List类c++实现(加强版)
c语言中list的实现方式
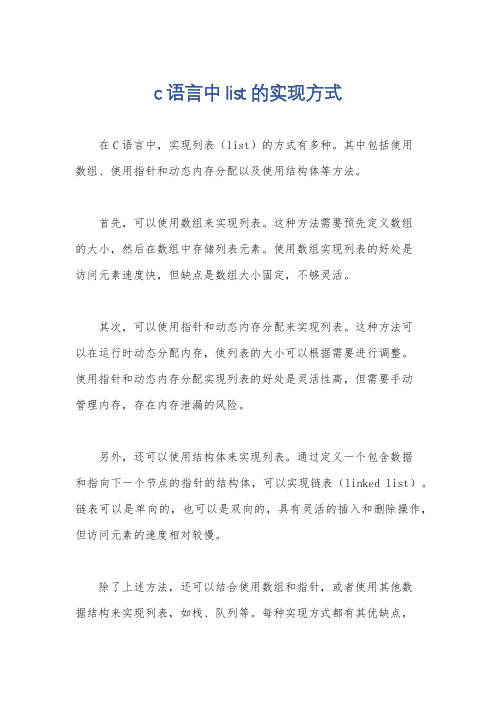
c语言中list的实现方式
在C语言中,实现列表(list)的方式有多种。
其中包括使用
数组、使用指针和动态内存分配以及使用结构体等方法。
首先,可以使用数组来实现列表。
这种方法需要预先定义数组
的大小,然后在数组中存储列表元素。
使用数组实现列表的好处是
访问元素速度快,但缺点是数组大小固定,不够灵活。
其次,可以使用指针和动态内存分配来实现列表。
这种方法可
以在运行时动态分配内存,使列表的大小可以根据需要进行调整。
使用指针和动态内存分配实现列表的好处是灵活性高,但需要手动
管理内存,存在内存泄漏的风险。
另外,还可以使用结构体来实现列表。
通过定义一个包含数据
和指向下一个节点的指针的结构体,可以实现链表(linked list)。
链表可以是单向的,也可以是双向的,具有灵活的插入和删除操作,但访问元素的速度相对较慢。
除了上述方法,还可以结合使用数组和指针,或者使用其他数
据结构来实现列表,如栈、队列等。
每种实现方式都有其优缺点,
选择合适的实现方式取决于具体的需求和应用场景。
总的来说,在C语言中,实现列表的方式有多种多样,开发人员可以根据实际情况选择最适合的方式来实现列表。
list c++ 用法

list c++ 用法C++是一种高效的编程语言,因为它结合了面向对象编程(OOP)和底层语言的特点。
C++的语法简单而且易读易写,因此被广泛用于开发各种软件,包括操作系统、数据库、游戏、图形用户界面等等。
在这篇文章中,我们将探讨C++的基本概念和用法。
1. 变量和数据类型- 整数类型(int、short、long、long long)- 无符号整数类型(unsigned int、unsigned short、unsigned long、unsigned long long)- 浮点型(float、double、long double)- 字符类型(char)- 布尔类型(bool)下面的代码声明了一个整数变量:```cppint myNumber;```这将声明一个名为“myNumber”的变量,该变量的类型为整数。
2. 运算符- 算术运算符:+、-、*、/、%- 关系运算符:==、!=、<、>、<=、>=- 逻辑运算符:&&、||、!计算两个整数之和的代码如下:```cppint a = 10;int b = 5;int sum = a + b;```3. 控制流语句- if语句:如果条件为真,则执行代码块- if-else语句:如果条件为真,则执行第一个代码块;否则,执行第二个代码块 - switch语句:根据一个变量的值执行不同的代码块- for循环:重复执行一段代码,直到达到指定条件- while循环:由于条件为真而一直重复执行代码- do-while循环:重复执行一段代码,直到条件为假```cppint myNumber = 5;if (myNumber > 0) {cout << "This number is positive." << endl;}```4. 函数1. 声明函数,指定函数类型、名称和参数2. 定义函数,编写执行任务的代码3. 调用函数,使程序执行函数所定义的任务5. 数组- 声明数组:指定数组类型、名称和大小- 初始化数组:指定数组的初始值- 访问数组元素:使用数组名称和元素索引访问数组中的值```cppint myArray[5] = {1, 2, 3, 4, 5};```6. 指针- 定义指针:指定指针类型和名称- 指针的值:存储另一个变量的内存地址- 指针的运算符:*号用于在指针中获取变量的值,&号用于获取变量的地址```cppint value = *myPointer; // 获取myNumber的值(值为10)```7. 类和对象- 定义类:指定类名称和成员变量、成员函数- 创建对象:使用类定义创建对象- 使用对象:调用对象的成员函数或变量```cppRectangle myRectangle;myRectangle.length = 5;myRectangle.width = 3;double area = myRectangle.calculateArea(); // 计算面积(值为15)```总结在本文中,我们涵盖了C++的基本概念和用法,包括变量和数据类型、运算符、控制流语句、函数、数组、指针以及类和对象。
c语言中list的用法

C语言中list的用法1.简介在C语言中,l is t是一种常用的数据结构,用于存储和管理多个元素。
它类似于数组,但具有更强大的灵活性和功能。
本文将介绍C语言中l i st的使用方法,包括创建、添加、删除和遍历等操作。
2.创建lis t要使用l is t,首先需要定义一个结构体来表示l is t的节点,节点中包含数据元素和指向下一个节点的指针。
然后,使用指向该结构体的指针来表示整个l is t。
以下是创建l is t的基本代码:t y pe de fs tr uc tN ode{i n td at a;s t ru ct No de*n ex t;}N od e;t y pe de fs tr uc t{N o de*h ea d;}L is t;3.添加元素要向li st中添加元素,可以使用以下代码:v o id ad dE le me nt(Li s t*li st,i nt ne wDa t a){N o de*n ew No de=(Nod e*)ma ll oc(s iz eof(No de));n e wN od e->d at a=new D at a;n e wN od e->n ex t=NUL L;i f(l is t->h ea d==NU L L){l i st->he ad=n ew Nod e;}e ls e{N o de*c ur re nt No de=l is t->h ea d;w h il e(cu rr en tN ode->n ex t!=N UL L){c u rr en tN od e=cu rre n tN od e->n ex t;}c u rr en tN od e->n ext=ne wN od e;}}4.删除元素要从li st中删除元素,可以使用以下代码:v o id re mo ve El em ent(Li st*l is t,in tta r ge t){ N o de*c ur re nt No de=l is t->h ea d;N o de*p re vN od e=NUL L;w h il e(cu rr en tN ode!=N UL L){i f(c ur re nt No de->d a ta==ta rg et){i f(p re vN od e==N ULL){l i st->he ad=c ur ren t No de->ne xt;}e ls e{p r ev No de->ne xt=cu r re nt No de->ne xt;}f r ee(c ur re nt No de);b r ea k;}p r ev No de=c ur re ntN o de;c u rr en tN od e=cu rre n tN od e->n ex t;}}5.遍历lis t要遍历l is t中的所有元素,可以使用以下代码:v o id tr av er se Li st(L is t*li st){N o de*c ur re nt No de=l is t->h ea d;w h il e(cu rr en tN ode!=N UL L){p r in tf("%d",cu rre n tN od e->d at a);c u rr en tN od e=cu rre n tN od e->n ex t;}}6.示例下面是一个使用l ist的示例:#i nc lu de<s td io.h>#i nc lu de<s td li b.h>t y pe de fs tr uc tN ode{i n td at a;s t ru ct No de*n ex t;}N od e;t y pe de fs tr uc t{N o de*h ea d;}L is t;v o id ad dE le me nt(Li s t*li st,i nt ne wDa t a){N o de*n ew No de=(Nod e*)ma ll oc(s iz eof(No de)); n e wN od e->d at a=new D at a;n e wN od e->n ex t=NUL L;i f(l is t->h ea d==NU L L){l i st->he ad=n ew Nod e;}e ls e{N o de*c ur re nt No de=l is t->h ea d;w h il e(cu rr en tN ode->n ex t!=N UL L){c u rr en tN od e=cu rre n tN od e->n ex t;}c u rr en tN od e->n ext=ne wN od e;}}v o id re mo ve El em ent(Li st*l is t,in tta r ge t){N o de*c ur re nt No de=l is t->h ea d;N o de*p re vN od e=NUL L;w h il e(cu rr en tN ode!=N UL L){i f(c ur re nt No de->d a ta==ta rg et){i f(p re vN od e==N ULL){l i st->he ad=c ur ren t No de->ne xt;}e ls e{p r ev No de->ne xt=cu r re nt No de->ne xt; }f r ee(c ur re nt No de);b r ea k;}p r ev No de=c ur re ntN o de;c u rr en tN od e=cu rre n tN od e->n ex t;}}v o id tr av er se Li st(L is t*li st){N o de*c ur re nt No de=l is t->h ea d;w h il e(cu rr en tN ode!=N UL L){p r in tf("%d",cu rre n tN od e->d at a);c u rr en tN od e=cu rre n tN od e->n ex t;}}i n tm ai n(){L i st my Li st;m y Li st.h ea d=NU LL;a d dE le me nt(&my Lis t,5);a d dE le me nt(&my Lis t,10);a d dE le me nt(&my Lis t,15);r e mo ve El em en t(&my L is t,10);t r av er se Li st(&myL i st);r e tu rn0;}7.总结使用li st可以轻松地管理多个元素,实现灵活的数据存储和操作。
