C语言模拟CPU调度
操作系统进程调度C语言代码

操作系统进程调度C语言代码操作系统是计算机系统中的重要组成部分,用于管理和控制计算机资源的分配和使用。
在操作系统中,进程调度是指将系统资源(如 CPU、内存、磁盘、网络等)分配给运行中的进程的过程。
进程调度是操作系统实现多任务、多用户和分时系统的关键。
进程调度的算法有多种。
最常见的是时间片轮转算法、优先级调度算法、最短进程优先算法和先到先服务算法等。
下面我们将介绍一下时间片轮转算法的 C 语言代码实现。
1. 时间片轮转算法时间片轮转算法是一种基于时间片的调度算法,它给每个进程分配一个时间片,当时间片用完后,系统将进程暂停,并将 CPU 分配给下一个进程。
时间片轮转算法可以让所有进程公平地使用 CPU 时间,并且可以避免进程饥饿的情况发生。
2. C 语言代码实现在 C 语言中,可以用结构体来表示一个进程,包括进程 ID、进程状态、优先级、到达时间和需要运行的时间片等属性。
下面是一个简单的进程结构体的定义:```struct Process processes[5];在实现时间片轮转算法之前,需要先实现一个进程调度函数,它的作用是找到就绪进程中优先级最高的进程,并返回它的位置。
下面是一个简单的进程调度函数:```int find_highest_priority_process(struct Process *processes, int n) {int highest_priority = -1;int highest_priority_index = -1;for (int i = 0; i < n; i++) {if (processes[i].state != 2 && processes[i].priority >highest_priority) {highest_priority = processes[i].priority;highest_priority_index = i;}}return highest_priority_index;}```在实现时间片轮转算法之前,需要先定义一些全局变量,包括就绪队列、当前时间、时间片大小和进程数量等。
操作系统进程调度算法(c语言实现)
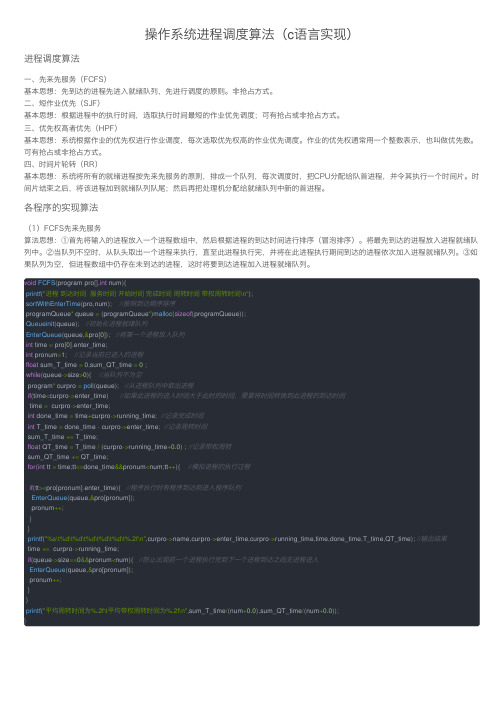
操作系统进程调度算法(c语⾔实现)进程调度算法⼀、先来先服务(FCFS)基本思想:先到达的进程先进⼊就绪队列,先进⾏调度的原则。
⾮抢占⽅式。
⼆、短作业优先(SJF)基本思想:根据进程中的执⾏时间,选取执⾏时间最短的作业优先调度;可有抢占或⾮抢占⽅式。
三、优先权⾼者优先(HPF)基本思想:系统根据作业的优先权进⾏作业调度,每次选取优先权⾼的作业优先调度。
作业的优先权通常⽤⼀个整数表⽰,也叫做优先数。
可有抢占或⾮抢占⽅式。
四、时间⽚轮转(RR)基本思想:系统将所有的就绪进程按先来先服务的原则,排成⼀个队列,每次调度时,把CPU分配给队⾸进程,并令其执⾏⼀个时间⽚。
时间⽚结束之后,将该进程加到就绪队列队尾;然后再把处理机分配给就绪队列中新的⾸进程。
各程序的实现算法(1)FCFS先来先服务算法思想:①⾸先将输⼊的进程放⼊⼀个进程数组中,然后根据进程的到达时间进⾏排序(冒泡排序)。
将最先到达的进程放⼊进程就绪队列中。
②当队列不空时,从队头取出⼀个进程来执⾏,直⾄此进程执⾏完,并将在此进程执⾏期间到达的进程依次加⼊进程就绪队列。
③如果队列为空,但进程数组中仍存在未到达的进程,这时将要到达进程加⼊进程就绪队列。
void FCFS(program pro[],int num){printf("进程到达时间服务时间开始时间完成时间周转时间带权周转时间\n");sortWithEnterTime(pro,num);//按照到达顺序排序programQueue* queue =(programQueue*)malloc(sizeof(programQueue));Queueinit(queue);//初始化进程就绪队列EnterQueue(queue,&pro[0]);//将第⼀个进程放⼊队列int time = pro[0].enter_time;int pronum=1;//记录当前已进⼊的进程float sum_T_time =0,sum_QT_time =0;while(queue->size>0){//当队列不为空program* curpro =poll(queue);//从进程队列中取出进程if(time<curpro->enter_time)//如果此进程的进⼊时间⼤于此时的时间,需要将时间转换到此进程的到达时间time = curpro->enter_time;int done_time = time+curpro->running_time;//记录完成时间int T_time = done_time - curpro->enter_time;//记录周转时间sum_T_time += T_time;float QT_time = T_time /(curpro->running_time+0.0);//记录带权周转sum_QT_time += QT_time;for(int tt = time;tt<=done_time&&pronum<num;tt++){//模拟进程的执⾏过程if(tt>=pro[pronum].enter_time){//程序执⾏时有程序到达则进⼊程序队列EnterQueue(queue,&pro[pronum]);pronum++;}}printf("%s\t%d\t%d\t%d\t%d\t%d\t%.2f\n",curpro->name,curpro->enter_time,curpro->running_time,time,done_time,T_time,QT_time);//输出结果time += curpro->running_time;if(queue->size==0&&pronum<num){//防⽌出现前⼀个进程执⾏完到下⼀个进程到达之间⽆进程进⼊EnterQueue(queue,&pro[pronum]);pronum++;}}printf("平均周转时间为%.2f\t平均带权周转时间为%.2f\n",sum_T_time/(num+0.0),sum_QT_time/(num+0.0));}(2)短作业优先(SJF)算法思想:①⾸先也是按进程的到达时间进⾏排序。
操作系统实验处理机调度C语言实现
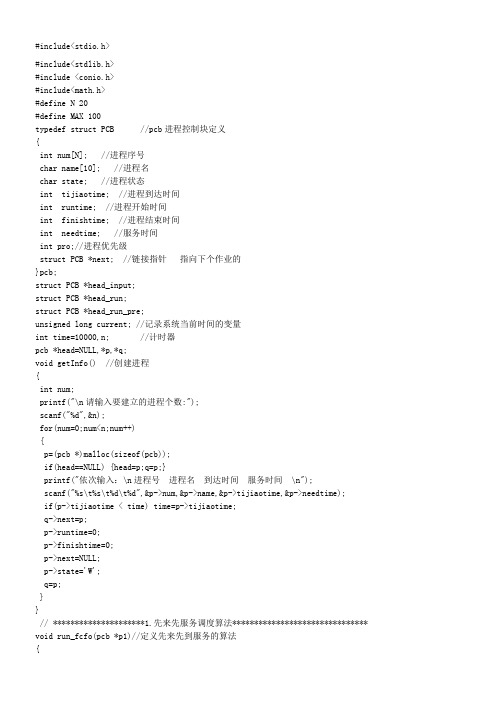
#include<stdio.h>#include<stdlib.h>#include <conio.h>#include<math.h>#define N 20#define MAX 100typedef struct PCB //pcb进程控制块定义{int num[N]; //进程序号char name[10]; //进程名char state; //进程状态int tijiaotime; //进程到达时间int runtime; //进程开始时间int finishtime; //进程结束时间int needtime; //服务时间int pro;//进程优先级struct PCB *next; //链接指针指向下个作业的}pcb;struct PCB *head_input;struct PCB *head_run;struct PCB *head_run_pre;unsigned long current; //记录系统当前时间的变量int time=10000,n; //计时器pcb *head=NULL,*p,*q;void getInfo() //创建进程{int num;printf("\n请输入要建立的进程个数:");scanf("%d",&n);for(num=0;num<n;num++){p=(pcb *)malloc(sizeof(pcb));if(head==NULL) {head=p;q=p;}printf("依次输入:\n进程号进程名到达时间服务时间 \n");scanf("%s\t%s\t%d\t%d",&p->num,&p->name,&p->tijiaotime,&p->needtime);if(p->tijiaotime < time) time=p->tijiaotime;q->next=p;p->runtime=0;p->finishtime=0;p->next=NULL;p->state='W';q=p;}}// *********************1.先来先服务调度算法******************************* void run_fcfo(pcb *p1)//定义先来先到服务的算法{time = p1->tijiaotime > time? p1->tijiaotime:time;p1->runtime=time;printf("\n现在时间是%d,开始运行进程%s\n",time,p1->name);time+=p1->needtime;p1->state='F';p1->finishtime=time;printf("进程名开始时间所需时间结束时间\n");printf("%s %d %d %d ",p1->name,p1->runtime,p1->needtime,p1->finishtime); }void fcfo()//定义运行进程函数{int i,j,t;for(j=0;j<n;j++){p=head;t=10000;for(i=0;i<n;i++) //找到当前未完成的进程{if(p->tijiaotime<t && p->state=='W'){t=p->tijiaotime;q=p; //标记当前未完成的进程}p=p->next;}run_fcfo(q);}}// ************************2.优先级调度服务算法************************************int readydata(){ //建立就绪队列if(head_input->next==NULL){return 0;}struct PCB *p1=head_input->next,*pmax,*p2;int maxpro=0xffff;pmax=p1;p2=head_input;while(p1!=NULL){if(p1->pro<maxpro){maxpro=p1->pro;head_run_pre=p2;pmax=p1;}p2=p1;p1=p1->next;}head_run=pmax;head_run_pre->next=head_run->next;return 1;}void runprocess() //运行进程函数{head_run->runtime-=10;head_run->pro++;struct PCB *p1,*p2;printf("时间片的大小 %d",current);current+=10;printf(" %s 开始\n",head_run->name);printf("时间片的大小 %d",current);printf(" %s 结束\n",head_run->name);if(head_run->runtime<=0){//判断进程是否运行结束}else{p1=head_input;p2=head_input->next;p1->next=head_run;head_run->next=p2;}}int readyprocess(){while(1){if(readydata()==0)return 0;else runprocess();}}void Init(){head_input=new PCB;head_input->next=NULL;current=0;int numpro;printf("请重新输入要建立的进程个数:");scanf("%d",&numpro);printf("请依次输入进程名运行时间优先级\n");for(int i=0;i<numpro;i++){struct PCB *p1=new PCB;scanf("%s",p1->name);scanf("%d",&p1->runtime);scanf("%d",&p1->pro);p1->state='C';p1->next=NULL;struct PCB *p2=head_input->next;head_input->next=p1;p1->next=p2;}}// ************************3.