Android超炫图片浏览器代码
Android图片加载利器之Picasso基本用法

Android图⽚加载利器之Picasso基本⽤法今天开始我们来学习⼀下Picasso,计划包括以下⼏⽅⾯的内容:⽬前市场上⽐较流⾏的图⽚加载框架主要有UniversalImageLoader,Picasso,Glide,Fresco。
下⾯简单介绍⼀下这⼏个框架:UniversalImageLoader:这个可以说是⾮常⾮常经典的⼀个了,相信每个app的开发⼈员都使⽤过,只可惜作者已经停⽌该项⽬的维护了,所以不太推荐使⽤。
Picasso:是Square公司出品的图⽚加载框架,Square出品必出精品,主要特点就是使⽤简单,扩展性强,⽀持各种来源的图⽚,包括⽹络、Resources、assets、files、content providers等。
内部集成了OkHttp的⽹络框架,所以如果你的项⽬中使⽤了Square公司的其他框架,那么推荐使⽤Picasso,兼容性会好⼀些。
⽬前在Github上的Star已经达到12758个。
Glide:是Google员⼯的开源项⽬,基于Picasso的⼀个框架,代码风格与Picasso⾮常相似,增加了更多的功能,⾮常重要的就是⽀持gif,当然它的包会⼤⼀些。
如果你的项⽬对图⽚的使⽤场景⾮常多,并且需要⽀持gif,则推荐使⽤。
⽬前在Github 上的Star已经达到13636个。
Fresco:是FB出品的开源框架,⽐较新,最⼤的优点就是在内存占⽤上的优化,极⼤的减少了OOM,功能上也包含了以上三种框架的功能,但是也带来了⼀个⽐较明显的缺点就是太⼤了,所以推荐使⽤在完全是做图⽚相关的app上,否则Picasso和Glide就完全够⽤了。
⽬前在Github上的Star已经达到11983个。
上⾯主要对各种框架做个简单的介绍,既然是讲解Picasso的,那么接下来看看Picasso都有哪些功能。
1 提供内存和磁盘缓存,默认开启,可以设置不进⾏缓存2 图⽚加载过程中默认显⽰的图⽚3 图⽚加载失败或出错后显⽰的图⽚4 图⽚加载成功或失败的回调5 ⾃定义图⽚⼤⼩、⾃动测量ImageView⼤⼩、裁剪、旋转图⽚等6 对图⽚进⾏转换7 标签管理,暂停和恢复图⽚加载8 请求优先级管理9 可以从不同来源加载图⽚,⽹络、Resources、assets、files、content providers10 更加丰富的扩展功能以上这些功能将会在下⾯的⽂章中进⾏详细讲解。
黑马程序员安卓教程:案例-网络图片查看器

案例-网络图片查看器通过该案例可以学习如何将网络图片显示在到用户界面。
需求如图1-3,在编辑框输入图片的url地址,然后点击右侧的确定按钮。
然后app就开始访问网络,将网络上的图片显示在界面。
图1-3 网络图片查看器编写布局【文件1-5】activity_main.xml1.<LinearLayout xmlns:android="/apk/res/android"2.xmlns:tools="/tools"3.android:layout_width="match_parent"4.android:layout_height="match_parent"5.android:orientation="vertical">6.7.<LinearLayout8. android:layout_width="match_parent"9. android:layout_height="wrap_content"10. android:orientation="horizontal" >11.12. <EditText13. android:id="@+id/et_url"14. android:layout_width="0dp"15. android:layout_height="wrap_content"16. android:layout_weight="1"17. android:text="/data/attachment/forum18. /201511/04/121947sci9qd732yyyyyh4.jpg"19. android:hint=" 请输入url 地址" />20.21. <Button22. android:layout_gravity="center_vertical"23. android:layout_width="wrap_content"24. android:layout_height="wrap_content"25. android:onClick="load"26. android:text=" 确定" />27. </LinearLayout>28.29.<ImageView30. android:layout_gravity="center_horizontal"31. android:id="@+id/iv"32. android:layout_width="wrap_content"33. android:layout_height="wrap_content" />34.35.</LinearLayout>注意:上面第17行布局代码中我将一个网络图片的地址“写死”了,因为图片地址的url太长了,因此仅仅为了方便演示才这么做的。
Android图片浏览器
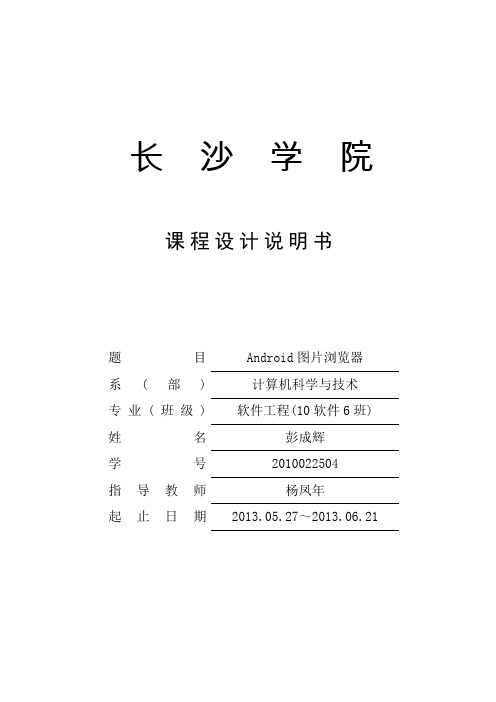
长沙学院课程设计说明书题目Android图片浏览器系(部) 计算机科学与技术专业(班级) 软件工程(10软件6班) 姓名彭成辉学号2010022504指导教师杨凤年起止日期2013.05.27~2013.06.21课程设计任务书课程名称:综合实训Ⅱ题目:Android图片浏览器已知技术参数和设计要求:设计基于Android平台的图片浏览器程序,具体要求如下:1.图片文件的管理功能。
在配置文件中配置访问SD卡文件的权限,使用File类访问SD卡上的图片文件。
2.用户浏览图片的功能。
用户通过触摸屏操作选择图片进行浏览,使用事件响应函数响应用户的操作,可以使用ImageView在屏幕中显示图片。
3.用户编辑图片的功能。
用户可以放大、缩小、旋转和裁剪图片。
4.设置壁纸功能。
将选择的图片设置为壁纸,可以使用WallPaper类来完成该功能。
5.图片文件的排序功能和查找功能。
根据文件的名称、日期或者其他属性进行排序,根据文件的名称、日期或者其他属性进行查找。
6.使用多种视图展示图片的功能。
可以使用ListView、GridView或者Gallery类来展示图片。
各阶段具体要求:(1)开发前的准备:Android开发环境的搭建。
(2)系统分析与设计:包括程序需求分析、UI设计、业务逻辑设计等。
(3)系统编码实现:根据以上六点要求,对Android图片浏览器进行编码实现。
(4)测试:编写测试用例对程序进行测试。
设计工作量:(1)软件设计:完成问题陈述中所提到的软件以及工作量要求。
(2)论文:要求撰写不少于3000个文字的文档,详细说明各阶段具体要求。
工作计划:安排4周时间进行综合实训。
第一周----Android开发环境的搭建,软件需求分析。
第二周----UI设计、业务逻辑设计。
第三、四周----Android图片浏览器的代码实现与软件测试。
注意事项⏹提交文档长沙学院课程设计任务书(每学生1份)长沙学院课程设计论文(每学生1份)长沙学院课程设计鉴定表(每学生1份)指导教师签名:日期:2013-5-26教研室主任签名:日期:系主任签名:日期:长沙学院课程设计鉴定表摘要本文档描述了基于Android平台如何开发图片浏览器应用软件,并附带介绍了Android开发环境的搭建方法。
android手把手开发一个图片浏览器

这次我给大家讲解一个Android图片浏览器的应用。
一:Android是什么Android是基于Linux内核的软件平台和操作系统,早期由Google开发,后由开放手机联盟Open Handset Alliance)开发。
它采用了软件堆层(software stack,又名以软件叠层)的架构,主要分为三部分。
低层以Linux内核工作为基础,只提供基本功能;其他的应用软件则由各公司自行开发,以Java作为编写程序的一部分。
另外,为了推广此技术,Google 和其它几十个手机公司建立了开放手机联盟。
Android在未公开之前常被传闻为Google电话或gPhone。
大多传闻认为Google开发的是自己的手机电话产品,而不是一套软件平台。
到了2010年1月,Google开始发表自家品牌手机电话的Nexus One。
目前最新版本为Android2.1。
下图是它的结构:简单来讲,Android就是一个开源的手机软件开发工具。
我主要给大家讲应用方面,大家如果有兴趣,可以了解相关基本知识。
