遍历二叉树(递归+非递归)实验资料报告材料
数据结构二叉树遍历实验报告

问题一:二叉树遍历1.问题描述设输入该二叉树得前序序列为:ABC##DE#G##F##HI##J#K##(#代表空子树)请编程完成下列任务:⑴请根据此输入来建立该二叉树,并输出该二叉树得前序、中序与后序序列;⑵按层次遍历得方法来输出该二叉树按层次遍历得序列;⑶求该二叉树得高度.2.设计描述(1)二叉树就是一种树形结构,遍历就就是要让树中得所有节点被且仅被访问一次,即按一定规律排列成一个线性队列。
二叉(子)树就是一种递归定义得结构,包含三个部分:根结点(N)、左子树(L)、右子树(R).根据这三个部分得访问次序对二叉树得遍历进行分类,总共有6种遍历方案:NLR、LNR、LRN、NR L、RNL与LNR。
研究二叉树得遍历就就是研究这6种具体得遍历方案,显然根据简单得对称性,左子树与右子树得遍历可互换,即NLR与NRL、LNR与RNL、LRN与RLN,分别相类似,因而只需研究NLR、LNR与LRN三种即可,分别称为“先序遍历”、“中序遍历”与“后序遍历”.采用递归方式就可以容易得实现二叉树得遍历,算法简单且直观。
(2)此外,二叉树得层次遍历即按照二叉树得层次结构进行遍历,按照从上到下,同一层从左到右得次序访问各节点。
遍历算法可以利用队列来实现,开始时将整个树得根节点入队,然后每从队列中删除一个节点并输出该节点得值时,都将它得非空得左右子树入队,当队列结束时算法结束.(3)计算二叉树高度也就是利用递归来实现:若一颗二叉树为空,则它得深度为0,否则深度等于左右子树得最大深度加一.3。
源程序1 23 4 5 6 7 8 9 10111213 #include 〈stdio、h>#include <stdlib、h>#include 〈malloc、h>#define ElemTypecharstructBTreeNode {ﻩElemType data;ﻩstruct BTreeNode* left;ﻩstruct BTreeNode* right;};void CreateBTree(struct BTreeNode** T){ﻩchar ch;ﻩscanf_s("\n%c”, &ch);14151617 18 19202122 23 24 252627 282930 31 32 333435 36373839404142 43 4445464748 495051 ﻩif (ch ==’#’)*T = NULL;ﻩelse {ﻩ(*T) = malloc(sizeof(struct BTreeNode)); ﻩﻩ(*T)->data = ch;CreateBTree(&((*T)—〉left));ﻩCreateBTree(&((*T)—>right));ﻩ}}void Preorder(struct BTreeNode*T){ﻩif (T != NULL) {ﻩﻩprintf("%c ", T->data);ﻩPreorder(T->left);ﻩPreorder(T->right);ﻩ}}void Inorder(struct BTreeNode*T){ﻩif (T != NULL) { ﻩInorder(T—>left);ﻩprintf("%c ",T->data);ﻩInorder(T—〉right);ﻩ}}voidPostorder(structBTreeNode*T){if (T !=NULL) {ﻩPostorder(T->left);Postorder(T-〉right);printf(”%c ", T-〉data);ﻩ}}voidLevelorder(struct BTreeNode* BT){struct BTreeNode*p;struct BTreeNode*q[30];ﻩint front=0,rear=0;ﻩif(BT!=NULL) {52 53545556 57585960 61626364 6566 6768 6970 7172 73 74 7576 7778 7980818283 848586 8788 89 ﻩﻩrear=(rear+1)%30;q[rear]=BT;ﻩ}while(front!=rear) {ﻩfront=(front+1)% 30;p=q[front];ﻩﻩprintf(”%c",p->data);if(p-〉left!=NULL) {ﻩrear=(rear+1)%30;ﻩﻩq[rear]=p->left;ﻩ}ﻩif(p—>right!=NULL) {ﻩrear=(rear+1)% 30;ﻩﻩﻩq[rear]=p-〉right;ﻩ}}}int getHeight(struct BTreeNode*T) {ﻩint lh,rh;ﻩif(T == NULL) return0;lh = getHeight(T->left);rh = getHeight(T—>right);return lh>rh ?lh + 1 : rh + 1; }void main(void){ﻩstruct BTreeNode*T;CreateBTree(&T);ﻩprintf("前序序列:\n”);Preorder(T);printf("\n");ﻩprintf("中序序列:\n”);ﻩInorder(T);ﻩprintf(”\n");printf("后序序列:\n");Postorder(T);printf("\n”);9091929394printf("层次遍历序列:\n”);ﻩLevelorder(T);printf(”\n”);printf("二叉树高度:%d\n”, getHeight(T));}4、运行结果问题二:哈夫曼编码、译码系统1.问题描述对一个ASCII编码得文本文件中得字符进行哈夫曼编码,生成编码文件;反过来,可将编码文件译码还原为一个文本文件(选做)。
二叉树的建立和遍历的实验报告doc

二叉树的建立和遍历的实验报告篇一:二叉树的建立及遍历实验报告实验三:二叉树的建立及遍历【实验目的】(1)掌握利用先序序列建立二叉树的二叉链表的过程。
(2)掌握二叉树的先序、中序和后序遍历算法。
【实验内容】1. 编写程序,实现二叉树的建立,并实现先序、中序和后序遍历。
如:输入先序序列abc###de###,则建立如下图所示的二叉树。
并显示其先序序列为:abcde中序序列为:cbaed后序序列为:cbeda【实验步骤】1.打开VC++。
2.建立工程:点File->New,选Project标签,在列表中选Win32 Console Application,再在右边的框里为工程起好名字,选好路径,点OK->finish。
至此工程建立完毕。
3.创建源文件或头文件:点File->New,选File标签,在列表里选C++ Source File。
给文件起好名字,选好路径,点OK。
至此一个源文件就被添加到了你刚创建的工程之中。
