进程调度算法c语言
进程调度[C语言实现]
![进程调度[C语言实现]](https://img.taocdn.com/s3/m/552d56c619e8b8f67c1cb9dc.png)
#include<stdio.h>#include<stdio.h>#include<malloc.h>typedef struct ProcessNode{ // 进程结点的基本结构char name; //进程名int service_time; //服务时间int arrive_time; //到达时间int priority; //优先级struct FCFS_time{ //先到先服务int finish_time; //完成时间int turnaround_time; //周转时间float weigtharound_time;//带权周转时间}FCFS_time;struct SJF_time{ //短作业优先int finish_time;int turnaround_time;float weigtharound_time;int flag;}SJF_time;struct RR_time{ //时间片轮转的结点int finish_time;int turnaround_time;float weigtharound_time;int flag_time;//赋值为进程的服务时间,为0则进程完成}RR_time;struct Pri_time{ //优先权非抢占式int finish_time;int turnaround_time;float weigtharound_time;}Pri_time;struct ProcessNode*next;}ProcessNode,*Linklist;void main(){int choice;Linklist p,head;Linklist read_information();Linklist FCFS_scheduling(Linklist head);Linklist SJF_scheduling(Linklist head);Linklist RR_scheduling(Linklist head);Linklist Pri_scheduling(Linklist head);head=read_information();//读入进程的基本信息do{p=head->next;printf("\n");printf("**********进程初始信息输出********** \n"); //输出初始化后的进程基本信息printf("\n");printf("进程名称");printf("到达时间");printf("服务时间");printf("优先级");printf("\n");while(p){printf(" %c ",p->name);printf(" %d ",p->arrive_time);printf(" %d ",p->service_time);printf(" %d ",p->priority);printf("\n");p=p->next;}printf("\n");printf("************************************ \n");//输出进程的调用选择项printf("\n");printf("1、FCFS----先到先服务\n");printf("2、SJF-----短作业优先\n");printf("3、RR------时间片轮转\n");printf("4、Pri-----优先权调度\n");printf("5、退出\n");printf("\n");printf("************************************ \n");printf("\n");printf("请在1—5之间选择: ");scanf("%d",&choice);printf("\n");printf("\n");switch(choice){case 1: FCFS_scheduling(head);break;case 2: SJF_scheduling(head);break;case 3: RR_scheduling(head);break;case 4: Pri_scheduling(head);break;// case 5: exit();}}while(choice!=5);}Linklist read_information()//进程读入函数{int i;int num;// ProcessNode ;Linklist pro;Linklist p;Linklist head;printf("\n");printf("************进程调度算法************ \n");printf("\n");printf("请输入进程的个数:");scanf("%d",&num);printf("\n");printf("*************初始化信息************* \n");printf("\n");head=(Linklist)malloc(sizeof(ProcessNode));//头结点head->next=NULL;p=head;for(i=1;i<=num;i++){pro=(Linklist)malloc(sizeof(ProcessNode));//创建进程结点printf(" 输入第%d个进程信息:\n",i);printf(" 请输入进程名: ");fflush(stdin);scanf("%c",&pro->name);printf(" 到达时间: ");scanf("%d",&pro->arrive_time);printf(" 服务时间: ");scanf("%d",&pro->service_time);printf(" 优先级↑: ");scanf("%d",&pro->priority);//pro->next=head->next; head->next=pro;//逆序建链p->next=pro; p=pro;//顺序建链//p++;pro->next=NULL;}printf("\n");return head;}Linklist FCFS_scheduling(Linklist head)//先到先服务算法函数{Linklist p;Linklist q;//指向前一进程p=head->next;while(p) //初始化进程的完成时间、周转时间、带权周转时间,初值均赋为0{p->FCFS_time.finish_time=0;p->FCFS_time.turnaround_time=0;p->FCFS_time.weigtharound_time=0;p=p->next;}p=q=head->next;p->FCFS_time.finish_time=p->arrive_time;//避免第一个进程到达时间不为0while(p){if(p->arrive_time<=q->FCFS_time.finish_time)//下一进程已到达,在等待中{p->FCFS_time.finish_time=(p->service_time)+(q->FCFS_time.finish_time);//服务时间p->FCFS_time.turnaround_time=(p->FCFS_time.finish_time)-(p->arrive_time);//周转时间p->FCFS_time.weigtharound_time=(float)(p->FCFS_time.turnaround_time)/(p->service_time);//带权周转时间}else{p->FCFS_time.finish_time=p->service_time+p->arrive_time;//服务时间p->FCFS_time.turnaround_time=(p->FCFS_time.finish_time)-(p->arrive_time);//周转时间p->FCFS_time.weigtharound_time=(float)(p->FCFS_time.turnaround_time)/(p->service_time);//带权周转时间}q=p;p=p->next;}p=head->next;printf("******************************** FCFS ******************************** \n");//输出先到先服务调度后的进程信息printf("\n");printf("进程名称");printf("到达时间");printf("服务时间");printf("优先级");printf("完成时间");printf("周转时间");printf("带权周转时间");printf("\n");while(p){printf(" %c ",p->name);printf(" %d ",p->arrive_time);printf(" %d ",p->service_time);printf(" %d ",p->priority);printf(" %d",p->FCFS_time.finish_time);printf(" %d",p->FCFS_time.turnaround_time);printf(" %0.2f",p->FCFS_time.weigtharound_time);printf("\n");p=p->next;}printf("\n");printf("********************************************************************** \n");printf("\n");return head;}Linklist SJF_scheduling(Linklist head)//短作业优先算法{Linklist p,r;Linklist q;//指向前一进程结点int num=0;//记录进程个数int add_flag=0;//进程完成服务个数int service_time_min;int arrive_time;int k;p=head->next;//首元结点while(p) //初始化进程的完成时间、周转时间、带权周转时间,初值均赋为0{p->SJF_time.finish_time=0;p->SJF_time.turnaround_time=0;p->SJF_time.weigtharound_time=0;p->SJF_time.flag=0;++num;q=p;p=p->next;}q->next=head->next;//将创建的进程队列变为循环队列p=head->next;q=p;p->SJF_time.finish_time=p->arrive_time+p->service_time;p->SJF_time.turnaround_time=(p->SJF_time.finish_time)-(p->arrive_time);//周转时间p->SJF_time.weigtharound_time=(float)(p->SJF_time.turnaround_time)/(p->service_time);//带权周转时间q->SJF_time.finish_time=p->SJF_time.finish_time;p->SJF_time.flag=1; add_flag=1;p=p->next;do{if(p->SJF_time.flag==1){p=p->next;}else if((p->arrive_time)>(q->SJF_time.finish_time)){service_time_min=p->service_time;arrive_time=p->arrive_time;while(p->arrive_time==arrive_time&&p->SJF_time.flag==0)//寻找最短的作业{if((p->next->service_time)<(p->service_time)){service_time_min=p->next->service_time;p=p->n ext;}else {p=p->next;}}p=q->next;r=q;while(p->service_time!=service_time_min){p=p->next;}//指针指向最短作业p->SJF_time.finish_time=p->arrive_time+p->service_time;p->SJF_time.flag=1;++add_flag;p->SJF_time.turnaround_time=(p->SJF_time.finish_time)-(p->arrive_time);//周转时间p->SJF_time.weigtharound_time=(float)(p->SJF_time.turnaround_time)/(p->service_time);//带权周转时间q=p;p=r->next;}else{k=0;service_time_min=p->service_time;while(((p->arrive_time)<=(q->SJF_time.finish_time))&&k<=num)//寻找最短的作业{if(p->SJF_time.flag==1) {p=p->next;++k;}elseif((p->SJF_time.flag!=1)&&((p->service_time)<service_time_min)){ service_time_min=p->service_time;p=p->next;++k;}else { p=p->next;++k; }}p=q->next;r=q;while(p->service_time!=service_time_min){p=p->next;}//指针指向最短作业p->SJF_time.finish_time=q->SJF_time.finish_time+p->service_time;p->SJF_time.turnaround_time=(p->SJF_time.finish_time)-(p->arrive_time);//周转时间p->SJF_time.weigtharound_time=(float)(p->SJF_time.turnaround_time)/(p->service_time);//带权周转时间p->SJF_time.flag=1;++add_flag;//q=p;p=p->next;q=p;p=r->next;}}while(add_flag!=num);for(p=head->next;num>0;num--)//断开循环队列{q=p;p=p->next;}q->next=NULL;p=head->next;//指向链首,输出短作业调度后的进程信息printf("\n");printf("******************************** SJF ********************************* \n");printf("\n");printf("进程名称");printf("到达时间");printf("服务时间");printf("优先级");printf("完成时间");printf("周转时间");printf("带权周转时间");printf("\n");while(p){printf(" %c ",p->name);printf(" %d ",p->arrive_time);printf(" %d ",p->service_time);printf(" %d ",p->priority);printf(" %d",p->SJF_time.finish_time);printf(" %d",p->SJF_time.turnaround_time);printf(" %0.2f",p->SJF_time.weigtharound_time);printf("\n");p=p->next;}printf("\n");printf("********************************************************************** \n");printf("\n");return head;}Linklist RR_scheduling(Linklist head)//时间片轮转算法{Linklist q;//指向前一进程结点Linklist p;int q_time;//时间片大小int num=0;//记录进程个数int add_flag=0;//进程完成服务个数printf("请输入时间片的大小: ");scanf("%d",&q_time);p=head->next;while(p) //初始化进程的完成时间、周转时间、带权周转时间,初值均赋为0{p->RR_time.finish_time=0;p->RR_time.turnaround_time=0;p->RR_time.weigtharound_time=0;p->RR_time.flag_time=p->service_time;q=p;++num;p=p->next;}q->next=head->next;//将创建的进程队列变为循环队列p=head->next;q->RR_time.finish_time=p->arrive_time;do{/* printf("\n");printf("************************************************************** \n");printf(" %c ",p->name);printf(" %d ",p->arrive_time);printf(" %d ",p->service_time);printf(" %d ",p->priority);printf(" %d",p->RR_time.finish_time);printf("\n"); */if((p->RR_time.flag_time)>(q_time))//服务时间大于时间片{p->RR_time.finish_time=(q->RR_time.finish_time)+(q_time);//累加完成时间p->RR_time.flag_time=(p->RR_time.flag_time)-(q_time);if((p->next->arrive_time)<=(p->RR_time.finish_time))//有进程等待{ q=p;p=p->next; }else //当前进程未完成,无进程等待,指针不向后移{ q=p; }}else if((p->RR_time.flag_time)==0)//进程已经完成{p=p->next;}else{p->RR_time.finish_time=(q->RR_time.finish_time)+(p->RR_time.flag_time);p->RR_time.flag_time=0; ++add_flag;p->RR_time.turnaround_time=(p->RR_time.finish_time)-(p->arrive_time);//周转时间p->RR_time.weigtharound_time=(float)(p->RR_time.turnaround_time)/(p->service_time);//带权周转时间if((p->next->arrive_time)<(p->RR_time.finish_time))//有进程等待{ q=p;p=p->next; }else //当前进程完成,无进程等待,指针向后移//{ q=p; q->RR_time.finish_time=p->next->arrive_time; }{p=p->next;q=p;q->RR_time.finish_time=p->arrive_time;}}}while(add_flag!=num);//}while(p->RR_time.flag==0);for(p=head->next;num>0;num--)//断开循环队列{q=p;p=p->next;}q->next=NULL;p=head->next;//指向链首,输出时间片轮转调度后的进程信息printf("\n");printf("******************************** RR ********************************** \n");printf("\n");printf("进程名称");printf("到达时间");printf("服务时间");printf("优先级");printf("完成时间");printf("周转时间");printf("带权周转时间");..WORD完美格式..printf("\n");while(p){printf(" %c ",p->name);printf(" %d ",p->arrive_time);printf(" %d ",p->service_time);printf(" %d ",p->priority);printf(" %d",p->RR_time.finish_time);printf(" %d",p->RR_time.turnaround_time);printf(" %0.2f",p->RR_time.weigtharound_time);printf("\n");p=p->next;}printf("\n");printf("********************************************************************** \n");printf("\n");return head;}Linklist Pri_scheduling(Linklist head)//优先权调度算法{printf(" 优先权调度算法\n");return head;}..专业知识编辑整理..。
先来先服务和优先数调度算法c语言

