学生成绩信息的管理
班级学生考试成绩管理规定

班级学生考试成绩管理规定一、引言在学校教育中,考试成绩是评价学生学习成绩的重要依据,也是激励学生提高学习能力的一种手段。
班级学生考试成绩管理规定旨在建立公平、公正、科学的考试评价体系,促进学生全面发展。
二、考试的设置为了全面评价学生的学习情况,班级设置了定期考试和小测验。
定期考试包括期中考试和期末考试,小测验则分为课堂测验和作业测验。
定期考试主要考察学生对学期内容的掌握情况,小测验则着重检验学生的学习进度和理解能力。
三、成绩的计算班级成绩以百分制计算,将学生在各类考试和测验中获得的分数进行加权平均。
其中,定期考试的权重较高,小测验的权重较低。
这样的设计能够充分反映学生在整个学期中的学习情况,避免只重视一次考试的结果。
四、成绩分布和评价为了评价学生在班级内的位置和水平,成绩分布采用成绩段的方式,将成绩按照固定的比例划分为较优、优秀、良好、及格和不及格等不同等级。
同时,针对学科特点,可以设置特殊奖励,例如对于成绩突出的学生可以给予表扬或奖励,鼓励学生们积极学习。
五、成绩公示为了保证透明公正,班级成绩将在一定时间内公示,让家长和学生对自己的学习情况有清晰的了解。
公示期间,家长可以与班主任进行交流,及时解决学生成绩方面的问题。
同时,班主任也会根据学生的成绩情况,制定相应的学习计划,帮助学生提高学习能力。
六、成绩讨论和反思为了提高班级整体学习水平,班级定期组织成绩讨论和反思活动。
在这些活动中,学生可以分享自己的学习方法和经验,互相学习借鉴,共同进步。
同时,班主任也会总结学生的共性问题,并提出解决方案,为学生指导学习方法。
七、监督和自查为了确保成绩的准确性和公正性,班级设立了监督和自查制度。
班主任会定期抽查学生试卷,核对成绩的计算过程和结果。
同时,为了避免学生作弊,班级也会采取相应的反作弊措施,例如随机座位、考场监控等,确保考试的公平性。
八、成绩记录和报告班级会为每个学生建立成绩档案,记录学生的考试成绩和评价。
中小学学生数据报送与管理的制度

中小学学生数据报送与管理的制度
1. 目的和意义
为了更好地满足教育管理的需求,提高教育质量,确保学生信
息的准确性、完整性和安全性,特制定本制度,规范中小学学生数
据的报送与管理。
2. 数据报送内容
中小学学生数据报送内容主要包括:学生基本信息、学业成绩、德育评价、体质健康、教师教学情况等相关数据。
3. 数据报送流程
3.1 数据收集
各学校应在每学期开始前,对学生的基本信息、学业成绩等进
行收集,确保数据的准确性。
3.2 数据审核
学校应对收集到的数据进行审核,确保数据的完整性和准确性。
3.3 数据报送
学校应按照教育行政部门的要求,定期将审核后的学生数据报
送至上级教育行政部门。
3.4 数据反馈
教育行政部门应对学校报送的数据进行审核,对发现的问题应
及时反馈给学校,并要求学校进行修正。
4. 数据管理
4.1 数据安全
学校应加强对学生数据的保护,防止数据泄露、损毁、丢失等,确保学生数据的安全。
4.2 数据更新
学校应定期对学生的数据进行更新,确保数据的时效性。
4.3 数据使用
学校应对学生数据进行合理使用,不得泄露学生个人隐私,不得用于非法用途。
5. 违规处理
对于违反本制度的行为,教育行政部门将依法依规进行处理,对直接负责的主管人员和其他直接责任人员依法依规给予处分。
6. 附则
本制度自发布之日起实施,如有未尽事宜,由教育行政部门另行规定。
---
以上就是中小学学生数据报送与管理的制度,希望各学校认真执行,共同维护学生数据的管理工作。
管理制度:中小学校的学生信息报送

管理制度:中小学校的学生信息报送1. 目的为了加强中小学校学生信息管理,确保学生信息准确、及时地上报,提高学校管理效率,根据相关法律法规,特制定本管理制度。
2. 适用范围本管理制度适用于我国所有中小学校的学生信息报送工作。
3. 学生信息内容学生信息包括基本信息、学业成绩、行为表现等方面,具体如下:- 基本信息:包括学生姓名、性别、出生日期、民族、身份证号码、家庭住址、联系方式等;- 学业成绩:包括各科目成绩、综合素质评价等;- 行为表现:包括奖励、惩罚等情况。
4. 信息报送流程4.1 信息收集各中小学校应指定专人负责学生信息的收集工作,确保信息准确、完整。
4.2 信息审核收集到的学生信息需经过相关部门审核,确保信息无误。
4.3 信息报送审核无误的学生信息,由学校指定的报送人员按照规定的格式和要求,报送至上级教育行政部门。
4.4 信息反馈上级教育行政部门收到学生信息后,应对报送情况进行反馈,对存在的问题要求学校及时整改。
5. 报送时间及频率学生信息报送时间及频率如下:- 每学期末进行一次全面的学生信息报送;- 如有特殊情况,上级教育行政部门可要求学校进行即时报送。
6. 保障措施6.1 人员保障各中小学校应确保有专人负责学生信息报送工作,并定期进行培训,提高业务水平。
6.2 技术保障学校应配备必要的计算机、打印机等办公设备,确保学生信息报送工作的顺利进行。
6.3 制度保障学校应将学生信息报送工作纳入常规管理,建立健全相关制度,确保工作落实到位。
7. 违规处理对于未按照规定要求进行学生信息报送的学校,上级教育行政部门将进行通报批评,并视情节严重程度,对相关责任人进行追责。
8. 附则本管理制度自发布之日起实施,原有相关规定与本管理制度不符的,以本管理制度为准。
如有未尽事宜,可根据实际情况予以补充。
{content}。
学生成绩管理系统数据流程图及数据字典

