function1
c语言function函数的用法

c语言function函数的用法C语言中的函数是一种非常重要的编程工具,它可以让程序员将代码分解成小块,使得代码更加模块化和易于维护。
在本文中,我们将探讨C语言中函数的用法,包括函数的定义、调用、参数传递和返回值等方面。
1. 函数的定义在C语言中,函数的定义通常包括函数名、参数列表和函数体。
函数名用于标识函数,参数列表用于传递参数,函数体则是函数的具体实现。
下面是一个简单的函数定义示例:```int add(int a, int b){return a + b;}```在上面的示例中,函数名为add,参数列表包括两个整型参数a和b,函数体中使用return语句返回a和b的和。
2. 函数的调用函数的调用是指在程序中使用函数的过程。
在C语言中,函数的调用通常使用函数名和参数列表来完成。
下面是一个简单的函数调用示例:```int result = add(1, 2);```在上面的示例中,我们调用了add函数,并将返回值赋值给result 变量。
函数调用时,需要传递与函数定义中参数列表相匹配的参数。
3. 参数传递在C语言中,函数的参数传递可以通过值传递和指针传递两种方式来实现。
值传递是指将参数的值复制一份传递给函数,而指针传递则是将参数的地址传递给函数。
下面是一个简单的值传递和指针传递示例:```void swap(int a, int b){int temp = a;a = b;b = temp;}void swap_ptr(int* a, int* b){int temp = *a;*a = *b;*b = temp;}int main(){int x = 1, y = 2;swap(x, y);printf("x=%d, y=%d\n", x, y); // 输出 x=1, y=2swap_ptr(&x, &y);printf("x=%d, y=%d\n", x, y); // 输出 x=2, y=1return 0;}```在上面的示例中,我们定义了两个函数swap和swap_ptr,分别用于值传递和指针传递。
_stdcall介绍

stdcall调用约定:stdcall很多时候被称为pascal调用约定,因为pascal是早期很常见的一种教学用计算机程序设计语言,其语法严谨,使用的函数调用约定就是stdcall。
在Microsoft C++系列的C/C++编译器中,常常用PASCAL宏来声明这个调用约定,类似的宏还有WINAPI和CALLBACK。
stdcall调用约定声明的语法为(以前文的那个函数为例):int __stdcall function(int a,int b)stdcall的调用约定意味着:1)参数从右向左压入堆栈,2)函数自身修改堆栈 3)函数名自动加前导的下划线,后面紧跟一个@符号,其后紧跟着参数的尺寸。
以上述这个函数为例,参数b首先被压栈,然后是参数a,函数调用function(1,2)调用处翻译成汇编语言将变成:push 2 第二个参数入栈push 1 第一个参数入栈call function 调用参数,注意此时自动把cs:eip入栈而对于函数自身,则可以翻译为:push ebp 保存ebp寄存器,该寄存器将用来保存堆栈的栈顶指针,可以在函数退出时恢复mov ebp,esp 保存堆栈指针mov eax,[ebp + 8H] 堆栈中ebp指向位置之前依次保存有ebp,cs:eip,a,b,ebp +8指向aadd eax,[ebp + 0CH] 堆栈中ebp + 12处保存了bmov esp,ebp 恢复esppop ebpret 8而在编译时,这个函数的名字被翻译成_function@8注意不同编译器会插入自己的汇编代码以提供编译的通用性,但是大体代码如此。
其中在函数开始处保留esp到ebp中,在函数结束恢复是编译器常用的方法。
从函数调用看,2和1依次被push进堆栈,而在函数中又通过相对于ebp(即刚进函数时的堆栈指针)的偏移量存取参数。
函数结束后,ret 8表示清理8个字节的堆栈,函数自己恢复了堆栈。
调用堆栈
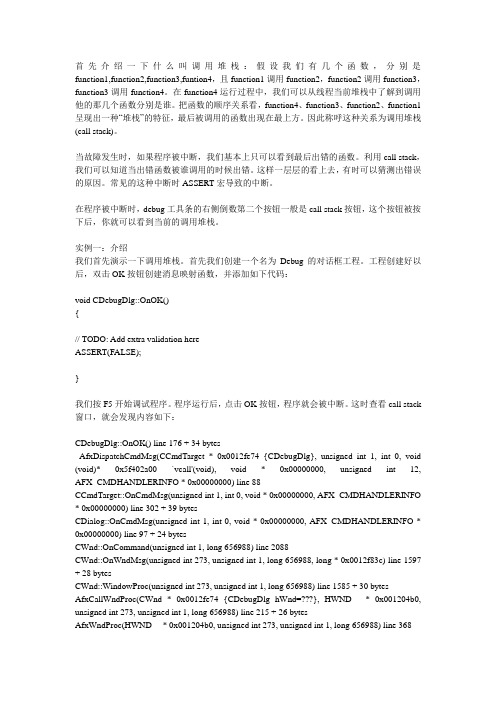
首先介绍一下什么叫调用堆栈:假设我们有几个函数,分别是function1,function2,function3,funtion4,且function1调用function2,function2调用function3,function3调用function4。
在function4运行过程中,我们可以从线程当前堆栈中了解到调用他的那几个函数分别是谁。
把函数的顺序关系看,function4、function3、function2、function1呈现出一种“堆栈”的特征,最后被调用的函数出现在最上方。
因此称呼这种关系为调用堆栈(call stack)。
当故障发生时,如果程序被中断,我们基本上只可以看到最后出错的函数。
利用call stack,我们可以知道当出错函数被谁调用的时候出错。
这样一层层的看上去,有时可以猜测出错误的原因。
常见的这种中断时ASSERT宏导致的中断。
在程序被中断时,debug工具条的右侧倒数第二个按钮一般是call stack按钮,这个按钮被按下后,你就可以看到当前的调用堆栈。
实例一:介绍我们首先演示一下调用堆栈。
首先我们创建一个名为Debug的对话框工程。
工程创建好以后,双击OK按钮创建消息映射函数,并添加如下代码:void CDebugDlg::OnOK(){// TODO: Add extra validation hereASSERT(FALSE);}我们按F5开始调试程序。
程序运行后,点击OK按钮,程序就会被中断。
