SE lecture 8(20120418)
visual basic 2012 大学教程(第8章)

8. FilesConsciousness ... does not appear to itself chopped up in bits. ... A “river”or a “stream” are the metaphors by which it is most naturally described.—William JamesI can only assume that a “Do Not File” document is filed in a “Do NotFile” file.—Senator Frank Church, Senate Intelligence Subcommittee Hearing, 1975ObjectivesIn this chapter you’ll learn:• To use file processing to implement a business app.• To create, write to and read from files.• To become familia r with sequential-access file processing.• To use classes StreamWriter and StreamReader to write text to and read textfrom files.• To organize GUI commands in menus.• To manage resources with Using statements and the Finally block of a Trystatement.Outline8.1 Introduction8.2 Data Hierarchy8.3 Files and Streams8.4 Test-Driving the Credit Inquiry App8.5 Writing Data Sequentially to a Text File8.5.1 Class CreateAccounts8.5.2 Opening the File8.5.3 Managing Resources with the Using Statement8.5.4 Adding an Account to the File8.5.5 Closing the File and Terminating the App8.6 Building Menus with the Windows Forms Designer8.7Credit Inquiry App: Reading Data Sequentially from a Text File8.7.1 Implementing the Credit Inquiry App8.7.2 Selecting the File to Process8.7.3 Specifying the Type of Records to Display8.7.4 Displaying the Records8.8 Wrap-UpSummary | Terminology | Self-Review Exercises | Answers to Self-Review Exercises | Exercises8.1. IntroductionVariables and arrays offer only temporary storage of data in memory—the data is lost, for example, when a local variable ―goes out of scope‖ or when the app terminates. By contrast, files (and databases, which we cover in Chapter 12) are used for long-term retention of large (and often vast) amounts of data, even after the app that created the data terminates, so data maintained in files is often called persistent data. Computers store files on secondary storage devices, such as magnetic disks, optical disks (like CDs, DVDs and Bluray Discs™), USB flash drives and magnet ic tapes. In this chapter, we explain how to create, write to and read from data files. We continue our treatment of GUIs, explaining how to organize commands in menus and showing how to use the Windows Forms Designer to rapidly create menus. We also discuss resource management—as apps execute, they often acquire resources, such as memory and files, that need to be returned to the system so they can be reused at a later point. We show how to ensure that resources are properly returned to the system when the y’re no longer needed.8.2. Data HierarchyUltimately, all data items that computers process are reduced to combinations of 0s and 1s. This occurs because it’s simple and economical to build electronic devices that can assume two stable states—one represents 0 and the other represents 1. It’s remarkable that the impressive functions performed by computers involve only the most fundamental manipulations of 0s and 1s!BitsThe smallest data item that computers support is called a bit, short for ―binary digit‖—a digit that can assume either the value 0 or the value 1. Computer circuitry performs various simple bit manipulations, such as examining the value of a bit, setting the value of a bit and reversing a bit (from 1 to 0 or from 0 to 1). For more information on the binary number system, see Appendix C, Number Systems.CharactersProgramming with data in the low-level form of bits is cumbersome. It’s preferable to program with data in forms such as decimal digits (that is, 0, 1, 2, 3, 4, 5, 6, 7, 8 and 9), letters (that is, the uppercase letters A–Z and the lowercase letters a–z) and special symbols (that is, $, @, %, &, *, (, ), -, +, ", :, ?, / and many others). Digits, letters and special symbols are referred to as characters. The set of all characters used to write programs and represent data items on a particular computer is called that computer’s character set. Because computers process only 0s and 1s, every character in a computer’s character set is represented as a pattern of 0s and 1s. Bytes are composed of eight bits. Visual Basic uses the Unicode character set, in which each character is typically composed of two bytes (and hence 16 bits). You create programs and data items with characters; computers manipulate and process these characters as patterns of bits. FieldsJust as characters are composed of bits, fields are composed of characters. A field is a group of characters that conveys meaning. For example, a field consisting of uppercase and lowercase letters can represent a person’s name.Data HierarchyData items processed by computers form a data hierarchy (Fig. 8.1), in which data items become larger and more complex in structure as we progress up the hierarchy from bits to characters to fields to larger data aggregates.Fig. 8.1. Data hierarchy (assuming that files are organized into records). RecordsTypically, a record is composed of several related fields. In a payroll system, for example, a record for a particular employee might include the following fields:1. Employee identification number2. Name3. Address4. Hourly pay rate5. Number of exemptions claimed6. Year-to-date earnings7. Amount of taxes withheldIn the preceding example, each field is associated with the same employee. A data file can be implemented as a group of related records.1A company’s payroll file normally contains one record for each employee. Companies typically have many files, some containing millions, billions or even trillions of characters of information.1. In some operating systems, a file is viewed as nothing more than a collection ofbytes, and any organization of the bytes in a file (such as organizing the data into records) is a view created by the programmer.To facilitate the retrieval of specific records from a file, at least one field in each record can be chosen as a record key, which identifies a record as belonging to a particular person or entity and distinguishes that record from all others. For example, in a payroll record, the employee identification number normally would be the record key. Sequential FilesThere are many ways to organize records in a file. A common organization is called a sequential file in which records typically are stored in order by a record-key field. In a payroll file, records usually are placed in order by employee identification number. DatabasesMost businesses use many different files to store data. For example, a company might have payroll files, accounts receivable files (listing money due from clients), accounts payable files (listing money due to suppliers), inventory files (listing facts about all the items handled by the business) and many other files. Related files often are stored in a database. A collection of programs designed to create and manage databases is called a database management system (DBMS). You’ll learn about databases in Chapter 12 and you’ll do additional work with databases in Chapter 13, Web App Development with , and online Chapters 24–25.8.3. Files and StreamsVisual Basic views a file simply as a sequential stream of bytes (Fig. 8.2). Depending on the operating system, each file ends either with an end-of-file marker or at a specific byte number that’s recorded in a system-maintained administrative data structure for the file. You open a file from a Visual Basic app by creating an object that enables communication between an app and a particular file, such as an object of class StreamWriter to write text to a file or an object of class StreamReader to read text from a file.Fig. 8.2. Visual Basic’s view of an n-byte file.8.4. Test-Driving the Credit Inquiry AppA credit manager would like you to implement a Credit Inquiry app that enables the credit manager to separately search for and display account information for customers with• debit balances—customers who owe the company money for previously received goods and services• credit balances—customers to whom the company owes money• zero balances—customers who do not owe the company moneyThe app reads records from a text file then displays the contents of each record that matches the type selected by the credit manager, whom we shall refer to from this point forward simply as ―the user.‖Opening the FileWhen the user initially executes the Credit Inquiry app, the Button s at the bottom of the window are disabled (Fig. 8.3(a))—the user cannot interact with them until a file has been selected. The company could have several files containing account data, so to begin processing a file of accounts, the user selects Open...from the app’s custom File menu (Fig. 8.3(b)), which you’ll create in Section 8.6. This displays an Open dialog (Fig.8.3(c)) that allows the user to specify the name and location of the file from which the records will be read. In our case, we stored the file in the folder C:\DataFiles and named the file Accounts.txt. The left side of the dialog allows the user to locate the file on disk. The user can then select the file in the right side of the dialog and click the Open Button to submit the file name to the app. The File menu also provides an Exit menu item that allows the user to terminate the app.a) Initial GUI with Buttons disabled until the user selects a file from which to readrecordsb) Selecting the Open... menu item from the File menu displays the Open dialog inpart (c)c) The Open dialog allows the user to specify the location and name of the fileFig. 8.3. GUI for the Credit Inquiry app.Displaying Accounts with Debit, Credit and Zero BalancesAfter selecting a file name, the user can click one of the Button s at the bottom of the window to display the records that match the specified account type. Figure 8.4(a) shows the accounts with debit balances. Figure 8.4(b) shows the accounts with credit balances. Figure 8.4(c) shows the accounts with zero balances.a) Clicking the Debit Balances Button displays the accounts with positive balances(that is, the people who owe the company money)b) Clicking the Credit Balances Button displays the accounts with negative balances(that is, the people to whom the company owes money)c) Clicking the Zero Balances Button displays the accounts with zero balances (that is, the people who do not have a balance because they’ve already paid or have nothad any recent transactions)Fig.8.4. GUI for Credit Inquiry app.8.5. Writing Data Sequentially to a Text FileBefore we can implement the Credit Inquiry app, we must create the file from which that app will read records. Our first app builds the sequential file containing the account information for the company’s clients. For each client, the app obtains through its GUI the c lient’s account number, first name, last name and balance—the amount of money that the client owes to the company for previously purchased goods and services. The data obtained for each client constitutes a ―record‖ for that client. In this app, the accoun t number is used as the record key—files are often maintained in order by their record keys. For simplicity, this app assumes that the user enters records in account number order.GUI for the Create Accounts AppThe GUI for the Create Accounts app is shown in Fig. 8.5. This app introduces the Menu-Strip control which enables you to place a menu bar in your window. It alsointroduces ToolStripMenuItem controls which are used to create menus and menu items. We show how use the IDE to build the menu and menu items in Section 8.6. There you’ll see that the menu and menu item variable names are generated by the IDE and begin with capital letters. Like other controls, you can change the variable names in theProperties window by modifying the (Name) property.Fig. 8.5. GUI for the Create Accounts app.Interacting with the Create Accounts AppWhen the user initially executes this app, the Close menu item, the TextBox es and the Add Account Button are disabled (Fig. 8.6(a))—the user can interact with these controls only after specifying the file into which the records will be saved. To begin creating a fileof accounts, the user selects File > New... (Fig. 8.6(b)), which displays a Save As dialog (Fig. 8.6(c)) that allows the user to specify the name and location of the file into which the records will be placed. The File menu provides two other menu items—Close to close the file so the user can create another file and Exit to terminate the app. After the user specifies a file name, the app opens the file and enables the controls, so the user can begin entering account information. Figure 8.6(d)–(h) shows the sample data being entered for five accounts. The app does not depict how the records are stored in the file. This is a text file, so after you close the app, you can open the file in any text editor to see its contents. Figure 8.6(j)shows the file’s contents in Notepad.a) Initial GUI before user selects a fileb) Selecting New... to create a filec) Save As dialog displayed when user selects New... from the File menu. In this case,the user is naming the file Accounts.txt and placing the file in the C:\DataFilesfolder.d) Creating account 100e) Creating account 200f) Creating account 300g) Creating account 400h) Creating account 500i) Closing the filej) The Accounts.txt file open in Notepad to show how the records were written to the file. Note the comma separators between the data itemsFig. 8.6. User creating a text file of account information.8.5.1. Class CreateAccountsLet’s now study the declaration of class CreateAccounts, which begins in Fig. 8.7. Framework Class Library classes are grouped by functionality into namespaces, which make it easier for you to find the classes needed to perform particular tasks. Line 3 is an Imports statement, which indicates that we’re using classes from the System.IO namespace. This namespace contains stream classes such as StreamWriter (for text output) and Stream-Reader (for text input). Line 6 declares fileWriter as an instance variable of type Stream-Writer. We’ll use this variable to interact with the file that the user selects.Click here to view code image1' Fig. 8.7: CreateAccounts.vb2' App that creates a text file of account information.3Imports System.IO ' using classes from this namespace45Public Class CreateAccounts6Dim fileWriter As StreamWriter' writes data to text file7Fig. 8.7. App that creates a text file of account information.You must import System.IO before you can use the namespace’s classes. In fact, all namespaces except System must be imported into a program to use the classes in those namespaces. Namespace System is imported by default into every program. Classes like String, Convert and Math that we’ve used frequently in earlier examples are declared in the System namespace. So far, we have not used Imports statements in any of our programs, but we have used many classes from namespaces that must be imported. For example, all of the GUI controls you’ve used so far are classes in theSystem.Windows.Forms namespace.So why were we able to compile those programs? When you create a project, each Visual Basic project type automatically imports several namespaces that are commonlyused with that project type. You can see the namespaces (Fig. 8.8) that were automatically imported into your project by right clicking the project’s name in the Solution Explorer window, selecting Properties from the menu and clicking the References tab. The list appears under Imported namespaces:—each namespace with a checkmark is automatically imported into the project. This app is a Windows Forms app. The System.IO namespace is not imported by default. To import a namespace, you can either use an Imports statement (as in line 3 of Fig. 8.7) or you can scroll through the list in Fig. 8.8 and check the checkbox for the namespace you wish to import.Fig. 8.8. Viewing the namespaces that are pre-Imported into a Windows Forms app.8.5.2. Opening the FileWhen the user selects File > New..., method NewToolStripMenuItem_Click (Fig. 8.9) is called to handle the New... m enu item’s Click event. This method opens the file. First, line 12 calls method CloseFile (Fig. 8.11, lines 102–111) in case the user previously opened another file during the current execution of the app. CloseFile closes the file associated with this app’s StreamWriter.Click here to view code image8' create a new file in which accounts can be stored9Private Sub NewToolStripMenuItem_Click(sender As Object,10 e As EventArgs) Handles NewToolStripMenuItem.Click1112 CloseFile() ' ensure that any prior file is closed13Dim result As DialogResult' stores result of Save dialog14Dim fileName As String' name of file to save data1516' display dialog so user can choose the name of the file to save17Using fileChooser As New SaveFileDialog()18 result = fileChooser.ShowDialog()19 fileName = fileChooser.FileName ' get specified file name20End Using' automatic call to fileChooser.Dispose() occurs here2122' if user did not click Cancel23If result <> Windows.Forms.DialogResult.Cancel Then24Try25' open or create file for writing26 fileWriter = New StreamWriter(fileName, True)2728' enable controls29 CloseToolStripMenuItem.Enabled = True30 addAccountButton.Enabled = True31 accountNumberTextBox.Enabled = True32 firstNameTextBox.Enabled = True33 lastNameTextBox.Enabled = True34 balanceTextBox.Enabled = True35Catch ex As IOException36 MessageBox.Show("Error Opening File", "Error",37MessageBoxButtons.OK, MessageBoxIcon.Error)38End Try39End If40End Sub' NewToolStripMenuItem_Click41Fig. 8.9. Using the SaveFileDialog to allow the user to select the file into whichrecords will be written.Next, lines 17–20 of Fig. 8.9 display the Save As dialog and get the file name specified by the user. First, line 17 creates the SaveFileDialog object (namespace System.Windows.Forms) named fileChooser. Line 18 calls its ShowDialog method to display the SaveFileDialog (Fig. 8.6(c)). This dialog prevents the user from interacting with any other window in the app until the user closes it by clicking either Save or Cancel, so it’s a modal dialog. The user selects the location where the file should be stored and specifies the file name, then clicks Save. Method ShowDialog returns a DialogResult enumeration constant specifying which button (Save or Cancel) the user clicked to close the dialog. This is assigned to the DialogResult variable result (line 18). Line 19 uses SaveFileDialog property FileName to obtain the location and name of the file.8.5.3. Managing Resources with the Using StatementLines 17–20 introduce the Using statement, which simplifies writing code in which you obtain, use and release a resource. In this case, the resource is a SaveFileDialog. Windows and dialogs are limited system resources that occupy memory and should be returned to the system (to free up that memory) as soon as they’re no longer needed. Inall our previous apps, this happens when the app terminates. In a long-running app, if resources are not returned to the system when they’re no longer needed, a resource leak occurs and the resources are not available for use in this or other apps. Objects that represent such resources typically provide a Dispose method that must be called to return