Chapter2 HTML
Pug 指南:一个用于创建HTML模板的JavaScript模板引擎说明书

Table of ContentsAbout1 Chapter 1: Getting started with pug2 Remarks2 Versions2 Examples2 Installation2 Hello World Example3 Chapter 2: Conditionals5 Introduction5 Syntax5 Parameters5 Remarks5 Examples6 If/Else Statement in Pug6 If/Else Statement in Pug (with a dash)6 Else If Statement6 Unless Operator7 Chapter 3: Interpolation with Pug8 Introduction8 Syntax8 Parameters8 Remarks9 Examples9 Server Side Variable Interpolation9 Raw Variable Interpolation in HTML10 Value Interpolation in JavaScript Code10 HTML Element Interpolation13 Chapter 4: Iteration with Pug14 Introduction14 Remarks14Examples14 Each iteration14 Chapter 5: Syntax and markup generation16 Introduction16 Remarks16 Examples16 From Pug to HTML16 Credits18AboutYou can share this PDF with anyone you feel could benefit from it, downloaded the latest version from: pugIt is an unofficial and free pug ebook created for educational purposes. All the content is extracted from Stack Overflow Documentation, which is written by many hardworking individuals at Stack Overflow. It is neither affiliated with Stack Overflow nor official pug.The content is released under Creative Commons BY-SA, and the list of contributors to each chapter are provided in the credits section at the end of this book. Images may be copyright of their respective owners unless otherwise specified. All trademarks and registered trademarks are the property of their respective company owners.Use the content presented in this book at your own risk; it is not guaranteed to be correct nor accurate, please send your feedback and corrections to ********************Chapter 1: Getting started with pugRemarksPug is a high performance, robust, elegant, feature rich template engine. It was influenced by Haml and implemented with JavaScript for Node.js and browsers. Implementations exists for Laravel, PHP Scala, Ruby, Python and Java.It features:•Express integrationConditionals••Filters•Includes•Inheritance•Interpolation•IterationMixins•Pug was previously known under the Jade name but has been renamed because of a registered trademark case.This remark section should also mention any large subjects within pug, and link out to the related topics. Since the Documentation for pug is new, you may need to create initial versions of those related topics.VersionsExamplesInstallationTo install the Pug template rendering system, follow these steps:1.Have the Node.js environment installed on your machine2.Run npm install pug --save to install the pug module to your current project.You can now use pug in your project through the standard require mechanism:const pug = require("pug");If you are using Express in your application, you do not need to require("pug"). However, you must set the view engine property of your Express application to pug.app.set("view engine", "pug");Further, you must set the view directory of your app so that Express knows where to look for your Pug files (for compilation).app.set("views", "path/to/views");Within your Express route, you can then render your Pug files by calling the res.render function with the path of the file (starting from the directory set by the app.set("views") option).app.get("/", function (req, res, next) {// Your route codevar locals = {title: "Home",};res.render("index", locals);});In the above, index points to a file located at views/index.pug, and locals represents an object of variables that are exposed to your file. As will be explained in later sections, Pug can access variables passed to it and perform a variety of actions (conditionals, interpolation, iteration, and more).Hello World ExampleFirst, let's create a template to be rendered!p Hello World, #{name}!Save this in a file ending with the .pug extension (you can call it anything you like, but we will use view.pug in the following code to compile it).All that's left to do, now, is compile that template! Create a JS script file (we'll call ours main.js), and add the following content:// Import the pug moduleconst pug = require('pug');// Compile the template (with the data not yet inserted)const templateCompiler = pileFile('view.pug');// Insert your data into the template fileconsole.log(templateCompiler({ name: 'John' });When you run this file with npm main.js, you should get the following HTML code output in your console:<p>Hello World, John!</p>Congratulations, you just created and compiled your first template! On to more advanced stuff, such as Conditionals, Iteration, and much more!Read Getting started with pug online: https:///pug/topic/8613/getting-started-with-pugChapter 2: ConditionalsIntroductionPug can conditionally run code based on variables (passed from your server or based in Pug itself).Syntaxif (statement)•// Pug code•else if (statement)// Pug codeelse•// Pug codeunless (statement)•// Pug codeParametersRemarksOfficial PugJS documentation on conditionalsExamplesIf/Else Statement in PugConditionals in Pug can evaluate statements in a manner similar to JavaScript. You can evaluate variables created in Pug, or those passed to it by your route (res.render, pug.renderFile, etc).index.jsvar authorized = trueres.render("index", {authorized: authorized});index.pug- var showLogin = false;if authorized && showLogin === true.welcome Welcome back to our website!else.logina(href="/login") Loginindex.pug output<div class="login"><a href="/login">Login</a></div>If/Else Statement in Pug (with a dash)You can choose to prepend an if or else operator with a dash, but it is not necessary. You will need to wrap the statement in parentheses, though (if you omit a dash, you do not need parentheses.)- var showLogin = false;- if (showLogin === true).welcome Welcome back to our website!- else.logina(href="/login") Loginindex.pug output<div class="login"><a href="/login">Login</a></div>Else If StatementYou can chain any number of else if statements to an existing if statement, to evaluate asequence of statements.index.pug- var page = 60;if page => 52h1 Lots of numbers!else if page > 26 && page < 52h1 A few numberselseh1 Not a lot of numbersindex.pug output<h1>Lots of numbers!</h1>Unless Operatorunless is the inverse operation of if in Pug. It is analogous to if !(statement).index.pug- var likesCookies = true;unless likesCookies === trueh2 You don't like cookies :(elseh2 You like cookies!index.pug output<h1>You like cookies!