操作系统文件管理实验代码
文件管理系统源代码
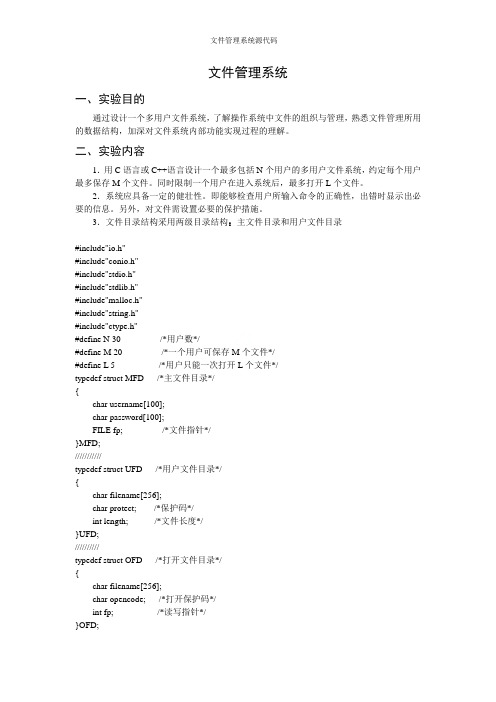
文件管理系统一、实验目的通过设计一个多用户文件系统,了解操作系统中文件的组织与管理,熟悉文件管理所用的数据结构,加深对文件系统内部功能实现过程的理解。
二、实验内容1.用C语言或C++语言设计一个最多包括N个用户的多用户文件系统,约定每个用户最多保存M个文件。
同时限制一个用户在进入系统后,最多打开L个文件。
2.系统应具备一定的健壮性。
即能够检查用户所输入命令的正确性,出错时显示出必要的信息。
另外,对文件需设置必要的保护措施。
3.文件目录结构采用两级目录结构:主文件目录和用户文件目录#include"io.h"#include"conio.h"#include"stdio.h"#include"stdlib.h"#include"malloc.h"#include"string.h"#include"ctype.h"#define N 30 /*用户数*/#define M 20 /*一个用户可保存M个文件*/#define L 5 /*用户只能一次打开L个文件*/typedef struct MFD /*主文件目录*/{char username[100];char password[100];FILE fp; /*文件指针*/}MFD;///////////typedef struct UFD /*用户文件目录*/{char filename[256];char protect; /*保护码*/int length; /*文件长度*/}UFD;//////////typedef struct OFD /*打开文件目录*/{char filename[256];char opencode; /*打开保护码*/int fp; /*读写指针*/}OFD;//////////typedef struct COMM /*命令串*/{char string[256]; /*命令*/struct COMM *next;/*后继指针*/}COMM;////////////MFD mainfd[N]; /*主文件目录数组*/UFD userfd[M]; /*用户文件目录数组*/OFD openfd[L]; /*打开文件目录数组*/////////COMM*command; /*命令串指针*/char username[10];int usernum,savenum,opennum;int workfile;void init();void init_ufd(char *username);/*初始化用户文件目录*/ void mesg(char *str); /*消息*/char *getpass(); /*设置口令函数声明*/ char *getuser(); /*设置用户函数声明*/ COMM *readcommand(); /*读命令串函数声明*/ void login(); /*用户登录*/void logout(); /*用户注销*/void setpass(); /*设置口令*/void create(); /*创建文件*/void mydelete(); /*删除文件*/void myread(); /*读文件*/void myopen(); /*打开文件*/void myclose(); /*关闭文件*/void mywrite(); /*写文件*/void help(); /*帮助*/void dir(); /*列文件目录*/void mycopy(); /*复制文件*/void myrename(); /*重命名文件名*//////////////void main(){init();for(;;){readcommand();if(strcmp(command->string,"create")==0)create();else if(strcmp(command->string,"delete")==0)mydelete();else if(strcmp(command->string,"open")==0)myopen();else if(strcmp(command->string,"close")==0)myclose();else if(strcmp(command->string,"read")==0)myread();else if(strcmp(command->string,"write")==0)mywrite();else if(strcmp(command->string,"copy")==0)mycopy();else if(strcmp(command->string,"rename")==0)myrename();else if(strcmp(command->string,"login")==0)login();else if(strcmp(command->string,"setpass")==0)setpass();else if(strcmp(command->string,"logout")==0)logout();else if(strcmp(command->string,"help")==0)help();else if(strcmp(command->string,"dir")==0)dir();else if(strcmp(command->string,"exit")==0)break;elsemesg("Bad command!");}}///////////////////void init(){FILE *fp;char tempname[20],temppass[20];int i=0;usernum=0;savenum=0;opennum=0;strcpy(username,"");if((fp=fopen("mainfile.txt","r"))!=NULL){while(!feof(fp)){strcpy(tempname,"");fgets(tempname,20,fp);if(strcmp(tempname,"")!=0){fgets(temppass,20,fp);tempname[strlen(tempname)-1]='\0';temppass[strlen(tempname)-1]='\0';strcpy(mainfd[usernum].username,tempname);strcpy(mainfd[usernum].password,tempname);usernum++;}}fclose(fp);}}///////////////////////void init_ufd(char *username)/*初始化用户文件目录*/{FILE *fp;char tempfile[100],tempprot;int templength;savenum=0;opennum=0;workfile=-1;if((fp=fopen(username,"w+"))!=NULL){while(!feof(fp)){strcpy(tempfile,"");fgets(tempfile,50,fp);if(strcmp(tempfile,"")!=0){fscanf(fp,"%c",&tempprot);fscanf(fp,"%d",&templength);tempfile[strlen(tempfile)-1]='\0';strcpy(userfd[savenum].filename,tempfile);userfd[savenum].protect=tempprot;userfd[savenum].length=templength;savenum++;fgets(tempfile,50,fp);}}}fclose(fp);}////////////////////void mesg(char *str){printf("\n %s\n",str);}////////////////////////////char *getuser(){char username[20];char temp;int i;username[0]='\0';for(i=0;i<10;){while(!kbhit());temp=getch();if(isalnum(temp)||temp=='_'||temp==13){username[i]=temp;if(username[i]==13){username[i]='\0';break;}putchar(temp);i++;username[i]='\0';}}return(username);}///////////////////char *getpass(){char password[20];char temp;int i;password[0]='\0';for(i=0;i<10;){while(!kbhit());temp=getch();if(isalnum(temp)||temp=='_'||temp==13){password[i]=temp;if(password[i]==13){password[i]='\0';break;}putchar('*');i++;password[i]='\0';}}return(password);}///////////////COMM *readcommand(){char temp[256];char line[256];int i,end;COMM *newp,*p;command=NULL;strcpy(line,"");while(strcmp(line,"")==0){printf("\nc:\\>");gets(line);}for(i=0;i<=strlen(line);i++){if(line[i]==' ')i++;end=0;while(line[i]!='\0'&&line[i]!=' '){temp[end]=line[i];end++;i++;}if(end>0){temp[end]='\0';newp=(COMM *)malloc(sizeof(COMM *));strcpy(newp->string,temp);newp->next=NULL;if(command==NULL)command=newp;else{p=command;while(p->next!=NULL)p=p->next;p->next=newp;}}}p=command;return(command);}/////////////////////////////void login() /*用户注册*/{FILE *fp;int i;char password[20],confirm[20],tempname[20];if(command->next==NULL){printf("\n User Name:");strcpy(tempname,getuser());}else if(command->next->next!=NULL){mesg("Too many parameters!");return;}elsestrcpy(tempname,command->next->string);for(i=0;i<usernum;i++)if(strcmp(mainfd[i].username,tempname)==0)break; /*从mainfd表中查找要注册的用户*/ if(i>=usernum) /*新用户*/{printf("\n new user account,enter your password twice!");printf("\n Password:");strcpy(password,getpass());/*输入口令且返回之*/printf("\n Password:");strcpy(confirm,getpass()); /*第二次输入口令*/if(strcmp(password,confirm)==0)/*用户数不能超过N*/{if(usernum>=N)mesg("Create new account false!number of user asscount limited.\n login fasle!");else{strcpy(mainfd[usernum].username,tempname);/*把新用户和口令填入mainfd中*/strcpy(mainfd[usernum].password,password);usernum++;strcpy(username,tempname);mesg("Create a new user!\n login success!");init_ufd(username); /*初始化用户文件目录*/fp=fopen("mainfile.txt","w+"); /*把新用户填入mainfile.txt文件中*/for(i=0;i<usernum;i++){fputs(mainfd[i].username,fp);fputs("\n",fp);fputs(mainfd[i].password,fp);fputs("\n",fp);}fclose(fp);}}else{mesg("Create new account false! Error password.");mesg("login false!");}}else{printf("\n Password:");strcpy(password,getpass());if(strcmp(mainfd[i].password,password)!=0)mesg("Login false! Error password.");else{mesg("Login success!");strcpy(username,tempname);init_ufd(username);}}}/////////////////////////void logout() /*用户注销*/{if(command->next!=NULL)mesg("Too many parameters!");else if(strcmp(username,"")==0)mesg("No user login!");else{strcpy(username,"");opennum=0;savenum=0;workfile=-1;mesg("User logout!");}}////////////////////void setpass() /*修改口令*/{int i=0;FILE *fp;char oldpass[20],newpass[20],confirm[20];if(strcmp(username,"")==0)mesg("No user login!");else{printf("\n Old password:");strcpy(oldpass,getpass());for(int i=0;i<usernum;i++){if(strcmp(mainfd[i].username,username)==0)break;}if(strcmp(mainfd[i].password,oldpass)!=0)mesg("Old password error!");else{printf("\n New password:");strcpy(newpass,getpass());printf("\n Confirm password:");strcpy(confirm,getpass());if(strcmp(newpass,confirm)!=0)mesg("Password not change! confirm different.");else{strcpy(mainfd[i].password,newpass);mesg(" Password change !");fp=fopen("mainfile.txt","w+");for(i=0;i<usernum;i++){fputs(mainfd[i].username,fp);fputs("\n",fp);fputs(mainfd[i].password,fp);fputs("\n",fp);}fclose(fp);}}}}////////////////void create(){int i=0;FILE *fp;if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next!=NULL)mesg("Too many parameters!");else{for(i=0;i<savenum;i++){if(strcmp(userfd[i].filename,command->next->string)==0)break;}if(i<savenum)mesg("Error! the file already existed!");else if(savenum>=M)mesg("Error! connot create file! number of files limited!");else{strcpy(userfd[savenum].filename,command->next->string);userfd[i].protect='r';userfd[i].length=rand();savenum++;mesg("Create file success!");fp=fopen(username,"w+");for(i=0;i<savenum;i++){fputs(userfd[i].filename,fp);fputs("\n",fp);fprintf(fp,"%c\n%d\n",userfd[i].protect,userfd[i].length);}fclose(fp);}}}/////////////////void mydelete() /*删除*/{int i=0;int tempsave=0;FILE *fp;if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next!=NULL)mesg("Too many parameters!");else{for(i=0;i<savenum;i++){if(strcmp(userfd[i].filename,command->next->string)==0)break;}if(i>=savenum)mesg("Error! the file not existed!");else{tempsave=i;for(i=0;i<opennum;i++){if(strcmp(command->next->string,openfd[i].filename)==0)break;}if(i>=opennum)mesg("File not open");////////////////////////////////////////////else{if(tempsave==savenum-1)savenum--;else{for(;tempsave<savenum-1;tempsave++){strcpy(userfd[tempsave].filename,userfd[tempsave+1].filename);userfd[tempsave].protect=userfd[tempsave+1].protect;userfd[tempsave].length=userfd[tempsave+1].length;}savenum--;}mesg("Delete file success!");fp=fopen(username,"w+");for(i=0;i<savenum;i++){fputs(userfd[i].filename,fp);fputs("\n",fp);fprintf(fp,"%c\n%d\n",userfd[i].protect,userfd[i].length);}fclose(fp);}}}}//////////////////void myread() /*读*/{int i=0;int tempsave=0;char tempfile[100];if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next!