C语言实现的list

C语言实现的list[cpp] view plain copy1.#ifndef __WINE_SERVER_LIST_H2.#define __WINE_SERVER_LIST_H3.4.#include <stddef.h>5.6.struct list7.{8.struct list *next;9.struct list *prev;10.};11.12./* add an element after the specified one */13.static inline void list_add_after( struct list *elem, struct list *to_add )14.{15.to_add->next = elem->next;16.to_add->prev = elem;17.elem->next->prev = to_add;18.elem->next = to_add;19.}20.21./* add an element before the specified one */22.static inline void list_add_before( struct list *elem, stru ct list *to_add )23.{24.to_add->next = elem;25.to_add->prev = elem->prev;26.elem->prev->next = to_add;27.elem->prev = to_add;28.}29.30./* add element at the head of the list */31.static inline void list_add_head( struct list *list, struct li st *elem )32.{33.list_add_after( list, elem );34.}35.36./* add element at the tail of the list */37.static inline void list_add_tail( struct list *list, struct list *elem )38.{39.list_add_before( list, elem );40.}41.42./* remove an element from its list */43.static inline void list_remove( struct list *elem )44.{45.elem->next->prev = elem->prev;46.elem->prev->next = elem->next;47.}48.49./* get the next element */50.static inline struct list *list_next( const struct list *list, c onst struct list *elem )51.{52.struct list *ret = elem->next;53.if (elem->next == list) ret = NULL;54.return ret;55.}56.57./* get the previous element */58.static inline struct list *list_prev( const struct list *list, c onst struct list *elem )59.{60.struct list *ret = elem->prev;61.if (elem->prev == list) ret = NULL;62.return ret;63.}64.65./* get the first element */66.static inline struct list *list_head( const struct list *list )67.{68.return list_next( list, list );69.}70.71./* get the last element */72.static inline struct list *list_tail( const struct list *list )73.{74.return list_prev( list, list );75.}76.77./* check if a list is empty */78.static inline int list_empty( const struct list *list )79.{80.return list->next == list;82.83./* initialize a list */84.static inline void list_init( struct list *list )85.{86.list->next = list->prev = list;87.}88.89./* count the elements of a list */90.static inline unsigned int list_count( const struct list *li st )91.{92.unsigned count = 0;93.const struct list *ptr;94.for (ptr = list->next; ptr != list; ptr = ptr->next) count ++;95.return count;96.}97.98./* move all elements from src to the tail of dst */99.static inline void list_move_tail( struct list *dst, struct li st *src )100.{101.if (list_empty(src)) return;102.103.dst->prev->next = src->next;104.src->next->prev = dst->prev;105.dst->prev = src->prev;106.src->prev->next = dst;107.list_init(src);109.110./* move all elements from src to the head of dst */111.static inline void list_move_head( struct list *dst, struct list *src )112.{113.if (list_empty(src)) return;114.115.dst->next->prev = src->prev;116.src->prev->next = dst->next;117.dst->next = src->next;118.src->next->prev = dst;119.list_init(src);120.}121.122./* iterate through the list */123.#define LIST_FOR_EACH(cursor,list) \124.for ((cursor) = (list)->next; (cursor) != (list); (cursor) = ( cursor)->next)125.126./* iterate through the list, with safety against removal */127.#define LIST_FOR_EACH_SAFE(cursor, cursor2, list) \ 128.for ((cursor) = (list)->next, (cursor2) = (cursor)->next; \129.(cursor) != (list); \130.(cursor) = (cursor2), (cursor2) = (cursor)->next)131.132./* iterate through the list using a list entry */133.#define LIST_FOR_EACH_ENTRY(elem, list, type, field) \134.for ((elem) = LIST_ENTRY((list)->next, type, field); \135.&(elem)->field != (list); \136.(elem) = LIST_ENTRY((elem)->field.next, type, field)) 137.138./* iterate through the list using a list entry, with safety against removal */139.#define LIST_FOR_EACH_ENTRY_SAFE(cursor, cursor2, l ist, type, field) \140.for ((cursor) = LIST_ENTRY((list)->next, type, field), \ 141.(cursor2) = LIST_ENTRY((cursor)->field.next, type, field ); \142.&(cursor)->field != (list); \143.(cursor) = (cursor2), \144.(cursor2) = LIST_ENTRY((cursor)->field.next, type, field ))145.146./* iterate through the list in reverse order */147.#define LIST_FOR_EACH_REV(cursor,list) \148.for ((cursor) = (list)->prev; (cursor) != (list); (cursor) = ( cursor)->prev)149.150./* iterate through the list in reverse order, with safety against removal */151.#define LIST_FOR_EACH_SAFE_REV(cursor, cursor2, list ) \152.for ((cursor) = (list)->prev, (cursor2) = (cursor)->prev; \153.(cursor) != (list); \154.(cursor) = (cursor2), (cursor2) = (cursor)->prev)155.156./* iterate through the list in reverse order using a list e ntry */157.#define LIST_FOR_EACH_ENTRY_REV(elem, list, type, fi eld) \158.for ((elem) = LIST_ENTRY((list)->prev, type, field); \ 159.