNS中的SRV、TXT、PTR三个标志符来通告服务的 名称,提供详细的信息,如对象名称,服务类型,域名和配 置参数等。
一. What is Bonjour?
二. Bonjour相关内容 三. Bonjour的工作过程
What is Bonjour?
2002年被称作Rendezvous而公布于世,后于 2005年重新命名为Bonjour,源自于法语,是 “你好”的意思,发音为:[bɔnzhur]
也称为零配置联网,能自动发现IP网络上的电 脑、设备和服务。
HP SmartStream Designer与HP Mosaic和HP Collage 产品说明书
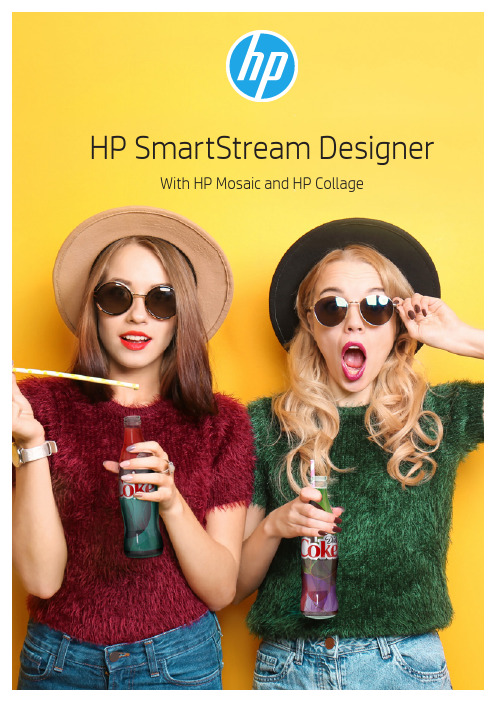
HP SmartStream Designer With HP Mosaic and HP CollageA software plug-in for Adobe® InDesign® or Adobe Illustrator®, HP SmartStream Designer makes it possible to personalize any job with images, text and designs, for maximum impact. It has an easy to-use interface and can be easily integrated with over a dozen third-party dynamic applications. It also features rich database logic and preflight capabilities.HP MosaicHP SmartStream Mosaic is variable design technology software provided withHP SmartStream Designer. It enables the creation of one-of-a-kind products fora memorable customer experience. Since its launch in 2014, it has helped renownedbrands like Coca-Cola, Planters Peanuts and Budweiser create millions of colorfuldesigns, each one of them unique.Using a patent-pending algorithm, HP Mosaic can generate virtually unlimited variations ofa seed pattern by using scaling, transposition and rotation.HP CollageHP SmartStream Collage is a new variable data tool available as part of HP SmartStream Designer. Like HP Mosaic, it can create large numbers of unique items, each with one-of- a-kind artwork. But unlike HP Mosaic, users begin the creative process by defining the basic components, or seed elements, that will be part of each and every variation.Users can control for variables like repetition, rotation and size, as well as the overlap and overall scattering of the elements. Control for overlap and overall scattering of the elements. This flexibility allows brands to retain control over the visual identity and assure brand integrity, while maximizing creative freedom.• H arness the power of variable data printing (VDP).• C reate one-of-a-kind products for a memorable customer experience.• P roduce mass customization and personalized campaigns.• E nable unique, engaging experiences for your customers— and their customers.Share with colleaguesTo find out more, visit /go/smartstreamdesigner • Rules-based variable data• HP Mosaic• HP Collage• S ecurity solutions, such as micro-text, micro QR, security fonts, Guilloché• Edge Printing and Spine Printing• E nables packing HPD for fast expansion on HP PrintOS Composer and Composer ServerIn addition, it can integrate with third-party solutions, such as:• Maps / geolocation• Personalized URLs• Barcode generationHP SmartStream Designer for Adobe InDesign• Mac OS X versions 10.13 or 10.14, Java JDK 10• Microsoft Windows 10, Java JRE 10• Minimum 16GB memory• Adobe InDesign CC 2019 64bit HP SmartStream Designer for Adobe Illustrator • Mac OS X versions 10.13 or 10.14, Java JDK 10• Microsoft Windows 10, Java JRE 10• Minimum 16GB memory • Adobe Illustrator CC 2019 64bit© Copyright 2018 HP Development Company, L.P. The information contained herein is subject to change without notice. The only warranties for HP products and services are set forth in the express warranty statements accompanying such products and services. Nothing herein should be construed as constituting an additional warranty. HP shall not be liable for technical or editorial errors or omissions contained herein.4AA6-4597EEW, November 2018Technical specifications。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
8
对服务的广告
• 应用程序提供一个服务名和端口 • 遵循跟DNS同样的“具体到一般”的模型 • 服务名 ._服务类型 . _传输协议名. 域名
■
传输协议名为TCP或UDP ■ 你自己的非常有创意的协议是不支持的...
Tuesday, November 18, 2008
9
服务命名
Canon MP780._ipp._tcp.local.
域名 协议 服务类型 (Internet Printing Protocol) (网络打印协议)
服务名称
Tuesday, November 18, 2008
10
发布一个服务
• NSNetService 被用来通过 Bonjour 发布服务
NSNetService *_service; _service = [[NSNetService alloc] initWithDomain:@”” type:@”_ipp._tcp” name:@”Canon MP780” port:4721];
• 状态以异步方式传达到代理:
- (void)netService:(NSNetService *)sender didNotResolve:(NSDictionary *)errorDict; • Same errorDict as before. - (void)netServiceDidResolveAddress:(NSNetService *)sender;
■
不需要DNS服务器来映射名字到地址 ■ 不需要目录服务器来查找服务
■
Tuesday, November 18, 2008
5
自动分配地址
• Bonjour 挑选一个随机的地址,检查是否被使用 •
如果没有被使用,就给你用 ■ 如果被使用了,再试 使用 “.local.” 作为一个虚拟的顶层域名 ■ 例如: iPhone3G.local.