时间片轮转调度服务算法************************************ void shijianpian(){ int b,i,X,t,k;int a[MAX];//存放进程的剩余时间int cnt[MAX];//存放进程调度次数printf("请输入进程数:");scanf("%d",&X);printf("\n请输入时间片t大小:");scanf("%d",&t);printf("\n请依次输入各个进程的服务时间");for(i=0;i<X;i++){scanf("%d",&a[i]);cnt[i]=0;}printf("被调度进程\t进程调度次数 \t本次运行时间结果\t剩余时间\n");k=1;while(k){for(i=0;i<X;i++){if(a[i]!=0)if(a[i]>=t){a[i]-=t;b+=t;cnt[i]=cnt[i]+1;printf("\n\t%d\t\t%d\t\t%d\t\t%d",i+1,cnt[i],b,a[i]);}else{b=b+a[i];cnt[i]=cnt[i]+1;a[i]=0;printf("\n\t%d\t\t%d\t\t%d\t\t%d",i+1,cnt[i],b,a[i]);}else continue;}for(i=0;i<X;i++)if(a[i]!=0){ k=1;break;}else continue;if(i>=X)k=0;}}void main(){printf(" *******************************");printf("\n 1. 按先来先到服务调度的算法模拟\n"); printf(" *******************************");getInfo();fcfo();printf("\n *******************************");printf("\n 2. 按优先级调度的算法模拟\n");printf("\n *******************************\n"); Init();readyprocess();printf("\n *******************************");printf("\n 3. 按时间片轮转调度的算法模拟\n");printf(" *******************************\n"); shijianpian();printf(" \n");}。
调度算法C语言实现

调度算法C语言实现调度算法是操作系统中的重要内容之一,它决定了进程在系统中的运行方式和顺序。
本文将介绍两种常见的调度算法,先来先服务(FCFS)和最短作业优先(SJF),并用C语言实现它们。
一、先来先服务(FCFS)调度算法先来先服务(FCFS)调度算法是最简单的调度算法之一、它按照进程到达的先后顺序进行调度,即谁先到达就先执行。
实现这个算法的关键是记录进程到达的顺序和每个进程的执行时间。
下面是一个用C语言实现先来先服务调度算法的示例程序:```c#include <stdio.h>//进程控制块结构体typedef structint pid; // 进程IDint arrivalTime; // 到达时间int burstTime; // 执行时间} Process;int maiint n; // 进程数量printf("请输入进程数量:");scanf("%d", &n);//输入每个进程的到达时间和执行时间Process process[n];for (int i = 0; i < n; i++)printf("请输入进程 %d 的到达时间和执行时间:", i);scanf("%d%d", &process[i].arrivalTime,&process[i].burstTime);process[i].pid = i;}//根据到达时间排序进程for (int i = 0; i < n - 1; i++)for (int j = i + 1; j < n; j++)if (process[i].arrivalTime > process[j].arrivalTime) Process temp = process[i];process[i] = process[j];process[j] = temp;}}}//计算平均等待时间和平均周转时间float totalWaitingTime = 0; // 总等待时间float totalTurnaroundTime = 0; // 总周转时间int currentTime = 0; // 当前时间for (int i = 0; i < n; i++)if (currentTime < process[i].arrivalTime)currentTime = process[i].arrivalTime;}totalWaitingTime += currentTime - process[i].arrivalTime;totalTurnaroundTime += (currentTime + process[i].burstTime) - process[i].arrivalTime;currentTime += process[i].burstTime;}//输出结果float avgWaitingTime = totalWaitingTime / n;float avgTurnaroundTime = totalTurnaroundTime / n;printf("平均等待时间:%f\n", avgWaitingTime);printf("平均周转时间:%f\n", avgTurnaroundTime);return 0;```以上程序实现了先来先服务(FCFS)调度算法,首先根据进程的到达时间排序,然后依次执行每个进程,并计算总等待时间和总周转时间。
处理器调度算法c语言
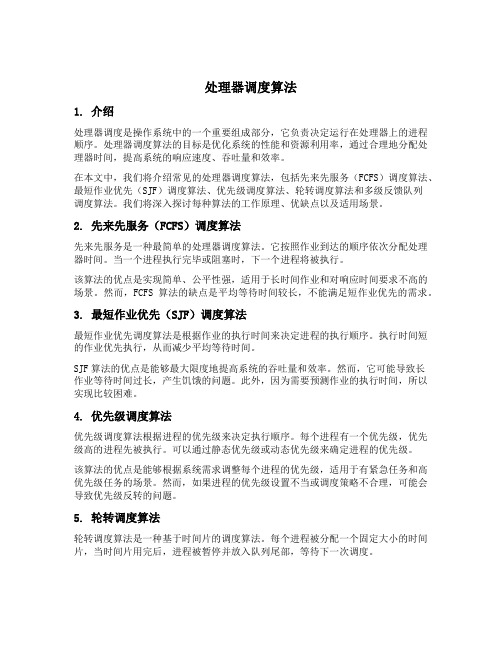
处理器调度算法1. 介绍处理器调度是操作系统中的一个重要组成部分,它负责决定运行在处理器上的进程顺序。
处理器调度算法的目标是优化系统的性能和资源利用率,通过合理地分配处理器时间,提高系统的响应速度、吞吐量和效率。
在本文中,我们将介绍常见的处理器调度算法,包括先来先服务(FCFS)调度算法、最短作业优先(SJF)调度算法、优先级调度算法、轮转调度算法和多级反馈队列调度算法。
我们将深入探讨每种算法的工作原理、优缺点以及适用场景。
2. 先来先服务(FCFS)调度算法先来先服务是一种最简单的处理器调度算法。
它按照作业到达的顺序依次分配处理器时间。
当一个进程执行完毕或阻塞时,下一个进程将被执行。
该算法的优点是实现简单、公平性强,适用于长时间作业和对响应时间要求不高的场景。
然而,FCFS算法的缺点是平均等待时间较长,不能满足短作业优先的需求。
3. 最短作业优先(SJF)调度算法最短作业优先调度算法是根据作业的执行时间来决定进程的执行顺序。
执行时间短的作业优先执行,从而减少平均等待时间。
SJF算法的优点是能够最大限度地提高系统的吞吐量和效率。
然而,它可能导致长作业等待时间过长,产生饥饿的问题。
此外,因为需要预测作业的执行时间,所以实现比较困难。
4. 优先级调度算法优先级调度算法根据进程的优先级来决定执行顺序。
每个进程有一个优先级,优先级高的进程先被执行。
可以通过静态优先级或动态优先级来确定进程的优先级。
该算法的优点是能够根据系统需求调整每个进程的优先级,适用于有紧急任务和高优先级任务的场景。
然而,如果进程的优先级设置不当或调度策略不合理,可能会导致优先级反转的问题。
5. 轮转调度算法轮转调度算法是一种基于时间片的调度算法。
每个进程被分配一个固定大小的时间片,当时间片用完后,进程被暂停并放入队列尾部,等待下一次调度。
轮转调度算法的优点是公平性好,能够合理分配处理器时间,适用于响应时间要求不高的场景。
但是,如果时间片过大,则可能导致长时间作业等待时间过长;如果时间片过小,则会导致上下文切换频繁,影响系统性能。
c语言实现进程调度算法

c语言实现进程调度算法进程调度算法是操作系统中的一个重要组成部分,用于决定在多道程序环境下,选择哪个进程来占用CPU并执行。
C语言是一种通用的编程语言,可以用于实现各种进程调度算法。
这里我将分别介绍三种常见的进程调度算法:先来先服务调度算法(FCFS)、最短作业优先调度算法(SJF)和轮转法调度算法(RR),并给出用C语言实现的示例代码。
首先,我们来看先来先服务调度算法(FCFS)。
此算法根据到达时间的先后顺序,按照先来后到的顺序进行处理。
下面是基于C语言的先来先服务调度算法实现示例代码:```c#include<stdio.h>struct Process};void FCFS(struct Process proc[], int n)for (int i = 1; i < n; i++)}printf("进程号到达时间服务时间完成时间等待时间周转时间\n");for (int i = 0; i < n; i++)}for (int i = 0; i < n; i++)}int maiint n;printf("请输入进程数:");scanf("%d", &n);struct Process proc[n];for (int i = 0; i < n; i++)printf("请输入进程%d的到达时间和服务时间(用空格分隔):", i + 1);}FCFS(proc, n);return 0;```其次,我们来看最短作业优先调度算法(SJF),该算法选择执行时间最短的进程先执行。
下面是基于C语言的最短作业优先调度算法实现示例代码:```c#include<stdio.h>struct Process};void SJF(struct Process proc[], int n)for (int i = 0; i < n; i++)for (int j = 0; j < i; j++)}shortest_job = i;for (int j = i + 1; j < n; j++)shortest_job = j;}}}for (int i = 1; i < n; i++)}printf("进程号到达时间服务时间完成时间等待时间周转时间\n");for (int i = 0; i < n; i++)}for (int i = 0; i < n; i++)}int maiint n;printf("请输入进程数:");scanf("%d", &n);struct Process proc[n];for (int i = 0; i < n; i++)printf("请输入进程%d的到达时间和服务时间(用空格分隔):", i + 1);}SJF(proc, n);return 0;```最后,我们来看轮转法调度算法(RR),该算法分配一个时间片给每个进程,当时间片用完后,将CPU分配给下一个进程。