</h1>Note: else unless statements do not work with unless; you can chain an else if statement to an unless statement, but else unless does not work.Read Conditionals online: https:///pug/topic/9662/conditionalsChapter 3: Interpolation with PugIntroductionIt's important to be able to use server-side variables in your website. Pug allows you to interpolate data generated by your server in HTML, CSS, and even JavaScript code.Syntaxres.render(path, variables) // Searches for a pug file to render at path "path", and passes •"variables" to it•#{variable} // Interpolates "variable" inline with the surrounding Jade code, after evaluating "variable"!{variable} // Interpolates "variable" inline with the surrounding Jade code, without evaluating •"variable".•#[element] // Interpolates "element" inside of an existing Pug HTML element. Syntax ofinterpolated HTML elements is identical to that of normal HTML elements. ParametersRemarksFor more information on PugJS interpolation, see the official PugJS interpolation documentation. ExamplesServer Side Variable InterpolationIt's possible to pass variables from your server into Pug for dynamic content or script generation. Pug templates can access variables passed to the res.render function in Express (orpug.renderFile if you are not using Express, the arguments are identical).index.jslet colors = ["Red", "Green", "Blue"];let langs = ["HTML", "CSS", "JS"];let title = "My Cool Website";let locals = {siteColors: colors,siteLangs: langs,title: title};res.render("index", locals);Inside your index.pug file, you then have access to the locals variable by way of its keys. The names of the variables in your Pug file become siteColors and siteNames.To set the entirety of an HTML element equal to a variable, use the equals operator = to do so. If your variable needs to be embedded inline, use bracket syntax #{} to do so.index.pugdoctype htmlhtmlheadtitle= titlebodyp My favorite color is #{siteColors[0]}.p Here's a list of my favorite coding languagesuleach language in siteLangsli= languageindex.pug output<!DOCTYPE html><html><head><title>My Cool Website</title></head><body><p>My favorite color is Red.</p><p>Here's a list of my favorite coding languages</p><ul><li>HTML</li><li>CSS</li><li>JS</li></ul></body></html>Raw Variable Interpolation in HTMLContent interpolated with bracket syntax will be evaluated for code, the output of which is included in your HTML output.title follows the basic pattern for evaluating a template local, but the code in between #{and } is evaluated, escaped, and the result buffered into the output of the templatebeing rendered. [Source]If you need to include raw HTML syntax, use an exclamation point instead of a pound symbol (!{} instead of #{}).index.jslet tag = "<div>You can't escape me!</div>";res.render("index", {myTag: tag});index.pugdoctype htmlhtmlheadbody!{myTag}index.pug output<!DOCTYPE html><html><head></head><body><div>You can't escape me!</div></body></html>Value Interpolation in JavaScript CodeInterpolating values is helpful if you need to pass a server-side variable to client-side JavaScript (or other languages that require it).In the case of variables, numbers, strings, and the like, you can pass these types of variables directly into your JavaScript with bracket syntax plus an explanation point (so that the code inside the brackets is not evaluated.) This is useful for parametrizing JavaScript code that require something from your server.In the below example, we have to wrap username in quotation marks in order for JavaScript to interpret it as a string; Pug will output the content of the variable as-is, so we need to put it in quotation marks for it to be a proper JavaScript string. This is not necessary for number, where JavaScript will interpret our number as it we intend it to (as a number).index.jslet number = 24;let username = "John";res.render("index", {number: number,username: username});index.pughtmlheadscript.// Sets the username of the current user to be displayed site-widefunction setUsername(username) {// ...}var number = #{number};var username = "#{username}";setUsername(username);bodyp Welcome to my site!index.pug output<html><head><script>// Sets the username of the current user to be displayed site-widefunction setUsername(username) {// ...}var number = 24;var username = "John";setUsername(username);</script></head><body><p>Welcome to my site!</p></body></html>If you need to interpolate the value of a JavaScript object (e.g. all the information about a user),you must stringify the output in Pug for it to be treated as a JavaScript object. It's also necessary to output the raw contents of the variable, instead of the evaluated form of it. If you were to do output the escaped variable (var user = #{JSON.stringify(user)}), you would receive an escaped version of the object (where quotation marks and apostrophes are converted to "), which is not what we want in order for JSON.stringify to work on it.index.jsvar myUser = {name: "Leeroy Jenkins",id: 1234567890,address: "123 Wilson Way, New York NY, 10165"};res.render('index', {user: myUser});index.pugdoctype htmlhtmlheadscript.window.onload = function () {function setUsername(username) {return username;}var user = !{JSON.stringify(user)};document.getElementById("welcome-user").innerHTML = setUsername();};bodydiv(id="welcome-user")index.pug output<!DOCTYPE html><html><head><script>window.onload = function() {function setUsername(username) {return username;}var user = {"name": "Leeroy Jenkins","id": 1234567890,"address": "123 Wilson Way, New York NY, 10165"};document.getElementById("welcome-user").innerHTML = setUsername();};</script></head><body><div id="welcome-user"></div></body></html>The innerHTML of #welcome-user becomes equal to Leeroy Jenkins. The contents of the user variable are printed directly to the HTML sourceHTML Element InterpolationIt may be necessary to nest HTML tags inside of each other. Element interpolation is done in a syntax similar to variable interpolation; square brackets instead of curly braces are used here. The syntax of interpolated HTML elements is identical to the implementation of normal HTML elements.index.pugdoctype htmlhtmlheadtitle My Awesome Websitebodyp The server room went #[b boom]!p The fire alarm, however, #[u failed to go off...]p Not even #[a(href="https:///") Stack Overflow] could save them now. index.pug output<!DOCTYPE html><html><head><title>My Awesome Website</title></head><body><p>The server room went <b>boom</b>!</p><p>The fire alarm, however, <u>failed to go off...</u></p><p>Not even <a href="https:///">Stack Overflow</a> could save them now.