学生成绩管理系统数据流程图及数据字典一、数据流程图学生成绩管理系统是一个用于管理学生的学习成绩的系统。
下面是该系统的数据流程图:1. 学生信息录入流程:- 学生信息管理员将学生的基本信息录入系统中,包括学生的姓名、学号、班级等。
- 系统生成一个惟一的学生ID,并将学生ID与学生的基本信息关联起来。
- 学生信息管理员将学生的课程信息录入系统中,包括课程名称、课程代码等。
- 系统生成一个惟一的课程ID,并将课程ID与课程信息关联起来。
2. 成绩录入流程:- 教师登录系统后,选择要录入成绩的课程。
- 系统显示该课程下的所有学生列表。
- 教师选择要录入成绩的学生,并输入学生的成绩。
- 系统将成绩与学生ID和课程ID关联起来,并存储在数据库中。
3. 成绩查询流程:- 学生登录系统后,选择要查询成绩的课程。
- 系统显示该课程下的该学生的成绩。
4. 成绩统计流程:- 教师登录系统后,选择要统计成绩的课程。
- 系统显示该课程下的所有学生列表及其成绩。
- 教师可以选择按照成绩排序,计算平均成绩等。
二、数据字典下面是学生成绩管理系统的数据字典,包括实体和属性的定义:1. 学生(Student)实体:- 学生ID(StudentID):惟一标识学生的ID。
- 姓名(Name):学生的姓名。
- 学号(StudentNumber):学生的学号。
- 班级(Class):学生所在的班级。
2. 课程(Course)实体:- 课程ID(CourseID):惟一标识课程的ID。
- 课程名称(CourseName):课程的名称。
- 课程代码(CourseCode):课程的代码。
3. 成绩(Grade)实体:- 学生ID(StudentID):学生的ID。
- 课程ID(CourseID):课程的ID。
- 成绩(Score):学生在该课程中的成绩。
4. 用户(User)实体:- 用户ID(UserID):惟一标识用户的ID。
- 用户名(Username):用户的用户名。
学生成绩管理系统设计报告
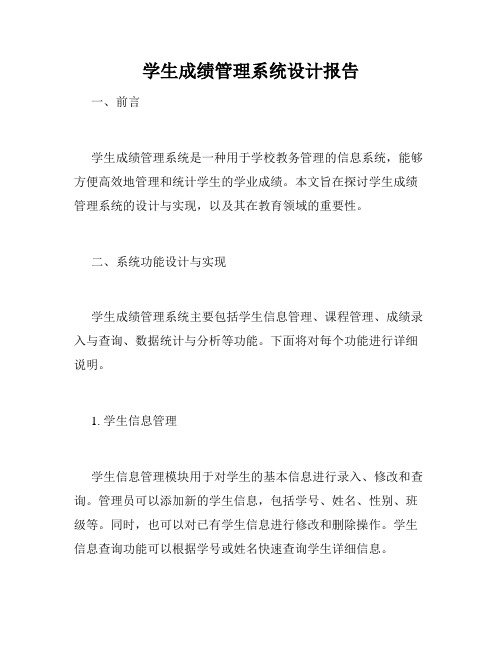
学生成绩管理系统设计报告一、前言学生成绩管理系统是一种用于学校教务管理的信息系统,能够方便高效地管理和统计学生的学业成绩。
本文旨在探讨学生成绩管理系统的设计与实现,以及其在教育领域的重要性。
二、系统功能设计与实现学生成绩管理系统主要包括学生信息管理、课程管理、成绩录入与查询、数据统计与分析等功能。
下面将对每个功能进行详细说明。
1. 学生信息管理学生信息管理模块用于对学生的基本信息进行录入、修改和查询。
管理员可以添加新的学生信息,包括学号、姓名、性别、班级等。
同时,也可以对已有学生信息进行修改和删除操作。
学生信息查询功能可以根据学号或姓名快速查询学生详细信息。
2. 课程管理课程管理模块用于管理学校的各门课程信息。
管理员可以添加新的课程,包括课程代码、名称、教师等。
此外,也可以对已有课程进行修改和删除操作。
课程查询功能可以根据课程代码或名称快速查询课程详细信息。
3. 成绩录入与查询成绩录入与查询模块用于记录学生的各门课程成绩,并提供查询功能。
教师可以通过该模块录入学生的考试成绩,包括课程代码、学号、成绩等。
学生和家长可以通过系统查询成绩,了解自己的学业表现。
成绩查询功能支持按学号或课程代码查询。
4. 数据统计与分析数据统计与分析模块用于对学生的成绩进行统计和分析。
系统可以根据各个维度(如班级、课程)对成绩数据进行汇总,生成报表和图表展示成绩情况。
通过数据分析,学校能够及时发现学生学习中存在的问题,帮助他们改进学习方法。
三、系统设计与技术实现学生成绩管理系统的设计与实现离不开合理的系统架构和技术支持。
下面介绍系统设计与技术实现的关键要素。
1. 系统架构学生成绩管理系统采用B/S架构,即基于浏览器的客户端/服务器模式。
通过将系统部署在服务器,用户只需要在浏览器中输入指定网址即可访问。
这样做不仅简化了系统的安装和维护,还提高了系统的稳定性和安全性。
2. 开发工具与技术系统的开发可以选用多种开发工具和技术,如HTML/CSS、JavaScript、PHP、MySQL等。
学生成绩信息管理系统
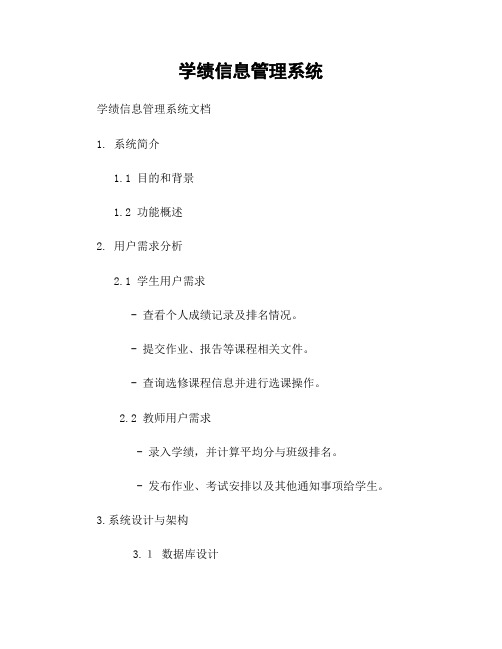
学绩信息管理系统学绩信息管理系统文档1. 系统简介1.1 目的和背景1.2 功能概述2. 用户需求分析2.1 学生用户需求- 查看个人成绩记录及排名情况。
- 提交作业、报告等课程相关文件。
- 查询选修课程信息并进行选课操作。
2.2 教师用户需求- 录入学绩,并计算平均分与班级排名。
- 发布作业、考试安排以及其他通知事项给学生。
3.系统设计与架构3.1数据库设计-设计数据库表结构,包括:学生表、教师表、科目表等。
3-2功能模块划分-划定各个子模块职责范围,如登录认证模块, 成绩录入/查询模块, 文件模块等。
4.详细功能描述4-1登陆注册–实现不同角色(管理员/老师/學生)登錄註冊功能,包含用戶驗證與權限控制部分。
4-2成績查詢–允许學生根据条件查询个人成绩,并显示排名情况。
4-3成績录入–允许教师输入学绩,系统自动计算平均分与班级排名,并提供相应的错误提示功能。
4-4 文件- 学生可以提交作业、报告等文件;老师可发布课程资料给学生。
5. 系统测试5.1 单元测试- 对每个模块进行单元测试以验证其正确性和稳定性。
6.部署与运行环境6-1硬件需求-列出服务器及客户端所需要的硬件配置要求。
6-2软件需求-列出操作系統, 数据库管理系统和其他必须安装在服务器上的软体需求。
7.附件8.法律名词及注释:- GDPR(General Data Protection Regulation):欧洲通用数据保护条例,是为了加强对于公民隐私权利和信息处理规范而制定的一项监管政策。
- COPPA (Children's Online Privacy Protection Act):儿童在线隐私保护法案,在美国旨在增强对13岁以下孩子们网络活动中收集到他们身份信息使用者责任意识方面做了规定。
- FERPA (Family Educational Rights and Privacy Act):家庭教育权利和隐私法案,是美国一项旨在保护学生个人信息的联邦法律。
班级学生成绩管理制度
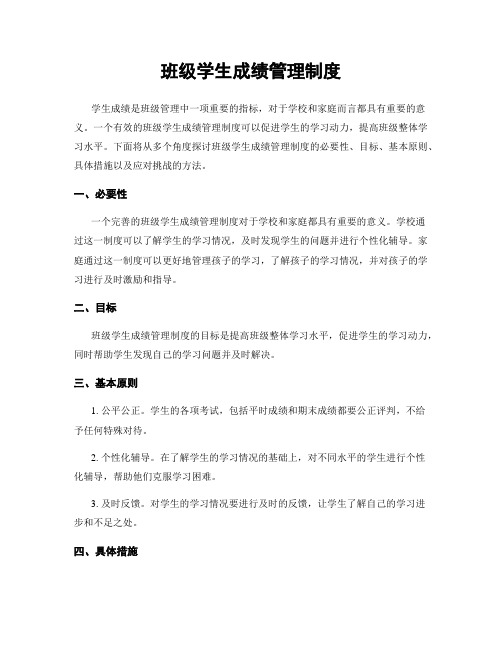
班级学生成绩管理制度学生成绩是班级管理中一项重要的指标,对于学校和家庭而言都具有重要的意义。
一个有效的班级学生成绩管理制度可以促进学生的学习动力,提高班级整体学习水平。
下面将从多个角度探讨班级学生成绩管理制度的必要性、目标、基本原则、具体措施以及应对挑战的方法。
一、必要性一个完善的班级学生成绩管理制度对于学校和家庭都具有重要的意义。
学校通过这一制度可以了解学生的学习情况,及时发现学生的问题并进行个性化辅导。
家庭通过这一制度可以更好地管理孩子的学习,了解孩子的学习情况,并对孩子的学习进行及时激励和指导。
二、目标班级学生成绩管理制度的目标是提高班级整体学习水平,促进学生的学习动力,同时帮助学生发现自己的学习问题并及时解决。
三、基本原则1. 公平公正。
学生的各项考试,包括平时成绩和期末成绩都要公正评判,不给予任何特殊对待。
2. 个性化辅导。
在了解学生的学习情况的基础上,对不同水平的学生进行个性化辅导,帮助他们克服学习困难。
3. 及时反馈。
对学生的学习情况要进行及时的反馈,让学生了解自己的学习进步和不足之处。
四、具体措施1. 教师定期组织考试,进行客观、公正的评卷。
同时,对学生的平时表现也要进行评价,反映学生的全面学习能力。
2. 学校建立家校联系机制,不定期邀请家长参加家长会,了解学生在家庭环境中的学习情况,并与家长共同制定学习计划。
3. 学校设立学习小组,由在学习方面表现优秀的学生带领,组织学生相互学习、竞争,提高整体学习水平。
4. 学校加大师资力量,增加教师数量,保证每个教师能够对每个学生进行系统的教学和辅导。
五、应对挑战在实施班级学生成绩管理制度的过程中,可能会遇到一些挑战,例如学生对学习的厌学情绪、学生之间的竞争等。
针对这些挑战,需要采取以下措施来解决。
1. 营造积极向上的学习氛围,引导学生树立正确的学习观念,培养学生主动学习的能力。
2. 引导学生正确看待竞争,将竞争看作是一种积极向上的推动力,而不是压力。
关于学生成绩管理的具体规定