先来先服务和优先数调度算法c语言先来先服务和优先数调度算法c语言一、前言操作系统中的进程调度是指在多道程序环境下,按照一定的规则从就绪队列中选择一个进程,将CPU分配给它运行。
常用的进程调度算法有先来先服务(FCFS)、最短作业优先(SJF)、时间片轮转(RR)等。
本文将介绍两种常见的进程调度算法:先来先服务和优先数调度算法,并给出相应的C语言实现。
二、先来先服务算法1. 算法原理FCFS即First Come First Served,也称为FIFO(First In First Out),是一种非抢占式的进程调度算法。
按照任务到达时间的顺序进行处理,即谁先到达谁就被处理。
2. 算法流程(1)按照任务到达时间排序;(2)依次执行每个任务,直至所有任务都完成。
3. C语言实现下面是一个简单的FCFS程序:```c#include <stdio.h>struct process {int pid; // 进程IDint arrival_time; // 到达时间int burst_time; // 执行时间int waiting_time; // 等待时间};int main() {struct process p[10];int n, i, j;float avg_waiting_time = 0;printf("请输入进程数:");scanf("%d", &n);for (i = 0; i < n; i++) {printf("请输入第%d个进程的信息:\n", i + 1); printf("进程ID:");scanf("%d", &p[i].pid);printf("到达时间:");scanf("%d", &p[i].arrival_time);printf("执行时间:");scanf("%d", &p[i].burst_time);}for (i = 0; i < n; i++) {for (j = 0; j < i; j++) {if (p[j].arrival_time > p[j + 1].arrival_time) { struct process temp = p[j];p[j] = p[j + 1];p[j + 1] = temp;}}}int current_time = p[0].arrival_time;for (i = 0; i < n; i++) {if (current_time < p[i].arrival_time) {current_time = p[i].arrival_time;}p[i].waiting_time = current_time - p[i].arrival_time;current_time += p[i].burst_time;}printf("进程ID\t到达时间\t执行时间\t等待时间\n");for (i = 0; i < n; i++) {printf("%d\t%d\t%d\t%d\n", p[i].pid, p[i].arrival_time, p[i].burst_time, p[i].waiting_time);avg_waiting_time += (float)p[i].waiting_time / n;}printf("平均等待时间:%f\n", avg_waiting_time);return 0;}```三、优先数调度算法1. 算法原理优先数调度算法是一种非抢占式的进程调度算法。
进程调度C语言实现

