编写一个简易计算器的源代码
简易计算器程序源代码

简易计算器程序源代码下面是一个简易计算器程序的源代码,它可以执行基本的四则运算:```python#定义加法函数def add(x, y):return x + y#定义减法函数def subtract(x, y):return x - y#定义乘法函数def multiply(x, y):return x * y#定义除法函数def divide(x, y):if y == 0:return "除数不能为0"else:return x / y#显示菜单print("选择操作:")print("1. 相加")print("2. 相减")print("3. 相乘")print("4. 相除")#获取用户输入choice = input("输入你的选择(1/2/3/4): ")#获取用户输入的两个数字num1 = float(input("输入第一个数字: "))num2 = float(input("输入第二个数字: "))#根据用户选择执行相应操作if choice == '1':print(num1, "+", num2, "=", add(num1, num2))elif choice == '2':print(num1, "-", num2, "=", subtract(num1, num2)) elif choice == '3':print(num1, "*", num2, "=", multiply(num1, num2)) elif choice == '4':print(num1, "/", num2, "=", divide(num1, num2))else:print("请输入有效的选择")```运行这个程序,你将看到一个简易的计算器菜单。
简单计算器c语言源码

#include <stdio.h>#include <string.h>#include <ctype.h>#include <math.h>//expression evaluate#define iMUL 0#define iDIV 1#define iADD 2#define iSUB 3#define iCap 4//#define LtKH 5//#define RtKH 6#define MaxSize 100void iPush(float);float iPop();float StaOperand[MaxSize];int iTop=-1;//char Srcexp[MaxSize];char Capaexp[MaxSize];char RevPolishexp[MaxSize];float NumCapaTab[26];char validexp[]="*/+-()";char NumSets[]="0123456789";char StackSymb[MaxSize];int operands;//void NumsToCapas(char [], int , char [], float []);int CheckExpress(char);int PriorChar(char,char);int GetOperator(char [], char);void counterPolishexp(char INexp[], int slen, char Outexp[]); float CalcRevPolishexp(char [], float [], char [], int);void main(){char ch;char s;int bl=1;while(bl==1){int ilen;float iResult=0.0;printf("输入计算表达式(最后以=号结束):\n");memset(StackSymb,0,MaxSize);memset(NumCapaTab,0,26); //A--NO.1, B--NO.2, etc.gets(Srcexp);ilen=strlen(Srcexp);NumsToCapas(Srcexp,ilen,Capaexp,NumCapaTab);ilen=strlen(Capaexp);counterPolishexp(Capaexp,ilen,RevPolishexp);ilen=strlen(RevPolishexp);iResult=CalcRevPolishexp(validexp, NumCapaTab, RevPolishexp,ilen);printf("\n计算结果:\n%.6f\n",iResult);printf("是否继续运算?(Y/N)\n");ch=getchar();s=getchar();//接受回车if(ch=='y'||ch=='Y'){bl=1;}else{bl=0;}}}void iPush(float value){if(iTop<MaxSize) StaOperand[++iTop]=value;}float iPop(){if(iTop>-1)return StaOperand[iTop--];return -1.0;}void NumsToCapas(char Srcexp[], int slen, char Capaexp[], float NumCapaTab[]) {char ch;int i, j, k, flg=0;int sign;float val=0.0,power=10.0;i=0; j=0; k=0;while (i<slen){ch=Srcexp[i];if (i==0){sign=(ch=='-')?-1:1;if(ch=='+'||ch=='-'){ch=Srcexp[++i];flg=1;}}if (isdigit(ch)){val=ch-'0';while (isdigit(ch=Srcexp[++i])) {val=val*10.0+ch-'0';}if (ch=='.'){while(isdigit(ch=Srcexp[++i])) {val=val+(ch-'0')/power; power*=10;}} //end ifif(flg){val*=sign;flg=0;}} //end if//write Capaexp array// write NO.j to arrayif(val){Capaexp[k++]='A'+j; Capaexp[k++]=ch;NumCapaTab[j++]=val; //A--0, B--1,and C, etc.}else{Capaexp[k++]=ch;}val=0.0;power=10.0;//i++;}Capaexp[k]='\0';operands=j;}float CalcRevPolishexp(char validexp[], float NumCapaTab[], char RevPolishexp[], int slen) {float sval=0.0, op1,op2;int i, rt;char ch;//recursive stacki=0;while((ch=RevPolishexp[i]) && i<slen){switch(rt=GetOperator(validexp, ch)){case iMUL: op2=iPop(); op1=iPop();sval=op1*op2;iPush(sval);break;case iDIV: op2=iPop(); op1=iPop();if(!fabs(op2)){printf("overflow\n");iPush(0);break;}sval=op1/op2;iPush(sval);break;case iADD: op2=iPop(); op1=iPop();sval=op1+op2;iPush(sval);break;case iSUB: op2=iPop(); op1=iPop();sval=op1-op2;iPush(sval);break;case iCap: iPush(NumCapaTab[ch-'A']); break;default: ;}++i;}while(iTop>-1){sval=iPop();}return sval;}int GetOperator(char validexp[],char oper) {int oplen,i=0;oplen=strlen(validexp);if (!oplen) return -1;if(isalpha(oper)) return 4;while(i<oplen && validexp[i]!=oper) ++i;if(i==oplen || i>=4) return -1;return i;}int CheckExpress(char ch){int i=0;char cc;while((cc=validexp[i]) && ch!=cc) ++i;if (!cc)return 0;return 1;}int PriorChar(char curch, char stach){//栈外优先级高于(>)栈顶优先级时,才入栈//否则(<=),一律出栈if (curch==stach) return 0; //等于时应该出栈else if (curch=='*' || curch=='/'){if(stach!='*' && stach!='/')return 1;}else if (curch=='+' || curch=='-'){if (stach=='(' || stach==')')return 1;}else if (curch=='('){if (stach==')')return 1;}return 0;}void counterPolishexp(char INexp[], int slen, char Outexp[]) {int i, j, k,pr;char t;i=0;j=k=0;while (INexp[i]!='=' && i<slen){if (INexp[i]=='(')StackSymb[k++]=INexp[i];//iPush(*(INexp+i));else if(INexp[i]==')'){//if((t=iPop())!=-1)while((t=StackSymb[k-1])!='('){Outexp[j++]=t;k--;}k--;}else if (CheckExpress(INexp[i])) // is oparator{// printf("operator %c k=%d\n",INexp[i],k);while (k){// iPush(*(INexp+i));if(pr=PriorChar(INexp[i],StackSymb[k-1])) break;else{//if ((t=iPop())!=-1)t=StackSymb[k-1]; k--;Outexp[j++]=t;}} //end whileStackSymb[k++]=INexp[i]; //common process }else //if() 变量名{// printf("operand %c k=%d\n",INexp[i],k); Outexp[j++]=INexp[i];}i++; //}while (k){t=StackSymb[k-1]; k--;Outexp[j++]=t;}Outexp[j]='\0';}。
简单计算器源代码
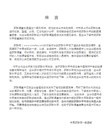
static Button b3=new Button("9");
static Button b4=new Button("/");
static Button b5=new Button("Close");
static Button C1=new Button("4");
{
int z=a.length();
int q1=a.indexOf("+");
int q2=a.indexOf("/");
int q3=a.indexOf("-");
int q4=a.indexOf("*");
if(q2>0)
}
if(q4>0)
{
String c=a.substring(0,q4);
float c1=Float.parseFloat(c);
String d=a.substring(q4+1);
String d=a.substring(q3+1);
float d1=Float.parseFloat(d);
float sum=c1-d1;
tf.setText(""+sum);
a=""+sum;
if(e.getSource()==C1)
a+="4";
if(e.getSource()==D1)
a+="1";
简易计算器源代码
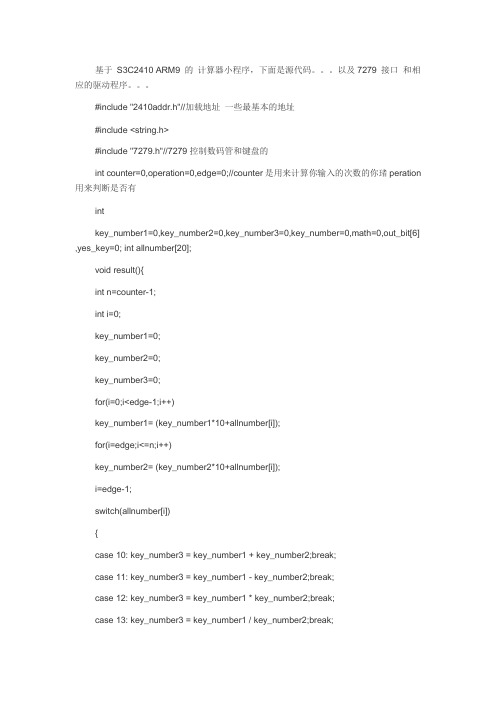
基于S3C2410 ARM9 的计算器小程序,下面是源代码。
以及7279 接口和相应的驱动程序。
