c语言第六章(苏小红版)
c语言课件ppt苏小红

文件的读写操作
文件读取
使用fscanf()、fgets()等函 数从文件中读取数据。
文件写入
使用fprintf()、fputs()等 函数将数据写入文件。
文件读写模式
根据需要选择不同的文件 读写模式,如只读、只写 、追加等。
程序调试技巧和方法
内存释放
动态分配的内存在使用完毕后需要释 放,以避免内存泄漏。C语言提供了 free函数来释放动态分配的内存。
07
文件操作和程序调试
文件的打开和关闭
01
02
03
文件打开
使用fopen()函数打开文 件,指定文件名和打开模 式。
文件关闭
使用fclose()函数关闭已打 开的文件,释放资源。
文件指针
03
控制结构
条件语句
条件语句
用于根据特定条件执行不同的 代码块。
if语句
根据条件判断,如果条件为真 ,执行if后面的代码块。
switch语句
根据表达式的值,执行不同的 代码块。
三元运算符
根据条件返回两个值中的一个 ,类似于if-else语句。
循环语句
循环语句
用于重复执行一段代码,直到满足特定条件 。
。
C语言最初的设计目的是为了编 写操作系统的内核,后来逐渐发
展成为一种通用的编程语言。
C语言的发展历程中,出现了许 多重要的版本和标准,如C89、
C99和C11等。
C语言的特点和应用领域
C语言是一种结构化编程语言,支持 过程化、面向对象和泛型编程范式。
C语言在操作系统、编译器、数据库 等领域的开发中扮演着重要的角色。
哈工大苏小红版C语言课件cha
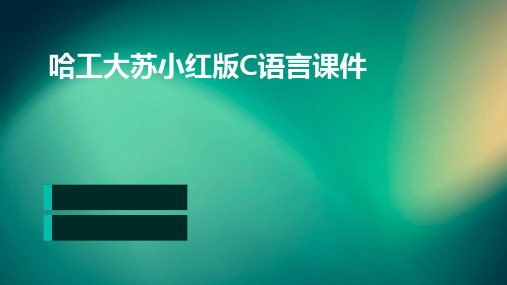
C语言的特点和应用领域
系统软件
嵌入式系统
操作系统、编译器等核心软件都是用 C语言编写的。
在嵌入式系统中,C语言被广泛应用 于开发底层驱动程序和操作系统内核。
应用软件
数据库、游戏、图形界面等应用软件 也广泛使用C语言开发。
C语言的基本语法结构
数据类型
运算符
控制结构
包括整型、浮点型、字 符型、数组类型、结构
短整型通常占用2个字节,整型 占用4个字节,长整型占用8个 字节。
浮点型数据类型
浮点型数据类型用于 存储小数,包括单精 度浮点数和双精度浮 点数。
浮点型数据类型用于 存储实数,包括正实 数、负实数和零。
单精度浮点数通常用 float表示,双精度浮 点数通常用double 表示。
字符型数据类型
01
05 指针和内存管理
指针的定义和使用
01
02
03
04
指针是变量,用于存储 内存地址。
指针变量必须先定义后 使用。
使用指针前需要先赋值。
指针可以指向同类型的 变量或数组元素。
内存管理的基本概念
01
02
03
04
内存分为堆区和栈区。
堆区用于动态内存分配,由程 序员管理。
栈区用于存储局部变量,由系 统自动管理。
内存管理涉及内存的申请、使 用和释放。
动态内存分配和释放
使用`malloc()`函数在堆区分配内存。
使用`realloc()`函数调整已分配内存 的大小。
使用`calloc()`函数在堆区分配并初始 化内存。
使用`free()`函数释放已分配的内存。
06 文件操作
文件的打开和关闭
要点一
打开文件
C语言程序设计苏小红版答案解析
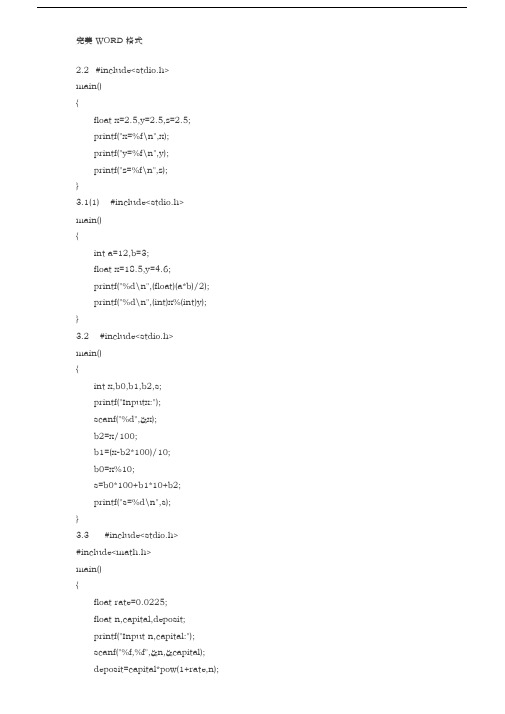
完美 WORD 格式2.2 #include<stdio.h>main(){float x=2.5,y=2.5,z=2.5;printf("x=%f\n",x);printf("y=%f\n",y);printf("z=%f\n",z);}3.1(1) #include<stdio.h>main(){int a=12,b=3;float x=18.5,y=4.6;printf("%d\n",(float)(a*b)/2);printf("%d\n",(int)x%(int)y);}3.2 #include<stdio.h>main(){int x,b0,b1,b2,s;printf("Inputx:");scanf("%d",&x);b2=x/100;b1=(x-b2*100)/10;b0=x%10;s=b0*100+b1*10+b2;printf("s=%d\n",s);}3.3 #include<stdio.h>#include<math.h>main(){float rate=0.0225;float n,capital,deposit;printf("Input n,capital:");scanf("%f,%f",&n,&capital);deposit=capital*pow(1+rate,n);printf("deposit=%f\n",deposit);}3.4 #include<stdio.h>#include<math.h>main(){float a,b,c;专业知识分享完美 WORD 格式double x,y;printf("Inputa,b,c:");scanf("%f,%f,%f",&a,&b,&c);x=(-b+sqrt(b*b-4*a*c))/(2*a);y=(-b-sqrt(b*b-4*a*c))/(2*a);printf("x=%f,y=%f\n",x,y);}4.1(1) #include<stdio.h>main(){char c1='a',c2='b',c3='c';printf("a%cb%cc%c\n",c1,c2,c3); }4.1(2) #include<stdio.h>main(){int a=12,b=15;printf("a=%d%%,b=%d%%\n",a,b); }4.1(3) #include<stdio.h>main(){int a,b;scanf("%2d%*2s%2d",&a,&b);printf("%d,%d\n",a,b);}4.2 #include<stdio.h>main(){long a,b;float x,y;scanf("%d,%d\n",&a,&b);scanf("%f,%f\n",&x,&y);printf("a=%d,b=%d\n",a,b);printf("x=%f,b=%f\n",x,y);}5.1 #include<stdio.h>main(){float a;printf("Innputa:");scanf("%f",&a);if(a>=0){专业知识分享完美 WORD 格式a=a;printf("a=%f\n",a);}else{a=-a;printf("a=%f\n",a);}}5.2 #include<stdio.h>main(){int a;printf("Inputa:");scanf("%d",&a);if(a%2==0){printf("a 是偶数 ");}else{printf("a 是奇数 ");}}5.3 #include<stdio.h>#include<math.h>main(){float a,b,c,s,area;printf("Inputa,b,c:");scanf("%f,%f,%f",&a,&b,&c);if(a+b>c&&a+c>b&&b+c>a){s=(a+b+c)/2;area=(float)sqrt(s*(s-a)*(s-b)*(s-c));printf("area=%f\n",area);}else{printf(" 不是三角形 ");}}5.4 #include<stdio.h>#include<math.h>专业知识分享完美 WORD 格式main(){float a,b,c,x,y;printf("Inputa,b,c:");scanf("%f,%f,%f",&a,&b,&c);if(a==0){printf(" 该方程不是一元二次方程\n");}if(b*b-4*a*c>0){x=(-b+sqrt(b*b-4*a*c))/(2*a);y=(-b-sqrt(b*b-4*a*c))/(2*a);printf("x=%f,y=%f\n",x,y);}else if(b*b-4*a*c==0){x=-b/(2*a);y=-b/(2*a);printf("x=%f,y=%f\n",x,y);}else{printf(" 该方程无实根\n");}}5.5 #include<stdio.h>main(){int year,flag;printf("Input a year:");scanf("%d",&year);if(year%4==0&&year%400!=0||year%400==0) {flag=1;}else{flag=0;}if(flag==1){printf("%d is a leap year !\n",year); }专业知识分享完美 WORD 格式else{printf("%d is not a leap year !\n",year);}}5.6 #include<stdio.h>main(){int year,flag;printf("Input a year:");scanf("%d",&year);flag=year%400==0||year%4==0&&year%100!=0?1:0;if(flag==1&&flag!=0){printf("%d is a leap year !\n",year);}else{printf("%d is not a leap year !\n",year);}}5.7 #include<stdio.h>main(){char ch;printf("Inputch:");scanf("%c",&ch);if(ch>='a'&&ch<='z'){ch=getchar();ch=ch-32;printf("%c,%d\n",ch,ch);}else if(ch>='A'&&ch<='Z'){ch=getchar();ch=ch+32;printf("%c,%d\n",ch,ch);}else{printf("%c",ch);}}专业知识分享完美 WORD 格式5.8 #include<stdio.h>main(){char ch;printf("Inputch:");scanf("%c",&ch);if(ch>=48&&ch<=57){printf("ch 是数字字符 \n");}else if(ch>=65&&ch<=90){printf("ch 是大写字母 \n");}else if(ch>=97&&ch<=122){printf("ch 是小写字母 \n");}else if(ch==32){printf("ch 是空格 \n");}else{printf("ch 是其他字符 \n");}}5.9 #include<stdio.h>main(){int score,grade;printf("Input score:");scanf("%d",&score);grade=score/10;if(score<0||score>100){printf("Input error\n");}if(score>=90&&score<=100){printf("%d--A\n",score);}else if(score>=80&&score<90){专业知识分享完美 WORD 格式printf("%d--B\n",score);}else if(score>=70&&score<80){printf("%d--C\n",score);}else if(score>=60&&score<70){printf("%d--D\n",score);}else if(score>=0&&score<60){printf("%d- -E\n",score);}}5.10 #include<stdio.h>main(){int year,month;printf("Input year,month:");scanf("%d,%d",&year,&month);if(month>12||month<=0){printf("error month\n");}else{switch(year,month){case 12:case 10:case 8:case 7:case 5:case 3:case 1:printf("31 天 \n");break;case 11:case 9:case 6:case 4:printf("30 天 \n");break;专业知识分享完美 WORD 格式case 2:if(year%4==0&&year!=0||year%400==0){printf("29 天 \n");}else{printf("28 天 \n");}break;default:printf("Input error\n");}}}6.1(1) #include<stdio.h>main(){int i,j,k;char space=' ';for(i=1;i<=4;i++){for(j=1;j<=i;j++){printf("%c",space);}for(k=1;k<=6;k++){printf("*");}printf("\n");}}6.1(2) #include<stdio.h>main(){int k=4,n;for(n=0;n<k;n++){if(n%2==0) continue;k--;}printf("k=%d\n,n=%d\n",k,n);}专业知识分享完美 WORD 格式6.1(3) #include<stdio.h>main(){int k=4,n;for(n=0;n<k;n++){if(n%2==0) break;k--;}printf("k=%d,n=%d\n",k,n); }6.2(1) #include<stdio.h> main(){int i,sum=0;for(i=1;i<=101;i++){sum=sum+i;}printf("sum=%d\n",sum); }6.2(2) #include<stdio.h>main(){long i;long term,sum=0;for(i=1;i<=101;i=i+2){term=i*(i+1)*(i+2);sum=sum+term;}printf("sum=%ld\n",sum); }6.2(4) #include<stdio.h>#include<math.h>main(){int n=1;float term=1.0,sign=1,sum=0; while(term<=-1e-4||term>=1e-4) {term=1.0/sign;sum=sum+term;sign=sign+n;专业知识分享完美 WORD 格式n++;}printf("sum=%f\n",sum);}6.2(5) #include<stdio.h>#include<math.h>main(){int n=1,count=1;float x;double sum,term;printf("Input x:");scanf("%f",&x);sum=x;term=x;do{term=-term*x*x/((n+1)*(n+2));sum=sum+term;n=n+2;count++;}while(fabs(term)>=1e-5);printf("sin(x)=%f,count=%d\n",sum,count);}6.3 #include<stdio.h>main(){int x=1,find=0;while(!find){if(x%2==1&&x%3==2&&x%5==4&&x%6==5&&x%7==0){printf("x=%d\n",x);find=1;x++;}}}/* int x,find=0;for (x=1;!find;x++){if(x%2==1&&x%3==2&&x%5==4&&x%6==5&&x%7==0){printf("x=%d\n",x);find=1;专业知识分享完美 WORD 格式}}}*/6.4 #include<stdio.h>main(){int i,n;long p=1,m=1;printf("Input n:");scanf("%d",&n);for(i=1;i<=n;i++){p=i*i;m=i*i*i;printf("p=%d,m=%d\n",i,p,i,m);}}6.5 #include<stdio.h>main(){float c,f;for(c=-40;c<=110;c=c+10){f=9/5*c+32;printf("f=%f\n",f);}}6.6 #include<stdio.h>#include<math.h>main(){int n;double c=0.01875,x;do{x=x*pow(1+c,12)-1000;n++;}while(x>0);printf("x=%d\n",x);}6.7 #include<stdio.h>main(){int n=0;专业知识分享完美 WORD 格式float a=100.0,c;printf("Inputc:");scanf("%f",&c);do{a=a*(1+c);n++;}while(a<=200);printf("n=%d\n",n);}6.8 #include<stdio.h>#include<math.h>main(){int n=1,count=1;double sum=1,term=1;while(fabs(term)>=1e-5){term=pow(-1,count)*(1.0/(n+2));sum=sum+term;n=n+2;count++;}sum=4*sum;printf( "sum=%f,count=%d\n",sum,count); }6.9 #include<stdio.h>#include<math.h>main(){int n=1,count=1;double sum=1,term=1;while(fabs(term)>=1e-5){term=term*(1.0/n);sum=sum+term;n++;count++;}printf("sum=%f,count=%d\n",sum,count); }6.10 #include<stdio.h>#include<math.h>专业知识分享完美 WORD 格式main(){int x;for(x=100;x<=999;x++){if(x==pow(x/100,3)+pow(x/10%10,3)+pow(x%10,3))printf("x=%d\n",x);}}6.11 #include<stdio.h>main(){int i=0,n;long sum=0,term=1;printf("Inputn:");scanf("%d",&n);do{i++;term=term*i;sum=sum+term;}while(sum<n);printf("%d\n",i-1);}6.12 #include<stdio.h>main(){int i,n,m,count=0,sum=0;printf("Input n:");scanf("%d",&n);for(i=1;i<=n;i++){printf("Inputm:");scanf("%d",&m);if(m>0){sum=sum+m;count++;}else{break;}printf("sum=%d,count=%d\n",sum,count);专业知识分享完美 WORD 格式}}6.13 #include<stdio.h>main(){int i,n,m,count=0,sum=0;printf("Inputn:");scanf("%d",&n);for(i=1;i<=n;i++){printf("Inputm:");scanf("%d",&m);if(m>0||m<0){sum=sum+m;count++;}else{break;}printf("sum=%d,count=%d\n",sum,count);}}6.14 #include<stdio.h>main(){int x,y,z;for(x=0;x<=17;x++){for(y=0;y<=25;y++){3*x+2*y+z==50;z=30-x-y;if( 3*x+2*y+z==50&&x+y+z==30)printf("x=%d,y=%d,z=%d\n",x,y,z);}}}6.15 #include<stdio.h>main(){int x,y;for(x=0;x<=98;x++)专业知识分享完美 WORD 格式{y=98-x;2*x+4*y==386;if(x+y==98&&2*x+4*y==386){printf("x=%d,y=%d\n",x,y);}}}6.16 #include<stdio.h>main(){int x,y,z;for(x=0;x<=20;x++){for(y=0;y<=33;y++){3*y+5*x+z/3.0==100;z=100-x-y;if (5*x+3*y+z/3.0==100&&z+x+y==100){printf("x=%d,y=%d,z=%d\n",x,y,z);}}}}6.17 #include<stdio.h>main(){int x,y,z;for(x=1;x<=9;x++){for(y=1;y<=17;y++){10*x+5*y+z==100;z=50-x-y;if( 10*x+5*y+z==100&&x+y+z==50&&z>0){printf("x=%d,y=%d,z=%d\n",x,y,z);}}}专业知识分享完美 WORD 格式}7.1 #include<stdio.h> int Square(int i){return i*i;}int main(){int i=0;i=Square(i);for( ;i<3;i++){static int i=1;i+=Square(i);printf("%d,",i);}printf("%d\n",i);return 0;}7.2 #include<stdio.h> int hour,minute,second; void update(){second++;if(second==60){second=0;minute++;}if(minute==60){minute=0;hour++;}if(hour==24)hour=0;}void display(){printf("%d,%d,%d\n",hour,minute,second);}void delay(){专业知识分享完美 WORD 格式int t;for(t=0;t<100000000;t++);}int main(){int i;void updaye(),display(),delay();for(i=0;i<1000000;i++){update();display();delay();}return 0;}7.3 #include<stdio.h>int GetMax(int a,int b);int main(){int x,y,max;printf("Inputx,y:");scanf("%d,%d",&x,&y);max=GetMax(x,y);printf("max=%d\n",max);return 0;}int GetMax(int m,int n){if(m>=n)return m;elsereturn n;}7.4 #include<stdio.h>int LCM(int n,int m);int main(){int a,b;printf("Inputa,b:");scanf("%d,%d",&a,&b);printf("%d\n",LCM(a,b));return 0;}int LCM(int n,int m)专业知识分享完美 WORD 格式{int x;int find=0;for(x=1;!find;x++){if(x%n==0&&x%m==0){find=1;}}return x-1;}7.5 #include<stdio.h>long Fact(int n);int main(){int m,a;printf("Inputm:");scanf("%d",&m);for(a=1;a<=m;a++){printf("%d!=%ld\n",a,Fact(a));}return 0;}long Fact(int n){int i;long result=1;for(i=2;i<=n;i++)result*=i;return result;}7.6 #include<stdio.h>long Fact(int n);int main(){int m;long ret;printf("Inputm:");scanf("%d",&m);ret=Fact(m);printf("ret=%d\n",ret);专业知识分享完美 WORD 格式return 0;}long Fact(int n){int i;long result=1,sum=0;for(i=2;i<=n;i++){result*=i;sum=sum+result;}return sum;}7.7(1) #include<stdio.h>int Gcd(int a,int b);int main(){int m,n;printf("Inputm,n:");scanf("%d,%d",&m,&n);printf("%d\n",Gcd(m,n));return 0;}int Gcd(int a,int b){int t,min,find=0;min=a<b?a:b;t=min;for(t=min;!find;t--){a%t==0;b%t==0;if(a%t==0&&b%t==0)return t;}find=1;}7.7(2) #include<stdio.h>int Gcd(int a,int b);int main(){int m,n;printf("Inputm,n:");scanf("%d,%d",&m,&n);专业知识分享完美 WORD 格式printf("%d\n",Gcd(m,n));return 0;}int Gcd(int a,int b){int r,temp;r=a%b;if(r==0)return b;elsedo{temp=b;b=r;a=temp;r=a%b;}while(r!