=NULL)mesg("Too many parameters!");elsestrcpy(tempfile,command->next->string);for(i=0;i<savenum;i++){if(strcmp(tempfile,userfd[i].filename)==0)break;}if(i>=savenum)mesg("File not existed!");else{tempsave=i;for(i=0;i<opennum;i++){if(strcmp(tempfile,openfd[i].filename)==0)break;}if(i>=opennum)mesg("File not opened");else{if(userfd[tempsave].length<1000)printf("\n The file size is %d KB",userfd[tempsave].length);else if(userfd[tempsave].length==1000)printf("\n The file size is 1000 KB");elseprintf("\n The file size is %d,%d KB",userfd[tempsave].length/1000,userfd[tempsave].length%1000);mesg("File read");}}}}/////////////////void myopen()/*打开*/{int i=0;int type=0;char tempcode;char tempfile[100];if(strcmp(username,"")==0)mesg("No user login!");else if(command->next==NULL)mesg("Too few parameters!");{strcpy(tempfile,"");tempcode='r';if(strcmp(command->next->string,"/r")==0){tempcode='r';type=1;}else if(strcmp(command->next->string,"/w")==0){tempcode='w';type=1;}else if(strcmp(command->next->string,"/d")==0){tempcode='d';type=1;}else if(command->next->string[0]=='/')mesg("Error! /r/w/d request!");else if(command->next->next!=NULL)mesg("Too many parameters!");elsestrcpy(tempfile,command->next->string);if(type==1){if(command->next->next!=NULL){if(command->next->next->next!=NULL)mesg("Too many parameters!");elsestrcpy(tempfile,command->next->next->string);}elsemesg("Too few parameters!");}if(strcmp(tempfile,"")){for(i=0;i<savenum;i++){if(strcmp(tempfile,userfd[i].filename)==0)break;}if(i>=savenum)mesg("File not existed!");else{for(i=0;i<opennum;i++){if(strcmp(tempfile,openfd[i].filename)==0)break;}if(i<opennum){mesg("File already opened!");if(openfd[i].opencode!=tempcode){openfd[i].opencode=tempcode;mesg("File permission changed!");}}else if(opennum>=L){mesg("Error! connot open file! number of opened files limited!");}else{strcpy(openfd[opennum].filename,tempfile);openfd[opennum].opencode=tempcode;workfile=opennum;opennum++;mesg("File open success!");}}}}}///////////////////////void myclose()/*关闭*/{int i=0;int tempsave=0;char tempfile[100];if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next!=NULL)mesg("Too many parameters!");else{strcpy(tempfile,command->next->string);for(i=0;i<savenum;i++){if(strcmp(tempfile,userfd[i].filename)==0)break;}if(i>=savenum)mesg("File not existed!");else{for(i=0;i<opennum;i++){if(strcmp(tempfile,openfd[i].filename)==0)break;}if(i>=opennum)mesg("File not opened");else{if(i==opennum-1)opennum--;else{for(;i<opennum-1;i++){strcpy(openfd[i].filename,openfd[i+1].filename);openfd[i].opencode=openfd[i+1].opencode;}opennum--;}mesg("Close file success!");}}}}/////////////////////////void mywrite()/*写*/{int i=0;int tempsave=0;char tempfile[100];if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next!=NULL)mesg("Too many parameters!");else{strcpy(tempfile,command->next->string);for(i=0;i<savenum;i++){if(strcmp(tempfile,userfd[i].filename)==0)break;}if(i>=savenum)mesg("File not existed!");else{tempsave=i;for(i=0;i<opennum;i++){if(strcmp(tempfile,openfd[i].filename)==0)break;}if(i>=opennum)mesg("File not opened");else{mesg("File write");int tt=rand();if(userfd[tempsave].length<=1000)printf("\n The file size from %d KB to %d KB",userfd[tempsave].length,userfd[tempsave].length+tt);/* else if(userfd[tempsave].lenght=1000)printf("\n The file size from 1000 KB");*/elseprintf("\n The file size form %d,%d KB to %d,%d KB",userfd[tempsave].length/1000,userfd[tempsave].length%1000,userfd[tempsave].length+tt/10 00,userfd[tempsave].length+tt%1000);userfd[tempsave].length=userfd[tempsave].length+tt;}}}}///////////////////void help()/*帮助*/{static char *cmd[]={"login","setpass","logout","create","open","read","write","close","delete","dir","help","exit","copy","rename"};static char *cmdhlp[]={"aommand format: login [<username>]","command format:setpass","command format:logout","command format:create <filename>","command format:open [/r/w/d] <filename>","command format:read <filename>","command format:write <filename>","command format:close <filename>","command format:delete <filename>","command format:dir [/u/o/f]","command format:help [<command>]","command format:exit","command format:copy <source filename> <destination filename>","command format:rename <old filename> <new filename>"};static char *detail[]={"explain:user login in the file system.","explain:modify the user password.","explain:user logout the file system.","explain:creat a new file.","explain:/r -- read only [deflaut]\n\t /w -- list opened files \n\t /d --read,modify and delete.","explain:read the file.","explain:modify the file.","explain:close the file.","explain:delete the file.","explain:/u -- list the user account\n\t /0 -- list opened files\n\t /f --list user file[deflaut].","explain:<command> -- list the command detail format and explain.\n\t [deflaut] list the command.","explain:exit from the file sysytem.","explain:copy from one file to another file.","explain:modify the file name."};int helpnum=14;int i=0;if(command->next==NULL){mesg(cmdhlp[10]);mesg(detail[10]);mesg("step 1: login");printf("\t if old user then login,if user not exist then create new user");mesg("step 2: open the file for read(/r),write(/w)or delete(/d)");printf("\t you can open one or more files.one command can open one file");mesg("step 3: read,write or delete some one file");printf("\t you can operate one of the opened files.one command can open one file");mesg("step 4: close the open file");printf("\t you can close one of the opened files.one command can open one file");mesg("step 5: user logout.close all the user opened files");printf("\n command list:");for(i=0;i<helpnum;i++){if(i%4==0)printf("\n");printf(" %-10s",cmd[i]);}}else if(command->next->next!=NULL)mesg("Too many parameters!");else{for(i=0;i<helpnum;i++){if(strcmp(command->next->string,cmd[i])==0)break;}if(i>=helpnum)mesg("the command not existed!");else{mesg(cmdhlp[i]);mesg(detail[i]);}}}//////////////////////void dir()/*列目录文件*/{int i=0;int type=0;char tempcode;if(strcmp(username,"")==0)mesg("No user login!");else{if(command->next==NULL){tempcode='f';}else{if(strcmp(command->next->string,"/u")==0){tempcode='u';}else if(strcmp(command->next->string,"/o")==0){tempcode='o';}else if(strcmp(command->next->string,"/f")==0){tempcode='f';}else if(command->next->string[0]=='/')mesg("Error! /u/o/f request!");else if(command->next->next!=NULL)mesg("Too many parameters!");}if('u'==tempcode){printf("list the user account\n");printf("usename\n");for(i=0;i<usernum;i++){printf("%s\n",mainfd[i].username);}}else if('f'==tempcode){printf("list opened files\n");printf("filename length protect\n");for(i=0;i<savenum;i++){printf("%s%s%d%s%s%c\n",userfd[i].filename," ",userfd[i].length,"KB"," ",userfd[i].protect);}}else if('o'==tempcode){printf("list user files\n");printf("filename opencode\n");for(i=0;i<opennum;i++){printf("%s%s%c\n",openfd[i].filename," ",openfd[i].opencode);}}}}/////////////////////////void mycopy()/*复制*/{char sourfile[256],destfile[256];int i=0,j=0,temp=0;FILE *fp;char tempchar;if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next==NULL)mesg("Too few parametets!");else if(command->next->next->next!