#include "2410addr.h"//加载地址一些最基本的地址#include <string.h>#include "7279.h"//7279控制数码管和键盘的int counter=0,operation=0,edge=0;//counter是用来计算你输入的次数的你琽peration 用来判断是否有intkey_number1=0,key_number2=0,key_number3=0,key_number=0,math=0,out_bit[6] ,yes_key=0; int allnumber[20];void result(){int n=counter-1;int i=0;key_number1=0;key_number2=0;key_number3=0;for(i=0;i<edge-1;i++)key_number1= (key_number1*10+allnumber[i]);for(i=edge;i<=n;i++)key_number2= (key_number2*10+allnumber[i]);i=edge-1;switch(allnumber[i]){case 10: key_number3 = key_number1 + key_number2;break;case 11: key_number3 = key_number1 - key_number2;break;case 12: key_number3 = key_number1 * key_number2;break;case 13: key_number3 = key_number1 / key_number2;break;}for(i=0;i<6;i++){out_bit[i]= key_number3%0x0a;key_number3 = key_number3/0x0a;write7279(DECODE1+i,out_bit[i]);}}void __irq Keyaddnumb(void){yes_key=1;rINTMSK|=(BIT_EINT2);//key_number=read7279(CMD_READ);key_number=read7279(CMD_READ);ClearPending(BIT_EINT2); //清除中断标志rINTMSK&=~(BIT_EINT2);//yes_key=0;}void Main(){int j=0;Init7279();//EINT2 RiserGPFCON = (rGPFCON&0xff0f)|0x0060; //GPF2:Interrupt;GPF3:Out 7279CS //extern int p=0;//for(p=0;p<20;p++)allnumber[p]=0;send_byte(CMD_RESET);write7279(0x98,0xff);rEXTINT0= (rEXTINT0&0xffff00FF)|0x00000400; pISR_EINT2=(unsigned)Keyaddnumb; rINTMOD=0x0; //设置为IRQ模式rINTMSK&=~(BIT_EINT2); //打开keyboard中断do{if(yes_key==1){switch(key_number){case 0x03: key_number = 0;operation=0;break; case 0x0b: key_number = 1;operation=0;break; case 0x13: key_number = 2;operation=0;break; case 0x1b: key_number = 3;operation=0;break; case 0x04: key_number = 4;operation=0;break; case 0x0c: key_number = 5;operation=0;break; case 0x14: key_number = 6;operation=0;break; case 0x1c: key_number = 7;operation=0;break; case 0x05: key_number = 8;operation=0;break; case 0x0d: key_number = 9;operation=0;break; case 0x15: key_number = 10;operation=1;break; case 0x1d: key_number = 11;operation=2;break; case 0x06: key_number = 12;operation=3;break; case 0x0e: key_number = 13;operation=4;break; case 0x16: key_number = 14;operation=5;break;case 0x1e: key_number = 15;operation=6;break; } if(key_number==14) { result(); for(j=0;j<19;j++) allnumber[j]=0; counter=0;edge=0; } else if(key_number ==15) { // while(1){ //for(j=0;j<1000000;j++)short_delay(); send_byte(CMD_RESET); } /*Init7279();//EINT2 Rise rGPFCON = (rGPFCON&0xff0f)|0x0060;//GPF2:Interrupt;GPF3:Out 7279CS rEXTINT0= (rEXTINT0&0xffff00FF)|0x00000400; pISR_EINT2=(unsigned)Keyaddnumb; rINTMOD=0x0; //设置为IRQ模式rINTMSK&=~(BIT_EINT2); //打开keyboard中断//result(); */ //break; // } else{ allnumber[counter++]=key_number; } yes_key=0; } if(yes_key==0&&operation==0) { int j=0,p; if(edge<1&&counter>0) { //if(edge<1){ for(j=1;j<=counter;j++)write7279(DECODE1+5-j+1,allnumber[j-1]); }elseif(edge>1&&counter>0){send_byte(CMD_RESET);write7279(0x98,0xff);for(j=1;j<=counter-edge;j++)write7279(DECODE0+5+1-j,allnumber[edge+j-1]);}//else send_byte(CMD_RESET);}elseif(0<operation<5){edge=counter;operation=0;}}while(1);#include "2410addr.h"#include "7279.h"void Init7279(void){rGPEUP = rGPEUP|0x3800; //使用GPE11 GPE12 GPE13,去掉对应上拉功能rGPECON = (rGPECON&0xf03fffff)|0x05000000; // GPE11:In Data;GPE12:Out Data;GPE13:Out CLKrGPFUP = rGPFUP|0x0c;//使用GPF2 GPF3//rGPFCON = (rGPFCON&0xff0f)|0x0040; //GPF2:In Key;GPF3:Out 7279CS rGPFCON = (rGPFCON&0xff0f)|0x0060; //GPF2:In Key;GPF3:Out 7279CSrGPFDAT = rGPFDAT|0x08; //GPF3=1rGPFDAT = rGPFDAT&0xf7; //GPF3=0 选通7279rGPGUP = rGPGUP|0x0040; //使用GPG6,去掉对应上拉功能rGPGCON = (rGPGCON&0xffffcfff)|0x01000; //GPG6:Out 74H125 C1&(~C2) rGPGDAT = rGPGDAT&0xffbf; //GPG6=0}void write7279(unsigned char cmd ,unsigned char dta){send_byte(cmd);send_byte(dta);}unsigned char read7279(unsigned char command) {send_byte(command);return(receive_byte());}void send_byte(unsigned char out_byte) { unsigned char i;setcsLOW;long_delay();for(i=0;i<8;i++){if(out_byte&0x80){setdatHIGH;}else{setdatLOW;}setclkHIGH;short_delay();setclkLOW;short_delay();out_byte= out_byte*2;}setdatLOW;}unsigned char receive_byte(void){unsigned char i, in_byte;setdatZ;short_delay();for(i=0;i<8;i++){setclkHIGH;short_delay();in_byte=in_byte*2;if(dat){in_byte = in_byte|0x01; } setclkLOW;short_delay();}setdatL;return (in_byte);}void long_delay(void) {unsigned char i;for(i=0;i<0x30;i++);}void short_delay(void) {unsigned char i;for(i=0;i<0x0a;i++);}void delay10ms(unsigned char time) {unsigned char i;unsigned int j;for(i=0;i<time;i++){for(j=0;j<0x3300;j++){if(!key){//key_int();}}}}#ifndef __7279_H__#define __7279_H__void write7279(unsigned char,unsigned char); unsigned char read7279(unsigned char); void send_byte(unsigned char);unsigned char receive_byte(void);。
编写一个简单的计算器程序

编写一个简单的计算器程序计算器程序是一种非常实用的工具,它可以帮助我们进行数学计算,并简化复杂的运算过程。
本文将介绍如何编写一个简单的计算器程序,实现基本的加减乘除运算。
首先,我们需要确定计算器程序的功能和界面设计。
在本文中,我们将使用Python编程语言来编写计算器程序,并使用命令行界面(CLI)进行交互。
这意味着我们将在终端窗口中输入表达式,并显示结果。
接下来,我们需要考虑计算器程序的基本运算功能。
一个简单的计算器需要实现四个基本的运算:加法、减法、乘法和除法。
我们将使用函数来实现每个运算功能。
以下是一个示例代码:```pythondef add(x, y):return x + ydef subtract(x, y):return x - ydef multiply(x, y):return x * ydef divide(x, y):return x / y```在这个示例代码中,我们定义了四个函数,每个函数接受两个参数,并返回计算结果。
接下来,我们需要处理输入表达式并调用相应的运算函数。
我们将使用一个循环来持续接收用户输入,并在用户输入“exit”时退出程序。
以下是一个示例代码:```pythonwhile True:expression = input("请输入一个表达式:")if expression == "exit":break#解析表达式,提取运算符和操作数operator = Nonefor op in ["+", "-", "*", "/"]:if op in expression:operator = opbreakif not operator:print("表达式错误,请重新输入!") continueoperands = expression.split(operator) x = float(operands[0])y = float(operands[1])if operator == "+":result = add(x, y)elif operator == "-":result = subtract(x, y)elif operator == "*":result = multiply(x, y)elif operator == "/":result = divide(x, y)print("运算结果:", result)print("谢谢使用,再见!")```在这个示例代码中,我们使用了一个无限循环来持续接收用户输入。
简易计算器源代码--java
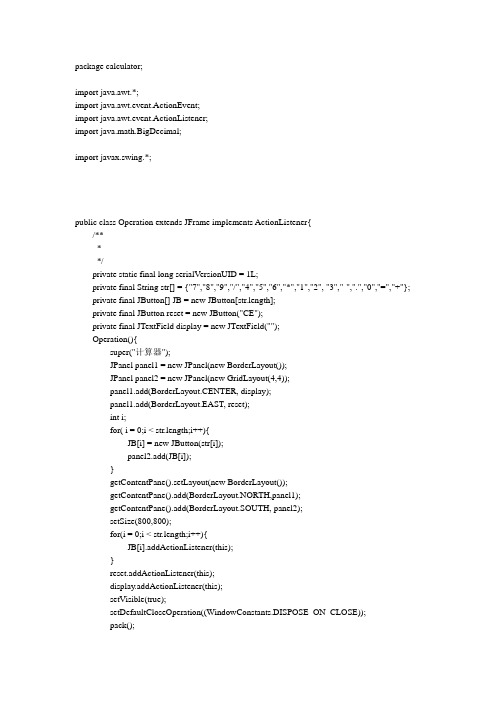
package calculator;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import java.math.BigDecimal;import javax.swing.*;public class Operation extends JFrame implements ActionListener{/****/private static final long serialVersionUID = 1L;private final String str[] = {"7","8","9","/","4","5","6","*","1","2", "3","-",".","0","=","+"};private final JButton[] JB = new JButton[str.length];private final JButton reset = new JButton("CE");private final JTextField display = new JTextField("");Operation(){super("计算器");JPanel panel1 = new JPanel(new BorderLayout());JPanel panel2 = new JPanel(new GridLayout(4,4));panel1.add(BorderLayout.CENTER, display);panel1.add(BorderLayout.EAST, reset);int i;for( i = 0;i < str.length;i++){JB[i] = new JButton(str[i]);panel2.add(JB[i]);}getContentPane().setLayout(new BorderLayout());getContentPane().add(BorderLayout.NORTH,panel1);getContentPane().add(BorderLayout.SOUTH, panel2);setSize(800,800);for(i = 0;i < str.length;i++){JB[i].addActionListener(this);}reset.addActionListener(this);display.addActionListener(this);setVisible(true);setDefaultCloseOperation((WindowConstants.DISPOSE_ON_CLOSE));pack();}public void actionPerformed(ActionEvent e) {// TODO 自动生成的方法存根Object target = e.getSource();String label = e.getActionCommand();if(target == reset){handlereset();}else if("0123456789.".indexOf(label)>=0){handleNumber(label);}elsehandleOperator(label);}private