数据结构课程设计_商品销售管理源代码
数据结构课程设计校园超市商品销售统计系统方案
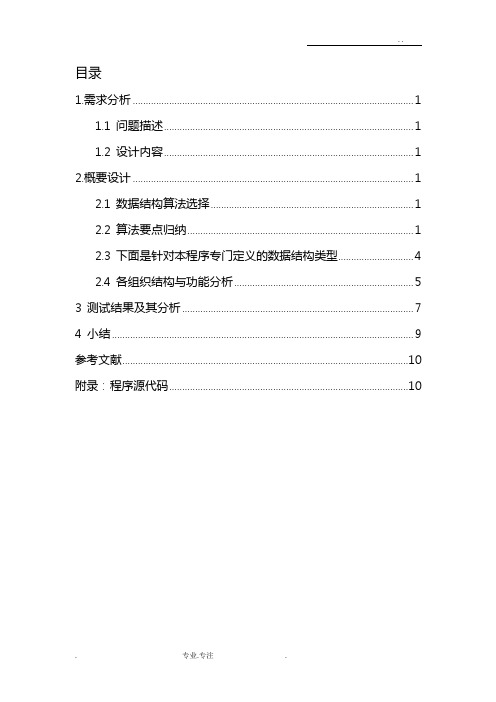
目录1.需求分析 (1)1.1 问题描述 (1)1.2 设计内容 (1)2.概要设计 (1)2.1 数据结构算法选择 (1)2.2 算法要点归纳 (1)2.3 下面是针对本程序专门定义的数据结构类型 (4)2.4 各组织结构与功能分析 (5)3 测试结果及其分析 (7)4 小结 (9)参考文献 (10)附录:程序源代码 (10)1.需求分析1.1 问题描述设计一系统,实现超市定期对销售各商品的记录进行统计,可按商品的编号、单价、销售量或销售额做出排名。
1.2 设计内容在本设计中,首先从数据文件中读出各商品的信息记录,存储在顺序表中。
各商品的信息包括:商品编号、商品名、单价、销出数量、销售额。
商品编号共4位,采用字母和数字混合编号,如:A125,前一位为大写字母,后三位为数字,按商品编号进行排序时,可采用基数排序法。
对各商品的单价、销售量或销售额进行排序时,可采用多种排序方法,如直接插入排序、冒泡排序、快速排序,直接选择排序等方法。
在本设计中,对单价的排序采用冒泡排序法,对销售量的排序采用快速排序法,对销售额的排序采用堆排序法。
2.概要设计2.1 数据结构算法选择本设计主要采用了顺序表。
共用四种排序方法:冒泡排序法,快速排序法,堆排序法,基数排序法。
2.2 算法要点归纳1.冒泡排序:冒泡排序的算法思想是:通过无序区中相邻元素关键字间的比较和位置的交换,使关键字最小的元素如气泡一般逐渐往上“漂浮”直至“水面”。
整个算法是从最下面的元素开始,对每两个相邻元素的关键字进行比较,且使关键字较小的元素换至关键字较大的元素之上,使得经过一趟冒泡排序后,关键字最小的元素到达最上端。
接着,再在剩下的元素中找关键字次小的元素,并把它换在第二个位置上。
依次类推,一直到所有元素都有序为止。
图1:冒泡排序算法思想2.快速排序:快速排序是对冒泡排序的一种改进。
它的基本思想是:通过一趟排序将待排记录分割成独立的两部分,其中一部分记录的关键字均比另一部分记录的关键字,则可分别对这两部分记录继续进行排序,以达到整个序列有序。
C语言销售管理系统设计(内含完整代码及附件)
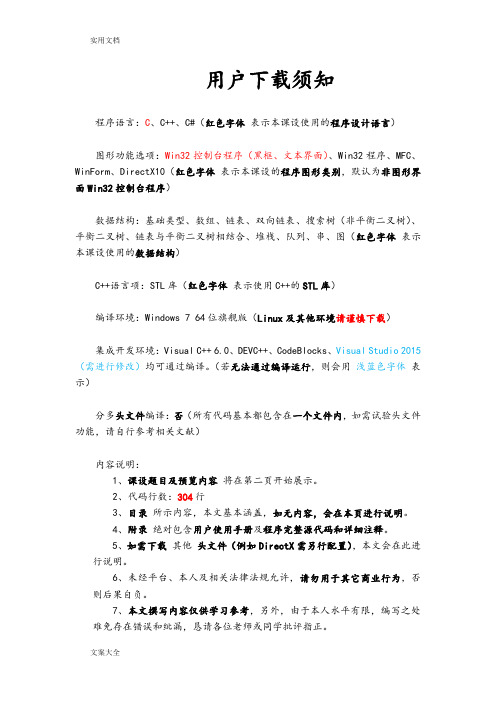
用户下载须知程序语言:C、C++、C#(红色字体表示本课设使用的程序设计语言)图形功能选项:Win32控制台程序(黑框、文本界面)、Win32程序、MFC、WinForm、DirectX10(红色字体表示本课设的程序图形类别,默认为非图形界面Win32控制台程序)数据结构:基础类型、数组、链表、双向链表、搜索树(非平衡二叉树)、平衡二叉树、链表与平衡二叉树相结合、堆栈、队列、串、图(红色字体表示本课设使用的数据结构)C++语言项:STL库(红色字体表示使用C++的STL库)编译环境:Windows 7 64位旗舰版(Linux及其他环境请谨慎下载)集成开发环境:Visual C++ 6.0、DEVC++、CodeBlocks、Visual Studio 2015(需进行修改)均可通过编译。
(若无法通过编译运行,则会用浅蓝色字体表示)分多头文件编译:否(所有代码基本都包含在一个文件内,如需试验头文件功能,请自行参考相关文献)内容说明:1、课设题目及预览内容将在第二页开始展示。
2、代码行数:304行3、目录所示内容,本文基本涵盖,如无内容,会在本页进行说明。
4、附录绝对包含用户使用手册及程序完整源代码和详细注释。
5、如需下载其他头文件(例如DirectX需另行配置),本文会在此进行说明。
6、未经平台、本人及相关法律法规允许,请勿用于其它商业行为,否则后果自负。
7、本文撰写内容仅供学习参考,另外,由于本人水平有限,编写之处难免存在错误和纰漏,恳请各位老师或同学批评指正。
销售管理系统设计某公司有四个销售员(编号:1-4),负责销售五种产品(编号:A-E)。
每个销售员都将当天出售的每种产品各写一张便条交上来。
每张便条包含内容:1)销售员的代号,2)产品的代号,3)这种产品当天的销售额。
每位销售员每天上缴1张便条。
系统基本功能:1、系统以菜单方式工作2、便条信息录入功能(便条信息用文件保存)3、收集到了上个月的所有便条后,读取销售情况1)计算每个人每种产品的销售额。
C语言商店销售管理系统课程设计
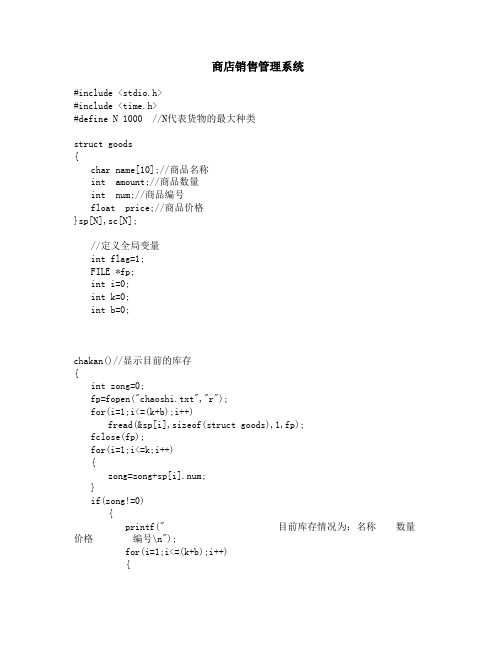
商店销售管理系统#include <stdio.h>#include <time.h>#define N 1000 //N代表货物的最大种类struct goods{char name[10];//商品名称int amount;//商品数量int num;//商品编号float price;//商品价格}sp[N],sc[N];//定义全局变量int flag=1;FILE *fp;int i=0;int k=0;int b=0;chakan()//显示目前的库存{int zong=0;fp=fopen("chaoshi.txt","r");for(i=1;i<=(k+b);i++)fread(&sp[i],sizeof(struct goods),1,fp);fclose(fp);for(i=1;i<=k;i++){zong=zong+sp[i].num;}if(zong!=0){printf(" 目前库存情况为:名称数量价格编号\n");for(i=1;i<=(k+b);i++){printf(" %s %d %f %d\n",sp[i].name,sp[i].amount,sp[i].price,sp[i].num);}}else printf("\n #####目前库存为#####\n");}jinhuo()//进货模块{do{k++;i++;printf(" 请输入商品的名称.数量.价格.标号(>0)例如:a 1 2.5 111\n\t\t\t");scanf("%s %d %f %d%c",sp[i].name,&sp[i].amount,&sp[i].price,&sp[i]. num);printf(" 是否继续,是(1),否(0)\n\t\t\t");scanf("%d",&flag);}while(flag!=0);fp=fopen("chaoshi.txt","w");for(i=1;i<=k;i++)fwrite(&sp[i],sizeof(struct goods),1,fp);fclose(fp);chakan();}xiaoshou()//销售模块{float m[100];int z=1;int j[100];int a[100];int g=0;int y=0;int h=0;float zong=0;fp=fopen("chaoshi.txt","r");for(i=1;i<=k;i++)fread(&sp[i],sizeof(struct goods),1,fp);fclose(fp);do{g++;y++;loop: printf(" 请输入商品编号和数量,例如:(100 20)\n\t\t\t");scanf("%d %d",&j[y],&a[y]);for(z=1;z<=k;z++){if(j[y]==sp[z].num){h++;sp[z].amount=sp[z].amount-a[y];m[y]=(float)a[y]*sp[z].price;sc[y]=sp[z];}}if(h==0){printf(" 输入有误,请重新输入\n\t\t\t");goto loop;}fp=fopen("chaoshi.txt","wt");for(i=1;i<=k;i++)fwrite(&sp[i],sizeof(struct goods),1,fp);fclose(fp);printf(" 是否继续,是(1),否(0)\n\t\t\t");scanf("%d",&flag);}while(flag!=0);printf("\n############################################\n");printf(" 编号名称单价数量总计\n");for(y=1;y<=g;y++){printf(" %4d%10s%8.2f%6d%13.2f\n",sc[y].num,sc[ y].name,sc[y].price,a[y],m[y]);zong=zong+m[y];}printf(" 您此次一共消费%f 元,欢迎您下次光临\n",zong);shijian();}zengjia()//增加商品数量模块{float m;int z=1;int j[100],a[100];int g=0;int y=0;int zong=0;int h=0;fp=fopen("chaoshi.txt","r");for(i=1;i<=k;i++)fread(&sp[i],sizeof(struct goods),1,fp);fclose(fp);do{g++;y++;loop: printf(" 请输入商品编号和数量,例如:(100 20)\n\t\t\t");scanf("%d %d",&j[y],&a[y]);for(z=1;z<=k;z++){if(j[y]==sp[z].num){h++;sp[z].amount=sp[z].amount+a[y];m=a[y]*sp[z].price;sc[y]=sp[z];}}if(h==0){printf(" 输入有误,请重新输入\n\t\t\t");goto loop;}fp=fopen("chaoshi.txt","wt");for(i=1;i<=k;i++)fwrite(&sp[i],sizeof(struct goods),1,fp);fclose(fp);printf(" 是否继续,是(1),否(0)\n\t\t\t");scanf("%d",&flag);}while(flag!=0);chakan();}shanchu()//删除商品模块{int z;int j[100],a[100];int c=0;int y=0;int h=0;do{c++;loop: printf(" 请输入您想删除的商品编号\n\t\t\t");scanf("%d",&j[y]);for(z=1;z<=k;z++){h++;if(j[y]==sp[z].num)sp[z]=sp[z+1];}if(h==0){printf(" 输入有误,请重新输入\n\t\t\t");goto loop;}fp=fopen("chaoshi.txt","wt");for(i=1;i<=(k-1);i++)fwrite(&sp[i],sizeof(struct goods),1,fp);fclose(fp);printf(" 是否继续,是(1),否(0)\n\t\t\t");scanf("%d",&flag);}while(flag!=0);chakan();}shijian(){struct tm when;time_t now;time(&now);when=*localtime(&now);printf(" %s\n",asctime(&when));}main()//主函数{int choice;do{printf("\n\n\n ********超市管理系统*******\n\n");printf(" 1.进货\n");printf(" 2.销售\n");printf(" 3.增加商品数量\n");printf(" 4.删除商品\n");printf(" 5.查看目前库存\n");printf(" 0.返回主菜单\n");printf(" 请选择您要进行的操作\n\t\t\t");scanf("%d",&choice);switch(choice){case 1:jinhuo();break;case 2:xiaoshou();break;case 3:zengjia();break;case 4:shanchu();break;case 5:chakan();break;case 0:break;default :printf(" 输入有误,请重新输入\n\t\t\t");}}while(choice!=0);}。
