C语言下的学生管理系统(含密码加密和验证码)
c语言学生管理系统代码

C语言学生管理系统代码
简介
学生管理系统是一个用于管理学生信息的简单程序,它可以实现添加学生信息、显示学生信息、修改学生信息和删除学生信息等功能。
通过这个系统,学校或机构可以更方便地管理和维护学生的相关数据。
功能
1.添加学生信息:通过输入学生的姓名、学号、年龄和性别等信息,将
学生信息存储在系统中。
每个学生信息包括学号、姓名、年龄和性别等关键信息。
2.显示学生信息:可以显示系统中所有学生的信息,包括学号、姓名、
年龄和性别。
3.修改学生信息:通过输入学生的学号,可以修改该学生的姓名、年龄
和性别等信息。
4.删除学生信息:通过输入学生的学号,可以删除该学生的信息。
实现
下面是一个简单的C语言学生管理系统代码示例:
```c #include <stdio.h> #include <string.h>
#define MAX_STUDENTS 100
// 定义学生结构体 struct Student { int id; char name[100]; int age; char
gender[10]; };
// 定义全局变量,用于存储学生信息和统计学生数量 struct Student
students[MAX_STUDENTS]; int num_students = 0;
// 添加学生 void addStudent() { if (num_students >= MAX_STUDENTS) { printf(。
C语言学生信息管理系统
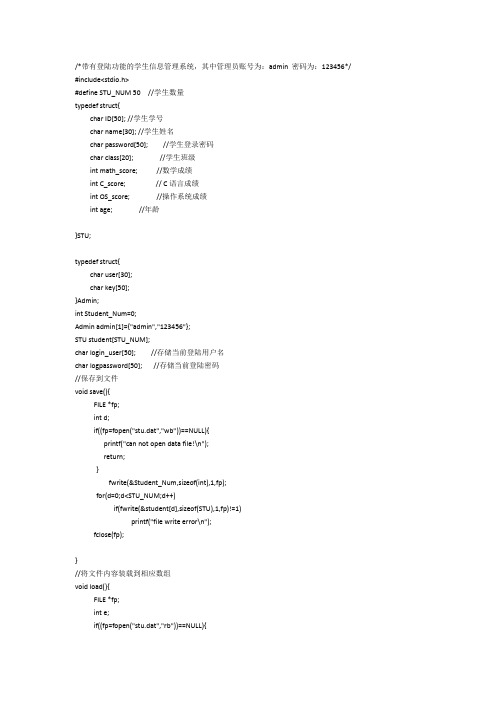
/*带有登陆功能的学生信息管理系统,其中管理员账号为:admin 密码为:123456*/ #include<stdio.h>#define STU_NUM 50 //学生数量typedef struct{char ID[50]; //学生学号char name[30]; //学生姓名char password[50]; //学生登录密码char class[20]; //学生班级int math_score; //数学成绩int C_score; // C语言成绩int OS_score; //操作系统成绩int age; //年龄}STU;typedef struct{char user[30];char key[50];}Admin;int Student_Num=0;Admin admin[1]={"admin","123456"};STU student[STU_NUM];char login_user[50]; //存储当前登陆用户名char logpassword[50]; //存储当前登陆密码//保存到文件void save(){FILE *fp;int d;if((fp=fopen("stu.dat","wb"))==NULL){printf("can not open data file!\n");return;}fwrite(&Student_Num,sizeof(int),1,fp);for(d=0;d<STU_NUM;d++)if(fwrite(&student[d],sizeof(STU),1,fp)!=1)printf("file write error\n");fclose(fp);}//将文件内容装载到相应数组void load(){FILE *fp;int e;if((fp=fopen("stu.dat","rb"))==NULL){printf("can not open file\n");return;}fread(&Student_Num,sizeof(int),1,fp);for(e=0;e<STU_NUM;e++)if(fread(&student[e],sizeof(STU),1,fp)!=1){if(feof(fp)){fclose(fp);return;}printf("file read error\n");}fclose(fp);}//管理员登陆int admin_login_confirm(){Admin temp;int wt=0;int confirm=0;do{printf("input your username:");scanf("%s",er);printf("input your password:");scanf("%s",temp.key);if(strcmp(er,admin[0].user)==0 && strcmp(temp.key,admin [0].key)==0){confirm=1;break;}else{confirm=0;wt++;printf("wrong password! \n");}}while(wt<3);return confirm;}int check(char ck[50]){int check=0;int ckflag=0;for(check=0;check<Student_Num;check++){if(strcmp(ck,student[check].ID)==0)ckflag=1;return ckflag;}return ckflag;}//学生登录int student_login(){int a;int ok=0;int wrong=0;STU tem;do{printf("user_ID:");scanf("%s",tem.ID);printf("password:");scanf("%s",tem.password);for(a=0;a<Student_Num;a++){if(strcmp(tem.ID,student[a].ID)==0 && strcmp(tem.password,student[a].password)==0){strcpy(login_user,student[a].ID);strcpy(logpassword,student[a].password);ok=1;break;}else{ok=0;}}if(ok==0){wrong++;printf("wrong password\n");}elsebreak;}while(wrong<3);return ok;}//添加学生信息void Add_student(){printf("ID:");scanf("%s",student[Student_Num].ID);while(check(student[Student_Num].ID)==1){printf("the ID is already have!\n");printf("ID:");scanf("%s",student[Student_Num].ID);}printf("name:");scanf("%s",student[Student_Num].name);printf("password:");scanf("%s",student[Student_Num].password);printf("class:");scanf("%s",student[Student_Num].class);printf("math_score:");scanf("%d",&student[Student_Num].math_score);printf("C_score:");scanf("%d",&student[Student_Num].C_score);printf("OS_score:");scanf("%d",&student[Student_Num].OS_score);printf("age:");scanf("%d",&student[Student_Num].age);Student_Num++;}//显示学生信息void display_student(){int b;printf("ID\tname\tclass\tmath_score\tC_score\t\tOS_score\tage\n");for(b=0;b<Student_Num;b++){printf("%s\t%s\t%s\t%d\t\t%d\t\t%d\t\t%d",student[b].ID,student[b].name,student[b].class,student[b].math_sc ore,student[b].C_score,student[b].OS_score,student[b].age);printf("\n");}}//更改学生信息void alter_student(){char alter[50];STU ch;int al,change=0;printf("the ID you want to change:");scanf("%s",alter);for(al=0;al<STU_NUM;al++){if(strcmp(alter,student[al].ID)==0){printf("ID:");scanf("%s",ch.ID);while(check(ch.ID)==1){printf("the ID is already have!\n");printf("ID:");scanf("%s",ch.ID);}printf("name:");scanf("%s",);printf("password:");scanf("%s",ch.password);printf("class:");scanf("%s",ch.class);printf("math_score:");scanf("%d",&ch.math_score);printf("C_score:");scanf("%d",&ch.C_score);printf("OS_score:");scanf("%d",&ch.OS_score);printf("age:");scanf("%d",&ch.age);printf("are you sure you want to change? (Y/N)[ ]\b\b");if(getchar()=='Y'||getchar()=='y'){student[al]=ch;change=1; break;}}}if(change==0) printf("no such user");save();}//查找void search(){char search[50];int sc;printf("the ID you want to search:");scanf("%s",search);for(sc=0;sc<STU_NUM;sc++){if(strcmp(search,student[sc].ID)==0) {printf("ID\tname\tclass\tmath_score\tC_score\t\tOS_score\tage\n");printf("%s\t%s\t%s\t%d\t\t%d\t\t%d\t\t%d",student[sc].ID,student[sc].name,student[sc].class,student[sc].math_ score,student[sc].C_score,student[sc].OS_score,student[sc].age);printf("\n");break;}}if(sc>=STU_NUM) printf("no such student!\n");}//删除void delete_student(){char del[50];STU dele;int de;printf("the ID you want to delete:");scanf("%s",del);for(de=0;de<STU_NUM;de++){if(strcmp(del,student[de].ID)==0) {printf("ID\tname\tclass\tmath_score\tC_score\t\tOS_score\tage\n");printf("%s\t%s\t%s\t%d\t\t%d\t\t%d\t\t%d",student[de].ID,student[de].name,student[de].class,student[de].mat h_score,student[de].C_score,student[de].OS_score,student[de].age);printf("\n");break;}}if(de>=STU_NUM) printf("no such student!\n");else{printf("are you sure you want to delete? y/n [ ]\b\b");if(getchar()=='Y'||getchar()=='y'){dele=student[de];student[de]=student[Student_Num-1];student[Student_Num-1]=dele;Student_Num--;}}save();}//更改学生登录密码void change_password(){char ps1[50],ps2[50],old[50];int point=0;int inputcnt=1;printf("please input your old password:");scanf("%s",old);while(strcmp(old,logpassword)!=0){printf("old password is wrong!\n");printf("please input your old password:");scanf("%s",old);if(inputcnt>=2) { printf("you have wrong 3 times!\n"); return;}inputcnt++;}printf("input new password:");scanf("%s",ps1);printf("confirm new password:");scanf("%s",ps2);if(strcmp(ps1,ps2)==0){printf("Are you sure to change? y/n [ ]\b\b");if(getchar()=='Y'||getchar()=='y'){for(point=0;point<Student_Num;point++){if(strcmp(login_user,student[point].ID)==0){strcpy(student[point].password,ps1);save();break;}}}}}//显示主菜单void display_Main_Menu(){printf("***************Welcome to Student Management System***************\n");printf("** **\n");printf("** 1.administrator Login er Login 3.exit system **\n");printf("** **\n");printf("******************************************************************\n");printf("your choice:[ ]\b\b");}//管理员功能菜单void display_admin_function(){printf("***********************************************\n");printf("**** M | 1.add student ****\n");printf("**** | 2.save stdudent information ****\n");printf("**** E | 3.alter student information ****\n");printf("**** | 4.search for student information ****\n");printf("**** N | 5.delete student information ****\n");printf("**** | 6.display student information ****\n");printf("**** U | 7.exit ****\n");printf("***********************************************\n");}//学生功能菜单void display_user_function(){printf("============student login============\n");printf("** 1.check information **\n");printf("** 2.change password **\n");printf("** 3.exit **\n");printf("=====================================\n");}//管理员功能选择int Admin_function(){int ach;while(1){display_admin_function();printf("select your choice[ ]\b\b");scanf("%d",&ach);switch(ach){case 1: Add_student(); break;case 2: save(); break;case 3: alter_student(); break;case 4: search(); break;case 5: delete_student(); break;case 6: display_student(); break;case 7: display_Main_Menu(); return 0;default: printf("repeat!\n"); break;}}}//学生功能选择int user_function(){int uch;int back=0;while(1){display_user_function();printf("hello,student make your choice[ ]\b\b");scanf("%d",&uch);switch(uch){case 1: display_student(); break;case 2: change_password(); break;case 3: back=1; return back;default: printf("everything is ok\n"); break;}return back;}int main(){int choice;load();display_Main_Menu();while(1){scanf("%d",&choice);switch(choice){case 1: if(admin_login_confirm()==1) {Admin_function(); break;}else{printf("you have input wrong password 3 times! exit the system...\n");return 0;}case 2: if(student_login()==1) {if(user_function()==1) return 0;elsebreak;}elseprintf("you have input wrong password 3 times! exit the system...\n");return 0;case 3: return 0;default: printf("please input 1~3\n");display_Main_Menu();break;}}}。
c语言程序设计学生信息管理系统
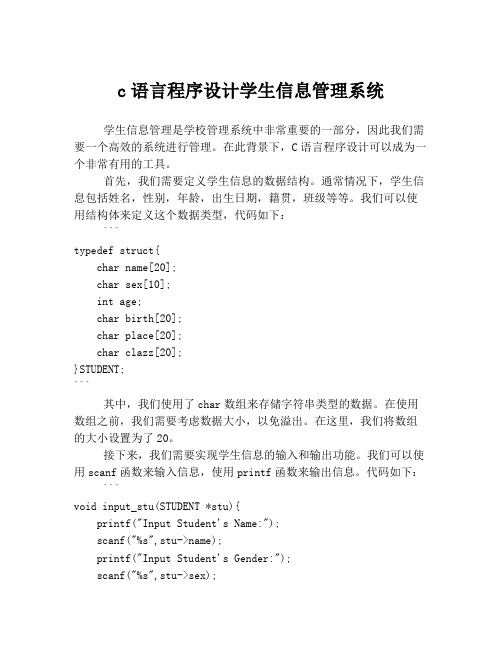
c语言程序设计学生信息管理系统学生信息管理是学校管理系统中非常重要的一部分,因此我们需要一个高效的系统进行管理。
在此背景下,C语言程序设计可以成为一个非常有用的工具。
首先,我们需要定义学生信息的数据结构。
通常情况下,学生信息包括姓名,性别,年龄,出生日期,籍贯,班级等等。
我们可以使用结构体来定义这个数据类型,代码如下:```typedef struct{char name[20];char sex[10];int age;char birth[20];char place[20];char clazz[20];}STUDENT;```其中,我们使用了char数组来存储字符串类型的数据。
在使用数组之前,我们需要考虑数据大小,以免溢出。
在这里,我们将数组的大小设置为了20。