void handleNumber(String key) {// TODO 自动生成的方法存根if ((isFirstDigit)&&("0123456789".indexOf(key)>=0))display.setText(key);else if ((key.equals(".")))display.setText(display.getText() + ".");else if (!key.equals("."))display.setText(display.getText() + key);isFirstDigit = false;}boolean isFirstDigit = true;double number1 = 0.0;double number2 = 0.0;String operator = "=";BigDecimal df ;private void handlereset() {// TODO 自动生成的方法存根display.setText("");isFirstDigit = true;operator = "=";}public void handleOperator(String key) {if (operator.equals("+")){number1 = Double.valueOf(display.getText());number2 = add(number1,number2);df = new BigDecimal(number2);display.setText(String.valueOf( df.setScale(5, BigDecimal.ROUND_HALF_UP).doubleValue()));}else if (operator.equals("-")){number1 = Double.valueOf(display.getText());number2 = sub(number2,number1);df = new BigDecimal(number2);display.setText(String.valueOf( df.setScale(5, BigDecimal.ROUND_HALF_UP).doubleValue()));}else if (operator.equals("*")){number1 = Double.valueOf(display.getText());number2 = mul(number1,number2);df = new BigDecimal(number2);display.setText(String.valueOf( df.setScale(5, BigDecimal.ROUND_HALF_UP).doubleValue()));}else if (operator.equals("/")){number1 = Double.valueOf(display.getText());if(number1 == 0)display.setText("error");else{number2 = div(number2,number1);df = new BigDecimal(number2);display.setText(String.valueOf( df.setScale(5, BigDecimal.ROUND_HALF_UP).doubleValue()));}}else if (operator.equals("=")){number2 = Double.valueOf(display.getText());display.setText(String.valueOf(number2));}operator = key;isFirstDigit = true;}private double div(double key1, double key2) {// TODO 自动生成的方法存根double result = 0.0;result = key1 / key2;return result;}private double mul(double key1, double key2) {// TODO 自动生成的方法存根double result = 0.0;result = key1 * key2;return result;}private double sub(double key1, double key2) { // TODO 自动生成的方法存根double result = 0.0;result = key1 - key2;return result;}private double add(double key1, double key2) { // TODO 自动生成的方法存根double result = 0.0;result = number1 + number2;return result;}public static void main(String[] args) {new Operation();}}。
编写一个简易计算器的源代码
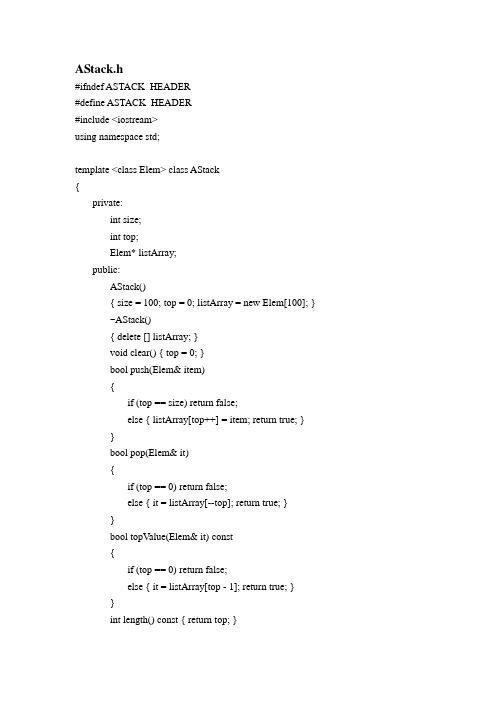
AStack.h#ifndef ASTACK_HEADER#define ASTACK_HEADER#include <iostream>using namespace std;template <class Elem> class AStack{private:int size;int top;Elem* listArray;public:AStack(){ size = 100; top = 0; listArray = new Elem[100]; }~AStack(){ delete [] listArray; }void clear() { top = 0; }bool push(Elem& item){if (top == size) return false;else { listArray[top++] = item; return true; } }bool pop(Elem& it){if (top == 0) return false;else { it = listArray[--top]; return true; }}bool topValue(Elem& it) const{if (top == 0) return false;else { it = listArray[top - 1]; return true; } }int length() const { return top; }};#endifFunction.cpp#include "function.h"#include "AStack.h"#include <math.h>#include <stdio.h>void calUserInfo(){cout<<"\t* 智能计算器V1.0*"<<endl;cout<<"\t*********************"<<endl;cout<<"\t* 1 * 2 * 3 * + * - *"<<endl;cout<<"\t* 4 * 5 * 6 * * * / *"<<endl;cout<<"\t* 7 * 8 * 9 * 0 * % *"<<endl;cout<<"\t* & * ^ * = * ( * ) *"<<endl;cout<<"\t*********************"<<endl; }int isp(char& ch){switch(ch){case '=':return 0;break;case '+':case '-':return 3;break;case '*':case '/':case '%':return 5;case '(':return 1;break;case ')':return 8;break;case '^':case '&':return 7;break;}}int osp(char& ch) {switch(ch) {case '=':return 0;break;case '+':case '-':return 2;break;case '*':case '/':case '%':return 4;break;case '(':return 8;break;case ')':break;case '^':case '&':return 6;break;}}double extract(double x,double y){return pow(x,1/y);}bool cal(char op, double x, double y, double& r) {int o = 0;switch(op){case '-':r = x - y;break;case '+':r = x + y;break;case '/':r = x / y;break;case '%':(int) o = (int)x % (int)y;r = (double)o;break;case '*':r = x * y;case '&':r = extract(x,y);break;case '^':r = pow(x,y);break;}return true;}bool isDigit(char ch){if (((int)ch >= 48) && ((int)ch <= 57))return true;else return false;}bool isPoint(char ch){if (ch == '.')return true;else return false;}bool isOperator(char ch){if ((ch == '=') || (ch == '-') || (ch == '+') || (ch == '(') || (ch == ')') || (ch == '*') || (ch == '&') ||(ch == '/') ||(ch == '%') ||(ch == '^'))return true;else return false;}double turnDigit(char ch){double value;value = (double)ch - 48;return value;}double newDigit(double prior_digit, double now_digit, bool isHavePoint, double point_num){double value;if(!isHavePoint){value = prior_digit*10 + now_digit;}else{value = prior_digit + now_digit/(pow(10,point_num));}return value;}Function.h#ifndef FUNCTION_HEADER#define FUNCTION_HEADER//友好的用户界面void calUserInfo();//用于记录处于栈内的优先级int isp(char& ch);//用于记录栈外的优先级int osp(char& ch);//相当于value = x op y;如果cal成功,然后将value的值返回r,用r来保存bool cal(char op, double x, double y, double& r);//判断是否为字符bool isDigit(char ch);//判断是否为小数点bool isPoint(char ch);//判断是否为操作运算符bool isOperator(char ch);//将数字的字符状态转化成double状态double turnDigit(char ch);//如果几个数字或加一个小数点连在一起,这说明他们是同一个数字的不同位数,此函数将不同连在一起的数字//组成新的数字double newDigit(double prior_digit, double now_digit, bool isHavePoint, double point_num);#endifMain.cpp#include "AStack.h"#include "function.h"#include <iostream>#include <stdlib.h>#include <string.h>using namespace std;int main(){char str[100] = "\0";AStack<double> opnd; //用于存放数据AStack<char> optr; //用于存放操作符char now_ch = '='; //用于记录当前字符char prior_ch = '\0'; //用于记录前一个字符double now_dig = 0; //用于记录当前一个数字double prior_dig = 0; //用于记录前一个数字double value = 0; //用于存放计算后的值bool point = false; //用于判断是否有小数点int point_num = 1; //用于记录数字后面的小数点位数char topValue; //用于记录opnd的top上的值char option = 'Y'; //用于判断是否要继续运算do{prior_dig = 0; //在opnd中提前放一个0now_ch = '=';opnd.push(prior_dig);optr.push(now_ch);system("cls");calUserInfo();cout << "请输入表达式(以等号结束):" << endl;cin >> str;bool exitPoint = false;/*对每个字符的处理*/for(int i = 0; i < strlen(str); i++){now_ch = str[i];/*判断是不是数字以及相关操作*/if (isDigit(now_ch)){now_dig = turnDigit(now_ch);if (isDigit(prior_ch)){opnd.pop(prior_dig);if(exitPoint){point = true;now_dig = newDigit(prior_dig, now_dig,point,point_num);point_num++;}elsenow_dig = newDigit(prior_dig, now_dig,point,point_num);}if(isPoint(prior_ch)){opnd.pop(prior_dig);now_dig = newDigit(prior_dig, now_dig,point,point_num);exitPoint = true;point_num++;}value = now_dig;opnd.push(now_dig);prior_ch = now_ch;}/*判断是不是小数点以及相关操作*/else if (isPoint(now_ch)){point = true;prior_ch = now_ch;}/*判断是不是操作符以及相关操作*/else if (isOperator(now_ch)){/*对用于数字操作的相关标记量进行清空,方便下一次数字操作*/point = false;point = 0;exitPoint = false;point_num = 1;/*看optr中是否有操作符存在,若不存在,则只放一个操作符进去*//* 但不进行任何操作*/if(optr.length() <= 1){optr.push(now_ch);prior_ch = now_ch;}/*optr已有操作符存在的话,开始进行优先级的比较*/else{optr.pop(topValue);/* 栈内优先级小于栈外优先级*/if(isp(topValue) < osp(now_ch)){optr.push(topValue);optr.push(now_ch);prior_ch = now_ch;}/*栈内优先级大于栈外优先级*/else if(isp(topValue) > osp(now_ch)){if(now_ch == ')' && topValue == '('){break;}do{double x = 1,y = 0;opnd.pop(x);opnd.pop(y);if( cal(topValue,y,x,value) ){opnd.push(value);}if(!optr.pop(topValue))break;}while(isp(topValue) > osp(now_ch));// if((topValue != '('))if (now_ch != ')'){optr.push(topValue);optr.push(now_ch);}prior_ch = now_ch;}/*其他情况报错*/elsebreak;}}/*其他情况报错*/elsecout << "输入的表达式错误,请检查!" << endl; }optr.pop(prior_ch);/*打印最后的计算值*/if(prior_ch == '='){cout << "最终得出的数据为: " << value << endl;}elsecout << "输入的表达式错误,请检查!" << endl;/*判断是否还要继续*/cout << "是否继续? ,继续'Y'/结束'N' :" << endl;cin >> option;/*清空数字栈和操作符栈*/opnd.clear();optr.clear();}while(option == 'Y');return 0;}。
自己写的计算器程序源代码
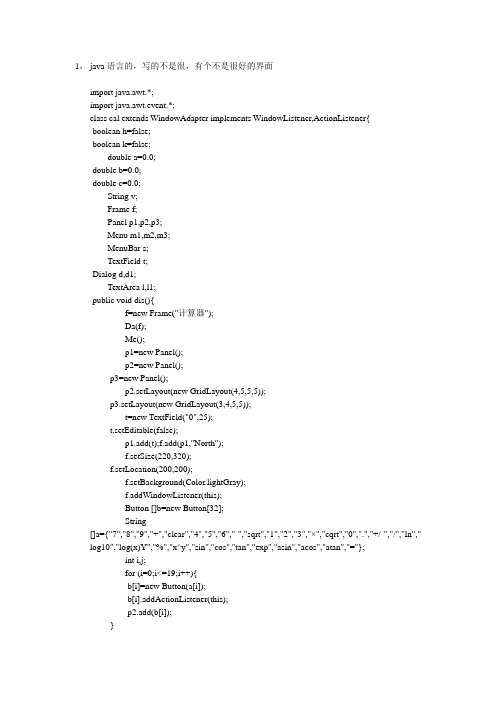
1,java语言的,写的不是很,有个不是很好的界面import java.awt.*;import java.awt.event.*;class cal extends WindowAdapter implements WindowListener,ActionListener{boolean h=false;boolean k=false;double a=0.0;double b=0.0;double c=0.0;String v;Frame f;Panel p1,p2,p3;Menu m1,m2,m3;MenuBar s;TextField t;Dialog d,d1;TextArea l,l1;public void dis(){f=new Frame("计算器");Da(f);Me();p1=new Panel();p2=new Panel();p3=new Panel();p2.setLayout(new GridLayout(4,5,5,5));p3.setLayout(new GridLayout(3,4,5,5));t=new TextField("0",25);t.setEditable(false);p1.add(t);f.add(p1,"North");f.setSize(220,320);f.setLocation(200,200);f.setBackground(Color.lightGray);f.addWindowListener(this);Button []b=new Button[32];String[]a={"7","8","9","+","clear","4","5","6","-","sqrt","1","2","3","×","cqrt","0",".","+/-","/","ln","log10","log(x)Y","%","x^y","sin","cos","tan","exp","asin","acos","atan","="};int i,j;for (i=0;i<=19;i++){b[i]=new Button(a[i]);b[i].addActionListener(this);p2.add(b[i]);}for (j=20;j<=31;j++){b[j]=new Button(a[j]);b[j].addActionListener(this);p3.add(b[j]);}f.add(p2,"Center");f.add(p3,"South");f.setV isible(true);}public void Me(){s=new MenuBar();f.setMenuBar(s);m1=new Menu("编辑(E)");m2=new Menu("查看(V)");m3=new Menu("帮助(H)");s.add(m1);s.add(m2);s.add(m3);m1.add(new MenuItem("Open"));m1.add(new MenuItem("Copy"));m1.add(new MenuItem("pastye"));m1.add(new MenuItem("Save",new MenuShortcut(KeyEvent.VK_S)));m1.add(new MenuItem("Exit"));m2.add(new MenuItem("Standard"));m2.add(new MenuItem("Science"));m1.addActionListener(this);m2.addActionListener(this);m3.add(new MenuItem("About"));m3.add(new MenuItem("Instructions"));m3.addActionListener(this);}public void windowClosing(WindowEvent e){if(e.getSource()==d)d.setV isible(false);else if(e.getSource()==d1)d1.setV isible(false);elseSystem.exit(0);}public void Da(Frame f){d=new Dialog(f,"Dialog",true);d.setSize(36,120);d.setLocation(200,200);d1=new Dialog(f,"使用说明",true);d1.setSize(200,202);d1.setLocation(200,200);l=new TextArea("",4,15,TextArea.SCROLLBARS_VERTICAL_ONLY);l1=new TextArea("",4,15,TextArea.SCROLLBARS_NONE);d.add(l);d1.add(l1);d.addWindowListener(this);d1.addWindowListener(this);}public void actionPerformed(ActionEvent e){float x;String q=e.getActionCommand();if(q=="Exit")System.exit(0);if(q=="About"){l.setText(" 该计算器实现加减乘除开方等基本运算,而且能够计算三角函数以及反三角函数的值,能够进行对数、乘方运算。
简单计算器设计源代码

Button btn0,btn1,btn2,btn3,btn4,btn5;
Button btn6,btn7,btn8,btn9;
EditText et=null;
Button btnC,btnadd,btnsub,btnmul,btndiv,btnequal,btnpoint;
<Button
android:id="@+id/btn_seven_main"
android:layout_width="0dp"
android:layout_height="60dp"
android:layout_weight="1"
android:gravity="center"
android:textSize="40sp"
android:text="/"/>
</LinearLayout>
</LinearLayout>
源代码:
package com.example.num;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
android:text="-"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layoutid:orientation="horizontal" >
计算器c语言代码

计算器c语言代码计算器C语言代码计算器是一种常见的工具,用于进行数值计算和数学运算。
计算器通常具有基本的四则运算功能,可以执行加法、减法、乘法和除法运算,同时还可以进行其他高级计算,比如开方、求幂、取余等。
在本文中,我们将介绍如何使用C语言编写一个简单的计算器。
我们需要定义计算器的基本功能。
我们可以使用函数来实现不同的计算操作。
下面是一个示例代码,实现了加法、减法、乘法和除法四种功能:```c#include <stdio.h>// 加法函数double add(double a, double b) {return a + b;}// 减法函数double subtract(double a, double b) {return a - b;}// 乘法函数double multiply(double a, double b) { return a * b;}// 除法函数double divide(double a, double b) { if (b == 0) {printf("除数不能为零!\n"); return 0;}return a / b;}int main() {double num1, num2;char operator;printf("请输入两个操作数:");scanf("%lf %lf", &num1, &num2); printf("请输入操作符:");scanf(" %c", &operator);switch (operator) {case '+':printf("结果:%lf\n", add(num1, num2));break;case '-':printf("结果:%lf\n", subtract(num1, num2));break;case '*':printf("结果:%lf\n", multiply(num1, num2));break;case '/':printf("结果:%lf\n", divide(num1, num2));break;default:printf("无效的操作符!\n");break;}return 0;}```在上面的代码中,我们使用了四个函数来实现不同的计算操作。
简单的计算器源代码
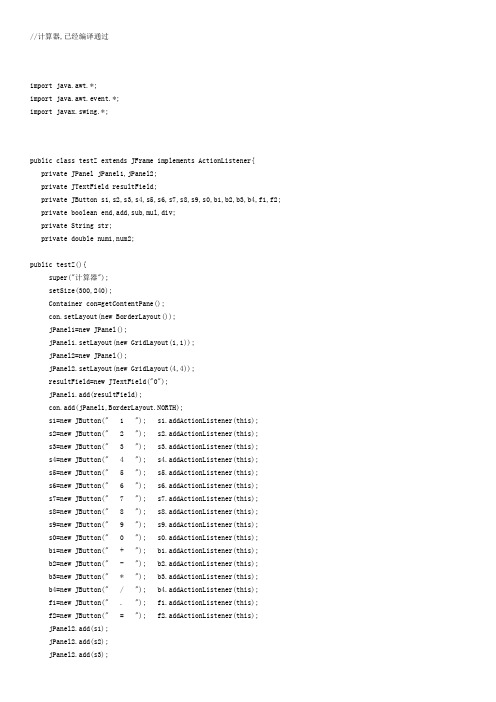
//计算器,已经编译通过import java.awt.*;import java.awt.event.*;import javax.swing.*;public class testZ extends JFrame implements ActionListener{private JPanel jPanel1,jPanel2;private JTextField resultField;private JButton s1,s2,s3,s4,s5,s6,s7,s8,s9,s0,b1,b2,b3,b4,f1,f2; private boolean end,add,sub,mul,div;private String str;private double num1,num2;public testZ(){super("计算器");setSize(300,240);Container con=getContentPane();con.setLayout(new BorderLayout());jPanel1=new JPanel();jPanel1.setLayout(new GridLayout(1,1));jPanel2=new JPanel();jPanel2.setLayout(new GridLayout(4,4));resultField=new JTextField("0");jPanel1.add(resultField);con.add(jPanel1,BorderLayout.NORTH);s1=new JButton(" 1 "); s1.addActionListener(this);s2=new JButton(" 2 "); s2.addActionListener(this);s3=new JButton(" 3 "); s3.addActionListener(this);s4=new JButton(" 4 "); s4.addActionListener(this);s5=new JButton(" 5 "); s5.addActionListener(this);s6=new JButton(" 6 "); s6.addActionListener(this);s7=new JButton(" 7 "); s7.addActionListener(this);s8=new JButton(" 8 "); s8.addActionListener(this);s9=new JButton(" 9 "); s9.addActionListener(this);s0=new JButton(" 0 "); s0.addActionListener(this);b1=new JButton(" + "); b1.addActionListener(this);b2=new