头插法和尾插法建立单链表
头插法和尾插法建立单链表
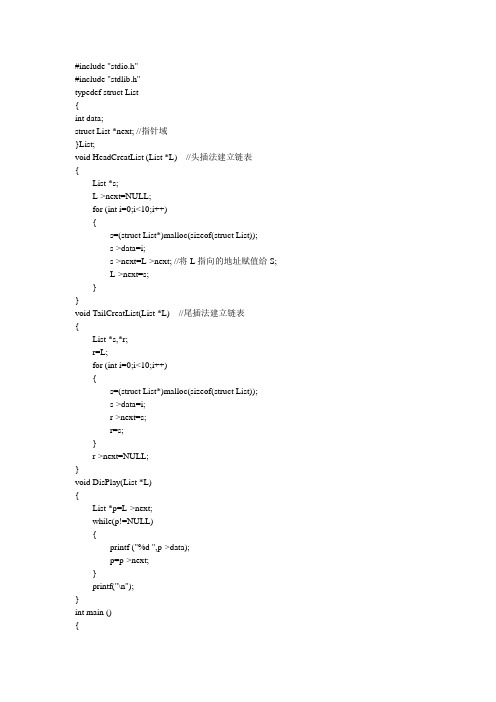
#include "stdio.h"#include "stdlib.h"typedef struct List{int data;struct List *next; //指针域}List;void HeadCreatList (List *L) //头插法建立链表{List *s;L->next=NULL;for (int i=0;i<10;i++){s=(struct List*)malloc(sizeof(struct List));s->data=i;s->next=L->next; //将L指向的地址赋值给S;L->next=s;}}void TailCreatList(List *L) //尾插法建立链表{List *s,*r;r=L;for (int i=0;i<10;i++){s=(struct List*)malloc(sizeof(struct List));s->data=i;r->next=s;r=s;}r->next=NULL;}void DisPlay(List *L){List *p=L->next;while(p!=NULL){printf ("%d ",p->data);p=p->next;}printf("\n");}int main (){List *L1,*L2;L1=(struct List*)malloc(sizeof(struct List));L2=(struct List*)malloc(sizeof(struct List)); HeadCreatList(L1);DisPlay(L1);TailCreatList(L2);DisPlay(L2);}//头插法创建链表#include <stdio.h>#include <stdlib.h>struct node{int data;struct node * next;};//建立只含头结点的空链表struct node * create_list(){struct node * head = NULL;head = (struct node *)malloc(sizeof(struct node));if (NULL == head){printf("memory out of use/n");return NULL;}head->next = NULL;head->data = 0;return head;}//头插法建立链表int insert_form_head(struct node * head, int num){struct node * head_t = head->next;struct node * new_node = NULL;new_node = (struct node *)malloc(sizeof(struct node));if (NULL == new_node){printf("memory out of use/n");return -1;}//将新结点插入到链表的最后new_node->data = num;new_node->next = head_t;head->next = new_node;return 0;}//打印链表int show_list(struct node * head){struct node * temp;temp = head->next;while(temp){printf("%d/n",temp->data);temp = temp->next;}return 0;}// 按值删除结点,头结点不被删除int delete_node(struct node *head, int data){//head_t 保存要删除结点的上一个结点struct node * head_t = head;struct node * temp = NULL;if (head == NULL){printf("delete node from empty list!/n");return -1;}//查找删除的结点的前一个结点//如果此处查找的是删除的结点,则需要另加一个指针保存删除结点的前一个指针while(NULL != head_t->next){if (data == head_t->next->data)break;head_t = head_t->next;}//如果要删除的结点不存在,直接返回if (NULL==head_t->next){printf("node not found/n");return -1;}//删除操作temp = head_t->next;head_t->next = head_t->next->next;free(temp);return 0;}void main(int argc, char* argv[]){struct node * head;head = create_list();if (NULL == head)printf("create_list error/n");insert_form_head(head,123);insert_form_head(head,456);show_list(head);printf("delete once!/n");delete_node(head,123);show_list(head);printf("delete second!/n");delete_node(head,456);show_list(head);delete_node(head,0);show_list(head);}/*//尾插法创建链表#include<stdio.h>#include<stdlib.h>struct Node{int data;struct Node * next;};//建立只含头结点的空链表struct Node * create_list(){struct Node * head = NULL;head = (struct Node *)malloc(sizeof(struct Node));if (NULL == head){printf("memory out of use/n");return NULL;}head->next = NULL;head->data = 0;return head;//尾插法建立链表int insert_form_tail(struct Node * head, int num){struct Node * temp = head;struct Node * new_node = NULL;new_node = (struct Node *)malloc(sizeof(struct Node));if (NULL == new_node){printf("memory out of use/n");return -1;}//寻找尾结点while (temp->next != NULL){temp = temp->next;}//将新结点插入到链表的最后new_node->data = num;new_node->next = NULL;temp->next = new_node;return 0;}//打印链表int show_list(struct Node * head){struct Node * temp;temp = head->next;while(temp){printf("%d/n",temp->data);temp = temp->next;}return 0;}void main(int argc, char* argv[]){struct Node * head;head = create_list();if (NULL == head)printf("create_list error/n");insert_form_tail(head,123);insert_form_tail(head,456);show_list(head);*/。
c语言链表头插法

c语言链表头插法C语言是一门广泛应用于嵌入式系统和操作系统开发等领域的语言,而链表头插法是其中一种非常常用的数据结构处理方法。
本文主要围绕C语言链表头插法展开阐述,分为以下几个步骤:1. 了解链表的概念链表是一种常见的数据结构,它由一个个结点通过指针相连而组成。
每个结点包含两个部分:数据域和指针域。
数据域存储实际数据,指针域存储下一个结点的地址。
链表中第一个结点称为头结点,最后一个结点称为尾结点。
链表的特点是可以在任意位置方便地添加、删除和查找元素。
2. 理解头插法的含义头插法是一种在链表头部插入新结点的方法,相应的还有尾插法。
在操作时,先将新结点的指针域指向原头结点,再将头结点更新为新结点,从而实现在头部插入新元素。
尾插法则是在链表尾部添加新结点。
3. 理解链表头文件中结构体的定义链表通常需要定义一个结构体,用于存储每个结点的数据和指针域信息。
在C语言中链表结构体通常包含两个部分,分别是数据域和指针域。
例如下面的结构体定义:```struct Node{int data;struct Node *next;};```其中data存储结点数据,next存储指向下一个结点的指针。
next也可以用来表示链表的结束,当其指向NULL时,链表结束。
4. 实现链表头插法链表头插法的具体实现如下:```void list_add_head(struct Node **head, int data){// 创建新结点struct Node *new_node = (structNode*)malloc(sizeof(struct Node));new_node->data = data;// 更新头结点为新结点的指针new_node->next = *head;*head = new_node;}```该函数的参数是指向头结点指针的指针以及要插入的数据。
首先,在堆内存中创建一个新结点,然后将其指针域指向原头结点。
《数据结构》-头插法建立单链表

LinkList head;
