SpringMVC单元测试之MockMVC 模拟登入用户
详解SpringMVC如何测试Controller(使用springmvcmock测试)

详解SpringMVC如何测试Controller(使⽤springmvcmock测试)在springmvc中⼀般的测试⽤例都是测试service层,今天我来演⽰下如何使⽤springmvc mock直接测试controller层代码。
1.什么是mock测试?mock测试就是在测试过程中,对于某些不容易构造或者不容易获取的对象,⽤⼀个虚拟的对象来创建以便测试的测试⽅法。
2.为什么要使⽤mock测试?使⽤Mock O bject进⾏测试,主要是⽤来模拟那些在应⽤中不容易构造(如HttpServletRequest必须在Servlet容器中才能构造出来)或者⽐较复杂的对象(如JDBC中的ResultSet对象)从⽽使测试顺利进⾏的⼯具。
3.常⽤注解RunWith(SpringJUnit4ClassRunner.class): 表⽰使⽤Spring Test组件进⾏单元测试;WebAppConfiguratio: 使⽤这个annotation会在跑单元测试的时候真实的启⼀个web服务,然后开始调⽤Controller的Rest API,待单元测试跑完之后再将web服务停掉;ContextConfiguration: 指定Bean的配置⽂件信息,可以有多种⽅式,这个例⼦使⽤的是⽂件路径形式,如果有多个配置⽂件,可以将括号中的信息配置为⼀个字符串数组来表⽰;4.安装测试环境spring mvc测试框架提供了两种⽅式,独⽴安装和集成Web环境测试(此种⽅式并不会集成真正的web环境,⽽是通过相应的Mock API进⾏模拟测试,⽆须启动服务器)。
独⽴安装测试⽅式MockMvcBuilders.standaloneSetup(Object... controllers):通过参数指定⼀组控制器,这样就不需要从上下⽂获取了;主要是两个步骤:(1)⾸先⾃⼰创建相应的控制器,注⼊相应的依赖(2)通过MockMvcBuilders.standaloneSetup模拟⼀个Mvc测试环境,通过build得到⼀个MockMvc代码如下:package com.xfs.test;import org.junit.Assert;import org.junit.Before;import org.junit.Test;import org.springframework.test.web.servlet.MockMvc;import org.springframework.test.web.servlet.MvcResult;import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;import org.springframework.test.web.servlet.result.MockMvcResultHandlers;import org.springframework.test.web.servlet.result.MockMvcResultMatchers;import org.springframework.test.web.servlet.setup.MockMvcBuilders;import com.alibaba.fastjson.JSON;import com.alibaba.fastjson.JSONObject;import com.xfs.web.controller.APIController;/*** 独⽴安装测试⽅式 springmvc mock测试** @author admin** 2017年11⽉23⽇上午10:39:49*/public class TestApiOne {private MockMvc mockMvc;@Beforepublic void setUp() {APIController apiController = new APIController();mockMvc = MockMvcBuilders.standaloneSetup(apiController).build();}@Testpublic void testGetSequence() {try {MvcResult mvcResult = mockMvc.perform(MockMvcRequestBuilders.post("/api/getSequence")).andExpect(MockMvcResultMatchers.status().is(200)).andDo(MockMvcResultHandlers.print()).andReturn();int status = mvcResult.getResponse().getStatus();System.out.println("请求状态码:" + status);String result = mvcResult.getResponse().getContentAsString();System.out.println("接⼝返回结果:" + result);JSONObject resultObj = JSON.parseObject(result);// 判断接⼝返回json中success字段是否为trueAssert.assertTrue(resultObj.getBooleanValue("success"));} catch (Exception e) {e.printStackTrace();}}}请求结果如下:集成Web环境⽅式MockMvcBuilders.webAppContextSetup(WebApplicationContext context):指定WebApplicationContext,将会从该上下⽂获取相应的控制器并得到相应的MockMvc;主要是三个步骤:(1)@WebAppConfiguration:测试环境使⽤,⽤来表⽰测试环境使⽤的ApplicationContext将是WebApplicationContext类型的;value指定web应⽤的根(2)通过@Autowired WebApplicationContext wac:注⼊web环境的ApplicationContext容器(3)然后通过MockMvcBuilders.webAppContextSetup(wac).build()创建⼀个MockMvc进⾏测试代码如下:package com.xfs.test;import org.junit.Assert;import org.junit.Before;import org.junit.Test;import org.junit.runner.RunWith;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.mock.web.MockHttpSession;import org.springframework.test.context.ContextConfiguration;import org.springframework.test.context.junit4.AbstractJUnit4SpringContextTests;import org.springframework.test.context.web.WebAppConfiguration;import org.springframework.test.web.servlet.MockMvc;import org.springframework.test.web.servlet.MvcResult;import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;import org.springframework.test.web.servlet.result.MockMvcResultHandlers;import org.springframework.test.web.servlet.result.MockMvcResultMatchers;import org.springframework.test.web.servlet.setup.MockMvcBuilders;import org.springframework.web.context.WebApplicationContext;import com.alibaba.fastjson.JSON;import com.alibaba.fastjson.JSONObject;/*** 集成Web环境⽅式 springmvc mock测试** @author admin** 2017年11⽉23⽇上午11:12:43*/@RunWith(JUnit4ClassRunner.class)@WebAppConfiguration@ContextConfiguration(locations = { "classpath*:spring/*.xml" })public class TestApiTwo extends AbstractJUnit4SpringContextTests {@Autowiredpublic WebApplicationContext wac;public MockMvc mockMvc;public MockHttpSession session;@Beforepublic void before() throws Exception {mockMvc = MockMvcBuilders.webAppContextSetup(wac).build();}@Testpublic void testGetSequence() {try {MvcResult mvcResult = mockMvc.perform(MockMvcRequestBuilders.post("/api/getSequence")).andExpect(MockMvcResultMatchers.status().is(200)).andDo(MockMvcResultHandlers.print()).andReturn();int status = mvcResult.getResponse().getStatus();System.out.println("请求状态码:" + status);String result = mvcResult.getResponse().getContentAsString();System.out.println("接⼝返回结果:" + result);JSONObject resultObj = JSON.parseObject(result);// 判断接⼝返回json中success字段是否为trueAssert.assertTrue(resultObj.getBooleanValue("success"));} catch (Exception e) {e.printStackTrace();}}}运⾏结果和上⾯独⽴测试时候⼀样。
MockMvc模拟对controller进行单元测试
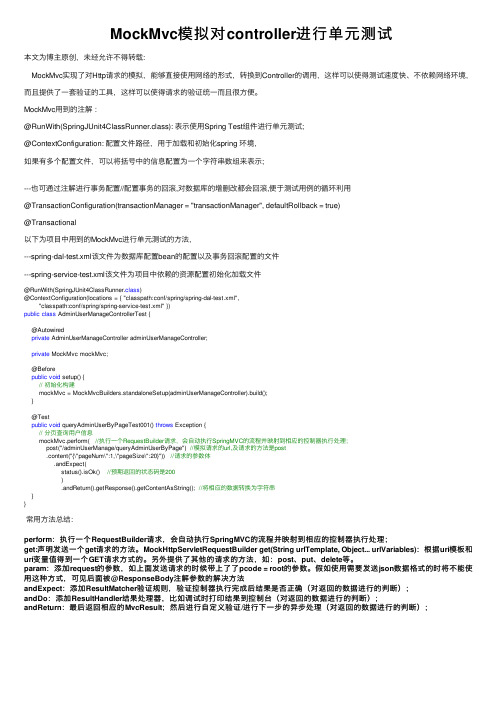
MockMvc模拟对controller进⾏单元测试本⽂为博主原创,未经允许不得转载: MockMvc实现了对Http请求的模拟,能够直接使⽤⽹络的形式,转换到Controller的调⽤,这样可以使得测试速度快、不依赖⽹络环境,⽽且提供了⼀套验证的⼯具,这样可以使得请求的验证统⼀⽽且很⽅便。
MockMvc⽤到的注解:@RunWith(SpringJUnit4ClassRunner.class): 表⽰使⽤Spring Test组件进⾏单元测试;@ContextConfiguration: 配置⽂件路径,⽤于加载和初始化spring 环境,如果有多个配置⽂件,可以将括号中的信息配置为⼀个字符串数组来表⽰;---也可通过注解进⾏事务配置//配置事务的回滚,对数据库的增删改都会回滚,便于测试⽤例的循环利⽤@TransactionConfiguration(transactionManager = "transactionManager", defaultRollback = true)@Transactional以下为项⽬中⽤到的MockMvc进⾏单元测试的⽅法,---spring-dal-test.xml该⽂件为数据库配置bean的配置以及事务回滚配置的⽂件---spring-service-test.xml该⽂件为项⽬中依赖的资源配置初始化加载⽂件@RunWith(SpringJUnit4ClassRunner.class)@ContextConfiguration(locations = { "classpath:conf/spring/spring-dal-test.xml","classpath:conf/spring/spring-service-test.xml" })public class AdminUserManageControllerTest {@Autowiredprivate AdminUserManageController adminUserManageController;private MockMvc mockMvc;@Beforepublic void setup() {// 初始化构建mockMvc = MockMvcBuilders.standaloneSetup(adminUserManageController).build();}@Testpublic void queryAdminUserByPageTest001() throws Exception {// 分页查询⽤户信息mockMvc.perform( //执⾏⼀个RequestBuilder请求,会⾃动执⾏SpringMVC的流程并映射到相应的控制器执⾏处理;post("/adminUserManage/queryAdminUserByPage") //模拟请求的url,及请求的⽅法是post.content("{\"pageNum\":1,\"pageSize\":20}")) //请求的参数体.andExpect(status().isOk() //预期返回的状态码是200).andReturn().getResponse().getContentAsString(); //将相应的数据转换为字符串}}常⽤⽅法总结:perform:执⾏⼀个RequestBuilder请求,会⾃动执⾏SpringMVC的流程并映射到相应的控制器执⾏处理;get:声明发送⼀个get请求的⽅法。