#include<stdio.h>#include<stdio.h>#include<malloc.h>typedef struct ProcessNode{ // 进程结点的基本结构char name; // 进程名int service_time; // 服务时间int arrive_time; // 到达时间int priority; // 优先级struct FCFS_time{ // 先到先服务int finish_time; // 完成时间int turnaround_time; // 周转时间float weigtharound_time;// 带权周转时间}FCFS_time;struct SJF_time{ // 短作业优先int finish_time;int turnaround_time;float weigtharound_time;int flag;}SJF_time;struct RR_time{ // 时间片轮转的结点int finish_time;int turnaround_time;float weigtharound_time;int flag_time;// 赋值为进程的服务时间,为0 则进程完成}RR_time;struct Pri_time{ // 优先权非抢占式int finish_time;int turnaround_time;float weigtharound_time;}Pri_time;struct ProcessNode*next;}ProcessNode,*Linklist;void main(){int choice;Linklist read_information();Linklist FCFS_scheduling(Linklist head);Linklist SJF_scheduling(Linklist head);Linklist RR_scheduling(Linklist head);Linklist Pri_scheduling(Linklist head);head=read_information();// 读入进程的基本信息do{p=head->next;printf("\n");printf("\n");printf(" 请在 1—5 之间选择 : ");scanf("%d",&choice);printf("\n");printf("\n"); printf(" ********** 进程初始信息输出 ********** \n");输出初始化后的进程基本信息 printf("\n");printf(" 进程名称 ");printf(" 到达时间 ");printf(" 服务时间 ");printf(" 优先级 ");printf("\n");while(p){printf(" %c ",p->name);printf(" %d ",p->arrive_time);printf(" %d ",p->service_time); printf(" %d",p->priority);printf("\n");p=p->next;}printf("\n");printf(" ************************************ \n");//输出进程的调用选择项 printf("\n");printf("1 、FCFS---先- 到先服务\n"); printf("2 、SJF 短作业优先\n");printf("3 、 RR ---- 时间片轮转 \n");printf("4 、Pri ----- 优先权调度 \n");printf("\n"); printf(" ************************************ \n");{case 1: FCFS_scheduling(head); break;case 2: SJF_scheduling(head);break;case 3: RR_scheduling(head); break;case 4: Pri_scheduling(head); break;// case 5: exit();}}while(choice!=5);Linklist read_information()// 进程读入函数{int i;int num;// ProcessNode ; Linklist pro; Linklist p; Linklist head;printf("\n");printf(" ************ 进程调度算法************ \n");printf("\n");printf(" 请输入进程的个数:");scanf("%d",&num);printf("\n");printf(" ************* 初始化信息************* \n");printf("\n");head=(Linklist)malloc(sizeof(ProcessNode));// 头结点head->next=NULL;p=head;for(i=1;i<=num;i++){pro=(Linklist)malloc(sizeof(ProcessNode));// 创建进程结点printf(" 输入第%d 个进程信息:\n",i);printf(" 请输入进程名: ");fflush(stdin);scanf("%c",&pro->name);printf(" 到达时间: ");scanf("%d",&pro->arrive_time);printf(" 服务时间: ");scanf("%d",&pro->service_time);printf(”优先级f :");scanf("%d",&pro->priority);//pro->next=head->next; head->next=pro;// 逆序建链p->next=pro; p=pro;// 顺序建链//p++;pro->next=NULL;}printf("\n");return head;}Linklist FCFS_scheduling(Linklist head)// 先到先服务算法函数Linklist p;Linklist q;// 指向前一进程p=head->next;while(p) // 初始化进程的完成时间、周转时间、带权周转时间,初值均赋为0{p->FCFS_time.finish_time=0;p->FCFS_time.turnaround_time=0;p->FCFS_time.weigtharound_time=0;p=p->next;}p=q=head->next;p->FCFS_time.finish_time=p->arrive_time;// 避免第一个进程到达时间不为0 while(p){if(p->arrive_time<=q->FCFS_time.finish_time)// 下一进程已到达,在等待中{p->FCFS_time.finish_time=(p->service_time)+(q->FCFS_time.finish_time);// 服务时间p->FCFS_time.turnaround_time=(p->FCFS_time.finish_time)-(p->arrive_time);// 周转时间p->FCFS_time.weigtharound_time=(float)(p->FCFS_time.turnaround_time)/(p->service_time);// 带权周转时间}else{p->FCFS_time.finish_time=p->service_time+p->arrive_time;// 服务时间p->FCFS_time.turnaround_time=(p->FCFS_time.finish_time)-(p->arrive_time);// 周转时间p->FCFS_time.weigtharound_time=(float)(p->FCFS_time.turnaround_time)/(p->service_time);// 带权周转时间}q=p;p=p->next;p=head->next;printf(" ******************************** FCFS ******************************** \n");// 输出先到先服务调度后的进程信息printf("\n");printf(" 进程名称"); printf(" 到达时间"); printf(" 服务时间");printf(" 优先级");printf(" 完成时间");printf(" 周转时间"); printf(" 带权周转时间");printf("\n");while(p){printf(" %c ",p->name);printf(" %d ",p->arrive_time);printf(" %d ",p->service_time);printf(" %d ",p->priority);printf(" %d",p->FCFS_time.finish_time);printf(" %d",p->FCFS_time.turnaround_time);printf(" %0.2f",p->FCFS_time.weigtharound_time); printf("\n");p=p->next;}printf("\n");printf(" ********************************************************************** \n");printf("\n");return head;Linklist SJF_scheduling(Linklist head)// 短作业优先算法{Linklist p,r;Linklist q;// 指向前一进程结点int num=0;// 记录进程个数int add_flag=0;// 进程完成服务个数int service_time_min;int arrive_time;int k;p=head->next;// 首元结点while(p) // 初始化进程的完成时间、周转时间、带权周转时间,初值均赋为0{p->SJF_time.finish_time=0; p->SJF_time.turnaround_time=0; p->SJF_time.weigtharound_time=0;p->SJF_time.flag=0;++num;q=p;p=p->next;}q->next=head->next;// 将创建的进程队列变为循环队列p=head->next;q=p;p->SJF_time.finish_time=p->arrive_time+p->service_time;p->SJF_time.turnaround_time=(p->SJF_time.finish_time)-(p->arrive_time);// 周转时间p->SJF_time.weigtharound_time=(float)(p->SJF_time.turnaround_time)/(p->service_time);// 带权周转时间q->SJF_time.finish_time=p->SJF_time.finish_time;p->SJF_time.flag=1; add_flag=1;p=p->next;do{ if(p->SJF_time.flag==1){p=p->next;} else if((p->arrive_time)>(q->SJF_time.finish_time)) {service_time_min=p->service_time;arrive_time=p->arrive_time;while(p->arrive_time==arrive_time&&p->SJF_time.flag==0)// 寻找最短的作业{if((p->next->service_time)<(p->service_time)){service_time_min=p->next->service_time;p=p->next;}else {p=p->next;}}p=q->next;r=q;while(p->service_time!=service_time_min){p=p->next;}// 指针指向最短作业p->SJF_time.finish_time=p->arrive_time+p->service_time;p->SJF_time.flag=1;++add_flag;p->SJF_time.turnaround_time=(p->SJF_time.finish_time)-(p->arrive_time);// 周转时间p->SJF_time.weigtharound_time=(float)(p->SJF_time.turnaround_time)/(p->service_time);// 带权周转时间q=p;p=r->next;}else{k=0;service_time_min=p->service_time;while(((p->arrive_time)<=(q->SJF_time.finish_time))&&k<=num)// 寻找最短的作业{if(p->SJF_time.flag==1) {p=p->next;++k;}else if((p->SJF_time.flag!=1)&&((p->service_time)<service_time_min)){ service_time_min=p->service_time;p=p->next;++k;}else { p=p->next;++k; }}p=q->next;r=q;while(p->service_time!=service_time_min){p=p->next;}// 指针指向最短作业p->SJF_time.finish_time=q->SJF_time.finish_time+p->service_time;p->SJF_time.turnaround_time=(p->SJF_time.finish_time)-(p->arrive_time);// 周转时间p->SJF_time.weigtharound_time=(float)(p->SJF_time.turnaround_time)/(p->service_time);// 带权周转时间p->SJF_time.flag=1;++add_flag;//q=p;p=p->next;q=p;p=r->next;}}while(add_flag!=num);for(p=head->next;num>0;num--)// 断开循环队列{ q=p;p=p->next;} q->next=NULL;p=head->next;// 指向链首,输出短作业调度后的进程信息printf("\n");printf(" ******************************** SJF ********************************* \n");printf("\n");printf(" 进程名称");printf(" 到达时间"); printf("服务时间"); printf(" 优先级");printf(" 完成时间");printf(" 周转时间"); printf("printf("\n");带权周转时间while(p){printf(" %c ",p->name);printf(" %d ",p->arrive_time);printf(" %d ",p->service_time);printf(" %d ",p->priority);printf(" %d",p->SJF_time.finish_time);printf(" %d",p->SJF_time.turnaround_time);printf(" %0.2f",p->SJF_time.weigtharound_time);printf("\n");p=p->next;}printf("\n");printf(" ********************************************************************** \n");printf("\n");return head;Linklist RR_scheduling(Linklist head)// 时间片轮转算法{Linklist q;// 指向前一进程结点Linklist p;int q_time;// 时间片大小int num=0;// 记录进程个数int add_flag=0;// 进程完成服务个数printf(" 请输入时间片的大小 : "); scanf("%d",&q_time);p=head->next;while(p) // 初始化进程的完成时间、周转时间、带权周转时间,初值均赋为 0 {p->RR_time.finish_time=0; p->RR_time.turnaround_time=0;p->RR_time.weigtharound_time=0; p->RR_time.flag_time=p->service_time;q=p;++num;p=p->next;}q->next=head->next;// 将创建的进程队列变为循环队列 p=head->next;q->RR_time.finish_time=p->arrive_time;do{/* printf("\n"); if((p->RR_time.flag_time)>(q_time))// 服务时间大于时间片{ p->RR_time.finish_time=(q->RR_time.finish_time)+(q_time);// 累加完成时间p->RR_time.flag_time=(p->RR_time.flag_time)-(q_time);if((p->next->arrive_time)<=(p->RR_time.finish_time))// 有进程等待{ q=p;p=p->next; }else // 当前进程未完成,无进程等待,指针不向后移{ q=p; }}else if((p->RR_time.flag_time)==0)// 进程已经完成{p=p->next;}else{ printf("%c ",p->name); printf("printf("printf("%d ",p->arrive_time); %d ",p->service_time); %d ",p->priority); printf("%d",p->RR_time.finish_time);printf("printf("\n"); */************************************************************** \n");p->RR_time.finish_time=(q->RR_time.finish_time)+(p->RR_time.flag_time);p->RR_time.flag_time=0; ++add_flag;p->RR_time.turnaround_time=(p->RR_time.finish_time)-(p->arrive_time);// 周转时间p->RR_time.weigtharound_time=(float)(p->RR_time.turnaround_time)/(p->service_time);// 带权周转时间if((p->next->arrive_time)<(p->RR_time.finish_time))// 有进程等待{ q=p;p=p->next; }else // 当前进程完成,无进程等待,指针向后移//{ q=p; q->RR_time.finish_time=p->next->arrive_time; }{p=p->next;q=p;q->RR_time.finish_time=p->arrive_time;}}}while(add_flag!=num);//}while(p->RR_time.flag==0);for(p=head->next;num>0;num--)// 断开循环队列{q=p;p=p->next;} q->next=NULL;p=head->next;// 指向链首,输出时间片轮转调度后的进程信息printf("\n");printf(" ******************************** RR ********************************** \n");printf("\n");printf(" 进程名称"); printf(" 到达时间"); printf(" 服务时间");printf(" 优先级");printf(" 完成时间"); printf(" 周转时间"); printf(" 带权周转时间");printf("\n");while(p){printf(" %c ",p->name);printf(" %d ",p->arrive_time);printf(" %d ",p->service_time);printf(" %d ",p->priority);printf(" %d",p->RR_time.finish_time);printf(" %d",p->RR_time.turnaround_time);printf(" %0.2f",p->RR_time.weigtharound_time);printf("\n");p=p->next;}printf("\n");printf(" ********************************************************************** \n");printf("\n");return head;}Linklist Pri_scheduling(Linklist head)// 优先权调度算法printf(" 优先权调度算法\n"); return head;}。
操作系统进程调度算法(c语言实现)
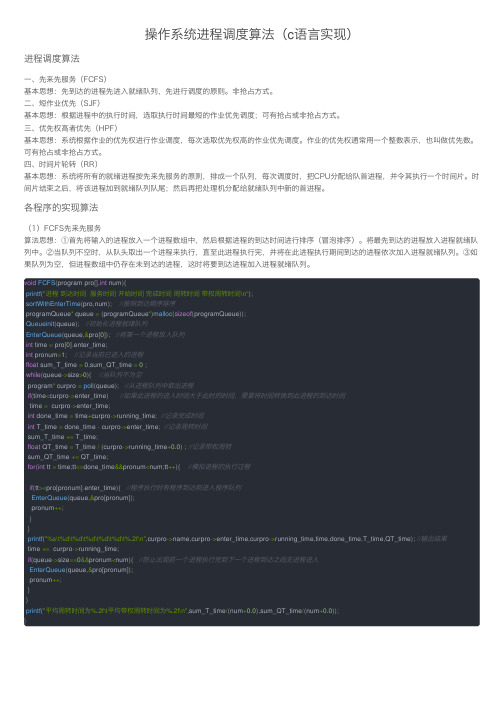
操作系统进程调度算法(c语⾔实现)进程调度算法⼀、先来先服务(FCFS)基本思想:先到达的进程先进⼊就绪队列,先进⾏调度的原则。
⾮抢占⽅式。
⼆、短作业优先(SJF)基本思想:根据进程中的执⾏时间,选取执⾏时间最短的作业优先调度;可有抢占或⾮抢占⽅式。
三、优先权⾼者优先(HPF)基本思想:系统根据作业的优先权进⾏作业调度,每次选取优先权⾼的作业优先调度。
作业的优先权通常⽤⼀个整数表⽰,也叫做优先数。
可有抢占或⾮抢占⽅式。
四、时间⽚轮转(RR)基本思想:系统将所有的就绪进程按先来先服务的原则,排成⼀个队列,每次调度时,把CPU分配给队⾸进程,并令其执⾏⼀个时间⽚。
时间⽚结束之后,将该进程加到就绪队列队尾;然后再把处理机分配给就绪队列中新的⾸进程。
各程序的实现算法(1)FCFS先来先服务算法思想:①⾸先将输⼊的进程放⼊⼀个进程数组中,然后根据进程的到达时间进⾏排序(冒泡排序)。
将最先到达的进程放⼊进程就绪队列中。
②当队列不空时,从队头取出⼀个进程来执⾏,直⾄此进程执⾏完,并将在此进程执⾏期间到达的进程依次加⼊进程就绪队列。
③如果队列为空,但进程数组中仍存在未到达的进程,这时将要到达进程加⼊进程就绪队列。
void FCFS(program pro[],int num){printf("进程到达时间服务时间开始时间完成时间周转时间带权周转时间\n");sortWithEnterTime(pro,num);//按照到达顺序排序programQueue* queue =(programQueue*)malloc(sizeof(programQueue));Queueinit(queue);//初始化进程就绪队列EnterQueue(queue,&pro[0]);//将第⼀个进程放⼊队列int time = pro[0].enter_time;int pronum=1;//记录当前已进⼊的进程float sum_T_time =0,sum_QT_time =0;while(queue->size>0){//当队列不为空program* curpro =poll(queue);//从进程队列中取出进程if(time<curpro->enter_time)//如果此进程的进⼊时间⼤于此时的时间,需要将时间转换到此进程的到达时间time = curpro->enter_time;int done_time = time+curpro->running_time;//记录完成时间int T_time = done_time - curpro->enter_time;//记录周转时间sum_T_time += T_time;float QT_time = T_time /(curpro->running_time+0.0);//记录带权周转sum_QT_time += QT_time;for(int tt = time;tt<=done_time&&pronum<num;tt++){//模拟进程的执⾏过程if(tt>=pro[pronum].enter_time){//程序执⾏时有程序到达则进⼊程序队列EnterQueue(queue,&pro[pronum]);pronum++;}}printf("%s\t%d\t%d\t%d\t%d\t%d\t%.2f\n",curpro->name,curpro->enter_time,curpro->running_time,time,done_time,T_time,QT_time);//输出结果time += curpro->running_time;if(queue->size==0&&pronum<num){//防⽌出现前⼀个进程执⾏完到下⼀个进程到达之间⽆进程进⼊EnterQueue(queue,&pro[pronum]);pronum++;}}printf("平均周转时间为%.2f\t平均带权周转时间为%.2f\n",sum_T_time/(num+0.0),sum_QT_time/(num+0.0));}(2)短作业优先(SJF)算法思想:①⾸先也是按进程的到达时间进⾏排序。
调度算法C语言实现