&(elem)->field != (list); \160.(elem) = LIST_ENTRY((elem)->field.prev, type, field)) 161.162./* iterate through the list in reverse order using a list e ntry, with safety against removal */163.#define LIST_FOR_EACH_ENTRY_SAFE_REV(cursor, curs or2, list, type, field) \164.for ((cursor) = LIST_ENTRY((list)->prev, type, field), \ 165.(cursor2) = LIST_ENTRY((cursor)->field.prev, type, field ); \166.&(cursor)->field != (list); \167.(cursor) = (cursor2), \168.(cursor2) = LIST_ENTRY((cursor)->field.prev, type, field ))169.170./* macros for statically initialized lists */171.#undef LIST_INIT172.#define LIST_INIT(list) { &(list), &(list) }173.174./* get pointer to object containing list element */175.#undef LIST_ENTRY176.#define LIST_ENTRY(elem, type, field) \177.((type *)((char *)(elem) - offsetof(type, field)))178.179.#endif /* __WINE_SERVER_LIST_H */用法简介:[cpp] view plain copy1./* Define a list like so:2.*3.* struct gadget4.* {5.* struct list entry; <-- doesn't have to be the first item in the struct6.* int a, b;7.* };8.*9.* static struct list global_gadgets = LIST_INIT( global_ga dgets );10.*11.* or12.*13.* struct some_global_thing14.* {15.* struct list gadgets;16.* };17.*18.* list_init( &some_global_thing->gadgets );19.*20.* Manipulate it like this:21.*22.* list_add_head( &global_gadgets, &new_gadget->e ntry );23.* list_remove( &new_gadget->entry );24.* list_add_after( &some_random_gadget->entry, &new_gadget->entry );25.*26.* And to iterate over it:27.*28.* struct gadget *gadget;29.* LIST_FOR_EACH_ENTRY( gadget, &global_gadgets, struct gadget, entry )30.* {31.* ...32.* }33.*34.*/。
c语言list用法

c语言list用法在C语言中,没有内置的List数据类型,但是可以使用数组来实现类似的功能。
下面是一种用数组实现List的常见方式:1. 定义一个结构体来表示List和相关的元素:ctypedef struct{int capacity; List最大容量int size; List当前元素个数int *data; List元素数组} List;2. 初始化List:cList* initList(int capacity){List *list = (List*)malloc(sizeof(List)); 分配内存list->capacity = capacity; 设置容量list->size = 0; 初始元素个数为0list->data = (int*)malloc(sizeof(int) * capacity); 分配数组内存return list;}3. 添加元素到List的末尾:cvoid add(List *list, int element){if(list->size == list->capacity){List已满,需要扩容list->capacity *= 2;list->data = (int*)realloc(list->data, sizeof(int) * list->capacity);}list->data[list->size++] = element;}4. 获取List指定位置的元素:cint get(List *list, int index){if(index < 0 index >= list->size){printf("Index out of range.\n");return -1; 返回一个错误值}return list->data[index];5. 删除List末尾的元素:cvoid removeLast(List *list){if(list->size > 0){list->size;}}6. 销毁List:cvoid destroyList(List *list){free(list->data); 释放数组内存free(list); 释放List内存}使用示例:cint main(){List *list = initList(5); 初始化容量为5的Listadd(list, 10);add(list, 20);add(list, 30);printf("List[0]: %d\n", get(list, 0)); 输出:List[0]: 10removeLast(list);printf("List[2]: %d\n", get(list, 2)); 输出:Index out of range. List[2]: -1destroyList(list); 销毁Listreturn 0;}这只是一种使用数组实现List的方式,根据实际需求可以进行相应的修改和扩展。
如何用C语言或C++实现一个List类?
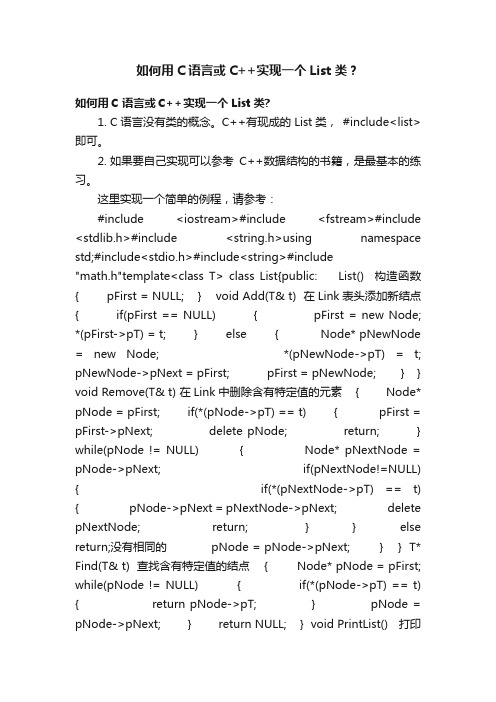
如何用C语言或C++实现一个List类?如何用C语言或C++实现一个List类?1.C语言没有类的概念。
C++有现成的List类,#include<list>即可。
2.如果要自己实现可以参考C++数据结构的书籍,是最基本的练习。
这里实现一个简单的例程,请参考:#include <iostream>#include <fstream>#include<stdlib.h>#include <string.h>using namespacestd;#include<stdio.h>#include<string>#include"math.h"template<class T> class List{public: List() 构造函数{ pFirst = NULL; } void Add(T& t) 在Link表头添加新结点{ if(pFirst == NULL) { pFirst = new Node; *(pFirst->pT) = t; } else { Node* pNewNode= new Node; *(pNewNode->pT) = t; pNewNode->pNext = pFirst; pFirst = pNewNode; } }void Remove(T& t) 在Link中删除含有特定值的元素 { Node*pNode = pFirst; if(*(pNode->pT) == t) { pFirst =pFirst->pNext; delete pNode; return; } while(pNode != NULL) { Node* pNextNode =pNode->pNext; if(pNextNode!=NULL) { if(*(pNextNode->pT) == t) { pNode->pNext = pNextNode->pNext; delete pNextNode; return; } } else return;没有相同的 pNode = pNode->pNext; } } T*Find(T& t) 查找含有特定值的结点 { Node* pNode = pFirst; while(pNode != NULL) { if(*(pNode->pT) == t) { return pNode->pT; } pNode =pNode->pNext; } return NULL; } void PrintList() 打印输出整个链表 { if(pFirst == NULL) { cout<<"列表为空列表!"<<endl; return; } Node* pNode = pFirst; while(pNode != NULL) { cout<<*(pNode->pT)<<endl; pNode = pNode->pNext; } } ~List() { Node* pNode = pFirst; while(pNode != NULL) { Node* pNextNode = pNode->pNext; delete pNode; pNode = pNextNode; } }protected: struct Node{ Node* pNext; T* pT; Node() { pNext = NULL; pT = new T; } ~Node() { delete pT; } }; Node *pFirst; 链首结点指针};class Student{public: char id[20]; 学号 char name[20]; 姓名 int age; 年龄 Student() { } ~Student() { } Student(const char* pid, const char* pname, int _age) { strcpy(id, pid); strcpy(name, pname); age = _age; } bool operator==(const Student& stu) { return strcmp(id, stu.id) == 0 && strcmp(id, stu.id) == 0 && age==stu.age; } Student& operator=(const