操作系统进程调度优先级算法C语言模拟

操作系统进程调度优先级算法C语言模拟```cstruct Processint pid; // 进程IDint priority; // 优先级};```接下来,我们使用一个简单的示例来说明操作系统进程调度优先级算法的模拟实现。
假设有5个进程需要调度执行,它们的初始优先级和运行时间如下:进程ID,优先级,已运行时间--------,--------,------------P1,4,2P2,3,4P3,1,6P4,2,1P5,5,3首先,我们需要将这些进程按照优先级排序,以得到调度队列。
可以使用冒泡排序算法实现,代码如下:```cvoid bubbleSort(struct Process *processes, int n)for (int i = 0; i < n - 1; i++)for (int j = 0; j < n - i - 1; j++)if (processes[j].priority > processes[j + 1].priority)struct Process temp = processes[j];processes[j] = processes[j + 1];processes[j + 1] = temp;}}}``````c#include <stdio.h>void bubbleSort(struct Process *processes, int n);int maistruct Process processes[] = {{1, 4, 2}, {2, 3, 4}, {3, 1, 6}, {4, 2, 1}, {5, 5, 3}};int n = sizeof(processes) / sizeof(struct Process);bubbleSort(processes, n);printf("初始调度队列:\n");printf("进程ID\t优先级\t已运行时间\n");for (int i = 0; i < n; i++)}//模拟进程调度printf("\n开始模拟进程调度...\n");int finished = 0;while (finished < n)struct Process *current_process = &processes[0];printf("执行进程 P%d\n", current_process->pid);finished++;printf("进程 P%d 执行完毕\n", current_process->pid);} else}bubbleSort(processes, n);}printf("\n所有进程执行完毕,调度队列的最终顺序为:\n"); printf("进程ID\t优先级\t已运行时间\n");for (int i = 0; i < n; i++)}return 0;```以上代码中,我们使用了一个变量`finished`来记录已完成的进程数量,当`finished`等于进程数量`n`时,所有进程执行完毕。
用c语言编写进程调度的算法

用c语言编写进程调度的算法进程调度是计算机操作系统中一个很重要的组成部分。
目的是通过选择适当的策略,合理地分配处理器时间,保证各个进程有公正的机会去执行它们的任务,从而达到提高系统性能,提高使用量的目的。
进程调度算法根据不同的策略的不同优先级进行分类,并根据优先级来确定在什么时候该执行进程。
下面,我们将讨论一下基于策略的进程调度算法。
1.先入先出调度(FIFO)先入先出调度算法是一种最基本的调度算法。
该算法的执行方式是根据进程请求进入内存的时间顺序,进行排队,进入队列最开始的进程被分配CPU处理时间并运行,直到进程完成或发生中断或问题撤销。
但是,这种方法存在一个明显的缺陷-平均等待时间相对较长,而优先级较高的任务需要等待一段时间才能执行。
当有一个长时间的任务进入系统时,整个系统的响应时间也很长。
2.短作业(SJF)调度短作业优先(SJF)调度算法,是指根据任务在CPU上运行所需的时间长度(即任务的长度)来选择进程。
以开始时间和运行时间和作业大小为基础的调度算法,目的是为了尽可能地减少平均等待时间和平均花费时间。
该算法中,进程会依照作业长度被分配执行时间。
因此,若进程需要执行较长的作业,可能会将优先权移交给较短的作业,而导致长时间等待。
3.基于优先级的调度算法基于优先级的调度算法是指根据不同作业或进程的优先级,选定当前优先级最高的进程进行执行。
该算法的优先级分类方式可以分为静态优先级和动态优先级。
静态优先级:这种算法分先分配好不同进程的不同优先级,每个进程都有自己的优先级。
在动态运行过程中,进程优先级不发生变化。
该算法的缺点是对于散乱的进程和许多小型进程,此算法的相反效果很快变现。
动态权重算法:这种算法分配给程序一个初始权重,不断根据进程的相对优先级而减小或增加权重。
当进程被选中或被执行时,权重会下降。
进程被阻止或者等待时,权重会增加使之在下一次调度时有更高的几率获得执行的机会。
4.多级反馈调度算法多级反馈调度算法是一种渐进式算法。
c语言课程设计处理机低级调度模拟系统

《高级程序设计语言》课程设计报告题目:处理机低级调度模拟系统专业:网络工程班级: 10….学号: 00000000000姓名: *********指导教师: *******完成日期: 2013年 3月 30 日一、课程设计的目的1、掌握C语言数组、函数、指针、结构体的综合应用。
2、掌握使用C语言,进行应用性的开发。
3、掌握系统数据结构与算法的设计。
二、课程设计的内容课程设计题目:处理机低级调度模拟系统课程设计内容:根据操作系统处理机不同的调度算法,使用C语言模拟实现处理机调度过程。
1、系统数据结构(1)进程控制块(pcb):进程名称、到达时间、进程要求运行时间、进程已运行时间、指针、进程状态等等(要根据不同算法的需要定义全面的数据结构)(2)进程队列(PQueue):链表……2、调度算法(1)先来先服务调度(FCFS):按照进程提交给系统的先后顺序来挑选进程,先提交的先被挑选。
(2)多级反馈队列调度(FB,第i级队列的时间片=2i-1):(a)应设置多个就绪队列,并为各个队列赋予不同的优先级。
(b)当一个新进程进入内存后,首先将它放入第一队列的末尾,按FCFS的原则排队等待调度。
当轮到该进程执行时,如他能在该时间片内完成,便可准备撤离系统;如果它在一个时间片结束时尚未完成,调度程序便将该进程转入第二队列的末尾,再同样地按FCFS原则等待调度执行;如果它在第二队列中运行一个时间片后仍未完成,再依次将它放入第三队列……,如此下去,当一个长作业进程从第一队列依次降到第N队列后,在第N队列中便采取时间片轮转的方式运行。
(c)仅当第一队列空闲时,调度程序才调度第二队列中的进程运行。
三、课程设计的要求1、按照给出的题目内容(1)完成系统数据结构设计与实现、系统算法设计与实现、系统模块设计与实现、系统总体的设计与实现。
(2)系统需要一个简单操作界面,例如:===========================1. 先来先服务调度2. 多级反馈队列调度3. 退出(按数字1、2、3、,选择操作)===========================(3)对每种调度算法都要求输出每个进程(进程数不少于5)开始运行时刻、完成时刻、周转时间,以及这组进程的平均周转时间。
用C语言编写进程调度的算法

q=q->next;
}
return OK;
}
Status Printr(READYQueue ready,FINISHQueue finish){ //打印就绪队列中的进程状态
int i=0 ;
while(ready.RUN->next!=NULL)
{
ready.RUN->next->pcb.CPUTIME++;
ready.RUN->next->pcb.NEEDTIME--;
ready.RUN->next->pcb.PRIO-=3;
if(ready.RUN->next->pcb.NEEDTIME==0)
Status Print(READYQueue ready,FINISHQueue finish);
Status Printr(READYQueue ready,FINISHQueue finish);
Status Fisrt(READYQueue &ready);
Status Insert1(READYQueue &ready);
QueuePtr s=(QueuePtr)malloc(sizeof(QNode));
s->pcb=ready.RUN->next->pcb;
s->next=NULL; //将未完成的进程插入就绪队列
ready.TAIL->next=s;
ready.TAIL=s;
//按优先数从大到小排序
处理器调度算法模拟

for (;VI != VII;){//VI作为一个控制变量,VII作为一个终止变量
VI++;
for (VIII = VI; VIII != VII;VIII++){//VIII作为一个控制变量
if (i == 0){//按照最短作业;pc = pc;
}
void switchRegister(registerInfo&p){
cout << "register is being switched..." << endl;
cout << "current CPU register info is:" << endl;
cout << "create a process..." << endl;
cout << "process is created." << endl;
cout << "process info is:" << endl;
cout << *this;
}
friend ostream& operator<<(ostream&cout, process&p){
a = t1.burstTime;
b = t2.burstTime;
break;
case 2://按照进程优先级的大小
a = t1.processPCB.priority;
c语言实现多级反馈队列调度的算法模拟进程的控制
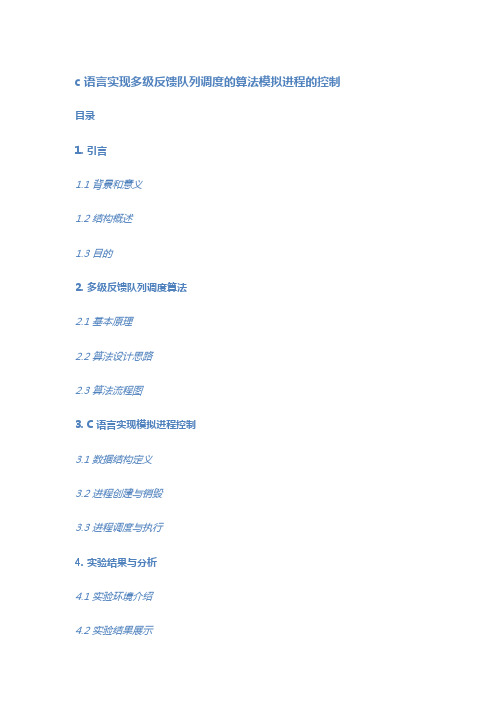
c语言实现多级反馈队列调度的算法模拟进程的控制目录1. 引言1.1 背景和意义1.2 结构概述1.3 目的2. 多级反馈队列调度算法2.1 基本原理2.2 算法设计思路2.3 算法流程图3. C语言实现模拟进程控制3.1 数据结构定义3.2 进程创建与销毁3.3 进程调度与执行4. 实验结果与分析4.1 实验环境介绍4.2 实验结果展示4.3 结果分析与讨论5. 结论与展望5.1 结论总结5.2 存在问题与改进方向1. 引言1.1 背景和意义在计算机科学领域,进程调度是操作系统中非常重要的一个功能。
它负责决定哪个进程可以使用CPU并以何种顺序执行。
进程调度算法的优劣直接影响了系统的性能和效率。
多级反馈队列调度算法是一种常用的进程调度算法之一,它可以根据进程的类型和优先级将进程划分到不同的队列中,并根据优先级来决定进程的执行顺序。