接下来,我们需要实现学生信息的输入和输出功能。
我们可以使用scanf函数来输入信息,使用printf函数来输出信息。
代码如下:```void input_stu(STUDENT *stu){printf("Input Student's Name:");scanf("%s",stu->name);printf("Input Student's Gender:");scanf("%s",stu->sex);printf("Input Student's Age:");scanf("%d",&stu->age);printf("Input Student's Birth:");scanf("%s",stu->birth);printf("Input Student's Place:");scanf("%s",stu->place);printf("Input Student's Class:");scanf("%s",stu->clazz);}void output_stu(STUDENT *stu){printf("Name:%s\n",stu->name);printf("Gender:%s\n",stu->sex);printf("Age:%d\n",stu->age);printf("Birth:%s\n",stu->birth);printf("Place:%s\n",stu->place);printf("Class:%s\n",stu->clazz);}```注意,在使用scanf函数输入字符串类型的数据时,应该使用%s 格式控制符。
学生信息管理系统c语言

学生信息管理系统c语言1. 简介学生信息管理系统是一个基于C语言开发的程序,用于管理学校或教育机构中的学生信息。
该系统可以实现学生信息的录入、查询、修改和删除等功能,并且支持数据的持久化存储。
本文档将介绍学生信息管理系统的功能、使用方法以及具体实现细节。
2. 功能学生信息管理系统具有以下主要功能:2.1 录入信息该系统可以通过用户输入的方式录入学生的基本信息,包括学号、姓名、性别、年龄、班级等。
其中,学号是唯一的标识符,用于区分不同的学生。
2.2 查询信息用户可以通过学号或姓名查询特定学生的信息。
系统会根据用户提供的查询条件,在学生信息数据库中查找匹配的学生记录,并将结果显示给用户。
2.3 修改信息用户可以修改已录入学生的信息,包括姓名、性别、年龄、班级等。
系统会根据用户提供的学号,在学生信息数据库中找到相应的学生记录,并将其修改为用户输入的新信息。
2.4 删除信息用户可以根据学号删除某个学生的信息。
系统会在学生信息数据库中找到匹配的学生记录,并将其从数据库中删除。
3. 使用方法学生信息管理系统的使用方法如下:3.1 编译和运行程序首先,需要将C源代码编译成可执行文件。
在命令行中输入以下命令:gcc main.c -o student_system然后,运行编译得到的可执行文件:./student_system3.2 界面操作系统运行后,会显示一个简单的命令行界面,用户可以通过键盘输入指定的命令来执行相应的操作。
以下是系统支持的命令列表:•add:录入学生信息•search:查询学生信息•modify:修改学生信息•delete:删除学生信息•exit:退出系统用户可以根据提示输入相应的命令,并按下回车键确认。
系统会根据用户输入执行相应的操作,然后返回到命令行界面,等待下一次输入。
4. 实现细节学生信息管理系统的实现细节如下:4.1 数据存储系统使用文件来持久化存储学生信息。
每个学生的信息被保存在一个独立的记录中,记录之间使用换行符进行分隔。
学生管理系统c语言简单版
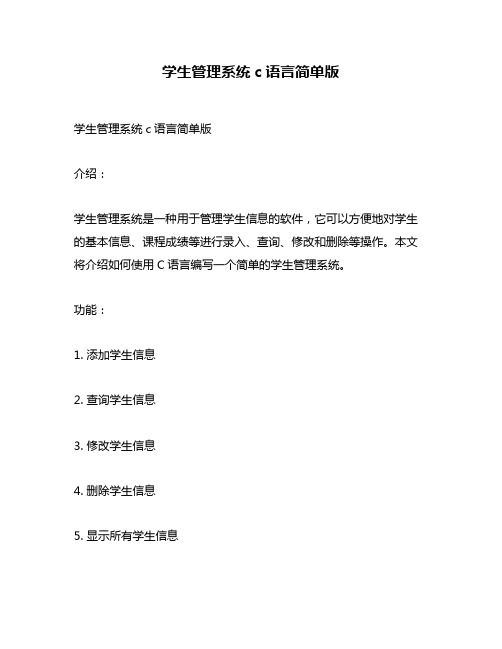
学生管理系统c语言简单版学生管理系统c语言简单版介绍:学生管理系统是一种用于管理学生信息的软件,它可以方便地对学生的基本信息、课程成绩等进行录入、查询、修改和删除等操作。
本文将介绍如何使用C语言编写一个简单的学生管理系统。
功能:1. 添加学生信息2. 查询学生信息3. 修改学生信息4. 删除学生信息5. 显示所有学生信息实现方法:1. 添加学生信息添加学生信息需要输入以下内容:姓名、性别、年龄、班级和电话号码。
我们可以定义一个结构体来存储这些信息,代码如下:```struct Student {char name[20];char sex[10];int age;char class[20];char phone[20];};```然后定义一个数组来存储多个学生的信息:```struct Student students[100];int count = 0; // 学生数量```接下来,我们可以编写一个函数来添加新的学生信息:```void addStudent() {struct Student student;printf("请输入姓名:");scanf("%s", );printf("请输入性别:");scanf("%s", student.sex);printf("请输入年龄:");scanf("%d", &student.age);printf("请输入班级:");scanf("%s", student.class);printf("请输入电话号码:");scanf("%s", student.phone);students[count++] = student; // 将新的学生信息存储到数组中 printf("添加成功!\n");}```2. 查询学生信息查询学生信息可以按照姓名或电话号码进行查询。
学生信息管理系统(c语言版)

return s1;
}
/*按照数据结构成绩排序*/
void DSpaixu(StuList s1)
{
int i,j;
StuList s;
s.length=1;
for(i=0;i<s1.length-1;i++)
{
for(j=s1.length-1;j>i;j--)
scanf("%f",&s1.data[i].Java);
scanf("%f",&s1.data[i].Vc);
printf("\n");
for(k=0;k<i;k++)
{
if(s1.data[k].id==s1.data[i].id)
{
printf("学号重复了,请重新输入");
}
/*按照学号查找学生信息*/
void xuehaozhao(StuList s1)
{
StuList s;
int x,i=0,k=0;
s.length=0;
printf("请输入您要查找的学生的学号:");
scanf("%d",&x);
for(int j=0;j<s1.length;j++)
scanf("%f",&s1.data[i].DS);
scanf("%f",&s1.data[i].Java);
scanf("%f",&s1.data[i].Vc);
C语言学生信息管理系统(源代码)
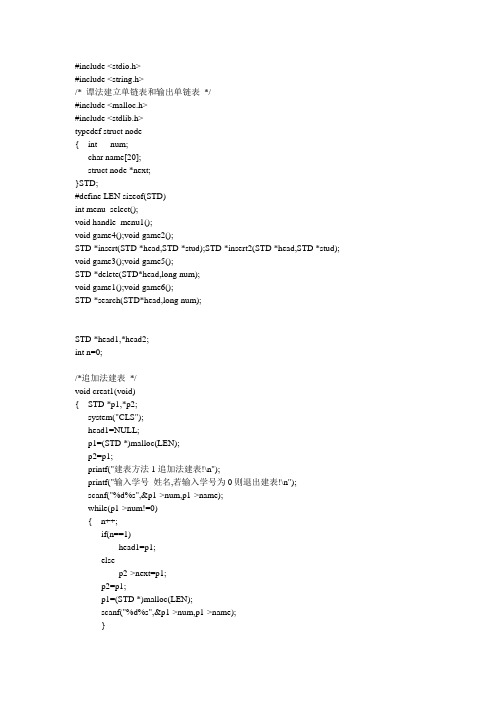
#include <stdio.h>#include <string.h>/* 谭法建立单链表和输出单链表*/#include <malloc.h>#include <stdlib.h>typedef struct node{ int num;char name[20];struct node *next;}STD;#define LEN sizeof(STD)int menu_select();void handle_menu1();void game4();void game2();STD *insert(STD *head,STD *stud);STD *insert2(STD *head,STD *stud); void game3();void game5();STD *delete(STD*head,long num);void game1();void game6();STD *search(STD*head,long num);STD *head1,*head2;int n=0;/*追加法建表*/void creat1(void){ STD *p1,*p2;system("CLS");head1=NULL;p1=(STD *)malloc(LEN);p2=p1;printf("建表方法1追加法建表!\n");printf("输入学号姓名,若输入学号为0则退出建表!\n");scanf("%d%s",&p1->num,p1->name);while(p1->num!=0){ n++;if(n==1)head1=p1;elsep2->next=p1;p2=p1;p1=(STD *)malloc(LEN);scanf("%d%s",&p1->num,p1->name);}free(p1); p2->next=NULL;scanf("%*c");printf("按回车键继续!\n");getchar();system("CLS");return ;}/* 插入法建表*/void creat2(void){ STD *p1;head2=NULL;system("CLS");p1=(STD *)malloc(LEN);printf("建表方法2插入法建表!\n");printf("输入学号姓名(输入学号以0结束)!\n");scanf("%d%s",&p1->num,p1->name);while(p1->num!=0){ p1->next=head2;head2=p1;p1=(STD *)malloc(LEN);scanf("%d%s",&p1->num,p1->name);}scanf("%*c");printf("按回车键继续!\n");getchar();system("CLS");free(p1);return ;}/* 链表输出*/void print(STD *head){ STD *p;p=head;while (p!=NULL){ printf("学号%3d 姓名%s\n",p->num,p->name); p=p->next;} printf("链表为空!\n");scanf("%*c");printf("按回车键继续!\n");getchar();system("CLS");}/*-------------------------------------------------*//*--------------表1插入结点函数-------*//*-------------------------------------------------*/void game4(){char ch;STD *stud,*head=head1;system("CLS");printf("\t仅限在表1中插入!\n");printf("\t输入要插入结点的学号和姓名(以空格隔开):");stud=(STD *)malloc(LEN);scanf("%*c");scanf("%d%s",&stud->num,&stud->name);if(stud->num<0){ printf("\t输入的学号不能为负数,请重新选择插入!\n");return;}while(stud->num>=0){head1=insert(head,stud);printf("\t继续插入新结点吗?(y/n):");ch=getchar();if(ch=='y'||ch=='Y'){ printf("\t输入新结点学号姓名:(输入n结束插入)");stud=(STD *)malloc(LEN);scanf("%*c");scanf("%d%s",&stud->num,&stud->name);}elsebreak;}printf("\t输入回车键返回!\n");getchar();system("CLS");printf("\n");}/*-------------------------------------------------*//*--------------表2插入结点函数-------*//*-------------------------------------------------*/void game2(){char ch;STD *stud,*head=head2;system("CLS");printf("\t仅限在表2中插入!\n");printf("\t输入要插入结点的学号和姓名(以空格隔开):");stud=(STD *)malloc(LEN);scanf("%*c");scanf("%d%s",&stud->num,&stud->name);if(stud->num<0){ printf("\t输入的学号不能为负数,请重新选择插入!\n");return;}while(stud->num>=0){head2=insert2(head,stud);printf("\t继续插入新结点吗?(y/n):");ch=getchar();if(ch=='y'||ch=='Y'){ printf("\t输入新结点学号姓名:(输入n结束插入)");stud=(STD *)malloc(LEN);scanf("%*c");scanf("%d%s",&stud->num,&stud->name);}elsebreak;}printf("\t输入回车键返回!\n");getchar();system("CLS");printf("\n");}/**************************************//* 插入模块*//**************************************/STD *insert(STD *head,STD *stud){STD *p0,*p1,*p2;p1=head;p0=stud;if(head==NULL){head=p0;p0->next=NULL;}else{while((p0->num>p1->num)&&(p1->next!=NULL)){p2=p1; p1=p1->next;}if(p0->num<=p1->num){if(head==p1)head=p0;else p2->next=p0;p0->next=p1;}else{p1->next=p0;p0->next=NULL;}}n=n+1;printf("按回车键继续!\n");getchar();system("CLS");return (head);}/**************************************//* 插入模块2 *//**************************************/STD *insert2(STD *head,STD *stud){STD *p0,*p1,*p2;p1=head;p0=stud;if(head==NULL){head=p0;p0->next=NULL;}else{while((p0->num<p1->num)&&(p1->next!=NULL)){p2=p1; p1=p1->next;}if(p0->num>=p1->num){if(head==p1)head=p0;else p2->next=p0;p0->next=p1;}else{p1->next=p0;p0->next=NULL;}}n=n+1;printf("按回车键继续!\n");getchar();system("CLS");return (head);}/*-------------------------------------------------*//*--------------表1删除学生信息-------*//*-------------------------------------------------*/void game3(){ int num;char ch;STD *head=head1;printf("\t仅限在表1中删除!\n");printf("\t输入要删除的学号:");scanf("%*c");scanf("%ld",&num);if(num<0){ printf("\t输入的学号不能为负数,请重新选择删除!\n");return;}while(num>=0){head=head1;head1=delete(head,num);printf("\t继续删除吗?(y/n):");scanf("%*c");ch=getchar();if(ch=='y'||ch=='Y'){ printf("\t输入要删除的学号:(输入n结束删除!)");scanf("%*c");scanf("%ld",&num);}elsebreak;}printf("\t输入回车键返回!\n");getchar();system("CLS");printf("\n");}/*-------------------------------------------------*//*--------------表2删除学生信息-------*//*-------------------------------------------------*/void game5(){ int num;char ch;STD *head=head2;printf("\t仅限在表2中删除!\n");printf("\t输入要删除的学号:");scanf("%*c");scanf("%ld",&num);if(num<0){ printf("\t输入的学号不能为负数,请重新选择删除!\n");return;}while(num>=0){head=head2;head2=delete(head,num);printf("\t继续删除吗?(y/n):");scanf("%*c");ch=getchar();if(ch=='y'||ch=='Y'){ printf("\t输入要删除的学号:(输入n结束删除!)");scanf("%*c");scanf("%ld",&num);}elsebreak;}printf("\t输入回车键返回!\n");getchar();system("CLS");printf("\n");}/**************************************//* 删除模块*//**************************************/STD *delete(STD*head,long num){STD *p1,*p2;if (head==NULL) {printf("\n没有找到该学号!\n");return head;} p1=head;while(num!=p1->num&&p1->next!=NULL){p2=p1;p1=p1->next;}if(num==p1->num){if(p1==head)head=p1->next;else p2->next=p1->next;printf("\n删除:%ld\n",num);n=n-1;}elseprintf("\n 没有发现此项! \n",num);system("CLS");return(head);}/*-------------------------------------------------*//*--------------表1查找学生信息-------*//*-------------------------------------------------*/void game1(){ int num;char ch;STD *head=head1;printf("\t仅限在表1中查找!\n");printf("\t输入要查找的学号:");scanf("%ld",&num);if(num<0){ printf("\t输入的学号不能为负数,请重新查找!\n");return;}while(num>=0){head=head1;head1=search(head,num);printf("\t继续查找吗?(y/n):");scanf("%d",&num);ch=getchar();if(ch=='y'||ch=='Y'){ printf("\t输入要查找的学号:(输入n结束查找)");scanf("%ld",&num);}elsebreak;}printf("\t输入回车键返回!\n");getchar();system("CLS");printf("\n");}/*-------------------------------------------------*//*--------------表2查找学生信息-------*//*-------------------------------------------------*/void game6(){ int num;char ch;STD *head=head2;printf("\t仅限在表2中查找!