7_Collection_and_Map
collection 用法

Collection 用法什么是 collection在计算机编程的领域里,collection(集合)是指把一组元素组织在一起的数据结构。
它可以用来存储和操作多个相关的元素,比如数字、字符串、对象等。
在不同的编程语言中,collection 有不同的实现方式和特点。
Collection 的类型常见的 collection 类型包括列表(list)、元组(tuple)、集合(set)和字典(dictionary)。
下面将对每种类型进行详细介绍。
列表(List)列表是一种有序的集合,可以包含任意类型的元素。
列表中的元素可以根据需要进行增加、删除和修改。
创建列表在大多数编程语言中,可以使用方括号([])来创建一个列表。
例如,在Python 中,可以使用以下代码创建一个包含整数的列表:numbers = [1, 2, 3, 4, 5]访问列表元素要访问列表中的元素,可以使用下标(index)来引用元素的位置。
在大多数编程语言中,列表的下标从0开始。
例如,在上面的列表中,要访问第一个元素(1),可以使用以下代码:first_number = numbers[0]列表的操作列表支持多种操作,包括向列表中添加元素(append())、删除元素(remove())和修改元素值。
例如,在Python中,可以使用以下代码示例来演示这些操作:numbers.append(6) # 向列表末尾添加元素numbers.remove(3) # 删除列表中的某个元素numbers[0] = 10 # 修改列表中的元素值元组(Tuple)元组是一种不可变的有序集合,可以包含任意类型的元素。
元组一经创建,其元素及其顺序不能改变。
创建元组在大多数编程语言中,可以使用圆括号(())来创建一个元组。
例如,在Python 中,可以使用以下代码创建一个包含整数和字符串的元组:person = (1, 'Alice', 25)访问元组元素访问元组中的元素与列表的访问方式相似,同样使用下标来引用元素的位置。
collections java方法
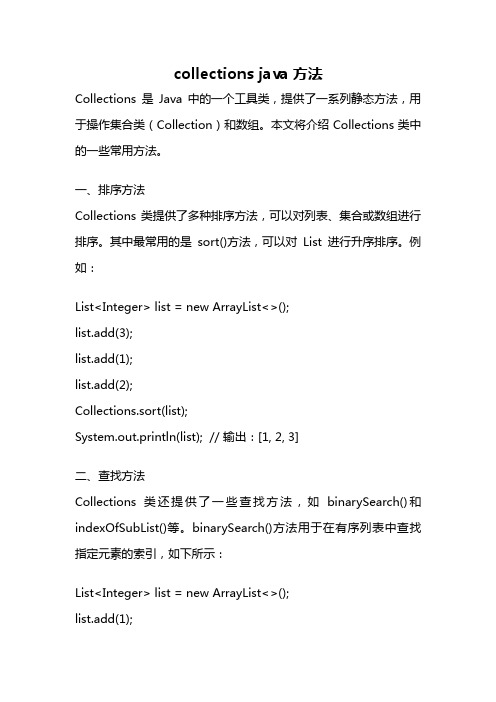
collections java方法Collections是Java中的一个工具类,提供了一系列静态方法,用于操作集合类(Collection)和数组。
本文将介绍Collections类中的一些常用方法。
一、排序方法Collections类提供了多种排序方法,可以对列表、集合或数组进行排序。
其中最常用的是sort()方法,可以对List进行升序排序。
例如:List<Integer> list = new ArrayList<>();list.add(3);list.add(1);list.add(2);Collections.sort(list);System.out.println(list); // 输出:[1, 2, 3]二、查找方法Collections类还提供了一些查找方法,如binarySearch()和indexOfSubList()等。
binarySearch()方法用于在有序列表中查找指定元素的索引,如下所示:List<Integer> list = new ArrayList<>();list.add(1);list.add(2);list.add(3);int index = Collections.binarySearch(list, 2);System.out.println(index); // 输出:1三、反转方法Collections类的reverse()方法可以用于反转List中元素的顺序。
例如:List<Integer> list = new ArrayList<>();list.add(1);list.add(2);list.add(3);Collections.reverse(list);System.out.println(list); // 输出:[3, 2, 1]四、填充方法Collections类的fill()方法可以将List中的所有元素替换为指定的元素。
collection的用法

collection的用法collection是一个英语单词,意思是“集合”或“收藏品”,它在计算机领域中也有广泛的应用。
在编程中,collection通常指的是一组数据元素的容器,可用于存储、检索和操作数据。
常见的collection包括List、Set、Map等。
在Java编程中,collection是一个非常重要的概念,Java提供了丰富的collection类库,包括java.util包下的List、Set、Map 等。
这些类可以用来存储和操作不同类型的数据,如字符串、数字、对象等。
List是一种有序的集合,它可以存储重复的元素。
常见的List 包括ArrayList和LinkedList。
Set是一种无序的集合,它不能包含重复的元素。
常见的Set包括HashSet和TreeSet。
Map是一种键值对的集合,它可以存储重复的value,但不允许重复的key。
常见的Map包括HashMap和TreeMap。
使用collection时,需要先创建一个集合对象,然后向其中添加元素或从中删除元素。
可以使用for循环或迭代器来遍历集合中的元素。
除了基本的添加、删除、查找操作之外,collection还提供了一些高级操作,如排序、查找最大值、查找最小值等。
在使用collection时,需要注意集合的数据类型和容量,避免数据类型不匹配或容量不足的问题。
此外,为了提高程序的效率,可以使用集合的子类或自定义集合类,实现更高效的数据操作。
综上所述,collection是Java编程中非常重要的一个概念,它可以帮助我们更方便、高效地操作数据。
了解和掌握collection的用法,将有助于提高我们的编程能力和效率。
collection底层原理

collection底层原理collection是Java中提供的一个顶层接口,它定义了一组集合操作的共通方法和规则,同时也是Java集合类的带头大哥。
它的底层原理主要依赖于数据结构和算法。
首先,我们来看java.util包下的Collection接口与其派生的子接口List、Set。
List是有序的、可以重复的集合,其中的元素存储的顺序就是它们在集合中的顺序;Set是无序的、不可重复的集合。
在Java中,我们使用这些集合类来管理数据的时候,底层会根据数据的特性采用不同的数据结构来实现。
ArrayList是一种动态数组,它提供了快速的随机访问能力,但是插入和删除元素需要移动其它元素,所以速度较慢;而LinkedList则是双向链表,插入和删除元素速度非常快,但是读取元素时需要遍历整个链表,速度较慢。
接着,我们来看一下Map接口及其实现类HashMap、TreeMap。
Map是一组成对的“键值对”数据,每一个“键值对”都是一个Entry对象,其中包含key和value两个属性;HashMap实现了基于哈希表的 Map接口,它通过哈希算法来快速定位元素,插入、删除、查找元素效率都非常高;而TreeMap则是一种基于红黑树(Balanced Tree)的实现,也就是平衡二叉树,通过红黑树的特性能够使元素在集合中有序存储,并且查找元素的效率也非常高。
最后,我们来看一下Java集合类的性能分析。
通常,对于集合中的元素数量很多,或者集合内元素的类型和值域均比较简单的情况下,我们可能更希望使用基于数组的List实现,如ArrayList,在执行随机访问、修改等操作上有着非常快的速度;而对于集合中的元素数量不太多,元素较为复杂、类型不同的情况下,我们可能会选择基于链表的List实现,如LinkedList,在元素的插入、删除等操作上有非常高的效率。
总之,Java中的集合类为我们提供了非常灵活、高效的数据管理方案,具有可扩展、易维护的优点。
小学上册第14次英语第4单元期末试卷(含答案)

