查字典项目的源代码
《C#程序设计(第2版)》电子教案 项目十六 字典查询

项目十六 字典查询分析
字典查询主要的步骤: 1.线程、委托、正规表达式、泛型字典知识 2.字典查询的实现
任务一 线程、委托、泛型知识的学习
1. 线程 2、委托
声明委托 使用委托 匿名委托 3、正规表达式 4、泛型
任务二 字典查询的实现 新建项目,并在窗体上创建字典查询界面和编程。
项目十六 字典查询 小结
学习者创建与电子字典相 似的界面,实现字典查询操作 ,通过本项目,学生能学会了 线程、委托、正规表达式、泛 型等知识的综合运用。
查字典项目的源代码

#include <stdio.h>#include <string.h>#include <stdlib.h>#define BUF_SIZE 4096typedef struct{char *key;int num;char **trans;}word_t;void sys_err(const char *msg){perror(msg);exit(1);}int word_num(const char *filename){FILE *fp;char buf[BUF_SIZE];int total = 0;fp = fopen(filename, "r");if(fp == NULL)sys_err("open file\n");while((fgets(buf, BUF_SIZE, fp)) != NULL) {if(buf[0] == '#')total++;}fclose(fp);return total;}int nword(const char *filename){FILE *fp;char buf[BUF_SIZE];int total = 0;fp = fopen(filename, "r+");if(fp == NULL)sys_err("open file\n");while((fgets(buf, BUF_SIZE, fp)) != NULL) {if(buf[0] == '#')total++;}return total;}word_t *init_word(int total, const char *filename)//将文本写入缓存中{FILE *fp;char buf[BUF_SIZE];int i, j;fp = fopen(filename, "r");if(fp == NULL)sys_err("open file\n");word_t *dict = (word_t *)malloc(total * sizeof(word_t));while((fgets(buf, BUF_SIZE, fp)) != NULL){if(buf[0] == '#'){dict->key = malloc(strlen(buf) - 1);buf[strlen(buf) - 1] = '\0';strcpy(dict->key, buf+1);}else{dict->num = 0;buf[strlen(buf)-1] = '@';for(i = 0; i < strlen(buf); i++)if(buf[i] == '@')dict->num++;dict->trans = (char **)malloc(sizeof(char *) * dict->num);int pos = -1;char *p = buf + 6;for(j = 6; buf[j] != '\0'; j++){if(buf[j] == '@'){pos++;buf[j] = '\0';dict->trans[pos] = (char *)malloc(sizeof(char) * (strlen(p) + 1));strcpy(dict->trans[pos], p);p = buf+ j + 1;}}dict++;}}dict = dict-total;return dict;}int partition(word_t *dict,int start, int end)//排序{int i, last = start;word_t tmp, *pivot;pivot = dict+start;for(i = start+1; i <= end; i++){if(strcmp((dict+i)->key, pivot->key) < 0){last++;tmp = *(dict+i);*(dict + i) = *(dict+last);*(dict+last) = tmp;}}tmp = *(dict +last);*(dict+last) = *(dict+start);*(dict+start) = tmp;return last;}void quicksort(word_t *dict, int start, int end)//排序{int mid;if(end > start){mid = partition(dict, start, end);quicksort(dict, start, mid-1);quicksort(dict, mid+1, end);}}word_t *binarysearch(word_t *dict,char *str, int total)//二分查找{int mid, start = 0, end = total - 1;while(start <= end){mid = (start + end) / 2;if(strcmp((dict+mid)->key, str) < 0)start = mid + 1;else if(strcmp((dict+mid)->key, str) > 0)end = mid - 1;elsereturn dict+mid;}return NULL;}void print(const word_t *dict)//打印单词和解释{int i;printf("---------------------------------\n");printf("word : <%s>\n", dict->key);for(i = 0;i < dict->num; i++)printf("Trans %d: %s\n", i+1,dict->trans[i]);printf("---------------------------------\n");}void insert_new_word(const char *filename)//添加新词到自己的词库{FILE *fp;char buf[BUF_SIZE];fp = fopen(filename, "a+");if(fp == NULL)sys_err("open file\n");printf("Please enter the word like this: (#hello)\n");fgets(buf, BUF_SIZE, stdin);fprintf(fp, "%s", buf);printf("Please enter the trans like this: (Trans:vt nihao@wei)\n");fgets(buf, BUF_SIZE, stdin);fprintf(fp,"%s", buf);fclose(fp);}void usr_txt(char *str,const char *filename)//用户自己的词库查找{int n_word, i;word_t *dict;n_word = nword(filename);dict = init_word(n_word, filename);for(i = 0; i < n_word; i++){if(strcmp(dict[i].key, str) == 0){print(dict+i);break;}}if(i == n_word){char ch[32];printf("This is a new word. can't find in %s.\nDo you want to save it in your word-library ? ( y or n)", filename);fgets(ch, 32, stdin);ch[strlen(ch)-1] = '\0';while(1){if(strcmp(ch, "y") == 0){insert_new_word(filename);break;}else if(strcmp(ch, "n") == 0)break;elseprintf("Undefind command : \"%s\".\nPlease input your choice again :(y or n) ", ch);}}}void print_sys(void){printf("---------------------------------\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* 欢迎使用电子辞典 *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* 1.Enter 2.Quit *\n");printf("* *\n");printf("---------------------------------\n");}int main(int argc, const char *argv[]){int end;int count = 0;char choice[32], filename[32];char str[32];word_t *dict;printf(" Be loading...