销售管理系统课程设计c语言

销售管理系统课程设计 c语言一、教学目标本课程旨在通过C语言编程,使学生掌握销售管理系统的开发方法。
教学目标包括:1.知识目标:使学生掌握C语言的基本语法、数据结构、算法和编程思想,理解销售管理系统的业务流程和架构设计。
2.技能目标:培养学生具备使用C语言进行程序设计的能力,能够独立完成销售管理系统的模块开发和调试。
3.情感态度价值观目标:培养学生对计算机编程的兴趣,增强其团队合作意识和解决问题的能力,使其认识到计算机技术在现代商业管理中的重要作用。
二、教学内容本课程的教学内容主要包括C语言基础知识、数据结构、算法、编程规范、销售管理系统的设计与实现等。
具体安排如下:1.C语言基础知识:介绍C语言的基本语法、关键字、运算符、表达式等。
2.数据结构:讲解常用的数据结构,如数组、链表、栈、队列、树、图等,以及其在销售管理系统中的应用。
3.算法:学习常见的排序算法、查找算法、动态规划算法等,分析其时间复杂度和空间复杂度。
4.编程规范:介绍C语言编程的规范和技巧,如代码、注释、命名规则等。
5.销售管理系统的设计与实现:分析销售管理系统的业务需求,设计系统架构,实现各功能模块,并进行调试与优化。
三、教学方法本课程采用讲授法、案例分析法、实验法等多种教学方法,以激发学生的学习兴趣和主动性。
1.讲授法:通过讲解C语言的基本语法、数据结构、算法等内容,使学生掌握相关知识。
2.案例分析法:分析实际的销售管理场景,引导学生运用所学知识解决实际问题。
3.实验法:安排实验课程,让学生动手实践,培养其编程能力和解决问题的能力。
四、教学资源本课程所需教学资源包括:1.教材:《C语言程序设计》等相关教材。
2.参考书:提供相关的编程指南、算法手册等参考资料。
3.多媒体资料:制作课件、演示视频等,以辅助教学。
4.实验设备:计算机、网络设备等,用于实验课程的开展。
五、教学评估本课程的评估方式包括平时表现、作业、考试等。
评估方式应客观、公正,能够全面反映学生的学习成果。
C语言课程设计《商品销售管理系统》

C语言课程设计《商品销售管理系统》摘要本文介绍的是C语言课程设计《商品销售管理系统》,通过使用C语言编程制作的电脑程序,能实现商品销售入库、库存管理、采购管理、商品销售及库存查询功能,设计的目的是为了让电脑为商店的销售管理工作提供帮助。
关键词:C语言课程设计;商品销售管理系统;商品入库;库存管理;采购管理。
1 绪论商品销售管理系统是一种电脑信息系统,是通过计算机程序实现库存管理、采购管理、销售管理、库存查询等基本功能,以便更有效地管理货物出售,节省人力与财力的。
因此,本文将使用C语言开发一个基于计算机的商品销售管理系统,以实现商品销售入库、库存管理、采购管理、商品销售及库存查询等功能。
2 功能需求2.1主菜单程序的功能菜单由主菜单控制,主菜单主要有以下几项功能:(1)商品入库(2)库存管理(3)采购管理(4)库存查询(5)商品销售(6)退出系统2.2 商品入库此功能需要实现商品信息录入功能,比如商品名称、售价、单位、购买数量等。
2.3 库存管理此功能的功能是实现对商品库存的管理,可以实现对商品库存的查看和管理功能,方便商家明确库存量。
2.4 采购管理此功能是实现采购管理,功能包括供货商信息管理、商品采购管理、采购账务记账等。
2.6 商品销售此功能用于实现对商品的销售,可以实现商品信息的查看及购买,销售的同时可以实现商品库存的查看。
3 系统建模系统建模是用逻辑模型来描述系统信息流程,包括实体-实体关系模型、关系模型及功能模型,实体-实体关系模型可以描述系统中各实体之间关系的抽象结构;关系模型能将系统中的关键实体和关联分析结构表示出来;功能模型能实现各种功能以及功能之间的关系结构。
4 系统设计本系统的设计重点是编写C语言程序,以实现商品销售管理系统功能。
核心算法包括以下:(1)无源文件init_menu(),用于初始化主菜单,根据用户输入调用不同的程序完成功能;(2)商品入库文件proc_input(),主要实现商品信息录入功能,需要获取用户输入的商品信息并存储;(3)库存管理文件proc_manage(),实现对商品库存的查看和管理功能,若商品库存不足,则可以选择跳转到商品采购管理程序;(4)采购管理文件proc_buy(),主要实现采购管理功能,包括供货商信息管理、商品采购管理、采购账务记账等;(5)库存查询文件proc_search(),用于实现对商品库存数量查询;(6)商品销售文件proc_sale(),用于实现商品的销售,销售的同时可以实现商品库存的查看。
服装销售管理系统—C语言课程设计

(二)查询打开文件,从键盘输入要查询的信息,若在文件里找到要查询的信息,则在界面输入信息,并调用系统暂停函数,返回界面;若没有找到查询的信息,调用系统暂停函数,返回界面。
(三)删除打开文件,从键盘输入要删除的信息,若在文件里找到要删除的信息存在,则把文件里要删除的那条信息删除掉,并调用系统暂停函数,返回界面;若没有找到删除的信息,调用系统暂停函数,返回界面。
(四)修改打开文件,从键盘输入要修改的信息,若在文件里找到要修改的信息存在,则按照提示信息依次输入要修改的信息,写入文件,并调用系统暂停函数,返回界面;若没有找到修改的信息,调用系统暂停函数,返回界面。
4、主要代码段分析/** 对系统进行初始化,建立用户记录和商品记录**/printf(" 商品数量: %d\n",tmpProduct->productCount);printf("商品附加信息: %s\n",tmpProduct->memo);ﻩﻩﻩsystem("pause");return ; }tmpProduct = tmpProduct->nex t; }printf("对不起,不存在该商品编号的商品!\n");ﻩﻩﻩsystem("pause");default:break; }}四、调试过程 (1 .测试数据设计 2 .测试结果分析)初始化用户名与密码管理员:admin admin店长:boss boss销售员:sel l sell(一)主界面(二)以管理员方式登陆系统,输入正确的用户账号admin和密码admin若登陆名或密码错误,则提示用户不存在登陆成功,进入管理员界面选择“(1)自身密码修改”,修改管理员密码选择“(2)用户信息管理”,进行用户的增、删、改、查功能选择“用户信息查看”,查看当前用户信息选择“用户信息添加”,添加用户信息选择“用户信息删除”,删除用户返回管理员界面,选择“(3)商品信息管理”,进行商品的增、删、改、查功能。
C语言课程设计《商品销售管理系统》

商品销售管理系统目录一、需求分析 (2)二、概要设计 (2)三、详细设计 (4)四、调试分析 (14)五、用户手册 (14)六、测试数据 (15)七、附录 (18)—1—一、需求分析商品销售管理程序商品信息:商品编号、商品名称、商品类别(普通电视机、DVD、带DVD的电视机,带DVD的电视机的售价为普通电视机和DVD单价之和的80%)、商品进货价格、商品销售价格、商品数量、供应商名称等。
记录每一次销售商品的数量和价格,并提供对已售出商品的价格、数量进行统计、排序功能,但允许用户退商品。
(要求:1.源文件采用多文件的工程结构2.数据存储采用文件形式3.标准的C输入输出4.功能完善,适当的注释,5.关于文件的内容需要自学)二、概要设计(一)按系统分析的功能要求将系统划分为以下几个主要功能模块:1、文件管理文件打开、关闭:对于刚输入或进行操作后的商品信息,在建立新的商品库存量后,可以把其保存在一个文件中,并对该文件可进行打开和关闭操作。
2、进出货管理(1)商品进货: 输入数据后商品信息能自动更新进行添加(2)商品销售: 输入数据后商品信息能自动更新进行减少3、商品数据管理(1)增加/删除商品: 添加或删除某条商品的信息(2)修改商品信息: 对某条商品的信息进行修改4、浏览商品信息管理(1)查询商品信息:可分别按商品编号、名称、类别或需要进货商品查询(2)排序商品信息: 可按商品编号、名称、类别分别排排序(3)浏览商品信息:可浏览输入、查询或排序后的数据。
(二)本程序结构(1)结构体设计struct goods{ long int num;char name[20];char sort[20];long int count;long int price;}goods[200];(2)各功能函数设计:void input() /*功能1:输入商品信息*/—2—void sequence() /*功能2:排序商品信息*/void purchase() /*功能3:进货商品信息*/void sell() /*功能4:销售商品信息*/void edit() /*功能5:添加或删除商品信息*/void correct() /*功能6:修改商品信息*/void search() /*功能7:查询商品信息*/void scan() /*功能8:浏览商品信息*/void reserve() /*功能9:保存文件*/void quit() /*退出系统*/(3)主函数设计:void main (){void input();void sequence();void purchase();void sell();void edit();void correct();void search();void scan();void reserve();lp: for(i=0;i<1;i++)scanf("%d",&k);system("cls");switch(k) /*用switch语句实现功能选择*/system("cls"); /*清屏*/goto lp; /*返回主界面*/}三、详细设计(1)各功能函数:void input() /*功能1:输入商品信息*/{printf("\n 请输入需要建立新库存的商品种类数量:");scanf("%d",&n);system("cls");printf("\n …………………………………………………………………………………………………\n");for(i=0;i<n;i++) /*用循环实现输入n个商品*/{printf("\n\t*请输入商品的编号:");scanf("%ld",&goods[i].num);printf("\n\t 