JButton(" - "); b2.addActionListener(this);b3=new JButton(" * "); b3.addActionListener(this);b4=new JButton(" / "); b4.addActionListener(this);f1=new JButton(" . "); f1.addActionListener(this);f2=new JButton(" = "); f2.addActionListener(this);jPanel2.add(s1);jPanel2.add(s2);jPanel2.add(s3);jPanel2.add(b1);jPanel2.add(s4);jPanel2.add(s5);jPanel2.add(s6);jPanel2.add(b2);jPanel2.add(s7);jPanel2.add(s8);jPanel2.add(s9);jPanel2.add(b3);jPanel2.add(s0);jPanel2.add(f1);jPanel2.add(f2);jPanel2.add(b4);con.add(jPanel2,BorderLayout.CENTER);}public void num(int i){String s = null;s=String.valueOf(i);if(end){//如果数字输入结束,则将文本框置零,重新输入resultField.setText("0");end=false;}if((resultField.getText()).equals("0")){//如果文本框的内容为零,则覆盖文本框的内容resultField.setText(s);}else{//如果文本框的内容不为零,则在内容后面添加数字str = resultField.getText() + s;resultField.setText(str);}}public void actionPerformed(ActionEvent e){ //数字事件 if(e.getSource()==s1)num(1);else if(e.getSource()==s2)num(2);else if(e.getSource()==s3)num(3);else if(e.getSource()==s4)num(4);else if(e.getSource()==s5)num(5);else if(e.getSource()==s6)num(6);else if(e.getSource()==s7)num(7);else if(e.getSource()==s8)num(8);else if(e.getSource()==s9)num(9);else if(e.getSource()==s0)num(0);//符号事件else if(e.getSource()==b1)sign(1);else if(e.getSource()==b2)sign(2);else if(e.getSource()==b3)sign(3);else if(e.getSource()==b4)sign(4);//等号else if(e.getSource()==f1){str=resultField.getText();if(str.indexOf(".")<=1){str+=".";resultField.setText(str);}}else if(e.getSource()==f2){num2=Double.parseDouble(resultField.getText());if(add){num1=num1 + num2;}else if(sub){num1=num1 - num2;}else if(mul){num1=num1 * num2;}else if(div){num1=num1 / num2;}resultField.setText(String.valueOf(num1));end=true;}}public void sign(int s){if(s==1){add=true;sub=false;mul=false;div=false;}else if(s==2){add=false;sub=true;mul=false;div=false;}else if(s==3){add=false;sub=false;mul=true;div=false;}else if(s==4){add=false;sub=false;mul=false;div=true;}num1=Double.parseDouble(resultField.getText()); end=true;}public static void main(String[] args){testZ th1=new testZ();th1.show();}}。
C编写简易计算器附源代码超详细
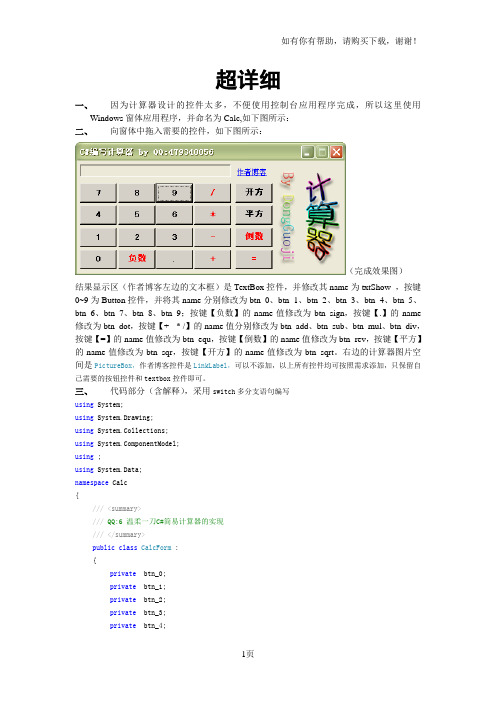
超详细一、因为计算器设计的控件太多,不便使用控制台应用程序完成,所以这里使用Windows窗体应用程序,并命名为Calc,如下图所示:二、向窗体中拖入需要的控件,如下图所示:(完成效果图)结果显示区(作者博客左边的文本框)是TextBox控件,并修改其name为txtShow ,按键0~9为Button控件,并将其name分别修改为btn_0、btn_1、btn_2、btn_3、btn_4、btn_5、btn_6、btn_7、btn_8、btn_9;按键【负数】的name值修改为btn_sign,按键【.】的name 修改为btn_dot,按键【+ - * /】的name值分别修改为btn_add、btn_sub、btn_mul、btn_div,按键【=】的name值修改为btn_equ,按键【倒数】的name值修改为btn_rev,按键【平方】的name值修改为btn_sqr,按键【开方】的name值修改为btn_sqrt。
右边的计算器图片空间是PictureBox,作者博客控件是LinkLabel,可以不添加,以上所有控件均可按照需求添加,只保留自己需要的按钮控件和textbox控件即可。
三、代码部分(含解释),采用switch多分支语句编写using System;using System.Drawing;using System.Collections;using ponentModel;using ;using System.Data;namespace Calc{///<summary>/// QQ:6 温柔一刀C#简易计算器的实现///</summary>public class CalcForm :{private btn_0;private btn_1;private btn_2;private btn_3;private btn_4;private btn_5;private btn_6;private btn_7;private btn_8;private btn_9;private btn_add;private btn_sub;private btn_mul;private btn_div;private btn_sqrt;private btn_sign;private btn_equ;private btn_dot;private btn_rev;private txtShow;private btn_sqr;private PictureBox pictureBox1;private LinkLabel linkLabel1;///<summary>///必需的设计器变量。
C语言编写的计算器源代码

C语言编写的计算器源代码```c#include<stdio.h>#include<stdlib.h>#include<string.h>#include<ctype.h>#define MAX_EXPRESSION_SIZE 100//栈结构定义typedef structint top;double data[MAX_EXPRESSION_SIZE];} Stack;//初始化栈void initStack(Stack *s)s->top = -1;//入栈void push(Stack *s, double value)if (s->top == MAX_EXPRESSION_SIZE - 1)printf("Stack is full. Cannot push element.\n");} elses->data[++(s->top)] = value;}//出栈double pop(Stack *s)if (s->top == -1)printf("Stack is empty. Cannot pop element.\n"); return -1;} elsereturn s->data[(s->top)--];}//获取栈顶元素double peek(Stack *s)if (s->top == -1)return -1;} elsereturn s->data[s->top];}//判断运算符的优先级int getPriority(char operator)switch (operator)case '+':case '-':return 1;case '*':case '/':return 2;case '^':return 3;default:return -1;}//执行四则运算double performOperation(double operand1, double operand2, char operator)switch (operator)case '+':return operand1 + operand2;case '-':return operand1 - operand2;case '*':return operand1 * operand2;case '/':if (operand2 != 0)return operand1 / operand2;} elseprintf("Error: Division by zero.\n");exit(1);}case '^':return pow(operand1, operand2);default:return 0;}//计算表达式结果double evaluateExpression(char *expression) Stack operandStack;Stack operatorStack;initStack(&operandStack);initStack(&operatorStack);int length = strlen(expression);for (int i = 0; i < length; i++)//忽略空格if (expression[i] == ' ')continue;}//数字直接入栈if (isdigit(expression[i]))double num = 0;while (i < length && (isdigit(expression[i]) , expression[i] == '.'))num = num * 10 + (expression[i] - '0');i++;}i--;push(&operandStack, num);}//左括号入栈else if (expression[i] == '(')push(&operatorStack, expression[i]);}//右括号出栈并执行运算,直到遇到左括号else if (expression[i] == ')')while (peek(&operatorStack) != '(')double operand2 = pop(&operandStack);double operand1 = pop(&operandStack);char operator = pop(&operatorStack);double result = performOperation(operand1, operand2, operator);push(&operandStack, result);}pop(&operatorStack);}//运算符出栈并执行运算,直到栈空或者遇到优先级较低的运算符elsewhile (peek(&operatorStack) != -1 &&getPriority(expression[i]) <= getPriority(peek(&operatorStack))) double operand2 = pop(&operandStack);double operand1 = pop(&operandStack);char operator = pop(&operatorStack);double result = performOperation(operand1, operand2, operator);push(&operandStack, result);}push(&operatorStack, expression[i]);}}//处理剩下的运算符while (peek(&operatorStack) != -1)double operand2 = pop(&operandStack);double operand1 = pop(&operandStack);char operator = pop(&operatorStack);double result = performOperation(operand1, operand2, operator);push(&operandStack, result);}return pop(&operandStack); // 返回最终结果int maichar expression[MAX_EXPRESSION_SIZE];printf("Enter an arithmetic expression: ");fgets(expression, MAX_EXPRESSION_SIZE, stdin);double result = evaluateExpression(expression);printf("Result = %.2f\n", result);return 0;```这个计算器可以实现基本的四则运算,支持括号和浮点数。
科学计算器源代码

科学计算器源代码以下是一个简单的科学计算器的源代码,包含了基本的数学运算、三角函数、指数和对数运算、阶乘和组合运算等功能。