该算法兼具公平性和高响应时间两个特点,适合应用于多任务环境下。
本文旨在使用C语言实现模拟多级反馈队列调度算法,并通过模拟进程控制过程,探讨其在实际应用中的表现。
通过实验结果与分析,我们将评估该算法在不同场景下的性能指标,并对其中存在问题提出改进方向。
1.2 结构概述本文共分为五个部分组成:•引言:介绍本文撰写目的、研究背景以及多级反馈队列调度算法在进程控制中的重要性。
•多级反馈队列调度算法:详细介绍多级反馈队列调度算法的基本原理、设计思路和算法流程图。
•C语言实现模拟进程控制:描述C语言中如何定义数据结构以及实现进程的创建、销毁以及调度和执行过程。
•实验结果与分析:介绍实验环境并展示实验结果,对结果进行分析和讨论。
•结论与展望:总结本文的研究成果,并提出该算法在应用过程中存在的问题以及改进方向。
通过以上结构安排,我们将全面深入地探讨多级反馈队列调度算法在模拟进程控制中的应用。
1.3 目的本文旨在通过使用C语言实现多级反馈队列调度算法,模拟进程控制过程。
通过对该算法进行分析和实验,目标如下:1.探讨多级反馈队列调度算法在不同场景下的优劣势,并对其性能指标进行评估。
处理器调度算法c语言

处理器调度算法c语言一、概述处理器调度算法是操作系统中一个非常重要的问题。
在多任务操作系统中,有多个进程同时运行,而处理器只有一个,因此需要对进程进行调度,使得每个进程都能够得到适当的执行时间。
二、常见的处理器调度算法1. 先来先服务(FCFS)FCFS算法是最简单的调度算法之一。
它按照进程到达时间的先后顺序进行调度,即先到达的进程先执行。
这种算法容易实现,但可能会导致长作业等待时间过长。
2. 最短作业优先(SJF)SJF算法是根据每个进程所需的CPU时间来进行排序,并按照顺序进行调度。
这种算法可以减少平均等待时间和平均周转时间,并且可以最大限度地利用CPU资源。
3. 优先级调度优先级调度是根据每个进程的优先级来进行排序,并按照顺序进行调度。
这种算法可以确保高优先级进程得到更多的CPU时间,但可能会出现低优先级进程饥饿问题。
4. 时间片轮转(RR)RR算法将CPU分配给每个任务一定量的时间片,在该时间片内运行任务。
如果任务在该时间片内未完成,则将其放回队列尾部,并分配给下一个任务时间片。
这种算法可以确保公平性,并且可以避免长作业等待时间过长。
三、C语言中的处理器调度算法实现1. FCFS算法实现#include <stdio.h>int main(){int n, i, j;float avg_waiting_time = 0, avg_turnaround_time = 0;printf("Enter the number of processes: ");scanf("%d", &n);int burst_time[n], waiting_time[n], turnaround_time[n];printf("Enter the burst time for each process:\n");for(i=0; i<n; i++)scanf("%d", &burst_time[i]);waiting_time[0] = 0;turnaround_time[0] = burst_time[0];for(i=1; i<n; i++){waiting_time[i] = waiting_time[i-1] + burst_time[i-1];turnaround_time[i] = waiting_time[i] + burst_time[i];avg_waiting_time += waiting_time[i];avg_turnaround_time += turnaround_time[i];}avg_waiting_time /= n;avg_turnaround_time /= n;printf("\nProcess\tBurst Time\tWaiting Time\tTurnaround Time\n");for(i=0; i<n; i++)printf("P%d\t%d\t\t%d\t\t%d\n", i+1, burst_time[i], waiting_time[i], turnaround_time[i]);printf("\nAverage Waiting Time: %.2f\n", avg_waiting_ time);printf("Average Turnaround Time: %.2f\n", avg_turnaround_ time);return 0;}2. SJF算法实现#include <stdio.h>int main(){int n, i, j, temp;float avg_waiting_time = 0, avg_turnaround_time = 0; printf("Enter the number of processes: ");scanf("%d", &n);int burst_time[n], waiting_time[n], turnaround_time[n]; printf("Enter the burst time for each process:\n");for(i=0; i<n; i++)scanf("%d", &burst_time[i]);for(i=0; i<n-1; i++)for(j=i+1; j<n; j++)if(burst_time[i] > burst_time[j]){temp = burst_time[i];burst_time[i] = burst_time[j]; burst_time[j] = temp;}waiting_time[0] = 0;turnaround_time[0] = burst_time[0];for(i=1; i<n; i++){waiting_time[i] = waiting_time[i-1] + burst_time[i-1];turnaround_time[i] = waiting_time[i] + burst_time[i];avg_waiting_time += waiting_time[i];avg_turnaround_time += turnaround_time[i];}avg_waiting_time /= n;avg_turnaround_time /= n;printf("\nProcess\tBurst Time\tWaiting Time\tTurnaround Time\n");for(i=0; i<n; i++)printf("P%d\t%d\t\t%d\t\t%d\n", i+1, burst_time[i], waiting_time[i], turnaround_time[i]);printf("\nAverage Waiting Time: %.2f\n", avg_waiting_ time);printf("Average Turnaround Time: %.2f\n", avg_turnaround_ time);return 0;}3. 优先级调度算法实现#include <stdio.h>int main(){int n, i, j, temp;float avg_waiting_time = 0, avg_turnaround_time = 0;printf("Enter the number of processes: ");scanf("%d", &n);int burst_time[n], waiting_time[n], turnaround_time[n], priority[n];printf("Enter the burst time and priority for each process:\n"); for(i=0; i<n; i++)scanf("%d %d", &burst_time[i], &priority[i]);for(i=0; i<n-1; i++)for(j=i+1; j<n; j++)if(priority[i] > priority[j]){temp = priority[i];priority[i] = priority[j];priority[j] = temp;temp = burst_time[i];burst_time[i] = burst_time[j]; burst_time[j] = temp;}waiting_time[0] = 0;turnaround_time[0] = burst_time[0];for(i=1; i<n; i++){waiting_time[i] = waiting_time[i-1] + burst_time[i-1];turnaround_time[i] = waiting_time[i] + burst_time[i];avg_waiting_ time += waiting_ time[i];avg_turnaround_ time += turnaround_ time[i];}avg_waiting_ time /= n;avg_turnaround_ time /= n;printf("\nProcess\tBurst Time\tPriority\tWaiting Time\tTurnaround Time\n");for(i=0; i<n; i++)printf("P%d\t%d\t\t%d\t\t%d\t\t%d\n", i+1, burst_ time[i], priority[i], waiting_time[i], turnaround_time[i]);printf("\nAverage Waiting Time: %.2f\n", avg_waiting_ time);printf("Average Turnaround Time: %.2f\n", avg_turnaround _ time);return 0;}4. RR算法实现#include <stdio.h>int main(){int n, i, j, time_quantum;float avg_waiting_time = 0, avg_turnaround_time = 0;printf("Enter the number of processes: ");scanf("%d", &n);int burst_time[n], remaining_time[n], waiting_time[n], turnaround_time[n];printf("Enter the burst time for each process:\n");for(i=0; i<n; i++)scanf("%d", &burst_time[i]);printf("Enter the time quantum: ");scanf("%d", &time_quantum);for(i=0; i<n; i++)remaining_time[i] = burst_time[i];int t=0;while(1){int done = 1;for(i=0; i<n; i++){if(remaining_time[i] > 0){done = 0;if(remaining_ time[i] > time_ quantum){t += time_ quantum;remaining_ time[i] -= time_ quantum;}else{t += remaining _ time[i];waiting_time[i] = t - burst_time[i];remaining_ time[i] = 0;turnaround_ time[i] = waiting_time[i] + burst_time[i];avg_waiting_ time += waiting_ time[i];avg_turnaround _ time += turnaround_ time[i];}}}if(done == 1)break;}avg_waiting_ time /= n;avg_turnaround_ time /= n;printf("\nProcess\tBurst Time\tWaiting Time\tTurnaround Time\n");for(i=0; i<n; i++)printf("P%d\t%d\t\t%d\t\t%d\n", i+1, burst_time[i], waiting_time[i], turnaround_time[i]);printf("\nAverage Waiting Time: %.2f\n", avg_waiting_ time);printf("Average Turnaround Time: %.2f\n", avg_turnaround _ time);return 0;}四、总结以上是常见的处理器调度算法的C语言实现方式。