Student& stu) { strcpy(id, stu.id); strcpy(name, ); age = stu.age; } friend ostream& operator<< (ostream&out,const Student& stu);};ostream & operator<< (ostream&out,const Student& stu){ out<<"id:"<<stu.id<<"\tname:"<<<<"\tage:"<<stu.age<<endl;}int main(){ List<Student> stuList; cout<<"添加学生前:"<<endl; stuList.PrintList(); Student stu1("1", "张三", 18); Student stu2("2", "李四", 18); Student stu3("3", "王五", 18); Student stu4("4", "至尊宝", 18); Student stu5("5", "猪八戒", 18); Student stu6("6", "唐僧", 18); Student stu7("7", "沙和尚", 18); Student stu8("8", "观音", 18); stuList.Add(stu1); stuList.Add(stu2); stuList.Add(stu3); stuList.Add(stu4); stuList.Add(stu5); stuList.Add(stu6); stuList.Add(stu7); stuList.Add(stu8); cout<<"添加学生后:"<<endl;stuList.PrintList(); Student stu11("1", "张三", 18); Student* pStu = stuList.Find(stu11); cout<<"查找到的同学是:"<<*pStu; stuList.Remove(stu11); cout<<"\n\n删除第一个后:"<<endl; stuList.PrintList(); return 0;}如何用C语言实现一个类似C++中vector的功能你先学习template,看看Vector是怎么造的,懂得了原理,vector简单的,说穿了就是数组,数组是有大小的,当你要扩大到一定的时候,就会重新分配一个较大的数组,把先前的复制到新的数组中,这个是vector的致命缺陷~当要插入的量大的时候,但优点是访问速度快。
C++中list的使用方法及常用list操作总结

C++中list的使⽤⽅法及常⽤list操作总结C++中list的使⽤⽅法及常⽤list操作总结⼀、List定义:List是stl实现的双向链表,与向量(vectors)相⽐, 它允许快速的插⼊和删除,但是随机访问却⽐较慢。
使⽤时需要添加头⽂件#include <list>⼆、List定义和初始化:list<int>lst1; //创建空listlist<int> lst2(5); //创建含有5个元素的listlist<int>lst3(3,2); //创建含有3个元素的listlist<int>lst4(lst2); //使⽤lst2初始化lst4list<int>lst5(lst2.begin(),lst2.end()); //同lst4三、List常⽤操作函数:Lst1.assign() 给list赋值Lst1.back() 返回最后⼀个元素Lst1.begin() 返回指向第⼀个元素的迭代器Lst1.clear() 删除所有元素Lst1.empty() 如果list是空的则返回trueLst1.end() 返回末尾的迭代器Lst1.erase() 删除⼀个元素Lst1.front() 返回第⼀个元素Lst1.get_allocator() 返回list的配置器Lst1.insert() 插⼊⼀个元素到list中Lst1.max_size() 返回list能容纳的最⼤元素数量Lst1.merge() 合并两个listLst1.pop_back() 删除最后⼀个元素Lst1.pop_front() 删除第⼀个元素Lst1.push_back() 在list的末尾添加⼀个元素Lst1.push_front() 在list的头部添加⼀个元素Lst1.rbegin() 返回指向第⼀个元素的逆向迭代器Lst1.remove() 从list删除元素Lst1.remove_if() 按指定条件删除元素Lst1.rend() 指向list末尾的逆向迭代器Lst1.resize() 改变list的⼤⼩Lst1.reverse() 把list的元素倒转Lst1.size() 返回list中的元素个数Lst1.sort() 给list排序Lst1.splice() 合并两个listLst1.swap() 交换两个listLst1.unique() 删除list中重复的元素四、List使⽤⽰例:⽰例1:遍历List//迭代器法for(list<int>::const_iteratoriter = lst1.begin();iter != lst1.end();iter++){cout<<*iter;}cout<<endl;⽰例2:#include <iostream>#include <list>#include <numeric>#include <algorithm>#include <windows.h>using namespace std;typedef list<int> LISTINT;typedef list<int> LISTCHAR;void main(){//⽤LISTINT创建⼀个list对象LISTINT listOne;//声明i为迭代器LISTINT::iterator i;listOne.push_front(3);listOne.push_front(2);listOne.push_front(1);listOne.push_back(4);listOne.push_back(5);listOne.push_back(6);cout << "listOne.begin()--- listOne.end():" << endl;for (i = listOne.begin(); i != listOne.end(); ++i)cout << *i << " ";cout << endl;LISTINT::reverse_iterator ir;cout << "listOne.rbegin()---listOne.rend():" << endl;for (ir = listOne.rbegin(); ir != listOne.rend(); ir++) {cout << *ir << " ";}cout << endl;int result = accumulate(listOne.begin(), listOne.end(), 0);cout << "Sum=" << result << endl;cout << "------------------" << endl;//⽤LISTCHAR创建⼀个list对象LISTCHAR listTwo;//声明i为迭代器LISTCHAR::iterator j;listTwo.push_front('C');listTwo.push_front('B');listTwo.push_front('A');listTwo.push_back('D');listTwo.push_back('E');listTwo.push_back('F');cout << "listTwo.begin()---listTwo.end():" << endl;for (j = listTwo.begin(); j != listTwo.end(); ++j)cout << char(*j) << " ";cout << endl;j = max_element(listTwo.begin(), listTwo.end());cout << "The maximum element in listTwo is: " << char(*j) << endl; Sleep(10000);}#include <iostream>#include <list>#include <windows.h>using namespace std;typedef list<int> INTLIST;//从前向后显⽰list队列的全部元素void put_list(INTLIST list, char *name){INTLIST::iterator plist;cout << "The contents of " << name << " : ";for (plist = list.begin(); plist != list.end(); plist++)cout << *plist << " ";cout << endl;}//测试list容器的功能void main(void){//list1对象初始为空INTLIST list1;INTLIST list2(5, 1);INTLIST list3(list2.begin(), --list2.end());//声明⼀个名为i的双向迭代器INTLIST::iterator i;put_list(list1, "list1");put_list(list2, "list2");put_list(list3, "list3");list1.push_back(7);list1.push_back(8);cout << "list1.push_back(7) and list1.push_back(8):" << endl;put_list(list1, "list1");list1.push_front(6);list1.push_front(5);cout << "list1.push_front(6) and list1.push_front(5):" << endl;put_list(list1, "list1");list1.insert(++list1.begin(), 3, 9);cout << "list1.insert(list1.begin()+1,3,9):" << endl;put_list(list1, "list1");//测试引⽤类函数cout << "list1.front()=" << list1.front() << endl;cout << "list1.back()=" << list1.back() << endl;list1.pop_front();list1.pop_back();cout << "list1.pop_front() and list1.pop_back():" << endl;put_list(list1, "list1");list1.erase(++list1.begin());cout << "list1.erase(++list1.begin()):" << endl;put_list(list1, "list1");list2.assign(8, 1);cout << "list2.assign(8,1):" << endl;put_list(list2, "list2");cout << "list1.max_size(): " << list1.max_size() << endl;cout << "list1.size(): " << list1.size() << endl;cout << "list1.empty(): " << list1.empty() << endl;put_list(list1, "list1");put_list(list3, "list3");cout << "list1>list3: " << (list1 > list3) << endl;cout << "list1<list3: " << (list1 < list3) << endl;list1.sort();put_list(list1, "list1");list1.splice(++list1.begin(), list3);put_list(list1, "list1");put_list(list3, "list3");Sleep(10000);}感谢阅读,希望能帮助到⼤家,谢谢⼤家对本站的⽀持!。
