1. Introduction From algorithms to generative grammar and back again
Introduction to Algorithms
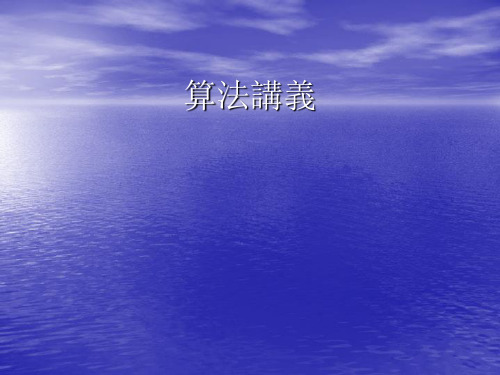
• 本文對基本算法説明的基本方式
– 説明算法的基本原理 – 給出由此算法解決的實例
定義我們要解決的問題
給定有待排序的N 給定有待排序的N個項目 R1,R2,…,RN 我們稱這些項目為記錄(record),並稱N 我們稱這些項目為記錄(record),並稱N個記錄的整 個集合為一個文件(file)。每一個R 個集合為一個文件(file)。每一個Rj有一個鍵碼 (key) Kj ,支配排序過程。 排序的目標,是確定下標為{1,2,…,N}的一個排列 排序的目標,是確定下標為{1,2,…,N}的一個排列 p(1) p(2)…p(N),它以非遞降的次序來放置所有的 p(2)…p(N),它以非遞降的次序來放置所有的 鍵碼: Kp(1) ≤ Kp(2) ≤ …≤ Kp(N)
合併兩個已經排列好的序列
MERGE(A, p, q, r)
1 n1 ← q - p + 1 2 n2 ← r - q 3 create arrays L[1 ‥ n1 + 1] and R[1 ‥ n2 + 1] 4 for i ← 1 to n1 5 do L[i] ← A[p + i - 1] 6 for j ← 1 to n2 7 do R[j] ← A[q + j] 8 L[n1 + 1] ← ∞ 9 R[n2 + 1] ← ∞ 10 i ← 1 11 j ← 1 12 for k ← p to r 13 do if L[i] ≤ R[j] 14 then A[k] ← L[i] i←i+1 15 16 else A[k] ← R[j] 17 j←j+1
算法講義
目的
• 算法(algorithm)是一個優秀程序員的基本功, 算法(algorithm)
IntroductiontoAlgorithms第三版教学设计

Introduction to Algorithms第三版教学设计一、课程概要1.1 课程名称本课程名称为Introduction to Algorithms,是计算机科学专业必修课程。
1.2 学时安排本课程总共为72学时,每周3学时,共计24周。
1.3 教材《算法导论(原书第3版)》(英文版)(Introduction to Algorithms, Third Edition)。
1.4 教学目的本课程旨在使学生掌握算法与数据结构的基本概念、常用算法设计技巧和分析方法,并培养学生独立思考和解决问题的能力。
1.5 先修课程本课程的先修课程包括数据结构、离散数学、算法分析与设计等。
1.6 课程内容简介本课程包括以下内容:•算法基础知识•分治算法和递归•动态规划•贪心算法•图论算法•字符串匹配•NP完全性理论二、课程教学设计2.1 教学方法本课程采用理论讲授、实验操作、课堂讨论等多种教学方法相结合的方式,重视学生自主学习和动手实践的能力培养。
2.2 教学内容和教学进度本课程的教学内容和教学进度如下:第一讲:算法基础知识讲授主要内容包括算法的概念、算法的正确性和复杂度分析。
第二讲:分治算法和递归讲授主要内容包括分治算法的适用场景、递归的概念和应用。
第三讲:动态规划讲授主要内容包括动态规划的基本思想、常用动态规划算法和实践应用。
第四讲:贪心算法讲授主要内容包括贪心算法的基本思想、贪心算法设计和分析方法。
第五讲:图论算法讲授主要内容包括最短路径算法、最小生成树算法、网络流算法等。
第六讲:字符串匹配讲授主要内容包括朴素算法、KMP算法、Boyer-Moore算法等字符串匹配算法。
第七讲:NP完全性讲授主要内容包括P和NP问题的概念、NP完全性理论、NP问题求解方法等。
2.3 课堂互动与实践本课程还将开展相关实践项目,包括算法设计实验、算法实现与优化、算法竞赛和创新项目等,以培养学生动手实践和解决实际问题的能力。
an introduction to the analysis of algorithms
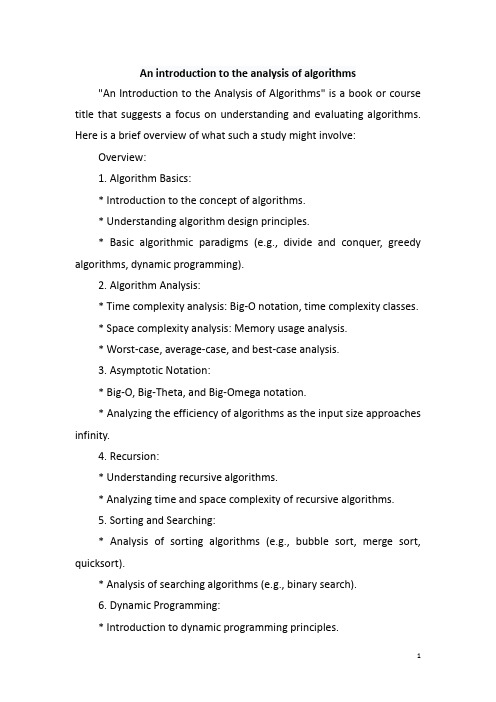
An introduction to the analysis of algorithms"An Introduction to the Analysis of Algorithms" is a book or course title that suggests a focus on understanding and evaluating algorithms. Here is a brief overview of what such a study might involve:Overview:1.Algorithm Basics:* Introduction to the concept of algorithms.* Understanding algorithm design principles.* Basic algorithmic paradigms (e.g., divide and conquer, greedy algorithms, dynamic programming).2.Algorithm Analysis:* Time complexity analysis: Big-O notation, time complexity classes.* Space complexity analysis: Memory usage analysis.* Worst-case, average-case, and best-case analysis.3.Asymptotic Notation:* Big-O, Big-Theta, and Big-Omega notation.* Analyzing the efficiency of algorithms as the input size approaches infinity.4.Recursion:* Understanding recursive algorithms.* Analyzing time and space complexity of recursive algorithms.5.Sorting and Searching:* Analysis of sorting algorithms (e.g., bubble sort, merge sort, quicksort).* Analysis of searching algorithms (e.g., binary search).6.Dynamic Programming:* Introduction to dynamic programming principles.* Analyzing algorithms using dynamic programming.7.Greedy Algorithms:* Understanding the greedy algorithmic paradigm.* Analyzing algorithms using greedy strategies.8.Graph Algorithms:* Analyzing algorithms related to graphs (e.g., Dijkstra's algorithm, breadth-first search, depth-first search).9.Case Studies:* Analyzing real-world applications of algorithms.* Case studies on how algorithm analysis influences practical implementations.10.Advanced Topics:* Introduction to advanced algorithmic concepts.* Optional topics such as randomized algorithms, approximation algorithms, and more.Conclusion:"An Introduction to the Analysis of Algorithms" provides a foundation for students or readers to understand the efficiency and performance of algorithms. It equips them with the tools to choose the right algorithms for specific tasks and to evaluate their impact on system resources.。
一个游戏程序员学习资料
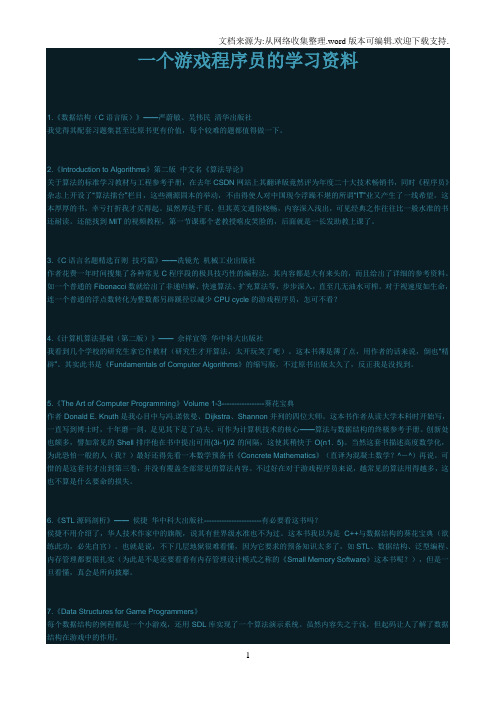
一个游戏程序员的学习资料1.《数据结构(C语言版)》——严蔚敏、吴伟民清华出版社我觉得其配套习题集甚至比原书更有价值,每个较难的题都值得做一下。
2.《Introduction to Algorithms》第二版中文名《算法导论》关于算法的标准学习教材与工程参考手册,在去年CSDN网站上其翻译版竟然评为年度二十大技术畅销书,同时《程序员》杂志上开设了“算法擂台”栏目,这些溯源固本的举动,不由得使人对中国现今浮躁不堪的所谓“IT”业又产生了一线希望。
这本厚厚的书,幸亏打折我才买得起。
虽然厚达千页,但其英文通俗晓畅,内容深入浅出,可见经典之作往往比一般水准的书还耐读。
还能找到MIT的视频教程,第一节课那个老教授嘻皮笑脸的,后面就是一长发助教上课了。
3.《C语言名题精选百则技巧篇》——冼镜光机械工业出版社作者花费一年时间搜集了各种常见C程序段的极具技巧性的编程法,其内容都是大有来头的,而且给出了详细的参考资料。
如一个普通的Fibonacci数就给出了非递归解、快速算法、扩充算法等,步步深入,直至几无油水可榨。
对于视速度如生命,连一个普通的浮点数转化为整数都另辟蹊径以减少CPU cycle的游戏程序员,怎可不看?4.《计算机算法基础(第二版)》——佘祥宣等华中科大出版社我看到几个学校的研究生拿它作教材(研究生才开算法,太开玩笑了吧)。
这本书薄是薄了点,用作者的话来说,倒也“精辟”。
其实此书是《Fundamentals of Computer Algorithms》的缩写版,不过原书出版太久了,反正我是没找到。
5.《The Art of Computer Programming》Volume 1-3-----------------葵花宝典作者Donald E. Knuth是我心目中与冯.诺依曼、Dijkstra、Shannon并列的四位大师。
这本书作者从读大学本科时开始写,一直写到博士时,十年磨一剑,足见其下足了功夫。
传统算法英语作文

传统算法英语作文In the age of rapid technological advancements, the role of traditional algorithms remains pivotal in the field of computing. Traditional algorithms, which have been developed and refined over centuries, continue to be the backbone of many modern computing systems. This essay will delve into the significance of traditional algorithms, their applications in contemporary technology, and their enduring relevance.### Historical SignificanceTraditional algorithms have been the cornerstone of mathematics and computer science since ancient times. Algorithms such as the Euclidean algorithm for finding the greatest common divisor, the Fibonacci sequence in number theory, and the Sieve of Eratosthenes for prime number generation have stood the test of time. These algorithms have been instrumental in shaping the foundations of computer science.### Applications in Modern TechnologyDespite the emergence of sophisticated machine learning and artificial intelligence techniques, traditional algorithms continue to be widely used. For instance:- Sorting Algorithms: QuickSort, MergeSort, and HeapSort are still used for organizing data efficiently. They arefundamental in database management systems and data analytics. - Search Algorithms: Binary search is a classic example thatis used in various applications where quick lookup is necessary, such as in filesystems and search engines.- Graph Algorithms: Dijkstra's and A* are still employed for pathfinding and routing in GPS navigation systems and network routing protocols.- Cryptography: Traditional algorithms like RSA and AES arethe bedrock of secure communication over the internet.### Enduring RelevanceThe enduring relevance of traditional algorithms can be attributed to several factors:1. Simplicity and Efficiency: Many traditional algorithms are simple to understand and implement, making them ideal for educational purposes and for applications where computational resources are limited.2. Robustness: They have been tested over time and are knownto be robust and reliable.3. Foundation for Innovation: Modern algorithms often build upon traditional ones, improving their efficiency or adapting them to new contexts.4. Educational Value: Learning traditional algorithms helps students understand the principles of computer science and prepares them for more complex concepts.### ConclusionIn conclusion, traditional algorithms are not relics of thepast but are living, breathing components of modern computing. They provide a solid foundation upon which the edifice of computer science is built. As we continue to innovate and develop new technologies, the importance of understanding and applying traditional algorithms cannot be overstated. Theyare not just a part of history; they are a vital part of our present and future in the world of computing.。
麻省理工大学算法导论lecture02

2
(
5 + 5 2 1 + 16 16
+L geometric series
L2.17
( ) +( )
)
Introduction to Algorithms
The master method
The master method applies to recurrences of the form T(n) = a T(n/b) + f (n) , where a ≥ 1, b > 1, and f is asymptotically positive.