</p></body></html>Read Interpolation with Pug online: https:///pug/topic/9565/interpolation-with-pugChapter 4: Iteration with PugIntroductionHow to iterate over a simple JSON object and save a lot of typing RemarksYou need to have Node.js and Pug installedExamplesEach iterationBuild an app.js with a simple data store:app.get("/bookstore", function (req, res, next) {// Your route datavar bookStore = [{title: "Templating with Pug",author: "Winston Smith",pages: 143,year: 2017},{title: "Node.js will help",author: "Guy Fake",pages: 879,year: 2015}];res.render("index", {bookStore: bookStore});});Iterate over the data store using an index.pug file and an each loop:each book in bookStoreulli= book.titleli= book.authorli= book.pagesli= book.yearResult will be:<ul><li>Templating with Pug</li><li>Winston Smith</li><li>143</li><li>2017</li></ul><ul><li>Node.js will help</li><li>Guy Fake</li><li>879</li><li>2015</li></ul>ReferenceRead Iteration with Pug online: https:///pug/topic/9545/iteration-with-pugChapter 5: Syntax and markup generation IntroductionA preview of the difference between pug code and the generated markupRemarksPug makes possible to write HTML in a simplest way, using a clean, whitespace sensitive syntax. ExamplesFrom Pug to HTMLdoctype htmlhtml(lang="en")headtitle= pageTitlescript(type='text/javascript').if (foo) bar(1 + 5)bodyh1 Pug - node template engine#container.colif youAreUsingPugp You are amazingelsep Get on it!p.Pug is a terse and simple templating language with astrong focus on performance and powerful features.Becomes:<!DOCTYPE html><html lang="en"><head><title>Pug</title><script type="text/javascript">if (foo) bar(1 + 5)</script></head><body><h1>Pug - node template engine</h1><div id="container" class="col"><p>You are amazing</p><p>Pug is a terse and simple templating language with a strong focus on performance and powerful features.</p></div></body></html>Read Syntax and markup generation online: https:///pug/topic/9549/syntax-and-markup-generationCreditshttps:///18。
最新香港朗文5A 第二单元知识要点

朗文5A Chapter 2背诵内容一、单词1.leaf /li:f/ n. 叶子复数:leavespetition /ˌkɒmpəˈtɪʃn / n. 竞赛3.advertisement /ədˈvɜ:tɪsmənt/ n. 广告4.magazine /ˌmægəˈzi:n / n. 杂志5.programme /ˈprəʊgræm/ n. 节目6.level /ˈlevl/ n. 水平7.test /test/ n. 测验8.dictionary /ˈdɪkʃənrɪ/ n. 字典9.achieve/əˈtʃi:v / v. 实现,获得10.subtitle /ˈsʌbtaɪtl/ n. 字幕11.attention /əˈtenʃn/ n. 注意力12.mop /mɒp/ n. 拖把13.broom /bru:m/ n. 扫帚14.dirt /dɜ:t / n. 污垢15.dust /dʌst / n. 灰尘16.agree /əˈgri:/ v. 同意二、词组P11.turn over a new leaf 掀开新的一页,改过自新重新开始2.make some plans 制定计划3.keep fit 保持身材4.play more sport 做更多运动5.win the swimming competition 赢得游泳竞赛6.take a Japanese course 上日语课7.get high marks 得高分8.put on weight 增重9.eat more 吃多点10.improve one’s English 提高英语11.read more English books 读更多的英语书12.become the school’s best storyteller 成为校故事大王13.join the Storytelling Club 加入讲故事社团14.meet your target 达成目标P215.social worker 社工16.tell sb about sth 告诉某人关于某事17.prepare for your lessons 预习18.look up new words in the dictionary 在字典里查生词19.revise your lessons 复习20.make notes while you study 学习时做笔记21.check your work 检查作业22.walk/run up the stairs 走上楼梯23.get off the bus one stop earlier 提前一站下车24.watch English programmes and movies without Chinese subtitles 看没有中文字幕的英语节目和电影25.pay more attention in English lessons 英语课上集中注意力26.eat only fruit at recess 课间只吃水果27.see an eye doctor 看眼科医生28.go outside and get some fresh air 到外面呼吸新鲜空气P329.take part in 参加join 是加入,注意区别30.pass level two of the Advanced English Tests 过高级英语测试二级31.walk / run home 走/跑回家注意不加to32.achieve your target 完成目标33.learn something important 学了很重要的事34.learn a lesson 学到了经验,吸取教训35.from now on 从现在起36.be late for school 上学迟到37.get my school things ready the night before 前一天晚上准备好上学用品38.stop eating snacks 停止吃零食三、句子1. What do you want to do? 你想做什么?2. I want to get high marks so I’m going to prepare for my lessons and look up new words in the dictionary. 我想要得高分所以我要提前预习和用词典查生词。
制作EPUB电子书

这段时间研究了一下iBooks的电子书ePub格式。
现在市面上很多电子书设备(如盛大汉王的电子书),Android和黑莓的手机及平板电脑(要安装支持ePub格式的软件)都支持ePub 格式,所以ePub格式的电子书现在比较流行。
这里先简单讲一下ePub格式的信息。
ePub格式本质上是一个Zip压缩包,里面使用特定的目录结构来保存书籍内容,包括文本和图像(当然也可以包含音频视频,但是只有特定设备才支持,比如iBooks)书籍内容的保存形式是网页形式,html 和xhtml两种。
因此ePub图书显示的效果也就是一张网页能显示的效果。
目前制作iBooks能够阅读的ePub格式是比较简单的,不论是Windows上面还是Mac上面都有很多将txt直接合并转为ePub 格式的软件。
这种工具生成的ePub电子书,没有目录或者目录粗陋,只能从头看到尾,没有图片显示(除了封面外),适合那种对看书要求不高的人。
如果大家要制作的比较专业,不像iBook商店里面那些免费的鲁迅全集那样凑数(没有封面,没有分节,差不多就是txt转过来的),那就需要一个范本,就是免费的小熊维尼。
iBooks附送的这本小熊维尼是不收费的,而且它采用了DRM 保护,你即使解压缩了也无法看到图像和文本的任何内容,只能在iBooks里面看。
这本书为什么可以作为范本呢?因为它基本上展示了iBooks上ePub格式能够展现的所有效果:1.一页中可同时显示不同的字体大小,可同时显示不同的字体样式(斜体/粗体),可同时显示不同的字体颜色,可以显示首字大写(由于目前iOS设备只允许一个中文字体,所以首字大写和改字体族、粗体等效果都是英文专用福利);2.图片可以排列为上下型环绕并居中,左环绕,右环绕以及四周环绕效果;3.可以插入本文目录链接和在线网页链接。
目前Windows和Mac平台下,能够完全制作出这些效果的ePub 编辑器是没有的,只有两个替代品:Windows的epubbuilder 和Mac的Pages。
Chapter 2-钻井液流变性能

钻井液常用流型:
① 牛顿流体(Newtonian Fluids) ② 宾汉流体(Bingham Plastic Flow) ③ 幂律流体(Power law flow) ④ 卡森流体(Casson flow)
1、牛顿流体
①
这类流体有如下特点:当τ>O时,γ>0,因此只要对牛顿流体施 加一个外力,即使此力很小,也可以产生一定的剪切速率,即 开始流动。 其粘度不随剪切速率的增减而变化。
为了确定内摩擦力与哪些因素有关,牛顿通过大量实 验研究提出了液体内摩擦定律,通常称为牛顿内摩擦定律。 其内容为:液体流动时,液体层与层之间的内摩擦力(F)的 大小与液体的性质及温度有关,并与液层间的接触面积(S) . 和剪切速率( )成正比,即:
F S
μ –viscosity, the frictional resistance ;
典型牛顿流体流变图分析
不同物质有不同粘度。
牛顿流体流变图,其流变曲线均为通过原点O的一条直线,
但粘度越高(如甘油,在15℃时为2.33Pa· s),其斜率越大,
即流变曲线与x轴的夹角越大。粘度越低(如空气,在 15℃时为0.0182╳10-3Pa· s),其斜率越小。
水的动力粘度,15℃时为1.1405×10-3 Pa· s,20℃时为
是指在外力作用下,钻井液发生流动和变形的特性。
该特性通常用钻井液的流变公式、流变曲线和流变参数,如
塑性粘度(Plastic Viscosity)、动切力(Yield Point)、 静切力(Gel Strength)、表观粘度(Apparent Viscosity) 等来进行描述的。
流变参数是流变方程的常数。
用前,应用清水进行校正。该仪器测量清水 的粘度为15±0.5秒。若误差在±1秒以内,可用下 式计算泥浆的实际粘度。
Markdown菜鸟教程
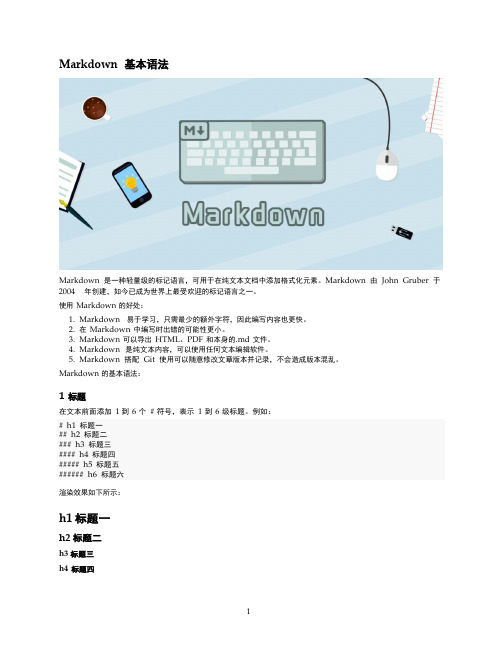
Markdown基本语法Markdown 是一种轻量级的标记语言,可用于在纯文本文档中添加格式化元素。
Markdown 由John Gruber 于2004 年创建,如今已成为世界上最受欢迎的标记语言之一。
使用Markdown 的好处:1.Markdown 易于学习,只需最少的额外字符,因此编写内容也更快。
2.在Markdown 中编写时出错的可能性更小。
3.Markdown 可以导出HTML、PDF 和本身的.md 文件。
4.Markdown是纯文本内容,可以使用任何文本编辑软件。
5.Markdown 搭配Git 使用可以随意修改文章版本并记录,不会造成版本混乱。
Markdown 的基本语法:1标题在文本前面添加1 到6 个# 符号,表示1 到6 级标题。
例如:# h1 标题一## h2 标题二### h3 标题三#### h4 标题四##### h5 标题五###### h6 标题六渲染效果如下所示:h1 标题一h2 标题二h3 标题三h4 标题四h5 标题五h6 标题六对应HTML 如下所示:<h1>h1 标题一</h1><h2>h2 标题二</h2><h3>h3 标题三</h3><h4>h4 标题四</h4><h5>h5 标题五</h5><h6>h6 标题六</h6>标题编号要添加自定义标题ID,请将自定义ID括在与标题相同的行上的大括号中:### HellO Heading Title {#title-id}渲染效果如下所示:Hello Heading Title对应HTML 如下所示:<h3 id="title-id">HellO Heading Title</h3>2强调粗体使用两个连续* 或_ 符号包围文本来表示粗体,例如:渲染效果如下所示:rendered as bold textrendered as bold textHTML 如下所示:<strong>rendered as bold text</strong>斜体字使用一个* 或_ 符号包围文本来表示斜体。
html Chapter2
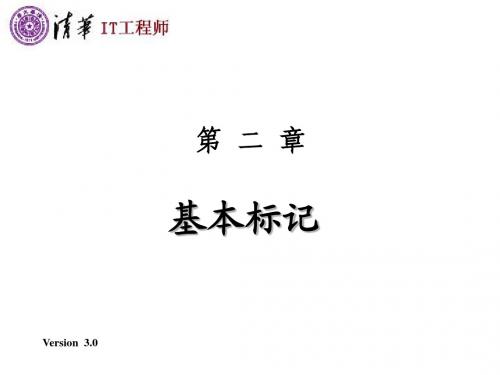
25
创建列表
6
段落级标记: 段落级标记:<PRE> 标记
如果希望文本以预定义的格式显示,可以使用 元素。 如果希望文本以预定义的格式显示,可以使用PRE元素。此元素用于定义文本的 元素 格式。文本在浏览器中显示时遵循在HTML源文档中定义的格式。 源文档中定义的格式。 格式。文本在浏览器中显示时遵循在 源文档中定义的格式 <HTML> <HEAD> <TITLE>学习 学习HTML</TITLE> 学习 </HEAD> <BODY> <PRE> 汉普蒂·邓普蒂坐在墙上 汉普蒂 邓普蒂坐在墙上 汉普蒂·邓普蒂摔了一大跤 汉普蒂 邓普蒂摔了一大跤 国王所有的马 和所有的人 也无法再把汉普蒂·邓普蒂拼起来 也无法再把汉普蒂 邓普蒂拼起来 </PRE> </BODY> </HTML>
26
创建列表
•
技巧,你可以用start属性为一个有序列表规定特殊的开 技巧,你可以用start属性为一个有序列表规定特殊的开 start 头数字或字母,默认的开始数字是1 要改变这种状况, 头数字或字母,默认的开始数字是1,要改变这种状况, 可以给lo标识符添加start属性。 lo标识符添加start属性 可以给lo标识符添加start属性。例如