请输入商品的名称:");—3—scanf("%s",goods[i].name);printf("\n\t 请输入商品的种类:");scanf("%s",goods[i].sort);printf("\n\t 请输入商品的数量:");scanf("%d",&goods[i].count);printf("\n\t 请输入商品的单价:");scanf("%d",&goods[i].price);printf("\n …………………………………………………………………………………………………\n");}printf("\tPlease press any key to continue:");getch();return; /*返回主界面*/}void sequence() /*功能2:排序商品信息*/{long temp;char p1[30],p2[30];for(k=0;k<n-1;k++) /*(1): 按编号排序*/for(i=0;i<n-k-1;i++) /*用起泡法排序*/if(goods[i].num>goods[i+1].num) /*按编号由小到大排序*/{temp=goods[i].num;goods[i].num=goods[i+1].num;goods[i+1].num=temp;strcpy(p1,goods[i].name);strcpy(goods[i].name,goods[i+1].name);strcpy(goods[i+1].name,p1);strcpy(p2,goods[i].sort);strcpy(goods[i].sort,goods[i+1].sort);strcpy(goods[i+1].sort,p2);}printf("--------------------------------------------------------------------------------\n");printf("\n\t\t按商品编号排序后的商品信息\n\n");printf("\t商品编号商品名称商品类别商品数量商品单价\n"); /*输出排序后商品信息*/for(i=0;i<n;i++){printf("\t %5ld %5s %5s %5ld %5ld\n",go ods[i].num,goods[i].name,goods[i].sort,goods[i].count,goods[i].price);—4—}for(k=0;k<n-1;k++) /*(2): 按商品名称排序*/for(i=0;i<n-k-1;i++)if(strcmp(goods[i].name,goods[i+1].name)>0){strcpy(p1,goods[i].name);strcpy(goods[i].name,goods[i+1].name);strcpy(goods[i+1].name,p1);temp=goods[i].num;goods[i].num=goods[i+1].num;goods[i+1].num=temp;strcpy(p2,goods[i].sort);strcpy(goods[i].sort,goods[i+1].sort);strcpy(goods[i+1].sort,p2);}printf("--------------------------------------------------------------------------------\n");printf("\n\t\t按商品名称排序后的商品信息\n");printf("\n\t商品名称商品编号商品类别商品数量商品单价\n"); /*输出排序后商品信息*/for(i=0;i<n;i++){printf("\t %5s %5ld %5s %5ld %5ld\n",goods[ i].name,goods[i].num,goods[i].sort,goods[i].count,goods[i].price);}for(k=0;k<n-1;k++) /*(3): 按商品类别排序*/for(i=0;i<n-k-1;i++) /*用起泡法排序*/if(strcmp(goods[i].sort,goods[i+1].sort)>0){strcpy(p2,goods[i].sort);strcpy(goods[i].sort,goods[i+1].sort);strcpy(goods[i+1].sort,p2);temp=goods[i].num;goods[i].num=goods[i+1].num;goods[i+1].num=temp;strcpy(p1,goods[i].name);strcpy(goods[i].name,goods[i+1].name);strcpy(goods[i+1].name,p1);}printf("-------------------------------------------------------------—5—-------------------");printf("\n\t\t按商品类别排序后的商品信息\n\n");printf("\t商品类别商品编号商品名称商品数量商品单价\n"); /*输出排序后商品信息*/for(i=0;i<n;i++){printf("\t %5s %5ld %5s %5ld %5ld\n",goods[i].sort,goods[i].num,goods[i].name,goods[i].count,goods[i].price);}printf("--------------------------------------------------------------------------------");printf("please press any key to continue:");getch();return; /*返回主界面*/}void purchase() /*功能3:进货商品信息*/ {long temp; /*定义临时变量表示刚进货的商品编号*/int j;printf("\n\t\5请输入刚进货的商品编号:");scanf("%ld",&temp);printf("\n\t\5请输入刚进货的商品数量:");scanf("%d",&j);printf("\n\n\n\tpress any key to contiue:");getch();system("cls");printf("\n\t输出进货后的商品信息:");for(i=0;i<n;i++) /*循环,寻找与进货相同的商品信息*/if(temp==goods[i].num)goods[i].count=goods[i].count+j; /*自动更改商品数量*/printf("\n\t商品编号商品名商品类别商品数量商品价格");for(i=0;i<n;i++) /*循环,输出进货后的商品信息*/{printf("\n\t %4ld %4s %4s %ld %4ld",goods[i].num,goods[i].name,goods[i].sort,goods[i].count,goods[i].price);}printf("\n\n\t\tPress any key to enter menu:");getch(); /*返回功能选择界面*/return;}—6—void sell() /*功能4:销售商品信息*/{long temp; /*定义临时变量temp表示刚销售的商品编号*/int j;printf("\n\t\5请输入刚销售的商品编号:");scanf("%ld",&temp);printf("\n\t\5请输入刚销售的商品数量:");scanf("%d",&j);for(i=0;i<n;i++) /*循环,找到刚销售的商品信息*/{if(temp==goods[i].num){for(;j>goods[i].count;) /*如果销售额大于库存量,则出现错误提示*/{printf("\n\t售出数量大于商品原有数量,出现错误,请重新输入:");scanf("%d",&j);}goods[i].count=goods[i].count-j; /*完成自动更改商品数量的功能*/}}printf("\n\tpress any key to contiue:");getch();system("cls"); /*清屏*/printf("\n\n *销售后商品信息:");printf("\n\t商品类别商品编号商品名称商品数量商品单价\n");for(i=0;i<n;i++) /*循环,输出销售后商品信息*/{printf("\t %4s %4ld %4s %4ld %4ld\n",g oods[i].sort,goods[i].num,goods[i].name,goods[i].count,goods[i].price );}printf("\n\tPress any key to enter menu:");getch();return; /*返回功能选择界面*/}void edit() /*功能5:添加或删除商品信息*/ {void add(); /*声明子函数*/void dele();—7—printf("\n\t请选择:1.添加商品信息; 2.删除商品信息;");printf("\t*请输入 1或2 继续:");scanf("%d",&k);switch(k) /*用switch语句实现功能选择*/{case 1: add();break;case 2: dele();break;}getch();return; /*返回主菜单*/}void add() /*添加商品信息*/{ /*定义子函数*/printf("\t*请输入所添加商品的商品编号:");scanf("%ld",&goods[n].num);printf("\n\t请输入所添加商品的名称:");scanf("%s",goods[n].name);printf("\n\t请输入所添加商品的类别:");scanf("%s",goods[n].sort);printf("\n\t请输入所添加商品的数量:");scanf("%d",&goods[n].count);printf("\n\t请输入所添加商品的价格:");scanf("%d",&goods[n].price);n=n+1;printf("\n");}void dele() /*删除商品信息*/{ /*定义子函数*/int temp; /*定义局部变量*/int j;for(i=0;i<n;i++){printf("\n\t商品编号商品名商品类别商品数量商品价格");printf("\n\t %5ld %5s %5s %5ld %5ld",goods[i].num,goods[i].name,goods[i].sort,goods[i].count,goods[i].price);printf("\n");}printf("\n");printf("\n*请输入要删除的商品编号:");scanf("%ld",&temp);printf("\n\n");—8—for(i=0;i<n;i++){if(temp==goods[i].num){for(j=i;j<n;j++){goods[j].num=goods[j+1].num;strcpy(goods[j].name,goods[j+1].name);strcpy(goods[j].sort,goods[j+1].sort);goods[j].count=goods[j+1].count;goods[j].price=goods[j+1].price;}n=n-1; /*商品总库存量减少*/}}for(i=0;i<n;i++){printf("\n\t商品编号商品名商品类别商品数量商品价格"); /*输出删除后的商品信息*/printf("\n\t %5ld %5s %5s %5ld %5ld",goods[i].num,goods[i].name,goods[i].sort,goods[i].count,goods[i].price);printf("\n");}printf("Please press any key to continue:");getch();return; /*返回主界面*/}void correct() /*功能6:修改商品信息*/ {long temp; /*定义局部变量*/printf("\n\t请输入要修改的商品的编号:");scanf("%ld",&temp); /*输入新的商品信息*/printf("\n");for(i=0;i<n;i++) /*保存新的商品信息*/{if(temp==goods[i].num){printf("\t请输入一个新的的商品编号:");scanf("%ld",&goods[i].num);printf("\n\t请输入新的商品的名称:");scanf("%s",goods[i].name);printf("\n\t请输入新的商品的类别:");—9—scanf("%s",goods[i].sort);printf("\n\t请输入新的商品的数量:");scanf("%d",&goods[i].count);printf("\n\t请输入新的商品价格:");scanf("%d",&goods[i].price);printf("\n");}}for(i=0;i<n;i++){printf("\n\t新的商品名称商品编号商品类别商品数量商品单价\n");printf("\t %4s %4ld %4s %4ld %4ld",goods[i].name,goods[i].num,goods[i].sort,goods[i].count,goods[ i].price);printf("\n");}printf("Please press any key to continue:"); /*返回功能选择界面*/getch();return; /*返回主菜单*/}void search() /*功能7:查询商品信息*/{int k; /*定义局部变量*/long temp1;char temp2[30],temp3[20];printf("\n\t\5按商品编号查询,请按1\t\t\t\5按商品名查询,请按2\n\t\5按商品类别查询,请按3\t\t\t\5查需要进货的商品,请按4\n");printf("\n\n\n\t\t\t请输入数字确定查询方式:");scanf("%d",&k);printf("\n");printf("Press any key to continue:");getch();system("cls");switch(k) /*实现查询方式的选择*/{case 1:{ printf("\n\n\n\n\n\t\t请输入要查询的商品的编号:"); /*按商品编号查询*/scanf("%ld",&temp1);system("cls"); /*清屏*/for(i=0;i<n;i++){—10—if(temp1==goods[i].num){printf("\n");printf("\n\t商品编号商品名商品类别商品数量商品价格");printf("\n\t %5ld %5s %5s %5ld %5ld",goods[i] .num,goods[i].name,goods[i].sort,goods[i].count,goods[i].price);}}}break; /*跳出switch语句*/case 2:{ printf("\n\n\n\n\n\t\t请输入要查询的商品名:"); /*按商品名查询数据*/scanf("%s",temp2);system("cls");for(i=0;i<n;i++){if(strcmp(temp2,goods[i].name)==0){printf("\n");printf("\n\t商品编号商品名商品类别商品数量商品价格");printf("\n\t %5ld %5s %5s %5ld %5ld",goods[i] .num,goods[i].name,goods[i].sort,goods[i].count,goods[i].price);}}}break; /*跳出switch语句*/case 3:{ printf("\n\n\n\n\n\t\t请输入要查询的商品类别:"); /*按商品类别查询数据*/scanf("%s",temp3);system("cls");for(i=0;i<n;i++){if(strcmp(temp3,goods[i].sort)==0)printf("\n");printf("\n\t商品编号商品名商品类别商品数量商品价格");printf("\n\t %5ld %5s %5s %5ld %5ld",goods[i].num,goods[i].name,goods[i].sort,goods[i].count,goods[i] — 11 —.price);}}}break; /*跳出switch语句*/case 4:{ int a=50;for(i=0;i<n;i++) /*查询需要进货的商品名*/{if(goods[i].count<a)printf("\n\t\t\4需要进货的商品名:%s 现有库存量:%ld\n",goods[i].name,goods[i].count);}}break; /*跳出switch语句*/}printf("\n\n\t\tPress any key to enter menu:");getch();return; /*返回主界面*/}void scan() /*功能8:浏览商品信息*/ {printf("\n\t浏览输入的商品信息,请按1\n\n\t浏览查询的商品信息,请按2\n\n\t浏览排序后商品信息,请按3\n");printf("请选择:");scanf("%d",&k);switch(k){case 1:{ printf("\t\t\t浏览输入的商品信息\n");printf("\t商品编号商品名商品类别商品数量商品价格\n");/*输出浏览商品信息*/for(i=0;i<n;i++){printf("\t%5ld %5s %5s %5ld %ld",goods[i].num,goods[i].name,goods[i].sort,goods[i].count,goods[i].price);printf("\n");printf("Press any key to enter menu:");getch();return;} break; /*跳出switch语句*/case 2:{ printf("\t\t\t浏览查询的商品信息\n");—12—printf("\t商品编号商品名商品类别商品数量商品价格\n");/*输出浏览商品信息*/for(i=0;i<n;i++){printf("\t%5ld %5s %5s %5ld %ld",goods[i] .num,goods[i].name,goods[i].sort,goods[i].count,goods[i].price);printf("\n");}printf("\nPress any key to enter menu:");getch();return;} break; /*跳出switch语句*/case 3:{ printf("\t\t\t浏览按商品编号排序的商品信息\n");printf("\t商品编号商品名商品类别商品数量商品价格\n");/*输出浏览商品信息*/for(i=0;i<n;i++){printf("\t%5ld %5s %5s %5ld %ld",goods[i].num ,goods[i].name,goods[i].sort,goods[i].count,goods[i].price);printf("\n");}printf("\nPress any key to enter menu:");getch();return; /*返回主界面*/} break; /*跳出switch语句*/}}void reserve() /*功能9:保存文件*/{FILE *fp;char file[15]; /*用来存放文件保存路径以及文件名*/ system("cls"); /*清屏*/printf("\n\t*请输入文件路径及文件名:");scanf("%s",file);fp=fopen("file","w+"); /*创建并打开一个文件,并得到该文件的地址*/fprintf(fp,"商品编号\t商品名 \t商品类别\t商品数量\t商品价格\n");printf("商品编号\t商品名 \t 商品类别\t 商品数量\t 商品价格\n");for(i=0;i<n;i++)—13—{fprintf(fp,"%ld\t\t%s\t\t%s\t\t%d\t\t%d\n",goods[i].num,goods[i].name ,goods[i].sort,goods[i].count,goods[i].price);printf("%ld\t\t%s\t\t%s\t\t%d\t\t%d\n",goods[i].num,goods[i].name,goo ds[i].sort,goods[i].count,goods[i].price);}fclose(fp); /*关闭文件*/printf("文件已经保存!\n");getch();system("cls"); /*清屏*/}void quit() /*退出系统*/{exit(0);}四、调试手册(1)、sp.c(225) : warning C4013: 'add' undefined; assuming extern returning int add()函数声明错误。
商品销售c语言课程设计

商品销售 c语言课程设计一、课程目标知识目标:1. 理解C语言在商品销售管理系统中的应用,掌握相关数据结构(如结构体、数组、链表)的使用;2. 学会使用C语言实现商品信息的增删改查功能,了解文件操作在商品数据存储中的应用;3. 了解模块化编程思想,掌握函数的定义、调用及参数传递。
技能目标:1. 培养学生运用C语言编写商品销售管理系统程序的能力,具备解决实际问题的编程技能;2. 培养学生分析问题、设计算法、编写代码、调试程序的能力;3. 提高学生的团队协作能力,学会与他人共同完成一个项目。
