JAVA堆排序和几种排序方法实现代码
java稳定的排序方法

java稳定的排序方法
Java是一种广泛使用的编程语言,其中排序是常见的操作。
在排序中,稳定性是一个重要的概念。
稳定的排序算法可以保留相等元素的原始顺序,而不稳定的排序算法不保证这一点。
下面介绍几种Java中稳定的排序方法:
1. 冒泡排序:该算法的基本思想是通过交换相邻的元素来将较大的元素逐步“冒泡”到数组的末尾。
冒泡排序是一种简单但效率较低的排序算法,时间复杂度为O(n^2)。
2. 插入排序:该算法的基本思想是将数组分为有序和无序两部分,从无序部分依次取出一个元素插入到有序部分的适当位置。
插入排序的时间复杂度也是O(n^2),但在实际应用中,它比冒泡排序更常用。
3. 归并排序:该算法的基本思想是将待排序数组分成两个子数组,并将每个子数组递归地进行排序,然后再将它们合并成一个有序数组。
归并排序的时间复杂度为O(nlogn),但它需要额外的空间来存储子数组。
4. 堆排序:该算法的基本思想是将待排序数组构建为一个最大堆(或最小堆),然后不断取出堆顶元素并重新调整堆,直到所有元素都被取出。
堆排序的时间复杂度为O(nlogn),但也需要额外的空间来存储堆。
总的来说,以上排序方法都是稳定的。
在实际应用中,我们需要根据数据规模、数据类型和性能需求等因素来选择适当的排序算法。
java倒序排序方法

java倒序排序方法java语言是一种面向对象的编程语言,具有强大的排序功能。
在java中,倒序排序是非常常见的操作,有多种实现方法。
一、使用Collections.reverseOrder()方法java中的Collections类提供了reverseOrder()方法,可以用于倒序排序,该方法返回一个比较器,可以将一个对象列表按照指定的顺序进行排序。
示例代码如下所示:```javaimport java.util.ArrayList;import java.util.Collections;import java.util.List;public class ReverseSortExample {public static void main(String[] args) {List<Integer> numbers = new ArrayList<>();numbers.add(5);numbers.add(2);numbers.add(9);numbers.add(1);numbers.add(7);System.out.println("排序前:" + numbers); Collections.sort(numbers, Collections.reverseOrder()); System.out.println("排序后:" + numbers);}}```输出结果如下所示:```排序前:[5, 2, 9, 1, 7]排序后:[9, 7, 5, 2, 1]```在这个示例中,我们创建了一个包含一些整数的列表,并使用Collections类的sort()方法对其进行排序。
通过传递`Collections.reverseOrder()`作为比较器参数,可以实现倒序排序。
值得注意的是,reverseOrder()方法返回的是一个比较器,它会根据元素的自然顺序进行排序。
arrays.sort();的多种使用方法

arrays.sort();的多种使用方法(原创版4篇)目录(篇1)1.引言2.`arrays.sort()`函数的基本用法3.`arrays.sort()`函数的多种使用方法4.结论正文(篇1)数组的排序在现代编程中是常见的操作。
Java的`arrays.sort()`函数提供了一种方便的方式来对数组进行排序。
它具有多种使用方法,能够满足不同类型和规模数组的排序需求。
以下是`arrays.sort()`函数的多种使用方法。
1.数组元素的直接比较排序t* 这是`arrays.sort()`函数的基本用法,它通过比较数组元素的值来进行排序。
t* 例如,对于一个整型数组,可以使用以下代码进行排序:t```tarduino`int[] arr = {5, 2, 8, 1, 9};tArrays.sort(arr); // 按照从小到大的顺序对数组进行排序`t```2.使用自定义的比较器进行排序t* 如果需要按照自定义的规则进行排序,可以使用`Arrays.sort()`函数的第二个参数,即自定义的比较器。
t* 比较器是一个函数,它接受两个参数,并返回一个整数值表示它们的相对顺序。
t* 例如,对于一个字符串数组,可以使用以下代码进行排序:t```tarduino`String[] arr = {"apple", "banana", "orange", "grape"};tArrays.sort(arr, new Comparatoru003cStringu003e() {t @Overridet public int compare(String s1, String s2) {t return s1.length() - s2.length(); // 按长度排序` t});`t```3.使用自定义的排序算法进行排序t* 如果需要使用自定义的排序算法进行排序,可以使用`Arrays.sort()`函数的第三个参数,即自定义的比较器。
java的几种排序方式

java的几种排序方式用Java语言实现的各种排序,包括插入排序、冒泡排序、选择排序、Shell排序、快速排序、归并排序、堆排序、SortUtil等。
插入排序:package org.rut.util.algorithm.support;import org.rut.util.algorithm.SortUtil;/*** @author treeroot* @since 2006-2-2* @version 1.0*/public class InsertSort implements SortUtil.Sort{/* (non-Javadoc)* @see org.rut.util.algorithm.SortUtil.Sort#sort(int[])*/public void sort(int[] data) {int temp;for(int i=1;i for(int j=i;(j>0)&&(data[j] SortUtil.swap(data,j,j-1);}}}}冒泡排序:package org.rut.util.algorithm.support;import org.rut.util.algorithm.SortUtil;/*** @author treeroot* @since 2006-2-2* @version 1.0*/public class BubbleSort implements SortUtil.Sort{/* (non-Javadoc)* @see org.rut.util.algorithm.SortUtil.Sort#sort(int[])*/public void sort(int[] data) {int temp;for(int i=0;i for(int j=data.length-1;j>i;j--){if(data[j] SortUtil.swap(data,j,j-1);}}}}}选择排序:package org.rut.util.algorithm.support;import org.rut.util.algorithm.SortUtil;/*** @author treeroot* @since 2006-2-2* @version 1.0*/public class SelectionSort implements SortUtil.Sort { /** (non-Javadoc)** @see org.rut.util.algorithm.SortUtil.Sort#sort(int[]) */public void sort(int[] data) {int temp;for (int i = 0; i < data.length; i++) {int lowIndex = i;for (int j = data.length - 1; j >i; j--) {if (data[j] < data[lowIndex]) {lowIndex = j;}}SortUtil.swap(data,i,lowIndex);}}}Shell排序:package org.rut.util.algorithm.support;import org.rut.util.algorithm.SortUtil;/*** @author treeroot* @since 2006-2-2* @version 1.0*/public class ShellSort implements SortUtil.Sort{/* (non-Javadoc)* @see org.rut.util.algorithm.SortUtil.Sort#sort(int[]) */public void sort(int[] data) {for(int i=data.length/2;i>2;i/=2){for(int j=0;j insertSort(data,j,i);}}insertSort(data,0,1);/*** @param data* @param j* @param i*/private void insertSort(int[] data, int start, int inc) {int temp;for(int i=start+inc;i for(int j=i;(j>=inc)&&(data[j] SortUtil.swap(data,j,j-inc); }}}}快速排序:package org.rut.util.algorithm.support;import org.rut.util.algorithm.SortUtil;/*** @author treeroot* @since 2006-2-2* @version 1.0*/public class QuickSort implements SortUtil.Sort{/* (non-Javadoc)* @see org.rut.util.algorithm.SortUtil.Sort#sort(int[])*/public void sort(int[] data) {quickSort(data,0,data.length-1);}private void quickSort(int[] data,int i,int j){int pivotIndex=(i+j)/2;//swapSortUtil.swap(data,pivotIndex,j);int k=partition(data,i-1,j,data[j]);SortUtil.swap(data,k,j);if((k-i)>1) quickSort(data,i,k-1);if((j-k)>1) quickSort(data,k+1,j);}/*** @param data* @param i* @param j* @return*/private int partition(int[] data, int l, int r,int pivot) {while(data[++l] while((r!=0)&&data[--r]>pivot);SortUtil.swap(data,l,r);}while(l SortUtil.swap(data,l,r);return l;}}改进后的快速排序:package org.rut.util.algorithm.support;import org.rut.util.algorithm.SortUtil;/*** @author treeroot* @since 2006-2-2* @version 1.0*/public class ImprovedQuickSort implements SortUtil.Sort { private static int MAX_STACK_SIZE=4096;private static int THRESHOLD=10;/* (non-Javadoc)* @see org.rut.util.algorithm.SortUtil.Sort#sort(int[]) */public void sort(int[] data) {int[] stack=new int[MAX_STACK_SIZE];int top=-1;int pivot;int pivotIndex,l,r;stack[++top]=0;stack[++top]=data.length-1;while(top>0){int j=stack[top--];int i=stack[top--];pivotIndex=(i+j)/2;pivot=data[pivotIndex];SortUtil.swap(data,pivotIndex,j);//partitionl=i-1;r=j;do{while(data[++l] while((r!