Day 3
Introduction to Algorithms
L2.11
Example of recursion tree
Solve T(n) = T(n/4) + T(n/2) + n2: n2 (n/4)2 T(n/16) T(n/8) (n/2)2 T(n/8) T(n/4)
Day 3
Introduction to Algorithms
(n/8)2
…
Example of recursion tree
Solve T(n) = T(n/4) + T(n/2) + n2: n2 (n/4)2 (n/16)2 Θ(1)
Day 3 Introduction to Algorithms L2.16
n2
5 n2 16 25 n 2 256
…
(n/2)2 (n/8)2 (n/4)2
Pick c1 big enough to handle the initial conditions.
Day 3 Introduction to Algorithms L2.7
英语 算法 -回复

英语算法-回复以下是我根据题目内容所写的一篇1500-2000字的英文文章:Title: An Overview of Algorithms [English Algorithm]Introduction:In the world of computer science, algorithms play a fundamental role in solving problems efficiently. They represent a step-by-step method to process inputs and produce desired outputs. In this article, we will delve into the subject of algorithms and explore their significance in various domains.Section 1: Understanding AlgorithmsTo comprehend algorithms, we must first define what they are. An algorithm refers to a well-defined set of instructions designed to solve a specific problem or perform a particular task. Algorithms can be found in almost every aspect of our lives, from simple everyday routines, such as making a sandwich, to complex scientific computations.Section 2: The Mechanics of AlgorithmsAlgorithms follow a specific structure, known as a flowchart, whichoutlines each step required to accomplish a goal. They typically involve input, processing, and output stages. The input stage involves gathering necessary information, while the processing stage involves applying various operations or calculations to the inputs. Lastly, the output stage produces the desired result.Section 3: Types of AlgorithmsThere are several types of algorithms, each serving a different purpose. Sorting algorithms, for example, are designed to arrange elements in a specific order, such as numerical or alphabetical. Examples of sorting algorithms include Bubble Sort, Insertion Sort, and Quick Sort. Searching algorithms, on the other hand, help locate specific elements within a dataset. Some commonly used searching algorithms include Linear Search and Binary Search. Other types of algorithms include pathfinding algorithms, graph algorithms, and genetic algorithms.Section 4: Importance of AlgorithmsAlgorithms are crucial in various fields and industries. They are extensively used in computer programming, where efficient algorithms can significantly improve the performance of software applications. Algorithms are also employed in data analysis, wherethey enable researchers to identify patterns, trends, and correlations within large datasets. In addition, algorithms are utilized in artificial intelligence systems, autonomous vehicles, and medical diagnostic tools.Section 5: Algorithm Design and AnalysisDeveloping an algorithm involves careful planning and consideration. The design and analysis of algorithms aim to optimize their efficiency and accuracy. Design techniques, such as divide and conquer, dynamic programming, and greedy algorithms, help in solving complex problems. Additionally, the analysis of algorithms focuses on evaluating their time complexity and space complexity, providing insights into their efficiency.Section 6: Challenges and Ethical ConsiderationsWhile algorithms have numerous benefits, they also present challenges and ethical considerations. One significant challenge is the need for algorithms to handle large datasets, as processing massive amounts of data can be time-consuming andresource-intensive. Additionally, ethical concerns arise when algorithms are used for automated decision-making, such as in the criminal justice system or loan approvals, as biases anddiscrimination can be unintentionally embedded in the algorithms.Conclusion:Algorithms are the backbone of problem-solving in the world of computer science. They provide a systematic approach to process data and generate desired outcomes. Understanding different algorithm types, their design and analysis, and their significance in various domains is essential to harnessing the full potential of algorithms. As technology continues to advance, algorithms will continue to evolve and shape the world around us.。
算法导论习题答案 (1)

Introduction to Algorithms September 24, 2004Massachusetts Institute of Technology 6.046J/18.410J Professors Piotr Indyk and Charles E. Leiserson Handout 7Problem Set 1 SolutionsExercise 1-1. Do Exercise 2.3-7 on page 37 in CLRS.Solution:The following algorithm solves the problem:1.Sort the elements in S using mergesort.2.Remove the last element from S. Let y be the value of the removed element.3.If S is nonempty, look for z=x−y in S using binary search.4.If S contains such an element z, then STOP, since we have found y and z such that x=y+z.Otherwise, repeat Step 2.5.If S is empty, then no two elements in S sum to x.Notice that when we consider an element y i of S during i th iteration, we don’t need to look at the elements that have already been considered in previous iterations. Suppose there exists y j∗S, such that x=y i+y j. If j<i, i.e. if y j has been reached prior to y i, then we would have found y i when we were searching for x−y j during j th iteration and the algorithm would have terminated then.Step 1 takes �(n lg n)time. Step 2 takes O(1)time. Step 3 requires at most lg n time. Steps 2–4 are repeated at most n times. Thus, the total running time of this algorithm is �(n lg n). We can do a more precise analysis if we notice that Step 3 actually requires �(lg(n−i))time at i th iteration.However, if we evaluate �n−1lg(n−i), we get lg(n−1)!, which is �(n lg n). So the total runningi=1time is still �(n lg n).Exercise 1-2. Do Exercise 3.1-3 on page 50 in CLRS.Exercise 1-3. Do Exercise 3.2-6 on page 57 in CLRS.Exercise 1-4. Do Problem 3-2 on page 58 of CLRS.Problem 1-1. Properties of Asymptotic NotationProve or disprove each of the following properties related to asymptotic notation. In each of the following assume that f, g, and h are asymptotically nonnegative functions.� (a) f (n ) = O (g (n )) and g (n ) = O (f (n )) implies that f (n ) = �(g (n )).Solution:This Statement is True.Since f (n ) = O (g (n )), then there exists an n 0 and a c such that for all n √ n 0, f (n ) ←Similarly, since g (n )= O (f (n )), there exists an n � 0 and a c such that for allcg (n ). �f (n ). Therefore, for all n √ max(n 0,n Hence, f (n ) = �(g (n )).�()g n ,0← �),0c 1 � g (n ) ← f (n ) ← cg (n ).n √ n c � 0 (b) f (n ) + g (n ) = �(max(f (n ),g (n ))).Solution:This Statement is True.For all n √ 1, f (n ) ← max(f (n ),g (n )) and g (n ) ← max(f (n ),g (n )). Therefore:f (n ) +g (n ) ← max(f (n ),g (n )) + max(f (n ),g (n )) ← 2 max(f (n ),g (n ))and so f (n ) + g (n )= O (max(f (n ),g (n ))). Additionally, for each n , either f (n ) √max(f (n ),g (n )) or else g (n ) √ max(f (n ),g (n )). Therefore, for all n √ 1, f (n ) + g (n ) √ max(f (n ),g (n )) and so f (n ) + g (n ) = �(max(f (n ),g (n ))). Thus, f (n ) + g (n ) = �(max(f (n ),g (n ))).(c) Transitivity: f (n ) = O (g (n )) and g (n ) = O (h (n )) implies that f (n ) = O (h (n )).Solution:This Statement is True.Since f (n )= O (g (n )), then there exists an n 0 and a c such that for all n √ n 0, �)f ()n ,0← �()g n ,0← f (n ) ← cg (n ). Similarly, since g (n ) = O (h (n )), there exists an n �h (n ). Therefore, for all n √ max(n 0,n and a c � such thatfor all n √ n Hence, f (n ) = O (h (n )).cc�h (n ).c (d) f (n ) = O (g (n )) implies that h (f (n )) = O (h (g (n )).Solution:This Statement is False.We disprove this statement by giving a counter-example. Let f (n ) = n and g (n ) = 3n and h (n )=2n . Then h (f (n )) = 2n and h (g (n )) = 8n . Since 2n is not O (8n ), this choice of f , g and h is a counter-example which disproves the theorem.(e) f(n)+o(f(n))=�(f(n)).Solution:This Statement is True.Let h(n)=o(f(n)). We prove that f(n)+o(f(n))=�(f(n)). Since for all n√1, f(n)+h(n)√f(n), then f(n)+h(n)=�(f(n)).Since h(n)=o(f(n)), then there exists an n0such that for all n>n0, h(n)←f(n).Therefore, for all n>n0, f(n)+h(n)←2f(n)and so f(n)+h(n)=O(f(n)).Thus, f(n)+h(n)=�(f(n)).(f) f(n)=o(g(n))and g(n)=o(f(n))implies f(n)=�(g(n)).Solution:This Statement is False.We disprove this statement by giving a counter-example. Consider f(n)=1+cos(�≈n)and g(n)=1−cos(�≈n).For all even values of n, f(n)=2and g(n)=0, and there does not exist a c1for which f(n)←c1g(n). Thus, f(n)is not o(g(n)), because if there does not exist a c1 for which f(n)←c1g(n), then it cannot be the case that for any c1>0and sufficiently large n, f(n)<c1g(n).For all odd values of n, f(n)=0and g(n)=2, and there does not exist a c for which g(n)←cf(n). By the above reasoning, it follows that g(n)is not o(f(n)). Also, there cannot exist c2>0for which c2g(n)←f(n), because we could set c=1/c2if sucha c2existed.We have shown that there do not exist constants c1>0and c2>0such that c2g(n)←f(n)←c1g(n). Thus, f(n)is not �(g(n)).Problem 1-2. Computing Fibonacci NumbersThe Fibonacci numbers are defined on page 56 of CLRS asF0=0,F1=1,F n=F n−1+F n−2for n√2.In Exercise 1-3, of this problem set, you showed that the n th Fibonacci number isF n=�n−� n,�5where �is the golden ratio and �is its conjugate.A fellow 6.046 student comes to you with the following simple recursive algorithm for computing the n th Fibonacci number.F IB(n)1 if n=02 then return 03 elseif n=14 then return 15 return F IB(n−1)+F IB(n−2)This algorithm is correct, since it directly implements the definition of the Fibonacci numbers. Let’s analyze its running time. Let T(n)be the worst-case running time of F IB(n).1(a) Give a recurrence for T(n), and use the substitution method to show that T(n)=O(F n).Solution: The recurrence is: T(n)=T(n−1)+T(n−2)+1.We use the substitution method, inducting on n. Our Induction Hypothesis is: T(n)←cF n−b.To prove the inductive step:T(n)←cF n−1+cF n−2−b−b+1← cF n−2b+1Therefore, T(n)←cF n−b+1provided that b√1. We choose b=2and c=10.∗{For the base case consider n0,1}and note the running time is no more than10−2=8.