情感态度价值观目标:1. 培养学生对计算机编程的兴趣,激发学习积极性,增强自信心;2. 培养学生认真负责的工作态度,养成良好的编程习惯;3. 通过项目实践,使学生认识到编程在解决实际问题中的价值,提高社会责任感。
分析课程性质、学生特点和教学要求,本课程目标旨在使学生掌握C语言在商品销售管理系统中的应用,培养其编程技能和实际解决问题的能力。
课程目标具体、可衡量,以便学生和教师在教学过程中能够明确课程预期成果,并为后续的教学设计和评估提供依据。
二、教学内容1. C语言基础回顾:数据类型、变量、运算符、控制结构(条件语句、循环语句);2. 函数:函数的定义、调用、参数传递、递归;3. 数据结构:结构体、数组、链表;4. 文件操作:文件的打开、关闭、读写、定位;5. 商品销售管理系统功能设计:- 商品信息的增删改查功能;- 销售记录的录入与查询;- 库存管理;- 用户登录与权限管理。
6. 系统实现:模块化编程、函数封装、代码优化;7. 项目实践:分组合作完成商品销售管理系统的设计与实现,并进行测试与调试。
教学内容按照教学大纲的安排,从C语言基础知识回顾开始,逐步深入到函数、数据结构、文件操作等高级内容。
在教学过程中,结合教材相关章节,让学生掌握商品销售管理系统的功能设计及实现方法。
教学内容科学、系统,符合学生的认知发展规律,确保学生能够学以致用,提高实际编程能力。
数据结构课程设计 商品货架管理系统
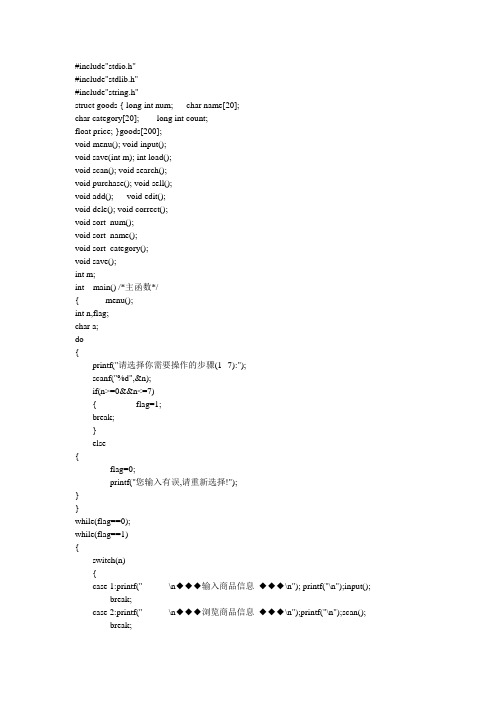
#include"stdio.h"#include"stdlib.h"#include"string.h"struct goods { long int num; char name[20];char category[20]; long int count;float price; }goods[200];void menu(); void input();void save(int m); int load();void scan(); void search();void purchase(); void sell();void add(); void edit();void dele(); void correct();void sort_num();void sort_name();void sort_category();void save();int m;int main() /*主函数*/{ menu();int n,flag;char a;do{printf("请选择你需要操作的步骤(1--7):");scanf("%d",&n);if(n>=0&&n<=7){ flag=1;break;}else{flag=0;printf("您输入有误,请重新选择!");}}while(flag==0);while(flag==1){switch(n){case 1:printf(" \n◆◆◆输入商品信息◆◆◆\n"); printf("\n");input();break;case 2:printf(" \n◆◆◆浏览商品信息◆◆◆\n");printf("\n");scan();break;case 3:printf(" \n◆◆◆查询商品信息◆◆◆\n");printf("\n");search();break;case 4:printf(" \n◆◆◆添加或删除商品◆◆◆\n");printf("\n");edit();break;case 5:printf(" \n◆◆◆修改商品信息◆◆◆\n");printf("\n");correct();break;case 6:printf(" \n◆◆◆进货管理◆◆◆\n");printf("\n");purchase();break;case 7:printf(" \n◆◆◆售货管理◆◆◆\n");printf("\n");sell();break;case 0:exit(0);break;default :break;}printf("\n");printf("是否继续进行(y or n):\n");scanf("%c",&a);if(a=='y'){flag=1;system("cls"); /*清屏*/menu(); /*调用菜单函数*/printf("请再次选择你需要操作的步骤(1--7):\n");scanf("%d",&n);printf("\n");}else exit(0);}}void menu() /*菜单函数*/{printf("\n\t************欢迎进入商品销售管理系统**********\n");printf("\n\t\t1.录入商品信息");printf("\t\t2.浏览商品信息\n");printf("\t\t3.查询商品信息");printf("\t\t4.添加或删除商品\n");printf("\t\t5.修改商品信息");printf("\t\t6.进货管理\n");printf("\t\t7.售货管理\n");printf("\t\t0.退出\n");printf("\n");printf("\n"); }void input() /*输入商品信息并保存*/{int i;printf ("\n 请输入需要建立新库存的商品种类数量:");scanf ("%d",&m);for (i=0; i<m; i++) /*用循环实现输入m 个商品*/{printf ("\n\t 请输入商品的编号:");scanf("%ld",&goods[i].num);printf ("\n\t 请输入商品的名称:");scanf("%s",goods[i].name);printf ("\n\t 请输入商品的种类:");scanf ("%s",goods[i].category);printf ("\n\t 请输入商品的数量:");scanf("%d",&goods[i].count);printf ("\n\t 请输入商品的单价:");scanf ("%f",&goods[i].price);printf("\n"); }save(m);return; /*返回主界面*/}void display(){int i;printf ("商品编号\t 商品名称\t 商品类别\t 商品数量\t 商品价格\n") ;/*输出商品信息*/ for(i=0;i<m;i++)printf("%ld\t\t%s\t\t%s\t\t%1d%\t\t%.2f\n",goods[i]. num,goods[i].name, goods[i].category, goods[i]. count, goods[i]. price);}void scan() /*浏览商品信息*/{int k;m=load();printf ("按商品编号浏览,请按l\t按商品名称浏览,请按2\t按商品类别浏览,请按3\n"); printf ("请选择浏览方式:");scanf ("%d",&k);switch (k) /*方式的选择*/{ case 1:sort_num();break;case 2:sort_name();break;case 3:sort_category();break;}return; /*返回主界面*/}void sort_num() /*⑴:按编号排序*/{long temp;char p1[30], p2[30];for (int k=0; k<m-1; k++)for (int i=0; i<m-k-1; i++) /* 用起泡法排序*/if (goods [i].num>goods[i+1]. num) /*按编号由小到大排序*/{temp=goods[i].num;goods[i].num=goods[i+1].num;goods[i+1].num=temp;strcpy (p1,goods[i].name);strcpy (goods[i].name, goods[i+1]. name);strcpy (goods[i+1].name, p1);strcpy (p2,goods[i].category);strcpy (goods[i].category, goods[i+1].category);strcpy (goods[i+1].category, p2);}display();return; /*返回主界面*/}void sort_name() /*(2):按商品名称排序*/{long temp;char p1[30], p2[30];for (int k=0;k<m-1;k++)for(int i=0;i<m-k-1;i++)if(strcmp(goods[i].name, goods[i+1].name)>0){strcpy(p1, goods[i].name);strcpy(goods[i].name, goods[i+1].name);strcpy(goods[i+1].name, p1);temp=goods[i].num;goods[i].num=goods[i+1].num;goods[i+1].num=temp;strcpy (p2,goods[i].category);strcpy (goods[i].category, goods[i+1].category);strcpy (goods[i+1].category, p2);}display();return; /*返回主界面*/}void sort_category() /*(3):按商品类别排序*/{long temp;char p1[30], p2[30];for (int k=0; k<m-1; k++)for (int i=0; i<m-k-1; i++) /* 用起泡法排序*/if(strcmp(goods[i].category, goods[i+1].category)>0){strcpy (p2, goods[i].category);strcpy(goods[i].category, goods[i+1].category);strcpy (goods[i+1].category, p2);temp=goods[i].num;goods[i].num=goods[i+1].num;goods[i+1].num=temp;strcpy (p1,goods[i].name);strcpy (goods[i].name, goods[i+1].name);strcpy (goods[i+1].name, p1);}display();return; /*返回主界面*/}void search_num ()/*(1):按商品编号查找*/{int long temp,i;printf ("\n 请输入要查询的商品编号:");scanf ("%ld",&temp);for(i=0;i<m;i++){if(temp==goods[i].num){printf ("\n 商品编号\t 商品名称\t 商品类别\t 商品数量\t 商品价格\n");printf("%1d\t\t%s\t\t%s\t\t%1d\t\t%.2f\n",goods[i].num, goods[i]. name, goods[i].category, goods[i]. count, goods[i]. price);}}}void