关于学生成绩管理的具体规定学生成绩是衡量学生学习成果和教学质量的重要依据,为了保证成绩管理的科学性、公正性和准确性,特制定以下具体规定。
一、成绩评定的原则和方式1、公平公正原则教师在评定学生成绩时,应遵循公平公正的原则,对所有学生一视同仁,不偏袒、不歧视任何学生。
2、多元化评定方式成绩评定应采用多元化的方式,包括但不限于考试、作业、课堂表现、实验报告、项目成果等。
考试可以分为平时测验、期中考试和期末考试等形式。
作业应包括书面作业、实践作业等。
课堂表现涵盖学生的参与度、提问回答、小组合作等方面。
实验报告和项目成果则根据具体的学科和课程要求进行评定。
3、权重分配不同的评定方式应赋予合理的权重。
一般来说,期末考试成绩在总成绩中所占比例不宜过高,应注重学生在整个学习过程中的表现。
例如,期末考试成绩占总成绩的 50%,平时测验成绩占 20%,作业成绩占 20%,课堂表现占 10%。
二、考试管理1、命题要求教师命题应依据教学大纲和课程目标,注重考查学生对知识的理解、应用和创新能力。
试题应具有一定的难度和区分度,避免出现过于简单或偏题、怪题。
2、考试组织考试安排应提前公布,包括考试时间、地点、考试形式等。
考试过程中,应严格遵守考场纪律,监考人员要认真履行职责,防止作弊行为的发生。
3、试卷评阅试卷评阅应按照统一的标准和评分细则进行,做到客观、公正、准确。
评阅后的试卷应进行复查,确保评分无误。
三、成绩录入与审核1、成绩录入教师应在规定的时间内将学生成绩录入教务管理系统,确保成绩的准确性和完整性。
2、成绩审核成绩录入后,应由教学管理人员进行审核。
审核内容包括成绩的录入是否准确、评定方式和权重是否符合规定等。
如有问题,应及时通知教师进行修改。
四、成绩查询与申诉1、成绩查询学生有权查询自己的成绩。
学校应提供便捷的成绩查询渠道,如教务管理系统、校园网等。
2、成绩申诉如果学生对自己的成绩有异议,可以在规定的时间内提出申诉。
学生成绩管理系统