要开发一个Android应用,首先得搭建Android开发环境:下载并安装Android sdk(Software Development Kit, 即软件开发工具包)。
由于Android开发是集成在Eclipse中,需下载并安装ADT(Eclipse集成Android sdk插件)。
搭建环境会用一个专门的章节为大家详细讲解,这里我就不再赘述。
二:Android程序的创建开发环境搭好后,我们先启动Eclipse创建一个Android的应用程序,然后在左上角单击File→New→Android Project,如下图所示:如果在图中Java Project找不到Android Project:在左上角单击File→New→Other:在弹出框中Android文件件中选中Android Project,然后单击Next进入下一步:弹出一个列表框:下面对这个列表的一些重要属性进行讲解:1.应用程序名称以及内容栏:2.工具栏:采用的编译工具即Android模拟器:3.属性栏:即应用程序中的相关属性:三:第一个Android应用程序这就是我们刚才创建的一个Android应用程序如下图:在这里面,我们最关心的是界面(main.xml)与后台(Test1.java):单击main.xml:我们先看看中间视图:这是一个页面编辑器模式:在左下角点击main.xml切换到界面编码模式:这就是刚才页面视图的源码:下面让我们看看后台(Test1.java)源码:单击Test1.java:以下将此类程序称之为activity(活动),该activity运行时会自动调用onCreate方法:而上图中onCreate方法是启动res文件夹下的layout下的main.xml界面。
Android图片浏览器
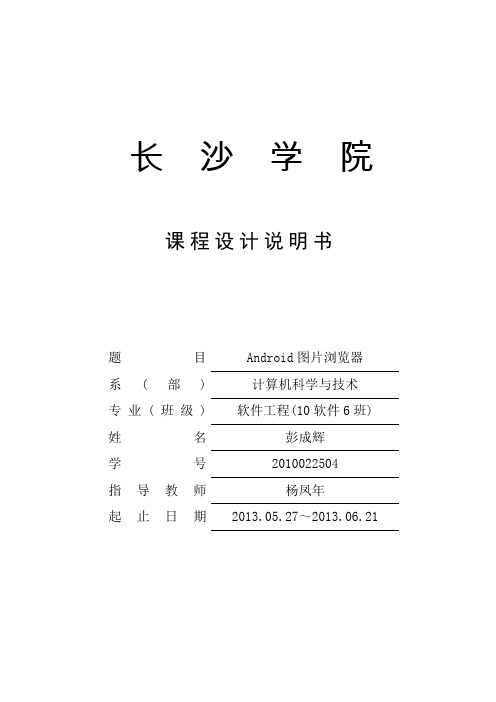
长沙学院课程设计说明书题目Android图片浏览器系(部) 计算机科学与技术专业(班级) 软件工程(10软件6班) 姓名彭成辉学号2010022504指导教师杨凤年起止日期2013.05.27~2013.06.21课程设计任务书课程名称:综合实训Ⅱ题目:Android图片浏览器已知技术参数和设计要求:设计基于Android平台的图片浏览器程序,具体要求如下:1.图片文件的管理功能。
在配置文件中配置访问SD卡文件的权限,使用File类访问SD卡上的图片文件。
2.用户浏览图片的功能。
用户通过触摸屏操作选择图片进行浏览,使用事件响应函数响应用户的操作,可以使用ImageView在屏幕中显示图片。
3.用户编辑图片的功能。
用户可以放大、缩小、旋转和裁剪图片。
4.设置壁纸功能。
将选择的图片设置为壁纸,可以使用WallPaper类来完成该功能。
5.图片文件的排序功能和查找功能。
根据文件的名称、日期或者其他属性进行排序,根据文件的名称、日期或者其他属性进行查找。
6.使用多种视图展示图片的功能。
可以使用ListView、GridView或者Gallery类来展示图片。
各阶段具体要求:(1)开发前的准备:Android开发环境的搭建。
(2)系统分析与设计:包括程序需求分析、UI设计、业务逻辑设计等。
(3)系统编码实现:根据以上六点要求,对Android图片浏览器进行编码实现。
(4)测试:编写测试用例对程序进行测试。
设计工作量:(1)软件设计:完成问题陈述中所提到的软件以及工作量要求。
(2)论文:要求撰写不少于3000个文字的文档,详细说明各阶段具体要求。
工作计划:安排4周时间进行综合实训。
第一周----Android开发环境的搭建,软件需求分析。
第二周----UI设计、业务逻辑设计。
第三、四周----Android图片浏览器的代码实现与软件测试。
注意事项⏹提交文档长沙学院课程设计任务书(每学生1份)长沙学院课程设计论文(每学生1份)长沙学院课程设计鉴定表(每学生1份)指导教师签名:日期:2013-5-26教研室主任签名:日期:系主任签名:日期:长沙学院课程设计鉴定表摘要本文档描述了基于Android平台如何开发图片浏览器应用软件,并附带介绍了Android开发环境的搭建方法。
分享45个android实例源码
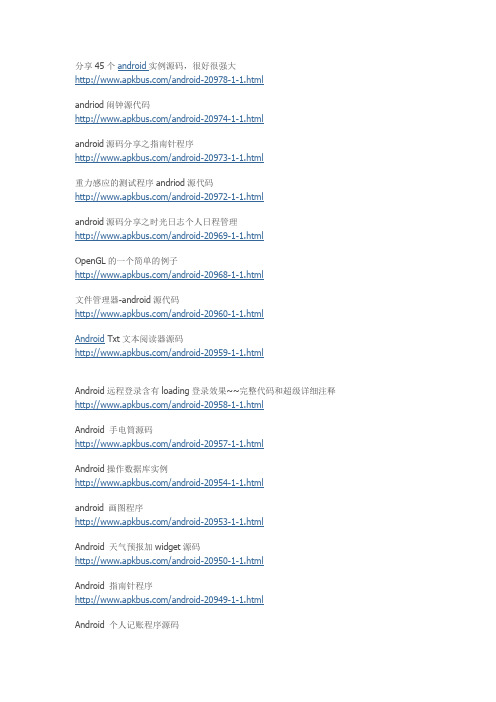
分享45个android实例源码,很好很强大/android-20978-1-1.htmlandriod闹钟源代码/android-20974-1-1.htmlandroid源码分享之指南针程序/android-20973-1-1.html重力感应的测试程序andriod源代码/android-20972-1-1.htmlandroid源码分享之时光日志个人日程管理/android-20969-1-1.htmlOpenGL的一个简单的例子/android-20968-1-1.html文件管理器-android源代码/android-20960-1-1.htmlAndroid Txt文本阅读器源码/android-20959-1-1.htmlAndroid远程登录含有loading登录效果~~完整代码和超级详细注释/android-20958-1-1.htmlAndroid 手电筒源码/android-20957-1-1.htmlAndroid操作数据库实例/android-20954-1-1.htmlandroid 画图程序/android-20953-1-1.htmlAndroid 天气预报加widget源码/android-20950-1-1.htmlAndroid 指南针程序/android-20949-1-1.htmlAndroid 个人记账程序源码Android游戏的心跳效果/android-20939-1-1.htmlAndroid PDF 阅读器源码/android-20858-1-1.htmlAndroid SqliteManager 源码/android-20857-1-1.htmlandroid 多点触控实例源码/android-20856-1-1.htmlAndroid 条码扫描程序源码/android-20855-1-1.htmlEditText插入QQ表情源码/android-20854-1-1.htmlAsyncTask进度条加载网站数据到ListView /android-20834-1-1.htmlandroid连接SQLite数据库-----增加改查+分页/android-20833-1-1.htmlAndroid 一个批量删除联系人的Demo/android-20832-1-1.htmlTXT 文本阅读器源码(android源码分享)/android-20827-1-1.htmlandroid 查询工具源代码/android-20824-1-1.htmlandroid进度条对话框Demo/android-20823-1-1.htmlAndroid实现渐显按钮的左右滑动效果/android-20752-1-1.html android天气预报源码Android 文件浏览器源码/android-20976-1-1.htmlandroid源码分享之私密通讯录源码/android-20975-1-1.htmlAndroid自定义泡泡效果源码/android-20956-1-1.htmlandroid 获取Gps信息的程序源码/android-20955-1-1.htmlandroid 超炫的图片浏览器/android-20952-1-1.htmlandroid 加载时闪烁点样式的启动画面/android-20951-1-1.html实现基于Android的英文电子词典/android-20948-1-1.html基于Android 的英文电子词典/android-20947-1-1.htmlandroid 源码之英语单词记忆程序源码/android-20936-1-1.htmlandorid 源码北京公交线路查询(离线)/android-20938-1-1.htmlAndroid 计算器源码/android-20935-1-1.html带文字的ProgressBar Demo源码/android-20831-1-1.htmlandroid自定义时钟(三种方法实现,秒针效果,详细注解)/android-20830-1-1.htmlAndroid 秒表源码分享/android-20829-1-1.htmlAndroid源代码定时情景模式切换/android-20828-1-1.htmlandroid 公交查询/android-20826-1-1.htmlandroid源码分享之带手势划动功能的日历源码/android-20825-1-1.html。
android浏览器源代码
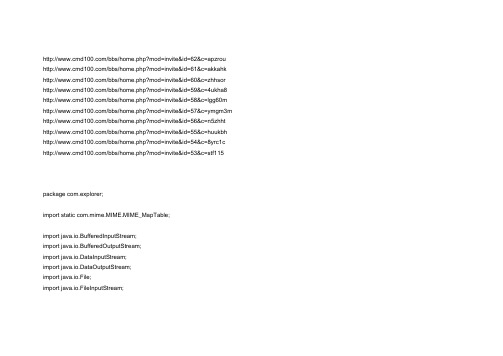