小学上册英语第4单元期末试卷(含答案)英语试题一、综合题(本题有100小题,每小题1分,共100分.每小题不选、错误,均不给分)1.What do we call the marks on a map that represent directions?A. ScaleB. Compass RoseC. LegendD. Grid答案: B2.My uncle is a skilled __________ (手工艺人).3.I have a wonderful _____ (老师).4. A ________ (山谷) is often fertile and green.5.The process of a liquid turning into a gas is called ______.6. A circuit must be _______ for electricity to flow.7.I have a robot ____ that does tricks. (玩具名称)8.I have a ___ (collection) of stickers.9.The gazelle can leap very _________ (远).10.He is a _____ (政治家) advocating for change.11.The __________ (水利工程) help manage resources.12.What do we call the study of the atmosphere and weather?A. MeteorologyB. GeographyC. AstronomyD. Climatology答案:A. Meteorology13.I want to help protect the _______ (环境). It’s important for future _______ (世代).14.The _____ is a star that can be seen during the day.15.The capital of Japan is _______.16.The chemical formula for ammonium sulfate is ______.17.我的朋友喜欢 _______ (活动). 她觉得这很 _______ (形容词)18. A _______ change can be reversed, but a chemical change cannot.19.The first successful human flight in space occurred in ________.20.I can’t believe how cool my __________ (玩具名) is!21.I think every toy has its own ________ (名词) and personality.22. A ____(public-private partnership) leverages resources for community benefit.23.The term "combustion" refers to a reaction that produces _______.24.The capital of Georgia is __________.25.What do you call the center of an atom?A. NeutronB. ProtonC. NucleusD. Electron答案:C26.I love _______ (去野餐) in the summer.27.The _____ (种植合作社) supports local farmers.28.Did you hear the _____ (小狗) yapping excitedly?29.My cat chases after ______ (小虫子).30.I love _______ (去图书馆).31.I love listening to __________ music because it makes me feel __________. My favorite singer is __________. I like to sing along to his/her songs when I am__________.32.I enjoy _____ with my friends. (playing)33. A whale is a type of ______ that lives in the ocean.34.I enjoy playing ________ games on my tablet.35. A __________ is a landform that rises significantly above its surroundings.36.I have a new ___. (shirt)37.I collect _______ (名词) as a hobby. Each item has its own _______ (故事).38.The _____ (车站) is busy.39. A __________ is a type of bond formed by sharing electrons.40.ts are used in ______ for their medicinal properties. (某些植物因其药用特性而用于传统医学。
小学六年级下册第3次英语第一单元期中试卷(答案和解释)

小学六年级下册英语第一单元期中试卷(答案和解释)英语试题一、综合题(本题有50小题,每小题2分,共100分.每小题不选、错误,均不给分)1.Which of these is used for cutting?A. ForkB. SpoonC. KnifeD. Plate2.What do we use to drink water?A. ForkB. KnifeC. CupD. Plate3.My father ______ a doctor.A. isB. areC. amD. be4.What is your favorite color?A. DogB. YellowC. MilkD. Chair5.My school is very __________. It has a big __________ and many __________. I like my __________ because it is very __________. There are many __________ in the classroom, and I sit by the __________. We learn __________ and __________ in the morning, and __________ in the afternoon. After school, I go home and do my__________.6.Which of these is a wild animal?A. DogB. CowC. ElephantD. Lion7.Which of the following is an animal?A. DogB. TableC. BookD. Chair8.What do we use to drink water?A. PlateB. ForkC. CupD. Knife9.Which one is a planet?A. MoonB. EarthC. SunD. Star10.Which of these is a color?A. GreenB. ChairC. DogD. Spoon11.Lily and Jack are at the beach. They are building a __________. Jack is digging a__________ while Lily is collecting __________ to decorate the castle. After finishing, they sit on a __________ and enjoy some __________.12.Which one is a type of weather?A. SunnyB. SpoonC. PlateD. Chair13.Lucy loves to read books about __________ and magic. Her favorite character is a__________ who can fly. She has a collection of __________ about wizards and fairies. Every night before bed, Lucy reads a chapter from her __________ to help her fall asleep.14.He ______ like ice cream.A. dontB. doesntC. notD. isnt15.What is the time when the sun is highest in the sky?A. MorningB. NoonC. AfternoonD. Evening16.Jack and his family are going on a road trip. They pack their __________ (suitcases) and put them in the __________ (car). Jack’s dad checks the __________ (map) to find the best __________ (route) for their trip. They stop at a __________ (gas station) to fill up the car with __________ (gas).17.Mark is at the __________ with his family. They are watching a __________ about animals. Mark loves to watch __________, and today, they are watching a movie about__________. During the movie, they eat __________ and drink __________.18.Which of these is a season?A. SummerB. MondayC. JanuaryD. April19.Which season comes after winter?A. AutumnB. SpringC. SummerD. Winter again20.Every Saturday, Lily goes to the __________ (1) to buy fresh fruit. Today, she bought some red __________ (2) and green __________ (3) to make a fruit salad. Her mother is going to make a delicious __________ (4) with the fruits for dessert. After theyfinished preparing the salad, Lily’s family sat at the __________ (5) and ate together. After dinner, Lily helped her mom __________ (6) the kitchen.21.I have a garden in my house. Every day, I water the flowers and __. My mom planted some vegetables like tomatoes, carrots, and __. We take care of the plants by giving them enough water and sunlight. Sometimes, I help my mom pick the vegetables when they are ready. It’s fun to grow our own food.22.Lucy is at the beach with her family. She is building a __________ (1) with her brother. They use __________ (2) to make the walls strong and __________ (3) to decorate it. After building, they go for a __________ (4) in the water.23.Which one is a color?A. TreeB. BlueC. DogD. Apple24.Every morning, I __________ (get) up at 6:30 and __________ (brush) my teeth. After that, I __________ (have) breakfast with my family. We __________ (eat) eggs, bread, and fruit. Then, I __________ (leave) for school at 7:30.25.Which of these is a shape?A. CircleB. CarC. TableD. Spoon26.Which of these is a tool used to cut things?A. KnifeB. SpoonC. PlateD. Fork27.David is visiting his grandparents. He brings his favorite __________ with him. His grandmother gives him a warm __________ to wear, and they go for a walk outside. David sees some __________ in the yard and tries to catch them. He feels __________ because he loves spending time with his grandparents.28.We went to the zoo last weekend and saw many animals. My favorite animal was the __. It was so big and strong. We also saw a cute little __ playing with its friends. After that, we visited the __ and watched them swim. It was a fun day at the zoo!29.What is 2 plus 3?A. 4B. 5C. 6D. 730.What’s the weather like today?A. It’s raining.B. It’s a cat.C. It’s a book.D. It’s Monday.31.Which one is a color?A. GreenB. SpoonC. PlateD. Knife32.Which of these is a color?A. RedB. CarC. ChairD. Dog33.Which one is a vegetable?A. BananaB. CarrotC. CherryD. Orange34.At the weekend, my family likes to go to the ______ to see a movie. We watch the latest ______ movie, which is very exciting. After the movie, we eat some ______ and drink cold ______. I think watching movies is a fun way to relax together as a family!35.Which of these is a body part?A. LegB. ChairC. SpoonD. Table36.What is the opposite of "fast"?A. SlowB. HighC. LightD. Heavy37.Which of these is a day of the week?A. SundayB. RedC. ChairD. Car38.I __________ (not understand) the math lesson yesterday, so I __________ (ask) my friend for help. She __________ (explain) everything very clearly. After that, I__________ (feel) more confident. Later, we __________ (do) some practice problems together. I __________ (realize) that I __________ (get) better at math after all the practice.39.Which of the following is an example of a fruit?A. LettuceB. CarrotC. AppleD. Potato40.Which of these is not a color?A. BlueB. GreenC. DogD. Red41.What is the opposite