junit4单元测试--web项目中模拟登录会话,做全流程测试

junit4单元测试--web项⽬中模拟登录会话,做全流程测试junit4相对于junit3,基于注解的⽅式写单元测试⽤例,使⽤过程中⽅便很多。
如下缩写均是代码⽚段,摘录其中关键部分,重要是理解其中知识点。
⼀、编写测试⽤例基类@RunWith(SpringJUnit4ClassRunner.class)@WebAppConfiguration@ContextConfiguration({"file:src/main/webapp/WEB-INF/applicationContext.xml", "file:src/main/webapp/WEB-INF/spring-servlet.xml","file:src/main/webapp/WEB-INF/conf/spring-redis.xml", "file:src/main/webapp/WEB-INF/conf/spring-resttemplate.xml"})public abstract class BaseJunit{/*** wac*/@Autowiredprivate WebApplicationContext wac;/*** MockMvc*/private MockMvc mockMvc;protected WebApplicationContext getWac(){return this.wac;}protected MockMvc getMockMvc(){return this.mockMvc;}/*** 初始化mocMvc** @see*/@Beforepublic void setUp(){this.mockMvc = webAppContextSetup(this.wac).build();}......}@RunWith(SpringJUnit4ClassRunner.class) 指定采⽤Spring的运⾏环境@WebAppConfiguration ⽤来声明这是⼀个web测试环境@ContextConfiguration ⽤来指定加载项⽬的配置⽂件⼆、抽出web系统登录⽅法public abstract class BaseLoginJunit extends BaseJunit{/*** MockMvc*/private MockHttpSession session;protected MockHttpSession getSession(){return session;}/*** 测试前,初始化系统登录** @see*/@Beforepublic void setUp(){super.setUp();this.session = (MockHttpSession)getLoginSession();}/*** 完成登录功能,返回当前登录会话** @return HttpSession* @see*/private HttpSession getLoginSession(){String url = "/xxx/login";String params = "{\"userName\":\"xxx\",\"password\":\"xxx\",\"verifyCode\":\"xxx\"}";MvcResult result = null;try{result = getMockMvc().perform(MockMvcRequestBuilders.post(url).accept(MediaType.APPLICATION_JSON).contentType(MediaType.APPLICATION_JSON_UTF8_VALUE).content( params)).andExpect(MockMvcResultMatchers.status().isOk()).andDo(MockMvcResultHandlers.print()).andReturn();}catch (Exception e){e.printStackTrace();}return result.getRequest().getSession();}......}三、编写spring控制器测试⽅法@FixMethodOrder(_ASCENDING) // 指定按字母顺序执⾏测试⽤例public class ResourceControllerTest extends BaseLoginJunit{/*** res id list*/private static List<String> RES_LIST = new ArrayList<>();/*** 测试 getResource** @see*/@Testpublic void testGetResource(){String url = "/xxx/get";MultiValueMap<String, String> map = new LinkedMultiValueMap<>();this.setPage(map);get(url, map);}/*** 测试 add** @see*/@Testpublic void testAddResource(){String url = "/xxx/add";ResourceBean bean = new ResourceBean();ResourceBean anotherBean = new ResourceBean();bean.setResName("test res1");anotherBean.setResName("test res2");MvcResult result = post(url, JSONObject.toJSONString(bean));ReturnVal returnVal = this.getReturnVal(result);RES_LIST.add(returnVal.getData().getId());MvcResult anotherResult = post(url, JSONObject.toJSONString(childBean));ReturnVal anotherReturnVal = this.getReturnVal(anotherResult);RES_LIST.add(anotherReturnVal.getData().getId());}/*** 测试updateResource** @see*/@Testpublic void testBupdateResource(){String url = "/xxx/update";ResourceBean bean = new ResourceBean();bean.setId(RES_LIST.get(0));bean.setResName("test res1");MvcResult result = post(url, JSONObject.toJSONString(bean));assertEquals(AbstractController.STATUS_SUCCESS, getReturnVal(result).getStatus());}/*** 测试delResource** @see*/@Testpublic void testCdelResource(){String url = "/xxx/delete";MultiValueMap<String, String> map = new LinkedMultiValueMap<>();map.add("id", RES_LIST.remove(0));MvcResult result = get(url, map);assertEquals(AbstractController.STATUS_SUCCESS, getReturnVal(result).getStatus());}/*** 测试batchDelResource** @see*/@Testpublic void testDbatchDelResource(){String url = "/xxx/batchDel";MultiValueMap<String, String> map = new LinkedMultiValueMap<>();StringBuilder params = new StringBuilder();for (int i = 0; i < RES_LIST.size(); i++ ){if (i == RES_LIST.size() - 1){params.append(RES_LIST.get(i));}else{params.append(RES_LIST.get(i)).append(",");}}map.add("id", params.toString());MvcResult result = get(url, map);assertEquals(AbstractController.STATUS_SUCCESS, getReturnVal(result).getStatus());}}以上测试⽤例中,@FixMethodOrder很关键,因为增、删、改、查需要指定测试的顺序。
springmvc之单元测试(MockMvc)-独立测试

springmvc之单元测试(MockMvc)-独⽴测试spring mvc测试框架提供了两种⽅式,独⽴安装和集成Web环境测试(此种⽅式并不会集成真正的web环境,⽽是通过相应的Mock API进⾏模拟测试,⽆须启动服务器)1、mockMvc.perform执⾏⼀个请求;2、MockMvcRequestBuilders.get("/user/1")构造⼀个请求3、ResultActions.andExpect添加执⾏完成后的断⾔4、ResultActions.andDo添加⼀个结果处理器,表⽰要对结果做点什么事情,⽐如此处使⽤MockMvcResultHandlers.print()输出整个响应结果信息。
5、ResultActions.andReturn表⽰执⾏完成后返回相应的结果。
MockMvcBuilder是⽤来构造MockMvc的构造器,其主要有两个实现:StandaloneMockMvcBuilder和DefaultMockMvcBuilder,StandaloneMockMvcBuilder继承了DefaultMockMvcBuilder。
直接使⽤静态⼯⼚MockMvcBuilders创建即可:MockMvcBuilders.webAppContextSetup(WebApplicationContext context):指定WebApplicationContext,将会从该上下⽂获取相应的控制器并得到相应的MockMvc;MockMvcBuilders.standaloneSetup(Object... controllers):通过参数指定⼀组控制器,这样就不需要从上下⽂获取了;其中DefaultMockMvcBuilder还提供了如下API:addFilters(Filter... filters)/addFilter(Filter filter, String... urlPatterns):添加javax.servlet.Filter过滤器defaultRequest(RequestBuilder requestBuilder):默认的RequestBuilder,每次执⾏时会合并到⾃定义的RequestBuilder中,即提供公共请求数据的;alwaysExpect(ResultMatcher resultMatcher):定义全局的结果验证器,即每次执⾏请求时都进⾏验证的规则;alwaysDo(ResultHandler resultHandler):定义全局结果处理器,即每次请求时都进⾏结果处理;dispatchOptions:DispatcherServlet是否分发OPTIONS请求⽅法到控制器;StandaloneMockMvcBuilder继承了DefaultMockMvcBuilder,⼜提供了如下API:setMessageConverters(HttpMessageConverter<?>...messageConverters):设置HTTP消息转换器;setValidator(Validator validator):设置验证器;setConversionService(FormattingConversionService conversionService):设置转换服务;addInterceptors(HandlerInterceptor... interceptors)/addMappedInterceptors(String[] pathPatterns, HandlerInterceptor... interceptors):添加spring mvc拦截器;setContentNegotiationManager(ContentNegotiationManager contentNegotiationManager):设置内容协商管理器;setAsyncRequestTimeout(long timeout):设置异步超时时间;setCustomArgumentResolvers(HandlerMethodArgumentResolver... argumentResolvers):设置⾃定义控制器⽅法参数解析器;setCustomReturnValueHandlers(HandlerMethodReturnValueHandler... handlers):设置⾃定义控制器⽅法返回值处理器;setHandlerExceptionResolvers(List<HandlerExceptionResolver>exceptionResolvers)/setHandlerExceptionResolvers(HandlerExceptionResolver... exceptionResolvers):设置异常解析器;setViewResolvers(ViewResolver...resolvers):设置视图解析器;setSingleView(View view):设置单个视图,即视图解析时总是解析到这⼀个(仅适⽤于只有⼀个视图的情况);setLocaleResolver(LocaleResolver localeResolver):设置Local解析器;setFlashMapManager(FlashMapManager flashMapManager):设置FlashMapManager,如存储重定向数据;setUseSuffixPatternMatch(boolean useSuffixPatternMatch):设置是否是后缀模式匹配,如“/user”是否匹配"/user.*",默认真即匹配;setUseTrailingSlashPatternMatch(boolean useTrailingSlashPatternMatch):设置是否⾃动后缀路径模式匹配,如“/user”是否匹配“/user/”,默认真即匹配;addPlaceHolderValue(String name, String value) :添加request mapping中的占位符替代;因为StandaloneMockMvcBuilder不会加载Spring MVC配置⽂件,因此就不会注册我们需要的⼀些组件,因此就提供了如上API⽤于注册我们需要的相应组件。