search_name ()/*(2):按商品名称查找*/{int i;char temp[20];printf ("\n\n 请输入要查询的商品名称:");scanf("%s",temp);for (i=0;i<m;i++){if (strcmp (temp, goods[i].name)==0){printf ("商品编号\t 商品名称\t 商品类别\t 商品数量\t 商品价格\n");printf("\t\t%1d\t\t%s\t\t%s\t\t%1d\t\t%.2f\n",goods[i].num, goods[i]. name, goods[i].category, goods[i]. count, goods[i]. price);}}if(i>m)printf("无此商品");return ;}void search_category ()/*(3):按商品类别查找*/{int i;char temp[20];printf ("\n\n 请输入要查询的商品类别:");scanf("%s",temp);printf ("\t 商品编号\t 商品名称商品类别\t 商品数量\t 商品价格");for(i=0;i<m;i++){if(strcmp(temp, goods[i].category)==0)printf("\t\t%1d\t\t%s\t\t%s\t\t%1d\t\t%.2f",goods[i].num, goods[i]. name, goods[i].category, goods[i]. count, goods[i].price);}}void search () /*查询商品信息*/{int k; /*定义局部变量*/m=load();printf ("\n 按商品编号查询3 请按l,\t 按商品名称查询请按2,\t 按商品类别查询请按3\n");printf ("\n\请输入查询方式:");scanf ("%d",&k);switch (k) /*查询方式的选择*/{case 1:search_num();break;/*按商品编号查询*/case 2:search_name();break;/*按商品名称查询*/case 3:search_category();break;/*按商品类别查询*/ }printf("\nPress any key to enter menu.....");getchar ();return; /*返回主界面*/}void purchase() /*进货商品信息*/{long temp; /*定义临时变量表示刚进货的商品编号*/int j;m=load();printf ("\n\t 请输入刚进货的商品编号:");scanf("%ld",&temp);printf ("\n\t 请输入刚进货的商品数量:");scanf("%d",&j);printf ("\n\t 输出进货后的商品信息:\n");for (int i=0;i<m;i++) /*循环,寻找与进货相同的商品信息*/if(temp==goods[i]. num) goods[i].count=goods[i].count+j; /* 自动更改商品数量*/display();save(m);printf("\n\n\t\tPress any key to enter menu.....");getchar() ;/*返回功能选择界面*/return;}void sell() /*销售商品信息*/{long temp; /*定义临时变量temp 表示刚销售的商品编号*/int j;m=load();printf ("\n 请输入刚销售的商品编号:");scanf("%ld",&temp);printf ("\n 请输入刚销售的商品数量:");scanf("%d",&j);for(int i=0;i<m;i++) /*循环,找到刚销售的商品信息*/{if(temp==goods[i].num){for(;j>goods[i].count;) /*如果销售额大于库存量,则出现错误提示*/{printf ("\t 售出数量大于商品原有数量,出现错误,请重新输入:");scanf("%d",&j);}goods [i].count=goods[i].count-j; /*完成自动更改商品数量的功能*/ }}display();printf ("\n\tpress any key to contiue.....");getchar();return; /*返回功能选择界面*/}void edit() /*添加或删除商品信息*/{int k;void add (); /*声明子函数*/void dele();printf ("\n\t1.添加商品信息;2.删除商品信息;\n");printf ("\n 请输入1 或2 继续:");scanf ("%d",&k);switch (k) /*用switch 语句实现功能选择*/{case 1: add();break;case 2: dele();break;}printf ("\n\tpress any key to contiue......");getchar ();return; /*返回主菜单*/}void add () /*添加商品*/{FILE *fp;int count,i;if ((fp=fopen("goods_list","ab"))==NULL){printf ("cannot open file\n");exit(0);}printf("\n 请输入增加的商品数目:");scanf("%d",&count);for(i=0;i<count;i++){printf ("\n 请输入所添加商品的商品编号:");scanf("%ld",&goods[i]. num);printf ("\n 请输入所添加商品的名称:");scanf("%s",goods[i]. name);printf ("\n 请输入所添加商品的类别:");scanf("%s",goods[i].category);printf ("\n 请输入所添加商品的数量:");scanf("%d",&goods[i].count);printf ("\n 请输入所添加商品的价格:");scanf("%f",&goods[i].price);}for (i=0;i<count;i++) /*将新增的商品信息输出到磁盘文件中去*/ if (fwrite(&goods[i],sizeof(struct goods),1,fp)!=1)printf("file write error\n");printf("\n 添加完毕!\n");fclose(fp);printf("\n");}void dele() /*删除商品信息*/{int temp;m=load();display();printf ("\n 请输入要删除的商品编号:");scanf("%ld", &temp);printf ("\n");for(int i=0;i<m;i++){if(temp==goods[i].num){for(int j=i;j<m;j++){goods[j].num=goods[j+1].num;strcpy(goods[j].name, goods[j+1].name);strcpy (goods[j].category, goods[j+1].category);goods[j].count=goods[j+1].count;goods[j].price=goods[j+1].price;}}}m=m-1; /*商品总库存量减少*/save(m);display();printf ("Please press any key to continue.....");getchar();return; /*返回主界面*/}void correct() /*修改商品信息*/{long temp; /*定义局部变量*/m=load();display();printf ("\n\t 请输入要修改的商品的编号:");scanf ("%ld",&temp) ; /*输入新的商品信息*/for (int i=0; i<m; i++) /*保存新的商品信息*/{if(temp==goods[i].num){printf ("\n\t 请输入新的商品的名称:");scanf("%s",goods[i].name);printf("\n\t 请输入新的商品的类别:");scanf("%s",goods[i].category);printf("\n\t 请输入新的商品价格:");scanf("%f",&goods[i].price);printf("\n");}}save(m);display();printf ("Please press any key to continue......") ; /* 返回功能选择界面*/ getchar(); return; /*返回主菜单*/ }int load() /*导入函数*/{FILE*fp;int n=0;if((fp=fopen("goods_list","rb"))==NULL){printf ("cannot open file\n");exit(0);}else{do{fread(&goods[n],sizeof(struct goods),1,fp);n++;}while(feof(fp)==0);}fclose(fp);return(n-1);}void save(int m) /*保存文件函数*/{int i;FILE*fp;if ((fp=fopen("goods_list","wb"))==NULL){printf ("cannot open file\n");exit(0);}for (i=0;i<m;i++) /*将内存中的商品信息输出到磁盘文件中去*/ if (fwrite(&goods[i],sizeof(struct goods),1,fp)!=1)printf("file write error\n");fclose(fp);}。
电脑销售管理系统的设计与实现,单链表代码
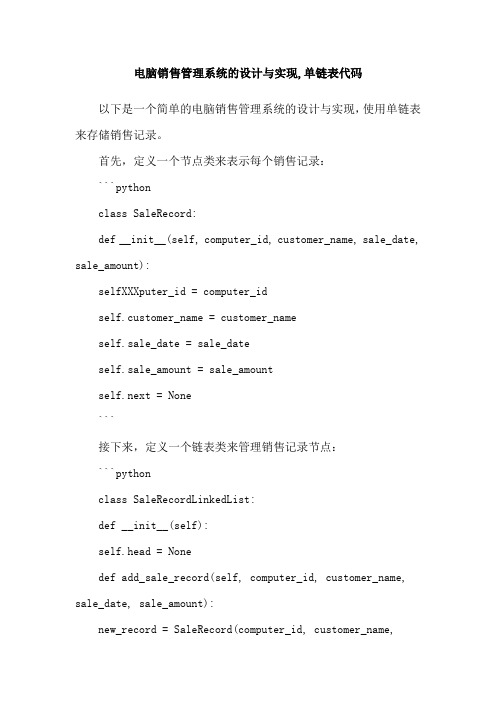
电脑销售管理系统的设计与实现,单链表代码以下是一个简单的电脑销售管理系统的设计与实现,使用单链表来存储销售记录。