the resources to the system. The Using statement in lines 17–20 creates a SaveFileDialog object, uses it in lines 18–19, then automatically calls its Dispose method to release the object’s resources as soon as End Using is reached, thusguaranteeing that the resources are returned to the system and the memory they occupy is freed up (even if an exception occurs).Line 23 tests whether the user clicked Cancel by comparing result to the constant Windows.Forms.DialogResult.Cancel. If not, line 26 creates a StreamWriter object that we’ll use to write data to the file. The two arguments are a String representing the location and name of the file, and a Boolean indicating what to do if the file already exists. If the file doesn’t exist, this statement creates the file. If the file does exist, the second argument (True) indicates that new data written to the file should be appended at the end of the file’s current contents. If the second argument is False and the file already exists, the file’s contents will be discarded and new data will be written starting at the beginning of the file. Lines 29–34 enable the Close menu item and the TextBox es and Button that are used to enter records into the app. Lines 35–37 catch an IOException if there’s a problem opening the file. If so, the app displays an error message. If no exception occurs, the file is opened for writing. Most file-processing operations have the potential to throw exceptions, so such operations are typically placed in Try statements.8.5.4. Adding an Account to the FileAfter typing information in each TextBox, the user clicks the Add Account Button, which calls method addAccountButton_Click (Fig. 8.10) to save the data into the file. If the user entered a valid account number (that is, an integer greater than zero), lines 56–59 write the record to the file by invoking the StreamWriter’s WriteLine method, which writes a sequence of characters to the file and positions the output cursor to the beginning of the next line in the file. We separate each field in the record with a comma in this example (this is known as a comma-delimited text file), and we place each record on its own line in the file. If an IOException occurs when attempting to write the record to the file, lines 64–66 Catch the exception and display an appropriate message to the user. Similarly, if the user entered invalid data in the accountNumberTextBox or balanceTextBox lines 67–69 catch the FormatExceptions thrown by class Convert’s methods and display an appropriate error message. Lines 73–77 clear the TextBox es and return the focus to the accountNumberTextBox so the user can enter the next record. Click here to view code image42' add an account to the file43Private Sub addAccountButton_Click(sender As Object,44 e As EventArgs) Handles addAccountButton.Click4546' determine whether TextBox account field is empty47If accountNumberTextBox.Text <> String.Empty Then48' try to store record to file49Try50' get account number51Dim accountNumber As Integer =52 Convert.ToInt32(accountNumberTextBox.Text)5354If accountNumber > 0Then' valid account number?55' write record data to file separating fields by commas56 fileWriter.WriteLine(accountNumber & "," &57 firstNameTextBox.Text & "," &58 lastNameTextBox.Text & "," &59 Convert.ToDecimal(balanceTextBox.Text))60Else61 MessageBox.Show("Invalid Account Number", "Error",62MessageBoxButtons.OK, MessageBoxIcon.Error)63End If64Catch ex As IOException65 MessageBox.Show("Error Writing to File", "Error",66MessageBoxButtons.OK, MessageBoxIcon.Error)67Catch ex As FormatException68 MessageBox.Show("Invalid account number or balance",69"Format Error", MessageBoxButtons.OK,MessageBoxIcon.Error)70End Try71End If7273 accountNumberTextBox.Clear()74 firstNameTextBox.Clear()75 lastNameTextBox.Clear()76 balanceTextBox.Clear()77 accountNumberTextBox.Focus()78End Sub' addAccountButton_Click79Fig. 8.10. Writing an account record to the file.8.5.5. Closing the File and Terminating the AppWhen the user selects File > Close, method CloseToolStripMenuItem_Click (Fig. 8.11, lines 81–91) calls method CloseFile (lines 102–111) to close the file. Then lines 85–90 disable the controls that should not be available when a file is not open.Click here to view code image80' close the currently open file and disable controls81Private Sub CloseToolStripMenuItem_Click(sender As Object,82 e As EventArgs) Handles CloseToolStripMenuItem.Click8384 CloseFile() ' close currently open file85 CloseToolStripMenuItem.Enabled = False86 addAccountButton.Enabled = False87 accountNumberTextBox.Enabled = False88 firstNameTextBox.Enabled = False89 lastNameTextBox.Enabled = False90 balanceTextBox.Enabled = False91End Sub' CloseToolStripMenuItem_Click9293' exit the app94Private Sub ExitToolStripMenuItem_Click(sender As Object,95 e As EventArgs) Handles ExitToolStripMenuItem.Click9697 CloseFile() ' close the file before terminating app98 Application.Exit() ' terminate the app99End Sub' ExitToolStripMenuItem_Click100101' close the file102Sub CloseFile()103If fileWriter IsNot Nothing Then104Try105 fileWriter.Close() ' close StreamWriter106Catch ex As IOException107 MessageBox.Show("Error closing file", "Error",108MessageBoxButtons.OK, MessageBoxIcon.Error)109End Try110End If111End Sub' CloseFile112End Class' CreateAccountsFig. 8.11. Closing the file and terminating the app.When the user clicks the Exit menu item, method ExitToolStripMenuItem_Click (lines 94–99) respo nds to the menu item’s Click event by exiting the app. Line 97 closes the StreamWriter and the associated file, then line 98 terminates the app. The call to method Close (line 105) is located in a Try block. Method Close throws an IOExceptionif the file cannot be closed properly. In this case, it’s important to notify the user that the information in the file or stream might be corrupted.8.6. Building Menus with the Windows Forms DesignerIn the test-drive of the Credit Inquiry app (Section 8.4) and in the overview of the Create Accounts app (Section 8.5), we demonstrated how menus provide a convenient way to organize the commands that you use to interact with an app without ―cluttering‖ its user interface. Menus contain groups of related commands. When a command is selected, the app performs a specific action (for example, select a file to open, exit the app, etc.).Menus make it simple and straightforward to locate an app’s commands. They can also make it easier for users to use apps. For example, many apps provide a File menu that contains an Exit menu item to terminate the app. If this menu item is always placed in the File menu, then users become accustomed to going to the File menu to terminate an app. When they use a new app and it has a File menu, they’ll already be familiar with the location of the Exit command.The menu that contains a menu ite m is that menu item’s parent menu. In the Create Accounts app, File is the parent menu that contains three menu items—Ne w..., Close and Exit.Adding a MenuStrip to the FormBefore you can place a menu on your app, you must provide a MenuStrip to organize and manage the app’s menus. Double click the MenuStrip control in the Toolbox. This creates a menu bar (the MenuStrip) across the top of the Form (below the title bar; Fig.8.12) and places a MenuStrip icon in the component tray (the gray area) at the bottom of the designer. You can access the MenuStrip’s properties in the Properties window by clicking the MenuStrip icon in the component tray. We set the MenuStrip’s (Name) property to applicationMenuStrip.。
lecture的意思用法大全
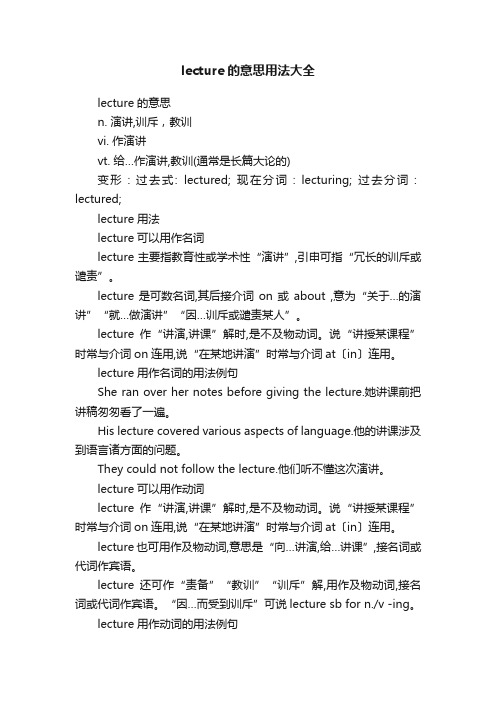
lecture的意思用法大全lecture的意思n. 演讲,训斥,教训vi. 作演讲vt. 给…作演讲,教训(通常是长篇大论的)变形:过去式: lectured; 现在分词:lecturing; 过去分词:lectured;lecture用法lecture可以用作名词lecture主要指教育性或学术性“演讲”,引申可指“冗长的训斥或谴责”。
lecture是可数名词,其后接介词on或about ,意为“关于…的演讲”“就…做演讲”“因…训斥或谴责某人”。
lecture作“讲演,讲课”解时,是不及物动词。
说“讲授某课程”时常与介词on连用,说“在某地讲演”时常与介词at〔in〕连用。
lecture用作名词的用法例句She ran over her notes before giving the lecture.她讲课前把讲稿匆匆看了一遍。
His lecture covered various aspects of language.他的讲课涉及到语言诸方面的问题。
They could not follow the lecture.他们听不懂这次演讲。
lecture可以用作动词lecture作“讲演,讲课”解时,是不及物动词。
说“讲授某课程”时常与介词on连用,说“在某地讲演”时常与介词at〔in〕连用。
lecture也可用作及物动词,意思是“向…讲演,给…讲课”,接名词或代词作宾语。
lecture还可作“责备”“教训”“训斥”解,用作及物动词,接名词或代词作宾语。
“因…而受到训斥”可说lecture sb for n./v -ing。
lecture用作动词的用法例句It was a shame for me to be lectured in front of the whole class.当着整个班级的面被训斥了一顿,真让我感到羞辱。
He lectured to his students on modern writers.他给学生们讲了关于现代作家的一课。
2012年英语专业八级真题解析

专八 2012 - 1 1
classified as "observation with intervention" or "observation without intervention". Observation with inter vention can be made in at least two ways, [ 11]participant observation and field experiment. In participant observation, observers, that is researchers, play a dual role: They observe people's behaviour and they par ticipate actively in the situation they are observing. If individuals who are being (!bserved lmow that the ob server is present to collect information about their behaviour, this is undisguised participant observation. But in disguised participant observation, those who are being observed do not lmow that they are being ob served.
托福听力tpo67全套对话讲座原文+题目+答案+译文

托福听力tpo67全套对话讲座原文+题目+答案+译文Section1 (1)Conversation1 (2)原文 (2)题目 (4)答案 (6)译文 (6)Lecture1 (8)原文 (8)题目 (10)答案 (12)译文 (13)Lecture2 (14)原文 (14)题目 (16)答案 (18)译文 (19)Section2 (20)Conversation2 (20)原文 (20)题目 (23)答案 (25)译文 (25)Lecture3 (27)原文 (27)题目 (29)答案 (31)译文 (32)Section1Conversation1原文Student:Hi.I know it's Friday afternoon and all,but this is kind of an emergency.Supervisor:Oh,what kind of emergency?Exactly?Student:Well,I mean,there's no danger or anything.It's like a personal emergency. It's about my apartment.Supervisor:Well,I really only deal with dormitories.The apartment facilities, supervisors,offices,next door room,208ask for Jim.Student:I just came from there.They sent me to you.It's a problem with my stove.Supervisor:And they sent you here.All right.Now,what's the problem?Student:My stove isn't working at all.It won't even turn on.Supervisor:It's electric?Student:Yes.Supervisor:Okay,our electrician is out today,his daughter is getting married tomorrow.So realistically he probably won't get to it until Monday afternoon. Perhaps Tuesday.Student:Really,we have to go without a stove for a whole weekend,possibly more?Supervisor:Yes,as you pointed out,this is not a dire emergency,so it's going to be handled under the normal maintenance schedule,which is Monday through Friday.And I know for a fact that Monday is already pretty tight,the electrician will have a lot to catch up on.So when I say possibly Tuesday,I'm just trying to be realistic.Student:But I really rely on that stove.I don't have any kind of on campus dining arrangement or contract.Supervisor:Well,I understand,but…Student:And it's not even the whole problem.I'm expecting a bunch of people to show up tomorrow night.I'm going to be hosting a meeting of the editorial staff of the school paper.And a dinner was scheduled.Supervisor:Now I see which you meant by a personal emergency,but all I can really do is put in a work request.I'm sorry.Student:I just got finished shopping for all the food for the meeting.Ah.I guess,I'll just have to call it off.Supervisor:Why would you cancel the meeting?Student:Well,I mean,I could do it next week.Supervisor:Couldn't you like use a neighbour or something?Student:I don't think so.I mean,the only neighbors I really know,well enough to ask the guys next door,if you saw the state of that kitchen,you'd understand.I'm not sure I could find the stove under all the mess.Supervisor:I see.Well,we could try to set you up in one of our conference rooms in the Johnson building.Student:Really?I thought that student groups couldn't book the rooms in Johnson.Supervisor:Well,normally they can't.However,given your situation,I can try to putin a word with some people and see if we can make an exception here.There is also a full kitchen in the Johnson building,so you'd be covered there.Student:Okay.Yes.That definitely would work.Um.Do you have any idea when you know if you can make this happen or not?Because I'll need to let people know.Supervisor:Yeah,I understand people need to know what's going on.Um.Let me get back to you in an hour or so on this.Can you leave me your phone number?Student:Sure.Thanks.题目1.Why does the woman go to see the facilities supervisor?A.To find out where there is a stove that she can useB.To complain about her treatment in another facilities officeC.To ask if a meeting can be moved to another locationD.To schedule repairs for a broken appliance2.Why does the woman believe that her problem is a serious one?[Click on2 answers.]A.She does not have an on-campus option for meals.B.She is concerned that the stove could be dangerous.C.She knows that other students have had similar problems.D.She was relying on using the stove for an upcoming event.3.What will the woman probably do next?A.Request an emergency repair for her stoveB.Prepare a meal that does not need to be cookedC.Move her event to a different locationD.Reschedule her event to the following week4.What does the woman imply about her next-door neighbors?A.Their kitchen is too dirty for her to use.B.Their stove is not functioning properly.C.They do not let other people use their stove.D.They will be using their kitchen this weekend.5.What can be inferred about the supervisor when he says this:Student:I just got finished shopping for all the food for the meeting.Ah.I guess,I'll just have to call it off.Supervisor:Why would you cancel the meeting?Student:Well,I mean,I could do it next week.A.He feels sorry for the woman.B.He believes that the woman's plan of action is not necessary.C.He wants to know the reason for the woman's decision.D.He wants the woman to confirm her plan.答案D AD C A B译文1.学生:嗨。
托福听力tpo39 全套对话讲座原文+题目+答案+译文

托福听力tpo39全套对话讲座原文+题目+答案+译文Section1 (2)Conversation1 (2)原文 (2)题目 (4)答案 (5)译文 (5)Lecture1 (7)原文 (7)题目 (9)答案 (11)译文 (11)Lecture2 (13)原文 (13)题目 (16)答案 (17)译文 (18)Section2 (20)Conversation2 (20)原文 (20)题目 (22)答案 (23)译文 (23)Lecture3 (25)原文 (25)题目 (27)答案 (29)译文 (29)Lecture4 (30)原文 (30)题目 (33)答案 (34)译文 (35)Section1Conversation1原文NARRATOR:Listen to a conversation between a student and a theater professor.MALE STUDENT:Hi,Professor Jones.FEMALE PROFESSOR:Hey,didn't I see you at the performance of Crimes of the Heart last night?MALE STUDENT:Yeah…actually my roommate had a small part in it.FEMALE PROFESSOR:Really?I was impressed with the performance—there sure are some talented people here!What did you think?MALE STUDENT:You know,Beth Henley's an OK playwright;she's written some decent stuff,but it was a little too traditional,a little too ordinary…especially considering the research I’m doing.FEMALE PROFESSOR:Oh,what’s that?MALE STUDENT:On the Polish theater director Jerzy Grotowski.FEMALE PROFESSOR:Grotowski,yeah,that's a little out of the mainstream…pretty experimental.MALE STUDENT:That’s what I wanted to talk to you about.I had a question about our essay and presentation.FEMALE PROFESSOR:OK…MALE STUDENT:Yeah,some of these ideas,uh,Grotowski's ideas,are really hard to understand—they're very abstract,philosophical—and,well,I thought the class would get more out of it if I acted out some of it to demonstrate.FEMALE PROFESSOR:Interesting idea…and what happens to the essay?MALE STUDENT:Well,I'll do the best I can with that,but supplement it with the performance—you know,bring it to life.FEMALE PROFESSOR:All right,but what exactly are we talking about here?Grotowski, as I'm sure you know,had several phases in his career.MALE STUDENT:Right.Well,I’m mainly interested in his idea from the late1960s…Poor theater,you know,a reaction against a lot of props,lights,fancy costumes,and all that…so,it’d be good for the classroom.I wouldn’t need anything special.FEMALE PROFESSOR:Yes.I’m sure a lot of your classmates are unfamiliar with Grotowski—this would be good for them.MALE STUDENT:Right,and this leads…I think there's overlap between his Poor theater phase and another phase of his,when he was concerned with the relationship between performers and the audience.I also want to read more and write about that.FEMALE PROFESSOR:You know,I saw a performance several years ago…it really threw me for a loop.You know,you're used to just watching a play,sitting back…but this performance,borrowing Grotowski's principles,was really confrontational—a little uncomfortable.The actors looked right in our eyes,even moved us around, involved us in the action.MALE STUDENT:Yeah,I hope I can do the same when I perform for the class.I'm a bit worried,since the acting is so physical,that there's so much physical preparation involved.FEMALE PROFESSOR:Well,some actors spend their whole lives working on this…so don't expect to get very far in a few weeks…but I'm sure you can bring a couple of points across.And,if you need some extra class time,let me know.MALE STUDENT:No,I think I can fit it into the regular time for the presentation.FEMALE PROFESSOR:OK.I think this'll provide for some good discussion about these ideas,and other aspects of the audience and their relationship to theatricalproductions.题目1.What are the speakers mainly discussing?A.A play by Grotowski that was discussed in class.B.A proposal that the student has for an assignment.C.A play that is currently being performed at the university.D.The main phases in Grotowski's career as a director.2.What does the student imply when he talks about the play he recently attended?A.He attended the play because he is writing an essay on it.B.He wished the play were more experimental.C.He thought his roommate showed great talent.D.He was not familiar with the author of the play.3.What are two characteristics of Grotowski's theater that the speakers mention?[Click on2answers.]A.The minimal equipment on the stage in his productions.B.The single stories that his plays are based on.C.The elaborate costumes the actors wear in his plays.D.The actions of the performers in his plays.4.Why does the professor mention a play she attended several years ago?A.To compare it to the play she saw the previous evening.B.To suggest that Grotowski's principles do not necessarily lead to effective theater.C.To show how different it was from Poor theater.D.To provide an example of one of the ideas the student wants to research.5.What does the professor imply about the acting the student wants to do?A.Audiences are no longer surprised by that type of acting.B.The acting requires less physical preparation than he thinks.C.He will not be able to master that style of acting easily.D.He should spend less time acting for the class and more time on class discussion.答案B B AD D C译文旁白:请听一段学生和戏剧学教授之间的对话。
lecture 8
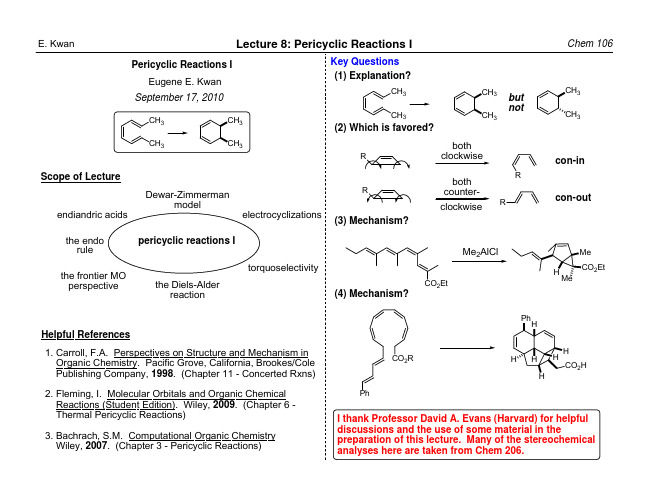
R R R
R
R
R
one phase inversion Möbius topology, 4 e, allowed
zero inversions Hückel topology, 4 e, forbidden
but not
In transition states, it is much easier to get to a Möbius topology. For example, dienes are known to close to cyclobutanes. We can draw arrows for this process:
R
However, the curly arrows don't give any guidance for whether the R groups end up syn or anti to each other. In fact, they end up syn. How did that happen?