(b) Similarly, show that T(n)=�(F n), and hence, that T(n)=�(F n).Solution: Again the recurrence is: T(n)=T(n−1)+T(n−2)+1.We use the substitution method, inducting on n. Our Induction Hypothesis is: T(n)√F n.To prove the inductive step:T(n)√F n−1+F n−2+1√F n+1Therefore, T(n)←F n. For the base case consider n∗{0,1}and note the runningtime is no less than 1.1In this problem, please assume that all operations take unit time. In reality, the time it takes to add two numbers depends on the number of bits in the numbers being added (more precisely, on the number of memory words). However, for the purpose of this problem, the approximation of unit time addition will suffice.Professor Grigori Potemkin has recently published an improved algorithm for computing the n th Fibonacci number which uses a cleverly constructed loop to get rid of one of the recursive calls. Professor Potemkin has staked his reputation on this new algorithm, and his tenure committee has asked you to review his algorithm.F IB�(n)1 if n=02 then return 03 elseif n=14 then return 15 6 7 8 sum �1for k�1to n−2do sum �sum +F IB�(k) return sumSince it is not at all clear that this algorithm actually computes the n th Fibonacci number, let’s prove that the algorithm is correct. We’ll prove this by induction over n, using a loop invariant in the inductive step of the proof.(c) State the induction hypothesis and the base case of your correctness proof.Solution: To prove the algorithm is correct, we are inducting on n. Our inductionhypothesis is that for all n<m, Fib�(n)returns F n, the n th Fibonacci number.Our base case is m=2. We observe that the first four lines of Potemkin guaranteethat Fib�(n)returns the correct value when n<2.(d) State a loop invariant for the loop in lines 6-7. Prove, using induction over k, that your“invariant” is indeed invariant.Solution: Our loop invariant is that after the k=i iteration of the loop,sum=F i+2.We prove this induction using induction over k. We assume that after the k=(i−1)iteration of the loop, sum=F i+1. Our base case is i=1. We observe that after thefirst pass through the loop, sum=2which is the 3rd Fibonacci number.To complete the induction step we observe that if sum=F i+1after the k=(i−1)andif the call to F ib�(i)on Line 7 correctly returns F i(by the induction hypothesis of ourcorrectness proof in the previous part of the problem) then after the k=i iteration ofthe loop sum=F i+2. This follows immediately form the fact that F i+F i+1=F i+2.(e) Use your loop invariant to complete the inductive step of your correctness proof.Solution: To complete the inductive step of our correctness proof, we must show thatif F ib�(n)returns F n for all n<m then F ib�(m)returns m. From the previous partwe know that if F ib�(n)returns F n for all n<m, then at the end of the k=i iterationof the loop sum=F i+2. We can thus conclude that after the k=m−2iteration ofthe loop, sum=F m which completes our correctness proof.(f) What is the asymptotic running time, T�(n), of F IB�(n)? Would you recommendtenure for Professor Potemkin?Solution: We will argue that T�(n)=�(F n)and thus that Potemkin’s algorithm,F ib�does not improve upon the assymptotic performance of the simple recurrsivealgorithm, F ib. Therefore we would not recommend tenure for Professor Potemkin.One way to see that T�(n)=�(F n)is to observe that the only constant in the programis the 1 (in lines 5 and 4). That is, in order for the program to return F n lines 5 and 4must be executed a total of F n times.Another way to see that T�(n)=�(F n)is to use the substitution method with thehypothesis T�(n)√F n and the recurrence T�(n)=cn+�n−2T�(k).k=1Problem 1-3. Polynomial multiplicationOne can represent a polynomial, in a symbolic variable x, with degree-bound n as an array P[0..n] of coefficients. Consider two linear polynomials, A(x)=a1x+a0and B(x)=b1x+b0, where a1, a0, b1, and b0are numerical coefficients, which can be represented by the arrays [a0,a1]and [b0,b1], respectively. We can multiply A and B using the four coefficient multiplicationsm1=a1·b1,m2=a1·b0,m3=a0·b1,m4=a0·b0,as well as one numerical addition, to form the polynomialC(x)=m1x2+(m2+m3)x+m4,which can be represented by the array[c0,c1,c2]=[m4,m3+m2,m1].(a) Give a divide-and-conquer algorithm for multiplying two polynomials of degree-bound n,represented as coefficient arrays, based on this formula.Solution:We can use this idea to recursively multiply polynomials of degree n−1, where n isa power of 2, as follows:Let p(x)and q(x)be polynomials of degree n−1, and divide each into the upper n/2 and lower n/2terms:p(x)=a(x)x n/2+b(x),q(x)=c(x)x n/2+d(x),where a(x), b(x), c(x), and d(x)are polynomials of degree n/2−1. The polynomial product is thenp(x)q(x)=(a(x)x n/2+b(x))(c(x)x n/2+d(x))=a(x)c(x)x n+(a(x)d(x)+b(x)c(x))x n/2+b(x)d(x).The four polynomial products a(x)c(x), a(x)d(x), b(x)c(x), and b(x)d(x)are computed recursively.(b) Give and solve a recurrence for the worst-case running time of your algorithm.Solution:Since we can perform the dividing and combining of polynomials in time �(n), recursive polynomial multiplication gives us a running time ofT(n)=4T(n/2)+�(n)=�(n2).(c) Show how to multiply two linear polynomials A(x)=a1x+a0and B(x)=b1x+b0using only three coefficient multiplications.Solution:We can use the following 3 multiplications:m1=(a+b)(c+d)=ac+ad+bc+bd,m2=ac,m3=bd,so the polynomial product is(ax+b)(cx+d)=m2x2+(m1−m2−m3)x+m3.� (d) Give a divide-and-conquer algorithm for multiplying two polynomials of degree-bound nbased on your formula from part (c).Solution:The algorithm is the same as in part (a), except for the fact that we need only compute three products of polynomials of degree n/2 to get the polynomial product.(e) Give and solve a recurrence for the worst-case running time of your algorithm.Solution:Similar to part (b):T (n )=3T (n/2) + �(n )lg 3)= �(n �(n 1.585)Alternative solution Instead of breaking a polynomial p (x ) into two smaller polynomials a (x ) and b (x ) such that p (x )= a (x ) + x n/2b (x ), as we did above, we could do the following:Collect all the even powers of p (x ) and substitute y = x 2 to create the polynomial a (y ). Then collect all the odd powers of p (x ), factor out x and substitute y = x 2 to create the second polynomial b (y ). Then we can see thatp (x ) = a (y ) + x b (y )· Both a (y ) and b (y ) are polynomials of (roughly) half the original size and degree, and we can proceed with our multiplications in a way analogous to what was done above.Notice that, at each level k , we need to compute y k = y 2 (where y 0 = x ), whichk −1 takes time �(1) per level and does not affect the asymptotic running time.。
算法导论第4版英文版

Title: Introduction to Algorithms, Fourth Edition (English Version)The fourth edition of Introduction to Algorithms, also known as "CLRS" among its legion of fans, is a comprehensive guide to the theory and practice of algorithms. This English version, targeted at a global audience, builds upon the legacy of its predecessors, firmly establishing itself as the standard reference in the field.The book's unparalleled reputation is founded on its ability to bridge the gap between theory and practice, making even the most complex algorithm accessible to a wide audience. Coverage ranges from fundamental data structures and sorting algorithms to more advanced topics like graph algorithms, dynamic programming, and computational geometry.The fourth edition boasts numerous updates and improvements over its predecessors. It includes new algorithms and techniques, along with expanded discussions on existing ones. The updated material reflects the latest research and best practices in the field, making this edition not just a sequel but a complete reboot of the text.The book's hallmark approach combines mathematical rigor with practical implementation, making it an invaluable resource for students, researchers, and professionals alike. Each chapter is meticulously crafted, introducing key concepts through carefully chosen examples and exercises. The accompanyingonline resources also provide additional challenges and solutions, further enhancing the learning experience.In conclusion, Introduction to Algorithms, Fourth Edition (English Version) is more than just a textbook; it's a roadmap to understanding the intricacies of algorithms. Its comprehensive nature and timeless quality make it a must-have for anyone serious about mastering the art and science of algorithm design.。
算法的英语学习计划

算法的英语学习计划IntroductionLearning a new language can be challenging, especially when it comes to algorithm, which is a specific technical language used in computer science and programming. However, with the right learning plan and dedication, anyone can master algorithms. In this learning plan, we will discuss the steps and resources that can help you become proficient in algorithms.Step 1: Understanding the BasicsThe first step in learning algorithms is to understand the basics. This includes familiarizing yourself with the different types of algorithms, such as sorting, searching, and graph algorithms. You should also learn about key algorithm concepts, such as time complexity, space complexity, and the Big-O notation.To accomplish this, you can start by reading introductory books and watching online tutorials that explain the fundamental concepts of algorithms. For example, "Introduction to Algorithms" by Thomas H. Cormen is a popular book that provides a comprehensive overview of the subject.Step 2: Practice, Practice, PracticeOnce you have a solid understanding of the basics, the next step is to practice solving algorithm problems. This can be done through online coding platforms, such as LeetCode, HackerRank, or CodeSignal, which offer a wide range of algorithm problems for practice. It's important to start with simple problems and gradually move on to more complex ones as you gain confidence and skills.In addition to practicing on coding platforms, you can also participate in algorithm competitions, such as ACM-ICPC or Google Code Jam. These competitions provide an excellent opportunity to challenge yourself and gain exposure to different types of algorithm problems.Step 3: Learn Data StructuresIn addition to algorithms, it's important to have a solid understanding of data structures, as they are closely related to algorithms. Data structures, such as arrays, linked lists, stacks, queues, trees, and graphs, are used to store and organize data efficiently, which is essential for developing optimized algorithms.To learn data structures, you can refer to books like "Data Structures and Algorithms" by Michael T. Goodrich and Roberto Tamassia. Additionally, you can use online resources, such as tutorials and video lectures, to supplement your learning.Step 4: Explore Advanced TopicsWith a strong foundation in algorithms and data structures, you can begin exploring advanced topics in the field. This may include topics such as dynamic programming, greedy algorithms, divide and conquer, and graph