\n");end = word_num("dict.txt");if(strcmp(argv[1], "-txt") == 0)dict = init_word(end, "dict.txt");if(strcmp(argv[1], "-bin") == 0){init_bin(end, "dict.txt");dict = fun_bin("dict.dat");}quicksort(dict, 0, end-1);system("clear");while(1){print_sys();printf("Please make your choice:");fgets(choice, 32, stdin);choice[strlen(choice)-1] = '\0';if(strcmp(choice, "1") == 0){system("clear");printf("if you want to return interface , input \"#\"\n");printf("else input the word you want to search .\n");while(1){printf("Input : ");fgets(str, 32, stdin);str[strlen(str)-1] = '\0';if(count > 0)system("clear");count++;if(strcmp(str, "#") == 0)break;if(binarysearch(dict, str, end) != NULL){print(binarysearch(dict, str, end));}else{char ch[32];printf("Sorry, can't find such word in system-library !\nDo you want to go to yourself's word-library ? ( y or n) ");while(1){fgets(ch, 32, stdin);ch[strlen(ch)-1] = '\0';if(strcmp(ch, "y") == 0){printf("Please input the filename of your word-library : ")while(1){fgets(filename, 32, stdin);filename[strlen(filename)-1] = '\0';FILE *fp;if((fp = fopen(filename, "r")) != NULL)break;elseprintf("Can't find such text which you inputed.\nPlease input the filename again: ");}usr_txt(str, filename);break;}else if(strcmp(ch, "n") == 0)break;elseprintf("Undefind command: \"%s\"\nPlease input your choice again :(y or n)", ch);}}}}else if(strcmp(choice, "2") == 0){system("clear");printf("*********************************\n");printf("* Byebye! *\n");printf("*********************************\n");return 1;}elseprintf("Undefind command: \"%s\"\n.Please input your choice again : ", choice);}int i, j;for (i = 0; i < end; i++){free(dict[i].key);for (j = 0; j < dict[i].num; j++)free(dict[i].trans[j]);free(dict[i].trans);}free(dict);return 0;}。
python 实现中文文本检索方法

(实用版3篇)编制人员:_______________审核人员:_______________审批人员:_______________编制单位:_______________编制时间:_______________序言小编为大家精心编写了3篇《python 实现中文文本检索方法》,供大家借鉴与参考。
下载文档后,可根据您的实际需要进行调整和使用,希望能够帮助到大家,谢射!(3篇)《python 实现中文文本检索方法》篇1要实现中文文本检索方法,可以使用 Python 中的各种自然语言处理 (NLP) 库。
以下是一些常用的方法:1. 使用 Python 中的 jieba 库进行分词jieba 是一个常用的中文分词库,可以使用 Python 中的 pip 安装。
使用jieba 库,可以对中文文本进行分词,并将其转换为词典形式,以便进行检索。
例如,下面的代码演示了如何使用 jieba 库对中文文本进行分词:```import jiebatext = "我爱人工智能助手"seg_list = jieba.cut(text)print(seg_list)```输出结果为:```我爱人工智能助手```2. 使用 Python 中的 docplex 库进行全文检索docplex 是一个用于全文检索的 Python 库,它支持多种文本格式,包括PDF、Word 文档、HTML 等。
使用 docplex 库,可以对文本进行全文检索,并返回匹配的文本行。
例如,下面的代码演示了如何使用 docplex 库对 PDF 文件进行全文检索:```import docplex# 打开 PDF 文件pdf = docplex.PDF("example.pdf")# 进行全文检索query = "人工智能"results = pdf.search(query)# 打印匹配的文本行for r in results:print(r.text)```输出结果为:```人工智能人工智能技术人工智能的发展```3. 使用 Python 中的 Whoosh 库进行文本检索Whoosh 是一个用于文本检索的 Python 库,它支持多种索引格式,包括Elasticsearch、Solr、Whoosh 等。
文件夹中所有文件中的内容搜索源代码

srFile.Close(); srFile.Dispose(); srFile = null;
}
MessageBox.Show(exp.Message, "Exception Found", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
lblStatus.Text = "Status: Search end."; }
// 搜索指定文件夹,并递归搜索其子文件夹 private void SearchDir(string dir) {
lblStatus.Text = "Search DIR: " + dir; string[] arrFolders = new string[0]; string[] arrFiles = new string[0];
try {
arrFolders = Directory.GetDirectories(dir); arrFiles = Directory.GetFiles(dir); } catch {
MessageBox.Show("Please set the correct Directory Path", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
return; }
for(int i=0; i"Search DIR: " + dir;
iIndex = stIndexOf('/'); if (iIndex < 0)
PHP搜索引擎源代码

$this->_sites['meta'] = $this->getMeta($content);
$this->_sites['title'] = $this->getTitle($content);
//$this->_sites['detail'] = $this->getDetail($content);
对某一网址进行检索获取网站基本信息同时提取网站的所有连接
PHP搜索引擎源代码
简单PHP搜索引擎源代码,需要开启PHP的cURL扩展。功能:对某一网址进行检索,获取网站基本信息,同时提取网站的所有连接。
<?php
class Engine{
private $_url = ''; //URL地址
private $_sites = ''; //页面信息
return substr($body,0,400);
}
//获取title内容
public function getTitle($content){
preg_match('/
$this->_sites['links'] = $this->getLinks($content);
}
//获取meta内容
public function getMeta($content){
$file = 'metaCache';
file_put_contents($file,$content);
如何查看网页源代码

如何查看网页源代码
好多初学程序员或者网页设计员或者是站长等经常会模仿别人的网页,看别人网页是怎么写的,这里呢就需要查看别人网页的源代码。
下面小编跟你分享查看网页源代码的方法。
查看网页源代码的方法
IE浏览器
1打开一个网页之后,右键---》查看源文件(IE10 为查看源),然后就会弹出网页的源文件。
2点击之后就会出现一个文本样式的代码了
3第二种方法就是根据浏览器状态栏或工具栏中的点击“查看”然后就用一项“查看源代码”,点击查看源代码即可查看此网页