调度算法C语言实现调度算法是操作系统中的重要内容之一,它决定了进程在系统中的运行方式和顺序。
本文将介绍两种常见的调度算法,先来先服务(FCFS)和最短作业优先(SJF),并用C语言实现它们。
一、先来先服务(FCFS)调度算法先来先服务(FCFS)调度算法是最简单的调度算法之一、它按照进程到达的先后顺序进行调度,即谁先到达就先执行。
实现这个算法的关键是记录进程到达的顺序和每个进程的执行时间。
下面是一个用C语言实现先来先服务调度算法的示例程序:```c#include <stdio.h>//进程控制块结构体typedef structint pid; // 进程IDint arrivalTime; // 到达时间int burstTime; // 执行时间} Process;int maiint n; // 进程数量printf("请输入进程数量:");scanf("%d", &n);//输入每个进程的到达时间和执行时间Process process[n];for (int i = 0; i < n; i++)printf("请输入进程 %d 的到达时间和执行时间:", i);scanf("%d%d", &process[i].arrivalTime,&process[i].burstTime);process[i].pid = i;}//根据到达时间排序进程for (int i = 0; i < n - 1; i++)for (int j = i + 1; j < n; j++)if (process[i].arrivalTime > process[j].arrivalTime) Process temp = process[i];process[i] = process[j];process[j] = temp;}}}//计算平均等待时间和平均周转时间float totalWaitingTime = 0; // 总等待时间float totalTurnaroundTime = 0; // 总周转时间int currentTime = 0; // 当前时间for (int i = 0; i < n; i++)if (currentTime < process[i].arrivalTime)currentTime = process[i].arrivalTime;}totalWaitingTime += currentTime - process[i].arrivalTime;totalTurnaroundTime += (currentTime + process[i].burstTime) - process[i].arrivalTime;currentTime += process[i].burstTime;}//输出结果float avgWaitingTime = totalWaitingTime / n;float avgTurnaroundTime = totalTurnaroundTime / n;printf("平均等待时间:%f\n", avgWaitingTime);printf("平均周转时间:%f\n", avgTurnaroundTime);return 0;```以上程序实现了先来先服务(FCFS)调度算法,首先根据进程的到达时间排序,然后依次执行每个进程,并计算总等待时间和总周转时间。
fcfs调度算法c语言实现 -回复

fcfs调度算法c语言实现-回复FCFS调度算法(First-Come, First-Served)是操作系统中的一种简单且常见的进程调度算法。
在该算法中,进程按照到达的先后顺序被依次执行,即先到先服务。
本文将详细介绍FCFS调度算法的实现原理和步骤,并通过C语言代码实现。
一、概述进程调度是操作系统中的重要组成部分,它负责按照一定的策略和规则决定哪个进程可以执行,并分配处理器时间给它。
FCFS调度算法是最简单的调度算法之一,它不考虑进程的优先级或执行时间,只关注进程到达的先后顺序。
二、FCFS调度算法的实现步骤下面是FCFS调度算法的实现步骤:1. 定义进程控制块(PCB)结构体:PCB是描述进程的数据结构,其中包括进程ID、到达时间、执行时间等信息。
可以使用C语言的结构体来定义PCB。
2. 输入进程信息:通过键盘输入进程的到达时间和执行时间,创建多个进程,并将它们存储在一个进程队列中。
3. 按到达时间排序:对进程队列按照到达时间进行排序,以确保进程按照到达的先后顺序执行。
可以使用C语言的排序算法(如冒泡排序)来实现。
4. 计算等待时间和周转时间:对于排好序的进程队列,逐个计算每个进程的等待时间和周转时间。
等待时间等于前面所有进程的执行时间之和,而周转时间等于等待时间加上执行时间。
5. 输出结果:打印每个进程的信息,包括进程ID、到达时间、执行时间、等待时间和周转时间。
同时,计算平均等待时间和平均周转时间并输出。
三、C语言实现代码以下是使用C语言实现FCFS调度算法的示例代码:c#include <stdio.h>typedef struct {int processId;int arrivalTime;int burstTime;int waitingTime;int turnaroundTime;} PCB;void sortByArrivalTime(PCB *processes, int n) {for (int i = 0; i < n - 1; i++) {for (int j = 0; j < n - i - 1; j++) {if (processes[j].arrivalTime > processes[j +1].arrivalTime) {PCB temp = processes[j];processes[j] = processes[j + 1];processes[j + 1] = temp;}}}}void calculateWaitingTime(PCB *processes, int n) {processes[0].waitingTime = 0;for (int i = 1; i < n; i++) {processes[i].waitingTime = processes[i - 1].waitingTime + processes[i - 1].burstTime;}}void calculateTurnaroundTime(PCB *processes, int n) { for (int i = 0; i < n; i++) {processes[i].turnaroundTime = processes[i].waitingTime + processes[i].burstTime;}void printResult(PCB *processes, int n) {printf("Process\tArrival Time\tBurst Time\tWaitingTime\tTurnaround Time\n");for (int i = 0; i < n; i++) {printf("d\td\t\td\t\td\t\td\n", processes[i].processId, processes[i].arrivalTime,processes[i].burstTime, processes[i].waitingTime, processes[i].turnaroundTime);}int totalWaitingTime = 0;int totalTurnaroundTime = 0;for (int i = 0; i < n; i++) {totalWaitingTime += processes[i].waitingTime;totalTurnaroundTime += processes[i].turnaroundTime;}printf("Average waiting time: .2f\n", (float) totalWaitingTime / n);printf("Average turnaround time: .2f\n", (float) totalTurnaroundTime / n);}int main() {int n;printf("Enter the number of processes: ");scanf("d", &n);PCB processes[n];printf("Enter the arrival time and burst time of each process:\n");for (int i = 0; i < n; i++) {printf("Process d: ", i + 1);scanf("dd", &processes[i].arrivalTime,&processes[i].burstTime);processes[i].processId = i + 1;}sortByArrivalTime(processes, n);calculateWaitingTime(processes, n);calculateTurnaroundTime(processes, n);printResult(processes, n);return 0;}以上代码首先定义了PCB结构体,然后通过键盘输入进程的到达时间和执行时间,创建进程队列。
操作系统进程调度C语言代码

