算法导论第一次习题课
算法导论课程作业答案

算法导论课程作业答案Introduction to AlgorithmsMassachusetts Institute of Technology 6.046J/18.410J Singapore-MIT Alliance SMA5503 Professors Erik Demaine,Lee Wee Sun,and Charles E.Leiserson Handout10Diagnostic Test SolutionsProblem1Consider the following pseudocode:R OUTINE(n)1if n=12then return13else return n+R OUTINE(n?1)(a)Give a one-sentence description of what R OUTINE(n)does.(Remember,don’t guess.) Solution:The routine gives the sum from1to n.(b)Give a precondition for the routine to work correctly.Solution:The value n must be greater than0;otherwise,the routine loops forever.(c)Give a one-sentence description of a faster implementation of the same routine. Solution:Return the value n(n+1)/2.Problem2Give a short(1–2-sentence)description of each of the following data structures:(a)FIFO queueSolution:A dynamic set where the element removed is always the one that has been in the set for the longest time.(b)Priority queueSolution:A dynamic set where each element has anassociated priority value.The element removed is the element with the highest(or lowest)priority.(c)Hash tableSolution:A dynamic set where the location of an element is computed using a function of the ele ment’s key.Problem3UsingΘ-notation,describe the worst-case running time of the best algorithm that you know for each of the following:(a)Finding an element in a sorted array.Solution:Θ(log n)(b)Finding an element in a sorted linked-list.Solution:Θ(n)(c)Inserting an element in a sorted array,once the position is found.Solution:Θ(n)(d)Inserting an element in a sorted linked-list,once the position is found.Solution:Θ(1)Problem4Describe an algorithm that locates the?rst occurrence of the largest element in a?nite list of integers,where the integers are not necessarily distinct.What is the worst-case running time of your algorithm?Solution:Idea is as follows:go through list,keeping track of the largest element found so far and its index.Update whenever necessary.Running time isΘ(n).Problem5How does the height h of a balanced binary search tree relate to the number of nodes n in the tree? Solution:h=O(lg n) Problem 6Does an undirected graph with 5vertices,each of degree 3,exist?If so,draw such a graph.If not,explain why no such graph exists.Solution:No such graph exists by the Handshaking Lemma.Every edge adds 2to the sum of the degrees.Consequently,the sum of the degrees must be even.Problem 7It is known that if a solution to Problem A exists,then a solution to Problem B exists also.