\n");printf("\t输入要查找的学号:");scanf("%ld",&num);if(num<0){ printf("\t输入的学号不能为负数,请重新查找!\n");return;}while(num>=0){head=head2;head2=search(head,num);printf("\t继续查找吗?(y/n):");scanf("%d",&num);ch=getchar();if(ch=='y'||ch=='Y'){ printf("\t输入要查找的学号:(输入n结束查找)");scanf("%ld",&num);}elsebreak;}printf("\t输入回车键返回!\n");getchar();system("CLS");printf("\n");}/**************************************//* 查找模块*//**************************************/STD *search(STD*head,long num){STD *p1,*p2;if (head==NULL) {printf("\n没有找到该学号!\n");return head;} p1=head;while(num!=p1->num&&p1->next!=NULL){p2=p1;p1=p1->next;}if(num==p1->num)printf("\n%d %s\n",p1->num,p1->name);elseprintf("\n 没有发现此项! \n",num);return(head);}/* void main(){printf(" *** 运行结果***\n");printf("输入学号姓名(输入学号以0结束)!\n");creat1();print(head1);creat2();print(head2);} *///******************************//* select.cpp:主程序文件*//******************************void main(){handle_menu1();}//*****************************//* 菜单处理函数*//*****************************void handle_menu1(){int h;for(;;){h=menu_select();switch(h){ case 1:/* printf("\t进入追加法建表\n"); */creat1();break; /* 调用功能模块*/case 2:/* printf("\t进入插入法建表\n"); */creat2();break;case 3:/* printf("\t输出追加法链表的结果\n"); */print(head1);break;case 4:/* printf("\t输出插入法链表的结果\n"); */print(head2);break;case 5:/* printf("\t链表1插入\n"); */game4();break;case 6:/* printf("\t链表2插入\n"); */game2();break;case 7:/* printf("\t链表1删除\n"); */game3();break;case 8:/* printf("\t链表2删除\n"); */game5();break;case 9:/* printf("\t链表1查找\n"); */game1();break;case 10:/* printf("\t链表2查找\n"); */game6();break;case 11:printf("\t再见,欢迎再次使用本系统!谢谢!\n");return;}}}//**********************************//* 菜单选择函数: int menu_select()*//**********************************int menu_select(){int cn;for(;;){printf("\ \n");printf("\t1.追加法建表\n");printf("\ \n");printf("\t2.插入法建表\n");printf("\ \n");printf("\t3.输出链表1\n");printf("\ \n");printf("\t4.输出链表2\n");printf("\ \n");printf("\t5.插入链表1 \n");printf("\ \n");printf("\t6.插入链表2 \n");printf("\ \n");printf("\t7.删除链表1 \n");printf("\ \n");printf("\t8.删除链表2 \n");printf("\ \n");printf("\t9.查找链表1 \n");printf("\ \n");printf("\t10.查找链表2 \n");printf("\ \n");printf("\t11.退出系统\n");printf("\ \n");printf("\t请您选择1-11:");scanf("%d",&cn);//gets(s);//cn=atoi(s);if(cn<1||cn>11)printf("\n\t输入错误,重选1-11:");elsebreak;}return cn;}。
C语言学生信息管理系统(完整版)

#define PRINT0 printf("name:%s\nsex:%s\nage:%d\nID_card:%d\naddress:%s\n",st[i].name,st[i].sex,st[i].age,st[i] .ID_card,st[i].addr)#define PRINT1 printf("prefession:%s\nstudent_number:%d\n*****score*****\nwuli:%d\n",st[i].prefession,st[i] .student_number,st[i].score.wuli)#define PRINT2 printf("gaoshu:%d\nyingyu:%d\ntiyu:%d\naverage: %d\n",st[i].score.gaoshu,st[i].score.yingyu,st[i ].score.tiyu,st[i].score.aver)#define print1 printf("________________________________")#define N 2#include "string.h"#include "stdio.h"int sum=0;struct score{int wuli;int gaoshu;int yingyu;int tiyu;int aver;};struct message{ char name[10];int age;char sex[5];int ID_card;char addr[30];char prefession[30];int student_number;struct score score;}st[100];/*************write message*************/write_message(){ int flag;char chioce;do{system("cls");flag=2; sum++;printf("_______________________________");printf("please input student's message:\n");printf("\n");print1;printf("%dth student message:",sum);print1;printf("\nname:");scanf("%s",st[sum].name);printf("\nsex:");scanf("%s",st[sum].sex);printf("\nage:");scanf("%d",&st[sum].age);printf("\nID_card:");scanf("%d",&st[sum].ID_card);printf("\naddress:");scanf("%s",st[sum].addr);printf("\nprefession:");scanf("%s",st[sum].prefession);printf("\nschool number:");scanf("%d",&st[sum].student_number);printf("\n*****score******\n");printf("wuli:");scanf("%d",&st[sum].score.wuli);printf("\ngaoshu:");scanf("%d",&st[sum].score.gaoshu);printf("\nyingyu:");scanf("%d",&st[sum].score.yingyu);printf("\ntiyu:");scanf("%d",&st[sum].score.tiyu);printf("\naverage:");scanf("%d",&st[sum].score.aver);do{printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");getchar();scanf("%c",&chioce);printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}else if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);save_message();}/*************save message****************/save_message(){ FILE *fp;int i;if((fp=fopen("student.txt","wb"))==NULL){printf("read error \n");printf("press any key back to menu\n");getch();exit(1);}for(i=0;i<sum;i++)if(fwrite(&st[i],sizeof(struct message),1,fp)!=1){printf("write error\n");fclose(fp);}fclose(fp);printf("\n********___OK!___**********\n___press any key back___");sum=i;bioskey(0);}/***************add message*****************/add_message(){int i,j,flag; char chioce;i=0;j=sum-1;flag=0;do{ system("cls");i++; j++;print1;printf("add %dth student's meaasge\n",i);print1;printf("\nname:");scanf("%s",st[j].name);printf("\nsex:");scanf("%s",st[j].sex);printf("\nage:");scanf("%d",&st[j].age);printf("\nID_card:");scanf("%d",&st[j].ID_card);printf("\naddress:");scanf("%s",st[j].addr);printf("\nprefession:");scanf("%s",st[j].prefession);printf("\nstudent_number:");scanf("%d",&st[j].student_number);printf("\n*****score******\n");printf("wuli:");scanf("%d",&st[j].score.wuli);printf("\ngaoshu:");scanf("%d",&st[j].score.gaoshu);printf("\nyingyu:");scanf("%d",&st[j].score.yingyu);printf("\ntiyu:");scanf("%d",&st[j].score.tiyu);printf("\naverage:");scanf("%d",&st[j].score.aver);printf("\n\nweather add %dth student's message: \n",i+1);do{printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");getchar();scanf("%c",&chioce);printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}else if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;}while(1);}while(flag==1);sum=j+1;save_message();}/**********inqiure message******/inqiure_message(){int chioce;do{system("cls");printf("**********choose 0-3**********\n\n\n");printf(" 1:name inquire\n\n\n");printf(" 2:IDcard inqiure\n\n\n");printf(" 3:student_number\n \n\n");printf(" 0:back menu\n\n\n");scanf("%d",&chioce);switch(chioce){case 1: name_inqiure();break;case 2: ID_card_inqiure();break;case 3: grade_inqiure();break;case 0:break;}}while(chioce!=0);}/**********name inqiure*********/name_inqiure(){char NAME[30];int i; int flag,k;char chioce;do{ system("cls");k=0;printf("please input the message you inqiure");printf("\nname:");scanf("%s",NAME);getchar();printf("\n");for(i=0;i<sum;i++){if(strcmp(st[i].name,NAME)==0){PRINT0;PRINT1;PRINT2;k=1;}}if(k==0) printf("without message you inqiure\n");do{ printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");scanf("%c",&chioce); getchar();printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);printf("press any key return to menu");bioskey(0);}/**********IDcard inqiure*********/ID_card_inqiure(){int card;int i; int flag,k;char chioce;do{ clrscr(); k=0;printf("please input the message you inqiure");printf("\nIDcard:");scanf("%d",&card);getchar();printf("\n");for(i=0;i<sum;i++){if(st[i].ID_card==card){PRINT0;PRINT1;PRINT2;k=1;}}if(k==0) printf("without message you inqiure\n");do{ printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");scanf("%c",&chioce); getchar();printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);printf("press any key return to menu");bioskey(0);}/*********grade inqiure***********/grade_inqiure(){int GRADE;int i; int flag,k;char chioce;do{ system("cls");k=0;printf("please input the message you inqiure");printf("\nstudent_number:");scanf("%d",&GRADE);getchar();printf("\n");for(i=0;i<sum;i++){if(st[i].student_number==GRADE){PRINT0;PRINT1;PRINT2;k=1;}}if(k==0) printf("without message you inqiure\n");do{ printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");scanf("%c",&chioce); getchar();printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);printf("press any key return to menu");bioskey(0);}/*********change message**********/change_message(){char pre[30],chioce;int i,gra,flag,num,s;s=0;do{system("cls");printf("please input message you want to change\n ");printf("student_number:");scanf("%d",&gra); getchar();for(i=0;i<sum;i++){if(st[i].student_number==gra){PRINT0;PRINT1;PRINT2;printf("\n********input message you want to change********\n");printf("0:name***1:sex***2:age***3:ID_card***4:address***\n5:prefession***6:wuli***7:stud ent_number***8\n:gaoshu***9:yingyu***10:tiyu***11:average***________\n");printf("choose 0-11\n");scanf("%d",&num); getchar();switch(num){case 0: printf("input the name changed\n");scanf("%s",st[i].name); getchar(); break;case 1: printf("input the sex changed\n");scanf("%s",st[i].sex); getchar(); break;case 2: printf("input the age changed\n");scanf("%d",&st[i].age); getchar(); break;case 3: printf("input the ID_card changed\n");scanf("%d",&st[i].ID_card); getchar(); break;case 4: printf("input the address changed\n");scanf("%s",st[i].addr); getchar(); break;case 5: printf("input the prefession changed\n");scanf("%s",st[i].prefession); getchar(); break;case 6: printf("input the wuli_score changed\n");scanf("%d",&st[i].score.wuli); getchar(); break;case7: printf("input the student_number changed\n");scanf("%d",&st[i].student_number); getchar(); break;case 8: printf("input the gaoshu_score changed\n");scanf("%d",&st[i].score.gaoshu); getchar(); break;case 9: printf("input the yingyu_score changed\n");scanf("%d",&st[i].score.yingyu); getchar(); break;case 10: printf("input the tiyu_score changed\n");scanf("%d",&st[i].score.tiyu); getchar(); break;case 11: printf("input the average_score changed\n");scanf("%d",&st[i].score.aver); getchar(); break;default: printf("input error\n"); break;}printf("\n*********the changed message*********\n\n");PRINT0;PRINT1;PRINT2;s=1;}}if(s!