=0)&&(data[--r]>pivot)); SortUtil.swap(data,l,r);}while(l SortUtil.swap(data,l,r);SortUtil.swap(data,l,j);if((l-i)>THRESHOLD){stack[++top]=i;stack[++top]=l-1;}if((j-l)>THRESHOLD){stack[++top]=l+1;stack[++top]=j;}}//new InsertSort().sort(data);insertSort(data);}/*** @param data*/private void insertSort(int[] data) {int temp;for(int i=1;i for(int j=i;(j>0)&&(data[j] SortUtil.swap(data,j,j-1); }}}}归并排序:package org.rut.util.algorithm.support;import org.rut.util.algorithm.SortUtil;/*** @author treeroot* @since 2006-2-2* @version 1.0*/public class MergeSort implements SortUtil.Sort{/* (non-Javadoc)* @see org.rut.util.algorithm.SortUtil.Sort#sort(int[])*/public void sort(int[] data) {int[] temp=new int[data.length];mergeSort(data,temp,0,data.length-1);}private void mergeSort(int[] data,int[] temp,int l,int r){int mid=(l+r)/2;if(l==r) return ;mergeSort(data,temp,l,mid);mergeSort(data,temp,mid+1,r);for(int i=l;i<=r;i++){temp=data;}int i1=l;int i2=mid+1;for(int cur=l;cur<=r;cur++){if(i1==mid+1)data[cur]=temp[i2++];else if(i2>r)data[cur]=temp[i1++];else if(temp[i1] data[cur]=temp[i1++];elsedata[cur]=temp[i2++];}}}改进后的归并排序:package org.rut.util.algorithm.support;import org.rut.util.algorithm.SortUtil;/*** @author treeroot* @since 2006-2-2* @version 1.0*/public class ImprovedMergeSort implements SortUtil.Sort { private static final int THRESHOLD = 10;/** (non-Javadoc)** @see org.rut.util.algorithm.SortUtil.Sort#sort(int[])*/public void sort(int[] data) {int[] temp=new int[data.length];mergeSort(data,temp,0,data.length-1);}private void mergeSort(int[] data, int[] temp, int l, int r) { int i, j, k;int mid = (l + r) / 2;if (l == r)return;if ((mid - l) >= THRESHOLD)mergeSort(data, temp, l, mid);elseinsertSort(data, l, mid - l + 1);if ((r - mid) >THRESHOLD)mergeSort(data, temp, mid + 1, r);elseinsertSort(data, mid + 1, r - mid);for (i = l; i <= mid; i++) {temp = data;}for (j = 1; j <= r - mid; j++) {temp[r - j + 1] = data[j + mid];}int a = temp[l];int b = temp[r];for (i = l, j = r, k = l; k <= r; k++) {if (a < b) {data[k] = temp;a = temp;} else {data[k] = temp[j--];b = temp[j];}}}/*** @param data* @param l* @param i*/private void insertSort(int[] data, int start, int len) {for(int i=start+1;i for(int j=i;(j>start) && data[j] SortUtil.swap(data,j,j-1); }}}}堆排序:package org.rut.util.algorithm.support;import org.rut.util.algorithm.SortUtil;/*** @author treeroot* @since 2006-2-2* @version 1.0*/public class HeapSort implements SortUtil.Sort{/* (non-Javadoc)* @see org.rut.util.algorithm.SortUtil.Sort#sort(int[])public void sort(int[] data) {MaxHeap h=new MaxHeap();h.init(data);for(int i=0;i h.remove();System.arraycopy(h.queue,1,data,0,data.length); }private static class MaxHeap{void init(int[] data){this.queue=new int[data.length+1];for(int i=0;i queue[++size]=data;fixUp(size);}}private int size=0;private int[] queue;public int get() {return queue[1];}public void remove() {SortUtil.swap(queue,1,size--);fixDown(1);}//fixdownprivate void fixDown(int k) {int j;while ((j = k << 1) <= size) {if (j < size && queue[j] j++;if (queue[k]>queue[j]) //不用交换break;SortUtil.swap(queue,j,k);k = j;}}private void fixUp(int k) {while (k >1) {int j = k >>1;if (queue[j]>queue[k])break;SortUtil.swap(queue,j,k);k = j;}}}SortUtil:package org.rut.util.algorithm;import org.rut.util.algorithm.support.BubbleSort;import org.rut.util.algorithm.support.HeapSort;import org.rut.util.algorithm.support.ImprovedMergeSort;import org.rut.util.algorithm.support.ImprovedQuickSort;import org.rut.util.algorithm.support.InsertSort;import org.rut.util.algorithm.support.MergeSort;import org.rut.util.algorithm.support.QuickSort;import org.rut.util.algorithm.support.SelectionSort;import org.rut.util.algorithm.support.ShellSort;/*** @author treeroot* @since 2006-2-2* @version 1.0*/public class SortUtil {public final static int INSERT = 1;public final static int BUBBLE = 2;public final static int SELECTION = 3;public final static int SHELL = 4;public final static int QUICK = 5;public final static int IMPROVED_QUICK = 6;public final static int MERGE = 7;public final static int IMPROVED_MERGE = 8;public final static int HEAP = 9;public static void sort(int[] data) {sort(data, IMPROVED_QUICK);}private static String[] name={"insert", "bubble", "selection", "shell", "quick", "improved_quick", "merge", "improved_merge", "heap"};private static Sort[] impl=new Sort[]{new InsertSort(),new BubbleSort(),new SelectionSort(),new ShellSort(),new QuickSort(),new ImprovedQuickSort(),new MergeSort(),new ImprovedMergeSort(),new HeapSort()public static String toString(int algorithm){return name[algorithm-1];}public static void sort(int[] data, int algorithm) { impl[algorithm-1].sort(data);}public static interface Sort {public void sort(int[] data);}public static void swap(int[] data, int i, int j) { int temp = data;data = data[j];data[j] = temp;}}。
java arraylist排序方法

java arraylist排序方法Java中的ArrayList是一种动态数组,它可以根据需要自动调整大小。
有时,我们需要对ArrayList中的元素进行排序,以便更好地进行数据处理。
在本文中,我们将介绍几种Java中ArrayList排序的方法。
1. 使用Collections.sort()方法Collections.sort()方法可用于对ArrayList进行排序。
该方法使用默认排序顺序对列表中的元素进行排序。