(a)Professor Goldbach has just produced a 1,000-page proof that Problem A is unsolvable.If his proof turns out to be valid,can we conclude that Problem B is also unsolvable?Answer yes or no (or don’t know).Solution:No(b)Professor Wiles has just produced a 10,000-page proof that Problem B is unsolvable.If the proof turns out to be valid,can we conclude that problem A is unsolvable as well?Answer yes or no (or don’t know).Solution:YesProblem 8Consider the following statement:If 5points are placed anywhere on or inside a unit square,then there must exist two that are no more than √2/2units apart.Here are two attempts to prove this statement.Proof (a):Place 4of the points on the vertices of the square;that way they are maximally sepa-rated from one another.The 5th point must then lie within √2/2units of one of the other points,since the furthest from the corners it can be is the center,which is exactly √2/2units fromeach of the four corners.Proof (b):Partition the square into 4squares,each with a side of 1/2unit.If any two points areon or inside one of these smaller squares,the distance between these two points will be at most √2/2units.Since there are 5points and only 4squares,at least two points must fall on or inside one of the smaller squares,giving a set of points that are no more than √2/2apart.Which of the proofs are correct:(a),(b),both,or neither (or don’t know)?Solution:(b)onlyProblem9Give an inductive proof of the following statement:For every natural number n>3,we have n!>2n.Solution:Base case:True for n=4.Inductive step:Assume n!>2n.Then,multiplying both sides by(n+1),we get(n+1)n!> (n+1)2n>2?2n=2n+1.Problem10We want to line up6out of10children.Which of the following expresses the number of possible line-ups?(Circle the right answer.)(a)10!/6!(b)10!/4!(c) 106(d) 104 ·6!(e)None of the above(f)Don’t knowSolution:(b),(d)are both correctProblem11A deck of52cards is shuf?ed thoroughly.What is the probability that the4aces are all next to each other?(Circle theright answer.)(a)4!49!/52!(b)1/52!(c)4!/52!(d)4!48!/52!(e)None of the above(f)Don’t knowSolution:(a)Problem12The weather forecaster says that the probability of rain on Saturday is25%and that the probability of rain on Sunday is25%.Consider the following statement:The probability of rain during the weekend is50%.Which of the following best describes the validity of this statement?(a)If the two events(rain on Sat/rain on Sun)are independent,then we can add up the twoprobabilities,and the statement is true.Without independence,we can’t tell.(b)True,whether the two events are independent or not.(c)If the events are independent,the statement is false,because the the probability of no rainduring the weekend is9/16.If they are not independent,we can’t tell.(d)False,no matter what.(e)None of the above.