c语言中操作list的方法 -回复

c语言中操作list的方法-回复C语言是一种广泛应用于系统开发和嵌入式设备的编程语言。
虽然C 语言本身不支持像Python或Java那样的内置列表数据类型,但可以使用数组和指针来操作一组数据。
本文将介绍如何在C语言中操作列表数据结构,从创建和初始化列表,到插入、删除元素和遍历列表。
1. 声明和初始化列表(数组):在C语言中,可以使用数组来表示列表。
数组是一种能够存储多个具有相同数据类型的元素的数据结构。
要声明一个列表,可以使用数组的声明形式,如下所示:cint myList[10]; 声明一个包含10个整数元素的列表上述代码段声明了一个具有10个整数元素的列表。
要初始化这个列表,可以使用赋值运算符和大括号来将值赋给每个元素,例如:cint myList[4] = {1, 2, 3, 4}; 声明并初始化一个包含4个整数的列表上述代码段定义了一个包含4个整数元素的列表,并且为每个元素赋值为1、2、3和4。
2. 访问列表中的元素:要访问列表中的特定元素,可以使用索引操作符([])。
在C语言中,索引从0开始,因此要访问列表中的第一个元素,可以使用索引0,如下所示:cint firstElement = myList[0]; 获取列表中的第一个元素3. 插入和删除元素:在C语言中,由于数组是一种固定长度的数据结构,因此无法像Python的列表那样动态插入或删除元素。
但是可以通过移动元素来模拟插入或删除操作。
下面是一个插入元素的示例代码:cint myList[5] = {1, 2, 3, 4};int length = 4;int insertIndex = 2;int newValue = 10;元素后移for (int i = length; i > insertIndex; i) {myList[i] = myList[i - 1];}插入新值myList[insertIndex] = newValue;length++;输出列表for (int i = 0; i < length; i++) {printf("d ", myList[i]);}上述代码段首先声明了一个包含4个整数元素的列表。
链表(list)的实现(c语言)
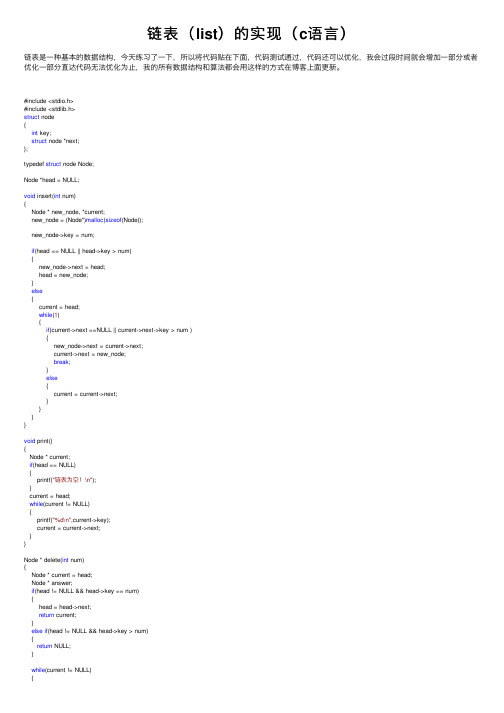
链表(list)的实现(c语⾔)链表是⼀种基本的数据结构,今天练习了⼀下,所以将代码贴在下⾯,代码测试通过,代码还可以优化,我会过段时间就会增加⼀部分或者优化⼀部分直达代码⽆法优化为⽌,我的所有数据结构和算法都会⽤这样的⽅式在博客上⾯更新。
#include <stdio.h>#include <stdlib.h>struct node{int key;struct node *next;};typedef struct node Node;Node *head = NULL;void insert(int num){Node * new_node, *current;new_node = (Node*)malloc(sizeof(Node));new_node->key = num;if(head == NULL || head->key > num){new_node->next = head;head = new_node;}else{current = head;while(1){if(current->next ==NULL || current->next->key > num ){new_node->next = current->next;current->next = new_node;break;}else{current = current->next;}}}}void print(){Node * current;if(head == NULL){printf("链表为空!\n");}current = head;while(current != NULL){printf("%d\n",current->key);current = current->next;}}Node * delete(int num){Node * current = head;Node * answer;if(head != NULL && head->key == num){head = head->next;return current;}else if(head != NULL && head->key > num){return NULL;}while(current != NULL){Node * answer;if(current->next != NULL && current->next->key == num){answer = current->next;current->next = current->next->next;return answer;}else if(current->next != NULL && current->next->key > num) {return NULL;}current = current->next;}return NULL;}int main(){Node * x;insert(14);insert(2);insert(545);insert(44);print();x = delete(44);if(x != NULL){free(x);}print();return0;}。
链表(list)--c实现

链表(list)--c实现做c的开发有1年多了,期间写过c++,感觉基础不够好,补上去,不丢⼈。
o(^▽^)oto better myself.#include <stdio.h>#include <stdlib.h>typedef struct node{int data;struct node* next;}Node;typedef Node* List;void pInitList(List*);List initList();void deleteList(List);int isNull(List);void insertNode(Node*, int);void deleteNode(List, Node*);Node* findLast(List);Node* findValue(List, int);void printList(List);void printList(List list){if(list == NULL){printf("empty list. printf nothing.\n");return;}Node* curNode = list->next;while(curNode != NULL ){if(curNode->next != NULL){printf("%d ->", curNode->data);}else{printf("%d \n", curNode->data);}curNode = curNode->next;}}Node* findValue(List list, int tarVal){if(list == NULL){printf("empty list.\n");return NULL;}Node* curNode = list->next;while((curNode->data != tarVal )&&(curNode != NULL)){curNode = curNode->next;}if(curNode == NULL) {printf("not find the value %d\n", tarVal);free(curNode);return NULL;}printf(" find the value %d\n", tarVal);return curNode;}Node* findLast(List list){if(list == NULL){printf("empty list.\n");return NULL;}Node* curNode = list->next;while(curNode->next != NULL){curNode = curNode->next;printf("find the last node value is %d.\n", curNode->data); return curNode;}void deleteNode(List list, Node* delNode){Node* curNode = (Node*)malloc(sizeof(Node));Node* freeNode = NULL;if(curNode == NULL){printf("malloc error at line %d.", __LINE__);return;}curNode = list->next;if(curNode->data == delNode->data){list->next = NULL;free(curNode);return ;}while((curNode != NULL)&& (curNode->next->data != delNode->data)&& (curNode->next != NULL)){curNode=curNode->next;}if(curNode->next == NULL || (curNode ==NULL)){printf("can not find the given node.