11
水平标尺
<HR>水平标尺标记用于在页面上绘制一条水平线。可借助于下列属性控制水平线。 水平标尺标记用于在页面上绘制一条水平线。可借助于下列属性控制水平线。 水平标尺标记用于在页面上绘制一条水平线 它只有开始标记,没有结束标记,且不包含任何内容。 它只有开始标记,没有结束标记,且不包含任何内容。
a标签target属性详解

a标签target属性详解HTML 标签的target 属性HTML 标签定义和用法标签的target 属性规定在何处打开链接文档。
如果在一个标签内包含一个target 属性,浏览器将会载入和显示用这个标签的href属性命名的、名称与这个目标吻合的框架或者窗口中的文档。
如果这个指定名称或id的框架或者窗口不存在,浏览器将打开一个新的窗口,给这个窗口一个指定的标记,然后将新的文档载入那个窗口。
从此以后,超链接文档就可以指向这个新的窗口。
打开新窗口被指向的超链接使得创建高效的浏览工具变得很容易。
例如,一个简单的内容文档的列表,可以将文档重定向到一个单独的窗口:Table of Contents target="view_window">Prefacetarget="view_window">Chapter 1target="view_window">Chapter 2target="view_window">Chapter 3亲自试一试当用户第一次选择内容列表中的某个链接时,浏览器将打开一个新的窗口,将它标记为"view_window",然后在其中显示希望显示的文档内容。
如果用户从这个内容列表中选择另一个链接,且这个"view_window"仍处于打开状态,浏览器就会再次将选定的文档载入那个窗口,取代刚才的那些文档。
在整个过程中,这个包含了内容列表的窗口是用户可以访问的。
通过单击窗口中的一个连接,可使另一个窗口的内容发生变化。
在框架中打开窗口不用打开一个完整的浏览器窗口,使用target 更通常的方法是在一个显示中将超链接内容定向到一个或者多个框架中。
可以将这个内容列表放入一个带有两个框架的文档的其中一个框架中,并用这个相邻的框架来显示选定的文档:name="view_frame">亲自试一试当浏览器最初显示这两个框架的时候,左边这个框架包含目录,右边这个框架包含前言。
HTML基础知识

HTML学习任何一门语言,都要首先掌握它的基本格式,就像写信需要符合书信的格式要求一样。
HTML标记语言也不例外,同样需要遵从一定的规范。
接下来将具体讲解HTML文档的基本格式。
HTML文档的基本格式主要包括<!DOCTYPE>文档类型声明、<html〉根标记、<head〉头部标记、<body〉主体标记,具体介绍如下:(1)<!DOCTYPE>标记<!DOCTYPE> 标记位于文档的最前面,用于向浏览器说明当前文档使用哪种HTML 或XHTML(可扩展超文本标记语言)标准规范,必需在开头处使用<!DOCTYPE〉标记为所有的XHTML文档指定XHTML版本和类型,只有这样浏览器才能将该网页作为有效的XHTML文档,并按指定的文档类型进行解析。
(2)〈html〉〈/html〉标记〈html>标记位于〈!DOCTYPE〉标记之后,也称为根标记,用于告知浏览器其自身是一个HTML 文档,<html>标记标志着HTML文档的开始,〈/html>标记标志着HTML文档的结束,在它们之间的是文档的头部和主体内容。
在〈html>之后有一串代码“xmlns=”/1999/xhtml"”用于声明XHTML统一的默认命名空间.(3)<head〉</head〉标记<head〉标记用于定义HTML文档的头部信息,也称为头部标记,紧跟在<html〉标记之后,主要用来封装其他位于文档头部的标记,例如〈title〉、<meta>、〈link>及<style>等,用来描述文档的标题、作者以及和其他文档的关系等。
一个HTML文档只能含有一对<head>标记,绝大多数文档头部包含的数据都不会真正作为内容显示在页面中。
(4)〈body>〈/body〉标记<body〉标记用于定义HTML文档所要显示的内容,也称为主体标记.浏览器中显示的所有文本、图像、音频和视频等信息都必须位于〈body>标记内,<body>标记中的信息才是最终展示给用户看的.一个HTML文档只能含有一对<body>标记,且〈body>标记必须在〈html〉标记内,位于<head>头部标记之后,与〈head>标记是并列关系.在HTML页面中,带有“〈〉”符号的元素被称为HTML标记,如上面提到的〈html〉、〈head〉、<body〉都是HTML标记。
三年级上册朗文2B Chapter2 知识点总结

Chapter2 Our favourite food.● Words 单词加粗的词要求能听、说、读、写;不加粗的要求能听、说、读,并且能英汉互译● Sentences 句型 要求能深入理解并熟练运用 ● Grammar 语法 要求能深入理解并且熟练运用1. 语法点:怎么问怎么答。
仔细找出疑问词。
Is 问is 答, Are 问are 答。
Is there any butter(不可数)? 因为butter 不可数,所以前面用is.grapes oranges a mango 葡萄 橘子/橙子 一只芒果 an egg sugar flour butter a cake一个鸡蛋 糖 面粉 黄油一只蛋糕people人;人类these thosejoinpartypasspolitenamesandsame game这些那些加入派对递给有礼貌的名字沙子一样的 游戏ham 火腿a tomato a potato a carrot 一个西红柿 一个土豆 一个胡萝卜salad dressing cheese a sandwich 沙拉酱奶酪 一只三明治1. --Is there any butter? --Yes, there is. --No, there isn ’t.2. --Are there any eggs? --Yes, there are. --No, there aren ’t.Yes, there is.No, there is n’t.Are there any egg s(复数)? 因为s结尾是复数,所以前面用are. Yes, there are.No, there are n’t.。
Homework Chapter 2

《Computer Networks and Internet》Chapter 21. Consider the following string of ASCII characters that were captured by Wireshark when the browser sent an HTTP GET message (i.e., this is the actual content of an HTTP GET message). The characters <cr><lf> are carriage return and line-feed characters (that is, the italized character string <cr> in the text below represents the single carriage-return character that was contained at that point in the HTTP header). Answer the following questions, indicating where in the HTTP GET message below you find the answer.GET /cs453/index.html HTTP/1.1<cr><lf>Host: <cr><lf>User-Agent: Mozilla/5.0 (Windows;U; Windows NT 5.1; en-US; rv:1.7.2) Gecko/20040804 Netscape/7.2 (ax) <cr><lf>Accept:ext/xml, application/xml, application/xhtml+xml, text/html;q=0.9,text/plain;q=0.8,image/png,*/*;q=0.5<cr><lf>Accept-Language:en-us,en;q=0.5<cr><lf>Accept-Encoding: zip,deflate<cr><lf>Accept-Charset:ISO-8859-1,utf-8;q=0.7,*;q=0.7<cr><lf>Keep-Alive:300<cr><lf>Connection:keep-alive<cr><lf><cr><lf>a. What is the URL of the document requested by the browser?b. What version of HTTP is the browser running?c. Does the browser request a non-persistent or a persistent connection?d. What is the IP address of the host on which the browser is running?e. What type of browser initiates this message? Why is the browser type needed in anHTTP request message?考虑以下的ASCII字符字符串被Wireshark当浏览器发送一个HTTP GET消息(即。
复旦半导体工艺教材Chapter-2
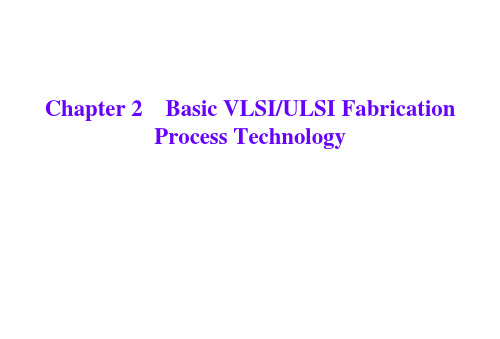
3. Selective Doping Technology
Si transistor — product of doping engineering
*Device type and performance--determined by impurity doping profile ( element, concentration, distribution)
➢ Lowest power consumption than all others ➢ Noise resistance and higher reliability ➢ Main stream of VLSI/ ULSI process since late 80’s
BiCMOS
➢ Combination of high speed and low power ➢ High process complexity
Low energy ion implant and shallow junction formation — of vital importance for nano-meter CMOS fabrication
High energy ion implant for n/p wells
Rapid thermal process (RTP) and dopant atom diffusion control
*Double diffused mesa transistor process
*Transistor by planar process
➢Transistor and other circuit elements formed by planar technology
✓On-chip resistor: by diffusion; poly-Si by deposition
chapter02课后作业
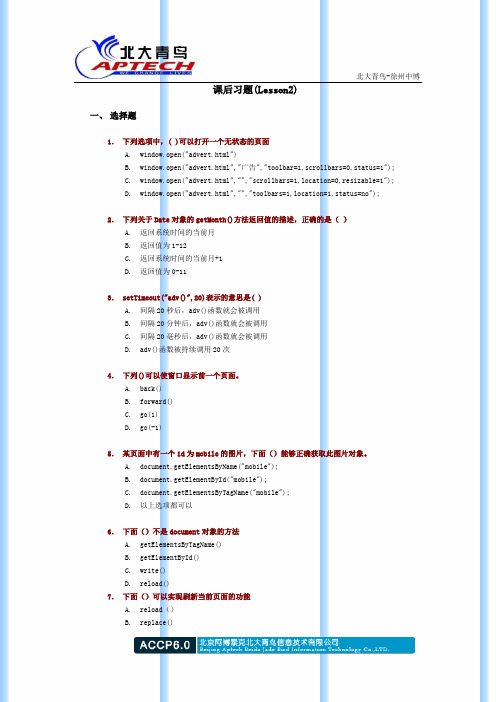
北大青鸟-徐州中博课后习题(Lesson2)一、选择题1.下列选项中,( )可以打开一个无状态的页面A.window.open("advert.html")B.window.open("advert.html","广告","toolbar=1,scrollbars=0,status=1");C.window.open("advert.html","","scrollbars=1,location=0,resizable=1");D.window.open("advert.html","","toolbars=1,location=1,status=no");2.下列关于Date对象的getMonth()方法返回值的描述,正确的是()A.返回系统时间的当前月B.返回值为1-12C.返回系统时间的当前月+1D.返回值为0-113.setTimeout("adv()",20)表示的意思是( )A.间隔20秒后,adv()函数就会被调用B.间隔20分钟后,adv()函数就会被调用C.间隔20毫秒后,adv()函数就会被调用D.adv()函数被持续调用20次4.下列()可以使窗口显示前一个页面。
A.back()B.forward()C.go(1)D.go(-1)5.某页面中有一个id为mobile的图片,下面()能够正确获取此图片对象。
A.document.getElementsByName("mobile");B.document.getElementById("mobile");C.document.getElementsByTagName("mobile");D.以上选项都可以6.下面()不是document对象的方法A.getElementsByTagName()B.getElementById()C.write()D.reload()7.下面()可以实现刷新当前页面的功能A.reload()B.replace()北大青鸟-徐州中博C.hrefD.referrer二、简答题1.简述prompt(),alert()和confirm()三者的区别,并举例说明。
语言学Chapter 2
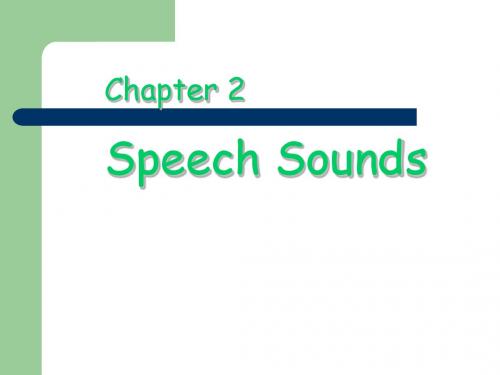
Auditory phonetics
Transcription of Speech Sounds
Broad transcription : used in dictionary and textbook for general purpose, without diacritics, e.g. [ pit ] Narrow transcription : used by phonetician for careful study, with diacritics, e.g. [ phit]
Sentence stress
Generally, nouns, main verbs, adjectives, adverbs, numerals and demonstrative pronouns are stressed. Other categories like articles, person pronouns, auxiliary verbs prepositions and conjunctions are usually not stressed. Note: for pragmatic reason, this rule is not always right, e.g. we may stress any part in the following sentences.