4.写好代码5.编译->链接->调试#include#include#define OK 1#define OVERFLOW -2typedef int Status;typedef char TElemType;typedef struct BiTNode{TElemType data;struct BiTNode *lchild, *rchild;}BiTNode,*BiTree;Status CreateBiTree(BiTree &T){TElemType ch;scanf("%c",&ch);if (ch=='#')T= NULL;else{if (!(T = (BiTNode *)malloc(sizeof(BiTNode))))return OVERFLOW;T->data = ch; CreateBiTree(T->lchild); CreateBiTree(T->rchild); }return OK;} // CreateBiTreevoid PreOrder(BiTree T) {if(T){printf("%c",T->data); PreOrder(T->lchild); PreOrder(T->rchild);}}void InOrder(BiTree T) {if(T){InOrder(T->lchild);printf("%c",T->data);InOrder(T->rchild);}}void PostOrder(BiTree T){if(T){PostOrder(T->lchild); PostOrder(T->rchild);printf("%c",T->data);}}void main(){BiTree T;CreateBiTree(T);printf("\n先序遍历序列:"); PreOrder(T);printf("\n中序遍历序列:"); InOrder(T);printf("\n后序遍历序列:"); PostOrder(T);}【实验心得】这次实验主要是通过先序序列建立二叉树,和二叉树的先序、中序、后续遍历算法。
二叉树的遍历算法实验报告

二叉树的遍历算法实验报告二叉树的遍历算法实验报告引言:二叉树是计算机科学中常用的数据结构之一,它是由节点组成的层次结构,每个节点最多有两个子节点。
在实际应用中,对二叉树进行遍历是一项重要的操作,可以帮助我们理解树的结构和节点之间的关系。
本文将介绍二叉树的三种遍历算法:前序遍历、中序遍历和后序遍历,并通过实验验证其正确性和效率。
一、前序遍历前序遍历是指先访问根节点,然后按照先左后右的顺序遍历左右子树。
具体的实现可以通过递归或者使用栈来实现。
我们以递归方式实现前序遍历算法,并进行实验验证。
实验步骤:1. 创建一个二叉树,并手动构造一些节点和它们之间的关系。
2. 实现前序遍历算法的递归函数,函数的输入为根节点。
3. 在递归函数中,首先访问当前节点,然后递归调用函数遍历左子树,最后递归调用函数遍历右子树。
4. 调用前序遍历函数,输出遍历结果。
实验结果:经过实验,我们得到了正确的前序遍历结果。
这证明了前序遍历算法的正确性。
二、中序遍历中序遍历是指按照先左后根再右的顺序遍历二叉树。
同样,我们可以使用递归或者栈来实现中序遍历算法。
在本实验中,我们选择使用递归方式来实现。
实验步骤:1. 继续使用前面创建的二叉树。
2. 实现中序遍历算法的递归函数,函数的输入为根节点。
3. 在递归函数中,首先递归调用函数遍历左子树,然后访问当前节点,最后递归调用函数遍历右子树。
4. 调用中序遍历函数,输出遍历结果。
实验结果:通过实验,我们得到了正确的中序遍历结果。
这证明了中序遍历算法的正确性。
三、后序遍历后序遍历是指按照先左后右再根的顺序遍历二叉树。
同样,我们可以使用递归或者栈来实现后序遍历算法。
在本实验中,我们选择使用递归方式来实现。
实验步骤:1. 继续使用前面创建的二叉树。
2. 实现后序遍历算法的递归函数,函数的输入为根节点。
3. 在递归函数中,首先递归调用函数遍历左子树,然后递归调用函数遍历右子树,最后访问当前节点。
4. 调用后序遍历函数,输出遍历结果。
二叉树存储与遍历实验报告
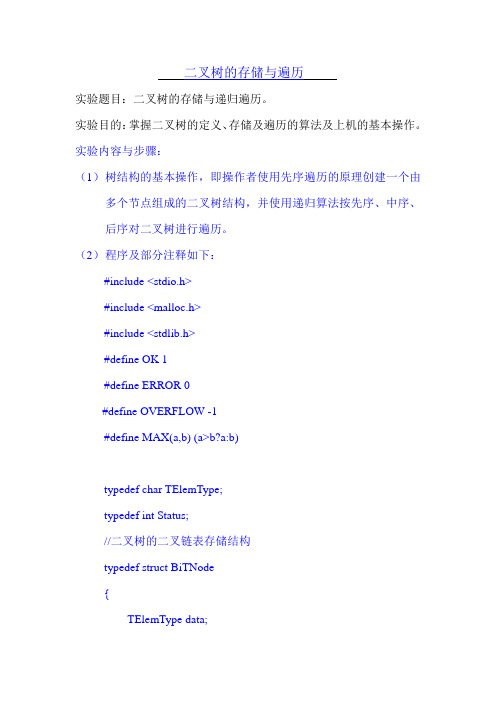
二叉树的存储与遍历实验题目:二叉树的存储与递归遍历。
实验目的:掌握二叉树的定义、存储及遍历的算法及上机的基本操作。
实验内容与步骤:(1)树结构的基本操作,即操作者使用先序遍历的原理创建一个由多个节点组成的二叉树结构,并使用递归算法按先序、中序、后序对二叉树进行遍历。
(2)程序及部分注释如下:#include <stdio.h>#include <malloc.h>#include <stdlib.h>#define OK 1#define ERROR 0#define OVERFLOW -1#define MAX(a,b) (a>b?a:b)typedef char TElemType;typedef int Status;//二叉树的二叉链表存储结构typedef struct BiTNode{TElemType data;struct BiTNode *lchild,*rchild;}BiTNode,*BiTree;//先序遍历生成二叉树Status CreatBiTree(BiTree &T){TElemType ch,temp;printf("输入一个元素: ");scanf("%c",&ch);temp=getchar(); //结束回车if(ch==' ') T=NULL; //输入空格表示结点为空树else{if(!(T=(BiTree)malloc(sizeof(BiTNode))))exit(OVERFLOW);T->data=ch; //生成根结点CreatBiTree(T->lchild); //构造左子树CreatBiTree(T->rchild); //构造右子树}return OK;}//打印元素Status PrintElem(TElemType e){printf("%c ",e);return OK;}//先序遍历二叉树Status PreOrderTraverse(BiTree T,Status (* Visit)(TElemType e)) {if(T){ //二叉树不为空时if(Visit(T->data)) //访问根结点if(PreOrderTraverse(T->lchild,Visit)) //先序遍历左子树if(PreOrderTraverse(T->rchild,Visit)) return OK; //先序遍历右子树return ERROR;}else return OK;}//中序遍历二叉树Status InOrderTraverse(BiTree T,Status (* Visit)(TElemType e)) {if(T){if(InOrderTraverse(T->lchild,Visit))if(Visit(T->data))if(InOrderTraverse(T->rchild,Visit)) return