of "fast"?A. SlowB. QuickC. FastestD. Speed42.Which of these is a season?A. WinterB. ChairC. SpoonD. Dog43.Which of these is a cold drink?A. LemonadeB. SoupC. CoffeeD. Tea44.They _______ (not/understand) the lesson because they _______ (not/listen) carefully to the teacher.45.Which one is the correct sentence?A. He don’t like pizzA.B. He doesn’t like pizzA.C. He not like pizzA.D. He no like pizzA.46.Which one is a kind of fruit?A. CarrotB. AppleC. PotatoD. Onion47.Which one is a vegetable?A. CarrotB. ChickenC. AppleD. Banana48.What is the opposite of "hot"?A. WarmB. ColdC. SoftD. Wet49.We ______ (study) math every day. Our teacher ______ (give) us homework at the end of each lesson. Yesterday, I ______ (finish) my homework early, so I ______ (have) some free time. I ______ (play) with my friends after school. We ______ (enjoy) playing soccer together.50.What is the opposite of "happy"?A. SadB. AngryC. ExcitedD. Sleepy(答案及解释)。
collections.sort 排序规则

Collections.sort排序规则一、介绍Collections是Java中的一个工具类,它提供了一系列静态方法来操作集合对象。
其中,sort方法可以对List集合进行排序操作。
在使用Collections.sort方法时,我们需要传入一个Comparator对象来指定排序规则。
本文将介绍Collections.sort的排序规则。
二、基本用法在使用Collections.sort方法时,通常需要在参数中指定一个Comparator对象。
Comparator是一个函数式接口,我们可以使用Lambda表达式或者匿名类来创建Comparator对象。
Comparator 接口中定义了一pare方法,用于比较两个对象的大小关系。
根pare 方法的返回值来决定排序的顺序。
pare方法返回值小于0时,表示第一个对象小于第二个对象;当返回值等于0时,表示两个对象相等;当返回值大于0时,表示第一个对象大于第二个对象。
三、排序规则在编写Comparator对象时,我们可以根据具体的需求来定义排序规则。
下面是一些常见的排序规则:1. 升序排序当需要对集合进行升序排序时,我们可以简单地实现一个Comparator对象,比较两个对象的大小关系,并根据大小关系返回相应的值。
例如:```javaList<String> list = new ArrayList<>();list.add("apple");list.add("banana");list.add("cherry");Collections.sort(list, (s1, s2) -> s1pareTo(s2));System.out.println(list); // 输出:[apple, banana, cherry]```在这个例子中,我们使用了Lambda表达式来创建Comparator对象,该Comparator对象使用StringpareTo方法来比较两个字符串的大小关系,从而实现升序排序。
小学下册P卷英语第1单元测验试卷
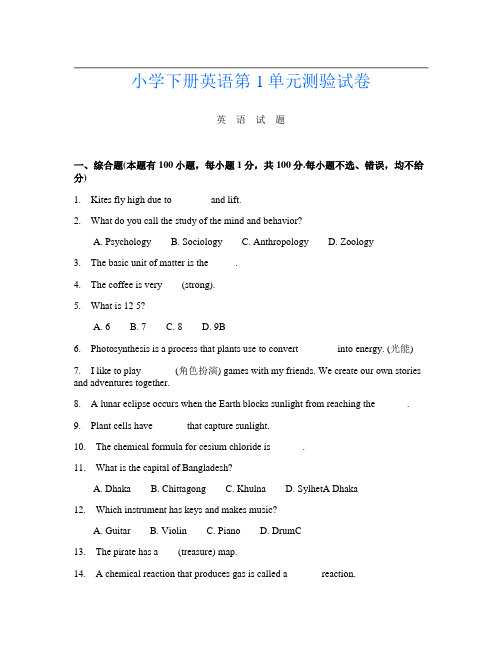
小学下册英语第1单元测验试卷英语试题一、综合题(本题有100小题,每小题1分,共100分.每小题不选、错误,均不给分)1.Kites fly high due to _______ and lift.2.What do you call the study of the mind and behavior?A. PsychologyB. SociologyC. AnthropologyD. Zoology3.The basic unit of matter is the _____.4.The coffee is very ___ (strong).5.What is 12 5?A. 6B. 7C. 8D. 9B6.Photosynthesis is a process that plants use to convert _______ into energy. (光能)7.I like to play ______ (角色扮演) games with my friends. We create our own stories and adventures together.8. A lunar eclipse occurs when the Earth blocks sunlight from reaching the ______.9.Plant cells have ______ that capture sunlight.10.The chemical formula for cesium chloride is ______.11.What is the capital of Bangladesh?A. DhakaB. ChittagongC. KhulnaD. SylhetA Dhaka12.Which instrument has keys and makes music?A. GuitarB. ViolinC. PianoD. DrumC13.The pirate has a ___ (treasure) map.14. A chemical reaction that produces gas is called a ______ reaction.15.The cake is _______ and delicious.16. A sound wave can be either _____ or transverse.17.I have a collection of _______ (我有一个_______的收藏).18.What do you call the time when it is dark outside?A. DaytimeB. NighttimeC. AfternoonD. MorningB19.I planted a __________ (花坛) in my backyard.20.The ancient Mayans were known for their ________ and astronomy.21.__________ help plants absorb water and nutrients from the soil.22.在中国,古代的________ (families) 注重传承与教育。
Java集合排序及java集合类详解(Collection、List、Map、Set)
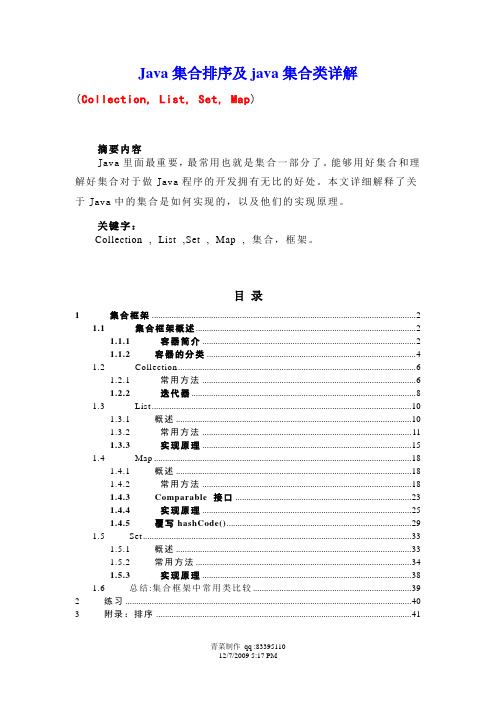
Java集合排序及java集合类详解(Collection, List, Set, Map)摘要内容Java里面最重要,最常用也就是集合一部分了。
能够用好集合和理解好集合对于做Java程序的开发拥有无比的好处。
本文详细解释了关于Java中的集合是如何实现的,以及他们的实现原理。
关键字:Collection , List ,Set , Map , 集合,框架。
目录1 集合框架 (2)1.1 集合框架概述 (2)1.1.1 容器简介 (2)1.1.2 容器的分类 (4)1.2 Collection (6)1.2.1 常用方法 (6)1.2.2 迭代器 (8)1.3 List (10)1.3.1 概述 (10)1.3.2 常用方法 (11)1.3.3 实现原理 (15)1.4 Map (18)1.4.1 概述 (18)1.4.2 常用方法 (18)1.4.3 Comparable 接口 (23)1.4.4 实现原理 (25)1.4.5 覆写hashCode() (29)1.5 Set (33)1.5.1 概述 (33)1.5.2 常用方法 (34)1.5.3 实现原理 (38)1.6 总结:集合框架中常用类比较 (39)2 练习 (40)3 附录:排序 (41)1集合框架1.1集合框架概述1.1.1容器简介到目前为止,我们已经学习了如何创建多个不同的对象,定义了这些对象以后,我们就可以利用它们来做一些有意义的事情。