ListNode *s;
head=NULL;
printf("请输入链表各结点的数据(字符型):\n");
while((ch=getchar())!='\n')
{
s=(ListNode *)malloc(sizeof(ListNode ));
if(s==NULL)
{
printf("申请存储空间失败!");return head;
2020
数据结构
Data structure
头插法建立单链表
讲授:XXX
0.5 头插法建立单链表
要 假三设求、线:性链表表中的结插点入的数据类型是字符型,逐个输入这些字符,并以换行符‘\n’作为输入结束的条
件,使用头插法动态地建立单链表(不带头结点)。
算法思路:
从一个空表开始,重复读入数据,生成新结点,将读入数据存放在新结点的数据域中,然后将新结 点插入到当前链表的表头上,直到读入换行符'\n'为止。
图2-6 新结点前插
图2-7 头指针指向新结点
重复进行第②步到第⑥步,便可建立一个含有多个结点的 不带头结点的单链表,如图2-8。
head s
d
c
b
a^
图2-8 头插法建单链表
04
0.5
05
头插法建立单链表
具体算法:
LinkList CreatListF(void)
{//头插法建立单链表
DataType ch;
算法步骤:
①将头指针head置为
②读取字符ch,判断ch与'\n'是否不相
NULL,转向②。如图2-3。 等,若是转向③,否则,返回head。
头插法与尾插法
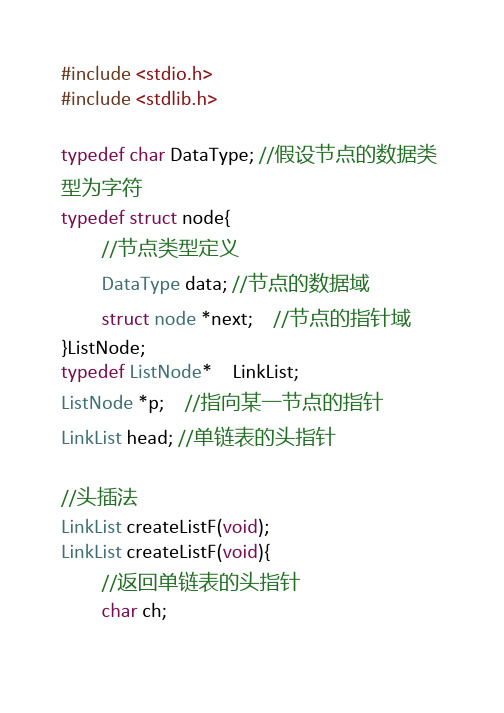
#include <stdio.h>#include <stdlib.h>typedef char DataType; //假设节点的数据类型为字符typedef struct node{//节点类型定义DataType data; //节点的数据域struct node *next; //节点的指针域}ListNode;typedef ListNode* LinkList;ListNode *p; //指向某一节点的指针LinkList head; //单链表的头指针//头插法LinkList createListF(void);LinkList createListF(void){//返回单链表的头指针char ch;LinkList head; //头指针ListNode *s; //工作指针head = NULL; //链表开始为空ch = getchar(); //读入第一个字符while (ch != '\n') {s =(ListNode*)malloc(sizeof(ListNode)); //生成新节点s->data = ch; //将读入的数据放入新节点的数据域中s->next = head;head = s;ch = getchar(); //读入下一个字符}return head;}//尾插法LinkList createListR(void);LinkList createListR(void){//返回头指针char ch;LinkList head; //头指针ListNode *s,*r; //工作指针head = NULL; //链表开始为空r = NULL; //尾指针初始值为空ch = getchar(); //读入第一个字符while (ch != '\n') {s =(ListNode*)malloc(sizeof(ListNode)); //生成新节点s->data=ch; //将读入的数据放入新节点的数据域中s->next=NULL;if (head==NULL) {head = s; //新节点插入空表,头节点指向插入的第一个节点r = s; //尾节点指向插入的第一个节点}else{r->next = s; //将新节点查到*r 之后r = s; //尾指针指向新表尾}ch = getchar();} //endwhilereturn head;}int main(int argc, const char * argv[]){LinkList l = createListR();while (l != NULL) {printf("%c",l->data);l = l->next;}printf("\n");return0; }。
CC++实现单向循环链表(尾指针,带头尾节点)
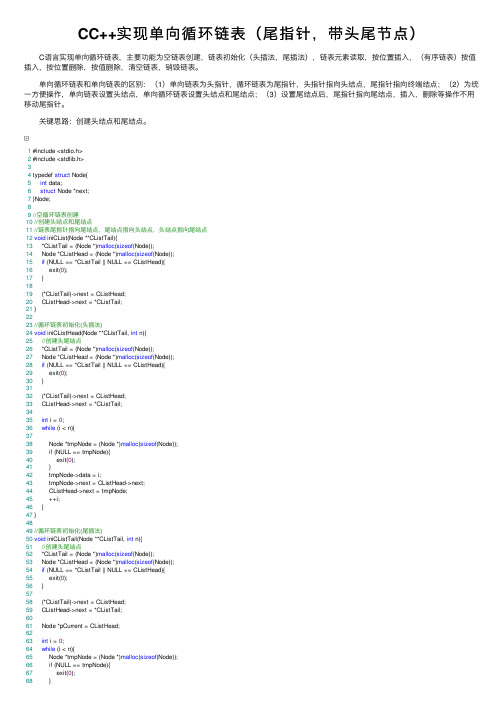
CC++实现单向循环链表(尾指针,带头尾节点) C语⾔实现单向循环链表,主要功能为空链表创建,链表初始化(头插法,尾插法),链表元素读取,按位置插⼊,(有序链表)按值插⼊,按位置删除,按值删除,清空链表,销毁链表。
单向循环链表和单向链表的区别:(1)单向链表为头指针,循环链表为尾指针,头指针指向头结点,尾指针指向终端结点;(2)为统⼀⽅便操作,单向链表设置头结点,单向循环链表设置头结点和尾结点;(3)设置尾结点后,尾指针指向尾结点,插⼊,删除等操作不⽤移动尾指针。
关键思路:创建头结点和尾结点。
1 #include <stdio.h>2 #include <stdlib.h>34 typedef struct Node{5int data;6struct Node *next;7 }Node;89//空循环链表创建10//创建头结点和尾结点11//链表尾指针指向尾结点,尾结点指向头结点,头结点指向尾结点12void iniCList(Node **CListTail){13 *CListTail = (Node *)malloc(sizeof(Node));14 Node *CListHead = (Node *)malloc(sizeof(Node));15if (NULL == *CListTail || NULL == CListHead){16 exit(0);17 }1819 (*CListTail)->next = CListHead;20 CListHead->next = *CListTail;21 }2223//循环链表初始化(头插法)24void iniCListHead(Node **CListTail, int n){25//创建头尾结点26 *CListTail = (Node *)malloc(sizeof(Node));27 Node *CListHead = (Node *)malloc(sizeof(Node));28if (NULL == *CListTail || NULL == CListHead){29 exit(0);30 }3132 (*CListTail)->next = CListHead;33 CListHead->next = *CListTail;3435int i = 0;36while (i < n){3738 Node *tmpNode = (Node *)malloc(sizeof(Node));39if (NULL == tmpNode){40 exit(0);41 }42 tmpNode->data = i;43 tmpNode->next = CListHead->next;44 CListHead->next = tmpNode;45 ++i;46 }47 }4849//循环链表初始化(尾插法)50void iniCListTail(Node **CListTail, int n){51//创建头尾结点52 *CListTail = (Node *)malloc(sizeof(Node));53 Node *CListHead = (Node *)malloc(sizeof(Node));54if (NULL == *CListTail || NULL == CListHead){55 exit(0);56 }5758 (*CListTail)->next = CListHead;59 CListHead->next = *CListTail;6061 Node *pCurrent = CListHead;6263int i = 0;64while (i < n){65 Node *tmpNode = (Node *)malloc(sizeof(Node));66if (NULL == tmpNode){67 exit(0);68 }69 tmpNode->data = i;70 tmpNode->next = *CListTail;71 pCurrent->next = tmpNode;72 pCurrent = tmpNode;7374 ++i;75 }76 }7778//循环链表按位置插⼊79void insertCListPos(Node *CList, int pos, int val){ 8081 Node *pCurrent = CList->next; //指向头结点82int i = 1;83while (pCurrent != CList && i < pos){84 pCurrent = pCurrent->next;85 ++i;86 }8788 Node *tmpNode = (Node *)malloc(sizeof(Node)); 89if (NULL == tmpNode){90 exit(0);91 }92 tmpNode->data = val;93 tmpNode->next = pCurrent->next;94 pCurrent->next = tmpNode;9596 }9798//有序循环链表,按值插⼊99void insertCListValue(Node *CList, int val){100 Node *pCur = CList->next->next;101 Node *pPer = CList->next;102103while (pCur != CList && pCur->data < val){ 104 pPer = pCur;105 pCur = pCur->next;106 }107108 Node *tmpNode = (Node *)malloc(sizeof(Node)); 109if (NULL == tmpNode){110 