OK;else return ERROR;}return OK;}//后序遍历二叉树Status PostOrderTraverse(BiTree T,Status (* Visit)(TElemType e)){ if(T){if(PostOrderTraverse(T->lchild,Visit))if(PostOrderTraverse(T->rchild,Visit))if(Visit(T->data)) return OK;else return ERROR;}return OK;}void main(){BiTree T;Status (* Visit)(TElemType);Visit=PrintElem;CreatBiTree(T);printf("\n先序遍历:");PreOrderTraverse(T,Visit);printf("\n中序遍历:");InOrderTraverse(T,Visit);printf("\n后序遍历:");PostOrderTraverse(T,Visit);printf("\n程序结束.\n");}分析与体会:(1)本程序采用的是递归的算法实现了二叉树的三种遍历,相对于非递归的算法较为简单。
二叉树遍历的实习报告

实习报告实习内容:二叉树遍历实习时间:2023实习单位:某高校计算机实验室一、实习目的本次实习的主要目的是通过实现二叉树的遍历,加深对二叉树数据结构的理解,掌握二叉树的常见操作,提高编程能力。
二、实习内容1. 理解二叉树的基本概念和性质,包括节点之间的关系、树的深度、高度等。
2. 掌握二叉树的存储结构,包括顺序存储和链式存储。
3. 实现二叉树的前序遍历、中序遍历和后序遍历。
4. 通过实际编程,验证二叉树遍历的正确性。
三、实习过程1. 二叉树的基本概念和性质:二叉树是一种非线性的数据结构,每个节点最多有两个子节点。
节点之间的关系包括父子关系、兄弟关系等。
树的深度是指从根节点到最远叶子节点的最长路径上的边数,高度是指从根节点到最远叶子节点的最长路径上的边数加1。
2. 二叉树的存储结构:二叉树可以用顺序存储结构或链式存储结构表示。
顺序存储结构使用数组来实现,每个节点存储在数组的一个位置中,节点之间的父子关系通过数组下标来表示。
链式存储结构使用链表来实现,每个节点包含数据域和两个指针域,分别指向左子节点和右子节点。
3. 二叉树的遍历:二叉树的遍历是指按照一定的顺序访问树中的所有节点。
常见的遍历方式有前序遍历、中序遍历和后序遍历。
前序遍历是指先访问根节点,然后递归遍历左子树,最后递归遍历右子树。
中序遍历是指先递归遍历左子树,然后访问根节点,最后递归遍历右子树。
后序遍历是指先递归遍历左子树,然后递归遍历右子树,最后访问根节点。
4. 编程实现:根据二叉树的存储结构和遍历方法,编写C语言程序实现二叉树的前序遍历、中序遍历和后序遍历。
程序中使用递归函数来实现遍历操作,通过建立链式存储结构,验证遍历的正确性。
四、实习心得通过本次实习,我对二叉树的数据结构有了更深入的了解,掌握了二叉树的存储方式和常见操作。
在实现二叉树遍历的过程中,我学会了如何使用递归函数解决问题,提高了编程能力。
同时,通过实际编程验证了二叉树遍历的正确性,增强了对算法理解的信心。
二叉树遍历实验报告

1.实验题目二叉树的建立与遍历[问题描述]建立一棵二叉树,并对其进行遍历(先序、中序、后序),打印输出遍历结果。
2.需求分析(1)输入的形式和输入值的范围:以字符形式按先序遍历输入(2)输出的形式:依次按递归先序、中序、后序遍历,非递归先序、中序、后序遍历结果输出(3) 程序所能达到的功能:从键盘接受输入(先序)进行遍历(先序、中序、后序),将遍历结果打印输。
(4) 测试数据:ABCффDEфGффFффф(其中ф表示空格字符)则输出结果为先序:ABCDEGF中序:CBEGDFA后序:CGBFDBA3.概要设计(1)struct btnode{char data; 数据struct btnode *Lchild;左子数指针struct btnode *Rchild; 右子数指针};struct btnode *createbt(struct btnode *bt )初始条件:空二叉树存在操作结果:先序建立二叉树void preOrder(struct btnode *bt)初始条件:二叉树存在递归先序遍历二叉树void preOrder1(struct btnode *bt)初始条件:二叉树存在操作结果:非递归先序遍历void midOrder(struct btnode *bt)初始条件:二叉树存在操作结果:递归中序遍历void midOrder1(struct btnode *bt)初始条件:二叉树存在操作结果:非递归中序遍历void postOrder(struct btnode *bt)初始条件:二叉树存在操作结果:递归后序遍历void postOrder1 (struct btnode *bt)初始条件:二叉树存在操作结果:非递归后序遍历void main() 主函数(2)void main() 主函数{*createbtpreOrderpreOrder1midOrdermidOrder1postOrderpostOrder1}4.详细设计struct btnode{char data;struct btnode *Lchild;struct btnode *Rchild;};struct btnode *createbt(struct btnode *bt ){ 输入结点数据c检查存储空间将c赋给结点参数p递归建立左子树递归建立右子树}void preOrder(struct btnode *bt){判断树是否为空输出根结点数data递归遍历左子树递归遍历右子树}void preOrder1(struct btnode *bt){定义栈,结点参数pWhile(栈或p是否为空){While(p!=null){输出根结点数data将根结点压栈遍历左子树}提取栈顶元素值栈顶元素出栈访问右子树}void midOrder(struct btnode *bt){判断树是否为空递归遍历左子树输出根结点数data递归遍历右子树}void midOrder1(struct btnode *bt){定义栈,结点参数pWhile(栈或p是否为空){While(p!=null){将根结点压栈遍历左子树}提取栈顶元素值输出根结点数data栈顶元素出栈访问右子树}void postOrder(struct btnode *bt){判断树是否为空递归遍历左子树递归遍历右子树输出根结点数data}void postOrder1 (struct btnode *bt){定义栈,结点参数p,prebt入栈While(栈或p是否为空){提取栈顶元素值if判断p是否为空或是pre的根结点输出根结点数data栈顶元素出栈栈顶元素p赋给pre记录else if右结点非空将右结点压栈if左结点将左结点压栈}}void main(){struct btnode *root=NULL;root=createbt(root);preOrder(root); midOrder(root); postOrder(root);preOrder1(root); midOrder1(root);postOrder1(root);}5.调试分析(1)先序建立二叉树时,虽用到递归建左右子树,但没有把他们赋值给根节点的左右指针,造成二叉树脱节。