操作系统实验处理机调度C语言实现

操作系统实验处理机调度C语言实现
一、实验目的
1.理解操作系统分时调度的概念;
2.熟悉程序跟踪法;
3.开发一个C语言程序,实现调度算法。
二、实验内容
1.了解操作系统的分时调度概念,理解调度算法的实现原理;
2.根据操作系统调度实验案例中的要求,编写C语言程序并调试;
3.按照调度实验案例要求,进行操作系统分时调度实验,实现调度算法;
4.使用程序跟踪方法,对实验结果进行分析,并分析程序的调度结果。
三、实验步骤
1.仔细阅读操作系统分时调度实验,了解其原理;
2.编写C语言程序,实现调度算法;
3.编写操作系统分时调度实验的调度规则,并利用前面编写的C语言
程序实现调度规则;
4.设置实验测试数据,对操作系统分时调度进行模拟实验;
5.利用程序跟踪方法,进行实验结果分析及性能测试;
6.根据实验结果,对调度算法进行改进,提高系统调度效率;
7.完成实验报告,并总结分析程序的调度结果。
四、实验结果分析
1.首先,本次实验中,编写的C语言程序完成了操作系统分时调度要求,可以正确的实现调度算法。
2.其次,在实验中。
c语言编写的进程调度算法

c语言编写的进程调度算法C语言编写的进程调度算法进程调度是操作系统的核心功能之一,它负责按照一定的策略和算法,合理地分配CPU资源给正在运行或即将运行的进程,从而提高操作系统的性能和资源利用率。
在操作系统中,存在多种不同的进程调度算法,本文将以C语言编写进程调度算法为主题,一步一步回答。
第一步:定义进程结构体首先,我们需要定义一个进程的数据结构体,以便在调度算法中使用。
进程结构体包括进程ID、进程优先级、进程状态等信息。
以下是一个简单的进程结构体示例:ctypedef struct {int pid; 进程IDint priority; 进程优先级int state; 进程状态} Process;第二步:初始化进程队列进程队列是存储所有待调度进程的数据结构,可以使用链表或数组来实现。
在初始化进程队列之前,需要先创建一个空的进程队列。
以下是一个简单的初始化进程队列函数:c#define MAX_PROCESSES 100 最大进程数Process processQueue[MAX_PROCESSES]; 进程队列int processCount = 0; 当前进程数void initProcessQueue() {processCount = 0;}第三步:添加进程到队列在调度算法中,需要将新创建或运行的进程添加到进程队列中,这样才能对其进行调度。
以下是一个简单的添加进程到队列的函数:void addProcess(int pid, int priority, int state) {if (processCount >= MAX_PROCESSES) {printf("进程队列已满,无法添加进程!\n");return;}Process newProcess;newProcess.pid = pid;newProcess.priority = priority;newProcess.state = state;processQueue[processCount] = newProcess;processCount++;}第四步:实现进程调度算法进程调度算法决定了操作系统如何决定哪个进程应该被调度并获得CPU 资源。
时间片轮转算法及优先级调度算法C语言模拟实现收藏

时间片轮转算法及优先级调度算法C语言模拟实现收藏时间片轮转算法是一种常见的CPU调度算法,通过将进程按照到达顺序放置在一个就绪队列中,并且给予每个进程相同的时间片,当进程用完时间片后,将其放到队列的尾部,轮流执行其他进程。
优先级调度算法是根据进程的优先级来决定下一个执行的进程。
下面是使用C语言模拟实现时间片轮转算法和优先级调度算法的代码:```c#include <stdio.h>typedef structchar name[10];int arrivalTime;int burstTime;int remainingTime;} Process;int totalTurnaroundTime = 0;int totalWaitingTime = 0;//初始化每个进程的剩余执行时间for (int i = 0; i < numProcesses; i++)processes[i].remainingTime = processes[i].burstTime;}while (1)int allProcessesFinished = 1; // 标记所有进程是否执行完毕for (int i = 0; i < numProcesses; i++)if (processes[i].remainingTime > 0)allProcessesFinished = 0; // 还有未执行完毕的进程processes[i].remainingTime = 0;} else}printf("%s执行完毕,剩余时间:%d\n", processes[i].name, processes[i].remainingTime);}}if (allProcessesFinished)break; // 所有进程执行完毕,退出循环}}//计算平均周转时间和平均等待时间float averageTurnaroundTime = (float)totalTurnaroundTime / numProcesses;float averageWaitingTime = (float)totalWaitingTime / numProcesses;printf("平均周转时间:%.2f\n", averageTurnaroundTime);printf("平均等待时间:%.2f\n", averageWaitingTime);int maiint numProcesses;printf("请输入进程数量:");scanf("%d", &numProcesses);Process processes[numProcesses];for (int i = 0; i < numProcesses; i++)printf("请输入进程%d的名称、到达时间和执行时间:", i+1);scanf("%s%d%d", processes[i].name, &processes[i].arrivalTime, &processes[i].burstTime);}printf("请输入时间片大小:");return 0;```优先级调度算法:```c#include <stdio.h>typedef structchar name[10];int arrivalTime;int burstTime;int priority;int waitingTime;int turnaroundTime;} Process;void runPriority(Process* processes, int numProcesses)int totalWaitingTime = 0;int totalTurnaroundTime = 0;//对进程按照到达时间进行排序for (int i = 0; i < numProcesses; i++)for (int j = 0; j < numProcesses-i-1; j++)if (processes[j].arrivalTime > processes[j+1].arrivalTime) Process temp = processes[j];processes[j] = processes[j+1];processes[j+1] = temp;}}}processes[0].waitingTime = 0;//计算每个进程的等待时间和周转时间for (int i = 1; i < numProcesses; i++)processes[i].waitingTime = processes[i-1].waitingTime + processes[i-1].burstTime;processes[i].turnaroundTime = processes[i].waitingTime + processes[i].burstTime;totalWaitingTime += processes[i].waitingTime;totalTurnaroundTime += processes[i].turnaroundTime;}//计算平均等待时间和平均周转时间float averageWaitingTime = (float)totalWaitingTime / numProcesses;float averageTurnaroundTime = (float)totalTurnaroundTime / numProcesses;printf("平均等待时间:%.2f\n", averageWaitingTime);printf("平均周转时间:%.2f\n", averageTurnaroundTime);int maiint numProcesses;printf("请输入进程数量:");scanf("%d", &numProcesses);Process processes[numProcesses];for (int i = 0; i < numProcesses; i++)printf("请输入进程%d的名称、到达时间、执行时间和优先级:", i+1);scanf("%s%d%d%d", processes[i].name,&processes[i].arrivalTime, &processes[i].burstTime,&processes[i].priority);}runPriority(processes, numProcesses);return 0;```通过输入进程的信息,可以计算出使用时间片轮转算法和优先级调度算法的平均等待时间和平均周转时间,从而比较它们的效果。
操作系统处理机调度算法的实现c语言源代码
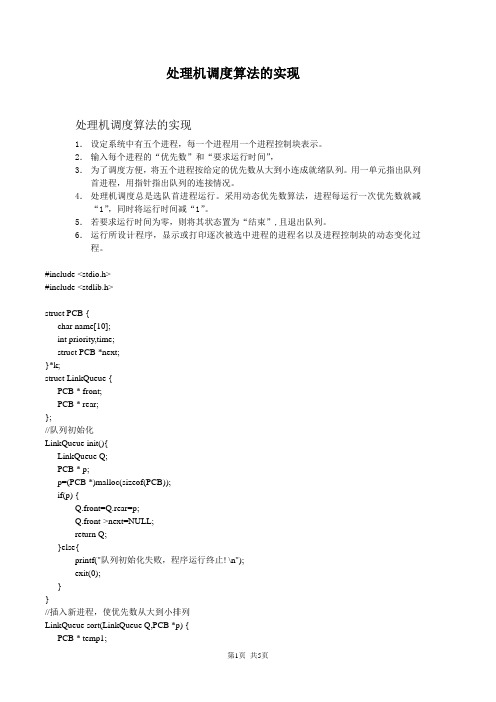