exit(0);111 }112 tmpNode->data = val;113 tmpNode->next = pPer->next;114 pPer->next = tmpNode;115 }116117//循环链表,按位置删除118void deleteCListPos(Node *CList, int pos){119 Node *pCur = CList->next;120121int i = 1;122while (pCur != CList && i < pos){123 pCur = pCur->next;124 ++i;125 }126127 Node *tmpNode = pCur->next;128 pCur->next = tmpNode->next;129free(tmpNode);130 }131132//循环链表,按值删除133//删除空链表为出问题134void deleteCListValue(Node *CList, int val){135 Node *pCur = CList->next->next;136 Node *pPer = CList->next;137138while (pCur != CList && pCur->data != val){ 139 pPer = pCur;140 pCur = pCur->next;141 }142if (pCur == CList)143return;144else{145 pPer->next = pCur->next;146free(pCur);147 }148 }149150//循环链表,清空链表151void claerCList(Node *CList){152 Node *p = CList->next->next;153 Node *q = NULL;154155while (p != CList){ //到达表尾156 q = p->next;157free(p);158 p = q;159 }160161 CList->next = CList; //将头结点指向尾结点162 }163164//循环链表,销毁链表165void destoryCList(Node **CList){166 Node *p = (*CList)->next;167 Node *q = NULL;168169while (p != (*CList)->next){ //到达表头170 q = p->next;171free(p);172 p = q;173 }174175 *CList = NULL;176 }177178//获取元素179void getCList(Node *CList, int pos, int *val){180 Node *pCur = CList->next->next;181int i = 1;182while (pCur != CList && i < pos){183 pCur = pCur->next;184 ++i;185 }186187 *val = pCur->data;188 }189//遍历输出元素190void printCList(Node *CList){191 Node * tmpNode = CList->next->next;192while (tmpNode != CList){ //到达表尾193 printf("%d\n", tmpNode->data);194 tmpNode = tmpNode->next;195 }196 }197198199int main(){200 Node *CList = NULL;201//iniCListHead(&CList, 8);202//iniCList(&CList);203 iniCListTail(&CList, 8);204205//insertCListPos(CList, 1, 2);206//insertCListPos(CList, 2, 4);207//insertCListPos(CList, 3, 6);208//209//insertCListValue(CList, 1);210//211//deleteCListPos(CList, 3);212//213//deleteCListValue(CList, 6);214215//claerCList(CList);216217int a = 0;218 getCList(CList, 2, &a);219 printf("%d\n", a);220221 printCList(CList);222223 printf("%d\n", CList);224 destoryCList(&CList);225 printf("%d\n", CList);226227 system("pause");228return0;229 }C语⾔完整代码 通过C++实现C语⾔的链表,主要区别:(1)struct可以不通过typedef,直接使⽤Node;(2)将malloc和free更换为new和delete1 #include <stdio.h>2 #include <stdlib.h>34struct Node{5int data;6struct Node *next;7 };89//空循环链表创建10//创建头结点和尾结点11//链表尾指针指向尾结点,尾结点指向头结点,头结点指向尾结点 12void iniCList(Node **CListTail){13 *CListTail = new Node;14 Node *CListHead = new Node;1516 (*CListTail)->next = CListHead;17 CListHead->next = *CListTail;18 }1920//循环链表初始化(头插法)21void iniCListHead(Node **CListTail, int n){22//创建头尾结点23 *CListTail = new Node;24 Node *CListHead = new Node;2526 (*CListTail)->next = CListHead;27 CListHead->next = *CListTail;2829int i = 0;30while (i < n){31 Node *tmpNode = new Node;3233 tmpNode->data = i;34 tmpNode->next = CListHead->next;35 CListHead->next = tmpNode;36 ++i;37 }38 }3940//循环链表初始化(尾插法)41void iniCListTail(Node **CListTail, int n){42//创建头尾结点43 *CListTail = new Node;44 Node *CListHead = new Node;4546 (*CListTail)->next = CListHead;47 CListHead->next = *CListTail;4849 Node *pCurrent = CListHead;5051int i = 0;52while (i < n){53 Node *tmpNode = new Node;5455 tmpNode->data = i;56 tmpNode->next = *CListTail;57 pCurrent->next = tmpNode;58 pCurrent = tmpNode;5960 ++i;61 }62 }6364//循环链表按位置插⼊65void insertCListPos(Node *CList, int pos, int val){6667 Node *pCurrent = CList->next; //指向头结点68int i = 1;69while (pCurrent != CList && i < pos){70 pCurrent = pCurrent->next;71 ++i;72 }7374 Node *tmpNode = new Node;7576 tmpNode->data = val;77 tmpNode->next = pCurrent->next;78 pCurrent->next = tmpNode;7980 }8182//有序循环链表,按值插⼊83void insertCListValue(Node *CList, int val){84 Node *pCur = CList->next->next;85 Node *pPer = CList->next;8687while (pCur != CList && pCur->data < val){88 pPer = pCur;89 pCur = pCur->next;90 }9192 Node *tmpNode = new Node;9394 tmpNode->data = val;95 tmpNode->next = pPer->next;96 pPer->next = tmpNode;97 }9899//循环链表,按位置删除100void deleteCListPos(Node *CList, int pos){ 101 Node *pCur = CList->next;102103int i = 1;104while (pCur != CList && i < pos){105 pCur = pCur->next;106 ++i;107 }108109 Node *tmpNode = pCur->next;110 pCur->next = tmpNode->next;111delete tmpNode;112 }113114//循环链表,按值删除115//删除空链表为出问题116void deleteCListValue(Node *CList, int val){ 117 Node *pCur = CList->next->next;118 Node *pPer = CList->next;119120while (pCur != CList && pCur->data != val){ 121 pPer = pCur;122 pCur = pCur->next;123 }124if (pCur == CList)125return;126else{127 pPer->next = pCur->next;128delete pCur;129 }130 }131132//循环链表,清空链表133void claerCList(Node *CList){134 Node *p = CList->next->next;135 Node *q = NULL;136137while (p != CList){ //到达表尾138 q = p->next;139delete p;140 p = q;141 }142143 CList->next = CList; //将头结点指向尾结点144 }145146//循环链表,销毁链表147void destoryCList(Node **CList){148 Node *p = (*CList)->next;149 Node *q = NULL;150151while (p != (*CList)->next){ //到达表头152 q = p->next;153delete p;154 p = q;155 }156157 *CList = NULL;158 }159160//获取元素161void getCList(Node *CList, int pos, int *val){ 162 Node *pCur = CList->next->next;163int i = 1;164while (pCur != CList && i < pos){165 pCur = pCur->next;166 ++i;167 }168169 *val = pCur->data;170 }171//遍历输出元素172void printCList(Node *CList){173 Node * tmpNode = CList->next->next;174while (tmpNode != CList){ //到达表尾175 printf("%d\n", tmpNode->data);176 tmpNode = tmpNode->next;177 }178 }179180181int main(){182 Node *CList = NULL;183//iniCListHead(&CList, 8);184//iniCList(&CList);185 iniCListTail(&CList, 8);186187//insertCListPos(CList, 1, 2);188//insertCListPos(CList, 2, 4);189//insertCListPos(CList, 3, 6);190//191//insertCListValue(CList, 1);192//193//deleteCListPos(CList, 3);194//195//deleteCListValue(CList, 6);196197//claerCList(CList);198199int a = 0;200 getCList(CList, 2, &a);201 printf("%d\n", a);202203 printCList(CList);204205 printf("%d\n", CList);206 destoryCList(&CList);207 printf("%d\n", CList);208209 system("pause");210return0;211 }C++完整代码单向循环链表 注意:(1)单向循环链表销毁时,需要将头结点和尾结点删除;(2)单向循环链表插⼊,删除,遍历,清空链表时,条件从头结点或第⼀节点始,判断指针是否达到尾结点;(3)清空链表时,最后将头结点指向尾结点;(4)销毁链表时,条件从头结点始,判断条件为指针是否到达头结点,最后将指针置空。
实验二:单链表基本运算实现

{
int data; //存放表结点值
struct node *next; //存放表结点的直接后驱元素的地址
} ListNode,*LinkList;
//头插法创建初始链表
LinkList create_h(int size)
{
;//将工作指针p指向后一个结点
;//计数器累加
}
return i;
}
//打印链表中的现有元素
printList(LinkList head)
{
LinkList p;
;//将工作指针p指向第1个结点
{
p=(LinkList)malloc(sizeof(ListNode));//申请新结点的存储空间
scanf("%d",&p->data);//读入新结点的值到data域中
;//将新增结点插到头结点的后面
;//将新增结点插到头结点的后面
printf("————头插法建立链表(1)\n");
printf("————尾插法建立链表(2)\n");
printf("————按位查找元素(3)\n");
printf("————按值查找元素(4)\n");
printf("————插入元素(5)\n");
printf("————删除元素(6)\n");
printList(h);
break;
case 5:
printf("插入元素,请输入要插入元素的值:\n");
链式存储(头插法、尾插法)

链式存储(头插法、尾插法)#include "stdio.h"#include "string.h"#include "ctype.h"#include "stdlib.h"#include "io.h"#include "math.h"#include "time.h"#define OK 1#define ERROR 0#define TRUE 1#define FALSE 0#define MAXSIZE 20 // 存储空间初始分配量typedef int Status;// Status是函数的类型,其值是函数结果状态代码。
如OK等typedef int ElemType;//ElemType类型依据实际情况⽽定,这⾥如果为intStatus visit(ElemType c){printf("%d ",c);return OK;}typedef struct Node{ElemType data;struct Node *next;}Node;typedef struct Node *LinkList; // 定义LinkList// 初始化顺序线性表Status InitList(LinkList *L){*L=(LinkList)malloc(sizeof(Node)); // 产⽣头结点,并使L指向此头结点if(!(*L)) // 存储分配失败return ERROR;(*L)->next=NULL; //指针域为空return OK;}//初始条件:顺序线性表L已存在。
操作结果:若L为空表,则返回TRUE,否则返回FALSEStatus ListEmpty(LinkList L){if(L->next)return FALSE;elsereturn TRUE;}// 初始条件:顺序线性表L已存在。
单链表的基本操作(查找,插入,删除)
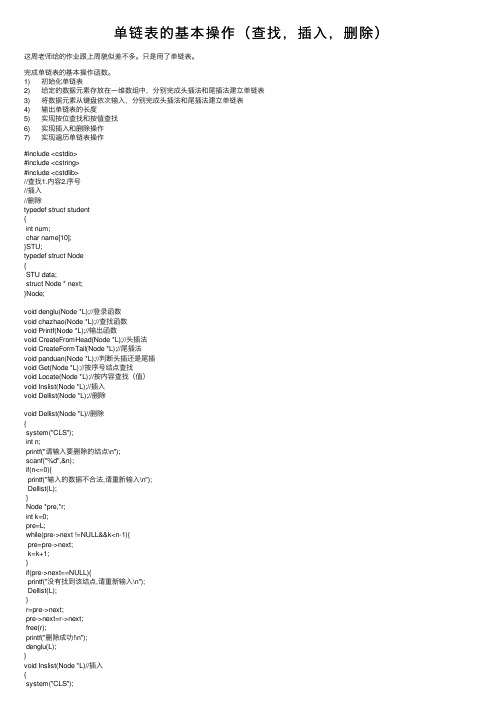
单链表的基本操作(查找,插⼊,删除)这周⽼师给的作业跟上周貌似差不多。
只是⽤了单链表。
完成单链表的基本操作函数。
1) 初始化单链表2) 给定的数据元素存放在⼀维数组中,分别完成头插法和尾插法建⽴单链表3) 将数据元素从键盘依次输⼊,分别完成头插法和尾插法建⽴单链表4) 输出单链表的长度5) 实现按位查找和按值查找6) 实现插⼊和删除操作7) 实现遍历单链表操作#include <cstdio>#include <cstring>#include <cstdlib>//查找1.内容2.序号//插⼊//删除typedef struct student{int num;char name[10];}STU;typedef struct Node{STU data;struct Node * next;}Node;void denglu(Node *L);//登录函数void chazhao(Node *L);//查找函数void Printf(Node *L);//输出函数void CreateFromHead(Node *L);//头插法void CreateFormTail(Node *L);//尾插法void panduan(Node *L);//判断头插还是尾插void Get(Node *L);//按序号结点查找void Locate(Node *L);//按内容查找(值)void Inslist(Node *L);//插⼊void Dellist(Node *L);//删除void Dellist(Node *L)//删除{system("CLS");int n;printf("请输⼊要删除的结点\n");scanf("%d",&n);if(n<=0){printf("输⼊的数据不合法,请重新输⼊\n");Dellist(L);}Node *pre,*r;int k=0;pre=L;while(pre->next !=NULL&&k<n-1){pre=pre->next;k=k+1;}if(pre->next==NULL){printf("没有找到该结点,请重新输⼊\n");Dellist(L);}r=pre->next;pre->next=r->next;free(r);printf("删除成功!\n");denglu(L);}void Inslist(Node *L)//插⼊{system("CLS");int n;printf("请输⼊在第⼏个位置插⼊数据\n");scanf("%d",&n);printf("请输⼊插⼊的学号,姓名\n");int num1;char name1[10];scanf("%d %s",&num1,name1);Node *pre,*s;int k=0;if(n<=0){printf("输⼊的数据不合法,请重新输⼊\n");Inslist(L);}pre=L;while(pre!