=1) printf("without message you want to change\n");do{ printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");scanf("%c",&chioce); getchar();printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);save_message();}/*********delete message**********/delete_message(){ int GRADE;int i,j,flag1,flag;char chioce1,chioce2;flag1=3;flag=3;do{ system("cls");printf("please input student's student_number you want to delete\n");printf("student_number:");scanf("%d",&GRADE); getchar();for(i=0;i<sum;i++)if(st[i].student_number==GRADE){do{PRINT0;PRINT1;PRINT2;printf("\n******************************\n_________________ _________________\n");printf("press y/Y deleted:\npress n/N cancel:\n");scanf("%c",&chioce1);getchar();system("cls");if(chioce1=='y'||chioce1=='Y') flag1=1;else if(chioce1=='n'||chioce1=='N') return;else {printf("***input error***\n___press any ker return___\n"); bioskey(0);}}while(flag1!=1);for(j=i;j<sum;j++){ st[j]=st[j+1];flag=2;printf("message was deleted\n");sum-=1; }}if(flag!=2) printf("without message you want to delete\n");do{printf("press y/Y continue:\npress n/N stop:\n");scanf("%c",&chioce2);printf("****************************\n");if(chioce2=='y'||chioce2=='Y') {flag=1; break;}else if(chioce2=='n'||chioce2=='N') {flag=0; break;}else {system("cls");printf("input error\n");}print1;printf("\n");}while(1);}while(flag==1);save_message();}/************insert message*************/insert_message(){ int chioce,flag,i; flag=2;do{system("cls");printf("please input the number of people you insert\n");scanf("%d",&chioce);if(chioce>=sum){printf("xin xi pai zai zui hou ");chioce=sum;}for(i=sum;i>chioce;i--) st[i]=st[i-1];printf("\nplease input message you insert");printf("\nname:");scanf("%s",st[i].name);printf("\nsex:");scanf("%s",st[i].sex);printf("\nage:");scanf("%d",&st[i].age);printf("\nID_card:");scanf("%d",&st[i].ID_card);printf("\naddress:");scanf("%s",st[i].addr);printf("\nprefession:");scanf("%s",st[i].prefession);printf("\ngrade:");scanf("%d",&st[i].student_number);printf("\n*****score******\n");printf("wuli:");scanf("%d",&st[i].score.wuli);printf("\ngaoshu:");scanf("%d",&st[i].score.gaoshu);printf("\nyingyu:");scanf("%d",&st[i].score.yingyu);printf("\ntiyu:");scanf("%d",&st[i].score.tiyu);printf("\naverage:");scanf("%d",&st[i].score.aver);sum+=1;do{printf("****************************\n");printf("press y/Y continue:\npress n/N stop:\n");getchar();scanf("%c",&chioce);printf("****************************\n");if(chioce=='y'||chioce=='Y') {flag=1; break;}else if(chioce=='n'||chioce=='N') {flag=0; break;}else printf("input error\n");print1;printf("\n");}while(1);}while(flag==1);save_message();}/*********school_number_order***********/grade_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].student_number>st[j].student_number){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("grade order from min to max\n");for(i=0;i<sum;i++){ print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);bioskey(0);}/***********wuli score order***************/wuli_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].score.wuli>st[j].score.wuli){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("wuli score order from min to max\n");for(i=0;i<sum;i++){print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);print1;bioskey(0);}/***********gaoshu score order***************/gaoshu_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].score.gaoshu>st[j].score.gaoshu){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("gaoshu score order from min to max\n");for(i=0;i<sum;i++){print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);print1;bioskey(0);}/***********yingyu score order***************/yingyu_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].score.yingyu>st[j].score.yingyu){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("yingyu score order from min to max\n");for(i=0;i<sum;i++){print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);print1;bioskey(0);}/***********tiyu score order***************/tiyu_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].score.tiyu>st[j].score.tiyu){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("yitu order from min to max\n");for(i=0;i<sum;i++){print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);print1;bioskey(0);}/***********average score order***************/average_order(){int i,j;system("cls");if(sum==0) {printf("**********no message**********\n____press any key return____");getch();return;}for(i=0;i<sum;i++)for(j=i+1;j<sum;j++)if(st[i].score.wuli>st[j].score.wuli){ st[sum+1]=st[i];st[i]=st[j];st[j]=st[sum+1];}printf("average score order from min to max\n");for(i=0;i<sum;i++){print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;getch();system("cls");}print1;printf("\ntotle num :%d\npress any key return to menu\n",sum);print1;bioskey(0);}/********order message****************/order_message(){int chioce;do{system("cls");printf("**********choose 0-6**********\n\n");printf(" 1:grade order\n\n");printf(" 2:wuli score order\n\n");printf(" 3:gaoshu score order\n \n");printf(" 4:ying yu score order\n\n");printf(" 5:tiyu score order\n\n");printf(" 6:average score order\n\n");printf(" 0:back menu\n\n");scanf("%d",&chioce);switch(chioce){case 1: grade_order();break;case 2: wuli_order();break;case 3: gaoshu_order();break;case 4: yingyu_order();break;case 5: tiyu_order();break;case 6: average_order();break;case 0:break;}}while(chioce!=0);}/**********answer secretory***********/mima_message(){int flag;char answer[10];char secret[10]="abcd";flag=2;do{system("cls");printf("\n______________________mi ma wei 'abcd'________________\n\n");printf("______________________qing shu ru mi ma:");scanf("%s",answer);getchar();if(strcmp(secret,answer)==0){flag=1;printf("\n\n =====throngh=====\n\n\n");printf("____________________press any key into next:\n");bioskey(0);}else{flag=0;printf("______________________input error:\n");printf("______________________press any key to return:\n");bioskey(0);}}while(flag!=1);}/*********read message****************/read_message(){int i;system("cls");if(sum<=0){ printf("without message\n");getch();return;}for(i=0;i<sum;i++){system("cls");print1;printf("\n%dth student's mssage\n",i+1);print1;printf("\n");PRINT0;PRINT1;PRINT2;printf("\n********press any key -show the next one********\n ");getch();}printf("________________totle num :%d________________\n",sum); printf("_____________press any key return______________\n"); bioskey(0);}/*************************************/main(){int chioce,flag;mima_message();do{system("cls");chioce=9;printf("**********************************************\n");printf("****welcome to system of managing students****\n");printf("**********************************************\n\n");printf("-------------------choose 0-8-----------------\n\n");printf(" 1:write message\n\n");printf(" 2:add message\n\n");printf(" 3:inqiure name\n\n");printf(" 4:change message\n\n");printf(" 5:insert message\n\n");printf(" 6:order message\n\n");printf(" 7:delete message\n\n");printf(" 8:read messaeg\n\n");printf(" 0:***exit***\n\n");scanf("%d",&chioce);getchar();switch(chioce){case 1: write_message();break;case 2: add_message();break;case 3: inqiure_message();break;case 4: change_message();break;case 5: insert_message();break;case 6: order_message();break;case 7: delete_message();break;case 8: read_message();break;case 0: printf("___sure press y/Y:___\n\n___no sure press n/N:___");scanf("%c",&chioce);getchar();if(chioce=='y'||chioce=='Y') flag=0;else flag=1;break;default : printf("\n ___input error___\n\n");printf("***press any key to go on***\n");getch();break;}}while(flag!=0);save_message();system("cls");printf("\n\n___message was saved___\n\n\n*****file name is student.txt*****\n");bioskey(0);}。
学生管理系统C语言附代码

if(i<1||i>L.length+1)
return 0;
for(k=i;k<=L.length;k++)
{
L.elem[k-1]=L.elem[k]; //将后面元素依次前移}
L.length--;
return 1;
}
四、实验体会
1、存在问题
1.
起初未将变量名启用数组的形式,通过调试发现姓名无法储存,学号只能储存一位数字,深刻体会存储字节的问题。
2.
关于结构体的嵌套,如何引用其中变量问题有了深刻体会。
3.
观察到老师给的代码中sqlist &L,而我均未使用&引用符号,依然可以运行。
于是查找资料编写
此时的Head(地址)的值与L相同!