mockmvc 用法
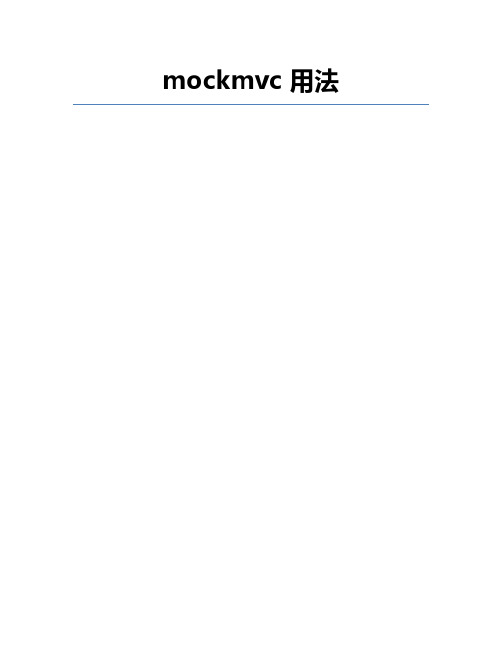
mockmvc 用法1. MockMVC简介MockMVC是一个模拟Spring MVC环境的测试框架,它可以模拟发出http请求,接收http响应,并对http请求和响应进行断言,从而实现对Spring MVC控制器的组件化测试。
MockMVC可以模拟Spring MVC控制器的行为,而不需要启动一个完整的Web服务器,从而提高了测试效率和准确性。
2. MockMVC的安装与配置MockMVC需要在Spring Boot项目中引入Spring Test模块,可以在pom.xml中添加以下依赖:```<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-test</artifactId><scope>test</scope></dependency>```MockMVC的配置需要使用@RunWith注解,在测试类中添加以下代码:```@RunWith(SpringRunner.class)@WebMvcTest```此外,还需要在测试类中注入MockMVC对象,可以使用@Autowired注解:```@Autowiredprivate MockMvc mockMvc;```### 3. MockMVC的基本使用MockMVC的基本使用包括创建MockMVC实例、配置请求参数、发送请求、验证响应结果等几个步骤。
1. 创建MockMVC实例:可以使用MockMvcBuilders类的standaloneSetup()方法创建MockMVC实例,传入要测试的Controller实例即可。
2. 配置请求参数:使用MockMvc实例的perform()方法,传入一个RequestBuilder实例,可以配置请求的参数,如请求方法、请求参数等。
mockmvcshirorequirerole注解
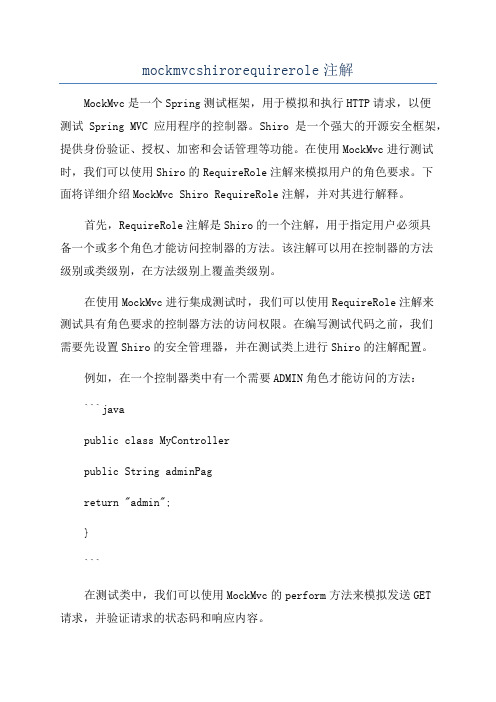
mockmvcshirorequirerole注解MockMvc是一个Spring测试框架,用于模拟和执行HTTP请求,以便测试Spring MVC应用程序的控制器。
Shiro是一个强大的开源安全框架,提供身份验证、授权、加密和会话管理等功能。
在使用MockMvc进行测试时,我们可以使用Shiro的RequireRole注解来模拟用户的角色要求。
下面将详细介绍MockMvc Shiro RequireRole注解,并对其进行解释。
首先,RequireRole注解是Shiro的一个注解,用于指定用户必须具备一个或多个角色才能访问控制器的方法。
该注解可以用在控制器的方法级别或类级别,在方法级别上覆盖类级别。
在使用MockMvc进行集成测试时,我们可以使用RequireRole注解来测试具有角色要求的控制器方法的访问权限。
在编写测试代码之前,我们需要先设置Shiro的安全管理器,并在测试类上进行Shiro的注解配置。
例如,在一个控制器类中有一个需要ADMIN角色才能访问的方法:```javapublic class MyControllerpublic String adminPagreturn "admin";}```在测试类中,我们可以使用MockMvc的perform方法来模拟发送GET请求,并验证请求的状态码和响应内容。
```javapublic class MyControllerTestprivate MockMvc mockMvc;public void testAdminPageAccess( throws ExceptionmockMvc.perform(get("/api/admin")).andExpect(status(.isOk().andExpect(content(.string("admin"));}```需要注意的是,RequireRole注解只是Shiro在控制器层面的角色授权机制之一、在实际应用中,我们可能还需要进行其他身份验证和权限控制。
Springboot单元测试简单介绍和启动所有测试类的方法
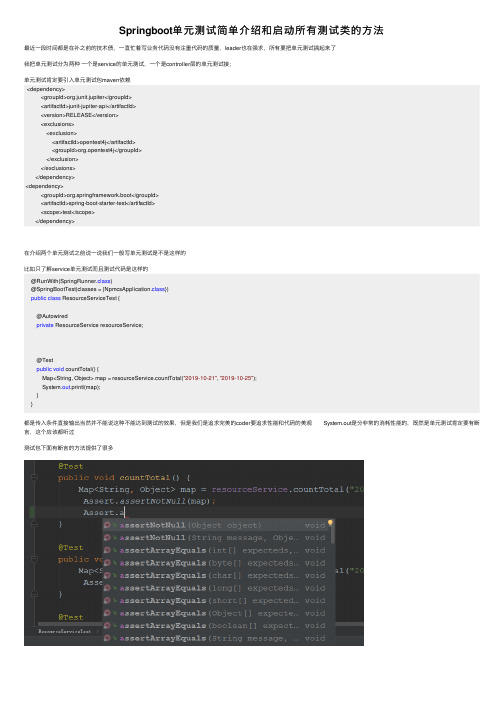
Springboot单元测试简单介绍和启动所有测试类的⽅法最近⼀段时间都是在补之前的技术债,⼀直忙着写业务代码没有注重代码的质量,leader也在强求,所有要把单元测试搞起来了我把单元测试分为两种⼀个是service的单元测试,⼀个是controller层的单元测试接;单元测试肯定要引⼊单元测试包maven依赖<dependency><groupId>org.junit.jupiter</groupId><artifactId>junit-jupiter-api</artifactId><version>RELEASE</version><exclusions><exclusion><artifactId>opentest4j</artifactId><groupId>org.opentest4j</groupId></exclusion></exclusions></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-test</artifactId><scope>test</scope></dependency>在介绍两个单元测试之前说⼀说我们⼀般写单元测试是不是这样的⽐如只了解service单元测试⽽且测试代码是这样的@RunWith(SpringRunner.class)@SpringBootTest(classes = {NpmcsApplication.class})public class ResourceServiceTest {@Autowiredprivate ResourceService resourceService;@Testpublic void countTotal() {Map<String, Object> map = resourceService.countTotal("2019-10-21", "2019-10-25");System.out.printl(map);}}都是传⼊条件直接输出当然并不能说这种不能达到测试的效果,但是我们是追求完美的coder要追求性能和代码的美观System.out是分⾮常的消耗性能的,既然是单元测试肯定要有断⾔,这个应该都听过测试包下⾯有断⾔的⽅法提供了很多这⾥有很多的断⾔⽅法⽐如上⾯的代代码可以修改为@RunWith(SpringRunner.class)@SpringBootTest(classes = {NpmcsApplication.class})public class ResourceServiceTest {@Autowiredprivate ResourceService resourceService;@Testpublic void countTotal() {Map<String, Object> map = resourceService.countTotal("2019-10-21", "2019-10-25");Assert.assertNotNull(map);}}上⾯代码重点是, 测试类加@RunWith注解, 还有加上 @SpringBootTest(classes = App.class) 注解, 这⾥的 App.class 是主程序java类. 主程序java程序必须是SpringBootApplication程序,否则测试⽤例会报如下错误:Unable to find a @SpringBootConfiguration, you need to use @ContextConfiguration or @SpringBootTest(classes=...) with your test ng.IllegalStateException.@RunWith是JUnit的⼀个注解, ⽤来告诉JUnit不要使⽤内置的⽅式进⾏单元测试, ⽽应该使⽤指定的类做单元测试对于Spring单元测试总是要使⽤ SpringRunner.class .@SpringBootTest ⽤来指定SpringBoot应⽤程序的⼊⼝类, 该注解默认会根据包名逐级往上找, ⼀直找到⼀个SpringBoot主程序class为⽌, 然后启动该类为单元测试准备Spring上下⽂环境. Spring单元测试并不在每个测试⽅法前都移动⼀个全新的Spring上下⽂, 因为这样做太耗费时间, ⽽是会缓存上下⽂环境. 如果某个测试⽅法需要重新准备Spring上下⽂, 需要在该⽅法上加@DirtiesContext 注解.@Test注解: JUnit在执⾏每个测试⽅法之前, 都会为测试类创建⼀个新的实例, 这样有助于隔离各个测试⽅法之前的相互影响.接下来说⼀下controller测试这个有点复杂⽤到的MockMvc,针对API测试的⼀个库话不多说直接刚代码@RunWith(SpringJUnit4ClassRunner.class)@SpringBootTestpublic class PressureViewContorllerTest {@Autowiredprivate WebApplicationContext context;private MockMvc mockMvc;private MockHttpSession session;@Beforepublic void before() throws Exception {mockMvc = MockMvcBuilders.webAppContextSetup(this.context).build();session = new MockHttpSession();User user = new User();user.setName("⼩明");session.setAttribute("user", user);}@Afterpublic void after() throws Exception {}/*** Method: showPressure()*/@Testpublic void testShowPressure() throws Exception {String json = "{\n" + "\t\"startDate\": \"2019-09-14\",\n" + "\t\"endDate\": \"2019-10-14\"\n" + "}";MultiValueMap<String, String> params = new LinkedMultiValueMap<String, String>();params.add("startDate", "2019-09-14");params.add("endDate", "2019-10-14");RequestBuilder request =MockMvcRequestBuilders.post("/pressure/pressureView").contentType(MediaType.APPLICATION_JSON).params(params);MvcResult mvcResult = mockMvc.perform(request).andExpect(MockMvcResultMatchers.status().isOk()).andDo(MockMvcResultHandlers.print()).andReturn();int status = mvcResult.getResponse().getStatus();String content = mvcResult.getResponse().getContentAsString();Assert.assertTrue("正确", status == 200);Assert.assertFalse("错误", status != 200);}/*** Method: pressureList()*/@Testpublic void testPressureList() throws Exception {MultiValueMap<String, String> params = new LinkedMultiValueMap<String, String>();params.add("startDate", "2019-09-14");params.add("endDate", "2019-10-14");RequestBuilder request =MockMvcRequestBuilders.post("/pressure/list").contentType(MediaType.APPLICATION_JSON_UTF8).params(params);MvcResult mvcResult = mockMvc.perform(request).andExpect(MockMvcResultMatchers.status().isOk()).andDo(MockMvcResultHandlers.print()).andReturn();int status = mvcResult.getResponse().getStatus();String content = mvcResult.getResponse().getContentAsString();Assert.assertTrue("正确", status == 200);Assert.assertFalse("错误", status != 200);}}代代码讲解:⾸先要注⼊MockMvc ,MockHttpSession ,WebApplicationContext 