举例来说,假设要存储许多雇员,不同的雇员的区别仅在于雇员的身份证号。
我们可以通过身份证号来顺序存储每个雇员,但是在内存中实现呢?是不是要准备足够的内存来存储1000个雇员,然后再将这些雇员逐一插入?如果已经插入了500条记录,这时需要插入一个身份证号较低的新雇员,该怎么办呢?是在内存中将500条记录全部下移后,再从开头插入新的记录? 还是创建一个映射来记住每个对象的位置?当决定如何存储对象的集合时,必须考虑如下问题。
collections的用法

collections的用法`collections` 是Python 标准库中的一个模块,提供了一些额外的数据结构类型,是对内置数据结构的扩展。
以下是一些常用的`collections` 模块中的数据结构及其用法:1. Counter(计数器):`Counter` 用于统计可迭代对象中各元素的出现次数,返回一个字典。
例如:```pythonfrom collections import Countermy_list = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]my_counter = Counter(my_list)print(my_counter)# 输出: Counter({4: 4, 3: 3, 2: 2, 1: 1})```2. defaultdict(默认字典):`defaultdict` 是`dict` 的子类,允许给字典的键指定默认值。
这样,即使键不存在,也不会抛出`KeyError`。
```pythonfrom collections import defaultdictmy_dict = defaultdict(int)my_dict['a'] += 1my_dict['b'] += 2print(my_dict)# 输出: defaultdict(<class 'int'>, {'a': 1, 'b': 2})```3. deque(双端队列):`deque` 是一个双向队列,支持在队列两端高效地进行元素的添加和弹出。
```pythonfrom collections import dequemy_deque = deque([1, 2, 3])my_deque.append(4) # 在右侧添加元素my_deque.appendleft(0) # 在左侧添加元素print(my_deque)# 输出: deque([0, 1, 2, 3, 4])```4. namedtuple(命名元组):`namedtuple` 创建一个带有字段名的元组,可以像类属性一样访问。
collection 用法

collection 用法Collection在英语中的意思是“收集”,在计算机科学中是一个非常常见的术语。
它是Java语言中的一个接口,用来表示一组对象,这些对象被称作元素。
Collection接口为许多常见的数据结构定义了通用操作,如添加、删除和遍历元素,提供了一种方便和统一的方式来操作这些数据结构。
Collection接口有两个子接口:List和Set。
List接口定义了一个序列,我们可以通过指定的索引访问其中的元素。
Set接口定义了一组不重复的元素,而且没有涉及到索引的概念。
除此之外,Collection还有自己的一些完整实现,如ArrayList、LinkedList、HashSet和TreeSet 等。
使用Collection可以来完成很多任务,如查找重复元素、获取元素个数、找出最大/最小元素等。
下面列举了一些Collection接口的使用示例:1. 创建一个ListList<String> list = new ArrayList<>();list.add("apple");list.add("banana");list.add("orange");2. 遍历一个Listfor (String fruit : list) {System.out.println(fruit);}3. 创建一个SetSet<Integer> set = new HashSet<>(); set.add(1);set.add(2);set.add(3);4. 判断Set是否包含某个元素if (set.contains(3)) {System.out.println("Set contains 3"); }5. 获取List中的元素个数int size = list.size();System.out.println("List contains " + size + " elements");6. 获取Set中的最大/最小值int max = Collections.max(set);int min = Collections.min(set);System.out.println("Max value: " + max);System.out.println("Min value: " + min);总之,Collection接口是Java集合框架中的一个重要组成部分。
kotlin _collection的map方法 -回复
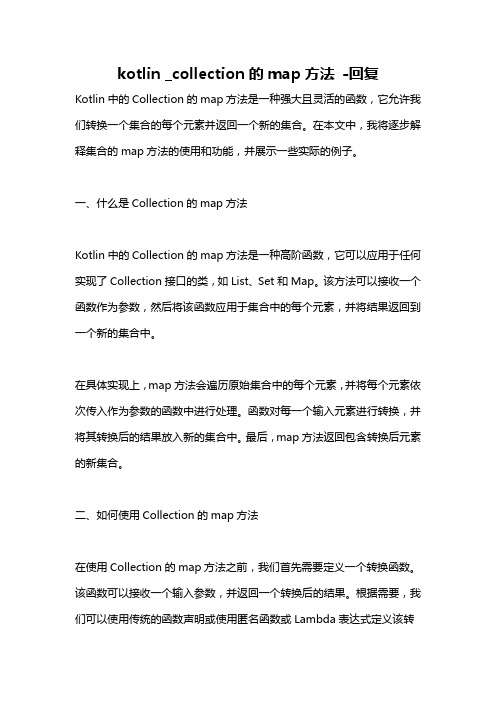
kotlin _collection的map方法-回复Kotlin中的Collection的map方法是一种强大且灵活的函数,它允许我们转换一个集合的每个元素并返回一个新的集合。
在本文中,我将逐步解释集合的map方法的使用和功能,并展示一些实际的例子。
一、什么是Collection的map方法Kotlin中的Collection的map方法是一种高阶函数,它可以应用于任何实现了Collection接口的类,如List、Set和Map。
该方法可以接收一个函数作为参数,然后将该函数应用于集合中的每个元素,并将结果返回到一个新的集合中。
在具体实现上,map方法会遍历原始集合中的每个元素,并将每个元素依次传入作为参数的函数中进行处理。
函数对每一个输入元素进行转换,并将其转换后的结果放入新的集合中。
最后,map方法返回包含转换后元素的新集合。
二、如何使用Collection的map方法在使用Collection的map方法之前,我们首先需要定义一个转换函数。
该函数可以接收一个输入参数,并返回一个转换后的结果。
根据需要,我们可以使用传统的函数声明或使用匿名函数或Lambda表达式定义该转换函数。
以下是一个使用Collection的map方法的基本语法:collection.map { element -> transform(element) }在上述语法中,collection表示一个具体的集合,element表示集合中的每个元素,transform表示转换函数。
更具体地说,我们可以通过以下步骤来使用Collection的map方法:1. 首先,创建一个原始的集合(如List或Set),并填充一些元素。
2. 定义一个转换函数,该函数可以接收一个元素作为参数,并返回一个转换后的结果。
3. 使用map方法对集合进行转换,并将转换函数作为参数传递给map 方法。
4. 遍历新的集合,查看转换结果是否正确。
三、实际例子让我们通过一些实际的例子来演示Collection的map方法的用法和功能。
map中collect用法
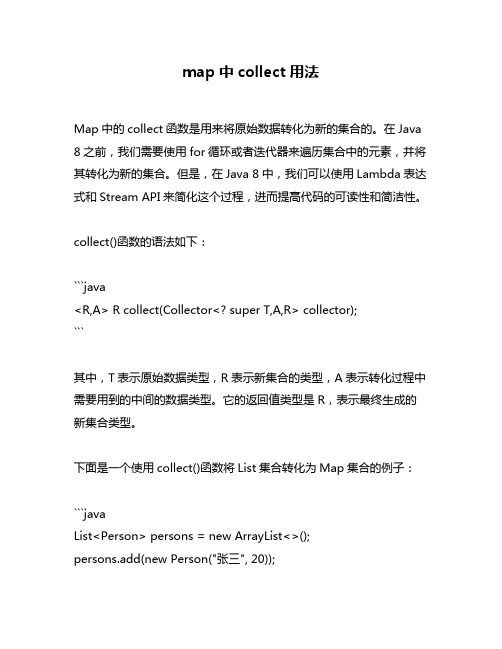
map中collect用法Map中的collect函数是用来将原始数据转化为新的集合的。
在Java 8之前,我们需要使用for循环或者迭代器来遍历集合中的元素,并将其转化为新的集合。
但是,在Java 8中,我们可以使用Lambda表达式和Stream API来简化这个过程,进而提高代码的可读性和简洁性。
collect()函数的语法如下:```java<R,A> R collect(Collector<? super T,A,R> collector);```其中,T表示原始数据类型,R表示新集合的类型,A表示转化过程中需要用到的中间的数据类型。
它的返回值类型是R,表示最终生成的新集合类型。