/bbs/home.php?mod=invite&id=62&c=apzrou /bbs/home.php?mod=invite&id=61&c=akkahk /bbs/home.php?mod=invite&id=60&c=zhhsor /bbs/home.php?mod=invite&id=59&c=4ukha8 /bbs/home.php?mod=invite&id=58&c=lgg60m /bbs/home.php?mod=invite&id=57&c=ymgm3m /bbs/home.php?mod=invite&id=56&c=n5zhht /bbs/home.php?mod=invite&id=55&c=huukbh /bbs/home.php?mod=invite&id=54&c=8yrc1c /bbs/home.php?mod=invite&id=53&c=stf115package com.explorer;import static com.mime.MIME.MIME_MapTable;import java.io.BufferedInputStream;import java.io.BufferedOutputStream;import java.io.DataInputStream;import java.io.DataOutputStream;import java.io.File;import java.io.FileInputStream;import java.io.FileOutputStream; import java.util.ArrayList;import java.util.Arrays;import parator;import java.util.HashMap;import java.util.List;import java.util.Map;import android.app.Activity;import android.app.AlertDialog;import android.app.Dialog;import android.app.ProgressDialog; import android.content.DialogInterface; import android.content.Intent;import .Uri;import android.os.Bundle;import android.os.Environment; import android.os.Handler;import android.os.Looper;import android.os.Message;import youtInflater; import android.view.Menu;import android.view.MenuInflater;import android.view.MenuItem;import android.view.View;import android.view.View.OnClickListener;import android.widget.AdapterView;import android.widget.AdapterView.OnItemClickListener;import android.widget.AdapterView.OnItemLongClickListener;import android.widget.Button;import android.widget.EditText;import android.widget.ListAdapter;import android.widget.ListView;import android.widget.SimpleAdapter;import android.widget.TextView;import android.widget.Toast;import com.file.R;public class MainActivity extends Activity implements OnClickListener, OnItemClickListener, OnItemLongClickListener { private TextView currentDir;private Button btnC;private Button btnE;private ListView listView;private File rootDir;private File copyPath;private String flag;private String startFilePath;private String desFilePath;private List<File> fileList = new ArrayList<File>();private ProgressDialog progressDialog;private int currentLen = 0;private long totaLength = 0;private Handler messageHandler;/** Called when the activity is first created. */@Overridepublic void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState);setContentView(yout.main);currentDir = (TextView) findViewById(R.id.currentDir);// fileName = (TextView) findViewById();btnC = (Button) findViewById(R.id.btnC);btnE = (Button) findViewById(R.id.btnE);btnC.setOnClickListener(this);btnE.setOnClickListener(this);listView = (ListView) findViewById(R.id.listView);listView.setOnItemClickListener(this);listView.setOnItemLongClickListener(this);//得到当前线程的Looper实例,由于当前线程是UI线程也可以通过Looper.getMainLooper()得到messageHandler = new MessageHandler(Looper.myLooper());if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) { rootDir = Environment.getExternalStorageDirectory();} else {rootDir = Environment.getRootDirectory();}loadFiles(rootDir);}//自定义Handlerclass MessageHandler extends Handler {public MessageHandler(Looper looper) {super(looper);}@Overridepublic void handleMessage(Message msg) {loadFiles(new File(currentDir.getText().toString()));}}@Overridepublic boolean onCreateOptionsMenu(Menu menu) {MenuInflater inflater = getMenuInflater();inflater.inflate(R.menu.menu, menu);return true;}@Overridepublic boolean onOptionsItemSelected(MenuItem item) {if (item.getItemId() == R.id.newFile) {LayoutInflater factory = LayoutInflater.from(MainActivity.this);final View view = factory.inflate(yout.rename, null);AlertDialog d = new AlertDialog.Builder(MainActivity.this).setCancelable(true).setMessage("文件夹名") .setView(view).setPositiveButton("确定", new DialogInterface.OnClickListener() {@Overridepublic void onClick(DialogInterface dialog, int which) {String dirName = ((EditText) view.findViewById(R.id.rename)).getText().toString();String newFile = currentDir.getText().toString() + "/" + dirName;if (new File(newFile).exists()) {Toast.makeText(MainActivity.this, "文件夹已存在", Toast.LENGTH_LONG).show();return;}File f = new File(currentDir.getText().toString(), dirName);f.mkdir();}}).create();d.show();} else if (item.getItemId() == R.id.about) {Dialog d = new AlertDialog.Builder(MainActivity.this).setTitle("文件浏览器1.0beta").setMessage("本程序由劲松Alex制作") .setPositiveButton("确定", null).create();d.show();} else if (item.getItemId() == R.id.exit) {MainActivity.this.finish();}return true;}/*** 加载当前文件夹列表* */public void loadFiles(File dir) {List<Map<String, Object>> list = new ArrayList<Map<String, Object>>();if (dir != null) {// 处理上级目录if (!dir.getAbsolutePath().equals(rootDir.getAbsolutePath())) {Map<String, Object> map = new HashMap<String, Object>();map.put("file", dir.getParentFile());map.put("name", "上一级目录");map.put("img", R.drawable.folder);list.add(map);}currentDir.setText(dir.getAbsolutePath());File[] files = dir.listFiles();sortFiles(files);if (files != null) {for (File f : files) {Map<String, Object> map = new HashMap<String, Object>();map.put("file", f);map.put("name", f.getName());map.put("img", f.isDirectory() ? R.drawable.folder: (f.getName().toLowerCase().endsWith(".zip") ? R.drawable.zip : R.drawable.text));list.add(map);}}} else {Toast.makeText(this, "目录不正确,请输入正确的目录!", Toast.LENGTH_LONG).show();}ListAdapter adapter = new SimpleAdapter(this, list, yout.item, new String[] { "name", "img" }, new int[] {, R.id.icon });// listView.setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);listView.setAdapter(adapter);}/*** 排序文件列表* */private void sortFiles(File[] files) {Arrays.sort(files, new Comparator<File>() {public int compare(File file1, File file2) {if (file1.isDirectory() && file2.isDirectory())return 1;if (file2.isDirectory())return 1;return -1;}});}/*** 打开文件** @param file*/private void openFile(File file) {Intent intent = new Intent();intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);// 设置intent的Action属性intent.setAction(Intent.ACTION_VIEW);// 获取文件file的MIME类型String type = getMIMEType(file);// 设置intent的data和Type属性。
android实战之实现图片滚动控件,自动播放

Android图片滚动,加入自动播放功能,使用自定义属性实现,霸气十足!大家好,记得上次我带着大家一起实现了一个类似与淘宝客户端中带有的图片滚动播放器的效果,但是在做完了之后,发现忘了加入图片自动播放的功能(或许是我有意忘记加的.....),结果图片只能通过手指滑动来播放。
于是今天我将再次带领大家,添加上之前遗漏的功能,让我们的图片播放器更加完善。
这次的程序开发将完全基于上一次的代码,如果有朋友还未看过上篇文章,请先阅读Android实现图片滚动控件,含页签功能,让你的应用像淘宝一样炫起来。
既然是要加入自动播放的功能,那么就有一个非常重要的问题需要考虑。
如果当前已经滚动到了最后一张图片,应该怎么办?由于我们目前的实现方案是,所有的图片都按照布局文件里面定义的顺序横向排列,然后通过偏移第一个图片的leftMargin,来决定显示哪一张图片。
因此当图片滚动在最后一张时,我们可以让程序迅速地回滚到第一张图片,然后从头开始滚动。
这种效果和淘宝客户端是有一定差异的(淘宝并没有回滚机制,而是很自然地由最后一张图片滚动到第一张图片),我也研究过淘宝图片滚动器的实现方法,并不难实现。
但是由于我们是基于上次的代码进行开发的,方案上无法实现和淘宝客户端一样的效果,因此这里也就不追求和它完全一致了,各有风格也挺好的。
好了,现在开始实现功能,首先是打开SlidingSwitcherView,在里面加入一个新的AsyncTask,专门用于回滚到第一张图片:[java]view plaincopy1.class ScrollToFirstItemTask extends AsyncTask<Integer, Integer, Integer> {2.3.@Override4.protected Integer doInBackground(Integer... speed) {5.int leftMargin = firstItemParams.leftMargin;6.while (true) {7. leftMargin = leftMargin + speed[0];8.// 当leftMargin大于0时,说明已经滚动到了第一个元素,跳出循环9.if (leftMargin > 0) {10. leftMargin = 0;11.break;12. }13. publishProgress(leftMargin);14. sleep(20);15. }16.return leftMargin;17. }18.19.@Override20.protected void onProgressUpdate(Integer... leftMargin) {21. firstItemParams.leftMargin = leftMargin[0];22. firstItem.setLayoutParams(firstItemParams);23. }24.25.@Override26.protected void onPostExecute(Integer leftMargin) {27. firstItemParams.leftMargin = leftMargin;28. firstItem.setLayoutParams(firstItemParams);29. }30.31.}然后在SlidingSwitcherView里面加入一个新的方法:[java]view plaincopy1./**2. * 滚动到第一个元素。
Android图片轮播代码

