Java完整代码
计算器Java编程完全代码

计算器Java编程代码1、界面截图1、程序代码import java.awt.*;import java.awt.event.*;import javax.swing.*;public class Counter implements ActionListener {// 改进小数问题private boolean append = false;JButton[] jb = new JButton[20];JTextField jtf = new JTextField(19);String[] st = { "Backs", "CE", "C", "+", " 7 ", " 8 ", " 9", "-"," 4 ", " 5 ", " 6", "*", " 1 ", " 2 ", " 3", "/"," . ", "+/-", " 0", "=", };String num1 = "0";String operator = "+";public Counter() {JFrame jf = new JFrame("计算器");// 界面设置GridLayout gl = new GridLayout(6, 1);jf.setLayout(gl);JPanel jp0 = new JPanel();jp0.add(jtf);jf.add(jp0);JPanel jp1 = new JPanel();for (int i = 0; i < 4; i++) {jb[i] = new JButton(st[i]);jp1.add(jb[i]);}jf.add(jp1);JPanel jp2 = new JPanel();for (int i = 4; i < 8; i++) {jb[i] = new JButton(st[i]);jp2.add(jb[i]);}jf.add(jp2);JPanel jp3 = new JPanel();for (int i = 8; i < 12; i++) {jb[i] = new JButton(st[i]);jp3.add(jb[i]);}jf.add(jp3);JPanel jp4 = new JPanel();for (int i = 12; i < 16; i++) { jb[i] = new JButton(st[i]);jp4.add(jb[i]);}jf.add(jp4);JPanel jp5 = new JPanel();for (int i = 16; i < 20; i++) { jb[i] = new JButton(st[i]);jp5.add(jb[i]);}jf.add(jp5);jtf.setEditable(false);// 文本框不可编辑jf.setResizable(false);// 窗口不可编辑jf.pack();// 自动调整窗口的大小// jf.setSize(240, 220);jf.setLocation(450, 300);jf.setVisible(true);jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);for (int i = 0; i < 20; i++) {// 注册监听jb[i].addActionListener(this);}}public void actionPerformed(ActionEvent ae) {String s = ae.getActionCommand();if (s.trim().matches("^\\d$")) {// 判断是输入的是否是数字if (append) {// 追加数字String ss = jtf.getText().trim();jtf.setText(ss + s.trim());} else {// 替换文本框原来的数字jtf.setText(s.trim());append = true;}} else if ("+-/*".indexOf(s.trim()) != -1) {// 判断按的是否是四则运算符num1 = jtf.getText();// 将第一次输入的数存储起来operator = s.trim();// 将输入的符号存储起来append = false;} else if ("=".equals(s.trim())) {String num2 = jtf.getText();double d1 = Double.parseDouble(num1);double d2 = Double.parseDouble(num2);if ("+".equals(operator)) {// 加法运算d1 = d1 + d2;} else if ("-".equals(operator)) {// 减法运算d1 = d1 - d2;} else if ("*".equals(operator)) {// 乘法运算d1 = d1 * d2;} else if ("/".equals(operator)) {// 除法运算d1 = d1 / d2;}jtf.setText(d1 + "");// 显示结果append = false;} else if (".".equals(s.trim())) {// 判断小数点String temp = jtf.getText();if (temp.indexOf(".") == -1) {jtf.setText(temp + ".");append = true;}} else if ("+/-".equals(s.trim())) {// 判断+/-String temp = jtf.getText();if(temp.startsWith("-")) {// 如果该数是负数则取负号后的数字jtf.setText(temp.substring(1));} else {// 如果是正数则在这个数前加上负号jtf.setText("-" + temp);}} else if ("CE".equals(s.trim()) || "C".equals(s.trim())) {// 判断复位键jtf.setText("0");append = false;} else if ("BackS".equals(s.trim())) {// 判断BackS键(删除)String temp = jtf.getText();if (temp.length() > 0) {jtf.setText(temp.substring(0, temp.length() - 1));}}}public static void main(String[] args) {new Counter();}}。
计算器完整代码(java)

1. Calculator 类import java.applet.*;import java.awt.*;import java.awt.event.*;import ng.*;import java.applet.Applet;import javax.swing.*;import javax.swing.border.*;public class Calculator extends JApplet implements ActionListener {private final String[] KEYS={"7","8","9","/","sqrt", "4","5","6","*","%","1","2","3","-","1/x","0","+/-",".","+","="};private final String[] COMMAND={"Backspace","CE","C"};private final String[] M={" ","MC","MR","MS","M+"};private JButton keys[]=new JButton[KEYS.length];private JButton commands[]=new JButton[COMMAND.length];private JButton m[]=new JButton[M.length];private JTextField display =new JTextField("0");// public JTextField setHorizontalAlignment(int alignment);// private JTextFielddisplay.setHorizontalAlignment(JTextField.RIGHT)=new JTextField("0"); private void setup(){display.setHorizontalAlignment(JTextField.RIGHT);JPanel calckeys=new JPanel();JPanel command=new JPanel();JPanel calms=new JPanel();calckeys.setLayout(new GridLayout(4,5,3,3));command.setLayout(new GridLayout(1,3,3,3));calms.setLayout(new GridLayout(5,1,3,3));for(int i=0;i<KEYS.length;i++){keys[i]=new JButton(KEYS[i]);calckeys.add(keys[i]);keys[i].setForeground(Color.blue);}keys[3].setForeground(Color.red);keys[4].setForeground(Color.red);keys[8].setForeground(Color.red);keys[9].setForeground(Color.red);keys[13].setForeground(Color.red);keys[14].setForeground(Color.red);keys[18].setForeground(Color.red);keys[19].setForeground(Color.red);for(int i=0;i<COMMAND.length;i++){commands[i]=new JButton(COMMAND[i]);command.add(commands[i]);commands[i].setForeground(Color.red);}for(int i=0;i<M.length;i++){m[i]=new JButton(M[i]);calms.add(m[i]);m[i].setForeground(Color.red);}JPanel panel1=new JPanel();panel1.setLayout(new BorderLayout(3,3));panel1.add("North",command);panel1.add("Center",calckeys);JPanel top=new JPanel();top.setLayout(new BorderLayout());display.setBackground(Color.WHITE);top.add("Center",display);getContentPane().setLayout(new BorderLayout(3,5)); getContentPane().add("North",top);getContentPane().add("Center",panel1); getContentPane().add("West",calms);}public void init(){setup();for(int i=0;i<KEYS.length;i++){keys[i].addActionListener(this);}for(int i=0;i<COMMAND.length;i++){commands[i].addActionListener(this);}for(int i=0;i<M.length;i++){m[i].addActionListener(this);}display.setEditable(false);}public void actionPerformed(ActionEvent e){String label=e.getActionCommand();// double zero=e.getActionCommand();if(label=="C")handleC();else if(label=="Backspace")handleBackspace();else if(label=="CE")display.setText("0");else if (label=="1/x")daoShu();else if(label=="sqrt"){display.setText(Math.sqrt(getNumberFromDisplay())+"");}else if("0123456789.".indexOf(label)>=0){handleNumber(label);// handlezero(zero);}elsehandleOperator(label);}private boolean firstDigit=true;private void handleNumber(String key){if(firstDigit){ if(key.equals("0"))display.setText(key);else if(key.equals(".")){display.setText("0.");firstDigit=false;}else if(!key.equals("0")&&!key.equals(".")){display.setText(key);firstDigit=false;}}else if((key.equals("."))&&(display.getText().indexOf(".")<0)) display.setText(display.getText()+".");else if(!key.equals(".")