下面是一个使用collect()函数将List集合转化为Map集合的例子:```javaList<Person> persons = new ArrayList<>();persons.add(new Person("张三", 20));persons.add(new Person("李四", 25));persons.add(new Person("王五", 30));Map<String, Integer> ageMap = persons.stream().collect(Collectors.toMap(Person::getName,Person::getAge));System.out.println(ageMap);```在上面的例子中,我们将存储Person对象的List集合转化为一个以name作为键,age作为值的Map集合。
在collect()函数中,我们使用了toMap()方法,它返回一个收集器(Collector)对象,将原始数据中的每一个元素转化为Map中的一个键值对。
resultmap collection用法

resultmap collection用法一、概述Mybatis是一款优秀的ORM框架,它使用XML或注解配置映射关系,将Java对象与数据库表进行映射。
其中,resultmap和collection是Mybatis中非常重要的两个标签。
本文将详细介绍resultmap和collection的用法。
二、resultmap的用法1.定义resultmap在Mybatis中,resultmap用于将查询结果集中的列与Java对象属性进行映射。
定义resultmap时需要指定id、type和column等属性。
示例代码:```<resultMap id="userMap" type="er"><id column="id" property="userId"/><result column="username" property="userName"/><result column="password" property="password"/></resultMap>```其中,id属性指定了该resultmap的唯一标识;type属性指定了映射结果所对应的Java类;column属性指定了查询结果集中对应列的名称;property属性指定了Java对象中对应属性的名称。
2.使用resultmap在SQL语句中使用select标签时,可以通过refid引用已经定义好的resultmap。
示例代码:```<select id="getUserById" resultMap="userMap">select * from user where id=#{userId}</select>```其中,getUserById为该select语句的唯一标识;resultMap属性引用了已经定义好的userMap。
flutter collection插件用法

flutter collection插件用法============Flutter框架在开发移动应用中具有很高的实用性,而collection 插件则为Flutter应用提供了丰富的数据集合操作功能。
本文将详细介绍flutter collection插件的用法。
一、插件简介-----flutter collection插件是Flutter框架中的一个重要插件,它提供了一系列用于操作数据集合的函数和方法,例如列表(List)、集合(Set)和映射(Map)等。
通过使用collection插件,开发者可以更加方便地处理数据,提高开发效率。
二、安装与导入------要使用flutter collection插件,首先需要在Flutter项目的`pubspec.yaml`文件中添加插件依赖。
在`dependencies`部分添加以下内容:```yamlflutter:uses-material-design: truecollection: ^x.x.x # 使用时请替换为最新版本```其中,`^x.x.x`表示插件的版本号,需要替换为实际安装的版本号。
完成配置后,可以通过运行`flutter pub get`命令来安装插件。
在Dart文件中,可以通过以下方式导入插件:```dartimport 'package:collection/collection.dart';```三、基本用法------使用flutter collection插件,可以通过以下方式创建列表、集合和映射:```dartList<String> list = [“元素1”, “元素2”, “元素3"];Set<int> set = <int>[1, 2, 3];Map<String, S tring> map = {“key1”: “value1”, “key2”: “value2"};```此外,还可以使用插件提供的函数和方法对集合进行操作,例如:* `addAll()`:将一个集合的所有元素添加到另一个集合中。
mybatis中resultmap的collection标签用法
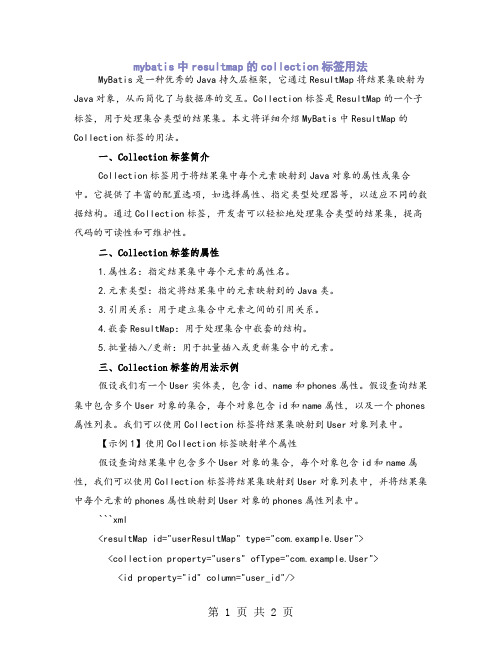
mybatis中resultmap的collection标签用法MyBatis是一种优秀的Java持久层框架,它通过ResultMap将结果集映射为Java对象,从而简化了与数据库的交互。
Collection标签是ResultMap的一个子标签,用于处理集合类型的结果集。
本文将详细介绍MyBatis中ResultMap的Collection标签的用法。
一、Collection标签简介Collection标签用于将结果集中每个元素映射到Java对象的属性或集合中。
它提供了丰富的配置选项,如选择属性、指定类型处理器等,以适应不同的数据结构。
通过Collection标签,开发者可以轻松地处理集合类型的结果集,提高代码的可读性和可维护性。
二、Collection标签的属性1.属性名:指定结果集中每个元素的属性名。
2.元素类型:指定将结果集中的元素映射到的Java类。
3.引用关系:用于建立集合中元素之间的引用关系。
4.嵌套ResultMap:用于处理集合中嵌套的结构。
5.批量插入/更新:用于批量插入或更新集合中的元素。
三、Collection标签的用法示例假设我们有一个User实体类,包含id、name和phones属性。
假设查询结果集中包含多个User对象的集合,每个对象包含id和name属性,以及一个phones属性列表。
我们可以使用Collection标签将结果集映射到User对象列表中。
【示例1】使用Collection标签映射单个属性假设查询结果集中包含多个User对象的集合,每个对象包含id和name属性,我们可以使用Collection标签将结果集映射到User对象列表中,并将结果集中每个元素的phones属性映射到User对象的phones属性列表中。
```xml<resultMap id="userResultMap" type="er"><collection property="users" ofType="er"><id property="id" column="user_id"/><result property="name" column="user_name"/><collection property="phones" ofType="java.util.List"><id property="phoneId" column="phone_id"/><result property="phoneNumber" column="phone_number"/></collection></collection></resultMap>```在上面的示例中,我们将users集合中的每个元素(即User对象)映射到查询结果集中的每行数据上。
collections.newsetfrommap用法
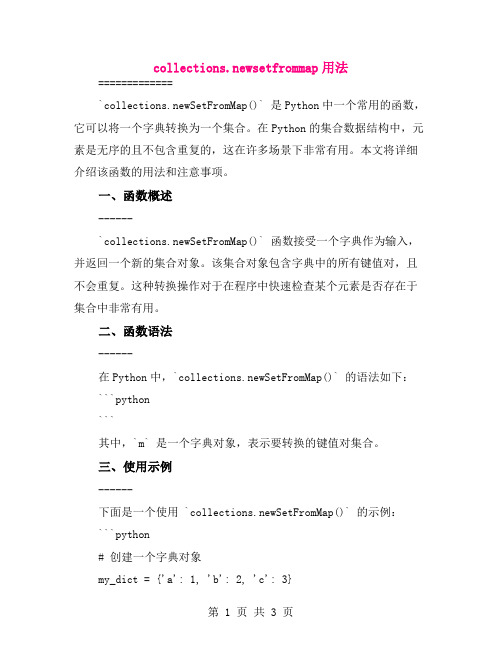
collections.newsetfrommap用法=============`collections.newSetFromMap()` 是Python中一个常用的函数,它可以将一个字典转换为一个集合。
在Python的集合数据结构中,元素是无序的且不包含重复的,这在许多场景下非常有用。
本文将详细介绍该函数的用法和注意事项。
一、函数概述------`collections.newSetFromMap()` 函数接受一个字典作为输入,并返回一个新的集合对象。
该集合对象包含字典中的所有键值对,且不会重复。
这种转换操作对于在程序中快速检查某个元素是否存在于集合中非常有用。
二、函数语法------在Python中,`collections.newSetFromMap()` 的语法如下:```python```其中,`m` 是一个字典对象,表示要转换的键值对集合。