树的遍历实验报告

实验项目:树的遍历1.实验目的:学会创建一棵二叉树,以及完成对树的简单操作。
2.实验内容:1)生成一棵以二叉链表存储的二叉树bt(不少于15个结点)2)分别用递归和非递归方法前序遍历bt,并以缩格形式打印bt 上各结点的信息。
3)编写算法,交换bt上所有结点的左、右子树,并以缩格形式打印出交换前后的bt结点信息。
3.程序简介:先创建一棵二叉树,递归非递归前序遍历,层次遍历,交换左右子树,缩格打印各结点的信息。
4.算法设计介绍:首先按照前序遍历的顺序递归创建一棵二叉树,然后序遍历的非递归使用堆栈完成的,即访问该结点的时候,如果有右孩子,让右孩子进栈,访问左孩子,当左孩子为空时,抛出栈顶的元素,访问出栈的这个元素的左右孩子。
缩格打印和层次遍历想法类似,都是借助队列完成的,把当前结点的左右孩子进队列之后,让这个结点出队列。
交换左右子树,就是当某个结点的左右子树不同时为空时,定义一个中间变量交换。
5.困难及解答一开始创建二叉树的参数我想用指向结构体的指针,后来才意识到得用指向指针的指针,想了好一段时间才想明白,因为某个结点的左右孩子是指向结点的指针,要想再指向一个指针,只能用指针的指针了。
6.心得树这一章我听得乱七八糟,上课能听懂,但就是不会编程,要不是书上有算法,我估计我肯定编不出来,看来还是得多编啊。
程序清单/*// 我真诚地保证:// 我独立完成了整个程序从分析、设计到编码的所有工作。
// 如果在上述过程中,我遇到了什么困难而求教于人,那么,我将在程序实习报告中// 详细地列举我所遇到的问题,以及别人给我的提示。
// 我的程序里中凡是引用到其他程序或文档之处,// 例如教材、课堂笔记、网上的源代码以及其他参考书上的代码段,// 我都已经在程序的注释里很清楚地注明了引用的出处。
// 我从未没抄袭过别人的程序,也没有盗用别人的程序,// 不管是修改式的抄袭还是原封不动的抄袭。
// 我编写这个程序,从来没有想过要去破坏或妨碍其他计算机系统的正常运转。
二叉树递归遍历数据结构实验报告
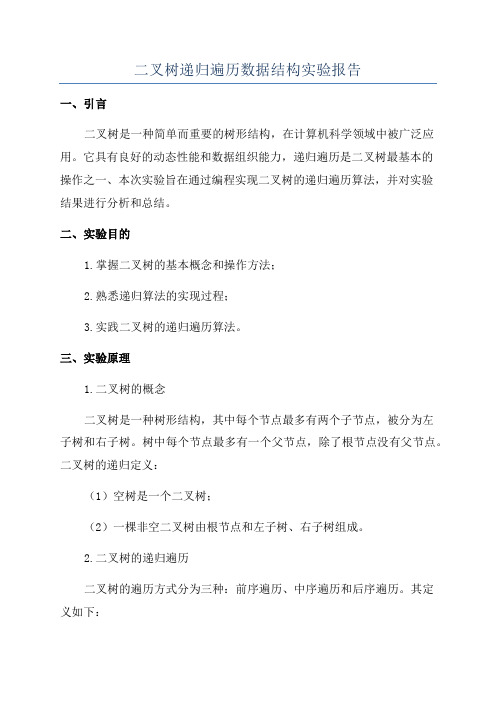
二叉树递归遍历数据结构实验报告一、引言二叉树是一种简单而重要的树形结构,在计算机科学领域中被广泛应用。
它具有良好的动态性能和数据组织能力,递归遍历是二叉树最基本的操作之一、本次实验旨在通过编程实现二叉树的递归遍历算法,并对实验结果进行分析和总结。
二、实验目的1.掌握二叉树的基本概念和操作方法;2.熟悉递归算法的实现过程;3.实践二叉树的递归遍历算法。
三、实验原理1.二叉树的概念二叉树是一种树形结构,其中每个节点最多有两个子节点,被分为左子树和右子树。
树中每个节点最多有一个父节点,除了根节点没有父节点。
二叉树的递归定义:(1)空树是一个二叉树;(2)一棵非空二叉树由根节点和左子树、右子树组成。
2.二叉树的递归遍历二叉树的遍历方式分为三种:前序遍历、中序遍历和后序遍历。
其定义如下:(1)前序遍历:根节点->左子树->右子树;(2)中序遍历:左子树->根节点->右子树;(3)后序遍历:左子树->右子树->根节点。
四、实验过程1.定义二叉树的数据结构和相关操作方法首先,我们需要定义二叉树的节点结构,包含数据域和左右子节点指针域。
然后,可定义插入节点、删除节点等操作方法。
2.实现递归遍历算法(1)前序遍历前序遍历的流程为:先访问根节点,再前序遍历左子树,最后前序遍历右子树。
通过递归调用即可实现。
伪代码如下:```void preOrder(Node* root)if (root != NULL)cout << root->data;preOrder(root->left);preOrder(root->right);}(2)中序遍历和后序遍历与前序遍历类似,中序遍历的流程为:先中序遍历左子树,再访问根节点,最后中序遍历右子树。
后序遍历的流程为:先后序遍历左子树,再后序遍历右子树,最后访问根节点。
也可以通过递归调用实现。
伪代码如下:```void inOrder(Node* root)if (root != NULL)inOrder(root->left);cout << root->data;inOrder(root->right);}void postOrder(Node* root)if (root != NULL)postOrder(root->left);postOrder(root->right);cout << root->data;}五、实验结果与分析我们通过编写测试数据并调用递归遍历算法进行遍历,得到以下结果:(1)前序遍历结果:ABDECFG(2)中序遍历结果:DBEAFCG(3)后序遍历结果:DEBFGCA实验结果与预期相符,表明递归遍历算法编写正确。
遍历二叉树实验报告
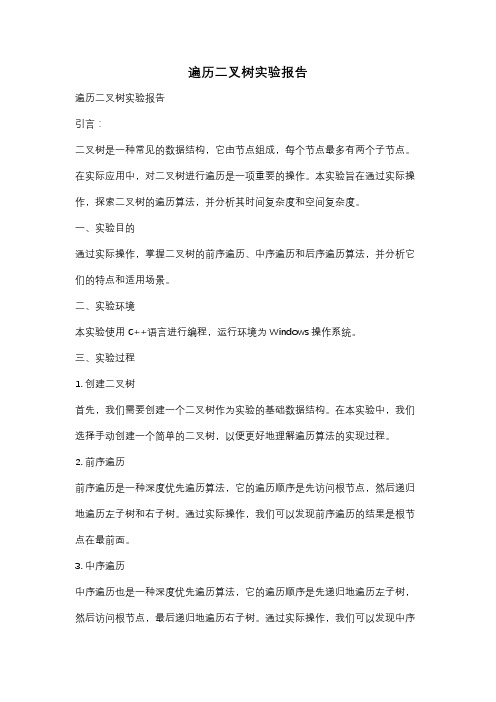
遍历二叉树实验报告遍历二叉树实验报告引言:二叉树是一种常见的数据结构,它由节点组成,每个节点最多有两个子节点。
在实际应用中,对二叉树进行遍历是一项重要的操作。
本实验旨在通过实际操作,探索二叉树的遍历算法,并分析其时间复杂度和空间复杂度。
一、实验目的通过实际操作,掌握二叉树的前序遍历、中序遍历和后序遍历算法,并分析它们的特点和适用场景。
二、实验环境本实验使用C++语言进行编程,运行环境为Windows操作系统。
三、实验过程1. 创建二叉树首先,我们需要创建一个二叉树作为实验的基础数据结构。
在本实验中,我们选择手动创建一个简单的二叉树,以便更好地理解遍历算法的实现过程。
2. 前序遍历前序遍历是一种深度优先遍历算法,它的遍历顺序是先访问根节点,然后递归地遍历左子树和右子树。
通过实际操作,我们可以发现前序遍历的结果是根节点在最前面。
3. 中序遍历中序遍历也是一种深度优先遍历算法,它的遍历顺序是先递归地遍历左子树,然后访问根节点,最后递归地遍历右子树。
通过实际操作,我们可以发现中序遍历的结果是根节点在中间。
4. 后序遍历后序遍历同样是一种深度优先遍历算法,它的遍历顺序是先递归地遍历左子树和右子树,最后访问根节点。
通过实际操作,我们可以发现后序遍历的结果是根节点在最后面。
5. 分析与总结通过对前序遍历、中序遍历和后序遍历的实际操作,我们可以得出以下结论:- 前序遍历适合于需要先处理根节点的场景,例如树的构建和复制。
