表达式二叉树·实验代码
二叉查找树实现代码及运行结果

temp=SearchBST(T,keyword,NULL,p);//查找
if(!temp) printf("%d isn't existed!\n",keyword); //没有找到
else printf("%d has been found!\n",keyword); //成功找到
}
else return 0; //树中已存在关键字为e的数据元素
}
int DeleteBST(BiTree &T,int key)
{//若二叉排序树T中存在关键字等于key的数据元素时,则删除该数据元素结点
//并返回1,否则返回0
int tmp1,tmp2;
tmp1=tmp2=0;
if(!T) return 0; //不存在关键字等于key的数据元素
//则指针p指向该数据元素,并返回1,否则指针指向查找路径上访问的最后一
//个结点并返回0,指针f指向T的双亲,其初始调用值为NULL
int tmp1,tmp2;
tmp1=tmp2=0;
if(!T) {p=f;return 0;} //查找不成功
else if(key==T->data) {p=T;return 1;} //查找成功
scanf("%d",&n);
printf("Please input every node:\n");
for(int i=0;i<n;i++)//输入树的结点个数
scanf("%d",&A[i]);
二叉树实现源代码
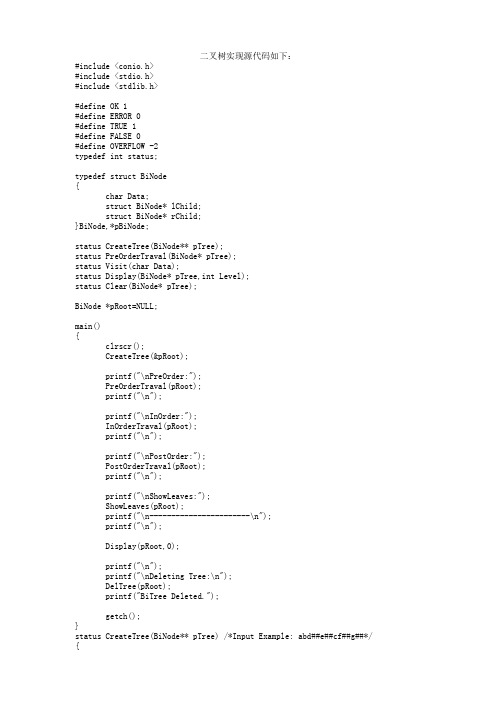
二叉树实现源代码如下:#include <conio.h>#include <stdio.h>#include <stdlib.h>#define OK 1#define ERROR 0#define TRUE 1#define FALSE 0#define OVERFLOW -2typedef int status;typedef struct BiNode{char Data;struct BiNode* lChild;struct BiNode* rChild;}BiNode,*pBiNode;status CreateTree(BiNode** pTree);status PreOrderTraval(BiNode* pTree);status Visit(char Data);status Display(BiNode* pTree,int Level);status Clear(BiNode* pTree);BiNode *pRoot=NULL;main(){clrscr();CreateTree(&pRoot);printf("\nPreOrder:");PreOrderTraval(pRoot);printf("\n");printf("\nInOrder:");InOrderTraval(pRoot);printf("\n");printf("\nPostOrder:");PostOrderTraval(pRoot);printf("\n");printf("\nShowLeaves:");ShowLeaves(pRoot);printf("\n-----------------------\n");printf("\n");Display(pRoot,0);printf("\n");printf("\nDeleting Tree:\n");DelTree(pRoot);printf("BiTree Deleted.");getch();}status CreateTree(BiNode** pTree) /*Input Example: abd##e##cf##g##*/ {char ch;scanf("%c",&ch);if(ch==‘#‘){(*pTree)=NULL;}else{if(!((*pTree)=(BiNode*)malloc(sizeof(BiNode)))) {exit(OVERFLOW);}(*pTree)->Data=ch;CreateTree(&((*pTree)->lChild));CreateTree(&((*pTree)->rChild));}return OK;}status PreOrderTraval(BiNode* pTree){if(pTree){if(Visit(pTree->Data)){if(PreOrderTraval(pTree->lChild)){if(PreOrderTraval(pTree->rChild)){return OK;}}}return ERROR;}else{return OK;}}status InOrderTraval(BiNode* pTree){if(pTree){if(InOrderTraval(pTree->lChild)){if(Visit(pTree->Data)){if(InOrderTraval(pTree->rChild)){return OK;}}return ERROR;}return ERROR;}else{return OK;}}status PostOrderTraval(BiNode* pTree){if(pTree){if(PostOrderTraval(pTree->lChild)){if(PostOrderTraval(pTree->rChild)){if(Visit(pTree->Data)){return OK;}return ERROR;}}return ERROR;}else{return OK;}}status Visit(char Data){printf("%c",Data);return OK;}status Display(BiNode* pTree,int Level){int i;if(pTree==NULL) return;Display(pTree->lChild,Level+1);for(i=0;i<Level-1;i++){printf(" ");}if(Level>=1){printf("--");}printf("%c\n",pTree->Data);Display(pTree->rChild,Level+1);}status ShowLeaves(BiNode* pTree){if(pTree){if(ShowLeaves(pTree->lChild)){if(ShowLeaves(pTree->rChild)){if((pTree->lChild==NULL)&&(pTree->rChild==NULL)) {if(!Visit(pTree->Data)){return ERROR;}}return OK;}}return ERROR;}else{return OK;}}status DelTree(BiNode* pTree){if(pTree){if(DelTree(pTree->lChild)){if(DelTree(pTree->rChild)){printf("Deleting %c\n",pTree->Data); free((void*)pTree);return OK;}}return ERROR;}else{return OK;}}。
数据结构实验二叉树C完整代码

#include<iostream>using namespace std;const int MAXSIZE = 100 ;template <class T > struct BiNode //二叉链表结点{T data;//数据域BiNode<T>* lch;//左指针域BiNode<T>* rch;//右指针域};template <class T> class BiTree //二叉树的实现{public:void Create (BiNode<T> * &R,T data[] ,int i);//创建二叉树void Release(BiNode<T> *R); //释放二叉树BiNode<T> *root; //根节点BiTree(T a[]){Create(root,a,1);};//构造函数BiNode<T> * Getroot(){return root;};//根节点地址返回void PrintWay(T data, T a[]);void PreOrder(BiNode<T> *R); //前序遍历void InOrder(BiNode<T> *R); //中序遍历void LevelOrder(BiNode<T> *R);//层序遍历void PostOrder(BiNode<T> *R); //后序遍历~BiTree();//析构函数int GetDepth(BiNode<T> *R,int d);//求二叉树深度void GetPath(T x,BiNode<T>*R);//求指定结点到根结点的路径};template <class T> void BiTree <T>::Create (BiNode<T> * &R,T data[] ,int i)//顺序结构存储二叉链表{//i表示位置,从1开始if(data[i-1]!=0){R=new BiNode<T>;//创建根节点R->data=data[i-1];Create(R->lch,data,2*i);//创建左子树Create(R->rch,data,2*i+1);//创建右子树}elseR=NULL;}template <class T> void BiTree <T>::PreOrder(BiNode<T> *R)//前序遍历的实现{if(R!=NULL){cout<<R->data;//访问结点PreOrder(R->lch);//遍历左子树PreOrder(R->rch);//遍历右子树}}template <class T> void BiTree <T>::InOrder(BiNode<T> *R)//中序遍历的实现{if(R!=NULL){PreOrder(R->lch);//遍历左子树cout<<R->data;//访问结点PreOrder(R->rch);//遍历右子树}}template <class T> void BiTree <T>::PostOrder(BiNode<T> *R)//后序遍历的实现{if(R!=NULL){PreOrder(R->lch);//遍历左子树PreOrder(R->rch);//遍历右子树cout<<R->data;//访问结点}}template <class T> void BiTree <T>::LevelOrder(BiNode<T> *R)//层序遍历的实现{BiNode<T> * queue[MAXSIZE];//利用队列实现层序遍历int f = 0,r = 0;//初始化空队列if(R!=NULL)queue[++r] = R;while(f!=r){BiNode<T> *p=queue[++f];//队头元素出列cout<<p->data;if(p->lch!=NULL)queue[++r]=p->lch;if(p->rch!=NULL)queue[++r]=p->rch;}}template <class T> void BiTree<T>::Release(BiNode<T> *R)//释放二叉树{if (R!=NULL){Release(R->lch);Release(R->rch);delete R;}}template <class T> BiTree<T>::~BiTree() //调用Release函数{Release(root);}template <class T> int BiTree<T>::GetDepth(BiNode<T> *R,int d) //求二叉树的深度{if (R==NULL) return d;if ((R->lch==NULL) && (R->rch==NULL))return d+1;else{int m = GetDepth(R->lch,d+1);int n = GetDepth(R->rch,d+1);return n>m? n:m;}}template<class T> void BiTree<T>::PrintWay(T data, T a[])//打印路径{int x;for(int i=1;i<100;i++){if(a[i-1]==data)//数组中各元素和指定元素比较、查找所在位置i{x=i;break;}}cout<<"该结点到根结点的路径为:"<<endl;while(x!=0){cout<<a[x-1]<<endl;x/=2;//子节点对应父节点编号}}void main(){char buf[MAXSIZE]={'j','u','s','t','l','o','v','e','u','f','o','r','e','v','e','r'};BiTree <char> Test(buf);BiNode<char> * A = Test.Getroot();cout<<"前序遍历:";Test.PreOrder(A);cout<<endl;cout<<"中序遍历:";Test.InOrder(A);cout<<endl;cout<<"后续遍历:";Test.PostOrder(A);cout<<endl;cout<<"层序遍历:";Test.LevelOrder(A);cout<<endl;int depth=0;cout<<"深度为";cout<<Test.GetDepth(A,depth)<<endl;cout<<endl;Test.PrintWay('f',buf);}。
c语言二叉树代码
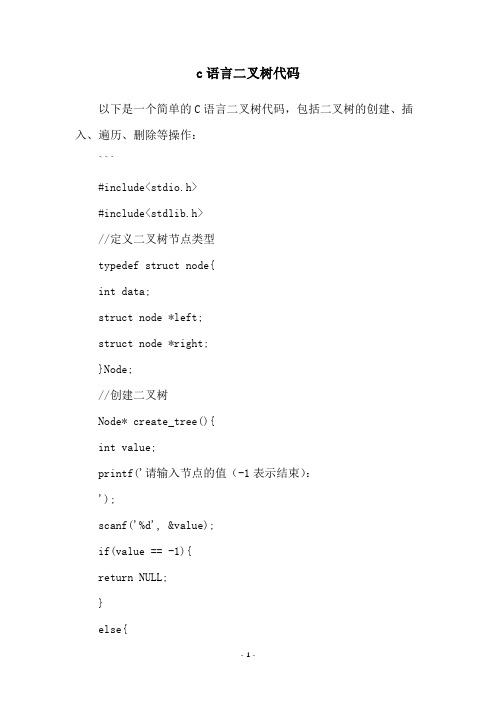
c语言二叉树代码以下是一个简单的C语言二叉树代码,包括二叉树的创建、插入、遍历、删除等操作:```#include<stdio.h>#include<stdlib.h>//定义二叉树节点类型typedef struct node{int data;struct node *left;struct node *right;}Node;//创建二叉树Node* create_tree(){int value;printf('请输入节点的值(-1表示结束):');scanf('%d', &value);if(value == -1){return NULL;}else{Node *p = (Node*)malloc(sizeof(Node));p->data = value;printf('请输入%d的左子节点:', value);p->left = create_tree();printf('请输入%d的右子节点:', value);p->right = create_tree();return p;}}//插入节点Node* insert_node(Node *root, int value){if(root == NULL){Node *p = (Node*)malloc(sizeof(Node));p->data = value;p->left = NULL;p->right = NULL;return p;}else if(value < root->data){root->left = insert_node(root->left, value);}else if(value > root->data){root->right = insert_node(root->right, value); }return root;}//先序遍历void preorder_traversal(Node *root){if(root != NULL){printf('%d ', root->data);preorder_traversal(root->left);preorder_traversal(root->right);}}//中序遍历void inorder_traversal(Node *root){if(root != NULL){inorder_traversal(root->left);printf('%d ', root->data);inorder_traversal(root->right);}}//后序遍历void postorder_traversal(Node *root){if(root != NULL){postorder_traversal(root->left);postorder_traversal(root->right);printf('%d ', root->data);}}//查找节点Node* search_node(Node *root, int value){ if(root == NULL){return NULL;}else if(root->data == value){return root;}else if(value < root->data){return search_node(root->left, value);}else{return search_node(root->right, value); }}//删除节点Node* delete_node(Node *root, int value){if(root == NULL){return NULL;}else if(value < root->data){root->left = delete_node(root->left, value); }else if(value > root->data){root->right = delete_node(root->right, value); }else{//情况一:被删除节点没有子节点if(root->left == NULL && root->right == NULL){ free(root);root = NULL;}//情况二:被删除节点只有一个子节点else if(root->left == NULL){Node *temp = root;root = root->right;free(temp);}else if(root->right == NULL){Node *temp = root;root = root->left;free(temp);}//情况三:被删除节点有两个子节点else{Node *temp = root->right;while(temp->left != NULL){temp = temp->left;}root->data = temp->data;root->right = delete_node(root->right, temp->data); }}return root;}//主函数int main(){Node *root = NULL;int choice, value;while(1){printf('请选择操作: ');printf('1.