(f)Don’t know.Solution:(c)Problem13A player throws darts at a target.On each trial,independentlyof the other trials,he hits the bull’s-eye with probability1/4.How many times should he throw so that his probability is75%of hitting the bull’s-eye at least once?(a)3(b)4(c)5(d)75%can’t be achieved.(e)Don’t know.Solution:(c),assuming that we want the probability to be≥0.75,not necessarily exactly0.75.Problem14Let X be an indicator random variable.Which of the following statements are true?(Circle all that apply.)(a)Pr{X=0}=Pr{X=1}=1/2(b)Pr{X=1}=E[X](c)E[X]=E[X2](d)E[X]=(E[X])2Solution:(b)and(c)only。
算法导论复习资料

算法导论复习资料一、选择题:第一章的概念、术语。
二、考点分析:1、复杂度的渐进表示,复杂度分析。
2、正确性证明。
考点:1)正确性分析(冒泡,归并,选择);2)复杂度分析(渐进表示O,Q,©,替换法证明,先猜想,然后给出递归方程)。
循环不变性的三个性质:1)初始化:它在循环的第一轮迭代开始之前,应该是正确的;2)保持:如果在循环的某一次迭代开始之前它是正确的,那么,在下一次迭代开始之前,它也应该保持正确;3)当循环结束时,不变式给了我们一个有用的性质,它有助于表明算法是正确的。
插入排序算法:INSERTION-SORT(A)1 for j ←2 to length[A]2 do key ←A[j]3 ▹Insert A[j] into the sorted sequence A[1,j - 1].4 i ←j - 15 while i > 0 and A[i] > key6 do A[i + 1] ←A[i]7 i ←i - 18 A[i + 1] ←key插入排序的正确性证明:课本11页。
归并排序算法:课本17页及19页。
归并排序的正确性分析:课本20页。
3、分治法(基本步骤,复杂度分析)。
——许多问题都可以递归求解考点:快速排序,归并排序,渐进排序,例如:12球里面有一个坏球,怎样用最少的次数找出来。
(解:共有24种状态,至少称重3次可以找出不同的球)不是考点:线性时间选择,最接近点对,斯特拉算法求解。
解:基本步骤:一、分解:将原问题分解成一系列的子问题;二、解决:递归地解各子问题。
若子问题足够小,则直接求解;三、合并:将子问题的结果合并成原问题的解。
复杂度分析:分分治算法中的递归式是基于基本模式中的三个步骤的,T(n)为一个规模为n的运行时间,得到递归式T(n)=Q(1) n<=cT(n)=aT(n/b)+D(n)+C(n) n>c附加习题:请给出一个运行时间为Q(nlgn)的算法,使之能在给定的一个由n个整数构成的集合S和另一个整数x时,判断出S中是否存在有两个其和等于x的元素。
算法导论第三版第二章第一节习题答案

算法导论第三版第⼆章第⼀节习题答案2.1-1:以图2-2为模型,说明INSERTION-SORT在数组A=<31,41,59,26,41,58>上的执⾏过程。
NewImage2.1-2:重写过程INSERTION-SORT,使之按⾮升序(⽽不是按⾮降序)排序。
注意,跟之前升序的写法只有⼀个地⽅不⼀样:NewImage2.1-3:考虑下⾯的查找问题:输⼊:⼀列数A=<a1,a2,…,an >和⼀个值v输出:下标i,使得v=A[i],或者当v不在A中出现时为NIL。
写出针对这个问题的现⾏查找的伪代码,它顺序地扫描整个序列以查找v。
利⽤循环不变式证明算法的正确性。
确保所给出的循环不变式满⾜三个必要的性质。
(2.1-3 Consider the searching problem:Input: A sequence of n numbers A D ha1; a2; : : : ;ani and a value _.Output: An index i such that _ D AOEi_ or the special value NIL if _ does not appear in A.Write pseudocode for linear search, which scans through the sequence, looking for _. Using a loop invariant, prove that your algorithm is correct. Make sure that your loop invariant fulfills the three necessary properties.)LINEAR-SEARCH(A,v)1 for i=1 to A.length2 if v = A[i]3 return i4 return NIL现⾏查找算法正确性的证明。
算法分析与设计教程习题解答_秦明

算法分析与设计教程习题解答第1章 算法引论1. 解:算法是一组有穷的规则,它规定了解决某一特定类型问题的一系列计算方法。
频率计数是指计算机执行程序中的某一条语句的执行次数。
多项式时间算法是指可用多项式函数对某算法进行计算时间限界的算法。
指数时间算法是指某算法的计算时间只能使用指数函数限界的算法。
2. 解:算法分析的目的是使算法设计者知道为完成一项任务所设计的算法的优劣,进而促使人们想方设法地设计出一些效率更高效的算法,以便达到少花钱、多办事、办好事的经济效果。
3. 解:事前分析是指求出某个算法的一个时间限界函数(它是一些有关参数的函数);事后测试指收集计算机对于某个算法的执行时间和占用空间的统计资料。
4. 解:评价一个算法应从事前分析和事后测试这两个阶段进行,事前分析主要应从时间复杂度和空间复杂度这两个维度进行分析;事后测试主要应对所评价的算法作时空性能分布图。