初始化这些对象@Before这个⽅法是在每个Test⽅法之前执⾏,模拟登录因为登录拦截检测是否有session信息mockMvc.perform()模仿页⾯调⽤接⼝ MockMvcRequestBuilders.post("/pressure/list") 这个是掉POST⽅法 GET直接.get(url)MultiValueMap<String, String> params = new LinkedMultiValueMap<String, String>();params.add("startDate", "2019-09-14");params.add("endDate", "2019-10-14");这个是模拟参数使⽤的是 MockMvcRequestBuilders.post("/pressure/list").contentType(MediaType.APPLICATION_JSON_UTF8) .params(params);也可以使⽤mvc.perform(MockMvcRequestBuilders.get("/manPower/countManPowerByTeam").param("startDate","2019-01-01").param("endDate","2019-12-31")).andExpect(MockMvcResultMatche 这段代码:mockMvc.perform(request).andExpect(MockMvcResultMatchers.status().isOk()).andDo(MockMvcResultHandlers.print()).andReturn();期望返回status是ok 并且打印放回的结果最后附上启动所有的测试类⽅法使⽤的是TestSuite/*** 启动所有的测试类* @Author zly* @Date 2018/11/2 09:54*/@RunWith(Suite.class)@Suite.SuiteClasses({TeamServiceTest.class,PressureViewContorllerTest.class,UserControllerTest.class,FlowViewControllerTest.class,HttpConnUtilTest.class, DemandPICControllerTest.class, DemandProtraitServiceTest.class})public class TestSuite {就是把所有的测试类注⼊进来有写的不好之处希望各路⼤佬指教共同成长。
Junit测试Controller(MockMVC使用),传输@RequestBody数据解决办法
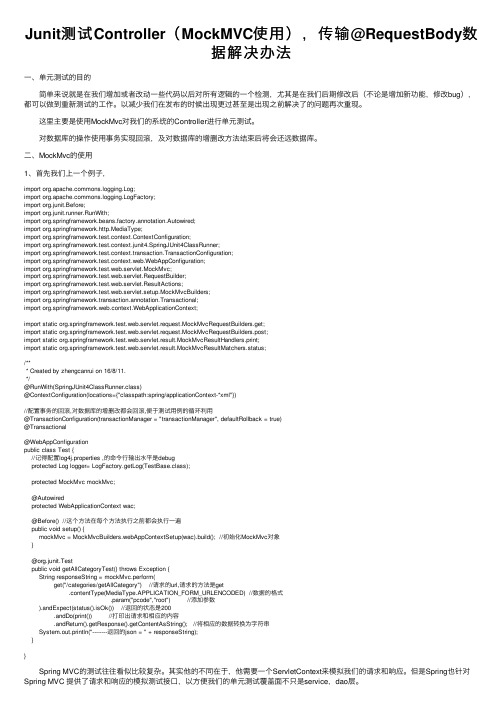
Junit测试Controller(MockMVC使⽤),传输@RequestBody数据解决办法⼀、单元测试的⽬的 简单来说就是在我们增加或者改动⼀些代码以后对所有逻辑的⼀个检测,尤其是在我们后期修改后(不论是增加新功能,修改bug),都可以做到重新测试的⼯作。
以减少我们在发布的时候出现更过甚⾄是出现之前解决了的问题再次重现。
这⾥主要是使⽤MockMvc对我们的系统的Controller进⾏单元测试。
对数据库的操作使⽤事务实现回滚,及对数据库的增删改⽅法结束后将会还远数据库。
⼆、MockMvc的使⽤1、⾸先我们上⼀个例⼦,import mons.logging.Log;import mons.logging.LogFactory;import org.junit.Before;import org.junit.runner.RunWith;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.http.MediaType;import org.springframework.test.context.ContextConfiguration;import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;import org.springframework.test.context.transaction.TransactionConfiguration;import org.springframework.test.context.web.WebAppConfiguration;import org.springframework.test.web.servlet.MockMvc;import org.springframework.test.web.servlet.RequestBuilder;import org.springframework.test.web.servlet.ResultActions;import org.springframework.test.web.servlet.setup.MockMvcBuilders;import org.springframework.transaction.annotation.Transactional;import org.springframework.web.context.WebApplicationContext;import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.post;import static org.springframework.test.web.servlet.result.MockMvcResultHandlers.print;import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;/*** Created by zhengcanrui on 16/8/11.*/@RunWith(SpringJUnit4ClassRunner.class)@ContextConfiguration(locations={"classpath:spring/applicationContext-*xml"})//配置事务的回滚,对数据库的增删改都会回滚,便于测试⽤例的循环利⽤@TransactionConfiguration(transactionManager = "transactionManager", defaultRollback = true)@Transactional@WebAppConfigurationpublic class Test {//记得配置log4j.properties ,的命令⾏输出⽔平是debugprotected Log logger= LogFactory.getLog(TestBase.class);protected MockMvc mockMvc;@Autowiredprotected WebApplicationContext wac;@Before() //这个⽅法在每个⽅法执⾏之前都会执⾏⼀遍public void setup() {mockMvc = MockMvcBuilders.webAppContextSetup(wac).build(); //初始化MockMvc对象}@org.junit.Testpublic void getAllCategoryTest() throws Exception {String responseString = mockMvc.perform(get("/categories/getAllCategory") //请求的url,请求的⽅法是get.contentType(MediaType.APPLICATION_FORM_URLENCODED) //数据的格式 .param("pcode","root") //添加参数).andExpect(status().isOk()) //返回的状态是200.andDo(print()) //打印出请求和相应的内容.andReturn().getResponse().getContentAsString(); //将相应的数据转换为字符串System.out.println("--------返回的json = " + responseString);}} Spring MVC的测试往往看似⽐较复杂。
MVC模式实现用户登录
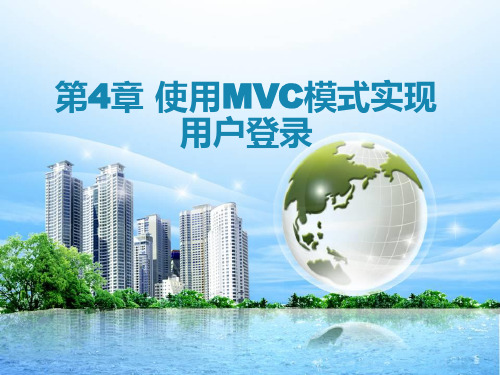
4 视图根据接收到的结果;将信息显示给用户&
13
4.4.2分为三块: com.restaurant.bean包中存放实体类; com.restaurant.dao包中存放数据访问类; com.restaurant.service存放业务逻辑类&
使用Servlet作为控制器 作用是接收用户的请求数据;选择合适的Controller处理具体 业务;处理完成后;根据Model返回的结果选择一个View显示数 据& 在com.restaurant.servlet的包中;新建一个LoginServlet. java 的Servlet文件;用来实现业务逻辑处理&
在 程 序 设 计 中 ; 把 采 用 模 型 Model 、 视 图 View 、 控 制 器 Controller的设计方式称为MVC设计模式&
7
4.2.2 MVC模式的定义及特点
什么是设计模式
设计模式是一套被反复使用、成功的代码设计经验总结&
MVC设计模式
MVC是一种流行的软件设计模式;分为以下3个模块
第4章 使用MVC模式实现 用户登录
目录
1 JSP开发模型 2 MVC模式的概述 3 JDBC技术 4 使用MVC模式实现用户登录模块
mockmvc 使用指南
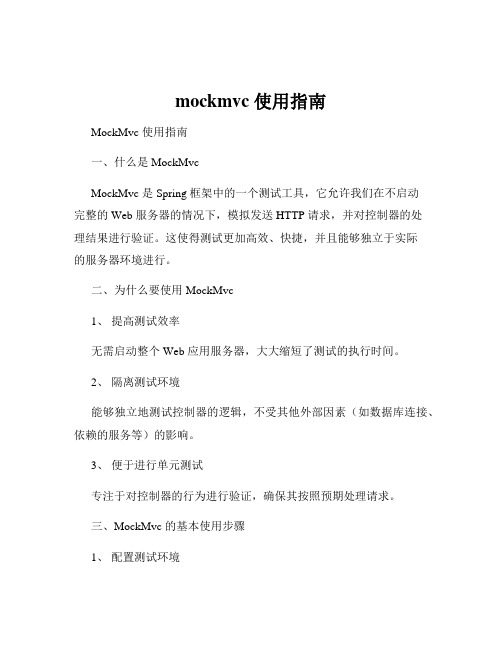
mockmvc 使用指南MockMvc 使用指南一、什么是 MockMvcMockMvc 是 Spring 框架中的一个测试工具,它允许我们在不启动完整的 Web 服务器的情况下,模拟发送 HTTP 请求,并对控制器的处理结果进行验证。
这使得测试更加高效、快捷,并且能够独立于实际的服务器环境进行。
二、为什么要使用 MockMvc1、提高测试效率无需启动整个 Web 应用服务器,大大缩短了测试的执行时间。
2、隔离测试环境能够独立地测试控制器的逻辑,不受其他外部因素(如数据库连接、依赖的服务等)的影响。
3、便于进行单元测试专注于对控制器的行为进行验证,确保其按照预期处理请求。
三、MockMvc 的基本使用步骤1、配置测试环境首先,需要在测试类中配置 Spring 的测试上下文。
这通常通过使用`@SpringBootTest` 或`@WebMvcTest` 等注解来完成。
```java@SpringBootTest@AutoConfigureMockMvcpublic class MyControllerTest {@Autowiredprivate MockMvc mockMvc;//测试方法}```2、构建请求使用`MockMvc` 提供的方法来构建 HTTP 请求。
可以设置请求的方法(GET、POST 等)、请求的路径、请求参数等。
```javaResultActions resultActions =mockMvcperform(MockMvcRequestBuildersget("/api/users"));```3、验证响应通过`andExpect` 方法来验证响应的状态码、响应体的内容等。
```javaresultActionsandExpect(status()isOk())andExpect(content()string("Expected response content"));```四、处理请求参数1、查询参数通过`param` 方法添加查询参数。
mock单元测试mockmvc详解
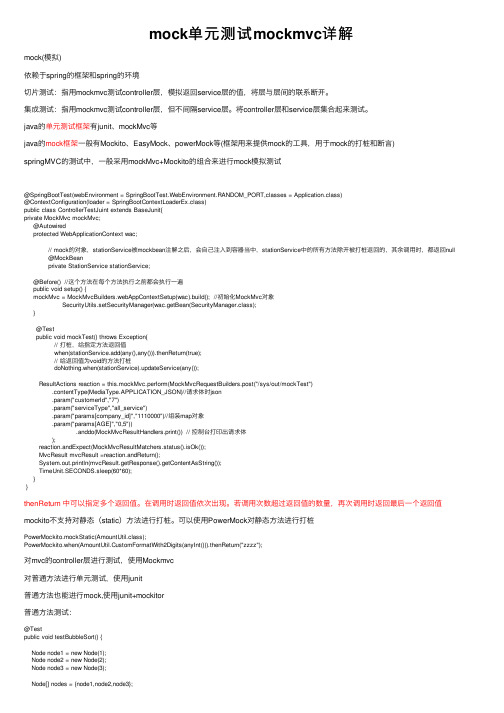
mock单元测试mockmvc详解mock(模拟)依赖于spring的框架和spring的环境切⽚测试:指⽤mockmvc测试controller层,模拟返回service层的值,将层与层间的联系断开。
集成测试:指⽤mockmvc测试controller层,但不间隔service层。
将controller层和service层集合起来测试。
java的单元测试框架有junit、mockMvc等java的mock框架⼀般有Mockito、EasyMock、powerMock等(框架⽤来提供mock的⼯具,⽤于mock的打桩和断⾔)springMVC的测试中,⼀般采⽤mockMvc+Mockito的组合来进⾏mock模拟测试@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT,classes = Application.class)@ContextConfiguration(loader = SpringBootContextLoaderEx.class)public class ControllerTestJuint extends BaseJunit{private MockMvc mockMvc;@Autowiredprotected WebApplicationContext wac; // mock的对象,stationService被mockbean注解之后,会⾃⼰注⼊到容器当中,stationService中的所有⽅法除开被打桩返回的,其余调⽤时,都返回null @MockBean private StationService stationService; @Before() //这个⽅法在每个⽅法执⾏之前都会执⾏⼀遍public void setup() {mockMvc = MockMvcBuilders.webAppContextSetup(wac).build(); //初始化MockMvc对象 SecurityUtils.setSecurityManager(wac.getBean(SecurityManager.class);} @Test public void mockTest() throws Exception{ // 打桩,给指定⽅法返回值 when(stationService.add(any(),any())).thenReturn(true); // 给返回值为void的⽅法打桩 doNothing.when(stationService).updateService(any());ResultActions reaction = this.mockMvc.perform(MockMvcRequestBuilders.post("/sys/out/mockTest").contentType(MediaType.APPLICATION_JSON)//请求体时json.param("customerId","7").param("serviceType","all_service").param("params[company_id]","1110000")//组装map对象.param("params[AGE]","0,5")) .anddo(MockMvcResultHandlers.print()) // 控制台打印出请求体);reaction.andExpect(MockMvcResultMatchers.status().isOk());MvcResult mvcResult =reaction.andReturn();System.out.println(mvcResult.getResponse().getContentAsString());TimeUnit.SECONDS.sleep(60*60);}}thenReturn 中可以指定多个返回值。