=NULL)mesg("Too many parameters!");else{strcpy(sourfile,command->next->string);strcpy(destfile,command->next->next->string);for(i=0;i<savenum;i++){if(strcmp(sourfile,userfd[i].filename)==0)break;}temp=i;if(i>=savenum)printf("\n the source file not eixsted!");else{for(i=0;i<opennum;i++){if(strcmp(sourfile,openfd[i].filename)==0)break;}if(i>=opennum)printf("\n the source file not opened!");else{for(j=0;j<opennum;j++){if(strcmp(destfile,openfd[i].filename)==0)break;}if(j<opennum)mesg("Error! the destination file opened,you must close it first.");else{for(j=0;j<savenum;j++){if(strcmp(destfile,userfd[i].filename)==0)break;}if(j<savenum){mesg("Warning! the destination file exist!");printf("\n Are you sure to recover if ? [Y/N]");while(!kbhit());tempchar=getch();if(tempchar=='N'||tempchar=='n')mesg("Cancel! file not copy.");else if(tempchar=='Y'||tempchar=='y'){mesg("File copy success!file recovered!");userfd[j].length=userfd[i].length;userfd[j].protect=userfd[i].protect;fp=fopen(username,"w+");for(i=0;i<savenum;i++){fputs(userfd[i].filename,fp);fputs("\n",fp);fprintf(fp,"%C\n%d\n",userfd[i].protect,userfd[i].length);}fclose(fp);}}else if(savenum>=M)mesg("copy false! file total limited.");else{strcpy(userfd[savenum].filename,destfile);userfd[savenum].length=userfd[temp].length;userfd[savenum].protect=userfd[temp].protect;savenum++;fp=fopen(username,"w+");for(i=0;i<savenum;i++){fputs(userfd[i].filename,fp);fputs("\n",fp);fprintf(fp,"%c\n%d\n",userfd[i].protect,userfd[i].length);}fclose(fp);mesg("File copy success!");}}}}}}///////////////////////void myrename()/*文件重命名*/{char oldfile[256],newfile[256];int i=0,temp=0;if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next==NULL)mesg("Too few parametets!");else if(command->next->next->next!=NULL)mesg("Too many parameters!");else{strcpy(oldfile,command->next->string);strcpy(newfile,command->next->next->string);for(i=0;i<savenum;i++){if(strcmp(oldfile,userfd[i].filename)==0)break;}temp=i;if(temp>=savenum)printf("\n the source file not eixsted!");else if(temp!=i&&i<savenum){printf("\n newfile and the existing files is the same name, please re-naming!");}else{for(i=0;i<opennum;i++){if(strcmp(oldfile,openfd[i].filename)==0)break;}if(i>=opennum)printf("\n the source file not opened!");else if(strcmp(oldfile,newfile)==0){printf("Rename false! oldfile and newfile can not be the same.");}else{strcpy(openfd[i].filename,newfile);strcpy(userfd[temp].filename,newfile);mesg("Rename file success!\n");}}}}。
操作系统文件管理系统模拟实验

操作系统文件管理系统模拟实验操作系统文件管理系统模拟实验一、实验目的本实验旨在通过模拟操作系统的文件管理系统,加深对操作系统文件管理的理解,锻炼操作系统的应用能力。
二、实验环境1、操作系统:Windows/Linux/MacOS2、编程语言:C/C++/Java/Python等三、实验内容1、初始化文件管理系统1.1 创建根目录,并初始化空文件目录1.2 初始化用户目录和权限设置2、文件操作2.1 创建文件2.1.1 检查文件名合法性2.1.2 检查文件是否已存在2.1.3 为新文件分配磁盘空间2.1.4 添加文件元数据信息2.2 打开文件2.2.1 检查文件是否存在2.2.2 检查用户权限2.3 读取文件内容2.3.1 读取文件权限检查2.3.2 读取文件内容2.4 写入文件内容2.4.1 写入文件权限检查2.4.2 写入文件内容2.5 删除文件2.5.1 检查文件是否存在2.5.2 检查用户权限2.5.3 释放文件占用的磁盘空间2.5.4 删除文件元数据信息3、目录操作3.1 创建子目录3.1.1 检查目录名合法性3.1.2 检查目录是否已存在3.1.3 添加目录元数据信息3.2 打开目录3.2.1 检查目录是否存在3.2.2 检查用户权限3.3 列出目录内容3.3.1 列出目录权限检查3.3.2 列出目录内容3.4 删除目录3.4.1 检查目录是否存在3.4.2 检查用户权限3.4.3 递归删除目录下所有文件和子目录3.4.4 删除目录元数据信息四、实验步骤1、根据实验环境的要求配置操作系统和编程语言环境。
2、初始化文件管理系统,创建根目录,并初始化用户目录和权限设置。
3、进行文件操作和目录操作。
五、实验结果分析根据实验步骤进行文件操作和目录操作,观察系统的运行情况并记录相关实验结果。
六、实验结论通过本实验,深入了解了操作系统中文件管理系统的相关原理和实现方式,并且通过实验操作进一步巩固了相应的应用能力。
操作系统实验参考代码

目录实验一WINDOWS进程初识 (2)实验二进程管理 (6)实验三进程同步的经典算法 (10)实验四存储管理 (14)试验五文件系统试验 (18)实验有关(参考)代码实验一WINDOWS进程初识1、实验目的(1)学会使用VC编写基本的Win32 Consol Application(控制台应用程序)。
(2)掌握WINDOWS API的使用方法。
(3)编写测试程序,理解用户态运行和核心态运行。
2、程序清单清单1-1 一个简单的Windows控制台应用程序// hello项目# include <iostream>void main(){std::cout << “Hello, Win32 Consol Application” << std :: endl ;}清单1-2 核心态运行和用户态运行时间比计算// proclist项目# include <windows.h># include <tlhelp32.h># include <iostream.h>// 当在用户模式机内核模式下都提供所耗时间时,在内核模式下进行所耗时间的64位计算的帮助方法DWORD GetKernelModePercentage(const FILETIME& ftKernel,const FILETIME& ftUser){// 将FILETIME结构转化为64位整数ULONGLONG qwKernel=(((ULONGLONG)ftKernel.dwHighDateTime)<<32)+ftKernel.dwLowDateTime;ULONGLONG qwUser=(((ULONGLONG)ftUser.dwHighDateTime)<<32)+ftUser.dwLowDateTime;// 将消耗时间相加,然后计算消耗在内核模式下的时间百分比ULONGLONG qwTotal=qwKernel+qwUser;DWORD dwPct=(DWORD)(((ULONGLONG)100*qwKernel)/qwTotal);return(dwPct);}// 以下是将当前运行过程名和消耗在内核模式下的时间百分数都显示出来的应用程序void main(int argc,char *argv[]){if(argc<2){cout<<"请给出你要查询的程序名"<<endl;exit(0);}// 对当前系统中运行的过程拍取“快照”HANDLE hSnapshot=::CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS,// 提取当前过程0);// 如果是当前过程,就将其忽略// 初始化过程入口PROCESSENTRY32 pe;::ZeroMemory(&pe,sizeof(pe));pe.dwSize=sizeof(pe);BOOL bMore=::Process32First(hSnapshot,&pe);BOOL found = FALSE;while(bMore){// 打开用于读取的过程if(!strcmp(pe.szExeFile,argv[1])){found = TRUE;HANDLE hProcess=::OpenProcess(PROCESS_QUERY_INFORMA TION,// 指明要得到信息FALSE,// 不必继承这一句柄pe.th32ProcessID);// 要打开的进程if (hProcess!=NULL){// 找出进程的时间FILETIME ftCreation,ftKernelMode,ftUserMode,ftExit;::GetProcessTimes(hProcess,// 所感兴趣的进程&ftCreation,// 进程的启动时间&ftExit,// 结束时间(如果有的话)&ftKernelMode,// 在内核模式下消耗的时间&ftUserMode);// 在用户模式下消耗的时间// 计算内核模式消耗的时间百分比DWORD dwPctKernel=::GetKernelModePercentage(ftKernelMode,// 在内核模式上消耗的时间ftUserMode);// 在用户模式下消耗的时间// 向用户显示进程的某些信息cout<< "process ID: " << pe.th32ProcessID<< ",EXE file:" << pe.szExeFile<< ",%d in Kernel mode: " << dwPctKernel << endl;// 消除句柄::CloseHandle(hProcess);}}// 转向下一个进程bMore=::Process32Next(hSnapshot,&pe);}if(found==FALSE){cout<<"当前系统没有这个可执行程序正在运行"<<endl;exit(0);}}清单1-3 核心态运行和用户态运行时间测试程序#include <stdio.h>main(){int i,j;while(1){for(i=0;i<1000;i++);for(j=1;j<1000;j++) printf(“enter kernel mode running.”);}}实验二进程管理1、实验目的1) 通过创建进程、观察正在运行的进程和终止进程的程序设计和调试操作,进一步熟悉操作系统的进程概念,理解Windows进程的“一生”。
文件管理系统源代码

文件管理系统一、实验目的通过设计一个多用户文件系统,了解操作系统中文件的组织与管理,熟悉文件管理所用的数据结构,加深对文件系统内部功能实现过程的理解。
二、实验内容1.用C语言或C++语言设计一个最多包括N个用户的多用户文件系统,约定每个用户最多保存M个文件。
同时限制一个用户在进入系统后,最多打开L个文件。
2.系统应具备一定的健壮性。
即能够检查用户所输入命令的正确性,出错时显示出必要的信息。
另外,对文件需设置必要的保护措施。
3.文件目录结构采用两级目录结构:主文件目录和用户文件目录#include"io.h"#include"conio.h"#include"stdio.h"#include"stdlib.h"#include"malloc.h"#include"string.h"#include"ctype.h"#define N 30 /*用户数*/#define M 20 /*一个用户可保存M个文件*/#define L 5 /*用户只能一次打开L个文件*/typedef struct MFD /*主文件目录*/{char username[100];char password[100];FILE fp; /*文件指针*/}MFD;///////////typedef struct UFD /*用户文件目录*/{char filename[256];char protect; /*保护码*/int length; /*文件长度*/}UFD;//////////typedef struct OFD /*打开文件目录*/{char filename[256];char opencode; /*打开保护码*/int fp; /*读写指针*/}OFD;//////////typedef struct COMM /*命令串*/{char string[256]; /*命令*/struct COMM *next;/*后继指针*/}COMM;////////////MFD mainfd[N]; /*主文件目录数组*/UFD userfd[M]; /*用户文件目录数组*/OFD openfd[L]; /*打开文件目录数组*/////////COMM*command; /*命令串指针*/char username[10];int usernum,savenum,opennum;int workfile;void init();void init_ufd(char *username);/*初始化用户文件目录*/ void mesg(char *str); /*消息*/char *getpass(); /*设置口令函数声明*/ char *getuser(); /*设置用户函数声明*/ COMM *readcommand(); /*读命令串函数声明*/ void login(); /*用户登录*/void logout(); /*用户注销*/void setpass(); /*设置口令*/void create(); /*创建文件*/void mydelete(); /*删除文件*/void myread(); /*读文件*/void myopen(); /*打开文件*/void myclose(); /*关闭文件*/void mywrite(); /*写文件*/void help(); /*帮助*/void dir(); /*列文件目录*/void mycopy(); /*复制文件*/void myrename(); /*重命名文件名*//////////////void main(){init();for(;;){readcommand();if(strcmp(command->string,"create")==0)create();else if(strcmp(command->string,"delete")==0)mydelete();else if(strcmp(command->string,"open")==0)myopen();else if(strcmp(command->string,"close")==0)myclose();else if(strcmp(command->string,"read")==0)myread();else if(strcmp(command->string,"write")==0)mywrite();else if(strcmp(command->string,"copy")==0)mycopy();else if(strcmp(command->string,"rename")==0)myrename();else if(strcmp(command->string,"login")==0)login();else if(strcmp(command->string,"setpass")==0)setpass();else if(strcmp(command->string,"logout")==0)logout();else if(strcmp(command->string,"help")==0)help();else if(strcmp(command->string,"dir")==0)dir();else if(strcmp(command->string,"exit")==0)break;elsemesg("Bad command!");}}///////////////////void init(){FILE *fp;char tempname[20],temppass[20];int i=0;usernum=0;savenum=0;opennum=0;strcpy(username,"");if((fp=fopen("mainfile.txt","r"))!=NULL){while(!feof(fp)){strcpy(tempname,"");fgets(tempname,20,fp);if(strcmp(tempname,"")!=0){fgets(temppass,20,fp);tempname[strlen(tempname)-1]='\0';temppass[strlen(tempname)-1]='\0';strcpy(mainfd[usernum].username,tempname);strcpy(mainfd[usernum].password,tempname);usernum++;}}fclose(fp);}}///////////////////////void init_ufd(char *username)/*初始化用户文件目录*/{FILE *fp;char tempfile[100],tempprot;int templength;savenum=0;opennum=0;workfile=-1;if((fp=fopen(username,"w+"))!=NULL){while(!feof(fp)){strcpy(tempfile,"");fgets(tempfile,50,fp);if(strcmp(tempfile,"")!