学生成绩管理系统学生成绩管理系统是一个用于管理、记录和分析学生学习成绩的工具。
它可以帮助学校、教师和家长更好地了解学生的学习情况,提供个性化的教学指导和辅导。
本文将介绍学生成绩管理系统的功能、优势以及在实际应用中的作用。
一、功能介绍1. 学生信息管理:学生成绩管理系统可以存储和管理学生的个人信息,包括姓名、班级、学号、家庭联系方式等。
教师和学校可以通过系统快速查找和更新学生信息。
2. 成绩录入与查询:学生的各科成绩可以通过系统进行录入和查询。
教师可以根据学科、班级或学生姓名进行成绩查询,及时了解学生的学习情况。
3. 成绩分析与报告:学生成绩管理系统可以根据学生的成绩数据生成详细的成绩分析报告。
通过分析学生在不同科目、不同时间段的表现,教师可以更好地评估学生的学习水平和进步空间,及时调整教学内容和方法。
4. 学习计划和目标设定:学生成绩管理系统可以帮助学校和教师制定学生个性化的学习计划和目标。
根据学生的历史成绩和评估结果,系统可以推荐适合学生的学习资源和教材,提供针对性的学习建议。
5. 家校互动平台:学生成绩管理系统还提供了家校互动的功能,家长可以通过系统查看学生的成绩和评语,与教师进行实时沟通。
教师可以向家长发布通知、作业、考试安排等信息,促进学校和家庭的紧密合作。
二、系统优势1. 提高工作效率:学生成绩管理系统可以自动化完成学生信息管理、成绩录入和查询等繁琐的工作,节省教师和学校的时间和精力。
2. 数据准确性:通过使用学生成绩管理系统,可以避免人为录入错误和纰漏,确保学生成绩数据的准确性和完整性。
3. 个性化分析:学生成绩管理系统提供了丰富的成绩分析功能,可以根据学生的特点和需求进行个性化评估和辅导,促进学生全面发展。
4. 促进教学改进:通过对学生成绩进行全面分析,教师可以及时发现学生的薄弱环节和问题,采取有针对性的教学改进措施,提高教学效果。
5. 加强家校联系:学生成绩管理系统提供了家校互动平台,方便教师和家长之间及时沟通,共同关注学生的学习情况和发展。
学生成绩管理系统

学生成绩管理系统简介学生成绩管理系统是一种用于管理学生的学业成绩和相关信息的软件系统。
它可以帮助学校、教师和学生有效地管理和查询学生的成绩,提供全面的成绩分析和统计功能,促进教学质量的提高。
功能学生成绩管理系统通常具有以下核心功能:1.学生信息管理:包括学生的基本信息、课程注册、班级信息等。
2.成绩录入:教师可以登录系统,录入学生成绩,并进行相应的成绩审核和修改。
3.成绩查询:学生、教师和学校管理者均可通过系统查询学生成绩,可以按照学生、班级、课程等维度进行灵活查询。
4.成绩统计与分析:系统可以对成绩数据进行统计和分析,生成各类成绩报告和分析图表,为教师和学校提供决策依据。
5.成绩排名:系统可以根据学生成绩进行排名,可以按照总分、班级、课程等维度进行排名。
6.数据导入导出:系统可以支持将学生成绩数据导入导出到Excel、CSV等格式,方便学校的数据管理。
优势学生成绩管理系统的应用带来诸多优势:1.提高工作效率:通过系统自动化处理和统计成绩数据,节省了大量的人力和时间成本。
2.提供准确的数据:系统可以准确地计算和记录学生成绩,避免了人工计算和录入带来的错误。
3.提供个性化服务:学生成绩管理系统可以根据学生的不同需求提供个性化的信息查询和分析功能,满足学生个性化的学习需求。
4.提供全面的分析和决策支持:系统可以生成各类成绩报告和分析图表,帮助教师和学校进行成绩分析和决策。
5.提高教学质量:通过系统对学生成绩进行全方位的管理和分析,有助于教师了解学生的学习状况,及时调整教学方法,提高教学质量。
使用场景学生成绩管理系统适用于各类学校、教育机构以及在线教育平台的学生成绩管理需求。
以下是几个典型的使用场景:1.学校管理者可以通过系统了解学校整体的学生成绩情况,进行综合分析和决策。
2.教师可以通过系统录入学生成绩、排名、查询历史成绩等,方便管理学生成绩和进行个性化教学。
3.学生可以通过系统查询自己的成绩、查看排名等,及时了解自己的学习状况。
3-学校对学生考试成绩进行备份和查阅的相关管理制度
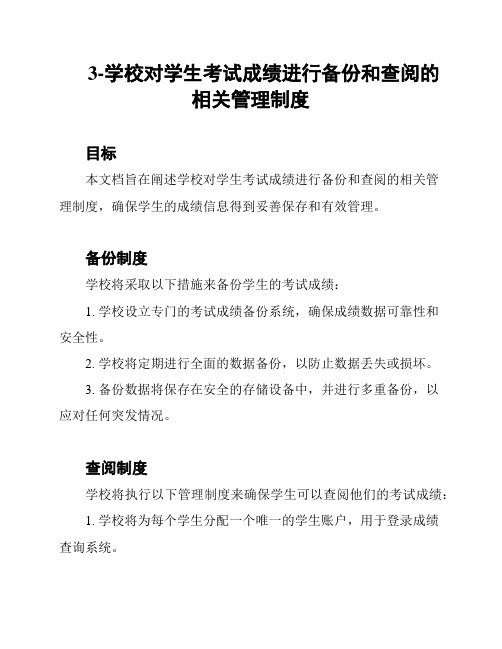
3-学校对学生考试成绩进行备份和查阅的相关管理制度目标本文档旨在阐述学校对学生考试成绩进行备份和查阅的相关管理制度,确保学生的成绩信息得到妥善保存和有效管理。
备份制度学校将采取以下措施来备份学生的考试成绩:1. 学校设立专门的考试成绩备份系统,确保成绩数据可靠性和安全性。
2. 学校将定期进行全面的数据备份,以防止数据丢失或损坏。
3. 备份数据将保存在安全的存储设备中,并进行多重备份,以应对任何突发情况。
查阅制度学校将执行以下管理制度来确保学生可以查阅他们的考试成绩:1. 学校将为每个学生分配一个唯一的学生账户,用于登录成绩查询系统。
2. 学生可以通过登录系统来查阅他们的考试成绩,系统将提供简洁明了的界面和操作指南。
3. 学生也可以选择向教务处提出成绩查询请求,教务处将协助学生查找和提供相关成绩信息。
4. 学校将确保成绩信息的及时更新和准确性,提供给学生最新的成绩数据。
数据保护学校将采取以下措施保护学生的成绩数据:1. 学校将严格限制对成绩数据的访问权限,只有授权人员才能查阅和修改成绩信息。
2. 学校将建立网络安全防护措施,防止未经授权的访问和数据泄露。
3. 学校将制定数据保护政策和操作规范,确保遵循相关的法律法规和隐私保护原则。
总结通过建立备份制度、查阅制度和数据保护措施,学校将确保对学生考试成绩的有效管理和保护。
这将有助于提升学生的信任感和学业积极性,同时促进学校的教学管理工作。
有关该管理制度的具体操作细则将在相关政策文件中详细规定。
以上为学校对学生考试成绩进行备份和查阅的相关管理制度的文档内容。
---注:该文档所描述的制度为简化版,无法覆盖所有法律复杂性。
具体的制度实施需要遵循相关法规和学校政策,以确保合法性和可行性。
学生成绩管理系统论文