- 中序遍历适合于需要按照节点值的大小顺序进行处理的场景,例如搜索二叉树的构建和排序。
- 后序遍历适合于需要先处理叶子节点的场景,例如树的销毁和内存释放。
四、实验结果通过实际操作,我们成功实现了二叉树的前序遍历、中序遍历和后序遍历算法,并得到了相应的遍历结果。
这些结果验证了我们对遍历算法的分析和总结的正确性。
五、实验总结本实验通过实际操作,深入探索了二叉树的遍历算法,并分析了它们的特点和适用场景。
二叉树的遍历实验报告
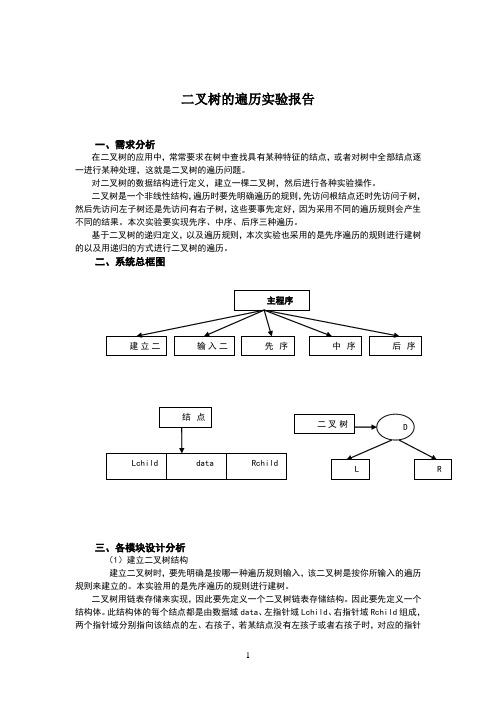
二叉树的遍历实验报告一、需求分析在二叉树的应用中,常常要求在树中查找具有某种特征的结点,或者对树中全部结点逐一进行某种处理,这就是二叉树的遍历问题。
对二叉树的数据结构进行定义,建立一棵二叉树,然后进行各种实验操作。
二叉树是一个非线性结构,遍历时要先明确遍历的规则,先访问根结点还时先访问子树,然后先访问左子树还是先访问有右子树,这些要事先定好,因为采用不同的遍历规则会产生不同的结果。
本次实验要实现先序、中序、后序三种遍历。
基于二叉树的递归定义,以及遍历规则,本次实验也采用的是先序遍历的规则进行建树的以及用递归的方式进行二叉树的遍历。
二、系统总框图三、各模块设计分析(1)建立二叉树结构建立二叉树时,要先明确是按哪一种遍历规则输入,该二叉树是按你所输入的遍历规则来建立的。
本实验用的是先序遍历的规则进行建树。
二叉树用链表存储来实现,因此要先定义一个二叉树链表存储结构。
因此要先定义一个结构体。
此结构体的每个结点都是由数据域data 、左指针域Lchild 、右指针域Rchild 组成,两个指针域分别指向该结点的左、右孩子,若某结点没有左孩子或者右孩子时,对应的指针域就为空。
最后,还需要一个链表的头指针指向根结点。
要注意的是,第一步的时候一定要先定义一个结束标志符号,例如空格键、#等。
当它遇到该标志时,就指向为空。
建立左右子树时,仍然是调用create()函数,依此递归进行下去,直到遇到结束标志时停止操作。
(2)输入二叉树元素输入二叉树时,是按上面所确定的遍历规则输入的。
最后,用一个返回值来表示所需要的结果。
(3)先序遍历二叉树当二叉树为非空时,执行以下三个操作:访问根结点、先序遍历左子树、先序遍历右子树。
(4)中序遍历二叉树当二叉树为非空时,程序执行以下三个操作:访问根结点、先序遍历左子树、先序遍历右子树。
(5)后序遍历二叉树当二叉树为非空时,程序执行以下三个操作:访问根结点、先序遍历左子树、先序遍历右子树。
二叉树遍历 实验报告

数据结构实验报告报告题目: 二叉树的基本操作学生班级:学生姓名: 学号:一. 实验目的1、基本要求: 深刻理解二叉树性质和各种存储结构的特点及适用范围;掌握用指针类型描述、访问和处理二叉树的运算;熟练掌握二叉树的遍历算法;。
2. 较高要求: 在遍历算法的基础上设计二叉树更复杂操作算法;认识哈夫曼树、哈夫曼编码的作用和意义;掌握树与森林的存储与便利。
二.实验学时:课内实验学时: 3学时课外实验学时: 6学时三. 实验题目1. 以二叉链表为存储结构, 实现二叉树的创建、遍历(实验类型: 验证型)1)问题描述:在主程序中设计一个简单的菜单, 分别调用相应的函数功能:1…建立树2…前序遍历树3…中序遍历树4…后序遍历树5…求二叉树的高度6…求二叉树的叶子节点7…非递归中序遍历树0…结束2)实验要求: 在程序中定义下述函数, 并实现要求的函数功能:CreateBinTree(BinTree &T): 按从键盘输入的前序序列, 创建树Preorder(BinTree &T): 前序遍历树(递归)Inorder(BinTree &T): 中序(递归)遍历树Postorder(BinTree &T): 后序遍历树(递归)PostTreeDepth(BinTree &T): 树的高度leaf(BinTree &T):树的叶子节点InorderN(BinTree &T): 中序(非递归)遍历树3)数据结构二叉链表存储数据类型定义typedef struct node{TElemType data;struct node *lchild,*rchild;}BinTNode;元素类型:int CreateBinTree(BinTree &T);void Preorder(BinTree &T);void Inorder(BinTree &T);void Postorder(BinTree &T);void InorderN(BinTree &T);int PostTreeDepth(BinTree &T);int leaf(BinTree &T);2.编写算法实现二叉树的非递归中序遍历和求二叉树高度。
数据结构实验报告:递归和非递归遍历二叉树

return false;
}//if
else
return true;
}
void outputTree(BiTree pbnode,int totalSpace)/*输出二叉树*/
{if(pbnode!=NULL)
{totalSpace+=8;/*右子树与根节点相距8个空格*/
CreateTree(T->lchild);
printf("请输入%c的右子树,否则输入#\n",T->data);
CreateTree(T->rchild);
}//else
}// CreateTree
//访问二叉树节点
Visit(BiTree p)
{ if(p)
{ printf(" %c ",p->data);
{totalSpace+=8;/*右子树与根节点相距8个空格*/
outputTree(pbnode->rchild,totalSpace);
for(int i=0;i<totalSpace;i++)
printf(" ");
printf("%c\n\n",pbnode->data);
outputTree(pbnode->lchild,totalSpace);/*递归调用左子树*/
count2=InOrderTraverAndCountLeaf(t);//非递推遍历二叉树
printf("\n非递推法遍历二叉树所得叶子节点数: %d\n\n",count2);
p=T;
SqStack s;
二叉树的遍历实验报告

二叉树的遍历实验报告一、实验目的1.了解二叉树的存储结构。
2.掌握二叉树的遍历方式。
二、实验原理1.二叉树的定义:二叉树是一种特殊的树形结构,它的每个结点最多只能有两个子结点,分别称为左子结点和右子结点。
一般有两种存储方式,分别是顺序存储和链式存储。
其中顺序存储需要用到数组,而链式存储则需要用到指针。
遍历二叉树的方式主要有三种,分别是前序遍历、中序遍历和后序遍历。
其中前序遍历是先遍历根节点,然后遍历左子树和右子树;中序遍历是先遍历左子树,然后遍历根节点和右子树;后序遍历是先遍历左子树和右子树,然后遍历根节点。