首先,定义一个节点类来表示每个销售记录:```pythonclass SaleRecord:def __init__(self, computer_id, customer_name, sale_date, sale_amount):selfXXXputer_id = computer_idself.customer_name = customer_nameself.sale_date = sale_dateself.sale_amount = sale_amountself.next = None```接下来,定义一个链表类来管理销售记录节点:```pythonclass SaleRecordLinkedList:def __init__(self):self.head = Nonedef add_sale_record(self, computer_id, customer_name, sale_date, sale_amount):new_record = SaleRecord(computer_id, customer_name,sale_date, sale_amount)if self.head is None:self.head = new_recordelse:current = self.headwhile current.next is not None:current = current.nextcurrent.next = new_recorddef display_sale_records(self):current = self.headwhile current is not None:print("Computer ID:" currentXXXputer_id)print("Customer Na" current.customer_name)print("Sale Date:" current.sale_date)print("Sale Amount:" current.sale_amount)print("------" current = current.next```最后,我们可以使用链表类来创建销售记录,并显示所有的销售记录:```pythonsale_records = SaleRecordLinkedList()sale_records.add_sale_record(1, "John Doe" "2021-01-01"1000)sale_records.add_sale_record(2, "Jane Smith""2021-01-02" 1500)sale_records.add_sale_record(3, "Bob Johnson""2021-01-03" 2000)sale_records.display_sale_records()```这样,就可以通过调用 `add_sale_record` 方法来添加销售记录,并通过调用 `display_sale_records` 方法来显示所有的销售记录。
数据结构课程设计报告-超市商品管理
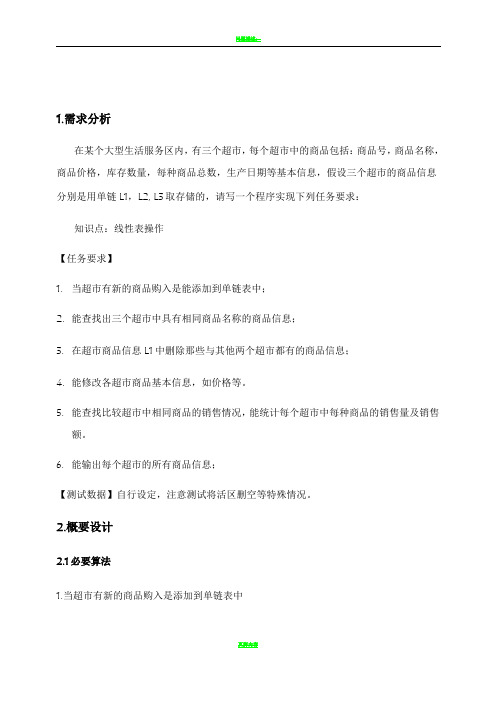
1.需求分析在某个大型生活服务区内,有三个超市,每个超市中的商品包括:商品号,商品名称,商品价格,库存数量,每种商品总数,生产日期等基本信息,假设三个超市的商品信息分别是用单链L1,L2, L3取存储的,请写一个程序实现下列任务要求:知识点:线性表操作【任务要求】1.当超市有新的商品购入是能添加到单链表中;2.能查找出三个超市中具有相同商品名称的商品信息;3.在超市商品信息L1中删除那些与其他两个超市都有的商品信息;4.能修改各超市商品基本信息,如价格等。
5.能查找比较超市中相同商品的销售情况,能统计每个超市中每种商品的销售量及销售额。
6.能输出每个超市的所有商品信息;【测试数据】自行设定,注意测试将活区删空等特殊情况。
2.概要设计2.1必要算法1.当超市有新的商品购入是添加到单链表中算法概要:运用单链表的基本操作——插入一个新结点,即分配一个新的储存空间,然后依次输入结点所含的数据,再插入到原结点的尾部即可。
此算法时间复杂度为O(1)。
2.查找出三个超市中具有相同商品名称的商品信息算法概要:1.寻找相同商品名称算法:先比较前两个链表(超市)l1,l2,运用比较字符串的strcmp()方法找出两个链表中含有相同的商品名称,然后将两个链表中具有相同商品名称的商品信息存储到新建链表fs中,再比较链表fs和l3,重复上述步骤,找出链表l1,l2,l3中都具有相同商品名称的商品,将其商品信息存储在链表fs中。
此算法时间复杂度为O(n)。
2.输出相同商品的商品信息:访问fs中每个结点中的商品名称,根据商品名称分别遍历原链表l1,l2,l3的商品信息找出原结点,然后将其信息输出。
此算法时间复杂度为O(n)。
3.在超市商品信息L1中删除那些与其他两个超市都有的商品信息算法概要:首先运用上述的寻找相同商品名称算法,找出l1中那些与其他两个超市都有的商品名称,再根据其名称遍历链表l1,找出其结点删除并释放——单链表的基本操作。
C语言课程设计报告——《商品销售管理》

湖北汽车工业学院电气与信息工程学院《C语言程序设计》课程设计课程设计题目:商品销售管理专业:电子信息科学与技术班级: T1023-11姓名:余勇成绩:目录一、需求分析 (3)1.2问题 (3)1.2系统 (3)1.3运行要求 (3)二、总体设计 (3)2.1系统流程设计 (3)2.2系统模块设计 (4)三、详细设计 (5)3.1数据结构设计 (5)3.2界面设计 (6)3.3模块实现 (13)四、功能模块的调试和测试 (21)五、课程设计总结 (24)六、致谢 (27)参考文献 (28)附录一、需求分析1、问题问题描述:已知一公司有10种产品(产品编号,产品名称,产品价格,产品产地,库存数量(最开始为1000个)),设计一程序,完成以下功能:①销售:从键盘输入顾客姓名,销售数量、销售日期,实现销售功能。
需要判断产品是否存在,销售数量是否小于库存数量,销售日期格式是否合法(格式为:YYYY-MM-DD,如2009-01-02))②计算时间段内各个产品的销售总额③能根据顾客姓名,查询购买历史④能显示所有顾客的姓名根据需求,该系统所应包含的信息有以下一些:商品销售的基本信息:顾客姓名、产品类型、销售数量、销售日期、库存数量、销售总额;根据需求,该系统所应实现的功能有以下一些:1. 各种基本数据的录入。
如:产品销售的基本信息的录入。
2. 基于各种基本数据的查询。
即允许对已经录入的数据进行查询。
例如根据产品编号查询产品的销售历史;根据顾客姓名,查询购买历史。
3. 各种基本数据的计算。
计算时间段内各个产品的销售总额。
4. 数据的显示:显示所有顾客的姓名。
5. 数据导出:将销售信息导出到指定文件。
6. 数据导入:从指定文件导入销售信息。
2、系统采用C语言实现,开发环境为VC6.0,可以运行在操作系统windows98及以上皆可。
3、运行要求1)能不受限制的录入销售信息;2)界面友好,操作方便,例如保存前需要提示用户是否保存;3)容错性强,例如数据导出时,如果文件不存在需要提示用户。
c语言课程设计(销售管理系统)【完整版】

c语言课程设计(销售管理系统)【完整版】
本管理系统实现了销售管理,可以完成客户管理、商品管理以及订单管理等功能,为管理者提供更多便利。
管理系统由主菜单、客户管理、商品管理、订单管理等模块组成,主菜单模块显示并实现了上述以及其他所有模块,可以方便快捷地进行相关操作。
客户管理模块包括新建客户信息、删除客户信息、更新客户信息、查询客户信息等功能,可以快速有效地管理客户信息;
订单管理模块包括新建订单、删除订单、更新订单、查询订单等功能,能够轻松地完成销售订单的管理。
另外,系统还采用了文件存储,只要上次保存数据正确,就可以实现每次登录之后的自动加载,让操作更加简单快捷。
系统采用C语言开发,使用字符操作实现图形界面;结构体链表数据结构实现数据存储与处理;文件存储实现数据加载与保存;采用函数式编程实现功能模块;不断尝试性地增加改进系统,以确保系统运行更加稳定可靠。
本项目以C语言编程实现对销售管理系统的全面而完整的管理,能满足日常销售管理的需求;同时,丰富的图形界面,实用的功能模块和可靠的文件存储,使系统操作变得更为简单快捷。
数据结构课程设计校园超市商品销售统计系统

目录1.需求分析 (1)1.1 问题描述 (1)1.2 设计内容 (1)2.概要设计 (1)2.1 数据结构算法选择 (1)2.2 算法要点归纳 (1)2.3 下面是针对本程序专门定义的数据结构类型 (4)2.4 各组织结构与功能分析 (5)3 测试结果及其分析 (6)4 小结 (8)参考文献 (9)附录:程序源代码 (9)1.需求分析1.1 问题描述设计一系统,实现超市定期对销售各商品的记录进行统计,可按商品的编号、单价、销售量或销售额做出排名。
1.2 设计内容在本设计中,首先从数据文件中读出各商品的信息记录,存储在顺序表中。
各商品的信息包括:商品编号、商品名、单价、销出数量、销售额。
商品编号共4位,采用字母和数字混合编号,如:A125,前一位为大写字母,后三位为数字,按商品编号进行排序时,可采用基数排序法。
对各商品的单价、销售量或销售额进行排序时,可采用多种排序方法,如直接插入排序、冒泡排序、快速排序,直接选择排序等方法。
在本设计中,对单价的排序采用冒泡排序法,对销售量的排序采用快速排序法,对销售额的排序采用堆排序法。
2.概要设计2.1 数据结构算法选择本设计主要采用了顺序表。
共用四种排序方法:冒泡排序法,快速排序法,堆排序法,基数排序法。
2.2 算法要点归纳1.冒泡排序:冒泡排序的算法思想是:通过无序区中相邻元素关键字间的比较和位置的交换,使关键字最小的元素如气泡一般逐渐往上“漂浮”直至“水面”。
整个算法是从最下面的元素开始,对每两个相邻元素的关键字进行比较,且使关键字较小的元素换至关键字较大的元素之上,使得经过一趟冒泡排序后,关键字最小的元素到达最上端。
接着,再在剩下的元素中找关键字次小的元素,并把它换在第二个位置上。
依次类推,一直到所有元素都有序为止。
图 1:冒泡排序算法思想2.快速排序:快速排序是对冒泡排序的一种改进。
它的基本思想是:通过一趟排序将待排记录分割成独立的两部分,其中一部分记录的关键字均比另一部分记录的关键字,则可分别对这两部分记录继续进行排序,以达到整个序列有序。
C语言设计实现销售管理系统源代码
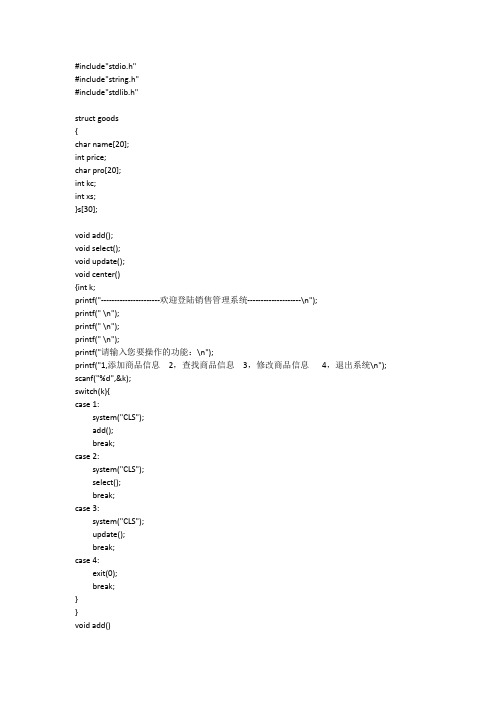