创建二叉树 ');printf('2.插入节点');printf('3.遍历二叉树 ');printf('4.查找节点');printf('5.删除节点');printf('6.退出程序');scanf('%d', &choice); switch(choice){case 1:root = create_tree(); break;case 2:printf('请输入要插入的节点值:');scanf('%d', &value);root = insert_node(root, value);break;case 3:printf('先序遍历:');preorder_traversal(root);printf('中序遍历:');inorder_traversal(root);printf('后序遍历:');postorder_traversal(root);printf('');break;case 4:printf('请输入要查找的节点值:');scanf('%d', &value);Node *result = search_node(root, value);if(result != NULL){printf('找到节点:%d', result->data);}else{printf('未找到节点:%d', value);}break;case 5:printf('请输入要删除的节点值:');scanf('%d', &value);root = delete_node(root, value); break;case 6:printf('程序已退出。
表达式类型的实现(二叉树)
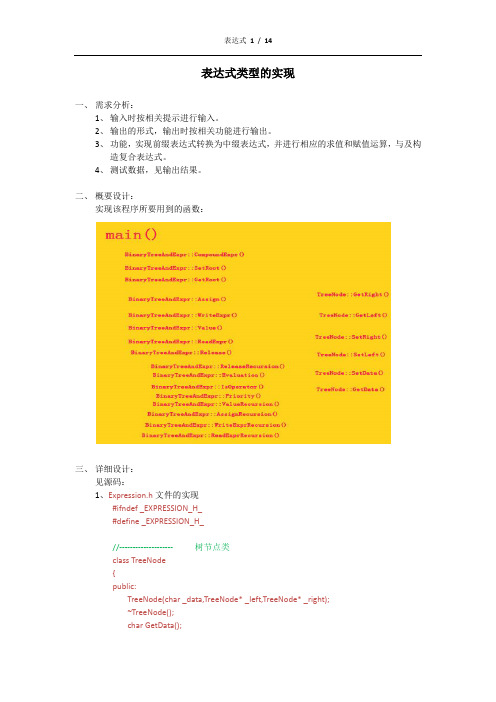
表达式类型的实现一、需求分析:1、输入时按相关提示进行输入。
2、输出的形式,输出时按相关功能进行输出。
3、功能,实现前缀表达式转换为中缀表达式,并进行相应的求值和赋值运算,与及构造复合表达式。
4、测试数据,见输出结果。
二、概要设计:实现该程序所要用到的函数:三、详细设计:见源码:1、Expression.h文件的实现#ifndef _EXPRESSION_H_#define _EXPRESSION_H_//-------------------- 树节点类class TreeNode{public:TreeNode(char _data,TreeNode* _left,TreeNode* _right);~TreeNode();char GetData();void SetData(char _data);void SetLeft(TreeNode* _left);void SetRight(TreeNode* _right);TreeNode* GetLeft();TreeNode* GetRight();private:char Data;TreeNode* left,*right;};//-------------------- 二叉树几及表达式类class BinaryTreeAndExpr{public:BinaryTreeAndExpr();~BinaryTreeAndExpr();TreeNode* GetRoot();void SetRoot(TreeNode* _root);void ReadExpr(char* E);void WriteExpr(char* E);void Assign(char v,int c);static int Value(char* E);static BinaryTreeAndExpr* CompoundExpr(char p,char* E1,char* E2);void Release();private:void ReleaseRecursion(TreeNode* &p);void ReadExprRecursion(TreeNode* &p,char* E);void WriteExprRecursion(TreeNode* p,char* E);void AssignRecursion(TreeNode* p,char v,int c);int ValueRecursion(TreeNode* p);int Priority(char c1,char c2);bool IsOperator(char c);int Evaluation(int a,char op,int b);TreeNode* root;int Expr_i,Expr_len;};#endif2、Expression.cpp文件的实现#include<iostream>#include<cmath>#include"Expression.h"using namespace std;//----------------------树节点类成员函数TreeNode::TreeNode(char _data,TreeNode* _left,TreeNode* _right) {Data=_data;left=_left;right=_right;}TreeNode::~TreeNode(){}char TreeNode::GetData(){return Data;}void TreeNode::SetLeft(TreeNode* _left){left=_left;}void TreeNode::SetRight(TreeNode* _right){right=_right;}TreeNode* TreeNode::GetLeft(){return left;}TreeNode* TreeNode::GetRight(){return right;}void TreeNode::SetData(char _data){Data=_data;}//---------------------------- 二叉树几及表达式类成员函数BinaryTreeAndExpr::BinaryTreeAndExpr():root(NULL){Expr_i=Expr_len=0;}BinaryTreeAndExpr::~BinaryTreeAndExpr(){}void BinaryTreeAndExpr::Release(){if(root!=NULL){ReleaseRecursion(root);delete(root);root=NULL;}}void BinaryTreeAndExpr::ReleaseRecursion(TreeNode* &p) {if(p->GetLeft()!=NULL){TreeNode* p1;p1=p->GetLeft();ReleaseRecursion(p1);delete(p1);}else if(p->GetRight()!=NULL){TreeNode*p2;p2=p->GetRight();ReleaseRecursion(p2);delete(p2);}p=NULL;}TreeNode* BinaryTreeAndExpr::GetRoot(){return root;}void BinaryTreeAndExpr::ReadExpr(char* E){if(root!=NULL) {Release();root=NULL;}Expr_i=0;Expr_len=strlen(E);if(Expr_len==0) return ;ReadExprRecursion(root,E);}void BinaryTreeAndExpr::ReadExprRecursion(TreeNode* &p,char* E) {if(Expr_i==Expr_len)return ;p=(TreeNode*)new TreeNode(E[Expr_i++],NULL,NULL);char temp=p->GetData();if(!IsOperator(temp)) return ;else{TreeNode* q1,* q2;ReadExprRecursion(q1,E);p->SetLeft(q1);ReadExprRecursion(q2,E);p->SetRight(q2);}}void BinaryTreeAndExpr::WriteExpr(char* E){if(root==NULL) {E[0]='\0';return ;}WriteExprRecursion(root,E);}void BinaryTreeAndExpr::WriteExprRecursion(TreeNode* p,char* E) {char c1,c2,c3[100],c4[100];if(p->GetLeft()==NULL || p->GetRight()==NULL){E[0]=p->GetData();E[1]='\0';return ;}c1=p->GetLeft()->GetData();c2=p->GetRight()->GetData();if(!IsOperator(c1) && !IsOperator(c2)){E[0]=c1;E[1]=p->GetData();E[2]=c2;E[3]='\0';}else if(IsOperator(c1) && !IsOperator(c2)){WriteExprRecursion(p->GetLeft(),c3);if(Priority(p->GetData(),p->GetLeft()->GetData())>0){E[0]='(';for(int i=0;i<strlen(c3);i++) E[i+1]=c3[i];E[i+1]=')';E[i+2]=p->GetData();E[i+3]=p->GetRight()->GetData();E[i+4]='\0';}else{for(int i=0;i<strlen(c3);i++) E[i]=c3[i];E[i]=p->GetData();E[i+1]=p->GetRight()->GetData();E[i+2]='\0';}}else if(!IsOperator(c1) && IsOperator(c2)){WriteExprRecursion(p->GetRight(),c3);if(Priority(p->GetData(),p->GetRight()->GetData())>0) {E[0]=p->GetLeft()->GetData();E[1]=p->GetData();E[2]='(';for(int i=0;i<strlen(c3);i++) E[i+3]=c3[i];E[i+3]=')';E[i+4]='\0';}else{E[0]=p->GetLeft()->GetData();E[1]=p->GetData();for(int i=0;i<strlen(c3);i++) E[i+2]=c3[i];E[i+2]='\0';}}else{WriteExprRecursion(p->GetLeft(),c3);WriteExprRecursion(p->GetRight(),c4);if(Priority(p->GetData(),p->GetLeft()->GetData())>0){E[0]='(';for(int i=0;i<strlen(c3);i++) E[i+1]=c3[i];E[i+1]=')';E[i+2]='\0';}else{for(int i=0;i<strlen(c3);i++) E[i]=c3[i];E[i]='\0';}int j=strlen(E);E[j]=p->GetData();if(Priority(p->GetData(),p->GetRight()->GetData())>0){E[j+1]='(';for(int i=0;i<strlen(c4);i++) E[j+2+i]=c4[i];E[j+2+i]=')';E[j+3+i]='\0';}else{for(int i=0;i<strlen(c4);i++) E[j+1+i]=c4[i];E[j+1+i]='\0';}}}int BinaryTreeAndExpr::Priority(char c1,char c2){switch(c1){case '+':case '-':return -1;case '*':switch(c2){case '+':case '-':return 1;}return -1;case '/':switch(c2){case '+':case '-':return 1;}return -1;case '^':return 1;}return 0;}bool BinaryTreeAndExpr::IsOperator(char c){return !(c>=97 && c<=122 || c>=48 && c<=57);}void BinaryTreeAndExpr::Assign(char v,int c){AssignRecursion(root,v,c);}void BinaryTreeAndExpr::AssignRecursion(TreeNode* p,char v,int c){if(p!