=0);return b;}8.1(1) #include<stdio.h> void Func(int x){x=2;}int main(){int x=10;Func(x);printf("%d",x);return 0;}8.1(2) #include<stdio.h> void Func(int b[]){int j;for(j=0;j<4;j++){b[j]=j;}}int main(){static int a[]={5,6,7,8},i;Func(a);for(i=0;i<4;i++)专业知识分享完美 WORD 格式{printf("%d",a[i]);}return 0;}8.2(1) int PositiveNum(int a[],int n) {int i,count=0;for(i=0;i<n;i++){if(a[i]>0)count++;}return 0;}8.2(2) void Fib(long f[],long n){int i;f[0]=0;f[1]=1;for(i=2;i<n;i++){f[i]=f[i-1]+f[i-2];}}8.2(3) #include<stdio.h>int main(){int a[10],n,max,min,maxPos,minPos;for(n=0;n<10;n++){scanf("%d",&a[n]);}max=min=a[0];maxPos=minPos=0;for(n=0;n<10;n++){if(a[n]>max){max=a[n];maxPos=n;}else if(a[n]<min){min=a[n];专业知识分享完美 WORD 格式minPos=n;}}printf("max=%d,pos=%d\n",max,maxPos);printf("min=%d,pos=%d\n",min,minPos);return 0;}8.3 void DivArray(int *pArray,int n){int i;for(i=0;i<n;i++){pArray[i]/=pAttay[0];}}8.4 #include<stdio.h>#define N 40int FailNum(int score[],int n);void ReadScore(int score[],int n);int main(){int score[N],n;printf("Input n:");scanf("%d",&n);ReadScore(score,n);FailNum(score,n);printf("FailNum students are %d\n",FailNum(score,n));return 0;}int FailNum(int score[],int n){int i,count=0;for(i=0;i<n;i++){if(score[i]<60)count++;}return count;}void ReadScore(int score[],int n){int i;printf("Input score:");for(i=0;i<n;i++)专业知识分享完美 WORD 格式{scanf("%d",&score[i]);}}8.5 #include<stdio.h>#define N 40int HighAver(int score[],int n);int ReadScore(int score[]);int main(){int score[N],n;n=ReadScore(score);printf("HighAver students are %d\n",HighAver(score,n));return 0;}int HighAver(int score[],int n){int i,count=0,sum=0,aver;for(i=0;i<n;i++){sum=sum+score[i];aver=sum/n;}for(i=0;i<n;i++){if(score[i]>aver)count++;}return count;}int ReadScore(int score[]){int i=-1;do{i++;printf("Input score:");scanf("%d",&score[i]);}while(score[i]>=0);return i;}8.6 #include<stdio.h>#define N 40int ReadScore(int score[],long num[]);int FindMax(int score[],long num[],int n);专业知识分享完美 WORD 格式int main(){int score[N],n,max,num,t;long num[N];t=FindMax(score,n);max=score[t];num=num[t];printf("max=%d,num=%d\n",max,num);return 0;}int ReadScore(int score[],long num[]){int i=-1;do{i++;printf("Input student's ID and score:");scanf("%ld%d",&num[i],&score[i]);}while(num[i]>0&&score[i]>=0);return i;}int FindMax(int score[],long num[],int n){int max,i;max=score[0];for(i=1;i<n;i++){if(score[i]>max)max=score[i];}return i;}8.7 #include<stdio.h>#define N 40int Read(int a[]);int ChangeArry(int a[],int n);int main(){int a[N],n;printf("%d\n",ChangeArry(a,n));return 0;}int Read(int a[]){int i;专业知识分享完美 WORD 格式for(i=0;i<9;i++){printf("Input a:");scanf("%d",&a[i]);return i;}int ChangeArry(int a[],int n){int max,min,i,maxpos,minpos,term;max=min=score[0];for(i=1;i<n;i++){if(a[i]>max)max=score[i];maxpos=n;else(a[i]<min)min=a[i];minpos=n;temp=maxpos;maxpos=minpos;minpos=temp;}return a[i];}8.10 #include<stdio.h>#define N 5void main(){int a[N][N];int sum = 0;int i, j;printf(" 请输入一个 %d*%d 的矩阵 \n",N,N);for(i = 0; i < N; i++){for(j = 0; j < N; j++){scanf("%d",&a[i][j]);}}for(i = 0; i < N; i++){for(j = 0; j < N; j++){专业知识分享。
C语言苏小红版第六章习题答案

第六章习题1。
(1)#include〈stdio。
h〉main(){int i,j,k;char space=’ ’;for(i=1;i〈=4;i++){for(j=1;j<=i;j++){printf("%c",space);}for(k=1;k<=6;k++){printf("*");}printf("\n”);}}(2)#include 〈stdio.h>main(){int k = 4, n;for (n = 0;n < k; n++){if (n %2 == 0)continue;k—-;}printf(”k = %d,n = %d\n”,k,n);}(3)#include 〈stdio。
h〉main(){int k = 4, n;for (n = 0; n < k; n++){if (n % 2 ==0)break;k—-;}printf(”k = %d, n = %d\n",k,n);}2.(1)计算1+3+5+……99+101=#include<stdio。
h>main(){int i,sum=0;for(i=1;i〈=101;i=i+2){sum=sum+i;}printf(”sum=%d\n",sum);}(2)计算1*2*3+3*4*5+。
..+99*100*101= #include〈stdio。
h〉main(){long i;long term,sum=0;for(i=1;i〈=99;i=i+2){term=i*(i+1)*(i+2);sum=sum+term;}printf(”sum=%ld",sum);}(3)计算a+aa+aaa+..。
+aa..。
a(n个a)的值#include〈stdio.h>main( ){long term=0,sum=0;int a,i,n;printf("input a,n:”);scanf(”%d,%d”,&a,&n);for(i=1;i〈=n;i=i++){term=term*10+a;sum=sum+term;}printf("sum=%ld\n”,sum);}(4 )计算1—1/2+1/3—。
C语言程序设计 苏小红版 第五六单元 答案
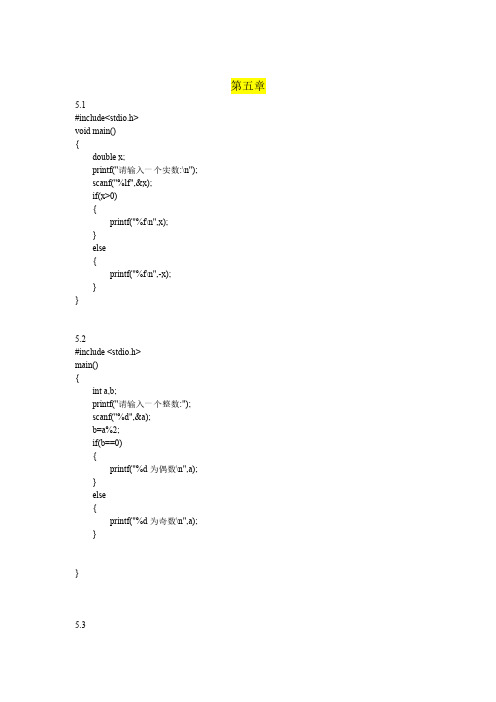
printf("该方程不是一元二次方程.\n"); } else if(b*b-4*a*c>0) {
d=sqrt(b*b-4*a*c); x1=(-b+d)/(2*a);
x2=(-b-d)/(2*a); printf("x1=%f,x2=%f\n",x1,x2); } else if(b*b-4*a*c==0)
#include<stdio.h> #include<math.h> main() {
float a,b,c,s,area; printf("请输入三边长:"); scanf("%f,%f,%f",&a,&b,&c); s=(a+b+c)/2; area=(float)sqrt(s*(s-a)*(s-b)*(s-c)); if((a+b>c)&&(a+c>b)&&(b+c>a)) {
printf("%d 年是闰年\n",year); } else {
printf("%d 年不是闰年\n",year); } }
5.7 #include<stdio.h> main() {
char ch; printf("请输入一个字母:"); ch=getchar(); if((ch>=65)&&(ch<=90)) {
int a,b; printf("请输入一个整数:"); scanf("%d",&a); b=a%2; if(b==0) {
《C语言程序设计》(苏小红)-课后习题答案-高等教育出版社

2.2#include<stdio.h>main(){float x=2.5,y=2.5,z=2.5;printf("x=%f\n",x);printf("y=%f\n",y);printf("z=%f\n",z);}3.1(1)#include<stdio.h>main(){int a=12,b=3;float x=18.5,y=4.6;}3.1(2)#include<stdio.h>main(){int x=32,y=81,p,q;p=x++;q=--y;printf("%d %d\n",p,q);printf("%d %d\n",x,y);}3.2#include<stdio.h>main(){int x,b0,b1,b2,s;printf("Inputx:");scanf("%d",&x);b2=x/100;b1=(x-b2*100)/10;//或(x%100)/10;或x/10%10;b0=x%10;s=b0*100+b1*10+b2;printf("s=%d\n",s);}3.3#include<stdio.h>#include<math.h>main(){float rate=0.0225;float n,capital,deposit;printf("Input n,capital:");scanf("%f,%f",&n,&capital);deposit=capital*pow(1+rate,n);printf("deposit=%f\n",deposit); }3.4#include<stdio.h>#include<math.h>main(){float a, b, c;double x, y;printf("Input a, b, c:");scanf("%f %f %f", &a, &b, &c);x=(-b+sqrt(b*b-4*a*c))/(2*a);y=(-b-sqrt(b*b-4*a*c))/(2*a);printf("x=%f,y=%f\n",x,y);}习题44.1(1)#include<stdio.h>main(){char c1='a',c2='b',c3='c';}4.1(2)#include<stdio.h>main(){int a=12,b=15;}4.1(3)#include<stdio.h>main(){int a,b;printf("%d,%d\n",a,b);}4.2#include<stdio.h>main(){long a,b;float x,y;scanf("%d,%d\n",&a,&b);scanf("%f,%f\n",&x,&y);printf("a=%d,b=%d\n",a,b);printf("x=%f,b=%f\n",x,y); }5.1#include<stdio.h>main(){float a;printf("Input a:");scanf("%f",&a);if(a>=0){a=a;printf("a=%f\n",a);}else{a=-a;printf("a=%f\n",a);}}5.2#include<stdio.h>main(){int a;printf("Input a:");scanf("%d", &a);if(a%2==0){printf("a是偶数");}else{printf("a是奇数");}}5.3#include<stdio.h>#include<math.h>main(){float a,b,c,s,area;printf("Input a, b, c:");scanf("%f %f %f", &a, &b, &c);if(a+b>c&&a+c>b&&b+c>a){s=(a+b+c)/2;area=(float)sqrt(s*(s-a)*(s-b)*(s-c));printf("area=%f\n",area);}else{printf("不是三角形");}}5.4#include<stdio.h>#include<math.h>main(){float a,b,c,x,y;printf("Inputa,b,c:");scanf("%f,%f,%f",&a,&b,&c);if(a==0){printf("该方程不是一元二次方程\n");}if(b*b-4*a*c>0){x=(-b+sqrt(b*b-4*a*c))/(2*a);y=(-b-sqrt(b*b-4*a*c))/(2*a);printf("x=%f,y=%f\n",x,y);}else if(b*b-4*a*c==0){x=-b/(2*a);y=-b/(2*a);printf("x=%f,y=%f\n",x,y);}else{printf("该方程无实根\n");}}5.5#include<stdio.h>main(){int year,flag;printf("Input a year:");scanf("%d",&year);if(year%4==0&&year%400!=0||year%400==0){flag=1;}else{flag=0;}if(flag==1){printf("%d is a leap year!\n",year);}else{printf("%d is not a leap year!\n",year);}}5.6#include<stdio.h>main(){int year,flag;printf("Input a year:");scanf("%d",&year);flag=year%400==0||year%4==0&&year%100!=0?1:0;if(flag==1&&flag!=0){printf("%d is a leap year!\n",year);}else{printf("%d is not a leap year!\n",year);}}5.7#include<stdio.h>main(){char ch;printf("Inputch:");scanf("%c",&ch);if(ch>='a'&&ch<='z'){ch=getchar();ch=ch-32;printf("%c,%d\n",ch,ch);}else if(ch>='A'&&ch<='Z'){ch=getchar();ch=ch+32;printf("%c,%d\n",ch,ch);}else{printf("%c",ch);}}5.8#include<stdio.h>main(){char ch;printf("Inputch:");scanf("%c",&ch);if(ch>=48&&ch<=57){printf("ch是数字字符\n");}else if(ch>=65&&ch<=90){printf("ch是大写字母\n");}else if(ch>=97&&ch<=122){printf("ch是小写字母\n");}else if(ch==32){printf("ch是空格\n");}else{printf("ch是其他字符\n");}}5.9#include<stdio.h>main(){int score,grade;printf("Input score:");scanf("%d",&score);grade=score/10;if(score<0||score>100){printf("Input error\n");}if(score>=90&&score<=100){printf("%d--A\n",score);}else if(score>=80&&score<90){printf("%d--B\n",score);}else if(score>=70&&score<80){printf("%d--C\n",score);}else if(score>=60&&score<70){printf("%d--D\n",score);}else if(score>=0&&score<60){printf("%d--E\n",score);}}5.10#include<stdio.h>main(){int year,month;printf("Input year,month:");scanf("%d,%d",&year,&month);if(month>12||month<=0){printf("error month\n");}else{switch(year,month){case12:case10:case8:case7:case5:case3:case1:printf("31天\n");break;case11:case9:case6:case4:printf("30天\n");break;case2:if(year%4==0&&year!=0||year%400==0){printf("29天\n");}else{printf("28天\n");}break;default:printf("Input error\n");}}}6.1(1)#include<stdio.h>main(){int i,j,k;char space='';for(i=1;i<=4;i++){for(j=1;j<=i;j++){printf("%c",space);}for(k=1;k<=6;k++){printf("*");}printf("\n");}}6.1(2)#include<stdio.h>main(){int k=4,n;for(n=0;n<k;n++){if(n%2==0)continue;k--;}printf("k=%d\n,n=%d\n",k,n);}6.1(3)#include<stdio.h>main(){int k=4,n;for(n=0;n<k;n++){if(n%2==0)break;k--;}printf("k=%d,n=%d\n",k,n);}6.2(1)#include<stdio.h>main(){int i,sum=0;for(i=1;i<=101;i++){sum=sum+i;}printf("sum=%d\n",sum);}6.2(2)#include<stdio.h>main(){long i;long term,sum=0;for(i=1;i<=101;i=i+2){term=i*(i+1)*(i+2);sum=sum+term;}printf("sum=%ld\n",sum);}6.2(4)#include<stdio.h>#include<math.h>main(){int n=1;float term=1.0,sign=1,sum=0;while(term<=-1e-4||term>=1e-4){term=1.0/sign;sum=sum+term;sign=sign+n;n++;}printf("sum=%f\n",sum);}6.2(5)#include<stdio.h>#include<math.h>main(){int n=1,count=1;float x;double sum,term;printf("Input x:");scanf("%f",&x);sum=x;term=x;do{term=-term*x*x/((n+1)*(n+2));sum=sum+term;n=n+2;count++;}while(fabs(term)>=1e-5);printf("sin(x)=%f,count=%d\n",sum,count);}6.3#include<stdio.h>main(){int x=1,find=0;while(!find){if(x%2==1&&x%3==2&&x%5==4&&x%6==5&&x%7==0){printf("x=%d\n",x);find=1;x++;}}}/*int x,find=0;for(x=1;!find;x++){if(x%2==1&&x%3==2&&x%5==4&&x%6==5&&x%7==0){printf("x=%d\n",x);find=1;}}}*/6.4#include<stdio.h>main(){int i,n;long p=1,m=1;printf("Input n:");scanf("%d",&n);for(i=1;i<=n;i++){p=i*i;m=i*i*i;printf("p=%d,m=%d\n",i,p,i,m);}}6.5#include<stdio.h>main(){float c,f;for(c=-40;c<=110;c=c+10){f=9/5*c+32;printf("f=%f\n",f);}}6.6#include<stdio.h>#include<math.h>main(){int n;double c=0.01875,x;do{x=x*pow(1+c,12)-1000;n++;}while(x>0);printf("x=%d\n",x);}6.7#include<stdio.h>main(){int n=0;float a=100.0,c;printf("Inputc:");scanf("%f",&c);do{a=a*(1+c);n++;}while(a<=200);printf("n=%d\n",n);}6.8#include<stdio.h>#include<math.h>main(){int n=1,count=1;double sum=1,term=1;while(fabs(term)>=1e-5){term=pow(-1,count)*(1.0/(n+2));sum=sum+term;n=n+2;count++;}sum=4*sum;printf("sum=%f,count=%d\n",sum,count); }6.9#include<stdio.h>#include<math.h>main(){int n=1,count=1;double