三、实验步骤typedef struct binaryTree {char data; //数据域struct binaryTree *left; //左子树struct binaryTree *right; //右子树} BTree;2.创建二叉树:BTree *createBTree(BTree *bt) {char ch;scanf("%c", &ch);if (ch == '#') {bt = NULL;}else {bt = (BTree*)malloc(sizeof(BTree));bt->data = ch;bt->left = createBTree(bt->left); //递归创建左子树bt->right = createBTree(bt->right); //递归创建右子树}return bt;}3.前序遍历:6.测试代码:四、实验结果分析测试所得结果如下:输入字符:AB#C##D#F##前序遍历结果:ABCFD中序遍历结果:BACFD后序遍历结果:BCFD A五、实验总结通过本次实验,我了解了二叉树的基本概念和存储结构,掌握了二叉树的前、中、后序遍历方式的实现方法。
这些知识对于我以后学习数据结构和算法,具有重要意义,对我的编程能力的提升也是有益的。
数据结构二叉树遍历实验报告简版
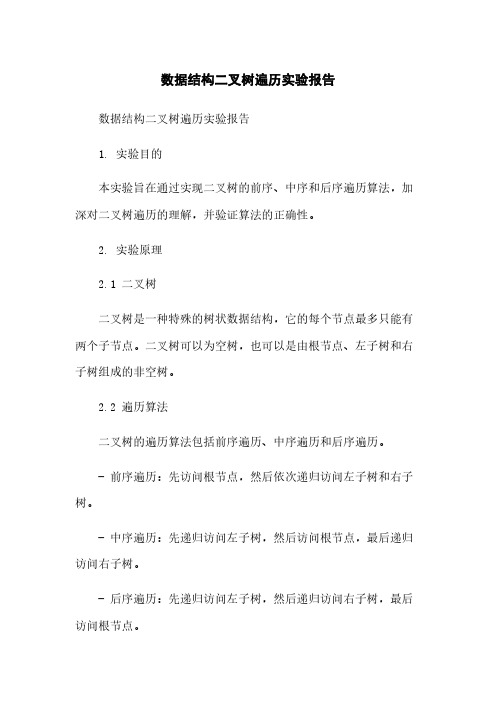
数据结构二叉树遍历实验报告数据结构二叉树遍历实验报告1. 实验目的本实验旨在通过实现二叉树的前序、中序和后序遍历算法,加深对二叉树遍历的理解,并验证算法的正确性。
2. 实验原理2.1 二叉树二叉树是一种特殊的树状数据结构,它的每个节点最多只能有两个子节点。
二叉树可以为空树,也可以是由根节点、左子树和右子树组成的非空树。
2.2 遍历算法二叉树的遍历算法包括前序遍历、中序遍历和后序遍历。
- 前序遍历:先访问根节点,然后依次递归访问左子树和右子树。
- 中序遍历:先递归访问左子树,然后访问根节点,最后递归访问右子树。
- 后序遍历:先递归访问左子树,然后递归访问右子树,最后访问根节点。
3. 实验过程3.1 数据结构设计首先,我们需要设计表示二叉树的数据结构。
在本次实验中,二叉树的每个节点包含三个成员变量:值、左子节点和右子节点。
我们可以使用面向对象编程语言提供的类来实现。
具体实现如下:```pythonclass TreeNode:def __init__(self, val=0, left=None, right=None): self.val = valself.left = leftself.right = right```3.2 前序遍历算法前序遍历算法的实现主要包括以下步骤:1. 若二叉树为空,则返回空列表。
2. 创建一个栈,用于存储遍历过程中的节点。
3. 将根节点入栈。
4. 循环执行以下步骤,直到栈为空:- 弹出栈顶节点,并将其值添加到结果列表中。
- 若当前节点存在右子节点,则将右子节点压入栈。
- 若当前节点存在左子节点,则将左子节点压入栈。
具体实现如下:```pythondef preorderTraversal(root):if not root:return []stack = []result = []stack.append(root)while stack:node = stack.pop()result.append(node.val)if node.right:stack.append(node.right)if node.left:stack.append(node.left)return result```3.3 中序遍历算法中序遍历算法的实现主要包括以下步骤:1. 若二叉树为空,则返回空列表。
二叉树的建立和遍历实验报告
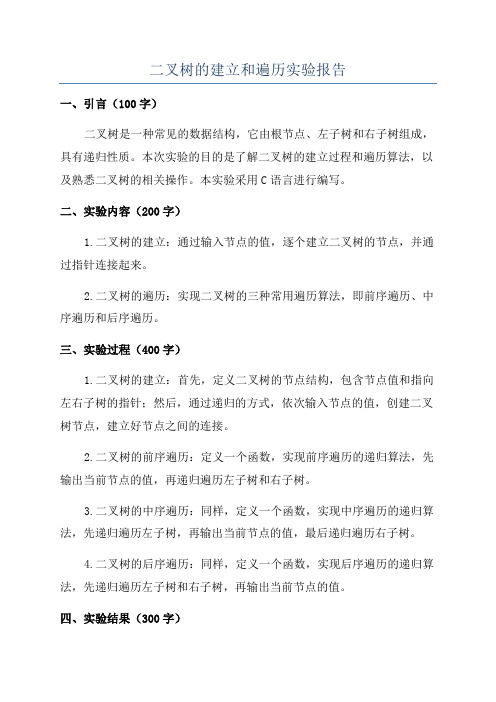
二叉树的建立和遍历实验报告一、引言(100字)二叉树是一种常见的数据结构,它由根节点、左子树和右子树组成,具有递归性质。
本次实验的目的是了解二叉树的建立过程和遍历算法,以及熟悉二叉树的相关操作。
本实验采用C语言进行编写。
二、实验内容(200字)1.二叉树的建立:通过输入节点的值,逐个建立二叉树的节点,并通过指针连接起来。
2.二叉树的遍历:实现二叉树的三种常用遍历算法,即前序遍历、中序遍历和后序遍历。
三、实验过程(400字)1.二叉树的建立:首先,定义二叉树的节点结构,包含节点值和指向左右子树的指针;然后,通过递归的方式,依次输入节点的值,创建二叉树节点,建立好节点之间的连接。
2.二叉树的前序遍历:定义一个函数,实现前序遍历的递归算法,先输出当前节点的值,再递归遍历左子树和右子树。
3.二叉树的中序遍历:同样,定义一个函数,实现中序遍历的递归算法,先递归遍历左子树,再输出当前节点的值,最后递归遍历右子树。
4.二叉树的后序遍历:同样,定义一个函数,实现后序遍历的递归算法,先递归遍历左子树和右子树,再输出当前节点的值。
四、实验结果(300字)通过实验,我成功建立了一个二叉树,并实现了三种遍历算法。
对于建立二叉树来说,只要按照递归的思路,先输入根节点的值,再分别输入左子树和右子树的值,即可依次建立好节点之间的连接。
建立好二叉树后,即可进行遍历操作。
在进行遍历算法的实现时,我首先定义了一个函数来进行递归遍历操作。
在每一次递归调用中,我首先判断当前节点是否为空,若为空则直接返回;若不为空,则按照特定的顺序进行遍历操作。
在前序遍历中,我先输出当前节点的值,再递归遍历左子树和右子树;在中序遍历中,我先递归遍历左子树,再输出当前节点的值,最后递归遍历右子树;在后序遍历中,我先递归遍历左子树和右子树,再输出当前节点的值。
通过运行程序,我成功进行了二叉树的建立和遍历,并得到了正确的结果。
可以看到,通过不同的遍历顺序,可以获得不同的遍历结果,这也是二叉树遍历算法的特性所在。
二叉树的遍历实验报告

二叉树的遍历实验报告一、实验目的1.了解二叉树的基本概念和性质;2.理解二叉树的遍历方式以及它们的实现方法;3.学会通过递归和非递归算法实现二叉树的遍历。
二、实验内容1.二叉树的定义在计算机科学中,二叉树是一种重要的数据结构,由节点及它们的左右儿子组成。
没有任何子节点的节点称为叶子节点,有一个子节点的节点称为一度点,有两个子节点的节点称为二度点。
二叉树的性质:1.每个节点最多有两个子节点;2.左右子节点的顺序不能颠倒,左边是父节点的左子节点,右边是父节点的右子节点;3.二叉树可以为空,也可以只有一个根节点;4.二叉树的高度是从根节点到最深叶子节点的层数;5.二叉树的深度是从最深叶子节点到根节点的层数;6.一个深度为d的二叉树最多有2^(d+1) -1个节点,其中d>=1;7.在二叉树的第i层上最多有2^(i-1)个节点,其中i>=1。
2.二叉树的遍历方式二叉树的遍历是指从根节点出发,按照一定的顺序遍历二叉树中的每个节点。
常用的二叉树遍历方式有三种:前序遍历、中序遍历和后序遍历。