R R
6. Finally, decide how many -electrons are involved. Here, there are four. As stated earlier: Aromatic (allowed) Hückel Topology Möbius Topology 4n+2 4n Anti-aromatic (not allowed) 4n 4n+2
Scope of Lecture Dewar-Zimmerman model endiandric acids the endo rule the frontier MO perspective pericyclic reactions I electrocyclizations
Contents COURSE STAFF......................................................................
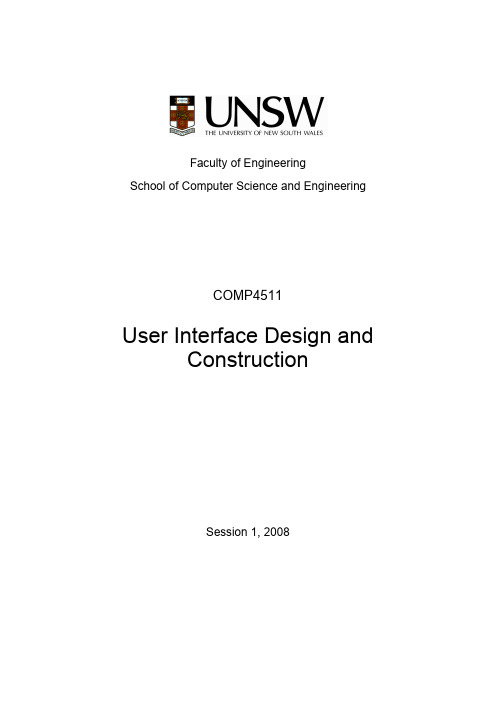
Faculty of EngineeringSchool of Computer Science and EngineeringCOMP4511User Interface Design andConstructionSession 1, 2008ContentsCOURSE STAFF (2)COURSE DETAILS (2)TIMES (2)COURSE AIMS (2)LEARNING OUTCOMES (4)RATIONALE (4)TEACHING STRATEGIES (4)ASSESSMENT (5)ACADEMIC HONESTY AND PLAGIARISM (6)COURSE SCHEDULE (7)RESOURCES FOR STUDENTS (7)COURSE EVALUATION AND DEVELOPMENT (8)OTHER MATTERS (8)Course staff•Daniel Woo, Lecturer in Chargeo Room 307-K17o9385 6495o danielw@.au•Outside of consultation times e-mail Daniel directly or locate on-line course content via .au/~cs4511Course details• 6 units of credit (UoC)•Pre- and co-requisiteso COMP3511/9511 Human Computer Interaction (User centred design) (pre-requisite)o COMP4001 Object-Oriented Software Development (C++, UML) (co-requisite)•The course is currently run as a 4th year elective.•Postgraduate students are permitted to undertake this course under the undergraduate course code. Postgraduate students are assigned an additional assignment exercise to write a technical paper on a topic relevant to user interface software developmentTimes•Lectures: Monday 11:00am-1:00pm, Wed 10:00-11:00am•Studio: Thursday 3:00-6:00pmCourse aims•Extend paper-based user-centred design techniques introduced in COMP3511/9511 and object-oriented design concepts introduced in COMP4001•Provide practical object-oriented software development skills specifically for graphical user interfaces•Understand the design and programming constraints user interfaces•Provide experience in usability testing of software applicationsLearning outcomes•Write applications in the Objective-C programming language•Design and implement graphical user interface (GUI) software•Write two user interface software applications as part of assignment work to first gain confidence in developing GUI applications and then to develop your skills with more complex behaviour •Understand the role design patterns in user interface software, notably the model view controller and state design patterns•Understand concepts such as event handling and views•Develop graphical user interface software with features that support copy, paste, undo, menus and responding to mouse and keyboard actions•Conduct peer usability evaluations of software developed in this course•Describe aspects of your software using object oriented techniques such as Unified Markup Language (UML)•Become competent with version control systems to maintain source code and other project related documents•Work collaboratively in a multidisciplinary environmentRationale•COMP4511 is a highly practical course that introduces you to the programming aspects of user interface software. We do not assume that you have developed such an application before but require that you feel competent in object-oriented principles and programming techniques •Usability evaluation is a key component in the software design lifecycle, so the designs that you develop in this course will be evaluated by your peers. This provides feedback to help improve your design and gives you further experience to evaluate software systems.•We build on the user centred design principles introduced in COMP3511 expecting that you will conduct user interviews to better understand requirements, develop paper or electronic prototypes of your design and conduct usability walkthroughs of your designs preferably before you write code (but we understand that this is possibly the first time that you have engaged in this process). •One of your assignment tasks will be to develop an interactive application in the domain that is of interest to you. We believe that by helping you create something that you are passionate about then your desire to excel and learn far outweighs completing a project for which you have no interest.•The lecture and studio are conducted in the CHI Lab (G11-K17) so that you have the tools and technologies immediately in front of you during class. This allows us experiment with the material whilst we are learning, addressing practical issues as they arise.•Video streaming content will be used to provide on-line course material. The Moodle class web site will host an on-line forum. We are very interested to better understand user/student needs for on-line course delivery•Based on the feedback we have had from both former students and some of their employers, the students who successfully completed this course have a balanced view of the software development process: they care about user needs, they can carry out the user centred design process, they understand the rigour and technical demands of software engineering, and they have applied knowledge in the area of usability evaluation•We train software aware students to be more than just programmersTeaching strategies•The CHI lab will be available for access to Mac based computers for self study programming exercises, conducting usability tests and hold team design meetings•Thursday 3-6pm is reserved for “studio” where we conduct workshop exercises that are focussed on usability, in-class design or coding exercises. Tutors and the lecturer will be available to discuss and review your progress•Thursday 3-4pm will be also be used for small group or individual consultation to review progress and assigned checkpoints•Additional lecture material will be provided on-line using streaming multimedia to cover design topics and more in-depth programming topics•The Moodle site will be used to conduct on-line discussion.•The overall format of the lectures and studios provide opportunity for feedback and discussionAssessmentThe exact assignment topics are still subject to change given that we are investigating collaboration with the school of industrial design and possibly working with a commercial organisation as a possible “client” that will help focus the work around a theme. Typically we would allow you to choose your own topic. This will be discussed in classes in Week 1.•Assignment 1: Introductory User Interface Involving Timeo Code Due Week 5, Fridayo15 %o Assessment will be based on Software Design, Code Implementation, User Interface Design, Version Control, Paper Prototyping, User Interviewso There will be other deliverables in studio to demonstrate progress•Assignment 2: Part 1 Design and Prototyping of an Interactive Applicationo Code Due Week 8, Thursday 2pmo15 %o Assessment will be based on Project Planning, User Centred Design, Paper Prototyping, Software Design, Code Prototyping, Version Control and UsabilityEvaluationso There will be other deliverables in studio to demonstrate progress•Assignment 2: Part 2 Implementation and Evaluation of an Interactive Applicationo Code Due Week 11, Thursday 2pmo25 %o Assessment will be based on Iterative Improvements, Additional Features, Code Implementations, Version Control, Usability Evaluations and Final Poster Presentation o There will be other deliverables in studio to demonstrate progress•Participation (Undergraduate)o5%o Studio Participationo Design Diary useo Reflection on Progress in Journal•Paper (Postgraduate)o5%o Research Paper on a topic related to interaction design and user centred designo Participation will be recorded•Final Examinationo40%o Written examination•Assignment 1 is an introductory assignment that allows you to learn about the Objective C language and develop a basic graphical user interface application that can respond to simple events generated by timers•Assignment 2 is in two parts and is designed to be your major project for the course. You may choose to develop an application that is interactive and supports the concept of direct manipulation. This will involve responding to mouse and keyboard events and drawing to the screen using the graphics functionality available in Cocoa. Examples could be a drawing application, a furniture layout application or a file system / Finder replacement.•Assignment source code is not explicitly submitted but will utilise the version control system (subversion) used in the course. On the due date, your assignment repository will be copied and the tagged branch will be copied and used as the assessable work. Written design reports should also be kept in the repository with any other non-electronic submissions handed in to class on the due date.•Assignment code will be kept in the repository trunk. Submissions will use a tagged branch.Documentation relating to the assignments will be kept in the repository and/or the on-line trac/wiki system (which is different from Moodle).•All electronic work submitted will be retained by the University of New South Wales and can be used for teaching, research and review purposes. We will acknowledge your contribution if you wish, or withhold your name should you choose to remain anonymous.•You also have the right to use your electronic submissions for your own personal use. You must retain any copyright notices contained in other code used in your submissions so the origin of the source is retained (eg. From class examples).•Any data provided as part of assignments (eg. Test data sets) may not be used for commercial purposes and must not be provided in any form to any other party.Academic honesty and plagiarismWhat is Plagiarism?Plagiarism is the presentation of the thoughts or work of another as one’s own.* Examples include:•direct duplication of the thoughts or work of another, including by copying material, ideas or concepts from a book, article, report or other written document (whether published orunpublished), composition, artwork, design, drawing, circuitry, computer program or software, web site, Internet, other electronic resource, or another person’s assignment without appropriateacknowledgement;•paraphrasing another person’s work with very minor changes keeping the meaning, form and/or progression of ideas of the original;•piecing together sections of the work of others into a new whole;•presenting an assessment item as independent work when it has been produced in whole or part in collusion with other people, for example, another student or a tutor; and•claiming credit for a proportion a work contributed to a group assessment item that is greater than that actually contributed.†For the purposes of this policy, submitting an assessment item that has already been submitted for academic credit elsewhere may be considered plagiarism.Knowingly permitting your work to be copied by another student may also be considered to be plagiarism.Note that an assessment item produced in oral, not written, form, or involving live presentation, may similarly contain plagiarised material.The inclusion of the thoughts or work of another with attribution appropriate to the academic discipline does not amount to plagiarism.The Learning Centre website is main repository for resources for staff and students on plagiarism and academic honesty. These resources can be located via:.au/plagiarismThe Learning Centre also provides substantial educational written materials, workshops, and tutorials to aid students, for example, in:•correct referencing practices;•paraphrasing, summarising, essay writing, and time management;•appropriate use of, and attribution for, a range of materials including text, images, formulae and concepts.Individual assistance is available on request from The Learning Centre.Students are also reminded that careful time management is an important part of study and one of the identified causes of plagiarism is poor time management. Students should allow sufficient time for research, drafting, and the proper referencing of sources in preparing all assessment items.* Based on that proposed to the University of Newcastle by the St James Ethics Centre. Used with kind permission from the University of Newcastle† Adapted with kind permission from the University of Melbourne.Course scheduleSubject to changesLectures Studio Assignment Deliverables Week 0Week 1 Course IntroductionGoal Directed DesignIntroduction Obj-CMemoryFoundation Classes Object Oriented Design Interface Builder Actions and Outlets Assignment 1 DesignWeek 2 Contacts ExampleModel View ControllerTablesTimers Coding StyleDebuggingVersion ControlAssignment 1 DesignA1 Concept DocumentMid Semester BreakWeek 3 ControlsArchivingMenusToolbarsDialogs Unit Testing Usability TestingWeek 4 User Centred DesignProcess Assignment 1Usability EvaluationA1 OO Design BriefWeek 5 ViewsDrawingEventsA1 Presentation A1 Code DueWeek 6 Graphics ApplicationBehaviour and Form A1 ReviewA2 ConceptsA2 Project PlanWeek 7 Graphics ApplicationState Design PatternA2 Walkthroughs A2 Concept DocumentWeek 8 Graphics ApplicationCopy / Paste / UndoNIB and Window ControllersA2 Usability Evaluations A2 Code Part 1 DueWeek 9 Interaction Details A2 Usability EvaluationsWeek 10 Document ArchitectureUser PreferencesA2 Usability Evaluations A2 Usability ReportWeek 11 Interaction Design Topics A2 Usability Evaluations A2 Code Part 2 Due Week 12 Project/UCD Reflection A2 Presentations A2 Final ReportResources for studentsRequired Text Books•Cooper, Reimann and Cronin (2007), About Face 3: The Essentials of Interaction Design, John Wiley•Hillegrass (2004), Cocoa Programming for Mac OS X (2nd Ed), Addison WesleyReferences from COMP3511/9511 or COMP4001•Preece, Rogers, Sharp (2007), Interaction Design Beyond Human Computer Interaction, John Wiley & Sons Inc.•Nielsen (1993), Usability Engineering, Morgan Kaufmann.•Gamma, Helm, Johnson and Vlissides (1995), Design Patterns: Elements of Reusable Object-Oriented Software, Addison-Wesley.Other References•Apple Computer Inc. (2001), Learning Cocoa, O’Reilly and Associates Inc.•Garfunkel and Mahoney (2002), Building Cocoa Applications, O’Reilly and Associates Inc. •Kochran, SG (2004), Programming in Objective-C, Sams Publishing•Students seeking resources can also obtain assistance from the UNSW Library. One starting point for assistance is:.au/web/services/services.htmlOther Materials•Design Diary A4 or A3 bound sketchpad for design work. This will be assessed during Studio consultation.•Post-it Notes™, coloured pens and pencils will be used as part of the design work. Please use only Blu-Tack™ for placing posters on walls. Do not use sticky or masking tape.Course evaluation and development•We will use both paper-based and electronic survey tools throughout the session to gather feedback about the course. This is used to assess the quality of the course in order to make on going improvements. We do take this feedback seriously and approach the design of this course using the user centred design philosophies.Other matters•Students are expected to attend all classes•Please review the official school policies available at .au/~studentoffice/policies/yellowform.html. It contains important information regarding use of laboratories, originality of assignment submissions and special consideration. Note that in order to receive a CSE login account you must have agreed to the conditions stated in that document.•The Yellow Form also states the supplementary assessment policy and outlines what to do in case illness or misadventure that affects your assessment, and supplementary examinations procedures within the School of Computer Science and Engineering•Please read and understand the School Policy in relation to laboratory conduct.•Note that no food or drink is permitted in the laboratory. CSE fines will apply.•The laboratory is to be secured at all times. No equipment or furniture can be removed from the laboratory.•You are not permitted to provide unauthorised access to this laboratory.•UNSW Occupational Health and Safety policies and expectations.au/ohs/ohs.shtml•Computer Ergonomics for Students.au/ergonomics/ergoadjust.html•OHS Responsibility and Accountability for Students.au/ergonomics/ohs.html•Students who have a disability are encouraged to discuss their study needs with the course convener prior to, or at the commencement of the course, or with the Equity Officer (Disability) in the Equity and Diversity Unit (9385 4734). Information for students with disabilities is available at: .au/disabil.htmlIssues to be discussed may include access to materials, signers or note-takers, the provision of services and additional examination and assessment arrangements. Early notification is essential to enable any necessary adjustments to be made. Information on designing courses and course outlines that take into account the needs of students with disabilities can be found at:.au/acboardcom/minutes/coe/disabilityguidelines.pdf。
lecture 08
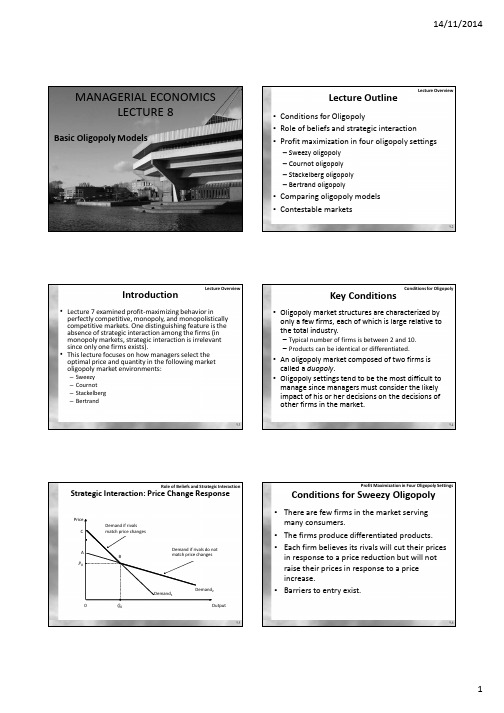
9-6
1
14/11/2014
Price A ܲ
0
Profit Maximization in Four Oligopoly Settings
Sweezy Oligopoly
Sweezy Demand B
C
E MR
ܳ
F MR2
MC0
MC1 Demand1 (rival holds price constant) MR1 Demand2 (rival matches price change)
and cost functions, ܥଵ ܳଵ ൌ ܿଵܳଵ and
ܥଶ ܳଶ ൌ ܿଶܳଶ the reactions functions are:
ܳଵ ൌ ݎଵ ܳଶ
=
ܽ
െ ܿଵ ʹܾ
−
1 2
ܳଶ
ܳଶ ൌ ݎଶ ܳଵ
=
ܽ
െ ܿଶ ʹܾ
−
1 2
ܳଵ
9-10
Profit Maximization in Four Oligopoly Settings
14/11/2014
MANAGERIAL ECONOMICS LECTURE 8
Basic Oligopoly Models
Lecture Outline
Lecture8
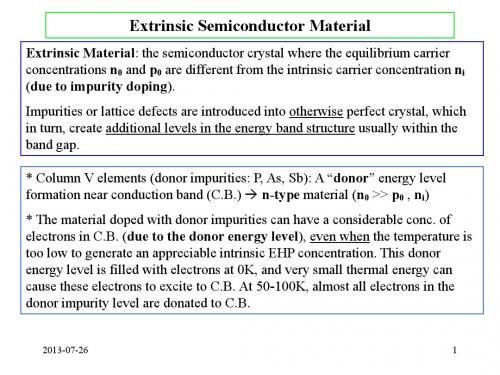
- Amphoteric impurities (Si, Ge) in III-V compound (in GaAs) :
- Si or Ge (column IV impurities) can serve as donors or acceptors, depending on whether they occupy the column III or column V sublattice of the crystal.