=NULL&&k<n-1){pre=pre->next;k=k+1;}if(pre==NULL){printf("⽆法找到该节点,请重新输⼊\n");Inslist(L);}s=(Node*)malloc(sizeof(Node));strcpy(s->data .name ,name1);s->data.num=num1;s->next =pre->next ;pre->next =s;printf("插⼊成功!\n");denglu(L);}void Locate(Node *L)//按内容查找(值){system("CLS");int n;printf("请输⼊要查找的学号\n");scanf("%d",&n);Node *p;p=L->next;while(p!=NULL){if(p->data.num!=n){p=p->next;}elsebreak;}printf("你要查找的学号所对应的信息为%d %s\n",p->data.num,p->);denglu(L);}void Get(Node *L)//按序号结点查找{system("CLS");int n;printf("请输⼊你要查找的结点\n");scanf("%d",&n);if(n<=0){printf("输⼊的数据不合法,请重新输⼊\n");Get(L);}Node *p;p=L;int j=0;while((p->next!=NULL)&&(j<n)){p=p->next;j++;}if(n==j){printf("你要查找的结点的储存位置的数据为%d %s\n",p->data.num,p->); }denglu(L);}void Printf(Node *L){int q=0;Node *p=L->next;while(p!=NULL){q++;printf("%d %s\n",p->data.num,p->); p=p->next;}printf("单链表长度为%d\n",q);denglu(L);}void chazhao(Node *L){printf("1.按序号查找\n");printf("2.按内容查找\n");printf("3.返回主界⾯\n");int aa;scanf("%d",&aa);switch(aa){case 1:Get(L);case 2:Locate(L);case 3:denglu(L);break;default:printf("输⼊错误请重新输⼊\n");chazhao(L);}}void denglu(Node *L){int a;printf("请选择你要做什么\n");printf("1.查找\n");printf("2.插⼊\n");printf("3.删除\n");printf("4.打印现有的学⽣信息及单链表长度\n"); printf("5.退出\n");scanf("%d",&a);switch(a){case 1:chazhao(L);case 2:Inslist(L);case 3:Dellist(L);case 4:Printf(L);case 5:printf("谢谢使⽤\n");exit(0);default:printf("输⼊错误请重新输⼊\n");denglu(L);}}void CreateFromHead(Node *L)//头插法{Node *s;int n;//n为元素个数printf("请输⼊元素个数\n");scanf("%d",&n);printf("请输⼊学号姓名\n");for(int i=1;i<=n;i++){s=(Node *)malloc(sizeof(Node));scanf("%d %s",&s->data.num,s->); s->next=L->next;L->next=s;}}void CreateFormTail(Node *L)//尾插法{Node *s,*r;r=L;int n;//n为元素个数printf("请输⼊元素个数\n");scanf("%d",&n);printf("请输⼊学号姓名\n");for(int i=1;i<=n;i++){s=(Node *)malloc(sizeof(Node));scanf("%d %s",&s->data.num,s->);r->next=s;r=s;if(i==n){r->next=NULL;}}}Node *InitList(Node *L)//初始化单链表{L=(Node *)malloc(sizeof(Node));L->next=NULL;return L;}void panduan(Node *L){int q;printf("请选择⽤哪种⽅式建⽴链表\n");printf("1.头插法\n");printf("2.尾插法\n");scanf("%d",&q);switch(q){case (1):CreateFromHead(L);printf("输⼊成功!\n");break;case (2):CreateFormTail(L);printf("输⼊成功!\n");break;default:printf("输⼊错误请重新输⼊\n");panduan(L);}}int main(){Node *L=NULL;L=InitList(L);panduan(L);denglu(L);return 0;}ps.贴上来的代码空格有点⼩奇怪啊。
数据结构___头插法和尾插法建立链表(各分有无头结点)

实验一链表的建立及基本操作方法实现一、【实验目的】1、理解和掌握单链表的类型定义方法和结点生成方法。
2、掌握利用头插法和尾插法建立单链表和显示单链表元素的算法。
3、掌握单链表的查找(按序号)算法。
4、掌握单链表的插入、删除算法。
二、【实验内容】1、利用头插法和尾插法建立一个无头结点单链表,并从屏幕显示单链表元素列表。
2、利用头插法和尾插法建立一个有头结点单链表,并从屏幕显示单链表元素列表。
3、将测试数据结果用截图的方式粘贴在程序代码后面。
重点和难点:尾插法和头插法建立单链表的区别。
建立带头结点和无头结点单链表的区别。
带头结点和无头结点单链表元素显示方法的区别三、【算法思想】1) 利用头插法和尾插法建立一个无头结点单链表链表无头结点,则在创建链表时,初始化链表指针L=NULL。
当用头插法插入元素时,首先要判断头指针是否为空,若为空,则直接将新结点赋给L,新结点next指向空,即L=p,p->next=NULL,若表中已经有元素了,则将新结点的next指向首结点,然后将新结点赋给L即(p->next=L,L=p)。
当用尾插法插入元素时,首先设置一个尾指针tailPointer以便随时指向最后一个结点,初始化tailPointer和头指针一样即tailPointer=L。
插入元素时,首先判断链表是否为空,若为空,则直接将新结点赋给L即L=p,若不为空,else将最后一个元素的next指向新结点即tailPointer->next=p,然后跳出这个if,else语句,将新结点next指向空,并且将tailPointer指向新结点即p->next=NULL,tailPointer=p。
2) 利用头插法和尾插法建立一个有头结点单链表链表有头结点,则在创建链表时,初始化链表指针L->next = NULL。
与无头结点区别在于,判断链表为空是根据L->next是否为空。
用头插法插入元素时,要判断链表是否为空,若为空则将新结点next指向空,作为表尾,若不为空,则直接插入,将新结点next指向头结点next的指向,再将头结点next指向新结点即p->next=L->next,L->next=p。
本题目要求利用尾插法建立单链表。
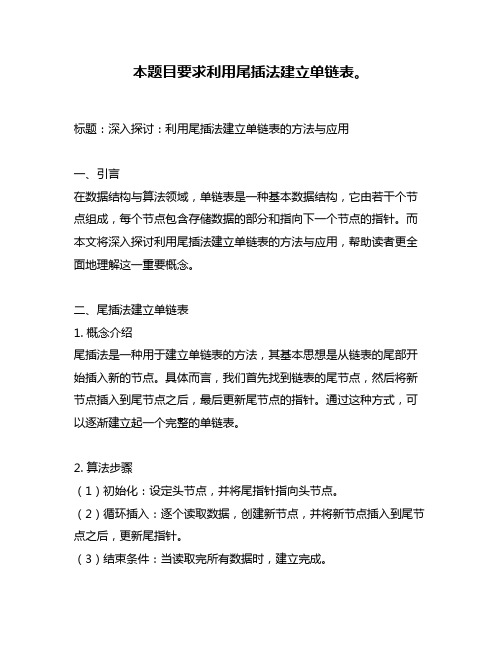
本题目要求利用尾插法建立单链表。
标题:深入探讨:利用尾插法建立单链表的方法与应用一、引言在数据结构与算法领域,单链表是一种基本数据结构,它由若干个节点组成,每个节点包含存储数据的部分和指向下一个节点的指针。
而本文将深入探讨利用尾插法建立单链表的方法与应用,帮助读者更全面地理解这一重要概念。
二、尾插法建立单链表1. 概念介绍尾插法是一种用于建立单链表的方法,其基本思想是从链表的尾部开始插入新的节点。
具体而言,我们首先找到链表的尾节点,然后将新节点插入到尾节点之后,最后更新尾节点的指针。
通过这种方式,可以逐渐建立起一个完整的单链表。
2. 算法步骤(1)初始化:设定头节点,并将尾指针指向头节点。
(2)循环插入:逐个读取数据,创建新节点,并将新节点插入到尾节点之后,更新尾指针。
(3)结束条件:当读取完所有数据时,建立完成。
3. 代码示例以下是用C语言实现尾插法建立单链表的简单示例代码:```c#include <stdio.h>#include <stdlib.h>typedef struct Node {int data;struct Node *next;} Node;Node* createList(int arr[], int n) {Node *head = (Node *)malloc(sizeof(Node));head->next = NULL;Node *tail = head;for (int i = 0; i < n; i++) {Node *newNode = (Node *)malloc(sizeof(Node)); newNode->data = arr[i];newNode->next = NULL;tail->next = newNode;tail = newNode;}return head;}void printList(Node* head) {Node *p = head->next;while (p != NULL) {printf("%d ", p->data);p = p->next;}}int main() {int arr[] = {1, 2, 3, 4, 5};int n = sizeof(arr) / sizeof(arr[0]); Node *head = createList(arr, n); printList(head);return 0;}```三、尾插法建立单链表的应用1. 数据输入尾插法建立单链表常用于动态读取一系列数据并创建相应的链表。