的源代码源文件。
火狐浏览器查看
1打开火狐浏览器Firefox,右键----查看页面源代码
2或者点击右上角的工具栏按钮如下图
3点击web开发者----》页面源代码
chrome(谷歌)浏览器
1打开谷歌浏览器,右键---》查看网页源代码,也可以点击浏览器,右上角“三横”控制图标---》工具----》查看源代码
2一般如果查看网页的源代码,利用右键--》查看网页源代码即可,这样子方便快速,其它浏览器基本大同小异,操作步骤和上述三个浏览器基本一致。
dict项目案例

dict项目案例
项目名称:Python词典(dict)
项目简介:一个Python语言编写的命令行词典,能够查询英文单词的词义、例句、音标等信息。
用户可以直接在命令行中输入要查询的单词,程序会通过网络获取该单词的相关信息并输出到屏幕上。
实现技术:Python、requests库、json库、argparse库
项目流程:
1. 接受命令行中输入的参数(单词)。
2. 使用requests库向在线词典API发送请求。
3. 解析API返回的JSON格式数据,提取需要的信息。
4. 将提取得到的信息格式化后输出到屏幕上。
5. 处理异常情况,如网络连接失败、单词不存在等情况。
优化方向:
1. 实现本地缓存,减少网络请求次数,提高程序运行效率。
2. 添加输入自动补全功能,提高用户体验。
3. 实现中英文互译功能,拓展使用范围。
4. 添加多语言支持,满足不同语种用户的需求。
以上是一个dict项目的简单实现方案,希望对你有所帮助。
关于搜索引擎的一些源代码

关于搜索引擎的一些源代码//第一个版本应该保存body和title,搜索结果形成超链接,不显示正文。
protected void Button1_Click(object sender, EventArgs e){string indexPath = "c:/index";//设置索引文件保存的路径//Directory表示索引文件(用来保存用户扔过来的数据的地方)保存的地方//是抽象类,两个子类FSDirectory(文件中)、RAMDire ctory (内存中)。
indexpath表示索引的文件夹路径FSDirectory directory = FSDirectory.Open(new DirectoryInfo(indexPath), new NativeFSLockFactory());//IndexReader的静态方法bool IndexExists(Directory directory)判断目录directory是否是一个索引目录。
bool isUpdate = IndexReader.IndexExists(directory);if (isUpdate)//假如该目录存在{//如果索引目录被锁定(比如索引过程中程序异常退出),则首先解锁if (IndexWriter.IsLocked(directory)){//在对目录写之前会先把目录锁定。
两个IndexWriter没法同时写一个索引文件。
IndexWriter在进行写操作的时候会自动加锁,close 的时候会自动解锁IndexWriter.Unlock(directory);}}//IndexReader对索引进行读取的类,对IndexWriter进行写的类IndexWriter writer = new IndexWriter(directory, new PanGuAnalyzer(), !isUpdate, .Index.IndexWriter.MaxFieldLength.UNLIMITED);WebClient wc = new WebClient();wc.Encoding = Encoding.UTF8;//否则下载的是乱码//todo:读取rss,获得第一个item中的链接的编号部分就是最大的帖子编号int maxId = GetMaxId();for (int i = 1; i <= maxId; i++){//依次获得地址string url = "-" + i + ".aspx";//根据地址依次将对应的该网页下载下来string html = wc.DownloadString(url);//将各个文档解析HTMLDocumentClass doc = new HTMLDocumentClass();doc.designMode = "on"; //不让解析引擎去尝试运行javascript doc.IHTMLDocument2_write(html);doc.close();string title = doc.title;string body = doc.body.innerText;//去掉标签//为避免重复索引,所以先删除number=i的记录,再重新添加writer.DeleteDocuments(new Term("number",i.T oString()));Document document = new Document();//只有对需要全文检索的字段才ANALYZEDdocument.Add(new Field("number", i.ToString(), Field.Store.YES, Field.Index.NOT_ANALYZED));document.Add(new Field("title", title, Field.Store.YES, Field.Index.NOT_ANALYZED));document.Add(new Field("body", body, Field.Store.YES, Field.Index.ANALYZED,.Documents.Field.TermVector.WITH_POSITIONS_OFFS ETS));writer.AddDocument(document);logger.Debug("索引" + i + "完毕");}writer.Close();directory.Close();//不要忘了Close,否则索引结果搜不到logger.Debug("全部索引完毕");}protected void Button2_Click(object sender, EventArgs e){string indexPath = "c:/index";string kw = TextBox1.Text;FSDirectory directory = FSDirectory.Open(new DirectoryInfo(indexPath), new NoLockFactory());IndexReader reader = IndexReader.Open(directory, true);IndexSearcher searcher = new IndexSearcher(reader);PhraseQuery query = new PhraseQuery();//todo:把用户输入的关键词进行拆词foreach (string word in CommonHelper.SplitWord(TextBox1.T ext))//先用空格,让用户去分词,空格分隔的就是词“计算机专业”{query.Add(new Term("body", word));}//query.Add(new T erm("body","计算机"));//query.Add(new T erm("body", "专业"));query.SetSlop(100);TopScoreDocCollector collector = TopScoreDocCollector.create(1000, true);searcher.Search(query, null, collector);ScoreDoc[] docs = collector.TopDocs(0, collector.GetT otalHits()).scoreDocs;List<SearchResult> listResult = new List<SearchResult>();for (int i = 0; i < docs.Length; i++){int docId = docs[i].doc;//取到文档的编号(主键,这个是Lucene .net分配的)//检索结果中只有文档的id,如果要取Document,则需要Doc 再去取//降低内容占用Document doc = searcher.Doc(docId);//根据id找Document string number = doc.Get("number");string title = doc.Get("title");string body = doc.Get("body");SearchResult result = new SearchResult();result.Number = number;result.Title = title;result.BodyPreview = Preview(body,TextBox1.T ext);listResult.Add(result);}repeaterResult.DataSource = listResult;repeaterResult.DataBind();}private static string Preview(string body,string keyword){//创建HTMLFormatter,参数为高亮单词的前后缀PanGu.HighLight.SimpleHTMLFormatter simpleHTMLFormatter =new PanGu.HighLight.SimpleHTMLFormatter("<font color=\"red\">", "</font>");//创建Highlighter ,输入HTMLFormatter 和盘古分词对象SemgentPanGu.HighLight.Highlighter highlighter =new PanGu.HighLight.Highlighter(simpleHTMLFormatter, new Segment());//设置每个摘要段的字符数highlighter.FragmentSize = 100;//获取最匹配的摘要段String bodyPreview = highlighter.GetBestFragment(keyword, body);return bodyPreview;}private int GetMaxId(){XDocument xdoc = XDocument.Load("");XElement channel = xdoc.Root.Element("channel");XElement firstItem = channel.Elements("item").First();XElement link = firstItem.Element("link");Match match =Regex.Match(link.Value, @"showtopic-(\d+)\.aspx");string id =match.Groups[1].Value;return Convert.ToInt32(id);}}}。
英汉字典源代码

字典最快速的实现方法是trie tree。
这个树是专门用来实现字典的。
但是trie tree的删除操作比较麻烦。
用二叉查找树可以实现,速度也可以很快。
AVL tree只不过是平衡的二叉树,在字典这个应用上没有客观的速度提升,因为字典不会产生极端化的二叉树(链表)。
下面是我的二叉查找树的代码。
二叉查找树的优点是实现容易,而且它的inorder traverse 既是按照字母顺序的输出。
//binary search tree, not self-balancing//by Qingxing Zhang, Dec 28,2009. prep for google interview#include <iostream>using namespace std;struct BST{int data;BST *left;BST *right;};//runtime: O(logn) on average, O(n) worst casebool search(BST *&root, int key)//return false if the key doesn't exist{if(root==NULL)return false;if(key < root->data)return search(root->left,key);else if(key > root->data)return search(root->right,key);elsereturn true;}//runtime: O(logn)on average, O(n) worst casebool insert(BST *&root, int key)//return false if the key already exists{if(root==NULL){BST *node = new BST;node->data = key;node->left = node->right = NULL;root = node;return true;}else if(key < root->data)return insert(root->left,key);else if(key > root->data)return insert(root->right,key);elsereturn false;}//runtime:O(logn) on average, O(n) worst casebool remove(BST *&root,int key)//return false if the key doesn't exist.{if(root==NULL)//no such keyreturn false;else if(key < root->data)return remove(root->left,key);else if(key > root->data)return remove(root->right,key);else//node found{if((root->left==NULL)&&(root->right==NULL))//no child(leaf node){BST *tmp = root;root = NULL;delete tmp;}else if((root->left==NULL)||(root->right==NULL))//one child{BST *tmp = root;if(root->left==NULL)root = root->right;elseroot = root->left;delete tmp;}else//two children:replace node value with inorder successor and delete that node {BST *tmp = root->right;while(tmp->left!=NULL)tmp = tmp->left;int tmpdata = tmp->data;remove(root,tmpdata);root->data = tmpdata;}return true;}}//runtime:O(n)void inorder(BST *&node) {if(node!=NULL){inorder(node->left);cout << node->data << " ";inorder(node->right);}}//runtime:O(n)void preorder(BST *&node) {if(node!=NULL){cout << node->data << " ";preorder(node->left);preorder(node->right);}}//runtime:O(n)void postorder(BST *&node) {if(node!=NULL){postorder(node->left);postorder(node->right);cout << node->data << " "; }}int main(){bool b;BST *root = NULL;b = insert(root,1);b = insert(root,7);b = insert(root,5);b = insert(root,77);b = insert(root,10);b = insert(root,4);b = insert(root,13);//inordercout << "In-order:";inorder(root);cout << endl;//preordercout << "Pre-order:";preorder(root);cout << endl;//postordercout << "Post-order:";postorder(root);cout << endl;// search for 7if(search(root,7))cout << "7 found!" << endl;elsecout << "7 doesn't exist!" << endl;b = remove(root,7);cout << "----------------" << endl;//inordercout << "In-order:";inorder(root);cout << endl;//preordercout << "Pre-order:";preorder(root);cout << endl;//postordercout << "Post-order:";postorder(root);cout << endl;cout << "7 found!" << endl;elsecout << "7 doesn't exist!" << endl; return 0;}。
C#Dictionary(字典)源码解析效率分析

C#Dictionary(字典)源码解析效率分析 通过查阅⽹上相关资料和查看微软源码,我对Dictionary有了更深的理解。
Dictionary,翻译为中⽂是字典,通过查看源码发现,它真的内部结构真的和平时⽤的字典思想⼀样。
我们平时⽤的字典主要包括两个两个部分,⽬录和正⽂,⽬录⽤来进⾏第⼀次的粗略查找,正⽂进⾏第⼆次精确查找。
通过将数据进⾏分组,形成⽬录,正⽂则是分组后的结果。
⽽Dictionary对应的是 int[] buckets 和 Entry[] entries,buckets⽤来记录要查询元素分组的起始位置(这么写是为了⽅便理解,其实是最后⼀个插⼊元素的位置没有元素为-1,查找同组元素通过 entries 元素中的 Next 遍历,后⾯会提到),entries记录所有元素。
分组依据是计算元素 Key 的哈希值与 buckets 的长度取余,余数就是分组,指向buckets 位置。
通过先查找 buckets 确定元素分组的起始位置,再遍历分组内元素查找到准确位置。
与对应的⽬录和正⽂相同,buckets的长度⼤于等于 entries(我理解是为扩展做准备的),buckets 的长度使⽤HashHelpers.GetPrime(capacity) 计算,是⼀个计算得到的最优值。
capacity是字典的容量,⼤于等于字典中实际存储元素个数。