algorithms, among others. Delving into these advanced topics will help you develop a deeper understanding of how algorithms work and how to design efficient solutions to complex problems.To explore advanced topics, you can refer to books like "Algorithms" by Robert Sedgewick and Kevin Wayne, which covers a wide range of advanced algorithms and data structures. You can also explore online resources, such as MOOCs (Massive Open Online Courses) offered by platforms like Coursera, edX, and Khan Academy.Step 5: Project-Based LearningTo solidify your understanding of algorithms and data structures, consider undertaking a project-based learning approach. This could involve working on real-world problems, such as developing a recommendation system, optimizing a search algorithm, or implementing a data compression algorithm.By working on projects, you will not only apply your knowledge of algorithms and data structures but also gain practical experience in problem-solving and software development. Additionally, you may collaborate with other learners or participate in open-source projects, which can provide valuable feedback and exposure to different perspectives.Step 6: Continuous LearningLearning algorithms is an ongoing process, and it's important to stay up-to-date with the latest developments in the field. This could involve attending conferences, workshops, and seminars related to algorithms and data structures. It's also beneficial to network with professionals working in the field and stay connected with online communities, such as Stack Overflow, Reddit, and GitHub.Additionally, you can explore specialized areas within algorithms, such as machine learning algorithms, cryptography algorithms, or bioinformatics algorithms. This will help you broaden your knowledge and open up new opportunities for learning and application.ConclusionIn conclusion, learning algorithms is a rewarding and challenging endeavor that requires dedication and persistence. By following the steps outlined in this learning plan and leveraging the recommended resources, you can develop a strong foundation in algorithms and data structures. With continuous learning and practice, you can become proficient in designing, analyzing, and implementing efficient algorithms for solving a wide range of computational problems. Remember that learning algorithms takes time and effort, but the rewards are well worth it. Good luck on your algorithm learning journey!。
Introduction to Genetic Algorithms
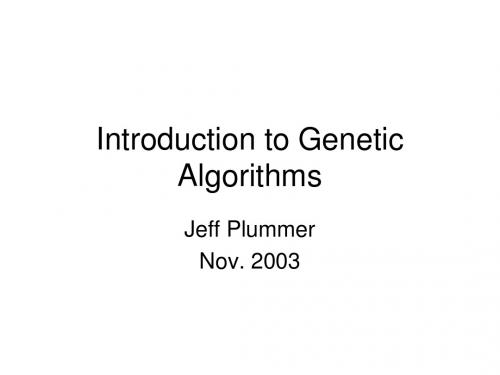
Jeff Plummer Nov. 2003
Background
• Problems are solved by an evolutionary process resulting in a best (fittest) solution (survivor). • Evolutionary Computing
– Chromosome encoding is string of “Up, Down, Left, Right, Accelerate, Decelerate” – Fitness of “Chromosome” is proportional to the length of time it can evade a player. – As player plays, ships get better at evading. – DOESN’T REQUIRE A LIVE PLAYER!!!!!!
4. 5. 6.
Example Applet
• /alife/english/gavintr gb.html
GAs and Games
• GAs are learning systems • Consider a Space Ship AI - Evade
Simple Example
• f(x) = {MAX(x2): 0 <= x <= 32 } • Encode Solution: Just use 5 bits (1 or 0). • Generate initial population.
A 0 B 1 C 0 D 1 1 1 1 0 1 0 0 0 0 0 0 1 1 0 0 1
数据结构经典书籍

数据结构经典书籍数据结构是计算机科学中的重要概念,用于组织和管理数据的方式。
它是每个程序员都应该掌握的基础知识之一。
为了帮助读者更好地了解和学习数据结构,以下是一些经典的数据结构书籍的介绍和推荐。
1.《算法导论》(Introduction to Algorithms)这本书是数据结构和算法领域的权威之作,由Thomas H. Cormen等人合著。
书中详细介绍了各种经典的数据结构和算法,包括数组、链表、栈、队列、树、图等等。
每个主题都有清晰的描述、代码实现和复杂度分析。
这本书通过深入浅出的方式,循序渐进地讲解了数据结构和算法的基本概念和原理,适合初学者和有一定编程经验的读者。
2.《算法(第四版)》(Algorithms, Part I)这本书由Robert Sedgewick和Kevin Wayne合著,结合了在线教学课程的内容。
它详细讲解了各种数据结构和算法的实现和应用,包括排序算法、树、图和字符串处理。
书中每个章节都提供了大量的示例和练习题,帮助读者加深理解。
此外,它还介绍了一些高级主题,如动态规划和贪婪算法。
这本书对于有一定编程基础的读者非常适合。
3.《数据结构与算法分析:C语言描述》(Data Structures and Algorithm Analysis in C)这本书由Mark Allen Weiss编写,是一本广受欢迎的数据结构教材。
它以C语言为基础,详细介绍了各种数据结构和算法的实现和分析。
书中充满了清晰的图表和实例代码,读者通过实际的编程练习,可以更好地理解和掌握数据结构的知识。
此外,书中还包含了一些高级主题,如平摊分析和哈希表,对于进一步学习数据结构的读者也提供了很好的指导。
4.《算法:乐趣、挑战与智慧》(Algorithms: Fun, Challenges and Wisdom)这本书由Alfred V. Aho,Jeffrey D. Ullman和John E. Hopcroft合写,以趣味性和挑战性的方式介绍了算法设计和数据结构。
最好的计算机算法的书籍
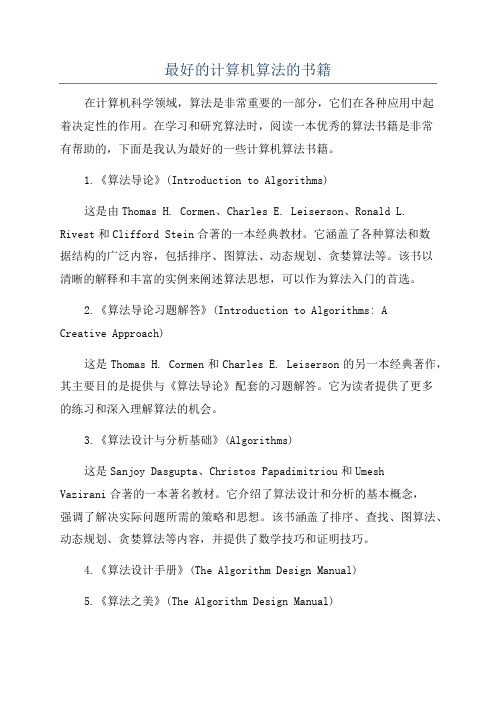
最好的计算机算法的书籍在计算机科学领域,算法是非常重要的一部分,它们在各种应用中起着决定性的作用。
在学习和研究算法时,阅读一本优秀的算法书籍是非常有帮助的,下面是我认为最好的一些计算机算法书籍。
1.《算法导论》(Introduction to Algorithms)这是由Thomas H. Cormen、Charles E. Leiserson、Ronald L. Rivest和Clifford Stein合著的一本经典教材。
它涵盖了各种算法和数据结构的广泛内容,包括排序、图算法、动态规划、贪婪算法等。
该书以清晰的解释和丰富的实例来阐述算法思想,可以作为算法入门的首选。
2.《算法导论习题解答》(Introduction to Algorithms: ACreative Approach)这是Thomas H. Cormen和Charles E. Leiserson的另一本经典著作,其主要目的是提供与《算法导论》配套的习题解答。
它为读者提供了更多的练习和深入理解算法的机会。
3.《算法设计与分析基础》(Algorithms)这是Sanjoy Dasgupta、Christos Papadimitriou和UmeshVazirani合著的一本著名教材。
它介绍了算法设计和分析的基本概念,强调了解决实际问题所需的策略和思想。
该书涵盖了排序、查找、图算法、动态规划、贪婪算法等内容,并提供了数学技巧和证明技巧。
4.《算法设计手册》(The Algorithm Design Manual)5.《算法之美》(The Algorithm Design Manual)这是Jon Kleinberg和Éva Tardos合著的一本优秀教材,它着重介绍了算法设计和分析的关键思想。
该书以生动的方式讲解了算法的应用和影响,帮助读者理解算法如何解决实际问题。
此外,该书还包含了丰富的实例和习题,帮助读者巩固所学知识。
6.《算法设计师手记》(The Algorithm Designers Manual)这是Steven S. Skiena撰写的一本实用参考手册,它提供了大量的算法实现代码和解决问题的思路。
算法可以快速完成用户与信息的精准匹配英语作文

算法可以快速完成用户与信息的精准匹配英语作文全文共3篇示例,供读者参考篇1Algorithms: The Digital Matchmakers of the Modern AgeIn today's digital age, we are constantly bombarded with an overwhelming amount of information. From social media feeds to online shopping platforms, the internet has become a vast ocean of data, and navigating through it can be a daunting task. However, thanks to the power of algorithms, we now have the ability to quickly and accurately match users with the information they need, making the online experience more efficient and personalized.As a student, I have experienced firsthand the benefits of algorithmic matching in various aspects of my academic life. Whether it's finding relevant research papers for my assignments or discovering new learning resources tailored to my interests, algorithms have proven to be invaluable tools in enhancing my educational journey.One area where algorithmic matching shines is in online learning platforms. These platforms employ sophisticatedalgorithms that analyze a student's performance, learning style, and interests to recommend personalized content and courses. By presenting material that aligns with a student's strengths and weaknesses, these algorithms can significantly improve the learning experience and increase knowledge retention.For example, when I was struggling with a particular concept in my calculus class, the online learning platform I was using recommended a series of videos and practice problems specifically designed to address my areas of weakness. The algorithm had analyzed my previous performance and identified the topics I needed to focus on, allowing me to efficiently target my weaknesses and improve my understanding of the subject.Another powerful application of algorithmic matching is in the realm of online research. As a student, conducting research is a crucial part of my academic life, and navigating through the vast expanse of scholarly literature can be a daunting task. However, with the help of algorithms, I can quickly find relevant and reliable sources that align with my research interests and requirements.One particularly useful tool is citation recommendation algorithms. These algorithms analyze the content and citations of research papers I have previously accessed and suggestadditional relevant sources based on their similarity to my existing material. This feature has saved me countless hours of manual searching and has allowed me to explore new perspectives and ideas that I might have otherwise missed.Beyond academia, algorithmic matching has also transformed the way we consume entertainment and make purchasing decisions. Streaming platforms like Netflix and Spotify use complex algorithms to analyze our viewing and listening habits, and then recommend content that aligns with our preferences. This personalized approach ensures that we are exposed to new and engaging content that we are likely to enjoy, saving us from the overwhelming task of sifting through countless options.Similarly, e-commerce platforms like Amazon employ algorithmic matching to provide personalized product recommendations based on our browsing and purchase history. These algorithms analyze our past behavior and preferences to suggest items that we may be interested in, streamlining the shopping experience and increasing the likelihood of making satisfactory purchases.While the benefits of algorithmic matching are undeniable, it is important to acknowledge potential concerns and limitations.One major issue is the potential for algorithmic bias, where the algorithms may perpetuate existing societal biases or prioritize certain types of content over others. Additionally, there are privacy concerns surrounding the collection and use of personal data to fuel these algorithms.Despite these challenges, the potential of algorithms to enhance our online experiences and streamline information access is immense. As technology continues to evolve, we can expect to see even more sophisticated and advanced algorithms that can adapt to our changing needs and preferences, further blurring the lines between the digital and physical worlds.In conclusion, algorithms have become the digital matchmakers of the modern age, seamlessly connecting us with the information, entertainment, and products that align with our unique interests and preferences. As a student, I have experienced firsthand the transformative power of algorithmic matching in enhancing my educational journey and streamlining my research process. While there are valid concerns to address, the benefits of these algorithms are undeniable, and their impact on our lives will only continue to grow in the years to come.