这时查看call stack 窗口,就会发现内容如下:CDebugDlg::OnOK() line 176 + 34 bytes_AfxDispatchCmdMsg(CCmdTarget * 0x0012fe74 {CDebugDlg}, unsigned int 1, int 0, void (void)* 0x5f402a00 `vcall'(void), void * 0x00000000, unsigned int 12, AFX_CMDHANDLERINFO * 0x00000000) line 88CCmdTarget::OnCmdMsg(unsigned int 1, int 0, void * 0x00000000, AFX_CMDHANDLERINFO * 0x00000000) line 302 + 39 bytesCDialog::OnCmdMsg(unsigned int 1, int 0, void * 0x00000000, AFX_CMDHANDLERINFO * 0x00000000) line 97 + 24 bytesCWnd::OnCommand(unsigned int 1, long 656988) line 2088CWnd::OnWndMsg(unsigned int 273, unsigned int 1, long 656988, long * 0x0012f83c) line 1597 + 28 bytesCWnd::WindowProc(unsigned int 273, unsigned int 1, long 656988) line 1585 + 30 bytes AfxCallWndProc(CWnd * 0x0012fe74 {CDebugDlg hWnd=???}, HWND__ * 0x001204b0, unsigned int 273, unsigned int 1, long 656988) line 215 + 26 bytesAfxWndProc(HWND__ * 0x001204b0, unsigned int 273, unsigned int 1, long 656988) line 368AfxWndProcBase(HWND__ * 0x001204b0, unsigned int 273, unsigned int 1, long 656988) line 220 + 21 bytesUSER32! 77d48709()USER32! 77d487eb()USER32! 77d4b368()USER32! 77d4b3b4()NTDLL! 7c90eae3()USER32! 77d4b7ab()USER32! 77d7fc9d()USER32! 77d76530()USER32! 77d58386()USER32! 77d5887a()USER32! 77d48709()USER32! 77d487eb()USER32! 77d489a5()USER32! 77d489e8()USER32! 77d6e819()USER32! 77d65ce2()CWnd::IsDialogMessageA(tagMSG * 0x004167d8 {msg=0x00000202 wp=0x00000000 lp=0x000f001c}) line 182CWnd::PreTranslateInput(tagMSG * 0x004167d8 {msg=0x00000202 wp=0x00000000 lp=0x000f001c}) line 3424CDialog::PreTranslateMessage(tagMSG * 0x004167d8 {msg=0x00000202 wp=0x00000000 lp=0x000f001c}) line 92CWnd::WalkPreTranslateTree(HWND__ * 0x001204b0, tagMSG * 0x004167d8 {msg=0x00000202 wp=0x00000000 lp=0x000f001c}) line 2667 + 18 bytesCWinThread::PreTranslateMessage(tagMSG * 0x004167d8 {msg=0x00000202 wp=0x00000000 lp=0x000f001c}) line 665 + 18 bytesCWinThread::PumpMessage() line 841 + 30 bytesCWnd::RunModalLoop(unsigned long 4) line 3478 + 19 bytesCDialog::DoModal() line 536 + 12 bytesCDebugApp::InitInstance() line 59 + 8 bytesAfxWinMain(HINSTANCE__ * 0x00400000, HINSTANCE__ * 0x00000000, char * 0x00141f00, int 1) line 39 + 11 bytesWinMain(HINSTANCE__ * 0x00400000, HINSTANCE__ * 0x00000000, char * 0x00141f00, int 1) line 30WinMainCRTStartup() line 330 + 54 bytesKERNEL32! 7c816d4f()这里,CDebugDialog::OnOK作为整个调用链中最后被调用的函数出现在call stack的最上方,而内核中程序的启动函数Kernel32! 7c816d4f()则作为栈底出现在最下方。
Function_1

8
当x > 0 当x = 0 当x < 0
x
2. Some Properties of Functions 2.1 Symmetry Let f be a function with domain D which is symmetric with respect to the origin (i.e.,x∈D implies that –x∈D). If f(x)=f(-x) for all x in D, then f is called an even function. If f(-x)=-f(x) for all x in D, f is called an odd function. If f is an even function, the graph of f is symmetric with respect to the y-axis, since if a point(x, f(x)) is on the graph, the point (-x, f(x)) is also on the graph. If f is an odd function, the graph of f is symmetric with respect to the origin as for any x in the domain of f, points (x, f(x)) and (-x, -f(x)) are both on the graph.