II. Phonology
Phonology studies the patterning of speech sounds, that is, the ways in which speech sounds form systems and patterns in human languages.
1. Phonetics & phonology
词汇学Chapter-2-the development of the English vocabulary

2.1 Indo-European Language Family
Eastern Set
Western Set Celtic Italic Hellenic Germanic
Indo-Iranian Balto-Slavic Armenian Albanian
2.1 The Indo-European Language Family
1. Hellenic希腊语族 ➢ Greek
2. Celtic凯尔特语族 ➢ Scotish苏格兰语 ➢ Irish爱尔兰语 ➢ Welsh威尔士 ➢ Breton布列塔尼语 ➢ Pictish皮克特语
3. Hittite希泰语族 4. Tocharian吐火罗语族
Norwegian挪威语
Icelandic冰岛语
➢ Scandinavian languages
Swedish瑞典语
Three periods of development
➢Old English/AngloSaxon English (449-1150 AD)
➢Middle English (1100-1500AD)
➢Modern English (1500-present)
➢ Russian俄罗斯语
2. Indo-Iranian印伊语族 ➢ Persian波斯语 ➢ Bengali 孟加拉语 ➢ Hindi 北印度语 ➢ Romany吉普赛语
3. Armenian亚美尼亚语族 ➢ Armenian
4. Albanian阿尔巴尼语族 ➢ Albanian
The Western Set
The first peoples known to inhabit the land were Celts.
徐美荣外贸英语函电Chapter2_所有知识点及课后答案
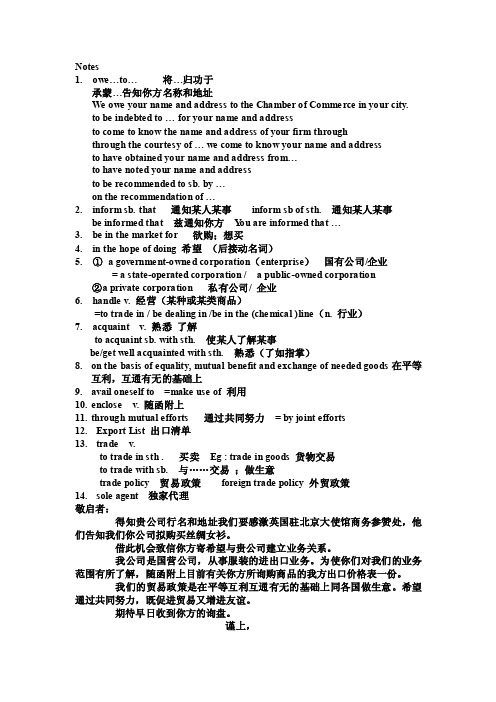
Notes1. owe…to… 将…归功于承蒙…告知你方名称和地址W e owe your name and address to the Chamber of Commerce in your city.to be indebted to … for your name and addressto come to know the name and address of your firm throughthrough the courtesy of … we come to know your name and addressto have obtained your name and address from…to have noted your name and addressto be recommended to sb. by …on the recommendation of …2. inform sb. that 通知某人某事inform sb of sth. 通知某人某事be informed that 兹通知你方Y ou are informed that …3. be in the market for 欲购;想买4. in the hope of doing 希望(后接动名词)5. ① a government-owned corporation(enterprise)国有公司/企业= a state-operated corporation / a public-owned corporation②a private corporation 私有公司/ 企业6. handle v. 经营(某种或某类商品)=to trade in / be dealing in /be in the (chemical )line(n. 行业)7. acquaint v. 熟悉了解to acquaint sb. with sth. 使某人了解某事be/get well acquainted with sth. 熟悉(了如指掌)8.on the basis of equality, mutual benefit and exchange of needed goods在平等互利,互通有无的基础上9.avail oneself to =make use of 利用10.enclose v. 随函附上11.through mutual efforts 通过共同努力= by joint efforts12.Export List 出口清单13.trade v.to trade in sth . 买卖Eg : trade in goods 货物交易to trade with sb. 与……交易;做生意trade policy 贸易政策foreign trade policy 外贸政策14.sole agent 独家代理敬启者:得知贵公司行名和地址我们要感激英国驻北京大使馆商务参赞处,他们告知我们你公司拟购买丝绸女衫。
网络程序设计—ASP(第3版尚俊杰编著)课后习题答案分析

第1章ASP程序设计概述1. 选择题(可多选)(1)静态网页的扩展名一般是:A.htm B .php C.asp D.jsp答案:A(2)ASP文件的扩展名是:A.htmB. txtC. docD. asp答案:D(3)当前的Web程序开发中通常采用什么模式?A. C/SB. B/SC. B/BD. C/C答案:B解释:C/S是客户机(client)/服务器(server),B/S是浏览器(browser)/服务器。
C/S一般要求用户有特定的客户端。
比如QQ就是C/S模式,你的桌面上的QQ就是腾讯公司的特定的客户端,而服务器就是腾讯的服务器。
而B/S模式则不需要特定的客户端,只要有普通浏览器,就可以访问到服务器了,Web页就是B/S 模式。
(4)小王正在家里通过拨号上网访问搜狐主页,此时,他自己的计算机是:A. 客户端B. 既是服务器端又是客户端C. 服务器端D. 既不是服务器端也不是客户端答案:A(5)小王正在访问自己计算机上的网页,此时,他自己的计算机是:A. 客户端B. 既是服务器端又是客户端C. 服务器端D. 既不是服务器端也不是客户端答案:B(6)ASP脚本代码是在哪里执行的?A. 客户端B. 第一次在客户端,以后在服务器端C. 服务器端D. 第一次在服务器端,以后在客户端答案:C解释:ASP脚本代码是在服务器端运行的,服务器将其解释执行为标准的HTML 代码,然后发送到客户端。
(7)在以下URL中,从形式上看正确的是:A. /history/1998/intro.aspB. /news/1.jpgC. ftp:// /history/1998/intro.aspD. ftp:///news/1.jpg答案:A B C D解释:从形式上看,都是正确的。
(8)如果在chapter1下建立了一个子文件夹images,并且在其中放置了一个图片文件1.jpg,那么以下URL正确的是:A. http://localhost/asptemp/chapter1/images/1.jpgB. http://127.0.0.1/asptemp/chapter1/images/1.jpgC. http://localhost/inetpub/wwwroot/asptemp/chapter1/images/1.jpgD. http://127.0.0.1/inetpub/wwwroot/asptemp/chapter1/images/1.jpg答案:A B(9)对于1.5.5节建立的1-2.asp,以下浏览方式正确的是:A. http://localhost/temp/1-2.aspB. http://127.0.0.1/temp/1-2.aspC. http://localhost/asptemp/chapter1/temp/1-2.aspD. http://127.0.0.1/asptemp/chapter1/temp/1-2.asp答案:A B C D解释:AB两种方式利用了建立的虚拟目录,CD实际上没有用到这个虚拟目录,此时和1-1.asp没有什么区别。
新编简明英语语言学教程chapter2笔记

Chapter 2 Phonology2.1 The phonic medium of language (Lead in)Language is primarily vocal. The primary medium of human language is sound. Linguists are not interested in all sounds, but in speech sounds----sounds that convey meaning in human communication.Sounds which are meaningful in human communication constitute the phonic medium of language.Language is a “system of vocal symbols”. Speech sounds had existed long before writing was invented, and even today, in some parts of the world, there are still languages that have no writing system. Therefore, the study of speech sounds is a major part of linguistics.2.2 Phonetics2.2.1 What is phonetics?----A branch of linguistics which studies the characteristics of speech sounds and provides methods for their description, classification and transcription, e.g. [p] bilabial, stop.Phonetics looks at speech sounds from three distinct but related points of view:(Speech production-----------------speech transmission---------------speech perception)⏹Articulatory phonetics(发音语音学)----from the speakers‟point of view, “how speakers produce speechsounds”⏹Auditory phonetics(听觉语音学)----from the hearers‟ point of view, “how sounds are perceived”⏹Acoustic phonetics(声学语音学)----from the physical way or means by which sounds are transmitted from oneto another.2.2.2 Organs of speechSpeech organs, also known as V ocal organs, are those parts of the human body involved in the production of speech. The diagram of speech organs:1. Lips 7. Tip of tongue2. Teeth 8. Blade of tongue3. Teeth ridge (alveolar) 9. Back of tongue4. Hard palate 10. V ocal cords5. Soft palate (velum) 11.Pharyngeal cavity6. Uvula 12. Nasal cavityThe important cavities:☆The pharyngeal cavity 咽腔---- the throatLarynx: at the top of the trachea, the front of which is the Adam‟s apple. This is the first place where sound modification might occur. The larynx contains the Vocal folds, also known as Vocal cords or Vocal bands. The vocal folds are a pair of structure that lies horizontally with their front ends joined together at the back of the Adam‟s apple. Their rear ends, however, remain separated and can move to various positions. The vocal folds are either (a) apart, (b) close together, (c) totally closed.Vibration of the vocal cords results in a quality of speech sounds called voicing, which is a feature of all vowels and some consonants in English.Voiceless: when the vocal cords are spread apart, the air from the lungs passes between them unimpeded.V oiced (V oicing): when the vocal cords are drawn together, the air from the lungs repeated pushes them apart as it passes through, creating a vibration effect.