In III-V Compounds (in GaAs) :
- Column VI impurities (S, Se, Te) occupying column V sites (As) serve as donors, and column II impurities (Be, Zn, Cd) occupying column III serve as acceptors.
2013-07-26
4
Electrons and Holes in Quantum Wells
1. Single-valued (discrete) energy levels in the band gap arising from doping (donor/acceptor energy level)
•This acceptor level is empty of electrons at 0K, and very small thermal energy can excite the electrons from V.B. to acceptor level. At 50-100K, almost all empty sites in the acceptor impurity level are filled with the electrons, leaving behind holes in V.B.
、幅度调制(PDF)

DSB-SC 的波形特征:当()m t 为负时,()s t 的包络被翻了过来(图4.2.2)DSB-SC 的频谱特征:和AM 一样,都是双边带,带宽是基带的2倍,但没有载频线谱(假设()m t 不含直流)。
见图4.2.3。
标准调幅AM 和DSB-SC 都属于DSB 。
不过缺省情况下,我们说的DSB 指DSB-SC 。
四 SSBDSB 的两个边带是对称的,已知其中一个边带,等于已知两个边带。
因此,发送DSB 的时候,如果我们用滤波器切去一个边带,收端也应该有办法复原出DSB 或者()m t 。
这样的设计叫SSB 。
对于上单边带调制,()USB s t 的频谱是 ()DSB s t 频谱的上边带,其复包络()USB s t %就是()m t 的正频率部分,也即()USB s t %就是()m t 的解析信号,即()()()ˆUSB s t m t jm t =+%()()()ˆcos 2sin 2USB c c s t m t f t m t f t ππ=−显然,通过相干解调就可以解出 ()m t 。
对于下单边带,同理可得()()()ˆcos 2sin 2LSB c c s t m t f t m t f t ππ=+总之就是()()()ˆcos 2sin 2USB c c c s t A m t f t m t f t ππ= µ这个表达式说明,()SSB s t是由两个载波互相正交的DSB 构成的,后一个DSB 所起的作用就是为了抵消前一个DSB 中的一个边带。
这个结构显示出SSB 也可以用两个DSB 调制来实现(图4.2.13),这种实现方式叫正交调制法,而图4.2.11的方法叫滤波法。
另外,SSB 也可以这样解调:插入大载波来包络检波()()ˆcos 2cos 2sin 2USB c c c c c s A f t A A m t f t A m t f t πππ+=+ µ。
MSE_SE-Guidelines-2012

GUIDELINES FOR M.S.E. DEGREE IN CIVIL ENGINEERING:CONCENTRATION IN STRUCTURAL ENGINEERING1GeneralAn applicant for the M.S.E. degree must present the equivalent of an undergraduate civil engineering program as preparation. If the applicant’s undergraduate degree is not in civil engineering, then some undergraduate prerequisite courses may be required. See the CEE Department Guidelines for additional information.CourseworkA student pursuing a M.S.E. degree in Structural Engineering must complete at least 30 credit hours of acceptable graduate work. (This usually corresponds to 10 courses.) A thesis is not required. In satisfying the credit hour requirement, the following requirements must be satisfied:∙At least 15 of the credit hours must be in Civil and Environmental Engineering (CEE) courses.∙At least 12 credit hours must correspond to courses within the Structures concentration area. Acceptable courses are listed below. However, no more than 21 credit hours from the courses listed below can be counted toward the MSE degree. Among the 12 credit hours required, at least 3 should be at the 600 level.CEE 510Finite Element Methods CEE 518Fiber-Reinforced Cement CompositesCEE 511Dynamics of Structures CEE 611Earthquake EngineeringCEE 512Theory of Structures CEE 613Metal Structural MembersCEE 513Plastic Analysis and Design of Frames CEE 614Advanced Prestressed ConcreteCEE 514Prestressed Concrete CEE 615Reinforced Concrete MembersCEE 515Advanced Design of R/C Structures CEE 617Random VibrationsCEE 516Bridge Structures CEE 619Adv. Struct. Dynamics and Smart Structures CEE 517Reliability of Structures CEE 910Structural Engineering ResearchCEE 519High-Perfor. Struct. Materials and Systems∙In addition to the minimum 12 credit hours of Structures courses, a student must complete 2 credit hours of the CEE 812 Structural Engineering Graduate Seminar. No more than 2 credit hours of CEE 812 will count towards the degree.∙ A student must satisfactorily complete at least two graduate level courses (cognate courses), with a minimum of 2 credit hours each, in a department other than Civil and Environmental Engineering. Cognate courses must be passed with a B- or better (see Rackham BULLETIN for more information). One of these cognate courses must be an advanced mathematics course. The list of courses on page 2 can be used as a guide to satisfy the cognate course requirement. Courses other than those listed should be approved by the student’s academic advisor in advance.Courses cross-listed with CEE courses do not qualify as cognates.∙No more than 6 credit hours of directed studies, seminars or research can be counted toward the 30-credit requirement. This covers credit hours received for CEE 910 and CEE 950.∙No more than 12 credit hours at the 400 level are acceptable. Of these 12 hours, a maximum of 9 hours can be in CEE courses. Structural engineering courses at the 400 level are not accepted for graduate credit unless approved in advance by the MSE graduate advisor in structural engineering.∙SGUS students are permitted to double count up to 9 credit hours, including CEE 413 and 415. However, students who double count 413 and 415 must take at least 3 structural engineering courses at the 500 level.∙ A maximum of 6 graduate level semester hours (with a grade of B or better) can be transferred from other institutions approved by Rackham.GradesThe grading system used for graduate studies is based on the following 9-point scale:A+ = 9; A = 8; A- = 7; B+ = 6; B = 5; B- = 4; C+ = 3; C = 2; C- = 1A minimum cumulative graduate grade point average (GPA) of 5 on this 9-point scale is required for all graduate courses taken for credit and applied toward the Master’s Degree.1 For additional information on M.S.E. degree requirements, see the Graduate Student Handbook (prepared by the Horace H. Rackham School of Graduate Studies) and the CEE Department Guidelines. The Graduate Student Handbook is available on the World Wide Web at /.To be considered for a master’s degree diploma, a student must submit a formal application to the Office of Graduate Academic Records of the Graduate School. The deadline for the Graduate School to receive the degree application form is four weeks after the first day of classes in a full term and one week after the first day of classes in a half term. These dates can usually be found on the Rackham Graduate School web site (/). Acceptable Cognate Courses for M.S.E. in Structural EngineeringShown below is a partial list of courses that can be used to satisfy the advanced math cognate course requirement for the CEE Department's M.S.E. degrees. In general, the math course should have a prerequisite of Math 215 or equivalent. Math 404 Intermediate Differential EquationsMath 412 Introduction to Modern Algebra BioStat 553 Applied BiostatisticsMath 416 Theory of AlgorithmsMath 417 Matrix Algebra IMath 419 Linear Spaces and Matrix TheoryIOE 510 Linear ProgrammingMath 433 Intro. to Differential GeometryMath 450 Adv. Math for Engineers IMath 451 Adv. Calculus IMath 454 Boundary Value Prob. for PDEMath 462 Mathematical ModelsMath 471 Intro. to Numerical MethodsMath 5XX Any 500 level math courseShown below is a partial list of courses that may be used to satisfy the second cognate course requirement for the CEE Department's M.S.E. degrees.ME 400 Mechanical Engineering Analysis Aero 416 Plates and ShellsME 401 Statistical Quality Control and Design Aero 513 Solid and Structural Mechanics IME 412 Advanced Strength of Materials Aero 514 Solid and Structural Mechanics IIME 515 Contact Mechanics Aero 516 Mechanics of CompositesME 501 Analytical Methods in Mechanics Aero 518 Theory of Elastic Stability IME 502 Methods of Diff. Eqns. In Mechanics Aero 565 Optimal Structural DesignME 511 Theory of Solid Continua Aero 611 Advanced Finite ElementsME 519 Theory of Plasticity IME 543 Analytical and Comp. Dynamics I MSE 514 Composite MaterialsME 555 Design OptimizationME 558 Discrete Design OptimizationME 563 Time Series ModelingME 564 Linear Systems TheoryME 605 Adv. Finite Element Methods in Mech.ME 619 Theory of Plasticity IIThere are many other courses in engineering, math, science, and architecture/urban planning that may satisfy the requirements for the non-math cognate course. (A cognate course must not be cross-listed with a CEE course, must be at the 400 level or higher, must be related to the field of specialization, and must be listed in the Rackham Bulletin. Cognate courses must be passed with a B- or better to count towards the degree.) Such courses must be approved for cognate credit in advance by the student’s academic advisor. Courses outside of engineering, math, science, and architecture/urban planning are generally not acceptable as cognate courses. Except as listed above, generally 400 level courses are not acceptable.Examples of courses accepted in the past: UP 538, UP 594, UP 565The checklist below can be used to monitor your progress toward your M.S.E. degree.Requirement Description CourseNumberCourse Description Credits Transfer1 Cognate–Math2 Cognate3 CEE(Concentration Area) 4 CEE(Concentration Area) 5 CEE(Concentration Area) 6 CEE(Concentration Area)600 level7 CEE8 OpenChoice9 OpenChoice10 NOT Structures andNOT Cognate11 CEE812(Graduate seminar) ExtraExtra。
NF EN 10028-1 - janv 2001 - Produits plats en acier pour appareils a pression - prescriptions genera

NF EN 10028-1Janvier 2001Ce document est à usage exclusif et non collectif des clients Normes en ligne.Toute mise en réseau, reproduction et rediffusion, sous quelque forme que ce soit,même partielle, sont strictement interdites.This document is intended for the exclusive and non collective use of AFNOR Webshop(Standards on line) customers. All network exploitation, reproduction and re-dissemination,even partial, whatever the form (hardcopy or other media), is strictly prohibited.Boutique AFNORPour : VICHEMCode client : 23412505Commande : N-20050120-095443-Tle 20/1/2005 - 15:25Diffusé par11992© AFNOR 2001AFNOR 20011er tirage 2001-01-F© A F N O R 2001 — T o u s d r o i t s r és e r v ésFA043128ISSN 0335-3931NF EN 10028-1Janvier 2001Indice de classement: A 36-205-1norme européenneProduits laminés pour appareils à pression BNS 10-10Membres de la commission de normalisationPrésident :M CRETONSecrétariat :BNSM BARRERE PUMM BOCQUET USINOR INDUSTEELMME BRUN-MAGUET AFNORM CRETON BNSM DE VEYRAC UGINE LA DEFENSEM DENOIX ETUDES ET PRODUCTIONS SCHLUMBERGERM DESPLACES SOLLAC MEDITERRANEE FOSM FINET USINOR INDUSTEELM FLANDRIN MINISTERE DE L’ECONOMIE, DES FINANCES ET DE L’INDSUTRIE — DARPMIM JARBOUI CETIMM LAGNEAUX MINISTERE DE L’ECONOMIE, DES FINANCES ET DE L’INDSUTRIE — DARPMIM LIEURADE CETIMM REGER EDF/SCF SQRM RIGAL GTS INDUSTRIESMME ROUMIER MINISTERE DE L'EQUIPEMENT, DES TRANSPORTS ET DU LOGEMENTM STAROPOLI GDFAvant-propos nationalRéférences aux normes françaisesLa correspondance entre les normes mentionnées à l'article «Références normatives» et les normes françaises identiques est la suivante :CR 10260:FD CR 10260 (indice de classement: A 02-005-3)EN 10002-1:NF EN 10002-1 (indice de classement: A 03-001)EN 10002-5:NF EN 10002-5 (indice de classement: A 03-005)EN 10020:NF EN 10020 (indice de classement: A 02-025)EN 10021:NF EN 10021 (indice de classement: A 00-100)EN 10027-1:NF EN 10027-1 (indice de classement: A 02-005-1)EN 10027-2:NF EN 10027-2 (indice de classement: A 02-005-2)EN 10028-7:NF EN 10028-7 (indice de classement: A 36-205-7)EN 10029:NF EN 10029 (indice de classement: A 46-503)EN 10045-1:NF EN 10045-1 (indice de classement: A 03-011)EN 10048:NF EN 10048 (indice de classement: A 46-101)—3—NF EN 10028-1:2001EN 10051:NF EN 10051 (indice de classement: A 46-501)EN 10052:NF EN 10052 (indice de classement: A 02-010)EN 10079:NF EN 10079 (indice de classement: A 40-001)EN 10088-1:NF EN 10088-1 (indice de classement: A 35-572)EN 10160:NF EN 10160 (indice de classement: A 04-305)EN 10163-2:NF EN 10163-2 (indice de classement: A 40-501-2)EN 10164:NF EN 10164 (indice de classement: A 36-202)EN 10168:NF EN 10168 (indice de classement: A 03-116) 1)EN 10204:NF EN 10204 (indice de classement: A 00-001)EN 10258:NF EN 10258 (indice de classement: A 46-110-1)EN 10259:NF EN 10259 (indice de classement: A 46-110-2)EN ISO 377:NF EN ISO 377 (indice de classement: A 03-112)EN ISO 2566-1:NF EN ISO 2566-1 (indice de classement: A 03-174)EN ISO 2566-2:NF EN ISO 2566-2 (indice de classement: A 03-175)EN ISO 3651-2:NF EN ISO 3651-2 (indice de classement: A 05-159)ISO 14284:NF EN ISO 14284 (indice de classement: A 06-002) 1)Modalités d’applicationLe fabricant, l’importateur ou le fournisseur qui, pour la vente de ses produits, se réfère au présent document ou à un texte qui fait référence à certains de ses articles, doit être en mesure de fournir à son client les éléments propres à justifier que les prescriptions normatives sont respectées.L’attribution de la marque NF aux produits conformes au présent document offre la garantie que ces éléments sont contrôlés sous l’égide d’AFNOR (certification par tierce partie).1)En préparation.NORME EUROPÉENNE EUROPÄISCHE NORM EUROPEAN STANDARDEN 10028-1Avril 2000La présente norme européenne a été adoptée par le CEN le 29 octobre 1999.Les membres du CEN sont tenus de se soumettre au Règlement Intérieur du CEN/CENELEC qui définit les conditions dans lesquelles doit être attribué, sans modification, le statut de norme nationale à la norme européenne.Les listes mises à jour et les références bibliographiques relatives à ces normes nationales peuvent être obtenues auprès du Secrétariat Central ou auprès des membres du CEN.La présente norme européenne existe en trois versions officielles (allemand, anglais, français). Une version faite dans une autre langue par traduction sous la responsabilité d'un membre du CEN dans sa langue nationale, et notifiée au Secrétariat Central, a le même statut que les versions officielles.Les membres du CEN sont les organismes nationaux de normalisation des pays suivants : Allemagne, Autriche,Belgique, Danemark, Espagne, Finlande, France, Grèce, Irlande, Islande, Italie, Luxembourg, Norvège, Pays-Bas, Portugal, République Tchèque, Royaume-Uni, Suède et Suisse.CENCOMITÉ EUROPÉEN DE NORMALISATION Europäisches Komitee für Normung European Committee for StandardizationSecrétariat Central : rue de Stassart 36, B-1050 Bruxelles© CEN 2000Tous droits d’exploitation sous quelque forme et de quelque manière que ce soit réservés dans le monde entier aux membres nationaux du CEN.Réf. n°EN 10028-1:2000 FICS :77.140.30; 77.140.50Remplace EN 10028-1:1992Version françaiseProduits plats en aciers pour appareils à pression —Partie 1: Prescriptions généralesFlacherzeugnisse aus Druckbehälterstählen —Teil 1: Allgemeine AnforderungenFlat products made of steels for pressure purposes —Part 1: General requirementsPage2EN 10028-1:2000SommairePage Avant-propos (3)1Domaine d’application (4)2Références normatives (4)3Définitions (5)4Dimensions et tolérances dimensionnelles (6)5Calcul de la masse (6)6Classification et désignation (6)6.1Classification (6)6.2Désignation (6)7Informations à fournir par l'acheteur (7)7.1Informations obligatoires (7)7.2Options (7)8Exigences (7)8.1Procédé d'élaboration (7)8.2État de livraison (7)8.3Composition chimique (8)8.4Caractéristiques mécaniques (8)8.5État de