5
2 x 1, Exampl f ( x ) = 2 x 1, e:1-7
x>0 x≤0
y = x2 1
y
y = 2x 1
o
x
6
example 1-8 设某药物的每天剂量为 (单位 毫克 , 剂量为y 单位 毫克) 单位:毫克 对于16岁以上的成年人用药剂量是一常数 设为2mg.而对 岁以上的成年人用药剂量是一常数,设为 对于 岁以上的成年人用药剂量是一常数 设为 而对 岁以下的未成年人,则每天用药剂量 于16岁以下的未成年人 则每天用药剂量 成比于年龄 , 岁以下的未成年人 则每天用药剂量y 成比于年龄x 比例常数为0.125mg/岁,其函数关系为 比例常数为 岁e 1 Given a function f(x)=√1-x2, find the domain and the range of f, and sketch its graph. Solution The domain of f is the set of all real numbers such that f(x) is real. As f(x) exists if and only if the radicand 1-x2 is nonnegative; i.e., 1-x2≥0, or equivalently, -1≤x≤1, the domain is [-1, 1]. As 0≤1-x2≤1, whenever x is in [-1, 1],it follows that 0≤√1-x2≤1. Hence , the range is [0, 1]. The graph of f(x) is the upper portion of the unit circle as illustrated in Figure 1.
JavaScript中如何判断一个变量是否为函数类型

JavaScript中如何判断一个变量是否为函数类型在JavaScript中,判断一个变量是否为函数类型有多种方法。
本文将介绍三种常用的方法:typeof运算符、instanceof运算符和使用Object.prototype.toString方法。
一、使用typeof运算符在JavaScript中,typeof运算符可以返回一个变量的类型。
当变量是函数类型时,typeof运算符会返回"function"。
因此,我们可以通过对变量使用typeof运算符,然后判断返回的结果是否为"function"来判断变量是否为函数类型。
示例代码如下:```javascriptfunction isFunction1(variable) {return typeof variable === 'function';}```二、使用instanceof运算符JavaScript中的instanceof运算符可以判断一个对象是否属于某个特定类。
在JavaScript中,函数也是对象的一种,每个函数都是Function类的实例。
因此,通过使用instanceof运算符可以判断一个变量是否为函数类型。
示例代码如下:```javascriptfunction isFunction2(variable) {return variable instanceof Function;}```三、使用Object.prototype.toString方法在JavaScript中,Object.prototype.toString方法是一个通用的方法,可以获取一个变量的类型。
通过使用这个方法,我们可以获取一个函数对象的类型字符串"[object Function]"。
进一步,我们可以使用正则表达式对这个类型字符串进行匹配,判断一个变量是否为函数类型。
示例代码如下:```javascriptfunction isFunction3(variable) {return Object.prototype.toString.call(variable) === '[object Function]';}```综上所述,我们可以使用typeof运算符、instanceof运算符和Object.prototype.toString方法来判断一个变量是否为函数类型。
function是什么意思中文翻译

function是什么意思中文翻译function既能做名词也能做动词,那么你知道function做名词和动词分别都是什么意思吗?下面店铺为大家带来function的英语意思解释和英语例句,供大家参考学习!function作名词的意思功能,作用;应变量,函数function作动词的意思有或起作用;行使职责function的英语音标英 [ˈfʌŋkʃən] 美 [ˈfʌŋkʃən]function的时态现在分词: functioning过去式: functioned过去分词: functionedfunction的英语例句1. Other causes of migraine are VDU screens and strip-lighting.偏头痛的其他诱因还有电脑的视频显示器和灯管照明。
2. Sensitive Cream will not strip away the skin's protective layer.敏感肌肤专用面霜不会破坏皮肤表面的保护层。
3. She'd managed to strip the bloodied rags away from Nellie's body.她已经设法将内利身上沾满血污的破烂衣衫脱掉了。
4. The yacht is built of cedar strip planking.游艇是用雪松木条建造的。
5. Mr Porter was subjected to a degrading strip-search.波特先生被迫接受有辱人格的裸身搜查。
6. All 23 of them were strip-searched for drugs.他们23个人均被裸体搜查,看是否携带毒品。
7. The doctor told me to strip down to my shorts.医生让我脱得只剩一条短裤.8. She banded the birthday gift with a silk strip.她用一根丝带扎好了生日礼物.9. Strip the children down ; get them out of those wet clothes.把孩子们的那些湿衣服脱光.10. The aircraft circled round [ around ] over the landing strip.飞机在着陆跑道上空兜圈子.11. Strip out any damaged wiring.清除所有损坏的配线.12. The dancer did a strip.跳舞者表演了脱衣舞.13. The bent strip can straighten up by itself.这弯曲的金属片自己可以变直.14. Make a Mobius strip out of a ribbon of mild steel and magnetise it.用一根软钢条做成麦比乌斯带,将其磁化。
c语言程序注释模板

竭诚为您提供优质文档/双击可除c语言程序注释模板篇一:c语言编写规范之注释1、头文件包含includes2、私有类型定义privatetypedef3、私有定义privatedefine4、私有宏定义privatemacro5、私有变量privatevariables6、私有函数原型privatefunctionprototypes7、私有函数privatefunctions8、私有函数前注释/************************************************** ******************************Functionname:Fsmc_noR_init*description:conf igurestheFsmcandgpiostointerfacewiththenoRmemory.*thisfunctionmustbecalledbeforeanywrite/readoperation *onthenoR.*input:none*output:none*Return:none*************************************************** ****************************/9、程序块采用缩进风格编写,缩进空格为4。