```#include <iostream>#include <cmath>using namespace std;//计算器类class ScientificCalculatorpublic://基本运算double add(double a, double b)return a + b;}double subtract(double a, double b)return a - b;}double multiply(double a, double b)return a * b;}double divide(double a, double b)if (b != 0)return a / b;} elsecout << "Error: Division by zero!" << endl; return 0;}}//三角函数运算double sine(double angle)return sin(angle);}double cosine(double angle)return cos(angle);}double tangent(double angle)return tan(angle);}//指数和对数运算double exponent(double x) return exp(x);}double logarithm(double x) return log10(x);}//阶乘运算double factorial(int n)if (n == 0)return 1;} elseint result = 1;for (int i = 1; i <= n; i++) result *= i;}return result;}}//组合运算if (n >= r)return factorial(n) / (factorial(r) * factorial(n - r));} elsecout << "Error: Invalid input!" << endl;return 0;}}};//主函数int maiScientificCalculator calculator;//进行一些简单的计算cout << "Addition: " << calculator.add(5, 3) << endl;cout << "Subtraction: " << calculator.subtract(5, 3) << endl;cout << "Multiplication: " << calculator.multiply(5, 3) << endl;cout << "Division: " << calculator.divide(5, 3) << endl;//三角函数运算cout << "Sine: " << calculator.sine(0.5) << endl;cout << "Cosine: " << calculator.cosine(0.5) << endl;cout << "Tangent: " << calculator.tangent(0.5) << endl;//指数和对数运算cout << "Exponent: " << calculator.exponent(2) << endl;cout << "Logarithm: " << calculator.logarithm(100) << endl;//阶乘和组合运算cout << "Factorial: " << calculator.factorial(5) << endl;return 0;```这个科学计算器的源代码使用了面向对象的编程思想,将不同的数学运算封装成类的成员函数。
计算器源代码
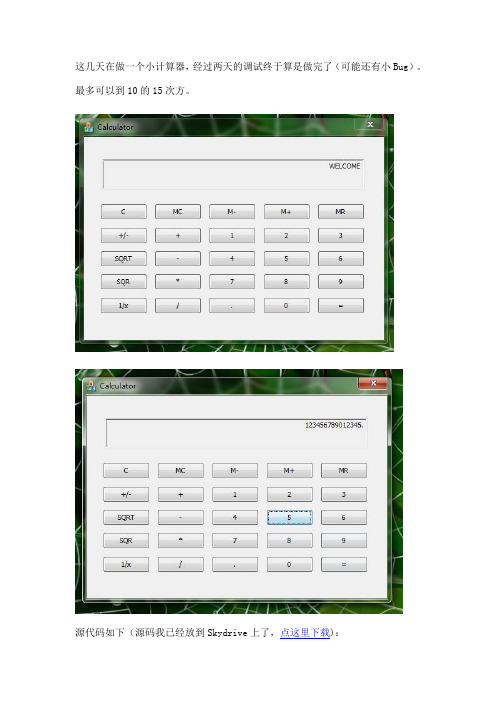
这几天在做一个小计算器,经过两天的调试终于算是做完了(可能还有小Bug)。
最多可以到10的15次方。
源代码如下(源码我已经放到Skydrive上了,点这里下载):// CalculatorDlg.cpp : implementation file//#include "stdafx.h"#include "Calculator.h"#include "CalculatorDlg.h"#include "math.h"#ifdef _DEBUG#define new DEBUG_NEW#endif// CAboutDlg dialog used for App AboutDisplay *Pdisplay;class CAboutDlg : public CDialog{public:CAboutDlg();// Dialog Dataenum { IDD = IDD_ABOUTBOX };protected:virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support// Implementationprotected:DECLARE_MESSAGE_MAP()};CAboutDlg::CAboutDlg() : CDialog(CAboutDlg::IDD){}void CAboutDlg::DoDataExchange(CDataExchange* pDX){CDialog::DoDataExchange(pDX);}BEGIN_MESSAGE_MAP(CAboutDlg, CDialog)END_MESSAGE_MAP()// CCalculatorDlg dialogCCalculatorDlg::CCalculatorDlg(CWnd* pParent /*=NULL*/): CDialog(CCalculatorDlg::IDD, pParent), Cal_display(_T("WELCOME")){m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);Pdisplay=new Display(this);}void CCalculatorDlg::DoDataExchange(CDataExchange* pDX){CDialog::DoDataExchange(pDX);DDX_Text(pDX, IDC_EDIT1, Cal_display);}BEGIN_MESSAGE_MAP(CCalculatorDlg, CDialog)ON_WM_SYSCOMMAND()ON_WM_PAINT()ON_WM_QUERYDRAGICON()//}}AFX_MSG_MAP// ON_WM_KEYDOWN()ON_BN_CLICKED(IDOK, &CCalculatorDlg::OnBnClickedOk)ON_BN_CLICKED(IDCANCEL, &CCalculatorDlg::OnBnClickedCancel)ON_BN_CLICKED(IDCANCEL2, &CCalculatorDlg::OnBnClickedCancel2) ON_BN_CLICKED(IDOK4, &CCalculatorDlg::OnBnClickedOk4)ON_BN_CLICKED(IDOK6, &CCalculatorDlg::OnBnClickedOk6)ON_BN_CLICKED(IDOK2, &CCalculatorDlg::OnBnClickedOk2)ON_BN_CLICKED(IDCANCEL3, &CCalculatorDlg::OnBnClickedCancel3) ON_BN_CLICKED(IDOK3, &CCalculatorDlg::OnBnClickedOk3)ON_BN_CLICKED(IDCANCEL4, &CCalculatorDlg::OnBnClickedCancel4) ON_BN_CLICKED(IDCANCEL6, &CCalculatorDlg::OnBnClickedCancel6) ON_BN_CLICKED(IDCANCEL5, &CCalculatorDlg::OnBnClickedCancel5) ON_BN_CLICKED(IDCANCEL8, &CCalculatorDlg::OnBnClickedCancel8) ON_BN_CLICKED(IDCANCEL9, &CCalculatorDlg::OnBnClickedCancel9) ON_BN_CLICKED(IDCANCEL7, &CCalculatorDlg::OnBnClickedCancel7) ON_BN_CLICKED(IDOK5, &CCalculatorDlg::OnBnClickedOk5)ON_BN_CLICKED(IDOK7, &CCalculatorDlg::OnBnClickedOk7)ON_BN_CLICKED(IDCANCEL10, &CCalculatorDlg::OnBnClickedCancel10) ON_BN_CLICKED(IDOK8, &CCalculatorDlg::OnBnClickedOk8)ON_BN_CLICKED(IDCANCEL12, &CCalculatorDlg::OnBnClickedCancel12)ON_BN_CLICKED(IDCANCEL11, &CCalculatorDlg::OnBnClickedCancel11)ON_BN_CLICKED(IDOK9, &CCalculatorDlg::OnBnClickedOk9)ON_BN_CLICKED(IDCANCEL13, &CCalculatorDlg::OnBnClickedCancel13)ON_BN_CLICKED(IDOK10, &CCalculatorDlg::OnBnClickedOk10)ON_BN_CLICKED(IDCANCEL15, &CCalculatorDlg::OnBnClickedCancel15)ON_BN_CLICKED(IDCANCEL14, &CCalculatorDlg::OnBnClickedCancel14)ON_WM_KEYUP()END_MESSAGE_MAP()// CCalculatorDlg message handlersBOOL CCalculatorDlg::OnInitDialog(){CDialog::OnInitDialog();// Add "About..." menu item to system menu.// IDM_ABOUTBOX must be in the system command range.ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);ASSERT(IDM_ABOUTBOX < 0xF000);CMenu* pSysMenu = GetSystemMenu(FALSE);if (pSysMenu != NULL){CString strAboutMenu;strAboutMenu.LoadString(IDS_ABOUTBOX);if (!strAboutMenu.IsEmpty()){pSysMenu->AppendMenu(MF_SEPARATOR);pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);}}// Set the icon for this dialog. The framework does this automatically // when the application's main window is not a dialogSetIcon(m_hIcon, TRUE); // Set big iconSetIcon(m_hIcon, FALSE); // Set small icon// TODO: Add extra initialization herereturn TRUE; // return TRUE unless you set the focus to a control}//--------------------------------------------------------------------------------------------------//void CCalculatorDlg::OnKeyDown(UINT nChar,UINT nRepCnt,UINT nFlags) //{//int a=1;//CDialog::OnKeyDown(nChar, nRepCnt, nFlags);//}//void CCalculatorDlg::OnKeyUp(UINT nChar, UINT nRepCnt, UINT nFlags) //{// int a=1;//// CDialog::OnKeyUp(nChar, nRepCnt, nFlags);//}void CCalculatorDlg::OnSysCommand(UINT nID, LPARAM lParam){if ((nID & 0xFFF0) == IDM_ABOUTBOX){CAboutDlg dlgAbout;dlgAbout.DoModal();}else if(nID==SC_CLOSE){CCalculatorDlg::OnOK();}else{CDialog::OnSysCommand(nID, lParam);}}// If you add a minimize button to your dialog, you will need the code below// to draw the icon. For MFC applications using the document/view model, // this is automatically done for you by the framework.void CCalculatorDlg::OnPaint(){if (IsIconic()){CPaintDC dc(this); // device context for paintingSendMessage(WM_ICONERASEBKGND, reinterpret_cast<WPARAM>(dc.GetSafeHdc()), 0);// Center icon in client rectangleint cxIcon = GetSystemMetrics(SM_CXICON);int cyIcon = GetSystemMetrics(SM_CYICON);CRect rect;GetClientRect(&rect);int x = (rect.Width() - cxIcon + 1) / 2;int y = (rect.Height() - cyIcon + 1) / 2;// Draw the icondc.DrawIcon(x, y, m_hIcon);}else{CDialog::OnPaint();}}// The system calls this function to obtain the cursor to display while the