=NULL){if(p->GetData()==v) p->SetData(c+48);AssignRecursion(p->GetLeft(),v,c);AssignRecursion(p->GetRight(),v,c);}}BinaryTreeAndExpr* BinaryTreeAndExpr::CompoundExpr(char p,char* E1,char* E2){BinaryTreeAndExpr BTAE1,BTAE2,*BTAE3;BTAE1.ReadExpr(E1);BTAE2.ReadExpr(E2);TreeNode* q=(TreeNode*)new TreeNode(p,NULL,NULL);q->SetLeft(BTAE1.GetRoot());q->SetRight(BTAE2.GetRoot());BTAE3=(BinaryTreeAndExpr*)new BinaryTreeAndExpr;BTAE3->SetRoot(q);return BTAE3;}void BinaryTreeAndExpr::SetRoot(TreeNode* _root){root=_root;}int BinaryTreeAndExpr::Value(char* E){BinaryTreeAndExpr btae;btae.ReadExpr(E);return btae.ValueRecursion(btae.GetRoot());}int BinaryTreeAndExpr::ValueRecursion(TreeNode* p){char c1,c2;int temp1,temp2;if(p->GetLeft()==NULL || p->GetRight()==NULL){c1=p->GetData();return (c1>=97 && c1<=122)?0:c1-48;}c1=p->GetLeft()->GetData();c2=p->GetRight()->GetData();if(!IsOperator(c1) && !IsOperator(c2)){if(c1>=97 && c1<=122) temp1=0;else temp1=c1-48;if(c2>=97 && c2<=122) temp2=0;else temp2=c2-48;return Evaluation(temp1,p->GetData(),temp2);}else if(IsOperator(c1) && !IsOperator(c2)){temp1=ValueRecursion(p->GetLeft());if(c2>=97 && c2<=122) temp2=0;else temp2=c2-48;return Evaluation(temp1,p->GetData(),temp2);}else if(!IsOperator(c1) && IsOperator(c2)){temp2=ValueRecursion(p->GetRight());if(c1>=97 && c1<=122) temp1=0;else temp1=c1-48;return Evaluation(temp1,p->GetData(),temp2);}else{temp1=ValueRecursion(p->GetLeft());temp2=ValueRecursion(p->GetRight());return Evaluation(temp1,p->GetData(),temp2);}}int BinaryTreeAndExpr::Evaluation(int a,char op,int b) {switch(op){case '+':return a+b;break;case '-':return a-b;break;case '*':return a*b;break;case '/':return a/b;break;case '^':return pow(a,b);break;}return 0;}3、ExpressionMain.cpp文件的实现#include<iostream>#include"Expression.h"using namespace std;int main(){BinaryTreeAndExpr btae,*btae1;char E1[100],E2[100],P,V;int switchs,c,switchs2;bool run=true,run2=true;while(run){cout<<"请选择功能,功能如下:"<<endl;cout<<"1.构造复合表达试并输出相应结果."<<endl;cout<<"2.输入前缀表达试并构造中缀表达试."<<endl;cout<<"3.对前缀表达试求值."<<endl;cout<<"4.退出."<<endl;cin>>switchs;switch(switchs){case 4:run=false;break;case 1:cout<<"请输入相关数据.前缀表达试"<<endl;getchar();scanf("%s %c %s",E1,&P,E2);btae1=BinaryTreeAndExpr::CompoundExpr(P,E1,E2);while(run2){cout<<"如有变量要赋值请输入1,否则输入2"<<endl;cin>>switchs2;if(switchs2==1){cout<<"请输入相关数据."<<endl;getchar();scanf("%c %d",&V,&c);btae1->Assign(V,c);}else run2=false;}btae1->WriteExpr(E1);cout<<"中缀表达试:"<<E1<<endl;btae1->Release();delete(btae1);run2=true;break;case 2:cout<<"请输入相关数据.前缀表达试"<<endl;cin>>E1;btae.ReadExpr(E1);while(run2){cout<<"如有变量要赋值请输入1,否则输入2"<<endl;cin>>switchs2;if(switchs2==1){cout<<"请输入相关数据."<<endl;getchar();scanf("%c %d",&V,&c);btae.Assign(V,c);}else run2=false;}cout<<"中缀表达试:";btae.WriteExpr(E2);cout<<E2<<endl;run2=true;break;case 3:cout<<"请输入相关数据.前缀表达试"<<endl;cin>>E1;btae.ReadExpr(E1);btae.WriteExpr(E2);cout<<"中缀表达试为:";cout<<E2<<endl;cout<<"计算结果为:";cout<<BinaryTreeAndExpr::Value(E1)<<endl;break;default:cout<<"你的输入无效!请重新输入."<<endl;break;}btae.Release();if(run) cout<<endl;}return 0;}四、调式分析:1、调式时递归函数不正确,出现一些逻辑错误,经改正后最终得到了正确的结果。
二叉树求解表达式值的代码编写。

二叉树求解表达式值的代码编写。
二叉树求解表达式值的代码编写是算法领域中的重要问题之一。
在本文中,我将一步一步解释如何编写这样的代码,并提供一个示例来说明算法的实现过程。
首先,让我们明确一下二叉树求解表达式值的问题。
给定一个二叉树,其中每个节点都是一个运算符或是一个操作数,我们需要计算整个表达式的值。
例如,考虑以下二叉树:*/ \+ -/ \ / \3 24 5在这个例子中,根节点是乘法运算符“*”,左子树是加法运算符“+”,右子树是减法运算符“-”,叶子节点是操作数3,2,4和5。
我们的目标是计算整个表达式的值,即(3+2) * (4-5) = 5 * -1 = -5.在解决这个问题之前,我们首先需要定义二叉树的节点。
每个节点可以表示一个运算符或是一个操作数。
我们可以使用面向对象的思想来实现这个节点类。
以下是一个简单的节点类的示例代码:pythonclass Node:def __init__(self, val):self.val = valself.left = Noneself.right = None在这个示例中,我们定义了一个Node类,它有一个val属性表示节点的值,并有left和right属性表示节点的左子树和右子树。
接下来,我们需要编写一个递归的求解表达式值的函数。
该函数将以一个二叉树节点作为参数,并返回该节点所表示的子树的表达式值。
以下是求解表达式值的函数的示例代码:pythondef evaluate_expression(root):# 如果节点为空,返回0if root is None:return 0# 如果为叶子节点,返回节点的值if root.left is None and root.right is None: return root.val# 递归求解左子树和右子树的表达式值left_value = evaluate_expression(root.left) right_value = evaluate_expression(root.right)# 根据节点的值进行相应的运算if root.val == '+':return left_value + right_valueelif root.val == '-':return left_value - right_valueelif root.val == '*':return left_value * right_valueelif root.val == '/':return left_value / right_valueelse:raise ValueError("Invalid operator")在这个示例中,我们首先检查节点是否为空,如果为空,则返回0。
(数据结构)二叉树实验源代码 二叉树各种遍历 叶子结点 分枝数 单分枝 双分枝 深度
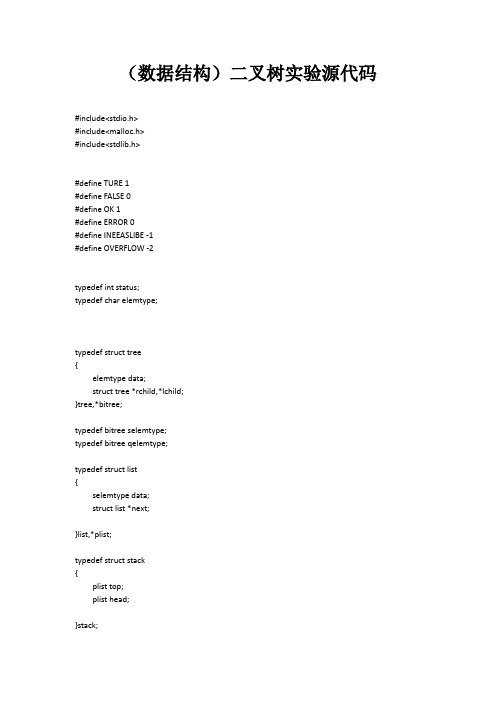
(数据结构)二叉树实验源代码#include<stdio.h>#include<malloc.h>#include<stdlib.h>#define TURE 1#define FALSE 0#define OK 1#define ERROR 0#define INEEASLIBE -1#define OVERFLOW -2typedefint status;typedef char elemtype;typedefstruct tree{elemtype data;struct tree *rchild,*lchild;}tree,*bitree;typedefbitreeselemtype;typedefbitreeqelemtype;typedefstruct list{selemtype data;struct list *next;}list,*plist;typedefstruct stack{plist top;plist head;}stack;typedefstruct node{qelemtype data;struct node *next;}node,*queueptr;typedefstruct{queueptr front;queueptr rear;}linkqueue;#include"header.h"statuscreatbitree(bitree&T){elemtype e;printf("请按先序输入二叉树:\n");scanf("%c",&e);getchar();if(e=='#')T=NULL;else{T=(bitree)malloc(sizeof(tree));if(!T){exit(0);}T->data=e;creatbitree(T->lchild);creatbitree(T->rchild);}return OK;}statuspretraverse(bitree T){if(T==NULL)return OK;else{printf("\t%c",T->data);pretraverse(T->lchild);pretraverse(T->rchild);}}statuscentertraverse(bitree T){if(T==NULL)return OK;else{centertraverse(T->lchild);printf("\t%c",T->data);centertraverse(T->rchild);}}statusnexttraverse(bitree T){if(T==NULL)return OK;else{nexttraverse(T->lchild);nexttraverse(T->rchild);printf("\t%c",T->data);}}statusinitstack(stack &s){s.head=(plist)malloc(sizeof(list));if(s.top==NULL)return ERROR;s.top=s.head;s.top->next=NULL;return OK;}status push(stack &s,selemtype e) {plist p;p=(plist)malloc(sizeof(list));p->data=e;p->next=s.head->next;s.head->next=p;s.top=p;return OK;}status pop(stack &s,selemtype&e){if(s.top==s.head){printf("the stack is no elemt\n");return ERROR;}e=s.top->data;s.head->next=s.top->next;plist p;p=s.top;if(p->next==NULL)s.top=s.head;elses.top=s.head->next;free(p);return OK;}statusstackemtpy(stack s){if(s.top==s.head){return OK;}else{return ERROR;}}statusgettop(stack s,selemtype&p){if(s.head==s.top)return ERROR;elsep=s.top->data;return OK;}statuscenterstrack(bitree T){stack s;initstack(s);push(s,T);bitree c;while(!stackemtpy(s)){while(gettop(s,c)&&c){push(s,c->lchild);}pop(s,c);if(!stackemtpy(s)){pop(s,c);printf("\t%c",c->data);push(s,c->rchild);}}return OK;}statusinitqueue(linkqueue&q){q.front=q.rear=(queueptr)malloc(sizeof(node));if(!q.front)return ERROR;q.front->next=NULL;return OK;}statusenqueue(linkqueue&q,qelemtype e) {queueptr p;p=(queueptr)malloc(sizeof(node));if(!p)return ERROR;p->data=e;p->next=NULL;q.rear->next=p;q.rear=p;return OK;}statusdequeue(linkqueue&q,qelemtype&e) {if(q.front==q.rear){printf("该队列中没有数据元素\n");return ERROR;}e=q.front->next->data;queueptr p;p=q.front->next;if(p==q.rear){q.rear=q.front;q.rear->next=NULL;}elseq.front->next=p->next;free(p);return OK;}statuslayertraverse(bitree T){linkqueue queue;initqueue(queue);if(T==NULL)return ERROR;bitree c=T;enqueue(queue,c);while(1)dequeue(queue,c);printf("\t%c",c->data);if(c->lchild!=NULL)enqueue(queue,c->lchild);if(c->rchild!=NULL)enqueue(queue,c->rchild);if(queue.front==queue.rear)break;}return OK;}statusleafcout(bitree T){if(T==NULL)return 0;else if(T->lchild==NULL&&T->rchild==NULL)return 1;else{returnleafcout(T->rchild)+leafcout(T->lchild);}}statusbranchcout(bitree T){if(T==NULL||(T->rchild==NULL&&T->lchild==NULL))return 0;elsereturnbranchcout(T->rchild)+branchcout(T->lchild)+1;}statussinglebranchcout(bitree T){if(T==NULL||(T->rchild==NULL&&T->lchild==NULL))return 0;if(T->rchild!