10、相对独立的程序块之间、变量说明之后必须加空行;11、较长的字符(>80字符)要分成多行书写,长表达式要在低优先级操作符划分新行,操作符放在新行之首,新行要恰当缩进,保持排版整齐;12、循环、判断等语句中若有较长的表达式或语句,则要进行适应的划分,长表达式要在低优先级操作符处划分新行,操作符放在新行之首;13、若函数或过程中的参数较长,则要进行适当的划分。
14、不允许把多个短语句写在一行中,即一行只写一条语句。
15、if、for、do、while、case、switch、default等语句自占一行,且if、for、do、while等语句的执行语句部分无论多少都要加括号{}。
函数 function 原型方法(一)

函数 function 原型方法(一)函数 function 原型什么是函数 function 原型?•函数 function 原型定义了函数的基本结构和功能。
•使用原型可以创建多个相似的函数实例。
为什么要使用函数 function 原型?•提高代码的复用性:通过使用原型,可以在不重复编写代码的情况下创建多个相似的函数实例。
•简化代码的维护:对于需要修改的功能,只需要修改原型中的代码,所有基于该原型创建的函数实例都会自动更新。
如何使用函数 function 原型?1.创建函数 function 原型对象:function MyFunction() {// 函数原型的代码}2.向函数原型添加方法和属性:MyFunction.prototype.methodName = function () {// 方法的代码};MyFunction.prototype.propertyName = value;3.基于函数原型创建函数实例:var myFunctionInstance = new MyFunction();基本语法解释•MyFunction:函数原型的名称,可根据实际需要自定义。
•methodName:方法名称,可根据实际需要自定义。
•propertyName:属性名称,可根据实际需要自定义。
•value:属性值,可以是基本类型或对象。
使用原型来定义方法•通过原型定义的方法可以在所有基于函数原型创建的实例中共享。
•这样可以节省内存空间,因为所有实例共享同一个方法的引用。
使用原型来定义属性•通过原型定义的属性同样可以在所有实例中共享。
•但是注意,如果属性是对象类型,修改其中一个实例的属性值可能会影响其他实例。
原型继承•可以通过原型链实现继承,使一个函数原型从另一个函数原型派生出来。
•派生的函数原型将会包含父原型中的所有方法和属性。
总结•函数 function 原型是创建多个相似函数实例的基础。
•使用原型可以提高代码的复用性和简化维护工作。
全新版大学英语听说教程1答案

Part ACommunicative Function1. How are you?/ I'd like you to meet my classmate.2. I'm.../ May I introduce...to you?/ Pleased to meet you.3. Come and meet my family./ ...this is Tom./ It's good to know you./ ...this is my sister.Part BTextExercise 1: 1. B 2. DExercise 2:1. Yang Weiping:China/ Chemistry/ Likes listening to English programs on radio and TV; enjoys English pop songs/ Started learning English several years ago/ Favorite activity: listening; Difficulty:speaking2. Virginia:Singapore/ Library science/ To get a good job, one has be to fluent in English./ Started learning English in high school./ Favorite activity: reading; Difficulty: writingPart CExercise:How to Improve Listening ComprehensionAmong the four skills of listening , speaking, reading and writing, I find listening most difficult, because I worry about the words I don't know. Now I am trying to focus on the general idea, not worrying about he new words. This makes me feel good, because I know I have understood something. Then, I listen again carefully and if I have any problems I play the difficult part again. In this way I come to understand better both the main idea and the details ofthe listening text.Part D (Refer to TextBook)Unit 2Part ACommunicative Function1. closing2. opening3. closing4. opening5. opening6. openingListening Strategy1. a2. b3. b4. a5. b6. a7. b8. a9. b 10. bPart BTextExercise 1:1. 1) b 2) c 3) aExercise 2:1. a. age b. money c. people's appearance2. a. ...say that again? I did not catch it./ b. ...speak more slowly, please?3. a....I really need to be going./ ...nice talking to you.Part C·I hear this idea: 1/2·I don't hear this idea but I can infer it: 4/5/6·I don't hear this idea and I can't infer it: 3Part D (Refer to TextBook)Unit 3Part ACommunicative FunctionMaggie likes swimming but she does not care for skiing. She loves flying on planes and traveling by train but she hates getting on buses because they are too crowded and dirty. she is not interested in playing the piano and she prefers reading to playing computer games. She loves going to Chinese restaurants and her favorite food is spicy Sichuan bean curd. After work she is keen on listening to music. She prefers light music to rock, because light music makes feel relaxed. She enjoys watching TV in the evening. She thinks a lot of news programs but sitcoms are the last kind of thing for her to watch.Listening Strategy1. /br/2. /pr/3. /kl/4. /tr/5. /sp/6. /pr/7. /pl/8. /str/9. /gr/ 10. /gl/Part BTextExercise 1: 1.c 2.dExercise 2:1. Private2. Halls of Residence3. Self-catering (rent per week)4. 37.86 (single)5. 