springmvc项目单元测试
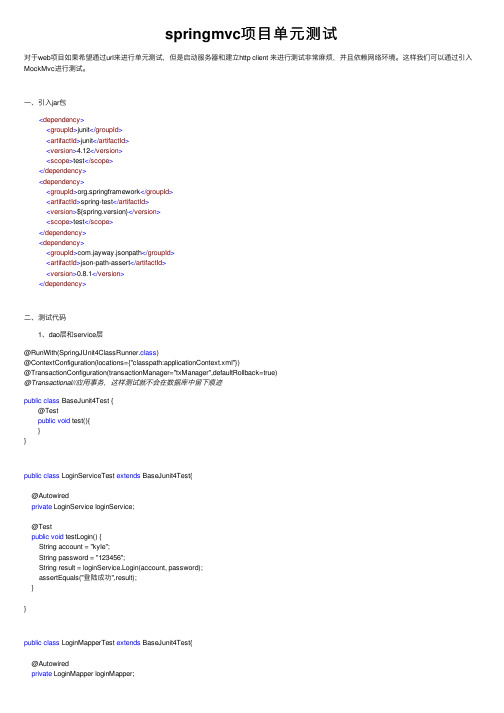
springmvc项⽬单元测试对于web项⽬如果希望通过url来进⾏单元测试,但是启动服务器和建⽴http client 来进⾏测试⾮常⿇烦,并且依赖⽹络环境。
这样我们可以通过引⼊MockMvc进⾏测试。
⼀、引⼊jar包 <dependency><groupId>junit</groupId><artifactId>junit</artifactId><version>4.12</version><scope>test</scope></dependency><dependency><groupId>org.springframework</groupId><artifactId>spring-test</artifactId><version>${spring.version}</version><scope>test</scope></dependency><dependency><groupId>com.jayway.jsonpath</groupId><artifactId>json-path-assert</artifactId><version>0.8.1</version></dependency>⼆、测试代码 1、dao层和service层@RunWith(SpringJUnit4ClassRunner.class)@ContextConfiguration(locations={"classpath:applicationContext.xml"})@TransactionConfiguration(transactionManager="txManager",defaultRollback=true)@Transactional//应⽤事务,这样测试就不会在数据库中留下痕迹public class BaseJunit4Test { @Test public void test(){ }}public class LoginServiceTest extends BaseJunit4Test{@Autowiredprivate LoginService loginService;@Testpublic void testLogin() {String account = "kyle";String password = "123456";String result = loginService.Login(account, password);assertEquals("登陆成功",result);}}public class LoginMapperTest extends BaseJunit4Test{@Autowiredprivate LoginMapper loginMapper;@Testpublic void testGetUserPwdByAccount() {String account = "kyle";String pwd = loginMapper.getUserPwdByAccount(account);assertEquals("123456",pwd);}} 2、web层测试@RunWith(SpringJUnit4ClassRunner.class)//使⽤Spring Test组件进⾏单元测试@ContextConfiguration(locations={"classpath:applicationContext.xml",//加载配置⽂件"classpath:spring-mvc.xml"})@WebAppConfiguration@TransactionConfiguration(transactionManager="txManager",defaultRollback=true) @Transactional//应⽤事务,这样测试就不会在数据库中留下痕迹public class BaseWebJunit4Test {protected MockMvc mockMvc;protected MockHttpSession mockHttpSession;@Autowiredprotected WebApplicationContext context;@Beforepublic void initMockMvc() throws Exception {mockMvc = MockMvcBuilders.webAppContextSetup(context).build();this.mockHttpSession = new MockHttpSession();mockMvc.perform(MockMvcRequestBuilders.post("/login").contentType(MediaType.APPLICATION_FORM_URLENCODED).param("account", "kyle").param("password", "123456").session(mockHttpSession)).andExpect(status().isOk()).andExpect(content().string("登陆成功")).andDo(print()).andReturn().getResponse().getContentAsString();}@Testpublic void test(){}}public class LoginControllerTest extends BaseWebJunit4Test{@Testpublic void testLogin() throws Exception {mockMvc.perform(MockMvcRequestBuilders.post("/login").contentType(MediaType.APPLICATION_FORM_URLENCODED).param("account", "kyle").param("password", "123456").session(mockHttpSession)).andExpect(status().isOk()).andExpect(content().string("登陆成功")).andDo(print()).andReturn().getResponse().getContentAsString();}@Testpublic void testGetUserInfo() throws Exception {mockMvc.perform(MockMvcRequestBuilders.post("/getUserInfo") .contentType(MediaType.APPLICATION_JSON).content("{\"account\":\"kyle\"}").session(mockHttpSession)).andExpect(status().isOk()).andExpect(jsonPath("$.password", is("123456"))).andDo(print()).andReturn().getResponse().getContentAsString();}}三、mock mvc 相关api。
SpringBoot+Junit5+MockMvc写单元测试
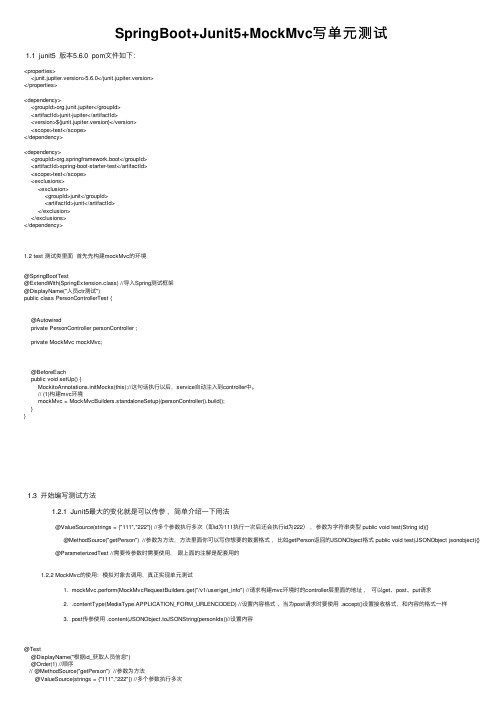
SpringBoot+Junit5+MockMvc写单元测试1.1 junit5 版本5.6.0 pom⽂件如下:<properties><junit.jupiter.version>5.6.0</junit.jupiter.version></properties><dependency><groupId>org.junit.jupiter</groupId><artifactId>junit-jupiter</artifactId><version>${junit.jupiter.version}</version><scope>test</scope></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-test</artifactId><scope>test</scope><exclusions><exclusion><groupId>junit</groupId><artifactId>junit</artifactId></exclusion></exclusions></dependency>1.2 test 测试类⾥⾯⾸先先构建mockMvc的环境@SpringBootTest@ExtendWith(SpringExtension.class) //导⼊Spring测试框架@DisplayName("⼈员ctr测试")public class PersonControllerTest {@Autowiredprivate PersonController personController ;private MockMvc mockMvc;@BeforeEachpublic void setUp() {MockitoAnnotations.initMocks(this);//这句话执⾏以后,service⾃动注⼊到controller中。
mockmvcshirorequirerole注解
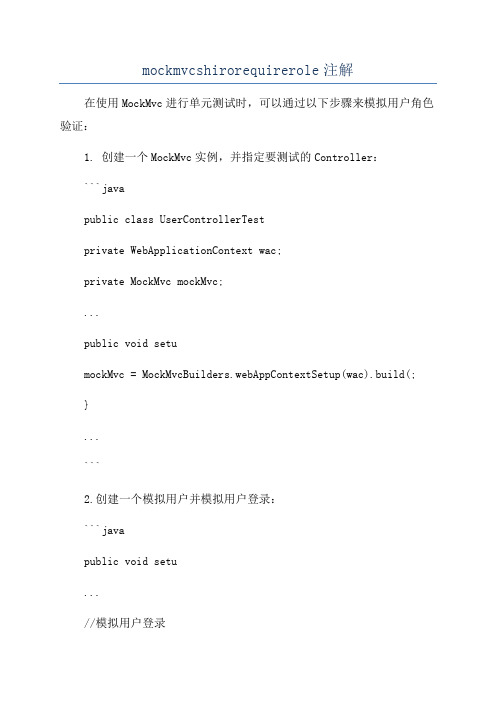
mockmvcshirorequirerole注解在使用MockMvc进行单元测试时,可以通过以下步骤来模拟用户角色验证:1. 创建一个MockMvc实例,并指定要测试的Controller:```javapublic class UserControllerTestprivate WebApplicationContext wac;private MockMvc mockMvc;...public void setumockMvc = MockMvcBuilders.webAppContextSetup(wac).build(;}...```2.创建一个模拟用户并模拟用户登录:```javapublic void setu...//模拟用户登录Subject subjectUnderTest = newSubject.Builder(.sessionId("testSessionId").authenticated(true).principals(new SimplePrincipalCollection("testUser", "testRealm")).buildSubject(;//将模拟用户设置到安全管理器中DefaultWebSubjectFactory subjectFactory = new DefaultWebSubjectFactory(;SecurityUtils.setSecurityManager(subjectFactory.createSecuri tyManager();SecurityUtils.getSubject(.login(subjectUnderTest.getPrincipa ls();...``````javapublic void testAdminRol...```通过以上步骤,我们就可以在使用MockMvc进行单元测试时,模拟用户角色验证了。
SpringBoot单元测试(Mock)
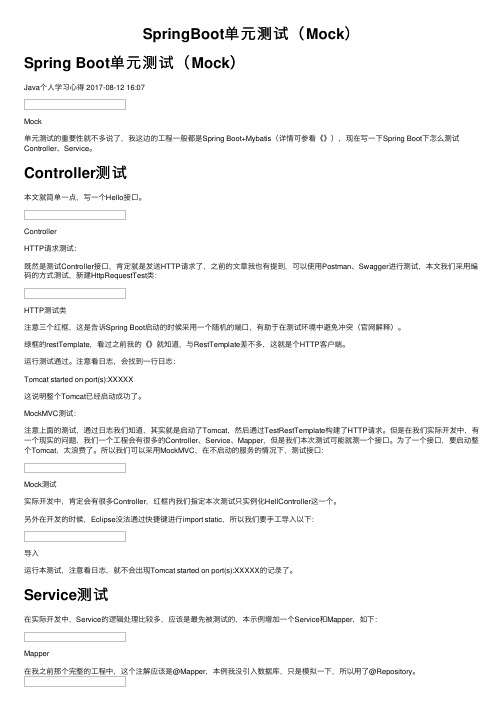
SpringBoot单元测试(Mock)Spring Boot单元测试(Mock)Java个⼈学习⼼得 2017-08-12 16:07Mock单元测试的重要性就不多说了,我这边的⼯程⼀般都是Spring Boot+Mybatis(详情可参看《》),现在写⼀下Spring Boot下怎么测试Controller、Service。
Controller测试本⽂就简单⼀点,写⼀个Hello接⼝。
ControllerHTTP请求测试:既然是测试Controller接⼝,肯定就是发送HTTP请求了,之前的⽂章我也有提到,可以使⽤Postman、Swagger进⾏测试,本⽂我们采⽤编码的⽅式测试,新建HttpRequestTest类:HTTP测试类注意三个红框,这是告诉Spring Boot启动的时候采⽤⼀个随机的端⼝,有助于在测试环境中避免冲突(官⽹解释)。
绿框的restTemplate,看过之前我的《》就知道,与RestTemplate差不多,这就是个HTTP客户端。
运⾏测试通过。
注意看⽇志,会找到⼀⾏⽇志:Tomcat started on port(s):XXXXX这说明整个Tomcat已经启动成功了。
MockMVC测试:注意上⾯的测试,通过⽇志我们知道,其实就是启动了Tomcat,然后通过TestRestTemplate构建了HTTP请求。
但是在我们实际开发中,有⼀个现实的问题,我们⼀个⼯程会有很多的Controller、Service、Mapper,但是我们本次测试可能就测⼀个接⼝。
为了⼀个接⼝,要启动整个Tomcat,太浪费了。
所以我们可以采⽤MockMVC,在不启动的服务的情况下,测试接⼝:Mock测试实际开发中,肯定会有很多Controller,红框内我们指定本次测试只实例化HellController这⼀个。