\n");return ;}else{freeNode = curNode->next;curNode->next = freeNode->next;free(freeNode);}}void insertNode(Node* curNode, int newValue){Node *newNode = (Node*) malloc(sizeof(Node));if(newNode == NULL){printf("malloc error at line %d.", __LINE__);return;}newNode->data = newValue;newNode->next = curNode->next;curNode->next = newNode;}/*return 0 means is not NULL*/int isNull(List list ){//printf("next value %d\n", list->next->data);return (list->next == NULL);}#if 1void pInitList(List* list){//*list = (Node*) malloc(sizeof(Node));(*list)->next = NULL;return;}#endifList initList(){List list;list = (Node*) malloc(sizeof(Node));list->next = NULL;return list;}void deleteList(List list){Node* freeNode = NULL;if(list == NULL) return;while(list->next != NULL)//get the free nodefreeNode = list->next;list->next = freeNode->next;free(freeNode);}return ;}int main(){printf("hello.\n");#if 0List newList = NULL;newList = initList();#elseList *list = NULL;//这⾥我第⼀次就没分配1级指针的内存,导致出错,感谢帮助我指出的⼈。
c语言 list 实例

c语言 list 实例C语言是一种广泛应用于编程开发的高级语言,它提供了丰富的数据结构和函数库,以支持各种应用场景。
其中之一便是列表(List),也被称为链表。
列表是一种非常重要的数据结构,可以用来存储和处理大量的数据。
在本文中,我们将介绍C语言中列表的基本概念、实现方式以及一些常见的列表操作。
列表是由一系列节点(Node)组成的数据结构,每个节点包含了一个数据项和一个指向下一个节点的指针。
通过这种方式,列表的元素可以按照一定的顺序进行连接,形成一个具有先后次序的数据集合。
在C语言中,我们通常使用结构体(Struct)来定义节点的数据结构。
下面是一个简单的C语言列表的定义:```typedef struct ListNode {int val;struct ListNode* next;} ListNode;```在这个定义中,`ListNode`是节点的类型名称,具有两个成员变量:`val`表示节点的值,`next`表示指向下一个节点的指针。
通过这样的定义,我们可以创建一个链表,并通过指针将各个节点连接在一起。
创建一个列表的过程通常包括三个步骤:创建节点、添加节点以及删除节点。
下面我们将针对这三个步骤进行详细的介绍。
首先,我们需要创建一个空列表,即一个空链表:```ListNode* createList() {return NULL;}```上述代码中的`createList`函数返回了一个指针,它指向一个空链表。
此时,该链表不包含任何节点,指针的值为`NULL`。
接下来,我们可以添加节点到链表中。
例如,我们定义一个`addNode`函数,用于在链表的末尾添加一个新的节点:```void addNode(ListNode** head, int val) {ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));newNode->val = val;newNode->next = NULL;if (*head == NULL) {*head = newNode;} else {ListNode* current = *head;while (current->next != NULL) {current = current->next;}current->next = newNode;}}```在上述代码中,首先使用`malloc`函数为新节点分配存储空间,然后设置新节点的`val`为指定的值,并将`next`指针指向`NULL`。
CList控件使用方法1
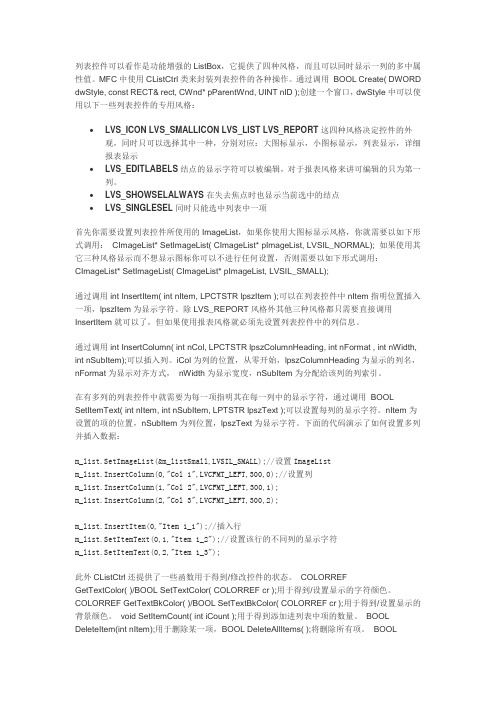
列表控件可以看作是功能增强的ListBox,它提供了四种风格,而且可以同时显示一列的多中属性值。
MFC中使用CListCtrl类来封装列表控件的各种操作。
通过调用BOOL Create( DWORD dwStyle, const RECT& rect, CWnd* pParentWnd, UINT nID );创建一个窗口,dwStyle中可以使用以下一些列表控件的专用风格:∙LVS_ICON LVS_SMALLICON LVS_LIST LVS_REPORT这四种风格决定控件的外观,同时只可以选择其中一种,分别对应:大图标显示,小图标显示,列表显示,详细报表显示∙LVS_EDITLABELS结点的显示字符可以被编辑,对于报表风格来讲可编辑的只为第一列。
∙LVS_SHOWSELALWAYS在失去焦点时也显示当前选中的结点∙LVS_SINGLESEL同时只能选中列表中一项首先你需要设置列表控件所使用的ImageList,如果你使用大图标显示风格,你就需要以如下形式调用:CImageList* SetImageList( CImageList* pImageList, LVSIL_NORMAL); 如果使用其它三种风格显示而不想显示图标你可以不进行任何设置,否则需要以如下形式调用:CImageList* SetImageList( CImageList* pImageList, LVSIL_SMALL);通过调用int InsertItem( int nItem, LPCTSTR lpszItem );可以在列表控件中nItem指明位置插入一项,lpszItem为显示字符。
除LVS_REPORT风格外其他三种风格都只需要直接调用InsertItem就可以了,但如果使用报表风格就必须先设置列表控件中的列信息。
通过调用int InsertColumn( int nCol, LPCTSTR lpszColumnHeading, int nFormat , int nWidth, int nSubItem);可以插入列。
c中list的用法

c中list的用法
C语言中的list是一种动态数组(dynamic array)的实现方式,也称作可变长
数组(variable-length array)。
它允许在程序运行过程中动态地分配内存,以存储
一组数据,并支持在任意位置插入、删除、查找和修改元素。
使用list,可以轻松
地实现各种数据结构和算法,如栈、队列、堆、哈希表等。
使用list时,需要先包含头文件<stdlib.h>,然后使用malloc函数分配一定大小的内存空间,然后使用realloc函数在需要的时候重新调整内存大小,使用free函
数释放不再需要的内存空间。
在对list进行插入和删除操作时,需要注意指针和数
组下标的正确使用,以防止内存泄漏和越界访问等错误。
list的使用方法比较灵活,可以根据需要自定义结构体类型,然后对结构体指
针进行动态内存分配。
此外,可以使用预定义的数据类型,如int、char、float等,也可以使用void类型指针,以实现更高级的数据结构和算法。
总之,C语言中的list是一种强大的数据结构,它允许动态地管理一组数据,
并提供了丰富的操作接口,可以用于实现各种数据结构和算法。
对于需要高效处理大量数据的程序,使用list可以大大提高程序的性能和可维护性。
c++list用法及源代码
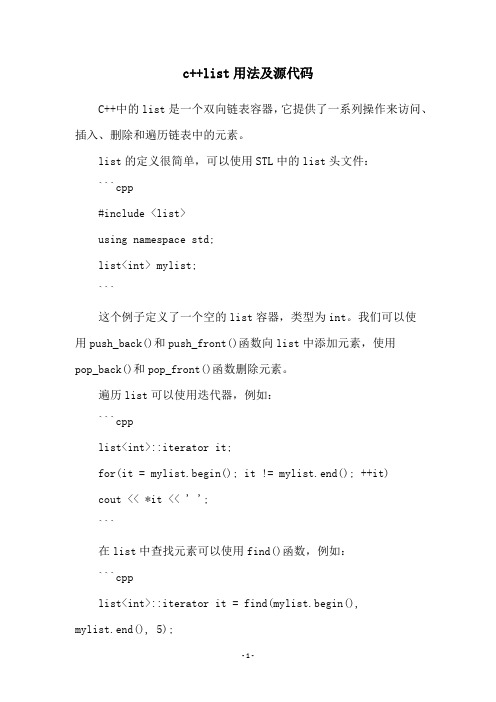
c++list用法及源代码C++中的list是一个双向链表容器,它提供了一系列操作来访问、插入、删除和遍历链表中的元素。
list的定义很简单,可以使用STL中的list头文件:```cpp#include <list>using namespace std;list<int> mylist;```这个例子定义了一个空的list容器,类型为int。
我们可以使用push_back()和push_front()函数向list中添加元素,使用pop_back()和pop_front()函数删除元素。
遍历list可以使用迭代器,例如:```cpplist<int>::iterator it;for(it = mylist.begin(); it != mylist.end(); ++it)cout << *it << ' ';```在list中查找元素可以使用find()函数,例如:```cpplist<int>::iterator it = find(mylist.begin(),mylist.end(), 5);if(it != mylist.end())cout << 'Element found: ' << *it << endl;elsecout << 'Element not found' << endl;```除了基本的操作,list还提供了一些特殊的操作,例如splice()函数可以将两个list合并起来。
下面是一个完整的list使用示例:```cpp#include <iostream>#include <list>using namespace std;void print_list(list<int>& mylist){list<int>::iterator it;for(it = mylist.begin(); it != mylist.end(); ++it)cout << *it << ' ';cout << endl;}int main(){list<int> mylist;mylist.push_back(1);mylist.push_back(2);mylist.push_back(3);mylist.push_front(0);print_list(mylist);mylist.pop_back();mylist.pop_front();print_list(mylist);list<int>::iterator it = find(mylist.begin(), mylist.end(), 2);mylist.insert(it, 10);print_list(mylist);mylist.remove(2);print_list(mylist);list<int> mylist2;mylist2.push_back(4);mylist2.push_back(5);mylist2.push_back(6);mylist.splice(mylist.end(), mylist2);print_list(mylist);return 0;}```输出结果为:```0 1 2 31 20 1 10 2 30 1 10 30 1 10 3 4 5 6```以上就是C++中list容器的基本用法和源代码。