52.78 (double)Part CExercise:1. A busy life2. Between 6 and 15 hours3. They must remain current in their fields.4. They will revise and update them.Part D (Refer to TextBook )Part ACommunicative Function1. Yeah/ By the way/ Who?/ Don't you think so?/ Yes./ Quite well.2. Like what?/ Yeah/ Hmmm, let me think./ Well./ Come to think of it.Listening Strategy1. 923812. 26083. 15404. 755. 1566. 9007. 842008. 17359. 9:4010. 5:45Part BTextExercise 1: 1. c 2.a 3. dExercise 2:1. At Carol's house on Saturday2. He's uncertain whether he can have a good time at the party or not.3. He is not good at small talk.4. one should talk about something other people are interested in.5. by getting them to talk about themselves.Part CExercise: 1. F 2.T 3. F 4.T 5.FPart D (Refer to TextBook )Unit 5Part ACommunicative Function1. Call Back David Johnson this afternoon2. Call Bill Green at 415-289-1074 this evening. It's important.3. Meet Judy outside the Art Museum at ten tomorrow morning.4. Don't forget to go to Tom's party this evening.Listening Strategy1. 6247-22552. 5404-99823. 612-930-9608Part BTextExercise 1: 1. b 2. aExercise 2:Telephone Message:For: Mr. Johnson of ABC ImportsCaller: Richard Alexander from Star ElectronicsMobile Phone Number: 909-555-2308Office number: 714-555-2000Message: Call Richard Alexander at office number before 6pm.Part CExercise:1. Brian Tong2. Good luck Company3. Computer sales representative4. a degree in Computer science5. a computer programmer in a trading company for thee years.6. 38839673Part D (Refer to TextBook)Unit 6Part ACommunicative Function1. He wants to know where he can buy a painting2. He found out how much the dress cost as well as where hi could buy it.3. She suggests that them man buy a tie for his cousin.Listening Strategy1. 20.502. 50.953. 175.404. 50.805. 594Part BTextExercise 1:1. In a department store2. there are four people speaking in the conversation. they are the receptionist, thesalesperson, Ann and Mark3. to buy a dress for AnnExercise 2: 1. a 2. d 3. b 4. d 5. cPart CExercise:1. ...some defective goods2. ...was absent/...had mistaken his shop for a second had goods store./ ...was careless3. ...the mistake/...exchange the ladies' purchases/...half the price.Part D (Refer to TextBook)Unit 7Part ACommunicative Function1.O,2.O3.F4.F5.O6.F7.O8.O9.F 10.O 11.O 12.FListening Strategy (omitted)Part BTextExercise 1: 1.a 2.dExercise 2:Steve Wellsa university juniorB averagea lifeguard for two summersin an apartmenthard working and reliableseldom absent from work and always on timepay the rent of the apartmenta clerk in the mailroom2 to 6 am Monday through FridayminimumPart CExercise:mentioned: 1,3not mentioned but can be inferred: 2,5not mentioned and can't be inferred: 4,6Part D (Refer to TextBook)Unit 8Part ACommunicative Function1. because he dialed the wrong number2. because she was late for work. she overslept.3. because he did not notify her earlier about quitting.4. because he could not hire the woman.Listening Strategy (omitted)Part BTextExercise 1: 1.c 2.b 3.cExercise 2:1. he was clumsy and spoiled everything he did.2. in a warehouse.3. he unpacked the goods newly arrived from the factory and put them in assigned places.4. Fred broke a large base.5. $3506. to deduct part of Fred's weekly wages until the base was paid for.7. as it would take a long time to deduct $350 from his wages, he could keep the job whilehe was paying for the vase.Part CExercise: 1.d 2.c 3.d 4.b 5.bPart D (Refer to TextBook )Unit 9Part ACommunicative Function1.Mrs. FaberOct. 20thThree nightsone double room130 dollars including breakfast2.Mr.Green8:00 tomorrow morningPudong AirportRoom 804, Park HotelListening Strategy1. March 122. May 23. 