另外在开发的时候,Eclipse没法通过快捷键进⾏import static,所以我们要⼿⼯导⼊以下:导⼊运⾏本测试,注意看⽇志,就不会出现Tomcat started on port(s):XXXXX的记录了。
mockmvc shiro requirerole 注解

mockmvc shiro requirerole 注解接下来,需要在测试方法中创建一个模拟的HTTP请求,并设置请求的URI、请求方法、请求内容等。
例如,可以使用MockHttpServletRequestBuilder的静态方法来创建GET请求:```MockHttpServletRequestBuilder request = MockMvcRequestBuilders.get("/e某ample")```然后,可以通过使用MockMvc的perform方法来执行模拟的HTTP请求,并得到一个ResultActions对象。
通过ResultActions对象,可以对响应结果进行进一步的验证。
例如,可以使用ResultActions的andE某pect方法来验证响应的状态码是否为200:```ResultActions result = mockMvc.perform(request);result.andE某pect(MockMvcResultMatchers.status(.isOk(;```接着,需要模拟登录用户的身份。
可以使用Shiro的SecurityUtils.getSubject(.login方法来进行登录操作,并设置当前用户的身份和角色信息。
最后,在执行模拟的HTTP请求之前,需要在请求之前设置上下文环境,以便Shiro能够正确地进行权限判断。
可以使用Shiro的Subject类的doAs方法来在请求执行前设置上下文环境。
例如,可以在测试方法中使用try-with-resources语句来确保在请求执行后清理上下文环境:```try (MockedSecurityManager mockedSecurityManager = new MockedSecurityManager。
mockedSecurityManager.mockLogin("username", "password", "role");mockedSecurityManager.mockSubject(.doAs(new CreateSubjectCallback。
springboot单元测试之六:用mockmvc模拟cookie(springboot2。。。
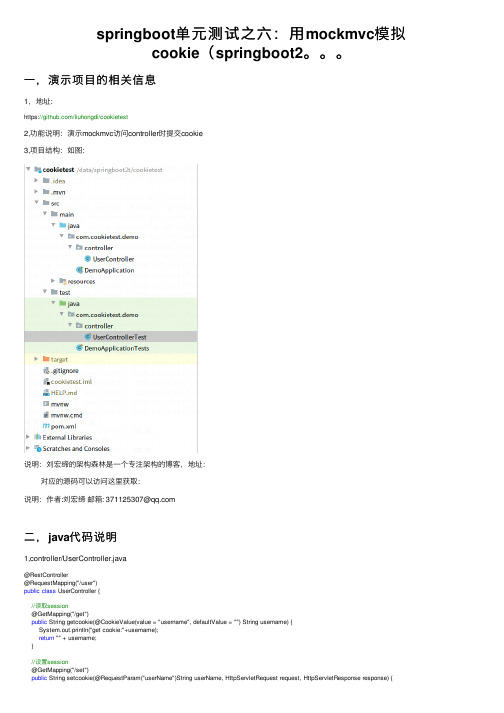
springboot单元测试之六:⽤mockmvc模拟cookie(springboot2。
⼀,演⽰项⽬的相关信息1,地址:https:///liuhongdi/cookietest2,功能说明:演⽰mockmvc访问controller时提交cookie3,项⽬结构:如图:说明:刘宏缔的架构森林是⼀个专注架构的博客,地址:对应的源码可以访问这⾥获取:说明:作者:刘宏缔邮箱: 371125307@⼆,java代码说明1,controller/UserController.java@RestController@RequestMapping("/user")public class UserController {//读取session@GetMapping("/get")public String getcookie(@CookieValue(value = "username", defaultValue = "") String username) {System.out.println("get cookie:"+username);return "" + username;}//设置session@GetMapping("/set")public String setcookie(@RequestParam("userName")String userName, HttpServletRequest request, HttpServletResponse response) {Cookie cookie = new Cookie("username", userName);//过期时间,单位是:秒(s)cookie.setMaxAge(30 * 24 * 60 * 60);//cookie.setPath(request.getContextPath());cookie.setPath("/");response.addCookie(cookie);System.out.println("set cookie:"+userName);return userName;}}2,controller/UserControllerTest.java@AutoConfigureMockMvc@SpringBootTestclass UserControllerTest {@Autowiredprivate UserController userController;@Autowiredprivate MockMvc mockMvc;@Test@DisplayName("测试读取cookie值")void getCookie() throws Exception{Cookie cookieu = new Cookie("username", "mr liu");//过期时间,单位是:秒(s)cookieu.setMaxAge(30 * 24 * 60 * 60);cookieu.setPath("/");//queryMvcResult mvcResult = mockMvc.perform(get("/user/get").cookie(cookieu).contentType(MediaType.APPLICATION_FORM_URLENCODED)).andReturn();String content = mvcResult.getResponse().getContentAsString();assertThat(content, equalTo("mr liu"));}@Test@DisplayName("测试读取cookie值失败")void getCookieFail() throws Exception{//queryMvcResult mvcResult = mockMvc.perform(get("/user/get")//.cookie(cookieu).contentType(MediaType.APPLICATION_FORM_URLENCODED)).andReturn();String content = mvcResult.getResponse().getContentAsString();assertThat(content, equalTo(""));}@Test@DisplayName("测试写cookie值")void setCookie() throws Exception{String cookieValue="laoliu123aaa";//queryMvcResult mvcResult = mockMvc.perform(get("/user/set?userName="+cookieValue) .contentType(MediaType.APPLICATION_FORM_URLENCODED)).andReturn();String content = mvcResult.getResponse().getContentAsString();assertThat(content, equalTo(cookieValue));}}三,效果测试1,查接访问url测试set cookie:http://127.0.0.1:8080/user/set?userName=laoliu123get cookie:http://127.0.0.1:8080/user/get返回:2,执⾏单元测试:四,查看spring boot的版本: . ____ _ __ _ _/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \\\/ ___)| |_)| | | | | || (_| | ) ) ) )' |____| .__|_| |_|_| |_\__, | / / / /=========|_|==============|___/=/_/_/_/:: Spring Boot :: (v2.4.3)。
springBoot单元测试-模拟MVC测试

springBoot单元测试-模拟MVC测试1)模拟mvc测试,和基础测试是⼀样的,都需要在pom⽂件中引⼊junit的⽀持。
略2)编写测试类 Application1TestMVC 在类头上除啦加⼊之前的@RunWith(SpringRunner.class)、@RunWith(SpringRunner.class) 之外还要加⼊新的注解 @AutoConfigureMockMvc // 注⼊MockMvc (当然你实在不想加也⾏,有其他办法,不过我不想说,⿇烦)package com.cx.springboot;import java.util.Date;import org.junit.Test;import org.junit.runner.RunWith;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;import org.springframework.boot.test.context.SpringBootTest;import org.springframework.http.MediaType;import org.springframework.mock.web.MockHttpServletResponse;import org.springframework.test.context.junit4.SpringRunner;import org.springframework.test.web.servlet.MockMvc;import org.springframework.test.web.servlet.MvcResult;import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;import com.alibaba.fastjson.JSON;import erModel;@RunWith(SpringRunner.class)@SpringBootTest@AutoConfigureMockMvc // 注⼊MockMvcpublic class Application1TestMVC {@Autowiredprivate MockMvc mvc;/**** @throws Exception* @创建时间 2018年7⽉13⽇* @功能描述通过链接传值,接受string 返回值*/@Testpublic void testString() throws Exception {//准备请求url 不⽤带ip、端⼝、项⽬名称等直接写接⼝的映射地址就可以了String url = "/app/get2/zhangsan/1";/* 构建request 发送请求GET请求* MockMvcRequestBuilders 中有很多请求⽅式。
springboot对shiro进行mock单元测试
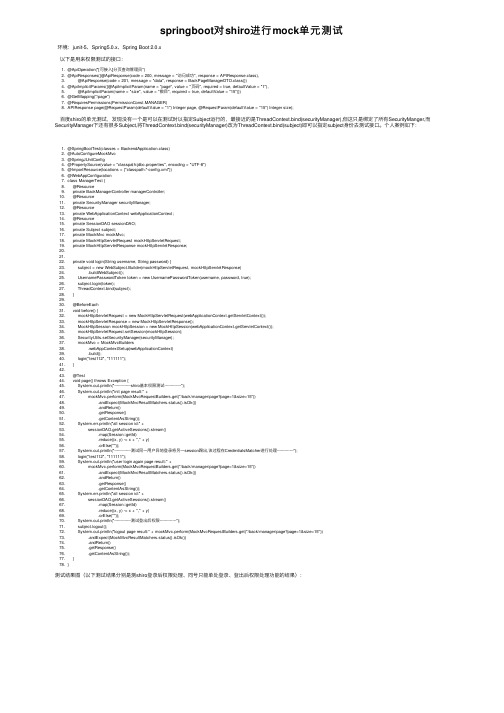
springboot对shiro进⾏mock单元测试环境:junit-5、Spring5.0.x、Spring Boot 2.0.x以下是⽤来权限测试的接⼝:1. @ApiOperation("[可接⼊]分页查询管理员")2. @ApiResponses({@ApiResponse(code = 200, message = "访问成功", response = APIResponse.class),3. @ApiResponse(code = 201, message = "data", response = BackPageManagerDTO.class)})4. @ApiImplicitParams({@ApiImplicitParam(name = "page", value = "页码", required = true, defaultValue = "1"),5. @ApiImplicitParam(name = "size", value = "数⽬", required = true, defaultValue = "15")})6. @GetMapping("/page")7. @RequiresPermissions(PermissionConst.MANAGER)8. APIResponse page(@RequestParam(defaultValue = "1") Integer page, @RequestParam(defaultValue = "15") Integer size);百度shiro的单元测试,发现没有⼀个是可以在测试时以指定Subject运⾏的,最接近的是ThreadContext.bind(securityManager),但这只是绑定了所有SecurityManger,⽽SecurityManager下还有很多Subject,将ThreadContext.bind(securityManager)改为ThreadContext.bind(subject)即可以指定subject⾝份去测试接⼝。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
目录(?)[-]1. pomxml2. controller层3. 单元测试类版权声明:本文为博主原创文章,转载请附明出处。