package ingbanner;import android.content.Context;import android.os.Bundle;import android.support.v7.app.AppCompatActivity;import android.util.Log;import android.widget.ImageView;import android.widget.Toast;import com.bumptech.glide.Glide;import com.youth.banner.Banner;import com.youth.banner.listener.OnBannerListener;import com.youth.banner.loader.ImageLoader;import java.util.ArrayList;import java.util.List;public class MainActivity extends AppCompatActivity implements OnBannerListener { private Banner banner;@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(yout.activity_main);List images= new ArrayList<>();images.add(R.mipmap.a);images.add(R.mipmap.b);images.add(R.mipmap.c);banner = (Banner) findViewById(R.id.banner);//设置图片加载器banner.setImageLoader(new GlideImageLoader());//设置图片集合banner.setImages(images);//banner设置方法全部调用完毕时最后调用banner.start();//设置监听器banner.setOnBannerListener(this);}@Overridepublic void OnBannerClick(int position) {Log.d("MainActivity","图片被点击");Toast.makeText(getApplicationContext(),"你点击了:"+position,Toast.LENGTH_SHORT).show();}private class GlideImageLoader extends ImageLoader {@Overridepublic void displayImage(Context context, Object path, ImageView imageView) { //Glide 加载图片Glide.with(context).load(path).into(imageView);}}//如果你需要考虑更好的体验,可以这么操作@Overrideprotected void onStart() {super.onStart();//开始轮播banner.startAutoPlay();}@Overrideprotected void onStop() {super.onStop();//结束轮播banner.stopAutoPlay();}}。
Android超大图长图浏览库SubsamplingScaleImageView源码解析

Android超⼤图长图浏览库SubsamplingScaleImageView源码解析⼀开始没打算分析 SubsamplingScaleImageView 这个开源的图⽚浏览器的,因为这个库在我们 App 中使⽤了,觉得⾃⼰对这个库还是⽐较熟悉的,结果某天再看看到源码介绍的时候,才发现⾃⼰对其了解并不够深⼊,所以这才打算再细细看看源码的实现,同时记录⽅便以后回顾。
那么 SubsamplingScaleImageView 有啥优点呢?1. 采⽤ GestureDetector 进⾏⼿势控制,⽀持图⽚的点击,双击,滑动等来控制的放⼤缩⼩;2. 使⽤了 BitmapRegionDecoder,具有分块加载功能;3. ⽀持查看长图,超⼤图上⾯的优点简直就是⾮常实⽤,基本上拿来就可以直接⽤,简单省⼒。
下⾯就是要来分析,它是如何满⾜这些优点的。
源码分析⾸先附上源码地址:使⽤说明如果可以拿到图⽚的资源id,assert或者⽂件路径,直接使⽤下⾯⽅式进⾏使⽤:SubsamplingScaleImageView imageView = (SubsamplingScaleImageView)findViewById(id.imageView);imageView.setImage(ImageSource.resource(R.drawable.monkey));// ... or ...imageView.setImage(ImageSource.asset("map.png"))// ... or ...imageView.setImage(ImageSource.uri("/sdcard/DCIM/DSCM00123.JPG"));如果可以拿到 bitmap 就可以这么使⽤:SubsamplingScaleImageView imageView = (SubsamplingScaleImageView)findViewById(id.imageView);imageView.setImage(ImageSource.bitmap(bitmap));可以看到使⽤是⾮常简单的。
Android实现图片点击预览效果(zoom动画)
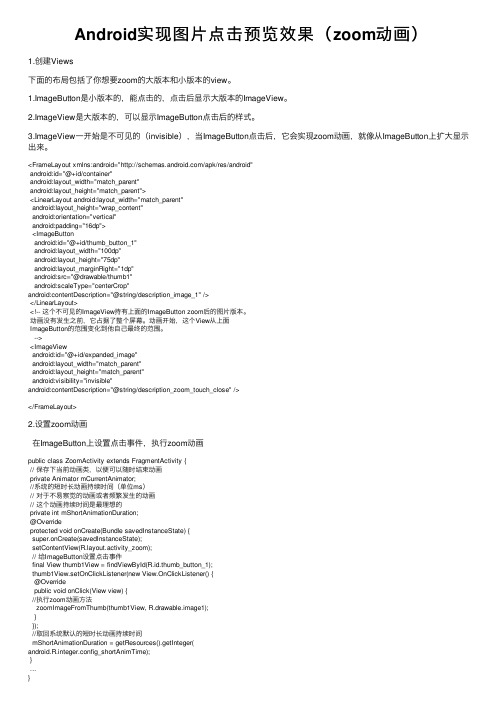
Android实现图⽚点击预览效果(zoom动画)1.创建Views下⾯的布局包括了你想要zoom的⼤版本和⼩版本的view。
1.ImageButton是⼩版本的,能点击的,点击后显⽰⼤版本的ImageView。
2.ImageView是⼤版本的,可以显⽰ImageButton点击后的样式。
3.ImageView⼀开始是不可见的(invisible),当ImageButton点击后,它会实现zoom动画,就像从ImageButton上扩⼤显⽰出来。
<FrameLayout xmlns:android="/apk/res/android"android:id="@+id/container"android:layout_width="match_parent"android:layout_height="match_parent"><LinearLayout android:layout_width="match_parent"android:layout_height="wrap_content"android:orientation="vertical"android:padding="16dp"><ImageButtonandroid:id="@+id/thumb_button_1"android:layout_width="100dp"android:layout_height="75dp"android:layout_marginRight="1dp"android:src="@drawable/thumb1"android:scaleType="centerCrop"android:contentDescription="@string/description_image_1" /></LinearLayout><!-- 这个不可见的ImageView持有上⾯的ImageButton zoom后的图⽚版本。
Android简单文件浏览器源代码(转)
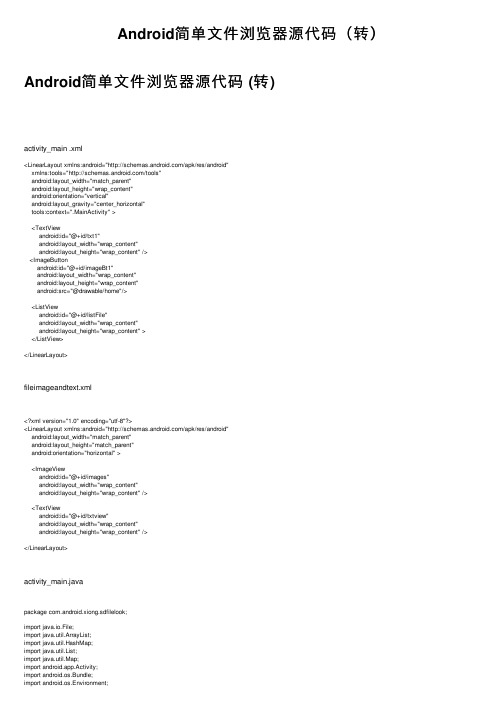