示例代码:```import java.util.ArrayList;import java.util.Collections;public class ArrayListSortingExample {public static void main(String[] args) {ArrayList<String> fruitsList = new ArrayList<String>(); fruitsList.add('Apple');fruitsList.add('Orange');fruitsList.add('Banana');fruitsList.add('Pineapple');fruitsList.add('Kiwi');// Sort the ArrayListCollections.sort(fruitsList);// Print the sorted ArrayListSystem.out.println('Sorted ArrayList: ');for (String fruit : fruitsList) {System.out.println(fruit);}}}```输出结果:```Sorted ArrayList:AppleBananaKiwiOrangePineapple```2. 使用自定义比较器进行排序如果我们需要使用自定义排序顺序对ArrayList中的元素进行排序,我们可以使用Comparator接口和Collections.sort()方法的重载版本。
java中sort方法

java中sort方法Java中sort方法1. 简介在Java中,sort方法是用于对数组或集合进行排序的常用方法。
它可以按照自然顺序或者指定的比较器来排序,使得元素按照一定的规则排列。
本文将详细介绍sort方法的用法和不同的排序方式。
2. 使用方法public static <T> void sort(List<T> list)public static <T> void sort(List<T> list, Comparator<? s uper T> c)public static void sort(int[] a)public static void sort(int[] a, int fromIndex, int toIn dex)public static void sort(long[] a)public static void sort(long[] a, int fromIndex, int toI ndex)public static void sort(short[] a)public static void sort(short[] a, int fromIndex, int to Index)public static void sort(char[] a)public static void sort(char[] a, int fromIndex, int toI ndex)public static void sort(byte[] a)public static void sort(byte[] a, int fromIndex, int toI ndex)public static void sort(float[] a)public static void sort(float[] a, int fromIndex, int to Index)public static void sort(double[] a)public static void sort(double[] a, int fromIndex, int t oIndex)public static <T> void sort(T[] a)public static <T> void sort(T[] a, int fromIndex, int to Index)sort方法有多个重载。
十大排序算法
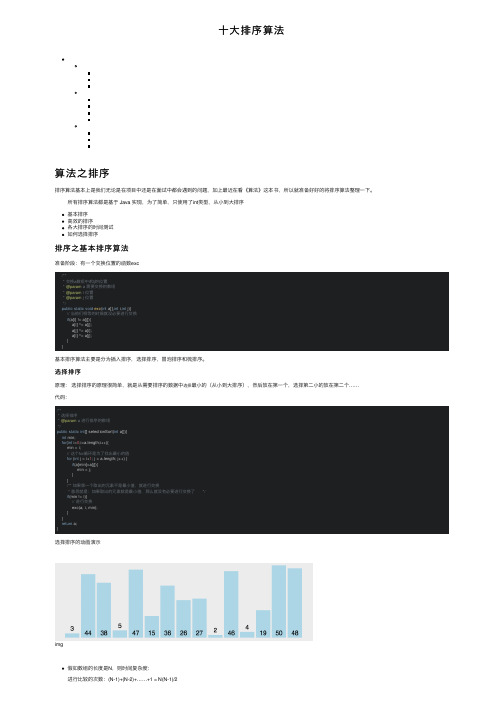
⼗⼤排序算法算法之排序排序算法基本上是我们⽆论是在项⽬中还是在⾯试中都会遇到的问题,加上最近在看《算法》这本书,所以就准备好好的将排序算法整理⼀下。
所有排序算法都是基于 Java 实现,为了简单,只使⽤了int类型,从⼩到⼤排序基本排序⾼效的排序各⼤排序的时间测试如何选择排序排序之基本排序算法准备阶段:有⼀个交换位置的函数exc/*** 交换a数组中i和j的位置* @param a 需要交换的数组* @param i 位置* @param j 位置*/public static void exc(int a[],int i,int j){// 当他们相等的时候就没必要进⾏交换if(a[i] != a[j]){a[i] ^= a[j];a[j] ^= a[i];a[i] ^= a[j];}}基本排序算法主要是分为插⼊排序,选择排序,冒泡排序和梳排序。
选择排序原理:选择排序的原理很简单,就是从需要排序的数据中选择最⼩的(从⼩到⼤排序),然后放在第⼀个,选择第⼆⼩的放在第⼆个……代码:/*** 选择排序* @param a 进⾏排序的数组*/public static int[] selectionSort(int a[]){int min;for(int i=0;i<a.length;i++){min = i;// 这个for循环是为了找出最⼩的值for (int j = i+1; j < a.length; j++) {if(a[min]>a[j]){min = j;}}/** 如果第⼀个取出的元素不是最⼩值,就进⾏交换* 意思就是:如果取出的元素就是最⼩值,那么就没有必要进⾏交换了 */if(min != i){// 进⾏交换exc(a, i, min);}}return a;}选择排序的动画演⽰img假如数组的长度是N,则时间复杂度:进⾏⽐较的次数:(N-1)+(N-2)+……+1 = N(N-1)/2进⾏交换的次数:N特点:(稳定)1. 运⾏时间与输⼊⽆关。
用Java实现常见的8种内部排序算法
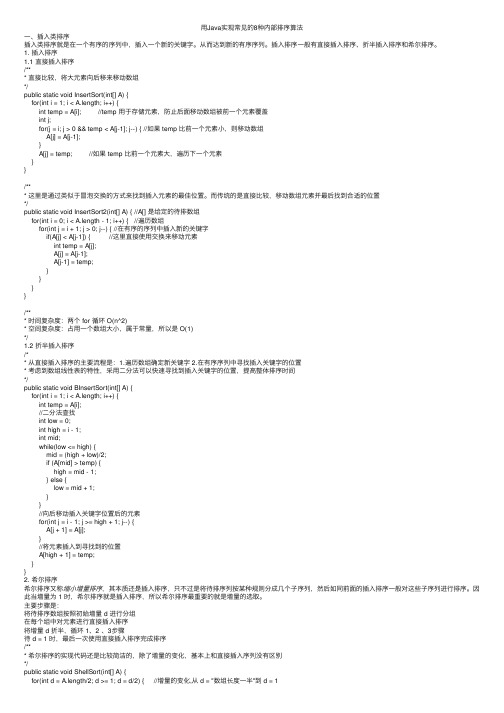
⽤Java实现常见的8种内部排序算法⼀、插⼊类排序插⼊类排序就是在⼀个有序的序列中,插⼊⼀个新的关键字。
从⽽达到新的有序序列。
插⼊排序⼀般有直接插⼊排序、折半插⼊排序和希尔排序。
1. 插⼊排序1.1 直接插⼊排序/*** 直接⽐较,将⼤元素向后移来移动数组*/public static void InsertSort(int[] A) {for(int i = 1; i < A.length; i++) {int temp = A[i]; //temp ⽤于存储元素,防⽌后⾯移动数组被前⼀个元素覆盖int j;for(j = i; j > 0 && temp < A[j-1]; j--) { //如果 temp ⽐前⼀个元素⼩,则移动数组A[j] = A[j-1];}A[j] = temp; //如果 temp ⽐前⼀个元素⼤,遍历下⼀个元素}}/*** 这⾥是通过类似于冒泡交换的⽅式来找到插⼊元素的最佳位置。
⽽传统的是直接⽐较,移动数组元素并最后找到合适的位置*/public static void InsertSort2(int[] A) { //A[] 是给定的待排数组for(int i = 0; i < A.length - 1; i++) { //遍历数组for(int j = i + 1; j > 0; j--) { //在有序的序列中插⼊新的关键字if(A[j] < A[j-1]) { //这⾥直接使⽤交换来移动元素int temp = A[j];A[j] = A[j-1];A[j-1] = temp;}}}}/*** 时间复杂度:两个 for 循环 O(n^2)* 空间复杂度:占⽤⼀个数组⼤⼩,属于常量,所以是 O(1)*/1.2 折半插⼊排序/** 从直接插⼊排序的主要流程是:1.遍历数组确定新关键字 2.在有序序列中寻找插⼊关键字的位置* 考虑到数组线性表的特性,采⽤⼆分法可以快速寻找到插⼊关键字的位置,提⾼整体排序时间*/public static void BInsertSort(int[] A) {for(int i = 1; i < A.length; i++) {int temp = A[i];//⼆分法查找int low = 0;int high = i - 1;int mid;while(low <= high) {mid = (high + low)/2;if (A[mid] > temp) {high = mid - 1;} else {low = mid + 1;}}//向后移动插⼊关键字位置后的元素for(int j = i - 1; j >= high + 1; j--) {A[j + 1] = A[j];}//将元素插⼊到寻找到的位置A[high + 1] = temp;}}2. 希尔排序希尔排序⼜称缩⼩增量排序,其本质还是插⼊排序,只不过是将待排序列按某种规则分成⼏个⼦序列,然后如同前⾯的插⼊排序⼀般对这些⼦序列进⾏排序。
Java实现堆排序(大根堆)

Java实现堆排序(⼤根堆) 堆排序是⼀种树形选择排序⽅法,它的特点是:在排序的过程中,将array[0,...,n-1]看成是⼀颗完全⼆叉树的顺序存储结构,利⽤完全⼆叉树中双亲节点和孩⼦结点之间的内在关系,在当前⽆序区中选择关键字最⼤(最⼩)的元素。
1. 若array[0,...,n-1]表⽰⼀颗完全⼆叉树的顺序存储模式,则双亲节点指针和孩⼦结点指针之间的内在关系如下: 任意⼀节点指针 i:⽗节点:i==0 ? null : (i-1)/2 左孩⼦:2*i + 1 右孩⼦:2*i + 22. 堆的定义:n个关键字序列array[0,...,n-1],当且仅当满⾜下列要求:(0 <= i <= (n-1)/2) ① array[i] <= array[2*i + 1] 且 array[i] <= array[2*i + 2];称为⼩根堆; ② array[i] >= array[2*i + 1] 且 array[i] >= array[2*i + 2];称为⼤根堆;3. 建⽴⼤根堆: n个节点的完全⼆叉树array[0,...,n-1],最后⼀个节点n-1是第(n-1-1)/2个节点的孩⼦。
对第(n-1-1)/2个节点为根的⼦树调整,使该⼦树称为堆。
对于⼤根堆,调整⽅法为:若【根节点的关键字】⼩于【左右⼦⼥中关键字较⼤者】,则交换。
之后向前依次对各节点((n-2)/2 - 1)~ 0为根的⼦树进⾏调整,看该节点值是否⼤于其左右⼦节点的值,若不是,将左右⼦节点中较⼤值与之交换,交换后可能会破坏下⼀级堆,于是继续采⽤上述⽅法构建下⼀级的堆,直到以该节点为根的⼦树构成堆为⽌。
反复利⽤上述调整堆的⽅法建堆,直到根节点。
4.堆排序:(⼤根堆) ①将存放在array[0,...,n-1]中的n个元素建成初始堆; ②将堆顶元素与堆底元素进⾏交换,则序列的最⼤值即已放到正确的位置; ③但此时堆被破坏,将堆顶元素向下调整使其继续保持⼤根堆的性质,再重复第②③步,直到堆中仅剩下⼀个元素为⽌。
8种排序算法
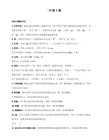
J=2(38) [38 49] 65 97 76 13 27 49
J=3(65) [38 49 65] 97 76 13 27 49
J=4(97) [38 49 65 97] 76 13 27 49
J=5(76) [38 49 65 76 97] 13 27 49
2. 堆的定义: N个元素的序列K1,K2,K3,...,Kn.称为堆,当且仅当该序列满足特性:
Ki≤K2i Ki ≤K2i+1(1≤ I≤ [N/2])
堆实质上是满足如下性质的完全二叉树:树中任一非叶子结点的关键字均大于等于其孩子结点的关键字。例如序列10,15,56,25,30,70就是一个堆,它对应的完全二叉树如上图所示。这种堆中根结点(称为堆顶)的关键字最小,我们把它称为小根堆。反之,若完全二叉树中任一非叶子结点的关键字均大于等于其孩子的关键字,则称之为大根堆。
(6)基数排序
基数排序是按照低位先排序,然后收集;再按照高位排序,然后再收集;依次类推,直到最高位。有时候有些属性是有优先级顺序的,先按低优先级排序,再按高优先级排序,最后的次序就是高优先级高的在前,高优先级相同的低优先级高的在前。基数排序基于分别排序,分别收集,所以其是稳定的排序算法。
2. 排序过程:
【示例】:
初始关键字 [49 38 65 97 76 13 27 49]
第一趟排序后 13 [38 65 97 76 49 27 49]
第二趟排序后 13 27 [65 97 76 49 38 49]
第三趟排序后 13 27 38 [97 76 49 65 49]
其次,说一下稳定性的好处。排序算法如果是稳定的,那么从一个键上排序,然后再从另一个键上排序,第一个键排序的结果可以为第二个键排序所用。基数排序就是这样,先按低位排序,逐次按高位排序,低位相同的元素其顺序再高位也相同时是不会改变的。另外,如果排序算法稳定,对基于比较的排序算法而言,元素交换的次数可能会少一些(个人感觉,没有证实)。
程序排序算法免费教程
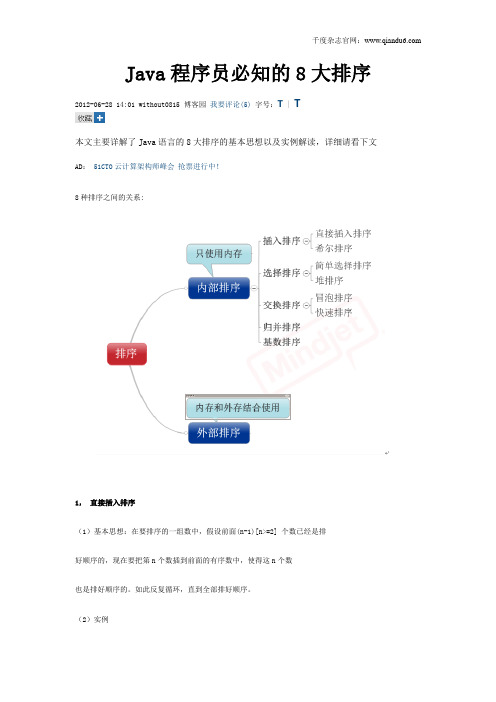