&&display.getText().length()<=32) display.setText(display.getText()+key);}private double number=0;private String operator="=";private void handleOperator(String key){if(operator.equals("/")){if(getNumberFromDisplay()==0){display.setText("除数不能为零");}else{number/=getNumberFromDisplay();}long t1;double t2;t1=(long)number;t2=number-t1;if(t2==0){display.setText(String.valueOf(t1));}else{display.setText(String.valueOf(number));}}else if(operator.equals("+"))number+=getNumberFromDisplay();else if(operator.equals("-"))number-=getNumberFromDisplay();else if(operator.equals("*"))number*=getNumberFromDisplay();else if(operator.equals("%"))number=number/100;else if(operator.equals("+/-"))number=number*(-1);else if(operator.equals("="))number=getNumberFromDisplay();long t1;double t2;t1=(long)number;t2=number-t1;if(t2==0)display.setText(String.valueOf(t1));elsedisplay.setText(String.valueOf(number));operator=key;firstDigit=true;}private double getNumberFromDisplay(){return Double.valueOf(display.getText()).doubleValue(); }private void handleC(){display.setText("0");firstDigit=true;operator="=";}private void handleBackspace(){String text=display.getText();int i=text.length();if(i>0){text=text.substring(0,i-1);if(text.length()==0){display.setText("0");firstDigit=true;operator="=";}elsedisplay.setText(text);}}private void daoShu(){if (display.getText().equals("0")){display.setText("除数不能为零");System.out.println(1);}else{long l;double d=1/getNumberFromDisplay();l=(long)d;System.out.println(d);System.out.println(l);display.setText(String.valueOf(d));}}}2.CalFrame 类import java.awt.Color;importimport java.awt.event.ActionListener;import java.awt.event.WindowAdapter;import java.awt.event.WindowEvent;import javax.swing.JApplet;import javax.swing.JFrame;public class Test {public static void main(String[] args) {JFrame f=new JFrame();f.getAccessibleContext();Calculator Calculator1=new Calculator(); Calculator1.init();f.getContentPane().add("Center",Calculator1);f.setVisible(true);f.setBounds(300, 200,380,245);f.setBackground(Color.LIGHT_GRAY);f.validate();f.setResizable(false);f.addWindowListener(new WindowAdapter(){public void windowClosing(WindowEvent e){System.exit(0);}});f.setTitle("计算器");}}。
Java记事本源代码(完整)
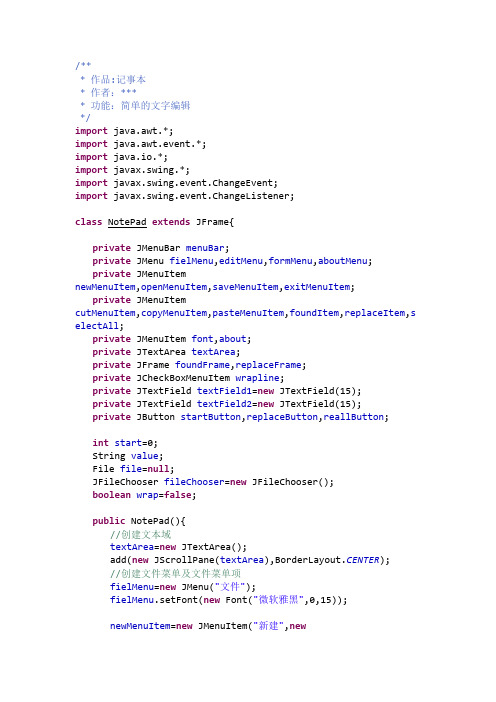
/*** 作品:记事本* 作者:**** 功能:简单的文字编辑*/import java.awt.*;import java.awt.event.*;import java.io.*;import javax.swing.*;import javax.swing.event.ChangeEvent;import javax.swing.event.ChangeListener;class NotePad extends JFrame{private JMenuBar menuBar;private JMenu fielMenu,editMenu,formMenu,aboutMenu;private JMenuItemnewMenuItem,openMenuItem,saveMenuItem,exitMenuItem;private JMenuItemcutMenuItem,copyMenuItem,pasteMenuItem,foundItem,replaceItem,s electAll;private JMenuItem font,about;private JTextArea textArea;private JFrame foundFrame,replaceFrame;private JCheckBoxMenuItem wrapline;private JTextField textField1=new JTextField(15);private JTextField textField2=new JTextField(15);private JButton startButton,replaceButton,reallButton;int start=0;String value;File file=null;JFileChooser fileChooser=new JFileChooser();boolean wrap=false;public NotePad(){//创建文本域textArea=new JTextArea();add(new JScrollPane(textArea),BorderLayout.CENTER);//创建文件菜单及文件菜单项fielMenu=new JMenu("文件");fielMenu.setFont(new Font("微软雅黑",0,15));newMenuItem=new JMenuItem("新建",newImageIcon("icons\\new24.gif"));newMenuItem.setFont(new Font("微软雅黑",Font.BOLD,13));newMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent. VK_N,InputEvent.CTRL_MASK));newMenuItem.addActionListener(listener);openMenuItem=new JMenuItem("打开",newImageIcon("icons\\open24.gif"));openMenuItem.setFont(new Font("微软雅黑",Font.BOLD,13));openMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent .VK_O,InputEvent.CTRL_MASK));openMenuItem.addActionListener(listener);saveMenuItem=new JMenuItem("保存",newImageIcon("icons\\save.gif"));saveMenuItem.setFont(new Font("微软雅黑",Font.BOLD,13));saveMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent .VK_S,InputEvent.CTRL_MASK));saveMenuItem.addActionListener(listener);exitMenuItem=new JMenuItem("退出",newImageIcon("icons\\exit24.gif"));exitMenuItem.setFont(new Font("微软雅黑",Font.BOLD,13));exitMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent .VK_E,InputEvent.CTRL_MASK));exitMenuItem.addActionListener(listener);//创建编辑菜单及菜单项editMenu=new JMenu("编辑");editMenu.setFont(new Font("微软雅黑",0,15));cutMenuItem=new JMenuItem("剪切",newImageIcon("icons\\cut24.gif"));cutMenuItem.setFont(new Font("微软雅黑",Font.BOLD,13));cutMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent. VK_X,InputEvent.CTRL_MASK));cutMenuItem.addActionListener(listener);copyMenuItem=new JMenuItem("复制",newImageIcon("icons\\copy24.gif"));copyMenuItem.setFont(new Font("微软雅黑",Font.BOLD,13));copyMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent .VK_C,InputEvent.CTRL_MASK));copyMenuItem.addActionListener(listener);pasteMenuItem=new JMenuItem("粘贴",newImageIcon("icons\\paste24.gif"));pasteMenuItem.setFont(new Font("微软雅黑",Font.BOLD,13));pasteMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEven t.VK_V,InputEvent.CTRL_MASK));pasteMenuItem.addActionListener(listener);foundItem=new JMenuItem("查找");foundItem.setFont(new Font("微软雅黑",Font.BOLD,13));foundItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK _F,InputEvent.CTRL_MASK));foundItem.addActionListener(listener);replaceItem=new JMenuItem("替换");replaceItem.setFont(new Font("微软雅黑",Font.BOLD,13));replaceItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent. VK_R,InputEvent.CTRL_MASK));replaceItem.addActionListener(listener);selectAll=new JMenuItem("全选");selectAll.setFont(new Font("微软雅黑",Font.BOLD,13));selectAll.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK _A,InputEvent.CTRL_MASK));selectAll.addActionListener(listener);//创建格式菜单及菜单项formMenu=new JMenu("格式");formMenu.setFont(new Font("微软雅黑",0,15));wrapline=new JCheckBoxMenuItem("自动换行");wrapline.setFont(new Font("微软雅黑",Font.BOLD,13));wrapline.addActionListener(listener);wrapline.addChangeListener(new ChangeListener() {public void stateChanged(ChangeEvent e) {if(wrapline.isSelected()){textArea.setLineWrap(true);}elsetextArea.setLineWrap(false);}});font=new JMenuItem("字体");font.setFont(new Font("微软雅黑",Font.BOLD,13)); font.addActionListener(listener);//创建关于菜单aboutMenu=new JMenu("关于");aboutMenu.setFont(new Font("微软雅黑",0,15)); about=new JMenuItem("记事本……");about.setFont(new Font("微软雅黑",Font.BOLD,13)); about.addActionListener(listener);//添加文件菜单项fielMenu.add(newMenuItem);fielMenu.add(openMenuItem);fielMenu.add(saveMenuItem);fielMenu.addSeparator();fielMenu.add(exitMenuItem);//添加编辑菜单项editMenu.add(cutMenuItem);editMenu.add(copyMenuItem);editMenu.add(pasteMenuItem);editMenu.add(foundItem);editMenu.add(replaceItem);editMenu.addSeparator();editMenu.add(selectAll);//添加格式菜单项formMenu.add(wrapline);formMenu.add(font);//添加关于菜单项aboutMenu.add(about);//添加菜单menuBar=new JMenuBar();menuBar.add(fielMenu);menuBar.add(editMenu);menuBar.add(formMenu);menuBar.add(aboutMenu);setJMenuBar(menuBar);//创建两个框架,用作查找和替换foundFrame=new JFrame();replaceFrame=new JFrame();//创建两个文本框textField1=new JTextField(15);textField2=new JTextField(15);startButton=new JButton("开始");startButton.addActionListener(listener);replaceButton=new JButton("替换为");replaceButton.addActionListener(listener);reallButton=new JButton("全部替换");reallButton.addActionListener(listener);}//创建菜单项事件监听器ActionListener listener=new ActionListener() {public void actionPerformed(ActionEvent e) {String name=e.getActionCommand();if(e.getSource() instanceof JMenuItem){if("新建".equals(name)){textArea.setText("");file=null;}if("打开".equals(name)){if(file!