=0){fscanf(fp,"%c",&tempprot);fscanf(fp,"%d",&templength);tempfile[strlen(tempfile)-1]='\0';strcpy(userfd[savenum].filename,tempfile);userfd[savenum].protect=tempprot;userfd[savenum].length=templength;savenum++;fgets(tempfile,50,fp);}}}fclose(fp);}////////////////////void mesg(char *str){printf("\n %s\n",str);}////////////////////////////char *getuser(){char username[20];char temp;int i;username[0]='\0';for(i=0;i<10;){while(!kbhit());temp=getch();if(isalnum(temp)||temp=='_'||temp==13){username[i]=temp;if(username[i]==13){username[i]='\0';break;}putchar(temp);i++;username[i]='\0';}}return(username);}///////////////////char *getpass(){char password[20];char temp;int i;password[0]='\0';for(i=0;i<10;){while(!kbhit());temp=getch();if(isalnum(temp)||temp=='_'||temp==13){password[i]=temp;if(password[i]==13){password[i]='\0';break;}putchar('*');i++;password[i]='\0';}}return(password);}///////////////COMM *readcommand(){char temp[256];char line[256];int i,end;COMM *newp,*p;command=NULL;strcpy(line,"");while(strcmp(line,"")==0){printf("\nc:\\>");gets(line);}for(i=0;i<=strlen(line);i++){if(line[i]==' ')i++;end=0;while(line[i]!='\0'&&line[i]!=' '){temp[end]=line[i];end++;i++;}if(end>0){temp[end]='\0';newp=(COMM *)malloc(sizeof(COMM *));strcpy(newp->string,temp);newp->next=NULL;if(command==NULL)command=newp;else{p=command;while(p->next!=NULL)p=p->next;p->next=newp;}}}p=command;return(command);}/////////////////////////////void login() /*用户注册*/{FILE *fp;int i;char password[20],confirm[20],tempname[20];if(command->next==NULL){printf("\n User Name:");strcpy(tempname,getuser());}else if(command->next->next!=NULL){mesg("Too many parameters!");return;}elsestrcpy(tempname,command->next->string);for(i=0;i<usernum;i++)if(strcmp(mainfd[i].username,tempname)==0)break; /*从mainfd表中查找要注册的用户*/ if(i>=usernum) /*新用户*/{printf("\n new user account,enter your password twice!");printf("\n Password:");strcpy(password,getpass());/*输入口令且返回之*/printf("\n Password:");strcpy(confirm,getpass()); /*第二次输入口令*/if(strcmp(password,confirm)==0)/*用户数不能超过N*/{if(usernum>=N)mesg("Create new account false!number of user asscount limited.\n login fasle!");else{strcpy(mainfd[usernum].username,tempname);/*把新用户和口令填入mainfd中*/strcpy(mainfd[usernum].password,password);usernum++;strcpy(username,tempname);mesg("Create a new user!\n login success!");init_ufd(username); /*初始化用户文件目录*/fp=fopen("mainfile.txt","w+"); /*把新用户填入mainfile.txt文件中*/for(i=0;i<usernum;i++){fputs(mainfd[i].username,fp);fputs("\n",fp);fputs(mainfd[i].password,fp);fputs("\n",fp);}fclose(fp);}}else{mesg("Create new account false! Error password.");mesg("login false!");}}else{printf("\n Password:");strcpy(password,getpass());if(strcmp(mainfd[i].password,password)!=0)mesg("Login false! Error password.");else{mesg("Login success!");strcpy(username,tempname);init_ufd(username);}}}/////////////////////////void logout() /*用户注销*/{if(command->next!=NULL)mesg("Too many parameters!");else if(strcmp(username,"")==0)mesg("No user login!");else{strcpy(username,"");opennum=0;savenum=0;workfile=-1;mesg("User logout!");}}////////////////////void setpass() /*修改口令*/{int i=0;FILE *fp;char oldpass[20],newpass[20],confirm[20];if(strcmp(username,"")==0)mesg("No user login!");else{printf("\n Old password:");strcpy(oldpass,getpass());for(int i=0;i<usernum;i++){if(strcmp(mainfd[i].username,username)==0)break;}if(strcmp(mainfd[i].password,oldpass)!=0)mesg("Old password error!");else{printf("\n New password:");strcpy(newpass,getpass());printf("\n Confirm password:");strcpy(confirm,getpass());if(strcmp(newpass,confirm)!=0)mesg("Password not change! confirm different.");else{strcpy(mainfd[i].password,newpass);mesg(" Password change !");fp=fopen("mainfile.txt","w+");for(i=0;i<usernum;i++){fputs(mainfd[i].username,fp);fputs("\n",fp);fputs(mainfd[i].password,fp);fputs("\n",fp);}fclose(fp);}}}}////////////////void create(){int i=0;FILE *fp;if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next!=NULL)mesg("Too many parameters!");else{for(i=0;i<savenum;i++){if(strcmp(userfd[i].filename,command->next->string)==0)break;}if(i<savenum)mesg("Error! the file already existed!");else if(savenum>=M)mesg("Error! connot create file! number of files limited!");else{strcpy(userfd[savenum].filename,command->next->string);userfd[i].protect='r';userfd[i].length=rand();savenum++;mesg("Create file success!");fp=fopen(username,"w+");for(i=0;i<savenum;i++){fputs(userfd[i].filename,fp);fputs("\n",fp);fprintf(fp,"%c\n%d\n",userfd[i].protect,userfd[i].length);}fclose(fp);}}}/////////////////void mydelete() /*删除*/{int i=0;int tempsave=0;FILE *fp;if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next!=NULL)mesg("Too many parameters!");else{for(i=0;i<savenum;i++){if(strcmp(userfd[i].filename,command->next->string)==0)break;}if(i>=savenum)mesg("Error! the file not existed!");else{tempsave=i;for(i=0;i<opennum;i++){if(strcmp(command->next->string,openfd[i].filename)==0)break;}if(i>=opennum)mesg("File not open");////////////////////////////////////////////else{if(tempsave==savenum-1)savenum--;else{for(;tempsave<savenum-1;tempsave++){strcpy(userfd[tempsave].filename,userfd[tempsave+1].filename);userfd[tempsave].protect=userfd[tempsave+1].protect;userfd[tempsave].length=userfd[tempsave+1].length;}savenum--;}mesg("Delete file success!");fp=fopen(username,"w+");for(i=0;i<savenum;i++){fputs(userfd[i].filename,fp);fputs("\n",fp);fprintf(fp,"%c\n%d\n",userfd[i].protect,userfd[i].length);}fclose(fp);}}}}//////////////////void myread() /*读*/{int i=0;int tempsave=0;char tempfile[100];if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next!=NULL)mesg("Too many parameters!");elsestrcpy(tempfile,command->next->string);for(i=0;i<savenum;i++){if(strcmp(tempfile,userfd[i].filename)==0)break;}if(i>=savenum)mesg("File not existed!");else{tempsave=i;for(i=0;i<opennum;i++){if(strcmp(tempfile,openfd[i].filename)==0)break;}if(i>=opennum)mesg("File not opened");else{if(userfd[tempsave].length<1000)printf("\n The file size is %d KB",userfd[tempsave].length);else if(userfd[tempsave].length==1000)printf("\n The file size is 1000 KB");elseprintf("\n The file size is %d,%d KB",userfd[tempsave].length/1000,userfd[tempsave].length%1000);mesg("File read");}}}}/////////////////void myopen()/*打开*/{int i=0;int type=0;char tempcode;char tempfile[100];if(strcmp(username,"")==0)mesg("No user login!");else if(command->next==NULL)mesg("Too few parameters!");{strcpy(tempfile,"");tempcode='r';if(strcmp(command->next->string,"/r")==0){tempcode='r';type=1;}else if(strcmp(command->next->string,"/w")==0){tempcode='w';type=1;}else if(strcmp(command->next->string,"/d")==0){tempcode='d';type=1;}else if(command->next->string[0]=='/')mesg("Error! /r/w/d request!");else if(command->next->next!=NULL)mesg("Too many parameters!");elsestrcpy(tempfile,command->next->string);if(type==1){if(command->next->next!=NULL){if(command->next->next->next!=NULL)mesg("Too many parameters!");elsestrcpy(tempfile,command->next->next->string);}elsemesg("Too few parameters!");}if(strcmp(tempfile,"")){for(i=0;i<savenum;i++){if(strcmp(tempfile,userfd[i].filename)==0)break;}if(i>=savenum)mesg("File not existed!");else{for(i=0;i<opennum;i++){if(strcmp(tempfile,openfd[i].filename)==0)break;}if(i<opennum){mesg("File already opened!");if(openfd[i].opencode!=tempcode){openfd[i].opencode=tempcode;mesg("File permission changed!");}}else if(opennum>=L){mesg("Error! connot open file! number of opened files limited!");}else{strcpy(openfd[opennum].filename,tempfile);openfd[opennum].opencode=tempcode;workfile=opennum;opennum++;mesg("File open success!");}}}}}///////////////////////void myclose()/*关闭*/{int i=0;int tempsave=0;char tempfile[100];if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next!=NULL)mesg("Too many parameters!");else{strcpy(tempfile,command->next->string);for(i=0;i<savenum;i++){if(strcmp(tempfile,userfd[i].filename)==0)break;}if(i>=savenum)mesg("File not existed!");else{for(i=0;i<opennum;i++){if(strcmp(tempfile,openfd[i].filename)==0)break;}if(i>=opennum)mesg("File not opened");else{if(i==opennum-1)opennum--;else{for(;i<opennum-1;i++){strcpy(openfd[i].filename,openfd[i+1].filename);openfd[i].opencode=openfd[i+1].opencode;}opennum--;}mesg("Close file success!");}}}}/////////////////////////void mywrite()/*写*/{int i=0;int tempsave=0;char tempfile[100];if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next!