6.运行所设计程序,显示或打印逐次被选中进程的进程名以及进程控制块的动态变化过程。
#include <stdio.h>
#include <stdlib.h>
struct PCB {
char name[10];
int prБайду номын сангаасority,time;
struct PCB *next;
}*k;
struct LinkQueue {
PCB * front;
PCB * rear;
};
//队列初始化
LinkQueue init(){
LinkQueue Q;
PCB * p;
p=(PCB *)malloc(sizeof(PCB));
if(p) {
Q.front=Q.rear=p;
}
LinkQueue input(LinkQueue Q) /*建立进程控制块函数*/
{
int i;
for(i=1;i<=5;i++)
{
printf("\n进程号No.%d:\n",i);
k=(PCB *)malloc(sizeof(PCB));
printf("\n输入进程名:");
scanf("%s",k->name);
temp2=temp1->next;
}
if(temp2->next==NULL && temp2->priority>=p->priority) {
temp2->next=p;
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
C语言模拟CPU调度C语言模拟CPU调度CPU在处理多个进程时,要根据各种情况对处理的进程进行调度。
这其中就包括对各个进程优先级的处理,和调度算法的处理。
下面这个C语言程序,是我在大学期间学习《操作系统》课程的CPU调度时编写的,模拟了CPU的两种调度模式和各种模式所对应的多种调度算法。
为了模拟得更为形象,采用了图形屏幕输出。
#include<stdlib.h>#include<stdio.h>#include<conio.h>#include<graphics.h>#include<math.h>#include<dos.h>#define NULL 0/*-----------------------------------------------------------------*/ struct event /*事件结点结构*/{int evtype; /*1:进程产生。
2:进程执行。
3:激活阻塞进程。
4:进程执行完5:进程阻塞* 6:返回事件*/int pnum; /*执行该事件的进程号*/int t; /*事件发生的时间*/int ifblock; /*如果是执行事件标准其有无阻塞,其它事件时无定义*/struct event *next;};struct process /*进程结点结构*/{int pnum; /*进程号*/int plong; /*进程长度*/int prior; /*进程优先级*/int blocknum; /*进程当前的阻塞数*/int runtime; /*执行次数*/struct process *next;};struct headnod /*队列头结点结构*/{struct process *head; /*队列头*/int totalpro; /*队列中的进程数*/struct process *tail; /*队列尾*/};/*============================================ =====================*/int Mode,Algorithm; /*选择的调度模式和算法*/ struct event *evhead; /*事件链表的头结点*/struct headnod *ready,*block; /*就绪队列、阻塞队列的头结点*/main(){int gdriver,gmode;struct process *runpro=NULL; /*当前正在执行的进程*/ struct process *wakepro; /*当前被唤醒的进程*/ struct process *newpro; /*新建进程*/int busy=0; /*标志cpu状态*/menu(); /*选择调度模式和算法的菜单*/gdriver=DETECT;initgraph(&gdriver, &gmode, "");setbkcolor(LIGHTBLUE);cleardevice();welcome(); /*显示欢迎屏幕*/start(); /*初始化*/while(evhead!=NULL){switch(evhead->evtype){case 1: /*产生新进程并在就绪队列中排队*/{randomize();newpro=(struct process *)malloc(sizeof(struct process));newpro->pnum=evhead->pnum;newpro->plong=rand()%3+1;if((Mode==2)&&(Algorithm==2))newpro->prior=rand()%3+1;elsenewpro->prior=0; /*优先级相等*/if((Mode==2)&&(Algorithm==1)){newpro->runtime=newpro->plong+1;newpro->blocknum=rand()%2;}else{newpro->blocknum=rand()%3;newpro->runtime=1;}newpro->next=NULL;drawcreate(newpro); /*画出产生一个新进程*/Orderp(1,newpro);drawQ(1);sleep(1);if(ready->head==newpro){if(busy==0)Runevent();elseif((Mode==2)&&(Algorithm==2)&&(newp ro->prior>runpro->prior)){Interupt(runpro);returnev(runpro);}}}break;case 2: /*执行事件*/ {runpro=ready->head;ready->head=ready->head->next; runpro->next=NULL;ready->totalpro--;busy=1;Run(runpro); /*画进入cpu*/ drawQ(1);sleep(1);if(evhead->ifblock==1)blockev(runpro);else{runpro->runtime--;if(runpro->runtime==0)overev(runpro); /*产生结束事件*/elsereturnev(runpro);}}break;case 3: /*激活事件*/ {wakepro=block->head;block->head=block->head->next;wakepro->next=NULL;block->totalpro--;wakeup(wakepro);/*移动激活进程*/if(block->totalpro!=0){drawQ(2);sleep(1);}Orderp(1,wakepro);drawQ(1);sleep(1);if(ready->head==wakepro){if(busy==0)Runevent();elseif((Mode==2)&&(Algorithm==2)&&(wake pro->prior>runpro->prior)){Interupt(runpro);returnev(runpro);}}wakepro=NULL;if(block->totalpro!=0)wakeupev();}break;case 4: /*结束事件*/{over(runpro);runpro=NULL;busy=0;if(ready->totalpro!=0)Runevent();}break;case 5: /*阻塞事件*/ {blocked(runpro);busy=0;if(ready->totalpro!=0)Runevent();Orderp(2,runpro);drawQ(2);sleep(1);if(block->head==runpro)wakeupev();runpro=NULL;}break;case 6: /*返回事件*/ {returnwait(runpro); /*画出返回*/ busy=0;Orderp(1,runpro);runpro=NULL;drawQ(1);sleep(1);Runevent();}break;}evhead=evhead->next;}setcolor(LIGHTRED);settextstyle(1,0,0);setusercharsize(1, 1, 2, 1);outtextxy(290,340,"END!");setcolor(15);settextstyle(1,0,1);outtextxy(105,420,"Author : ZHOUWUBO CLASS : 01-1 NO.17");getch();getch();closegraph();}/*============================================ =====================*/menu() /*选择菜单*/{char wrong;textbackground(LIGHTBLUE);clrscr();window(20,3,60,23);textbackground(LIGHTRED);textcolor(YELLOW);clrscr();gotoy(2,2);cprintf("Please select the Scheduling Mode:");gotoxy(5,3);cprintf("1.Non-Preemptive");gotoxy(5,4);cprintf("2.Preemptive");gotoxy(7,5);cprintf(">> ");cscanf("%d",&Mode);switch(Mode){case 1:{gotoxy(2,7);cprintf("Please select the Scheduling Algorithm:");gotoxy(5,8);cprintf("1.FCFS");gotoxy(5,9);cprintf("2.SPF");gotoxy(7,10);cprintf(">> ");cscanf("%d",&Algorithm);if((Algorithm!=1)&&(Algorithm!=2)){gotoxy(2,12);cprintf("Your select is wrong! Run once again!");scanf("%c",&wrong);}break;}case 2:{gotoxy(2,7);cprintf("Please select the Scheduling Algorithm:");gotoxy(5,8);cprintf("1.Round Robin");gotoxy(5,9);cprintf("2.Priority");gotoxy(7,10);cprintf(">> ");cscanf("%d",&Algorithm);if((Algorithm!=1)&&(Algorithm!