=null){fileChooser.setSelectedFile(file);}intreturnVal=fileChooser.showOpenDialog(NotePad.this);if(returnVal==JFileChooser.APPROVE_OPTION){file=fileChooser.getSelectedFile();}try{FileReader reader=new FileReader(file);int len=(int)file.length();char[] array=new char[len];reader.read(array,0,len);reader.close();textArea.setText(new String(array));}catch(Exception e_open){e_open.printStackTrace();}}}if("保存".equals(name)){if(file!=null){fileChooser.setSelectedFile(file);}intreturnVal=fileChooser.showSaveDialog(NotePad.this);if(returnVal==JFileChooser.APPROVE_OPTION){file=fileChooser.getSelectedFile();}try{FileWriter writer=new FileWriter(file);writer.write(textArea.getText());writer.close();}catch (Exception e_save) {e_save.getStackTrace();}}if("退出".equals(name)){System.exit(0);}if("剪切".equals(name)){textArea.cut();}if("复制".equals(name)){textArea.copy();}if("粘贴".equals(name)){textArea.paste();}if("查找".equals(name)){value=textArea.getText();foundFrame.add(textField1,BorderLayout.CENTER);foundFrame.add(startButton,BorderLayout.SOUTH);foundFrame.setLocation(300,300);foundFrame.setTitle("查找");foundFrame.pack();foundFrame.setVisible(true);foundFrame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE );}if("替换".equals(name)){value=textArea.getText();JLabel label1=new JLabel("查找内容:");JLabel label2=new JLabel("替换为:");JPanel panel1=new JPanel();panel1.setLayout(new GridLayout(2,2));JPanel panel2=new JPanel();panel2.setLayout(new GridLayout(1,3));replaceFrame.add(panel1,BorderLayout.NORTH);replaceFrame.add(panel2,BorderLayout.CENTER);panel1.add(label1);panel1.add(textField1);panel1.add(label2);panel1.add(textField2);panel2.add(startButton);panel2.add(replaceButton);panel2.add(reallButton);replaceFrame.setTitle("替换");replaceFrame.setLocation(300,300);replaceFrame.pack();replaceFrame.setVisible(true);replaceFrame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLO SE);}if("开始".equals(name)||"下一个".equals(name)){String temp=textField1.getText();int s=value.indexOf(temp,start);if(value.indexOf(temp,start)!=-1){textArea.setSelectionStart(s);textArea.setSelectionEnd(s+temp.length());textArea.setSelectedTextColor(Color.GREEN);start=s+1;startButton.setText("下一个");}else {JOptionPane.showMessageDialog(foundFrame, "查找完毕!", "提示", 0,new ImageIcon("icons\\search.gif"));foundFrame.dispose();}}if("替换为".equals(name)){String temp=textField1.getText();int s=value.indexOf(temp,start);if(value.indexOf(temp,start)!=-1){textArea.setSelectionStart(s);textArea.setSelectionEnd(s+temp.length());textArea.setSelectedTextColor(Color.GREEN);start=s+1;textArea.replaceSelection(textField2.getText());}else {JOptionPane.showMessageDialog(foundFrame, "查找完毕!", "提示", 0,new ImageIcon("icons\\search.gif"));foundFrame.dispose();}}if("全部替换".equals(name)){String temp=textArea.getText();temp=temp.replaceAll(textField1.getText(),textField2.getTex t());textArea.setText(temp);}if("全选".equals(name)){textArea.selectAll();}if("字体".equals(name)){FontDialog fontDialog=newFontDialog(NotePad.this);fontDialog.setVisible(true);if(textArea.getFont()!=fontDialog.getFont()){textArea.setFont(fontDialog.getFont());}}if("记事本……".equals(name)){AboutDialog aboutDialog=newAboutDialog(NotePad.this);aboutDialog.setVisible(true);}}};//创建字体设置对话面板,并添加相应事件监听器class FontDialog extends JDialog implements ItemListener, ActionListener, WindowListener{public JCheckBox Bold=new JCheckBox("Bold",false);public JCheckBox Italic=new JCheckBox("Italic",false);public List Size,Name;public int FontName;public int FontStyle;public int FontSize;public JButton OK=new JButton("OK");public JButton Cancel=new JButton("Cancel");public JTextArea Text=new JTextArea("字体预览文本域\n0123456789\nAaBbCcXxYyZz");public FontDialog(JFrame owner) {super(owner,"字体设置",true);GraphicsEnvironmentg=GraphicsEnvironment.getLocalGraphicsEnvironment();String name[]=g.getAvailableFontFamilyNames();Name=new List();Size=new List();FontName=0;FontStyle=0;FontSize=8;int i=0;Name.add("Default Value");for(i=0;i<name.length;i++)Name.add(name[i]);for(i=8;i<257;i++)Size.add(String.valueOf(i));this.setLayout(null);this.setBounds(250,200,480, 306);this.setResizable(false);OK.setFocusable(false);Cancel.setFocusable(false);Bold.setFocusable(false);Italic.setFocusable(false);Name.setFocusable(false);Size.setFocusable(false);Name.setBounds(10, 10, 212, 259);this.add(Name);Bold.setBounds(314, 10, 64, 22);this.add(Bold);Italic.setBounds(388, 10, 64, 22);this.add(Italic);Size.setBounds(232, 10, 64, 259);this.add(Size);Text.setBounds(306, 40, 157, 157);this.add(Text);OK.setBounds(306, 243, 74, 26);this.add(OK);Cancel.setBounds(390, 243, 74, 26);this.add(Cancel);Name.select(FontName);Size.select(FontSize);Text.setFont(getFont());Name.addItemListener(this);Size.addItemListener(this);Bold.addItemListener(this);Italic.addItemListener(this);OK.addActionListener(this);Cancel.addActionListener(this);this.addWindowListener(this);}public void itemStateChanged(ItemEvent e) {Text.setFont(getFont());}public void actionPerformed(ActionEvent e) {if(e.getSource()==OK){FontName=Name.getSelectedIndex();FontStyle=getStyle();FontSize=Size.getSelectedIndex();this.setVisible(false);}else cancel();}public void windowClosing(WindowEvent e) {cancel();}public Font getFont(){if(Name.getSelectedIndex()==0) return new Font("新宋体",getStyle(),Size.getSelectedIndex()+8);else return newFont(Name.getSelectedItem(),getStyle(),Size.getSelectedIndex() +8);}public void cancel(){Name.select(FontName);Size.select(FontSize);setStyle();Text.setFont(getFont());this.setVisible(false);}public void setStyle(){if(FontStyle==0 || FontStyle==2)Bold.setSelected(false);else Bold.setSelected(true);if(FontStyle==0 || FontStyle==1)Italic.setSelected(false);else Italic.setSelected(true);}public int getStyle(){int bold=0,italic=0;if(Bold.isSelected()) bold=1;if(Italic.isSelected()) italic=1;return bold+italic*2;}public void windowActivated(WindowEvent arg0) {}public void windowClosed(WindowEvent arg0) {}public void windowDeactivated(WindowEvent arg0) {}public void windowDeiconified(WindowEvent arg0) {}public void windowIconified(WindowEvent arg0) {}public void