单链表的头插法和尾插法
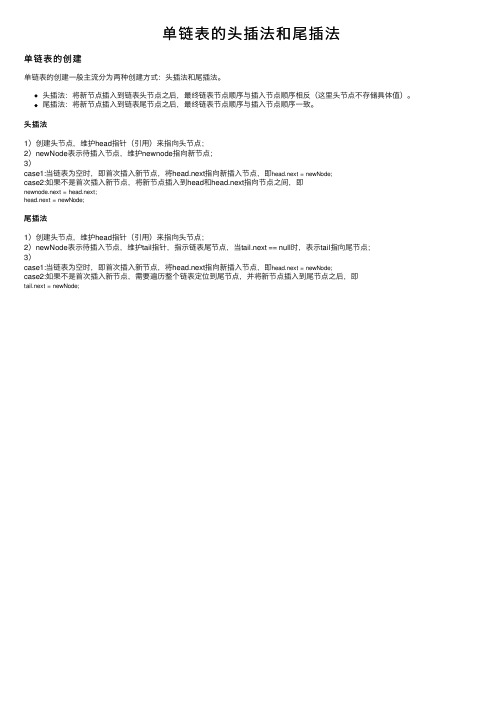
单链表的头插法和尾插法
单链表的创建
单链表的创建⼀般主流分为两种创建⽅式:头插法和尾插法。
头插法:将新节点插⼊到链表头节点之后,最终链表节点顺序与插⼊节点顺序相反(这⾥头节点不存储具体值)。
尾插法:将新节点插⼊到链表尾节点之后,最终链表节点顺序与插⼊节点顺序⼀致。
头插法
1)创建头节点,维护head指针(引⽤)来指向头节点;
2)newNode表⽰待插⼊节点,维护newnode指向新节点;
3)
case1:当链表为空时,即⾸次插⼊新节点,将head.next指向新插⼊节点,即head.next = newNode;
case2:如果不是⾸次插⼊新节点,将新节点插⼊到head和head.next指向节点之间,即
newnode.next = head.next;
head.next = newNode;
尾插法
1)创建头节点,维护head指针(引⽤)来指向头节点;
2)newNode表⽰待插⼊节点,维护tail指针,指⽰链表尾节点,当tail.next == null时,表⽰tail指向尾节点;
3)
case1:当链表为空时,即⾸次插⼊新节点,将head.next指向新插⼊节点,即head.next = newNode;
case2:如果不是⾸次插⼊新节点,需要遍历整个链表定位到尾节点,并将新节点插⼊到尾节点之后,即
tail.next = newNode;。
数据结构实验报告-单链表
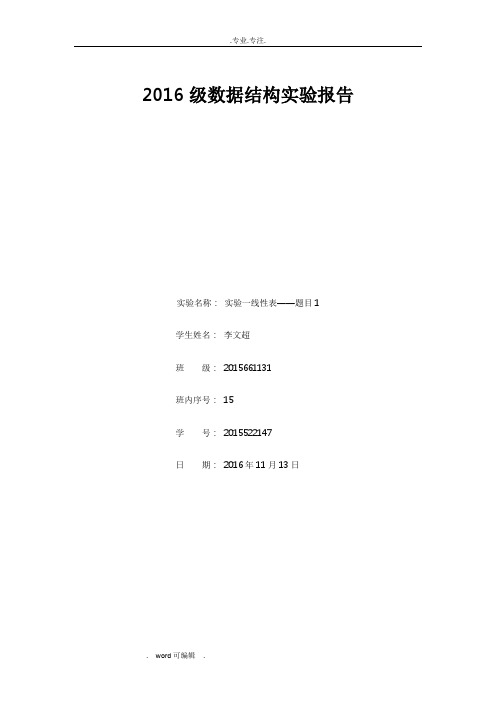
2016级数据结构实验报告实验名称:实验一线性表——题目1学生姓名:李文超班级:2015661131班内序号:15学号:2015522147日期:2016年11月13日1.实验要求实验目的:根据线性表的抽象数据类型的定义,选择下面任一种链式结构实现线性表,并完成线性表的基本功能。
线性表存储结构(五选一):1、带头结点的单链表2、不带头结点的单链表3、循环链表4、双链表5、静态链表线性表的基本功能:1、构造:使用头插法、尾插法两种方法2、插入:要求建立的链表按照关键字从小到大有序3、删除4、查找5、获取链表长度6、销毁7、其他:可自行定义编写测试main()函数测试线性表的正确性。
2.程序分析2.1 存储结构单链表的存储:(1)链表用一组任意的存储单元来存放线性表的结点。
这组存储单元既可以是连续的,也可以是不连续的,甚至零散地分布在内存的某些位置。
(2)链表中结点的逻辑次序和物理次序不一定相同。
为了能正确表示结点间的逻辑关系,在存储每个元素值的同时,还要存储该元素的直接后继元素的位置信息,这个信息称为指针或链。
结点结构┌──┬──┐ data 域---存放结点值的数据域│data │next │ next 域---存放结点的直接后继的地址的指针域└──┴──┘单链表在内存中的存储示意地址 内存单元1000H头指针 1020H1080H10C0H2.2 关键算法分析1、关键算法:(1)头插法自然语言描述:a:在堆中建立新结点b:将a[i]写入到新结点的数据域c:修改新结点的指针域d:修改头结点的指针域。
将新结点加入链表中伪代码描述a:Node <T> * s=new Node <T>b:s->data=a[i]c:s->next=front->next;d:front->next=s(2)尾插法自然语言描述:a:在堆中建立新结点:b:将a[i]写入到新结点的数据域:c:将新结点加入到链表中d:修改修改尾指针伪代码描述a:Node <T> * s=new Node <T>b:s->data=a[i]c:r->next=s;d:r=s(3)遍历打印函数自然语言描述:a:判断该链表是否为空链表,如果是,报错b:如果不是空链表,新建立一个temp指针c:将temp指针指向头结点d:打印temp指针的data域e:逐个往后移动temp指针,直到temp指针的指向的指针的next域为空伪代码描述a: If front->next==NULL①Throw ”an empty list ”②Node<T>* temp=front->next;b:while(temp->next)c:cout<<temp->data<<" ";d:temp=temp->next;(4) 获取链表长度函数自然语言描述:a:判断该链表是否为空链表,如果是,输出长度0b:如果不是空链表,新建立一个temp指针,初始化整形数n为0c:将temp指针指向头结点d:判断temp指针指向的结点的next域是否为空,如果不是,n加一,否则return ne: 使temp指针逐个后移,重复d操作,直到temp指针指向的结点的next域为0,返回n伪代码描述a:if ront->next==NULLb:Node<T>* temp=front->next;c:while(temp->next)d:temp=temp->next;(5)析构/删除函数自然语言描述:a:新建立一个指针,指向头结点b:判断要释放的结点是否存在,c:暂时保存要释放的结点d:移动a中建立的指针e:释放要释放的指针伪代码描述a:Node <T> * p=frontb:while(p)c:front=pd:p=p->nexte:delete front(6)按位查找函数自然语言描述:a:初始化工作指针p和计数器j,p指向第一个结点,j=1b:循环以下操作,直到p为空或者j等于1①:p指向下一个结点②:j加1c:若p为空,说明第i个元素不存在,抛出异常d:否则,说明p指向的元素就是所查找的元素,返回元素地址伪代码描述a:Node <T> * p=front->next;j=1;b:while(p&&j!=1)①:p=p->next②:j++c:if(!p) throw ”error”d:return p(7)按位查找函数自然语言描述:a:初始化工作指针p和计数器j,p指向第一个结点,j=1b:循环以下操作,找到这个元素或者p指向最后一个结点①:判断p指向的结点是不是要查找的值,如果是,返回j,否则p指向下一个结点,并且j的值加一c:如果找到最后一个结点还没有找到要查找的元素,返回查找失败信息伪代码描述a:Node <T> * p=front->next;j=1;b:while(p)①: if(p->next==x) return jp=p->nextj++c:return “error”(8)插入函数自然语言描述:a:在堆中建立新结点b:将要插入的结点的数据写入到新结点的数据域c:修改新结点的指针域d:修改前一个指针的指针域,使其指向新插入的结点的位置伪代码描述a:Node <T> * s=new Node <T>;b:s-data=p->datac:s->next=p->nextd:p->next=se:p->data=x(9)删除函数自然语言描述:a:从第一个结点开始,查找要删除的位数i前一个位置i-1的结点b:设q指向第i个元素c:将q元素从链表中删除d:保存q元素的数据e:释放q元素伪代码描述a:q=p->nextb:p->next=q->nextc:x=q->datad:delete q2、代码详细分析(插入):(1)从第一个结点开始,查找节点,使它的数据比x大,设p指向该结点:while (x>p->data) { p=p->next;}(2)新建一个节点s,把p的数据赋给s:s->data=p->data;(3)把s加到p后面:s->next=p->next; p->next=s;(4)p节点的数据用x替换:p->data=x;示意图如图所示xp->datas3、关键算法的时间复杂度:O(1)3.程序运行结果1. 流程图:2、结果截图3.测试结论:可以正确的对链表进行插入,删除,取长度,输出操作。
国家开放大学《数据结构》课程实验报告(实验2——线性表)参考答案
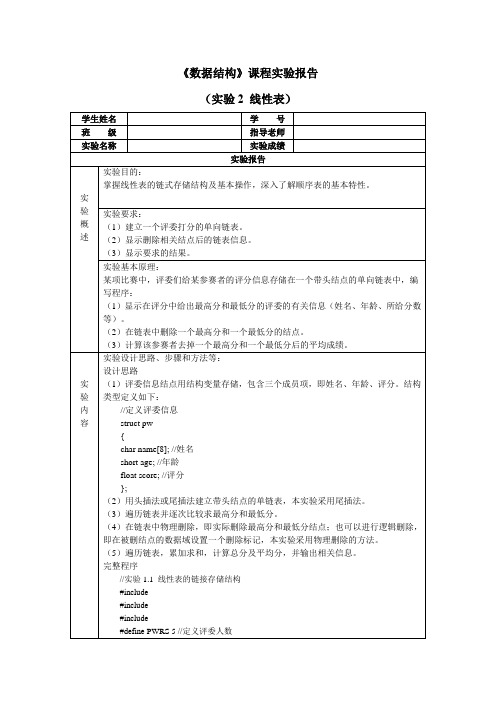
//在链表中删除最高分和最低分结点
for(q=head,p=head->next;p!=NULL;q=p,p=p->next)
{
if(p==pmin) { q->next=p->next; p=q; } //删除最低分结点
};
typedef struct pw PW;
//定义链表结点
struct node
{
PW data;
struct node * next;
};
typedef struct node NODE;
NODE *create(int n); //建立单链表
void input(NODE *s,int i); //输入第i个评委信息
(5)遍历链表,累加求和,计算总分及平均分,并输出相关信息。
完整程序
//实验1.1线性表的链接存储结构
#include
#include
#include
#define PWRS 5 //定义评委人数
//定义评委信息
struct pw
{
char name[8]; //姓名
short age; //年龄
float score; //评分
NODE *create(int n)
{
NODE *head,*p,*q;
inti;
p=(NODE*)malloc(sizeof(NODE));
head=p; q=p; p->next=NULL;
for(i=1; i<=n; i++)
{
p=(NODE*)malloc(sizeof(NODE));
数据结构c语言版上机报告单链表

数据结构C语言版上机报告:单链表序在数据结构课程中,单链表是一个重要的概念,也是C语言中常用的数据结构之一。
本次报告将深入探讨单链表的基本概念、操作方法以及应用场景,帮助读者更深入地理解和掌握这一数据结构。
一、概述1.1 单链表的定义单链表是一种线性表,它由一系列节点组成,每个节点包含两部分:数据域和指针域。
数据域用于存储数据元素,指针域用于指向下一个节点,通过指针将这些节点串联在一起,形成一个链表结构。