=2)){gotoxy(2,12);cprintf("Your select is wrong! Run once again!");scanf("%c",&wrong);}break;}default:{gotoxy(2,7);cprintf("Your select is wrong! Run once again!"); scanf("%c",&wrong);}}/*-----------------------------------------------------------------*/ welcome() /*显示欢迎屏幕*/{int i;setcolor(14);outtextxy(45,178,"create");outtextxy(40,188,"process");outtextxy(555,210,"Finish");line(100,204,170,204);line(170,204,160,199);line(160,199,165,204);line(100,226,170,226);line(170,226,160,231);line(160,231,165,226);rectangle(370,40,420,60);rectangle(425,40,475,60);rectangle(480,40,530,60);rectangle(535,40,588,60);setcolor(LIGHTRED);if((Mode==1)&&(Algorithm==1))int arw[8]={395,62,385,77,405,77,395,62};setlinestyle(0, 0, 3);drawpoly(4,arw);}if((Mode==1)&&(Algorithm==2)) {int arw[8]={450,62,440,77,460,77,450,62};setlinestyle(0, 0, 3);drawpoly(4,arw);}if((Mode==2)&&(Algorithm==1)) {int arw[8]={505,62,495,77,515,77,505,62};setlinestyle(0, 0, 3);drawpoly(4,arw);}if((Mode==2)&&(Algorithm==2)) {int arw[8]={561,62,551,77,571,77,561,62};setlinestyle(0, 0, 3);drawpoly(4,arw);setlinestyle(0, 0, 1);settextstyle(2,0,7);outtextxy(373,40,"FCFS");outtextxy(434,40,"SPF");outtextxy(495,40,"RR");settextstyle(2,0,5);outtextxy(537,42,"Priorty"); settextstyle(1,0,0);setusercharsize(1, 1, 2, 1);outtextxy(82,18, "CPU SCHEDULING"); outtextxy(280,360,"start!");setcolor(14);line(220,204,230,204);line(230,204,230,200);line(230,200,380,200);line(380,200,380,204);line(380,204,390,204);line(390,204,424,180);line(424,180,424,170);line(456,180,456,100);line(456,100,180,100);line(180,204,175,194);line(175,194,180,199);line(456,180,490,204);line(490,204,510,204); settextstyle(1,0,1);outtextxy(275,180,"READY"); line(220,226,230,226);line(230,226,230,230);line(230,230,380,230);line(380,230,380,226);line(380,226,390,226);line(390,226,424,250);line(424,250,424,260);line(456,250,456,330);line(456,330,410,330);line(410,330,410,334);line(410,334,260,334);line(260,334,260,330);line(260,330,180,330);line(180,330,180,226);line(180,226,175,236);line(420,308,410,308);line(410,308,410,304);line(410,304,260,304);line(260,304,260,308);line(260,308,250,308);line(456,250,490,226);line(490,226,510,226);outtextxy(305,285,"BLOCK");circle(440,215,30);sleep(2);setcolor(LIGHTBLUE);for(i=0;i<=43;i++){line(280+i,360,280+i,420);line(365-i,360,365-i,420);delay(5000);}}/*-----------------------------------------------------------------*/ start() /*初始化*/{int i;int t;struct event *p1,*p2;p1=(struct event *)malloc(sizeof(struct event));p1->evtype=1;p1->pnum=1;p1->t=0;p1->ifblock=0; /*在进程产生事件中无意义*/ p1->next=NULL;t=p1->t;evhead=p1;randomize();for(i=2;i<=5;i++){p2=(struct event *)malloc(sizeof(struct event)); p2->evtype=1;p2->pnum=i;p2->t=t+rand()%2+1;p2->ifblock=0;p2->next=NULL;t=p2->t;p1->next=p2;p1=p1->next;}ready=(struct headnod*)malloc(sizeof(struct headnod));ready->head=NULL;ready->totalpro=0;ready->tail=NULL;block=(struct headnod*)malloc(sizeof(struct headnod));block->head=NULL;block->totalpro=0;block->tail=NULL;}/*-----------------------------------------------------------------*/ drawcreate(struct process *p) /*画出进程的产生过程*/ {void *buf;int i,size;setfillstyle(1,p->pnum);bar(56,206,55+10*p->plong,224);size=imagesize(55,205,56+10*p->plong,225);buf=malloc(size);getimage(55,205,56+10*p->plong,225,buf);for(i=0; i<134; i++){putimage(55+i,205,buf,COPY_PUT);delay(3500);}free(buf);}/*-----------------------------------------------------------------*/ Orderp(int flag,struct process *p)/*1:就绪队列排队2:阻塞队列排队*/{struct process *q;if(flag==1) /*就绪队列*/{if(ready->totalpro==0){ready->head=p;ready->tail=p;ready->totalpro++;}else{if((Mode==1)&&(Algorithm==1)) /*FCFS*/{ready->tail->next=p;ready->tail=ready->tail->next;ready->totalpro++;}if((Mode==1)&&(Algorithm==2)) /*短进程优先*/{if(p->plong<ready->head->plong) /*长度小于当前队头*/{p->next=ready->head;ready->head=p;ready->totalpro++;}else /*长度大于当前队头*/{q=ready->head;while(q->next!=NULL){if(p->plong<q->next->plong) {p->next=q->next;q->next=p;ready->totalpro++;break;}elseq=q->next;}if(q->next==NULL){q->next=p;ready->totalpro++;ready->tail=p;}}}if((Mode==2)&&(Algorithm==1)) /*时间片轮转*/{ready->tail->next=p;ready->tail=ready->tail->next;ready->totalpro++;if((Mode==2)&&(Algorithm==2)) /*抢占优先级*/{if(p->prior>ready->head->prior) /*优先级高于当前队头*/{p->next=ready->head;ready->head=p;ready->totalpro++;}else /*优先级低于当前队头*/{q=ready->head;while(q->next!=NULL){if(p->prior>q->next->prior) {p->next=q->next;q->next=p;ready->totalpro++;break;elseq=q->next;}if(q->next==NULL) {q->next=p;ready->totalpro++;ready->tail=p;}}}}}if(flag==2) /*阻塞队列*/{if(block->totalpro==0){block->head=p;block->totalpro++;block->tail=p;}else{block->tail->next=p;block->tail=block->tail->next;block->totalpro++;}}}/*-----------------------------------------------------------------*/ drawQ(int flag) /*画出队列,flag=1:就绪队列,flag=2:阻塞队列*/{int i,j;struct process *p;if(flag==1){setcolor(LIGHTBLUE);for(i=0;i<=150;i++)line(230+i,205,230+i,225);for(i=0;i<=30;i++)line(189+i,203,189+i,227);p=ready->head;j=379;for(i=1;i<=ready->totalpro;i++) {setfillstyle(1,p->pnum);bar(j,206,j-10*p->plong+1,224);j=j-10*p->plong-1;p=p->next;}}if(flag==2){setcolor(LIGHTBLUE);for(i=0;i<=150;i++)line(260+i,309,260+i,39);for(i=0;i<=30;i++)line(420+i,308,420+i,329);p=block->head;j=261;for(i=1;i<=block->totalpro;i++) {setfillstyle(1,p->pnum);bar(j,309,j+10*p->plong-1,328);j=j+10*p->plong+1;p=p->next;}}}/*-----------------------------------------------------------------*/ Runevent() /*产生执行事件*/{struct event *p1;struct process *p;p=ready->head;randomize();p1=(struct event *)malloc(sizeof(struct event));p1->evtype=2;p1->pnum=p->pnum;p1->t=evhead->t;if(p->blocknum!