windowOpened(WindowEvent arg0) {} }//创建关于对话框class AboutDialog extends JDialog implements ActionListener{ public JButton OK,Icon;public JLabel Name,Version,Author,Java;public JPanel Panel;AboutDialog(JFrame owner) {super(owner,"关于",true);OK=new JButton("OK");Icon=new JButton(new ImageIcon("icons\\edit.gif"));Name=new JLabel("Notepad");Version=new JLabel("Version 1.0");Java=new JLabel("JRE Version 6.0");Author=new JLabel("Copyright (c) 11-5-2012 By Jianmin Chen");Panel=new JPanel();Color c=new Color(0,95,191);Name.setForeground(c);Version.setForeground(c);Java.setForeground(c);Author.setForeground(c);Panel.setBackground(Color.white);OK.setFocusable(false);this.setBounds(250,200,280, 180);this.setResizable(false);this.setLayout(null);Panel.setLayout(null);OK.addActionListener(this);Icon.setFocusable(false);Icon.setBorderPainted(false);Author.setFont(new Font(null,Font.PLAIN,11));Panel.add(Icon);Panel.add(Name);Panel.add(Version);Panel.add(Author);Panel.add(Java);this.add(Panel);this.add(OK);Panel.setBounds(0, 0, 280, 100);OK.setBounds(180, 114, 72, 26);Name.setBounds(80, 10, 160, 20);Version.setBounds(80, 27, 160, 20);Author.setBounds(15, 70, 250, 20);Java.setBounds(80, 44, 160, 20);Icon.setBounds(16, 14, 48, 48);}public void actionPerformed(ActionEvent e) { this.setVisible(false);}}}//创建记事本public class ChenJianmin {public static void main(String[] args){EventQueue.invokeLater(new Runnable() {public void run() {NotePad notePad=new NotePad();notePad.setTitle("记事本");notePad.setVisible(true);notePad.setBounds(100,100,800,600);notePad.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);}});}}。
简单的java代码

简单的java代码简单的java代码Java是一种面向对象的编程语言,它具有简单、可移植、安全和高性能等特点。
在Java中,我们可以编写各种各样的代码,从简单的“Hello World”程序到复杂的企业级应用程序都可以使用Java来实现。
在本文中,我们将介绍一些简单的Java代码示例。
一、Hello World程序“Hello World”程序是任何编程语言中最基本和最常见的程序之一。
在Java中,我们可以使用以下代码来实现:```public class HelloWorld {public static void main(String[] args) {System.out.println("Hello, World!");}}```这个程序很简单,它定义了一个名为“HelloWorld”的类,并在其中定义了一个名为“main”的方法。
该方法通过调用System.out.println()方法来输出“Hello, World!”字符串。
二、计算两个数之和下面是一个简单的Java程序,用于计算两个数之和:```import java.util.Scanner;public class AddTwoNumbers {public static void main(String[] args) {int num1, num2, sum;Scanner input = new Scanner(System.in);System.out.print("Enter first number: ");num1 = input.nextInt();System.out.print("Enter second number: ");num2 = input.nextInt();sum = num1 + num2;System.out.println("Sum of the two numbers is " + sum); }}该程序首先导入了java.util.Scanner类,以便从控制台读取输入。
java实验报告代码

java实验报告代码Java 实验报告代码引言:Java 是一种面向对象的编程语言,广泛应用于软件开发领域。
本实验报告将介绍我在学习 Java 过程中的实验代码,并对其进行分析和总结。
一、实验背景在学习 Java 编程语言时,实验是一种非常重要的学习方式。
通过实验,我们可以将理论知识应用于实际项目中,提升自己的编程能力和解决问题的能力。
二、实验目的本次实验的目的是通过编写 Java 代码,实现一些基本的功能,包括输入输出、条件语句、循环语句、数组和函数等。
三、实验过程1. 输入输出在 Java 中,可以使用 Scanner 类来实现输入输出操作。
下面是一个示例代码:```javaimport java.util.Scanner;public class InputOutputExample {public static void main(String[] args) {Scanner scanner = new Scanner(System.in);System.out.print("请输入一个整数:");int num = scanner.nextInt();System.out.println("您输入的整数是:" + num);}}```上述代码中,我们使用 Scanner 类的 nextInt() 方法来读取用户输入的整数,并使用 System.out.println() 方法将结果输出到控制台。
2. 条件语句条件语句是根据条件判断来执行不同的代码块。
下面是一个示例代码:```javapublic class ConditionalStatementExample {public static void main(String[] args) {int num = 10;if (num > 0) {System.out.println("这个数是正数");} else if (num < 0) {System.out.println("这个数是负数");} else {System.out.println("这个数是零");}}}```上述代码中,我们使用 if-else 语句来判断一个数是正数、负数还是零,并根据不同的条件输出不同的结果。
第一行java代码
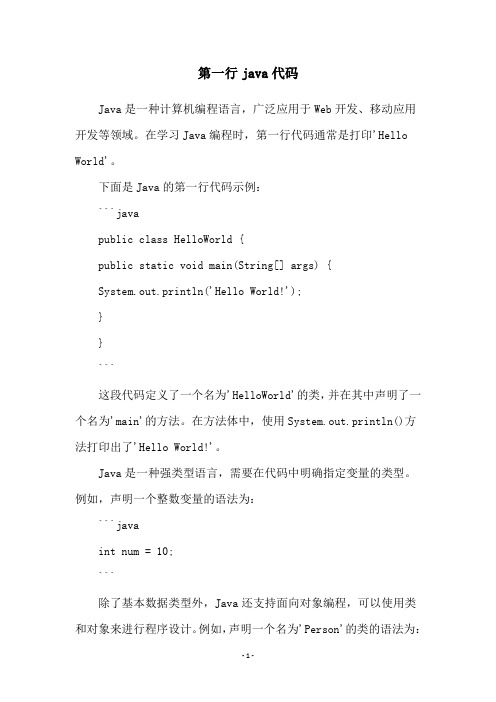
第一行java代码Java是一种计算机编程语言,广泛应用于Web开发、移动应用开发等领域。
在学习Java编程时,第一行代码通常是打印'Hello World'。
下面是Java的第一行代码示例:```javapublic class HelloWorld {public static void main(String[] args) {System.out.println('Hello World!');}}```这段代码定义了一个名为'HelloWorld'的类,并在其中声明了一个名为'main'的方法。
在方法体中,使用System.out.println()方法打印出了'Hello World!'。
Java是一种强类型语言,需要在代码中明确指定变量的类型。
例如,声明一个整数变量的语法为:```javaint num = 10;```除了基本数据类型外,Java还支持面向对象编程,可以使用类和对象来进行程序设计。
例如,声明一个名为'Person'的类的语法为:```javapublic class Person {String name;int age;double height;}```在实际使用中,可以创建Person对象,并对其属性进行赋值和访问:```javaPerson p = new Person(); = '张三';p.age = 20;p.height = 1.8;```Java还有许多其他特性和语法,需要逐步学习和掌握。
(完整word版)JAVA代码规范详细版

JAVA代码规范本Java代码规范以SUN的标准Java代码规范为基础,为适应我们公司的实际需要,可能会做一些修改。
本文档中没有说明的地方,请参看SUN Java标准代码规范。
如果两边有冲突,以SUN Java标准为准。
1. 标识符命名规范1.1 概述标识符的命名力求做到统一、达意和简洁。
1.1.1 统一统一是指,对于同一个概念,在程序中用同一种表示方法,比如对于供应商,既可以用supplier,也可以用provider,但是我们只能选定一个使用,至少在一个Java项目中保持统一。
统一是作为重要的,如果对同一概念有不同的表示方法,会使代码混乱难以理解。
即使不能取得好的名称,但是只要统一,阅读起来也不会太困难,因为阅读者只要理解一次。
1.1.2 达意达意是指,标识符能准确的表达出它所代表的意义,比如:newSupplier, OrderPaymentGatewayService等;而supplier1, service2,idtts等则不是好的命名方式。
准确有两成含义,一是正确,而是丰富。
如果给一个代表供应商的变量起名是order,显然没有正确表达。
同样的,supplier1, 远没有targetSupplier意义丰富。
1.1.3 简洁简洁是指,在统一和达意的前提下,用尽量少的标识符。
如果不能达意,宁愿不要简洁。
比如:theOrderNameOfTheTargetSupplierWhichIsTransfered 太长,transferedTargetSupplierOrderName则较好,但是transTgtSplOrdNm就不好了。
省略元音的缩写方式不要使用,我们的英语往往还没有好到看得懂奇怪的缩写。
1.1.4 骆驼法则Java中,除了包名,静态常量等特殊情况,大部分情况下标识符使用骆驼法则,即单词之间不使用特殊符号分割,而是通过首字母大写来分割。
比如: supplierName, addNewContract,而不是supplier_name, add_new_contract。
JAVA代码大全
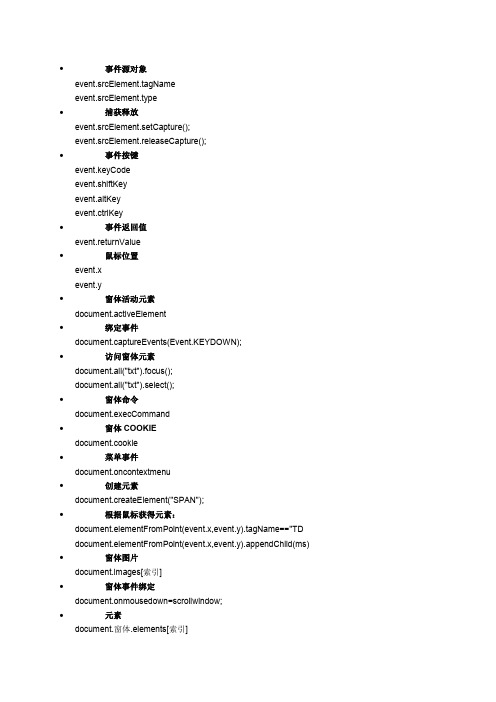
UNCODE 编码
escape() ,unescape
父对象
obj.parentElement(dhtml)
obj.parentNode(dom)
交换表的行
TableID.moveRow(2,1)
替换 CSS
document.all.csss.href = "a.css";
并排显示
display:inline
//过滤数字
<input type=text onkeypress="return event.keyCode>=48&&event.keyCode<=57||(t his.value.indexOf(‘.‘)<0?event.keyCode==46:false)" onpaste="return !clipboar dData.getData(‘text‘).match(/\D/)" ondragenter="return false">
encodeURIComponent 对":"、"/"、";" 和 "?"也编码
表格行指示
<tr onmouseover="this.bgColor=‘#f0f0f0‘" onmouseout="this.bgColor=‘#ffffff‘">
//各种尺寸
s += "\r\n 网页可见区域宽:"+ document.body.clientWidth; s += "\r\n 网页可见区域高:"+ document.body.clientHeight; s += "\r\n 网页可见区域高:"+ document.body.offsetWeight +" (包括边线的宽)"; s += "\r\n 网页可见区域高:"+ document.body.offsetHeight +" (包括边线的宽)"; s += "\r\n 网页正文全文宽:"+ document.body.scrollWidth; s += "\r\n 网页正文全文高:"+ document.body.scrollHeight; s += "\r\n 网页被卷去的高:"+ document.body.scrollTop; s += "\r\n 网页被卷去的左:"+ document.body.scrollLeft; s += "\r\n 网页正文部分上:"+ window.screenTop; s += "\r\n 网页正文部分左:"+ window.screenLeft; s += "\r\n 屏幕分辨率的高:"+ window.screen.height; s += "\r\n 屏幕分辨率的宽:"+ window.screen.width; s += "\r\n 屏幕可用工作区高度:"+ window.screen.availHeight; s += "\r\n 屏幕可用工作区宽度:"+ window.screen.availWidth;
java项目代码
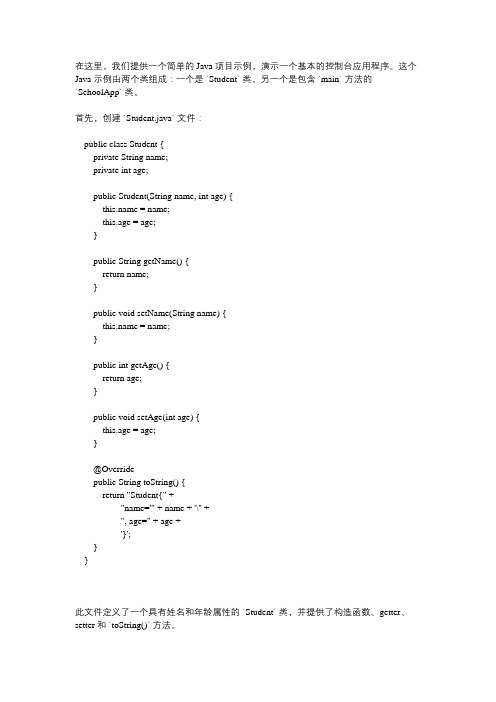
在这里,我们提供一个简单的 Java 项目示例,演示一个基本的控制台应用程序。
这个Java 示例由两个类组成:一个是 `Student` 类,另一个是包含 `main` 方法的`SchoolApp` 类。