user drags// the minimized window.HCURSOR CCalculatorDlg::OnQueryDragIcon(){return static_cast<HCURSOR>(m_hIcon);}//------------------------------OnButtonnClicked 部分-------------------------------------------------------------------------void CCalculatorDlg::OnBnClickedOk(){Caldata.CLEAR();UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel(){Caldata.MC();UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel2() {Caldata.MR();UpdateDisplay();}void CCalculatorDlg::OnBnClickedOk4() {Caldata.KEYIN(1);UpdateDisplay();}void CCalculatorDlg::OnBnClickedOk6() {Caldata.KEYIN(4);UpdateDisplay();}void CCalculatorDlg::OnBnClickedOk2() {Caldata.MM();UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel3() {Caldata.MA();UpdateDisplay();}void CCalculatorDlg::OnBnClickedOk3() {Caldata.PN();UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel4() {Caldata.ADD();UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel6() {Caldata.KEYIN(2);UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel5() {Caldata.KEYIN(3);UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel8() {Caldata.KEYIN(6);UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel9() {Caldata.KEYIN(5);UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel7() {Caldata.MINUS();UpdateDisplay();}void CCalculatorDlg::OnBnClickedOk5(){Caldata.Mode_Error=Caldata.SQRT(); UpdateDisplay();}void CCalculatorDlg::OnBnClickedOk7(){Caldata.SQR();UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel10() {Caldata.BY();UpdateDisplay();}void CCalculatorDlg::OnBnClickedOk8(){Caldata.KEYIN(7);UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel12() {Caldata.KEYIN(8);UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel11() {Caldata.KEYIN(9);UpdateDisplay();}void CCalculatorDlg::OnBnClickedOk9(){Caldata.Mode_Error=Caldata.REC(); UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel13() {Caldata.DIV();UpdateDisplay();}void CCalculatorDlg::OnBnClickedOk10() {Caldata.Dot();UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel15() {Caldata.KEYIN(0);UpdateDisplay();}void CCalculatorDlg::OnBnClickedCancel14() {Caldata.Mode_Error=Caldata.EQUAL();UpdateDisplay();}//------------------------------------------Display部分-----------------------------------------------------------------void CCalculatorDlg::UpdateDisplay(){if(!Caldata.Mode_Error){if(!(Caldata.Mode_Dot)){Cal_display.Format(_T("%lf"),Caldata.Data_Display);for(int i=Cal_display.GetLength()-1;i>1;i--) //去掉尾部多余的0,这个方法可共用,可以写成STATIC{if(Cal_display.GetAt(i)=='.')break;if(Cal_display.GetAt(i)=='0')Cal_display.Delete(i);else break;}}else {if(Caldata.Data_Display!=0){int j=0;Cal_display.Format(_T("%lf"),Caldata.Data_Display);for(int i=Cal_display.GetLength()-1;i>1;i--){if(Cal_display.GetAt(i)=='.')break;if(Cal_display.GetAt(i)=='0')Cal_display.Delete(i);else break;}for(int i=Cal_display.GetLength()-1;i>1;i--){ if(Cal_display.GetAt(i)=='.') break;j++;}if(j<=Caldata.Digi_Dot)for(int i=0;i<Caldata.Digi_Dot-j;i++) //小数输入,尾部补0Cal_display.Append(_T("0")); }else{Cal_display.Format(_T("0."));for(int i=0;i<Caldata.Digi_Dot;i++){Cal_display.Append(_T("0"));}}}}else Cal_display.Format(_T("ERROR"));UpdateData(false);}void CCalculatorDlg::ResetDisplay(){}//--------------------------------CALDATA 部分-----------------------------------------------------------Cal_Data::Cal_Data(){Data_Display=0;Data_Remember=0;Data_Last=0;Mode_Remenber=false;Mode_Input=false;Mode_Dot=false;Mode_Error=false;Digi_Dot=0;calaction=Cal_Action::Action_Null;}void Cal_Data::CLEAR(){Data_Display=0;Data_Last=0;Mode_Input=false;Mode_Error=false;Mode_Dot=false;calaction=Cal_Action::Action_Null;}void Cal_Data::MA(){Data_Remember=Data_Remember+Data_Display; }void Cal_Data::MM(){Data_Remember=Data_Remember-Data_Display; }void Cal_Data::MR(){Data_Display=Data_Remember;Mode_Input=false;}void Cal_Data::MC(){Data_Remember=0;Mode_Remenber=false;}void Cal_Data::ADD(){Data_Last=Data_Display;Mode_Dot=Mode_Input=false; calaction=Cal_Action::Action_Add;}void Cal_Data::MINUS(){Data_Last=Data_Display;Mode_Dot=Mode_Input=false;calaction=Cal_Action::Action_Minus;}void Cal_Data::BY(){Data_Last=Data_Display;Mode_Dot=Mode_Input=false;calaction=Cal_Action::Action_By;}void Cal_Data::DIV(){Data_Last=Data_Display;Mode_Dot=Mode_Input=false;calaction=Cal_Action::Action_Divide;}bool Cal_Data::EQUAL(){double TMP;Digi_Dot=0;Mode_Dot=false;switch(calaction){case Cal_Action::Action_Add : Data_Display+=Data_Last; Mode_Dot=Mode_Input=false;break;case Cal_Action::Action_Minus: Data_Display=Data_Last-Data_Display;Mode_Dot=Mode_Input=false;break;case Cal_Action::Action_By: Data_Display*=Data_Last;Mode_Dot=Mode_Input=false;break;case Cal_Action::Action_Divide: if(Data_Display==0)return true;else Data_Display=Data_Last/Data_Display;Mode_Dot=Mode_Input=false;break;case Cal_Action::Action_Null: Mode_Dot=Mode_Input=false;break;}calaction=Cal_Action::Action_Null;return false;}void Cal_Data::SQR(){Data_Display*=Data_Display;Mode_Dot=Mode_Input=false;}bool Cal_Data::SQRT(){Mode_Dot=Mode_Input=false;if(Data_Display<0)return true;Data_Display=sqrt(Data_Display);return false;}bool Cal_Data::REC(){Mode_Dot=Mode_Input=false;if(Data_Display==0)return true;else Data_Display=1/Data_Display;return false;}void Cal_Data::PN(){if(Data_Display!=0) Data_Display=-Data_Display;}void Cal_Data::Dot(){if(!Mode_Dot) { Mode_Dot=true;Digi_Dot=0;}}void Cal_Data::KEYIN(int value){ if(Data_Display>=100000000000000) //double型的数大于100000000000000后精度不高,所以限定为15位return;if(!Mode_Input){ Mode_Input=true;Data_Display=0;}if(!Mode_Dot){Data_Display*=10.0;Data_Display=Data_Display>=0? Data_Display+(double)value: Data_Display-(double)value;}else{if(Digi_Dot>=6) //小数点后面最多输入到第6位,否者会出错(double的精度不高)return;Digi_Dot++;double TMP=value;for(int i=0;i<Digi_Dot;i++){TMP=TMP/10.0;}Data_Display=Data_Display>=0? Data_Display+TMP:Data_Display-TMP;。
C语言简单计算器程序源代码

C语言简单计算器程序源代码以下是一个简单的C语言计算器程序的源代码:```c#include <stdio.h>int maichar operator;double num1, num2;printf("请输入运算符:");scanf("%c", &operator);printf("请输入两个数字:");scanf("%lf %lf", &num1, &num2);switch (operator)case '+':printf("%.2lf + %.2lf = %.2lf", num1, num2, num1 + num2); break;case '-':printf("%.2lf - %.2lf = %.2lf", num1, num2, num1 - num2); break;case '*':printf("%.2lf * %.2lf = %.2lf", num1, num2, num1 * num2);break;case '/':if (num2 != 0)printf("%.2lf / %.2lf = %.2lf", num1, num2, num1 / num2);elseprintf("除数不能为0");break;default:printf("无效的运算符");}return 0;```这个程序首先提示用户输入一个运算符,然后再提示输入两个数字。