Android简单⽂件浏览器源代码(转)Android简单⽂件浏览器源代码 (转)activity_main .xml<LinearLayout xmlns:android="/apk/res/android"xmlns:tools="/tools"android:layout_width="match_parent"android:layout_height="wrap_content"android:orientation="vertical"android:layout_gravity="center_horizontal"tools:context=".MainActivity" ><TextViewandroid:id="@+id/txt1"android:layout_width="wrap_content"android:layout_height="wrap_content" /><ImageButtonandroid:id="@+id/imageBt1"android:layout_width="wrap_content"android:layout_height="wrap_content"android:src="@drawable/home"/><ListViewandroid:id="@+id/listFile"android:layout_width="wrap_content"android:layout_height="wrap_content" ></ListView></LinearLayout>fileimageandtext.xml<?xml version="1.0" encoding="utf-8"?><LinearLayout xmlns:android="/apk/res/android"android:layout_width="match_parent"android:layout_height="match_parent"android:orientation="horizontal" ><ImageViewandroid:id="@+id/images"android:layout_width="wrap_content"android:layout_height="wrap_content" /><TextViewandroid:id="@+id/txtview"android:layout_width="wrap_content"android:layout_height="wrap_content" /></LinearLayout>activity_main.javapackage com.android.xiong.sdfilelook;import java.io.File;import java.util.ArrayList;import java.util.HashMap;import java.util.List;import java.util.Map;import android.app.Activity;import android.os.Bundle;import android.os.Environment;import android.view.Menu;import android.view.View;import android.view.View.OnClickListener;import android.widget.AdapterView;import android.widget.AdapterView.OnItemClickListener;import android.widget.ImageButton;import android.widget.ImageView;import android.widget.ListView;import android.widget.SimpleAdapter;import android.widget.TextView;public class MainActivity extends Activity {private ListView listfile;//当前⽂件⽬录private String currentpath;private TextView txt1;private ImageView images;private TextView textview;private ImageButton imagebt1;private int[] img = { R.drawable.file, R.drawable.folder, R.drawable.home }; private File[] files;private SimpleAdapter simple;@Overrideprotected void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);setContentView(yout.activity_main);listfile = (ListView) findViewById(R.id.listFile);txt1 = (TextView) findViewById(R.id.txt1);imagebt1 = (ImageButton) findViewById(R.id.imageBt1);init(Environment.getExternalStorageDirectory());listfile.setOnItemClickListener(new OnItemClickListener() {@Overridepublic void onItemClick(AdapterView<?> arg0, View arg1, int arg2,long arg3) {// TODO Auto-generated method stub// 获取单击的⽂件或⽂件夹的名称String folder = ((TextView) arg1.findViewById(R.id.txtview)).getText().toString();try {File filef = new File(currentpath + '/'+ folder);init(filef);} catch (Exception e) {e.printStackTrace();}}});//回根⽬录imagebt1.setOnClickListener(new OnClickListener() {@Overridepublic void onClick(View v) {init(Environment.getExternalStorageDirectory());}});}// 界⾯初始化public void init(File f) {if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {// 获取SDcard⽬录下所有⽂件名files = f.listFiles();if (!files.equals(null)) {currentpath=f.getPath();txt1.setText("当前⽬录为:"+f.getPath());List<Map<String, Object>> list = new ArrayList<Map<String, Object>>(); for (int i = 0; i < files.length; i++) {Map<String, Object> maps = new HashMap<String, Object>();if (files[i].isFile())maps.put("image", img[0]);elsemaps.put("image", img[1]);maps.put("filenames", files[i].getName());list.add(maps);}simple = new SimpleAdapter(this, list,yout.fileimageandtext, new String[] { "image","filenames" }, new int[] { R.id.images,R.id.txtview });listfile.setAdapter(simple);}} else {System.out.println("该⽂件为空");}}@Overridepublic boolean onCreateOptionsMenu(Menu menu) {// Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu);return true;}}。
Android最流行的的图片加载源码分析
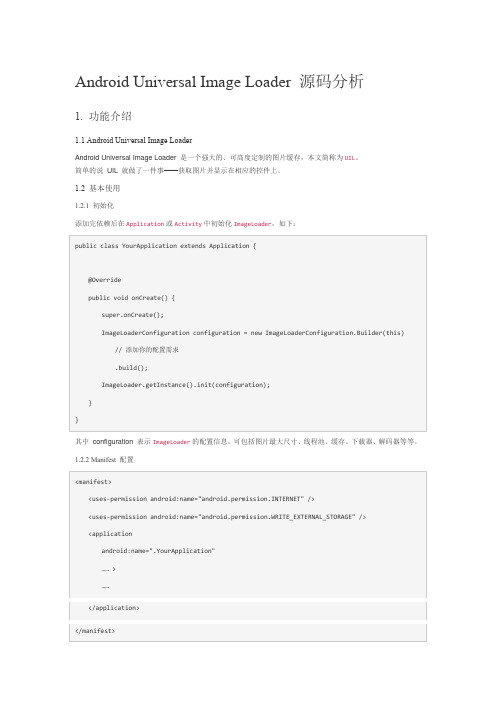
Android Universal Image Loader 源码分析1. 功能介绍1.1 Android Universal Image LoaderAndroid Universal Image Loader 是一个强大的、可高度定制的图片缓存,本文简称为UIL。
简单的说UIL 就做了一件事——获取图片并显示在相应的控件上。
1.2 基本使用1.2.1 初始化添加完依赖后在Application或Activity中初始化ImageLoader,如下:其中configuration 表示ImageLoader的配置信息,可包括图片最大尺寸、线程池、缓存、下载器、解码器等等。
1.2.2 Manifest 配置添加网络权限。
如果允许磁盘缓存,需要添加写外设的权限。
1.2.3 下载显示图片下载图片,解析为Bitmap 并在ImageView 中显示。
下载图片,解析为Bitmap 传递给回调接口。
以上是简单使用,更复杂API 见本文详细设计。
1.3 特点∙可配置度高。
支持任务线程池、下载器、解码器、内存及磁盘缓存、显示选项等等的配置。