surface (8)8.6•• Santé interne (8)9Contrôles (8)9.1Type et contenu des documents de contrôle (8)9.2Contrôles à effectuer (9)9.3Contre-essais (9)10Échantillonnage (9)10.1Fréquence des essais (9)10.2Prélèvement et préparation des échantillons et des éprouvettes (10)11Méthodes d'essai (11)11.1•• Analyse chimique (11)11.2Essai de traction à température ambiante (11)11.3Essai de traction à température élevée (11)11.4Essai de flexion par choc (11)11.5Autres essais (12)12Marquage (12)Annexe ZA (informative) Articles de la présente norme européenneconcernant les exigences essentielles ou d'autres dispositions des Directives UE (17)Page3EN 10028-1:2000Avant-proposLa présente norme a été élaborée par le Comité Technique ECISS/TC22 «Aciers pour appareils soumis àpression — Prescriptions de qualité».La présente norme européenne remplace l’EN10028-1:1992 et prend en considération les normes complémen-taires de la série EN 10028.Les autres parties de la présente norme européenne sont:EN 10028-2, Produits plats en acier pour appareils à pression — Partie 2 : Aciers non alliés et alliés avec carac-téristiques spécifiées à température élevée.EN 10028-3, Produits plats en acier pour appareils à pression — Partie 3 : Aciers soudables à grains fins normalisés.EN 10028-4, Produits plats en acier pour appareils à pression — Partie 4 : Aciers alliés au nickel avec caractéris-tiques spécifiées à basse température.EN 10028-5, Produits plats en acier pour appareils à pression — Partie 5 : Aciers soudables à grains fins, laminés thermomécaniquement.EN 10028-6, Produits plats en acier pour appareils à pression — Partie 6 : Aciers soudables à grains fins, trempés et revenus.EN 10028-7, Produits plats en acier pour appareils à pression — Partie 7 : Aciers inoxydables.Cette norme européenne devra recevoir le statut de norme nationale, soit par publication d'un texte identique, soit par entérinement, au plus tard en octobre 2000 et toutes les normes nationales en contradiction devront être reti-rées au plus tard en octobre 2000.La présente norme européenne a été élaborée dans le cadre d'un mandat donné au CEN par la Commission Européenne et l'Association Européenne de Libre Échange, et vient à l'appui des exigences essentielles de la (de) Directive(s) UE.Pour la relation avec la (les) Directive(s) UE, voir l'annexe ZA informative, qui fait partie intégrante de la présente norme.Selon le Règlement Intérieur du CEN/CENELEC, les instituts de normalisation nationaux des pays suivants sont tenus de mettre le présent document en application : Allemagne, Autriche, Belgique, Danemark, Espagne, Fin-lande, France, Grèce, Irlande, Islande, Italie, Luxembourg, Norvège, Pays-Bas, Portugal, République Tchèque, Royaume-Uni, Suède et Suisse.NOTE Dans les paragraphes marqués d'un point (•) se trouvent des informations relatives aux accords qui doivent être conclus lors de l'appel d'offres et de la commande. Les paragraphes marqués de deux points (••) contiennent des informa-tions relatives aux accords qui doivent être conclus lors de l'appel d'offres et de la commande.Page4EN 10028-1:20001Domaine d’applicationLa présente norme européenne EN 10028-1 spécifie les conditions techniques générales de livraison des produits plats utilisés principalement dans la construction des appareils à pression.Les conditions techniques générales de livraison de l'EN 10021 s'appliquent également aux produits livrés conformément à la présente norme européenne.2Références normativesCette norme européenne comporte par référence datée ou non datée des dispositions d'autres publications. Ces références normatives sont citées aux endroits appropriés dans le texte et les publications sont énumérées ci-après. Pour les références datées, les amendements ou révisions ultérieurs de l'une quelconque de ces publica-tions ne s'appliquent à cette norme que s'ils y ont été incorporés par amendement ou révision. Pour les références non datées, la dernière édition de la publication à laquelle il est fait référence s'applique.CR 10260, Système de désignation des aciers — Symboles additionnels pour la désignation des aciers (Rapport CEN).EN 10002-1, Matériaux métalliques — Essai de traction — Partie 1 : Méthode d'essai (à température ambiante). EN 10002-5, Matériaux métalliques — Essai de traction — Partie 5 : Méthode d'essai à température élevée.EN 10020, Définition et classification des nuances d'acier.EN 10021, Aciers et produits sidérurgiques — Conditions générales techniques de livraison.EN 10027-1, Système de désignation des aciers — Partie 1 : Désignations symboliques, symboles principaux. EN 10027-2, Système de désignation des aciers — Partie 2 : Système numérique.EN 10028-7, Produits plats en acier pour appareils à pression — Partie 7 : Aciers inoxydables.EN 10029, Tôles en acier laminées à chaud d'épaisseur égale ou supérieure à 3 mm — Tolérances sur les dimen-sions, la forme et la masse.EN 10045-1, Matériaux métalliques — Essai de flexion par choc sur éprouvettes Charpy — Partie 1 : Méthode d'essai.EN 10048, Feuillards laminés à chaud — Tolérances de dimensions et de forme.EN 10051, Tôles, larges bandes et larges bandes refendues laminées à chaud en continu en aciers alliés et non alliés — Tolérances sur les dimensions, la forme et la masse.EN 10052, Vocabulaire du traitement thermique des produits ferreux.EN 10079, Définition des produits en acier.EN 10088-1, Aciers inoxydables — Partie 1 : Liste des aciers inoxydables.EN 10160, Contrôle ultrasonore des produits plats en acier d'épaisseur égale ou supérieure à 6 mm (méthode par réflection).EN 10163-2, Conditions de livraison relatives à l'état de surface des produits en acier laminés à chaud — Partie2: Tôles et larges plats.EN 10164, Aciers de construction à caractéristiques de déformation améliorées dans le sens perpendiculaire à la surface du produit — Conditions techniques de livraison.Page5EN 10028-1:2000 EN 10168 1), Produits sidérurgiques — Contenu des documents de contrôle — Liste et description des informations.EN 10204, Produits métalliques — Types de documents de contrôle (y compris amendement A1:1995).EN 10258, Feuillards ou feuillards coupés à longueur en acier inoxydable laminés à froid — Tolérances sur les dimensions et la forme.EN 10259, Larges bandes et tôles en acier inoxydable laminées à froid — Tolérances sur les dimensions et la forme.EN ISO 377, Aciers et produits en acier — Position et préparation des échantillons et éprouvettes pour essais mécaniques (ISO 377:1997).EN ISO 3651-2, Détermination de la résistance à la corrosion intergranulaire des aciers inoxydables — Partie 2 : Aciers ferritiques, austénitiques et austéno-ferritiques (duplex) — Essais de corrosion en milieux contenant de l'acide sulfurique (ISO 3651-2:1998).EN ISO 2566-1, Conversion des valeurs d'allongement — Partie 1 : Aciers non alliés et faiblement alliés (ISO2566-1:1984).EN ISO 2566-2, Conversion des valeurs d'allongement — Partie 2 : Aciers austénitiques (ISO 2566-2:1984). ISO 14284, Fontes et aciers — Prélèvement et préparation des échantillons pour la détermination de la composi-tion chimique.3DéfinitionsPour les besoins de la présente norme européenne, les définitions des normes :—EN 10020, pour la classification des aciers ;—EN 10079, pour les formes des produits ; et—EN 10052, pour les types de traitement thermique,s'appliquent.La définition 3.1 est différente de celle de l'EN 10052, et la définition 3.2 s'y ajoute.3.1laminage normalisantprocédé de laminage dans lequel la déformation finale est effectuée dans une certaine gamme de températures conduisant à un état du matériau équivalent à celui obtenu après normalisation, de sorte que les valeurs spécifiées de caractéristiques mécaniques sont maintenues même après un traitement de normalisation. La désignation de cet état de livraison et de celui obtenu par un traitement thermique de normalisation est N3.2en plus des définitions relatives au traitement thermomécanique, à la trempe et au revenu, il convient de noter les points suivants :NOTE 1Le laminage thermomécanique (symbole M) peut inclure des procédés d'accélération des vitesses de refroidis-sement avec ou sans revenu y compris auto-revenu mais à l'exclusion totale de la trempe directe suivie d'un revenu.NOTE 2Le traitement de trempe et revenu (symbole QT) englobe également le durcissement par trempe directe suivi d'un revenu.1)En cours d’élaboration; en attendant la publication de ce document sous le statut de Norme européenne, il ya lieu de convenir au moment de l’appel d’offres et de la commande d’une norme nationale correspondante.3.3acheteurpersonne ou organisation qui commande des produits selon la présente norme. L’acheteur n’est pas nécessaire-ment, mais peut être, un fabricant d’équipements sous pression selon la Directive UE listée en annexe ZA. Lorsqu'un acheteur a des responsabilités selon cette Directive UE, la présente norme européenne donnera pré-somption de conformité aux Exigences Essentielles de la Directive identifiée dans l’annexe ZA4Dimensions et tolérances dimensionnelles• Les dimensions nominales et tolérances sur les dimensions des produits doivent faire l'objet d'un accord lors de l'appel d'offres et de la commande sur la base des normes dimensionnelles indiquées ci-dessous :4.1Pour les tôles en acier laminées à chaud, en non-continu, se référer à l'EN 10029.•• Sauf accord contraire lors de l'appel d'offres et à la commande, la tolérance d'épaisseur des tôles doit corres-pondre à la classe B de l'EN 10029.4.2Pour les bobines laminées à chaud en continu et les tôles découpées à partir de bobines (laminées en largeur≥600mm) et les bobines refendues laminées à chaud en largeur < 600 mm, se référer à l'EN10051. 4.3Pour les feuillards laminés à chaud (laminés en largeur < 600 mm), se référer à l'EN10048.4.4Pour les tôles et bandes laminées à froid, les bobines, et les bobines refendues laminées à froid (en largeur≥ 600 mm) en aciers inoxydables, se référer à l’EN 10259, et pour les bobines et les bobines refendues laminées à froid en largeur < 600 mm en aciers inoxydables, se référer à l'EN10258.NOTE L'EN 10258 et l'EN 10259 comportent des options qui offrent des possibilités dimensionnelles plus larges.5Calcul de la masseLe calcul de la masse nominale dérivée des dimensions nominales doit être effectué, pour tous les aciers des EN10028 parties 2 à 6, sur la base d'une masse volumique de 7,85 kg/dm3. Pour les masses volumiques des aciers résistant à la corrosion, voir l'annexe A de l'EN10088-1. Pour la masse volumique des aciers résistant au fluage, voir l'annexe A de l'EN10028-7.6Classification et désignation6.1Classification6.1.1La classification des nuances d'acier conformément à l'EN 10020 est donnée dans les parties spécifiques de l'EN 10028.6.1.2Les aciers traités dans l'EN 10028-7 sont, en outre, classés en fonction de leur structure en :—aciers ferritiques ;—aciers martensitiques ;—aciers austénitiques ;—aciers austéno-ferritiques.NOTE Pour plus de détails, voir l'EN 10088-1.6.2DésignationLes nuances d'acier spécifiées dans les diverses parties de l'EN 10028 sont désignées par des désignations sym-boliques et numériques. Les désignations symboliques ont été attribuées conformément à l'EN 10027-1 et au CR10260. Les désignations numériques correspondantes ont été attribuées conformément à l'EN 10027-2.7Informations à fournir par l'acheteur7.1Informations obligatoiresLes informations suivantes doivent être fournies par l'acheteur au moment de l'appel d'offres et de la commande :a)la quantité désirée ;b)le type de produit plat ;c)la norme européenne spécifiant les tolérances de dimensions, de forme et de masse (voir article 4) et, si lanorme européenne en question laisse au client le choix entre plusieurs possibilités, par exemple diverses fini-tions de rives ou diverses classes de tolérances, des informations détaillées relatives à ces spécificités ;d)les dimensions nominales du produit ;e)l'indice de la présente norme européenne ;f)la désignation symbolique ou numérique de la nuance d'acier ;g)l'état de livraison s'il diffère de l'état habituel spécifié dans la partie spécifique de l'EN10028 ; pour les aciersinoxydables, la gamme de fabrication choisie dans le tableau concerné de l'EN10028-7 ;h)le document de contrôle à produire (voir 9.1.1).7.2OptionsUn certain nombre d'options sont spécifiées dans la présente partie de l'EN 10028 et données dans la liste ci-dessous. Si l'acheteur n'indique pas les options qu'il souhaite voir mises en œuvre au moment de l'appel d'offres et de la commande, les produits doivent être fournis conformément aux spécifications de base (voir 7.1) :a)classe de tolérance différente (voir 4.1) ;b)spécification relative au procédé d'élaboration de l'acier (voir 8.1.1) ;c)caractéristiques mécaniques après traitement thermique supplémentaire (voir 8.4.1) ;d)spécifications de classes particulières pour le coefficient de striction (voir 8.4.2) ;e)essais supplémentaires (voir 9.2.2) ;f)fréquence d'essais différente (voir 10.1.1 et 10.1.3) ;g)conditions de livraison différentes (voir 10.2.1.3) ;h)utilisation d'éprouvettes longitudinales pour l'essai de flexion par choc (voir 10.2.2.3) ;i)spécification d'une méthode d'analyse (voir 11.1) ;j)température de l'essai de traction à température élevée (voir 11.3) ;k)température d'essai différente pour l'essai de flexion par choc (voir 11.4) ;l)méthode de marquage (voir 12.1) ;m)marquage spécial (voir 12.2 et 12.3) ;n)informations devant être données par le marquage (voir tableau 1).8Exigences8.1Procédé d'élaboration8.1.1•• Sauf si un procédé d'élaboration particulier a fait l'objet d'un accord lors de l'appel d'offres et de la commande, le procédé d'élaboration des aciers de la présente norme européenne doit être laissé au choix du producteur.8.1.2Les aciers autres que les aciers inoxydables doivent être totalement calmés.8.2État de livraisonVoir les parties spécifiques de l'EN 10028 (voir aussi 3.1 et 3.2).8.3Composition chimique8.3.1Analyse de couléeL'analyse de coulée donnée par le producteur de l'acier doit s'appliquer et satisfaire aux exigences des parties spécifiques de l'EN 10028.8.3.2Analyse sur produitLes écarts admissibles de l'analyse sur produit par rapport aux valeurs limites données pour l'analyse de coulée sont spécifiés dans les parties concernées de l'EN 10028.8.4Caractéristiques mécaniques8.4.1Les valeurs données dans les parties spécifiques de l'EN 10028 s'appliquent pour les éprouvettes préle-vées et préparées selon les indications du 10.2.2. Ces valeurs ont été obtenues sur des produits d'épaisseur nomi-nale (épaisseurs commandées) et à l'état normal de livraison (voir parties spécifiques de l'EN 10028).