Dictionary与真实的字典不同之处在于,真实字典的分组结果的物理位置是连续的,⽽ Dictionary 不是,他的物理位置顺序就是插⼊的顺序,⽽分组信息记录在 entries 元素中的 Next 中,Next 是个 int 字段,⽤来记录同组元素的下⼀个位置(若当前为该组第⼀个插⼊元素则记录-1,第⼀个插⼊元素在分组遍历的最后⼀个)解析⼀下Dictionary的⼏个关键⽅法1.Add(Insert 新增&更新⽅法) Add和使⽤[]更新实际就是调⽤的Insert,代码如下。
一个完整的从语言写的电子字典源码

C语言项目——查字典【项目需求描述】一、单词查询给定文本文件“dict.txt”,该文件用于存储词库。
词库为“英-汉”,“汉-英”双语词典,每个单词和其解释的格式固定,如下所示:#单词Trans:解释1@解释2@…解释n每个新单词由“#”开头,解释之间使用“@”隔开。
一个词可能有多个解释,解释均存储在一行里,行首固定以“Trans:”开头。
下面是一个典型的例子:#abyssinianTrans:a. 阿比西尼亚的@n. 阿比西尼亚人;依索比亚人该词有两个解释,一个是“a. 阿比西尼亚的”;另一个是“n. 阿比西尼亚人;依索比亚人”。
要求编写程序将词库文件读取到内存中,接受用户输入的单词,在字典中查找单词,并且将解释输出到屏幕上。
用户可以反复输入,直到用户输入“exit”字典程序退出。
程序执行格式如下所示:./app –test2-test2表示使用文本词库进行单词查找。
二、建立索引,并且使用索引进行单词查询要求建立二进制索引,索引格式如下图所示。
将文本文件“dict.txt”文件转换为上图所示索引文件“dict.dat”,使用索引文件实现单词查找。
程序执行格式如下:./app –index-index表示使用文本词库dict.txt建立二进制索引词库dict.dat./app –test2-test2表示使用二进制索引词库进行单词查找。
三、支持用户自添加新词用户添加的新词存放在指定文件中。
如果待查单词在词库中找不到,则使用用户提供的词库。
用户的词库使用文本形式保存,便于用户修改。
程序执行格式图1-1所示。
./app 词库选择选项-f 用户词库文件名词库选项为-test1,或者-test2,表示使用文本词库或者二进制索引词库。
-f为固定参数,用来指定用户词库文件名。
图1-1【项目要求】❑尽量考虑程序执行的效率,尽量减少开销,提高程序速度❑尽量考虑模块化程序设计思想,能够引入面向对象的设计模式和方法❑保证代码的可读性,紧凑的组织代码❑清晰设计思想和设计思路,代码实现尽量简洁❑可以完成相应的拓展功能,例如用户自添加单词,建立索引以提高查找速度等【考察知识点】(1)变量数据类型(2)数组(3)结构体(4)typedef关键字的使用(5)控制结构(6)函数接口设计(7)static关键字的使用(8)文件拆分与代码组织(9)模块化设计思想(10)简单的面向对象程序设计思想(11)指针与指针控制(12)const关键字的使用(13)C语言程序的命令行参数(14)多文件符号解析(15)头文件包含(16)宏(17)条件编译(18)字符串操作(19)malloc函数(20)常用的字符串库函数(21)文件操作(22)简单的出错处理(23)排序算法和二分查找算法(24)二进制文件和文本文件的区别(25)链表操作(26)makefile的使用(27)编程工具的使用(vi,gcc,gdb)(28)文档组织和项目规划【未考察到的知识点】(1)变参函数(2)函数指针(3)泛型算法(4)复杂链表的链表操作(5)栈和队列(6)二叉树。
c语言数据查询程序简单的代码

C语言数据查询程序简单的代码一、背景介绍C语言作为一种通用的编程语言,在软件开发领域有着广泛的应用。
数据查询是软件开发中常见的需求之一,因此编写一个数据查询程序是很有必要的。
本文将介绍如何用C语言编写一个简单的数据查询程序的代码。
二、程序设计在C语言中,要实现数据查询,可以使用数组或链表等数据结构来存储数据,然后通过循环或递归等方式来进行数据的查询操作。
下面是一个简单的C语言代码示例,实现了一个基于数组的数据查询程序。
```c#include <stdio.h>// 定义数据结构typedef struct {int id;char name[20];int score;} Student;// 查询函数int query(Student students[], int n, int targetId) {for (int i = 0; i < n; i++) {if (students[i].id == targetId) {return i;}}return -1;}int m本人n() {// 初始化数据Student students[3] = {{1, "张三", 80},{2, "李四", 90},{3, "王五", 85}};// 查询数据int targetId = 2;int index = query(students, 3, targetId);if (index != -1) {printf("学号:d,尊称:s,成绩:d\n", students[index].id,students[index].name, students[index].score);} else {printf("未找到该学生\n");}return 0;}```三、代码解析1. 定义了一个包含学生学号、尊称和成绩的数据结构Student。
VC6.0下电子词典源代码
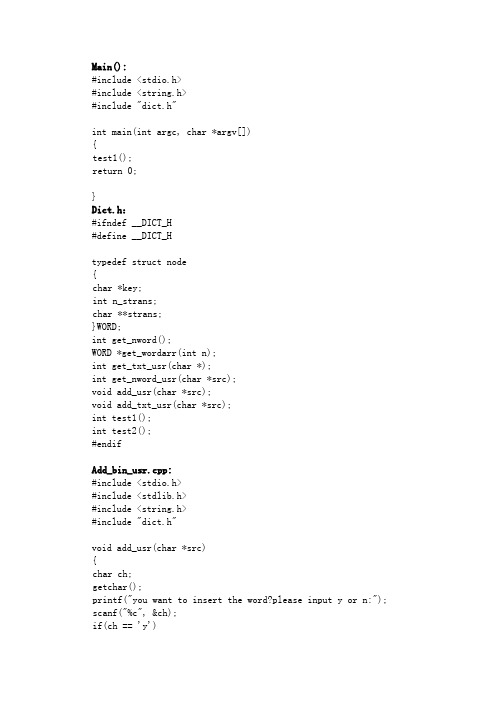
Main():#include <stdio.h>#include <string.h>#include "dict.h"int main(int argc, char *argv[]){t est1();r eturn 0;}Dict.h:#ifndef __DICT_H#define __DICT_Htypedef struct node{c har *key;i nt n_strans;c har **strans;}WORD;int get_nword();WORD *get_wordarr(int n);int get_txt_usr(char *);int get_nword_usr(char *src);void add_usr(char *src);void add_txt_usr(char *src);int test1();int test2();#endifAdd_bin_usr.cpp:#include <stdio.h>#include <stdlib.h>#include <string.h>#include "dict.h"void add_usr(char *src){c har ch;g etchar();p rintf("you want to insert the word?please input y or n:"); s canf("%c", &ch);i f(ch == 'y'){int n_strans, i;char buf[512];FILE *fw;int len;if((fw = fopen("dict_bin_usr.txt", "a")) == NULL){perror("dict_bin_usr.txt open failuren");exit(-1);}len = strlen(src)+1;fwrite(&len, 4, 1, fw);fwrite(src,1, len, fw);printf("input word information:input n_strans:");scanf("%d",&n_strans);fwrite(&n_strans, 4, 1, fw);printf("input word information:input strans:");getchar();for(i=0;i<n_strans; i++){fgets(buf,sizeof(buf),stdin);len = strlen(buf);buf[len-1] = '\0';fwrite(&len, 4, 1, fw);fwrite(buf, 1, len, fw);}fclose(fw);}}Add_txt_usr.cpp:#include <stdio.h>#include <string.h>#include <stdlib.h>#include "dict.h"void add_txt_usr(char *src){c har ch;c har buf[100];p rintf("you want to insert the word?please input y or n:"); s canf("%c", &ch);i f(ch== 'y'){FILE *fw;int len;char str[100];if((fw = fopen("dict_usr.txt", "a")) == NULL){perror("dict_usr.txt open failuren");exit(-1);}fprintf(fw,"%s\n", src);printf("input word information:input strans:");getchar();fgets(buf,sizeof(buf),stdin);len = strlen(buf);buf[len-1] = '\0';fprintf(fw, "%s\n", buf);fclose(fw);}i f(ch == 'n')fgets(buf,sizeof(buf),stdin);}Dict1.cpp:#include <stdio.h>#include <string.h>#include <stdlib.h>#include "dict.h"int search(WORD *word, char *str, int n){i nt mid, i;i nt start = 0;i nt end = n-1;i nt flag = 1;w hile(start <= end){mid = (start+end)/2;if((strcmp(str, word[mid].key))==0){flag = 0;printf("%s\n", word[mid].key);for(i=0; i<word[mid].n_strans; i++)printf("%s\n",word[mid].strans[i]);return 0;}else if(strcmp(str, word[mid].key)>0)start = mid+1;elseend = mid-1;}p rintf("in database These is no the word\n");r eturn flag;}static void free_word(WORD *word,int n){i nt i,j;f or(i=n-1; i>=0; i--){for(j=word[i].n_strans-1;j>=0; j--)free(word[i].strans[j]);free(word[i].strans);free(word[i].key);}f ree(word);// word =NULL;}int test1(void){i nt n;n = get_nword();W ORD *pword = get_wordarr(n);c har a[50];i nt flag = 0;i nt len;p rintf("******************************************************\n" );p rintf("please input you want to search word(input exit end):");f gets(a,50, stdin);l en = strlen(a);a[len-1] = '\0';w hile(strcmp(a, "exit") != 0){flag = search(pword, a, n);//找到返回0,找不到返回1if(flag == 1){flag = get_txt_usr(a);if(flag == 1)add_txt_usr(a);}printf("******************************************************\n" );printf("please input you want to search word(input exit end):");//system("pause");fgets(a,50, stdin);len = strlen(a);a[len-1] = '\0';}f ree_word(pword, n);return 0;}Dict3.cpp:#include <stdio.h>#include <string.h>#include <stdlib.h>#include "dict.h"int N;static WORD *get_bin_nword(){i nt n, i, j, n_strans;F ILE *fr;i nt len;i f((fr = fopen("dict_bin.txt", "r")) == NULL){perror("dict_bin.txt open failuren");exit(-1);}f read(&n, 4, 1, fr);N = n;W ORD *wordarr=(WORD *)malloc(sizeof(WORD)*N);f or(i=0; i<n; i++){fread(&len, 4, 1, fr);wordarr[i].key = (char *)malloc(len);fread(wordarr[i].key, 1, len, fr);fread(&n_strans, 4, 1, fr);wordarr[i].n_strans = n_strans;wordarr[i].strans =(char **) malloc(sizeof(*(wordarr[i].strans)) * n_strans);for(j=0; j<wordarr[i].n_strans; j++){fread(&len, 4, 1, fr);wordarr[i].strans[j] =(char *) malloc(len);fread(wordarr[i].strans[j], 1, len, fr);}}f close(fr);r eturn wordarr;}static int search(WORD *wordarr, int n, char *src){i nt start = 0;i nt end = n-1;i nt mid;i nt i, flag = 1;w hile(start <= end){mid = (start+end)/2;if((strcmp(src, wordarr[mid].key))==0){flag = 0;printf("%s\n", wordarr[mid].key);for(i=0; i<wordarr[mid].n_strans; i++)printf("%s\n",wordarr[mid].strans[i]);return 0;}else if(strcmp(src, wordarr[mid].key)>0)start = mid+1;elseend = mid-1;}p rintf("****************************************\n");p rintf(" in database These is no the word\n");p rintf("****************************************\n");r eturn flag;}static void free_word(WORD *word,int n){i nt i,j;f or(i=n-1; i>=0; i--){for(j=word[i].n_strans-1;j>=0; j--)free(word[i].strans[j]);free(word[i].strans);free(word[i].key);}f ree(word);w ord =NULL;}Get_bin_wordaar_txt.cpp:#include <stdio.h>#include <stdlib.h>#include <string.h>#include "dict.h"WORD *get_wordarr(int n) //从文件中读取内容,放在数据结构里面{W ORD *word = (WORD *)malloc(sizeof(WORD)*n);c har buf[512], bufcp[512];c har *token, *begin;i nt i = 0,j;i nt len;F ILE *fr, *fw;i f((fr = fopen("dict.txt", "r")) == NULL){perror("dict.txt open failure\n");exit(-1);}i f((fw = fopen("dict_bin.txt", "w")) == NULL){perror("dict_bin.txt open failuren");exit(-1);}f write(&n, 4, 1, fw);w hile(fgets(buf, sizeof(buf), fr)){len = strlen(buf)-1;buf[len] = '\0';word[i].key = (char *)malloc(len);strcpy(word[i].key, &buf[1]);fwrite(&len, 4, 1, fw);fwrite(word[i].key, 1, len, fw);fgets(buf, sizeof(buf), fr);{strcpy(bufcp, buf);for(j=0,begin=buf; (token=strtok(begin, "@"))!=NULL; begin=NULL,j++)word[i].n_strans = j;fwrite(&j, 4, 1, fw);word[i].strans = (char **)malloc(sizeof(*(word[i].strans)) * j);for(j=0,begin=bufcp; (token=strtok(begin, "@"))!