=NULL)mesg("Too many parameters!");else{strcpy(tempfile,command->next->string);for(i=0;i<savenum;i++){if(strcmp(tempfile,userfd[i].filename)==0)break;}if(i>=savenum)mesg("File not existed!");else{tempsave=i;for(i=0;i<opennum;i++){if(strcmp(tempfile,openfd[i].filename)==0)break;}if(i>=opennum)mesg("File not opened");else{mesg("File write");int tt=rand();if(userfd[tempsave].length<=1000)printf("\n The file size from %d KB to %d KB",userfd[tempsave].length,userfd[tempsave].length+tt);/* else if(userfd[tempsave].lenght=1000)printf("\n The file size from 1000 KB");*/elseprintf("\n The file size form %d,%d KB to %d,%d KB",userfd[tempsave].length/1000,userfd[tempsave].length%1000,userfd[tempsave].length+tt/10 00,userfd[tempsave].length+tt%1000);userfd[tempsave].length=userfd[tempsave].length+tt;}}}}///////////////////void help()/*帮助*/{static char *cmd[]={"login","setpass","logout","create","open","read","write","close","delete","dir","help","exit","copy","rename"};static char *cmdhlp[]={"aommand format: login [<username>]","command format:setpass","command format:logout","command format:create <filename>","command format:open [/r/w/d] <filename>","command format:read <filename>","command format:write <filename>","command format:close <filename>","command format:delete <filename>","command format:dir [/u/o/f]","command format:help [<command>]","command format:exit","command format:copy <source filename> <destination filename>","command format:rename <old filename> <new filename>"};static char *detail[]={"explain:user login in the file system.","explain:modify the user password.","explain:user logout the file system.","explain:creat a new file.","explain:/r -- read only [deflaut]\n\t /w -- list opened files \n\t /d --read,modify and delete.","explain:read the file.","explain:modify the file.","explain:close the file.","explain:delete the file.","explain:/u -- list the user account\n\t /0 -- list opened files\n\t /f --list user file[deflaut].","explain:<command> -- list the command detail format and explain.\n\t [deflaut] list the command.","explain:exit from the file sysytem.","explain:copy from one file to another file.","explain:modify the file name."};int helpnum=14;int i=0;if(command->next==NULL){mesg(cmdhlp[10]);mesg(detail[10]);mesg("step 1: login");printf("\t if old user then login,if user not exist then create new user");mesg("step 2: open the file for read(/r),write(/w)or delete(/d)");printf("\t you can open one or more files.one command can open one file");mesg("step 3: read,write or delete some one file");printf("\t you can operate one of the opened files.one command can open one file");mesg("step 4: close the open file");printf("\t you can close one of the opened files.one command can open one file");mesg("step 5: user logout.close all the user opened files");printf("\n command list:");for(i=0;i<helpnum;i++){if(i%4==0)printf("\n");printf(" %-10s",cmd[i]);}}else if(command->next->next!=NULL)mesg("Too many parameters!");else{for(i=0;i<helpnum;i++){if(strcmp(command->next->string,cmd[i])==0)break;}if(i>=helpnum)mesg("the command not existed!");else{mesg(cmdhlp[i]);mesg(detail[i]);}}}//////////////////////void dir()/*列目录文件*/{int i=0;int type=0;char tempcode;if(strcmp(username,"")==0)mesg("No user login!");else{if(command->next==NULL){tempcode='f';}else{if(strcmp(command->next->string,"/u")==0){tempcode='u';}else if(strcmp(command->next->string,"/o")==0){tempcode='o';}else if(strcmp(command->next->string,"/f")==0){tempcode='f';}else if(command->next->string[0]=='/')mesg("Error! /u/o/f request!");else if(command->next->next!=NULL)mesg("Too many parameters!");}if('u'==tempcode){printf("list the user account\n");printf("usename\n");for(i=0;i<usernum;i++){printf("%s\n",mainfd[i].username);}}else if('f'==tempcode){printf("list opened files\n");printf("filename length protect\n");for(i=0;i<savenum;i++){printf("%s%s%d%s%s%c\n",userfd[i].filename," ",userfd[i].length,"KB"," ",userfd[i].protect);}}else if('o'==tempcode){printf("list user files\n");printf("filename opencode\n");for(i=0;i<opennum;i++){printf("%s%s%c\n",openfd[i].filename," ",openfd[i].opencode);}}}}/////////////////////////void mycopy()/*复制*/{char sourfile[256],destfile[256];int i=0,j=0,temp=0;FILE *fp;char tempchar;if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next==NULL)mesg("Too few parametets!");else if(command->next->next->next!=NULL)mesg("Too many parameters!");else{strcpy(sourfile,command->next->string);strcpy(destfile,command->next->next->string);for(i=0;i<savenum;i++){if(strcmp(sourfile,userfd[i].filename)==0)break;}temp=i;if(i>=savenum)printf("\n the source file not eixsted!");else{for(i=0;i<opennum;i++){if(strcmp(sourfile,openfd[i].filename)==0)break;}if(i>=opennum)printf("\n the source file not opened!");else{for(j=0;j<opennum;j++){if(strcmp(destfile,openfd[i].filename)==0)break;}if(j<opennum)mesg("Error! the destination file opened,you must close it first.");else{for(j=0;j<savenum;j++){if(strcmp(destfile,userfd[i].filename)==0)break;}if(j<savenum){mesg("Warning! the destination file exist!");printf("\n Are you sure to recover if ? [Y/N]");while(!kbhit());tempchar=getch();if(tempchar=='N'||tempchar=='n')mesg("Cancel! file not copy.");else if(tempchar=='Y'||tempchar=='y'){mesg("File copy success!file recovered!");userfd[j].length=userfd[i].length;userfd[j].protect=userfd[i].protect;fp=fopen(username,"w+");for(i=0;i<savenum;i++){fputs(userfd[i].filename,fp);fputs("\n",fp);fprintf(fp,"%C\n%d\n",userfd[i].protect,userfd[i].length);}fclose(fp);}}else if(savenum>=M)mesg("copy false! file total limited.");else{strcpy(userfd[savenum].filename,destfile);userfd[savenum].length=userfd[temp].length;userfd[savenum].protect=userfd[temp].protect;savenum++;fp=fopen(username,"w+");for(i=0;i<savenum;i++){fputs(userfd[i].filename,fp);fputs("\n",fp);fprintf(fp,"%c\n%d\n",userfd[i].protect,userfd[i].length);}fclose(fp);mesg("File copy success!");}}}}}}///////////////////////void myrename()/*文件重命名*/{char oldfile[256],newfile[256];int i=0,temp=0;if(strcmp(username,"")==0)mesg("No user lgoin!");else if(command->next==NULL)mesg("Too few parametets!");else if(command->next->next==NULL)mesg("Too few parametets!");else if(command->next->next->next!=NULL)mesg("Too many parameters!");else{strcpy(oldfile,command->next->string);strcpy(newfile,command->next->next->string);for(i=0;i<savenum;i++){if(strcmp(oldfile,userfd[i].filename)==0)break;}temp=i;if(temp>=savenum)printf("\n the source file not eixsted!");else if(temp!=i&&i<savenum){printf("\n newfile and the existing files is the same name, please re-naming!");}else{for(i=0;i<opennum;i++){if(strcmp(oldfile,openfd[i].filename)==0)break;}if(i>=opennum)printf("\n the source file not opened!");else if(strcmp(oldfile,newfile)==0){printf("Rename false! oldfile and newfile can not be the same.");}else{strcpy(openfd[i].filename,newfile);strcpy(userfd[temp].filename,newfile);mesg("Rename file success!\n");}}}}。
操作系统上机实验代码

操作系统上机实验代码修改后的md命令程序:int MdComd(int k) //md命令处理函数{// 命令形式:md <目录名>// 功能:在指定路径下创建指定目录,若没有指定路径,则在当前目录下创建指定目录。
// 对于重名目录给出错误信息。
目录与文件也不能重名。
// 学生可以考虑命令中加“属性”参数,用于创建指定属性的子目录。
命令形式如下:// md <目录名>[ <属性>]// 属性包括R、H、S以及它们的组合(不区分大小写,顺序也不限)。
例如:// md user rh// 其功能是在当前目录中创建具有“只读”和“隐藏”属性的子目录user。
short i,s,s0,kk;char attrib=(char)16,*DirName;FCB *p;char str[20]="|";kk=SIZE/sizeof(FCB);if (k<1){cout<<"\错误:命令中没有目录名。
\";return -1;}if (k>2){cout<<"\错误:命令参数太多。
\";return -1;}s=ProcessPath(comd[1],DirName,k,0,attrib);if (s<0)return s; //失败,返回if (!IsName(DirName)) //若名字不符合规则{cout<<"\命令中的新目录名错误。
\";return -1;}i=FindFCB(DirName,s,attrib,p);if (i>0){cout<<"\错误:目录重名!\";return -1;}if (k==2) //命令形式:md <目录名> <属性符>{i=GetAttrib(strcat(str,comd[2]),attrib);//由i=GetAttrib(comd[2],attrib);if (i<0)return i;}s0=FindBlankFCB(s,p);//找空白目录项if (s0<0) //磁盘满return s0;s0=M_NewDir(DirName,p,s,attrib); //在p所指位置创建一新子目录项if (s0<0) //创建失败{cout<<"\磁盘空间已满,创建目录失败。
操作系统实验文件管理C++代码
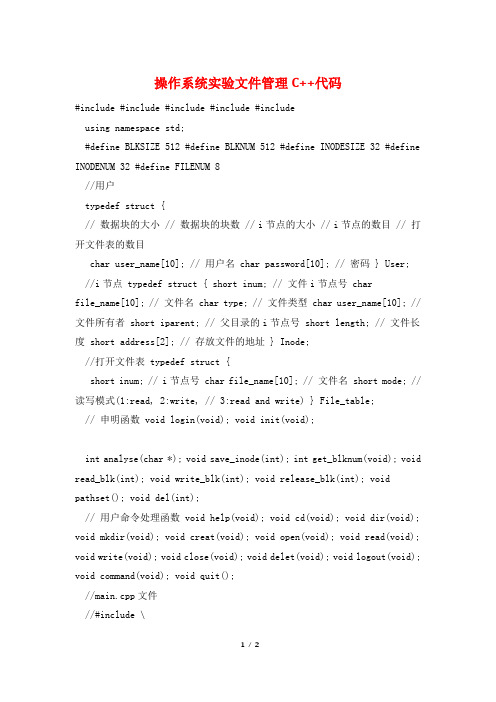