操作系统进程调度C语言代码#include <stdio.h>#define MAX 20//进程控制块typedef struct PCBchar name[10]; // 进程名int AT; // 到达时间int BT; // 服务时间int Pri; // 优先数int FT; // 完成时间int WT; //等待时间int RT; // 响应时间int position; // 第几号进程int flag; // 用来判断进程是否执行过}PCB;//进程调度void schedule(PCB a[], int n, int alg)int i, j, k, flag, temp;int count = 0;int pri_max = 0;float ATAT = 0.0;float AWT = 0.0;float ART = 0.0;PCBt;//各种算法的调度if (alg == 1)printf("采用先来先服务调度:\n"); //根据到达时间执行for (i = 0; i < n; i++)for (j = i + 1; j < n; j++)if (a[i].AT > a[j].AT)t=a[i];a[i]=a[j];a[j]=t;}//按到达时间依次执行for (i = 0; count != n; i++)for (j = 0; j < n; j++)//查找第一个到达时间小于等于当前时间的进程if (a[j].AT <= i && a[j].flag == 0)//记录运行时间a[j].BT--;//如果运行完成,记录完成时间、等待时间、响应时间if (a[j].BT == 0)a[j].FT=i+1;a[j].WT = a[j].FT - a[j].AT - a[j].Pri;a[j].RT=a[j].WT;a[j].flag = 1;count++;}elsebreak;}}}else if (alg == 2)printf("采用最短服务时间优先(非抢占)调度:\n");for (i = 0; count != n; i++)//找出服务时间最短的进程,并将其放置到最前面for (j = 0; j < n; j++)。
进程调度算法(fcfs,sjf,gxyb)C语言

#include<stdio.h>#include<stdlib.h>struct process{char pname;float arrivetime;float servetime;float finishtime;float roundtime;float droundtime;float waittime;float yxq; //优先权};struct process pro[100];//声明函数void fcfs(struct process pro[],int n);void sjf(struct process pro[],int n);void gxyb(struct process pro[],int n);struct process *sortarrivetime(struct process pro[],int n); void print(struct process pro[],int n);//主函数void main(){int n,i;printf("请输入有几个进程:\n");scanf("%d",&n);for(i=0;i<n;i++){fflush(stdin);printf("请输入第%d个进程名称(char):\n",i+1); scanf("%c",&pro[i].pname);printf("到达时间\n");scanf("%f",&pro[i].arrivetime);printf("服务时间\n");scanf("%f",&pro[i].servetime);}fcfs(pro,n);sjf(pro,n);gxyb(pro,n);}//按到达时间进行冒泡法排序struct process *sortarrivetime(struct process pro[],int n) {int i,j;struct process itemp;int flag;for(i=1;i<n;i++){flag=0;for(j=0;j<n-i;j++){if(pro[j].arrivetime>pro[j+1].arrivetime){itemp=pro[j];pro[j]=pro[j+1];pro[j+1]=itemp;flag=1; //交换标志}}if(flag==0) //如果一趟排序中没发生任何交换,则排序结束 break;}return pro;}//算平均数+输出函数void print(struct process pro[],int n){int i;float Sumroundtime=0,Sumdroundtime=0;float averoundtime,avedroundtime;for(i=0;i<n;i++)//算平均值{Sumroundtime+=pro[i].roundtime;Sumdroundtime+=pro[i].droundtime;}averoundtime=Sumroundtime/n;avedroundtime=Sumdroundtime/n;printf("进程名\t到达时间\t服务时间\t完成时间\t周转时间\t带全周转"); for(i=0;i<n;i++)//输出{printf("%c\t%.2f\t\t%.2f\t\t%.2f\t\t%.2f\t\t%.2f\n",pro[i].pname,pro[ i].arrivetime,pro[i].servetime,pro[i].finishtime,pro[i].roundtime,pro[i ].droundtime);}printf("平均值\t\t\t\t\t\t\t%.2f\t\t%.2f\n",averoundtime,avedroundtime); }//先来先服务算法void fcfs(struct process pro[],int n){int i;pro=sortarrivetime(pro,n);pro[0].finishtime=pro[0].arrivetime+pro[0].servetime;pro[0].roundtime=pro[0].finishtime-pro[0].arrivetime;pro[0].droundtime=pro[0].roundtime/pro[0].servetime;for(i=1;i<n;i++){if(pro[i].arrivetime<=pro[i-1].finishtime){pro[i].finishtime=pro[i-1].finishtime+pro[i].servetime; pro[i].roundtime=pro[i].finishtime-pro[i].arrivetime; pro[i].droundtime=pro[i].roundtime/pro[i].servetime;}else{pro[i].finishtime=pro[i].arrivetime+pro[i].servetime; pro[i].roundtime=pro[i].finishtime-pro[i].arrivetime;pro[i].droundtime=pro[i].roundtime/pro[i].servetime; }}printf("\n\n先来先服务:\n\n");print(pro,n);}//短作业优先算法void sjf(struct process pro[],int n){int i;pro=sortarrivetime(pro,n);pro[0].finishtime=pro[0].arrivetime+pro[0].servetime; for(i=1;i<n;i++){if(pro[i].arrivetime>pro[i-1].finishtime){pro[i].finishtime=pro[i].arrivetime+pro[i].servetime; }else{int k=0;for(int m=i;m<n;m++)//第i-1个进程完成时,找出k个进程已经到达。
c语言实现进程调度算法

c语言实现进程调度算法进程调度算法是操作系统中的一个重要组成部分,用于决定在多道程序环境下,选择哪个进程来占用CPU并执行。
C语言是一种通用的编程语言,可以用于实现各种进程调度算法。
这里我将分别介绍三种常见的进程调度算法:先来先服务调度算法(FCFS)、最短作业优先调度算法(SJF)和轮转法调度算法(RR),并给出用C语言实现的示例代码。
首先,我们来看先来先服务调度算法(FCFS)。
此算法根据到达时间的先后顺序,按照先来后到的顺序进行处理。
下面是基于C语言的先来先服务调度算法实现示例代码:```c#include<stdio.h>struct Process};void FCFS(struct Process proc[], int n)for (int i = 1; i < n; i++)}printf("进程号到达时间服务时间完成时间等待时间周转时间\n");for (int i = 0; i < n; i++)}for (int i = 0; i < n; i++)}int maiint n;printf("请输入进程数:");scanf("%d", &n);struct Process proc[n];for (int i = 0; i < n; i++)printf("请输入进程%d的到达时间和服务时间(用空格分隔):", i + 1);}FCFS(proc, n);return 0;```其次,我们来看最短作业优先调度算法(SJF),该算法选择执行时间最短的进程先执行。
下面是基于C语言的最短作业优先调度算法实现示例代码:```c#include<stdio.h>struct Process};void SJF(struct Process proc[], int n)for (int i = 0; i < n; i++)for (int j = 0; j < i; j++)}shortest_job = i;for (int j = i + 1; j < n; j++)shortest_job = j;}}}for (int i = 1; i < n; i++)}printf("进程号到达时间服务时间完成时间等待时间周转时间\n");for (int i = 0; i < n; i++)}for (int i = 0; i < n; i++)}int maiint n;printf("请输入进程数:");scanf("%d", &n);struct Process proc[n];for (int i = 0; i < n; i++)printf("请输入进程%d的到达时间和服务时间(用空格分隔):", i + 1);}SJF(proc, n);return 0;```最后,我们来看轮转法调度算法(RR),该算法分配一个时间片给每个进程,当时间片用完后,将CPU分配给下一个进程。
操作系统进程调度优先级算法C语言模拟

操作系统进程调度优先级算法C语言模拟```cstruct Processint pid; // 进程IDint priority; // 优先级};```接下来,我们使用一个简单的示例来说明操作系统进程调度优先级算法的模拟实现。
假设有5个进程需要调度执行,它们的初始优先级和运行时间如下:进程ID,优先级,已运行时间--------,--------,------------P1,4,2P2,3,4P3,1,6P4,2,1P5,5,3首先,我们需要将这些进程按照优先级排序,以得到调度队列。
可以使用冒泡排序算法实现,代码如下:```cvoid bubbleSort(struct Process *processes, int n)for (int i = 0; i < n - 1; i++)for (int j = 0; j < n - i - 1; j++)if (processes[j].priority > processes[j + 1].priority)struct Process temp = processes[j];processes[j] = processes[j + 1];processes[j + 1] = temp;}}}``````c#include <stdio.h>void bubbleSort(struct Process *processes, int n);int maistruct Process processes[] = {{1, 4, 2}, {2, 3, 4}, {3, 1, 6}, {4, 2, 1}, {5, 5, 3}};int n = sizeof(processes) / sizeof(struct Process);bubbleSort(processes, n);printf("初始调度队列:\n");printf("进程ID\t优先级\t已运行时间\n");for (int i = 0; i < n; i++)}//模拟进程调度printf("\n开始模拟进程调度...\n");int finished = 0;while (finished < n)struct Process *current_process = &processes[0];printf("执行进程 P%d\n", current_process->pid);finished++;printf("进程 P%d 执行完毕\n", current_process->pid);} else}bubbleSort(processes, n);}printf("\n所有进程执行完毕,调度队列的最终顺序为:\n"); printf("进程ID\t优先级\t已运行时间\n");for (int i = 0; i < n; i++)}return 0;```以上代码中,我们使用了一个变量`finished`来记录已完成的进程数量,当`finished`等于进程数量`n`时,所有进程执行完毕。
操作系统五种进程调度算法的代码