sum=1,term=1;while(fabs(term)>=1e-5){term=term*(1.0/n);sum=sum+term;n++;count++;}printf("sum=%f,count=%d\n",sum,count);}6.10#include<stdio.h>#include<math.h>main(){int x;for(x=100;x<=999;x++){if(x==pow(x/100,3)+pow(x/10%10,3)+pow(x%10,3))printf("x=%d\n",x);}}6.11#include<stdio.h>main(){int i=0,n;long sum=0,term=1;printf("Inputn:");scanf("%d",&n);do{i++;term=term*i;sum=sum+term;}while(sum<n);printf("%d\n",i-1);}6.12#include<stdio.h>main(){int i,n,m,count=0,sum=0;printf("Input n:");scanf("%d",&n);for(i=1;i<=n;i++){printf("Inputm:");scanf("%d",&m);if(m>0){sum=sum+m;count++;}else{break;}printf("sum=%d,count=%d\n",sum,count);}}6.13#include<stdio.h>main(){int i,n,m,count=0,sum=0;printf("Inputn:");scanf("%d",&n);for(i=1;i<=n;i++){printf("Inputm:");scanf("%d",&m);if(m>0||m<0){sum=sum+m;count++;}else{break;}printf("sum=%d,count=%d\n",sum,count);}}6.14#include<stdio.h>main(){int x,y,z;for(x=0;x<=17;x++){for(y=0;y<=25;y++){3*x+2*y+z==50;z=30-x-y;if(3*x+2*y+z==50&&x+y+z==30)printf("x=%d,y=%d,z=%d\n",x,y,z);}}}6.15#include<stdio.h>main(){int x,y;for(x=0;x<=98;x++){y=98-x;2*x+4*y==386;if(x+y==98&&2*x+4*y==386){printf("x=%d,y=%d\n",x,y);}}}6.16#include<stdio.h>main(){int x,y,z;for(x=0;x<=20;x++){for(y=0;y<=33;y++){3*y+5*x+z/3.0==100;z=100-x-y;if(5*x+3*y+z/3.0==100&&z+x+y==100){printf("x=%d,y=%d,z=%d\n",x,y,z);}}}}6.17#include<stdio.h>main(){int x,y,z;for(x=1;x<=9;x++){for(y=1;y<=17;y++){10*x+5*y+z==100;z=50-x-y;if(10*x+5*y+z==100&&x+y+z==50&&z>0){printf("x=%d,y=%d,z=%d\n",x,y,z);}}}}7.1#include<stdio.h>int Square(int i){return i*i;}int main(){int i=0;i=Square(i);for(;i<3;i++){static int i=1;i+=Square(i);printf("%d,",i);}printf("%d\n",i);return0;}7.2#include<stdio.h>int hour,minute,second;void update(){second++;if(second==60){second=0;minute++;}if(minute==60){minute=0;hour++;}if(hour==24)hour=0;}void display(){printf("%d,%d,%d\n",hour,minute,second); }void delay(){int t;for(t=0;t<100000000;t++);}int main(){int i;void updaye(),display(),delay();for(i=0;i<1000000;i++){update();display();delay();}return0;}7.3#include<stdio.h>int GetMax(int a,int b);int main(){int x,y,max;printf("Inputx,y:");scanf("%d,%d",&x,&y);max=GetMax(x,y);printf("max=%d\n",max);return0;}int GetMax(int m,int n){if(m>=n)return m;elsereturn n;}7.4#include<stdio.h>int LCM(int n,int m);int main(){int a,b;printf("Inputa,b:");scanf("%d,%d",&a,&b);printf("%d\n",LCM(a,b));return0;}int LCM(int n,int m){int x;int find=0;for(x=1;!find;x++){if(x%n==0&&x%m==0){find=1;}}return x-1;}7.5#include<stdio.h>long Fact(int n);int main(){int m,a;printf("Inputm:");scanf("%d",&m);for(a=1;a<=m;a++){printf("%d!=%ld\n",a,Fact(a));}return0;}long Fact(int n){int i;long result=1;for(i=2;i<=n;i++)result*=i;return result;}7.6#include<stdio.h>long Fact(int n);int main(){int m;long ret;printf("Inputm:");scanf("%d",&m);ret=Fact(m);printf("ret=%d\n",ret);return0;}long Fact(int n){int i;long result=1,sum=0;for(i=2;i<=n;i++){result*=i;sum=sum+result;}return sum;}7.7(1)#include<stdio.h>int Gcd(int a,int b);int main(){int m,n;printf("Inputm,n:");scanf("%d,%d",&m,&n);printf("%d\n",Gcd(m,n));return0;}int Gcd(int a,int b){int t,min,find=0;min=a<b?a:b;t=min;for(t=min;!find;t--){a%t==0;b%t==0;if(a%t==0&&b%t==0)return t;}find=1;}7.7(2)#include<stdio.h> int Gcd(int a,int b);int main(){int m,n;printf("Inputm,n:");scanf("%d,%d",&m,&n);printf("%d\n",Gcd(m,n));return0;}int Gcd(int a,int b){int r,temp;r=a%b;if(r==0)return b;elsedo{temp=b;b=r;a=temp;r=a%b;}while(r!=0);return b;}8.1(1)#include<stdio.h> void Func(int x){x=2;}int main(){int x=10;Func(x);printf("%d",x);return0;}8.1(2)#include<stdio.h> void Func(int b[]){int j;for(j=0;j<4;j++){b[j]=j;}}int main(){static int a[]={5,6,7,8},i;Func(a);for(i=0;i<4;i++){printf("%d",a[i]);}return0;}8.2(1)int PositiveNum(int a[],int n) {int i,count=0;for(i=0;i<n;i++){if(a[i]>0)count++;}return0;}8.2(2)void Fib(long f[],long n){int i;f[0]=0;f[1]=1;for(i=2;i<n;i++){f[i]=f[i-1]+f[i-2];}}8.2(3)#include<stdio.h>int main(){int a[10],n,max,min,maxPos,minPos;for(n=0;n<10;n++){scanf("%d",&a[n]);}max=min=a[0];maxPos=minPos=0;for(n=0;n<10;n++){if(a[n]>max){max=a[n];maxPos=n;}else if(a[n]<min){min=a[n];minPos=n;}}printf("max=%d,pos=%d\n",max,maxPos);printf("min=%d,pos=%d\n",min,minPos);return0;}8.3void DivArray(int*pArray,int n){int i;for(i=0;i<n;i++){pArray[i]/=pAttay[0];}}8.4#include<stdio.h>#define N40int FailNum(int score[],int n);void ReadScore(int score[],int n);int main(){int score[N],n;printf("Input n:");scanf("%d",&n);ReadScore(score,n);FailNum(score,n);printf("FailNum students are%d\n",FailNum(score,n));return0;}int FailNum(int score[],int n){int i,count=0;for(i=0;i<n;i++){if(score[i]<60)count++;}return count;}void ReadScore(int score[],int n){int i;printf("Input score:");for(i=0;i<n;i++){scanf("%d",&score[i]);}}8.5#include<stdio.h>#define N40int HighAver(int score[],int n);int ReadScore(int score[]);int main(){int score[N],n;n=ReadScore(score);printf("HighAver students are%d\n",HighAver(score,n));return0;}int HighAver(int score[],int n){int i,count=0,sum=0,aver;for(i=0;i<n;i++){sum=sum+score[i];aver=sum/n;}for(i=0;i<n;i++){if(score[i]>aver)count++;}return count;}int ReadScore(int score[]){int i=-1;do{i++;printf("Input score:");scanf("%d",&score[i]);}while(score[i]>=0);return i;}8.6#include<stdio.h>#define N40int ReadScore(int score[],long num[]);int FindMax(int score[],long num[],int n);int main(){int score[N],n,max,num,t;long num[N];t=FindMax(score,n);max=score[t];num=num[t];printf("max=%d,num=%d\n",max,num);return0;}int ReadScore(int score[],long num[]){int i=-1;do{i++;printf("Input student's ID and score:");scanf("%ld%d",&num[i],&score[i]);}while(num[i]>0&&score[i]>=0);return i;}int FindMax(int score[],long num[],int n){int max,i;max=score[0];for(i=1;i<n;i++){if(score[i]>max)max=score[i];}return i;}8.7#include<stdio.h>#define N40int Read(int a[]);int ChangeArry(int a[],int n);int main(){int a[N],n;printf("%d\n",ChangeArry(a,n));return0;}int Read(int a[]){int i;for(i=0;i<9;i++){printf("Input a:");scanf("%d",&a[i]);return i;}int ChangeArry(int a[],int n){int max,min,i,maxpos,minpos,term;max=min=score[0];for(i=1;i<n;i++){if(a[i]>max)max=score[i];maxpos=n;else(a[i]<min)min=a[i];minpos=n;temp=maxpos;maxpos=minpos;minpos=temp;}return a[i];}8.10#include<stdio.h>#define N5void main(){int a[N][N];int sum=0;int i,j;printf("请输入一个%d*%d的矩阵\n",N,N);for(i=0;i<N;i++){for(j=0;j<N;j++){scanf("%d",&a[i][j]);}}for(i=0;i<N;i++){for(j=0;j<N;j++){if(i==j||i+j==N-1)sum=sum+a[i][j];}}printf("对角线元素之和为:%d\n",sum);}8.11#include<stdio.h>#define N2#define M3void main(){int a[N][M],b[N][M];int i,j,sum1=0,sum2=0,sum=0;printf("请输入一个%d*%d的矩阵\n",N,M);for(i=0;i<N;i++){for(j=0;j<M;j++){scanf("%d",&a[i][j]);sum1=sum1+a[i][j];}}printf("请输入一个%d*%d的矩阵\n",N,M);for(i=0;i<N;i++){for(j=0;j<M;j++){scanf("%d",&b[i][j]);sum2=sum2+a[i][j];}}sum=sum1+sum2;printf("sum=%d\n",sum);}8.12#include<stdio.h>#define N7void YangHui(int a[N][N],int n);void main(){int i,j,n,b[N][N];printf("Input n:");scanf("%d",&n);YangHui(b,n);for(i=0;i<n;i++){for(j=0;j<=i;j++){printf("%4d",b[i][j]);}printf("\n");}}void YangHui(int a[N][N],int n){int i,j;for(i=0;i<n;i++){a[i][0]=1;a[i][i]=1;}for(i=2;i<n;i++){for(j=1;j<i;j++)a[i][j]=a[i-1][j-1]+a[i-1][j];}}8.13#include<stdio.h>#define N12void main(){int i;int f[N]={1,1};printf("%d:%d\n",0,f[0]);printf("%d:%d\n",1,f[1]);for(i=2;i<N;i++){f[i]=f[i-1]+f[i-2];printf("%d:%d\n",i+1,f[i]);}}8.14#include<stdio.h>#include<stdlib.h>#include<time.h>#define N6000void main(){int a[N],i;int b1=0,b2=0,b3=0,b4=0,b5=0,b6=0;float p1,p2,p3,p4,p5,p6;srand(time(NULL));for(i=0;i<N;i++){a[i]=rand()%6+1;printf("%3d",a[i]);if(a[i]==1)b1++;if(a[i]==2)b2++;if(a[i]==3)b3++;if(a[i]==4)b4++;if(a[i]==5)b5++;if(a[i]==6)b6++;p1=(float)(b1/6000.0);p2=(float)(b2/6000.0);p3=(float)(b3/6000.0);p4=(float)(b4/6000.0);p5=(float)(b5/6000.0);p6=(float)(b6/6000.0);}printf("骰子出现1的概率:%.2f\n",p1);printf("骰子出现2的概率:%.2f\n",p2);printf("骰子出现3的概率:%.2f\n",p3);printf("骰子出现4的概率:%.2f\n",p4);printf("骰子出现5的概率:%.2f\n",p5);printf("骰子出现6的概率:%.2f\n",p6);}9.1(1)void Swap(int*x,int*y){int*pTemp;//*pTemp未进行初始化,*pTemp指向哪里位置*pTemp=*x;*x=*y;*y=*pTemp;}9.1(2)void Swap(int*x,int*y){int*pTemp;pTemp=x;x=y;y=pTemp;}//错误,x,y没有指向类型9.2#include<stdio.h>int main(){int a[]={1,2,3,4,5};int*p=a;printf("%d,%d,%d,%d,%d,%d,%d\n",*p,*(++p),(*p)++,*p,*p--,--(*p),*p);return0;}9.3#include<stdio.h>void Swap(int*x,int*y);int main(){int a[6]={1,2,3,4,5,6},b[6]={11,22,33,44,55,66};int i;printf("Before Array\n");for(i=0;i<6;i++){printf("%d\n",a[i]);}for(i=0;i<6;i++){printf("%d\n",b[i]);}for(i=0;i<6;i++){Swap(&a[i],&b[i]);}printf("After Array\n");for(i=0;i<6;i++){printf("%d\n",a[i]);}for(i=0;i<6;i++){printf("%d\n",b[i]);}return0;}void Swap(int*x,int*y){int temp;temp=*x;*x=*y;*y=temp;}9.4#include<stdio.h>#define N10void FindMaxMin(int a[],int*pMaxa,int*pMaxnum,int*pMina,int*pMinnum);int main(){int a[N];int i,maxa,mina,maxnum,minnum;printf("Input a:");for(i=0;i<10;i++){scanf("%d",&a[i]);}FindMaxMin(a,&maxa,&maxnum,&mina,&minnum);printf("maxa=%d,maxnum=%d,mina=%d,minnum=%d\n",maxa,maxnum,mina,minnum);return0;}void FindMaxMin(int a[],int*pMaxa,int*pMaxnum,int*pMina,int*pMinnum){int i;*pMaxa=a[0];*pMina=a[0];*pMaxnum=0;*pMinnum=0;for(i=0;i<10;i++){if(a[i]>*pMaxa){*pMaxa=a[i];*pMaxnum=i;}else if(a[i]<*pMina){*pMina=a[i];*pMinnum=i;}}}10.1void MYStrcpy(char*dstStr,const char*srcStr){while((*dstStr++=*srcStr++)!='\0'){}}10.2#include<stdio.h>main(){char*a="main(){char*a=%c%s%c;printf(a,34,a,34);}";printf(a,34,a,34);} 10.3(1)unsigned int MyStrlen(char*p)main(){unsigned int len;len=0;for(;*pa!='\0';pa++){len++;}return len;}10.3(2)unsigned int MyStrlen(char s[]){char*p=s;while(*p!='\0'){p++;}return(p-*p);}10.3(3)int MyStrcmp(char*p1,char*p2){for(;*p1==*p2;p1++,p2++){if(*p1=='\0')return-1;}return1;}10.3(4)int MyStrcmp(char s[],char t[]){int i;for(i=0;s[i]==t[i];i++){if(s[i]=='\0')return0;}return i;}10.3(5)#include<stdio.h>#include<string.h>int main(){char password[7]="secret";char userInput[81];printf("Input Password:");scanf("%s",userInput);if(strcmp(userInput,password)==0)printf("Coorrect password!Welcome to the system...\n");else if(strcmp(userInput,password)<0)printf("Invalid password!user input<password...\n");elseprintf("Invalid password!user input>password...\n");return0;}10.4#include<stdio.h>#define N24unsigned int CountLetter(char str[]);int main(){char a[N];printf("Input a letter:\n");gets(a);printf("The length of the letter is:%d\n",CountLetter(a));return0;}unsigned int CountLetter(char str[]){char*p=str;int c=0,flag=0;while(*p!='\0'){if(*p!='')flag=1;else if(flag==1){c++;flag=0;}p++;}return c+1;}12.1#include<stdio.h>typedef struct data{int year;int month;int day;}DATA;typedef struct work{char a[14];char b[10];char c[6];}WORK;typedef struct student{char studentName[10];char studentSex;DATA birthday;WORK profession;}STUDENT;int main(){STUDENT stu={"王刚",'M',{1991,5,19},{"信息工程系","学习人员","学生"}};printf("stu:%2s%3c%6d/%02d/%02d%4s,%4s,%4s\n",stu.studentName,stu.studentSex,st u.birthday.year,stu.birthday.month,stu.birthday.day,stu.profession.a,stu.profession.b,stu.profession.c);return0;}12.4#include<stdio.h>#include<string.h>#define N10typedef struct people{char peopleName[10];}PEOPLE;int main(){int sum1=0,sum2=0,sum3=0,sum4=0;int i;char name[N][N];PEOPLE stu[5]={"zhang","li","wang"};printf("Enter their name:\n");for(i=0;i<10;i++){gets(name[i]);if(strcmp(name[i],stu[0].peopleName)==0)sum1++;else if(strcmp(name[i],stu[1].peopleName)==0) sum2++;else if(strcmp(name[i],stu[2].peopleName)==0) sum3++;elsesum4++;}printf("stu:%4s%2d\n",stu[i].peopleName,sum[j]);printf("stu:%4s%2d\n",stu[1].peopleName,sum2);printf("stu:%4s%2d\n",stu[2].peopleName,sum3);printf("%2d\n",sum4);return0;}。