首先,创建 `Student.java` 文件:public class Student {private String name;private int age;public Student(String name, int age) { = name;this.age = age;}public String getName() {return name;}public void setName(String name) { = name;}public int getAge() {return age;}public void setAge(int age) {this.age = age;}@Overridepublic String toString() {return "Student{" +"name='" + name + '\'' +", age=" + age +'}';}}此文件定义了一个具有姓名和年龄属性的 `Student` 类,并提供了构造函数、getter、setter 和 `toString()` 方法。
接下来,创建包含 `main` 方法的 `SchoolApp.java` 文件:import java.util.ArrayList;import java.util.List;public class SchoolApp {public static void main(String[] args) {// 创建一个学生列表List<Student> students = new ArrayList<>();// 添加三名学生students.add(new Student("Alice", 20));students.add(new Student("Bob", 22));students.add(new Student("Charlie", 19));// 输出学生信息System.out.println("学生列表:");for (Student student : students) {System.out.println(student);}// 更改其中一名学生的姓名students.get(0).setName("Alicia");System.out.println("\n更新后的学生列表:");for (Student student : students) {System.out.println(student);}}}在这个文件中,我们创建了一个 `Student` 对象的列表,并向其中添加了一些学生。
最让你惊艳的java代码
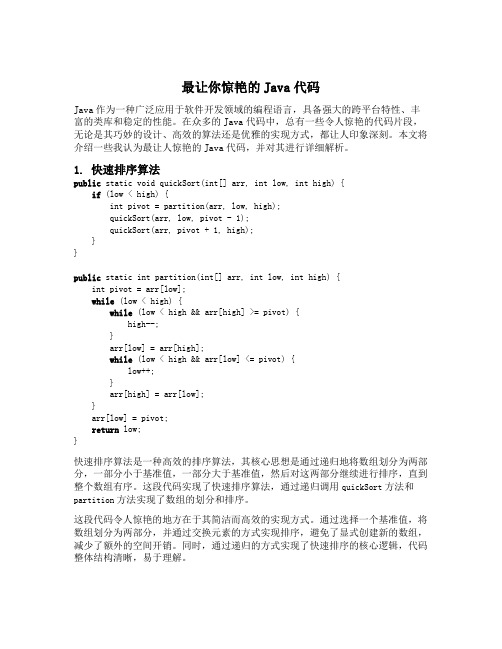
最让你惊艳的Java代码Java作为一种广泛应用于软件开发领域的编程语言,具备强大的跨平台特性、丰富的类库和稳定的性能。
在众多的Java代码中,总有一些令人惊艳的代码片段,无论是其巧妙的设计、高效的算法还是优雅的实现方式,都让人印象深刻。
本文将介绍一些我认为最让人惊艳的Java代码,并对其进行详细解析。
1. 快速排序算法public static void quickSort(int[] arr, int low, int high) {if (low < high) {int pivot = partition(arr, low, high);quickSort(arr, low, pivot - 1);quickSort(arr, pivot + 1, high);}}public static int partition(int[] arr, int low, int high) {int pivot = arr[low];while (low < high) {while (low < high && arr[high] >= pivot) {high--;}arr[low] = arr[high];while (low < high && arr[low] <= pivot) {low++;}arr[high] = arr[low];}arr[low] = pivot;return low;}快速排序算法是一种高效的排序算法,其核心思想是通过递归地将数组划分为两部分,一部分小于基准值,一部分大于基准值,然后对这两部分继续进行排序,直到整个数组有序。
这段代码实现了快速排序算法,通过递归调用quickSort方法和partition方法实现了数组的划分和排序。
这段代码令人惊艳的地方在于其简洁而高效的实现方式。
通过选择一个基准值,将数组划分为两部分,并通过交换元素的方式实现排序,避免了显式创建新的数组,减少了额外的空间开销。
java常用代码(20条案例)

java常用代码(20条案例)1. 输出Hello World字符串public class Main {public static void main(String[] args) {// 使用System.out.println()方法输出字符串"Hello World"System.out.println("Hello World");}}2. 定义一个整型变量并进行赋值public class Main {public static void main(String[] args) {// 定义一个名为num的整型变量并将其赋值为10int num = 10;// 使用System.out.println()方法输出变量num的值System.out.println(num);}}3. 循环打印数字1到10public class Main {public static void main(String[] args) {// 使用for循环遍历数字1到10for (int i = 1; i <= 10; i++) {// 使用System.out.println()方法输出每个数字System.out.println(i);}}}4. 实现输入输出import java.util.Scanner;public class Main {public static void main(String[] args) {// 创建一个Scanner对象scanner,以便接受用户的输入Scanner scanner = new Scanner(System.in);// 使用scanner.nextLine()方法获取用户输入的字符串String input = scanner.nextLine();// 使用System.out.println()方法输出输入的内容System.out.println("输入的是:" + input);}}5. 实现条件分支public class Main {public static void main(String[] args) {// 定义一个整型变量num并将其赋值为10int num = 10;// 使用if语句判断num是否大于0,如果是,则输出"这个数是正数",否则输出"这个数是负数"if (num > 0) {System.out.println("这个数是正数");} else {System.out.println("这个数是负数");}}}6. 使用数组存储数据public class Main {public static void main(String[] args) {// 定义一个整型数组nums,其中包含数字1到5int[] nums = new int[]{1, 2, 3, 4, 5};// 使用for循环遍历数组for (int i = 0; i < nums.length; i++) {// 使用System.out.println()方法输出每个数组元素的值System.out.println(nums[i]);}}}7. 打印字符串长度public class Main {public static void main(String[] args) {// 定义一个字符串变量str并将其赋值为"HelloWorld"String str = "Hello World";// 使用str.length()方法获取字符串的长度,并使用System.out.println()方法输出长度System.out.println(str.length());}}8. 字符串拼接public class Main {public static void main(String[] args) {// 定义两个字符串变量str1和str2,并分别赋值为"Hello"和"World"String str1 = "Hello";String str2 = "World";// 使用"+"号将两个字符串拼接成一个新字符串,并使用System.out.println()方法输出拼接后的结果System.out.println(str1 + " " + str2);}}9. 使用方法进行多次调用public class Main {public static void main(String[] args) {// 定义一个名为str的字符串变量并将其赋值为"Hello World"String str = "Hello World";// 调用printStr()方法,打印字符串变量str的值printStr(str);// 调用add()方法,计算两个整数的和并输出结果int result = add(1, 2);System.out.println(result);}// 定义一个静态方法printStr,用于打印字符串public static void printStr(String str) {System.out.println(str);}// 定义一个静态方法add,用于计算两个整数的和public static int add(int a, int b) {return a + b;}}10. 使用继承实现多态public class Main {public static void main(String[] args) {// 创建一个Animal对象animal,并调用move()方法Animal animal = new Animal();animal.move();// 创建一个Dog对象dog,并调用move()方法Dog dog = new Dog();dog.move();// 创建一个Animal对象animal2,但其实际指向一个Dog对象,同样调用move()方法Animal animal2 = new Dog();animal2.move();}}// 定义一个Animal类class Animal {public void move() {System.out.println("动物在移动");}}// 定义一个Dog类,继承自Animal,并重写了move()方法class Dog extends Animal {public void move() {System.out.println("狗在奔跑");}}11. 输入多个数并求和import java.util.Scanner;public class Main {public static void main(String[] args) {// 创建一个Scanner对象scanner,以便接受用户的输入Scanner scanner = new Scanner(System.in);// 定义一个整型变量sum并将其赋值为0int sum = 0;// 使用while循环持续获取用户输入的整数并计算总和,直到用户输入为0时结束循环while (true) {System.out.println("请输入一个整数(输入0退出):");int num = scanner.nextInt();if (num == 0) {break;}sum += num;}// 使用System.out.println()方法输出总和System.out.println("所有输入的数的和为:" + sum);}}12. 判断一个年份是否为闰年import java.util.Scanner;public class Main {public static void main(String[] args) {// 创建一个Scanner对象scanner,以便接受用户的输入Scanner scanner = new Scanner(System.in);// 使用scanner.nextInt()方法获取用户输入的年份System.out.println("请输入一个年份:");int year = scanner.nextInt();// 使用if语句判断年份是否为闰年,如果是,则输出"是闰年",否则输出"不是闰年"if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {System.out.println(year + "年是闰年");} else {System.out.println(year + "年不是闰年");}}}13. 使用递归实现斐波那契数列import java.util.Scanner;public class Main {public static void main(String[] args) {// 创建一个Scanner对象scanner,以便接受用户的输入Scanner scanner = new Scanner(System.in);// 使用scanner.nextInt()方法获取用户输入的正整数nSystem.out.println("请输入一个正整数:");int n = scanner.nextInt();// 使用for循环遍历斐波那契数列for (int i = 1; i <= n; i++) {System.out.print(fibonacci(i) + " ");}}// 定义一个静态方法fibonacci,使用递归计算斐波那契数列的第n项public static int fibonacci(int n) {if (n <= 2) {return 1;} else {return fibonacci(n - 1) + fibonacci(n - 2);}}}14. 输出九九乘法表public class Main {public static void main(String[] args) {// 使用两层for循环打印九九乘法表for (int i = 1; i <= 9; i++) {for (int j = 1; j <= i; j++) {System.out.print(j + "*" + i + "=" + (i * j) + "\t");}System.out.println();}}}15. 使用try-catch-finally处理异常import java.util.Scanner;public class Main {public static void main(String[] args) {// 创建一个Scanner对象scanner,以便接受用户的输入Scanner scanner = new Scanner(System.in);try {// 使用scanner.nextInt()方法获取用户输入的整数a和bSystem.out.println("请输入两个整数:");int a = scanner.nextInt();int b = scanner.nextInt();// 对a进行除以b的运算int result = a / b;// 使用System.out.println()方法输出结果System.out.println("计算结果为:" + result);} catch (ArithmeticException e) {// 如果除数为0,会抛出ArithmeticException异常,捕获异常并使用System.out.println()方法输出提示信息System.out.println("除数不能为0");} finally {// 使用System.out.println()方法输出提示信息System.out.println("程序结束");}}}16. 