SerialPort访问接口获取电子称数据
利用C#通过串口提取GPS定位信息
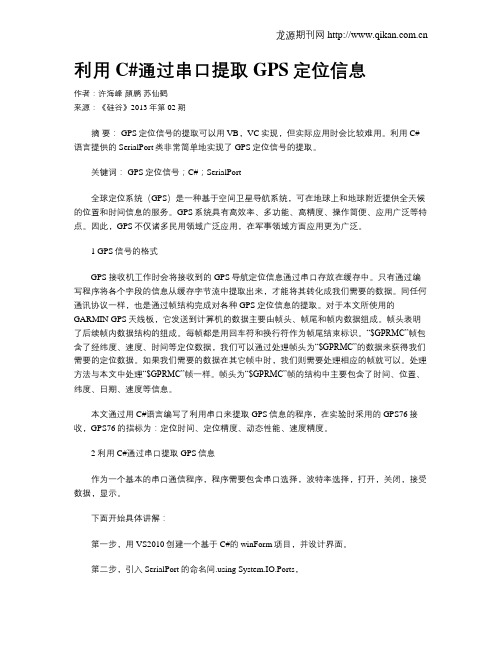
利用C#通过串口提取GPS定位信息作者:许海峰顔鹏苏仙鹤来源:《硅谷》2013年第02期摘要: GPS定位信号的提取可以用VB,VC实现,但实际应用时会比较难用。
利用C#语言提供的SerialPort类非常简单地实现了GPS定位信号的提取。
关键词: GPS定位信号;C#;SerialPort全球定位系统(GPS)是一种基于空间卫星导航系统,可在地球上和地球附近提供全天候的位置和时间信息的服务。
GPS系统具有高效率、多功能、高精度、操作简便、应用广泛等特点。
因此,GPS不仅诸多民用领域广泛应用,在军事领域方面应用更为广泛。
1 GPS信号的格式GPS接收机工作时会将接收到的GPS导航定位信息通过串口存放在缓存中。
只有通过编写程序将各个字段的信息从缓存字节流中提取出来,才能将其转化成我们需要的数据。
同任何通讯协议一样,也是通过帧结构完成对各种GPS定位信息的提取。
对于本文所使用的GARMIN GPS天线板,它发送到计算机的数据主要由帧头、帧尾和帧内数据组成。
帧头表明了后续帧内数据结构的组成。
每帧都是用回车符和换行符作为帧尾结束标识。
“$GPRMC”帧包含了经纬度、速度、时间等定位数据,我们可以通过处理帧头为“$GPRMC”的数据来获得我们需要的定位数据。
如果我们需要的数据在其它帧中时,我们则需要处理相应的帧就可以。
处理方法与本文中处理“$GPRMC”帧一样。
帧头为“$GPRMC”帧的结构中主要包含了时间、位置、纬度、日期、速度等信息。
本文通过用C#语言编写了利用串口来提取GPS信息的程序,在实验时采用的GPS76接收,GPS76的指标为:定位时间、定位精度、动态性能、速度精度。
2 利用C#通过串口提取GPS信息作为一个基本的串口通信程序,程序需要包含串口选择,波特率选择,打开,关闭,接受数据,显示。
下面开始具体讲解:第一步,用VS2010创建一个基于C#的winForm项目,并设计界面。
第二步,引入SerialPort的命名间.using System.IO.Ports,并实例化串口对象private SerialPort my = new SerialPort(),定义存储GPS文本信息的变量string gpstext = ""。
串口读取地磅电子秤C#源代码
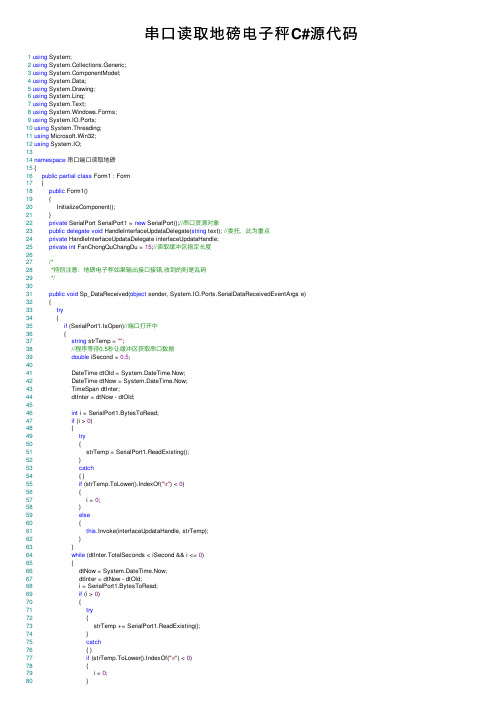
串⼝读取地磅电⼦秤C#源代码 1using System;2using System.Collections.Generic;3using ponentModel;4using System.Data;5using System.Drawing;6using System.Linq;7using System.Text;8using System.Windows.Forms;9using System.IO.Ports;10using System.Threading;11using Microsoft.Win32;12using System.IO;1314namespace串⼝端⼝读取地磅15 {16public partial class Form1 : Form17 {18public Form1()19 {20 InitializeComponent();21 }22private SerialPort SerialPort1 = new SerialPort();//串⼝资源对象23public delegate void HandleInterfaceUpdataDelegate(string text); //委托,此为重点24private HandleInterfaceUpdataDelegate interfaceUpdataHandle;25private int FanChongQuChangDu = 15;//读取缓冲区指定长度2627/*28 *特别注意:地磅电⼦秤如果输出接⼝接错,收到的则是乱码29*/3031public void Sp_DataReceived(object sender, System.IO.Ports.SerialDataReceivedEventArgs e)32 {33try34 {35if (SerialPort1.IsOpen)//端⼝打开中36 {37string strTemp = "";38//程序等待0.5秒让缓冲区获取串⼝数据39double iSecond = 0.5;4041 DateTime dtOld = System.DateTime.Now;42 DateTime dtNow = System.DateTime.Now;43 TimeSpan dtInter;44 dtInter = dtNow - dtOld;4546int i = SerialPort1.BytesToRead;47if (i > 0)48 {49try50 {51 strTemp = SerialPort1.ReadExisting();52 }53catch54 { }55if (strTemp.ToLower().IndexOf("\r") < 0)56 {57 i = 0;58 }59else60 {61this.Invoke(interfaceUpdataHandle, strTemp);62 }63 }64while (dtInter.TotalSeconds < iSecond && i <= 0)65 {66 dtNow = System.DateTime.Now;67 dtInter = dtNow - dtOld;68 i = SerialPort1.BytesToRead;69if (i > 0)70 {71try72 {73 strTemp += SerialPort1.ReadExisting();74 }75catch76 { }77if (strTemp.ToLower().IndexOf("\r") < 0)78 {79 i = 0;81else82 {83this.Invoke(interfaceUpdataHandle, strTemp);84 }85 }86 }87 }88 }89catch (Exception cw)90 {91 MessageBox.Show(cw.ToString());92return ;93 }9495 }9697private void UpdateTextBox(string text)98 {99100 txtShiShiZhongLiang.Text = text;101102if (SerialPort1.IsOpen)//端⼝打开103 {104 txtState.Text = "端⼝连接中";105 }106else107 {108 txtState.Text = "端⼝已断开";109 }110 }111112///<summary>113///执⾏AT指令并返回成功失败114///</summary>115///<param name="ATCmd">AT指令</param>116///<param name="StCmd">AT指令标准结束标识</param>117///<returns></returns>118private void ATCommand3(string ATCmd, string StCmd)119 {120string response = "";121 response = ATCommand(ATCmd, StCmd);122 }123124///<summary>125///执⾏AT指令并返回结果字符126///</summary>127///<param name="ATCmd">AT指令</param>128///<param name="StCmd">AT指令标准结束标识</param>129///<returns></returns>130private string ATCommand(string ATCmd, string StCmd)131 {132string response = "";133int i;134if (!ATCmd.EndsWith("\x01a"))135 {136if (!(ATCmd.EndsWith("\r") || ATCmd.EndsWith("\r\n")))137 {138 ATCmd = ATCmd + "\r";139 }140 }141 SerialPort1.WriteLine(ATCmd);142143//第⼀次读响应数据144if (SerialPort1.BytesToRead > 0)145 {146 response = SerialPort1.ReadExisting();147148//去除前端多可能多读取的字符149if (response.IndexOf(ATCmd) > 0)150 {151 response = response.Substring(response.IndexOf(ATCmd)); 152 }153else154 {155156 }157158if (response == "" || response.IndexOf(StCmd) < 0)159 {160if (response != "")161 {162if (response.Trim() == "ERROR")163 {165 }166if (response.IndexOf("+CMS ERROR") >= 0)167 {168string[] cols = new string[100];169 cols = response.Split(';');170if (cols.Length > 1)171 {172string errorCode = cols[1];173 }174 }175 }176 }177 }178179//读第⼀次没有读完的响应数据,直到读到特征数据或超时180for (i = 0; i < 3; i++)181 {182 Thread.Sleep(1000);183 response = response + SerialPort1.ReadExisting();184if (response.IndexOf(StCmd) >= 0)185 {186break;187 }188 }189190return response;191192193 }194195///<summary>196///从注册表获取系统串⼝列表197///</summary>198private void GetComList()199 {200 RegistryKey keyCom = Registry.LocalMachine.OpenSubKey("Hardware\\DeviceMap\\SerialComm"); 201if (keyCom != null)202 {203string[] sSubKeys = keyCom.GetValueNames();204this.cbxDuanKouHao.Items.Clear();205foreach (string sName in sSubKeys)206 {207string sValue = (string)keyCom.GetValue(sName);208this.cbxDuanKouHao.Items.Add(sValue);209 }210 }211 }212213private void cmID_DropDown(object sender, EventArgs e)214 {215 GetComList();216 }217218private void Form1_Load(object sender, EventArgs e)219 {220221 GetComList();222 btPause.Enabled = false;223 }224225//暂停监听读取数值226private void btPause_Click(object sender, EventArgs e)227 {228 SerialPort1.Close();229if (SerialPort1.IsOpen)//端⼝打开230 {231 txtState.Text = "端⼝连接中";232 }233else234 {235 txtState.Text = "端⼝已断开";236 }237 btENT.Enabled = true;238 btPause.Enabled = false;239240 }241//⼀直监听读取数值242private void btENT_Click(object sender, EventArgs e)243 {244if ((this.cbxDuanKouHao.Text.Trim() != "") && (this.cbxBoTeLv.Text != ""))245 {246if (SerialPort1 != null )247 {249 SerialPort1.DataReceived += new SerialDataReceivedEventHandler(Sp_DataReceived);250//串⼝更改251if (SerialPort1.PortName != this.cbxDuanKouHao.Text.Trim())252 {253 SerialPort1.Close();254 SerialPort1.Dispose();255 SerialPort1 = new SerialPort();//串⼝资源对象256 interfaceUpdataHandle = new HandleInterfaceUpdataDelegate(UpdateTextBox);//实例化委托对象257 SerialPort1.PortName = this.cbxDuanKouHao.Text.Trim();258 SerialPort1.BaudRate = Convert.ToInt32(this.cbxBoTeLv.Text.Trim());259 SerialPort1.Parity = JiaoYanWei(cbxJiaoYanWei.Text);260 SerialPort1.StopBits = TingZhiWei(cbxTingZhiWei.Text);261 SerialPort1.Encoding = Encoding.ASCII;//获取传输字符编码262 SerialPort1.DataReceived += new SerialDataReceivedEventHandler(Sp_DataReceived);263 SerialPort1.ReceivedBytesThreshold = 1;264try265 {266 SerialPort1.Open();267268 ATCommand3("AT+CLIP=1\r", "OK");269270271 btPause.Enabled = true;272 btENT.Enabled = false;273 }274catch (Exception)275 {276 MessageBox.Show("端⼝" + this.cbxDuanKouHao.Text.Trim() + "打开失败!");277 }278 }279else//串⼝未更改280 {281if(SerialPort1.IsOpen)//端⼝已打开282 {283 btPause.Enabled = true;284 btENT.Enabled = false;285 }286else287 {288 SerialPort1.Open();289 btPause.Enabled = true;290 btENT.Enabled = false;291 }292 }293 }294else295 {296 interfaceUpdataHandle = new HandleInterfaceUpdataDelegate(UpdateTextBox);//实例化委托对象297 SerialPort1.PortName = this.cbxDuanKouHao.Text.Trim();298 SerialPort1.BaudRate = Convert.ToInt32(this.cbxBoTeLv.Text.Trim());299 SerialPort1.Parity = JiaoYanWei(cbxJiaoYanWei.Text);300 SerialPort1.StopBits = TingZhiWei(cbxTingZhiWei.Text);301 SerialPort1.Encoding = Encoding.ASCII;//获取传输字符编码302 SerialPort1.DataReceived += new SerialDataReceivedEventHandler(Sp_DataReceived);303 SerialPort1.ReceivedBytesThreshold = 1;304try305 {306 SerialPort1.Open();307308 ATCommand3("AT+CLIP=1\r", "OK");309310311 btPause.Enabled = true;312 btENT.Enabled = false;313 }314catch (Exception)315 {316 MessageBox.Show("端⼝" + this.cbxDuanKouHao.Text.Trim() + "打开失败!");317 }318 }319 }320else321 {322 MessageBox.Show("请输⼊正确的端⼝号和波特率!");323this.cbxDuanKouHao.Focus();324 }325 }326327///<summary>328///读⼀次数:注意电⼦秤设置的发送信息规则:如果不是连续发送,是稳定后发送,则有时候读不到数329///</summary>330///<param name="sender"></param>331///<param name="e"></param>334try335 {336if ((this.cbxDuanKouHao.Text.Trim() != "") && (this.cbxBoTeLv.Text != ""))337 {338if (SerialPort1 != null)339 {340 SerialPort1.Close();341 SerialPort1.Dispose();342 SerialPort1 = new SerialPort();//重新创建串⼝资源对象,否则导致程序卡死343//串⼝更改344if (SerialPort1.PortName != this.cbxDuanKouHao.Text.Trim())345 {346 SerialPort1.PortName = this.cbxDuanKouHao.Text.Trim();347 SerialPort1.BaudRate = Convert.ToInt32(this.cbxBoTeLv.Text.Trim());348 SerialPort1.Parity = JiaoYanWei(cbxJiaoYanWei.Text);349 SerialPort1.StopBits = TingZhiWei(cbxTingZhiWei.Text);350 SerialPort1.Encoding = Encoding.ASCII;//获取传输字符编码351 SerialPort1.ReceivedBytesThreshold = 1;352try353 {354 SerialPort1.Open();355356 ATCommand3("AT+CLIP=1\r", "OK");357this.txtShiShiZhongLiang.Text = dushu();358 }359catch (Exception)360 {361 MessageBox.Show("端⼝" + this.cbxDuanKouHao.Text.Trim() + "打开失败!"); 362 }363 }364else//串⼝未更改365 {366if (SerialPort1.IsOpen)//端⼝已打开367 {368 ATCommand3("AT+CLIP=1\r", "OK");369this.txtShiShiZhongLiang.Text = dushu();370 }371else372 {373 SerialPort1.Open();374 ATCommand3("AT+CLIP=1\r", "OK");375this.txtShiShiZhongLiang.Text = dushu();376 }377 }378 }379else380 {381382 SerialPort1.PortName = this.cbxDuanKouHao.Text.Trim();383 SerialPort1.BaudRate = Convert.ToInt32(this.cbxBoTeLv.Text.Trim());384 SerialPort1.Parity = JiaoYanWei(cbxJiaoYanWei.Text);385 SerialPort1.StopBits = TingZhiWei(cbxTingZhiWei.Text);386387 SerialPort1.ReceivedBytesThreshold = 1;388try389 {390 SerialPort1.Open();391392 ATCommand3("AT+CLIP=1\r", "OK");393this.txtShiShiZhongLiang.Text = dushu();394 }395catch (Exception)396 {397 MessageBox.Show("端⼝" + this.cbxDuanKouHao.Text.Trim() + "打开失败!"); 398 }399 }400 }401else402 {403 MessageBox.Show("请输⼊正确的端⼝号和波特率!");404this.cbxDuanKouHao.Focus();405 }406if (SerialPort1.IsOpen)407 {408 SerialPort1.Close();409 }410 }411catch (Exception)412 {413414return;415 }419///读取缓冲区指定长度数值420///</summary>421///<param name="changdu"></param>422///<returns></returns>423private string dushu()424 {425string strTemp = "";426try427 {428if (SerialPort1.IsOpen)//端⼝打开中429 {430431int i = SerialPort1.BytesToRead;//获取缓冲区字节数432433#region程序等待0.5秒让缓冲区获取串⼝数据434double iSecond = 0.5;435436 DateTime dtOld = System.DateTime.Now;437 DateTime dtNow = System.DateTime.Now;438 TimeSpan dtInter;439 dtInter = dtNow - dtOld;440441while (dtInter.TotalSeconds < iSecond && i <= 0)442 {443 dtNow = System.DateTime.Now;444 dtInter = dtNow - dtOld;445 i = SerialPort1.BytesToRead;446 }447#endregion448if (i > 0)449 {450try451 {452453byte[] buf = new byte[FanChongQuChangDu];// 定义接收缓冲区454 SerialPort1.Read(buf, 0, FanChongQuChangDu);455for (int j = 0; j < FanChongQuChangDu; j++)456 {457458 ASCIIEncoding ASCIITochar = new ASCIIEncoding();459char[] ascii = ASCIITochar.GetChars(buf); // 将接收字节解码为ASCII字符数组460 strTemp += ascii[j];461//已读到换⾏符就跳出462if (strTemp.ToLower().IndexOf("\r") > 0)463 {464break;465 }466 }467 }468catch469 { }470 }471 }472 }473catch (Exception)474 {475476return strTemp;477 }478return strTemp;479 }480481private void btnZhuaQu_Click(object sender, EventArgs e)482 {483this.txtZhuaQuZhi.Text = this.txtShiShiZhongLiang.Text;484 }485///<summary>486///返回校验位487///</summary>488///<param name="zhi"></param>489///<returns></returns>490private Parity JiaoYanWei(string zhi)491 {492switch (zhi)493 {494case"Even": return Parity.Even;495case"Mark": return Parity.Mark;496case"None": return Parity.None;497case"Odd": return Parity.Odd;498case"Space": return Parity.Space;499default: return Parity.None;503///返回停⽌位504///</summary>505///<param name="zhi"></param>506///<returns></returns>507private StopBits TingZhiWei(string zhi)508 {509switch (zhi)510 {511case"0": return StopBits.None;512case"1": return StopBits.One;513case"1.5": return StopBits.OnePointFive;514case"2": return StopBits.Two;515default: return StopBits.One;516 }517 }518///<summary>519/// ASCII码转字符520///</summary>521///<param name="asciiCode"></param>522///<returns></returns>523public static string asciiToString(int asciiCode)524 {525if (asciiCode >= 0 && asciiCode <= 255)526 {527 System.Text.ASCIIEncoding asciiEncoding = new System.Text.ASCIIEncoding();528byte[] byteArray = new byte[] { (byte)asciiCode };529string strCharacter = asciiEncoding.GetString(byteArray);530return (strCharacter);531 }532else533 {534throw new Exception("ASCII Code is not valid.");535 }536 }537//⽤封装对象来实现538private void button1_Click(object sender, EventArgs e)539 {540try541 {542 mycom mycom1 = new mycom();543 mycom1.PortNum = cbxDuanKouHao.Text;544 mycom1.BaudRate = Convert.ToInt32(cbxBoTeLv.Text);545 mycom1.StopBits = 8;546 mycom1.Parity = 0;547 mycom1.StopBits = Convert.ToByte(cbxTingZhiWei.Text);548 mycom1.ReadTimeout = 10;549550 mycom1.Open();551string dushu = "";552byte[] buf = mycom1.Read(12);553554for (int j = 0; j < 12; j++)555 {556557 ASCIIEncoding ASCIITochar = new ASCIIEncoding();558char[] ascii = ASCIITochar.GetChars(buf); // 将接收字节解码为ASCII字符数组559 dushu += ascii[j];560561 }562563 txtShiShiZhongLiang.Text = dushu;564565 mycom1.Close();566 }567catch (Exception cuowu)568 {569 MessageBox.Show(cuowu.Message);570return ;571 }572573 }574 }575 }。
电子秤使用串口与计算机进行通讯

BE01型仪表通讯编程现有电子秤一台,使用串口与计算机进行通讯。
编写VB程序来访问串口,达到读取电子秤上显示的数据。
该电子秤为BE01型仪表,输出为RS-232C标准接口,波特率为300-9600、偶校验、7个数据位、2个停止位。
所有字符均发送11位ASCII码,一个起始位。
在VB中与串口通讯需要引入控件MSComm 串口通讯控件(在Microsoft Comm Control 6.0中)。
具体程序如下:控件简称:MSCDim Out(12) As Byte '接收var中的值Dim var As Variant '接收MSC.input中的数值Dim nRece As Integer '计算MSC.inputbuffer的个数Dim i As Integer, j As Integer '随即变量,计算循环******************************************************************** ********Private Sub Form_Load()ClearTextWith MSC.CommPort = 1 '设置Com1为通信端口.Settings = "9600,E,7,2" '设置通信端口参数 9600赫兹、偶校验、7个数据位、1个停止位.(这里需要进一步说明的是:.Setting=”BBBB,P,D,S”。