•• Le cas échéant, les valeurs des caractéristiques mécaniques garanties après traitement thermique complé-mentaire doivent faire l'objet d'un accord au moment de l'appel d'offres et de la commande.8.4.2•• Pour les produits (excepté ceux en acier inoxydable) d'épaisseur ≥ 15 mm, il peut être convenu, lors de l'appel d'offres et de la commande, de respecter les exigences fixées pour l'une des classes de qualité Z15, Z 25 ou Z 35 de l'EN 10164 caractérisées par des valeurs minimales de striction dans le sens de l'épaisseur. 8.5État de surfacePour les tôles, les exigences de qualité de surface spécifiées dans l'EN10163-2 doivent être appliquées de la manière suivante :a)pour les tôles conformes à l'EN 10028-2 à -6 : classe B2;b)pour les tôles conformes à l'EN 10028-7 : classe B3.8.6•• Santé interneLes exigences relatives à la santé interne ainsi que les conditions de sa vérification (voir 7.2.e et 11.5.3) peuvent, le cas échéant, être spécifiées au moment de l'appel d'offres et de la commande.9Contrôles9.1Type et contenu des documents de contrôle9.1.1• La conformité aux exigences de la commande des produits livrés, conformément à la présente norme européenne, doit faire l'objet d'un contrôle spécifique.L'acheteur doit préciser le type de document de contrôle souhaité (3.1.A, 3.1.B, 3.1.C ou 3.2) conformément àl'EN10204. Si un document de contrôle 3.1.A, 3.1.C ou 3.2 est commandé, l'acheteur doit notifier au producteur le nom et l'adresse de l'organisme ou de la personne chargée d'effectuer le contrôle et de produire le document correspondant. S'il s'agit d'un procès-verbal de réception 3.2, on doit convenir de la partie chargée de publier le certificat.9.1.2Le document de contrôle doit contenir, conformément à l'EN 10168, les codes et les informations suivants :a)les groupes d'informations A, B et Z ; la température de revenu doit également être donnée dans le cas desproduits trempés et revenus ;b)les résultats de l'analyse de coulée, conformément aux rubriques C 71 à C 92 ;c)les résultats des essais de traction à température ambiante, conformément aux rubriques C00 à C03 et C10à C13 ;d)les résultats de l'essai de flexion par choc sauf pour les aciers austénitiques de l'EN10028-7, conformémentaux rubriques C00 à C03 et C40 à C43 ;e)les résultats du contrôle visuel des produits (voir groupe d'informations D) ;f)si une ou plusieurs des options suivantes ont fait l'objet d'un accord lors de l'appel d'offres et de la commande,les informations correspondantes :1)le procédé d'élaboration de l'acier (rubrique C 70) ;2)l'analyse sur produit conformément aux rubriques C 71 à C 92 ;3)les résultats de l'essai de traction à température élevée (voir 9.2.2), conformément aux rubriques C 00àC03, C 10 et C 11 ;4)la valeur minimale de striction dans le sens de l'épaisseur, conformément aux rubriques C 00 à C 03, C 10et C 14 à C 29 ;5)le contrôle de santé interne par ultrasons (groupe d'informations F) ;6)les caractéristiques de flexion par choc des aciers austénitiques à température ambiante, conformémentaux rubriques C 00 à C 03 et C 40 à C 43 ;7)vérification des caractéristiques de flexion par choc des aciers inoxydables à basse température, confor-mément aux rubriques C 00 à C 03 et C 40 à C 43 ;8)résistance à la corrosion intergranulaire des aciers inoxydables, conformément aux rubriques C 60 à C69.9.2Contrôles à effectuer9.2.1Les contrôles suivants doivent être effectués :—essai de traction à température ambiante ;—essai de flexion par choc (sauf pour les aciers austénitiques de l'EN 10028-7), mais voir 10.2.2.3 ;—contrôle dimensionnel ;—examen visuel de l'état de surface.9.2.2•• Les essais suivants peuvent faire l'objet d'un accord :—analyse sur produit ;—essai de traction pour vérifier la limite d'élasticité conventionnelle à 0,2 % à température élevée (sauf pour les aciers des EN10028-4 et EN 10028-5) ;—essai de traction pour vérifier simultanément à température élevée pour les aciers austénitiques de l'EN10028-7 un, plusieurs ou l’ensemble des paramètres suivants: R p0,2, R p1,0 et R m ;—essai de traction dans le sens de l'épaisseur (sauf pour les aciers de l'EN10028-7) ;—essais de flexion par choc à température ambiante des aciers austénitiques de l'EN10028-7;—essais de flexion par choc à basse température des aciers de l'EN 10028-7 (sauf pour les aciers ferritiques) ;—contrôle aux ultrasons pour vérifier la santé interne ;—détermination de la résistance à la corrosion intergranulaire des aciers de l'EN 10028-7.9.3Contre-essaisVoir l'EN 10021.10Échantillonnage10.1Fréquence des essais10.1.1•• Pour l'analyse sur produit et sauf accord contraire, une seule éprouvette par coulée doit être prélevée pour déterminer, pour la nuance considérée, la teneur des éléments indiqués avec des valeurs numériques dans les divers tableaux des parties spécifiques de l'EN 10028.。
2012年英语专业四级考试真题及答案
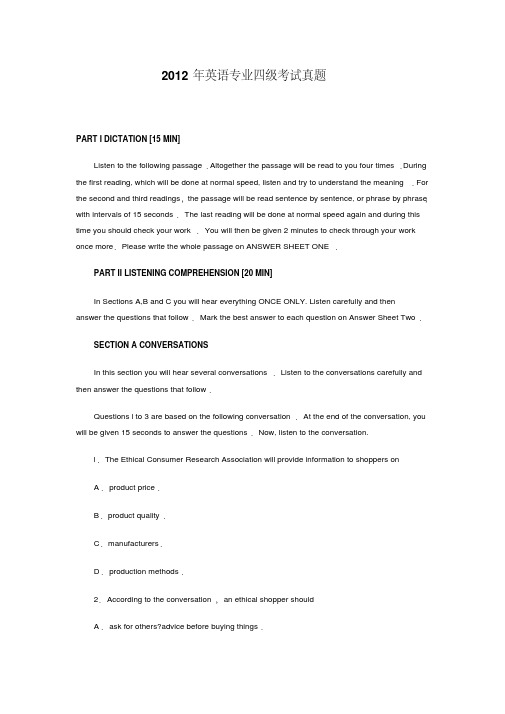
2012年英语专业四级考试真题PART I DICTATION [15 MIN]Listen to the following passage.Altogether the passage will be read to you four times.During the first reading, which will be done at normal speed, listen and try to understand the meaning.For the second and third readings,the passage will be read sentence by sentence, or phrase by phrase,with intervals of 15 seconds.The last reading will be done at normal speed again and during this time you should check your work.You will then be given 2 minutes to check through your work once more.Please write the whole passage on ANSWER SHEET ONE.PART II LISTENING COMPREHENSION [20 MIN]In Sections A,B and C you will hear everything ONCE ONLY. Listen carefully and thenanswer the questions that follow.Mark the best answer to each question on Answer Sheet Two.SECTION A CONVERSATIONSIn this section you will hear several conversations.Listen to the conversations carefully and then answer the questions that follow.Questions l to 3 are based on the following conversation.At the end of the conversation, you will be given 15 seconds to answer the questions.Now, listen to the conversation.l.The Ethical Consumer Research Association will provide information to shoppers onA.product price.B.product quality.C.manufacturers.D.production methods.2.According to the conversation,an ethical shopper shouldA.ask for others?advice before buying things.B.consider the worth of something to be bought.C.postpone buying things whenever possible.D.search for things that are less costly.3.According to the conversation,ethical shoppers can be best described asA.shrewd.B.thrifty.C.extravagant.D.cautious.Questions 4 to 7 are based on the following conversation.At the end of the conversation,you will be given 20 seconds to answer the questions.Now,listen to the conversation.4.Which of the following statements is CORRECT about Mary?A.She is enjoying her language study.B.She is enjoying her management study.C.She is not feeling very well at the moment.D.She is not happy about her study pressure.5.What does Mary think of the course initially?A.It is useful.B.It is difficult.C.It is challenging.D.It is interesting.6.What is Mary?s problem of living in a family house?A.She dislikes the food she eats.B.She is unable to sleep well.C.She has no chance to make friends.D.She finds the rent high.7.Which of the following is Mr.Davies?advice?A.To tryto make more friends.B.To try to change accommodation.C.To spend more time on English.D.To stop attending language classes.Questions 8 to 10 are based on the following conversation.At the end of the conversation,you will be given 15 seconds to answer the questions.Now,listen to the conversation.8.According to the conversation,the day is special becauseA.many people are surfing the net on that day.B.it is an anniversary of the internet.C.the net brought about no changes until that day.D.big changes will take place on that day.9.We learn from the conversation that peopleA.cannot Jive without the internet.B.cannot work without the internet.C.all use the internet to keep in touch.D.have varied opinions about internet use.10.At the end of the conversation.the speakers talk aboutA.the future of the internet.B.the type of office furniture.C.when changes will come.D.how people will use the internet.SECTION B PASSAGESIn this section,you will hear several passages.Listen to the passages carefully and then answer the questions that follow.Questions 11 to 13 are based on the following passage.At the end of the passage, you will be given 15 seconds to answer the questions.Now, listen to the passage.11.In order to open a bank account,you need to produce____in addition to your passport.A.a library card B.a registration formC.a telephone bill D.a receipt12.Which of the following might NOT be included in the,utility bill??A.Rent.B.Gas.C.Water.D.Telephone.13.According to the passage,what can one do in the post office?A.Getting contact details.B.Obtaining tax forms.C.Paying housing rents.D.Applying for loans.Questions 14 to 17 are based on the following passage.At the end of the passage, you will be given 20 seconds to answer the questions.Now, listen to the passage.14.According to the passage,,scheduling?means that youA.need to be efficient in work.B.plan your work properly.C.try to finish work ahead of time.D.know how to work in teams.15.According to the passage, one of the activities to relax could beA.protecting wild animals.B.spending time with your family.C.learning how to read efficiently.D.learning how to do gardening.16.One of the ways to reduce stress is toA.do better than anyone else.B.fulfill high ambitions in one's work.C.work and have reasonable aims.D.start with a relatively low aim.17.According to the passage,to reduce stress has something to do with the following EXCEPTA.one's position.B.one's interest.C.one's health.D.one's mood.Questions 18 to 20 are based on the following passage.At the end of the passage, you will be given 15 seconds to answer the questions.Now, listen to the passage.18.According to the passage,new words tend to come fromA.world politics.B.advances in science.C.areas of life.D.all the above.19.The passage explains the larger and richer vocabulary of English mainly from a viewpoint.A.historical B.culturalC.commercial D.colonial20.According to the passage,which of the following statements best describes the English language?A.It is outdated in grammar.B.It accepts new words from science.C.It has begun taking in new words.D.It tends to embrace new words.SECTION C NEWS BROADCASTIn this section,you will hear several news items.Listen to them carefully and then answer the questions that follow.Questions 21 and 22 are based OH the following news.At the end of the news item,you will be given 10 seconds to answer the questions.Now listen to the news.21.Where was the marble statue found?A.Out in the sea.B.Inside a bath house.C.On a cliff along the coast.D.On the coast outside Jerusalem.22.Which of the following best describes the condition of the statue?A.It was incomplete.B.It was recent artwork.C.It was fairly tall.D.It was in pieces.Questions 23 and 24 are based on the following news.At the end of the news item.you will be given 10 seconds to answer the questions.Now, listen to the news.23.The rescue efforts concentrated mainly onA.the U.S.-Canada border B.snow-stricken regions.C.highways.D.city streets.24.According to the news,the last group of people might have been stranded in their vehiclesfor more than ____ hours before being rescued.A.24 B.25 C.40 D.48Questions 25 and 26 are based on the following news.At the end of the news item, you will be given 10 seconds to answer the questions.Now, listen to the news.25.According to the 2006 anti-smoking restrictions,smoking was NOT allowed inA.offices.B.restaurants.C.bars.D.school playgrounds.26.According to the news,which of the following groups reacts negatively to the new law?A.Television producers.B.Hotel owners.C.Medical workers.D.Hospital management.Questions 27 and 28 are based on the following news.At the end of the news item,you will be given 10 seconds to answer the questions.Now, listen to the news.27.According to the news,who first discovered the fraud?A.A client.B.A bank manager.C.The police.D.Bank headquarters.28.When did the bank employee hand himself in?A.A month before the fraud was discovered.B.A day before the fraud was discovered.C.A day after the police launched investigation.D.A month after he transferred the money.Question 29 is based on the following news.At the end of the news item, you will be given 5 seconds to answer the question.Now, listen to the news.29.What is this news item mainly about?A.How to open Hotmail accounts.B.How to retrieve missing e-mails.C.New e-mail service by Microsoft.D.Problems and complaints about e-mails.Question30 is based on the following news.At the end of the news item, you will be given 5 seconds to answer the question.Now, listen to the news.30.Compared with 2009,which of the following figures remained about the same in 2010?A.Number of tickets sold.B.Box office revenues.C.Attendance rate. D Number of cinemasPART III CLOZE 【15 MIN】Decide which of the choices given below would best complete the passage if inserted in the corresponding blanks.Mark the best choice for each blank on Answer Sheet Two.The earthquake of 26th December 2004 resulted in one of the worst natural disasters in living memory.It was a (31) _____ underwater quake and occurred in the Indian Ocean.It (32) ____ coastlines,communities and brought death to many people.Why do earthquakes happen?The surface of the earth has not always looked as it does today;it is moving(33)____ (although very slowly)and has done so for billions of years.This is one(34)____ of earthquakes,when one section of the earth (tectonic plate)(35)____ another.Scientists can predict where but not(36)____ this might happen and the area between plates is called a fault line.On one fault line in Kobe,Japan in 1923 over 200,000 people were killed.