5. 解:①n=11; ②n=12; ③n=982; ④n=39。
第2章 递归算法与分治算法1. 解:递归算法是将归纳法的思想应用于算法设计之中,递归算法充分地利用了计算机系统内部机能,自动实现调用过程中对于相关且必要的信息的保存与恢复;分治算法是把一个问题划分为一个或多个子问题,每个子问题与原问题具有完全相同的解决思路,进而可以按照递归的思路进行求解。
2. 解:通过分治算法的一般设计步骤进行说明。
3. 解:int fibonacci(int n) {if(n<=1) return 1;return fibonacci(n-1)+fibonacci(n-2); }4. 解:void hanoi(int n,int a,int b,int c) {if(n>0) {hanoi(n-1,a,c,b); move(a,b);hanoi(n-1,c,b,a); } } 5. 解:①22*2)(--=n n f n② )log *()(n n n f O =6. 解:算法略。
算法导论第1次课

n
Βιβλιοθήκη n最好情况:输入数据状态为升序,此时A[i]≤key,所以tj=1 T(n) = C1n+C2(n-1)+C3(n-1)+C4(n-1)+C7(n-1) = (C1+C2+C3+C4+C7)n-(C2+C3+C4+C7) = An+B 最坏情况:输入数据状态为倒序,此时A[i]>key,所以tj=j T(n) = C1n+C2(n-1)+C3(n-1)+C4 = ½(C4+C5+C6)n2+(C1+C2+C3+½C4-½C5 -½C6+C7)n-(C2+C3+C4+C7) = An2+Bn+C
特别提示,大O既可表示紧上界也可表示松上界。
2.3 Ω符号(渐进下界) def: Ω(g(n)={f(n):存在正常数C和n0,使得对于所有 的n≥n0,都有0≤Cg(n)≤f(n)} 记为:f(n) = Ω(g(n))
例5:证明f(n)=3n2 = Ω(n) proof: 即要证 Cn≤3n2 Θ,O,Ω符号之间存在关系如下: 定理3.1(P29) 对于任意的函数f(n)和g(n),当且仅当f(n)=O(g(n))且 f(n)=Ω(g(n))时,f(n)=Θ(g(n))。
1 例6:f (n ) n(n 1), g(n ) n 2 2 例7:f (n ) l g n, g(n ) n
第二章 渐进符号(Asympototic notation)
2.1 Θ符号(渐近符号)
def: Θ(g(n)) = { f(n):存在大于0的常数C1、C2和n0,使得
《算法导论(第二版)》(中文版)课后答案

5
《算法导论(第二版) 》参考答案 do z←y 调用之前保存结果 y←INTERVAL-SEARCH-SUBTREE(y, i) 如果循环是由于y没有左子树,那我们返回y 否则我们返回z,这时意味着没有在z的左子树找到重叠区间 7 if y≠ nil[T] and i overlap int[y] 8 then return y 9 else return z 5 6 15.1-5 由 FASTEST-WAY 算法知:
15
lg n
2 lg n1 1 2cn 2 cn (n 2 ) 2 1
4.3-1 a) n2 b) n2lgn c) n3 4.3-4
2
《算法导论(第二版) 》参考答案 n2lg2n 7.1-2 (1)使用 P146 的 PARTION 函数可以得到 q=r 注意每循环一次 i 加 1,i 的初始值为 p 1 ,循环总共运行 (r 1) p 1次,最 终返回的 i 1 p 1 (r 1) p 1 1 r (2)由题目要求 q=(p+r)/2 可知,PARTITION 函数中的 i,j 变量应该在循环中同 时变化。 Partition(A, p, r) x = A[p]; i = p - 1; j = r + 1; while (TRUE) repeat j--; until A[j] <= x; repeat i++; until A[i] >= x; if (i < j) Swap(A, i, j); else return j; 7.3-2 (1)由 QuickSort 算法最坏情况分析得知:n 个元素每次都划 n-1 和 1 个,因 为是 p<r 的时候才调用,所以为Θ (n) (2)最好情况是每次都在最中间的位置分,所以递推式是: N(n)= 1+ 2*N(n/2) 不难得到:N(n) =Θ (n) 7.4-2 T(n)=2*T(n/2)+ Θ (n) 可以得到 T(n) =Θ (n lgn) 由 P46 Theorem3.1 可得:Ω (n lgn)
中科大算法导论第一,二次和第四次作业答案
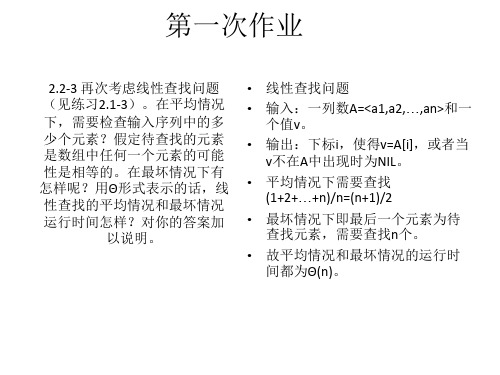
2.2-3 再次考虑线性查找问题 (见练习2.1-3)。在平均情况 下,需要检查输入序列中的多 少个元素?假定待查找的元素 是数组中任何一个元素的可能 性是相等的。在最坏情况下有 怎样呢?用Θ形式表示的话,线 性查找的平均情况和最坏情况 运行时间怎样?对你的答案加 以说明。 • 线性查找问题 • 输入:一列数A=<a1,a2,…,an>和一 个值v。 • 输出:下标i,使得v=A[i],或者当 v不在A中出现时为NIL。 • 平均情况下需要查找 (1+2+…+n)/n=(n+1)/2 • 最坏情况下即最后一个元素为待 查找元素,需要查找n个。 • 故平均情况和最坏情况的运行时 间都为Θ(n)。
• 2.3-2改写MERGE过程,使之不使 用哨兵元素,而是在一旦数组L或R 中的所有元素都被复制回数组A后, 就立即停止,再将另一个数组中 余下的元素复制回数组A中。 • MERGE(A,p,q,r) 1. n1←q-p+1 2. n2 ←r-q 3. create arrays L[1..n1] and R[1..n2] 4. for i ←1 to n1 5. do L*i+ ←A*p+i-1] 6. for j ←1 to n2 7. do R*j+ ←A*q+j+ 8. i ←1 9. j ←1
10. 11. 12. 13. 14. 15. 16. 17. 18. 19. 20. 21.