含义是:B:Baud Rate(波特率);P:Parity(奇偶);D:Data Bit;S:Stop Bit).InBufferSize = 40 '设置缓冲区接收数据为40字节.InputLen = 1 '设置Input一次从接收缓冲读取字节数为1.RThreshold = 1 '设置接收一个字节就产生OnComm事件End WithEnd Sub******************************************************************** ********Private Sub ClearText()Text3.Text = ""Text2.Text = "5"Text1.Text = ""End SubPrivate Sub Command1_Click()ClearText' nRece = 0 '计数器清零With MSC.InputMode = comInputModeBinary '设置数据接收模式为二进制形式.InBufferCount = 0 '清除接收缓冲区If Not .PortOpen Then.PortOpen = True '打开通信端口End IfEnd WithEnd SubPrivate Sub MSC_OnComm()DelayTime ‘用来延续时间ClearTextWith MSCSelect Case .CommEvent '判断通信事件Case comEvReceive: '收到Rthreshold个字节产生的接收事件SwichVar 1If Out(1) = 2 Then '判断是否为数据的开始标志.RThreshold = 0 '关闭OnComm事件接收End IfDoDoEventsLoop Until .InBufferCount >= 3 '循环等待接收缓冲区>=3个字节' nRece = nRece + 1For i = 2 To 12SwichVar iText1.Text = Text1.Text & Chr(Out(i))NextText1.Text = LTrim(Text1.Text)Text2.Text = Text2.Text & CStr(nRece).RThreshold = 1 '打开MSComm事件接收Case Else' .PortOpen = FalseEnd SelectEnd WithEnd Sub******************************************************************** ********Private Sub DelayTime()Dim bDT As BooleanDim sPrevious As Single, sLast As SinglebDT = TruesPrevious = Timer (Timer可以计算从子夜到现在所经过的秒数,在Microsoft Windows中,Timer函数可以返回一秒的小数部分)Do While bDTIf Timer - sPrevious >= 0.3 Then bDT = FalseLoopbDT = TrueEnd Sub(通信传输速率为9600bps,则最快速度1.04ms发送一个字节,仪表每秒发送50帧数据,每帧数据有4个字节,即每秒发送200个字节,平均5.0ms 发送一个字节,连续读取串口数据时要在程序中添加循环等待程序)Private Sub SwichVar(ByVal nNum As Integer)DelayTimevar = Nullvar = MSC.InputOut(nNum) = var(0)End Sub(设置接收数据模式采用二进制形式,即 InputMode=comInputModeBinary,但用Input属性读取数据时,不能直接赋值给 Byte 类型变量,只能通过先赋值给一个 Variant 类型变量,返回一个二进制数据的数组,再转换保存到Byte 类型数变量中。
c++中的serialport用法
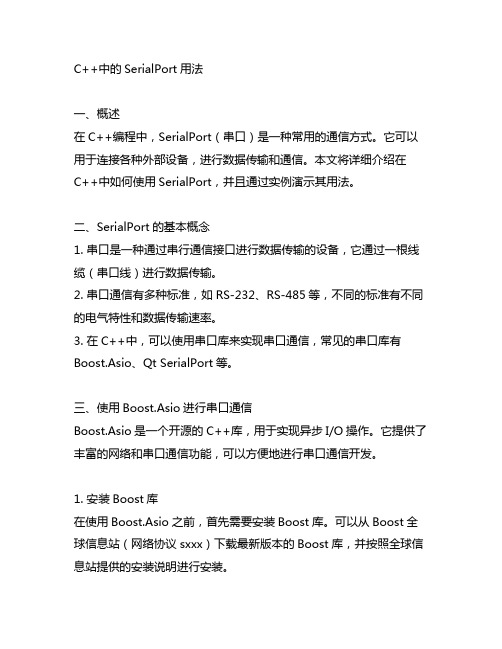
C++中的SerialPort用法一、概述在C++编程中,SerialPort(串口)是一种常用的通信方式。
它可以用于连接各种外部设备,进行数据传输和通信。
本文将详细介绍在C++中如何使用SerialPort,并且通过实例演示其用法。
二、SerialPort的基本概念1. 串口是一种通过串行通信接口进行数据传输的设备,它通过一根线缆(串口线)进行数据传输。
2. 串口通信有多种标准,如RS-232、RS-485等,不同的标准有不同的电气特性和数据传输速率。
3. 在C++中,可以使用串口库来实现串口通信,常见的串口库有Boost.Asio、Qt SerialPort等。
三、使用Boost.Asio进行串口通信Boost.Asio是一个开源的C++库,用于实现异步I/O操作。
它提供了丰富的网络和串口通信功能,可以方便地进行串口通信开发。
1. 安装Boost库在使用Boost.Asio之前,首先需要安装Boost库。
可以从Boost全球信息站(网络协议sxxx)下载最新版本的Boost库,并按照全球信息站提供的安装说明进行安装。
2. 创建SerialPort对象在C++中使用Boost.Asio库进行串口通信,首先需要创建一个SerialPort对象,并指定串口名称、波特率等参数。
```c++#include <boost/asio.hpp>using namespace boost::asio;// 创建SerialPort对象io_service io;serial_port serial(io, "COM1"); // 指定串口名称serial.set_option(serial_port::baud_rate(9600)); // 设置波特率```3. 读写串口数据创建好SerialPort对象之后,即可通过它进行串口数据的读写操作。
```c++// 向串口写入数据std::string write_data = "Hello, SerialPort!";write(serial, buffer(write_data));// 从串口读取数据char read_data[100];size_t len = read(serial, buffer(read_data, 100));```4. 异步串口通信Boost.Asio库支持异步串口通信,可以通过回调函数处理串口数据的读写操作。
有关JavaScript获取电子秤串口数据的编程代码

//绑定事件 fn(); } } catch(err) { alert(err.on(){ functionMSComm1::OnComm(){ MSComm1_OnComm(); } } //事件响应 functionMSComm1_OnComm() {
{ MSComm1=newAcTIveXObject(MSCOMMLib.MSComm.1); if((typeof(MSComm1)==undefined)||(MSComm1==null)) { alert(创建 MSComm1 对象失败!); } else {
{ if(MSComm1.PortOpen==true) { try{MSComm1.PortOpen=false; SKButton1.value=打开串口; }catch(ex) {alert(ex.message);} }
有关 JavaScript 获取电子秤串口数据的编程代码
JavaScript 一种直译式脚本语言,是一种动态类型、弱类型、基于原型的 语言,内置支持类型。它的解释器被称为 JavaScript 引擎,为浏览器的一部 分,广泛用于客户端的脚本语言,最早是在 HTML(标准通用标记语言下的 一个应用)网页上使用,用来给 HTML 网页增加动态功能。 本文介绍有关 JavaScript 获取电子秤串口数据的编程代码。 有关 JavaScript 获取电子秤串口数据的完整代码 //创建 MSComm 对象 funcTIonuf_GetSerPortData() { try
else{ try{MSComm1.PortOpen=true; MSComm1.InBufferCount=0; SKButton1.value=关闭串口; }catch(ex) {alert(ex.message);} } }
利用C#通过串口提取GPS定位信息
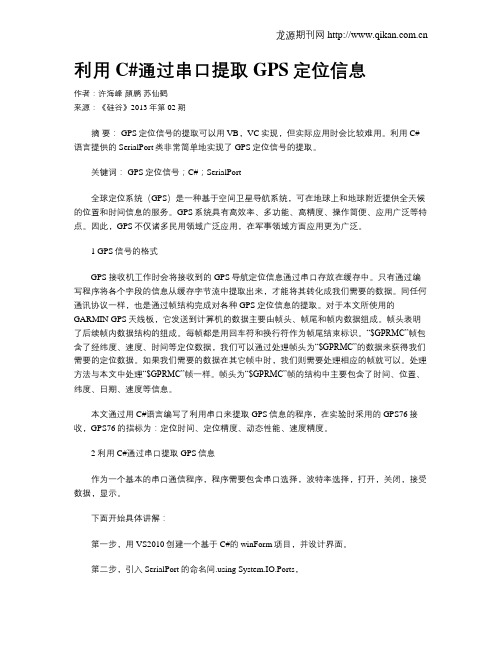
利用C#通过串口提取GPS定位信息作者:许海峰顔鹏苏仙鹤来源:《硅谷》2013年第02期摘要: GPS定位信号的提取可以用VB,VC实现,但实际应用时会比较难用。
利用C#语言提供的SerialPort类非常简单地实现了GPS定位信号的提取。
关键词: GPS定位信号;C#;SerialPort全球定位系统(GPS)是一种基于空间卫星导航系统,可在地球上和地球附近提供全天候的位置和时间信息的服务。
GPS系统具有高效率、多功能、高精度、操作简便、应用广泛等特点。
因此,GPS不仅诸多民用领域广泛应用,在军事领域方面应用更为广泛。
1 GPS信号的格式GPS接收机工作时会将接收到的GPS导航定位信息通过串口存放在缓存中。
只有通过编写程序将各个字段的信息从缓存字节流中提取出来,才能将其转化成我们需要的数据。
同任何通讯协议一样,也是通过帧结构完成对各种GPS定位信息的提取。
对于本文所使用的GARMIN GPS天线板,它发送到计算机的数据主要由帧头、帧尾和帧内数据组成。
帧头表明了后续帧内数据结构的组成。
每帧都是用回车符和换行符作为帧尾结束标识。
“$GPRMC”帧包含了经纬度、速度、时间等定位数据,我们可以通过处理帧头为“$GPRMC”的数据来获得我们需要的定位数据。
如果我们需要的数据在其它帧中时,我们则需要处理相应的帧就可以。
处理方法与本文中处理“$GPRMC”帧一样。
帧头为“$GPRMC”帧的结构中主要包含了时间、位置、纬度、日期、速度等信息。
本文通过用C#语言编写了利用串口来提取GPS信息的程序,在实验时采用的GPS76接收,GPS76的指标为:定位时间、定位精度、动态性能、速度精度。
2 利用C#通过串口提取GPS信息作为一个基本的串口通信程序,程序需要包含串口选择,波特率选择,打开,关闭,接受数据,显示。
下面开始具体讲解:第一步,用VS2010创建一个基于C#的winForm项目,并设计界面。
第二步,引入SerialPort的命名间.using System.IO.Ports,并实例化串口对象private SerialPort my = new SerialPort(),定义存储GPS文本信息的变量string gpstext = ""。
串口读取数据的方法
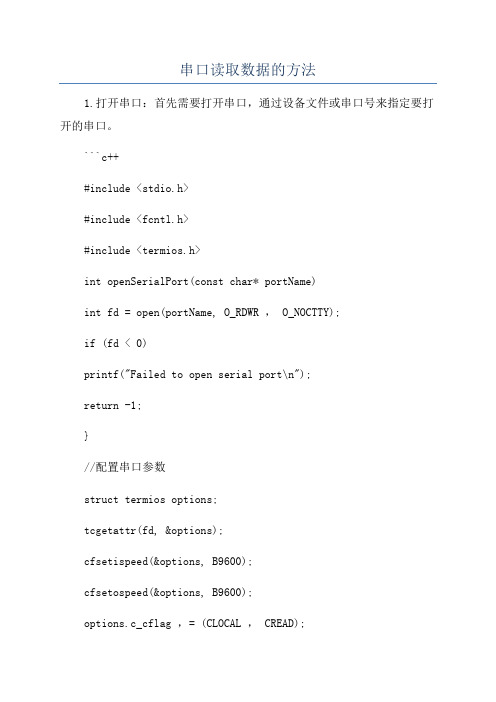
串口读取数据的方法1.打开串口:首先需要打开串口,通过设备文件或串口号来指定要打开的串口。
```c++#include <stdio.h>#include <fcntl.h>#include <termios.h>int openSerialPort(const char* portName)int fd = open(portName, O_RDWR , O_NOCTTY);if (fd < 0)printf("Failed to open serial port\n");return -1;}//配置串口参数struct termios options;tcgetattr(fd, &options);cfsetispeed(&options, B9600);cfsetospeed(&options, B9600);options.c_cflag ,= (CLOCAL , CREAD);tcsetattr(fd, TCSANOW, &options);return fd;}```2.读取串口数据:打开串口之后,可以通过读取文件描述符来读取串口数据。