12
NSNetService 代理方法
• 冲突的解决被自动处理 • 状态被传达到代理
- (void)netServiceWillPublish:(NSNetService *)sender - (void)netServiceDidPublish:(NSNetService *)sender - (void)netService:(NSNetService *)sender didNotPublish:(NSDictionary *)errorDict
Tuesday, November 18, 2008
17
服务解析
• 由NSNetServicesowser 找到的 NSNetService 必须在使用之前
解析他们的地址:
[netService setDelegate:self]; [netService resolveWithTimeout:5];
19
服务解析
• NSNetService 会为你生成 NSStream 实例
NSInputStream *inputStream = nil; NSOutputStream *outputStream = nil; [netService getInputStream:&inputStream outputStream:&outputStream];
22
什么是 Run Loop?
• 轻松的道这个!
Events
Timers
NSRunLoop
• 将NSStream 安排在 NSRunLoop 的计划表里促使他在
Tuesday, November 18, 2008
16
服务解析
Tuesday, November 18, 2008
17
服务解析
• 由NSNetServicesowser 找到的 NSNetService 必须在使用之前
解析他们的地址:
[netService setDelegate:self]; [netService resolveWithTimeout:5];
Tuesday, November 18, 2008
17
服务解析
• 由NSNetServicesowser 找到的 NSNetService 必须在使用之前
解析他们的地址:
[netService setDelegate:self]; [netService resolveWithTimeout:5];
Tuesday, November 18, 2008
19
NSStream是什么?
• 类似于 sockets ,但没有 select • 状态改变以异步方式传送给代理 • 写/读仍然是同步方式 • 你可以支持多个streams而仍然在一个线程上操作 • 设备未知 - 我们会使用sockets,但很可能是文件,内存
3
Bonjour
• 3个主要功能:
自动分配地址和映射名字 ■ 发布一个服务的可用 ■ 发现可用的服务
■
• 苹果递交给IETF的开放协议
■
Tuesday, November 18, 2008
4
Bonjour
• 使局域网自行配置
不需要管理 ■ 不需要DHCP服务器来分配地址
7
对服务的广告
• 应用程序提供一个服务名和端口 • 遵循跟DNS同样的“具体到一般”的模型 • 服务名 ._服务类型 . _传输协议名. 域名
■
服务类型是一个在IANA注册过的协议名 ■ 最多14个字符 ■ 格式是 [a-z0-9]([a-z0-9\-]*[a-z0-9])?
Tuesday, November 18, 2008
Tuesday, November 18, 2008
15
NSNetServiceBrowser 代理方法
• NSNetServiceBrowser 浏览也是异步的 • 代理方法在服务到来和离去时被调用
- (void)netServiceBrowserWillSearch:(NSNetServiceBrowser *)browser - (void)netServiceBrowserDidStopSearch:(NSNetServiceBrowser *)browser - (void)netServiceBrowser:(NSNetServiceBrowser *)browser didNotSearch:(NSDictionary *)errorInfo - (void)netServiceBrowser:(NSNetServiceBrowser *)browser didFindService:(NSNetService *)service moreComing:(BOOL)more - (void)netServiceBrowser:(NSNetServiceBrowser *)browser didRemoveService:(NSNetService *)service moreComing:(BOOL)more
Tuesday, November 18, 2008
17
服务解析
• 由NSNetServicesowser 找到的 NSNetService 必须在使用之前
解析他们的地址:
[netService setDelegate:self]; [netService resolveWithTimeout:5];
CS193P - 讲座 17
iPhone 应用程序开发
使用Bonjour和NSStream类处理网络
Tuesday, November 18, 2008
1
主题
• Bonjour
■
自动设置
• NSStream
■
异步通讯
Tuesday, November 18, 2008
2
僵尸节!
Tuesday, November 18, 2008
也能获得一个NSStreams指向这个位置
Tuesday, November 18, 2008
14
发现一个服务
• NSNetServiceBrowser 被用来在网络上搜索服务。
NSNetServiceBrowser *_browser; _browser = [[NSNetServiceBrowser alloc] init]; [_browser setDelegate:self]; [_browser searchForServicesOfType:@”_ipp._tcp.” inDomain:@””];
■
Tuesday, November 18, 2008
6
对服务的广告
• 应用程序提供一个服务名和端口 • 遵循跟DNS同样的“具体到一般”的模型 • 服务名 ._服务类型 . _传输协议名. 域名
■
服务名是一个人可以阅读的描述性的名字 ■ 最多63个UIF-8字符 ■ 所有字符都允许
Tuesday, November 18, 2008
• 状态以异步方式传达到代理:
- (void)netService:(NSNetService *)sender didNotResolve:(NSDictionary *)errorDict; • Same errorDict as before. - (void)netServiceDidResolveAddress:(NSNetService *)sender;
• 关闭一个 stream
[stream close]; [stream removeFromRunLoop:[NSRunLoop currentRunLoop] forMode:NSRunLoopCommonModes]; [stream setDelegate:nil];
Tuesday, November 18, 2008