今天介绍一下springMVC的单元测试,可以参考spring 官方文档进行前提准备,springmvc的demo工程,这里就不做叙述了pom.xmlcontroller层[html]01.<d e p e n d e n c y > 02.<g r o u p I d >o r g .s p r i n g f r a m e w o r k </g r o u p I d > 03.<a r t i f a c t I d >s p ri n g -c o r e </a r t i f a c t I d > 04.</d e p e n d e n c y > 05.<d e p e n d e n c y > 06.<g r o u p I d >o r g .s p r i n g f r a m e w o r k </g r o u p I d > 07.<a r t i f a c t I d >s p r i n g -b e a n s </a r t i f a c t I d > 08.</d e p e n d e n c y > 09.<d e p e n d e n c y > 10.<g r o u p I d >o r g .s p r i n g f r a m e w o r k </g r o u p I d > 11.<a r t i f a c t I d >s p r i n g -c o n t e x t </a r t i f a c t I d > 12.</d e p e n d e n c y > 13.<d e p e n d e n c y > 14.<g r o u p I d >o r g .s p r i n g f r a m e w o r k </g r o u p I d > 15.<a r t i f a c t I d >s p r i n g -c o n t e x t -s u p p o r t </a r t i f a c t I d > 16.</d e p e n d e n c y > 17.<d e p e n d e n c y > 18.<g r o u p I d >o r g .s p r i n g f r a m e w o r k </g r o u p I d > 19.<a r t i f a c t I d >s p r i n g -w e b </a r t i f a c t I d > 20.</d e p e n d e n c y > 21.<d e p e n d e n c y > 22.<g r o u p I d >o r g .s p r i n g f r a m e w o r k </g r o u p I d > 23.<a r t i f a c t I d >s p r i n g -w e b m v c </a r t i f a c t I d > 24.</d e p e n d e n c y > 25.<d e p e n d e n c y > 26.<g r o u p I d >o r g .s p r i n g f r a m e w o r k </g r o u p I d > 27.<a r t i f a c t I d >s p r i n g -o r m </a r t i f a c t I d > 28.</d e p e n d e n c y > 29.<d e p e n d e n c y > 30.<g r o u p I d >o r g .s p r i n g f r a m e w o r k </g r o u p I d > 31.<a r t i f a c t I d >s p r i n g -t x </a r t i f a c t I d > 32.</d e p e n d e n c y > 33.<d e p e n d e n c y > 34.<g r o u p I d >o r g .s p r i n g f r a m e w o r k </g r o u p I d > 35.<a r t i f a c t I d >s p r i n g -t e s t </a r t i f a c t I d > 36.</d e p e n d e n c y > 37.<d e p e n d e n c y > 38.<g r o u p I d >j u n i t </g r o u p I d > 39.<a r t i f a c t I d >j u n i t </a r t i f a c t I d > 40. </d e p e n d e n c y >[java]01. p a c k a g e c o n t r o l l e r ;02.03. i m p o r t j a v a x .s e r v l e t .h t t p .H t t p S e s s i o n ;04.05.i m p o r t o r g.s p r i n g f r a m e w o r k.b e a n s.f a c t o r y.a n n o t a t i o n.A u t o w i r e d;06.i m p o r t o r g.s p r i n g f r a m e w o r k.w e b.b i n d.a n n o t a t i o n.P a t h V a r i a b l e;07.i m p o r t o r g.s p r i n g f r a m e w o r k.w e b.b i n d.a n n o t a t i o n.R e q u e s t M a p p i n g;08.i m p o r t o r g.s p r i n g f r a m e w o r k.w e b.b i n d.a n n o t a t i o n.R e q u e s t M e t h o d;09.i m p o r t o r g.s p r i n g f r a m e w o r k.w e b.b i n d.a n n o t a t i o n.R e q u e s t P a r a m;10.i m p o r t o r g.s p r i n g f r a m e w o r k.w e b.b i n d.a n n o t a t i o n.R e s t C o n t r o l l e r;11.12.i m p o r t s e r v i c e.U s e r S e r v i c e;13.i m p o r t d o m a i n.U s e r;14.15./**16.*U s e r C o n t r o l l e r.17.*@a u t h o r L e o n L e e18.*/19.@R e s t C o n t r o l l e r20.@R e q u e s t M a p p i n g(v a l u e="u s e r")21.p u b l i c c l a s s U s e r C o n t r o l l e r{22.23./**24.*U s e r S e r v i c e i n t e r f a c e.25.*/26.@A u t o w i r e d27.p r i v a t e U s e r S e r v i c e u s e r S e r v i c e;28.29./**30.*G e t u s e r M S G.31.*@p a r a m u s e r I d32.*@r e t u r n u s e r M s g33.*/34.@R e q u e s t M a p p i n g(v a l u e="u s e r M s g/{u s e r I d}",m e t h o d=R e q u e s t M e t h o d.G E T)35.p u b l i c U s e r g e t U s e r M s g(@P a t h V a r i a b l e(v a l u e="u s e r I d")S t r i n g u s e r I d){36.r e t u r n u s e r S e r v i c e.g e t U s e r M s g(u s e r I d);37.}38.39./**40.*U p d a t e u s e r M S G.41.*@p a r a m u s e r I d42.*@p a r a m u s e r N a m e43.*@r e t u r n u p d a t e d u s e r M S G44.*/45.@R e q u e s t M a p p i n g(v a l u e="u s e r M s g/{u s e r I d}",m e t h o d=R e q u e s t M e t h o d.P U T)46.p u b l i c U s e r p u t U s e r M s g(@P a t h V a r i a b l e(v a l u e="u s e r I d")S t r i n g u s e r I d,@R e q u e s t P a r a m S t r i n g u s e r N a m e,H t t p S e s s i o n s e s s i o n){47.i f(n u l l==(S t r i n g)s e s s i o n.g e t A t t r i b u t e("l o g i n U s e r"))48.r e t u r n n e w U s e r();49.S y s t e m.o u t.p r i n t l n((S t r i n g)s e s s i o n.g e t A t t r i b u t e("l o g i n U s e r"));50.r e t u r n u s e r S e r v i c e.p u t U s e r M s g(u s e r I d,u s e r N a m e);51.}52.53./**54.*D e l e t e u s e r.55.*@p a r a m u s e r I d56.*@r e t u r n d e l e t e d u s e r M S G57.*/58.@R e q u e s t M a p p i n g(v a l u e="u s e r M s g/{u s e r I d}",m e t h o d=R e q u e s t M e t h o d.D E L E T E)59.p u b l i c U s e r d e l U s e r M s g(@P a t h V a r i a b l e(v a l u e="u s e r I d")S t r i n g u s e r I d){60.r e t u r n u s e r S e r v i c e.d e l U s e r M s g(u s e r I d);61.}62.63./**64.*A d d u s e r.65.*@p a r a m u s e r N a m e66.*@r e t u r n a d d e d u s e r M S G67.*/68.@R e q u e s t M a p p i n g(v a l u e="u s e r M s g",m e t h o d=R e q u e s t M e t h o d.P O S T)69.p u b l i c U s e r p o s t U s e r M s g(@R e q u e s t P a r a m S t r i n g u s e r N a m e){70.r e t u r n u s e r S e r v i c e.p o s t U s e r M s g(u s e r N a m e);71.}72.73./**74.*l o g i n U s e r.N o t e t h a t d o n o t s e n d p a s s w o r d a s u r l.单元测试类这里的静态导入比较重要,有时候没办法自动导入的就是下面的 import static xxx.*另一点,代表的是加载的配置文件,可以根据需要进行添加75. * @p a r a m u s e r I d76. * @p a r a m p a s s w o r d77. * @r e t u r n78.*/ 79.@R e q u e s t M a p p i n g (v a l u e = "u s e r M s g /{u s e r I d }/{p a s s w o r d }", m e t h o d = R e q u e s t M e t h o d .G E T ) 80.p u b l i c b o o l e a n l o g i n U s e r (@P a t h V a r i a b l e S t r i n g u s e r I d , @P a t h V a r i a b l e S t r i n g p a s s w o r d , H t t p S e s s i o n s e s s i o n ){ 81.i f ("l o g i n U s e r ".e q u a l s (u s e r I d )&&"l o g i n U s e r ".e q u a l s (p a s s w o r d )){ 82.s e s s i o n .s e t A t t r i b u t e ("l o g i n U s e r ", u s e r I d ); 83.r e t u r n t r u e ; 84.} 85. r e t u r n f a l s e ;86. }87. }[java]01. @C o n t e x t C o n f i g u r a t i o n (l o c a t i o n s = {"c l a s s p a t h :a p p l i c a t i o n C o n t e x t .x m l ","c l a s s p a t h :a p p l i c a t i o n C o n t e x t .m v c .x m l "})[java]01.p a c k a g e c o n t r o l l e r .t e s t ; 02.03.i m p o r t s t a t i c o r g .s p r i n g f r a m e w o r k .t e s t .w e b .s e r v l e t .s e t u p .M o c k M v c B u i l d e r s .