∙包含内存缓存和磁盘缓存两级缓存。
∙支持多线程,支持异步和同步加载。
∙支持多种缓存算法、下载进度监听、ListView 图片错乱解决等。
2. 总体设计2.1. 总体设计图上面是UIL 的总体设计图。
整个库分为ImageLoaderEngine,Cache及ImageDownloader,ImageDecoder,BitmapDisplayer,BitmapProcessor五大模块,其中Cache分为MemoryCache和DiskCache两部分。
简单的讲就是ImageLoader收到加载及显示图片的任务,并将它交给ImageLoaderEngine,ImageLoaderEngine分发任务到具体线程池去执行,任务通过Cache及ImageDownloader获取图片,中间可能经过BitmapProcessor和ImageDecoder处理,最终转换为Bitmap交给BitmapDisplayer在ImageAware中显示。
简单手机程序--图片浏览器(可拖动)
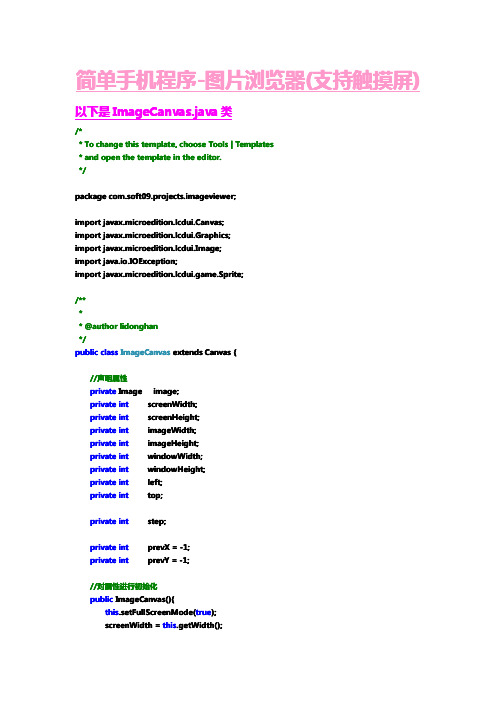
imageWidth;
private int
imageHeight;
private int
windowWidth;
private int
windowHeight;
private int
left;
private int
top;
private int
step;
private int private int
image = Image.createImage("/1.jpg"); //加载图片 imageWidth = image.getWidth(); imageHeight = image.getHeight(); windowWidth = screenWidth < imageWidth? screenWidth:imageWidth; windowHeight = screenHeight < imageHeight? screenHeight:imageHeight; }catch(IOException e){ System.err.println("无法装入图像!"); } } public void paint(Graphics g){ if(image != null){ g.drawImage(Image.createImage(image,
import javax.microedition.lcdui.Canvas; import javax.microedition.lcdui.Graphics; import javax.microedition.lcdui.Image; import java.io.IOException; import javax.microedition.lcdui.game.Sprite;
android自定义ImageView实现缩放-回弹效果

android自定义ImageView实现缩放,回弹效果androidImageview缩放回弹话不多说上代码:MainActivity.javapublic class MainActivity extends Activity{private LinearLayout ll_viewArea;private youtParams parm;private ViewArea viewArea;@Overridepublic void onCreate(Bundle savedInstanceState) {super.onCreate(savedInstanceState);//去除titlerequestWindowFeature(Window.FEATURE_NO_TITLE);//去掉Activity上面的状态栏getWindow().setFlags(youtParams.FLAG_FULLSCREEN,youtPara ms. FLAG_FULLSCREEN);setContentView(yout.main);ll_viewArea = (LinearLayout)findViewById(R.id.ll_viewArea);parm = newyoutParams(youtParams.FILL_PARENT, youtParams.FILL_PARENT);viewArea = newViewArea(MainActivity.this,R.drawable.psu); //自定义布局控件,用来初始化并存放自定义imageViewll_viewArea.addView(viewArea,parm);}}这段代码中要注意的问题是去掉title和状态栏两句代码必须放到setContentView(yout.main);话的前面。
而且这两句话必须有,因为后面计算回弹距离是根据全屏计算的(我的i9000就是480x800),如果不去掉title 和状态栏,后面的回弹会有误差,总是回弹不到想要的位置。
图片查看器部分代码
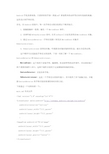
Android手机查看相册,只需轻轻的手指一滑就ok?看似简单的动作背后却有很深的奥秘,这里设计到手势识别。
首先,在Android系统中,每一次手势交互都会依照以下顺序执行。
1. 接触接触屏一刹那,触发一个MotionEvent事件。
2. 该事件被OnTouchListener监听,在其onTouch()方法里获得该MotionEvent对象。
3. 通过GestureDetector(手势识别器)转发次MotionEvent对象至OnGestureListener。
4. OnGestureListener获得该对象,听根据该对象封装的的信息,做出合适的反馈。
这个顺序可以说就是手势交互的原理,下面一同来了解一下MotionEvent、GestureDetector和OnGestureListener。
MotionEvent:这个类用于封装手势、触摸笔、轨迹球等等的动作事件。
其内部封装了两个重要的属性X和Y,这两个属性分别用于记录横轴和纵轴的坐标。
GestureDetector:识别各种手势。
OnGestureListener:这是一个手势交互的监听接口,其中提供了多个抽象方法,并根据GestureDetector的手势识别结果调用相对应的方法。
下面通过一个实例来看一下:main.xml布局文件<?xml version="1.0" encoding="utf-8"?><LinearLayout xmlns:android="/apk/res/android"android:orientation="vertical"android:layout_width="fill_parent"android:layout_height="fill_parent"><ImageView android:id="@+id/image"android:layout_width="fill_parent"android:layout_height="fill_parent"android:layout_gravity="center"/></LinearLayout>布局文件很简单,就是一个ImageView。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Android超炫图片浏览器代码使用过Android自带的gallery组件的人都知道,gallery实现的效果就是拖动浏览一组图片,相比iphone里也是用于拖动浏览图片的coverflow,显然逊色不少。
实际上,可以通过扩展gallery,通过伪3D变换可以基本实现coverflow的效果。
本文通过源代码解析这一功能的实现。
具体代码作用可参照注释。
最终实现效果如下:要使用gallery,我们必须首先给其指定一个adapter。
在这里,我们实现了一个自定义的ImageAdapter,为图片制作倒影效果。
传入参数为context和程序内drawable中的图片ID数组。
之后调用其中的createReflectedImages()方法分别创造每一个图像的倒影效果,生成对应的ImageView数组,最后在getView()中返回。