(37)____,earthquakes do not alwayshappen on fault lines,(38)____ is why they are so dangerous and (39)____.Where do volcanoes happen?Volcanoes happen where the earth's(40)____ is thin:lava,dust and gases(41)____ from beneath the earth.They can rise into a huge cone shape like a mountain and erupt,(42)____ they can be so violent(43)____ they just explode directly from the earth with no warning.There are 1511(44)'____' volcanoes in the world.This means that they may(45)____ be dangerous.In 1985 the Colombian volcano Nevado del Ruiz erupted.The lava melted a glacier and sent tones ofmud(46)____ the town below.Twenty thousand people died.Natural disasters like volcanic eruptions are often unpredictable.We regularly do not know when they(47)____ pen,or (48)____ where they will happen.In the future,scientists may be able to watch and predict(49)____ before they happen.This could(50)____ many lives.31.A.massive B.significant C.great D.grand32.A.changed B.converted C.destroyed D.transformed33.A.frequently B.continuously C.regularly D.periodically34.A.source B.reason C.movement D.cause35.A.collides with B.confronts with C.meets with D.faces with36.A.how B.why C.when D.what37.A.Generally B.However C.Similarly D.Anyway38.A.that B.it C.this D.which39.A.unpredictable B.unaccountable C.inevitable D.irresistible40.A.surface B.appearance C.crust D.cover41.A.flowed out B.burst out C.1eaked out D.trickled out42.A.or B.and C.nor D.but43.A.like B.for C.as D.that44.A.living B.active C.alive D.live45.A.relatively B.hardly C.still D.gradually46.A.down B.on C.across D.beyond47.A.are to B.should C.must D.might48.A.else B.even C.though D.whether49.A.accidents B.incidents C.occasions D.events50.A.rescue B.save C.preserve D.shelterPART IV GRAMMAR &VOCABULARY 【15 MIN】There are thirty sentences in this section.Beneath each sentence there are four words, phrases or statements marked A,B, C and D.Choose one word, phrase or statement that best completes the sentence.Mark your answers on Answer Sheet Two.51.Which of the following sentences is INCORRECT?A.Twenty miles seems like a long walk to him.B.No one except his supporters agree with him.C.Neither Julia nor I were going to the party.D.Few students in my class are really lazy.52.Which of the following determiners(限定词)can be placed before both singular count nouns and plural count nouns?A.many a B.few C.such D.the next53.Which of the following reflexive pronouns(反身代词)is used as an appositive(同位语)?A,He promised himself rapid progress.B.The manager herself will interview Mary.C.I have nothing to say for myself.D.They quarreled themselves red in the face.54.My boss ordered that the legal documents ____ to him before lunch.A.be sent B.were sent C.were to be sent D.must be sent55.Which of the following sentences expresses WILLINGNESS?A.By now she will be eating dinner.B.I shall never do that again.C.My brother will help you with the luggage.D.You shall get a promotion.56.Which of the following sentences is INCORRECT? A.How strange feelings they are!B.How dare you speak to me like that!C.What noise they are making!D. What a mess we are in!57.which of the italicized parts functions as a subject?A.We never doubt that her brother is honest.B.The problem is not who will go but who will stay.C.You must give it back to whoever it belongs to。
Lecture 1-8(中英)
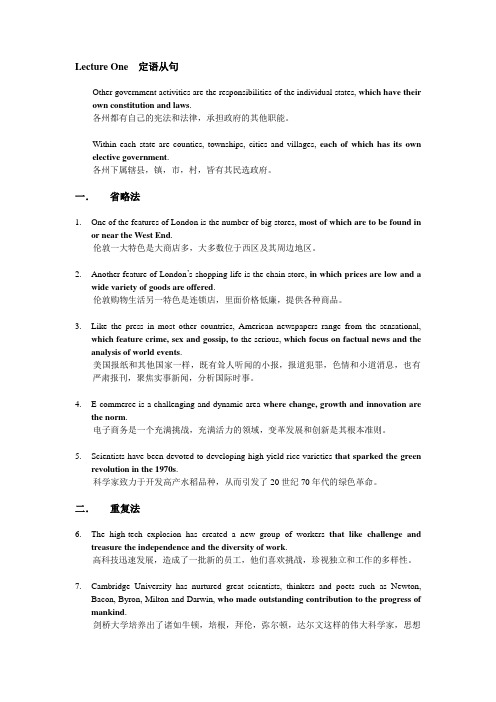
Lecture One 定语从句Other government activities are the responsibilities of the individual states, which have their own constitution and laws.各州都有自己的宪法和法律,承担政府的其他职能。
Within each state are counties, townships, cities and villages, each of which has its own elective government.各州下属辖县,镇,市,村,皆有其民选政府。
一.省略法1.One of the features of London is the number of big stores, most of which are to be found inor near the West End.伦敦一大特色是大商店多,大多数位于西区及其周边地区。
2.Another feature of London’s shopping life is the chain store, in which prices are low and awide variety of goods are offered.伦敦购物生活另一特色是连锁店,里面价格低廉,提供各种商品。
3.Like the press in most other countries, American newspapers range from the sensational,which feature crime, sex and gossip, to the serious, which focus on factual news and the analysis of world events.美国报纸和其他国家一样,既有耸人听闻的小报,报道犯罪,色情和小道消息,也有严肃报刊,聚焦实事新闻,分析国际时事。
lecture08
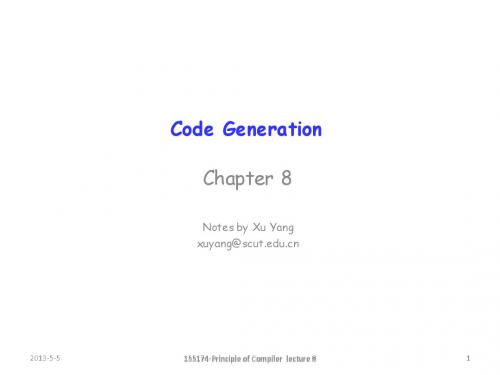
4
Code generation Steps • Code generation is typically broken into several steps
1) Intermediate code generation 2) Generate some form of assembly code instead of actual executable code 3) Optimization To improve the speed and size of the target code
2010-12-13
Three-address code for it read x t1=0<x if-false t1 goto L1 fact=1 label L2 t2=fact*x fact=t2 t3=x-1 x=t3 t4=x==0 if_false t4 goto L2 write fact Label L1
• Popular forms of intermediate code: Three-address code
2010-12-13
155174-Principle of Compiler lecture 8
11
8.1.1 Three-Address Code
• The most basic instruction of three address code has the general form x=y op z
2010-12-13
155174-Principle of Compiler lecture 8
3
Overview of Code Generation • The task of code generation is to generate executable code for a target machine that is a faithful representation of the semantics of the source code • Code generation depends on
英语新选修八讲义Unit4SectionⅣLearningaboutLanguageUsingLan

Section_ⅣLearning_about_Language_&_Using_Language[原文呈现]Act Two, Scene Ⅰ MAKING THE BET①It is 11 am in Henry Higgins' house the next day. Henry Higgins and Colonel Pickering are sitting deep in②con v ersation③.H:Do you want to hear any more sounds?CP:No, thank you. I rather fancied myself④because I can pronounce twenty-four distinct vowel⑤sounds; but your one hundred and thirty beat me. I can't distinguish⑥most of them.H:(laughing) Well, that es with practice.There is a knock and Mrs Pearce⑦(MP), the housekeeper⑧,es in w ith cookies⑨,a teapot ⑩,some cream⑪and t w o cups.MP:(hesitating) A young girl is asking to see you.H:A young girl! What does she want?MP:Well, she's quite a mon kind of girl with dirty nails⑫. I thought perhaps you wanted her to talk into your machines.H:Why⑬Has she got an interesting accent? We'll see. Show her in⑭,Mrs Pearce.MP:(only half resigned⑮to it) Very well, sir. (goes do w nstairs)H:This is a bit⑯of luck. I'll show you how I make records⑰on wax⑱disks⑲...MP:(returning) This is the young girl, sir. (Eliza es into the room shyly follo w ing Mrs Pearce⑳. She is dirty and w earing a shabby○21dress. She curtsies○22to the t w o men.)[读文清障]①bet n.& v t.打赌②deep in 专心于;深陷;全神贯注于③deep in conversation是形容词短语,在句中作伴随状语。
Unit 4 Section 1 人教版英语选修8配套课件讲解

Unit 4 Pygmalion
Ⅱ.短语互译 1.(把某人)改变或冒充成 ___p_a_s_s_.._.o_f_f_a_s_.._.______ 2.结识;与……相见____m_a_k_e_o_n_e_'_s_a_c_q_u_a_in_t_a_n_c_e____ 3.一般来说 ___g_e_n_e_ra_l_ly__sp_e_a_k_i_n_g_____ 4.就……来说;从……角度__i_n_t_e_rm__s_o_f_____ 5.in all directions 朝__四__面__八__方_ 6.hand over _交__出__;__移__交____ 7.a handful of_少__数__(_人__或__物__)___ 8.in disguise _伪__装__(_的__);__假__扮__(_的__)__ 9.in amazement_震__惊__;__惊__讶____
返回导航
Unit 4 Pygmalion
2.Eliza greeted the gentleman in order to __A___. A.ask him to buy some flowers from her B.talk with him C.ask him to teach her D.beg some money from him 3.Why did Eliza begin to cry? Because __A___. A.she thought Professor Higgins would arrest her B.the gentleman didn't give her some money C.Pickering beat and scolded her D.there was no reason
决策会计 比率分析 RATIO ANALYSIS
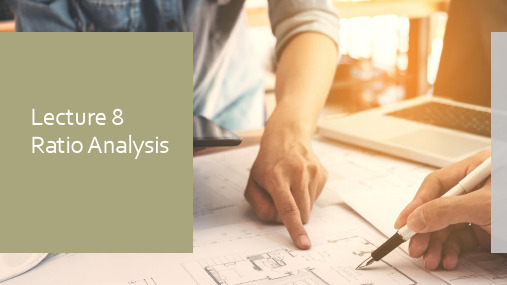
Lecture 8 Ratio AnalysisA method of analyzing financial statements by comparisons of related facts.Ratio gives a mathematical expression to a significant relationship between two related facts, and in this case two financial figures. By bringing two facts together, ratios generate new information. Ratios are often compared to:•Ratios from past periods •Industries averages •Budgeted ratios or StandardsRATIOS•the indicated quotient of twomathematical expressions•the relationship in quantity,amount, or size between two ormore things :PROPORTIONClasses ofRatios LIQUIDITY SOLVENCY PROFITABILITY OPERATINGmeasures the ability tomeet shorttermobligations measures the extend of the business financed by debt and itsability to meetlong termobligations measure the effectiveness of manager measures efficiency of day to day operationsLIQUIDITY RATIOSmeasures the ability to meet short term obligations•CURRENT RATIO = Current Assets / Current Liabilities•QUICK RATIO = [Cash + Acct. Receivable + Mkt. Securities] / C. Liabilities •WORKING CAPITAL = Current Assets –Current Liabilities•WORKING CAPITAL TURNOVER = Revenue / Average Working Capital •ACCOUNT RECEIVABLES TURNOVER = Revenue / Average Account Receivables •AVERAGE COLLECTION PERIOD = 365 / Account Receivables Turnover •INVENTORY TURNOVER = Cost Of Good Sold / Average InventoryLIQUIDITY RATIO Liquidity ratio measures the ability of the business to meet its short termobligations. Current Ratio (CR)The most common liquidity ratio is the current ratio, which is also the ratio of current assets to current liabilities. It is also expressed as how many times the assets can cover the liabilities. The calculation for current asset is shown below:Formula:Current Ratio = Current Assets ÷Current LiabilitiesCurrent Ratio = $110,000 ÷$70,000 = 1.57 times or 1.57 to 1 Therefore for $1.00 of current liabilities there is $1.57 of current assets to cover.As a creditor, you would like to have as high a current ratio as possible Rule of thumb for all businesses is 2:1. This was set because some businesses have high non-liquid assets. In hospitality industry, a ratio of 1.5 : 1.0 would be acceptable.LIQUIDITY RATIO Liquidity ratio measures the ability of the business to meet its short termobligations. Quick Ratio (QR) –Acid Test RatioThis is similar to current ratio except that current assets is replaced by quick assets.Quick assets are assets that can be easily converted into cash. Formula:Quick Ratio = [Cash + Mkt. Sec. + Accounts Receivable] ÷C. Liabilities Example:Cash = $10,000Marketable Securities= $50,000 Accounts Receivable = $20,000Current Liabilities = $70,000 QUICK RATIO = $80,000 ÷$70,000 = 1.14Therefore for $1.00 of current liabilities there is $1.14 of LIQUID assets to cover.As a creditor, you would at least a 1:1 in quick ratioAs owner/manager, quick ratio that is less than 1:1 may create problem in acquiring short term loan/debt.LIQUIDITY RATIO Liquidity ratio measures the ability of the business to meet its short termobligations.LIQUIDITY RATIO Liquidity ratio measures the ability of the business to meet its short term obligations. CURRENT ASSETS CURRENT LIABILITIESCash12,900Accounts Payable19,200Account Receivables43,100Accrued Wages Payable3,800Marketable Securities15,400Taxes Payable12,300Inventories9,900Short Term Loan26,900Prepaid Expenses4,800Accrued Utilities500TOTAL86,100TOTAL62,700CURRENT ASSETS CURRENT LIABILITIESCash12,900Accounts Payable4,200Account Receivables43,100Accrued Wages Payable3,800Marketable Securities400Taxes Payable12,300Inventories9,900Short Term Loan26,900Prepaid Expenses4,800Accrued Utilities500TOTAL71,100TOTAL47,700Working Capital = Current Assets –Current Liabilities = $23,400Current Ratio= 86,100/62,700= 1.37Current Ratio= 71,100/47,700= 1.49LIQUIDITY RATIO Liquidity ratio measures the ability of the business to meet its short termobligations. Working Capital Turnover Ratio (WCT)The ratio compares the working capital to the revenue of the business. Formula: Revenue ÷Average Working CapitalExample:Working Capital 2011 = 60,000Working Capital 2012 = 80,000Average WC = (60,000 + 80,000) ÷2 = 70,000Revenue = $500,000Therefore, WCT = 500,000 ÷70,000 = 7.14The above ratio means that the working capital of $70,000 was used 7.14 times during the year. The owner may prefer a lower working capital, thus high turnover rate, because available funds should or can be use to make long term investments that may maximize profit for the business. Creditors are looking for high current ratio that usually means a low turnover rate.LIQUIDITY RATIO Liquidity ratio measures the ability of the business to meet its short termobligations. Accounts Receivable Turnover (ART)Measures the rapidity of converting accounts receivable to cash.The higher the speed of conversion or turnover rate, the current ratio and the quick-ratio will have more credibility.Formula: Total Revenue÷Average Accounts ReceivableExample:Total Revenue = $500,000Average Accounts Receivable= (Opening Balance + Ending Balance) ÷2= (30,000 + 20,000) ÷2 = 25,000Therefore ART = 500,000 ÷25,000 = 20Owners or managers would like to see a high turnover rate. Accounts receivable in hospitality establishments are generally interest free, the sooner it is converted into cash, the better chances you have in putting the cash into productive investments.LIQUIDITY RATIO Liquidity ratio measures the ability of the business to meet its short termobligations. Average Collection Period (ACP)Measures the efficiency of collection policy of the business. The ratio translate the ART into a more understandable result.Formula: 365 ÷Accounts Receivable TurnoverExample:ART = 20Therefore, ACP = 365 ÷20 = 18.25The ACP of 18.25 means that on an average of 18.25 days through out the accounting year, the business was collecting all its accounts receivable. The lower the ACP generally reflects a efficient/tight collection or credit policy.The above formula also assume that all sales are cash sales. Management may adjust the ACP according to the percentage of credit sales to total sales. For example, if 50% of sales is credit sales, the ACP should be 18.25 ÷0.5 = 36.5LIQUIDITY RATIO Liquidity ratio measures the ability of the business to meet its short term obligations. INVENTORY TURNOVERFrequency of using the entire inventory during an accounting period.High turnover rate may reflect high frequency in ordering inventory due to low level of inventory.Low turnover rate may reflect low frequency in ordering due to high level of inventory.FORMULA: Costs of Good Sold ÷Average InventoryAverage Inventory = (Year 1 + Y ear 2) / 2FACTORS AFFECTING INVENTORY TURNOVER:•Types of service or restaurants•Location•store room space availability•bulk discountsPRACTICE 1BALANCE SHEET20112012 CURRENT ASSETS:Cash$26,950.00 $31,500.00Marketable Securities85,400.00 $60,500.00Accounts Receivable$34,000.00 $45,600.00Notes Receivable$44,000.00 $57,450.00Inventories$62,400.00 $58,900.00Total Current Assets:$252,750.00 $253,950.00 FIXED ASSETS:$1,086,450.00 $1,188,300.00 TOTAL ASSETS$1,339,200.00 $1,442,250.00 CURRENT LIABILITIES:$107,700.00 $78,250.00LONG TERM LIABIILTIES:$681,500.00 $644,000.00$789,200.00 $722,250.00 OWNERS EQUITY:$550,000.00 $720,000.00TOTAL EQUITY &LIABILITIES$1,339,200.00 $1,442,250.00CONDENSED P&L20112012REVENUE$883,400.00COST OF GOODS SOLD$212,275.00 Current Ratio Quick Ratio Accounts Receivable Turnover (ART) Average Collection PeriodWorking Capital Working Capital Turnover Inventory TurnoverPRACTICE 2BALANCE SHEET YEAR 1YEAR 2 CURRENT ASSETS:$252,750.00$253,950.00FIXED ASSETS:$1,086,450.00 $1,188,300.00 TOTAL ASSETS$1,339,200.00 $1,442,250.00 CURRENT LIABILITIES:$107,700.00 $78,250.00LONG TERM LIABIILTIES:$681,500.00 $644,000.00$789,200.00 $722,250.00 OWNERS EQUITY:$550,000.00 $720,000.00TOTAL EQUITY & LIABILITIES$1,339,200.00 $1,442,250.00CONDENSED P&L YEAR 1YEAR 2REVENUE$883,400.00COST OF GOODS SOLD$212,275.00NET INCOME BEFORE INTEREST & TAX$280,000.00INCOME AFTER INTEREST AND TAX$200,000.00ADDITIONAL INFORMATIONSHARES OUTSTANDING400,000.00 500,000.00MARKET PRICE PER SHARE $1.45 Solvency RatioDebt To Equity Ratio Total Liability To Total Equity RatioReturn on Assets Return on Investment (Equity)Earnings Per Share Price Earnings RatioCURRENT ASSETS:YEAR 1YEAR 2Cash$20,000$30,000Inventories$36,000$28,000Marketable Securities $12,000$6,000Accounts Receivable $16,000$8,000$84,000$72,000FIXED