三、使用示例------下面是一个使用 `collections.newSetFromMap()` 的示例:```python# 创建一个字典对象my_dict = {'a': 1, 'b': 2, 'c': 3}# 使用 collections.newSetFromMap() 函数转换字典为集合my_set = collections.newSetFromMap(my_dict)# 输出集合中的元素print(my_set) # 输出: {'a', 'b', 'c'}```在上面的示例中,我们首先创建了一个包含三个键值对的字典`my_dict`。
然后,我们使用 `collections.newSetFromMap()` 函数将其转换为一个集合 `my_set`。
最后,我们输出了集合中的元素。
可以看到,集合中只包含字典中的键值对,且不包含重复元素。
四、注意事项------使用 `collections.newSetFromMap()` 时需要注意以下几点:1. 字典中的键必须是不可变类型(如字符串、数字)或可迭代对象(如列表)。
小学上册T卷英语第二单元期末试卷
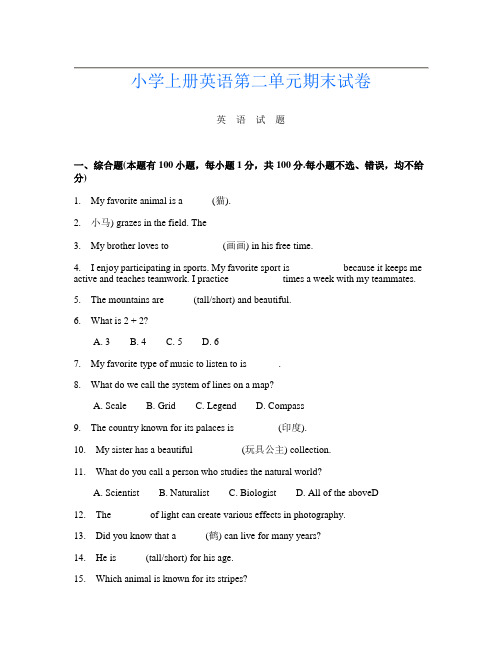
小学上册英语第二单元期末试卷英语试题一、综合题(本题有100小题,每小题1分,共100分.每小题不选、错误,均不给分)1.My favorite animal is a _____ (猫).2.小马) grazes in the field. The ___3.My brother loves to __________ (画画) in his free time.4.I enjoy participating in sports. My favorite sport is __________ because it keeps me active and teaches teamwork. I practice __________ times a week with my teammates.5.The mountains are _____ (tall/short) and beautiful.6.What is 2 + 2?A. 3B. 4C. 5D. 67.My favorite type of music to listen to is ______.8.What do we call the system of lines on a map?A. ScaleB. GridC. LegendD. Compass9.The country known for its palaces is ________ (印度).10.My sister has a beautiful _________ (玩具公主) collection.11.What do you call a person who studies the natural world?A. ScientistB. NaturalistC. BiologistD. All of the aboveD12.The _______ of light can create various effects in photography.13.Did you know that a _____ (鹤) can live for many years?14.He is _____ (tall/short) for his age.15.Which animal is known for its stripes?A. ZebraB. LeopardC. CheetahD. TigerA16.The butterfly's life cycle includes a ________________ (蛹).17.The water is ___. (clear)18.The _____ (植物标本) helps preserve plant species for study.19.My cousin is a ______. She enjoys playing the flute.20.I go to school by _______.21.The fish swims in the _________. (水)22.The ________ (农业创新) drives progress.23. A covalent bond forms when atoms _____ electrons.24.The color change in litmus paper indicates the presence of an ______ or base.25. A ________ (花) blooms in the spring.26.What animal is known for having a long neck?A. RhinoB. GiraffeC. ZebraD. HippoB27.Which season comes after winter?A. SummerB. SpringC. FallD. AutumnB28.My mom is ______ years old. (我妈妈____岁。
collections sort排序原理
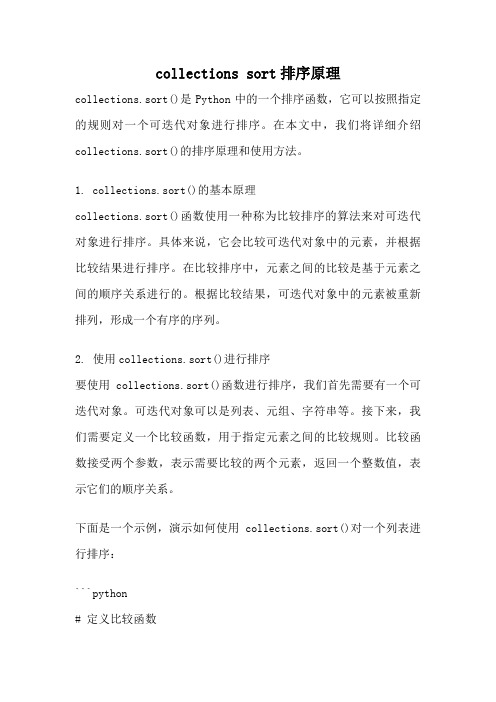
collections sort排序原理collections.sort()是Python中的一个排序函数,它可以按照指定的规则对一个可迭代对象进行排序。
在本文中,我们将详细介绍collections.sort()的排序原理和使用方法。
1. collections.sort()的基本原理collections.sort()函数使用一种称为比较排序的算法来对可迭代对象进行排序。
具体来说,它会比较可迭代对象中的元素,并根据比较结果进行排序。
在比较排序中,元素之间的比较是基于元素之间的顺序关系进行的。
根据比较结果,可迭代对象中的元素被重新排列,形成一个有序的序列。
2. 使用collections.sort()进行排序要使用collections.sort()函数进行排序,我们首先需要有一个可迭代对象。
可迭代对象可以是列表、元组、字符串等。
接下来,我们需要定义一个比较函数,用于指定元素之间的比较规则。
比较函数接受两个参数,表示需要比较的两个元素,返回一个整数值,表示它们的顺序关系。
下面是一个示例,演示如何使用collections.sort()对一个列表进行排序:```python# 定义比较函数def compare(a, b):if a < b:return -1elif a > b:return 1else:return 0# 定义待排序的列表lst = [3, 1, 2, 5, 4]# 使用collections.sort()进行排序collections.sort(lst, compare)# 打印排序结果print(lst)```上述代码中,我们定义了一个比较函数compare,它通过比较两个元素的大小来确定它们的顺序关系。
然后,我们定义了一个待排序的列表lst,并使用collections.sort()对其进行排序。
最后,打印排序结果。
3. collections.sort()的性能分析collections.sort()使用的是一种高效的排序算法,称为快速排序。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
1. Collection<E>接口单词Collection的含义是“收集在一起的一堆东西”,用在Java的SDK中译为“集群”。
Collection是各类“集群”上所有接口的根。
一个collection表示一组对象,这些对象称作该collection的元素。
有些collection容许重复元素存在,有些collection则不容许。
有些collection是有序的,有些collection则不是。
JA V A2的SDK并不提供Collection接口的直接实现,而是提供了更专门的子接口,像Set,List等接口。
真正实现的正是这些子接口,而且同一个子接口往往有几个各具特色的实现。
Collection接口主要用于以最一般的方式操作collection对象。
以下是接口Collection及其子接口List和Set,以及这两个子接口的实现。