/** Copyright (C) 2010 Neil Davies** Licensed under the Apache License, Version 2.0 (the "License");* you may not use this file except in compliance with the License.* You may obtain a copy of the License at** /licenses/LICENSE-2.0** Unless required by applicable law or agreed to in writing, software* distributed under the License is distributed on an "AS IS" BASIS,* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.* See the License for the specific language governing permissions and* limitations under the License.** This code is base on the Android Gallery widget and was Created* by Neil Davies neild001 'at' gmail dot com to be a Coverflow widget** @author Neil Davies*/public class ImageAdapter extends BaseAdapter {int mGalleryItemBackground;private Context mContext;private Integer[] mImageIds ;private ImageView[] mImages;public ImageAdapter(Context c, int[] ImageIds) {mContext = c;mImageIds = ImageIds;mImages = new ImageView[mImageIds.length];}public boolean createReflectedImages() {// The gap we want between the reflection and the original image final int reflectionGap = 4;int index = 0;for (int imageId : mImageIds) {Bitmap originalImage = BitmapFactory.decodeResource( mContext.getResources(), imageId);int width = originalImage.getWidth();int height = originalImage.getHeight();// This will not scale but will flip on the Y axisMatrix matrix = new Matrix();matrix.preScale(1, -1);// Create a Bitmap with the flip matrix applied to it.// We only want the bottom half of the imageBitmap reflectionImage = Bitmap.createBitmap(originalImage, 0, height / 2, width, height / 2, matrix, false);// Create a new bitmap with same width but taller to fit// reflectionBitmap bitmapWithReflection = Bitmap.createBitmap(width, (height + height / 2), Config.ARGB_8888);// Create a new Canvas with the bitmap that's big enough for// the image plus gap plus reflectionCanvas canvas = new Canvas(bitmapWithReflection);// Draw in the original imagecanvas.drawBitmap(originalImage, 0, 0, null);// Draw in the gapPaint deafaultPaint = new Paint();canvas.drawRect(0, height, width, height + reflectionGap, deafaultPaint);// Draw in the reflectioncanvas.drawBitmap(reflectionImage, 0, height + reflectionGap,null);// Create a shader that is a linear gradient that covers the// reflectionPaint paint = new Paint();LinearGradient shader = new LinearGradient(0,originalImage.getHeight(), 0,bitmapWithReflection.getHeight() + reflectionGap,0x70ffffff, 0x00ffffff, TileMode.CLAMP);// Set the paint to use this shader (linear gradient)paint.setShader(shader);// Set the Transfer mode to be porter duff and destination inpaint.setXfermode(new PorterDuffXfermode(Mode.DST_IN));// Draw a rectangle using the paint with our linear gradientcanvas.drawRect(0, height, width,bitmapWithReflection.getHeight() + reflectionGap, paint);ImageView imageView = new ImageView(mContext);imageView.setImageBitmap(bitmapWithReflection);imageView.setLayoutParams(new youtParams(160, 240));// imageView.setScaleType(ScaleType.MATRIX);mImages[index++] = imageView;}return true;}public int getCount() {return mImageIds.length;}public Object getItem(int position) {return position;}public long getItemId(int position) {return position;}public View getView(int position, View convertView, ViewGroup parent) { // Use this code if you want to load from resources/** ImageView i = new ImageView(mContext);* i.setImageResource(mImageIds[position]); i.setLayoutParams(new* youtParams(350,350));* i.setScaleType(ImageView.ScaleType.CENTER_INSIDE);** //Make sure we set anti-aliasing otherwise we get jaggies* BitmapDrawable drawable = (BitmapDrawable) i.getDrawable();* drawable.setAntiAlias(true); return i;*/return mImages[position];}/*** Returns the size (0.0f to 1.0f) of the views depending on the* 'offset' to the center.*/public float getScale(boolean focused, int offset) {/* Formula: 1 / (2 ^ offset) */return Math.max(0, 1.0f / (float) Math.pow(2, Math.abs(offset)));}}}复制代码仅仅实现了图片的倒影效果还不够,因为在coverflow中图片切换是有旋转和缩放效果的,而自带的gallery中并没有实现。