Java程序员必知的8大排序2012-06-28 14:01 without0815 博客园我要评论(5)字号:T | T本文主要详解了Java语言的8大排序的基本思想以及实例解读,详细请看下文AD:51CTO云计算架构师峰会抢票进行中!8种排序之间的关系:1,直接插入排序(1)基本思想:在要排序的一组数中,假设前面(n-1)[n>=2] 个数已经是排好顺序的,现在要把第n个数插到前面的有序数中,使得这n个数也是排好顺序的。
如此反复循环,直到全部排好顺序。
(2)实例(3)用java实现1.package com.njue;2.3.public class insertSort {4.public insertSort(){5.inta[]={49,38,65,97,76,13,27,49,78,34,12,64,5,4,62,99,98,54,56,17,18,23,34,15,35,25,53,51};6.int temp=0;7.for(int i=1;i<a.length;i++){8.int j=i-1;9. temp=a[i];10.for(;j>=0&&temp<a[j];j--){11. a[j+1]=a[j]; //将大于temp的值整体后移一个单位12. }13. a[j+1]=temp;14. }15.for(int i=0;i<a.length;i++)16. System.out.println(a[i]);17.}18.}2,希尔排序(最小增量排序)(1)基本思想:算法先将要排序的一组数按某个增量d(n/2,n为要排序数的个数)分成若干组,每组中记录的下标相差d.对每组中全部元素进行直接插入排序,然后再用一个较小的增量(d/2)对它进行分组,在每组中再进行直接插入排序。
当增量减到1时,进行直接插入排序后,排序完成。
(2)实例:(3)用java实现1.public class shellSort {2.public shellSort(){3.int a[]={1,54,6,3,78,34,12,45,56,100};4.double d1=a.length;5.int temp=0;6.while(true){7. d1= Math.ceil(d1/2);8.int d=(int) d1;9.for(int x=0;x<d;x++){10.for(int i=x+d;i<a.length;i+=d){11.int j=i-d;12. temp=a[i];13.for(;j>=0&&temp<a[j];j-=d){14. a[j+d]=a[j];15. }16. a[j+d]=temp;17. }18. }19.if(d==1)20.break;21. }22.for(int i=0;i<a.length;i++)23. System.out.println(a[i]);24.}25.}3.简单选择排序(1)基本思想:在要排序的一组数中,选出最小的一个数与第一个位置的数交换;然后在剩下的数当中再找最小的与第二个位置的数交换,如此循环到倒数第二个数和最后一个数比较为止。
java list map 排序方法

java list map 排序方法Java中的List和Map是常用的数据结构,它们在实际开发中经常用于存储和操作一组数据。
而对于List和Map的排序操作,是开发中经常用到的功能之一。
本文将介绍Java中List和Map的排序方法。
一、List的排序方法在Java中,List是一个有序的集合,可以按照元素的插入顺序来访问元素。
List提供了多种排序方法,常用的有以下几种:1. Collections.sort(List<T> list):对List进行升序排序,要求List中的元素实现Comparable接口,即自定义类需要实现Comparable接口并重写compareTo方法。
2. Collections.sort(List<T> list, Comparator<? super T> c):对List进行自定义排序,可以根据Comparator接口中的compare 方法来实现自定义的排序规则。
3. List.sort(Comparator<? super E> c):从Java 8开始,List 提供了sort方法来对List进行排序,使用方式与Collections.sort方法类似。
下面是一个示例代码,演示了如何使用Collections.sort方法对List进行排序:```javaimport java.util.ArrayList;import java.util.Collections;import java.util.List;public class ListSortExample {public static void main(String[] args) {List<String> list = new ArrayList<>();list.add("banana");list.add("apple");list.add("orange");list.add("grape");// 使用Collections.sort方法对List进行排序 Collections.sort(list);// 输出排序后的结果for (String fruit : list) {System.out.println(fruit);}}}```运行上述代码,输出结果为:applebananagrapeorange二、Map的排序方法在Java中,Map是一种键值对的数据结构,它可以存储任意类型的键和值。
java中让数组从大到小排序的方法
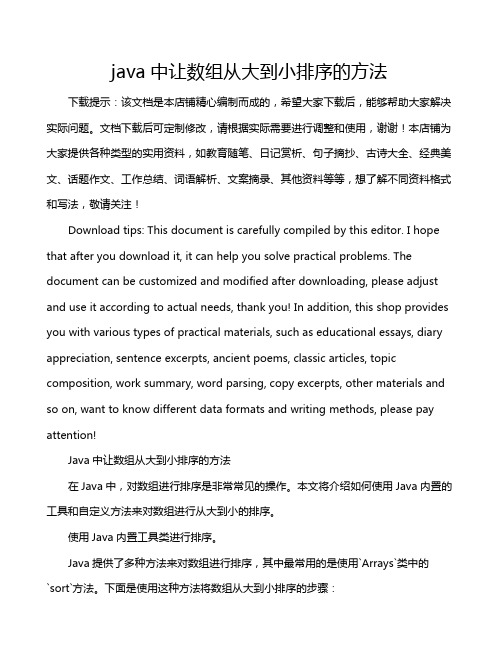
java中让数组从大到小排序的方法下载提示:该文档是本店铺精心编制而成的,希望大家下载后,能够帮助大家解决实际问题。
文档下载后可定制修改,请根据实际需要进行调整和使用,谢谢!本店铺为大家提供各种类型的实用资料,如教育随笔、日记赏析、句子摘抄、古诗大全、经典美文、话题作文、工作总结、词语解析、文案摘录、其他资料等等,想了解不同资料格式和写法,敬请关注!Download tips: This document is carefully compiled by this editor. I hope that after you download it, it can help you solve practical problems. The document can be customized and modified after downloading, please adjust and use it according to actual needs, thank you! In addition, this shop provides you with various types of practical materials, such as educational essays, diary appreciation, sentence excerpts, ancient poems, classic articles, topic composition, work summary, word parsing, copy excerpts, other materials and so on, want to know different data formats and writing methods, please pay attention!Java中让数组从大到小排序的方法在Java中,对数组进行排序是非常常见的操作。
排列组合三种算法java实现

1.<strong>1、全排列算法</strong>[java]view plaincopy1.import java.util.ArrayList;2./**3. * 全排列算法4. *5. */6.public class Arrange {7.8.private int total = 0;9.private ArrayList<string></string> arrangeList = new ArrayList<string></string>();10.11.public Arrange() {12. }13.14.private void swap(String list[], int k, int i) {15. String c3 = list[k];16. list[k] = list[i];17. list[i] = c3;18. }19.20.public void perm(String list[], int k, int m) {21.if (k > m) {22. StringBuffer sb = new StringBuffer();23.for (int i = 0; i <= m; i++) {24. sb.append(list[i]).append(",");25. }26.if (sb.length()>0) {27. sb.setLength(sb.length()-1);28. }29. arrangeList.add(sb.toString());30. total++;31. } else {32.for (int i = k; i <= m; i++) {33. swap(list, k, i);34. perm(list, k + 1, m);35. swap(list, k, i);36. }37. }38. }39.40.public int getTotal() {41.return total;42. }43.44.public ArrayList<string></string> getArrangeList() {45.return arrangeList;46. }47.48.public static void main(String args[]) {49. String list[] = { "1", "2", "3", "4", "5" };50. Arrange ts = new Arrange();51. ts.perm(list, 0, list.length-1);52.for (int i = 0; i < ts.getArrangeList().size(); i++) {53. System.out.println(ts.getArrangeList().get(i));54. }55. System.out.println("total:" + ts.total);56. }57.58.}2、组合算法[java]view plaincopy1.import java.util.ArrayList;2.import java.util.BitSet;3.4.public class Combination {5.6.private ArrayList<string></string> combList= new ArrayList<string></string>();7.8.public void mn(String[] array, int n) {9.int m = array.length;10.if (m < n)11.throw new IllegalArgumentException("Error m < n");12. BitSet bs = new BitSet(m);13.for (int i = 0; i < n; i++) {14. bs.set(i, true);15. }16.do {17. printAll(array, bs);18. } while (moveNext(bs, m));19.20. }21./**22. * 1、start 第一个true片段的起始位,end截止位23. * 2、把第一个true片段都置false24. * 3、数组从0下标起始到以第一个true片段元素数量减一为下标的位置都置true25. * 4、把第一个true片段end截止位置true26. *27. * @param bs 数组是否显示的标志位28. * @param m 数组长度29. * @return boolean 是否还有其他组合30. */31.private boolean moveNext(BitSet bs, int m) {32.int start = -1;33.while (start < m)34.if (bs.get(++start))35.break;36.if (start >= m)37.return false;38.39.int end = start;40.while (end < m)41.if (!bs.get(++end))42.break;43.if (end >= m)44.return false;45.for (int i = start; i < end; i++)46. bs.set(i, false);47.for (int i = 0; i < end - start - 1; i++)48. bs.set(i);49. bs.set(end);50.return true;51. }52.53./**54. * 输出生成的组合结果55. *56. * @param array 数组57. * @param bs 数组元素是否显示的标志位集合58. */59.private void printAll(String[] array, BitSet bs) {60. StringBuffer sb = new StringBuffer();61.for (int i = 0; i < array.length; i++)62.if (bs.get(i)) {63. sb.append(array[i]).append(',');64. }65. sb.setLength(sb.length() - 1);66. combList.add(sb.toString());67. }68.69.public ArrayList<string></string> getCombList() {70.return combList;71. }72.73.public static void main(String[] args) throws Exception {74. Combination comb = new Combination();75. comb.mn(new String[]{"1","2","3","4","5","6"}, 3);76.for (int i = 0; i < comb.getCombList().size(); i++) {77. System.out.println(comb.getCombList().get(i));78. String[] list = comb.getCombList().get(i).split(",");79. Arrange ts = new Arrange();80. ts.perm(list, 0, list.length-1);81.for (int j = 0; j < ts.getArrangeList().size(); j++) {82. System.out.println("/u0009"+ts.getArrangeList().get(j));83. }84. }85. }86.87.}[java]view plaincopy1.3、调用排列组合算法工具类[java]view plaincopy1.<pre class="java" name="code">import java.util.ArrayList;2.3.public class ArrangeCombine {4.5.public static ArrayList<string></string> getArrangeOrCombine(String[] args,int n, boolean isArrange) throws Exception{6.if (args.length<=0) {7.throw new Exception("array.length<=0");8. }9.if (n>args.length) {10.throw new Exception(" n>array.length");11. }12. Combination comb = new Combination();13. comb.mn(args, n);14.if (!isArrange) {15.return comb.getCombList();16. }17. ArrayList<string></string> arrangeList = new ArrayList<string></string>();18.for (int i = 0; i < comb.getCombList().size(); i++) {19. String[] list = comb.getCombList().get(i).split(",");20. Arrange ts = new Arrange();21. ts.perm(list, 0, list.length-1);22.for (int j = 0; j < ts.getArrangeList().size(); j++) {23. arrangeList.add(ts.getArrangeList().get(j));24. }25. }26.return arrangeList;27. }28.29.public static void main(String[] args) {30.try {31. ArrayList<string></string> arrangeOrCombine = ArrangeCombine.getArrangeOrCombine(new String[]{"1","2","3","4","5","6"}, 3, false);32. ArrayList<string></string> arrangeOrCombine2 = ArrangeCombine.getArrangeOrCombine(new String[]{"1","2","3","4","5","6"}, 3, true);33.for (int i = 0; i < arrangeOrCombine.size(); i++) {34. System.out.println(arrangeOrCombine.get(i));35. }36. System.out.println("Total:"+arrangeOrCombine.size());37. System.out.println("=============================");38.for (int i = 0; i < arrangeOrCombine2.size(); i++) {39. System.out.println(arrangeOrCombine2.get(i));40. }41. System.out.println("Total:"+arrangeOrCombine2.size());42. } catch (Exception e) {43. e.printStackTrace();44. }45. }46.47.}。
堆排序(Java实现)

堆排序(Java实现)《算法导论》中堆排序主要将其分为堆的性质、维护堆的性质、建堆、堆排序算法堆的性质:给定⼀个结点的下标i,很容易计算得到它的⽗结点、左孩⼦和右孩⼦的下标(伪代码):PARENT(i)return i/2LEFT(i)return 2iRIGHT(i)return 2i+1这⾥针对下标从1开始的数组,然⽽实际上我们涉及的数组都是从0开始。
为了改进上⾯的伪代码,可以使⽤移位来解决,其伪代码:PARENT(i)return (i-1)>>1LEFT(i)return ((i+1)<<1)-1RIGHT(i)return (i+1)<<1维护堆的性质(MAX-HEAPIFY):MAX-HEAPIFY是⽤于维护最⼤堆性质的重要过程。
他输⼊为⼀个数据A和⼀个下标i,在调⽤MAX-HEAPIFY的时候,假定根节点为LEFT(i)和RIGHT(i)的⼆叉树都是最⼤堆,但这时A[i]有可能⼩于其孩⼦,这样就违背了最⼤堆的性质。
MAX-HEAPIFY通过让A[i]的值在最⼤堆中“逐级下降”,从⽽使得以下标i为根结点的⼦树重新遵循最⼤堆的性质。
其伪代码如下所⽰:MAX-HEAPIFY(A, i)l = LEFT(i);r = RIGHT(i);if l <= A.heap-size and A[l] > A[i]largest = largestelse largest = i;if r <= A.heap-size and A[r] > A[largest]largest = rif largest != iexchangeA[i]withA[largest]MAX-HEAPIFY(A, largest)⽤Java语⾔实现维护堆的性质:MAXHeapify.javapackage heapsort;public class MaxHeapify {public void heapAdjust(int[] A, int i, int len){int l = Left(i);int r = Right(i);int largest = i;//假设⽗节点值最⼤if (l < len && A[l] > A[i]) {//左孩⼦值⼤于⽗节点值largest = l;}if (r < len && A[r] > A[largest]) {//右孩⼦值⼤于⽗节点值largest = r;}if (largest != i) {//exchange A[i]withA[largest]int tmp = A[i] ;A[i] = A[largest];A[largest] = tmp;heapAdjust(A, largest, len);}}private int Right(int i) {//右孩⼦坐标return ((i+1)<<1);// return 2*i+1;}private int Left(int i) {//左孩⼦坐标return ((i+1)<<1)-1;// return 2*i;}}测试维护堆的性质代码即:MaxHeapifyTest.javapackage heapsort;public class MaxHeapifyTest {public static void main(String[] args) {int[] A ={16, 4, 10, 14, 7, 9, 3, 2, 8, 1};// int[] A={5, 2, 4, 6, 1, 3, 2, 6};MaxHeapify maxHeapify = new MaxHeapify();maxHeapify.heapAdjust(A, 1, A.length);for (int i = 0; i < A.length; i++) {System.out.print(A[i]+" ");}}}测试结果:16 14 10 8 7 9 3 2 4 1建堆(BUILD-MAX-HEAPIFY):⽤⾃底向上的⽅法利⽤过程MAX-HEAPIFY把⼀个⼤⼩为n=A.length的数组A[1..n]转换为最⼤堆。
Java对象集合List排序的5种方式

Java对象集合List排序的5种⽅式⽬标明确排序对象类public class Student{private String name;private Integer age;public Student(String name, Integer age) { = name;this.age = age;}public Student() {}@Overridepublic String toString() {return "Student{" +"name='" + name + '\'' +", age=" + age +'}';}public String getName() {return name;}public void setName(String name) { = name;}public Integer getAge() {return age;}public void setAge(Integer age) {this.age = age;}}⽅式⼀:排序对象类实现Comparable接⼝的compareTo⽅法Student类public class Student implements Comparable<Student>{private String name;private Integer age;public Student(String name, Integer age) { = name;this.age = age;}public Student() {}@Overridepublic String toString() {return "Student{" +"name='" + name + '\'' +", age=" + age +'}';}public String getName() {return name;}public void setName(String name) { = name;}public