=NULL; begin=NULL,j++){len = strlen(token)+1;word[i].strans[j] = (char *)malloc(len);strcpy(word[i].strans[j],token);fwrite(&len, 4, 1, fw);fwrite(token, 1, len, fw);}}i++;}f close(fr);f close(fw);r eturn word;}Get_nword.cpp:#include <stdio.h>#include "dict.h"int get_nword(){F ILE *fp;i nt count = 0;c har buf[512];i f((fp=(fopen("dict.txt", "r")))==NULL){perror("file open failure\n");return -1;}w hile(fgets(buf, sizeof(buf), fp)){count++;}c ount /= 2;f close(fp);r eturn count;}Search_bin_usr.cpp:#include <stdio.h>#include <string.h>#include <stdlib.h>#include "dict.h"int get_nword_usr(char *src){int j, n_strans;FILE *fr;c har str[512];i nt len =0;i f((fr = fopen("dict_bin_usr.txt", "r")) == NULL) {perror("file open failuren");exit(-1);}w hile(fread(&len, 4, 1, fr)){fread(str, 1, len, fr);if(strcmp(str, src) == 0){printf("the word in usr database\n");printf("%s\n", str);fread(&n_strans, 4, 1, fr);for(j=0; j<n_strans; j++){fread(&len, 4, 1, fr);fread(str, 1, len, fr);printf("%s\n", str);}return 0;}fread(&n_strans, 4, 1, fr);for(j=0; j<n_strans; j++){fread(&len, 4, 1, fr);fread(str, 1, len, fr);}}p rintf("in usr these no the word\n");f close(fr);r eturn 1;}Seach_usr_txt.cpp:#include <stdio.h>#include <string.h>#include <stdlib.h>#include "dict.h"int get_txt_usr(char *src){F ILE *fr;c har str[512];i nt len =0;i f((fr = fopen("dict_usr.txt", "r")) == NULL){perror("dict_usr.txt open failuren");exit(-1);}w hile(fgets(str,sizeof(str), fr)){len = strlen(str);str[len-1] = '\0';if(strcmp(str, src) == 0){printf("****************************************\n");printf("the word in usr database\n");printf("%s\n", str);fgets(str, sizeof(str), fr);printf("%s\n", str);printf("****************************************\n");return 0;}}p rintf("****************************************\n");p rintf("in usr these no the word\n");p rintf("****************************************\n");f close(fr);r eturn 1;}。
基于Python的新华字典api调用代码实例

基于Python的新华字典api调用代码实例接口描述:基于Python的新华字典api调用代码实例接口平台:聚合数据#!/usr/bin/python# -*- coding: utf-8 -*-import json, urllibfrom urllib import urlencode#----------------------------------# 新华字典调用示例代码-聚合数据# 在线接口文档:/docs/156#----------------------------------def main():#配置您申请的APPKeyappkey ="*********************"#1.根据汉字查询字典request1(appkey,"GET")#2.汉字部首列表request2(appkey,"GET")#3.汉字拼音列表request3(appkey,"GET")#4.根据部首查询汉字request4(appkey,"GET")#5.根据拼音查询汉字request5(appkey,"GET")#6.根据id查询汉字完整信息request6(appkey,"GET")#根据汉字查询字典def request1(appkey, m="GET"):url ="/xhzd/query"params ={"word": "", #填写需要查询的汉字,UTF8 urlencode编码"key": appkey, #应用APPKEY(应用详细页查询)"dtype": "", #返回数据的格式,xml或json,默认json}params =urlencode(params)if m =="GET":f =urllib.urlopen("%s?%s"%(url, params))else:f =urllib.urlopen(url, params)content =f.read()res =json.loads(content)if res:error_code =res["error_code"]if error_code ==0:#成功请求print res["result"]else:print"%s:%s"%(res["error_code"],res["reason"]) else:print"request api error"#汉字部首列表def request2(appkey, m="GET"):url ="/xhzd/bushou"params ={"key": appkey, #应用APPKEY(应用详细页查询)"dtype": "", #返回数据的格式,xml或json,默认json}params =urlencode(params)if m =="GET":f =urllib.urlopen("%s?%s"%(url, params))else:f =urllib.urlopen(url, params)content =f.read()res =json.loads(content)if res:error_code =res["error_code"]if error_code ==0:#成功请求print res["result"]else:print"%s:%s"%(res["error_code"],res["reason"]) else:print"request api error"#汉字拼音列表def request3(appkey, m="GET"):url ="/xhzd/pinyin"params ={"key": appkey, #应用APPKEY(应用详细页查询)"dtype": "", #返回数据的格式,xml或json,默认json}params =urlencode(params)if m =="GET":f =urllib.urlopen("%s?%s"%(url, params))else:f =urllib.urlopen(url, params)content =f.read()res =json.loads(content)if res:error_code =res["error_code"]if error_code ==0:#成功请求print res["result"]else:print"%s:%s"%(res["error_code"],res["reason"]) else:print"request api error"#根据部首查询汉字def request4(appkey, m="GET"):url ="/xhzd/querybs"params ={"word": "", #填写需要查询的汉字部首,UTF8 urlencode编码"key": appkey, #应用APPKEY(应用详细页查询)"dtype": "", #返回数据的格式,xml或json,默认json"page": "", #页数,默认1"pageszie": "", #每页返回条数,默认10 最大50"isjijie": "", #是否显示简解,1显示 0不显示默认1"isxiangjie": "", #是否显示详解,1显示 0不显示默认1}params =urlencode(params)if m =="GET":f =urllib.urlopen("%s?%s"%(url, params))else:f =urllib.urlopen(url, params)content =f.read()res =json.loads(content)if res:error_code =res["error_code"]if error_code ==0:#成功请求print res["result"]else:print"%s:%s"%(res["error_code"],res["reason"]) else:print"request api error"#根据拼音查询汉字def request5(appkey, m="GET"):url ="/xhzd/querypy"params ={"word": "", #填写需要查询的拼音"key": appkey, #应用APPKEY(应用详细页查询)"dtype": "", #返回数据的格式,xml或json,默认json"page": "", #页数,默认1"pageszie": "", #每页返回条数,默认10 最大50"isjijie": "", #是否显示简解,1显示 0不显示默认1"isxiangjie": "", #是否显示详解,1显示 0不显示默认1}params =urlencode(params)if m =="GET":f =urllib.urlopen("%s?