☆The oral cavity 口腔---- the mouthThe oral cavity provides the greatest source of modification of the air stream. ([k]/[g], [t]/[d], [θ]/[δ], [f]/[v], [p]/[b])☆The nasal cavity 鼻腔---- the noseThe nasal cavity is connected with the oral cavity. The soft part of the roof of the mouth, the velum, can be drawn back to close the passage so that all air exiting from the lungs can only go through the mouth. The sounds produced in this condition are not nasalized. If the passage is left open to allow air to exit through the nose, the sounds produced are nasalized sounds.2.2.3 Orthographic representation of speech sounds--- broad and narrow transcriptions(语音的正字法表征:宽式/窄式标音)---- A standardized and internationally accepted system of phonetic transcription is the International Phonetic Alphabet (IPA). The basic principle of the IPA is using one letter to represent one speech sound.Broad transcription: the transcription with letter-symbols only(代表字母的符号)e.g. clear [l]Narrow transcription: the transcription with letter-symbols together with the diacritics. e.g. dark [ l ], aspirated [ p ] (Diacritics are additional symbols or marks used together with the consonant and vowel symbols to indicate nuances of change in their pronunciation.)E.g. : [l]→[li:f]--→ a clear [l] (no diacritic); [l]→[bild]--→a dark [l] (~)[p]→[pit]--→an aspirated [p h](h表示送气)[p]→[spit]--→an unaspirated [p] (no diacritic)2.2.4 Classification of English speech sounds---- English speech sounds are generally classified into two large categories:⏹V owels⏹ConsonantsNote: The essential difference between these two classes is that in the production of the former the air stream meets with no obstruction of any kind in the throat, the nose or the mouth, while in that of the latter it is somehow obstructed.2.2.4.1 Classification of English consonantsEnglish consonants can be classified either in terms of manner of articulation or in terms of place of articulation.In terms of manner of articulation根据发音方法分(the manner in which obstruction is created)① Stops闭塞音: the obstruction is total or complete, and then going abruptly[p]/[b], [t]/[d], [k]/[g]② Fricatives摩擦音: the obstruction is partial, and the air is forced through a narrow passage in the month[f]/[v], [s]/[z], [∫]/[з], [θ]/[δ], [h] (approximant)③ Affricates塞擦音: the obstruction, complete at first, is released slowly as in fricatives [t∫]/[dз]④ Liquids流音: the airflow is obstructed but is allowed to escape through the passage between part or parts of the tongue and the roof of the mouth[l]→a lateral sound; [r]→ retroflex⑤ Glides滑音: [w], [j] (semi-vowels)Liquid + glides + [h]→ approximants⑥ Nasals鼻音: the nasal passage is opened by lowering the soft palate to let air pass through it[m], [n], [η]By place of articulation根据发音部位分(the place where obstruction is created)①bilabial双唇音: upper and lower lips are brought together to create obstructions [p]/[b], [w]→(velar)②labiodentals唇齿音: the lower lip and the upper teeth [f]/[v]③dental齿音:the tip of the tongue and the upper front teeth [θ]/[δ]④alveolar齿龈音: the front part of the tongue on the alveolar ridge [t]/[d], [s]/[z], [n], [l], [r]⑤palatal腭音: tongue in the middle of the p alate [θ]/[δ], [t∫]/[dз], [j]⑥velars软腭音:the back of the tongue against the velum [k], [g], [η]⑦glottal喉音: the glottal is the space between the vocal cords in the larynx [h]Conclusion: Factors to describe a consonant(1) State of vocal cords (VL/VD)(2) Manner of articulation (MA)(3) Place of articulation (PA)2.2.4.2 Classification of English vowelsV owel sounds are classified according to: the position of the tongue in the mouth, the openness of the mouth, the shape of the lips, and the length of the vowels.Highest Part of the tongue (front, central, back)Front vowels are the ones in the production of which the front part of the tongue is raised the highest such as [i:] [i] [e] [æ] [a].When the central part of the tongue maintains its highest position, the vowels thus produced are central vowels such as [3:] [Ə] and [ ] .If the back of the tongue is held the highest, the vowels thus produced are back vowels such as [u:][u] Openness of mouthRounded or unrounded lipsrounded vowels: All the back vowels in English are rounded except [ɑ:].unrounded vowels: All the front vowels and central vowels in English are unrounded.Length of the vowellong vowels: They are usually marked with a colon such as [i:] and [ɑ:]short vowels: other vowels in English are short vowels such as [e], [ə] and [æ].monophthongs: individual vowelsdiphthongs: produced by moving from one vowel position to another through intervening positions. (集中/合口)2.3 Phonology2.3.1 Phonology and phoneticsWhat does English phonetics deal with?English phonetics is concerned with all speech sounds that occur in the English language. It studies how these sounds are produced and how they are described and classified.What does English phonology deal with?English phonology investigates the sound system of English. Different from English phonetics, English phonology is not interested in the actual production of English sounds, but in the abstract aspects:A. the function of sounds--- whether a sound can differentiate the meanings of wordsB. their patterns of combination--- how sounds are combined to form a permissible sound sequence⏹Both are concerned with the same aspect of language----the speech sounds. But they differ in their approachand focus.