25 days4. June 9Part BTextExercise 1: 1.d 2.b,d,e,f,gExercise 2: 1.c 2.d 3.b 4.bPart CExercise:1. they will have two leisurely weks on the beach2. expensive/ a train or a bus3. share the expenses/ cost too much4. have enough time/ the new semester5. good food/ casual clothes/ their homePart D (Refer to TextBook)Unit 10Part ACommunicative Function1.·big/exciting/crowded·expensive·lovely/historic2.1) very pretty2) lovely views3) /4) fascinating5) large shopping malls6) stores not too expensiveListening Strategy (ommitted)Part BTextExercise 1: 1.a,f 2.d,gExercise 2:Located in: Catcotin Mountain in Maryland because it is cool and safe. Composed of: an office for the president and living areas for his family and guests as well as a swimming pool and areas to play golf and other sports.Set up by President Roosevelt in 1942Present name given by: President Eisenhower for his grandson in 1953Used as : official presidential holiday resort since 1945Used by: several presidents for important meetings and talks during World War Two and in1959, 1978, and in July 2000.Part CExercise: 1.T 2.F 3.F 4.T 5.FPart D (Refer to TextBook )Unit 11Part ACommunicative FunctionB: Why don't you buy him a dog? Dogs are so friendly.B: How about a rabbit?B: Have you thought about bu7ying him a bird?B: Then you can buy him some tropical fish. They are pretty.B: The market. Shall we go right now?Listening Strategy1. once a week2. twice a week3. once a month4. every other day5. four nights a week6. neverPart BTextExercise 1: 1.b 2.cExercise 2: 1.F 2.F 3.F 4.T 5.F 6.TPart CExercise: 1.a 2.b 3.d 4.c 5.dPart D (Refer to TextBook )Unit 12Part ACommunicative Function: 1.c 2.dListening Strategy1. ...there are more and more ways...2. ...interested in...3. An average day...costs a dog owner...4. ...but only for a few weeks at a time5. Small talk is easy, isn't it?6. ...fill in a form...7. When I put my card in, the machine ate it.Part BTextExercise 1: 1.b 2.c 3.dExercise 2:1. A customer's credit card got stuck in a ATM machine.2. ...a wrong code numger three times3. go to the counter/ fill in a form with his account number and the date/ Purpose: to get thecustomer a new card4. in about a weekPart CExercise: 1.F 2.F 3.T 4.T 5.FPart D (Refer to TextBook)Unit 13Part ACommunicative Function1. he went for a visit to his hometown2. he went for an autumn walk in the hills3. he went on a river trip4. she did nothing but lie in bed5. she came down with the fluListening Strategy (ommitted)Part BTextExercise 1: 1.c 2.dExercise 2:1. Hid belief that one day he would become a movie star2. parking cars for one of Hollywood's big restaurants3. No, his pay was only basic. but he got generous tips form guests driving into therestaurant.4. Larry parked the car of a famous film director and was able to introduce himself to theman.5. He was amused by Larry's usual way of recommending himself.Part CExercise: 1.b 2.a 3.c 4.d 5.bPart D (Refer to TextBook)Unit 14Part ACommunicative Functioncolor: orangecomposition: woolusage: to keep warmthe present: a woolen scarfListening Strategy (ommitted)Part BTextExercise 1: 1.a 2.dExercise 2:1. ...form pictures in your own mind2. ...stay in the room where the radio set is3. ...do something else, like driving in the car, jogging, or even just walking around.4. ...half an hour or hourly intervals. ...variety of topics.5. ...the radio station they are listening to...opinions.Part CExercise: 1.F 2.T 3.F 4.T 5.F 6.TPart D (Refer to TextBook )Unit 15Part ACommunicative FunctionAGREE: 1,2,5,8DISAGREE: 3,4,6,7Listening Strategy : 1.b 2.a 3.b 4.a 5.a 6.bPart BTextExercise 1: 1.b 2.d 3.dExercise 2:1. Roommate2. female roommate3. fifth avenue4. three blocks5. rent6. September 17. 555067928. 59. 9 p.m.10. for sale11. sofa12. easy chair13. excellent condition14. $35015. offer16. 555-679217. 518. 9 p.m.Part CExercise: 1.F 2.T 3.F 4.T 5.FPart D (Refer to TextBook)Unit 16Part ACommunicative Functionsimilarities: ...family reuniondifferences:...New Year's Eve's dinnerTV's Spring Festival Special...firecrackers...Christmas trees...presents under the treeListening Strategy :Yes: 2, 3, 5, 7No: 1, 4, 6, 8Part BTextExercise 1: 1.c,g 2.aExercise 2: 1.d 2.a 3.bPart CExercise:mentioned: 5not mentioned but can be inferred: 2 not mentioned and can't be inferred: 1.3.4.6 Part D (Refer to TextBook精选。