若将引用符号&删去,则L不将改变Head的值,故&有种将传进去的参数“带出来”的作用。
于是发现即便插入了数据,再次输出时未显示插入的数据,于是进行改进加上&
果然插入的信息可以输出。
2、心得体会
1.复习了switch case 以及strcmp比较函数的用法;
2.学会增删操作中元素前后移的实现;
3.这次实验让我真正的明白了sqList &L的使用:在函数调用中引用变量一定要初始化才可以使用。
要返回到main里面进行操作、改变的顺序表需要带上&,如果只在对应函数操作的顺序表则不用。
c语言-学生信息管理系统(完整版)
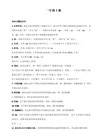
}
count=fwrite(stu,sizeof(Student),current,fs);
fclose(fs);
if(count!=current)
{
printf("保存失败\n");
return 1;
case'4':delete();break;
case'5':list();break;
case'6':save();break;
case'7':load();break;
case'0':if(stu!=NULL)
#include<string.h>
#include<malloc.h>
#include"Student.h"
#include"function.h"
Student*stu=NULL;
int current=0;
int total=0;
int main()
{
char choice;
printf("地址:%s\n",stu[i].address);
return 0;
}
}
printf("该学生不存在\n");
return 1;
}
int update()
{
char name[40];
printf(" 7.加载学生信息\n");
printf(" 0.退出程序\n");
c语言下的学生管理系统(含密码加密和验证码)

case 1:stu_search(userNO);break;
case 2:modify(userNO);break;
case 3:printf("\t\t
退出成功! \n");
exit(0);break;
default:printf("\t\t
你所选择的项目错误!重新选择! ");
student(userNO);break;
**********************************\n");
printf("\t\t
请输入学号: \n");
printf("\t\t");
fflush(stdin);
scanf("%d",&userNO);
欢迎进入学生登录界面
printf("\t\t===================================================================
请输入验证码: %s\n",code);
printf("\t\t===================================================================
======================\n");
if(strcmp(code,str)==0)
{
for(j=0;stu[j].no!=0;j++) {
if(stu[i].score.sum<stu[j].score.sum) {
count++; } } stu[i].ranking=count; count=1; } save(); }
学生信息管理系统(C语言实现)

#include <stdio.h>#include <stdlib.h>#include <string.h>struct student{int studentID;char studentName[20];char studentage[3];char studentsex[3];char studentbirth[10];//如199204char address[100];//填所在学院及班级char call[12];};struct student stud[100];int num=0;/*通过搜索学生学号查询结构体数组的下标*/ int student_searchby_ID(int ID){int i;for(i=0;i<num;i++){if(stud[i].studentID==ID){return i;}}return -1;}/* 通过搜索学生姓名查询结构体数组的下标*/int student_searchby_NAME(char NAME[]){int i;for(i=0;i<num;i++){if(strcmp(stud[i].studentName,NAME)==0){return i;}}return -1;}/*按学号给学生排序*/void student_sortby_line(){int i,j;struct student tmp;for (i=0;i<num;i++){for (j=1;j<num-i;j++){if (stud[j-1].studentID>stud[j].studentID) {tmp=stud[j-1];stud[j-1]=stud[j];stud[j]=tmp;}}}}/*显示某个学生的信息*/void student_display(int i){printf("%-8s%-8s%-8s%-8s%-15s%-15s%-15s\n","学号","姓名","年龄","性别","出生年月","地址","电话");printf("---------------------------------------------------------------------------\n");printf("%4d%8s%8s%8s%10s%20s%15s\n",stud[i].studentID,stud[i].studentName ,stud[i].studentage,stud[i].studentsex,stud[i].studentbirth,stud[i].addres s,stud[i].call);}/*显示全体学生信息*/void student_display_all(){int i;printf("%-8s%-8s%-8s%-8s%-8s%-15s%-15s%-15s\n","序号","学号","姓名","年龄","性别","出生年月","地址","电话");printf("----------------------------------------------------------------------------\n");for (i=0;i<num;i++){printf("%4d%6d%8s%8s%8s%10s%20s%15s\n",i+1,stud[i].studentID,stud[i].stud entName,stud[i].studentage,stud[i].studentsex,stud[i].studentbirth,stud[i].addres s,stud[i].call);}}/*插入某个学生的信息*/void student_insert(){while(1){printf("请输入学号:");scanf("%d",&stud[num].studentID);printf("请输入姓名:");scanf("%s",&stud[num].studentName);printf("请输入年龄:");scanf("%s",&stud[num].studentage);printf("请输入性别:");scanf("%s",&stud[num].studentsex);printf("请输入出生年月:");scanf("%s",&stud[num].studentbirth); printf("请输入地址:");scanf("%s",&stud[num].address);printf("请输入电话:");scanf("%s",&stud[num].call);num++;printf("是否继续?(y/n):");getchar();if (getchar()=='n') break;}}/*修改某个学生信息*/void student_modify(){while(1){int id;int i;printf("请输入要修改的学生的学号:"); scanf("%d",&id);i=student_searchby_ID(id);if (i==-1){printf("该学生不存在!\n");}else{printf("你要修改的学生信息为:\n"); student_display(i);printf("-- 请输入新值--\n");printf("请输入学号:");scanf("%d",&stud[i].studentID);printf("请输入姓名:");scanf("%s",&stud[i].studentName);printf("请输入年龄:");scanf("%s",&stud[i].studentage); printf("请输入性别:");scanf("%s",&stud[i].studentsex); printf("请输入出生年月:");scanf("%s",&stud[i].studentbirth);printf("请输入地址:");scanf("%s",&stud[i].address);printf("请输入电话:");scanf("%s",&stud[i].call);}printf("是否继续?(y/n):");getchar();if (getchar()=='n') break;}}/*删除某个学生信息*/void student_delete(){//int j;while(1)int id;int i;printf("请输入要删除的学生的学号:");scanf("%d",&id);i=student_searchby_ID(id);if (i==-1) printf("学生不存在!\n");else{printf("你要删除的学生信息为:\n");student_display(i);printf("是否真的要删除?(y/n):");getchar();if (getchar()=='y'){for(;i<num-1;i++) stud[i]=stud[i+1]; num--;}}printf("是否继续?(y/n)");getchar();if (getchar()=='n') break;}}/*按学生姓名查询*/void student_selectby_NAME(){while(1)char name[20];int i;printf("请输入要查询的学生的姓名:");scanf("%s",&name);i=student_searchby_NAME(name);if (i==-1) printf("学生不存在!\n");else{printf("你要查询的学生信息为:\n"); student_display(i);}printf("是否继续?(y/n)");getchar();if (getchar()=='n') break;}}/*按学生学号查询*/void student_selectby_ID(){while(1){int ID;int i;printf("请输入要查询的学生的学号:");scanf("%d",&ID);i=student_searchby_ID(ID);if (i==-1) printf("该学生不存在!\n"); else{printf("你要查询的学生信息为:\n"); student_display(i);}printf("是否继续?(y/n)");getchar();if (getchar()=='n') break;}}/*将学生信息从文件读出*/void readfile(){FILE *fp;int i;if ((fp=fopen("data.txt","r"))==NULL){printf("不能打开文件!\n");return;}if (fread(&num,sizeof(int),1,fp)!=1) {num=-1;}else{for(i=0;i<num;i++){fread(&stud[i],sizeof(struct student),1,fp);}}fclose(fp);}/*将学生信息写入文件*/void writefile(){FILE *fp;int i;fp=fopen("data.txt","w");if (fwrite(&num,sizeof(int),1,fp)!=1){printf("写入文件错误!\n");}for (i=0;i<num;i++){if (fwrite(&stud[i],sizeof(struct student),1,fp)!=1) {printf("写入文件错误!\n");}fputs( "\n ",fp);}fclose(fp);}/*主程序*/void main(){int choice;readfile();while(1){/*主菜单*/printf("\n------ 学生信息管理系统------\n");printf("1. 增加学生记录\n");printf("2. 修改学生记录\n");printf("3. 删除学生记录\n");printf("4. 按姓名查询学生记录\n");printf("5. 按学号查询学生记录\n");printf("6. 导出全体学生信息表\n");printf("7. 导出全体学生信息表(按学号大小)\n");printf("8. 退出\n");printf("请选择(1-8):");scanf("%d",&choice);switch(choice){case 1: student_insert(); break;case 2: student_modify(); break;case 3: student_delete(); break;case 4: student_selectby_NAME(); break;case 5: student_selectby_ID(); break;case 6: student_display_all(); break;case 7: student_sortby_line();student_display_all(); break; case 8: exit(0); break;}writefile(); }}。
学生管理系统c语言
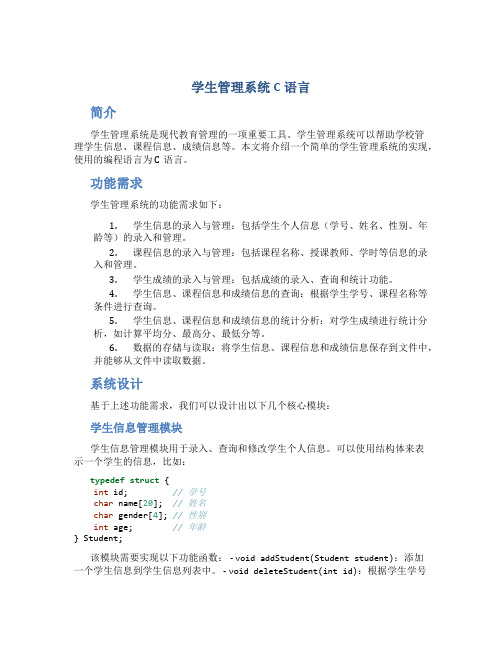
学生管理系统C语言简介学生管理系统是现代教育管理的一项重要工具。
学生管理系统可以帮助学校管理学生信息、课程信息、成绩信息等。
本文将介绍一个简单的学生管理系统的实现,使用的编程语言为C语言。
功能需求学生管理系统的功能需求如下:1.学生信息的录入与管理:包括学生个人信息(学号、姓名、性别、年龄等)的录入和管理。
2.课程信息的录入与管理:包括课程名称、授课教师、学时等信息的录入和管理。
3.学生成绩的录入与管理:包括成绩的录入、查询和统计功能。
4.学生信息、课程信息和成绩信息的查询:根据学生学号、课程名称等条件进行查询。
5.学生信息、课程信息和成绩信息的统计分析:对学生成绩进行统计分析,如计算平均分、最高分、最低分等。
6.数据的存储与读取:将学生信息、课程信息和成绩信息保存到文件中,并能够从文件中读取数据。
系统设计基于上述功能需求,我们可以设计出以下几个核心模块:学生信息管理模块学生信息管理模块用于录入、查询和修改学生个人信息。
可以使用结构体来表示一个学生的信息,比如:typedef struct {int id; // 学号char name[20]; // 姓名char gender[4]; // 性别int age; // 年龄} Student;该模块需要实现以下功能函数: - void addStudent(Student student):添加一个学生信息到学生信息列表中。
- void deleteStudent(int id):根据学生学号删除学生信息。
- void updateStudent(Student student):更新学生的个人信息。
- Student* searchStudent(int id):根据学生学号查询学生信息。
课程信息管理模块课程信息管理模块用于录入、查询和修改课程信息。
可以使用结构体来表示一个课程的信息,比如:typedef struct {char name[20]; // 课程名称char teacher[20]; // 授课教师int hours; // 学时} Course;该模块需要实现以下功能函数: - void addCourse(Course course):添加一个课程信息到课程信息列表中。
C语言 学生信息管理系统(完整版)
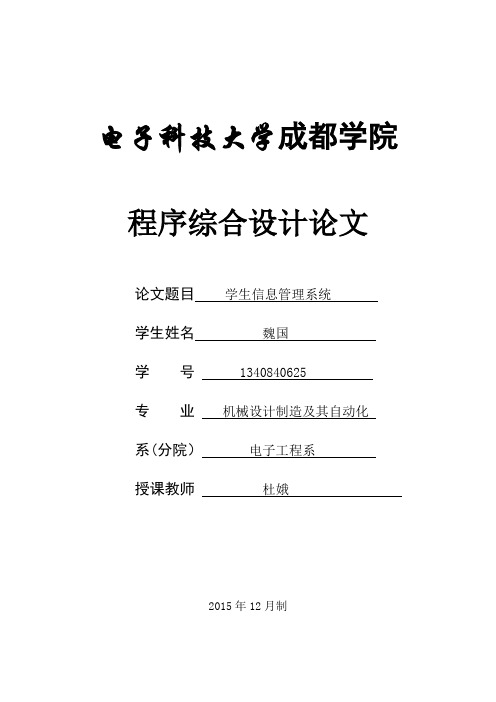
电子科技大学成都学院程序综合设计论文论文题目学生信息管理系统学生姓名魏国学号1340840625专业机械设计制造及其自动化系(分院)电子工程系授课教师杜娥2015年12月制摘要随着经济的发展,社会的进步,计算机越来越深入到我们日常的工作学习及生活中,成为我们日常生活不可或缺的辅助工具。
随着科学技术的不断提高,计算机科学日渐成熟,其强大的功能已成为人们深刻认识,它已为人们深刻认识,它已进入人类社会的各个领域并发挥着越来越重要的作用。
现在由于学校规模进一步扩大,学生人数逐渐上升,在学校的学生信息管理中,虽然已经存在许多学生信息管理系统,但由于学校之间的管理差异很信息的不同,各个学校的学生信息管理的要求不一致,这样我们需要根据具体学习的具体要求来开发学生信息管理系统以方便学生管理。
本系统主要对学生各种信息进行处理。
本系统采用C语言编写,设计从实用性出发,设计开发出一个操作简单且符合实际需要的学生信息管理系统。
本文设计出一个可以添加、修改、查询、删除、统计的学生信息管理系统;最后,通过测试分析,力求将学到的只是在学生信息管理系统的得到全面运用,并使系统在实际的操作中能按照设计的要求安全有效的正确运行。
学生信息管理系统是为了实现学校对学生信息管理的系统化、规范化和自动化,从而提高学校管理效率而设计的。
它完全取代了原来一直用人工管理的工作方式,避免了由于管理人员的工作疏忽以及管理质量问题所造成的各种错误,为及时、准确、高效的完成学生信息管理提供了强有力的工具和管理手段。
学生信息管理系统是一个中小型数据库管理系统,它界面美观、操作简单、安全性高,基本满足了学生信息管理的要求。
学生信息管理系统在运行阶段,效果好,数据准确性高,提高了工作效率,同时也实现了学生信息管理计算机化。
关键字:学生信息,管理系统,数据库,C语言编写第一章系统功能和组成模块1.1系统功能学生信息管理系统存放了每个学生的学号,姓名,性别,年龄,出生年月,家庭住址,政治面貌等信息的数据库。
学生信息管理系统(C语言)
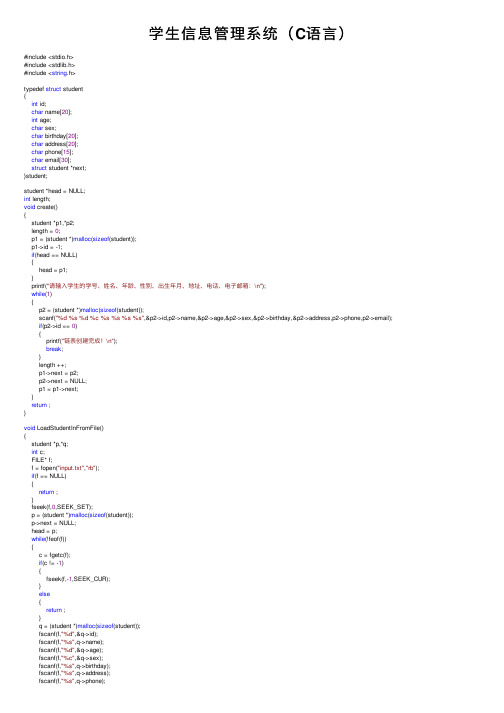