Integer getAge() {return age;}public void setAge(Integer age) {this.age = age;}/*** 需要实现的⽅法,实现升序排序,降序请反写* this表⽰当前的对象* @param o ⽐较时传⼊的对象* @param o ⽐较时传⼊的对象* @return*/@Overridepublic int compareTo(Student o) {return this.age-o.age;}}Mainpublic class Test {public static void main(String[] args) {//数据准备List<Student> list = new ArrayList<>();list.add(new Student("⼩明",1));list.add(new Student("⼩红",4));list.add(new Student("⼩刚",3));list.add(new Student("⼩鸡",5));list.add(new Student("⼩狗",2));//使⽤Collections集合⼯具类进⾏排序Collections.sort(list);for (Student student : list) {System.out.println(student);}}}compareTo⽅法实际上是⼀个⽐较⼤⼩的⽅法,只要是排序,我们必须⽤到⽐较,若果是简单的整数数组排序,我们只需要⽤ > 、 < 等进⾏⽐较,但是对于对象来说,Collections集合⼯具类在进⾏排序时,每次⽐较,都是调⽤的我们实现的compareTo⽅法,this表⽰当前对象,o表⽰要进⾏⽐较的传⼊对象,返回是⼀个int类型的整数返回值>0:表⽰当前对象⽐传⼊对象⼤(年龄)返回值=0:表⽰当前对象和传⼊对象⼀样⼤(年龄)返回值<0:表⽰当前对象⽐传⼊对象⼩(年龄)排序结果:Student{name='⼩明', age=1}Student{name='⼩狗', age=2}Student{name='⼩刚', age=3}Student{name='⼩红', age=4}Student{name='⼩鸡', age=5}Process finished with exit code⽅式⼆:使⽤Comparator接⼝⾃定义⾏为使⽤⽅式⼀我们必须在Student类上⾯进⾏修改,这显然不是最好的办法,如果我们不想按年龄排序,想要按照姓名排序,或者我们有⼀个⽅法需要按照年龄,另⼀个⽅法需要按照姓名,那么重写compareTo⽅法显然就没法完成我们的⽬标了,Collections的重载sort⽅法可以允许我们在排序对象外部⾃定义⼀个⽐较器(Comparator接⼝的实现类),因为我们仅需要实现compare()⽅法(实际上Comparator接⼝是⼀个函数式接⼝,⽆伤⼤雅最后解释,想了解的看最后),没必要在定义⼀个类,我们直接使⽤匿名内部类的⽅式。
堆排序算法详解
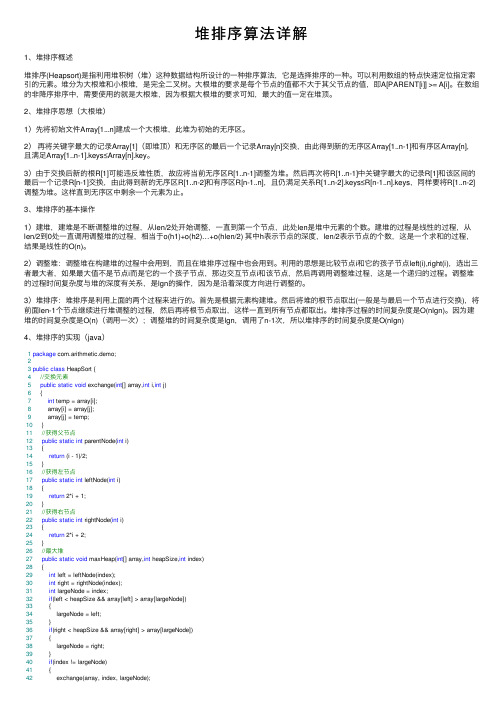
堆排序算法详解1、堆排序概述堆排序(Heapsort)是指利⽤堆积树(堆)这种数据结构所设计的⼀种排序算法,它是选择排序的⼀种。
可以利⽤数组的特点快速定位指定索引的元素。
堆分为⼤根堆和⼩根堆,是完全⼆叉树。
⼤根堆的要求是每个节点的值都不⼤于其⽗节点的值,即A[PARENT[i]] >= A[i]。
在数组的⾮降序排序中,需要使⽤的就是⼤根堆,因为根据⼤根堆的要求可知,最⼤的值⼀定在堆顶。
2、堆排序思想(⼤根堆)1)先将初始⽂件Array[1...n]建成⼀个⼤根堆,此堆为初始的⽆序区。
2)再将关键字最⼤的记录Array[1](即堆顶)和⽆序区的最后⼀个记录Array[n]交换,由此得到新的⽆序区Array[1..n-1]和有序区Array[n],且满⾜Array[1..n-1].keys≤Array[n].key。
3)由于交换后新的根R[1]可能违反堆性质,故应将当前⽆序区R[1..n-1]调整为堆。
然后再次将R[1..n-1]中关键字最⼤的记录R[1]和该区间的最后⼀个记录R[n-1]交换,由此得到新的⽆序区R[1..n-2]和有序区R[n-1..n],且仍满⾜关系R[1..n-2].keys≤R[n-1..n].keys,同样要将R[1..n-2]调整为堆。
这样直到⽆序区中剩余⼀个元素为⽌。
3、堆排序的基本操作1)建堆,建堆是不断调整堆的过程,从len/2处开始调整,⼀直到第⼀个节点,此处len是堆中元素的个数。
建堆的过程是线性的过程,从len/2到0处⼀直调⽤调整堆的过程,相当于o(h1)+o(h2)…+o(hlen/2) 其中h表⽰节点的深度,len/2表⽰节点的个数,这是⼀个求和的过程,结果是线性的O(n)。
2)调整堆:调整堆在构建堆的过程中会⽤到,⽽且在堆排序过程中也会⽤到。
利⽤的思想是⽐较节点i和它的孩⼦节点left(i),right(i),选出三者最⼤者,如果最⼤值不是节点i⽽是它的⼀个孩⼦节点,那边交互节点i和该节点,然后再调⽤调整堆过程,这是⼀个递归的过程。
java算法代码实现

java算法代码实现一、Java算法概述Java算法是指在Java编程语言中实现的各种计算机算法。
它们可以用于解决各种问题,例如排序、搜索、图形处理和数据分析等。
Java算法通常由一组指令组成,这些指令按照特定的顺序执行,以达到预期的结果。
二、Java算法的分类根据不同的标准,Java算法可以分为不同的类别。
以下是常见的分类方式:1. 基本排序算法:包括冒泡排序、选择排序和插入排序等。
2. 高级排序算法:包括快速排序、归并排序和堆排序等。
3. 搜索算法:包括线性搜索和二分搜索等。
4. 图形处理算法:包括图像滤波和边缘检测等。
5. 数据分析算法:包括聚类分析和分类器等。
三、Java基本排序算法代码实现以下是三种基本排序算法(冒泡排序、选择排序和插入排序)的Java 代码实现:1. 冒泡排序public static void bubbleSort(int[] arr) { int n = arr.length;for (int i = 0; i < n - 1; i++) {for (int j = 0; j < n - i - 1; j++) {if (arr[j] > arr[j + 1]) {int temp = arr[j];arr[j] = arr[j + 1];arr[j + 1] = temp;}}}}2. 选择排序public static void selectionSort(int[] arr) { int n = arr.length;for (int i = 0; i < n - 1; i++) {int minIndex = i;for (int j = i + 1; j < n; j++) {if (arr[j] < arr[minIndex]) {minIndex = j;}}int temp = arr[i];arr[i] = arr[minIndex];arr[minIndex] = temp;}}3. 插入排序public static void insertionSort(int[] arr) { int n = arr.length;for (int i = 1; i < n; i++) {int key = arr[i];int j = i - 1;while (j >= 0 && key < arr[j]) {arr[j + 1] = arr[j];j--;}arr[j + 1] = key;}}四、Java高级排序算法代码实现以下是三种高级排序算法(快速排序、归并排序和堆排序)的Java代码实现:1. 快速排序public static void quickSort(int[] arr, int low, int high) {if (low < high) {int pivotIndex = partition(arr, low, high);quickSort(arr, low, pivotIndex - 1);quickSort(arr, pivotIndex + 1, high);}}private static int partition(int[] arr, int low, int high) {int pivotValue = arr[high];int i = low - 1;for (int j = low; j < high; j++) {if (arr[j] < pivotValue) {i++;int temp = arr[i];arr[i] = arr[j];arr[j] = temp;}}int temp = arr[i + 1];arr[i + 1] = arr[high];arr[high] = temp;return i + 1;}2. 归并排序public static void mergeSort(int[] arr, int low, int high) {if (low < high) {int mid = (low + high) / 2;mergeSort(arr, low, mid);mergeSort(arr, mid + 1, high);merge(arr, low, mid, high);}}private static void merge(int[] arr, int low, int mid, int high) { int[] tempArr = new int[arr.length];for (int i = low; i <= high; i++) {tempArr[i] = arr[i];}int i = low;int j = mid + 1;int k = low;while (i <= mid && j <= high) {if (tempArr[i] <= tempArr[j]) {arr[k++] = tempArr[i++];} else {arr[k++] = tempArr[j++];}}while (i <= mid) {arr[k++] = tempArr[i++];}}3. 堆排序public static void heapSort(int[] arr) { buildMaxHeap(arr);for (int i = arr.length - 1; i >= 0; i--) { swap(arr, 0, i);maxHeapify(arr, 0, i);}}private static void buildMaxHeap(int[] arr) {int n = arr.length;for (int i = n / 2 - 1; i >= 0; i--) {maxHeapify(arr, i, n);}}private static void maxHeapify(int[] arr, int i, int heapSize) { int left = i * 2 + 1;int right = i * 2 + 2;int largestIndex = i;if (left < heapSize && arr[left] > arr[largestIndex]) {largestIndex = left;}if (right < heapSize && arr[right] > arr[largestIndex]) { largestIndex = right;}if (largestIndex != i) {swap(arr, i, largestIndex);maxHeapify(arr, largestIndex, heapSize);}}private static void swap(int[] arr, int a, int b) {int temp = arr[a];arr[a] = arr[b];arr[b] = temp;}五、Java搜索算法代码实现以下是两种搜索算法(线性搜索和二分搜索)的Java代码实现:1. 线性搜索public static boolean linearSearch(int[] arr, int target) {for (int num : arr) {if (num == target) {return true;}}return false;}2. 二分搜索public static boolean binarySearch(int[] sortedArr, int target) { int low = 0;int high = sortedArr.length - 1;while (low <= high) {int mid = (low + high) / 2;if (sortedArr[mid] == target) {return true;} else if (sortedArr[mid] < target) {low = mid + 1;} else {high = mid - 1;}}return false;}六、Java图形处理算法代码实现以下是两种图形处理算法(图像滤波和边缘检测)的Java代码实现:1. 