*; 04.i m p o r t s t a t i c o r g .s p r i n g f r a m e w o r k .t e s t .w e b .s e r v l e t .r e q u e s t .M o c k M v c R e q u e s t B u i l d e r s .*; 05.i m p o r t s t a t i c o r g .s p r i n g f r a m e w o r k .t e s t .w e b .s e r v l e t .r e s u l t .M o c k M v c R e s u l t H a n d l e r s .*; 06.i m p o r t s t a t i c o r g .s p r i n g f r a m e w o r k .t e s t .w e b .s e r v l e t .r e s u l t .M o c k M v c R e s u l t M a t c h e r s .*; 07.08.i m p o r t j a v a x .s e r v l e t .h t t p .H t t p S e s s i o n ; 09.10.i m p o r t o r g .j u n i t .B e f o r e ; 11.i m p o r t o r g .j u n i t .T e s t ; 12.i m p o r t o r g .j u n i t .r u n n e r .R u n W i t h ; 13.i m p o r t o r g .s p r i n g f r a m e w o r k .b e a n s .f a c t o r y .a n n o t a t i o n .A u t o w i r e d ; 14.i m p o r t o r g .s p r i n g f r a m e w o r k .h t t p .M e d i a T y p e ; 15.i m p o r t o r g .s p r i n g f r a m e w o r k .m o c k .w e b .M o c k H t t p S e s s i o n ; 16.i m p o r t o r g .s p r i n g f r a m e w o r k .t e s t .a n n o t a t i o n .R o l l b a c k ; 17.i m p o r t o r g .s p r i n g f r a m e w o r k .t e s t .c o n t e x t .C o n t e x t C o n f i g u r a t i o n ; 18.i m p o r t o r g .s p r i n g f r a m e w o r k .t e s t .c o n t e x t .j u n i t 4.S p r i n g J U n i t 4C l a s s R u n n e r ; 19.i m p o r t o r g .s p r i n g f r a m e w o r k .t e s t .c o n t e x t .t r a n s a c t i o n .T r a n s a c t i o n C o n f i g u r a t i o n ; 20.i m p o r t o r g .s p r i n g f r a m e w o r k .t e s t .c o n t e x t .w e b .W e b A p p C o n f i g u r a t i o n ; 21.i m p o r t o r g .s p r i n g f r a m e w o r k .t e s t .w e b .s e r v l e t .M o c k M v c ; 22.i m p o r t o r g .s p r i n g f r a m e w o r k .t e s t .w e b .s e r v l e t .M v c R e s u l t ; 23.i m p o r t o r g .s p r i n g f r a m e w o r k .t r a n s a c t i o n .a n n o t a t i o n .T r a n s a c t i o n a l ; 24.i m p o r t o r g .s p r i n g f r a m e w o r k .w e b .c o n t e x t .W e b A p p l i c a t i o n C o n t e x t ; 25.26./** 27.* s p r i n g m v c T e s t . 28.* @a u t h o r L e o n L e e 29.* @s i n c e s p r i n g -4.1.7 30.*/ 31.// s p r i n g 4.3 c h a n g e t o S p r i n g R u n n e r .c l a s s 32. @R u n W i t h (S p r i n g J U n i t 4C l a s s R u n n e r .c l a s s )33.@W e b A p p C o n f i g u r a t i o n34.@C o n t e x t C o n f i g u r a t i o n(l o c a t i o n s={"c l a s s p a t h:a p p l i c a t i o n C o n t e x t.x m l","c l a s s p a t h:a p p l i c a t i o n C o n t e x t.m v c.x m l"})35.//d o r o l l b a c k36.@T r a n s a c t i o n C o n f i g u r a t i o n(d e f a u l t R o l l b a c k=t r u e)37.@T r a n s a c t i o n a l38.p u b l i c c l a s s T e s t T e m p l a t e{39.@A u t o w i r e d40.p r i v a t e W e b A p p l i c a t i o n C o n t e x t w a c;41.42.p r i v a t e M o c k M v c m o c k M v c;43.p r i v a t e M o c k H t t p S e s s i o n s e s s i o n;44.45.@B e f o r e46.p u b l i c v o i d s e t u p(){47.//i n i t a p p l i c a t i o n C o n t e x t48.t h i s.m o c k M v c=w e b A p p C o n t e x t S e t u p(t h i s.w a c).b u i l d();49.t h i s.s e s s i o n=n e w M o c k H t t p S e s s i o n();50.}51.52.@T e s t53.p u b l i c v o i d g e t U s e r M s g()t h r o w s E x c e p t i o n{54.//g e t u s i n g g e t55.t h i s.m o c k M v c56..p e r f o r m((g e t("/u s e r/u s e r M s g/003"))57..a c c e p t(M e d i a T y p e.p a r s e M e d i a T y p e("a p p l i c a t i o n/j s o n;c h a r s e t=U T F-8")))58..a n d E x p e c t(s t a t u s().i s O k())59..a n d E x p e c t(c o n t e n t().c o n t e n t T y p e("a p p l i c a t i o n/j s o n;c h a r s e t=U T F-8"))60..a n d D o(p r i n t());//p r i n t61.}62.63.@T e s t64.//d o n't r o l l b a c k65.@R o l l b a c k(f a l s e)66.p u b l i c v o i d p u t U s e r M s g()t h r o w s E x c e p t i o n{67.//u p d a t e u s i n g p u t68.t h i s.m o c k M v c69..p e r f o r m((p u t("/u s e r/u s e r M s g/003"))70..c o n t e n t T y p e(M e d i a T y p e.A P P L I C A T I O N_F O R M_U R L E N C O D E D)71..p a r a m("u s e r N a m e","新名字03号")72..s e s s i o n((M o c k H t t p S e s s i o n)g e t L o g i n S e s s i o n())73..a c c e p t(M e d i a T y p e.p a r s e M e d i a T y p e("a p p l i c a t i o n/j s o n;c h a r s e t=U T F-8"))74.)75..a n d E x p e c t(s t a t u s().i s O k())76..a n d E x p e c t(c o n t e n t().c o n t e n t T y p e("a p p l i c a t i o n/j s o n;c h a r s e t=U T F-8"))77..a n d D o(p r i n t());//p r i n t78.}79.80.@T e s t81.p u b l i c v o i d d e l U s e r()t h r o w s E x c e p t i o n{82.//d e l e t e u s i n g d e l e t e83.t h i s.m o c k M v c84..p e r f o r m((d e l e t e("/u s e r/u s e r M s g/004"))85..a c c e p t(M e d i a T y p e.p a r s e M e d i a T y p e("a p p l i c a t i o n/j s o n;c h a r s e t=U T F-8"))86.)87..a n d E x p e c t(s t a t u s().i s O k())88..a n d E x p e c t(c o n t e n t().c o n t e n t T y p e("a p p l i c a t i o n/j s o n;c h a r s e t=U T F-8"))89..a n d D o(p r i n t());//p r i n t90.}91.92.@T e s t93.//d o n't r o l l b a c k94.@R o l l b a c k(f a l s e)95.p u b l i c v o i d p o s t U s e r()t h r o w s E x c e p t i o n{96.//a d d u s i n g p o s t97.t h i s.m o c k M v c98..p e r f o r m((p o s t("/u s e r/u s e r M s g"))99..p a r a m("u s e r N a m e","最新的用户")100..a c c e p t(M e d i a T y p e.p a r s e M e d i a T y p e("a p p l i c a t i o n/j s o n;c h a r s e t=U T F-8"))101.)102..a n d E x p e c t(s t a t u s().i s O k())103..a n d E x p e c t(c o n t e n t().c o n t e n t T y p e("a p p l i c a t i o n/j s o n;c h a r s e t=U T F-8"))104..a n d D o(p r i n t());//p r i n t105.}106.107./**108.*获取登入信息s e s s i o n109.*@r e t u r n110.*@t h r o w s E x c e p t i o n111.*/112.p r i v a t e H t t p S e s s i o n g e t L o g i n S e s s i o n()t h r o w s E x c e p t i o n{113.//m o c k r e q u e s t g e t l o g i n s e s s i o n114.//u r l=/x x x/x x x/{u s e r n a m e}/{p a s s w o r d}115.M v c R e s u l t r e s u l t=t h i s.m o c k M v c116..p e r f o r m((g e t("/u s e r/u s e r M s g/l o g i n U s e r/l o g i n U s e r"))) 117..a n d E x p e c t(s t a t u s().i s O k())118..a n d R e t u r n();119.r e t u r n r e s u l t.g e t R e q u e s t().g e t S e s s i o n();120.}121.}。