```c++int readSerialData(int fd, unsigned char* buffer, int bufferSize)int bytesRead = read(fd, buffer, bufferSize);if (bytesRead < 0)printf("Failed to read serial data\n");}return bytesRead;}```3.解析串口数据:读取到的数据可能是原始的字节流,需要根据具体的协议和数据格式进行解析。
```c++void parseData(unsigned char* buffer, int bufferSize)//解析数据的逻辑}```4.循环读取数据:可以使用循环来不断读取串口数据,并进行解析和处理。
java电子秤读取数据

package serial;import gnu.io.SerialPort;import java.util.HashMap;public class CommTest {public static void main(String[] args) {HashMap<String, Comparable> params = new HashMap<String, Comparable>(); params.put(SerialReader.PARAMS_PORT, "COM8"); // 端口名称params.put(SerialReader.PARAMS_RATE, 9600); // 波特率params.put(SerialReader.PARAMS_TIMEOUT, 1000); // 设备超时时间1秒params.put(SerialReader.PARAMS_DELAY, 200); // 端口数据准备时间1秒params.put(SerialReader.PARAMS_DATABITS, SerialPort.DATABITS_8); // 数据位params.put(SerialReader.PARAMS_STOPBITS, SerialPort.STOPBITS_1); // 停止位params.put(SerialReader.PARAMS_PARITY, SerialPort.PARITY_NONE); // 无奇偶校验SerialReader sr = new SerialReader(params);CommDataObserver bob = new CommDataObserver("bob");CommDataObserver joe = new CommDataObserver("joe");sr.addObserver(joe);sr.addObserver(bob);}}---------------- 2 -----------------package serial;import mPort;import mPortIdentifier;import gnu.io.NoSuchPortException;import gnu.io.PortInUseException;import gnu.io.SerialPort;import gnu.io.SerialPortEvent;import gnu.io.SerialPortEventListener;import gnu.io.UnsupportedCommOperationException;import java.io.IOException;import java.io.InputStream;import java.util.Enumeration;import java.util.HashMap;import java.util.HashSet;import java.util.Observable;import java.util.TooManyListenersException;/*** 串口数据读取类,用于windows的串口数据读取**/public class SerialReader extends Observable implements Runnable, SerialPortEventListener {static CommPortIdentifier portId;int delayRead = 200;int numBytes; // buffer中的实际数据字节数private static byte[] readBuffer = new byte[4096]; // 4k的buffer空间,缓存串口读入的数据static Enumeration portList;InputStream inputStream;SerialPort serialPort;HashMap serialParams;// 端口读入数据事件触发后,等待n毫秒后再读取,以便让数据一次性读完public static final String PARAMS_DELAY = "delay read"; // 延时等待端口数据准备的时间public static final String PARAMS_TIMEOUT = "timeout"; // 超时时间public static final String PARAMS_PORT = "port name"; // 端口名称public static final String PARAMS_DATABITS = "data bits"; // 数据位public static final String PARAMS_STOPBITS = "stop bits"; // 停止位public static final String PARAMS_PARITY = "parity"; // 奇偶校验public static final String PARAMS_RATE = "rate"; // 波特率/*** 初始化端口操作的参数.*** @see*/public SerialReader(HashMap params) {serialParams = params;init();}private void init() {try {// 参数初始化int timeout = Integer.parseInt(serialParams.get(PARAMS_TIMEOUT).toString());int rate = Integer.parseInt(serialParams.get(PARAMS_RATE).toString());int dataBits = Integer.parseInt(serialParams.get(PARAMS_DATABITS).toString());int stopBits = Integer.parseInt(serialParams.get(PARAMS_STOPBITS).toString());int parity = Integer.parseInt(serialParams.get(PARAMS_PARITY).toString());delayRead = Integer.parseInt(serialParams.get(PARAMS_DELAY).toString());String port = serialParams.get(PARAMS_PORT).toString();// 打开端口portId = CommPortIdentifier.getPortIdentifier(port);serialPort = (SerialPort) portId.open("SerialReader", timeout);inputStream = serialPort.getInputStream();serialPort.addEventListener(this);serialPort.notifyOnDataAvailable(true);serialPort.setSerialPortParams(rate, dataBits, stopBits, parity);} catch (PortInUseException e) {System.out.println("端口已经被占用!");e.printStackTrace();} catch (TooManyListenersException e) {System.out.println("端口监听者过多!");e.printStackTrace();} catch (UnsupportedCommOperationException e) {System.out.println("端口操作命令不支持!");e.printStackTrace();} catch (NoSuchPortException e) {System.out.println("端口不存在!");e.printStackTrace();} catch (IOException e) {e.printStackTrace();}Thread readThread = new Thread(this);readThread.start();}/*** Method declaration*** @see*/public void run() {try {Thread.sleep(100);} catch (InterruptedException e) {}}/*** Method declaration*** @param event** @see*/public void serialEvent(SerialPortEvent event) {try {// 等待1秒钟让串口把数据全部接收后在处理Thread.sleep(delayRead);System.out.print("serialEvent[" + event.getEventType() + "] "); } catch (InterruptedException e) {e.printStackTrace();}switch (event.getEventType()) {case SerialPortEvent.BI: // 10case SerialPortEvent.OE: // 7case SerialPortEvent.FE: // 9case SerialPortEvent.PE: // 8case SerialPortEvent.CD: // 6case SerialPortEvent.CTS: // 3case SerialPortEvent.DSR: // 4case SerialPortEvent.RI: // 5case SerialPortEvent.OUTPUT_BUFFER_EMPTY: // 2break;case SerialPortEvent.DATA_AVAILABLE: // 1try {// 多次读取,将所有数据读入// while (inputStream.available() > 0) {// numBytes = inputStream.read(readBuffer);// }numBytes = inputStream.read(readBuffer);changeMessage(readBuffer, numBytes);} catch (IOException e) {e.printStackTrace();}break;}}// 通过observer pattern将收到的数据发送给observer// 将buffer中的空字节删除后再发送更新消息,通知观察者public void changeMessage(byte[] message, int length) {setChanged();byte[] temp = new byte[length];System.arraycopy(message, 0, temp, 0, length);// System.out.println("msg[" + numBytes + "]: [" + new String(temp) + "]"); notifyObservers(temp);}static void listPorts() {Enumeration portEnum = CommPortIdentifier.getPortIdentifiers();while (portEnum.hasMoreElements()) {CommPortIdentifier portIdentifier = (CommPortIdentifier) portEnum.nextElement(); System.out.println(portIdentifier.getName() + " - "+ getPortTypeName(portIdentifier.getPortType()));}}static String getPortTypeName(int portType) {switch (portType) {case CommPortIdentifier.PORT_I2C:return "I2C";case CommPortIdentifier.PORT_PARALLEL:return "Parallel";case CommPortIdentifier.PORT_RAW:return "Raw";case CommPortIdentifier.PORT_RS485:return "RS485";case CommPortIdentifier.PORT_SERIAL:return "Serial";default:return "unknown type";}}/*** @return A HashSet containing the CommPortIdentifier for all serial ports that are not * currently being used.*/public static HashSet<CommPortIdentifier> getAvailableSerialPorts() {HashSet<CommPortIdentifier> h = new HashSet<CommPortIdentifier>(); Enumeration thePorts = CommPortIdentifier.getPortIdentifiers();while (thePorts.hasMoreElements()) {CommPortIdentifier com = (CommPortIdentifier) thePorts.nextElement();switch (com.getPortType()) {case CommPortIdentifier.PORT_SERIAL:try {CommPort thePort = com.open("CommUtil", 50);thePort.close();h.add(com);} catch (PortInUseException e) {System.out.println("Port, " + com.getName() + ", is in use.");} catch (Exception e) {System.out.println("Failed to open port " + com.getName() + e);}}}return h;}}---------- 3 -----------------package serial;import java.util.Observable;import java.util.Observer;class CommDataObserver implements Observer {String name;public CommDataObserver(String name) { = name;}public void update(Observable o, Object arg) {System.out.println("[" + name + "] GetMessage:\n [" + new String((byte[]) arg) + "]"); }}。
serialport_datareceived用法

serialport_datareceived用法使用串口数据接收是在开发中经常遇到的一种情况。
无论是使用串口与外部设备进行通信,还是与其他设备进行数据交换,SerialPort类都是非常常用的工具之一。
在这篇文章中,我们将会一步一步地回答关于串口数据接收的问题,帮助读者全面了解这个主题。
第一步:了解SerialPort类在开始之前,我们首先需要对SerialPort类进行一个简单的介绍。
SerialPort 类是.NET框架提供的一个用于串行通信的类库,主要用于直接通过串口与外设进行数据交换。
通过SerialPort类,我们可以方便地发送和接收数据,监测串口的状态,并对串口进行各种设置。
第二步:初始化SerialPort对象在开始接收数据之前,我们需要首先初始化一个SerialPort对象。
我们可以通过以下步骤来完成初始化:1. 引入命名空间:在代码文件的开头,我们需要引入命名空间System.IO.Ports,这个命名空间提供了SerialPort类的定义。
2. 创建SerialPort对象:我们可以使用关键字new来实例化一个SerialPort对象,并指定串口号和波特率等参数。
3. 配置串口参数:通过SerialPort对象的属性,我们可以对串口进行各种设置,如波特率、数据位、停止位、奇偶校验等。
第三步:设置数据接收事件接下来,我们需要设置一个数据接收事件,以便在接收到数据时进行相应的操作。
在SerialPort类中,有一个名为DataReceived的事件,它会在接收到数据时触发。
通过订阅这个事件,我们就可以在数据到达时执行自定义的处理函数。
1. 创建事件处理函数:首先,我们需要创建一个事件处理函数,用于处理接收到的数据。
这个函数可以是一个无返回值的方法,接受两个参数:一个是触发事件的对象,另一个是包含事件数据的EventArgs对象。
2. 订阅DataReceived事件:通过将事件处理函数与DataReceived事件进行绑定,我们可以在数据接收到时自动触发事件处理函数。
串口读取称或是地磅数据

串⼝读取称或是地磅数据论坛⾥经常碰到称或是地磅对接的问题,想当年我也是⼀路问过来啊,⾃动⼀⼈在客户那磨练下就知道怎么做的了,今天总结下吧。
我接触的称或是地磅都是串⼝输出数据,貌似都是串⼝输出(可能有别的我没接触到的)。
1、利⽤串⼝调试助⼿接收数据⾸先我们来了解称是怎么把数据传输出来的,⼀般情况称传出模式有2中, 模式⼀是连续发送,换句话说就是⼀直向外发数据,接收到数据后按照协议规则剥离出你要的数据就可以。
模式⼆种是应答式,就是按照协议格式发送⼀组字符串或是16进制字符串,称提给你回应,按照规则你剥离出你要的数据就可以了。
知道称数据发送⽅式了,那下步我们要知道怎么去接受传输过来的数据, 我⽤⼀个⽐喻来形容下:引⽔灌溉,⽔库的⽔引到庄稼地浇灌 ⽔库的⽔外放,肯定有规则的,浇灌多少地,⽔流量多⼤,传输多远,对于⽔库的管理者都有规定,这好⽐称⼚家的协议。
对于庄稼户,要⽤⽔,肯定要修渠道,渠道是多宽合适,这个根据⽔库管理者的建议,这个好⽐对照称称⼚家的协议。
例⼦只是⼀个例⼦不算很得当,希望阅读者能够理解。
哪些是要设置的协议呢:串⼝号,波特率,校验位,数据位,停⽌位。
串⼝号:本机要接收数据的串⼝号;波特率,校验位,数据库,停⽌位,这些都是有⼚家来给你提供的,只要按照他们给的标准设置就可以了,如下图当然还有重要的⼀点⼀定要把称和电脑连接上,⼀般选择屏蔽线作为传输介质。
如果没有问题就可以接收到数据了(连续发送模式)。
2、程序接收数据 想必通过上⾯的说明你应该了解怎么接收数据了,⾃⼰写程序接收数据,道理⼀样的,1、程序加载后把对应的串⼝,波特率,校验位,停⽌位设置正确mycom1.PortNum=iPort;mycom1.BaudRate=iRate;mycom1.ByteSize=bSize;mycom1.Parity=bParity;mycom1.StopBits=bStopBits;mycom1.ReadTimeout=iTimeout;if(this.OpenCom())msg.AppendText("串⼝初始化成功……");elsemsg.AppendText("串⼝初始化失败!");2、打开串⼝,接收发过来的数据//开串⼝public bool OpenCom(){try{ if (mycom1.Opened) { mycom1.Close(); mycom1.Open(); //打开串⼝ } else { mycom1.Open();//打开串⼝ } return true;}catch(Exception e){ MessageBox.Show("错误:" + e.Message); return false;}}接收数据public string dis_package(byte[] reb){string temp="";foreach(byte b in reb)temp+=b.ToString("X2")+" ";return temp;}数据怎么处理看你的需求了在次声明下,我⽤到基础函数是这个博主写的,关于串⼝的⼀个demo 如果你还不知道怎么做,去下个demo细细的研究下吧。