前序遍历:先遍历根节点,再遍历左子树,最后遍历右子树;中序遍历:先遍历左子树,再遍历根节点,最后遍历右子树;后序遍历:先遍历左子树,再遍历右子树,最后遍历根节点。
递归算法:利用函数调用,递归实现二叉树的遍历;非递归算法:利用栈或队列,对二叉树进行遍历。
三、实验步骤1.创建二叉树数据结构并插入节点;2.实现二叉树的前序遍历、中序遍历、后序遍历递归算法;3.实现二叉树的前序遍历、中序遍历、后序遍历非递归算法;4.测试算法功能。
四、实验结果1.创建二叉树数据结构并插入节点为了测试三种遍历方式的算法实现,我们需要创建一个二叉树并插入节点,代码如下:```c++//定义二叉树节点struct TreeNode {int val;TreeNode* left;TreeNode* right;TreeNode(int x) : val(x), left(NULL), right(NULL) {}};递归算法是实现二叉树遍历的最简单方法,代码如下:```c++//前序遍历非递归算法vector<int> preorderTraversal(TreeNode* root) {stack<TreeNode*> s;vector<int> res;if (!root) return res;s.push(root);while (!s.empty()) {TreeNode* tmp = s.top();s.pop();res.push_back(tmp->val);if (tmp->right) s.push(tmp->right);if (tmp->left) s.push(tmp->left);}return res;}4.测试算法功能return 0;}```测试结果如下:preorderTraversal: 4 2 1 3 6 5 7inorderTraversal: 1 2 3 4 5 6 7postorderTraversal: 1 3 2 5 7 6 4preorderTraversalNonRecursive: 4 2 1 3 6 5 7inorderTraversalNonRecursive: 1 2 3 4 5 6 7postorderTraversalNonRecursive: 1 3 2 5 7 6 4本次实验通过实现二叉树的递归和非递归遍历算法,加深了对二叉树的理解,并熟悉了遍历算法的实现方法。
实现二叉树的各种遍历算法实验报告

实现二叉树的各种遍历算法实验报告一实验题目: 实现二叉树的各种遍历算法二实验要求:2.1:(1)输出二叉树 b(2)输出H节点的左右孩子节点值(3)输出二叉树b 的深度(4)输出二叉树 b的宽度(5)输出二叉树 b的节点个数(6)输出二叉树 b的叶子节点个数(7)释放二叉树 b2.2:(1)实现二叉树的先序遍历(2)实现二叉树的中序遍历(3)实现二叉树的后序遍历三实验内容:3.1 树的抽象数据类型:ADT Tree{数据对象D:D是具有相同特性的数据元素的集合。
数据关系R:若D为空集,则称为空树;若D仅含有一个数据元素,则R为空集,否则R={H},H是如下二元关系:(1) 在D中存在唯一的称为根的数据元素root,它在关系H下无前驱;(2) 若D-{root}≠NULL,则存在D-{root}的一个划分D1,D2,D3, …,Dm(m>0),对于任意j≠k(1≤j,k≤m)有Dj∩Dk=NULL,且对任意的i(1≤i≤m),唯一存在数据元素xi∈Di有<root,xi>∈H;(3) 对应于D-{root}的划分,H-{<root,xi>,…,<root,xm>}有唯一的一个划分H1,H2,…,Hm(m>0),对任意j≠k(1≤j,k≤m)有Hj∩Hk=NULL,且对任意i(1≤i≤m),Hi是Di上的二元关系,(Di,{Hi})是一棵符合本定义的树,称为根root的子树。
基本操作P:InitTree(&T);操作结果:构造空树T。
DestroyTree(&T);初始条件:树T存在。
操作结果:销毁树T。
CreateTree(&T,definition);初始条件:definition给出树T的定义。
操作结果:按definition构造树T。
ClearTree(&T);初始条件:树T存在。
操作结果:将树T清为空树。
TreeEmpty(T);初始条件:树T存在。
数据结构二叉树遍历实验报告[1]简版
![数据结构二叉树遍历实验报告[1]简版](https://img.taocdn.com/s3/m/c3328b25a9114431b90d6c85ec3a87c240288a2f.png)
数据结构二叉树遍历实验报告数据结构二叉树遍历实验报告实验目的本实验旨在通过二叉树的遍历方法,加深对二叉树结构的理解,并掌握其遍历的实现方法。
实验内容实验内容包括以下两个部分:1. 实现二叉树的先序遍历方法;2. 实现二叉树的中序遍历方法。
实验原理和实现方法1. 先序遍历先序遍历即从根节点开始,先输出当前节点的值,然后先序遍历左子树,最后先序遍历右子树。
先序遍历的实现方法有递归和迭代两种。
递归实现递归实现的核心是先输出当前节点的值,并递归调用函数对左子树和右子树进行先序遍历。
以下是递归实现的伪代码示例:```pythondef preOrderTraversal(node):if node is None:returnprint(node.value)preOrderTraversal(node.left)preOrderTraversal(node.right)```迭代实现迭代实现需要借助栈来保存节点的信息。
整体思路是先将根节点入栈,然后循环执行以下步骤:弹出栈顶节点并输出,将栈顶节点的右子节点和左子节点依次入栈。
当栈为空时,遍历结束。
以下是迭代实现的伪代码示例:```pythondef preOrderTraversal(node):if node is None:returnstack = [node]while stack:curr = stack.pop()print(curr.value)if curr.right:stack.append(curr.right)if curr.left:stack.append(curr.left)```2. 中序遍历中序遍历即从根节点开始,先中序遍历左子树,然后输出当前节点的值,最后中序遍历右子树。
中序遍历的实现方法同样有递归和迭代两种。
递归实现递归实现的核心是先中序遍历左子树,并输出当前节点的值,最后递归调用函数对右子树进行中序遍历。
以下是递归实现的伪代码示例:```pythondef inOrderTraversal(node):if node is None:returninOrderTraversal(node.left)print(node.value)inOrderTraversal(node.right)```迭代实现迭代实现同样需要借助栈来保存节点的信息。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