最新《C语言程序设计》(苏小红) 课后习题答案 高等教育出版社资料

2.2 #include<stdio.h>main(){float x=2.5,y=2.5,z=2.5;printf("x=%f\n",x);printf("y=%f\n",y);printf("z=%f\n",z);}3.1(1)#include<stdio.h>main(){int a=12,b=3;float x=18.5,y=4.6;printf("%f\n",(float)(a*b)/2);printf("%d\n",(int)x%(int)y);}3.1(2)#include<stdio.h>main(){int x=32,y=81,p,q;p=x++;q=--y;printf("%d %d\n",p,q); printf("%d %d\n",x,y); }3.2#include<stdio.h>main(){int x,b0,b1,b2,s;printf("Inputx:");scanf("%d",&x);b2=x/100;b1=(x-b2*100)/10;//或(x%100)/10;或x/10%10;b0=x%10;s=b0*100+b1*10+b2;printf("s=%d\n",s);}3.3#include<stdio.h>#include<math.h>main(){float rate=0.0225;float n,capital,deposit;printf("Input n,capital:");scanf("%f,%f",&n,&capital);deposit=capital*pow(1+rate,n);printf("deposit=%f\n",deposit);}3.4#include<stdio.h>#include<math.h>main(){float a, b, c;double x, y;printf("Input a, b, c:");scanf("%f %f %f", &a, &b, &c);x=(-b+sqrt(b*b-4*a*c))/(2*a);y=(-b-sqrt(b*b-4*a*c))/(2*a);printf("x=%f,y=%f\n",x,y);}习题4 Array 4.1(1)#include<stdio.h>main(){char c1='a',c2='b',c3='c';printf("a%cb%cc%c\n",c1,c2,c3);}4.1(2)main(){int a=12,b=15;printf("a=%d%%,b=%d%%\n",a,b);}#include<stdio.h>main(){int a,b;printf("%d,%d\n",a,b);}4.2#include<stdio.h>main(){long a,b;float x,y;scanf("%d,%d\n",&a,&b);scanf("%f,%f\n",&x,&y);printf("a=%d,b=%d\n",a,b);printf("x=%f,b=%f\n",x,y); }5.1#include<stdio.h>main(){float a;printf("Input a:");scanf("%f",&a);if(a>=0){a=a;printf("a=%f\n",a);}else{a=-a;printf("a=%f\n",a);}}5.2#include<stdio.h>main(){int a;printf("Input a:");scanf("%d", &a);if(a%2==0)printf("a是偶数");}else{printf("a是奇数");}}5.3#include<stdio.h>#include<math.h>main(){float a,b,c,s,area;printf("Input a, b, c:");scanf("%f %f %f", &a, &b, &c);if(a+b>c&&a+c>b&&b+c>a){s=(a+b+c)/2;area=(float)sqrt(s*(s-a)*(s-b)*(s-c));printf("area=%f\n",area);}else{printf("不是三角形");}}5.4#include<stdio.h>#include<math.h>main(){float a,b,c,x,y;printf("Inputa,b,c:");scanf("%f,%f,%f",&a,&b,&c);if(a==0){printf("该方程不是一元二次方程\n");}if(b*b-4*a*c>0){x=(-b+sqrt(b*b-4*a*c))/(2*a);y=(-b-sqrt(b*b-4*a*c))/(2*a);printf("x=%f,y=%f\n",x,y);}else if(b*b-4*a*c==0)x=-b/(2*a);y=-b/(2*a);printf("x=%f,y=%f\n",x,y);}else{printf("该方程无实根\n");}}5.5#include<stdio.h>main(){int year,flag;printf("Input a year:");scanf("%d",&year);if(year%4==0&&year%400!=0||year%400==0){flag=1;}else{flag=0;}if(flag==1){printf("%d is a leap year!\n",year);}else{printf("%d is not a leap year!\n",year);}}5.6#include<stdio.h>main(){int year,flag;printf("Input a year:");scanf("%d",&year);flag=year%400==0||year%4==0&&year%100!=0?1:0;if(flag==1&&flag!=0){printf("%d is a leap year!\n",year);}else{printf("%d is not a leap year!\n",year);}}5.7#include<stdio.h>main(){char ch;printf("Inputch:");scanf("%c",&ch);if(ch>='a'&&ch<='z'){ch=getchar();ch=ch-32;printf("%c,%d\n",ch,ch);}else if(ch>='A'&&ch<='Z'){ch=getchar();ch=ch+32;printf("%c,%d\n",ch,ch);}else{printf("%c",ch);}}5.8#include<stdio.h>main(){char ch;printf("Inputch:");scanf("%c",&ch);if(ch>=48&&ch<=57){printf("ch是数字字符\n");}else if(ch>=65&&ch<=90){printf("ch是大写字母\n");}else if(ch>=97&&ch<=122){printf("ch是小写字母\n");}else if(ch==32){printf("ch是空格\n");}else{printf("ch是其他字符\n");}}5.9#include<stdio.h>main(){int score,grade;printf("Input score:");scanf("%d",&score);grade=score/10;if(score<0||score>100){printf("Input error\n");}if(score>=90&&score<=100){printf("%d--A\n",score);}else if(score>=80&&score<90){printf("%d--B\n",score);}else if(score>=70&&score<80){printf("%d--C\n",score);}else if(score>=60&&score<70){printf("%d--D\n",score);}else if(score>=0&&score<60){printf("%d--E\n",score);}}5.10#include<stdio.h>main(){int year,month;printf("Input year,month:");scanf("%d,%d",&year,&month);if(month>12||month<=0){printf("error month\n");}else{switch(year,month){case12:case10:case8:case7:case5:case3:case1:printf("31天\n");break;case11:case9:case6:case4:printf("30天\n");break;case2:if(year%4==0&&year!=0||year%400==0){printf("29天\n");}else{printf("28天\n");}break;default:printf("Input error\n");}}}6.1(1)#include<stdio.h>main(){int i,j,k;char space='';for(i=1;i<=4;i++){for(j=1;j<=i;j++){printf("%c",space);}for(k=1;k<=6;k++){printf("*");}printf("\n");}}6.1(2)#include<stdio.h>main(){int k=4,n;for(n=0;n<k;n++){if(n%2==0)continue;k--;}printf("k=%d\n,n=%d\n",k,n);}6.1(3)#include<stdio.h>main(){int k=4,n;for(n=0;n<k;n++){if(n%2==0)break;k--;}printf("k=%d,n=%d\n",k,n);}6.2(1)#include<stdio.h>main(){int i,sum=0;for(i=1;i<=101;i++){sum=sum+i;}printf("sum=%d\n",sum);}6.2(2)#include<stdio.h>main(){long i;long term,sum=0;for(i=1;i<=101;i=i+2){term=i*(i+1)*(i+2);sum=sum+term;}printf("sum=%ld\n",sum);}6.2(4)#include<stdio.h>#include<math.h>main(){int n=1;float term=1.0,sign=1,sum=0;while(term<=-1e-4||term>=1e-4){term=1.0/sign;sum=sum+term;sign=sign+n;n++;}printf("sum=%f\n",sum);}6.2(5)#include<stdio.h>#include<math.h>main(){int n=1,count=1;float x;double sum,term;printf("Input x:");scanf("%f",&x);sum=x;term=x;do{term=-term*x*x/((n+1)*(n+2));sum=sum+term;n=n+2;count++;}while(fabs(term)>=1e-5);printf("sin(x)=%f,count=%d\n",sum,count);}6.3#include<stdio.h>main(){int x=1,find=0;while(!find){if(x%2==1&&x%3==2&&x%5==4&&x%6==5&&x%7==0){printf("x=%d\n",x);find=1;x++;}}}/*int x,find=0;for(x=1;!find;x++){if(x%2==1&&x%3==2&&x%5==4&&x%6==5&&x%7==0){printf("x=%d\n",x);find=1;}}}*/6.4#include<stdio.h>main(){int i,n;long p=1,m=1;printf("Input n:");scanf("%d",&n);for(i=1;i<=n;i++){p=i*i;m=i*i*i;printf("p=%d,m=%d\n",i,p,i,m);}}6.5#include<stdio.h>main(){float c,f;for(c=-40;c<=110;c=c+10){f=9/5*c+32;printf("f=%f\n",f);}}6.6#include<stdio.h>#include<math.h>main(){int n;double c=0.01875,x;do{x=x*pow(1+c,12)-1000;n++;}while(x>0);printf("x=%d\n",x);}6.7#include<stdio.h>main(){int n=0;float a=100.0,c;printf("Inputc:");scanf("%f",&c);do{a=a*(1+c);n++;}while(a<=200);printf("n=%d\n",n);}6.8#include<stdio.h>#include<math.h>main(){int n=1,count=1;double sum=1,term=1;while(fabs(term)>=1e-5){term=pow(-1,count)*(1.0/(n+2));sum=sum+term;n=n+2;count++;}sum=4*sum;printf("sum=%f,count=%d\n",sum,count); }6.9#include<stdio.h>#include<math.h>main(){int n=1,count=1;double sum=1,term=1;while(fabs(term)>=1e-5){term=term*(1.0/n);sum=sum+term;n++;count++;}printf("sum=%f,count=%d\n",sum,count);}6.10#include<stdio.h>#include<math.h>main(){int x;for(x=100;x<=999;x++){if(x==pow(x/100,3)+pow(x/10%10,3)+pow(x%10,3))printf("x=%d\n",x);}}6.11#include<stdio.h>main(){int i=0,n;long sum=0,term=1;printf("Inputn:");scanf("%d",&n);do{i++;term=term*i;sum=sum+term;}while(sum<n);printf("%d\n",i-1);}6.12#include<stdio.h>main(){int i,n,m,count=0,sum=0;printf("Input n:");scanf("%d",&n);for(i=1;i<=n;i++){printf("Inputm:");scanf("%d",&m);if(m>0){sum=sum+m;count++;}else{break;}printf("sum=%d,count=%d\n",sum,count);}}6.13#include<stdio.h>main(){int i,n,m,count=0,sum=0;printf("Inputn:");scanf("%d",&n);for(i=1;i<=n;i++){printf("Inputm:");scanf("%d",&m);if(m>0||m<0){sum=sum+m;count++;}else{break;}printf("sum=%d,count=%d\n",sum,count);}}6.14#include<stdio.h>main(){int x,y,z;for(x=0;x<=17;x++){for(y=0;y<=25;y++){3*x+2*y+z==50;z=30-x-y;if(3*x+2*y+z==50&&x+y+z==30)printf("x=%d,y=%d,z=%d\n",x,y,z);}}}6.15#include<stdio.h>main(){int x,y;for(x=0;x<=98;x++){y=98-x;2*x+4*y==386;if(x+y==98&&2*x+4*y==386){printf("x=%d,y=%d\n",x,y);}}}6.16#include<stdio.h>main(){int x,y,z;for(x=0;x<=20;x++){for(y=0;y<=33;y++){3*y+5*x+z/3.0==100;z=100-x-y;if(5*x+3*y+z/3.0==100&&z+x+y==100){printf("x=%d,y=%d,z=%d\n",x,y,z);}}}}6.17#include<stdio.h>main(){int x,y,z;for(x=1;x<=9;x++){for(y=1;y<=17;y++){10*x+5*y+z==100;z=50-x-y;if(10*x+5*y+z==100&&x+y+z==50&&z>0){printf("x=%d,y=%d,z=%d\n",x,y,z);}}}}7.1#include<stdio.h>int Square(int i){return i*i;}int main(){int i=0;i=Square(i);for(;i<3;i++){static int i=1;i+=Square(i);printf("%d,",i);}printf("%d\n",i);return0;}7.2#include<stdio.h>int hour,minute,second;void update(){second++;if(second==60){second=0;minute++;}if(minute==60){minute=0;hour++;}if(hour==24)hour=0;}void display(){printf("%d,%d,%d\n",hour,minute,second); }void delay(){int t;for(t=0;t<100000000;t++);}int main(){int i;void updaye(),display(),delay();for(i=0;i<1000000;i++){update();display();delay();}return0;}7.3#include<stdio.h>int GetMax(int a,int b);int main(){int x,y,max;printf("Inputx,y:");scanf("%d,%d",&x,&y);max=GetMax(x,y);printf("max=%d\n",max);return0;}int GetMax(int m,int n){if(m>=n)return m;elsereturn n;}7.4#include<stdio.h>int LCM(int n,int m);int main(){int a,b;printf("Inputa,b:");scanf("%d,%d",&a,&b);printf("%d\n",LCM(a,b));return0;}int LCM(int n,int m){int x;int find=0;for(x=1;!find;x++){if(x%n==0&&x%m==0){find=1;}}return x-1;}7.5#include<stdio.h>long Fact(int n);int main(){int m,a;printf("Inputm:");scanf("%d",&m);for(a=1;a<=m;a++){printf("%d!=%ld\n",a,Fact(a));}return0;}long Fact(int n){int i;long result=1;for(i=2;i<=n;i++)result*=i;return result;}7.6#include<stdio.h>long Fact(int n);int main(){int m;long ret;printf("Inputm:");scanf("%d",&m);ret=Fact(m);printf("ret=%d\n",ret);return0;}long Fact(int n){int i;long result=1,sum=0;for(i=2;i<=n;i++){result*=i;sum=sum+result;}return sum;}7.7(1)#include<stdio.h>int Gcd(int a,int b);int main(){int m,n;printf("Inputm,n:");scanf("%d,%d",&m,&n);printf("%d\n",Gcd(m,n));return0;}int Gcd(int a,int b){int t,min,find=0;min=a<b?a:b;t=min;for(t=min;!find;t--){a%t==0;b%t==0;if(a%t==0&&b%t==0)return t;}find=1;}7.7(2)#include<stdio.h> int Gcd(int a,int b);int main(){int m,n;printf("Inputm,n:");scanf("%d,%d",&m,&n);printf("%d\n",Gcd(m,n));return0;}int Gcd(int a,int b){int r,temp;r=a%b;if(r==0)return b;elsedo{temp=b;b=r;a=temp;r=a%b;}while(r!