pgsqlfunction系列之一:返回结果集

pgsqlfunction系列之一:返回结果集pgsql function 系列之一:返回结果集--------------------------------------------------------------------------------我们在编写postgresql数据库的函数(或称为存储过程)时,时常会遇到需要返回一个结果集的情况,如何返回一个结果集,返回一个结果集有多少种方式,以及如何选择一个合适的方式返回结果集,这是一个需要仔细考虑的问题。
本文仅简单的罗列出各种返回结果集的方式并试图分析他们的特点,而采用何种方式则留给大家自己去判断。
阅读本文需要一定的postgresql数据库端编程知识,如果你缺乏这方面的知识,请先翻阅postgresql文档。
----------------------------------------------------分割线-------------------------------------------------------第一种选择:声明setof 某表/某视图返回类型这是postgresql官方推荐的返回结果集的形式,当你查阅postgresql官方文档的时候,你会看到的就是这种形式。
如果采用这种形式,你的function代码看起来会像这样:CREATE OR REPLACE FUNCTION function1 () RETURNS setof table1 AS$body$DECLAREresult record;BEGINfor result in select * from table1 loopreturn next result;end loop;return;END;$body$LANGUAGE 'plpgsql' VOLATILE CALLED ON NULL INPUT SECURITY INVOKER;这是使用pl/pgsql语言的风格,你甚至可以使用sql语言让代码看起来更简单:CREATE OR REPLACE FUNCTION function1 () RETURNS SETOF table1 AS$body$SELECT * from table1;$body$LANGUAGE 'sql' VOLATILE CALLED ON NULL INPUT SECURITY INVOKER;以下是分析:首先我们说优点,第一、这是官方推荐的;第二、当使用pl/pgsql语言的时候,我们可以在循环中加上判断语句,仅返回我们需要的行;第三、在jdbc调用中,我们可以像查询普通的表一样使用这个function,例如:"select * from function1()"。
simulink中matlabfunction用法(一)

simulink中matlabfunction用法(一)Simulink中matlabfunction用法简介Simulink中的matlabfunction是一种模块,在模型中使用Matlab代码实现自定义的算法或功能。
它可以帮助用户扩展Simulink 库功能,使其更加灵活、强大。
基本用法1.添加matlabfunction模块在Simulink模型中,找到Simulink Library Browser,展开Simulink文件夹,然后将matlabfunction模块拖动到编辑区。
2.编写Matlab代码双击matlabfunction模块,进入编辑界面,编写自定义的Matlab代码。
可以使用Matlab语言的全部功能。
3.输入输出在模块界面,用户可以定义输入和输出。
可以指定输入端口数目、名称和类型。
同样可以定义输出端口数目、名称和类型。
高级用法1.多输入输出在matlabfunction模块中,可以定义多个输入和输出。
只需在输入输出界面添加相应的输入输出端口即可。
2.参数传递可以向matlabfunction模块传递参数。
在模块界面添加参数输入端口,并在代码中使用这些参数。
3.构建矩阵和向量matlabfunction模块支持构建矩阵和向量的操作。
用户可以在Matlab代码中使用矩阵和向量的各种操作,如矩阵相乘、转置等。
4.使用Simulink信号可以使用信号或信号线连接到matlabfunction模块中的输入端口,以及从matlabfunction模块输出到信号线。
5.参数设置在Simulink模型中,可以通过设置参数,修改matlabfunction模块的行为。
例如,可以设置代码生成选项或优化选项。
总结通过matlabfunction模块,我们可以在Simulink模型中使用自定义的Matlab代码,实现更丰富的功能。
它的灵活性和强大性使得Simulink模型更加适应各种应用场景。
英文function是什么中文意思
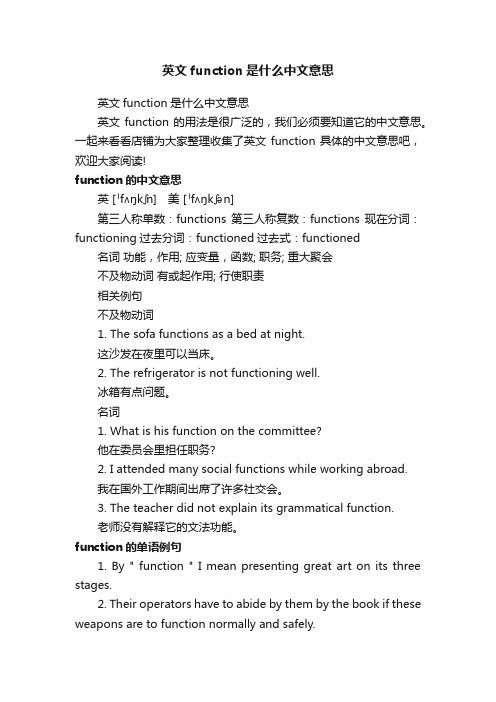
英文function是什么中文意思英文function是什么中文意思英文function的用法是很广泛的,我们必须要知道它的中文意思。
一起来看看店铺为大家整理收集了英文function具体的中文意思吧,欢迎大家阅读!function的中文意思英 [ˈfʌŋkʃn] 美 [ˈfʌŋkʃən]第三人称单数:functions第三人称复数:functions现在分词:functioning过去分词:functioned过去式:functioned名词功能,作用; 应变量,函数; 职务; 重大聚会不及物动词有或起作用; 行使职责相关例句不及物动词1. The sofa functions as a bed at night.这沙发在夜里可以当床。
2. The refrigerator is not functioning well.冰箱有点问题。
名词1. What is his function on the committee?他在委员会里担任职务?2. I attended many social functions while working abroad.我在国外工作期间出席了许多社交会。
3. The teacher did not explain its grammatical function.老师没有解释它的文法功能。