c中list的用法

c中list的用法下面我就跟你们具体介绍下c中list的用法的用法,希望对你们有用。
c中list的用法的用法如下:这几天在做图像处理方面的商量,其中有一部分是关于图像分割方面的,图像目标在分割出来之后要做进一步的处理,因此有必要将目标图像的信息保存在一个变量里面,一开始想到的是数组,但是马上就觉察使用数组的缺点:数组长度固定,动态支配内存很简洁导致错误发生。
最重要的一点是我要保存目标图像的每一点的坐标值,使用数组就有点无能为力了。
因此到百度、Google大神上面找思路,最终被我觉察在c++的标准库里面还有这么一个模板类:list,下面就是对找到的资料的汇总和加工。
vc6自带的msdn关怀文档的解释以下是引自msdn关怀文档:The template class describes an object that controls a varying-length sequenceof elements of type T. The sequence is stored as a bidirectional linked list of elements, each containing a member of type T.本模板类描述了一个对象,这个对象是类型为T的可变长度的序列元素。
这个序列接受双向链表的方式存储每一个元素,其中每一个元素的数据流行都是T。
The object allocates and frees storage for the sequence it controls through a protected object named allocator, of class A. Such an allocator object must have the same external interface as an object of template class allocator. Note that allocatoris not copied when the object is assigned.对序列对象的支配和释放操作通过一个受爱惜的对象allocator进行。
链表list
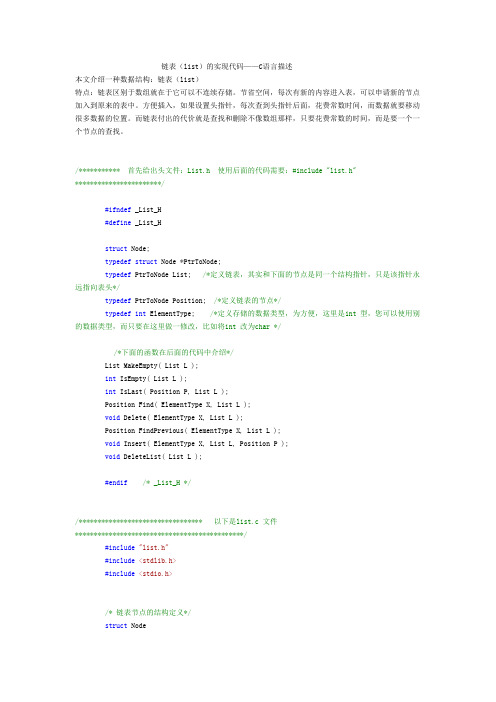
链表(list)的实现代码——C语言描述本文介绍一种数据结构:链表(list)特点:链表区别于数组就在于它可以不连续存储。
节省空间,每次有新的内容进入表,可以申请新的节点加入到原来的表中。
方便插入,如果设置头指针,每次查到头指针后面,花费常数时间,而数据就要移动很多数据的位置。
而链表付出的代价就是查找和删除不像数组那样,只要花费常数的时间,而是要一个一个节点的查找。
/*********** 首先给出头文件:List.h 使用后面的代码需要:#include "list.h"***********************/#ifndef _List_H#define _List_Hstruct Node;typedef struct Node *PtrToNode;typedef PtrToNode List; /*定义链表,其实和下面的节点是同一个结构指针,只是该指针永远指向表头*/typedef PtrToNode Position; /*定义链表的节点*/typedef int ElementType; /*定义存储的数据类型,为方便,这里是int 型,您可以使用别的数据类型,而只要在这里做一修改,比如将int 改为char *//*下面的函数在后面的代码中介绍*/List MakeEmpty( List L );int IsEmpty( List L );int IsLast( Position P, List L );Position Find( ElementType X, List L );void Delete( ElementType X, List L );Position FindPrevious( ElementType X, List L );void Insert( ElementType X, List L, Position P );void DeleteList( List L );#endif/* _List_H *//********************************* 以下是list.c 文件*********************************************/#include"list.h"#include<stdlib.h>#include<stdio.h>/* 链表节点的结构定义*/struct Node{ElementType Element;Position Next;};/*使链表清空,List这种数据类型定义在头文件中:typedef PtrToNode List; */ListMakeEmpty( List L ){if( L != NULL )DeleteList( L );L = (struct Node *)malloc( sizeof( struct Node ) );if( L == NULL )printf( "Out of memory!" );L->Next = NULL;return L;}/* 检查链表是否为空,因为L指向头指针,而头指针不存放数据(方便插入:每次插在头指针后面,花费常数时间),所以检验L->Next,如果是NULL(空),返回*/intIsEmpty( List L ){return L->Next == NULL;}/* 检查P所指向的节点是不是最后一个位置,如果是,则P->Next 是NULL,此时返回,Position 这种数据类型定义在头文件中:typedef PtrToNode Position;*/int IsLast( Position P, List L ){return P->Next == NULL;}/* 返回关键词X所在的位置;如果未找到X,则返回NULL */PositionFind( ElementType X, List L ){Position P;P = L->Next;while( P != NULL && P->Element != X )P = P->Next;return P;}/* 在链表L中,删除关键词X所在的节点*/voidDelete( ElementType X, List L ){Position P, TmpCell;P = FindPrevious( X, L ); /*找X所在节点的前一个位置,以便于删除。
c语言中操作list的方法
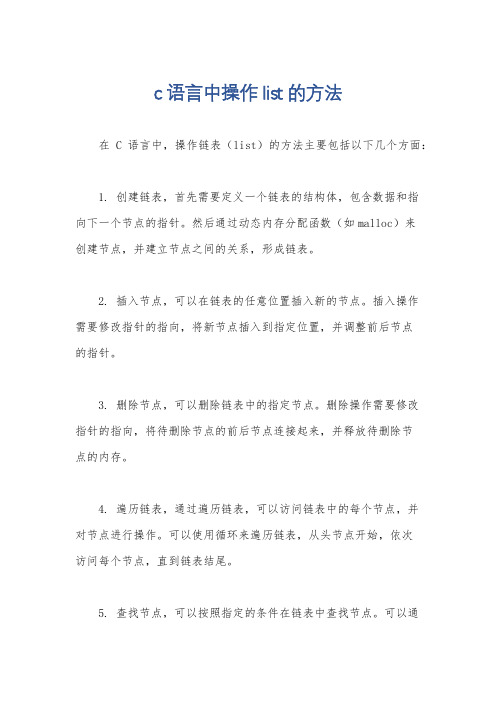
c语言中操作list的方法
在C语言中,操作链表(list)的方法主要包括以下几个方面:
1. 创建链表,首先需要定义一个链表的结构体,包含数据和指
向下一个节点的指针。
然后通过动态内存分配函数(如malloc)来
创建节点,并建立节点之间的关系,形成链表。
2. 插入节点,可以在链表的任意位置插入新的节点。
插入操作
需要修改指针的指向,将新节点插入到指定位置,并调整前后节点
的指针。
3. 删除节点,可以删除链表中的指定节点。
删除操作需要修改
指针的指向,将待删除节点的前后节点连接起来,并释放待删除节
点的内存。
4. 遍历链表,通过遍历链表,可以访问链表中的每个节点,并
对节点进行操作。
可以使用循环来遍历链表,从头节点开始,依次
访问每个节点,直到链表结尾。
5. 查找节点,可以按照指定的条件在链表中查找节点。
可以通
过遍历链表,逐个比较节点的值,找到符合条件的节点。
6. 修改节点,可以修改链表中指定节点的值。
可以通过遍历链表,找到需要修改的节点,然后修改节点的值。
7. 销毁链表,当链表不再使用时,需要释放链表所占用的内存空间。
可以通过遍历链表,释放每个节点的内存,最后释放链表的头节点。
总结起来,操作链表的方法包括创建链表、插入节点、删除节点、遍历链表、查找节点、修改节点和销毁链表。
通过这些方法,可以对链表进行各种操作,实现对数据的存储和处理。
在具体实现过程中,需要注意指针的操作和内存管理,确保链表的正确性和高效性。
c语言list函数

c语言list函数在C语言中,列表(List)是一种常见的数据结构,用于存储一系列有序的元素。
C语言标准库提供了许多用于操作列表的函数,其中包括一些用于创建、遍历和操作列表的函数。
本文将介绍C语言中常用的列表函数,帮助读者更好地理解和使用列表数据结构。
一、创建列表在C语言中,可以使用多种方式创建列表。
其中,最常用的是使用数组和结构体。
1. 数组创建列表使用数组创建列表非常简单,只需要指定数组的大小即可。
例如,创建一个大小为5的整数数组,用于存储一组有序的数字:```cint list[5];list[0] = 1;list[1] = 2;list[2] = 3;list[3] = 4;list[4] = 5;```此时,数组中的元素就形成了一个简单的列表。
可以使用循环遍历该列表,并使用数组下标访问每个元素。
2. 结构体创建列表除了使用数组,还可以使用结构体创建列表。
结构体是一种自定义的数据类型,可以包含多个不同类型的数据成员。
例如,创建一个包含字符串和整数的结构体,用于存储一组有序的数据:```ctypedef struct {char *str;int num;} ListElement;ListElement list[5];list[0].str = "Hello";list[0].num = 1;list[1].str = "World";list[1].num = 2;```此时,结构体中的成员就组成了一个简单的列表。
可以使用结构体指针遍历该列表,并访问每个元素。
二、操作列表C语言标准库提供了许多用于操作列表的函数,包括添加、删除、遍历等操作。
以下是一些常用的列表函数:1. `append()` 函数:将一个元素添加到列表的末尾。
可以使用该函数将一个整数添加到之前创建的结构体列表末尾:```clist[n].num++; // 将当前列表中的所有元素加1```可以使用指针来调用该函数,例如:`append(&list[n], num)`。
c实现的list

c实现的list // clist.cpp : 定义控制台应⽤程序的⼊⼝点。