学生成绩管理系统论文引言学生成绩管理系统是指通过信息化技术对学生学业成绩进行记录、分析和管理的一种系统。
在传统的学校教学中,教师往往需要通过手工记录学生的成绩情况,而学生成绩管理系统的出现极大地提高了教务工作的效率和准确性。
本论文将介绍学生成绩管理系统的设计与实现,并分析其在教育领域中的重要性。
设计与实现功能需求学生成绩管理系统主要包含以下功能:1.学生信息管理:包括学生基本信息的录入、修改和查询等操作。
2.课程管理:包括课程的添加、删除、修改和查询等操作。
3.成绩录入:教师可以录入学生的成绩信息。
4.成绩查询:学生、教师和教务管理人员可以通过系统查询学生的成绩。
5.成绩统计与分析:系统能够对学生成绩进行统计和分析,生成相应的成绩报表和图表,方便教师和教务管理人员进行评估和分析。
技术选型学生成绩管理系统的设计与实现使用了以下技术:1.后端开发:采用了Node.js作为后端开发语言,结合Express.js框架进行开发。
Node.js具有高效的异步IO和事件驱动机制,并且拥有强大的社区支持。
2.前端开发:采用了HTML、CSS和JavaScript进行前端开发,结合Bootstrap框架进行页面布局和样式设计。
3.数据库:采用了MongoDB作为数据库存储学生和成绩信息。
MongoDB是一种非关系型数据库,具有高性能、可扩展性和灵活的数据模型。
系统架构学生成绩管理系统的整体架构包括前端界面、后端逻辑和数据库三个部分。
前端界面通过浏览器与后端逻辑进行交互,后端逻辑通过与数据库的交互实现具体的功能。
系统架构图如下:系统架构图系统架构图实现过程学生成绩管理系统的实现过程如下:1.设计数据库:根据系统需求,设计MongoDB数据库的结构,包括学生信息表和成绩信息表。
2.实现后端逻辑:使用Node.js和Express.js框架开发后端逻辑,包括学生信息管理、课程管理、成绩录入和查询等功能。
3.实现前端界面:使用HTML、CSS和JavaScript开发前端界面,通过Ajax技术与后端进行数据交互,实现用户的操作和数据展示。
学生成绩管理系统活动方案

学生成绩管理系统活动方案一、引言学生成绩管理是学校教育管理的重要方面。
为了提高学校学生成绩管理的效率和质量,我们提出了学生成绩管理系统的活动方案。
本方案旨在利用信息化技术,建立一个全面、高效、准确的学生成绩管理系统,以便更好地跟踪学生的学业进展,提供精准的学生评估和有效的教育干预措施。
二、背景分析随着教育信息化的发展,学生成绩管理系统成为学校管理的必备工具。
传统的纸质成绩管理方式已经无法满足现代学生管理的需求,存在着信息不准确、传递效率低、统计工作繁琐等问题。
因此,建立一个现代化的学生成绩管理系统势在必行。
三、系统建设目标1. 提高学生成绩管理效率:通过建立学生成绩数据库,实现成绩录入、查询、统计分析等功能,减少管理人力成本,提高工作效率。
2. 精确评估学生学业水平:通过系统自动生成学生成绩报告单,全面、准确地评估学生的学术表现,为学生、教师和家长提供关键的参考指标。
3. 提供个性化教育干预措施:通过学生成绩数据的分析,帮助教师及时发现学生的学习问题,制定个性化的教育干预措施,提高学生成绩和学习动力。
4. 促进学校与家长的沟通:学生成绩管理系统将成绩数据与家长关联,实现学校与家庭之间的信息共享与传递,增强学校与家长之间的合作与沟通。
四、系统功能介绍1. 学生信息管理:学生个人信息录入、查询、修改和删除等功能,保证学生信息的准确性和完整性。
2. 教师信息管理:教师个人信息录入、查询、修改和删除等功能,方便学校管理教师信息。
3. 成绩录入与统计:教师可将学生成绩录入系统,并根据需求进行统计分析,如年级成绩统计、班级成绩排名等。
4. 学生成绩报告单生成:根据学生成绩数据,系统将自动生成学生的成绩报告单,并提供打印和导出功能,方便学校、教师和家长查阅。
5. 个性化教育干预措施:系统根据学生成绩数据,自动生成学生学业发展曲线图,帮助教师及时发现学生的学习问题,并提供相关的教育干预措施。
6. 家校互动平台:学生的成绩数据公开与家长关联,家长可以通过系统查看学生的成绩、教师评语等信息,并与教师进行互动沟通。
管理信息系统课程设计 学生成绩管理系统