学⽣信息管理系统(C语⾔)#include <stdio.h>#include <stdlib.h>#include <string.h>typedef struct student{int id;char name[20];int age;char sex;char birthday[20];char address[20];char phone[15];char email[30];struct student *next;}student;student *head = NULL;int length;void create(){student *p1,*p2;length = 0;p1 = (student *)malloc(sizeof(student));p1->id = -1;if(head == NULL){head = p1;}printf("请输⼊学⽣的学号、姓名、年龄、性别、出⽣年⽉、地址、电话、电⼦邮箱:\n");while(1){p2 = (student *)malloc(sizeof(student));scanf("%d %s %d %c %s %s %s %s",&p2->id,p2->name,&p2->age,&p2->sex,&p2->birthday,&p2->address,p2->phone,p2->email);if(p2->id == 0){printf("链表创建完成!\n");break;}length ++;p1->next = p2;p2->next = NULL;p1 = p1->next;}return ;}void LoadStudentInFromFile(){student *p,*q;int c;FILE* f;f = fopen("input.txt","rb");if(f == NULL){return ;}fseek(f,0,SEEK_SET);p = (student *)malloc(sizeof(student));p->next = NULL;head = p;while(!feof(f)){c = fgetc(f);if(c != -1){fseek(f,-1,SEEK_CUR);}else{return ;}q = (student *)malloc(sizeof(student));fscanf(f,"%d",&q->id);fscanf(f,"%s",q->name);fscanf(f,"%d",&q->age);fscanf(f,"%c",&q->sex);fscanf(f,"%s",q->birthday);fscanf(f,"%s",q->address);fscanf(f,"%s",q->phone);fscanf(f,"%s",q->email);q->next = NULL;p->next = q;p = p->next;length ++;//链表长度}}void ModifyStudentInfo(){student *p = head->next;int num;printf("请输⼊要修改的学⽣的学号:");scanf("%d",&num);while(p != NULL){if(p->id == num){printf("修改前,学号为%d的学⽣信息如下:\n",num);printf("%d %s %d %c %s %s %s %s",p->id,p->name,p->age,p->sex,p->birthday,p->address,p->phone,p->email); printf("请输⼊学⽣的新电话:");getchar();gets(p->phone);printf("请输⼊学⽣的新地址:");gets(p->address);printf("修改后,学号为%d的学⽣信息如下:\n",num);printf("%d %s %d %c %s %s %s %s",&p->id,p->name,&p->age,p->sex,p->birthday,p->address,p->phone,p->email);return ;}p = p->next;}if(p == NULL){printf("该学号不存在!\n");return ;}}void display(){student *p = head->next;printf("链表中所有的学⽣信息如下:\n");while(p != NULL){printf("%d %s %d %c %s %s %s %s",p->id,p->name,p->age,p->sex,p->birthday,p->address,p->phone,p->email);printf("\n");p = p->next;}return ;}void search(){int num,x;char name[20];student *p = head->next;printf("请选择查询⽅式:\n");printf("1、按学号查询\t2、按姓名查询\n");scanf("%d",&x);if(x == 1){printf("需要查找的学⽣学号为:");scanf("%d",num);while(p != NULL){if(p->id == num){printf("学号为%d的学⽣信息如下:\n",num);printf("%d %s %d %c %s %s %s %s",p->id,p->name,p->age,p->sex,p->birthday,p->address,p->phone,p->email);return ;}p = p->next;}if(p == NULL){printf("⽆此记录!\n");}}else if(x == 2){printf("需要查找的学⽣姓名为:");getchar();gets(name);p = head->next;while(p != NULL){if(strcmp(p->name,name) == 0){printf("学⽣姓名为%s的学⽣信息如下:\n",name);printf("%d %s %d %c %s %s %s %s",p->id,p->name,p->age,p->sex,p->birthday,p->address,p->phone,p->email);return ;}p = p->next;}if(p == NULL){printf("⽆此记录!\n");}}return ;}void insert(){int num,i;student *p,*q;p = head;printf("请输⼊你要插⼊的位置:");scanf("%d",&num);if(num > length){printf("找不到插⼊的位置\n");return ;}else{printf("请输⼊你要插⼊的学⽣的信息:\n");q = (student *)malloc(sizeof(student));scanf("%d %s %d %c %s %s %s %s",&q->id,q->name,&q->age,&q->sex,q->birthday,q->address,q->phone,q->email);while(p != NULL){if(p->id == q->id){printf("该学号已经存在,⽆法插⼊!\n");return ;}p = p->next;}p = head;for(i=0; i<num; ++i){p = p->next;}q->next = p->next;p->next = q;length ++;printf("插⼊成功!\n");return ;}}void Delete(){int num;student *p,*q;q = head;p = head->next;printf("请输⼊要删除的学⽣的学号:\n");scanf("%d",&num);while(p != NULL){if(p->id == num){q->next = p->next;free(p);length --;printf("删除成功!\n");return ;}p = p->next;q = q->next;}if(p == NULL){printf("找不到要删除的编号!\n");return ;}}void menu(){printf("___________________________________________________\n"); printf("| 学⽣信息管理系统 |\n");printf("| 0、退出系统 |\n");printf("| 1、录⼊学⽣信息 |\n");printf("| 2、建⽴链表 |\n");printf("| 3、显⽰链表 |\n");printf("| 4、查找链表中的某个元素 |\n");printf("| 5、删除链表中指定学号的结点 |\n");printf("| 6、指定位置上插⼊⼀个新结点 |\n");printf("| 7、修改学⽣信息 |\n");printf("__________________________________________________\n"); return ;}int main(void){int a;menu();while(1){printf("请输⼊相应的功能:");scanf("%d",&a);switch(a){case0:return0;case1:LoadStudentInFromFile();menu();break;case2:create();menu();break;case3:if(head){display();menu();}else{printf("链表为空,请先建⽴链表!\n");menu();}break;case4:if(head){search();menu();}else{printf("链表为空,请先建⽴链表!\n");menu();}break;case5:if(head){Delete();menu();}else{printf("链表为空,请先建⽴链表!\n");menu();}break;case6:if(head){insert();menu();}else{printf("链表为空,请先建⽴链表!\n");menu();}break;case7:if(head){ModifyStudentInfo();menu();}else{printf("链表为空,请先建⽴链表!\n"); menu();}break;default:break;}}system("pause");return0;}。
C语言学生信息管理系统

C语⾔学⽣信息管理系统本⽂实例为⼤家分享了C语⾔学⽣信息管理系统的具体代码,供⼤家参考,具体内容如下列表内容系统以菜单⽅式⼯作学⽣信息录⼊功能(学⽣信息⽤⽂件保存)—输⼊学⽣信息浏览功能——输出查询、排序功能——算法1、按学号查询2、按姓名查询学⽣信息的删除与修改界⾯简单明了;有⼀定的容错能⼒,⽐如输⼊的成绩不在0~100之间,就提⽰不合法,要求重新输⼊;⽤链表的⽅式实现。
#include <stdio.h>#include <string.h>#include <stdlib.h>#include <windows.h>#include <string.h>typedef struct Student{char name[100]; //姓名char num[100]; //学号char sex;//性别 (w代表⼥⽣m代表男⽣)int age;//年龄int score;//成绩}stu;typedef struct LNode{stu data;struct LNode *next;}LinkList;char nam[100];//名字char nu[100];//学号char s;//性别int ag;//年龄int sc;//成绩void welocome()//登陆界⾯{system("color b1");printf("````````````````````````````````````````````````````````````````````````````````");printf("\n");printf("\n");printf("\n");printf(" *********************** 欢迎登录学⽣信息管理平台 ************************* \n");printf("\n");printf("\n");printf("\n");printf("````````````````````````````````````````````````````````````````````````````````");}void menu()//功能菜单{system("color e3");printf(" |________________________________________________|\n");printf(" | |\n");printf(" | 学⽣信息管理系统 |\n");printf(" | |\n");printf(" | 0、退出系统 |\n");printf(" | 1、增加学⽣信息 |\n");printf(" | 2、删除学⽣信息 |\n");printf(" | 3、修改学⽣信息 |\n");printf(" | 4、查找学⽣的信息 |\n");printf(" | 5、按照学⽣成绩排序 |\n");printf(" | 6、浏览全部学⽣信息 |\n");printf(" | 7、保存学⽣信息到⽂件 |\n");printf(" | |\n");printf(" |________________________________________________|\n"); return ;}void InitList( LinkList *&L)//初始化链表{L=(LinkList *)malloc(sizeof(LinkList));L->next=NULL;}void ListInsert(LinkList *&L,LinkList *p)//插⼊新的节点{LinkList *q=NULL;q=L;p->next=q->next;q->next=p;}void addstu(LinkList *&L)//增加新的学⽣{system("color f2");printf("请输⼊学⽣的信息:\n");printf("学号:");scanf("%s",nu);LinkList *q=L->next;while(q!=NULL ){if(strcmp(q->data.num,nu)==0)//判断是否存在{printf("该⽣已存在\n");break;}q=q->next;}if(q==NULL){LinkList *p;InitList(p);strcpy(p->data.num,nu);printf("姓名:") ;scanf("%s",nam) ;strcpy(p->,nam);printf("性别:(w为男 m为⼥)");scanf(" %c",&s);p->data.sex=s;printf("年龄:");scanf("%d",&ag);p->data.age=ag;printf("总成绩:");scanf("%d",&sc);while(sc>100||sc<0){printf("输⼊有误,请重新输⼊\n");scanf("%d",&sc);}p->data.score=sc;ListInsert(L,p);}}void deletestu(LinkList *L)//删除学⽣{system("color f4");printf("请输⼊您要删除的学⽣的学号:");scanf("%s",nu);//判断LinkList *p,*pre;if(L->next==NULL){printf("还没有学⽣信息,请增加学⽣信息\n");return;}pre=L;p=pre->next;int judge=0;while(p){if(strcmp(p->data.num,nu)==0){judge=1;pre->next =p->next;free(p);printf("删除学⽣成功\n");break;}pre=p;p=p->next;}if(judge==0)printf("该⽣不存在\n");}void changestu(LinkList *L)//改变学⽣信息{int judge=1;system("color e4");printf("请输⼊您要修改学⽣的学号:\n");scanf("%s",nu);LinkList *q=L->next;while(q!=NULL ){if(strcmp(q->data.num,nu)==0){judge=1;printf("请输⼊您要修改的信息选项:1.姓名 2. 总成绩 3.年龄 \n"); int n;scanf("%d",&n);switch(n){case 1:printf("请输⼊您要修改的名字:");scanf("%s",nam);printf("修改的名字为:%s\n",nam);strcpy(q->,nam);printf("修改名字成功!\n");break;case 2:printf("请输⼊您要修改的总成绩");scanf("%d",&sc);printf("修改的总成绩为:%d\n",sc);q->data.score=sc;printf("修改总成绩成功!\n");break;case 3:printf("请输⼊您要修改的年龄:");scanf("%d",&ag);printf("修改的年龄为:%d\n",ag);q->data.age=ag;printf("修改年龄成功!\n");break;default :printf("请输⼊正确的选项\n");break;}}q=q->next;}if(judge==0){printf("该⽣不存在\n");}}void findstu(LinkList *L)//按学号或者姓名查找学⽣并输出该⽣信息{int flag;system("color b1");printf("1.按学号查询:\n");printf("2.按姓名查询:\n");printf("请输⼊查询⽅式:");scanf("%d",&flag);if(flag==1){printf("请输⼊该⽣学号:");scanf("%s",nu);//判断LinkList *q=L->next;while(q!=NULL ){if(strcmp(q->data.num,nu)==0){printf("姓名:%s\n",q->);printf("学号:%s\n",q->data.num);printf("性别:%c\n",q->data.sex);printf("年龄:%d\n",q->data.age);printf("总成绩:%d\n",q->data.score);break;}q=q->next;}if(q==NULL)printf("该⽣不存在\n");}else{printf("请输⼊该⽣姓名:");scanf("%s",nam);LinkList *q=L->next;while(q!=NULL ){if(strcmp(q->,nam)==0){printf("姓名:%s\n",q->);printf("学号:%s\n",q->data.num);printf("性别:%c\n",q->data.sex);printf("年龄:%d\n",q->data.age);printf("总成绩:%d\n",q->data.score);break;}q=q->next;}if(q==NULL)printf("该⽣不存在\n");}}void display(LinkList *&L)//浏览全部学⽣信息{LinkList *q=L->next;if(q==NULL){printf("还没有学⽣信息,请增加学⽣信息\n");return;}while(q){system("color c0");printf(" 学号:%s 名字:%s 年龄:%d 性别:%c 总成绩:%d \n",q->data.num,q->,q->data.age,q->data.sex, q->data.score);q=q->next;}}void paixu(LinkList *L)//按成绩排序排序并输出排序后的结果{system("color f9");LinkList *q,*p,*r=L->next;//判断if(r==NULL){printf("还没有学⽣信息,请增加学⽣信息\n");return;}while(r) //两层循环完成排序{p=r;q=r->next;LinkList *tmp;//⽤于排序时暂存节点InitList(tmp);while(q){if(q->data.score > p->data.score){/*先复制q结点信息到tmp*/strcpy(tmp->data.num,q->data.num);strcpy(tmp->,q->);tmp->data.sex=q->data.sex;tmp->data.age=q->data.age;tmp->data.score=q->data.score;/*再复制p结点信息到q*/strcpy(q->data.num,p->data.num);strcpy(q->,p->);q->data.sex=p->data.sex;q->data.age=p->data.age;q->data.score=p->data.score;/*最后复制exchange结点信息到p*/strcpy(p->data.num,tmp->data.num);strcpy(p->,tmp->);p->data.sex=tmp->data.sex;p->data.age=tmp->data.age;p->data.score=tmp->data.score;}q=q->next;}r=r->next;}printf("排序后的学⽣信息是:\n");display(L);}void saveStuDentFile(LinkList * &L)//保存学⽣信息到⽂件{FILE *fp;LinkList *p=L->next;if((fp=fopen("student.txt","w"))==NULL)// 以可写的⽅式打开当前⽬录下的.txt{printf("不能打开此⽂件,请按任意键退出\n");exit(1);}while(p){fprintf(fp,"%s %s %c %d %d \n",p->data.num,p->,p->data.sex,p->data.age,p->data.score); p=p->next;printf("保存成功\n");}fclose(fp);}void readStuDentput (LinkList *&L) //运⾏前把⽂件内容读取到电脑内存{FILE *fp;fp=fopen("student.txt","rb"); //以只读⽅式打开当前⽬录下的.txtif(fp==NULL){printf("不存在打开⽂件\n");exit(0); //终⽌程序}int i=0;while(!feof(fp)){char nu[100];//学号char nam[100];//名字char s;//性别int ag;//年龄int sc;//成绩fscanf(fp," %s %s %c %d %d",nu,nam,&s,&ag,&sc);i++;}fclose(fp);FILE *FP;FP=fopen("student.txt","rb"); //以只读⽅式打开当前⽬录下的.txtif(FP==NULL){printf("⽆法打开⽂件\n");exit(0); //终⽌程序}int b=i-1;int j=1;while(!feof(FP)){fscanf(FP,"%s %s %c %d %d",nu,nam,&s,&ag,&sc);LinkList *n=(LinkList *)malloc(sizeof(LinkList));strcpy(n->data.num,nu);//把后者的内容拷贝到前者中strcpy(n->,nam);//把后者的内容拷贝到前者中n->data.sex=s;n->data.age=ag;n->data.score=sc;ListInsert(L,n);//插⼊新的节点n=n->next;if(j==b)break;j++;}fclose(FP); //关闭⽂件}int main(){system("cls");//清屏welocome();//登陆界⾯Sleep(3000);//延缓3秒LinkList *L;InitList(L);readStuDentput (L);//运⾏前把⽂件内容读取到电脑int a;int choose;while(1){printf("请输⼊您要选择的功能键:\n");menu();//功能菜单scanf("%d",&choose);switch(choose){case 0://退出printf("谢谢使⽤!欢迎下次光临");exit(0);case 1://增加学⽣信息addstu(L);//增加新的学⽣break;case 2://删除所有学⽣信息deletestu(L);//删除学⽣break;case 3://改变个学⽣的信息changestu(L);//改变学⽣信息break;case 4://查找某个学⽣的信息findstu(L);//按学号查找学⽣并输出该⽣信息break;case 5:// 对学⽣成绩进⾏排序paixu(L);break;case 6://输出所有学⽣的信息display(L);break;case 7://保存学⽣信息到⽂件saveStuDentFile(L);break;default:printf("请输⼊正确的选择\n");break;}}}以上就是本⽂的全部内容,希望对⼤家的学习有所帮助,也希望⼤家多多⽀持。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