实验报告
附:源程序:
递归算法程序
#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
#define maxsize 100
#define FALSE 0
#define TRUE 1
typedef struct node //二叉树结构体类型定义{
char data;
struct node *lchild;
struct node *rchild;
}bitnode,*bitree;
/*扩展先序序列创建二叉链表*/
void cteatebitree(bitree *bt)
{
char ch;
ch=getchar();
if(ch=='.')*bt=NULL;
else
{
*bt=(bitree)malloc(sizeof(bitnode));
(*bt)->data=ch;
cteatebitree(&((*bt)->lchild));
cteatebitree(&((*bt)->rchild));
}
}
/*先序递归遍历*/
void preorder(bitree root)
{
if(root!=NULL)
{
printf("%c ",root->data);
preorder(root->lchild);
preorder(root->rchild);
}
}
/*中序递归遍历*/
void inorder(bitree root)
{
if(root!=NULL)
{
preorder(root->lchild);
printf("%c ",root->data);
preorder(root->rchild);
}
}
/*后序递归遍历*/
void postorder(bitree root)
{
if(root!=NULL)
{
preorder(root->lchild);
preorder(root->rchild);
printf("%c ",root->data);
}
}
void main()
{
bitree bt;
cteatebitree(&bt);
printf("先序递归遍历序列:\n");
preorder(bt);
printf("\n");
printf("中序递归遍历序列:\n");
inorder(bt);
printf("\n");
printf("后序递归遍历序列:\n");
postorder(bt);
printf("\n");
}
非递归算法程序
#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
#define FALSE 0
#define TRUE 1
#define maxsize 100
typedef struct node //二叉树结构体定义{
char data;
struct node *lchild;
struct node *rchild;
}bitnode,*bitree;
typedef struct //顺序栈结构体定义{
bitree elem[maxsize];
int top;
}seqstack;
int push(seqstack *s,bitree x) //入栈
{
if(s->top==maxsize-1)
return(FALSE);
s->top++;
s->elem[s->top]=x;
return(TRUE);
}
bitree pop(seqstack *s,bitree x) //出栈
{
if(s->top==-1) return NULL;
else
{
x=s->elem[s->top];
s->top--;
return x;
}
}
int gettop(seqstack *s,bitree *x) //读取栈顶元素
{
if(s->top==-1) return FALSE;
else
{
*x=s->elem[s->top];
return TRUE;
}
}
void createbitree(bitree *bt) //扩展先序序列创建二叉链表{
char ch;
ch=getchar();
if(ch=='.')*bt=NULL;
else
{
*bt=(bitree)malloc(sizeof(bitnode));
(*bt)->data=ch;
createbitree(&((*bt)->lchild));
createbitree(&((*bt)->rchild));
}
}
void preorder1(bitree root,seqstack s) //先序遍历
{
bitnode *p;
p=root;
while(p!=NULL||!(s.top==-1))
{
while(p!=NULL)
{
printf("%c",p->data);
push(&s,p);
p=p->lchild;
}
if(!(s.top==-1))
{
p=pop(&s,p);
p=p->rchild;
}
}
}
void inorder1(bitree root,seqstack s) //中序遍历{
bitnode *p;
s.top=-1;
p=root;
while(p!=NULL||!(s.top==-1))
{
if(p!=NULL)
{
push(&s,p);
p=p->lchild;
}
else
{
p=pop(&s,p);
printf("%c",p->data);
p=p->rchild;
}
}
}
void postorder1(bitree root) //后序遍历{
bitnode *p,*q;
seqstack s;
q=NULL;
p=root;
s.top=-1;
//printf("%c",p->data);
while(p!=NULL||!(s.top==-1))
{while(p!=NULL)
{
push(&s,p); p=p->lchild;
}
if(!(s.top==-1))
{
gettop(&s,&p);
if((p->rchild==NULL)||(p->rchild==q))
{
printf("%c",p->data);
q=p;
p=pop(&s,p);
p=NULL;
}
else p=p->rchild;
}
}
}
void main()
{
printf("先序序列创建二叉树\n");
seqstack s;
s.top=-1;
bitree root;
createbitree(&root);
printf("先序遍历序列:\n");
preorder1(root,s);
printf("\n");
printf("中序遍历序列:\n");
inorder1(root,s);
printf("\n");
printf("后序遍历序列:\n");
postorder1(root);
printf("\n");
}。