%s"%(url, params))else:f =urllib.urlopen(url, params)content =f.read()res =json.loads(content)if res:error_code =res["error_code"]if error_code ==0:#成功请求print res["result"]else:print"%s:%s"%(res["error_code"],res["reason"]) else:print"request api error"#根据id查询汉字完整信息def request6(appkey, m="GET"):url ="/xhzd/queryid"params ={"word": "", #填写需要查询的汉字id"key": appkey, #应用APPKEY(应用详细页查询)"dtype": "", #返回数据的格式,xml或json,默认json}params =urlencode(params)if m =="GET":f =urllib.urlopen("%s?%s"%(url, params))else:f =urllib.urlopen(url, params)content =f.read()res =json.loads(content)if res:error_code =res["error_code"]if error_code ==0:#成功请求print res["result"]else:print"%s:%s"%(res["error_code"],res["reason"]) else:print"request api error"if__name__ =='__main__':main()。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
#include <>#include <>#include <>#define BUF_SIZE 4096typedef struct{char *key;int num;char **trans;}word_t;void sys_err(const char *msg){perror(msg);exit(1);}int word_num(const char *filename){FILE *fp;char buf[BUF_SIZE];int total = 0;fp = fopen(filename, "r");if(fp == NULL)sys_err("open file\n");while((fgets(buf, BUF_SIZE, fp)) != NULL) {if(buf[0] == '#')total++;}fclose(fp);return total;}int nword(const char *filename){FILE *fp;char buf[BUF_SIZE];int total = 0;fp = fopen(filename, "r+");if(fp == NULL)sys_err("open file\n");while((fgets(buf, BUF_SIZE, fp)) != NULL) {if(buf[0] == '#')total++;}fclose(fp);return total;}word_t *init_word(int total, const char *filename)ey, str) == 0){print(dict+i);break;}}if(i == n_word){char ch[32];printf("This is a new word. can't find in %s.\nDo you want to save it in your word-library ? ( y or n)", filename);fgets(ch, 32, stdin);ch[strlen(ch)-1] = '\0';while(1){if(strcmp(ch, "y") == 0){insert_new_word(filename);break;}else if(strcmp(ch, "n") == 0)break;elseprintf("Undefind command : \"%s\".\nPlease input your choice again :(y or n) ", ch);}}}void print_sys(void){printf("---------------------------------\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* 欢迎使用电子辞典 *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("* *\n");printf("---------------------------------\n");}int main(int argc, const char *argv[]){int end;int count = 0;char choice[32], filename[32];char str[32];word_t *dict;printf(" Be loading...\n");end = word_num("");if(strcmp(argv[1], "-txt") == 0)dict = init_word(end, "");if(strcmp(argv[1], "-bin") == 0){init_bin(end, "");dict = fun_bin("");}quicksort(dict, 0, end-1);system("clear");while(1){print_sys();printf("Please make your choice:");fgets(choice, 32, stdin);choice[strlen(choice)-1] = '\0';if(strcmp(choice, "1") == 0){system("clear");printf("if you want to return interface , input \"#\"\n");printf("else input the word you want to search .\n");while(1){printf("Input : ");fgets(str, 32, stdin);str[strlen(str)-1] = '\0';if(count > 0)system("clear");count++;if(strcmp(str, "#") == 0)break;if(binarysearch(dict, str, end) != NULL){print(binarysearch(dict, str, end));}else{char ch[32];printf("Sorry, can't find such word in system-library !\nDo you want to go to yourself's word-library ? ( y or n) ");while(1){fgets(ch, 32, stdin);ch[strlen(ch)-1] = '\0';if(strcmp(ch, "y") == 0){printf("Please input the filename of your word-library : ")while(1){fgets(filename, 32, stdin);filename[strlen(filename)-1] = '\0';FILE *fp;if((fp = fopen(filename, "r")) != NULL)break;elseprintf("Can't find such text which you inputed.\nPlease input the filename again: ");}usr_txt(str, filename);break;}else if(strcmp(ch, "n") == 0)break;elseprintf("Undefind command: \"%s\"\nPlease input your choice again :(y or n)", ch);}}}}else if(strcmp(choice, "2") == 0){system("clear");printf("*********************************\n");printf("* Byebye! *\n");printf("*********************************\n");return 1;}elseprintf("Undefind command: \"%s\"\ input your choice again : ", choice);}int i, j;for (i = 0; i < end; i++){free(dict[i].key);for (j = 0; j < dict[i].num; j++)free(dict[i].trans[j]);free(dict[i].trans);}free(dict);return 0;}。