=NULL&&T->lchild!=NULL)returnsinglebranchcout(T->rchild)+singlebranchcout(T->lchild);returnsinglebranchcout(T->rchild)+singlebranchcout(T->lchild)+1;}statusdoublebranchcout(bitree T){if(T==NULL||(T->rchild==NULL&&T->lchild==NULL))return 0;if(T->rchild!=NULL&&T->lchild!=NULL)returndoublebranchcout(T->rchild)+doublebranchcout(T->lchild)+1;elsereturndoublebranchcout(T->rchild)+doublebranchcout(T->lchild);}statusnodecout(bitree T){if(T==NULL)return 0;if(T->rchild==NULL&&T->lchild==NULL)return 1;elsereturnnodecout(T->lchild)+nodecout(T->rchild)+1;}statustreedepth(bitree T){if(T==NULL)return 0;if(T->rchild==NULL&&T->lchild==NULL)return 1;elsereturn(treedepth(T->lchild)>treedepth(T->rchild)?treedepth(T->lchild):treedepth(T->rchild))+1; }void main(){bitree T;creatbitree(T);printf("先序遍历为:");pretraverse(T);printf("\n");printf("\n\n中序遍历为:");centertraverse(T);printf("\n");printf("\n\n后序序遍历为:");nexttraverse(T);printf("\n");printf("\n\n非递归中序遍历为:\n\t");centerstrack(T);printf("\n");printf("\n\n按层次遍历为:\n\t");layertraverse(T);printf("\n");printf("\n\n该树的结点数目为:%d\n\n",nodecout(T));printf("该树的叶子结点数目为:%d\n\n",leafcout(T));printf("该树的分枝数目为:%d\n\n",branchcout(T));printf("该树的单分枝数目为:%d\n\n",singlebranchcout(T));printf("该树的双分枝数目为:%d\n\n",doublebranchcout(T));printf("该树的深度为:%d\n\n",treedepth(T));}。
c语言二叉树代码

c语言二叉树代码对于c语言的二叉树代码,我们可以先了解一下二叉树的性质。
二叉树是一种树形结构,每个节点最多有两个子节点。
根据二叉树的性质,我们可以定义一个结构体来表示二叉树的节点。
struct TreeNode {int val; // 节点的值struct TreeNode *left; // 左子节点struct TreeNode *right; // 右子节点};接下来就是实现二叉树的增删改查:1. 增加节点void addNode(struct TreeNode **node, int val) {if (*node == NULL) {struct TreeNode *newNode = (structTreeNode*)malloc(sizeof(struct TreeNode));newNode->val = val;newNode->left = NULL;newNode->right = NULL;*node = newNode;} else {if (val < (*node)->val) {addNode(&((*node)->left), val);} else {addNode(&((*node)->right), val);}}}2. 删除节点void deleteNode(struct TreeNode **node, int val) {if (*node == NULL) {return;}if ((*node)->val == val) {if ((*node)->left != NULL && (*node)->right != NULL) { struct TreeNode *minNode = (*node)->right;while (minNode->left != NULL) {minNode = minNode->left;}(*node)->val = minNode->val;deleteNode(&((*node)->right), minNode->val);} else {struct TreeNode *temp = *node;if ((*node)->left != NULL) {*node = (*node)->left;} else {*node = (*node)->right;}free(temp);}} else if (val < (*node)->val) {deleteNode(&((*node)->left), val);} else {deleteNode(&((*node)->right), val);}}3. 查找节点struct TreeNode* searchNode(struct TreeNode* node, int val) { if (node == NULL) {return NULL;}if (node->val == val) {return node;} else if (val < node->val) {return searchNode(node->left, val);} else {return searchNode(node->right, val);}}以上就是c语言实现二叉树的基本代码。
数据结构实验六二叉树操作代码实现
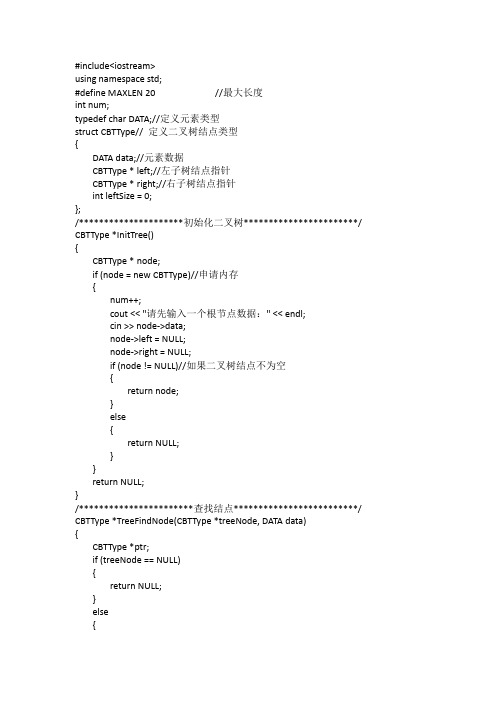
#include<iostream>using namespace std;#define MAXLEN 20 //最大长度int num;typedef char DATA;//定义元素类型struct CBTType// 定义二叉树结点类型{DATA data;//元素数据CBTType * left;//左子树结点指针CBTType * right;//右子树结点指针int leftSize = 0;};/*********************初始化二叉树***********************/ CBTType *InitTree(){CBTType * node;if (node = new CBTType)//申请内存{num++;cout << "请先输入一个根节点数据:" << endl;cin >> node->data;node->left = NULL;node->right = NULL;if (node != NULL)//如果二叉树结点不为空{return node;}else{return NULL;}}return NULL;}/***********************查找结点*************************/ CBTType *TreeFindNode(CBTType *treeNode, DATA data){CBTType *ptr;if (treeNode == NULL){return NULL;}else{if (treeNode->data == data){return treeNode;}else//分别向左右子树查找{if (ptr = TreeFindNode(treeNode->left, data))//左子树递归查找{return ptr;}else if (ptr = TreeFindNode(treeNode->right, data))//右子树递归查找{return ptr;}else{return NULL;}}}}/**********************添加结点*************************/void AddTreeNode(CBTType *treeNode){CBTType *pnode, *parent;DATA data;char menusel;if (pnode = new CBTType) //分配内存{cout << "输入添加的二叉树结点数据:" << endl;cin >> pnode->data;pnode->left = NULL; //设置左子树为空pnode->right = NULL; //设置左子树为空cout << "输入该结点的父结点数据:" << endl;cin >> data;parent = TreeFindNode(treeNode, data); //查找父结点,获得结点指针if (!parent) //没找到{cout << "没有找到父结点!" << endl;delete pnode;return;}cout << "**********************" << endl;cout << "*请输入相应数字:*" << endl;cout << "*1.添加该结点到左子树*" << endl;cout << "*2.添加该结点到右子树*" << endl;cout << "**********************" << endl;do{cin >> menusel;if (menusel == '1' || menusel == '2'){switch (menusel){case '1': //添加结点到左子树if (parent->left) //左子树不为空{cout << "左子树结点不为空" << endl;}else{parent->left = pnode;parent->leftSize++;num++;cout << "数据添加成功!" << endl;}break;case '2': //添加结点到右子树if (parent->right) //右子树不为空{cout << "右子树结点不为空" << endl;}else{parent->right = pnode;num++;cout << "数据添加成功!" << endl;}break;default:cout << "子节点选择错误!" << endl;break;}}} while (menusel != '1'&&menusel != '2');}}/***********************计算二叉树的深度********************************/int TreeDepth(CBTType *treeNode){int depleft, depright;if (treeNode == NULL){return 0; //结点为空的时候,深度为0}else{depleft = TreeDepth(treeNode->left); //左子树深度(递归调用)depright = TreeDepth(treeNode->right); //右子树深度(递归调用)if (depleft){return ++depleft;}else{return ++depright;}}}/*************************显示结点数据*********************************/void ShowNodeData(CBTType *treeNode){cout << treeNode->data << " ";}/***********************清空二叉树************************************/void ClearTree(CBTType *treeNode){if (treeNode)//判断当前树不为空{ClearTree(treeNode->left); //清空左子树ClearTree(treeNode->right); //清空右子树delete treeNode; //释放当前结点所占用的内存}}/**************************按层遍历算法*********************************/void LevelTree(CBTType *treeNode){CBTType *p;CBTType *q[MAXLEN]; //定义一个队列int head = 0, tail = 0;if (treeNode) //如果队首指针不为空{tail = (tail + 1) % MAXLEN; //计算循环队列队尾序号q[tail] = treeNode; //二叉树根指针进入队列while (head != tail){head = (head + 1) % MAXLEN; //计算循环队列的队首序号p = q[head]; //获取队首元素ShowNodeData(p); //输出队首元素if (p->left != NULL) //如果存在左子树{tail = (tail + 1) % MAXLEN; //计算队列的队尾序号q[tail] = p->left; //左子树入队}if (p->right != NULL) //如果存在右子树{tail = (tail + 1) % MAXLEN; //计算队列的队尾序号q[tail] = p->right; //右子树入队}}}}/*************************先序遍历算法**********************************/void DLRTree(CBTType *treeNode){if (treeNode){ShowNodeData(treeNode); //显示结点内容DLRTree(treeNode->left); //显示左子树内容DLRTree(treeNode->right); //显示右子树内容}}/***********************中序遍历算法************************************/void LDRTree(CBTType *treeNode){if (treeNode){LDRTree(treeNode->left); //显示左子树内容ShowNodeData(treeNode); //显示结点内容DLRTree(treeNode->right); //显示右子树内容}}/***********************后序遍历算法************************************/void LRDTree(CBTType *treeNode){if (treeNode){LRDTree(treeNode->left); //显示左子树内容DLRTree(treeNode->right); //显示右子树内容ShowNodeData(treeNode); //显示结点内容}}char find(int theKey,CBTType* root){CBTType *p = root;while (p != NULL)if (theKey < p->leftSize)p = p->left;elseif (theKey > p->leftSize){theKey -= (p->leftSize+1);p = p->right;}elsereturn p->data;//return NULL;}struct TreeNode{struct TreeNode* left;struct TreeNode* right;char elem;};void BinaryTreeFromOrderings(char* inorder, char* preorder, int length){if (length == 0){//cout<<"invalid length";return;}TreeNode* node = new TreeNode;//Noice that [new] should be written out.node->elem = *preorder;int rootIndex = 0;for (; rootIndex < length; rootIndex++){if (inorder[rootIndex] == *preorder)break;}//LeftBinaryTreeFromOrderings(inorder, preorder + 1, rootIndex);//RightBinaryTreeFromOrderings(inorder + rootIndex + 1, preorder + rootIndex + 1, length - (rootIndex + 1));cout << node->elem << ' ';return;}/*************************主函数部分************************************/int main(){int cycle = 1;while (cycle == 1)//主循环{int x;cout << "----------------------------------" << endl;cout << " 主菜单" << endl;cout << "**********************************" << endl;cout << "*0.