接下来,程序会根据输入的运算符执行相应的计算,并打印结果。
在计算除法时,程序会检查除数是否为0,如果为0,则打印错误信息。
请注意,这只是一个非常简单的计算器程序,没有考虑很多错误处理情况。
c语言实现虚拟计算器代码
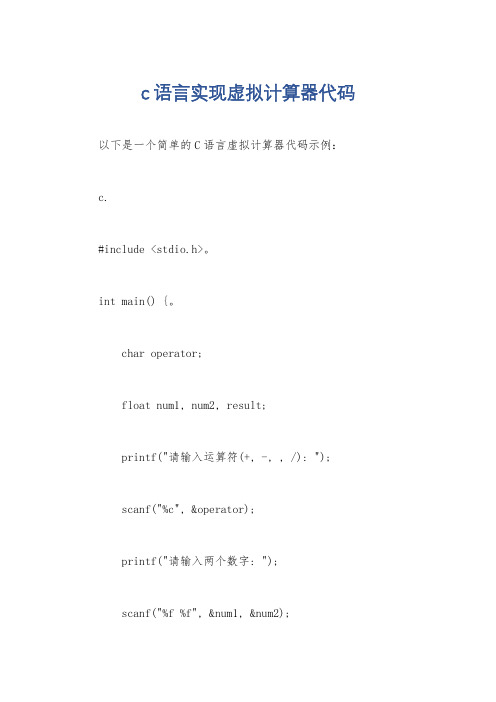
c语言实现虚拟计算器代码以下是一个简单的C语言虚拟计算器代码示例: c.#include <stdio.h>。
int main() {。
char operator;float num1, num2, result;printf("请输入运算符(+, -, , /): ");scanf("%c", &operator);printf("请输入两个数字: ");scanf("%f %f", &num1, &num2);switch(operator) {。
case '+':result = num1 + num2; break;case '-':result = num1 num2; break;case '':result = num1 num2; break;case '/':if(num2 != 0) {。
result = num1 / num2;} else {。
printf("错误,除数不能为0\n"); return 1; // 退出程序。
}。
break;default:printf("错误,无效的运算符\n");return 1; // 退出程序。
}。
printf("结果: %f %c %f = %f\n", num1, operator,num2, result);return 0;}。
这段代码首先要求用户输入运算符和两个数字,然后根据用户输入的运算符进行相应的计算,并输出结果。
在这个示例中,我们使用了switch语句来根据不同的运算符执行不同的计算操作。
另外,我们还对除数为0的情况进行了处理,以避免出现除以0的错误。
当然,这只是一个简单的示例代码,实际的虚拟计算器可能会更复杂,包括更多的功能和更完善的用户界面。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
AStack.h#ifndef ASTACK_HEADER#define ASTACK_HEADER#include <iostream>using namespace std;template <class Elem> class AStack{private:int size;int top;Elem* listArray;public:AStack(){ size = 100; top = 0; listArray = new Elem[100]; }~AStack(){ delete [] listArray; }void clear() { top = 0; }bool push(Elem& item){if (top == size) return false;else { listArray[top++] = item; return true; } }bool pop(Elem& it){if (top == 0) return false;else { it = listArray[--top]; return true; }}bool topValue(Elem& it) const{if (top == 0) return false;else { it = listArray[top - 1]; return true; } }int length() const { return top; }};#endifFunction.cpp#include "function.h"#include "AStack.h"#include <math.h>#include <stdio.h>void calUserInfo(){cout<<"\t* 智能计算器V1.0*"<<endl;cout<<"\t*********************"<<endl;cout<<"\t* 1 * 2 * 3 * + * - *"<<endl;cout<<"\t* 4 * 5 * 6 * * * / *"<<endl;cout<<"\t* 7 * 8 * 9 * 0 * % *"<<endl;cout<<"\t* & * ^ * = * ( * ) *"<<endl;cout<<"\t*********************"<<endl; }int isp(char& ch){switch(ch){case '=':return 0;break;case '+':case '-':return 3;break;case '*':case '/':case '%':return 5;case '(':return 1;break;case ')':return 8;break;case '^':case '&':return 7;break;}}int osp(char& ch) {switch(ch) {case '=':return 0;break;case '+':case '-':return 2;break;case '*':case '/':case '%':return 4;break;case '(':return 8;break;case ')':break;case '^':case '&':return 6;break;}}double extract(double x,double y){return pow(x,1/y);}bool cal(char op, double x, double y, double& r) {int o = 0;switch(op){case '-':r = x - y;break;case '+':r = x + y;break;case '/':r = x / y;break;case '%':(int) o = (int)x % (int)y;r = (double)o;break;case '*':r = x * y;case '&':r = extract(x,y);break;case '^':r = pow(x,y);break;}return true;}bool isDigit(char ch){if (((int)ch >= 48) && ((int)ch <= 57))return true;else return false;}bool isPoint(char ch){if (ch == '.')return true;else return false;}bool isOperator(char ch){if ((ch == '=') || (ch == '-') || (ch == '+') || (ch == '(') || (ch == ')') || (ch == '*') || (ch == '&') ||(ch == '/') ||(ch == '%') ||(ch == '^'))return true;else return false;}double turnDigit(char ch){double value;value = (double)ch - 48;return value;}double newDigit(double prior_digit, double now_digit, bool isHavePoint, double point_num){double value;if(!isHavePoint){value = prior_digit*10 + now_digit;}else{value = prior_digit + now_digit/(pow(10,point_num));}return value;}Function.h#ifndef FUNCTION_HEADER#define FUNCTION_HEADER//友好的用户界面void calUserInfo();//用于记录处于栈内的优先级int isp(char& ch);//用于记录栈外的优先级int osp(char& ch);//相当于value = x op y;如果cal成功,然后将value的值返回r,用r来保存bool cal(char op, double x, double y, double& r);//判断是否为字符bool isDigit(char ch);//判断是否为小数点bool isPoint(char ch);//判断是否为操作运算符bool isOperator(char ch);//将数字的字符状态转化成double状态double turnDigit(char ch);//如果几个数字或加一个小数点连在一起,这说明他们是同一个数字的不同位数,此函数将不同连在一起的数字//组成新的数字double newDigit(double prior_digit, double now_digit, bool isHavePoint, double point_num);#endifMain.cpp#include "AStack.h"#include "function.h"#include <iostream>#include <stdlib.h>#include <string.h>using namespace std;int main(){char str[100] = "\0";AStack<double> opnd; //用于存放数据AStack<char> optr; //用于存放操作符char now_ch = '='; //用于记录当前字符char prior_ch = '\0'; //用于记录前一个字符double now_dig = 0; //用于记录当前一个数字double prior_dig = 0; //用于记录前一个数字double value = 0; //用于存放计算后的值bool point = false; //用于判断是否有小数点int point_num = 1; //用于记录数字后面的小数点位数char topValue; //用于记录opnd的top上的值char option = 'Y'; //用于判断是否要继续运算do{prior_dig = 0; //在opnd中提前放一个0now_ch = '=';opnd.push(prior_dig);optr.push(now_ch);system("cls");calUserInfo();cout << "请输入表达式(以等号结束):" << endl;cin >> str;bool exitPoint = false;/*对每个字符的处理*/for(int i = 0; i < strlen(str); i++){now_ch = str[i];/*判断是不是数字以及相关操作*/if (isDigit(now_ch)){now_dig = turnDigit(now_ch);if (isDigit(prior_ch)){opnd.pop(prior_dig);if(exitPoint){point = true;now_dig = newDigit(prior_dig, now_dig,point,point_num);point_num++;}elsenow_dig = newDigit(prior_dig, now_dig,point,point_num);}if(isPoint(prior_ch)){opnd.pop(prior_dig);now_dig = newDigit(prior_dig, now_dig,point,point_num);exitPoint = true;point_num++;}value = now_dig;opnd.push(now_dig);prior_ch = now_ch;}/*判断是不是小数点以及相关操作*/else if (isPoint(now_ch)){point = true;prior_ch = now_ch;}/*判断是不是操作符以及相关操作*/else if (isOperator(now_ch)){/*对用于数字操作的相关标记量进行清空,方便下一次数字操作*/point = false;point = 0;exitPoint = false;point_num = 1;/*看optr中是否有操作符存在,若不存在,则只放一个操作符进去*//* 但不进行任何操作*/if(optr.length() <= 1){optr.push(now_ch);prior_ch = now_ch;}/*optr已有操作符存在的话,开始进行优先级的比较*/else{optr.pop(topValue);/* 栈内优先级小于栈外优先级*/if(isp(topValue) < osp(now_ch)){optr.push(topValue);optr.push(now_ch);prior_ch = now_ch;}/*栈内优先级大于栈外优先级*/else if(isp(topValue) > osp(now_ch)){if(now_ch == ')' && topValue == '('){break;}do{double x = 1,y = 0;opnd.pop(x);opnd.pop(y);if( cal(topValue,y,x,value) ){opnd.push(value);}if(!optr.pop(topValue))break;}while(isp(topValue) > osp(now_ch));// if((topValue != '('))if (now_ch != ')'){optr.push(topValue);optr.push(now_ch);}prior_ch = now_ch;}/*其他情况报错*/elsebreak;}}/*其他情况报错*/elsecout << "输入的表达式错误,请检查!" << endl; }optr.pop(prior_ch);/*打印最后的计算值*/if(prior_ch == '='){cout << "最终得出的数据为: " << value << endl;}elsecout << "输入的表达式错误,请检查!" << endl;/*判断是否还要继续*/cout << "是否继续? ,继续'Y'/结束'N' :" << endl;cin >> option;/*清空数字栈和操作符栈*/opnd.clear();optr.clear();}while(option == 'Y');return 0;}。