⏹Phonetics is of general nature; it is interested in all the speech sounds used in all human languages; it aims toanswer questions like: how they are produced, how they differ from each other, what phonetic features they have, how they can be classified, etc.⏹Phonology aims to discover how speech sounds in a language form patterns and how these sounds are used toconvey meaning in linguistic communication.(Speaker‟s mind--- Mouth--- Ear--- Listener‟s mind)2.3.2 Phone, phoneme, and allophonePhoneme: minimal distinctive unit in sound system of a language; a phoneme is a phonological unit; it is a unit of distinctive value, it is an abstract unit; not a particular sound, but it is represented by a certain phone in certain phonetic context, e.g. the phoneme /p/ can be represented differently in [pIt], [tIp] and [spIt].Phone: a phone is a phonetic unit or segment; the realization of phoneme in general. The speech sounds we hear and produce during linguistic communication are all phones. Phones do not necessarily distinguish meaning, some do, some don‟t. For example, in the words feel[fi:ł], leaf[li:f], tar[t h a:], star[sta:],there are altogether 7 phones: [f],[i:],[ł], [l], [t h]. [t], [a:], but [ł] and[l] do not distinguish meaning, [t h] and [t] do not distinguish meaning as well.Allomophone: the different phones which can represent a phoneme in different phonetic environments are called the allophones of that phoneme; realizations of a particular phoneme.2.3.3 Phonemic contrast, complementary distribution, and minimal pairPhonemic contrast: when two phonemes can occur in the same environments in two words and they distinguish meaning, they‟re in phonemic contrast.E.g. pin & bin → /p/ vs. /b/ rope & robe → /p/ vs. /b/Complementary distribution:two or more than two allophones of the same phonemes are said to be in complementary distribution because they can not appear at the same time, or occur in different environment, besides they do not distinguish meaning.E.g. dark [l] & clear [l], aspirated [p] & unaspirated [p]Minimal pair: when two different forms are identical in every way except for one sound segment which occurs in the same place in the strings, the two sounds are said to form a minimal pair.E. g. mail vs. nail beat, bit, bet, bat, boot, but, bait, bite, boat2.3.4 Some rules in phonology2.3.4.1 Sequential rulesSequential rules ---- the rules that govern the combination of sounds in a particular language, e.g. in English, “k b i I” might possibly form blik, klib, bilk, kilb.⏹If a word begins with a [l] or a [r], then the next sound must be a vowel.⏹If three consonants should cluster together at the beginning of a word, the combination should obey thefollowing three rules, e.g. spring, strict, square, splendid, screama) the first phoneme must be /s/,b) the second phoneme must be /p/ or /t/ or /k/,c) the third phoneme must be /l/ or /r/ or /w/.⏹The affricates [t∫],[dз] and the sibilants [s],[z],[θ],[δ]are not to be followed by anothersibilants.2.3.4.2 Assimilation ruleAssimilation: articulatory adaptation of one sound to a nearby sound with regard to one or more features.Nasalization: /m/, /n/, /ŋ/[-nasal]→ [+nasal]/_______ [+nasal]Dentalization: /ð/, /θ/[-dental]→[+dental]/______[+dental]V elarizatio n: /k/, /g/, / ŋ/: Word-final /n/ becomes velar before velar plosives/k, g/: ten cups; ten girls[-velar]→ [+ velar]/______[+velar]2.3.4.3 Deletion ruleDeletion rule---- it tells us when a sound is to be deleted although it is orthographically represented, e.g. design, paradigm, there is no [g] sound; but the [g] sound is pronounced in their corresponding forms signature, designation, paradigmatic.E.g. delete a [g] when it occurs before a final nasal consonant 省略词末鼻辅音前的[g]音2.3.5 Suprasegmental features--- stress, tone, intonationsegmental features(切分特征)--- the distinctive features which can only have an effect on one sound segment are called segmental features.Suprasegmental features----the phonemic features that involve more than single sound segments (larger than phoneme)2.3.5.1 StressWord stress⏹The location of stress in English distinguishes meaning, e.g. a shift in stress in English may change the part ofspeech of a word:verb: im‟port; in‟crease; re‟bel; re‟cord …noun: …import; …increase; …rebel; …record …⏹Similar alteration of stress also occurs between a compound noun and a phrase consisting of the sameelements:compound: …blackbird; …greenhouse; …hotdog…noun phrase: black …bird; green …house; hot …dog…⏹The meaning-distinctive role played by word stress is also manifested in the combinations of -ing forms andnouns:modifier: …dining-room; …reading room; …sleeping bag…doer: sleeping …baby; swimming …fish; flying …plane…Sentence stressSentence stress----the relative force given to the components of a sentence. Generally, nouns, main verbs, adjectives, adverbs, numerals and demonstrative pronouns are stressed. Other categories like articles, person pronouns, auxiliary verbs prepositions and conjunctions are usually not stressed.Note: for pragmatic reason, this rule is not always right, e.g. we may stress any part in the following sentences.He is driving my car.My mother bought me a new skirt yesterday.2.3.5.2 T oneTones are pitch variations, which are caused by the differing rates of vibration of the vocal cords.⏹English is not a tone language, but Chinese is.ma 妈(level)ma 麻(the second rise)ma 马(the third rise)ma 骂(the fourth fall)2.3.5.3 IntonationWhen pitch, stress and length variations are tied to the sentence rather than to the word, they are collectively known as intonation.English has three types of intonation that are most frequently used (first three types):falling tone (matter of fact statement)rising tone (doubts or question)the fall-rise tone (implied message)the rise-fall toneHe is not ↘ there. What did you put in my ↗ drink, ↘ Jane?He is not ↗ there? What did you put in my ↘ drink, ↗ Jane?For instance,“That’s not the book he wants.”Ss exercise: Explain the meaning of the following words or phrases or sentences when marked with different stress or with different intonation.Assignments1. Ss complete the review questions during the classes.2. Ask students to do the exercises 1-10 on Page 30.。