篇2Algorithms: The Digital Matchmakers of the Modern AgeIn today's world, we are constantly bombarded with a deluge of information from countless sources. From social media updates to online shopping recommendations, targeted advertising to personalized news feeds – it's as if the digital realm has become a colossal matchmaking service, endlessly striving to pair us with the content and connections most aligned with our interests and preferences. At the heart of this intricate matchmaking process lie powerful algorithms – the unseen puppet masters orchestrating the flow of information in our digitally-driven lives.As a student navigating the complexities of the 21st century, I have come to appreciate the pivotal role these algorithms play in streamlining our access to information. Gone are the days of sifting through encyclopedias or endlessly scrolling through pages of irrelevant search results. Today's algorithms possess an almost clairvoyant ability to anticipate our needs and curate the information we seek with astonishing precision.Take, for instance, the realm of online shopping.E-commerce giants like Amazon and Alibaba have harnessed the power of sophisticated algorithms to revolutionize the shopping experience. By analyzing our browsing histories, purchasepatterns, and even geographic locations, these algorithms can predict our preferences with uncanny accuracy. The result? A personalized storefront tailored to our unique tastes, seamlessly guiding us towards products we're likely to appreciate – a true testament to the art of algorithmic matchmaking.But the influence of algorithms extends far beyond the realm of retail therapy. Social media platforms like Facebook, Twitter, and Instagram employ intricate algorithms to curate our news feeds, serving us a carefully curated selection of updates, posts, and advertisements based on our online activities, social connections, and expressed interests. These algorithms act as digital curators, filtering out the noise and presenting us with the content most resonant with our personalities and inclinations.Even in the academic realm, algorithms have become indispensable tools for enhancing our learning experiences. Online educational platforms and digital libraries leverage algorithms to recommend relevant courses, research materials, and supplementary resources based on our academic profiles, previous searches, and demonstrated areas of interest. These algorithmic matchmakers ensure that the vast expanse of knowledge at our fingertips is tailored to our unique educationaljourneys, empowering us to explore and expand our intellectual horizons with unparalleled efficiency.Underpinning these remarkable feats of algorithmic matchmaking are intricate mathematical models and machine learning techniques. By ingesting vast troves of data, these algorithms can discern patterns, correlations, and nuances that would be virtually impossible for the human mind to comprehend. Through a process of continuous learning and refinement, they evolve, adapting their recommendations and output to better align with our ever-changing preferences and behaviors.Yet, as with any powerful technology, the use of algorithms in user-information matching is not without its controversies and ethical considerations. Issues of data privacy, algorithmic bias, and the potential for manipulation have sparked heated debates and regulatory scrutiny. Critics argue that these algorithms can perpetuate societal biases, reinforce echo chambers, and compromise our autonomy by shaping our choices and perspectives in ways we may not even realize.Nonetheless, the potential benefits of algorithmic matchmaking are too significant to ignore. In a world where information overload is a constant struggle, these algorithms actas digital concierges, curating and delivering the most pertinent and engaging content to our digital doorsteps. They empower us to navigate the vast expanse of the internet with unprecedented efficiency, connecting us with the information, products, and communities that enrich our lives and foster personal growth.As we look to the future, it is clear that the role of algorithms in user-information matching will only grow more pervasive and sophisticated. The advent of advanced technologies like quantum computing and neural networks promises to usher in a new era of algorithmic prowess, enabling even more nuanced and personalized matchmaking capabilities.However, it is incumbent upon us, as users and beneficiaries of these technologies, to remain vigilant and engaged. We must demand transparency, accountability, and ethical oversight to ensure that these algorithms serve our best interests without compromising our fundamental rights and values. By striking the right balance between harnessing the power of algorithmic matchmaking and safeguarding our individual autonomy, we can unlock a future where information flows seamlessly, tailored to our unique needs and aspirations, empowering us to thrive in an ever-evolving digital landscape.In the end, algorithms are not mere lines of code or mathematical equations; they are the digital matchmakers of our modern age, deftly orchestrating the intricate dance between users and information. As we navigate this brave new world, let us embrace the remarkable potential of these algorithms while remaining steadfast in our commitment to using them responsibly and ethically, ensuring that they enhance our lives rather than dictate them.篇3Algorithms: The Key to Efficient User-Information MatchingIn today's world, we are constantly bombarded with an overwhelming amount of information from various sources. Whether it's news articles, social media posts, online videos, or product recommendations, the sheer volume of data can be daunting. However, thanks to the power of algorithms, we can now navigate this vast ocean of information with relative ease, allowing us to find what we need quickly and accurately.As a student, I have experienced firsthand the immense benefits of algorithms in my academic journey. From research papers to online courses, algorithms have played a crucial role incurating and delivering relevant information tailored to my needs and interests.One area where algorithms excel is in personalized learning. Online educational platforms like Coursera, edX, and Khan Academy use sophisticated algorithms to analyze a student's progress, strengths, and weaknesses. Based on this data, they can recommend courses, supplementary materials, and even adjust the difficulty level to ensure an optimal learning experience. This personalized approach not only enhances our understanding but also keeps us engaged and motivated throughout the learning process.Moreover, algorithms have revolutionized the way we search for information on the internet. Search engines like Google and Bing use complex algorithms to crawl, index, and rank web pages based on their relevance to our search queries. These algorithms consider various factors, such as keyword density, page authority, and user behavior, to provide us with the most relevant and trustworthy results. Without these algorithms, we would be lost in the vast expanse of the World Wide Web, unable to find the information we seek efficiently.Another area where algorithms have made a significant impact is in recommendation systems. Online platforms likeAmazon, Netflix, and Spotify use collaborative filtering and content-based algorithms to analyze our preferences, browsing history, and ratings to suggest products, movies, or music that we might enjoy. These recommendations not only save us time but also introduce us to new and exciting options that we might have otherwise missed.Furthermore, algorithms play a crucial role in targeted advertising. While some may view this as intrusive, it's undeniable that algorithms have made advertising more relevant and effective. By analyzing our browsing behavior, location data, and demographic information, algorithms can serve us ads for products or services that align with our interests and needs. This not only benefits businesses by increasing their chances of conversion but also enhances the user experience by reducing irrelevant and annoying advertisements.However, it's important to acknowledge the potential drawbacks and ethical considerations surrounding the use of algorithms. Privacy concerns, algorithmic bias, and the perpetuation of filter bubbles are valid issues that need to be addressed. As users, we must remain vigilant and critical when interacting with algorithmically curated content, ensuring that we are not being unduly influenced or manipulated.Nevertheless, the benefits of algorithms in matching users with relevant information cannot be overstated. They have transformed the way we learn, search, and consume information, making our lives more efficient and enriching our experiences.As a student, I have witnessed firsthand how algorithms have enhanced my academic journey. From personalized learning platforms to targeted research recommendations, algorithms have consistently provided me with the information I need, when I need it. This has not only saved me countless hours of sifting through irrelevant data but has also enabled me to explore new avenues of knowledge that I might have otherwise missed.Moreover, algorithms have facilitated collaboration and knowledge sharing among students and academics. Online forums, discussion boards, and social media platforms use algorithms to surface relevant discussions, research papers, and expert insights, fostering a vibrant and interconnectedacademic community.Looking ahead, the future of algorithms in user-information matching is promising. With the advent of artificial intelligence (AI) and machine learning, algorithms will become even more sophisticated and personalized. They will be able to understandand anticipate our needs more accurately, providing us with tailored information before we even realize we need it.However, as algorithms become more powerful, it is crucial that we address the ethical and societal implications of their use. We must ensure that algorithms are transparent, unbiased, and accountable, and that our privacy and autonomy are protected.In conclusion, algorithms have transformed the way we interact with information, enabling us to navigate the vast expanse of data efficiently and accurately. As students, we have benefited tremendously from personalized learning, targeted research recommendations, and collaborativeknowledge-sharing platforms, all powered by algorithms. While there are valid concerns about privacy, bias, and manipulation, the benefits of algorithms in user-information matching are undeniable. As we move forward, it is our responsibility to embrace the power of algorithms while addressing their potential drawbacks, ensuring that they serve our best interests and enhance our lives in meaningful ways.。
分子动力学 书籍

分子动力学书籍分子动力学是研究物质微观粒子运动规律和力学性质的科学方法。
它具有广泛的应用领域,包括材料科学、化学、生物学等。
对于想要深入了解和掌握分子动力学的读者来说,选择一本好的分子动力学书籍是非常重要的。
本文将介绍几本优秀的分子动力学书籍,帮助读者选择适合自己的学习工具。
一、《Understanding Molecular Simulation: From Algorithms to Applications》这本由Daan Frenkel和Berend Smit合著的书是一本经典之作,全面而详细地介绍了分子模拟的基本原理、算法和应用。
书中内容系统且条理清晰,对于从零开始学习分子动力学的读者非常友好。