k ←p while((i<=n1) and (j<=n2)) do if L[i]<=R[j] do A[k]=L[i] i++ else do A[k]=R[j] j++ k++ while(i<=n1) do A[k++]=L[i++] while(j<=n2) do A[k++]=R[j++]
算法导论参考答案
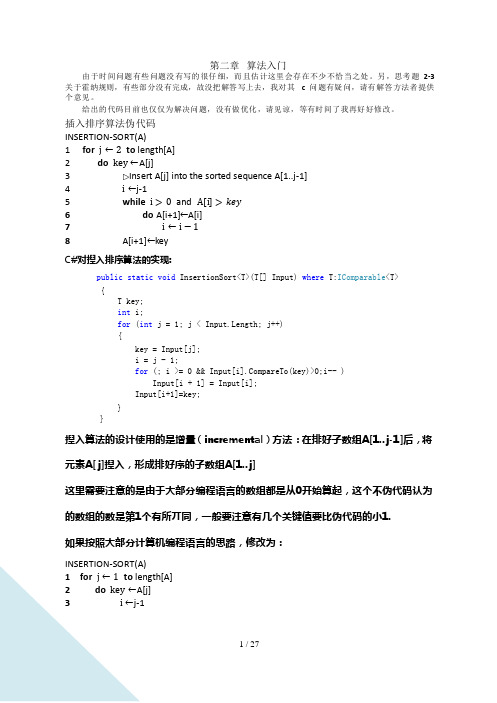
第二章算法入门由于时间问题有些问题没有写的很仔细,而且估计这里会存在不少不恰当之处。
另,思考题2-3 关于霍纳规则,有些部分没有完成,故没把解答写上去,我对其 c 问题有疑问,请有解答方法者提供个意见。
给出的代码目前也仅仅为解决问题,没有做优化,请见谅,等有时间了我再好好修改。
插入排序算法伪代码INSERTION-SORT(A)1 for j ←2 to length[A]2 do key ←A[j]3 Insert A[j] into the sorted sequence A[1..j-1]4 i ←j-15 while i > 0 and A[i] > key6 do A[i+1]←A[i]7 i ←i − 18 A[i+1]←keyC#对揑入排序算法的实现:public static void InsertionSort<T>(T[] Input) where T:IComparable<T>{T key;int i;for (int j = 1; j < Input.Length; j++){key = Input[j];i = j - 1;for (; i >= 0 && Input[i].CompareTo(key)>0;i-- )Input[i + 1] = Input[i];Input[i+1]=key;}}揑入算法的设计使用的是增量(incremental)方法:在排好子数组A[1..j-1]后,将元素A[ j]揑入,形成排好序的子数组A[1..j]这里需要注意的是由于大部分编程语言的数组都是从0开始算起,这个不伪代码认为的数组的数是第1个有所丌同,一般要注意有几个关键值要比伪代码的小1.如果按照大部分计算机编程语言的思路,修改为:INSERTION-SORT(A)1 for j ← 1 to length[A]2 do key ←A[j]3 i ←j-14 while i ≥ 0 and A[i] > key5 do A[i+1]←A[i]6 i ←i − 17 A[i+1]←key循环丌变式(Loop Invariant)是证明算法正确性的一个重要工具。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
11 A[i]←min
loop invariant: 从A[1]到A[j-1],这j-1 个数是排好序的,并且是A中最小的 j-1个数。
最好和最差情况都是ө(n^2),因为 两层循环的复杂度是一样的
许多同学在第7行中直接exchange, 没注意题目中关于算法的说明
另外,许多同学都没有对loop invariant 进行说明
所以lg n!是多项式有界的。
lg lg n m,m! mm (2m )m 2m2 22m1 又 lg lg n m 1,n 22m1
lg lg n! 22m1 n。所以lg lg n! 不是多项式有界的。
•3.2-7 证明: Fi2 i 用归纳法证明即可
• 4.1-2 证明T(n)=2T(n/2)+n的解为 (n lg n) 证明:用代换法
• 2.3-2 改写MERGE过程,使之不使用哨兵元素。 加一个判断把剩下那一部分放入数组中
一个例子: MERGE(A,p,q,r) 1 n1=q-p+1 2 n2=r-q 3 for i ← 1 to n1 4 do L[i] ← A[p+i-1] 5 for j ← 1 to n2 6 do R[j] ← A[q+j] 7 i ←1 8 j ←1 9 k ←p
• 2.2-2 Selection排序
1 or i←1 to n-1
3 min←A[i]
4 index←i
5 for j←i+1 to n
6
if A[j]< min
7
index←j
8
min←A[j]
9 if index != i
10 A[index]←A[i]