退出*" << endl;cout << "*1.对二叉树进行操作*" << endl;cout << "*2.输入前序序列和中序序列转为后序*" << endl;cout << "**********************************" << endl;cin >> x;CBTType *root = NULL;switch (x){//二叉树操作case 1:{char menusel;//设置根结点root = InitTree();//添加结点do{cout << "你想为二叉树添加结点吗?(y/n)" << endl;cin >> menusel;switch (menusel){case 'y':AddTreeNode(root);break;case 'n':break;default:cout << "添加结点错误!" << endl;break;}} while (menusel != 'n');//输出树的深度cout << "--------------------" << endl;cout << "二叉树建立完毕!" << endl;cout << "二叉树树高为:" << TreeDepth(root) << endl;//输出结点内容cout << "二叉树节点个数:" << num << endl;cout << "--------------------" << endl;do{cout << "请选择菜单遍历二叉树,输入0表示退出:" << endl;cout << "1.层次遍历" << endl;cout << "2.先序遍历" << endl;cout << "3.中序遍历" << endl;cout << "4.后序遍历" << endl;cout << "-------------------------------------" << endl;cin >> menusel;switch (menusel){case '0':break;case '1':cout << "按层遍历的结果:" << endl;LevelTree(root);cout << endl;break;case '2':cout << "先序遍历的结果:" << endl;DLRTree(root);cout << endl;break;case '3':cout << "中序遍历的结果:" << endl;LDRTree(root);cout << endl;break;case '4':cout << "后序遍历的结果:" << endl;LRDTree(root);cout << endl;break;default:cout << "选择出错!" << endl;break;}} while (menusel != '0');//清空二叉树ClearTree(root);break;}case 2:{ int n;printf("请输入元素个数:\n");cin >> n;char *pr = new char[n];printf("请输入前序序列:\n");for (int i = 0; i < n; i++){cin >> pr[i];}char *in = new char[n];printf("请输入中序序列:\n");for (int i = 0; i < n; i++){cin >> in[i];}printf("转换成后序序列为:\n"); BinaryTreeFromOrderings(in, pr, n);cout << endl;break;}case 0:cycle = 0;cout << endl;cout << "**************已退出**************" << endl;break;}}return 0;}。
二叉树实验代码
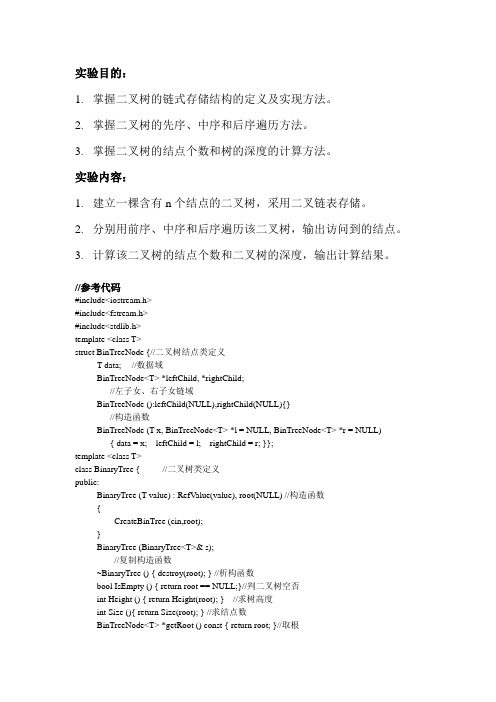
实验目的:1.掌握二叉树的链式存储结构的定义及实现方法。
2.掌握二叉树的先序、中序和后序遍历方法。
3.掌握二叉树的结点个数和树的深度的计算方法。
实验内容:1.建立一棵含有n个结点的二叉树,采用二叉链表存储。
2.分别用前序、中序和后序遍历该二叉树,输出访问到的结点。
3.计算该二叉树的结点个数和二叉树的深度,输出计算结果。
//参考代码#include<iostream.h>#include<fstream.h>#include<stdlib.h>template <class T>struct BinTreeNode {//二叉树结点类定义T data; //数据域BinTreeNode<T> *leftChild, *rightChild;//左子女、右子女链域BinTreeNode ():leftChild(NULL),rightChild(NULL){}//构造函数BinTreeNode (T x, BinTreeNode<T> *l = NULL, BinTreeNode<T> *r = NULL) { data = x; leftChild = l; rightChild = r; }};template <class T>class BinaryTree { //二叉树类定义public:BinaryTree (T value) : RefValue(value), root(NULL) //构造函数{CreateBinTree (cin,root);}BinaryTree (BinaryTree<T>& s);//复制构造函数~BinaryTree () { destroy(root); } //析构函数bool IsEmpty () { return root == NULL;}//判二叉树空否int Height () { return Height(root); } //求树高度int Size (){ return Size(root); } //求结点数BinTreeNode<T> *getRoot () const { return root; }//取根void preOrder (void (*visit) (BinTreeNode<T> *p)) //前序遍历{ preOrder (root, visit); }void inOrder (void (*visit) (BinTreeNode<T> *p)) //中序遍历{ inOrder (root, visit); }void postOrder (void (*visit) (BinTreeNode<T> *p)) //后序遍历{ postOrder (root, visit); }protected:BinTreeNode<T> *root; //二叉树的根指针T RefValue; //数据输入停止标志void CreateBinTree (istream& in,BinTreeNode<T> *& subTree); //从文件读入建树void destroy (BinTreeNode<T> * subTree);void preOrder (BinTreeNode<T>* subTree, void (*visit) (BinTreeNode<T> *p)); //前序遍历void inOrder (BinTreeNode<T>* subTree, void (*visit) (BinTreeNode<T> *p)); //中序遍历void postOrder (BinTreeNode<T>* subTree, void (*visit) (BinTreeNode<T> *p)); //后序遍历int Size (BinTreeNode<T> *subTree) const; //返回结点数int Height ( BinTreeNode<T> * subTree);//返回树高度//其他函数略};template<class T>void BinaryTree<T>::destroy (BinTreeNode<T> * subTree) {//删除根为subTree的子树if (subTree != NULL) {destroy (subTree->leftChild); //删除左子树destroy (subTree->rightChild); //删除右子树delete subTree; //删除根结点}}template<class T>void BinaryTree<T>::CreateBinTree (istream& in, BinTreeNode<T> *& subTree) { T item;if ( !in.eof () ){ //未读完, 读入并建树in >> item; //读入根结点的值if (item != RefValue){subTree = new BinTreeNode<T>(item);//建立根结点if (subTree == NULL){cerr << "存储分配错!"<< endl; exit (1);}CreateBinTree (in, subTree->leftChild);//递归建立左子树CreateBinTree (in, subTree->rightChild);//递归建立右子树}else subTree = NULL; //封闭指向空子树的指针}}template <class T>//前序void BinaryTree<T>::preOrder (BinTreeNode<T> * subTree, void (*visit) (BinTreeNode<T> *p)) {if (subTree!= NULL) {visit (subTree); //访问根结点preOrder (subTree->leftChild, visit); //遍历左子树preOrder (subTree->rightChild, visit); //遍历右子树}}template <class T>//中序void BinaryTree<T>::inOrder (BinTreeNode<T> * subTree, void (*visit) (BinTreeNode<T> *p)) {if (subTree != NULL) {inOrder (subTree->leftChild, visit); //遍历左子树visit (subTree); //访问根结点inOrder (subTree->rightChild, visit); //遍历右子树}}template <class T>//后序void BinaryTree<T>::postOrder (BinTreeNode<T> * subTree, void (*visit) (BinTreeNode<T> *p)) {if (subTree != NULL ) {postOrder (subTree->leftChild, visit);//遍历左子树postOrder (subTree->rightChild, visit); //遍历右子树visit (subTree); //访问根结点}}template <class T>int BinaryTree<T>::Size (BinTreeNode<T> * subTree) const {if (subTree == NULL) return 0; //空树else return 1+Size (subTree->leftChild)+ Size (subTree->rightChild);}template <class T>int BinaryTree<T>::Height ( BinTreeNode<T> * subTree){ if (subTree == NULL) return 0; //空树高度为0else {int i = Height (subTree->leftChild);int j = Height (subTree->rightChild);return (i < j) ? j+1 : i+1; }}void print(BinTreeNode<char> *p){cout<<p->data;}void main(){BinaryTree<char> T('#');cout<<"前序遍历结果为:";T.preOrder(print);cout<<endl<<"中序遍历结果为:";T.inOrder(print);cout<<endl<<"后序遍历结果为:";T.postOrder(print);cout<<endl;int i=T.Height();cout<<endl<<"树的高度是:"<<i;i=T.Size();cout<<endl<<"树的结点个数是:"<<i<<endl;}测试数据:输入:ab##cd##e##输出:说明:输入中的#号是分支结束的标志。
关于实现二叉树,平衡树的代码及每一行代码的解释