虚线框是接口,实线框是类Collection接口定义是public interface Collection<E> extends Iterable<E>这里的接口Iterable<E>中只含有一个方法Iterator<T> iterator()它返回一个T类型集群上的一个迭代器. 方法:添加删除boolean add(E e)boolean addAll(Collection<? extends E> c) void clear()boolean remove(Object o)boolean removeAll(Collection<?> c)逻辑判断boolean contains(Object o) ∈boolean containsAll(Collection<?> c) ⊆boolean retainAll(Collection<?> c) ∩boolean isEmpty()boolean equals(Object o)int hashCode()int size()迭代器Iterator<E> iterator()成数组Object[] toArray()<T> T[] toArray(T[] a)2. List<E>接口public interface List<E> extends Collection <E>boolean add(E e) 尾部添加,增强void add(int index, E element) 新增boolean addAll(Collection<? extends E> c)尾部添加,增强boolean addAll(int index, Collection<? extends E> c)新增E get(int index) 新增int indexOf(Object o)新增Iterator<E> iterator()int lastIndexOf(Object o) 新增ListIterator<E> listIterator()新增ListIterator<E> listIterator(int index)新增E remove(int index)新增E set(int index, E element)新增List<E> subList(int fromIndex, int toIndex) 新增3. Iterator<E>接口boolean hasNext()E next()void remove()4. ListIterator接口boolean hasNext()boolean hasPrevious()E next()E previous()int nextIndex()int previousIndex()void remove()Removes from the list the last element that was returned by next or previousvoid set(E e)Replaces the last element returned by next or previous with the specified elementvoid add(E e) Inserts the specified element into the list. 5. Set接口接口Set继承自Collection。
public interface Set<E> extends Collection <E>Set是一个不含重复元素的Collection。
更形式地说,Set不容许元素e1和e2存在于其中,使e1.equals(e2)为真,并且至多含有一个null。
Set接口模型化了数学上的集合概念。
set接口的方法与Collection接口的方法相同,只是实现接口Set的类的每个对象都表示一个集合,不能含有相同的元素。
需要特别说明的Set方法包括:boolean add(E e)boolean addAll(Collection<? extends E> c)只要当前集合发生变化,返回值即为true.6. Collection接口的实现.Collection接口没有直接被实现。
实际实现的是接口List和Set.List有两个不同的实现,一个是ArrayList,它是用数组实现的List接口。
另一个实现是LinkedList,它是用双向链表实现的List接口。
Set接口也有两个实现,一个是HashSet,它是用hash表实现的Set接口。
另一个是TreeSet它是用红黑树(一种二叉平衡树)实现的Set接口。
ArrayList,LinkedList,HashSet,TreeSet都是泛型类。
7. Map接口.Map的含义是“映射”,用Map命名接口,也正是取它的这个含义。
每个Map类的对象map都可以保存有限个(个数不限)key-value对,而且map不能含有重复的key,每个key 至多可以对应一个值。
因此map把每个key都对应到一个值,这就是把这个接口命名为Map的原因。
实际上map就是形如key-value的有序对的集合。
Map接口提供3个collection views,可以把map的内容分别看成:1.key的集合2.value的集群3.key-value对的集合。
map的collection views上的iterator返回元素的顺序就定义成这个map的顺序。
有些map的实现(如TreeMap类)对顺序有特别的保证,有些实现(如HashMap类)则不然。
注意:如果易变的对象用作key,则要十分小心。
对于一个作为key的对象,如果它的值被改变,而且这个改变影响了equals的比较,那么这个map的行为不被规定。
Collection接口和Map接口功能相似,但是是互相独立的两个接口,相互之间没有继承关系。
方法删除void clear()Removes all mappings from this map (optional operation).V remove(Object key)Removes the mapping for this key from this map if present (optional operation).添加<key,value>V put(K key, V value)把<key,value>插入当前map.void putAll(Map<? extends K,? extends V> m)把map m中的所有<key,value>插入当前map..获取属性值V get(Object key)返回键等于key的有序对<key,value>的value.boolean containsKey(Object key)∈boolean containsValue(Object value)返回true,如果当前map有以value为值的有序对。
.boolean isEmpty()Φint size()三种viewSet<Map.Entry<K,V>> entrySet()返回当前map所有有序对的集合Set<K> keySet()返回当前map所有key的集合Collection<V> values()返回当前map所有value的集群其它boolean equals(Object o)int hashCode()接口Map.Entry ---Map的嵌套接口该接口定义了对map的一个条目的操作方法。
表示一个map 的条目。
Map接口中的entrySet方法返回该Map的一个collection-view,该collection-view的元素就是实现该接口某个类的元素。
只能通过这个collection-view的iterator获取一个map entry 的引用。
这些Map.Entry对象只在迭代期间才合法。
更形式地说,在map entry被iterator返回之后,如果backing map已经被修改,那么这个map entry的行为不定,除非操作是iterator自己执行的remove操作,或者在iterator返回的map entry上的setValue操作。
方法获取key部分,value部分,修改value部分K getKey()返回有序对的key.V getValue()返回有序对的value.V setValue(V value)用value替换有序对的值部分.其它boolean equals(Object o)int hashCode()例.定义student类,含有域1.学号id,int型2.姓名name,String型3.成绩scores,Map型,它的Key是String型,表示课程名,value是Integer型,表示成绩。
构造函数.初始化学号和姓名,同时初始化scores成空map。
方法1.写输入一门课程成绩的方法public void input_score(String subject,int score) 2.写输出各科成绩的方法public void output_score()3.写修改一门课程成绩的方法public int modify_score(String subject,int score) 4.写查找某门课程成绩的方法public int search_score(String subject) 5.写方法String[] getsubjects(int i,int j),返回成绩在i,j之间(含i,j且i<=j)的所有课程名称。