=0);return b;}8.1(1)#include<stdio.h> void Func(int x){x=2;}int main(){int x=10;Func(x);printf("%d",x);return0;}8.1(2)#include<stdio.h> void Func(int b[]){int j;for(j=0;j<4;j++){b[j]=j;}}int main(){static int a[]={5,6,7,8},i;Func(a);for(i=0;i<4;i++){printf("%d",a[i]);}return0;}8.2(1)int PositiveNum(int a[],int n) {int i,count=0;for(i=0;i<n;i++){if(a[i]>0)count++;}return0;}8.2(2)void Fib(long f[],long n){int i;f[0]=0;f[1]=1;for(i=2;i<n;i++){f[i]=f[i-1]+f[i-2];}}8.2(3)#include<stdio.h>int main(){int a[10],n,max,min,maxPos,minPos;for(n=0;n<10;n++){scanf("%d",&a[n]);}max=min=a[0];maxPos=minPos=0;for(n=0;n<10;n++){if(a[n]>max){max=a[n];maxPos=n;}else if(a[n]<min){min=a[n];minPos=n;}}printf("max=%d,pos=%d\n",max,maxPos);printf("min=%d,pos=%d\n",min,minPos);return0;}8.3void DivArray(int*pArray,int n){int i;for(i=0;i<n;i++){pArray[i]/=pAttay[0];}}8.4#include<stdio.h>#define N40int FailNum(int score[],int n);void ReadScore(int score[],int n);int main(){int score[N],n;printf("Input n:");scanf("%d",&n);ReadScore(score,n);FailNum(score,n);printf("FailNum students are%d\n",FailNum(score,n));return0;}int FailNum(int score[],int n){int i,count=0;for(i=0;i<n;i++){if(score[i]<60)count++;}return count;}void ReadScore(int score[],int n){int i;printf("Input score:");for(i=0;i<n;i++){scanf("%d",&score[i]);}}8.5#include<stdio.h>#define N40int HighAver(int score[],int n);int ReadScore(int score[]);int main(){int score[N],n;n=ReadScore(score);printf("HighAver students are%d\n",HighAver(score,n));return0;}int HighAver(int score[],int n){int i,count=0,sum=0,aver;for(i=0;i<n;i++){sum=sum+score[i];aver=sum/n;}for(i=0;i<n;i++){if(score[i]>aver)count++;}return count;}int ReadScore(int score[]){int i=-1;do{i++;printf("Input score:");scanf("%d",&score[i]);}while(score[i]>=0);return i;}8.6#include<stdio.h>#define N40int ReadScore(int score[],long num[]);int FindMax(int score[],long num[],int n);int main(){int score[N],n,max,num,t;long num[N];t=FindMax(score,n);max=score[t];num=num[t];printf("max=%d,num=%d\n",max,num);return0;}int ReadScore(int score[],long num[]){int i=-1;do{i++;printf("Input student's ID and score:");scanf("%ld%d",&num[i],&score[i]);}while(num[i]>0&&score[i]>=0);return i;}int FindMax(int score[],long num[],int n){int max,i;max=score[0];for(i=1;i<n;i++){if(score[i]>max)max=score[i];}return i;}8.7#include<stdio.h>#define N40int Read(int a[]);int ChangeArry(int a[],int n);int main(){int a[N],n;printf("%d\n",ChangeArry(a,n));return0;}int Read(int a[]){int i;for(i=0;i<9;i++){printf("Input a:");scanf("%d",&a[i]);return i;}int ChangeArry(int a[],int n){int max,min,i,maxpos,minpos,term;max=min=score[0];for(i=1;i<n;i++){if(a[i]>max)max=score[i];maxpos=n;else(a[i]<min)min=a[i];minpos=n;temp=maxpos;maxpos=minpos;minpos=temp;}return a[i];}8.10#include<stdio.h>#define N5void main(){int a[N][N];int sum=0;int i,j;printf("请输入一个%d*%d的矩阵\n",N,N);for(i=0;i<N;i++){for(j=0;j<N;j++){scanf("%d",&a[i][j]);}}for(i=0;i<N;i++){for(j=0;j<N;j++){if(i==j||i+j==N-1)sum=sum+a[i][j];}}printf("对角线元素之和为:%d\n",sum);}8.11#include<stdio.h>#define N2#define M3void main(){int a[N][M],b[N][M];int i,j,sum1=0,sum2=0,sum=0;printf("请输入一个%d*%d的矩阵\n",N,M);for(i=0;i<N;i++){for(j=0;j<M;j++){scanf("%d",&a[i][j]);sum1=sum1+a[i][j];}}printf("请输入一个%d*%d的矩阵\n",N,M);for(i=0;i<N;i++){for(j=0;j<M;j++){scanf("%d",&b[i][j]);sum2=sum2+a[i][j];}}sum=sum1+sum2;printf("sum=%d\n",sum);}8.12#include<stdio.h>#define N7void YangHui(int a[N][N],int n);void main(){int i,j,n,b[N][N];printf("Input n:");scanf("%d",&n);YangHui(b,n);for(i=0;i<n;i++){for(j=0;j<=i;j++){printf("%4d",b[i][j]);}printf("\n");}}void YangHui(int a[N][N],int n){int i,j;for(i=0;i<n;i++){a[i][0]=1;a[i][i]=1;}for(i=2;i<n;i++){for(j=1;j<i;j++)a[i][j]=a[i-1][j-1]+a[i-1][j];}}8.13#include<stdio.h>#define N12void main(){int i;int f[N]={1,1};printf("%d:%d\n",0,f[0]);printf("%d:%d\n",1,f[1]);for(i=2;i<N;i++){f[i]=f[i-1]+f[i-2];printf("%d:%d\n",i+1,f[i]);}}8.14#include<stdio.h>#include<stdlib.h>#include<time.h>#define N6000void main(){int a[N],i;int b1=0,b2=0,b3=0,b4=0,b5=0,b6=0;float p1,p2,p3,p4,p5,p6;srand(time(NULL));for(i=0;i<N;i++){a[i]=rand()%6+1;printf("%3d",a[i]);if(a[i]==1)b1++;if(a[i]==2)b2++;if(a[i]==3)b3++;if(a[i]==4)b4++;if(a[i]==5)b5++;if(a[i]==6)b6++;p1=(float)(b1/6000.0);p2=(float)(b2/6000.0);p3=(float)(b3/6000.0);p4=(float)(b4/6000.0);p5=(float)(b5/6000.0);p6=(float)(b6/6000.0);}printf("骰子出现1的概率:%.2f\n",p1);printf("骰子出现2的概率:%.2f\n",p2);printf("骰子出现3的概率:%.2f\n",p3);printf("骰子出现4的概率:%.2f\n",p4);printf("骰子出现5的概率:%.2f\n",p5);printf("骰子出现6的概率:%.2f\n",p6);}9.1(1)void Swap(int*x,int*y){int*pTemp;//*pTemp未进行初始化,*pTemp指向哪里位置*pTemp=*x;*x=*y;*y=*pTemp;}9.1(2)void Swap(int*x,int*y){int*pTemp;pTemp=x;x=y;y=pTemp;}//错误,x,y没有指向类型9.2#include<stdio.h>int main(){int a[]={1,2,3,4,5};int*p=a;printf("%d,%d,%d,%d,%d,%d,%d\n",*p,*(++p),(*p)++,*p,*p--,--(*p),*p);return0;}9.3#include<stdio.h>void Swap(int*x,int*y);int main(){int a[6]={1,2,3,4,5,6},b[6]={11,22,33,44,55,66};int i;printf("Before Array\n");for(i=0;i<6;i++){printf("%d\n",a[i]);}for(i=0;i<6;i++){printf("%d\n",b[i]);}for(i=0;i<6;i++){Swap(&a[i],&b[i]);}printf("After Array\n");for(i=0;i<6;i++){printf("%d\n",a[i]);}for(i=0;i<6;i++){printf("%d\n",b[i]);}return0;}void Swap(int*x,int*y){int temp;temp=*x;*x=*y;*y=temp;}9.4#include<stdio.h>#define N10void FindMaxMin(int a[],int*pMaxa,int*pMaxnum,int*pMina,int*pMinnum);int main(){int a[N];int i,maxa,mina,maxnum,minnum;printf("Input a:");for(i=0;i<10;i++){scanf("%d",&a[i]);}FindMaxMin(a,&maxa,&maxnum,&mina,&minnum);printf("maxa=%d,maxnum=%d,mina=%d,minnum=%d\n",maxa,maxnum,mina,minnum);return0;}void FindMaxMin(int a[],int*pMaxa,int*pMaxnum,int*pMina,int*pMinnum){int i;*pMaxa=a[0];*pMina=a[0];*pMaxnum=0;*pMinnum=0;for(i=0;i<10;i++){if(a[i]>*pMaxa){*pMaxa=a[i];*pMaxnum=i;}else if(a[i]<*pMina){*pMina=a[i];*pMinnum=i;}}}10.1void MYStrcpy(char*dstStr,const char*srcStr){while((*dstStr++=*srcStr++)!='\0'){}}10.2#include<stdio.h>main(){char*a="main(){char*a=%c%s%c;printf(a,34,a,34);}";printf(a,34,a,34);} 10.3(1)unsigned int MyStrlen(char*p)main(){unsigned int len;len=0;for(;*pa!='\0';pa++){len++;}return len;}10.3(2)unsigned int MyStrlen(char s[]){char*p=s;while(*p!='\0'){p++;}return(p-*p);}10.3(3)int MyStrcmp(char*p1,char*p2){for(;*p1==*p2;p1++,p2++){if(*p1=='\0')return-1;}return1;}10.3(4)int MyStrcmp(char s[],char t[]){int i;for(i=0;s[i]==t[i];i++){if(s[i]=='\0')return0;}return i;}10.3(5)#include<stdio.h>#include<string.h>int main(){char password[7]="secret";char userInput[81];printf("Input Password:");scanf("%s",userInput);if(strcmp(userInput,password)==0)printf("Coorrect password!Welcome to the system...\n");else if(strcmp(userInput,password)<0)printf("Invalid password!user input<password...\n");elseprintf("Invalid password!user input>password...\n");return0;}10.4#include<stdio.h>#define N24unsigned int CountLetter(char str[]);int main(){char a[N];printf("Input a letter:\n");gets(a);printf("The length of the letter is:%d\n",CountLetter(a));return0;}unsigned int CountLetter(char str[]){char*p=str;int c=0,flag=0;while(*p!='\0'){if(*p!='')flag=1;else if(flag==1){c++;flag=0;}p++;}return c+1;}12.1#include<stdio.h>typedef struct data{int year;int month;int day;}DATA;typedef struct work{char a[14];char b[10];char c[6];}WORK;typedef struct student{char studentName[10];char studentSex;DATA birthday;WORK profession;}STUDENT;int main(){STUDENT stu={"王刚",'M',{1991,5,19},{"信息工程系","学习人员","学生"}};printf("stu:%2s%3c%6d/%02d/%02d%4s,%4s,%4s\n",stu.studentName,stu.studentSex,st u.birthday.year,stu.birthday.month,stu.birthday.day,stu.profession.a,stu.profession.b,stu.profession.c);return0;}12.4#include<stdio.h>#include<string.h>#define N10typedef struct people{char peopleName[10];}PEOPLE;int main(){int sum1=0,sum2=0,sum3=0,sum4=0;int i;char name[N][N];PEOPLE stu[5]={"zhang","li","wang"};printf("Enter their name:\n");for(i=0;i<10;i++){gets(name[i]);if(strcmp(name[i],stu[0].peopleName)==0)sum1++;else if(strcmp(name[i],stu[1].peopleName)==0) sum2++;else if(strcmp(name[i],stu[2].peopleName)==0) sum3++;elsesum4++;}printf("stu:%4s%2d\n",stu[i].peopleName,sum[j]);printf("stu:%4s%2d\n",stu[1].peopleName,sum2);printf("stu:%4s%2d\n",stu[2].peopleName,sum3);printf("%2d\n",sum4);return0;}精品文档。
《C语言程序设计》(苏小红) 课后习题参考答案 高等教育出版社
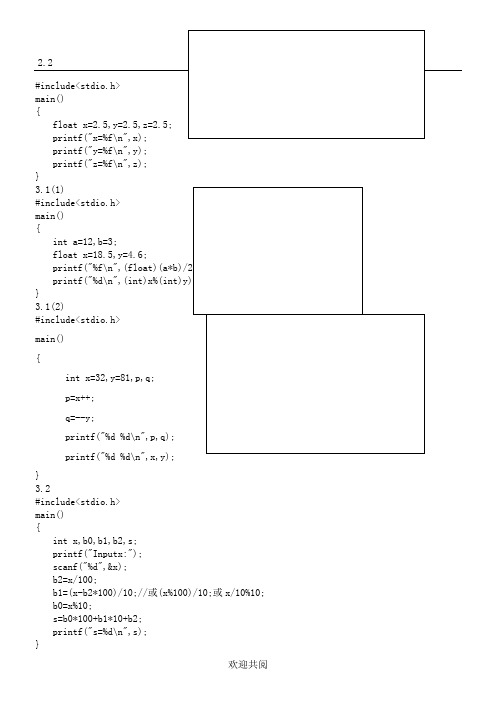
printf("y=%f\n",y);printf("z=%f\n",z);}3.1(1)main(){}3.1(2)main(){}3.2main(){int x,b0,b1,b2,s;printf("Inputx:");scanf("%d",&x);b2=x/100;b1=(x-b2*100)/10;//或(x%100)/10;或x/10%10;b0=x%10;s=b0*100+b1*10+b2;printf("s=%d\n",s);}3.3#include<stdio.h>#include<math.h>main(){float rate=0.0225;float n,capital,deposit;printf("Input n,capital:");scanf("%f,%f",&n,&capital);deposit=capital*pow(1+rate,n);printf("deposit=%f\n",deposit); }3.4main(){}习题44.1(1)main(){}4.1(2)main(){}4.1(3)main(){}4.2#include<stdio.h>main(){long a,b;float x,y;scanf("%d,%d\n",&a,&b);scanf("%f,%f\n",&x,&y);printf("a=%d,b=%d\n",a,b);printf("x=%f,b=%f\n",x,y); }5.1main(){{}{}}5.2main(){intscanf("%d", &a);if(a%2==0){printf("a是偶数");}else{printf("a是奇数");}}5.3#include<stdio.h>#include<math.h>main(){float a,b,c,s,area;printf("Input a, b, c:");scanf("%f %f %f", &a, &b, &c);if(a+b>c&&a+c>b&&b+c>a){s=(a+b+c)/2;area=(float)sqrt(s*(s-a)*(s-b)*(s-c));printf("area=%f\n",area);}{}}5.4main(){{}{}else if(b*b-4*a*c==0){x=-b/(2*a);y=-b/(2*a);printf("x=%f,y=%f\n",x,y);}else{printf("该方程无实根\n");}}5.5#include<stdio.h>main(){int year,flag;printf("Input a year:");scanf("%d",&year);if(year%4==0&&year%400!=0||year%400==0) {flag=1;}else{}{}{}}5.6main(){int{}else{printf("%d is not a leap year!\n",year);}}5.7#include<stdio.h>main(){char ch;printf("Inputch:");scanf("%c",&ch);if(ch>='a'&&ch<='z'){ch=getchar();ch=ch-32;printf("%c,%d\n",ch,ch);}else if(ch>='A'&&ch<='Z'){ch=getchar();ch=ch+32;printf("%c,%d\n",ch,ch);}{}}5.8#include<stdio.h>main(){char ch;printf("Inputch:");scanf("%c",&ch);if(ch>=48&&ch<=57){printf("ch是数字字符\n");grade=score/10;if(score<0||score>100){printf("Input error\n");}if(score>=90&&score<=100){printf("%d--A\n",score);}else if(score>=80&&score<90){printf("%d--B\n",score);}else if(score>=70&&score<80){printf("%d--C\n",score);}else if(score>=60&&score<70){printf("%d--D\n",score);}case3:case1:printf("31天\n");break;case11:case9:case6:case4:printf("30天\n");break;case2:if(year%4==0&&year!