关于实现二叉树,平衡树的代码及每一行代码的解释以下为python实现二叉树的代码,每一行代码的解释已在注释中说明:# 定义二叉树的节点class BinaryTree:def __init__(self, value):# 节点的值self.value = value# 左子树self.leftchild = None# 右子树self.rightchild = None# 添加左子树节点def add_leftchild(self, node):self.leftchild = node# 添加右子树节点def add_rightchild(self, node):self.rightchild = node# 创建二叉树root = BinaryTree(1)node2 = BinaryTree(2)node3 = BinaryTree(3)node4 = BinaryTree(4)node5 = BinaryTree(5)root.add_leftchild(node2)root.add_rightchild(node3)node2.add_leftchild(node4)node2.add_rightchild(node5)以下为python实现平衡树的代码(基于AVL树),每一行代码的解释已在注释中说明:# 定义AVL树节点class AVLNode:def __init__(self, value):# 节点值self.value = value# 左子树self.leftchild = None# 右子树self.rightchild = None# 高度self.height = 1# 定义AVL树class AVLTree:def __init__(self):# 根节点self.root = None# 获取树高def get_height(self, root):if root is None:return 0else:return root.height# 获取平衡因子def get_balance_factor(self, root): if root is None:return 0else:return self.get_height(root.leftchild) -self.get_height(root.rightchild)# 右旋转def right_rotate(self, root):lefttree = root.leftchildrighttree = lefttree.rightchild# 右旋转lefttree.rightchild = rootroot.leftchild = righttree# 更新节点高度root.height = 1 + max(self.get_height(root.leftchild), self.get_height(root.rightchild))lefttree.height = 1 + max(self.get_height(lefttree.leftchild), self.get_height(lefttree.rightchild))# 返回新的根节点return lefttree# 左旋转def left_rotate(self, root):righttree = root.rightchildlefttree = righttree.leftchild# 左旋转righttree.leftchild = rootroot.rightchild = lefttree# 更新节点高度root.height = 1 + max(self.get_height(root.leftchild),self.get_height(root.rightchild))righttree.height = 1 + max(self.get_height(righttree.leftchild), self.get_height(righttree.rightchild))# 返回新的根节点return righttree# 插入节点def insert_node(self, root, value):# 如果树为空,则插入新节点if root is None:return AVLNode(value)# 如果插入的值小于根节点,则插入到左子树中if value < root.value:root.leftchild = self.insert_node(root.leftchild, value) # 如果插入的值大于根节点,则插入到右子树中else:root.rightchild = self.insert_node(root.rightchild, value)# 更新节点高度root.height = 1 + max(self.get_height(root.leftchild), self.get_height(root.rightchild))# 获取平衡因子balance_factor = self.get_balance_factor(root)# 平衡树# 左左情况if balance_factor > 1 and value < root.leftchild.value:return self.right_rotate(root)# 左右情况if balance_factor > 1 and value > root.leftchild.value:root.leftchild = self.left_rotate(root.leftchild)return self.right_rotate(root)# 右右情况if balance_factor < -1 and value > root.rightchild.value: return self.left_rotate(root)# 右左情况if balance_factor < -1 and value < root.rightchild.value: root.rightchild = self.right_rotate(root.rightchild)return self.left_rotate(root)return root# 中序遍历def in_order(self, root):res = []if root is not None:res = self.in_order(root.leftchild)res.append(root.value)res = res + self.in_order(root.rightchild)return res# 创建AVL树avl_tree = AVLTree()# 插入节点root = Noneroot = avl_tree.insert_node(root, 10) root = avl_tree.insert_node(root, 20) root = avl_tree.insert_node(root, 30) root = avl_tree.insert_node(root, 40) root = avl_tree.insert_node(root, 50) root = avl_tree.insert_node(root, 25)# 中序遍历print(avl_tree.in_order(root))。
二叉树的基本操作实验代码

二叉树的基本操作实验代码二叉树的基本操作实验代码介绍二叉树是一种非常常见的数据结构,它具有以下特点:1. 每个节点最多有两个子节点;2. 左子节点比父节点小,右子节点比父节点大;3. 左右子树也是二叉树。
在本篇文章中,我们将介绍二叉树的基本操作,并提供相应的实验代码。
创建二叉树创建一个二叉树需要两个步骤:首先创建一个空的根节点,然后逐个添加子节点。
下面是创建一个简单的二叉树的示例代码:```c++#include <iostream>using namespace std;struct TreeNode {int val;TreeNode *left;TreeNode *right;TreeNode(int x) : val(x), left(NULL), right(NULL) {} };TreeNode* createTree() {TreeNode* root = new TreeNode(1);root->left = new TreeNode(2);root->right = new TreeNode(3);root->left->left = new TreeNode(4);root->left->right = new TreeNode(5);return root;}int main() {TreeNode* root = createTree();}```遍历二叉树遍历二叉树有三种方式:前序遍历、中序遍历和后序遍历。
下面是三种遍历方式的示例代码:前序遍历:```c++void preorderTraversal(TreeNode* root) {if (!root) return;cout << root->val << " ";preorderTraversal(root->left);preorderTraversal(root->right);}```中序遍历:```c++void inorderTraversal(TreeNode* root) {if (!root) return;inorderTraversal(root->left);cout << root->val << " ";inorderTraversal(root->right);}```后序遍历:```c++void postorderTraversal(TreeNode* root) {if (!root) return;postorderTraversal(root->left);postorderTraversal(root->right);cout << root->val << " ";}```查找二叉树查找二叉树需要递归地遍历每个节点,直到找到目标节点。
二叉树的完整代码实现
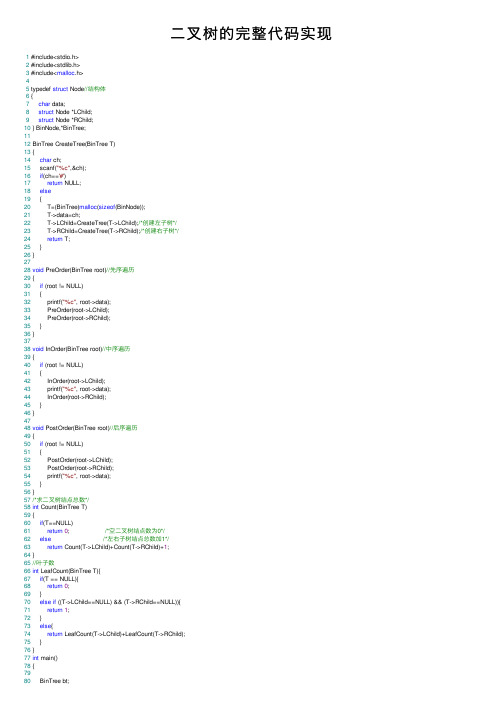
⼆叉树的完整代码实现1 #include<stdio.h>2 #include<stdlib.h>3 #include<malloc.h>45 typedef struct Node//结构体6 {7char data;8struct Node *LChild;9struct Node *RChild;10 } BinNode,*BinTree;1112 BinTree CreateTree(BinTree T)13 {14char ch;15 scanf("%c",&ch);16if(ch=='#')17return NULL;18else19 {20 T=(BinTree)malloc(sizeof(BinNode));21 T->data=ch;22 T->LChild=CreateTree(T->LChild);/*创建左⼦树*/23 T->RChild=CreateTree(T->RChild);/*创建右⼦树*/24return T;25 }26 }2728void PreOrder(BinTree root)//先序遍历29 {30if (root != NULL)31 {32 printf("%c", root->data);33 PreOrder(root->LChild);34 PreOrder(root->RChild);35 }36 }3738void InOrder(BinTree root)//中序遍历39 {40if (root != NULL)41 {42 InOrder(root->LChild);43 printf("%c", root->data);44 InOrder(root->RChild);45 }46 }4748void PostOrder(BinTree root)//后序遍历49 {50if (root != NULL)51 {52 PostOrder(root->LChild);53 PostOrder(root->RChild);54 printf("%c", root->data);55 }56 }57/*求⼆叉树结点总数*/58int Count(BinTree T)59 {60if(T==NULL)61return0; /*空⼆叉树结点数为0*/62else/*左右⼦树结点总数加1*/63return Count(T->LChild)+Count(T->RChild)+1;64 }65//叶⼦数66int LeafCount(BinTree T){67if(T == NULL){68return0;69 }70else if ((T->LChild==NULL) && (T->RChild==NULL)){71return1;72 }73else{74return LeafCount(T->LChild)+LeafCount(T->RChild);75 }76 }77int main()78 {7980 BinTree bt;81 printf("⼀、请按先序的⽅式输⼊⼆叉树的结点元素(注:输⼊#表⽰节点为空)如:ABC##DE#G##F###\n");82 bt=CreateTree(bt);83 printf("⼆、前序遍历⼆叉树:\n");84 PreOrder(bt);85 printf("\n");86 printf("三、中序遍历⼆叉树:\n");87 InOrder(bt);88 printf("\n");89 printf("四、后序遍历⼆叉树:\n");90 PostOrder(bt);91 printf("\n");92 printf("五、⼆叉树结点数: %d\n",Count(bt));93 printf("六、叶⼦节点的个数:%d \n",LeafCount(bt));94 system("pause");95 }。
数据结构二叉树实验报告(附代码)
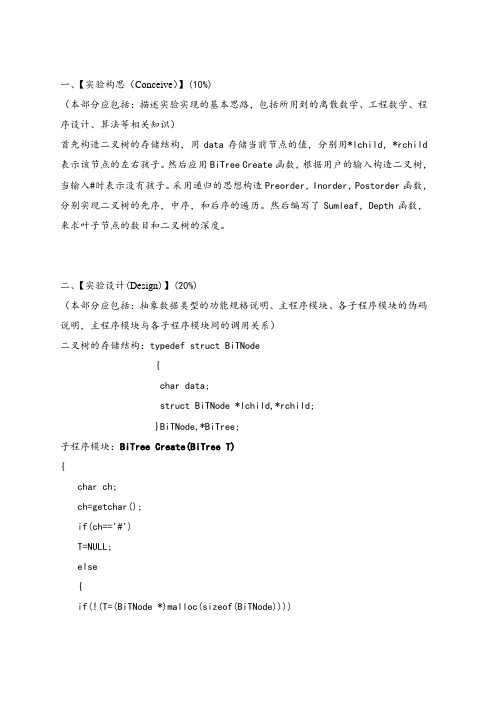
一、【实验构思(Conceive)】(10%)(本部分应包括:描述实验实现的基本思路,包括所用到的离散数学、工程数学、程序设计、算法等相关知识)首先构造二叉树的存储结构,用data存储当前节点的值,分别用*lchild,*rchild 表示该节点的左右孩子。
然后应用BiTree Create函数,根据用户的输入构造二叉树,当输入#时表示没有孩子。
采用递归的思想构造Preorder,Inorder,Postorder函数,分别实现二叉树的先序,中序,和后序的遍历。
然后编写了Sumleaf,Depth函数,来求叶子节点的数目和二叉树的深度。
二、【实验设计(Design)】(20%)(本部分应包括:抽象数据类型的功能规格说明、主程序模块、各子程序模块的伪码说明,主程序模块与各子程序模块间的调用关系)二叉树的存储结构:typedef struct BiTNode{char data;struct BiTNode *lchild,*rchild;}BiTNode,*BiTree;子程序模块:BiTree Create(BiTree T){char ch;ch=getchar();if(ch=='#')T=NULL;else{if(!(T=(BiTNode *)malloc(sizeof(BiTNode))))printf("Error!");T->data=ch;T->lchild=Create(T->lchild);T->rchild=Create(T->rchild);}return T;}void Preorder(BiTree T){if(T){printf("%c",T->data);Preorder(T->lchild);Preorder(T->rchild);}}int Sumleaf(BiTree T){int sum=0,m,n;if(T){if((!T->lchild)&&(!T->rchild)) sum++;m=Sumleaf(T->lchild);sum+=m;n=Sumleaf(T->rchild);sum+=n;}return sum;}void Inorder(BiTree T) {if(T){Inorder(T->lchild); printf("%c",T->data); Inorder(T->rchild); }}void Postorder(BiTree T) {if(T){Postorder(T->lchild); Postorder(T->rchild); printf("%c",T->data); }}int Depth(BiTree T){int dep=0,depl,depr;if(!T)dep=0;else{depl=Depth(T->lchild);depr=Depth(T->rchild);dep=1+(depl>depr?depl:depr);}return dep;}主程序模块:int main(){BiTree T = 0;int sum,dep;printf("请输入你需要建立的二叉树\n");printf("例如输入序列ABC##DE#G##F###(其中的#表示空)\n并且输入过程中不要加回车\n输入完之后可以按回车退出\n");T=Create(T);printf("先序遍历的结果是:\n");Preorder(T);printf("\n");printf("中序遍历的结果是:\n");Inorder(T);printf("\n");printf("后序遍历的结果是:\n");Postorder(T);printf("\n");printf("统计的叶子数:\n");sum=Sumleaf(T);printf("%d",sum);printf("\n统计树的深度:\n");dep=Depth(T);printf("\n%d\n",dep);}三、【实现描述(Implement)】(30%)(本部分应包括:抽象数据类型具体实现的函数原型说明、关键操作实现的伪码算法、函数设计、函数间的调用关系,关键的程序流程图等,给出关键算法的时间复杂度分析。
表达式二叉树表达式二叉树T第一操...