基于Java实现的Modbus通信模块电气设备数据采集

设计应用Modbus通信模块电气设备数据采集王勇平,张英豪(广州致新电力科技有限公司,广东广州作为一种工业通信标准,已经被广泛应用于工业实践。
根据通信中网络类型的不同,Modbus串行两种。
TCP/IP方式的底层实现依赖于方式的底层实现则依赖于异步串行传输(通常是RS-232/422/485)即串口通信。
基于此,主要研究和分析这两种通信方式下,不同电气设备数据采集中的传感器通信、通信中的数据格式、数据解析以通信可以快速建立主从机之间的通信,采集和存储环境温湿度、电流电压功率以及光强信号等数据,在一般的工业实践中具有较好的运用价值。
Modbus;TCP;RTU;传感器;数据采集Data Acquisition of Electrical Equipment in Modbus Communication Module Based on JavaWANG Yongping,ZHANG YinghaoGuangzhou Zhixin Power Technology Co.,Ltd.communication standard,Modbus况和校验码,以说明数据的接收是否准确和读写操作Modbus TCP协议要求每个通信对象必须要知道此通信链路中每个节点的地址和端口号[9]。
根据地址和端口号确定一个通信节点,识别通信节点中发送的数据,并且产生对应的响应。
响应时,通信发出。
其他网络中包含了此数据转换的包结构。
这种转换为网络解决节将传感器与其接收装置接电后,通过网线将传连接进行网络通信。
此通信是基技术,通过例转换关系。
数据解析以下位机响应报文(0292 FF小于0 ℃转10进制是实温度为在应用程序中,使用的开发语言是据实际业务需求在在程序中使用定时任务将采集的数据解析后封装为对应的对象,并使用3.5 用在Java使用的实现方式也不相同,主要分为Modbus RTU3.5.1用程序中, 2020年10月25日第37卷第20期接口获取串口连接对象。
使用.net的SerialPort串口监控控件接收串口数据

前述:在一个项目中,需要从串口通信的磁卡读卡器中读取刷卡的卡号,以便后续的业务操作。
该刷卡 器的型号为:SMR-RU2,使用USB 接入电脑,通过USB 转串口,Windows 会根据USB 口分配器固定的串口号。
本人使用了.net 中自带的SerialPort 串口监控控件来读取磁卡读卡器所读取的卡号,下面讲述具体细节。
使用SerialPort 接受串口信号:首先在创建一个From ,并且在工具箱中拖入SerialPort 控件,在这里我命名为mySerialPort ,并拖进一个TextBox,一遍显示信号数据。
接着初始化SerialPort 控件,代码如下:1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 <span style="white-space:pre"> </span>/// 初始化控件/// </summary>/// <param name="portName"></param> private void InitMySerialPort(String portName) { if (mySerialPort != null) { mySerialPort.PortName = portName;//端口号,这里可以电脑已经连接的COM 口,如COM1; mySerialPort.DataBits = 8;//设置每个字节的数据位,在这里我设置为8位,可以为7位 mySerialPort.DiscardNull = false; mySerialPort.DtrEnable = false; mySerialPort.Handshake = Handshake.None; mySerialPort.Parity = Parity.None; mySerialPort.ParityReplace = Convert.ToByte("63"); mySerialPort.RtsEnable = false; mySerialPort.StopBits = StopBits.One; } if (!mySerialPort.IsOpen) { mySerialPort.Open();//打开端口,进行监控 } mySerialPort.DataReceived += new SerialDataReceivedEventHandler(serialPort_DataReceived);//这个事件为最关键点,一旦端口 收到信号,就会触发该事件,这个事件就是真正读取信号,以便做接下的业务。
关于电子秤串口配置与把枪串口配置问题帮助
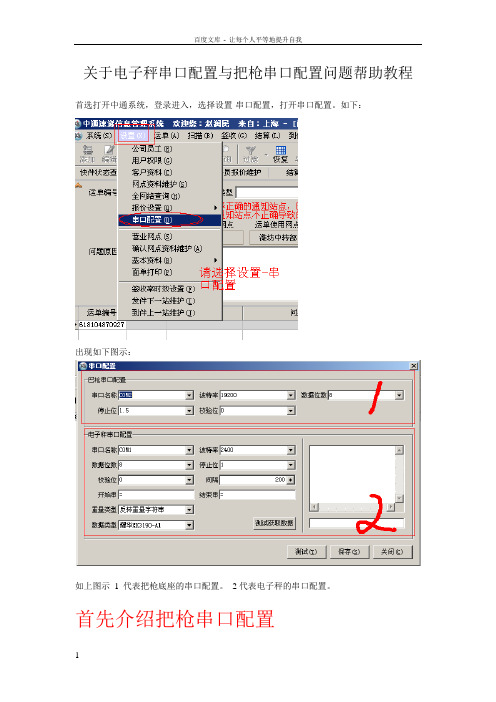
关于电子秤串口配置与把枪串口配置问题帮助教程首选打开中通系统,登录进入,选择设置-串口配置,打开串口配置。
如下:出现如下图示:如上图示1 代表把枪底座的串口配置。
2代表电子秤的串口配置。
首先介绍把枪串口配置把枪底座有两种连接方式,一为USB连接线,二为9针对9孔的串口线加单独电源线。
如何查看自己把枪底座的串口是多少呢?返回桌面,右键点击我的电脑图标,出现右键菜单,选择管理。
出现管理界面,选着设备管理器。
双击端口出现COM口配置找到CP2101 USB to UART Bridge Controler 选项查看后面端口号为COM2,及是中通管理系统里面的把枪串口的端口号更改为COM2 其他固定不动。
然后保存。
这样底座串口就算配置成功接下来是如何配置电子秤串口了一般电子秤串口默认是COM1的。
也就是端口不用调。
当然也不排除有其他端口号的存在。
这个只有慢慢测试了。
一般默认的是通信端口的第一个。
COM1 或者COM2.打开中通系统选择—设置—串口配置,出现如下图信息连接好电子秤后单击—测试获取数据,如出现下图所显示信息,说明电子秤连接完成:选择保存并关闭,即可使用扫描下面的扫描选项,正常使用电子秤。
如出现如下图所示:则更改波特率:一般电子秤在1200-2400之间,也不排除有其他波段的波特率出现,各网点可更改波特率后点击保存按钮,然后点击测试获取数据。
知道右边出现正常数字为准。
如出现下图所示:请查看串口名称是否正确,如确认正确可尝试一下操作方法:一、返回桌面,右键单击我的电脑小图标选择管理二、打开管理界面后,选择设备管理器,选择端口选项双击:三、出现如下图所示:四、选择电子秤正在使用的端口右键单击—选择卸载弹出提示窗口,选择确定。
卸载完成后。
请电脑重启。
重启之后。
即可正常使用电子秤扫描如出现如下图所示,请检查电子秤串口配置是否正确(如:串口名称是否符合、数据位数、停止位、校验为、开始串、结束串等是否符合上图所示),电子秤与电脑是否连接正确(如检查线路是否反接、线路是否存在损坏、串口连接是否正确),串口配置名称是否正确,连接线是否故障,电脑串口、电子秤串口是否损坏。
C#一台电脑对接多个串口通讯实例(电子天平称)

C#⼀台电脑对接多个串⼝通讯实例(电⼦天平称)⽐如:我们在对接串⼝硬件时。
实时获取硬件发送过来的数据、⽐如获取电⼦称发送过来的结果。
我们程序需要获取。
还有⼀种情况是⼀台电脑对接多个串⼝设备情况下如何开发。
1.封装⼀个OpenSerialPort实体类using System;using System.Collections.Generic;using System.IO.Ports;using System.Linq;using System.Text;using System.Threading.Tasks;namespace TestGetUnitek{public class OpenSerialPort{private SerialPort Sp = new SerialPort();public class SerialPortModel{///<summary>///端⼝号///</summary>public string PortName { get; set; }///<summary>///波特率///</summary>public int BaudRate { get; set; }///<summary>///奇偶验证///</summary>public Parity Parity { get; set; }///<summary>///停⽌位///</summary>public StopBits StopBits { get; set; }///<summary>///数据位///</summary>public int DataBits { get; set; }}public OpenSerialPort(SerialPortModel entity){//绑定对象信息Sp.PortName = entity.PortName;Sp.BaudRate = entity.BaudRate;Sp.Parity = entity.Parity;Sp.StopBits = entity.StopBits;Sp.DataReceived += new SerialDataReceivedEventHandler(Sp_DataReceived);Sp.ReceivedBytesThreshold = 1;Sp.DataBits = entity.DataBits;}///<summary>///打开端⼝///</summary>///<returns></returns>public string Open(){try{Sp.Open();return"1";}catch (Exception a){return a.Message;}}//实时获取数据public void Sp_DataReceived(object sender, System.IO.Ports.SerialDataReceivedEventArgs e){System.Threading.Thread.Sleep(100);//延迟100接收Byte[] reData = new byte[Sp.BytesToRead];//获取Sp.Read(reData, 0, reData.Length);string msg = Encoding.ASCII.GetString(reData);string ComName = Sp.PortName;//释放Sp.DiscardInBuffer();}//关闭端⼝public string Close(){try{Sp.Close();return"1";}catch (Exception a){return a.Message;}}}}2.然后在执⾏的地⽅。
SerialPort类的常用属性及方法.