=0||year%400==0){printf("29天\n");}else{printf("28天\n");}break;main(){int k=4,n;for(n=0;n<k;n++){if(n%2==0)continue;k--;}printf("k=%d\n,n=%d\n",k,n);}6.1(3)#include<stdio.h>main(){int k=4,n;for(n=0;n<k;n++){if(n%2==0)break;k--;}printf("k=%d,n=%d\n",k,n);main(){int n=1;float term=1.0,sign=1,sum=0;while(term<=-1e-4||term>=1e-4){term=1.0/sign;sum=sum+term;sign=sign+n;n++;}printf("sum=%f\n",sum);}6.2(5)#include<stdio.h>#include<math.h>main(){int n=1,count=1;float x;}}/*int x,find=0;for(x=1;!find;x++){if(x%2==1&&x%3==2&&x%5==4&&x%6==5&&x%7==0){printf("x=%d\n",x);find=1;}}}*/6.4#include<stdio.h>main(){int i,n;long p=1,m=1;printf("Input n:");scanf("%d",&n);x=x*pow(1+c,12)-1000;n++;}while(x>0);printf("x=%d\n",x);}6.7#include<stdio.h>main(){int n=0;float a=100.0,c;printf("Inputc:");scanf("%f",&c);do{a=a*(1+c);n++;}while(a<=200);printf("n=%d\n",n);}term=term*(1.0/n);sum=sum+term;n++;count++;}printf("sum=%f,count=%d\n",sum,count); }6.10#include<stdio.h>#include<math.h>main(){int x;for(x=100;x<=999;x++){if(x==pow(x/100,3)+pow(x/10%10,3)+pow(x%10,3))printf("x=%d\n",x);}}{sum=sum+m;count++;}else{break;}printf("sum=%d,count=%d\n",sum,count);}}6.13#include<stdio.h>main(){int i,n,m,count=0,sum=0;printf("Inputn:");scanf("%d",&n);for(i=1;i<=n;i++){printf("x=%d,y=%d,z=%d\n",x,y,z);}}}6.15#include<stdio.h>main(){int x,y;for(x=0;x<=98;x++){y=98-x;2*x+4*y==386;if(x+y==98&&2*x+4*y==386){printf("x=%d,y=%d\n",x,y);}}}6.16#include<stdio.h>{10*x+5*y+z==100;z=50-x-y;if(10*x+5*y+z==100&&x+y+z==50&&z>0){printf("x=%d,y=%d,z=%d\n",x,y,z);}}}}7.1#include<stdio.h>int Square(int i){return i*i;}int main(){int i=0;i=Square(i);hour=0;}void display(){printf("%d,%d,%d\n",hour,minute,second); }void delay(){int t;for(t=0;t<100000000;t++);}int main(){int i;void updaye(),display(),delay();for(i=0;i<1000000;i++){update();int main(){int a,b;printf("Inputa,b:");scanf("%d,%d",&a,&b);printf("%d\n",LCM(a,b));return0;}int LCM(int n,int m){int x;int find=0;for(x=1;!find;x++){if(x%n==0&&x%m==0){find=1;}long Fact(int n);int main(){int m;long ret;printf("Inputm:");scanf("%d",&m);ret=Fact(m);printf("ret=%d\n",ret);return0;}long Fact(int n){int i;long result=1,sum=0;for(i=2;i<=n;i++){result*=i;sum=sum+result;find=1;}7.7(2)#include<stdio.h> int Gcd(int a,int b);int main(){int m,n;printf("Inputm,n:");scanf("%d,%d",&m,&n);printf("%d\n",Gcd(m,n));return0;}int Gcd(int a,int b){int r,temp;r=a%b;if(r==0)return b;else{b[j]=j;}}int main(){static int a[]={5,6,7,8},i;Func(a);for(i=0;i<4;i++){printf("%d",a[i]);}return0;}8.2(1)int PositiveNum(int a[],int n) {int i,count=0;for(i=0;i<n;i++){{if(a[n]>max){max=a[n];maxPos=n;}else if(a[n]<min){min=a[n];minPos=n;}}printf("max=%d,pos=%d\n",max,maxPos);printf("min=%d,pos=%d\n",min,minPos);return0;}8.3void DivArray(int*pArray,int n){int i;count++;}return count;}void ReadScore(int score[],int n){int i;printf("Input score:");for(i=0;i<n;i++){scanf("%d",&score[i]);}}8.5#include<stdio.h>#define N40int HighAver(int score[],int n);int ReadScore(int score[]);int main(){printf("Input score:");scanf("%d",&score[i]);}while(score[i]>=0);return i;}8.6#include<stdio.h>#define N40int ReadScore(int score[],long num[]); int FindMax(int score[],long num[],int n);int main(){int score[N],n,max,num,t;long num[N];t=FindMax(score,n);max=score[t];num=num[t];printf("max=%d,num=%d\n",max,num);return0;}int main(){int a[N],n;printf("%d\n",ChangeArry(a,n));return0;}int Read(int a[]){int i;for(i=0;i<9;i++){printf("Input a:");scanf("%d",&a[i]);return i;}int ChangeArry(int a[],int n){int max,min,i,maxpos,minpos,term;max=min=score[0];for(j=0;j<N;j++){scanf("%d",&a[i][j]);}}for(i=0;i<N;i++){for(j=0;j<N;j++){if(i==j||i+j==N-1)sum=sum+a[i][j];}}printf("对角线元素之和为:%d\n",sum); }8.11#include<stdio.h>#define N2#define M3void main()#define N7void YangHui(int a[N][N],int n);void main(){int i,j,n,b[N][N];printf("Input n:");scanf("%d",&n);YangHui(b,n);for(i=0;i<n;i++){for(j=0;j<=i;j++){printf("%4d",b[i][j]);}printf("\n");}}void YangHui(int a[N][N],int n){}8.14#include<stdio.h>#include<stdlib.h>#include<time.h>#define N6000void main(){int a[N],i;int b1=0,b2=0,b3=0,b4=0,b5=0,b6=0;float p1,p2,p3,p4,p5,p6;srand(time(NULL));for(i=0;i<N;i++){a[i]=rand()%6+1;printf("%3d",a[i]);if(a[i]==1)b1++;if(a[i]==2)b2++;*pTemp=*x;*x=*y;*y=*pTemp;}9.1(2)void Swap(int*x,int*y) {int*pTemp;pTemp=x;x=y;y=pTemp;}//错误,x,y没有指向类型9.2#include<stdio.h>int main(){int a[]={1,2,3,4,5};int*p=a;printf("%d,%d,%d,%d,%d,%d,%d\n",*p,*(++p),(*p)++,*p,*p--,--(*p),*p);return0;for(i=0;i<6;i++){printf("%d\n",b[i]);}return0;}void Swap(int*x,int*y){int temp;temp=*x;*x=*y;*y=temp;}9.4#include<stdio.h>#define N10void FindMaxMin(int a[],int*pMaxa,int*pMaxnum,int*pMina,int*pMinnum); int main(){int a[N];{*pMina=a[i];*pMinnum=i;}}}10.1void MYStrcpy(char*dstStr,const char*srcStr){while((*dstStr++=*srcStr++)!='\0'){}}10.2#include<stdio.h>main(){char*a="main(){char*a=%c%s%c;printf(a,34,a,34);}";printf(a,34,a,34);} 10.3(1)unsigned int MyStrlen(char*p)main(){unsigned int len;{int i;for(i=0;s[i]==t[i];i++){if(s[i]=='\0')return0;}return i;}10.3(5)#include<stdio.h>#include<string.h>int main(){char password[7]="secret";char userInput[81];printf("Input Password:");scanf("%s",userInput);if(strcmp(userInput,password)==0)printf("Coorrect password!Welcome to the system...\n");else if(strcmp(userInput,password)<0){c++;flag=0;}p++;}return c+1;}12.1#include<stdio.h>typedef struct data{int year;int month;int day;}DATA;typedef struct work{char a[14];char b[10];int sum1=0,sum2=0,sum3=0,sum4=0;int i;char name[N][N];PEOPLE stu[5]={"zhang","li","wang"};printf("Enter their name:\n");for(i=0;i<10;i++){gets(name[i]);if(strcmp(name[i],stu[0].peopleName)==0) sum1++;else if(strcmp(name[i],stu[1].peopleName)==0) sum2++;else if(strcmp(name[i],stu[2].peopleName)==0) sum3++;elsesum4++;}printf("stu:%4s%2d\n",stu[i].peopleName,sum[j]);。
C语言程序设计苏小红版答案

#include<>main(){float x=,y=,z=;printf("x=%f\n",x);printf("y=%f\n",y);printf("z=%f\n",z);}(1) #include<>main(){int a=12,b=3;float x=,y=;printf("%d\n",(float)(a*b)/2);printf("%d\n",(int)x%(int)y);}#include<>main(){int x,b0,b1,b2,s;printf("Inputx:");scanf("%d",&x);b2=x/100;b1=(x-b2*100)/10;b0=x%10;s=b0*100+b1*10+b2;printf("s=%d\n",s);}#include<>#include<>main(){float rate=;float n,capital,deposit;printf("Input n,capital:");scanf("%f,%f",&n,&capital);deposit=capital*pow(1+rate,n);printf("deposit=%f\n",deposit); }#include<>#include<>main(){float a,b,c;double x,y;printf("Inputa,b,c:");scanf("%f,%f,%f",&a,&b,&c);x=(-b+sqrt(b*b-4*a*c))/(2*a);y=(-b-sqrt(b*b-4*a*c))/(2*a);printf("x=%f,y=%f\n",x,y);}(1) #include<>main(){char c1='a',c2='b',c3='c';printf("a%cb%cc%c\n",c1,c2,c3); }(2) #include<>main(){int a=12,b=15;printf("a=%d%%,b=%d%%\n",a,b); }(3) #include<>main(){int a,b;scanf("%2d%*2s%2d",&a,&b);printf("%d,%d\n",a,b);}#include<>main(){long a,b;float x,y;scanf("%d,%d\n",&a,&b);scanf("%f,%f\n",&x,&y);printf("a=%d,b=%d\n",a,b);printf("x=%f,b=%f\n",x,y);}#include<>main(){float a;printf("Innputa:");scanf("%f",&a);if(a>=0){a=a;printf("a=%f\n",a);}else{a=-a;printf("a=%f\n",a);}}#include<>main(){int a;printf("Inputa:");scanf("%d",&a);if(a%2==0){printf("a是偶数");}else{printf("a是奇数");}}#include<>#include<>main(){float a,b,c,s,area;printf("Inputa,b,c:");scanf("%f,%f,%f",&a,&b,&c);if(a+b>c&&a+c>b&&b+c>a){s=(a+b+c)/2;area=(float)sqrt(s*(s-a)*(s-b)*(s-c));printf("area=%f\n",area);}else{printf("不是三角形");}}#include<>#include<>{float a,b,c,x,y;printf("Inputa,b,c:");scanf("%f,%f,%f",&a,&b,&c);if(a==0){printf("该方程不是一元二次方程\n");}if(b*b-4*a*c>0){x=(-b+sqrt(b*b-4*a*c))/(2*a);y=(-b-sqrt(b*b-4*a*c))/(2*a);printf("x=%f,y=%f\n",x,y);}else if(b*b-4*a*c==0){x=-b/(2*a);y=-b/(2*a);printf("x=%f,y=%f\n",x,y);}else{printf("该方程无实根\n");}}#include<>main(){int year,flag;printf("Input a year:");scanf("%d",&year);if(year%4==0&&year%400!=0||year%400==0) {flag=1;}else{flag=0;}if(flag==1){printf("%d is a leap year !\n",year);}{printf("%d is not a leap year !\n",year);}}#include<>main(){int year,flag;printf("Input a year:");scanf("%d",&year);flag=year%400==0||year%4==0&&year%100!=01:0;if(flag==1&&flag!=0){printf("%d is a leap year !\n",year);}else{printf("%d is not a leap year !\n",year);}}#include<>main(){char ch;printf("Inputch:");scanf("%c",&ch);if(ch>='a'&&ch<='z'){ch=getchar();ch=ch-32;printf("%c,%d\n",ch,ch);}else if(ch>='A'&&ch<='Z'){ch=getchar();ch=ch+32;printf("%c,%d\n",ch,ch);}else{printf("%c",ch);}}#include<>main(){char ch;printf("Inputch:");scanf("%c",&ch);if(ch>=48&&ch<=57){printf("ch是数字字符\n");}else if(ch>=65&&ch<=90){printf("ch是大写字母\n");}else if(ch>=97&&ch<=122){printf("ch是小写字母\n");}else if(ch==32){printf("ch是空格\n");}else{printf("ch是其他字符\n");}}#include<>main(){int score,grade;printf("Input score:");scanf("%d",&score);grade=score/10;if(score<0||score>100){printf("Input error\n");}if(score>=90&&score<=100){printf("%d--A\n",score);}else if(score>=80&&score<90){printf("%d--B\n",score);}else if(score>=70&&score<80){printf("%d--C\n",score);}else if(score>=60&&score<70){printf("%d--D\n",score);}else if(score>=0&&score<60){printf("%d- -E\n",score);}}#include<>main(){int year,month;printf("Input year,month:");scanf("%d,%d",&year,&month);if(month>12||month<=0){printf("error month\n");}else{switch(year,month){case 12:case 10:case 8:case 7:case 5:case 3:case 1:printf("31天\n");break;case 11:case 9:case 6:case 4:printf("30天\n");break;case 2:if(year%4==0&&year!=0||year%400==0){printf("29天\n");}else{printf("28天\n");}break;default:printf("Input error\n");}}}(1) #include<>main(){int i,j,k;char space=' ';for(i=1;i<=4;i++){for(j=1;j<=i;j++){printf("%c",space);}for(k=1;k<=6;k++){printf("*");}printf("\n");}}(2) #include<>main(){int k=4,n;for(n=0;n<k;n++){if(n%2==0) continue;k--;}printf("k=%d\n,n=%d\n",k,n);}(3) #include<>main(){int k=4,n;for(n=0;n<k;n++){if(n%2==0) break;k--;}printf("k=%d,n=%d\n",k,n);}(1) #include<>main(){int i,sum=0;for(i=1;i<=101;i++){sum=sum+i;}printf("sum=%d\n",sum);}(2) #include<>main(){long i;long term,sum=0;for(i=1;i<=101;i=i+2){term=i*(i+1)*(i+2);sum=sum+term;}printf("sum=%ld\n",sum);}(4) #include<>#include<>main(){int n=1;float term=,sign=1,sum=0;while(term<=-1e-4||term>=1e-4){term=sign;sum=sum+term;sign=sign+n;n++;}printf("sum=%f\n",sum);}(5) #include<>#include<>main(){int n=1,count=1;float x;double sum,term;printf("Input x:");scanf("%f",&x);sum=x;term=x;do{term=-term*x*x/((n+1)*(n+2));sum=sum+term;n=n+2;count++;}while(fabs(term)>=1e-5);printf("sin(x)=%f,count=%d\n",sum,count);}#include<>main(){int x=1,find=0;while(!find){if(x%2==1&&x%3==2&&x%5==4&&x%6==5&&x%7==0){printf("x=%d\n",x);find=1;x++;}}}/* int x,find=0;for (x=1;!find;x++){if(x%2==1&&x%3==2&&x%5==4&&x%6==5&&x%7==0){printf("x=%d\n",x);find=1;}}}*/#include<>main(){int i,n;long p=1,m=1;printf("Input n:");scanf("%d",&n);for(i=1;i<=n;i++){p=i*i;m=i*i*i;printf("p=%d,m=%d\n",i,p,i,m);}}#include<>main(){float c,f;for(c=-40;c<=110;c=c+10){f=9/5*c+32;printf("f=%f\n",f);}}#include<>#include<>main(){int n;double c=,x;do{x=x*pow(1+c,12)-1000;n++;}while(x>0);printf("x=%d\n",x);}#include<>main(){int n=0;float a=,c;printf("Inputc:");scanf("%f",&c);do{a=a*(1+c);n++;}while(a<=200);printf("n=%d\n",n);}#include<>#include<>main(){int n=1,count=1;double sum=1,term=1;while(fabs(term)>=1e-5){term=pow(-1,count)*(n+2));sum=sum+term;n=n+2;count++;}sum=4*sum;printf( "sum=%f,count=%d\n",sum,count); }#include<>#include<>main(){int n=1,count=1;double sum=1,term=1;while(fabs(term)>=1e-5){term=term*n);sum=sum+term;n++;count++;}printf("sum=%f,count=%d\n",sum,count);}#include<>#include<>main(){int x;for(x=100;x<=999;x++){if(x==pow(x/100,3)+pow(x/10%10,3)+pow(x%10,3))printf("x=%d\n",x);}}#include<>main(){int i=0,n;long sum=0,term=1;printf("Inputn:");scanf("%d",&n);do{i++;term=term*i;sum=sum+term;}while(sum<n);printf("%d\n",i-1);}#include<>main(){int i,n,m,count=0,sum=0;printf("Input n:");scanf("%d",&n);for(i=1;i<=n;i++){printf("Inputm:");scanf("%d",&m);if(m>0){sum=sum+m;count++;}else{break;}printf("sum=%d,count=%d\n",sum,count);}}#include<>main(){int i,n,m,count=0,sum=0;printf("Inputn:");scanf("%d",&n);for(i=1;i<=n;i++){printf("Inputm:");scanf("%d",&m);if(m>0||m<0){sum=sum+m;count++;}else{break;}printf("sum=%d,count=%d\n",sum,count);}}#include<>main(){int x,y,z;for(x=0;x<=17;x++){for(y=0;y<=25;y++){3*x+2*y+z==50;z=30-x-y;if( 3*x+2*y+z==50&&x+y+z==30)printf("x=%d,y=%d,z=%d\n",x,y,z);}}}#include<>main(){int x,y;for(x=0;x<=98;x++){y=98-x;2*x+4*y==386;if(x+y==98&&2*x+4*y==386){printf("x=%d,y=%d\n",x,y);}}}#include<>main(){int x,y,z;for(x=0;x<=20;x++){for(y=0;y<=33;y++){3*y+5*x+z/==100;z=100-x-y;if (5*x+3*y+z/==100&&z+x+y==100){printf("x=%d,y=%d,z=%d\n",x,y,z);}}}}#include<>main(){int x,y,z;for(x=1;x<=9;x++){for(y=1;y<=17;y++){10*x+5*y+z==100;z=50-x-y;if( 10*x+5*y+z==100&&x+y+z==50&&z>0){printf("x=%d,y=%d,z=%d\n",x,y,z);}}}}#include<>int Square(int i){return i*i;}int main(){int i=0;i=Square(i);for( ;i<3;i++){static int i=1;i+=Square(i);printf("%d,",i);}printf("%d\n",i);return 0;}#include<>int hour,minute,second;void update(){second++;if(second==60){second=0;minute++;}if(minute==60){minute=0;hour++;}if(hour==24)hour=0;}void display(){printf("%d,%d,%d\n",hour,minute,second);}void delay(){int t;for(t=0;t<0;t++);}int main(){int i;void updaye(),display(),delay();for(i=0;i<1000000;i++){update();display();delay();}return 0;}#include<>int GetMax(int a,int b);int main(){int x,y,max;printf("Inputx,y:");scanf("%d,%d",&x,&y);max=GetMax(x,y);printf("max=%d\n",max);return 0;}int GetMax(int m,int n){if(m>=n)return m;elsereturn n;}#include<>int LCM(int n,int m);int main(){int a,b;printf("Inputa,b:");scanf("%d,%d",&a,&b);printf("%d\n",LCM(a,b));return 0;}int LCM(int n,int m){int x;int find=0;for(x=1;!find;x++){if(x%n==0&&x%m==0){find=1;}}return x-1;}#include<>long Fact(int n);int main(){int m,a;printf("Inputm:");scanf("%d",&m);for(a=1;a<=m;a++){printf("%d!