1.2 单链表的特点单链表具有以下特点:(1)动态性:单链表的长度可以动态地增加或减少,不需要预先分配固定大小的空间。
(2)插入和删除操作高效:在单链表中进行插入和删除操作时,只需要修改指针的指向,时间复杂度为O(1)。
(3)随机访问效率低:由于单链表采用链式存储结构,无法通过下标直接访问元素,需要从头节点开始依次遍历,时间复杂度为O(n)。
1.3 单链表的基本操作单链表的基本操作包括:创建、插入、删除、查找等。
这些操作是使用单链表时常常会涉及到的,下面将逐一介绍这些操作的具体实现方法和应用场景。
二、创建2.1 头插法和尾插法在C语言中,可以通过头插法和尾插法来创建单链表。
头插法是将新节点插入到链表的头部,尾插法是将新节点插入到链表的尾部,这两种方法各有优缺点,可以根据具体应用场景来选择。
2.2 应用场景头插法适合于链表的逆序建立,尾插法适合于链表的顺序建立。
三、插入3.1 在指定位置插入节点在单链表中,插入节点需要考虑两种情况:在链表头部插入和在链表中间插入。
通过对指针的操作,可以实现在指定位置插入节点的功能。
3.2 应用场景在实际应用中,经常会有需要在指定位置插入节点的情况,比如排序操作、合并两个有序链表等。
四、删除4.1 删除指定节点在单链表中,删除节点同样需要考虑两种情况:删除头节点和删除中间节点。
通过对指针的操作,可以实现删除指定节点的功能。
4.2 应用场景在实际应用中,经常会有需要删除指定节点的情况,比如删除链表中特定数值的节点等。
数据结构头插法和尾插法建立链表(各分有无头结点)

实验一链表的建立及基本操作方法实现一、【实验目的】1、理解和掌握单链表的类型定义方法和结点生成方法。
2、掌握利用头插法和尾插法建立单链表和显示单链表元素的算法。
3、掌握单链表的查找(按序号)算法。
4、掌握单链表的插入、删除算法。
二、【实验内容】1、利用头插法和尾插法建立一个无头结点单链表,并从屏幕显示单链表元素列表。
2、利用头插法和尾插法建立一个有头结点单链表,并从屏幕显示单链表元素列表。
3、将测试数据结果用截图的方式粘贴在程序代码后面。
重点和难点:尾插法和头插法建立单链表的区别。
建立带头结点和无头结点单链表的区别。
带头结点和无头结点单链表元素显示方法的区别三、【算法思想】1) 利用头插法和尾插法建立一个无头结点单链表链表无头结点,则在创建链表时,初始化链表指针L=NULL。
当用头插法插入元素时,首先要判断头指针是否为空,若为空,则直接将新结点赋给L,新结点nex t指向空,即L=p,p->next=NULL,若表中已经有元素了,则将新结点的n ext指向首结点,然后将新结点赋给L即(p->next=L,L=p)。
当用尾插法插入元素时,首先设置一个尾指针ta i lPoi nter以便随时指向最后一个结点,初始化tai lPoin ter和头指针一样即tailP ointe r=L。
插入元素时,首先判断链表是否为空,若为空,则直接将新结点赋给L即L=p,若不为空,else将最后一个元素的next指向新结点即tai l Point er->next=p,然后跳出这个i f,else语句,将新结点ne xt指向空,并且将tai lPoin ter指向新结点即p->next=NULL,tailPoi nter=p。
c语言单链表头插法
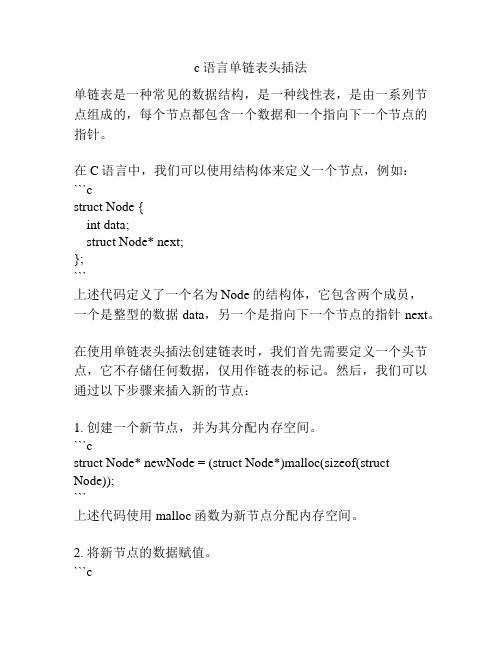
c语言单链表头插法单链表是一种常见的数据结构,是一种线性表,是由一系列节点组成的,每个节点都包含一个数据和一个指向下一个节点的指针。
在C语言中,我们可以使用结构体来定义一个节点,例如:```cstruct Node {int data;struct Node* next;};```上述代码定义了一个名为Node的结构体,它包含两个成员,一个是整型的数据data,另一个是指向下一个节点的指针next。
在使用单链表头插法创建链表时,我们首先需要定义一个头节点,它不存储任何数据,仅用作链表的标记。
然后,我们可以通过以下步骤来插入新的节点:1. 创建一个新节点,并为其分配内存空间。
```cstruct Node* newNode = (struct Node*)malloc(sizeof(struct Node));```上述代码使用malloc函数为新节点分配内存空间。
2. 将新节点的数据赋值。
```cnewNode->data = value;```上述代码将新节点的data成员设置为value。
3. 将新节点的next指针指向头节点的next指针所指向的节点。
```cnewNode->next = head->next;```上述代码将新节点的next指针指向头节点的next指针指向的节点,即将新节点插入到头节点之后。
4. 将头节点的next指针指向新节点。
```chead->next = newNode;```上述代码将头节点的next指针指向新节点,即将新节点设置为链表的第一个节点。
完整的头插法创建链表的函数如下:```cstruct Node* createList(int values[], int n) {struct Node* head = (struct Node*)malloc(sizeof(struct Node));head->next = NULL;for (int i = 0; i < n; i++) {struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));newNode->data = values[i];newNode->next = head->next;}return head;}```上述代码中的createList函数接受一个整型数组values和一个整数n作为参数,返回一个头指针。
数据结构单链表实验报告

数据结构单链表实验报告实验目的:本实验的目的是通过实现单链表数据结构,加深对数据结构的理解,并掌握单链表的基本操作和算法。
实验内容:1、单链表的定义单链表由若干个节点组成,每个节点包含数据域和指针域,数据域存储具体数据,指针域指向下一个节点。
单链表的头指针指向链表的第一个节点。
2、单链表的基本操作2.1 初始化链表初始化链表时,将头指针置空,表示链表为空。
2.2 插入节点插入节点可以分为头插法和尾插法。
- 头插法:将新节点插入链表头部,新节点的指针域指向原头节点,头指针指向新节点。
- 尾插法:将新节点插入链表尾部,新节点的指针域置空,原尾节点的指针域指向新节点。
2.3 删除节点删除节点可以分为按位置删除和按值删除两种方式。
- 按位置删除:给定要删除节点的位置,修改前一节点的指针域即可。
- 按值删除:给定要删除节点的值,遍历链表找到对应节点,修改前一节点的指针域即可。
2.4 遍历链表遍历链表即按顺序访问链表的每个节点,并输出节点的数据。
2.5 查找节点查找节点可以分为按位置查找和按值查找两种方式。
- 按位置查找:给定节点的位置,通过遍历链表找到对应节点。
- 按值查找:给定节点的值,通过遍历链表找到第一个匹配的节点。
实验步骤:1、根据实验目的,定义单链表的结构体和基本操作函数。
2、实现初始化链表的函数,将头指针置空。
3、实现头插法或尾插法插入节点的函数。
4、实现按位置删除节点的函数。
5、实现按值删除节点的函数。
6、实现遍历链表的函数,输出节点的数据。
7、实现按位置查找节点的函数。
8、实现按值查找节点的函数。
9、设计实验样例,测试单链表的各种操作。
实验结果与分析:通过测试实验样例,我们可以验证单链表的各种操作是否正确。
如果出现异常情况,可通过调试找出问题所在,并进行修改。
单链表的操作时间复杂度与操作的位置有关,对于查找操作,时间复杂度为O(n);对于插入和删除操作,时间复杂度也为O(n)。
附件:1、单链表的定义和基本操作的源代码文件。
尾插法创建链表
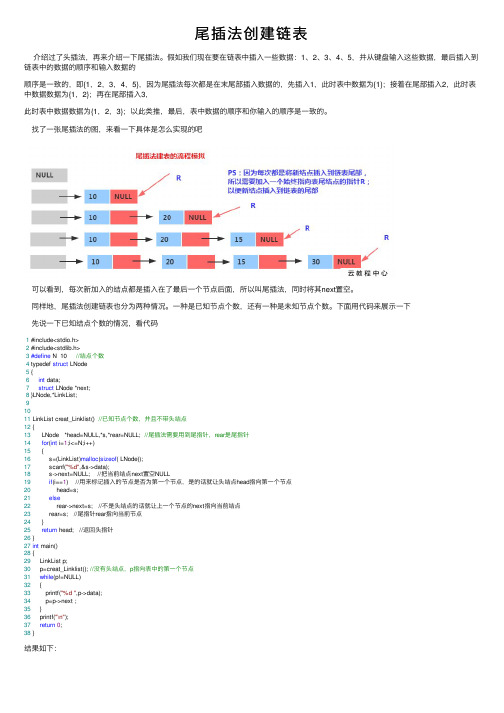
尾插法创建链表介绍过了头插法,再来介绍⼀下尾插法。
假如我们现在要在链表中插⼊⼀些数据:1、2、3、4、5,并从键盘输⼊这些数据,最后插⼊到链表中的数据的顺序和输⼊数据的顺序是⼀致的,即{1,2,3,4,5},因为尾插法每次都是在末尾部插⼊数据的,先插⼊1,此时表中数据为{1};接着在尾部插⼊2,此时表中数据数据为{1,2};再在尾部插⼊3,此时表中数据数据为{1,2,3};以此类推,最后,表中数据的顺序和你输⼊的顺序是⼀致的。
找了⼀张尾插法的图,来看⼀下具体是怎么实现的吧可以看到,每次新加⼊的结点都是插⼊在了最后⼀个节点后⾯,所以叫尾插法,同时将其next置空。
同样地,尾插法创建链表也分为两种情况。
⼀种是已知节点个数,还有⼀种是未知节点个数。