SerialPort类的常用属性及方法.一、 SerialPort类的常用属性三、数据发送示例Private Sub fasong()serialPort1.PortName = "COM1"serialPort1.BaudRate = 9600serialPort1.Open()Dim data As Byte() = Encoding.Unicode.GetBytes(textBox1.Text)Dim str As String = Convert.ToBase64String(data)serialPort1.WriteLine(str)MessageBox.Show("数据发送成功!", "系统提示")End Sub四、接受示例Private Sub jieshou()Dim data As Byte() = Convert.FromBase64String(serialPort1.ReadLine())textBox2.Text = Encoding.Unicode.GetString(data)serialPort1.Close()MessageBox.Show("数据接收成功!", "系统提示")End Sub五、通过串口关闭对方计算机(1)新建一个项目,命名为Ex13_02,默认窗体为Form1。
(2)在Form1窗体中,主要添加两个Button控件,分别用于打开通信串口和关闭对方计算机。
(3)主要程序代码。
Private Sub button1_Click(sender As Object, e As EventArgs) '打开串口serialPort1.PortName = "COM1"serialPort1.Open()button1.Enabled = Falsebutton2.Enabled = TrueEnd Sub'数据接收事件,等待接收关机命令Private Sub serialPort1_DataReceived(sender As Object, e As SerialDataReceivedEventArgs)Dim data As Byte() = Convert.FromBase64String(serialPort1.ReadLine())Dim str As String = Encoding.Unicode.GetString(data)serialPort1.Close()If str = "关机" ThenDim p As New Process()p.StartInfo.FileName = "cmd.exe"eShellExecute = Falsep.StartInfo.RedirectStandardInput = Truep.StartInfo.RedirectStandardOutput = Truep.StartInfo.RedirectStandardError = Truep.StartInfo.CreateNoWindow = Truep.Start()p.StandardInput.WriteLine("shutdown /s")p.StandardInput.WriteLine("exit")End IfEnd Sub'发送关机命令Private Sub button2_Click(sender As Object, e As EventArgs) If button2.Text = "关闭计算机" Then'发送关机命令数据Dim data As Byte() = Encoding.Unicode.GetBytes("关机") Dim str As String = Convert.ToBase64String(data) serialPort1.WriteLine(str)button2.Text = "取消关机"Elsebutton2.Text = "关闭计算机"button1.Enabled = Truebutton2.Enabled = False'取消关机Dim p As New Process()p.StartInfo.FileName = "cmd.exe"eShellExecute = Falsep.StartInfo.RedirectStandardInput = Truep.StartInfo.RedirectStandardOutput = Truep.StartInfo.RedirectStandardError = Truep.StartInfo.CreateNoWindow = Truep.Start()p.StandardInput.WriteLine("shutdown /a")p.StandardInput.WriteLine("exit")End If End Sub。
浅析使用COM口读取梅特勒托利多天平称重结果的方法

图4 常用9针串口外观 1—载波检测(CD) 2—接受数据(RXD) 3—发出数据(TXD)
4—数据终端准备好(DTR) 5—信号地线(SG) 6—数据准备好(DSR) 7—请求发送(RTS) 8—清除发送(CTS) 9—振铃指示(RI)
对于称重设备来说,以梅特勒托利多ICS429系 列产品为例,其背面接口如图5所示[1]。
Abstract:In order to meet the requirements of high display accuracy for human-computer interface(HMI)
. AlsloftwRaireg,hatnsewRmeestheord vtheadt c.an get the weighing results accurately by communicate with the METTLER TOLEDO
事件驱动型的,当计算机相应串行通讯端口接收到
数据时会触发其“OnComm”事件。 我们需要在其
“OnComm” 事 件 中 编 写 相 关 脚 本 才 能 将 称 重 设 备
发送过来的重量数据提取出来。
Private Sub MS1_OnComm()
Dim sTemp As String
变量定义
Select Case mEvent
“打印”键来读取数据。 如何能让称重设备周期性 地将其称重结果发送给计算机呢? 接下来将要使 用的是通讯指令。 梅特勒托利多针对其产品有着一 套 专 用 的 通 讯 指 令 MT-SICS(Standard Interface
Command Set),以便实现各种各样的功能。 这套指 令被分为4个级别level 0~level 3,所包含的命令由 简单到复杂,像传送称重结果这样的简单指令被包 含在了level 0中。 通过相关手册,查找到指令“SI”可 以命令称重设备将当前重量立即发送给计算机[2]。 2.5 编程实现 2.5.1 数据读取
通过计算机串口读取电子天平信息

通过计算机串口读取电子天平信息
刘安杰
【期刊名称】《《可编程控制器与工厂自动化(PLC FA)》》
【年(卷),期】2005(000)009
【摘要】本文接合一个具体的实例,分析了串口通信的特点和注意事项,以及如何利用VB中的MSComm控件,通过计算机的串口来读取一些智能设备中的信息。
【总页数】3页(P122-124)
【作者】刘安杰
【作者单位】滨州盟威BBS轮毂有限公司
【正文语种】中文
【中图分类】TP273
【相关文献】
1.PC机基于串口与89C52单片机通讯读取DS18B20的数据 [J], 文骁阳
2.存储器24C08的串口读取与写入程序 [J], 邹士洪
3.基于LabVIEW读取串口数据技术 [J], 夏锴;杨增宝
4.基于电子天平串口通讯探究 Leidenf rost现象 [J], 何勒铭;原媛
5.基于U盘读取文件机制打开超级终端串口的研究 [J], 陈文敏
因版权原因,仅展示原文概要,查看原文内容请购买。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
using System;using System.IO.Ports;using System.Text;using System.Threading;/// <summary>/// 封装COM口数据/// </summary>public class ComInformation{string _wdata;string _wunit;string _qdata;string _qunit;string _percentage;/// <summary>/// 获取或设置重量/// </summary>public string WData { get { return this._wdata; } set { this._wdata = value; } }/// <summary>/// 获取或设置重量单位/// </summary>public string WUnit { get { return this._wunit; } set { this._wunit = value; } }/// <summary>/// 获取或设置数量/// </summary>public string QData { get { return this._qdata; } set { this._qdata = value; } }/// <summary>/// 获取或设置数量单位/// </summary>public string QUnit { get { return this._qunit; } set { this._qunit = value; } }/// <summary>/// 获取或设置百分数/// </summary>public string Percentage { get { return this._percentage; } set { this._percentage =value; }}}/// <summary>/// 电子称数据读取类/// </summary>public class WeightReader : IDisposable#region 字段、属性与构造函数SerialPort sp;int _speed = 300;/// <summary>/// 获取或设置电脑取COM数据缓冲时间,单位毫秒/// </summary>public int Speed{get{return this._speed;}set{if (value < 300)throw new Exception("串口读取缓冲时间不能小于300毫秒!");this._speed = value;}}public bool InitCom(string PortName){return this.InitCom(PortName, 4800, 300);}/// <summary>/// 初始化串口/// </summary>/// <param name="PortName">数据传输端口</param>/// <param name="BaudRate">波特率</param>/// <param name="Speed">串口读数缓冲时间</param>/// <returns></returns>public bool InitCom(string PortName, int BaudRate,int Speed){try{sp = new SerialPort(PortName, BaudRate, Parity.None, 8);sp.ReceivedBytesThreshold = 10;sp.Handshake = Handshake.RequestToSend;sp.Parity = Parity.None;sp.ReadTimeout = 600;sp.WriteTimeout = 600;this.Speed = Speed;if (!sp.IsOpen){sp.Open();}return true;}catch{throw new Exception(string.Format("无法初始化串口{0}!",PortName));}}#endregion#region 串口数据读取方法public WeightInformation ReadInfo(){string src = this.ReadCom();WeightInformation info = new WeightInformation();info.WData = this.DecodeWeightData(src);info.WUnit = this.DecodeWeightUnit(src);info.Percentage = this.DecodePercentage(src);info.QData = this.DecodeQualityData(src);info.QUnit = this.DecodeQualityUnit(src);return info;}/// <summary>/// 将COM口缓存数据全部读取/// </summary>/// <returns></returns>private string ReadCom()//返回信息{if (this.sp.IsOpen){Thread.Sleep(this._speed);string res = "";//for (int i = 0; i < 5; i++)//{byte[] buffer = new byte[sp.BytesToRead];sp.Read(buffer, 0, buffer.Length);res = System.Text.Encoding.ASCII.GetString(buffer);//if (res != "")// break;//}if (res == ""){throw new Exception("串口读取数据为空,参数设置是否正确!");}return res;}return "";}#endregion#region 基本取数方法/// <summary>/// 从字符串中取值/// </summary>/// <param name="head">起始字符串</param>/// <param name="intervalLen">间隔位长度</param>/// <param name="valueLen">值长度</param>/// <param name="src">源字符串</param>/// <returns></returns>private string DecodeValue(string head, int intervalLen, int valueLen,string src) {int index = src.IndexOf(head);return src.Substring(index + intervalLen, valueLen);}/// <summary>/// 取重量/// </summary>/// <param name="srcString">源字符串</param>/// <returns></returns>private string DecodeWeightData(string srcString){return this.DecodeValue("GS,", 3, 8,srcString);}/// <summary>/// 取重量单位/// </summary>/// <param name="srcString">源字符串</param>/// <returns></returns>private string DecodeWeightUnit(string srcString){return this.DecodeValue("GS,", 12, 2, srcString);}/// <summary>/// 取百分数/// </summary>/// <param name="srcString">源字符串</param>/// <returns></returns>private string DecodePercentage(string srcString){return this.DecodeValue("U.W.", 4, 14, srcString);}/// <summary>/// 取数量/// </summary>/// <param name="srcString">源字符串</param>/// <returns></returns>private string DecodeQualityData(string srcString){return this.DecodeValue("PCS", 3, 9, srcString);}/// <summary>/// 取数量单位/// </summary>/// <param name="srcString">源字符串</param>/// <returns></returns>private string DecodeQualityUnit(string srcString){return this.DecodeValue("PCS", 12, 3, srcString);}#endregion#region 释放所有资源public void Dispose(){if (sp != null && sp.IsOpen){sp.Close();}}#endregion}。