使用集合存储数据并遍历import java.util.ArrayList;import java.util.List;public class Main {public static void main(String[] args) {// 创建一个名为list的List集合,并添加多个字符串元素List<String> list = new ArrayList<>();list.add("Java");list.add("Python");list.add("C++");list.add("JavaScript");// 使用for循环遍历List集合并使用System.out.println()方法输出每个元素的值for (int i = 0; i < list.size(); i++) {System.out.println(list.get(i));}}}17. 使用Map存储数据并遍历import java.util.HashMap;import java.util.Map;public class Main {public static void main(String[] args) {// 创建一个名为map的Map对象,并添加多组键值对Map<Integer, String> map = new HashMap<>();map.put(1, "Java");map.put(2, "Python");map.put(3, "C++");map.put(4, "JavaScript");// 使用for-each循环遍历Map对象并使用System.out.println()方法输出每个键值对的值for (Map.Entry<Integer, String> entry :map.entrySet()) {System.out.println("key=" + entry.getKey() + ", value=" + entry.getValue());}}}18. 使用lambda表达式进行排序import java.util.ArrayList;import java.util.Collections;import parator;import java.util.List;public class Main {public static void main(String[] args) {// 创建一个名为list的List集合,并添加多个字符串元素List<String> list = new ArrayList<>();list.add("Java");list.add("Python");list.add("C++");list.add("JavaScript");// 使用lambda表达式定义Comparator接口的compare()方法,按照字符串长度进行排序Comparator<String> stringLengthComparator = (s1, s2) -> s1.length() - s2.length();// 使用Collections.sort()方法将List集合进行排序Collections.sort(list, stringLengthComparator);// 使用for-each循环遍历List集合并使用System.out.println()方法输出每个元素的值for (String str : list) {System.out.println(str);}}}19. 使用线程池执行任务import java.util.concurrent.ExecutorService;import java.util.concurrent.Executors;public class Main {public static void main(String[] args) {// 创建一个名为executor的线程池对象,其中包含2个线程ExecutorService executor =Executors.newFixedThreadPool(2);// 使用executor.execute()方法将多个Runnable任务加入线程池中进行执行executor.execute(new MyTask("任务1"));executor.execute(new MyTask("任务2"));executor.execute(new MyTask("任务3"));// 调用executor.shutdown()方法关闭线程池executor.shutdown();}}// 定义一个MyTask类,实现Runnable接口,用于代表一个任务class MyTask implements Runnable {private String name;public MyTask(String name) { = name;}@Overridepublic void run() {System.out.println("线程" +Thread.currentThread().getName() + "正在执行任务:" + name);try {Thread.sleep(2000);} catch (InterruptedException e) {e.printStackTrace();}System.out.println("线程" +Thread.currentThread().getName() + "完成任务:" + name);}}20. 使用JavaFX创建图形用户界面import javafx.application.Application;import javafx.scene.Scene;import javafx.scene.control.Button;import yout.StackPane;import javafx.stage.Stage;public class Main extends Application {@Overridepublic void start(Stage primaryStage) throws Exception { // 创建一个Button对象btn,并设置按钮名称Button btn = new Button("点击我");// 创建一个StackPane对象pane,并将btn添加到pane中StackPane pane = new StackPane();pane.getChildren().add(btn);// 创建一个Scene对象scene,并将pane作为参数传入Scene scene = new Scene(pane, 200, 100);// 将scene设置为primaryStage的场景primaryStage.setScene(scene);// 将primaryStage的标题设置为"JavaFX窗口"primaryStage.setTitle("JavaFX窗口");// 调用primaryStage.show()方法显示窗口primaryStage.show();}public static void main(String[] args) { launch(args);}}。
JAVA代码大全
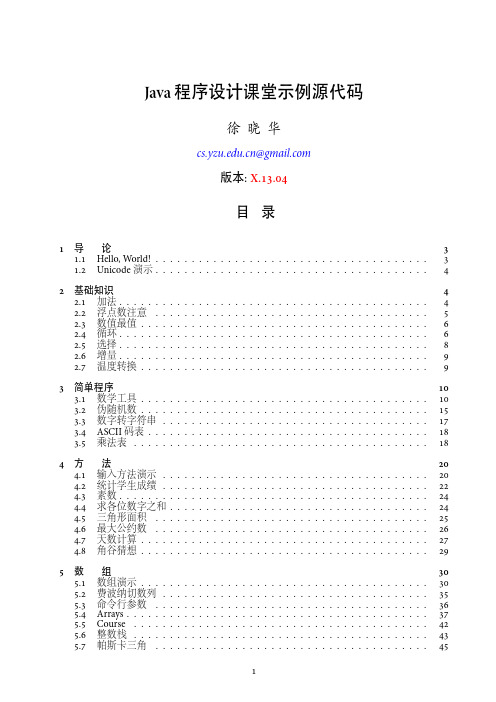
. 命令行参数 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. Arrays . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
方 法
. 输入方法演示 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. 统计学生成绩 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. ASCII 码表 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. 乘法表 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. Course . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. 整数栈 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
System.out.println(”Hello, World!”);
}
java新手代码大全

java新手代码大全Java新手代码大全。
Java是一种广泛使用的编程语言,对于新手来说,学习Java可能会遇到一些困难。
本文将为新手提供一些常见的Java代码示例,帮助他们更好地理解和掌握Java编程。
1. Hello World。
```java。
public class HelloWorld {。
public static void main(String[] args) {。
System.out.println("Hello, World!");}。
}。
```。
这是Java中最简单的程序,用于打印"Hello, World!"。
新手可以通过这个示例来了解一个基本的Java程序的结构和语法。
2. 变量和数据类型。
```java。
public class Variables {。
public static void main(String[] args) {。
int num1 = 10;double num2 = 5.5;String str = "Hello";System.out.println(num1);System.out.println(num2);System.out.println(str);}。
}。
```。
这个示例展示了Java中的基本数据类型和变量的声明和使用。
新手可以通过这个示例来学习如何定义和使用整型、浮点型和字符串类型的变量。
3. 条件语句。
```java。
public class ConditionalStatement {。
public static void main(String[] args) {。
int num = 10;if (num > 0) {。
System.out.println("Positive number");} else if (num < 0) {。
Java实现画图的详细步骤(完整代码)
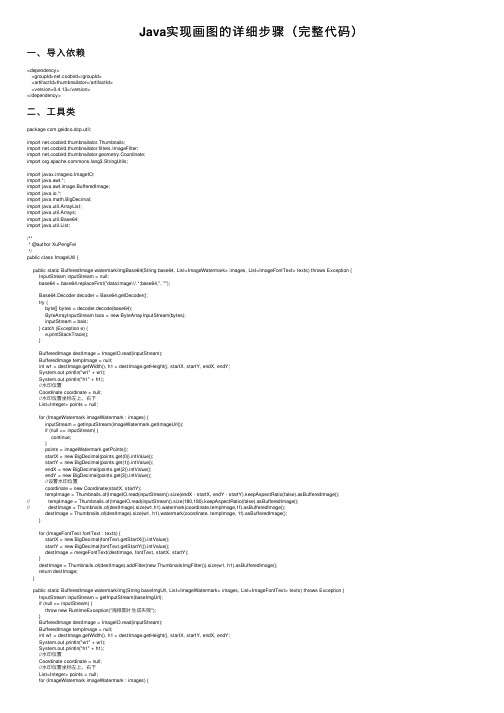
Java实现画图的详细步骤(完整代码)⼀、导⼊依赖<dependency><groupId>net.coobird</groupId><artifactId>thumbnailator</artifactId><version>0.4.13</version></dependency>⼆、⼯具类package com.geidco.dcp.util;import net.coobird.thumbnailator.Thumbnails;import net.coobird.thumbnailator.filters.ImageFilter;import net.coobird.thumbnailator.geometry.Coordinate;import ng3.StringUtils;import javax.imageio.ImageIO;import java.awt.*;import java.awt.image.BufferedImage;import java.io.*;import java.math.BigDecimal;import java.util.ArrayList;import java.util.Arrays;import java.util.Base64;import java.util.List;/*** @author XuPengFei*/public class ImageUtil {public static BufferedImage watermarkImgBase64(String base64, List<ImageWatermark> images, List<ImageFontText> texts) throws Exception {InputStream inputStream = null;base64 = base64.replaceFirst("data:image\\/.*;base64,", "");Base64.Decoder decoder = Base64.getDecoder();try {byte[] bytes = decoder.decode(base64);ByteArrayInputStream bais = new ByteArrayInputStream(bytes);inputStream = bais;} catch (Exception e) {e.printStackTrace();}BufferedImage destImage = ImageIO.read(inputStream);BufferedImage tempImage = null;int w1 = destImage.getWidth(), h1 = destImage.getHeight(), startX, startY, endX, endY;System.out.println("w1" + w1);System.out.println("h1" + h1);//⽔印位置Coordinate coordinate = null;//⽔印位置坐标左上、右下List<Integer> points = null;for (ImageWatermark imageWatermark : images) {inputStream = getInputStream(imageWatermark.getImageUrl());if (null == inputStream) {continue;}points = imageWatermark.getPoints();startX = new BigDecimal(points.get(0)).intValue();startY = new BigDecimal(points.get(1)).intValue();endX = new BigDecimal(points.get(2)).intValue();endY = new BigDecimal(points.get(3)).intValue();//设置⽔印位置coordinate = new Coordinate(startX, startY);tempImage = Thumbnails.of(ImageIO.read(inputStream)).size(endX - startX, endY - startY).keepAspectRatio(false).asBufferedImage();// tempImage = Thumbnails.of(ImageIO.read(inputStream)).size(180,180).keepAspectRatio(false).asBufferedImage();// destImage = Thumbnails.of(destImage).size(w1,h1).watermark(coordinate,tempImage,1f).asBufferedImage();destImage = Thumbnails.of(destImage).size(w1, h1).watermark(coordinate, tempImage, 1f).asBufferedImage();}for (ImageFontText fontText : texts) {startX = new BigDecimal(fontText.getStartX()).intValue();startY = new BigDecimal(fontText.getStartY()).intValue();destImage = mergeFontText(destImage, fontText, startX, startY);}destImage = Thumbnails.of(destImage).addFilter(new ThumbnailsImgFilter()).size(w1, h1).asBufferedImage();return destImage;}public static BufferedImage watermarkImg(String baseImgUrl, List<ImageWatermark> images, List<ImageFontText> texts) throws Exception {InputStream inputStream = getInputStream(baseImgUrl);if (null == inputStream) {throw new RuntimeException("海报图⽚⽣成失败");}BufferedImage destImage = ImageIO.read(inputStream);BufferedImage tempImage = null;int w1 = destImage.getWidth(), h1 = destImage.getHeight(), startX, startY, endX, endY;System.out.println("w1" + w1);System.out.println("h1" + h1);//⽔印位置Coordinate coordinate = null;//⽔印位置坐标左上、右下List<Integer> points = null;for (ImageWatermark imageWatermark : images) {inputStream = getInputStream(imageWatermark.getImageUrl());if (null == inputStream) {continue;}points = imageWatermark.getPoints();startX = new BigDecimal(points.get(0)).intValue();startY = new BigDecimal(points.get(1)).intValue();endX = new BigDecimal(points.get(2)).intValue();endY = new BigDecimal(points.get(3)).intValue();//设置⽔印位置coordinate = new Coordinate(startX, startY);tempImage = Thumbnails.of(ImageIO.read(inputStream)).size(endX - startX, endY - startY).keepAspectRatio(false).asBufferedImage();// tempImage = Thumbnails.of(ImageIO.read(inputStream)).size(180,180).keepAspectRatio(false).asBufferedImage();// destImage = Thumbnails.of(destImage).size(w1,h1).watermark(coordinate,tempImage,1f).asBufferedImage();destImage = Thumbnails.of(destImage).addFilter(new ThumbnailsImgFilter()).size(w1, h1).watermark(coordinate, tempImage, 1f).asBufferedImage(); }for (ImageFontText fontText : texts) {startX = new BigDecimal(fontText.getStartX()).intValue();startY = new BigDecimal(fontText.getStartY()).intValue();destImage = mergeFontText(destImage, fontText, startX, startY);}return destImage;}private static BufferedImage mergeFontText(BufferedImage bufferedImage, ImageFontText fontText,int left, int top) throws Exception {Graphics2D g = bufferedImage.createGraphics();g.setColor(getColor(fontText.getTextColor()));Font font = new Font(fontText.getTextFont(), Font.BOLD, fontText.getTextSize());g.setFont(font);g.setBackground(Color.white);if (fontText.getStartX() == -1) {//昵称居中设置FontMetrics fmNick = g.getFontMetrics(font);int nickWidth = fmNick.stringWidth(fontText.getText());int nickWidthX = (bufferedImage.getWidth() - nickWidth) / 2;//绘制⽂字g.drawString(new String(fontText.getText().getBytes(), "utf-8"), nickWidthX, top);} else {g.drawString(new String(fontText.getText().getBytes(), "utf-8"), left, top);}g.dispose();return bufferedImage;// AttributedString ats = new AttributedString("我是\n⼩⾬哈哈哈");// ats.addAttribute(TextAttribute.FOREGROUND, f, 0,2 );// AttributedCharacterIterator iter = ats.getIterator();// g.drawString(iter,left,top);}private static Color getColor(String color) {if (StringUtils.isBlank(color) || color.length() < 7) {return null;}try {int r = Integer.parseInt(color.substring(1, 3), 16);int g = Integer.parseInt(color.substring(3, 5), 16);int b = Integer.parseInt(color.substring(5), 16);return new Color(r, g, b);} catch (NumberFormatException nfe) {return null;}}public static InputStream getInputStream(String baseUrl) {if (StringUtils.isBlank(baseUrl)) {return null;}try {InputStream inputStream = new FileInputStream(baseUrl);return inputStream;} catch (IOException e) {e.printStackTrace();}// try {// URL url = new URL(baseUrl);// HttpURLConnection connection = (HttpURLConnection) url.openConnection();// connection.setConnectTimeout(6000);// connection.setReadTimeout(6000);// int code = connection.getResponseCode();// if (HttpURLConnection.HTTP_OK == code) {// return connection.getInputStream();// }// } catch (Exception e) {// e.printStackTrace();// }return null;}/*** 将透明背景设置为⽩⾊*/public static class ThumbnailsImgFilter implements ImageFilter {@Overridepublic BufferedImage apply(BufferedImage img) {int w = img.getWidth();int h = img.getHeight();BufferedImage newImage = new BufferedImage(w, h, BufferedImage.TYPE_INT_RGB);Graphics2D graphic = newImage.createGraphics();graphic.setColor(Color.white);//背景设置为⽩⾊graphic.fillRect(0, 0, w, h);graphic.drawRenderedImage(img, null);graphic.dispose();return newImage;}}}三、⼯具类package com.geidco.dcp.util;import lombok.AllArgsConstructor;import lombok.Data;import lombok.NoArgsConstructor;/*** @author XuPengFei*/@Data@NoArgsConstructor@AllArgsConstructorpublic class ImageFontText {private String text;private Integer textSize = 50;private String textColor = "#ff0000";private String textFont = "宋体";private Integer startX;private Integer startY;}四、⼯具类package com.geidco.dcp.util;import lombok.AllArgsConstructor;import lombok.Data;import lombok.NoArgsConstructor;import java.util.List;/*** @author XuPengFei*/@Data@NoArgsConstructor@AllArgsConstructorpublic class ImageWatermark {/*** 图⽚地址*/private String imageUrl;/*** ⽔印图⽚左上、右下标*/private List<Integer> points;}五、测试接⼝package com.geidco.dcp.controller.meetingApply;import com.geidco.dcp.pojo.meetingApply.MeetingApply;import com.geidco.dcp.pojo.meetingApply.MeetingGuestCardTemplate;import com.geidco.dcp.service.meetingApply.MeetingApplyService;import com.geidco.dcp.service.meetingApply.MeetingGuestCardTemplateService; import com.geidco.dcp.util.*;import mon.annotation.Log;import ng.StringUtils;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.beans.factory.annotation.Value;import org.springframework.util.ResourceUtils;import org.springframework.web.bind.annotation.PostMapping;import org.springframework.web.bind.annotation.RequestBody;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RestController;import javax.imageio.ImageIO;import javax.servlet.http.HttpServletResponse;import java.awt.image.BufferedImage;import java.io.*;import java.text.SimpleDateFormat;import java.util.