下⾯⽤代码来展⽰⼀下先说⼀下已知结点个数的情况,看代码1 #include<stdio.h>2 #include<stdlib.h>3#define N 10 //结点个数4 typedef struct LNode5 {6int data;7struct LNode *next;8 }LNode,*LinkList;91011 LinkList creat_Linklist() //已知节点个数,并且不带头结点12 {13 LNode *head=NULL,*s,*rear=NULL; //尾插法需要⽤到尾指针,rear是尾指针14for(int i=1;i<=N;i++)15 {16 s=(LinkList)malloc(sizeof( LNode));17 scanf("%d",&s->data);18 s->next=NULL; //把当前结点next置空NULL19if(i==1) //⽤来标记插⼊的节点是否为第⼀个节点,是的话就让头结点head指向第⼀个节点20 head=s;21else22 rear->next=s; //不是头结点的话就让上⼀个节点的next指向当前结点23 rear=s; //尾指针rear指向当前节点24 }25return head; //返回头指针26 }27int main()28 {29 LinkList p;30 p=creat_Linklist(); //没有头结点,p指向表中的第⼀个节点31while(p!=NULL)32 {33 printf("%d ",p->data);34 p=p->next ;35 }36 printf("\n");37return0;38 }结果如下:再说⼀下未知结点个数的情况,看代码1 #include<stdio.h>2 #include<stdlib.h>3 typedef struct LNode4 {5int data;6struct LNode *next;7 }LNode,*LinkList;89 LinkList create_Linklist( ) //未知结点个数,并且带头结点10 {11 LNode *head=NULL,*s,*rear=NULL; //尾插法需要⽤到尾指针,rear是尾指针12int e;char ch;13 head=(LinkList)malloc(sizeof( LNode));14 head->next=NULL;15 rear=head;do{scanf("%d",&e);19 s=(LinkList)malloc(sizeof( LNode));20 s->data=e;21 s->next=NULL; //把当前结点next置空NULL22 rear->next=s; //s操作上⼀个结点,让上⼀个节点的next指向当前结点23 rear=s; //尾指针指向当前结点}while((ch=getchar())!='\n');26return head; //返回头指针27 }28int main()29 {30 LinkList p;31 p=create_Linklist( );32 p=p->next; //有头结点,让p跳过头结点,指向表中的第⼀个节点33while(p!=NULL)34 {35 printf("%d ",p->data);36 p=p->next ;37 }38 printf("\n");39return0;40 }结果如下:其实尾插法创建链表的时候,头结点可有可⽆,根据需要来选择。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
#include "stdio.h"#include "stdlib.h"typedef struct List{int data;struct List *next; //指针域}List;void HeadCreatList (List *L) //头插法建立链表{List *s;L->next=NULL;for (int i=0;i<10;i++){s=(struct List*)malloc(sizeof(struct List));s->data=i;s->next=L->next; //将L指向的地址赋值给S;L->next=s;}}void TailCreatList(List *L) //尾插法建立链表{List *s,*r;r=L;for (int i=0;i<10;i++){s=(struct List*)malloc(sizeof(struct List));s->data=i;r->next=s;r=s;}r->next=NULL;}void DisPlay(List *L){List *p=L->next;while(p!=NULL){printf ("%d ",p->data);p=p->next;}printf("\n");}int main (){List *L1,*L2;L1=(struct List*)malloc(sizeof(struct List));L2=(struct List*)malloc(sizeof(struct List)); HeadCreatList(L1);DisPlay(L1);TailCreatList(L2);DisPlay(L2);}//头插法创建链表#include <stdio.h>#include <stdlib.h>struct node{int data;struct node * next;};//建立只含头结点的空链表struct node * create_list(){struct node * head = NULL;head = (struct node *)malloc(sizeof(struct node));if (NULL == head){printf("memory out of use/n");return NULL;}head->next = NULL;head->data = 0;return head;}//头插法建立链表int insert_form_head(struct node * head, int num){struct node * head_t = head->next;struct node * new_node = NULL;new_node = (struct node *)malloc(sizeof(struct node));if (NULL == new_node){printf("memory out of use/n");return -1;}//将新结点插入到链表的最后new_node->data = num;new_node->next = head_t;head->next = new_node;return 0;}//打印链表int show_list(struct node * head){struct node * temp;temp = head->next;while(temp){printf("%d/n",temp->data);temp = temp->next;}return 0;}// 按值删除结点,头结点不被删除int delete_node(struct node *head, int data) {//head_t 保存要删除结点的上一个结点struct node * head_t = head;struct node * temp = NULL;if (head == NULL){printf("delete node from empty list!/n");return -1;}//查找删除的结点的前一个结点//如果此处查找的是删除的结点,则需要另加一个指针保存删除结点的前一个指针while(NULL != head_t->next){if (data == head_t->next->data)break;head_t = head_t->next;}//如果要删除的结点不存在,直接返回if (NULL==head_t->next){printf("node not found/n");return -1;}//删除操作temp = head_t->next;head_t->next = head_t->next->next;free(temp);.return 0;}void main(int argc, char* argv[]){struct node * head;head = create_list();if (NULL == head)printf("create_list error/n");insert_form_head(head,123);insert_form_head(head,456);show_list(head);printf("delete once!/n");delete_node(head,123);show_list(head);printf("delete second!/n");delete_node(head,456);show_list(head);delete_node(head,0);show_list(head);}/*//尾插法创建链表#include<stdio.h>#include<stdlib.h>struct Node{int data;struct Node * next;};//建立只含头结点的空链表struct Node * create_list(){struct Node * head = NULL;head = (struct Node *)malloc(sizeof(struct Node));if (NULL == head){printf("memory out of use/n");return NULL;}head->next = NULL;head->data = 0;return head;}//尾插法建立链表int insert_form_tail(struct Node * head, int num){struct Node * temp = head;struct Node * new_node = NULL;new_node = (struct Node *)malloc(sizeof(struct Node));if (NULL == new_node){printf("memory out of use/n");return -1;}//寻找尾结点while (temp->next != NULL){temp = temp->next;}//将新结点插入到链表的最后new_node->data = num;new_node->next = NULL;temp->next = new_node;.return 0;}//打印链表int show_list(struct Node * head){struct Node * temp;temp = head->next;while(temp){printf("%d/n",temp->data);temp = temp->next;}return 0;}void main(int argc, char* argv[]){struct Node * head;head = create_list();if (NULL == head)printf("create_list error/n");insert_form_tail(head,123);insert_form_tail(head,456);.show_list(head);}*/可编辑。