操作系统五种进程调度算法的代码一、先来先服务(FCFS)调度算法先来先服务(FCFS)调度算法是操作系统处理进程调度时比较常用的算法,它的基本思想是按照进程的提交时间的先后顺序依次调度进程,新提交的进程会在当前运行进程之后排队,下面通过C语言代码来实现先来先服务(FCFS)调度算法:#include <stdio.h>#include <stdlib.h>//定义进程的数据结构struct Processint pid; // 进程标识符int at; // 到达时间int bt; // 执行时间};//进程调度函数void fcfs_schedule(struct Process *processes, int n)int i, j;//根据进程的到达时间排序for(i = 0; i < n; i++)for(j = i+1; j < n; j++)if(processes[i].at > processes[j].at) struct Process temp = processes[i]; processes[i] = processes[j];processes[j] = temp;//获取各个进程执行完毕的时间int ct[n];ct[0] = processes[0].at + processes[0].bt; for(i = 1; i < n; i++)if(ct[i-1] > processes[i].at)ct[i] = ct[i-1] + processes[i].bt;elsect[i] = processes[i].at + processes[i].bt; //计算各个进程的周转时间和带权周转时间int tat[n], wt[n], wt_r[n];for(i = 0; i < n; i++)tat[i] = ct[i] - processes[i].at;wt[i] = tat[i] - processes[i].bt;wt_r[i] = wt[i] / processes[i].bt;printf("P%d:\tAT=%d\tBT=%d\tCT=%d\tTAT=%d\tWT=%d\tWT_R=%f\n", processes[i].pid, processes[i].at, processes[i].bt, ct[i], tat[i], wt[i], wt_r[i]);//主函数int mainstruct Process processes[] ={1,0,3},{2,3,5},{3,4,6},{4,5,2},{5,6,4}};fcfs_schedule(processes, 5);return 0;输出:。
进程调度--动态优先数法(C语言实现)

进程调度--动态优先数法(C语言实现)#include"stdio.h"#include"stdlib.h"#include"string.h"typedef struct node{char name[10]; //进程标志符int prio; //进程优先数int cputime; //进程占用cpu时间int needtime; //进程到完成还要的时间char state; //进程的状态struct node *next; //链指针}PCB;PCB *finish,*ready,*tail,*run; //队列指针int N; //进程数//将就绪队列的第一个进程投入运行firstin(){run=ready; //就绪队列头指针赋值给运行头指针run->state='R'; //进程状态变为运行态ready=ready->next; //就绪列头指针后移到下一进程}//标题输出函数void prt1(char a){printf("进程号 cpu时间所需时间优先数状态\n");}//进程PCB输出void prt2(char a,PCB *q){ //优先数算法输出printf(" % -10s% -10d% -10d% -10d %c\n",q->name,q->cputime,q->needtime,q->prio,q->state );}//输出函数void prt(char algo){PCB *p;prt1(algo); //输出标题if(run!=NULL) //如果运行标题指针不空prt2(algo,run); //输出当前正在运行的PCBp=ready; //输出就绪队列PCBwhile(p!=NULL){prt2(algo,p);p=p->next;}p=finish; //输出完成队列的PCB while(p!=NULL){prt2(algo,p);p=p->next;}getchar(); //按任意键继续}//优先数的算法插入算法insert1(PCB *q){PCB *p1,*s,*r;int b;s=q; //待插入的PCB指针p1=ready; //就绪队列头指针r=p1; //r做p1的前驱指针b=1;while((p1!=NULL)&&b) //根据优先数确定插入位置if(p1->prio>=s->prio){r=p1;p1=p1->next;}elseb=0;if(r!=p1) //如果条件成立说明插入在r与p1之间{r->next=s;s->next=p1;}else{s->next=p1; //否则插入在就绪队列的头ready=s;}}//优先数创建初始PCB信息void create1(char alg){PCB *p;int i,time;char na[10];ready=NULL; //就绪队列头文件finish=NULL; //完成队列头文件run=NULL; //运行队列头文件printf("输入进程号和运行时间:\n"); //输入进程标志和所需时间创建PCBfor(i=1;i<=N;i++){p=(PCB *)malloc(sizeof(PCB));scanf("%s",na);scanf("%d",&time);strcpy(p->name,na);p->cputime=0;p->needtime=time;p->state='w';p->prio=50-time;if(ready!=NULL) //就绪队列不空,调用插入函数插入insert1(p);else{p->next=ready; //创建就绪队列的第一个PCBready=p;}}//clrscr();printf(" 优先数算法输出信息:\n");printf("***********************************************\n"); prt(alg); //输出进程PCB信息run=ready; //将就绪队列的第一个进程投入运行ready=ready->next;run->state='R';}//优先数调度算法void priority(char alg){while(run!=NULL) //当运行队列不空时,有进程正在运行{run->cputime=run->cputime+1;run->needtime=run->needtime-1;run->prio=run->prio-3; //每运行一次优先数降低3个单位if(run->needtime==0) //如所需时间为0将其插入完成队列{run->next=finish;finish=run;run->state='F'; //置状态为完成态run=NULL; //运行队列头指针为空if(ready!=NULL) //如就绪队列不空firstin(); //将就绪队列的第一个进程投入运行}else //没有运行完同时优先数不是最大,则将其变为就绪态插入到就绪队列if((ready!=NULL)&&(run->prioprio)){run->state='W';insert1(run);firstin(); //将就绪队列的第一个进程投入运行}prt(alg); //输出进程PCB信息}}//主函数void main(){char algo; //算法标记//clrscr();printf("输入进程数:\n"); scanf("%d",&N); //输入进程数create1(algo); //优先数算法priority(algo);}。
用c语言编写进程调度的算法

用c语言编写进程调度的算法进程调度是计算机操作系统中一个很重要的组成部分。
目的是通过选择适当的策略,合理地分配处理器时间,保证各个进程有公正的机会去执行它们的任务,从而达到提高系统性能,提高使用量的目的。
进程调度算法根据不同的策略的不同优先级进行分类,并根据优先级来确定在什么时候该执行进程。
下面,我们将讨论一下基于策略的进程调度算法。
1.先入先出调度(FIFO)先入先出调度算法是一种最基本的调度算法。
该算法的执行方式是根据进程请求进入内存的时间顺序,进行排队,进入队列最开始的进程被分配CPU处理时间并运行,直到进程完成或发生中断或问题撤销。
但是,这种方法存在一个明显的缺陷-平均等待时间相对较长,而优先级较高的任务需要等待一段时间才能执行。
当有一个长时间的任务进入系统时,整个系统的响应时间也很长。
2.短作业(SJF)调度短作业优先(SJF)调度算法,是指根据任务在CPU上运行所需的时间长度(即任务的长度)来选择进程。
以开始时间和运行时间和作业大小为基础的调度算法,目的是为了尽可能地减少平均等待时间和平均花费时间。
该算法中,进程会依照作业长度被分配执行时间。
因此,若进程需要执行较长的作业,可能会将优先权移交给较短的作业,而导致长时间等待。
3.基于优先级的调度算法基于优先级的调度算法是指根据不同作业或进程的优先级,选定当前优先级最高的进程进行执行。
该算法的优先级分类方式可以分为静态优先级和动态优先级。
静态优先级:这种算法分先分配好不同进程的不同优先级,每个进程都有自己的优先级。
在动态运行过程中,进程优先级不发生变化。
该算法的缺点是对于散乱的进程和许多小型进程,此算法的相反效果很快变现。
动态权重算法:这种算法分配给程序一个初始权重,不断根据进程的相对优先级而减小或增加权重。
当进程被选中或被执行时,权重会下降。
进程被阻止或者等待时,权重会增加使之在下一次调度时有更高的几率获得执行的机会。
4.多级反馈调度算法多级反馈调度算法是一种渐进式算法。
用C语言编写进程调度的算法

q=q->next;
}
return OK;
}
Status Printr(READYQueue ready,FINISHQueue finish){ //打印就绪队列中的进程状态
int i=0 ;
while(ready.RUN->next!=NULL)
{
ready.RUN->next->pcb.CPUTIME++;
ready.RUN->next->pcb.NEEDTIME--;
ready.RUN->next->pcb.PRIO-=3;
if(ready.RUN->next->pcb.NEEDTIME==0)
Status Print(READYQueue ready,FINISHQueue finish);
Status Printr(READYQueue ready,FINISHQueue finish);
Status Fisrt(READYQueue &ready);
Status Insert1(READYQueue &ready);
QueuePtr s=(QueuePtr)malloc(sizeof(QNode));
s->pcb=ready.RUN->next->pcb;
s->next=NULL; //将未完成的进程插入就绪队列
ready.TAIL->next=s;
ready.TAIL=s;
//按优先数从大到小排序
fcfs调度算法c语言实现