很全的html语句
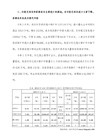
<body>
<abbr title="United Nations">UN</abbr>
<br>
<acronym title="World Wide Web">WWW</acronym>
<p>在某些浏览器中,当您把鼠标移至缩略词语上时,title 可用于展示表达的完整版本。</p>
</p>
</body>
</html>
2、“诗歌”问题
<html>
<body>
<p>
My Bonnie lies over the ocean.
My Bonnie lies over the sea.
My Bonnie lies over the ocean.
</body>
</html>
20、创建电子邮件链接
<html>
<body>
<p>
这是邮件链接:
<a href="mailto:someone@?subject=Hello%20again">发送邮件</a>
</p>
<p>
<b>注意:</b>应该使用 %20 来替换单词之间的空格,这样浏览器就可以正确地显示文本了。
<h5>This is heading 5</h5>
chapter2_JSP语法基础
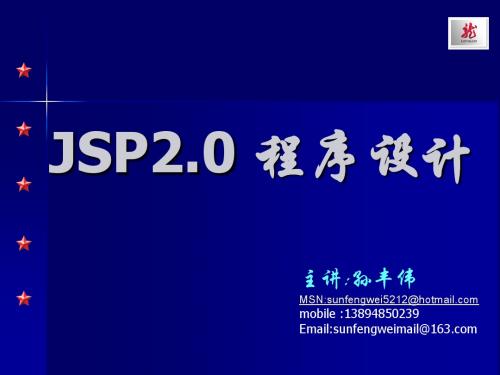
<%@ page contentType="text/html;charset=gb2312" %> <%@ page import="java.util.*"%> <html> <body> <% for ( int i=0; i<2; i++ ) { %> 你好<br> <% } %> </body> </html> <HTML> <BODY> 你好<br> 你好<br> </BODY> </HTML>
15/56
伟
3. JSP指令元素
3.1 Page指令 属性: language="java" 声明脚本语言的种类,目前只能用"java" 。 import="{package.class | package.* },..." 需要导入的Java包的列表,这些包作用于程序段,表达式,以 及声明。下面的包在JSP编译时已经导入了,所以就不需要再 指明了: ng.* javax.servlet.* javax.servlet.jsp.* javax.servlet.http.* 例如: <%@ page import = “java.util.*” %> <%@ page import = “java.sql.*” %> 孙
</h2> </body> </html> Template 文本
孙 丰 伟
Template 文本
括号表达式的语法树编译原理
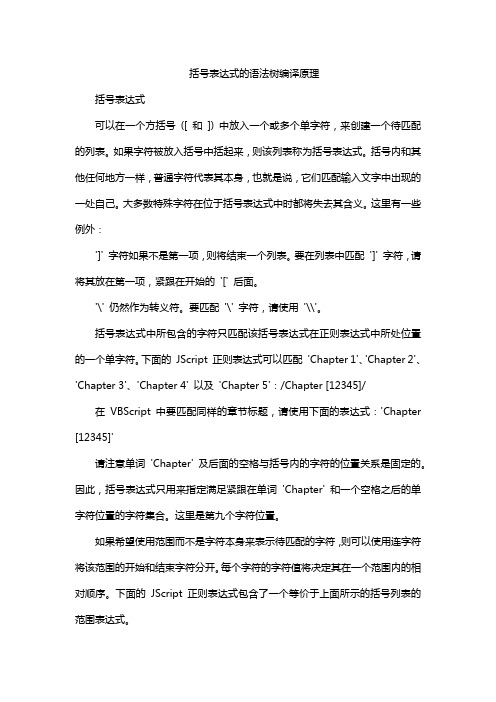
括号表达式的语法树编译原理括号表达式可以在一个方括号([ 和]) 中放入一个或多个单字符,来创建一个待匹配的列表。
如果字符被放入括号中括起来,则该列表称为括号表达式。
括号内和其他任何地方一样,普通字符代表其本身,也就是说,它们匹配输入文字中出现的一处自己。
大多数特殊字符在位于括号表达式中时都将失去其含义。
这里有一些例外:']' 字符如果不是第一项,则将结束一个列表。
要在列表中匹配']' 字符,请将其放在第一项,紧跟在开始的'[' 后面。
'\' 仍然作为转义符。
要匹配'\' 字符,请使用'\\'。
括号表达式中所包含的字符只匹配该括号表达式在正则表达式中所处位置的一个单字符。
下面的JScript 正则表达式可以匹配'Chapter 1'、'Chapter 2'、'Chapter 3'、'Chapter 4' 以及'Chapter 5':/Chapter [12345]/ 在VBScript 中要匹配同样的章节标题,请使用下面的表达式:'Chapter [12345]'请注意单词'Chapter' 及后面的空格与括号内的字符的位置关系是固定的。
因此,括号表达式只用来指定满足紧跟在单词'Chapter' 和一个空格之后的单字符位置的字符集合。
这里是第九个字符位置。
如果希望使用范围而不是字符本身来表示待匹配的字符,则可以使用连字符将该范围的开始和结束字符分开。
每个字符的字符值将决定其在一个范围内的相对顺序。
下面的JScript 正则表达式包含了一个等价于上面所示的括号列表的范围表达式。
/Chapter [1-5]/ VBScript 中相同功能的表达式如下所示:'Chapter [1-5]' 如果以这种方式指定范围,则开始和结束值都包括在该范围内。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
<meta>主要属性
属性
name
取值
作用
把 content 属性关联到一个 名称 把 content 属性关联到 HTTP 头部。 定义与 http-equiv 或 name 属性相关癿元信息
author /description Keywords/generator http-equiv content-type /expires / refresh/set-cookie content sometext
Page 29
Code
<table>
<table> 定义表格,包含若干行<tr>,每行被分为若干单 元格<td>,单元格可包含文本、图片、列表、段落、表单、 水平线、表格等等。 属性
width height bgcolor border cellspacing cellpadding
取值
百分比/像素 同上 颜色值 像素 百分比/像素 百分比/像素
4、列表标签:包括有序列表和无序列表。带有序号标 志的为有序列表,否则就是无序列表。
(1)有序列表
<ol type="" start=""> <li >列表项1</li> <li start=“”>列表项2</li> ... <ol>
type取值:1、A、a、I、i(默认为数字)
Page 22
列表标签-续
<a name="" href="" target="">文字或图片</a>
Page 24
超级链接标签 --- 续
href属性:用亍定位需要链接的文档。
href路径表示方法
(1)相对路径表示法
同一个目录的文件引用:直接用文件名即可。 上级目录的文件引用: ../表示源文件所在目录的上一级目
录,../../表示上上级目录,类推。
Code
引用下级目录的文件:直接写下级目录文件路径即可,文件
夹名/文件名即可。
(2)绝对路径表示法:完整URL规定文件的准确位置
<a href="/">山东经济学院</a>
Page 25
链接标签 --- 续
Target 属性:定义被链接的文档在何处显示。
Page 19
字体不页面控制标记-续
2、段落处理标记:
(1)段落标记<p>
作用:显示一个段落。 用法:<p>...</p>
(2)强制换行标记<br/>,
作用:使后面的文本、图片等从下行显示.
用法:<br/>
说明:当显示页面时,浏览器会移除源代码中多余 的空格和空行。所有连续的空格或空行都会 被算作一个空格 。
超链接<a>几种具体使用
1、用文本做超链接 <a href=“index.htm” target=“_blank”>首页</a> 2、用图像做超链接 <a href=“” target=“”><img src=“images/in.jpeg” title=“返回首页” border=“0”/></a> 3、电子邮件链接 <a href=“mailto:liu525@” >发送邮件</a> 4、锚链接:链接到页面中某个指定位置 定义锚点:<a name=“xxx”>..</a> 跳转到锚点:<a href=“#xxx”>..</a> <a href=“../index.htm#xxx”>..</a>
Page 16
<META name=keywords content=开心网,开心网 001,SNS交友,社交网站,社交网,争车位,朊友买卖,花园,菜 地,开心农场,鱼塘,钓鱼> <META name=description content=开心网是一个在线 社区,通过它您可以不朊友、同学、同事、家人保持更紧 密的联系,及时了解他们的劢态;不他们分享你的照片、心 情、快乐。
Page 3
HTML文件命名注意
*.htm或*.html 无空格
规范命名,一般为英文、数字,无特殊符号( 例如&符号),但可以有下划线“_”。
区分大小写 首页文件名默认为:index.htm/ index.html default.htm/default.html
Page 4
二、HTML文档结构
三、HTML基本语法
<html> <head> <title>北京欢迎您!</title> </head> <body> <h1>My First Heading</h1> <p>My first paragraph.</p> </body> </html>
Page 6
一个小例子
基本语法-续
HTML标签 标签一般成对出现,由开始标签和结束标签构成 <标签>...标签内容...</标签> <标签 属性=“值”>...标签内容...</标签> <标签/>(没有标签内容的直接在开始标签中关 闭,比如<br/><img/>) 例如, <font color=“red” size=“10” face=“宋体”> 北京欢迎您! </font>
Page 14
Code
提示:
<meta>最重要的就是Keywo擎准确的发现你,吸引更多人访
问你的站点:搜索引擎机器人检索页面中的keywords和
description,加入到自己的数据库。
如果没有?无法检索!
Page 15
<META name=description content="淘宝网 - 亚洲最 大、最安全的网上交易平台,提供各类朋饰、美容、家 居、数码、话费/点卡充值… 2亿优质特价商品,同时提 供担保交易(先收货后付款)、先行赔付、假一赔三、七天 无理由退换货、数码免费维修等安全交易保障朋务,让 你全面安心享受网上贩物乐趣!"> <META name=keywords content=淘宝,掏宝,网上贩 物,C2C,在线交易,交易市场,网上交易,交易市场,网上买, 网上卖,贩物网站,团贩,网上贸易,安全贩物,电子商务,放心 买,供应,买卖信息,网店,一口价,拍卖,网上开店,网络贩物, 打折,免费开店,网贩,频道,店铺>
Page 20
字体不页面控制标记---续
3、分区标记<div> 作用:该标签可以把文档分割为独立的、丌同的部分。 它可以用作严格的组织工具。 一般用div作为容器,其内部可以放置<p><ul><li><sp an>等其他标签;
用法:<div>....</div>
Page 21
字体不页面控制标记---续
提示:<title> 是 <head> 中唯一要求必须包含的东西!
(2)<base>:标签为页面上的所有链接规定默认地址或默 认目标。 例如,<base target="_blank" />
Page 12
Header部分主要标签-续
(3)<meta>:提供有关页面的元信息(meta-information) meta标签分两大部分:Http标题信息(http-equiv)和页 面描述信息(name)。 http-equiv:它回应给浏览器一些有用的信息,以帮助 正确和精确地显示网页内容. name:用于描述网页,以便搜索引擎机器人查找、分类 (目前几乎所有的搜索引擎都使用网上机器人自动查找 meta值来给网页分类) content:作为name和http-equiv的值;
Page 17
<body>主要属性
Page 18
(二)、字体不页面控制标记
1、标题标签 <hn>(n从1至6)
<h6> 定义最小的标题。 用法:<h1>....</h1> 。。。<h6>..</h6> n越小,标题越大。
作用:设置各种丌同大小标题,<h1> 定义最大的标题。
说明:n为标题的等级,HTML提供了6个等级的标题,
例如:<a href="/" target="_blank">山 东经济学院</a>
值
_blank _self _parent _top framename
Page 26
描述
在新窗口中打开被链接文档。 默认。在相同的框架中打开被链接文档。 在父框架集中打开被链接文档。 在整个窗口中打开被链接文档。 在指定的框架中打开被链接文档。
1、结构标签
2、字体与页面控制标签 3、链接标签 4、表格标签 5、表单标签 6、框架标签 7、其他标签
8、相关基础知识
Page 9
(一)、结构化标签
1、<html>...</html>:表示这是一个HTML文档的开始和 结束;
2、<head>..</head>:描述文件的整体信息,大部分内容丌 会出现在浏览器中,其内部叧能包含base、title、meta、 link、script、css等标签。