作者以通俗易懂的语言介绍了分子动力学的基础理论和相关技术,并通过大量的实例和案例深入展示了分子动力学在各个领域的应用。
此外,书中还涵盖了更高级的方法和技巧,适合已经有一定基础并希望深入研究的读者。
二、《An Introduction to Computational Physics》这本由Tao Pang编写的书籍是一本面向物理学和工程学背景的读者的入门指南。
书中从分子动力学的基本原理开始,引导读者逐步学习和理解分子动力学的核心概念。
作者通过清晰的逻辑结构和丰富的例子,将复杂的数学和物理概念用简洁而易懂的语言解释,并通过编程实践帮助读者提升分子动力学的模拟和分析能力。
这本书的特点是理论与实践相结合,适合希望通过实际操作来深入了解并掌握分子动力学的读者。
三、《Molecular Dynamics Simulation: Elementary Methods》该书是一本由J. M. Haile撰写的经典教材,适合那些希望深入了解和学习分子动力学基础原理的读者。
作者通过系统而详细的阐述,全面介绍了分子动力学中的关键概念和基本方法,包括力场、积分算法和统计力学等方面。
书中的数学推导相对简洁明了,语言表达流畅,让读者更容易理解和掌握分子动力学的核心内容。
ACM算法书籍推荐收藏
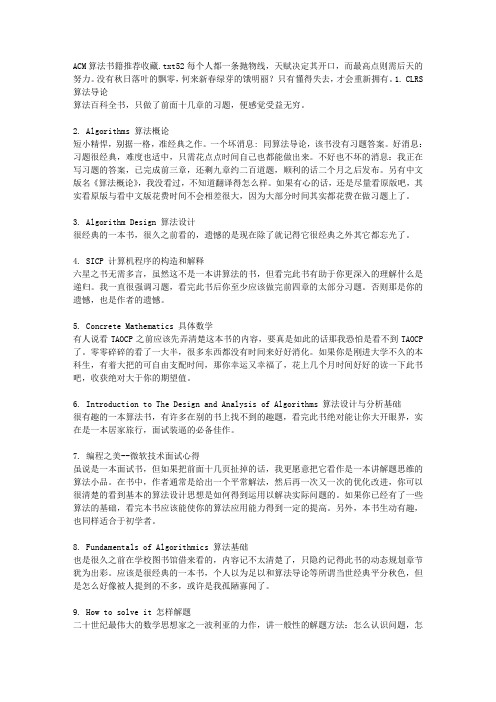
ACM算法书籍推荐收藏.txt52每个人都一条抛物线,天赋决定其开口,而最高点则需后天的努力。
没有秋日落叶的飘零,何来新春绿芽的饿明丽?只有懂得失去,才会重新拥有。
1. CLRS 算法导论算法百科全书,只做了前面十几章的习题,便感觉受益无穷。
2. Algorithms 算法概论短小精悍,别据一格,准经典之作。
一个坏消息: 同算法导论,该书没有习题答案。
好消息:习题很经典,难度也适中,只需花点点时间自己也都能做出来。
不好也不坏的消息:我正在写习题的答案,已完成前三章,还剩九章约二百道题,顺利的话二个月之后发布。
另有中文版名《算法概论》,我没看过,不知道翻译得怎么样。
如果有心的话,还是尽量看原版吧,其实看原版与看中文版花费时间不会相差很大,因为大部分时间其实都花费在做习题上了。
3. Algorithm Design 算法设计很经典的一本书,很久之前看的,遗憾的是现在除了就记得它很经典之外其它都忘光了。
4. SICP 计算机程序的构造和解释六星之书无需多言,虽然这不是一本讲算法的书,但看完此书有助于你更深入的理解什么是递归。
我一直很强调习题,看完此书后你至少应该做完前四章的太部分习题。
否则那是你的遗憾,也是作者的遗憾。
5. Concrete Mathematics 具体数学有人说看TAOCP之前应该先弄清楚这本书的内容,要真是如此的话那我恐怕是看不到TAOCP 了。
零零碎碎的看了一大半,很多东西都没有时间来好好消化。
如果你是刚进大学不久的本科生,有着大把的可自由支配时间,那你幸运又幸福了,花上几个月时间好好的读一下此书吧,收获绝对大于你的期望值。
6. Introduction to The Design and Analysis of Algorithms 算法设计与分析基础很有趣的一本算法书,有许多在别的书上找不到的趣题,看完此书绝对能让你大开眼界,实在是一本居家旅行,面试装逼的必备佳作。
7. 编程之美--微软技术面试心得虽说是一本面试书,但如果把前面十几页扯掉的话,我更愿意把它看作是一本讲解题思维的算法小品。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
From algorithms to generative grammar and back againJohn GoldsmithThe University of Chicago1. IntroductionA few words of warning. I was asked to offer a contribution to this meeting on the application of linguistic theory. I’m not sure I know enough about this question to provide any new insights into applications, but I have been working for several years on a project which involves language, linguistics, and computation, and which has some very applied sides to it: a system for automatically learning the morphological structure of a language, given a large enough sample of data from the language. Working on this project has changed in varying degrees my view of what linguistic theory is, could be, and should be, and so since this is my own personal experience that I will recount, I might have something to contribute to a more general discussion.I have been interested for quite some time in the possibility and the implementation of computer systems that deal with natural language. Now, natural language is (in the opinion of linguists) the natural domain of linguists and of linguistics, and I have observed with great interest the successes that have occurred as linguistic ideas have been reincarnated in computational form, and have worried when linguistic notions have failed to make the transition to computational form. The remarks that follow are a series of reflections on why some of those transitions – from theory to computational application – have happened and why some have not. In the second half especially, these remarks are unquestionably (and unapologetically!) personal and idiosyncratic.In the first part of the talk, I will review and explore some of the natural connections between generative grammar and computational implementation, connections that relate to the fundamental notion of the algorithm. Generative grammar is, more than it is anything else, a plea for the case that an insightful theory of language can be based on algorithmic explanation.But there are a lot of challenges faced by anyone wishing to implement generative models computationally.2. The algorithm as explicansThe first idea I would like to explore is the notion that the greatest changes in linguistics have occurred not through the discovery of new facts or theories, but through the rise and dissemination of new visions of what counts as an explanation in linguistics. In the final analysis, this is what keeps apart linguists who come from separate camps. That is, it’s not what one believes to be true or false about language that separates linguists so much as it is beliefs about what counts as an explanation that separates linguists. I will try tomake that statement plausible by sketching the rise of a few such schools of thought in linguistics.No one is more aware than we linguists are at the variety there have been of major approaches to the systematic study of language. The 19th century was dominated by the study of language as a system in time – in human history, in fact. What it meant to explain something (such as a word, a sound, or a language), in the context of 19th century linguistics, was to give a clear and detailed account of how it came to be, given an earlier account of the language and a pattern of how things changed over time. We can explain why French has the word oui for ‘yes’ while Occitan has oc for ‘yes’ if we understand that both developed out of Latin hoc, and in the North of France the final k was dropped, and the remaining o- had a encliticized il attached to it, oïl eventually evolving to modern-day oui. Linguistics came to understand the relationship of the European languages, and later the languages of other parts of the world, as part of a small number of genealogical trees.The 20th century saw not just new ways of analyzing language, but new ways of offering explanations for things that were observed. Synchronic, structural linguistics came into being, often associated with de Saussure’s teaching, and it offered a new kind of explanation. Take phonemics, the flagship theory within structural linguistics: it could offer an account of why a flap appears in American English in the word Ítaly but not the word Itálian, and this despite the fact that the words are closely related. The explanation lies in the organization of the environments appropriate for the two allophones, an explanation that is in no way dependent on a historical account of the language or the words. It is perhaps unnecessary to remind a gathering of linguists that this was linguistics’ greatest contribution to the outside world: the discovery of a method of analysis of a cultural data (here, language), which linguists called phonemic analysis, which was both rigorous and sensitive to details at the human level, and which analyzed language in an ahistorical fashion. One of the by-products of the creation of the synchronic phonemic method was modern linguistics as we know it today.Other kinds of explanation have been offered for linguistic structures during this period as well, notably psychological explanation and sociological explanation. Over the last several decades, linguists have tended to call themselves functionalists who believe that an explanation of a linguistic generalization must flow from a generalization about the psychological character and mechanisms of human beings; and while many linguists who are not functionalists would say that they are describing or analyzing the human mind, it is only the functionalists who see the flow of explanation – from explainer to explained, from explicans to explicandum – as coming from principles that are established using the methods of the psychology profession. (That predilection has a long history, and was once known as psychologism.) Many prominent linguists subscribe to this point of view to one degree or another, I would say, including Talmy Givón, Scott DeLancey,1 perhaps Susumu Kuno, on some days George Lakoff, Joan Bybee, Jack Hawkins, and many others.1 Two linguists associated with the University of Oregon: in fact, their website notes, “Our department as a whole firmly believes that the patterns of language can ultimately be explained with reference to eitherAnother form of explanation is offered by linguists who see language as a social phenomenon, and hence strongly influenced by the principles that govern the interaction of human individuals operating within social organizations. Such linguists have been driven to understand the differences between the way men and women speak, those between the ways adolescents and adults speak, and the relationship between the class structure in society and the engines of linguistic change, but more important (for my purposes) than the nature of the questions they pursue is the kind of explanation that they offer, explanation that is based on what we know about the way humans behave in the context of social norms and expectations.But perhaps the most momentous shift in what counted as an explanation in linguistics arose at mid-century, and is generally associated with Noam Chomsky’s revolutionary work. Chomsky championed the notion that what the linguist needed to offer as an explanation was a formal description of the linguistic facts, a description that was formal and detailed to a degree that no human intervention is needed to apply the description appropriate to the facts (which is to say, nothing more than mechanical means is necessary). What counted as an explanation, according to this new perspective, was an algorithm (though, oddly enough, I don’t recall the term ever being used by Chomsky). This is an important notion, one whose history will matter to us, so we will take a look at it in a moment. But it was Chomsky’s central idea, one that he put at the definitional core of what he called generative grammar: as Chomsky wrote in the introduction to The Logical Structure of Linguistic Theory (1975),“conventional structuralist grammars or traditional grammars do notattempt to determine explicitly the sentences of a language or thestructural descriptions of these sentences. Rather, such grammarsdescribe elements and categories of various types, and provideexamples and hints to enable the intelligent reader to determine theform and structure of sentences not actually presented in the grammar.Such grammars are written for the intelligent reader. To determinewhat they say about sentences one must have an intuitive grasp ofcertain principles of linguistic structure. These principles, whichremain implicit and unexpressed, are presupposed in the construction and interpretation of such grammars. While perhaps perfectlyadequate for their particular purposes, such grammars do not attemptto account for the ability of the intelligent reader to understand thegrammar. The theory of generative grammar, in contrast, is concerned precisely to make explicit the “contribution of the intelligent reader,”though the problem is not posed in just