操作系统实验文件管理C++代码#include #include #include #include #includeusing namespace std;#define BLKSIZE 512 #define BLKNUM 512 #define INODESIZE 32 #define INODENUM 32 #define FILENUM 8//用户typedef struct {// 数据块的大小 // 数据块的块数 // i节点的大小 // i节点的数目 // 打开文件表的数目char user_name[10]; // 用户名 char password[10]; // 密码 } User; //i节点 typedef struct { short inum; // 文件i节点号 charfile_name[10]; // 文件名 char type; // 文件类型 char user_name[10]; // 文件所有者 short iparent; // 父目录的i节点号 short length; // 文件长度 short address[2]; // 存放文件的地址 } Inode;//打开文件表 typedef struct {short inum; // i节点号 char file_name[10]; // 文件名 short mode; // 读写模式(1:read, 2:write, // 3:read and write) } File_table;// 申明函数 void login(void); void init(void);int analyse(char *); void save_inode(int); int get_blknum(void); void read_blk(int); void write_blk(int); void release_blk(int); void pathset(); void del(int);// 用户命令处理函数 void help(void); void cd(void); void dir(void); void mkdir(void); void creat(void); void open(void); void read(void); void write(void); void close(void); void delet(void); void logout(void); void command(void); void quit();//main.cpp文件//#include \//定义全局变量 char choice; int argc; // 用户命令的参数个数 char*argv[5]; // 用户命令的参数 int inum_cur; // 当前目录 chartemp[2*BLKSIZE]; // 缓冲区 User user; // 当前的用户 char bitmap[BLKNUM]; // 位图数组Inode inode_array[INODENUM]; // i节点数组 File_tablefile_array[FILENUM]; // 打开文件表数组 char image_name[10] = \// 文件系统名称 FILE *fp; // 打开文件指针//创建映像hd,并将所有用户和文件清除 void format(void) { int i;Inode inode; printf(\ printf(\ printf(\ scanf(\getchar(); if((choice == 'y') || (choice == 'Y')){ if((fp=fopen(image_name, \ { printf(\ exit(-1); } for(i = 0; i。
操作系统实验(金虎)实验四文件管理源代码

if(flag1==0)//将指针指向申请fat表块数第一块
{
p->f=r;
flag1++;
}
p->length++;
r->next=NULL;
while(t->next!=NULL)
t=t->next;
int i=0,j=0;
for(i=0;i<8;i++)
{
for(j=0;j<8;j++)
cout<<map[i][j]<<" ";
cout<<endl;
}
}
void mk()
{
Fname *p,*q,*s;
Fat *r,*t;
q=temp;
s=temp;
t=Ft;
cuur = time(NULL);
strftime(tmp,sizeof(tmp),"%Y-%m-%d %X",localtime(&cuur));//显示时间
int i,j,a=0,num,k=0,flag1=0;
string name;
cin>>name;
cout<<"请输入文件大小:"<<endl;
q=q->brother;
}
}
while(r->file!=NULL) //计算文件所占字节
{
f=r->file->f;
y=r->file->length;
操作系统文件管理实验
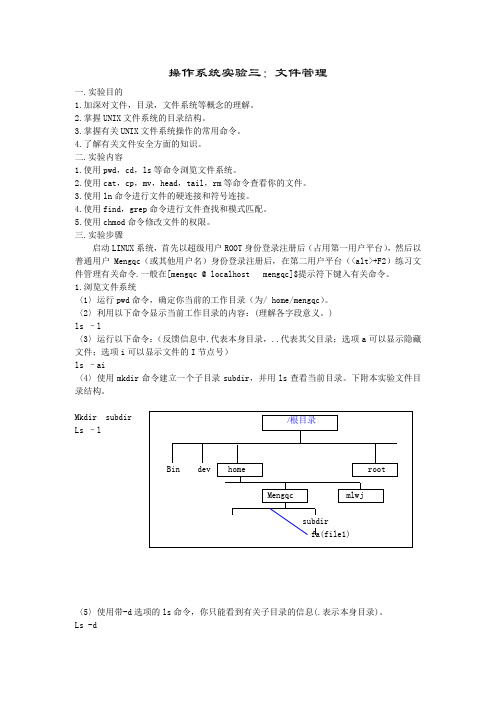
操作系统实验三:文件管理一.实验目的1.加深对文件,目录,文件系统等概念的理解。
2.掌握UNIX文件系统的目录结构。
3.掌握有关UNIX文件系统操作的常用命令。
4.了解有关文件安全方面的知识。
二.实验内容1.使用pwd,cd,ls等命令浏览文件系统。
2.使用cat,cp,mv,head,tail,rm等命令查看你的文件。
3.使用ln命令进行文件的硬连接和符号连接。
4.使用find,grep命令进行文件查找和模式匹配。
5.使用chmod命令修改文件的权限。
三.实验步骤启动LINUX系统,首先以超级用户ROOT身份登录注册后(占用第一用户平台),然后以普通用户Mengqc(或其他用户名)身份登录注册后,在第二用户平台(<alt>+F2)练习文件管理有关命令.一般在[mengqc @ localhost mengqc]$提示符下键入有关命令。
1.浏览文件系统〈1〉运行pwd命令,确定你当前的工作目录(为/ home/mengqc)。
〈2〉利用以下命令显示当前工作目录的内容:(理解各字段意义。
)ls –l〈3〉运行以下命令:(反馈信息中.代表本身目录,..代表其父目录;选项a可以显示隐藏文件;选项i可以显示文件的I节点号)ls –ai〈4〉使用mkdir命令建立一个子目录subdir,并用ls查看当前目录。
下附本实验文件目录结构。
Mkdir subdir/根目录Ls –lBin dev home rootMengqc mlwjsubdirfa(file1)〈5〉使用带-d选项的ls命令,你只能看到有关子目录的信息(.表示本身目录)。
Ls -d〈6〉使用cd命令,将工作目录改到根目录(/)上。
①用相对路径将工作目录改到根目录。
Pwd 显示当前目录为 /home/mengqcCd .. 相对路径,返回上一级。
Pwd 反馈显示当前目录为 /homeCd .. 相对路径,再返回上一级。
Pwd 反馈显示当前目录为 /Cd /home/mengqc 恢复本身目录(也可以cd↙ ).Pwd 反馈显示当前目录为 /home/mengqc②用绝对路径将工作目录改到根目录。
操作系统实验报告5——目录与文件管理

实验序号: 05 实验项目名称: linux下文件管理
学 号
2009406012
姓 名
吴松娇
Hale Waihona Puke 专业、班09计本(1)实验地点
32404
指导教师
汤敏丽
时间
2012.6.6
一、实验目的
1.加深对操作系统文件管理功能的理解。
2.熟练掌握linux下文件管理命令。
二、实验环境
装有linux系统的计算机。
(4)再次输入命令“ls -1”,确认两个目录和一个文件是否成功创建。
(5)输入命令“mv–f dir2 dir1”,将dir2目录移动到dirl目录;输入命令“mv–f cs.txt dir1”,将cs.txt目录移动到dirl目录。
(6)输入命令“cd dirl”,切换到dirl目录,再输入“ls”命令,查看到dir2目录。
13、分屏显示文件内容:more例子:ls | more /说明:每次分屏地查看由ls命令显示的/下子目录清单。
14、磁盘操作工具:du例子:du -sh .说明:列出当前目录下的所有子目录和文件占用的空间。
15、查找文件所在目录命令:which例子:which rm说明:查找rm命令保存在哪个目录。
(7)删除dir2目录,输入命令“rmdir dir2。”
2.文件操作
1.创建命令: touch例子: touch skxiao.xml说明:创建文件skxiao.xml
2.删除命令: rm例子: rm -r /home/shenkxiao/说明:删除/home/shenkxiao/下的所有文件
3.复制命令: cp例子: cp skxiao.xml /home/shenkxiao说明:将当前目录下的skxiao.xml文件拷贝到/home/shenkxiao目录下
操作系统+文件管理+部分代码

}
注销用户
void Logout(int a)
{ //注销用户,清除所有文件的登记栏
string str;
char temp[5]="";
for(int i=0;i<L;i++)
{ int i=0;
while(strcmp(MFD[i].Username,"")!=0&&(i<N))//查看MFD,检测用户数是否已满
{ i++; }
if(i>=N){ //如果用户数已满
cout<<"用户数已满. 你必须删除一个用户才继续新建用户"<<endl;
2.3 数据结构设计
系统管理员
typedef struct user //系统管理员
{ char name[5] ; // 登录用户名
char password[10] ; // 登录密码
}user;
用户文件目录
struct UFD
cin>>fil;4) goto Re;//如果文件名长度不符合要求,则要求重新输入
loop:
cout<<"文件属性(r,rw) :";//输入文件属性,读或是读写
open 打开文件
close 关闭文件
read 读文件
write 写文件
(2)列目录时要列出文件名、物理地址、保护码和文件长度;
(3)源文件可以进行读写保护。
提示:
(1)首先应确定文件系统的数据结构:主目录、子目录及活动文件等。主目录和子目录都以文件的形式存放于磁盘,这样便于查找和修改。
操作系统实验5文件系统:Linux文件管理

(1)掌握Linux 提供的文件系统调用的使用方法;
(2)熟悉文件和目录操作的系统调用用户接口;
(3)了解操作系统文件系统的工作原理和工作方式。
(1) 利用Linux 有关系统调用函数编写一个文件工具filetools,要求具有下列功能:***********
0. 退出
1. 创建新文件
2. 写文件
3. 读文件
4. 复制文件
5. 修改文件权限
6. 查看文件权限
7. 创建子目录
8. 删除子目录
9. 改变当前目录到指定目录
10. 链接操作
通过这次实验掌握Linux 提供的文件系统调用的使用方法;熟悉文件和目录操作的调用用户接口,了解操作系统文件系统的工作原理和工作方式。
实验3 操作系统文件管理实验报告

实验3 操作系统文件管理
班级_________________ 学号_________________ 姓名_________________ 1.DOS目录、文件夹和文件
1)说明以下命令的功能。
a)dir命令
b)cd命令
c)mkdir命令
d)copy命令
e)echo命令
f)copy命令
g)del命令
h)rd命令
(提示:可以使用/?开关参数来查看命令的说明。
)
2)回答以下问题
a)dir、dir /p 和dir /w显示结果有什么不同?
b)当输入错误命令时,会发生什么现象?
c)当输入dir /?会显示些什么?命令参数“/?”有什么意义?
d)如何显示驱动器C上以及驱动器D上的目录?
e)如何删除以“new”开头的文件?
f)如何创建名为paper的目录?
g)如何把扩展名为.doc的文件复制到paper目录?
h)如何删除paper目录?
i)在当前目录下使用dir,显示出来的文件信息中包括:“.”和“..”两个目录分别什么含义?2.实验心得体会:。
操作系统实验文件管理代码
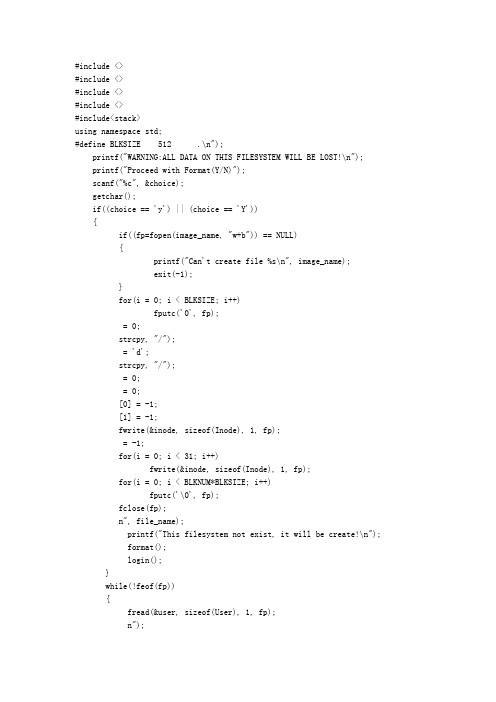
#include <>#include <>#include <>#include <>#include<stack>using namespace std;#define BLKSIZE 512 .\n");printf("WARNING:ALL DATA ON THIS FILESYSTEM WILL BE LOST!\n");printf("Proceed with Format(Y/N)");scanf("%c", &choice);getchar();if((choice == 'y') || (choice == 'Y')){if((fp=fopen(image_name, "w+b")) == NULL){printf("Can't create file %s\n", image_name);exit(-1);}for(i = 0; i < BLKSIZE; i++)fputc('0', fp);= 0;strcpy, "/");= 'd';strcpy, "/");= 0;= 0;[0] = -1;[1] = -1;fwrite(&inode, sizeof(Inode), 1, fp);= -1;for(i = 0; i < 31; i++)fwrite(&inode, sizeof(Inode), 1, fp);for(i = 0; i < BLKNUM*BLKSIZE; i++)fputc('\0', fp);fclose(fp);n", file_name);printf("This filesystem not exist, it will be create!\n");format();login();}while(!feof(fp)){fread(&user, sizeof(User), 1, fp);n");flag = 1;fclose(fp);break;}}if(flag == 0) break;}while(flag);n", image_name);exit(-1);}num = -1;}n");exit(-1);}bitmap[i] = '1';if((fp=fopen(image_name, "r+b")) == NULL){printf("Can't open file %s\n", image_name);exit(-1);}fseek(fp, i, SEEK_SET);fputc('1', fp);fclose(fp);return i;}ength;add0 = inode_array[num].address[0];if(len > 512)add1 = inode_array[num].address[1];if((fp = fopen(image_name, "r+b")) == NULL){printf("Can't open file %s.\n", image_name);exit(-1);}fseek(fp, BLKSIZE+INODESIZE*INODENUM +add0*BLKSIZE, SEEK_SET);ch = fgetc(fp);for(i=0; (i < len) && (ch != '\0') && (i < 512); i++){temp[i] = ch;ch = fgetc(fp);}if(i >= 512){fseek(fp,BLKSIZE+INODESIZE*INODENUM+add1*BLKSIZE, SEEK_SET);ch = fgetc(fp);for(; (i < len) && (ch != '\0'); i++){temp[i] = ch;ch = fgetc(fp);}}temp[i] = '\0';fclose(fp);}ddress[0];len = inode_array[num].length;if((fp = fopen(image_name, "r+b")) == NULL){printf("Can't open file %s.\n", image_name);exit(-1);}fseek(fp, BLKSIZE+INODESIZE*INODENUM+add0*BLKSIZE, SEEK_SET);for(i=0; (i<len)&&(temp[i]!='\0')&&(i < 512); i++)fputc(temp[i], fp);if(i == 512){add1 = inode_array[num].address[1];fseek(fp, BLKSIZE+INODESIZE*INODENUM+add1*BLKSIZE, SEEK_SET);for(; (i < len) && (temp[i] != '\0'); i++)fputc(temp[i], fp);}fputc('\0', fp);fclose(fp);}num == 0)strcpy(path,;else{strcpy(path,;m=0;n=inum_cur;while(m != inum_cur){while(inode_array[n].iparent != m){n = inode_array[n].iparent;}strcat(path,"/");strcat(path,inode_array[n].file_name);m = n;n = inum_cur;}}printf("[%s]$",path);}或者 cd dir1)void cd(void){int i;if(argc != 2){printf("Command cd must have two args. \n");return ;}if(!strcmp(argv[1], ".."))inum_cur = inode_array[inum_cur].iparent;else{for(i = 0; i < INODENUM; i++)if((inode_array[i].inum>0)&&(inode_array[i].type=='d')&&(inode_array[i].iparent==inum_cur)&& !strcmp(inode_array[i].file_name,argv[1])&&!strcmp(inode_array[i].user_name,)break;if(i == INODENUM)printf("This directory isn't exsited.\n");elseinum_cur = i;}}\n");return ;}num> 0) &&(inode_array[i].iparent == inum_cur)&&!strcmp(inode_array[i].user_name,){if(inode_array[i].type == 'd'){dcount++;printf("%-20s<DIR>\n", inode_array[i].file_name);}else{fcount++;bcount+=inode_array[i].length;printf("%-20s%12d bytes\n", inode_array[i].file_name,inode_array[i].length);}}printf("\n %d file(s)%11d bytes\n",fcount,bcount);printf(" %d dir(s) %11d bytes FreeSpace\n",dcount,1024*1024-bcount);}\n");return ;}num < 0) break;if(i == INODENUM){printf("Inode is full.\n");exit(-1);}inode_array[i].inum = i;strcpy(inode_array[i].file_name, argv[1]);inode_array[i].type = 'd';strcpy(inode_array[i].user_name,;inode_array[i].iparent = inum_cur;inode_array[i].length = 0;save_inode(i);}\n");return ;}for(i = 0; i < INODENUM; i++){if((inode_array[i].inum > 0) &&(inode_array[i].type == 'f') &&!strcmp(inode_array[i].file_name, argv[1])){printf("This file is exsit.