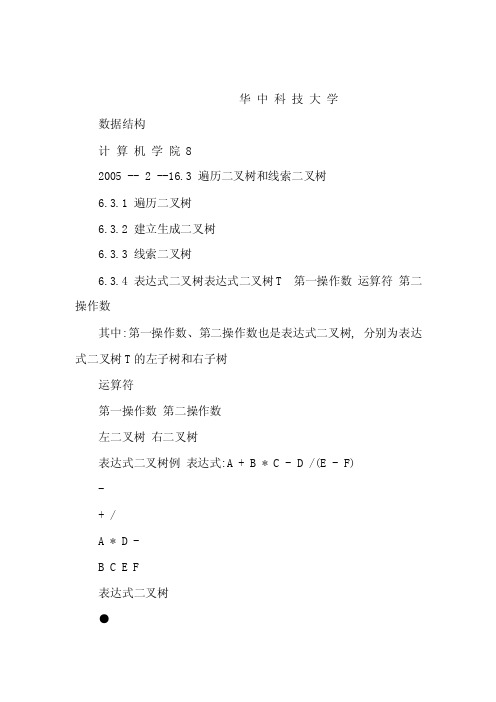
华中科技大学数据结构计算机学院 82005 -- 2 --16.3 遍历二叉树和线索二叉树6.3.1 遍历二叉树6.3.2 建立生成二叉树6.3.3 线索二叉树6.3.4 表达式二叉树表达式二叉树T 第一操作数运算符第二操作数其中:第一操作数、第二操作数也是表达式二叉树, 分别为表达式二叉树T的左子树和右子树运算符第一操作数第二操作数左二叉树右二叉树表达式二叉树例表达式:A + B * C - D /(E - F)-+ /A * D -B C E F表达式二叉树●前序遍历:- + A * B C / D - E F 前缀表示,前缀表达式,波兰式●中序遍历: A + B * C - D / E - F 中缀表示,中缀表达式●后序遍历: A B C * + D E F - / - 后缀表示,后缀表达式,逆波兰式6.3.5 中序遍历序列和前或后序序列确定唯一棵二叉树由前序序列:A D E B CA A和或后序序列:E D C B AD B D B不能确定唯一棵二叉树(如图T1,T2)E C E CT1 T2例1. 给定二叉树T的中序序列:B A C D E G FE 前序序列:E A B C DF GA F如何求二叉树T?EB C GB,A,C,D G,FD6.3.5 中序遍历序列和前或后序序列确定唯一棵二叉树例2. 给定二叉树T的后序序列:B G H E C A J I F D 中序序列:B A C G E H D I J F 如何求二叉树T?(根结点,左右子树结点)DB,A,CI,J,FG,E,H6.3.5 中序遍历序列和前或后序序列确定唯一棵二叉树例2. 给定二叉树T的后序序列:B G H E C A J I F D 中序序列:B A C G E H D I J F如何求二叉树T?DA FDB C IB,A,CI,J,FG,E,HE JG H二叉树Troot6.3.6 遍历二叉树算法AAB C∧∧∧B CDDE F∧∧∧∧EF二叉树二叉链表递归算法1.前序遍历以root为根指针的二叉树preorderstruct nodeb *rootif root //为非空二叉树 printf"%c",root-data; //访问根结点preorderroot-lchild;//递归遍历左子树preorderroot-rchild;//递归遍历右子树地址栈根指针栈执行preorderstruct nodeb *t;tAL1 tt1∧∧∧B C1.输出A;L1,t进栈t2DL1 t1∧∧ L1 t∧∧EF2.t指向B,记作t1;输出B;preorderstruct nodeb *tL1,t1进栈if t printf"%c",t-data;t1l2 preordert-lchild;L1 ttL1L1: preordert-rchild;3.t指向NULL,记作t2;退栈,L2:返回到L1,恢复t1;L2,t1进栈执行preorderstruct nodeb *t; tAL1 t3L2 t1t1∧∧∧B CL1 tt2t34.t指向D,记作t3;输出DD;L1、t3进栈t4∧∧∧∧EL1 t4preorderstruct nodeb *tL1 t3if tL2 t1 printf"%c",t-L1 tdata;5.t指向E,记作t4; preordert-lchild;输出E;L1,t4进栈L1: preordert-rchild;L2:执行preorderstruct nodeb *t;L2 t4tL1 t3A L1 t3L2 t1L2 t1L1 tt1L1 t∧∧∧6.t指向NULL,记作t5;t3D退栈,返回到L1,恢复t4;t4∧∧∧∧E L2、t4进栈Ft5 t6L1 t3L2t3preorderstruct nodeb *t L2 t1 L2 t1L2t1if tL1 t tt printf"%c",t- L1L17.t指向NULL,记作t6; preordert-lchild;退栈,返回到L2,恢复t4;退栈,返回到L1,恢复t3;L1: preordert-rchild;L2、t3进栈L2:执行preorderstruct nodeb *t;tL1 t7AL2 t3t1 L2 t1∧∧∧B CL1 tt3D8.t指向F,记作t7t7;输出F;L1,t7进栈∧∧∧∧EFt8L2 t7preorderstruct nodeb *tL2 t3L2 t3if tL2 t1L2 t1 printf"%c",t-L1 tL1 tdata; preordert-lchild;9.t指向NULL,记作t8;L1: preordert-rchild;退栈,返回到L1,恢复t7;L2,t7进栈L2:执行preorderstruct nodeb *t;tAL2 t3L2 t1 L2 t1t1∧∧∧B CL1 t L1 t10.t指向NULL,记作t9;t3Dt7退栈,返回到L2,恢复t7;∧∧∧∧退栈,返回到L2,恢复EFt3;t9preorderstruct nodeb *t if tL2 printf"%c",t- L1 t t data; preordert-lchild; 退栈,返回到L2,恢复t1;L1: preordert-rchild;退栈,返回到L1,恢复t;L2:L2、t进栈执行preorderstruct nodeb *t; tAt10L1t10∧∧∧B CL2tt1111.t指向C,记作t10;D 输出C;L1,t10进栈∧∧∧∧EFpreorderstruct nodeb *tif tt10L2 printf"%c",t-tL2 t L2data; preordert-lchild;12.t指向NULL,记作t11; 退栈,返回到L1,恢复L1: preordert-rchild;t10;L2: L2、t10进栈执行preorderstruct nodeb *t;tAt10∧∧∧B CL2tt1213.t指向NUII,记作t12;D 退栈,返回到L2,恢复t10∧∧∧∧EFpreorderstruct nodeb *tif t printf"%c",t-data; preordert-lchild;退栈,返回到L2,恢复t;L1: preordert-rchild;preorderstruct nodeb *tL2: 执行结束。
二叉树 C++ 实验代码

#include<iostream>#include<string>using namespace std;void visit (char c){cout<<"["<<c<<"]";}struct BiTNode{int data;BiTNode *lchild,*rchild;BiTNode(char ch,BiTNode *left,BiTNode *right) {data=ch;lchild=left;rchild=right;}BiTNode(){}};struct BiTree{BiTNode *root;BiTree(){root=NULL;}void PreOrderTraverse();void InOrderTraverse();void PostOrderTraverse();void InOrderTraverse2();BiTNode* SearchBST1(BiTNode *T, int key);void InsertBST1(BiTNode* &T, int e);private:void PreOrder(BiTNode *p);void InOrder(BiTNode *p);void PostOrder(BiTNode *p);void InOrder2(BiTNode *p);};BiTNode* BiTree::SearchBST1(BiTNode *T, int key){if (T == NULL) return T; // 查找不成功else if (key < T->data) return SearchBST1(T->lchild, key);else if (key > T->data) return SearchBST1(T->rchild, key);else return T; // 查找成功key==T->data}void BiTree:: InsertBST1(BiTNode* &T, int e){if (T==NULL) T=new BiTNode(e, NULL, NULL);else if (e < T->data) InsertBST1(T->lchild, e);else if (e > T->data) InsertBST1(T->rchild, e);}int cntLeaf(BiTNode *p){if(p==NULL) return 0;int cnt=0;if(p->lchild==NULL&&p->rchild==NULL){return 1;}else{return cntLeaf(p->lchild)+cntLeaf(p->rchild);}}void BiTree::PreOrderTraverse(){PreOrder(root);}void BiTree::InOrderTraverse(){InOrder(root);}void BiTree::InOrderTraverse2(){InOrder2(root);}void BiTree::PostOrderTraverse(){PostOrder(root);}void BiTree::PreOrder(BiTNode *p){if(p==NULL) return;cout<<p->data;PreOrder(p->lchild);PreOrder(p->rchild);}void BiTree::InOrder(BiTNode *p){if(p==NULL) return;PreOrder(p->lchild);cout<<p->data;PreOrder(p->rchild);}void BiTree::InOrder2(BiTNode *p){if(p==NULL) return;PreOrder(p->rchild);cout<<p->data;PreOrder(p->lchild);}void BiTree::PostOrder(BiTNode *p){if(p==NULL) return;PostOrder(p->lchild);PostOrder(p->rchild);cout<<p->data;}BiTNode *CopyTree(BiTNode *T){if (T==NULL ) return NULL;BiTNode*l= CopyTree(T->lchild);//复制左子树BiTNode*r = CopyTree(T->rchild);//复制右子树BiTNode *newT=new BiTNode(T->data,l,r);return newT;}int main(){BiTree T;T.InsertBST1(T.root,10);T.InsertBST1(T.root,5);//T.PreOrderTraverse();//cout<<endl;T.InOrderTraverse();cout<<endl;//T.PostOrderTraverse();//cout<<endl;T.InOrderTraverse2();cout<<endl;//cout<<cntLeaf(T.root)<<endl;return 0;}。
表达式转化成二叉树代码
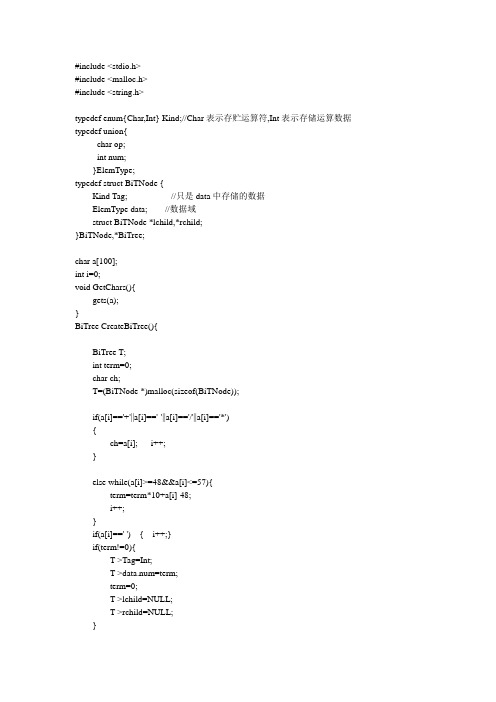
#include <stdio.h>#include <malloc.h>#include <string.h>typedef enum{Char,Int} Kind;//Char表示存贮运算符,Int表示存储运算数据typedef union{char op;int num;}ElemType;typedef struct BiTNode {Kind Tag; //只是data中存储的数据ElemType data; //数据域struct BiTNode *lchild,*rchild;}BiTNode,*BiTree;char a[100];int i=0;void GetChars(){gets(a);}BiTree CreateBiTree(){BiTree T;int term=0;char ch;T=(BiTNode *)malloc(sizeof(BiTNode));if(a[i]=='+'||a[i]=='-'||a[i]=='/'||a[i]=='*'){ch=a[i]; i++;}else while(a[i]>=48&&a[i]<=57){term=term*10+a[i]-48;i++;}if(a[i]==' ') { i++;}if(term!=0){T->Tag=Int;T->data.num=term;term=0;T->lchild=NULL;T->rchild=NULL;}else{T->Tag=Char;T->data.op=ch;T->lchild=CreateBiTree();T->rchild=CreateBiTree();}return T;}void InOrder(BiTree T){if(T){InOrder(T->lchild); //遍历左子树if(T->Tag==Int) printf("%d",T->data.num);else printf("%c",T->data.op); //访问结点InOrder(T->rchild); //遍历右子树}}void PostOrder(BiTree T){if(T){PostOrder(T->lchild); //遍历左子树PostOrder(T->rchild); //遍历右子树if(T->Tag==Int) printf("%d ",T->data.num);else printf("%c",T->data.op); //访问结点}}main(){BiTree B;GetChars();B=CreateBiTree();InOrder(B); printf("\n");PostOrder(B);}。
实验五 二叉树基本操作的编程实现

实验五二叉树基本操作的编程实现【实验目的】内容:二叉树基本操作的编程实现要求:二叉树基本操作的编程实现(2学时,验证型),掌握二叉树的建立、遍历、插入、删除等基本操作的编程实现,也可以进一步编程实现查找等操作,存储结构主要采用顺序或链接结构。
也鼓励学生利用基本操作进行一些应用的程序设计。
【实验性质】验证性实验(学时数:2H)【实验内容】以下的选题都可以作为本次实验的推荐题目1.建立二叉树,并以前序遍历的方式将结点内容输出。