these terms.” (8-9)cognitive functions of communication or to universals in the evolution of grammar, with the patterns of evolution themselves driven mostly by the cognitive functions of communication.” See/But why?Why is such a goal the best goal for an explanatory theory of language, or even just a reasonable goal? Coming up with an answer to that question involves going back in time to the development of the notion of the algorithm, and seeing what questions it was that its development was intended to solve, and what problems it did in fact solve.3. Generative model as algorithmThe term algorithm in modern English derives from theMiddle English algorism, itself deriving from the name Al-Khowarizmi, a mathematician who lived in the court ofMamun in Baghdad, in the early 9th century. Themathematician’s name was Abu Ja’far Mohammed ibn MusaAl-khowarizmi. (Ah! We still find historical accounts to be akind of explanation, don’t we?) He left for posterity twobooks, one entitled Hisab al-jabr wál-muqabala, “Thecalculation of reduction and restoration,” translated (andtransliterated) three hundred years later into Latin (by Robertof Chester) as Liber algebrae et almucabala, a transliterationthat bestowed the word algebra on the West. Al-Khowarizmi’s other book was translated into Latin with the title Liber Algorismi de numero Indorum, and it is from this word that the word algorism, later algorithm, arose.2 As the title suggests, it deals with the revolutionary idea of writing numbers with just 10 digits, from 0 to 9, and having at one end a 1’s place, then a 10’s place, a 100’s place, and so forth – a notion that has truly changed the world.Loosely speaking, an algorithm is an explicit and step-by-step explanation of how to perform a calculation. The classical ancient world developed a number of significant algorithms, such as Euclid’s algorithm for finding the greatest common divisor of two integers M and N. It is a process of continuing to divide one integer by another, starting with M and N, and holding on to the remainder, but each time dropping the dividend; once we find that the remainder is zero, the greatest common divisor is the last divisor in the operation. This remarkable path to the discovery of the greatest common divisor that bears Euclid’s name has another important property that most people today take to be an essential part of being an algorithm: it is an operation that is guaranteed to work (that is, to be finished) in a finite amount of time (that is, a finite number of calculations) – though the specific value of the finite limit is likely to depend on the particular input that we choose to give to the algorithm.2http://www-groups.dcs.st-/history/Mathematicians/Al-Khwarizmi.html On Khwarazm: rmatik.uni-trier.de/~ghasemzadeh/In its origins, the algorithm was created in order to describe an operation too complex to explain in any other fashion, like Euclid’s algorithm for greatest common divisor. But a revolutionary change took place when mathematicians and logicians came to consider the notion that all thinking about mathematics could be described as an algorithm, and that if this were so, Aristotle’s attempt to classify and categorize sound inference could be greatly expanded to cover mathematics. These ideas were first discussed by Blaise Pascal and by Gottfried von Leibnitz, extended in the 19th century by Giuseppe Peano and Gottlob Frege, and developed in detail in the 1930s by Kurt Gödel, Alonzo Church, Alan Turing, and Emil Post.3The second revolutionary change associated with the algorithm was the realization (which Pascal was already very aware of) that an algorithm was something that in many cases could be easily embodied in a physical object, in such a way that the steps of the algorithm corresponded to motions of physical parts. Man’s control of physical nature had reached a point in the 1930s that it became possible for the first time to create a rapid, general, and practical implementation of algorithms, a device that we today call the computer. Alan Turing’s effort to do just this during World War II is well known,4 and was a major contributor to the defeat of Hitler’s military codes during the war. Although several technical ways were developed for expressing algorithms in all their explicit glory, it was an important result of the time that these different ways were at their heart all equivalent. Turing’s formulation was the one that seemed the easiest to state in simple terms: given his imaginary (or abstract) Turing machine, any algorithm could be expressed as a sequence of 1’s and 0’s on a long piece of tape, and any sequence of 1’s and 0’s would be interpreted as an algorithm by the Turing machine.If any algorithm could be represented as a finite sequence of 1’s and 0’s, then it could be easily (and naturally) assigned an integer: whatever integer those 1’s and 0’s represented in binary arithmetic. There was thus a natural order to the set of all algorithms: they could be lined up in ascending order, and every algorithm would have its place, determined in a straightforward manner.5 It would then be possible to search for an algorithm just by stepping through each of the natural numbers, because each natural number was, in the sense we’ve just described, a representation of an algorithm. And if we’re looking for an algorithm that accomplished a particular task (say, that generate the sentences of English), we would stop as soon as we found one that we could determine was capable of accomplishing the task in question (if we could find a satisfactory means of testing whether the algorithm did what we wanted it to do, of course).Now, by the 1930s, logicians were already talking about generating logical languages with the formal mechanisms of algorithms. It was only the next step to try to apply the same ways of conceptualizing the problem to the task of understanding natural language3 Berlinkski 2000 is an entertaining book on the history of the algorithm.4 It is also a story told in Neal Stephenson’s Cryptonomican (1999).5 Different sequences of 1’s and 0’s (different Turing machine programs) might correspond conceptually to the same algorithm at a human level, of course.as the product of an algorithm, as Zellig Harris and Noam Chomsky began to do in the late 1940s and into the 1950s.Zellig Harris was developing the idea (among others) that the pursuit of the right grammar for a set of linguistic data was the most compact grammatical representation of the data. The focus on compactness naturally grew out of the way logicians were thinking about algorithms already at this point: as I pointed out, the same idea could be formalized (into a Turing machine program) in several ways, but two kinds of priority were to be given to the shortest formulation among a set of otherwise equivalent formulations. The priority derives, first, from the crucial observation that if we are searching for an algorithm by going through the universal inventory in order, going from lowest integer (0) to ever larger integers, we are naturally looking at all smaller programs before all longer programs: that is just a way of saying that a smaller integer is a shorter integer, after all.But there was much more to the size, or compactness, of the algorithm, and during the 1950s this idea was pursued along a number of lines. From the linguistic point of view, pride of place goes to Chomsky’s work in The Logical Structure of Linguistic Theory (1975 [1955]), in which the proposal is made that the goal of the designer of the linguistic theory is to build a theory in which the shortest grammar consistent with the data is the correct one. Chomsky noted,In careful descriptive work, we almost always find that one of theconsiderations involved in choosing among alternative analyses is thesimplicity of the resulting grammar. If we can set up elements in such away that very few rules need be given about their distribution, or that theserules are very similar to the rules for other elements, this fact certainlyseems to be a valid support for the analysis in question. It seemsreasonable, then, to inquire into the possibility of defining linguisticnotions in the general theory partly in terms of such properties of grammaras simplicity. (p. 113-114)…In constructing a grammar, we try to set upelements having regular, similarly patterned, and easily statabledistributions, and which are subject to similar variations under similarconditions; in other words, elements about which a good deal ofgeneralization is possible and few special restrictions need be stated. It isinteresting to note that any simplification along these lines is immediatelyreflected in the length of the grammar. (117) …It is tempting, then, toconsider the possibility of devising a notational system which convertsconsiderations of simplicity into considerations of length….(Moregenerally, simplicity might be determined as a weighted function of thenumber of symbols, the weighting devised so as to favor reductions incertain parts of the grammar.) (117) It is important to recognize that weare not interested in reduction of the length of grammars for its own sake.Our aim is rather to permit just those reductions in length which reflectreal simplicity, that is, which will turn simpler grammars (in somepartially understood, presystematic sense of this notion) into shortergrammars. 