#include <stdio.h>#include <string.h>#include <stdlib.h>#include <conio.h>#include <time.h>#define N 20void input();//增加学生信息void del();//删除学生信息void change();//修改学生信息void find();//查询学生信息void sorting();//排序void save();//保存到文件void read();//读取文件void stu_search();//学生查询信息void modify();//学生修改自己密码struct score_stu//定义分数结构体{int score1;int score2;int score3;int sum;}score;struct student //定义学生结构体{int no;char name[20];char spwd[20];int classnum;struct score_stu score;int age;int ranking;}stu[N],*p;void inputPasswd(char passwd[])//隐藏密码{char s[20];char temp[2];int n;//strcpy(s," ");s[0]='\0';while(1){while(1){n=getch();if(n == 13)//13为回车'\r'{break;}printf("*");memset(temp,0,sizeof(temp));//将temp里面的数据用0替换sprintf(temp,"%c",n);//将n打印成一个字符保存到temp里面strcat(s,temp);//把temp所指字符串添加到s结尾处(覆盖s结尾处的'\0') }break;}printf("\n");strcpy(passwd, s);}void captcha(char str[],int n)//验证码{char a[]="1234567890abcdefghijkmnopqrstuvwxyzABCDEFGHIJLMNPQRSTUVWXYZ";int i,j,k;k=strlen(a);//k表示字符串的长度srand(time(0));//随机数初始化for(i=0;i<n;i++){j=rand()%k;//取0-58的随机数str[i]=a[j];}str[i]='\0';}void admin()//管理员操作{int choose1;printf("\t\t1:增加学生信息\t2:删除学生信息\t3:修改学生信息\t4:查询学生信息\t5:排序学生信息\t6:退出程序\n");printf("\t\t");scanf("%d",&choose1);printf("\t\t=================================================================== ======================\n");fflush(stdin);switch(choose1){case 1: input();break;case 2: del();break;case 3: change();break;case 4: find();break;case 5: sorting();break;case 6: printf("\t\t退出成功!\n");exit(0);break;default :printf("\t\t你所选择的操作不是上述操作,请重新选择\n"); admin();break;}}void admin_login()//管理员登录{static int i=0;int j=3;char username[10];char pwd[10];char code[N],str[N];printf("\t\t**********************************欢迎进入管理员登录界面**********************************\n");printf("\t\t请输入帐号:\n");printf("\t\t");gets(username);printf("\t\t=================================================================== ======================\n");printf("\t\t请输入密码:\n");printf("\t\t");inputPasswd(pwd);printf("\t\t=================================================================== ======================\n");fflush(stdin);if((strcmp(username,"admin")==0) &&(strcmp(pwd,"admin")==0)){while(1){captcha(code,4);printf("\t\t请输入验证码:%s\n",code);printf("\t\t");scanf("%s",str);printf("\t\t=================================================================== ======================\n");if(strcmp(code,str)==0){printf("\n\t\t 您已成功登录 \n ");printf("\t\t请选择你要执行的操作:\n");admin();}else{j--;if(j==0){printf("\t\t错误次数过多,系统自动退出\n");exit(0);}}}}else{while(i<2){printf("\n 用户名和/或密码无效请重新输入 \n ");i++;admin_login();}printf("登录次数超限,自动退出!\n");exit(0);}}void student(int userNO)//学生操作{int x;printf("\t\t1:查询个人信息\t2:修改密码\t3:退出\n");printf("\t\t");scanf("%d",&x);printf("\t\t=========================================================================================\n");switch(x){case 1:stu_search(userNO);break;case 2:modify(userNO);break;case 3:printf("\t\t退出成功!\n");exit(0);break;default:printf("\t\t你所选择的项目错误!重新选择!");student(userNO);break;}}int student_login()//学生登录{static int j=0;int i;int k=3;int userNO;char pwd[20];char code[N+1],str[N+1];printf("\t\t**********************************欢迎进入学生登录界面**********************************\n");printf("\t\t请输入学号:\n");printf("\t\t");fflush(stdin);scanf("%d",&userNO);printf("\t\t=================================================================== ======================\n");printf("\t\t请输入密码:\n");printf("\t\t");fflush(stdin);inputPasswd(pwd);printf("\t\t=================================================================== ======================\n");read();for(i=0;i<N;i++){if((userNO == stu[i].no)&&(strcmp(pwd,stu[i].spwd)==0)){while(1){captcha(code,4);printf("\t\t请输入验证码:%s\n",code);printf("\t\t");scanf("%s",str);printf("\t\t=================================================================== ======================\n");if(strcmp(code,str)==0){printf("\t\t登录成功!\n");printf("\t\t请选择操作!\n");student(userNO);}else{k--;if(k==0){printf("\t\t错误次数过多,系统自动退出\n");exit(0);}}}}else{while(j<2){printf("\t\t登录失败,请重新登录!\n");j++;student_login();}printf("\t\t登陆次数超限!系统自动退出!");exit(0);}}return userNO;}void stu_ranking()//计算排名{int i,j;int count=1;read();for(i=0;stu[i].no!=0;i++){for(j=0;stu[j].no!=0;j++){if(stu[i].score.sum<stu[j].score.sum){count++;}}stu[i].ranking=count;count=1;}save();}void stu_search(int userNO)//学生查询个人信息{int i,x;read();for(i=0;i<N;i++){if(userNO == stu[i].no){printf("\t\t学号\t\t密码\t班级\t成绩一\t成绩二\t成绩三\t总分\t 年龄\t排名\n");printf("\t\t%d\t%s\t%s\t%d\t%d\t%d\t%d\t%d\t%d\t%d\n\n",stu[i].no,stu[i].name,s tu[i].spwd,stu[i].classnum,stu[i].score.score1,stu[i].score.score2,stu[i].score.score3,stu[i].score.sum, stu[i].age,stu[i].ranking);printf("\t\t=================================================================== ======================\n");printf("\t\t请选择退出系统或返回主菜单\n");printf("\t\t1:返回主菜单\t2:退出\n");printf("\t\t");scanf("%d",&x);printf("\t\t=================================================================== ======================\n");switch(x){case 1:student(userNO);break;case 2:printf("\t\t退出成功!\n");exit(0);}}else{printf("\t\t输入错误,系统自动返回主菜单!\n");student(userNO);}}}void modify(int userNO)//学生修改个人密码{fflush(stdin);static int k=0;int i;char pwd_old[20];char pwd_new[20];char pwd_new1[20];read();for(i=0;i<N;i++){if(userNO == stu[i].no){printf("\t\t请输入旧密码:\n");printf("\t\t");gets(pwd_old);printf("\t\t=================================================================== ======================\n");if(strcmp(pwd_old,stu[i].spwd)==0){printf("\t\t请输入新密码:\n");printf("\t\t");gets(pwd_new);printf("\t\t=================================================================== ======================\n");printf("\t\t请再次输入新密码:\n");printf("\t\t");gets(pwd_new1);printf("\t\t=================================================================== ======================\n");if(strcmp(pwd_new,pwd_new1)==0){printf("\t\t修改完成!\n");strcpy(stu[i].spwd,pwd_new);printf("\t\t=================================================================== ======================\n");break;}else{while(1){k++;if(k==3){printf("\t\t错误次数过多,自动返回主菜单!\n");printf("\t\t=================================================================== ======================\n");student(userNO);}else{printf("\t\t输入两次密码不一致请重新进入!\n");printf("\t\t=================================================================== ======================\n");modify(userNO);}}}}else{printf("\t\t密码输入不正确!自动返回主菜单\n");printf("\t\t=================================================================== ======================\n");student(userNO);}}}save();student(userNO);}void input()//增加学生信息{char ch;int i,n,count= 0;printf("\t\t请输入添加学生信息的条数:\n");printf("\t\t");scanf("%d",&n);printf("\t\t=================================================================== ======================\n");if(n<1||n>N){printf("\t\t输入不合法,请重新输入:\n");input();}printf("\t\t请添加学生信息:\n");printf("\t\t学号\t\t密码\t班级\t成绩一\t成绩二\t成绩三\t年龄\n"); read();for(i=0;i<N;i++){if(count == n){break;}if(stu[i].no==0){fflush(stdin);printf("\t\t");scanf("%d%s%s%d%d%d%d%d",&stu[i].no,stu[i].name,stu[i].spwd,&stu[i].classnum, &stu[i].score.score1,&stu[i].score.score2,&stu[i].score.score3,&stu[i].age);stu[i].score.sum=stu[i].score.score1+stu[i].score.score2+stu[i].score.score3; count++;}}save();stu_ranking();printf("\t\t=================================================================== ======================\n");printf("\t\t添加学生信息成功!\n");printf("\t\t是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);if(ch=='Y'||ch=='y'){input();}admin();}void del()//删除学生信息{fflush(stdin);int i,j;char ch;int del_no;printf("\t\t请输入想要删除记录的ID:\n");printf("\t\t");scanf("%d",&del_no);printf("\t\t=================================================================== ======================\n");read();for(i=0;stu[i].no!=0;i++){if(del_no == stu[i].no){for(j=i;j<N-1;j++){stu[j]=stu[j+1];}}}save();stu_ranking();printf("\t\t=========================================================================================\n");printf("\t\t删除信息成功!\n");printf("\t\t是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);if(ch=='Y'||ch=='y'){del();}admin();}void change()//修改学生信息{fflush(stdin);int i;char ch;int change_no;printf("\t\t请输入想要修改记录的ID:\n");printf("\t\t");scanf("%d",&change_no);printf("\t\t=================================================================== ======================\n");read();printf("\t\t学号\t\t密码\t班级\t成绩一\t成绩二\t成绩三\t年龄\n"); for(i=0;stu[i].no!=0;i++){if(change_no == stu[i].no){printf("\t\t%d\t%s\t%s\t%d\t%d\t%d\t%d\t%d\n",stu[i].no,stu[i].name,stu[i].spwd ,stu[i].classnum,stu[i].score.score1,stu[i].score.score2,stu[i].score.score3,stu [i].age);printf("\t\t请输入你要修改后的信息!\n");printf("\t\t=================================================================== ======================\n");printf("\t\t");scanf("%d%s%s%d%d%d%d%d",&stu[i].no,stu[i].name,stu[i].spwd,&stu[i].classnum,&stu[i].score.score1,&stu[i].score.score2,&stu[i].score.score3,&stu[i].age); }stu[i].score.sum=stu[i].score.score1+stu[i].score.score2+stu[i].score.score3; }save();stu_ranking();printf("\t\t=================================================================== ======================\n");printf("\t\t修改学生信息成功!