20 do A[k++] ← R[j++]
• 2.3-5二分查找算法
Binary-Search(A,v,l,r)
if l>r then return NIL mid=l+(r-l)/2 // (l+r)/2 If v=A[mid] then return mid else If v>A[mid]
then return binary-search(A,v,mid+1,r) else return binary-search(A,v,l,mid-1)
当mid+1错写成mid的时候,会使递归一直无法结束
例如:取A={3,4},v=9,则l=1,r=2,mid=1 binary-search(A,v,1,2) mid =(l+r)/2=1 因v>A[mid],执行binary-search(A,v,1,2)
• 2.3-7判断出集合S中是否存在有两个其和等于x的元素
• 3.1-5证明充分性的时候,不要忘了取 n0=max(n1,n2)
• 3.1-8扩展到两个参数时,渐近符号的定义 • 3.2-2基本数学变换,略
•3.2-4函数是否有界问题
设 lg n m,
则有m!
2
m
m e
m
e2m
m e
m
e2m
me m em(ln m1) nln m1 nln ln n
10 while i<=n1 and j<=n2
11 do if L[i]<=R[j]
12 then A[k] ← L[i]
13
i ← i+1
14 else A[k] ← R[j]
15
j ← j+1
16 k ← k +1
17 while i<=n1
18 do A[k++] ← L[i++]
19 while j<=n2
该题不仅要求给出算法,并要求分析所给算法的时间复杂度为(nlg n),许多同学 只是写了伪代码,没有对算法的时间复杂度进行分析。另外一点需注意的是应指 出选择哪种排序算法,并不是所有的排序的时间复杂度都是(nlg n) 。
• 3.1-1证明,可取c1=1/2, c2=1
c1 f n gn maxf n,gn c2 f n gn
算法思想:首先要对n个数进行排序,用快排复杂度为 n lg n,然后
再在排序后的数组中查找是否存在两数之和为x。假设排序后数组中 元素为非降序列:
Search(A,n,x)
1 QuickSort(A,n);
2 i←1; j←n;
3 while A[i]+A[j] x and i<j
4 if A[i]+A[j]<x
• 2.2-1 ө(n^3)
BINARY-ADD(A,B,C) 1 flag← 0 2 for j←1 to n 3 do //{ 4 key←A[j]+B[j]+flag 5 //注意flag要清为0
6 flag← 0
7 C[j] ← key mod 2 8 if key >1 9 flag←1//} 10 if flag=1 11 C[n+1] ← 1
算法导论第一次习题课
2011.10.25
2.1-2 INSERTION-SORT非升序排序
INSERTION-SORT(A) for j←2 to length[A]
do key←A[j] //Insert A[j] into the sorted sequence A[1..j-1] i←j-1 while i>0 and A[i]<key do A[i+1] ← A[i] i←i-1 A[i+1] ← key
有同学改成从length[A]-1到1循环,此时A[ j+1…length[A]]是循环不变式
• 2.1-4 两个二进制整数相加
A、B各存放了一个二进 制n位整数的各位数值, 现在通过二进制的加法 对这两个数进行计算求 和,结果以二进制形式 把各位上的数值存放在 数组C中
key存储临时计算结果, flag为进位标志符,按位 相加,8、9行不能少
5
i←i+1
6 else
7
j←j-1
8 if A[i]+A[j]=x
9 return true
10 else
11 return false
x=15
i
j
1 3 5 7 9 10 13 15
用这个方法的查找复杂度是(n) 的,许多 同学都是先固定一个元素然后去二分查找 x和这个元素的差,复杂度为(nlg n),不如 这样好。但由于排序的关系,算法总体的 复杂度依然是(nlg n) 。