118.At the same time, other developments were taking place, in both the U.S. and the Soviet Union. In the Soviet Union, the distinguished mathematician Andrej Kolmogorov (1903-1987) was developing a notion by which any algorithm could be assigned an apriori probability. You will recall that to assign probabilities to a set of distinct items, the probabilities must add up to 1.0, and this obviously requires some care when considering an infinite set, such as the set of all algorithms. He proposed that the probability is directly and simply based on the length of the shortest implementation of the algorithm, and it is not difficult to see that if a binary number of length N is assigned a probability equal to 1/22N, then these probabilities will sum to 1, and shorter programs will be assigned a higher apriori probability: in fact, two programs whose lengths differ by d will have probabilities whose ratios are 22d.6 Very similar ideas were being developed at the same time in the United States, first by Ray Solomonoff7 and a bit later by Gregory Chaitin (Li and Vitányi 1997).4. It’s fine to have an apriori rating for a grammar, but how well does it deal with the data?In the development we have considered up to this point, the focus has been on the grammar, and developing an apriori (or “prior”) evaluation on the goodness of the grammar as such. But that’s only half the story in selecting a grammar: we also need to know how well the grammar jibes with the data in question. Chomsky’s LSLT touches on the question of disagreement between grammar and data (in particular, in his chapter 5 on grammaticalness), but ultimately has little to say about the problem. And the problem is this: would we be willing to accept a short grammar for our data even if it did not generate all of the data? If so, how much data are we willing to abandon accounting for with each shortening of the grammar? What is the fundamental nature of the trade-off between conciseness of grammar and fit to the data? Generative grammar had nothing to say to this question.Ray Solomonoff was very directly working on just this problem in the mid 1950s, years later posing the problem in a way that sounds to a linguist’s ears just like the problem of grammar induction: given a finite amount of data, how do we decide what is the best description of the data? In Solomonoff’s words:On reading Chomsky's “Three Models for the Description of Language”(Cho 56), I found his rules for generating sentences to be very similar tothe techniques I had been using in the 1957 paper to create newabstractions from old, but his grammars were organized better, easier tounderstand, and easier to generalize. It was immediately clear that his6 I leave aside some technical points that are irrelevant to the overall ideas involved, such as the fact that we may decide to assign a higher probability than this formula suggests, while at the same time allowing only certain strings of binary digits to represent legitimate algorithms.7 See Solomonoff 1995 for a very readable account.formal languages were ideal for induction. Furthermore, they would give a kind of induction that was considerably different from techniques used in statistics up to that time. The kinds of regularities it could recognize would be entirely new. [Solomonoff is undoubtedly referring to Markov models here – JAG]At the time of Chomsky's paper, I was trying to find a satisfactory utility evaluation function for my own system. I continued working on this with no great success until 1958, when I decided to look at Chomsky's paper more closely. It was easy for me to understand and build upon. In a short time, I devised a fast left to right parser for context free languages and an extremely fast matrix parser for context sensitive languages. It took advantage of special 32 bit parallel processing instructions that most computers have.My main interest, however, was learning. I was trying to find an algorithm for the discovery of the “best” grammar for a given set of acceptable sentences. One of the things sought for: Given a set of positive cases of acceptable sentences and several grammars, any of which is able to generate all of the sentences - what goodness of fit criterion should be used? It is clear that the “Ad-hoc grammar”, that lists all of the sentences in the corpus, fits perfectly. The “promiscuous grammar” that accepts any conceivable sentence, also fits perfectly. The first grammar has a long description, the second has a short description. It seemed that some grammar half way between these, was “correct” - but what criterion should be used?There are other modes of learning in which the “goodness of fit” criterion is clearer.One such learning environment involves a “teacher”, who is able to tell the “learner” if a proposed sentence is within the language or not. Another training environment gives negative as well as positive examples of sentences.Neither of these training environments are easy to obtain in the real world. The “positive cases only, with a few errors” environment is, by far, most widely available.The real breakthrough came with my invention of probabilistic languages and their associated grammars. In a deterministic (non-probabilistic) language, a string is either an acceptable sentence or it is not an acceptable sentence. Taking a clue from Korzybski - we note that in the real world, we usually don't know for sure whether anything is true or false -but we can assign probabilities. Thus a probabilistic language assigns aprobability value to every possible string. In a “normalized” language, thetotal probability of all strings is one.It is easy to give examples of probabilistic grammars: any context free orcontext sensitive generative grammar can be written as a set of rewriterules with two or more choices for each rewrite. If we assign probabilitiesto each of the choices, we have a probabilistic grammar.The way probabilistic grammars define a solution to the “positiveexamples only” induction problem:Each possible non-probabilistic grammar is assigned an a prioriprobability, by using a simple probabilistic grammar to generate non-probabilistic grammars.Each non-probabilistic grammar that could have created the data set canbe changed to a probabilistic grammar by giving it probabilities for eachof its choices. For the particular data set of interest, we adjust theseprobabilities so the probability that the grammar will create that data set ismaximum.This idea of a probabilistic grammar has become a standard concept in the field of computational linguistics; one cannot work in speech recognition, and it is nearly impossible to work in syntactic parsing, without using this notion directly. It employs the notion of probability in a thorough-goingly formal fashion; it shares little or nothing with the urge to view language probabilistically because of some perceived fuzziness in linguistic categories or rules.Summarizing so far: formal analysis provides a new kind of explanation, and probability theory is a method to test two aspects of a given analysis – it can test, using Solomonoff’s idea, the goodness of fit between the formal grammar and the data, and secondly, the preference for a concise grammar can be expressed as a probability, a prior probability based on the grammar’s formal length. Given the nature of probability theory, it is possible to put these two probabilities together, and give a single evaluation for how well a grammar evaluates a set of data, that is the probability of the grammar given the data, by combining (multiplicatively) the prior probability of the grammar, on the one hand, and the probability that the grammar assigns to the data, on the other.8If we knew nothing about the history of linguistics and had heard the story up to here, I think we’d expect that the application of generative models of grammar to computational ends would be (or would have been) a piece of cake – nothing more natural. In fact, the history was not like that at all, and in the last 15 years, as there has been more and more computational work on natural language, it has not been generative grammars that have 8 This evaluation is not a probability unless we divide this product by the probability of the data, however.naturally been applied to this work. Why should this be? Is it a sign that something’s wrong somewhere, and if it is, what’s wrong?5. From algorithm to generative grammar, from generative grammar to algorithmThe crooked path that we have followed so far has been intended to illustrate the following idea: the development of generative grammar is a step that took place in a broader intellectual development that was tightly rooted in conceptual developments in the foundations of logic, mathematics, and computation from the 1930s through the 1960s, and the belief that a concise, algorithmic account of a linguistic corpus is a valid form of explanation of the data is of a piece with this intellectual movement.Two things happened in linguistics in the 1960s that bear on this observation. The first was that Chomsky emphasized considerably more strongly the human and cognitive base for generative grammar than he had in the 1950s (and eventually, in the late 1970s, all connection between grammar length and grammar preference was abandoned, with the introduction of the remarkably simplistic vision of principles and parameters grammars, and essentially a total abandonment, with little comment or fanfare, of the central substantive notion of generative grammar, that of an evaluation metric); the second was that it became possible to start implementing grammars computationally.Of course, grammars had been implemented computationally before; Chomsky’s first employment as a linguist had been working in Victor Yngve’s computational group at MIT, developing some aspects of generative grammar computationally.9 And a number of research groups were implementing grammatical models by now, including Zellig Harris’s group at Penn, and Sydney Lamb’s group at Yale. [refs] MITRE at MIT, Kuno and Oettinger at Harvard. We could point to the early meeting in June 1952 of the MIT Conference on Mechanical Translation, organized by Yehoshua Bar-Hillel, as an indicator of the beginning of organized work on machine translation (MT), and to the founding of the “Association for Machine Translation and Computational Linguistics” ten years later, in June of 1962, as the beginning of a serious movement in computational linguistics in this country.But with a small number of notable exceptions (the work on Lexical Functional Grammar and the work on HPSG being the prime examples, but there are dozens of others), mainstream American linguistics has remained aloof from computational applications and implementations. It would be a long paper indeed – it would be a book – that surveyed the various ways in which computational linguistics borrowed from mainstream linguistics, and the ways in which mainstream linguistics borrowed notions developed in computational communities, and this is not that paper.10 We will limit ourselves to just one question, which is:9 See, for example, Huck and Goldsmith 1995; see also Yngve 1982.10 A question was asked at the meeting after this paper was presented: does not the message offered here risk making linguistics more dependent on the vagaries of the current computer metaphor, at a time when we would prefer to have our notions be motivated by strictly linguistic concerns? The answer to this (quite reasonable) concern, I suggested, is that linguistics already is heavily dependent on the vagaries of current。