=%ld\n",a,Fact(a));}return 0;}long Fact(int n){int i;long result=1;for(i=2;i<=n;i++)result*=i;return result;}#include<>long Fact(int n);int main(){int m;long ret;printf("Inputm:");scanf("%d",&m);ret=Fact(m);printf("ret=%d\n",ret);return 0;}long Fact(int n){int i;long result=1,sum=0;for(i=2;i<=n;i++){result*=i;sum=sum+result;}return sum;}(1) #include<>int Gcd(int a,int b);int main(){int m,n;printf("Inputm,n:");scanf("%d,%d",&m,&n);printf("%d\n",Gcd(m,n));return 0;}int Gcd(int a,int b){int t,min,find=0;min=a<ba:b;t=min;for(t=min;!find;t--){a%t==0;b%t==0;if(a%t==0&&b%t==0)return t;}find=1;}(2) #include<>int Gcd(int a,int b);int main(){int m,n;printf("Inputm,n:");scanf("%d,%d",&m,&n);printf("%d\n",Gcd(m,n));return 0;}int Gcd(int a,int b){int r,temp;r=a%b;if(r==0)return b;elsedo{temp=b;b=r;a=temp;r=a%b;}while(r!=0);return b;}(1) #include<>void Func(int x){x=2;}int main(){int x=10;Func(x);printf("%d",x);return 0;}(2) #include<>void Func(int b[]){int j;for(j=0;j<4;j++){b[j]=j;}}int main(){static int a[]={5,6,7,8},i;Func(a);for(i=0;i<4;i++){printf("%d",a[i]);}return 0;}(1) int PositiveNum(int a[],int n){int i,count=0;for(i=0;i<n;i++){if(a[i]>0)count++;}return 0;}(2) void Fib(long f[],long n){int i;f[0]=0;f[1]=1;for(i=2;i<n;i++){f[i]=f[i-1]+f[i-2];}}(3) #include<>int main(){int a[10],n,max,min,maxPos,minPos;for(n=0;n<10;n++){scanf("%d",&a[n]);}max=min=a[0];maxPos=minPos=0;for(n=0;n<10;n++){if(a[n]>max){max=a[n];maxPos=n;}else if(a[n]<min){min=a[n];minPos=n;}}printf("max=%d,pos=%d\n",max,maxPos);printf("min=%d,pos=%d\n",min,minPos);return 0;}void DivArray(int *pArray,int n){int i;for(i=0;i<n;i++){pArray[i]/=pAttay[0];}}#include<>#define N 40int FailNum(int score[],int n);void ReadScore(int score[],int n);int main(){int score[N],n;printf("Input n:");scanf("%d",&n);ReadScore(score,n);FailNum(score,n);printf("FailNum students are %d\n",FailNum(score,n));return 0;}int FailNum(int score[],int n){int i,count=0;for(i=0;i<n;i++){if(score[i]<60)count++;}return count;}void ReadScore(int score[],int n){int i;printf("Input score:");for(i=0;i<n;i++){scanf("%d",&score[i]);}}#include<>#define N 40int HighAver(int score[],int n);int ReadScore(int score[]);int main(){int score[N],n;n=ReadScore(score);printf("HighAver students are %d\n",HighAver(score,n));return 0;}int HighAver(int score[],int n){int i,count=0,sum=0,aver;for(i=0;i<n;i++){sum=sum+score[i];aver=sum/n;}for(i=0;i<n;i++){if(score[i]>aver)count++;}return count;}int ReadScore(int score[]){int i=-1;do{i++;printf("Input score:");scanf("%d",&score[i]);}while(score[i]>=0);return i;}#include<>#define N 40int ReadScore(int score[],long num[]);int FindMax(int score[],long num[],int n);int main(){int score[N],n,max,num,t;long num[N];t=FindMax(score,n);max=score[t];num=num[t];printf("max=%d,num=%d\n",max,num);return 0;}int ReadScore(int score[],long num[]){int i=-1;do{i++;printf("Input student's ID and score:");scanf("%ld%d",&num[i],&score[i]);}while(num[i]>0&&score[i]>=0);return i;}int FindMax(int score[],long num[],int n){int max,i;max=score[0];for(i=1;i<n;i++){if(score[i]>max)max=score[i];}return i;}#include<>#define N 40int Read(int a[]);int ChangeArry(int a[],int n);int main(){int a[N],n;printf("%d\n",ChangeArry(a,n));return 0;}int Read(int a[]){int i;for(i=0;i<9;i++){printf("Input a:");scanf("%d",&a[i]);return i;}int ChangeArry(int a[],int n){int max,min,i,maxpos,minpos,term;max=min=score[0];for(i=1;i<n;i++){if(a[i]>max)max=score[i];maxpos=n;else(a[i]<min)min=a[i];minpos=n;temp=maxpos;maxpos=minpos;minpos=temp;}return a[i];}#include<>#define N 5void main(){int a[N][N];int sum = 0;int i, j;printf("请输入一个%d*%d的矩阵\n",N,N);for(i = 0; i < N; i++){for(j = 0; j < N; j++){scanf("%d",&a[i][j]);}}for(i = 0; i < N; i++){for(j = 0; j < N; j++){if(i == j || i + j == N - 1)sum=sum+a[i][j];}}printf("对角线元素之和为:%d\n",sum);}#include<>#define N 2#define M 3void main(){int a[N][M],b[N][M];int i,j,sum1=0,sum2=0,sum=0;printf("请输入一个%d*%d的矩阵\n",N,M);for(i = 0; i<N; i++){for(j = 0; j< M; j++){scanf("%d",&a[i][j]);sum1=sum1+a[i][j];}}printf("请输入一个%d*%d的矩阵\n",N,M);for(i = 0; i <N; i++){for(j = 0; j <M; j++){scanf("%d",&b[i][j]);sum2=sum2+a[i][j];}}sum=sum1+sum2;printf("sum=%d\n",sum);}#include<>#define N 7void YangHui(int a[N][N],int n);void main(){int i,j,n,b[N][N];printf("Input n:");scanf("%d",&n);YangHui(b,n);for(i=0;i<n;i++){for(j=0;j<=i;j++){printf("%4d",b[i][j]);}printf("\n");}}void YangHui(int a[N][N],int n){int i,j;for(i=0;i<n;i++){a[i][0]=1;a[i][i]=1;}for(i=2;i<n;i++){for(j=1;j<i;j++)a[i][j]=a[i-1][j-1]+a[i-1][j];}}#include<>#define N 12void main(){int i;int f[N]={1,1};printf("%d:%d\n",0,f[0]);printf("%d:%d\n",1,f[1]);for(i=2;i<N;i++){f[i]=f[i-1]+f[i-2];printf("%d:%d\n",i+1,f[i]);}}#include<>#include<>#include<>#define N 6000void main(){int a[N],i;int b1=0,b2=0,b3=0,b4=0,b5=0,b6=0;float p1,p2,p3,p4,p5,p6;srand(time(NULL));for(i=0;i<N;i++){a[i]=rand()%6+1;printf("%3d",a[i]);if(a[i]==1)b1++;if(a[i]==2)b2++;if(a[i]==3)b3++;if(a[i]==4)b4++;if(a[i]==5)b5++;if(a[i]==6)b6++;p1=(float)(b1/;p2=(float)(b2/;p3=(float)(b3/;p4=(float)(b4/;p5=(float)(b5/;p6=(float)(b6/;}printf("骰子出现1的概率:%.2f\n",p1);printf("骰子出现2的概率:%.2f\n",p2);printf("骰子出现3的概率:%.2f\n",p3);printf("骰子出现4的概率:%.2f\n",p4);printf("骰子出现5的概率:%.2f\n",p5);printf("骰子出现6的概率:%.2f\n",p6);}(1) void Swap(int *x,int *y){int *pTemp;.\n");else if(strcmp(userInput,password)<0)printf("Invalid password!user input<password...\n");elseprintf("Invalid password!user input>password...\n");return 0;}#include<>#define N 24unsigned int CountLetter(char str[]);{char a[N];printf("Input a letter:\n");gets(a);printf("The length of the letter is:%d\n",CountLetter(a));return 0;}unsigned int CountLetter(char str[]){char *p=str;int c=0,flag=0;while(*p!='\0'){if(*p!=' ')flag=1;else if(flag==1){c++;flag=0;}p++;}return c+1;}#include<>typedef struct data{int year;int month;int day;}DATA;typedef struct work{char a[14];char b[10];char c[6];}WORK;typedef struct student{char studentName[10];char studentSex;DATA birthday;WORK profession;int main(){STUDENT stu={"王刚",'M',{1991,5,19},{"信息工程系","学习人员","学生"}};printf("stu:%2s%3c%6d/%02d/%02d%4s,%4s,%4s\n",,, return 0; }#include<>#include<>#define N 10typedef struct people{char peopleName[10];}PEOPLE;int main(){int sum1=0,sum2=0,sum3=0,sum4=0;int i;char name[N][N];PEOPLE stu[5]={"zhang","li","wang"};printf("Enter their name:\n");for(i=0;i<10;i++){gets(name[i]);if(strcmp(name[i],stu[0].peopleName)==0)sum1++;else if(strcmp(name[i],stu[1].peopleName)==0)sum2++;else if(strcmp(name[i],stu[2].peopleName)==0)sum3++;elsesum4++;}printf("stu:%4s%2d\n",stu[i].peopleName,sum[j]);printf("stu:%4s%2d\n",stu[1].peopleName,sum2);printf("stu:%4s%2d\n",stu[2].peopleName,sum3);printf("%2d\n",sum4);return 0;}。
C语言程序设计苏小红版答案解析

2.2#iiiclude<stdio.h>main(){float x=2・5,y=2・5,z=2・5;pnntf(M x=%far\x); pnntf(M y=%fur\y);pmitf(”z=%f\n”,z);}3.1(1) #iiiclude<stdio.h> main(){int a=12,b=3;float x=18・5,y=4・6;printf(,,%d,di,\(float)(a*b)/2); pmitf(・%d\n役(int)x%(mt)y);}3.2#iiiclude<stdio.h>main(){int x,b0,bl,b2,s; pnntf(M Iiiputx:H);scanfC%dt&x);b2=x/100; bl=(x-b2*100)/10;b0=x%10; s=b0*100+bl*10+b2;}3.3# iiiclude<stdio.h>#mclude<math.h>main(){float rate=0.0225;float n,capital,deposit;printf(M Iiiput n,capital:H);scanf("%f,%f;&n,&capital);deposit=capital*pow(l+iate,n);printf(M deposit=%fji'\deposit);}3.4#iiiclude<stdio.h>#mclude<math.h>main()float 久b,c;double x,y;printf(M Iiiputa,b,c:H);scanf(H%f,%f,%f\&a.&b、&c);x=(-b+sqit(b*b-4*a*c))/(2 *a);y=(-b-sqrt(b*b-4*a*c))/(2*a); pnntfC,x=%fy=%fur\x,y);}4.1(1) #iiiclude<stdio.h>main(){char cl=,a\c2=,b\c3=t c,;printf(,l a%cb%cc%c\n,\c 1 ,c2,c3);}4.1(2) #iiiclude<stdio.h>main(){int a=12,b=15;pmHfTa=%d%%,b=%d%%S”,a,b);}4.1(3) #iiiclude<stdio.h>main(){int a、b;scanfC%2d%*2s%2d”,&a,&b);piiDtfp%d.%d\ir;a,b);}4.2#iiiclude<stdio.h>main(){long a,b;float x、y;scanfC%d,%d\n 役&a.&b);scanf(H%f,%fur\&x.&y);piintf(M a=%d.b=%d,,ii,\a.b); pnntfC,x=%fb=%far\x,v);}5.1 #iiiclude<stdio.h>main(){float a;scanf(H%f\&a);if(a>=0)a=a; pnntf「a=%f\n=);}else{a=-a; pnntf「a=%f\n=);}}5 ・ 2 #iiiclude<stdio.h>main(){int a;pnntf(H Iiiputa:H);scanff%cT;&a);if(a%2==0){prmtf("a 是偶数”);}else{piuitf("a 是奇数”);}}5.3#iiiclude<stdio.h>#mclude<math.h>main(){float a,b,c,s,area; pnntH M Iiiputa,b,c:H);scanf(H%f,%f,%f\&a,&b,&c);if(a+b>c&&a+c>b&&b+c>a) {s=(a+b+c)/2;area=(float)sqrt(s * (s-a) * (s-b)* (s-c));priiitf(n aiea=%f.n,\area);}else{pnntf(”不是三角形”);}}5.4#iiiclude<stdio.h>#iiiclude<math.li>mam()float a、b,c,x,y;pmitfClnputabcJ);if(a=0){ pnntf(”该方程不是一元二次方程®”);}if(b*b-4*a*c>0){ x=(-b+sqrt(b *b-4*a *c))/(2*a);v=(-b-sqrt(b*b-4*a*c))/(2*a); pnntf「x=%f,y=%fm役x,y);}else if(b*b-4*a*c=0){x=-b/(2*a);v=-b/(2*a); pnntf「x=%f,y=%fm 役x,y);}else{ pnntf(”该方程无实根\n”);}}5.5#iiiclude<stdio.h>main(){int yeai;flag;printf(M Iiiput a vear:H);scanf^^d^&yeai);if(y 亡ai%4==0&&yeai-%400! =01 |year%400==0) { flag=l;}else{flag=0;}if(flag==l)piiiitf(n%d is a leap year !\n”,yeaQ;elsepiiiitf(,,%d is not a leap year l^^year);}}5.6#iiiclude<stdio.h>main(){int yeai;flag;printf(M Iiiput a vear:H);scanfl^d^&yeai);flag=veai-%400=0||year%4==0&&y 亡ai%100!=0H:0;if(flag==l && flag! =0){priiitf(n%d is a leap year !\n,\vear);}else{is not a leap year l^^year);}}5.7#iiiclude<stdio.h>main(){char ch;pnntf(M Iiiputch:H);scanf(”%c-&ch);if(ch>=,a,&&ch<=N){ch=getchai();ch=ch・32;printf(n%c,%d\n,\ch,ch);}else if(ch>=,A,&&cli<='Z f){ch=getchai();ch=ch+32;printf(n%c,%d\n,\ch,ch);}elsepnntff%L,ch);5 ・ 8 #iiiclude<stdio.h> main()char ch; pnntf(M Iiiputch:H); scanf(”%ct&ch);if(ch>=48&&ch<=57){pruitf("ch是数字字符\n");}else if(ch>=65&&ch<=90){pimtfC'ch是大写字母\n");}else if(ch>=97&&ch<=122){ pnntf(”ch是小写字母\n");}else if(ch==32){printf(” ch 是空格\n”);}else{ pimtfC'ch是其他字符\n”);}}5.9 #iiiclude<stdio.h>main(){int score,grade; printf(M Iiiput score:n); scanf(H%d,\&scoie);grade=score/10; if(score<0|| score> 100){priiitf(n Input enor\n H);}if(score>=90&&score<= 100){prmtf(H%d-A\ii,\score);}else if(score>=80&&score<90)(n \H<ormuud&use。