图像滤波public static BufferedImage blurFilter(BufferedImage image, int radius) {int width = image.getWidth();int height = image.getHeight();BufferedImage resultImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);for (int y = 0; y < height; y++) {for (int x = 0; x < width; x++) {int rgbSumRed = 0;int rgbSumGreen = 0;int rgbSumBlue = 0;int count = 0;for (int i = -radius; i <= radius; i++) {for (int j = -radius; j <= radius; j++) {if (x + i >= 0 && x + i < width && y + j >= 0 && y + j < height) {int rgbValue = image.getRGB(x + i, y + j);rgbSumRed += (rgbValue >> 16) & 255;rgbSumGreen += (rgbValue >> 8) & 255;rgbSumBlue += rgbValue & 255;count++;}}}int avgRed = rgbSumRed / count;int avgGreen = rgbSumGreen / count;int avgBlue = rgbSumBlue / count;resultImage.setRGB(x, y, (avgRed << 16) | (avgGreen << 8) | avgBlue);}}return resultImage;}2. 边缘检测public static BufferedImage edgeDetection(BufferedImage image) {int width = image.getWidth();int height = image.getHeight();BufferedImage resultImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);int[][] gx = {{-1, 0, 1}, {-2, 0, 2}, {-1, 0, 1}};int[][] gy = {{-1, -2, -1}, {0, 0, 0}, {1, 2, 1}};for (int y = 0; y < height; y++) {for (int x = 0; x < width; x++) {int gxSumRed = 0;int gxSumGreen = 0;int gxSumBlue = 0;int gySumRed = 0;int gySumGreen = 0;int gySumBlue = 0;for (int i = -1; i <= 1; i++) {for (int j = -1; j <= 1; j++) {if (x + i >= 0 && x + i < width && y + j >= 0 && y + j < height) {Color colorValue = new Color(image.getRGB(x + i, y + j));gxSumRed += colorValue.getRed() * gx[i + 1][j + 1];gxSumGreen += colorValue.getGreen() * gx[i + 1][j + 1];gxSumBlue += colorValue.getBlue() * gx[i + 1][j + 1];gySumRed += colorValue.getRed() * gy[i + 1][j + 1];gySumGreen += colorValue.getGreen() * gy[i + 1][j + 1];gySumBlue += colorValue.getBlue() * gy[i + 1][j + 1];}}}int red = (int) Math.sqrt(gxSumRed * gxSumRed + gySumRed * gySumRed);int green = (int) Math.sqrt(gxSumGreen * gxSumGreen + gySumGreen * gySumGreen);int blue = (int) Math.sqrt(gxSumBlue * gxSumBlue + gySumBlue * gySumBlue);red = Math.min(red, 255);green = Math.min(green, 255);blue = Math.min(blue, 255);resultImage.setRGB(x, y, (red << 16) | (green << 8) | blue);}}return resultImage;}七、Java数据分析算法代码实现以下是两种数据分析算法(聚类分析和分类器)的Java代码实现:1. 聚类分析public static List<List<double[]>> kMeansClustering(List<double[]> dataList, int k) {List<List<double[]>> clustersList = new ArrayList<>(); for (int i = 0; i < k; i++) {clustersList.add(new ArrayList<>());}double[][] centroidsArr = newdouble[k][dataList.get(0).length];for (int i = 0; i < k; i++) {centroidsArr[i] = dataList.get(i);clustersList.get(i).add(dataList.get(i));}while (true) {boolean changed = false;for (double[] data : dataList) {int nearestCentroidIndex = 0;double nearestDistance = Double.MAX_VALUE; for (int i = 0; i < k; i++) {double distance = euclideanDistance(data, centroidsArr[i]);if (distance < nearestDistance) {nearestCentroidIndex = i;nearestDistance = distance;}}if (!clustersList.get(nearestCentroidIndex).contains(data)) {for (List<double[]> cluster : clustersList) {if (cluster.contains(data)) {cluster.remove(data);break;}}clustersList.get(nearestCentroidIndex).add(data);changed = true;}}if (!changed) {break;}for (int i = 0; i < k; i++) {double[] newCentroid =calculateCentroid(clustersList.get(i));centroidsArr[i] = newCentroid;}}return clustersList;}private static double euclideanDistance(double[] a, double[] b) { double sumSquares = 0.0;for (int i = 0; i < a.length; i++) {sumSquares += Math.pow(a[i] - b[i], 2);}return Math.sqrt(sumSquares);}private static double[] calculateCentroid(List<double[]> dataList) {int dimensions = dataList.get(0).length;double[] centroidArr = new double[dimensions];for (int d = 0; d < dimensions; d++) {double sumValuesInDimension = 0.0;for (double[] data : dataList) {sumValuesInDimension += data[d];}centroidArr[d] = sumValuesInDimension / dataList.size(); }return centroidArr;}2. 分类器public static String kNearestNeighborsClassifier(List<double[]> trainingDataList, List<String> trainingLabelList,double[] testData, int k) {double[][] distancesAndLabelsArr = newdouble[trainingDataList.size()][2];for (int i = 0; i < trainingDataList.size(); i++) {distancesAndLabelsArr[i][0] = euclideanDistance(testData, trainingDataList.get(i));distancesAndLabelsArr[i][1] =Double.parseDouble(trainingLabelList.get(i));}Arrays.sort(distancesAndLabelsArr,paringDouble(a -> a[0]));Map<Double, Integer> labelCountMap = new HashMap<>(); for (int i = 0; i < k; i++) {double label = distancesAndLabelsArr[i][1];if (labelCountMap.containsKey(label)) {labelCountMap.put(label, labelCountMap.get(label) + 1); } else {labelCountMap.put(label, 1);}}double mostFrequentLabel = -1;int maxLabelCount = -1;for (double label : labelCountMap.keySet()) {int count = labelCountMap.get(label);if (count > maxLabelCount) {maxLabelCount = count;mostFrequentLabel = label;}}return Double.toString(mostFrequentLabel);}private static double euclideanDistance(double[] a, double[] b) { double sumSquares = 0.0;for (int i = 0; i < a.length; i++) {sumSquares += Math.pow(a[i] - b[i], 2);}return Math.sqrt(sumSquares);}八、总结Java算法是计算机科学中的重要部分,可以用于解决各种问题。
java数组排序sort方法

Java 数组排序算法详解Java 数组排序算法是 Java 语言中一个重要的组成部分,是进行数据排序的重要工具。
Java 提供了多种数组排序算法,包括快速排序、归并排序、堆排序等。
本文将对 Java 数组排序算法进行详细介绍,并针对不同的算法提供具体的实现代码。