\n");return ;}}for(i = 0; i < INODENUM; i++)if(inode_array[i].inum < 0) break;if(i == INODENUM){printf("Inode is full.\n");exit(-1);}inode_array[i].inum = i;strcpy(inode_array[i].file_name, argv[1]);inode_array[i].type = 'f';strcpy(inode_array[i].user_name, ;inode_array[i].iparent = inum_cur;inode_array[i].length = 0;save_inode(i);}\n");return ;}for(i = 0; i < INODENUM; i++)if((inode_array[i].inum > 0) &&(inode_array[i].type == 'f') &&!strcmp(inode_array[i].file_name,argv[1])&&!strcmp(inode_array[i].user_name,)break;if(i == INODENUM){printf("The file you want to open doesn't exsited.\n");return ;}inum = i;printf("Please input open mode:(1: read, 2: write, 3: read and write):");scanf("%d", &mode);getchar();if((mode < 1) || (mode > 3)){printf("Open mode is wrong.\n");return;}for(i = 0; i < FILENUM; i++)if(file_array[i].inum < 0) break;if(i == FILENUM){printf("The file table is full, please close some file.\n");return ;}filenum = i;file_array[filenum].inum = inum;strcpy(file_array[filenum].file_name, inode_array[inum].file_name);file_array[filenum].mode = mode;printf("Open file %s by ", file_array[filenum].file_name);if(mode == 1) printf("read only.\n");else if(mode == 2) printf("write only.\n");else printf("read and write.\n");}\n");return;}for(i = 0; i < FILENUM; i++)if((file_array[i].inum > 0) &&!strcmp(file_array[i].file_name,argv[1]))break;if(i == FILENUM){printf("Open %s first.\n", argv[1]);return ;}else if(file_array[i].mode == 2){printf("Can't read %s.\n", argv[1]);return ;}inum = file_array[i].inum;printf("The length of %s:%d.\n", argv[1], inode_array[inum].length);if(inode_array[inum].length > 0){read_blk(inum);for(i = 0; (i < inode_array[inum].length) && (temp[i] != '\0'); i++) printf("%c", temp[i]);}}\n");return ;}for(i = 0; i < FILENUM; i++)if((file_array[i].inum>0)&&!strcmp(file_array[i].file_name,argv[1])) break;if(i == FILENUM){printf("Open %s first.\n", argv[1]);return ;}else if(file_array[i].mode == 1){printf("Can't write %s.\n", argv[1]);return ;}inum = file_array[i].inum;printf("The length of %s:%d\n", inode_array[inum].file_name, inode_array[inum].length);if(inode_array[inum].length == 0){i=0;inode_array[inum].address[0] = get_blknum();printf("Input the data(CTRL+Z to end):\n");while(i<1023&&(temp[i]=getchar())!=EOF) i++;temp[i]='\0';length=strlen(temp)+1;inode_array[inum].length=length;if(length > 512)inode_array[inum].address[1] = get_blknum();save_inode(inum);write_blk(inum);}elseprintf("This file can't be written.\n");}\n");return ;}for(i = 0; i < FILENUM; i++)if((file_array[i].inum > 0) &&!strcmp(file_array[i].file_name, argv[1])) break;if(i == FILENUM){printf("This file doesn't be opened.\n");return ;}else{file_array[i].inum = -1;printf("Close %s success!\n", argv[1]);}}num = -1;if(inode_array[i].length > 0){release_blk(inode_array[i].address[0]);if(inode_array[i].length >= 512)release_blk(inode_array[i].address[1]);}save_inode(i);}\n");return ;}int n,t,i;stack<int> istk;for(i = 0; i < INODENUM; i++)num >=0) &&(inode_array[i].iparent == inum_cur)&&(!strcmp(inode_array[i].file_name,argv[1]))&&(!strcmp(inode_array[i].user_name,)){n=inode_array[i].inum;break;}if(i==INODENUM) puts("Directory ERROR");else{(n);while(!()){t=();();del(t);for(i = 0; i < INODENUM; i++)if((inode_array[i].inum >=0) &&(inode_array[i].iparent == t)) (i);}}}// 功能: 退出当前用户(logout)void logout(){printf("Do you want to exit this user(y/n)");scanf("%c", &choice);getchar();if((choice == 'y') || (choice == 'Y')){printf("\nCurrent user has exited!\n");login();}return ;}// 功能: 退出文件系统(quit)void quit(){printf("Do you want to exist(y/n):");scanf("%c", &choice);getchar();if((choice == 'y') || (choice == 'Y'))exit(0);}// 功能: 显示错误void errcmd(){printf("Command Error!!!\n");}//清空内存中存在的用户名void free_user(){int i;for(i=0;i<10;i++)[i]='\0';}// 功能: 循环执行用户输入的命令, 直到logout// "help", "cd", "dir", "mkdir", "creat", "open","read", "write", "close", "delete", "logout", "clear", "format","quit"void command(void){char cmd[100];system("cls");do{pathset();gets(cmd);switch(analyse(cmd)){case 0:help(); break;case 1:cd(); break;case 2:dir(); break;case 3:mkdir(); break;case 4:create(); break;case 5:open(); break;case 6:read(); break;case 7:write(); break;case 8:close(); break;case 9:delet(); break;case 10:logout();break;case 11:system("cls");break; case 12:format();init();free_user();login();break;case 13:quit(); break;case 14:errcmd(); break;default:break;}}while(1);}// 主函数int main(void){login();init();command();return 0;}。
操作系统试验—快速文件系统源代码
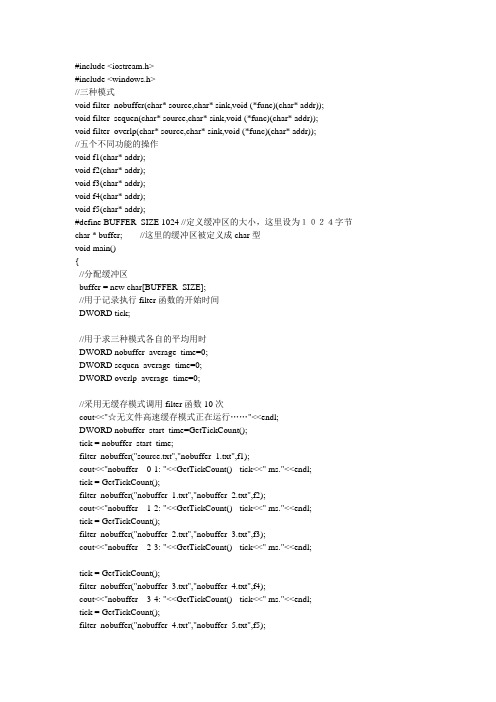
#include <iostream.h>#include <windows.h>//三种模式void filter_nobuffer(char* source,char* sink,void (*func)(char* addr));void filter_sequen(char* source,char* sink,void (*func)(char* addr));void filter_overlp(char* source,char* sink,void (*func)(char* addr));//五个不同功能的操作void f1(char* addr);void f2(char* addr);void f3(char* addr);void f4(char* addr);void f5(char* addr);#define BUFFER_SIZE 1024 //定义缓冲区的大小,这里设为1024字节char * buffer; //这里的缓冲区被定义成char型void main(){//分配缓冲区buffer = new char[BUFFER_SIZE];//用于记录执行filter函数的开始时间DWORD tick;//用于求三种模式各自的平均用时DWORD nobuffer_average_time=0;DWORD sequen_average_time=0;DWORD overlp_average_time=0;//采用无缓存模式调用filter函数10次cout<<"☆无文件高速缓存模式正在运行……"<<endl;DWORD nobuffer_start_time=GetTickCount();tick = nobuffer_start_time;filter_nobuffer("source.txt","nobuffer_1.txt",f1);cout<<"nobuffer 0-1: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_nobuffer("nobuffer_1.txt","nobuffer_2.txt",f2);cout<<"nobuffer 1-2: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_nobuffer("nobuffer_2.txt","nobuffer_3.txt",f3);cout<<"nobuffer 2-3: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_nobuffer("nobuffer_3.txt","nobuffer_4.txt",f4);cout<<"nobuffer 3-4: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_nobuffer("nobuffer_4.txt","nobuffer_5.txt",f5);tick = GetTickCount();filter_nobuffer("nobuffer_5.txt","nobuffer_6.txt",f1);cout<<"nobuffer 5-6: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_nobuffer("nobuffer_6.txt","nobuffer_7.txt",f2);cout<<"nobuffer 6-7: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_nobuffer("nobuffer_7.txt","nobuffer_8.txt",f3);cout<<"nobuffer 7-8: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_nobuffer("nobuffer_8.txt","nobuffer_9.txt",f4);cout<<"nobuffer 8-9: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_nobuffer("nobuffer_9.txt","nobuffer_10.txt",f5);DWORD nobuffer_end_time=GetTickCount();cout<<"nobuffer 9-10: "<<nobuffer_end_time - tick<<" ms."<<endl<<endl; //采用高速缓存模式调用filter函数10次cout<<"★使用文件高速缓存模式正在运行……"<<endl;DWORD sequen_start_time=GetTickCount();tick = sequen_start_time;filter_sequen("source.txt","sequen_1.txt",f1);cout<<"sequen 0-1: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_sequen("sequen_1.txt","sequen_2.txt",f2);cout<<"sequen 1-2: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_sequen("sequen_2.txt","sequen_3.txt",f3);cout<<"sequen 2-3: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_sequen("sequen_3.txt","sequen_4.txt",f4);cout<<"sequen 3-4: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_sequen("sequen_4.txt","sequen_5.txt",f5);cout<<"sequen 4-5: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_sequen("sequen_5.txt","sequen_6.txt",f1);cout<<"sequen 5-6: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_sequen("sequen_6.txt","sequen_7.txt",f2);cout<<"sequen 6-7: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_sequen("sequen_7.txt","sequen_8.txt",f3);tick = GetTickCount();filter_sequen("sequen_8.txt","sequen_9.txt",f4);cout<<"sequen 8-9: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_sequen("sequen_9.txt","sequen_10.txt",f5);DWORD sequen_end_time=GetTickCount();cout<<"sequen 9-10: "<<sequen_end_time - tick<<" ms."