FCFS(First-Come, First-Served)调度算法是一种简单的调度算法,其基本思想是按照进程到达的顺序进行调度,先到达的进程优先获得CPU。
以下是使用C语言实现FCFS调度算法的示例代码:c#include <stdio.h>#include <stdlib.h>#define MAX_PROCESS 100struct process {int id;int arrival_time;int burst_time;int start_time;int end_time;};void fcfs_scheduling(struct process proc[], int n) {int i, j;struct process temp;for (i = 0; i < n; i++) {proc[i].start_time = proc[i].arrival_time;proc[i].end_time = proc[i].start_time + proc[i].burst_time;printf("Process %d: Start time = %d, End time = %d\n", proc[i].id, proc[i].start_time, proc[i].end_time);}}int main() {struct process proc[MAX_PROCESS];int n, i;printf("Enter the number of processes: ");scanf("%d", &n);printf("Enter the details of each process:\n");for (i = 0; i < n; i++) {printf("Process %d: ", i + 1);printf("ID = ");scanf("%d", &proc[i].id);printf("Arrival time = ");scanf("%d", &proc[i].arrival_time);printf("Burst time = ");scanf("%d", &proc[i].burst_time);}fcfs_scheduling(proc, n);return 0;}在上面的代码中,我们首先定义了一个结构体process,其中包含进程的ID、到达时间、执行时间、开始时间和结束时间。
处理器调度算法c语言

处理器调度算法c语言一、概述处理器调度算法是操作系统中一个非常重要的问题。
在多任务操作系统中,有多个进程同时运行,而处理器只有一个,因此需要对进程进行调度,使得每个进程都能够得到适当的执行时间。
二、常见的处理器调度算法1. 先来先服务(FCFS)FCFS算法是最简单的调度算法之一。
它按照进程到达时间的先后顺序进行调度,即先到达的进程先执行。
这种算法容易实现,但可能会导致长作业等待时间过长。
2. 最短作业优先(SJF)SJF算法是根据每个进程所需的CPU时间来进行排序,并按照顺序进行调度。
这种算法可以减少平均等待时间和平均周转时间,并且可以最大限度地利用CPU资源。
3. 优先级调度优先级调度是根据每个进程的优先级来进行排序,并按照顺序进行调度。
这种算法可以确保高优先级进程得到更多的CPU时间,但可能会出现低优先级进程饥饿问题。
4. 时间片轮转(RR)RR算法将CPU分配给每个任务一定量的时间片,在该时间片内运行任务。
如果任务在该时间片内未完成,则将其放回队列尾部,并分配给下一个任务时间片。
这种算法可以确保公平性,并且可以避免长作业等待时间过长。
三、C语言中的处理器调度算法实现1. FCFS算法实现#include <stdio.h>int main(){int n, i, j;float avg_waiting_time = 0, avg_turnaround_time = 0;printf("Enter the number of processes: ");scanf("%d", &n);int burst_time[n], waiting_time[n], turnaround_time[n];printf("Enter the burst time for each process:\n");for(i=0; i<n; i++)scanf("%d", &burst_time[i]);waiting_time[0] = 0;turnaround_time[0] = burst_time[0];for(i=1; i<n; i++){waiting_time[i] = waiting_time[i-1] + burst_time[i-1];turnaround_time[i] = waiting_time[i] + burst_time[i];avg_waiting_time += waiting_time[i];avg_turnaround_time += turnaround_time[i];}avg_waiting_time /= n;avg_turnaround_time /= n;printf("\nProcess\tBurst Time\tWaiting Time\tTurnaround Time\n");for(i=0; i<n; i++)printf("P%d\t%d\t\t%d\t\t%d\n", i+1, burst_time[i], waiting_time[i], turnaround_time[i]);printf("\nAverage Waiting Time: %.2f\n", avg_waiting_ time);printf("Average Turnaround Time: %.2f\n", avg_turnaround_ time);return 0;}2. SJF算法实现#include <stdio.h>int main(){int n, i, j, temp;float avg_waiting_time = 0, avg_turnaround_time = 0; printf("Enter the number of processes: ");scanf("%d", &n);int burst_time[n], waiting_time[n], turnaround_time[n]; printf("Enter the burst time for each process:\n");for(i=0; i<n; i++)scanf("%d", &burst_time[i]);for(i=0; i<n-1; i++)for(j=i+1; j<n; j++)if(burst_time[i] > burst_time[j]){temp = burst_time[i];burst_time[i] = burst_time[j]; burst_time[j] = temp;}waiting_time[0] = 0;turnaround_time[0] = burst_time[0];for(i=1; i<n; i++){waiting_time[i] = waiting_time[i-1] + burst_time[i-1];turnaround_time[i] = waiting_time[i] + burst_time[i];avg_waiting_time += waiting_time[i];avg_turnaround_time += turnaround_time[i];}avg_waiting_time /= n;avg_turnaround_time /= n;printf("\nProcess\tBurst Time\tWaiting Time\tTurnaround Time\n");for(i=0; i<n; i++)printf("P%d\t%d\t\t%d\t\t%d\n", i+1, burst_time[i], waiting_time[i], turnaround_time[i]);printf("\nAverage Waiting Time: %.2f\n", avg_waiting_ time);printf("Average Turnaround Time: %.2f\n", avg_turnaround_ time);return 0;}3. 优先级调度算法实现#include <stdio.h>int main(){int n, i, j, temp;float avg_waiting_time = 0, avg_turnaround_time = 0;printf("Enter the number of processes: ");scanf("%d", &n);int burst_time[n], waiting_time[n], turnaround_time[n], priority[n];printf("Enter the burst time and priority for each process:\n"); for(i=0; i<n; i++)scanf("%d %d", &burst_time[i], &priority[i]);for(i=0; i<n-1; i++)for(j=i+1; j<n; j++)if(priority[i] > priority[j]){temp = priority[i];priority[i] = priority[j];priority[j] = temp;temp = burst_time[i];burst_time[i] = burst_time[j]; burst_time[j] = temp;}waiting_time[0] = 0;turnaround_time[0] = burst_time[0];for(i=1; i<n; i++){waiting_time[i] = waiting_time[i-1] + burst_time[i-1];turnaround_time[i] = waiting_time[i] + burst_time[i];avg_waiting_ time += waiting_ time[i];avg_turnaround_ time += turnaround_ time[i];}avg_waiting_ time /= n;avg_turnaround_ time /= n;printf("\nProcess\tBurst Time\tPriority\tWaiting Time\tTurnaround Time\n");for(i=0; i<n; i++)printf("P%d\t%d\t\t%d\t\t%d\t\t%d\n", i+1, burst_ time[i], priority[i], waiting_time[i], turnaround_time[i]);printf("\nAverage Waiting Time: %.2f\n", avg_waiting_ time);printf("Average Turnaround Time: %.2f\n", avg_turnaround _ time);return 0;}4. RR算法实现#include <stdio.h>int main(){int n, i, j, time_quantum;float avg_waiting_time = 0, avg_turnaround_time = 0;printf("Enter the number of processes: ");scanf("%d", &n);int burst_time[n], remaining_time[n], waiting_time[n], turnaround_time[n];printf("Enter the burst time for each process:\n");for(i=0; i<n; i++)scanf("%d", &burst_time[i]);printf("Enter the time quantum: ");scanf("%d", &time_quantum);for(i=0; i<n; i++)remaining_time[i] = burst_time[i];int t=0;while(1){int done = 1;for(i=0; i<n; i++){if(remaining_time[i] > 0){done = 0;if(remaining_ time[i] > time_ quantum){t += time_ quantum;remaining_ time[i] -= time_ quantum;}else{t += remaining _ time[i];waiting_time[i] = t - burst_time[i];remaining_ time[i] = 0;turnaround_ time[i] = waiting_time[i] + burst_time[i];avg_waiting_ time += waiting_ time[i];avg_turnaround _ time += turnaround_ time[i];}}}if(done == 1)break;}avg_waiting_ time /= n;avg_turnaround_ time /= n;printf("\nProcess\tBurst Time\tWaiting Time\tTurnaround Time\n");for(i=0; i<n; i++)printf("P%d\t%d\t\t%d\t\t%d\n", i+1, burst_time[i], waiting_time[i], turnaround_time[i]);printf("\nAverage Waiting Time: %.2f\n", avg_waiting_ time);printf("Average Turnaround Time: %.2f\n", avg_turnaround _ time);return 0;}四、总结以上是常见的处理器调度算法的C语言实现方式。
c语言编写的进程调度算法

c语言编写的进程调度算法C语言编写的进程调度算法进程调度是操作系统的核心功能之一,它负责按照一定的策略和算法,合理地分配CPU资源给正在运行或即将运行的进程,从而提高操作系统的性能和资源利用率。
在操作系统中,存在多种不同的进程调度算法,本文将以C语言编写进程调度算法为主题,一步一步回答。
第一步:定义进程结构体首先,我们需要定义一个进程的数据结构体,以便在调度算法中使用。
进程结构体包括进程ID、进程优先级、进程状态等信息。
以下是一个简单的进程结构体示例:ctypedef struct {int pid; 进程IDint priority; 进程优先级int state; 进程状态} Process;第二步:初始化进程队列进程队列是存储所有待调度进程的数据结构,可以使用链表或数组来实现。
在初始化进程队列之前,需要先创建一个空的进程队列。
以下是一个简单的初始化进程队列函数:c#define MAX_PROCESSES 100 最大进程数Process processQueue[MAX_PROCESSES]; 进程队列int processCount = 0; 当前进程数void initProcessQueue() {processCount = 0;}第三步:添加进程到队列在调度算法中,需要将新创建或运行的进程添加到进程队列中,这样才能对其进行调度。
以下是一个简单的添加进程到队列的函数:void addProcess(int pid, int priority, int state) {if (processCount >= MAX_PROCESSES) {printf("进程队列已满,无法添加进程!\n");return;}Process newProcess;newProcess.pid = pid;newProcess.priority = priority;newProcess.state = state;processQueue[processCount] = newProcess;processCount++;}第四步:实现进程调度算法进程调度算法决定了操作系统如何决定哪个进程应该被调度并获得CPU 资源。
进程调度c语言版