管理信息系统课程设计学生成绩管理系统一、引言在当今教育领域,学生成绩管理是学校教学管理的重要组成部分。
随着学校规模的不断扩大,学生人数的日益增加,传统的手工成绩管理方式已经无法满足高效、准确、便捷的管理需求。
因此,开发一个功能齐全、操作简便的学生成绩管理系统具有重要的现实意义。
二、系统需求分析(一)功能需求1、学生信息管理能够录入、修改、查询和删除学生的基本信息,如学号、姓名、班级等。
2、课程信息管理对学校开设的课程进行管理,包括课程名称、课程代码、学分、授课教师等信息的录入、修改和查询。
3、成绩录入与修改教师能够方便地录入学生的考试成绩,并支持成绩的修改和调整。
4、成绩查询与统计学生和教师可以按照不同的条件查询成绩,如学号、课程名称等。
同时,系统能够提供成绩统计功能,如平均分、最高分、最低分等。
5、权限管理为不同的用户设置不同的权限,如学生只能查询自己的成绩,教师可以录入和修改所授课程的成绩,管理员拥有系统的最高权限。
(二)性能需求1、响应时间系统在进行数据录入、查询和统计等操作时,响应时间应控制在合理范围内,确保用户的操作能够及时得到反馈。
2、稳定性系统应具备良好的稳定性,能够在长时间运行的情况下不出现故障或数据丢失。
3、安全性保证系统数据的安全性,防止非法用户的入侵和数据的篡改。
(三)数据需求1、学生信息包括学号、姓名、性别、出生日期、班级等。
2、课程信息课程代码、课程名称、学分、授课教师等。
3、成绩信息学号、课程代码、成绩等。
三、系统设计(一)总体设计1、系统架构采用 B/S(浏览器/服务器)架构,用户通过浏览器访问系统,服务器端负责数据的处理和存储。
2、模块划分系统主要分为学生信息管理模块、课程信息管理模块、成绩管理模块、查询统计模块和权限管理模块。
(二)数据库设计1、数据库概念模型根据系统需求,设计出学生、课程、成绩等实体以及它们之间的关系。
2、数据库表结构创建学生表(Student)、课程表(Course)、成绩表(Score)等,并定义相应的字段和数据类型。
教师学生成绩与评价管理制度

教师学生成绩与评价管理制度一、背景介绍学生成绩与评价是教育领域中的重要问题。
如何科学、客观地评价学生的学习成果,如何通过评价结果来促进学生进步,已经成为各个学校和教师面临的挑战。
在这一背景下,建立健全的教师学生成绩与评价管理制度显得尤为重要。
二、提高学生学习动力教师学生成绩与评价管理制度能够激发学生学习的积极性和主动性。
通过科学的评价,学生能够清晰地了解到自己的学习成绩和不足之处,并知道如何去改进,这将帮助他们形成自我驱动力,提高学习动力。
三、确保评价公平公正教师学生成绩与评价管理制度需要确保评价的公平公正性。
教师应该根据学生成绩、作业质量、参与度、课堂表现等多个维度全面评价学生,避免片面侧重某一项指标而使评价结果失真。
同时,教师也要确保评价标准明确透明,让学生和家长都能够理解和认同。
四、培养综合素质教师学生成绩与评价管理制度应该注重培养学生的综合素质。
除了学习成绩,学生还应该在评价中体现出对知识的理解能力、分析思考能力、创新能力、合作能力等综合素质的培养。
这样,评价结果才能真正反映学生的全面发展。
五、建立奖惩机制教师学生成绩与评价管理制度应该合理建立奖惩机制。
优秀的学生可以获得鼓励和奖励,以激发他们更进一步的学习动力;而对于表现不佳的学生,应该给予适当的惩罚与引导,帮助他们认识到问题所在,并积极改进。
六、加强与家长的沟通教师学生成绩与评价管理制度需要加强与家长的沟通。
通过及时向家长反馈学生的评价结果和问题所在,家长可以更好地了解孩子的学习情况,及时进行学习辅导和指导。
同时,家长的参与也可以促进评价结果的客观性和公正性。
七、细化评价指标教师学生成绩与评价管理制度应该细化评价指标。
除了基础的学科成绩之外,还应该考虑到学生的实践能力、思维能力、创新能力等方面的评价。
通过细化评价指标,可以更准确地了解学生在不同方面的发展情况,有针对性地进行教学。
八、加强自我评价教师学生成绩与评价管理制度需要教师进行自我评价。
学生成绩管理系统专业术语

学生成绩管理系统专业术语
学生成绩管理系统是一个用于管理学生成绩的工具,包括以下专业术语:
1、学生信息:包括学生姓名、学号、班级、课程信息等。
2、课程信息:包括课程名称、课程代码、任课教师、学分等信息。
3、成绩录入:将学生参加的考试成绩进行录入,系统会自动计算总分、平均分、及格率等成绩指标。
4、成绩查询:可以按照学生姓名、学号、班级、课程名称等条件查询学生的成绩信息。
5、成绩分析:系统可以根据录入的学生成绩信息,进行分析和统计,生成各种图表和报表,帮助教师和学生更好地了解学生的学习情况。
6、成绩排名:系统可以根据学生的成绩信息,进行排名,让学生和教师了解学生的成绩在班级或全校中的排名情况。
7、成绩预警:系统可以根据学生的成绩情况,自动生成预警信息,提醒学生及时自己的学习成绩,以及时采取措施提高学习水平。
8、成绩导出:可以将录入的成绩信息导出为Excel等格式,方便学生进行备份和处理。
9、系统设置:包括学生信息管理、课程信息管理、成绩录入方式、查询条件、分析指标、预警规则等设置。
10、权限管理:可以对不同用户的权限进行管理,确保系统的安全性和稳定性。
这些术语是学生成绩管理系统中的常见词汇,对于使用和维护该系统具有重要意义。
学生成绩管理系统数据流程图及数据字典