<<endl<<endl; //采用异步模式调用filter函数10次cout<<"◎异步传输模式正在运行……"<<endl;DWORD overlp_start_time=GetTickCount();tick = overlp_start_time;filter_overlp("source.txt","overlp_1.txt",f1);cout<<"overlp 0-1: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_overlp("overlp_1.txt","overlp_2.txt",f2);cout<<"overlp 1-2: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_overlp("overlp_2.txt","overlp_3.txt",f3);cout<<"overlp 2-3: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_overlp("overlp_3.txt","overlp_4.txt",f4);cout<<"overlp 3-4: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_overlp("overlp_4.txt","overlp_5.txt",f5);cout<<"overlp 4-5: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_overlp("overlp_5.txt","overlp_6.txt",f1);cout<<"overlp 5-6: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_overlp("overlp_6.txt","overlp_7.txt",f2);cout<<"overlp 6-7: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_overlp("overlp_7.txt","overlp_8.txt",f3);cout<<"overlp 7-8: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_overlp("overlp_8.txt","overlp_9.txt",f4);cout<<"overlp 8-9: "<<GetTickCount() - tick<<" ms."<<endl;tick = GetTickCount();filter_overlp("overlp_9.txt","overlp_10.txt",f5);DWORD overlp_end_time=GetTickCount();cout<<"overlp 9-10: "<<overlp_end_time - tick<<" ms."<<endl<<endl;//输出三种模式下的平均时间以做对比cout<<"■三种模式的平均用时如下:"<<endl;cout<<"·无文件高速缓存模式平均用时:"<<(nobuffer_end_time-nobuffer_start_time)/10<<" ms."<<endl;cout<<"·使用文件高速缓存模式平均用时:"<<(sequen_end_time-sequen_start_time)/10<<" ms."<<endl;cout<<"·异步传输模式平均用时:"<<(overlp_end_time-overlp_start_time)/10<<" ms."<<endl<<endl;return;}//对文件内容进行的5种操作(可以任意定义),本程序仅用了五个很简单的操作//f1 +1//f2 -1//f3 *1//f4 >>//f5 <<void f1(char* addr){ *addr = (unsigned char)*addr + 1; }void f2(char* addr){ *addr = (unsigned char)*addr - 1; }void f3(char* addr){ *addr = (unsigned char)*addr * 1; }void f4(char* addr){ *addr = (unsigned char)*addr >> 1;}void f5(char* addr){ *addr = (unsigned char)*addr << 1;}//没有文件高速缓存的filter函数void filter_nobuffer(char* source, char* sink, void (*func) (char* addr)){HANDLE handle_src,handle_dst; //定义源文件与目标文件的句柄BOOL cycle; //用以判断是否满一个缓冲区DWORD NumberOfBytesRead,NumberOfBytesWrite,index; //读的字节数、写的字节数//打开源文件handle_src=CreateFile(source,GENERIC_READ,NULL,NULL,OPEN_EXISTING,FILE_FLAG _NO_BUFFERING,NULL);//创建目标文件handle_dst = CreateFile(sink,GENERIC_WRITE,NULL,NULL,CREATE_ALWAYS,NULL,NULL);//如果打开或创建失败,则报错if( handle_src == INVALID_HANDLE_VALUE || handle_dst == INVALID_HANDLE_VALUE){cout<<"CreateFile Invocation Error!"<<endl;exit(1);}cycle = TRUE;//用cycle判断文件什么时候读完while(cycle){//从源文件读数据送入缓冲区if(ReadFile(handle_src,buffer,BUFFER_SIZE,&NumberOfBytesRead,NULL) == FALSE){cout<<"ReadFile Error!"<<endl;exit(1);}//当读不满一个缓冲区时,说明达到文件末尾,结束循环if(NumberOfBytesRead < BUFFER_SIZE)cycle = FALSE;//对文件内容进行的操作for(index = 0;index < NumberOfBytesRead;index++)func(&buffer[index]);//将缓冲区中的数据写入目标文件if(WriteFile(handle_dst,buffer,NumberOfBytesRead,&NumberOfBytesWrite,NULL) == FALSE){cout<<"WriteFile Error!"<<endl;exit(1);}}//关闭文件句柄CloseHandle(handle_src);CloseHandle(handle_dst);}void filter_sequen(char* source, char* sink, void (*func) (char* addr)){HANDLE handle_src,handle_dst; //定义源文件与目标文件的句柄BOOL cycle; //用以判断是否满一个缓冲区DWORD NumberOfBytesRead,NumberOfBytesWrite,index; //读的字节数、写的字节数//CreateFile函数设置参数FILE_FLAG_SEQUENTIAL_SCAN:使用文件高速缓存//打开源文件handle_src=CreateFile(source,GENERIC_READ,NULL,NULL,OPEN_EXISTING,FILE_FLAG _SEQUENTIAL_SCAN,NULL);//创建目标文件handle_dst=CreateFile(sink,GENERIC_WRITE,NULL,NULL,CREATE_ALWAYS,FILE_FLAG _SEQUENTIAL_SCAN,NULL);//如果打开或创建失败,则报错if( handle_src == INVALID_HANDLE_VALUE || handle_dst ==INVALID_HANDLE_VALUE){cout<<"CreateFile Invocation Error!"<<endl;exit(1);}cycle = TRUE;//用cycle判断文件什么时候读完while(cycle){//从源文件读数据送入缓冲区if(ReadFile(handle_src,buffer,BUFFER_SIZE,&NumberOfBytesRead,NULL)==FALSE) {cout<<"ReadFile Error!"<<endl;exit(1);}//当读不满一个缓冲区时,说明达到文件末尾,结束循环if(NumberOfBytesRead < BUFFER_SIZE)cycle = FALSE;//对文件内容进行的操作for(index = 0;index < NumberOfBytesRead;index++)func(&buffer[index]);//将缓冲区中的数据写入目标文件if(WriteFile(handle_dst,buffer,NumberOfBytesRead,&NumberOfBytesWrite,NULL) == FALSE){cout<<"WriteFile Error!"<<endl;exit(1);}}//关闭文件句柄CloseHandle(handle_src);CloseHandle(handle_dst);}void filter_overlp(char* source, char* sink, void (*func) (char* addr)){HANDLE handle_src,handle_dst; //定义源文件与目标文件的句柄BOOL cycle; //用以判断是否满一个缓冲区//读的字节数、写的字节数、GetLastError函数的返回值DWORD NumberOfBytesRead,NumberOfBytesWrite,index,dwError; OVERLAPPED overlapped; //overlapped 结构//打开源文件handle_src=CreateFile(source,GENERIC_READ,NULL,NULL,OPEN_EXISTING,FILE_FLAG_NO_BUFFERING | FILE_FLAG_OVERLAPPED,NULL);//创建目标文件handle_dst=CreateFile(sink,GENERIC_WRITE,NULL,NULL,CREATE_ALWAYS,NULL,NUL L);//如果打开或创建失败,则报错if( handle_src == INVALID_HANDLE_VALUE || handle_dst == INVALID_HANDLE_VALUE){cout<<"CreateFile Invocation Error"<<endl;exit(1);}//对overlapped结构初始化overlapped.hEvent=NULL;overlapped.Offset=-BUFFER_SIZE;overlapped.OffsetHigh=0;cycle = TRUE;//用cycle判断文件什么时候读完while(cycle){//计算文件的偏移量overlapped.Offset = overlapped.Offset + BUFFER_SIZE;//读源文件if(ReadFile(handle_src,buffer,BUFFER_SIZE,&NumberOfBytesRead,&overlapped) == FALSE){switch(dwError = GetLastError()){//读到文件结尾case ERROR_HANDLE_EOF:cycle = FALSE;break;//异步传输正在进行case ERROR_IO_PENDING:if(GetOverlappedResult(handle_src,&overlapped,&NumberOfBytesRead,TRUE) == FALSE){cout<<"GetOverlappedResult Error!"<<endl;exit(1);}break;default:break;}}//当读不满一个缓冲区时,说明达到文件末尾,结束循环if(NumberOfBytesRead < BUFFER_SIZE)cycle = FALSE;//对文件内容进行的操作for(index = 0;index < NumberOfBytesRead;index++)func(&buffer[index]);//将缓冲区中的数据写入目标文件if(WriteFile(handle_dst,buffer,NumberOfBytesRead,&NumberOfBytesWrite, NULL) == FALSE){cout<<"WriteFile Error!"<<endl;exit(1);}}//关闭文件句柄CloseHandle(handle_src);CloseHandle(handle_dst);}。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
}
}
m->one=tempone;
}//else if(length<=(beginsize+LENGTH))
else if(length<=(beginsize+LENGTH+LENGTH*LENGTH))
{//当用二级索引时候
for (k=0;k<beginsize;k++)
{
m->begin[k]=-1;
}
m->one=NULL;
m->two=NULL;
m->three=NULL;
if(length<=beginsize)
{//直接地址够用时候
for(k=0;k<length;k++)
for(k=0;k<beginsize;k++)
{//直接的存储上
for(i=0;i<16;i++)
{
for(j=0;j<16;j++)
{
if(Bitmap[i][j]==0)
{
m->begin[k]=i*16+j;
int type;//是文件还是目录
int size;//如果是文件给出大小
struct NODE *next;//兄弟结点
struct NODE * sub;//子节点
struct NODE * father;//父亲节点
Mixtab * table;
}FCB;
//文件控制块
temp->size=0;
Addpoint(temp);
}
}
//创建文件
void Mkfile()
{//处理二级时候有错误
char listname[50];
int length,i,j,k,leg=0;
cin>>listname;
FCB * temp;
if(getchar()=='\n')
tempone->data[k]=i*16+j;
Bitmap[i][j]=1;
leg=1;
break;
}
}
if(leg==1)
{
leg=0;
break;
}
}
}
leg=0;
Indireone * tempone=(Indireone *)malloc(sizeof (Indireone));
for (k=0;k<LENGTH;k++)
{
tempone->data[k]=-1;
{
//
for(i=0;i<16;i++)
{
for(j=0;j<16;j++)
{
if(Bitmap[i][j]==0)
{
m->begin[k]=i*16+j;
Bitmap[i][j]=1;
}
for(k=0;k<(length-beginsize);k++)
{//一级索引分配
for(i=0;i<16;i++)
{
for(j=0;j<16;j++)
{
if(Bitmap[i][j]==0)
{
leg=1;
break;
}
}
if(leg==1)
{
leg=0;
break;
}
}
}
}//if(length<=beginsize)
else if(length<=(beginsize+LENGTH))
root->size=0;
root->next=NULL;
root->father=root;
root->sub=NULL;
for(i=0;i<16;i++)
{
for(j=0;j<16;j++)
{
if(Bitmap[i][j]==0)
{
leftbit++;
temp=present->sub;
while(temp!=NULL)
{
if((!strcmp(temp->name,ary))&&(temp->type==x))
{
finding=temp;
return 1;
}
temp=temp->next;
for(i=0;i<16;i++)
{//初始化位示图
for(j=0;j<16;j++)
{
Bitmap[i][j]=rand()%2;
}
}
root=(FCB *)malloc(sizeof(FCB));
strcpy(root->name,"\\");
root->type=0;
#include<iostream>
#include<stdlib.h>
#include<time.h>
#include <locale.h>
//#include<string>
using namespace std;
//
#define beginsize 5
#define LENGTH 3
{//用一级索引的时候
for(k=0;k<beginsize;k++)
{//直接的存储上
for(i=0;i<16;i++)
{
for(j=0;j<16;j++)
{
if(Bitmap[i][j]==0)
{
void Delpoint(FCB *f)
{
FCB * temp=present->sub;
if(temp==f)
{
present->sub=temp->next;
delete(f);
}
else
{
while(temp->next!=f)
{
temp=temp->next;
//利用交互式命令实现树型目录结构和文件管理,同时利用位示图表示外存的分配情况,新建文件时分配必要的空间,模拟文件分配表记录文件在外存上的存储方式。了解系统对文件的操作。
//在文件中保存目录内容,创建文件或子目录可以用命令行命令:MD、CD、RD、MK(创建文件)、DEL(删除文件)和DIR
}
temp->next=f->next;
delete(f);
}
}
//查找是不是已经存在
int Isexist(char ary[],int x)
{
FCB * temp;
if(present->sub==NULL)
{
return 0;
}
else
{
}
}
}
}
//判断分配外存时候是不是足够
int Judgeenough(int n)
{
if(leftbit>=n)
return 1;
else return 0;
}
//添加时候用
void Addpoint(FCB * f)
{
FCB * temp;
if(present->sub==NULL)
m->begin[k]=i*16+j;
Bitmap[i][j]=1;
leg=1;
break;
}
}
if(leg==1)
{
leg=0;
break;
}Indirethree;
typedef struct Node
{
int begin[beginsize];
Indireone * one;
Indiretwo * two;
Indirethree * three;
}Mixtab;
typedef struct NODE { Biblioteka char name[50];
Indireone * tempone=(Indireone *)malloc(sizeof (Indireone));
for(k=0;k<LENGTH;k++)
{//一级索引分配
for(i=0;i<16;i++)
{
for(j=0;j<16;j++)
}
return 0;
}
}
//创建目录
void Mdlist()
{
char listname[50];
cin>>listname;
FCB * temp;
if(Isexist(listname,0))
{
cout<<"子目录或文件"<<listname<<"已存在。"<<endl;