#include <stdio.h>#include <stdlib.h>#include <STDLIB.H>#include <string.h>#include <CONIO.H>#define null 0#define ready '0'#define run '1'struct pcb{char name[10];int prionity;char state;int needtime;int runtime;struct pcb*next;}*pcb;struct pcb *creatlist(int n);void dy(struct pcb *la);void sl(struct pcb *la);void sjf(struct pcb *la);struct pcb *copylist(struct pcb *la);void main(){struct pcb* la;int n,flag=1,i=1,m;printf("......0......tuichu....................\n"); printf("......1......chuangjianjincheng........\n"); printf("......2......duanzuoye.................\n"); printf("......3......dongtai...................\n"); printf("......4......lunzhuang.................\n");while(i==1){flag=1;while(flag==1){ printf("\nxuanzecaozuo:\n");scanf("%d",&n);if(n>=0&&n<=4)elseprintf("\nshurucuowu.");}switch(n){case 0:i=0;break;case 1:printf("\njinchengchangdu:");scanf("%d",&m);la=creatlist(m);break;case 2:sjf(la);break;case 3:dy(la);break;case 4:sl(la);break;}}}struct pcb *creatlist(int m){struct pcb *p;int i;struct pcb* la=(struct pcb*)malloc(sizeof(struct pcb)); la->next=null;for(i=0;i<m;i++){p=(struct pcb*)malloc(sizeof(struct pcb));printf("\nname:");scanf("%s",p->name);printf("\nneeddtime:");scanf("%d",&(p->needtime));p->runtime=0;printf("\nruntime:%d\n",p->runtime);printf("\nprionity:");scanf("%d",&(p->prionity));printf("\nstate:%c\n",p->state);p->next=null;p->next=la->next;la->next=p;}printf("\n create pcb ok!");return(la);}struct pcb *copylist(struct pcb *la){struct pcb *p,*s,*lb;int i;p=la->next;lb=(struct pcb*)malloc(sizeof(struct pcb)); lb->next=null;while(p!=null){s=(struct pcb*)malloc(sizeof(struct pcb)); strcpy(s->name,p->name);s->needtime=p->needtime;s->runtime=p->runtime;s->prionity=p->prionity;s->state=p->state;s->next=null;s->next=lb->next;lb->next=s;p=p->next;}return(lb);}void sjf(struct pcb *la){struct pcb *p,*q,*r,*a;struct pcb *s;s=copylist(la);while(s->next!=null){p=s->next;q=p->next;while(q!=null){if(p->needtime>=q->needtime){p=q;}q=q->next;}p->state=run;printf("\nname:%s,",p->name); printf("needtime:%d,",p->needtime); printf("state:%c\n",p->state);a=s->next;printf("the ready pcb\n");while(a!=null){if(a->state==ready)printf("\nname:%s\n",a->name);a=a->next;}r=s;while(r!=null){if(r->next->name==p->name){r->next=p->next;free(p);}r=r->next;}}}void dy(struct pcb *la){struct pcb *p,*q,*r;struct pcb *s;s=copylist(la);while(s->next!=null){p=s->next;q=p->next;r=s;while(q!=null){if(p->prionity<q->prionity){p=q;}q=q->next;}p->state=run;p->runtime=p->runtime+1;p->prionity=p->prionity-1;printf("\nname:%s",p->name); printf(",state:%c",p->state);printf(",prionity:%d",p->prionity); printf(",runtime:%d\n",p->runtime); q=s->next;printf("the ready pcb\n");while(q!=null){if(q->state==ready){printf("\nname:%s,\n",q->name);}q=q->next;}if(p->needtime==p->runtime){ r=s;while(r!=null){if(r->next->name==p->name){r->next=p->next;free(p);}r=r->next;}}else p->state=ready;}}void sl(struct pcb *la){struct pcb *p,*q,*r;struct pcb *s;int n;printf("\nplease put in time n:"); scanf("%d",&n);s=copylist(la);r=s;while(s->next!=null){p=s->next;{p->state=run;printf("\nname:%s",p->name);printf(",state:%c",p->state);p->runtime=p->runtime+n;printf(",runtime:%d",p->runtime); printf(",needtime:%d\n",p->needtime); q=s->next;printf("the ready pcb");while(q!=null){printf("\nname:%s\n",q->name);q=q->next;}if(p->runtime==p->needtime){s->next=p->next;free(p);}else{s->next=p->next;while(r->next!=null){r=r->next;}r->next=p;p->next=null;}}}。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
struct pcb *next;
}pcb,ready[10];
struct pcb *creat(k)//测试通过
{
int a,t=0;
struct pcb *head,*p1,*p2;
{ int i; //printf函数只需输出一次就绪态或执行后的进程队伍就可以了,
struct pcb *p; //不需要执行所有的时间片,把时间片初和末轮转和时间片轮转都放到main函数里面
p=head;//将链表的头指针赋给p
for(i=0,j=0;i<n&&p!=null;i++)
{ if(p->artime<time)
{ ready[j++]=*p;//将进程赋给ready[0],进程数k加1
p=p->next;//p向后移一位
}
else
{swap(p+n,p+n+1);
}
}
}
head=p;
}
return head;
}
void print(struct pcb *head,int number,int q)//head为ready的头指针,number是就绪态进程的个数……测试通过
head=creat(i);
printf(" 就绪进队伍打印表 \n");
printf(" 时间片 名称 到达时间 需要时间 优先级 已用时间 状态\n");
srand(time(0));
p1=head=(struct pcb *)malloc(LEN);
p1->netime=1+(int)(20.0*rand()/(RAND_MAX+1.0));
p1->priority=1+(int)(32.0*rand()/(RAND_MAX+1.0));
{ struct pcb *p;
int m,n;
p=head;
if(k>1)
{ for(m=0;m<k-1;m++)
{for(n=0;n<k-1-m;n++)
{if(p[n].priority>p[n+1].priority)//优先级越小越先执行
for(;q!=null;)
{ if(strcmp((*p).name,(*q).name)==0)
{q->priority=q->priority+1;
q->ustime=q->ustime+1;
break;
}
else
{q=q->next;
}
p=head;
for(i=0;i<number;i++)
{ if(h%2==1)
{printf("第%2d时间片初",q);
}
else
printf("第%2d时间片末",q);
printf("%7s",&(*p).name);
printf("%8d",p->artime);
for(a=1;a<k;a++)
{p2=(struct pcb *)malloc(LEN);
p2->netime=1+(int)(20.0*rand()/(RAND_MAX+1.0));
p2->priority=1+(int)(32.0*rand()/(RAND_MAX+1.0));
printf("%11d",p->netime);
printf("%10d",p->priority);
printf("%10d",p->ustime);
printf("%9c",p->state);
p++;
printf("\n");
}
printf(" ---------------------------------------------------------\n");
h=h+1;
}
void run(struct pcb *p,struct pcb *head)//进程执行优先级+1已用时间+1
{ struct pcb *q;
q=head;
p->priority=p->priority+1;
p->ustime=p->ustime+1;
p2->artime=t++;
p2->ustime=0;
p2->state='W'ห้องสมุดไป่ตู้
scanf("%s",&(*p2).name);printf("\n");
printf("进程名称:%s\n",&(*p2).name);
printf("进程到达时间:%d\n",p2->artime);
x=0;//重置x的值
ready0=join(head,i,j);//重新加入重新排序
ready0=sort(ready0,x);
}
else
{ready0=sort(ready0,x);//再次排序就可以了
}
print(ready0,x,j);//打印出执行后的进程队伍
printf("进程需要时间片:%d\n",p1->netime);
printf("进程优先级:%d\n",p1->priority);
printf("进程已用时间:%d\n",p1->ustime);
printf("进程状态:%c\n",'W');
p1->next=NULL;
{head=p->next;
break;
}
else
{if(strcmp((p->next)->name,(*n).name)==0)
{p->next=p->next->next;
break;
}
else
{p=p->next;
#include<stdio.h>
#include<stdio.h>
#include<stdlib.h>
#include<time.h>
#include<string.h>
#define LEN sizeof(struct pcb)
#define null 0
static int x=0,h=1;
p1->next=p2;
p1=p1->next;
}
return head;
}
struct pcb *join(struct pcb *head,int n,int time)//…………测试通过
{ int i,j;
struct pcb *p;//p表示进程的指针,q表示就绪态的指针
p=p->next;
}
x=j;
return ready;
}
void swap(struct pcb *m,struct pcb *n)
{
struct pcb tmp;
tmp=*m;
*m=*n;
*n=tmp;
}
void *sort(struct pcb *head,int k)//sort函数是为了实现就绪态进程数组中的进程排序
p1->artime=t++;
p1->ustime=0;
p1->state='W';
printf("进程赋值:\n");
scanf("%s",&(*p1).name);printf("\n");
printf("进程名称:%s\n",&(*p1).name);
printf("进程到达时间:%d\n",p1->artime);
printf("进程需要时间片:%d\n",p2->netime);
printf("进程优先级:%d\n",p2->priority);
printf("进程已用时间:%d\n",p2->ustime);
printf("进程状态:%c\n",'W');
p2->next=NULL;
}
}
void *delete(struct pcb *n,struct pcb *head,int number)
{ int a;
struct pcb *p;
p=head;
for(a=0;a<number;)
{ if(strcmp((*p).name,(*n).name)==0)//头结点是要删除的结点