学生成绩管理系统数据流程图及数据字典一、数据流程图数据流程图是一种图形化的表示方式,用于描述系统中数据的流动和处理过程。
以下是学生成绩管理系统的数据流程图:1. 整体数据流程图学生成绩管理系统的整体数据流程图如下所示:[插入整体数据流程图]2. 学生信息管理流程图学生信息管理是学生成绩管理系统的核心功能之一。
以下是学生信息管理的数据流程图:[插入学生信息管理流程图]3. 成绩录入流程图成绩录入是学生成绩管理系统的重要功能之一。
以下是成绩录入的数据流程图:[插入成绩录入流程图]4. 成绩查询流程图成绩查询是学生成绩管理系统的常用功能之一。
以下是成绩查询的数据流程图:[插入成绩查询流程图]二、数据字典数据字典是对系统中所使用的数据项进行定义和说明的文档。
以下是学生成绩管理系统的数据字典:1. 学生信息表(Student Information)数据项:- 学生ID(Student ID):学生的唯一标识符,由系统自动生成。
- 姓名(Name):学生的姓名。
- 年级(Grade):学生所在的年级。
- 班级(Class):学生所在的班级。
- 性别(Gender):学生的性别。
- 出生日期(Date of Birth):学生的出生日期。
- 联系方式(Contact Information):学生的联系方式。
2. 课程信息表(Course Information)数据项:- 课程ID(Course ID):课程的唯一标识符,由系统自动生成。
- 课程名称(Course Name):课程的名称。
- 课程学分(Course Credit):课程的学分。
3. 成绩信息表(Grade Information)数据项:- 成绩ID(Grade ID):成绩的唯一标识符,由系统自动生成。
- 学生ID(Student ID):学生的唯一标识符。
- 课程ID(Course ID):课程的唯一标识符。
- 成绩(Grade):学生在该门课程中的成绩。
学生成绩管理系统设计方案

学生成绩管理系统设计方案概述:学生成绩管理系统是一种应用于学校或教育机构管理学生学业成绩的信息化工具。
本文将讨论学生成绩管理系统的设计方案,包括系统的功能需求、系统的模块设计、数据库设计以及用户界面设计等方面。
一、功能需求1. 学生信息管理:系统应能够记录学生的基本信息,包括姓名、性别、年龄、班级等,并能够进行信息的查询和修改。
2. 课程管理:系统应能够管理学校开设的各门课程,包括课程的名称、教师、授课时间等信息,并能够进行课程信息的录入和修改。
3. 成绩录入:系统应能够允许教师录入学生的考试成绩,包括平时成绩和考试成绩,并能够对成绩进行统计和分析。
4. 成绩查询:系统应能够提供学生和教师查询学生成绩的功能,包括按学生姓名、班级、课程等条件进行查询,并能够生成成绩报表。
5. 数据分析:系统应能够对学生成绩进行分析,包括成绩的平均值、最高分、最低分等统计指标的计算,并能够生成相应的图表进行可视化展示。
6. 系统管理:系统应具备用户权限管理功能,包括管理员、教师和学生角色的权限设置,以及对用户账号的管理和维护。
二、系统的模块设计1. 用户管理模块:实现管理员对用户账号的管理和权限设置功能。
2. 学生信息管理模块:实现学生基本信息的录入、查询和修改功能。
3. 课程管理模块:实现课程信息的录入、查询和修改功能。
4. 成绩录入模块:实现教师对学生成绩的录入功能。
5. 成绩查询模块:提供学生和教师查询学生成绩的功能。
6. 数据分析模块:实现对学生成绩进行统计和分析的功能。
三、数据库设计1. 学生表:包括学生的学号、姓名、性别、年龄、班级等字段。
2. 课程表:包括课程的编号、名称、教师、授课时间等字段。
3. 成绩表:包括学生的学号、课程编号、平时成绩、考试成绩等字段。
4. 用户表:包括用户的账号、密码、角色等字段。
四、用户界面设计1. 登录界面:提供用户登录系统的入口,输入账号和密码进行身份认证。
2. 学生信息管理界面:显示学生的基本信息列表,并提供查询和修改功能。
学生成绩考核评定和管理的细则

XXXX学校学生成绩考核评定和管理的细则学生学业成绩考核,不仅是对学生学习成绩结果作出的评价,也是对照教学目标检查教学质量的信息反馈,并根据这些信息对教学质量进行分析、监控、研究改进教学工作的重要手段。
一、成绩考核成绩考核分考试和考查两种。
每学期考试和考查的课程门数按实施性教学计划规定执行。
要根据课程的特点和学生的负担,每学期一般安排2~4门考试课程。
考试是对学生所学知识和技能进行总结性考核的方法。
可采用课堂理论考试,实验、实际操作、技能考评,大型作业,案例分析等多种方法进行。
一般可分为期中、期末考试,由教务管理部门统一组织,安排在该课程结束后或学期末,集中一段时间复习和考试。
考试形式可用笔试、口试、实操三种。
可根据课程的特点和需要,灵活采用,应坚持理论与实践的考核并重的原则。
笔试又可分为闭卷考试和开卷考试。
采用开卷考试的课程必须报教务管理部门批准方可实施。
笔试时间一般为100分钟,口试的准备时间为40分钟,答题最多20分钟,实操考试时间视考试内容具体确定。
考查是对学生所学知识和技能进行平时考核的方法。
可依据平时课堂提问、课堂作业、实际操作、单元测试等方法采集学生学习成绩,考查不得在学期末及考试周的前一周内集中测验或变相考试。
二、命题管理1、考试命题要以教学大纲为依据,全面测量学生应具备的知识和能力。
考试范围原则上是该课程本学期的全部内容。
应注意覆盖面要广和突出教学重点,基础知识题、综合分析题、应用题比例恰当。
2、命题先由教研组制定命题方案(或编制双向细目表),组卷试题应有一定的梯度,基本题、水平题、提高题结构合理,区分度强,题量适中,便于分辨学生成绩的优劣,考试成绩力求符合正态分布。
已考过的试卷不宜未作修改又做下一次考卷。
3、用同一学期授课计划、教学内容和进度相同的班级采用同一试卷。
每门课程应拟定水平、分量相当的两份试卷,并附有标准答案及评分标准。
实行学分制管理的,可采用A、B制命题。
实行分层教学管理的,应按分层教学大纲命题。
学生成绩管理系统功能概述