function的单语例句1. By " function " I mean presenting great art on its three stages.2. Their operators have to abide by them by the book if these weapons are to function normally and safely.3. These can effectively activate the immune system of human body and promote the immune function of cells and body fluid.4. Hu also called for scientifically regulating the relationship between Party committees and people's congresses, and to support people's congresses to function according to law.5. While the more religious individuals were more physically active and also less likely to smoke, these differences didn't account for their better lung function.6. I cannot help but wonder what the function of a new Yuanmingyuan would be.7. " Drought and environmental degradation have hampered the reservoir's water holding capacity and supply function, " Wang said.8. Cardiomyopathy is a disease in which the heart muscle becomes inflamed and doesn't function properly.9. Endothelial function is a measure of the activity of endothelial cells that line the inside of the blood vessels.10. The degree to which exposure to these contaminants suppresses immune system function has been " underestimated, " Carpenter added.function的词典解释1. 功能;作用;职责The function of something or someone is the useful thing that they do or are intended to do.e.g. The main function of the merchant banks is to raise capital for industry.商业银行的'主要职能是为产业融资。
function可数吗

function可数吗
"Function" 作为一个词,既可以作为可数名词,也可以作为不可数名词。
作为可数名词,"function" 可以指代一个具体的、特定的功能或职责。
例如,在编程中,"function" 指的是一段具有特定目的的代码块,可以执行一个特定的任务。
在数学中,"function" 表示一个变量与另一个变量之间的关系。
另一方面,"function" 作为不可数名词时,通常用于描述一个对象、设备或系统的整体功能或作用。
例如,"The television is a multi-function device",这里"function" 指的是电视机的多种功能。
因此,"function" 的可数或不可数取决于上下文和用法。
一般来说,当它指的是一个具体的、特定的功能或职责时,它是一个可数名词;当它描述一个对象、设备或系统的整体功能或作用时,它是一个不可数名词。
Python函数编程案例
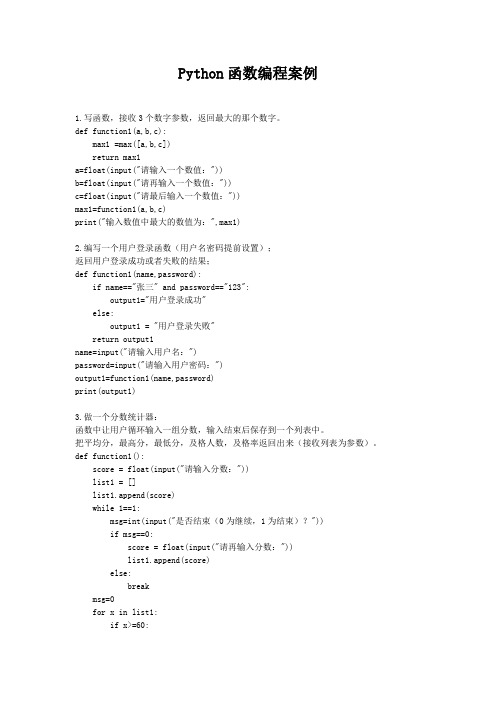
Python函数编程案例1.写函数,接收3个数字参数,返回最大的那个数字。
def function1(a,b,c):max1 =max([a,b,c])return max1a=float(input("请输入一个数值:"))b=float(input("请再输入一个数值:"))c=float(input("请最后输入一个数值:"))max1=function1(a,b,c)print("输入数值中最大的数值为:",max1)2.编写一个用户登录函数(用户名密码提前设置);返回用户登录成功或者失败的结果;def function1(name,password):if name=="张三" and password=="123":output1="用户登录成功"else:output1 = "用户登录失败"return output1name=input("请输入用户名:")password=input("请输入用户密码:")output1=function1(name,password)print(output1)3.做一个分数统计器:函数中让用户循环输入一组分数,输入结束后保存到一个列表中。
把平均分,最高分,最低分,及格人数,及格率返回出来(接收列表为参数)。
def function1():score = float(input("请输入分数:"))list1 = []list1.append(score)while 1==1:msg=int(input("是否结束(0为继续,1为结束)?"))if msg==0:score = float(input("请再输入分数:"))list1.append(score)else:breakmsg=0for x in list1:if x>=60:msg=msg+1aver = sum(list1)/len(list1)max1 = max(list1)min1 = min(list1)num1 = msgaver1 = msg/len(list1)*100return aver,max1,min1,num1,aver1aver,max1,min1,num1,aver1=function1()print("平均分为:",round(aver,2),"分,最高分为:",round(max1,2),"分,最低分为:",round(min1,2),"分,及格人数为:",num1,"人,及格率为:",round(aver1,2))。
vue在多方法执行完后再执行另一个方法(等待请求完数据再执行)

vue在 多 方 法 执 行 完 后 再 执 行 另 一 个 方 法 ( 等 待 请 求 完 数 据 再 执 行)
vue在一个方法执行完后执行另一个方法
用Promise.all来实现。 Promise是ES6的新特性,用于处理异步操作逻辑,用过给Promise添加then和catch函数,处理成功和失败的情况
ES7中新提出async搭配await,建议使用async搭配await。 使用方法:
示例:
function fun1(){ return new Promise((resolve, reject) => { /* 你的逻辑代码 */ console.log("1"); });
}, function fun2(){
return new Promise((resolve, reject) => { /* 你的逻辑代码 */ console.log("2");
}); }, function fun3(){
rห้องสมุดไป่ตู้turn new Promise((resolve, reject) => { /* 你的逻辑代码 */ console.log("3");
}); }, /* 调用 */ function run(){
Promise.all([ this.fun1(), this.fun2(), this.fun3()
]).then(res => { /* 你的逻辑代码 */ console.log("run");
}) }
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 ABS(绝对值) AND AVERAGE COLUMN CONCATENATE COUNTIF(条件计数) DATEБайду номын сангаасDATEDIF DAY DCOUNT FREQUENCY IF(条件语句) INDEX INT ISERROR LEFT(左边取值)
LEN(计算文本字符长度(含空格)
实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例
19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
MAX MID(取中间值) MIN MOD MONTH NOW OR RANK(成绩排序等) RIGHT(从右边取值) SUBTOTAL SUM SUMIF TEXT TODAY VALUE VLOOKUP(查找) WEEKDAY TRIM(清除非打印乱码) LEN(字符串的长度) EXACT(字符相同否) If countif (查相同)
INDEX返回表或区域中的值或值的引用
实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例 实例
MATCH(定位)
Good ISBLANK(数据透视后填充)
实例
实例