ASSETS:$340,000$423,000TOTAL ASSETS $424,000$495,000CURRENT LIABILITIES:Accounts Payable $24,000$28,000Accrued Expenses $12,000$25,000Wages Payable$8,000$12,000$44,000$65,000LONG TERM LIABILITIES:$100,000$100,000OWNERS EQUITY:$280,000$330,000TOTAL LIABILITIES & EQUITY $424,000$495,000Additional information :20112012Total Revenue$ 268,000 Income Before Interest & Tax $ 60,000 Income After Interest &Tax $ 40,000Oustanding Shares280,000 330,000PRACTICE 31CURRENT RATIO 2QUICK RATIO3ACCOUNT RECEIVABLE TURNOVER4AVERAGE COLLECTION PERIOD5WORKING CAPITAL 6WORKING CAPITAL TURNOVER7INVENTORY TURNOVER•TOTAL ASSETS TO TOTAL LIABILITIES = Total Assets / Total Liabilities•TOTAL LIABILITIES TO TOTAL ASSETS = Total Liabilities / Total Assets •DEBT TO TOTAL EQUITY = Total Liabilities / Total Equity SOLVENCY RATIOSmeasures the measures the extend of the business financed by debt and its ability to meet long term obligationsSOLVENCY RATIOS Solvency ratios measures the extend of the business financed by debt and its ability to meetlong termobligations Total Assets to Total Liabilities RatioFormula:Total Assets ÷Total LiabilitiesExample:Total Assets = $540,000Total Liabilities = $480,000Therefore, TA:TL = 540,000 ÷480,000 = 1.13The ratio show us that there is $1.13 in assets to cover every dollar in liabilities.The creditors would like to see a high TA:TL ratio, in the above example, there is a cushion of $0.13 for every $1.00 in liabilities. The higher the ratio, the greater the cushion.The assets of the business can be discounted by a total of 11.5% ($0.13 ÷$1.13) and the business will still be able to pay its creditors.SOLVENCY RATIOS Solvency ratios measures the extend of the business financed by debt and its ability to meetlong termobligations Total Liabilities to Total Assets RatioThis ratio measures the level of debt financing in the business.Formula:Total Liabilities ÷Total AssetsExample:Total Assets = $540,000Total Liabilities = $480,000Therefore, TL:TA = 480,000 ÷540,000 = 0.89The above example show us that each $1.00 of asset is financed by $0.89 by debt. The higher the ratio, the more difficult it is for the business to acquire loans.A high ratio also reflects the business use of financial leverage. The owner would like a high leverage to maximize return on investment, creditors would like a low leverage to protect their interest in the business.SOLVENCY RATIOS Solvency ratios measures the extend of the business financed by debt and its ability to meetlong termobligations Debt to Equity RatioThis ratio compares the level of debt versus equity financing.Formula:Total Liabilities ÷Total EquityExample:Total Equity = $60,000Total Liabilities = $480,000Therefore, TL:TE = 480,000 ÷60,000 = 8The above calculation show us that for each $1.00 invested by the stockholders, the creditors have invested $8.00! The higher the ratio, the more difficult it is for the business to acquire further debt financing and it also brings higher risks to the creditors.Financial LeverageThe use of debt in place of equity dollars to finance operations and increase the return on the equity dollars already invested.•high return on equity•releases additional equity funds•risk is transferred from the owner to the creditors•The greater the leverage used by the business, the lower the solvency ratio. •The creditors would like to see a high solvency ratio to ensure the return of their moneyFINANCIAL LEVERAGEFINANCIAL LEVERAGE Investment Needed$100,000$100,000Equity Financing$ 20,000$ 80,000Debt Financing$ 80,000$ 20,000Income Before Interest & Taxes (EBIT)$50,000$50,000Interest @ 10%$ 8,000$ 2,000Income After Interest$42,000$48,000Income Tax @ 40%$16,800$19,200Income After Interest & Taxes$25,200$28,800Return on Equity126%36%When financial leverage is applied, the additional $60,000 of equity funds can be diverted to be invested in other ventures or starts another restaurant.PROFITABILITY RATIOS These ratios measures management's overall effectiveness. Profitability ratios reflect the results of all areas of management's responsibilities.Return on Assets (ROA)This ratio measures the effectiveness of management's use of the organization's assets. This ratio is sometimes refer to as Gross Return on Assets.Formula:Income Before Interest & Tax ÷Total Average AssetsExample:Assets 1901 = $840,000Assets 1902 = $900,000Average Assets = ($840,000 + $900,000) ÷2 = $870,000Income Before Interest & Tax = $150,000Therefore, ROA = 150,000 ÷870,000 = 0.1724 or 17.24%The ROA above show us that if we use any of our assets for investment, we will have a return of 17.24%. If we borrowed money at 10% to financing expansion, we should have no problem paying the cost of the debt.A low ROA may be the result of low profit or excessive assets in the business.PROFITABILITY RATIOS These ratios measures management's overall effectiveness. Profitability ratios reflect the results of all areas of management's Return on Assets (NROA)This ratio is a variation of ROA, however this calculation also show us the advisability of seeking equity (as opposed to debt) financing for the business.The formula uses the net income instead of the income before interest & tax. This is because dividends or profit is only payable to the owners/stockholders after payment of interest and taxes.Formula:Net Income ÷Total Average AssetsExample:Assets 1901 = $840,000Assets 1902 = $900,000Avg Assets = ($840,000 + $900,000) ÷2 = $870,000Net Income (After Interest & Tax) = $80,000Therefore, NROA = 80,000 ÷870,000 = 0.092 or 9.20%The above calculations show us that for every $1.00 invested, the owner received $0.092 or 9.2% as return. Stockholders and owners will assume any further investment on their part will only yield the same return.PROFITABILITY RATIOS These ratios measures management's overall effectiveness. Profitability ratios reflect the results of all areas of management's responsibilities.Return on Investment or Equity (ROI or ROE)This ratio measures the effectiveness of management's use of equity funds. This ratio is sometimes refer to Return on Stockholders Equity.Formula:Net Income ÷Average EquityExample:Equity 1901 = $365,000Equity 1902 = $400,000Avg Equity = ($365,000 + $400,000) ÷2 = $382,500Net Income = $80,000Therefore, ROI = 80,000 ÷382,500 = 0.2092 or 20.92%The above calculation shows that the management is able to generate a return of 20.47% from the money the stockholders have invested. The stockholders can either put their money in the bank and get about 9% interest or invest with the company (with risks involved) for a better return.PROFITABILITY RATIOS These ratios measures management's overall effectiveness. Profitability ratios reflect the results of all areas of management's responsibilities.Earnings Per Share (EPS)This ratio calculates the earning per share, that is, comparing the net income to the total number of shares outstanding.Formula:Net Income ÷Avg Outstanding SharesExample:Shares Outstanding 1901 = 30,000Shares Outstanding 1902 = 60,000Avg Shares Outstanding = (30000+60000) ÷2 = 45,000Net Income = $80,000Therefore, EPS = 80,000 ÷45,000 = $1.78The above calculations show us that the earning per share is $1.78 for this accounting year(period).The EPS is generally calculated to also be use in another ratio called the Price Earning Ratio.PROFITABILITY RATIOS These ratios measures management's overall effectiveness. Profitability ratios reflect the results of all areas of management's responsibilities.Price Earning Ratio (PE Ratio)This ratio compares the earning per share with the market price to forecast investment possibilities.Generally, if the price of the share is several times higher than the earning potential of that share, we can conclude that there the high demand for the shares. The reason for this high demand are generally attributed to the believe of the public that the company is growing.Formula:Market Price ÷Earning Per ShareExample:Earning Per Share = $1.78Market Price = $15.00PE Ratio = $15 ÷$1.78 = 8.43Therefore, the Price of the share is 8.43 times of its earning potential.OPERATING RATIOSmeasures management's effectiveness in using its resources. Management are supposed to use assets to generate earnings for the business, therefore it is essential that the management use these resources effectively.OPERATINGRATIOSmeasuresmanagement's effectiveness in using itsresources.Inventory TurnoverThe inventory calculates how often the inventory is being used. Generally, the faster inventory is being used the better it is for the business. It is expensive to maintain a large inventory and money (that may be invested elsewhere) are tied up in inventory that are not used.Formula:Cost of Goods Sold ÷Average InventoryExample:Inventory 12/31/1901 = $20,000Inventory 12/31/1902 = $40,000Average Inventory = ($20,000 + $40,000) ÷2 = $30,000Cost of Goods Sold = $165,000Therefore, Inventory Turnover = 165,000 ÷30,000 = 5.5OPERATINGRATIOSmeasuresmanagement's effectiveness in using itsresources.Occupancy PercentageFormula: Rooms Occupied ÷Available RoomsExample:Rooms Occupied = 50Available Rooms = 120Therefore, Occupancy Percentage = 41.67%Multiple OccupancyFormula: Rooms Occupied by two or more guests ÷Rooms Occupied Example:Rooms Occupied by two or more guests = 15Rooms Occupied = 50Therefore, Multiple Occupancy Percentage = 30%OPERATINGRATIOSmeasuresmanagement's effectiveness in using itsresources.Average Room Rate or Average Daily Rate (ADR) Formula: Rooms Revenues ÷Number of Rooms SoldExample:Rooms Revenues = $3,400Rooms Sold = 50Therefore, ADR = $68.00Revenue Per Available Room (RevPar)Formula: Rooms Revenues ÷Number of Rooms SoldExample:Rooms Revenues = $3,400Rooms Available = 120Therefore, RevPar$28.33OPERATINGRATIOSmeasuresmanagement's effectiveness in using itsresources.Other Operating Ratios•Food Cost Percentage = Food Cost ÷Food Sales •Beverage Cost Percentage = Beverage Cost ÷Beverage Sales •Labor Cost Percentage = Labor Cost ÷Total Sales•Sales Mix = Individual Sales ÷Total SalesThank you。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Tom: My favorite is basketball. To me, that’s much more exciting. I like to play in a team. You know, it is not just skill. You have to have clever strategy. John: It’s too competitive for me. Many people play rough. You may easily get injured. Tom: That’s true. But I just love it! John: Our sports meeting is due next Saturday. Have you entered for anything? Tom: I’m not good at track and field. But I may take part in the basketball match. How about you? John: I’ll go for the one-hundred-meter dash.
Jean: Well, actually I’ve tried a few times, but they make me feel awful. Tom: That’s because you seldom exercise. Believe me, you’ll soon feel better. But of course you must begin step by step. And you have to keep it up. Jean: Perhaps I should learn from my grandma and play mahjong. Mahjong will soon become an Olympic game, I hear. Tom: I’m talking about physical exercises. Jean: Mahjong is very physical, believe me. Try playing it seven hours a day for a week, and see how you feel. Well, I’m just kidding. OK, I’ll take your advicet? (4 people with different professions) Main points included: Name for the exercise: Reasons for you: Time and place:
How to keep fit
Make up your own sentences: • in great shape • in good health • depend on • it’s great fun to do • take part in
Act out: 1) Neck exercise • Slowly lean your head to the left, with your ear approaching your shoulder. Keep this position for 3 seconds. • Then bring your head back to vertical and relax. Do the same to the other side. • Turn your head to the left and look back over your shoulder. Hold for 3 seconds and do the same to the right. • Tuck your chin in, pull your head straight back, and hold for 3 seconds. Then tilt your face to the ceiling and hold for 3 seconds. Bring your head back to vertical and relax.
Lecture 8 sports
1. Useful expressions 2. Dialogues 3. Discussions 4. Additional information
1. Useful expressions
Keep fit Be in good shape Work out in the gym • How well do you play basketball? • Pretty well. How good are you at sports? • About average, I guess. • Not very well. • Not too bad.
• It comes from ancient Sanskrit meaning “union’; and it is one of the techniques of an ancient Indian discipline. • People have used Yoga to help search for happiness and contentment for thousands of years. Nowadays, keep peace and fit? • Actions in yoga: 1)the lotus position (fold your legs over one another) 2)meditation
Action At Gym
• • • • • • •
go to the gym show them your membership card change into sweat pants in the changing room lock all your stuff in the locker drink some water before you start to work out find a mat and put it on the ground stretch your body and exercise your hands and feet
Outline of teaching
Lecture 0: Review + Topic Lecture 1: Words about hospital and dialogues Lecture 2-3: Going to the Hospital or Clinic Lecture 4-5: Seeing a Doctor Lecture 5-6: In/Out-patient Service Lecture 6-8: Habits Lecture 8-9: Sports lecture 10-11: Hosting a Party Lecture 11-12: hosting a meeting summary
Questions
1. Why doesn’t Jean do exercises? 2. What does Jean think of exercise? 3. What does Tom say to Jean about persuading her to do exercises? 4. what joke does Jean make about doing exercise?
•
• do 40 push-ups • do 30 sit- ups • work out on a running machine for 30 minutes • slow down and regulate your breathing • take a shower • go home
About Yoga
2. dialogues
1): why don’t you do exercises? Tom: Why don’t you do exercises, Jean? Jean: I’m busy. I have no time. Tom: That’s no excuse. Jean: Well, I’m different from you. You’re bursting with energy. Exercise is fun to you, and can keep you fit. I’m weak. Exercise tires me out and makes me too fatigued to do anything for the rest of the day. Tom: You are weak because you don’t do exercises. If you did, you’d be strong, energetic and efficient. You wouldn’t feel so burnt out and you wouldn’t be complaining about your unfinished work.
Words: gym/fitness center aerobics kick boxing 搏击操(dummy假人) do some curls workout ball 健身球 exercise bike健身车 Latin dance treadmill (running machine) dumbbell yoga push-up sit- up sauna
2) Shoulder rolls • Stand up straight, with feet slightly apart. • Move your shoulders forward. Roll them up toward your ears and then behind you.
Working out at the Gym
2). Favorite Sports Tom: Hey, John. You’re in great shape! John: Thanks. You’re in good health too. Tom: How do you manage it? Do you exercise regularly? John: Yes. I work hard at it. I just love to exercise. Tom: What are your favorite sports? John: It depends on the season. I like swimming, jogging, and tennis. But I guess my favorite is badminton. It’s great fun and physically demanding with all the running and smashing.