(2)学生信息的查询,包括查询学生基本信息和成绩.
(3)学生信息的修改,包括修改学生基本信息和成绩。
(4)学生信息的删除,包括修改学生基本信息和成绩。
(5)登录用户密码修改,用户登录到系统可进行相应的用户密码修改。
1学生成绩管理系统本系统主要用于学校学生信息管理总体任务是实现学生信息关系的系统化规范化和自动化其主要任务是用计算机对学生信息进行日常管理如查询修改增加删除另外还考虑到用户登录的权限针对学生信息和权限登录的学生成绩管理系统
学生成绩管理系统功能概述
学生成绩管理系统
本系统主要用于学校学生信息管理,总体任务是实现学生信息关系的系统化、规范化和自动化,其主要任务是用计算机对学生信息进行日常管理,如查询、修改 、增加、删除,另外还考虑到用户登录的权限,针对学生信息和权限登录的学生成绩管理系统。
(6)管理员用户对用户名的管理,包括添加新用户、删除用户。
1
系统功能流程图
学生成绩管理系统
用户登录
学生成绩管理系统
学生成绩管理系统 教师管理系统 登录管理员系统
各科成绩 学生 所有成绩
添加
详细信息 查找 老师
修改 修改密码 学生
升序排序 删除
老师 退出
修改密码
退出
退出
图 3。1 系统功能流程
2
3
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
/* 用链表实现学生成绩信息的管理*/
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
struct stud_node{
int num;
char name[20];
int score;
struct stud_node *next;
};
struct stud_node * Create_Stu_Doc(); /* 新建链表*/
struct stud_node * InsertDoc(struct stud_node * head, struct stud_node *stud); /* 插入*/ struct stud_node * DeleteDoc(struct stud_node * head, int num); /* 删除*/
void Print_Stu_Doc(struct stud_node * head); /* 遍历*/
int main(void)
{
struct stud_node *head,*p;
int choice, num, score;
char name[20];
int size = sizeof(struct stud_node);
printf("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~~~\n");
printf("\n");
printf(" Welcome to use the management of students' performance information\n"); printf("\n");
printf(" Make by Aibilly\n");
printf("\n");
printf("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~~~\n");
do{
printf("please choose 1:Create 2:Insert \n 3:Delete 4:Print 0:Exit\n");
scanf("%d", &choice);
switch(choice){
case 1:
head=Create_Stu_Doc();
break;
case 2:
printf("Input num,name and score:\n");
scanf("%d%s%d", &num,name, &score);
p = (struct stud_node *) malloc(size);
p->num = num;
strcpy(p->name, name);
p->score = score;
head=InsertDoc(head, p);
break;
case 3:
printf("Input num:\n");
scanf("%d", &num);
head = DeleteDoc(head, num);
break;
case 4:
Print_Stu_Doc(head);
break;
case 0:
break;
}
}while(choice != 0);
return 0;
}
/*新建链表*/
struct stud_node * Create_Stu_Doc()
{
struct stud_node * head,*p;
int num,score;
char name[20];
int size = sizeof(struct stud_node);
head=NULL;
printf("Input num,name and score:\n");
scanf("%d%s%d", &num,name, &score);
while(num != 0){
p = (struct stud_node *) malloc(size);
p->num = num;
strcpy(p->name, name);
p->score = score;
head=InsertDoc(head, p); /* 调用插入函数*/
scanf("%d%s%d", &num, name, &score);
}
return head;
}
/* 插入操作*/
struct stud_node * InsertDoc(struct stud_node * head, struct stud_node *stud)
{
struct stud_node *ptr ,*ptr1, *ptr2;
ptr2 = head;
ptr = stud; /* ptr指向待插入的新的学生记录结点*/
/* 原链表为空时的插入*/
if(head == NULL){
head = ptr; /* 新插入结点成为头结点*/
head->next = NULL;
}
else{ /* 原链表不为空时的插入*/
while((ptr->num > ptr2->num) && (ptr2->next != NULL)){
ptr1 = ptr2; /* ptr1, ptr2各后移一个结点*/
ptr2 = ptr2->next;
}
if(ptr->num <= ptr2->num){ /* 在ptr1与ptr2之间插入新结点*/ if(head==ptr2) head = ptr;
else ptr1->next = ptr;
ptr->next = ptr2;
}
else{ /* 新插入结点成为尾结点*/
ptr2->next =ptr;
ptr->next = NULL;
}
}
return head;
}
/* 删除操作*/
struct stud_node * DeleteDoc(struct stud_node * head, int num)
{
struct stud_node *ptr1, *ptr2;
/* 要被删除结点为表头结点*/
while(head!=NULL && head->num == num){
ptr2 = head;
head = head->next;
free(ptr2);
}
if(head == NULL) /*链表空*/
return NULL;
/* 要被删除结点为非表头结点*/
ptr1 = head;
ptr2 = head->next; /*从表头的下一个结点搜索所有符合删除要求的结点*/ while(ptr2!=NULL){
if(ptr2->num == num){ /* ptr2所指结点符合删除要求*/
ptr1->next = ptr2->next;
free(ptr2);
}
else
ptr1 = ptr2; /* ptr1后移一个结点*/
ptr2 = ptr1->next; /* ptr2指向ptr1的后一个结点*/ }
return head;
}
/*遍历操作*/
void Print_Stu_Doc(struct stud_node * head)
{ struct stud_node * ptr;
if(head == NULL){
printf("\nNo Records\n");
return;
}
printf("\nThe Students' Records Are: \n");
printf(" Num Name Score\n");
for(ptr = head; ptr; ptr = ptr->next)
printf("%8d%20s%6d \n", ptr->num, ptr->name, ptr->score);
}。