//#include "stdafx.h"#include <stdio.h>#include <malloc.h> //动态分配内存#include <stdlib.h> //exit 函数#include <stdbool.h> //布尔值函数#include <string.h>struct node {char * str;int len;node* next;//node* first;//node* last;};node* first = NULL;node* last = NULL;node* prev = NULL;node* search(const char * str);/*node* del(node* list) {if (list == NULL)return;free(list->str);return list->next;}*/node* new_node() {node* n = (node*)malloc(sizeof(node) + 1);return n;}node* first_node() {return first;}node* last_node() {return last;}void delete_node(const char* str) {node* node_to_del = search(str);prev->next = node_to_del->next;free(node_to_del->str);node_to_del->str = NULL;free(node_to_del);node_to_del = NULL;prev = first;}void update(const char* str, const char* newstr) {node* node_to_update = search(str);strcpy(node_to_update->str, newstr);node_to_update->len = strlen(newstr);node_to_update->str[node_to_update->len] = '\0';}node* list_init(const char* first_string) {first = last = new_node();first->str = (char*)malloc(sizeof(first_string)+1);strcpy(first->str, first_string);first->len = strlen(first_string);first->str[first->len] = '\0';first->next = NULL;return first;}void add(const char * str) {node* n = new_node();n->str = (char*)malloc(sizeof(str)+1);n->next = NULL;n->len = strlen(str);n->str[n->len] = '\0';strcpy(n->str, str);last->next = n;last = n;}node* search(const char * str) {node* n = first;prev = first;while (n != NULL && n->next != NULL) { if (strcmp(str, n->str) == 0)return n;prev = n;n = n->next;}return n;}int main(){node* head = list_init("head");add("hello,first");add("hello,second");add("cc");add("dd");add("ee");add("ea");add("eb");add("ec");add("ed");add("ee");node* dd_node = search("ee");delete_node("ee");update("hello,second", "second");return 0;}。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
public:
void clear();
~List() {this->clear();}
public:
class Iterator
{
friend class List<T>;
protected:
node *Iter;
Iterator(node *iter)
if(i>=size)
{
cout<<"Overflow error!!"<<endl;
exit(0);
}
{
T data;
node *next;
node *last;
};
private:
node *head;
node *rear;
int size;
public: //构造函数
List();
List(const List<T>&);
List(T,int );
{
Iter =Iter->last;
return *this;
}
Iterator &operator = (Iterator& it)
{
Iter =it.Iter;
return *this;
head=p1;head->last=NULL;
rear=p1;rear->next=NULL; size=1;
return *this;
}
node *p0=list.head;
node *p2=(node*)malloc(sizeof(node));
#include<iostream>
#include<stdlib.h>
#include<string>
using namespace std;
template <class T>
class List
{
friend class Iterator;
protected:
struct node
return Iter!=iter.Iter;
}
T operator *()
{
return Iter->data;
}
Iterator &operator[] (int n)
{
for(int i=0;i<n;i++)
rear->next=NULL;
node *p1=p4->next,*p3=p4;
while(p1->next!=NULL)
{
p1->last=p3;
p1=p1->next;
p3=p3->next;
}
T &back() {return rear->data;}
bool empty(){ return size==0;}
void link(List<T> &list0){ *this+=list0;}
void reset(Iterator &it,T val){ (it.Iter)->data=val;}
}
bool operator ==(const Iterator &iter)
{
return Iter==iter.Iter;
}
bool operator !=(const Iterator &iter)
{
this->rear=NULL;
return *this;
}
if(list.size==1)
{
node *p1=(node*)malloc(sizeof(node));
p1->data=list.head->data;
head->last=NULL;free(tp);
return Iterator(head);
}
if(it.Iter==rear)
{
node *tp=rear;
rear=rear->last;
rear->next=NULL;free(tp);
p1->last=p3;
size+=list.size;
}
T &operator[] (int i)
{
if(i>=size)
{
cout<<"Overflow error!!"<<endl;
exit(0);
}
{
node *p0=list.head;
node *p2=(node*)malloc(sizeof(node));
p2->data=p0->data;
node *p4=rear;
rear->next=p2; rear=p2;
while(p0->next!=NULL)
}
return Iterator(NULL);
}
Iterator begin(){ return Iterator(head);}
Iterator end(){ return Iterator(NULL);}
T &front(){return head->data;}
{
p0=p0->next;
node *p2=(node*)malloc(sizeof(node));
p2->data=p0->data;
rear->next=p2; rear=p2;
}
return Iterator(head);
}
(it.Iter->last)->next=it.Iter->next;
(it.Iter->next)->last=it.Iter->last;
node *ans=it.Iter->next; free(it.Iter);
}
};
public: //功能函数
List<T> sublist(Iterator&, Iterator&);
List<T> sublist( Iterator&,int);
List<T> sublist( Iterator&);
void push_back(const T);
p2->data=p0->data;
head=p2;rear=p2;
head->last=NULL;
while(p0->next!=NULL)
{
p0=p0->next;
node *p2=(node*)malloc(sizeof(node));
return Iterator(ans);
}
Iterator find(T val)
{
node *tp=head;
for(;tp!=NULL;tp=tp->next)
{
if((tp->data)==val) return Iterator(tp);
{
if(Iter==NULL)
{
cout<<"Overflow error!!"<<endl;
exit(0);
}
Iter=Iter->next;
}
return *this;
(it2.Iter)->data=tp;
}
Iterator erase(Iterator &it)
{
if(size==0)
{
cout<<"List is empty!!"<<endl;
exit(0);
}
size--;
if(size==0)
bool down_order(); //降序
int get_size(){ return size;}
friend void swap(Iterator &it1,Iterator &it2)
{
T tp=(it1.Iter)->data;
(it1.Iter)->data=(it2.Iter)->data;
p1->last=p3;
p1=p1->next;
p3=p3->next;
}
p1->last=p3;
size=list.size;
return *this;
}
void operator += (const List<T>&list)
p2->data=p0->data;
rear->next=p2; rear=p2;
}
rear->next=NULL;
node *p1=head->next,*p3=head;
while(p1->next!=NULL)
{
{
Iter=iter;
}
public:
Iterator(){}