2.将一个表示二叉树的数组结构转换成链表结构。
3.将表达式二叉树方式存入数组,以递归方式建立表达式之二叉树状结构,再分别输出前序、中序及后序遍历结果,并计算出表达式之结果。
【思考问题】1.二叉树是树吗?它的定义为什么是递归的?2.三种根序遍历主要思路是什么?3.如果不用遍历算法一般启用什么数据结构实现后序遍历?4.举出二叉树的应用范例?【参考代码】(一)建立二叉树,并以前序遍历的方式将结点内容输出*//*===============================================*//*程序构思:*//*输入元素值后建立二叉树,以递归的方式做前序遍历,*//*其顺序为:结点-左子-右子树,并将二叉树结点内容输出。
*/#include<stdlib.h>#include<stdio.h>struct tree /*声明树的结构*/{struct tree *left; /*存放左子树的指针*/int data; /*存放结点数据内容*/struct tree *right; /*存放右子树的指针*/};typedef struct tree treenode; /*声明新类型树结构*/typedef treenode *b_tree; /*声明二叉树的链表*//*===============================================*//*插入二叉树结点*//*===============================================*/b_tree insert_node (b_tree root, int node){b_tree newnode; /*声明树根指针*/b_tree currentnode; /*声明目前结点指针*/b_tree parentnode; /*声明父结点指针*//*建立新结点的内存空间*/newnode=(b_tree )malloc (sizeof(treenode));newnode->data=node; /*存入结点内容*/ newnode->right=NULL; /*设置右指针初值*/ newnode->left=NULL; /*设置左指针初值*/if (root==NULL)return newnode; /*返回新结点的位置*/ else{currentnode=root; /*存储目前结点指针*/while (currentnode!=NULL){parentnode=currentnode; /*存储父结点指针*/if (currentnode->data>node) /*比较结点的数值大小*/currentnode=currentnode->left; /*左子树*/elsecurrentnode=currentnode->right; /*右子树*/ }if (parentnode->data>node) /*将父结点和子结点做连结*/ parentnode->left=newnode; /*子结点为父结点之左子树*/ elseparentnode->right=newnode; /*子结点为父结点之右子树*/ }return root; /*返回根结点之指针*/ }/*===============================================*//*建立二叉树*//*===============================================*/b_tree create_btree (int *data, int len){b_tree root=NULL; /*根结点指针*/ int i;for (i=0; i<len; i++) /*建立树状结构*/ root=insert_node(root, );return root;}/*===============================================*//*二叉树前序遍历*//*===============================================*/void preorder (b_tree point){if (point!=NULL) /*遍历的终止条件*/ {printf ("%2d", point->data); /*处理打印结点内容*/preorder (point->left); /*处理左子树*//*处理右子树*/}}/*==================================================*//*主程序:建立二叉树,并以前序遍历输出二叉树结点内容*//*==================================================*/void main(){b_tree root=NULL; /*树根指针*/int i, index;int value; /*读入输入值所使用的暂存变量*/ int nodelist[20]; /*声明存储输入数据之数组*/printf ("\n Please input the elements of binary tree(Exit for 0): \n");index=0;/*------------------读取数值存到数组中------------------*/scanf ("%d", &value); /*读取输入值存到变量value*/while (value!=0){nodelist[index]=value;;scanf ("%d", &value);}/*----------------------建立二叉树----------------------*/root=create_btree(nodelist, index);/*--------------------前序遍历二叉树--------------------*/printf ("\n The preorder traversal result is (");preorder( );printf (") \n");}/*希望的结果*/ /*Please input the elements of binary tree(Exit for 0): *//*6 3 1 9 5 7 4 8 0 *//* */ /*The preorder traversal result is (6 3 1 5 4 9 7 8) */(二)将一个表示二叉树的数组结构转换成链表结构/*===============================================*//*程序构思:*//*给定一个二叉树数组结构,使用递归方式建立一棵二叉树,*//*并中序遍历的方式输出二叉树结点内容。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
实验:表达式二叉树实验要求:建立表达式二叉树,并打印(可逆时针旋转90度打印,也可尝试正向打印)以中缀表达式a*b+(c-d)/e-f 为例,建立以"-"号为根的表达式二叉树。
提示:若直接利用中缀表达式建立困难,可先将中缀表达式转化为后缀表达式(可利用栈中的中缀到后缀转化程序实现中缀表达式到后缀表达式的转换,再根据后缀表达式建立表达式二叉树,可参考栈中后缀表达式计算方法)。
实验代码:#include <iostream>#include <stack>#include <string>#include <queue>#include <vector>using namespace std;class tnode;void buildexptree(const string &exp);void printexptree();int judgerank(char ch);bool isoperator(char ch);void test();void setxy(tnode *tn, int y);int X = 0;int Y = 0;int depth = 0;vector<tnode*>vt;class tnode{public:char nodeValue;int x, y;tnode *left;tnode *right;tnode(){x = 0;y = 0;}tnode(char &item, tnode *lptr = NULL, tnode *rptr = NULL):nodeV alue(item), left(lptr), right(rptr){x = 0;y = 0;}};tnode *myNode;bool cmp(tnode *t1, tnode *t2){if(t1->y != t2->y)return (t1->y) > (t2->y);return (t1->x) < (t2->x);}class zpair{ //将operator和rank打包为一体,临时存储在stk2中public:char op;int rank;zpair(){}zpair(char _op, int _rank){op = _op;rank = _rank;}};int judgerank(char ch){if(ch == '(') return -1;if(ch == '+' || ch == '-') return 1;if(ch == '*' || ch == '/') return 2;return 0;}bool isoperator(char ch){if(ch == '(' || ch == ')' || ch == '+' || ch == '-' || ch == '*' || ch == '/') return true;elsereturn false;}void buildexptree(const string &exp){stack<tnode*>stk1;stack<zpair>stk2;int i;int len = exp.length();char ch;zpair tz;zpair ztop;for(i=0; i<len; i++){ch = exp[i];if(!isoperator(ch)){ //exp[i] is an operand, so create a tnode using exp[i] and push to stk1tnode *ptr1 = new tnode(ch);stk1.push(ptr1);}else {tz.op = ch;tz.rank = judgerank(ch);if(stk2.empty())stk2.push(tz);else if(ch == '(')stk2.push(tz);else if(ch == ')'){ztop = stk2.top();while(ztop.op != '('){tnode *ptr2 = stk1.top();stk1.pop();tnode *ptr3 = stk1.top();stk1.pop();tnode *ptr4 = new tnode(ztop.op, ptr3, ptr2);stk1.push(ptr4);stk2.pop();ztop = stk2.top();}stk2.pop();}else{ztop = stk2.top();while(tz.rank <= ztop.rank){tnode *ptr5 = stk1.top();stk1.pop();tnode *ptr6 = stk1.top();stk1.pop();tnode *ptr7 = new tnode(ztop.op, ptr6, ptr5);stk1.push(ptr7);stk2.pop();if(stk2.empty()) break;else ztop = stk2.top();}stk2.push(tz);}}}while(!stk2.empty()){tnode *ptr8 = stk1.top();stk1.pop();tnode *ptr9 = stk1.top();stk1.pop();tnode *ptr10 = new tnode(stk2.top().op, ptr9, ptr8);stk1.push(ptr10);stk2.pop();}myNode = stk1.top();}int main(){freopen("chris.txt","r",stdin);string exp;cout << "Please input an expression:" << endl;cin >> exp;buildexptree(exp);cout << "The expression tree is: " << endl;printexptree();return 0;}void test(){ //测试函数cout << "testing..." << endl;}void printexptree(){setxy(myNode, Y);sort(vt.begin(),vt.end(),cmp);int tmpY = 1;int tmpX = 0;for(int i=0; i<vt.size(); i++){if(vt[i]->y < tmpY){cout << endl;tmpX = 0;tmpY--;}cout << string(vt[i]->x - tmpX - 1 , ' ') << vt[i]->nodeValue;tmpX = vt[i]->x;}cout << endl;}void setxy(tnode *tn, int y){ //设定每一个节点的横坐标if(tn == NULL) return;setxy(tn->left, y-1);tn->x = ++X;tn->y = y;vt.push_back(tn);/* cout << "tn->nodeValue=" << tn->nodeValue << " " << "tn->x=" << tn->x << " " << "tn->y=" << tn->y << endl;*/setxy(tn->right,y-1);depth++;}实验截图:实验分析:通过类tnode构造了一个表达式二叉树,除了节点值nodeValue,左分支left 和右分支right,还引入了x,y作为标定的坐标,打印时使用。
首先完成infix2postfix,这其中使用了stk1,stk2两个栈分别存储子树和zpair量,zpair是将operator与其优先级打包的一个整体,然后通过比较优先级,调整stk1中的元素,构造了后缀表达式。
打印过程中,先调用setxy()方法来设定每个节点的坐标值,使用的是中序遍历,同时声明了存储各个节点的向量vt,将所有节点压入vt后先按y再按x排序,然后按格式输出表达式二叉树。