C语言程序设计苏小红版答案
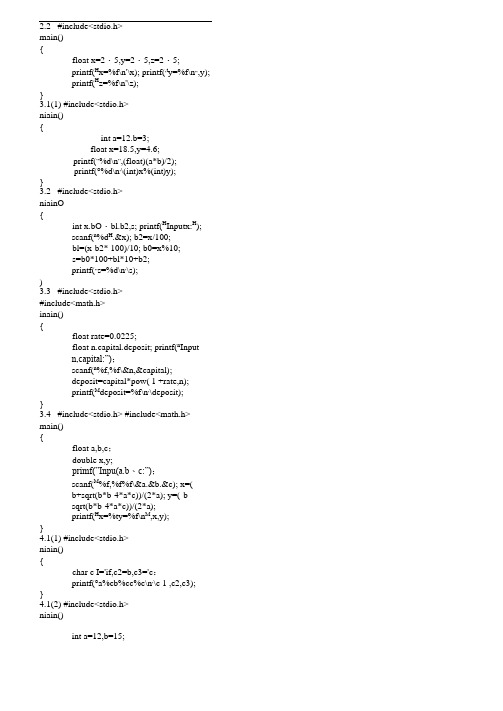
2.2#include<stdio.h>main(){float x=2・5,y=2・5,z=2・5;printf(H x=%f\n'\x); printf(,t y=%f\n,,,y);printf(H z=%f\n'\z);}3.1(1) #include<stdio.h>niain(){int a=12.b=3;float x=18.5,y=4.6;printf(,,%d\n,,,(float)(a*b)/2);printf(°%d\n,\(int)x%(int)y);}3.2#include<stdio.h>niainO{int x.bO・bl.b2,s; printf(H Inputx:H);scanf(n%d H.&x); b2=x/100;bl=(x-b2* 100)/10; b0=x%10;s=b0*100+bl*10+b2;printf(,,s=%d\n,\s);)3.3#include<stdio.h>#include<math.h>inain(){float rate=0.0225;float n.capital.deposit; printf(n Inputn,capital:”);scanf(n%f,%f\&n,&capital);deposit=capital*pow( 1 +rate,n);printf(M deposit=%f\n,\deposit);}3.4#include<stdio.h> #include<math.h> main(){float a,b,c;double x,y;primf(”Inpu(a.b、c:”);scanf(M%f,%f%f\&a.&b.&c); x=(-b+sqrt(b*b-4*a*c))/(2*a); y=(-b-sqrt(b*b-4*a*c))/(2*a);printf(H x=%ty=%f\n M,x,y);}4.1(1) #include<stdio.h>niain(){char c I='if,c2=b,c3='c:printf(°a%cb%cc%c\n,\c 1 ,c2,c3);}4.1(2) #include<stdio.h>niain()int a=12,b=15;printf(H a=%d%%,b=%d%%\n\a,b); )4.1(3) #include<stdio.h> main(){int a,b;scanf(n%2d%*2s%2d M,&a.&b); printf(u%d.%d\n,\a,b); }4.2 #include<stdio.h>niain(){long a.b;float x,y; scanf(n%d,%d\n*\&a,&b);scanf(,,%f,%f\n,\&x.&y); printf(u a=%d,b=%d\n,\a,b):printf(H x=%tb=%f\n,\x,y);}5.1#include<stdio.h>niain(){float a;printf(u Innputa:H); scanf(M%f\&a);if(a>=0){a=a:printf(H a=%f\n H,a);}else{a=-a;printf(,,a=%f\n,\a);})5.2#include<stdio.h>main(){int a;printf(H Inputa:M);scanf(M%d M.&a);if(a%2==0){printf(°a 是偶数”);}else{ printf(u a 是奇数”);}}53 #include<stdio.h>#include<math.h>inain(){float a,b,c,s,area;primf(”Inpu(abc:”); scanf(H%f,%L%f\&a.&b.&c);if(a+b>c&&a+c>b&&b+c>a)s=(a+b+c)/2; area=(float)sqrt(s*(s-a)*(s-b)*(s-c)); printf(,,area=%f\n,\area);}else{ printf(H不是三角形”);}}5.4#include<stdio.h>#include<math.h>niain(){float a.b、c,x,y;printf(,,Inputa.bx:H);if(a==O){primf(”该方程不是一元二次方程\n”);} if(b*b-4*a*c>0){ x=(-b+sqrt(b*b-4*a*c))/(2*a); y=(-b-sqrt(b*b-4*a*c))/(2*a); printf(,,x=%f,y=%f\n H,x,y);}else if(b*b-4*a*c==0){x=-b/(2*a);y=-b/(2*a): printf(,,x=%fy=%f\n H,x,y);}else{ printfC*该方程无实根\n”);})5.5#include<stdio.h>main(){int yearflag;printf(n Input a year:0); scanf(,,%d M.&year);if(year%4==0&&vear%400!=0llyear%400==0) {flag=l;}else{flag=O;} if(flag=l){- printf(H%d is a leap year !\n H,year);}else{ printf(H%d is not a leap year !\n M,year);}}5.6#include<stdio.h>main()int yeanflag; printf(M Input a year:0); scanf(,,%d H,&year);flag=year%400==0llyear%4==0&&year%100!=0? 1:0;if(flag=l&&flag!=O){〜 ~printf(u%d is a leap year !\n f\vear);}else{ printf(u%d is not a leap year !\n*\year);}}5.7#include<stdio.h>inain() { char ch: printf(°I nputch:"); scanf(H%c*\&ch);if(ch>=,a,&&ch<=,z,) { ch=getchar(); ch=ch-32;printf(n%c.%d\n'\ch,ch);}else if(ch>=*A'&&chv=Z){ch=getchar(); ch=ch+32;printf(H%c.%d\n,\ch,ch);}else{ primiT%c”,ch);}}5.8#include<stdio.h>main() {char ch;printf(u I nputch:,r); scanf(”%c”,&ch);if(ch>=48&&ch<=57){printf(Hch是数字字符\nj;}else if(ch>=65&&ch<=90){printf(Hch是大写字母\nj;}else if(ch>=97&&ch<=122){printf(Hch是小写字母\nj;}else if(ch=32)printf(°ch 是空格\nj;}else printf(°ch是瓦他字符\n J;}5.9#include<stdio.h>main(){int score.gradc; printf(u Input score:H);scanf(H%d H,&score);grade=score/10; if(score<Ollscore> 100){printfC'I nput error\n H);}if(score>=90&&score<=100){ printf(u%d-A\n,\score);}else if(score>=80&&score<90){printf(u%d-B\n,\score);}else if(score>=70&&score<80){printf(,,%d-C\n M,score);}else if(score>=60&&score<70){printf(H%d-D\n H,score);}else if(score>=0&&score<60){ printf(u%d- -E\n M,score);}}5.10#include<stdio.h>inain(){int yeanmonth: printf(°Input year,month:”);scanf(H%d,%d H.&yean &m onth); if(month> 12llmonth<=0) {printf(H error month\n M);}else{switch(year,month){case 12:case 10:case &case 7:case 5:case 3:case 1:printf(,r31 天5"); break;case 11:case 9:case 6:case 4:printf(n30 天\n»);break:case 2:if(year%4==0&&year!=0llyear%400==0) {printf(H29 天\nj;}else{ printf(H28 天\nj;}break;default:printf(*'I 叩ut error\n H);}}}6.1(1) #include<stdio.h>inain(){int ij,k;char space=* :for(i=l;i<=4;i++){ for(j=l;j<=i;j++) Iprimf("%c”,space);} for(k=l;k<=6;k++) {printfC*");} printf(°\n M);})6.1 (2) #include<stdio.h>main(){int k=4,n;for(n=0;n<k:n++){if(n%2==0) continue: k—;} printf(u k=%d\nji=%d\n,\k,n);}6.1(3) #include<stdio.h>niain(){int k=4,n;for(n=0;n<k:n++){if(n%2==0) break; k—;}printf(u k=%d.n=%d\n,\k.n);}6.2(1) #include<stdio.h>niain()int i,sum=O;for(i=l;i<=101;i++){ sum=sum+i;}printf(,,sum=%d\n,\sum);}6.2(2) #include<stdio.h>main(){long i;long ternksum=O;for(i= I ;i<= 101 ;i=i+2){ term=i*(i+l)*(i+2); sum=sum+term:} printf(,,sum=%ld\n*\sum);}6.2(4) #include<stdio.h>#include<math.h>niain(){int n=l;float term= 1.0.sign= 1 ,sum=O; while(term<=-1 e-4llterm>= 1 c-4) {term=1.0/sign; sum=sum+term: sign=sign+n;n++;} printf(,,sum=%f\n,\sum);}6.2(5) #include<stdio.h>#include<math.h>inain(){int n=Lcount=l;float x;double suniterni: printfCI nput x:N); scanf(H%f\&x);sum=x;term=x;do{ term=-term*x*x/((n+1 )*(n+2)); sum=sum+term;n=n+2; count++;} while(fabs(term)>= 1 e-5); printf(H sin(x>%f,count=%d\n",sum,count); } 6.3#include<stdio.h>niain(){int x=l,find=0;while(!find)if(x%2==l&&x%3==2&&x%5==4&&x%6==5&&x%7==0)printf(u x=%d\n M,x);find=l;x++;}}}/* int x,find=O:for (x=l;!find;x++){if(x%2==l&&x%3==2&&x%5==4&&x%6==5&&x%7==0) {printf(u x=%d\n M,x);find=l;}}}*/6.4#include<stdio.h>niainO{int i.n;long p=Lm=l;printf(u I nput n:N);scanf(M%d M.&n);for(i=l;i<=n:i++){p=i*i;printf(,,p=%d.m=%d\n,\i.p,i.m);}}6.5#includc<stdio.h>main(){float c,f;for(c=-40;c<=l 10;c=c+10){f=9/5*c+32;printf(,,f=%f\n,\f);}}6.6#include<stdio.h>#include<math.h>niainO{int n;double c=0.01875.x;do{x=x*pow(l+cj 2)-1000;n++;}whiIe(x>0);printf(,,x=%d\n,\x);}6.7#include<stdio.h>niainO{int n=0;float a=100.0,c;printf(H I nputc:,r);scanf(H%f\&c);doa=a*(l+c);n++; }while(a<=200);printf(H n=%d\n,\n);}6.8#include<stdio.h>#include<math.h>main(){int n=l,count=l;double sum= 1 ,term= 1;。
c语言程序设计苏小红课后答案
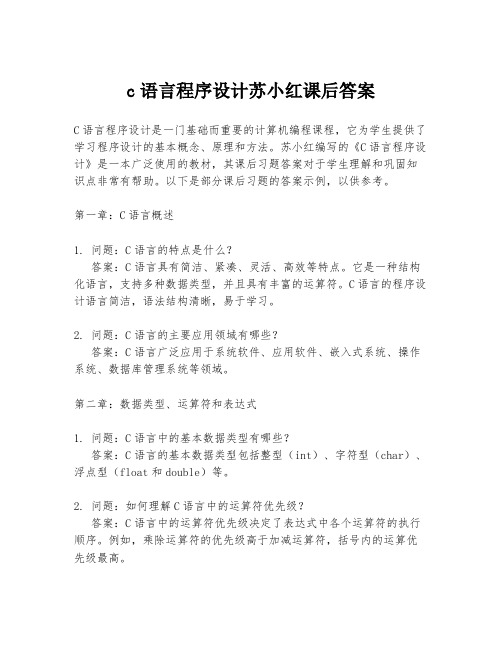
c语言程序设计苏小红课后答案C语言程序设计是一门基础而重要的计算机编程课程,它为学生提供了学习程序设计的基本概念、原理和方法。
苏小红编写的《C语言程序设计》是一本广泛使用的教材,其课后习题答案对于学生理解和巩固知识点非常有帮助。
以下是部分课后习题的答案示例,以供参考。
第一章:C语言概述1. 问题:C语言的特点是什么?答案:C语言具有简洁、紧凑、灵活、高效等特点。
它是一种结构化语言,支持多种数据类型,并且具有丰富的运算符。
C语言的程序设计语言简洁,语法结构清晰,易于学习。
2. 问题:C语言的主要应用领域有哪些?答案:C语言广泛应用于系统软件、应用软件、嵌入式系统、操作系统、数据库管理系统等领域。
第二章:数据类型、运算符和表达式1. 问题:C语言中的基本数据类型有哪些?答案:C语言的基本数据类型包括整型(int)、字符型(char)、浮点型(float和double)等。
2. 问题:如何理解C语言中的运算符优先级?答案:C语言中的运算符优先级决定了表达式中各个运算符的执行顺序。
例如,乘除运算符的优先级高于加减运算符,括号内的运算优先级最高。
第三章:控制结构1. 问题:C语言中的三种基本控制结构是什么?答案:C语言中的三种基本控制结构是顺序结构、选择结构和循环结构。
2. 问题:if语句的基本形式有哪些?答案:if语句的基本形式包括单分支if语句、双分支if-else语句和多分支if-else if-else语句。
第四章:数组1. 问题:什么是一维数组?答案:一维数组是具有相同数据类型元素的集合,这些元素在内存中连续存储,可以通过索引访问。
2. 问题:如何声明和初始化一个一维数组?答案:声明一维数组的语法是 `type arrayName[arraySize];`。
初始化可以使用 `{value1, value2, ...}` 的方式,例如 `intarr[5] = {1, 2, 3, 4, 5};`。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
的最大值32767
产生[0,b-1] 之间的随机数 – magic = rand()%b; 产生[a,a+b-1] 之间的随机数 – magic = rand()%b + a;
2014-4-20 31/62
#include <stdlib.h> #include <stdio.h> main() { int magic; /*计算机"想"的数*/ int guess; /*人猜的数*/ magic = rand()%100 + 1;
1!, 2!, 3!, … , n!
2014-4-20
24/62
【例6.4】输入n值,计算并输出
1! + 2! + 3! + … + n!
利用前项 计算后项
2014-4-20
25/62
【例6.4】输入n值,计算并输出
1! + 2! + 3! + … + n!
每次单独计算 累加项
2014-4-20
test counter true
false
循环体 (Body of Loop)
step x update counter step n
2014-4-20 7/62
for循环语句
当型循环——Condition is tested first 计数控制 ——Loop is controlled by a counter 循环起始条件 循环结束条件 循环变量增值 Syntax for (initial value ; condition; update counter) 循环变量控制循环次 statement;
2014-4-20 33/62
猜数游戏用到的库函数
每次运行程序时计算机所“想”的数都是一样的, 这是什么原因呢? – 函数rand()产生的只是伪随机数 随机函数srand – 为函数rand()设置随机数种子来实现对函数rand所产
生的伪随机数的 “随机化”
通过输入随机数种子,产生[0,100]之间的随机数
counter<6 true input n sum ← sum + n counter++ output sum
n
2 3 5 6 4
counter-controlled 计数器每次增1
使用了3个变量
2014-4-20
end
6/62
6.2计数控制的循环
counter ← initial value
Or
数,不要在循环体内 改变这个变量的值
for (initial value ; condition; update counter) 复合语句 { compound statement statement; 被当做一条语句看待 statement; 2014-4-20 }
8/62
start
i ← 0, sum ← 0
scanf("%u", &seed); srand(seed); magic = rand() % 100 + 1;
2014-4-20
34/62
#include <stdlib.h> #include <stdio.h> main() { int magic; int guess; int counter; /*记录人猜次数的计数器变量*/ unsigned int seed; printf("Please enter seed:"); scanf("%u", &seed); srand(seed); magic = rand() % 100 + 1; counter = 0; /*计数器变量count初始化为0*/ do{ printf("Please guess a magic number:"); scanf("%d", &guess); counter ++; /*计数器变量count加1*/ if (guess > magic) printf("Wrong! Too high!\n"); else if (guess < magic) printf("Wrong! Too low!\n"); else printf("Right!\n"); }while (guess != magic); printf("counter = %d \n", counter); 2014-4-20 }
第6章 循环控制结构
哈尔滨工业大学
计算机科学与技术学院
苏小红 sxh@
本章学习内容
计数控制的循环 条件控制的循环 for语句,while语句,do-while语句 continue语句,break语句 嵌套循环 结构化程序设计的基本思想 程序调试与排错
主要用在循环语句中,同时对多个变量赋初值等
for (i = 1 , j = 100; i < j; i++, j--)
循环起始条件
2014-4-20
循环结束条件
循环变量增值
22/62
【例6.2】计算并输出 n! = 1 × 2 × 3 × … × n
2014-4-20
23/62
【例6.3】计算并输出
【例6.1】计算并输出1+2+3+…+n的值
循环次数已知,计数控制的循环
sum = 0的作用?
2014-4-20
14/62
【例6.1】计算并输出1+2+3+…+n的值
循环次数已知,计数控制的循环
2014-4-20
15/62
【例6.1】计算并输出1+2+3+…+n的值
2014-4-20
循环条件第一次就为假(如 输入-1)时会怎样?
标记控制 Sentinel Controlled
5/62
Assume input example: 2 3 4 5 6
start
6.2计数控制的循环
counter sum false 1 2 3 6 4< 5 <6 6 true false true
counter ← 1, sum ← 0
1 2 3 4 6 5 14 20 0 2 14 5 9 0+5 2 5 9 2 3 4 6
语法
do{
statement; statement;
Don’t forget the semicolon!!
} while (condition) ;
statement;
2014-4-20 12/62
【例6.1】计算并输出1+2+3+…+n的值
循环次数已知,计数控制的循环
2014-4-20
13/62
【例6.1】计算并输出1+2+3+…+n的值
如何减少循环的次数?
2014-4-20
20/62
【例6.1】计算并输出1+2+3+…+n的值
2014-4-20
21/62
逗号运算符(Comma Operator)
表达式1, 表达式2, … , 表达式n
多数情况下,并不使用整个逗号表达式的值,更 常见的情况是要分别得到各表达式的值
只猜1次
例6.6
/*“想”一个[1,100]之间的数magic*/
printf("Please guess a magic number:"); scanf("%d", &guess); if (guess > magic) { printf("Wrong! Too high!\n"); } else if (guess < magic) { printf("Wrong! Too low!\n"); } else { printf("Right! \n"); printf("The number is:%d \n", magic); } 2014-4-20
26/62
6.3嵌套循环
使用嵌套循环的注意事项 使用复合语句,以保证逻辑上的正确性 – 即用一对花括号将各层循环体语句括起来 内层和外层循环控制变量不能同名,以免造 成混乱 采用右缩进格式书写,以保证层次的清晰性
2014-4-20
27/62
选择三种循环的一般原则
如果循环次数已知,计数控制的循环 – 用for
false
for循环语句
int i, sum, n; sum = 0; for (i = 0; i < 5; i++) { scanf(“%d”, &n); sum = sum + n; } printf(“%d”, sum);
9/62
i< 5
true
input n sum←sum+ n i++ output sum
2014-4-20
end
条件控制的循环
当 型 循 环
假 假 真 假
条 件P
A
条 件P
真
A
直 到 型 循 环
2014-4-20
10/62
while循环语句
当型循环——Condition is tested first