一、快速排序快速排序是一种常用的排序算法,它采用分治的思想,通过递归地将数组划分为较小和较大的两个子数组,然后递归地排序两个子数组。
快速排序是不稳定的排序算法,其平均时间复杂度为 O(nlogn)。
快速排序的实现代码如下:```javapublic static void quickSort(int[] arr, int left, int right) {if (left < right) {int pivotIndex = partition(arr, left, right);quickSort(arr, left, pivotIndex - 1);quickSort(arr, pivotIndex + 1, right);}}private static int partition(int[] arr, int left, int right) {int pivot = arr[left];int i = left + 1;int j = right;while (i <= j) {while (i <= j && arr[i] < pivot) {i++;}while (i <= j && arr[j] > pivot) {j--;}if (i <= j) {swap(arr, i, j);i++;j--;}}swap(arr, left, j);return j;}private static void swap(int[] arr, int i, int j) { int temp = arr[i];arr[i] = arr[j];arr[j] = temp;}```二、归并排序归并排序是一种高效的排序算法,它采用分治的思想,将数组划分为较小和较大的两个子数组,然后递归地排序两个子数组。
数据结构之java排序javasort

数据结构排序思想和算法分析(java 版)一、排序的概念:1、设 n 个记录的序列为 { R 1 , R 2 , R 3 , . . . , R n }其相应的关键字序列为 { K 1 , K 2 , K 3 , . . . , K n }若规定 1 , 2 , 3 , . . . , n 的一个排列 p 1 , p 2 , p 3 , . . . , p n ,使得相应的关键字满足如下非递减关系:K p1 ≤ K p2 ≤ K p3 ≤ . . . ≤ K pn则原序列变为一个按关键字有序的序列:R p1 , R p2 , R p3 , . . . , R pn此操作过程称为排序。
2、排序问题一般分为内排序( internal sorting )和外排序( external sorting )两类:2.1. 内排序:待排序的表中记录个数较少,整个排序过程中所有的记录都可以保留在内存中;按照排序过程中所依据的原则的不同可以分类为:插入排序(直接插入排序、折半插入排序、希尔排序)交换排序(快速排序) (冒泡泡排序、快速排序)选择排序(直接选择排序、堆排序)归并排序基数排序二叉排序树排序2.2.外排序:待排序的记录个数足够多,以至于他们必须存储在磁带、磁盘上组成外部文件,排序过程中需要多次访问外存。
3、排序的时间复杂性:排序过程主要是对记录的排序码进行比较和记录的移动过程。
因此排序的时间复杂性可以算法执行中的数据比较次数及数据移动次数来衡量。
当一种排序方法使排序过程在最坏或平均情况下所进行的比较和移动次数越少,则认为该方法的时间复杂性就越好,分析一种排序方法,不仅要分析它的时间复杂性,而且要分析它的空间复杂性、稳定性和简单性等。
二、各种排序算法及代码详解:1、插入类排序--直接插入排序插入类排序算法思想:主要就是对于一个已经有序的序列中,插入一个新的记录,但要求插入后此数据序列仍然有序,这个时候就要用到插入排序法。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
* @param end
*
结束位置
*/
private static void quickDeal(int[] array, int begin, int end,int method) {
if (end > begin) {
int pos = begin + (int) (Math.random() * (end - begin + 1)); // 计算分隔位置
public static int[] quickOrder(int[] array, int method) {
quickDeal(array,0,array.length - 1,method); return array;
}
/**
*
* @param array
* @param begin
*
开始位置
for (int i = 0; i < orderarray.length; i++) { System.out.println(orderarray[i]);
} }
/**
* 取随机数据,初始化一个数组
*
* @param min
*
随机数的最小值
* @param max
*
最大值
* @param size
位置的值时移动 array[j + 1] = tmp; //插入排序值
} } else if (method == 2) {
if (array[i - 1] < array[i]) { int tmp = array[i]; int j = i - 1; do { array[j + 1] = array[j]; j--; } while (j >= 0 && tmp > array[j]); array[j + 1] = tmp;
for (int i = 1; i < array.length; i++) { if (method == 1) { if (array[i - 1] > array[i]) { int tmp = array[i]; // int j = i - 1; do { array[j + 1] = array[j]; j--; } while (j >= 0 && tmp < array[j]); //当 j>=0 并且 当前值大于数据中 j
}
}
/** * 插入排序方法 * 排序原理:抽出一个数,做为排序基序列,然后依次抽出其它数与,与此序列中的
数进行比较,放入合适的位置
* @param array * @param method * @return */ public static int[] insertOrder(int[] array, int method) {
}
/** * 交换值,交换数组的两个值 * @param array * @param i * @param j */
private static void swap(int[] array,int i, int j) {
int tmp = array[i]; array[i] = array[j]; array[j] = tmp; }
} } return array; }
/** * 双向冒泡排序 * 原理:类似于冒泡排序,只不过是双向的 * @param array * @param method * @return */
public static int[] doubleBubbleOrder(int[] array,int method) {
各种排序方法的实现
import ng.Math; import java.util.Random;
/** * 排序 * * @author javajack */
public class OrderTest {
public static void main(String args[]) { OrderTest.ExecOrder(2);
while(j <= n-1) {
if(j < n-1 && a[j] < a[j+1])
j++;
if(temp >= a[j]) break;
a[(j-1)/2] = a[j];
j = 2 * j + 1; }
a[(j-1)/2] = temp; }
public static void Display(int[] a, String str) {
/**
*
* @param method
*
1 为升序,2 为降序
*/
public static void ExecOrder(int method) {
int[] array = null;
array = initArray(10, 210, 10);
//int[] orderarray = bubbleOrder(array,method); int[] orderarray = doubleBubbleOrder(array,method); //int[] orderarray = insertOrder(array, method); //int [] orderarray = quickOrder(array,method); //int[] orderarray = selectOrder(array, method);
int n = a.length; int temp = 0;
Display(a, "Before sort : ");
for(int i=n/2; i>0; i--) Adjust(a, i-1, n);
for(int i=n-2; i>=0; i--) {
temp = a[i+1]; a[i+1] = a[0]; a[0] = temp;
也就是向前移动
swap(array,pos,i);
pos++; //移动后 pos 增 1
}
}
}
swap(array,pos,end); //end 位置的值前移
quickDeal(array,begin,pos -1,method);
quickDeal(array,pos+1,end,method);
} return init; }
/** * 交换排序方法 * 原理:依次交换值 * @param array * @return */
public static int[] convertOrder(int[] array, int method) { for (int i = 0; i < array.length; i++) { for (int j = i + 1; j < array.length; j++) { if (method==2) { if (array[i] < array[j]) swap(array,i,j); }else if (method == 1) { if (array[i] > array[j]) swap(array,i,j);
System.out.println(str);
for(int i=0; i<a.length; i++) System.out.print(a[i] + " ");
System.out.println(); } }
输出结果为:
Before sort : 26 5 77 1 61 11 59 15 48 19 After sort : 1 5 11 15 19 26 48 59 61 77
int left = 0; int right = array.length -1 ; while (left < right) {
for(int i=left;i<=right;i++)
{ if (method==1) { if (array[left] > array[i]) swap(array,left,i); }else { if (array[left] < array[i]) swap(array,left,i); }
for(int i=0;i<array.length;i++) {
for (int j=array.length -1 ;j>i;j--) {
if (method==2) {
if (array[i] < array[j]) swap(array,i,j);
}else if (method==1) if (array[i] > array[j]) swap(array,i,j);
} } left++; right--; } return array; }
/** * 快速排序方法,运用到递归 * 排序原理:随机找到一个值,然后以此值大小进行分为两个数组,大的放左边,小
的放右边, * 然后再对左右两侧的数据依次排序根据 * @param array * @param method * @return */
堆排序(1)
public class HeapSort {
public static void main(String[] args) {
int[] a = {26, 5, 77, 1, 61, 11, 59, 15, 48, 19};
Sort(a); }