\n");printf("\t\t是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);if(ch=='Y'||ch=='y'){change();}admin();}void find1()//按查询{fflush(stdin);int i;char ch;int count=0;char find_name[20];printf("\t\t请输入想要查询的学生:\n");printf("\t\t");gets(find_name);printf("\t\t=================================================================== ======================\n");read();for(i=0;stu[i].no!=0;i++){if(strcmp(find_name,stu[i].name)==0){printf("\t\t学号\t\t密码\t班级\t成绩一\t成绩二\t成绩三\t总分\t年龄\t排名\n");printf("\t\t%d\t%s\t%s\t%d\t%d\t%d\t%d\t%d\t%d\t%d\n",stu[i].no,stu[i].name,stu [i].spwd,stu[i].classnum,stu[i].score.score1,stu[i].score.score2,stu[i].score.score3, stu[i].score.sum,stu[i].age,stu[i].ranking);count++;}}if(count==0){printf("\t\t操作错误是否继续?(Y/N)\n");printf("\t\t");scanf("%c",&ch);printf("\t\t=================================================================== ======================\n");if(ch=='Y'||ch=='y'){find1();}else{find();}}else{printf("\t\t查询学生信息成功!\n");printf("\t\t是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);printf("=\t\t================================================================== ======================\n");if(ch=='Y'||ch=='y'){find1();}else{find();}}}void find2()//按成绩一查询{fflush(stdin);int i;char ch;int count=0;int score;printf("\t\t请输入你要查询的成绩一分数\n");printf("\t\t");scanf("%d",&score);printf("\t\t=================================================================== ======================\n");read();printf("\t\t学号\t\t密码\t班级\t成绩一\t成绩二\t成绩三\t总分\t年龄\t 排名\n");for(i=0;stu[i].no!=0;i++){if(stu[i].score.score1 == score){printf("\t\t%d\t%s\t%s\t%d\t%d\t%d\t%d\t%d\t%d\t%d\n",stu[i].no,stu[i].name,stu [i].spwd,stu[i].classnum,stu[i].score.score1,stu[i].score.score2,stu[i].score.score3, stu[i].score.sum,stu[i].age,stu[i].ranking);count++;}}if(count==0){printf("\t\t操作错误是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);printf("\t\t=================================================================== ======================\n");if(ch=='Y'||ch=='y'){find2();}else{find();}}else{printf("\t\t查询学生信息成功!\n");printf("\t\t是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);printf("\t\t=================================================================== ======================\n");if(ch=='Y'||ch=='y'){find2();}else{find();}}}void find3()//按成绩二查询{fflush(stdin);int i;char ch;int count=0;int score;printf("\t\t请输入你要查询的成绩二分数\n");printf("\t\t");scanf("%d",&score);printf("\t\t=================================================================== ======================\n");read();printf("\t\t学号\t\t密码\t班级\t成绩一\t成绩二\t成绩三\t总分\t年龄\t 排名\n");for(i=0;stu[i].no!=0;i++){if(stu[i].score.score2 == score){printf("\t\t%d\t%s\t%s\t%d\t%d\t%d\t%d\t%d\t%d\t%d\n",stu[i].no,stu[i].name,stu [i].spwd,stu[i].classnum,stu[i].score.score1,stu[i].score.score2,stu[i].score.score3, stu[i].score.sum,stu[i].age,stu[i].ranking);count++;}}if(count==0){printf("\t\t操作错误是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);printf("\t\t=================================================================== ======================\n");if(ch=='Y'||ch=='y'){find3();}else{find();}}else{printf("\t\t查询学生信息成功!\n");printf("\t\t是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);printf("\t\t=================================================================== ======================\n");if(ch=='Y'||ch=='y'){find3();}else{find();}}}void find4()//按成绩三查询{fflush(stdin);int i;char ch;int count=0;int score;printf("\t\t请输入你要查询的成绩三分数\n");printf("\t\t");scanf("%d",&score);printf("\t\t=================================================================== ======================\n");read();printf("\t\t学号\t\t密码\t班级\t成绩一\t成绩二\t成绩三\t总分\t年龄\t 排名\n");for(i=0;stu[i].no!=0;i++){if(stu[i].score.score3 == score){printf("\t\t%d\t%s\t%s\t%d\t%d\t%d\t%d\t%d\t%d\t%d\n",stu[i].no,stu[i].name,stu [i].spwd,stu[i].classnum,stu[i].score.score1,stu[i].score.score2,stu[i].score.score3,stu[i].score.sum, stu[i].age,stu[i].ranking);count++;}}if(count==0){printf("\t\t操作错误是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);printf("\t\t=================================================================== ======================\n");if(ch=='Y'||ch=='y'){find4();}else{find();}}else{printf("\t\t查询学生信息成功!\n");printf("\t\t是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);if(ch=='Y'||ch=='y'){find4();}else{find();}}}void find5()//按排名查询{fflush(stdin);int i;char ch;int count=0;int ranking;printf("\t\t请输入想要查询的学生排名:\n");printf("\t\t");scanf("%d",&ranking);printf("\t\t=================================================================== ======================\n");read();for(i=0;stu[i].no!=0;i++){if(ranking==stu[i].ranking){printf("\t\t学号\t\t密码\t班级\t成绩一\t成绩二\t成绩三\t总分\t年龄\t排名\n");printf("\t\t%d\t%s\t%s\t%d\t%d\t%d\t%d\t%d\t%d\t%d\n",stu[i].no,stu[i].name,stu [i].spwd,stu[i].classnum,stu[i].score.score1,stu[i].score.score2,stu[i].score.score3, stu[i].score.sum,stu[i].age,stu[i].ranking);count++;}}if(count==0){printf("\t\t操作错误是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);printf("\t\t=================================================================== ======================\n");if(ch=='Y'||ch=='y'){find5();}else{find();}}else{printf("\t\t查询学生信息成功!\n");printf("\t\t是否继续?(Y/N)\n");fflush(stdin);printf("\t\t");scanf("%c",&ch);printf("\t\t=================================================================== ======================\n");if(ch=='Y'||ch=='y'){find5();}else{find();}}}void find6()//全部查询{int i,j;read();printf("\t\t学号\t\t密码\t班级\t成绩一\t成绩二\t成绩三\t总分\t年龄\t排名\n");for(i=0;stu[i].no!=0;i++){printf("\t\t%d\t%s\t%s\t%d\t%d\t%d\t%d\t%d\t%d\t%d\n",stu[i].no,stu[i].name,stu [i].spwd,stu[i].classnum,stu[i].score.score1,stu[i].score.score2,stu[i].score.score3, stu[i].score.sum,stu[i].age,stu[i].ranking);}printf("\t\t返回上一级还是退出?(1,返回|2,退出)\n\n");printf("\t\t");scanf("%d",&j);printf("\t\t=================================================================== ======================\n");if(j==1){find();}else{exit(0);}}void find()//查询学生信息{int choose2;printf("\t\t选择你要查询的方式:\n");printf("\t\t0:根据查询\t1:根据单科成绩一查询\t2:根据单科成绩二查询\t3:根据单科成绩三查询\n");printf("\t\t4:根据排名\t5:全部查询\t6:返回主菜单\n");printf("\t\t");scanf("%d",&choose2);printf("\t\t=================================================================== ======================\n");switch(choose2){case 0:find1();break;case 1:find2();break;case 2:find3();break;case 3:find4();break;case 4:find5();break;case 5:find6();break;case 6:admin();break;default :printf("\t\t你所选择的操作不是上述操作,请重新选择\n");find();}}void sorting1()//按排序{int i,j;struct student temp;read();for(i=0;stu[i].no!=0;i++){for(j=i;stu[j].no!=0;j++){if(strcmp(stu[i].name,stu[j].name)==1){temp=stu[i];stu[i]=stu[j];stu[j]=temp;}}}save();stu_ranking();printf("\t\t排序成功!\n");printf("\t\t=================================================================== ======================\n\n");sorting();}void sorting2()//按成绩一排序{int i,j;struct student temp;read();for(i=0;stu[i].no!=0;i++){for(j=i;stu[j].no!=0;j++){if(stu[i].score.score1<stu[j].score.score1){temp=stu[i];stu[i]=stu[j];stu[j]=temp;}}}save();stu_ranking();printf("\t\t排序成功!\n");printf("\t\t=================================================================== ======================\n");sorting();}void sorting3()//按成绩二排序{int i,j;struct student temp;read();for(i=0;stu[i].no!=0;i++){for(j=i;stu[j].no!=0;j++){if(stu[i].score.score2<stu[j].score.score2){temp=stu[i];stu[i]=stu[j];stu[j]=temp;}}}save();stu_ranking();printf("\t\t排序成功!\n");printf("\t\t=================================================================== ======================\n");sorting();}void sorting4()//按成绩三排序{int i,j;struct student temp;read();for(i=0;stu[i].no!=0;i++){for(j=i;stu[j].no!=0;j++){if(stu[i].score.score3<stu[j].score.score3){temp=stu[i];stu[i]=stu[j];stu[j]=temp;}}}save();stu_ranking();printf("\t\t排序成功!\n");printf("\t\t=================================================================== ======================\n");sorting();}void sorting5()//按排名排序{int i,j;struct student temp;read();for(i=0;stu[i].no!=0;i++){for(j=i;stu[j].no!=0;j++){if(stu[i].ranking>stu[j].ranking){temp=stu[i];stu[i]=stu[j];stu[j]=temp;}}}save();stu_ranking();printf("\t\t排序成功!\n");printf("\t\t=================================================================== ======================\n");sorting();}void sorting()//排序学生信息{int choose3;printf("\t\t请选择排序的方式\n");printf("\t\t0:根据排序\t1:根据单科成绩一排序\t2:根据单科成绩二排序\t3:根据单科成绩三排序\n\t\t4:根据排名进行排序\t5:返回主菜单\n");printf("\t\t");scanf("%d",&choose3);printf("\t\t=================================================================== ======================\n");switch(choose3){case 0: sorting1();break;case 1: sorting2();break;case 2: sorting3();break;case 3: sorting4();break;case 4: sorting5();break;case 5: admin();break;default :printf("\t\t你所选择的操作不是上述操作,请重新选择!\n");sorting();}}void save()//保存所有信息{int i;FILE *fp;fp=fopen("C:\\Users\\Administrator\\Desktop\\student.txt","wb+");for(i=0;stu[i].no!=0;i++){fwrite(&stu[i],sizeof(struct student),1,fp);}fclose(fp);}void read()//读出文件的信息{FILE *fp;fp=fopen("C:\\Users\\Administrator\\Desktop\\student.txt","rb");fread(&stu[0],sizeof(struct student),N,fp);fclose(fp);}void title()//开始介绍{printf("\t\t\t\t\t项目名称:C语言下的学生管理系统\n");printf("\t\t\t\t\t制作人:王顺\n");printf("\t\t\t\t\t制作时间:2016-10\n");printf("\t\t\t\t\t第九城市教育创游科技有限公司\n");}int main()//主函数{title();system("pause");system("cls");int userNO;int choose;printf("\t\t**********************************C语言下的学生管理系统**********************************\n");printf("\t\t你好!,请选择登录身份!\n");printf("\t\t0:管理员\t 1:学生 \t2:退出\n");printf("\t\t");scanf("%d",&choose);fflush(stdin);switch(choose){case 0:{admin_login();break;}case 1:{userNO = student_login();student(userNO);break;}case 2:printf("\t\t退出成功!\n");break;default :printf("\t\t你选择的身份不正确,请重新选择\n\n\n"); main();break;}return 0;}。