#include"stdio.h"#include"string.h"#include"stdlib.h"struct goods{char name[20];int price;char pro[20];int kc;int xs;}s[30];void add();void select();void update();void center(){int k;printf("----------------------欢迎登陆销售管理系统--------------------\n");printf(" \n");printf(" \n");printf(" \n");printf("请输入您要操作的功能:\n");printf("1,添加商品信息2,查找商品信息3,修改商品信息4,退出系统\n"); scanf("%d",&k);switch(k){case 1:system("CLS");add();break;case 2:system("CLS");select();break;case 3:system("CLS");update();break;case 4:exit(0);break;}}void add()FILE *fp;fp=fopen("goods.txt","a");printf("请输入商品名称单价生产厂家库存量销售量\n"); scanf("%s%d%s%d%d",&s[0].name,&s[0].price,&s[0].pro,&s[0].kc,&s[0].xs); fwrite(&s[0],sizeof(struct goods),1,fp);printf("添加成功\n");fclose(fp);center();}void select(){int i;char name[20];int money,k;FILE *fp;fp=fopen("goods.txt","rb");printf("您是要查找1;按商品名称2;生产厂家:\n");scanf("%d",&k);switch(k){case 1:printf("请输入商品名称:\n");scanf("%s",&name);for(i=0;i<30;i++){fread(&s[i],sizeof(struct goods),1,fp);if(strcmp(s[i].name,name)==0){printf("销售量金额库存量为:\n");money=s[i].price*s[i].xs;printf("%d\t%d\t%d\n",s[i].xs,money,s[i].kc);}}break;case 2:printf("请输入生产厂家:\n");scanf("%s",&name);for(i=0;i<30;i++){fread(&s[i],sizeof(struct goods),1,fp);if(strcmp(s[i].pro,name)==0){printf("销售量金额库存量为:\n");money=s[i].price*s[i].xs;printf("%d\t%d\t%d\n",s[i].xs,money,s[i].kc);}break;}fclose(fp);center();}void update(){int i;char name[20];int up,k;FILE *fp;fp=fopen("goods.txt","rb");for(i=0;i<30;i++){fread(&s[i],sizeof(struct goods),1,fp);}fclose(fp);if((fp=fopen("goods.txt","wb"))!=NULL)printf("请输入您要修改的商品名称:\n"); scanf("%s",name);printf("您要修改:1,库存量2,销售量\n"); scanf("%d",&k);switch(k){case 1:for(i=0;i<30;i++){if(strcmp(s[i].name,name)==0){printf("请输入您要修改的值:\n");scanf("%d",&up);s[i].kc=up;printf("修改成功\n");}fwrite(&s[i],sizeof(struct goods),1,fp);}break;case 2:for(i=0;i<30;i++){if(strcmp(s[i].name,name)==0){printf("请输入您要修改的值:\n");scanf("%d",&up);s[i].xs=up;printf("修改成功\n");}fwrite(&s[i],sizeof(struct goods),1,fp); }break;}fclose(fp);center();}int main(){center();}。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
sum = p->danjia*n + sum; //计算购买金额总和
cout << "☆ 按 0 继续购买,其他任意键退出: "; //输入start2=0继续购买,其他退出,可以进行多次购买
cin >> start2;
cout << "\t\t* 【 5 】: 修改商品信息 *\n";
cout << "\t\t* 【 6 】: 查询商品信息 *\n";
cout << "\t\t* 【 0 】: 退出管理系统 *\n";
case 3:head = insert(head);baocun(head); break; //3、插入功能
case 4:head = del(head), n--;baocun(head); break; //4、删除功能
int buy,flag; //buy购买的商品
struct thing *next;
};
struct thing *product(struct thing *p); //商品信息
float sum = 0.0;
struct thing *p;
p = head;
start2=0;
while (start2 == 0)
{
cout << "☆ 请输入购买商品的编号: ";
cin >> num;
cout << "☆ 请输入购买的数量: ";
cout << "\t\t* 【 2 】: 购买商品 *\n";
cout << "\t\t* 【 3 】: 增新商品 *\n";
cout << "\t\t* 【 4 】: 删除商品 *\n";
p = p->next;
} while (p != NULL);
return head;
}
/*******商品出售********/
struct thing *sell(struct thing *head)
{
int num, n, start2; //num购买编号,n购买数量,start2购买标志
cout << "\t\t\t*\t\t ☆欢迎使用商店管理系统☆ *\n";
cout << "\t\t* *\n";
cout << "\t\t* 【 1 】: 商品列表 *\n";
int choose; //功能选择标志
do{
/*********************** 菜单栏 *******************************/
system("cls");
cout << "\t\t◤*****************************************************◥";
p->kucun = p->kucun - n; //购买n个商品,库存就会减少n
}
else
{
cout << "** 对不起,没有此商品! **\n\n\n"; //输入购买的商品编号,库存没有时,输出“对不起,没有此商品!”
goto end;
case 0: cout<<"\t\t\t** 欢迎使用商店管理系统! **\n";exit(0); //0、退出系统并保存
}
}
else cout << " ** 输入错误! **\n";
cout << "\n\t\t\t【1】请选择: 1 菜单"; //输入start1=1,继续进入菜单
int kucun; //kucun库存量
int shuliang; //shuliang卖出去的数量
float danjia; //danjia商品价格
{
switch (choose)
{
case 1:head = product(head);break; //1、显示所有商品信息
case 2:head = product(head); head = sell(head); baocun(head); break; //2、购买商品
/*******************************************/
/* 数据结构课程设计 */
/* 商品销售管理 */
/* */
goto end;
}
if (p->num == num)
{
p->shuliang=n;
p->buy=p->buy+n; //p->buy一次性购买商品总量
p->flag=1; //p->flag记录p->num是否购买,便于购买清单打印
struct thing *del(struct thing *p); //删除商品信息
struct thing *sell(struct thing *head); //商品出售
cout << "\n\t\t\t【2】请选择: 其他任意键结束\n";
cin >> start1;
if (start1 != 1) //输入其他任意键退出
cin >> n;
while (p->num != num&&p->next != NULL)
{
p = p->next;
}
if (p->kucun < n) //购买数量大于库存,显示商品库存不足
{
cout << "** 商品库存不足! **\n\n\n\n";
{
system("color 3a"); //设置系统颜色
int n = 0,start1; //start1开始标志
thing *head = duqu(); //读取
struct thing *insert(struct thing *head); //商品插入
struct thing *review(struct thing *head); //商品修改
struct thing *chaxun(struct thing *head); //商品查询
#include <fstream>
#include <iostream>
using namespace std;
struct thing
{
char name[100], producer[100]; //name商品名称,producer生产商
int num; //num商品标号
case 5:head = review(head);baocun(head); break; //5、修改商品信息
case 6:head = chaxun(head); break; //6、查询商品信息
{ cout<<"\t\t\t** 欢迎使用商店管理系统! **\n";exit(0); }
} while (1);
}
/*******商品信息********/
thing *product(thing *head) பைடு நூலகம்
{
system("color 2c");
/* */
/* */
/*******************************************/
/****** 商品销售管理系统 *****/
cout << "\t\t◣*****************************************************◢\n\n";
cout << "☆ 请输入要选择的功能: ";
cin >> choose;
if (choose >= 0 && choose <= 6)
cout << "\t产品编号\t产品名字\t单价\t库存\t生产商\n";
do
{
cout << "\t" << p->num << "\t\t" << p->name << "\t\t"<< p->danjia << "\t" << p->kucun << "\t" << p->producer << "\n";
struct thing *duqu(); //文件读取
void baocun(struct thing *head); //文件保存
/********* 主函数 ***********/
void main()
thing * p = head;
if (p == NULL)