=0){p1->ifblock=rand()%2;if(p1->ifblock==1)p->blocknum--;}elsep1->ifblock=0;p1->next=NULL;Orderev(p1);}/*-----------------------------------------------------------------*/ Interupt(struct process *p) /*中断当前正在执行的进程*/ {struct event *q1,*q2;if(evhead->next==NULL){if(evhead->evtype==4)p->runtime++;evhead=NULL;}else{q1=evhead->next;q2=evhead;}while(q1!=NULL){if(q1->pnum==p->pnum){if(q1->evtype==4)p->runtime++;q2->next=q1->next;break;}else{q2=q1;q1=q1->next;}}}/*-----------------------------------------------------------------*/ Orderev(struct event *q) /*在事件链表中插入结点*/ {struct event *p;p=evhead;while(p->next!=NULL){if(q->t<p->next->t){q->next=p->next;p->next=q;break;}elsep=p->next;}if(p->next==NULL)p->next=q;}/*----------------------------------------------------------------*/ blockev(struct process *p) /*产生阻塞事件*/{struct event *q;randomize();q=(struct event *)malloc(sizeof(struct event));q->evtype=5;q->pnum=p->pnum;q->t=evhead->t+rand()%4+2;q->ifblock=0;q->next=NULL;Orderev(q);}/*-----------------------------------------------------------------*/ blocked(struct process *p) /*画出阻塞过程*/{int i,size;void *buf;setcolor(LIGHTBLUE);for(i=1;i<=28;i++)circle(440,215,i);setfillstyle(1,p->pnum);bar(426,251,426+10*p->plong-2,269);size=imagesize(425,250,426+10*p->plong-1,270);buf=malloc(size);getimage(425,250,426+10*p->plong-1,270,buf);for(i=0; i<59; i++){putimage(425,250+i,buf,COPY_PUT);delay(3500);}for(i=0;i<5;i++){putimage(425-i,308,buf,COPY_PUT);delay(3500);}free(buf);}/*-----------------------------------------------------------------*/ wakeupev() /*产生唤醒事件*/{struct event *q;struct process *p;p=block->head;randomize();q=(struct event *)malloc(sizeof(struct event));q->evtype=3;q->pnum=p->pnum;q->t=evhead->t+rand()%3+4;q->ifblock=0; /*此时无意义*/q->next=NULL;Orderev(q);}/*-----------------------------------------------------------------*/ Run(struct process *p) /*画出执行*/int i,size;void *buf;size=imagesize(380,205,380-10*p->plong-1,225);buf=malloc(size);getimage(380,205,380-10*p->plong-1,225,buf);for(i=0; i<29; i++){putimage(380-10*p->plong-1+i,205,buf,COPY_PUT);delay(3500);}setcolor(LIGHTBLUE);for(i=0;i<29;i++)line(380+i,205,380+i,225);setcolor(p->pnum);for(i=1;i<=28;i++)circle(440,215,i);free(buf);}/*-----------------------------------------------------------------*/ overev(struct process *p) /*产生结束事件*/struct event *q;randomize();q=(struct event *)malloc(sizeof(struct event));q->evtype=4;q->pnum=p->pnum;if((Mode==2)&&(Algorithm==1))q->t=evhead->t+2; /*时间片为2*/elseq->t=evhead->t+3*(p->plong);/*时间由进程长度确定*/q->ifblock=0;q->next=NULL;Orderev(q);}/*-----------------------------------------------------------------*/ returnev(struct process *p) /*产生返回事件*/{struct event *q;randomize();q=(struct event *)malloc(sizeof(struct event));q->evtype=6;q->pnum=p->pnum;if((Mode==2)&&(Algorithm==2))q->t=evhead->t;elseq->t=evhead->t+2; /*时间片为2*/q->ifblock=0;q->next=NULL;Orderev(q);}/*-----------------------------------------------------------------*/ wakeup(struct process *p)/*画出唤醒过程*/{int i,size;void *buf;size=imagesize(260,309,261+10*p->plong,329);buf=malloc(size);getimage(260,309,261+10*p->plong,329,buf);for(i=0; i<72; i++){putimage(260-i,309,buf,COPY_PUT);delay(3500);}for(i=0;i<104;i++){putimage(189,309-i,buf,COPY_PUT);delay(3500);}free(buf);}/*-----------------------------------------------------------------*/ returnwait(struct process *p)/*画出返回过程*/{int i,size;void *buf;setcolor(LIGHTBLUE);for(i=1;i<=28;i++)circle(440,215,i);setfillstyle(1,p->pnum);bar(426,161,426+10*p->plong-2,179);size=imagesize(425,160,426+10*p->plong-1,180);buf=malloc(size);getimage(425,160,426+10*p->plong-1,180,buf);for(i=0; i<59; i++){putimage(425,160-i,buf,COPY_PUT);delay(3500);}for(i=0;i<237;i++){putimage(425-i,101,buf,COPY_PUT);delay(3500);}for(i=0;i<105;i++){putimage(189,101+i,buf,COPY_PUT);delay(3500);}free(buf);}/*-----------------------------------------------------------------*/ over(struct process *p) /*画出结束过程*/{int i,size;void *buf;int k=evhead->pnum;setcolor(LIGHTBLUE);for(i=1;i<=28;i++)circle(440,215,i);setfillstyle(1,k);bar(476,206,476+10*p->plong,224);size=imagesize(475,205,476+10*p->plong+1,225);buf=malloc(size);getimage(475,205,476+10*p->plong+1,225,buf);for(i=0; i<=45; i++){putimage(475+i,205,buf,COPY_PUT);delay(4000);}setcolor(LIGHTBLUE);for(i=0;i<=31;i++){line(520+i,205,520+i,225);delay(4000);}free(buf);}。