*;@RestController@RequestMapping("/meetingGuestCard")public class MeetingGuestCardController {@Value("${config.ldir}")String ldir;@Value("${config.wdir}")String wdir;@Autowiredprivate MeetingApplyService meetingApplyService;@Autowiredprivate IdWorker idWorker;private MeetingGuestCardTemplateService meetingGuestCardTemplateService;@PostMapping("/generateGuestCard")@Log("绘制电⼦嘉宾证")public void generateGuestCard(@RequestBody Map<String, String> formData, HttpServletResponse response) {String templateId = formData.get("templateId");String ids = formData.get("meetingApplyId");List<String> meetingApplyIds = Arrays.asList(ids.split(","));List<MeetingApply> meetingApplys = meetingApplyService.getByKeys(meetingApplyIds);String uuid = UUID.randomUUID().toString().replaceAll("-", "");String datePath = new SimpleDateFormat("yyyy/MM/dd").format(new Date()) + "/" + uuid + "/";String zipPath = SysMeetingUtil.getAnnexFilePath() + "generateGuestCard/" + datePath;File file = new File(zipPath);if (!file.exists()) {file.mkdirs();}// 上传的位置String path = System.getProperty("").toLowerCase().indexOf("win") >= 0 ? wdir : ldir;MeetingGuestCardTemplate cardTemplate = meetingGuestCardTemplateService.findById(templateId);// 底图String baseImgUrl = cardTemplate.getBaseMapUrl();try {for (int i = 0; i < meetingApplys.size(); i++) {MeetingApply meetingApply = meetingApplys.get(i);String name = null;String unitName = null;String postName = null;String photoUrl = path;if ("zh".equals(meetingApply.getApplyWay())) {name = meetingApply.getName();unitName = meetingApply.getUnitName();postName = meetingApply.getPositionName();photoUrl = photoUrl + meetingApply.getPhotoUrl();} else {name = meetingApply.getNameEn();unitName = meetingApply.getUnitNameEn();postName = meetingApply.getPositionNameEn();photoUrl = photoUrl + meetingApply.getPhotoUrl();}List<ImageWatermark> images = new ArrayList<>();List<ImageFontText> texts = new ArrayList<>();// 查询模板详情List<MeetingGuestCardTemplate> templates = meetingGuestCardTemplateService.findByTemplateId(templateId);for (MeetingGuestCardTemplate template : templates) {String dict = template.getElementTypeDict();if ("1".equals(dict)) {ImageFontText imageFontText = new ImageFontText(name, StringUtils.isNotBlank(template.getFontSize()) ? Integer.parseInt(template.getFontSize()) : 30,StringUtils.isNotBlank(template.getFontColor()) ? template.getFontColor() : "#333333",StringUtils.isNotBlank(template.getFontType()) ? template.getFontType() : "宋体",template.getStartX(), template.getStartY());texts.add(imageFontText);}if ("2".equals(dict)) {ImageFontText imageFontText = new ImageFontText(unitName, StringUtils.isNotBlank(template.getFontSize()) ? Integer.parseInt(template.getFontSize()) : 30, StringUtils.isNotBlank(template.getFontColor()) ? template.getFontColor() : "#333333",StringUtils.isNotBlank(template.getFontType()) ? template.getFontType() : "宋体",template.getStartX(), template.getStartY());texts.add(imageFontText);}if ("3".equals(dict)) {ImageFontText imageFontText = new ImageFontText(postName, StringUtils.isNotBlank(template.getFontSize()) ? Integer.parseInt(template.getFontSize()) : 30, StringUtils.isNotBlank(template.getFontColor()) ? template.getFontColor() : "#333333",StringUtils.isNotBlank(template.getFontType()) ? template.getFontType() : "宋体",template.getStartX(), template.getStartY());texts.add(imageFontText);}if ("100".equals(dict)) {Integer startX = template.getStartX();Integer startY = template.getStartY();Integer endX = template.getEndX();Integer endY = template.getEndY();List<Integer> imagePoints = Arrays.asList(startX, startY, endX, endY);ImageWatermark image = new ImageWatermark(photoUrl, imagePoints);images.add(image);}}try {BufferedImage bufferedImage = ImageUtil.watermarkImgBase64(baseImgUrl, images, texts);OutputStream os = new FileOutputStream(zipPath + String.valueOf(i + 1) + name + ".jpg");ImageIO.write(bufferedImage, "jpg", os);//更新meetingApply的状态MeetingApply apply = new MeetingApply();apply.setId(meetingApply.getId());apply.setCreateCardDate(new Date());apply.setIsCreateCard("Yes");apply.setCardUrl("generateGuestCard/" + datePath + String.valueOf(i + 1) + name + ".jpg");meetingApplyService.update(apply);} catch (Exception e) {e.printStackTrace();}String zipFile = CompressUtil.zipFile(new File(zipPath), "zip");response.setContentType("APPLICATION/OCTET-STREAM");String fileName = "guestCard" + uuid + ".zip";response.setHeader("Content-Disposition", "attachment; filename=" + fileName);//ZipOutputStream out = new ZipOutputStream(response.getOutputStream());OutputStream out = response.getOutputStream();File ftp = ResourceUtils.getFile(zipFile);InputStream in = new FileInputStream(ftp);// 循环取出流中的数据byte[] b = new byte[100];int len;while ((len = in.read(b)) != -1) {out.write(b, 0, len);}in.close();out.close();} catch (Exception e) {e.printStackTrace();}}}六、页⾯效果到此这篇关于Java实现画图的详细步骤(完整代码)的⽂章就介绍到这了,更多相关Java实现画图内容请搜索以前的⽂章或继续浏览下⾯的相关⽂章希望⼤家以后多多⽀持!。
java类和对象简单的例子代码

Java类和对象简单的例子代码1. 简介在Java编程中,类和对象是非常重要的概念。
类是对象的模板,可以用来创建对象。
对象是类的实例,它可以拥有自己的属性和行为。
通过类和对象的使用,我们可以实现面向对象编程的思想,使我们的程序更加模块化和易于维护。
2. 创建类下面是一个简单的Java类的例子:```javapublic class Car {String brand;String color;int maxSpeed;void displayInfo() {System.out.println("Brand: " + brand);System.out.println("Color: " + color);System.out.println("Max Speed: " + maxSpeed);}}```在这个例子中,我们创建了一个名为Car的类。
该类有三个属性:brand、color和maxSpeed,并且有一个方法displayInfo用来展示车辆的信息。
3. 创建对象要创建Car类的对象,我们可以使用以下代码:```javaCar myCar = new Car();```这行代码创建了一个名为myCar的Car对象。
我们使用关键字new 来实例化Car类,并且将该实例赋值给myCar变量。
4. 访问对象的属性一旦我们创建了Car对象,我们就可以访问它的属性并为其赋值。
例如:```javamyCar.brand = "Toyota";myCar.color = "Red";myCar.maxSpeed = 180;```这些代码展示了如何为myCar对象的属性赋值。
我们可以使用点号操作符来访问对象的属性。
5. 调用对象的方法除了访问对象的属性,我们还可以调用对象的方法。
我们可以使用以下代码来展示myCar对象的信息:```javamyCar.displayInfo();```这行代码会调用myCar对象的displayInfo方法,从而展示该车辆的信息。
贪吃蛇JAVA源代码完整版

游戏贪吃蛇的JAVA源代码一.文档说明a)本代码主要功能为实现贪吃蛇游戏,GUI界面做到尽量简洁和原游戏相仿。
目前版本包含计分,统计最高分,长度自动缩短计时功能。
b)本代码受计算机系大神指点,经许可后发布如下,向Java_online网致敬c)运行时请把.java文件放入default package 即可运行。
二.运行截图a)文件位置b)进入游戏c)游戏进行中三.JAVA代码import java.awt.*;import java.awt.event.*;import static ng.String.format;import java.util.*;import java.util.List;import javax.swing.*;public class Snake extends JPanel implements Runnable { enum Dir {up(0, -1), right(1, 0), down(0, 1), left(-1, 0);Dir(int x, int y) {this.x = x; this.y = y;}final int x, y;}static final Random rand = new Random();static final int WALL = -1;static final int MAX_ENERGY = 1500;volatile boolean gameOver = true;Thread gameThread;int score, hiScore;int nRows = 44;int nCols = 64;Dir dir;int energy;int[][] grid;List<Point> snake, treats;Font smallFont;public Snake() {setPreferredSize(new Dimension(640, 440));setBackground(Color.white);setFont(new Font("SansSerif", Font.BOLD, 48));setFocusable(true);smallFont = getFont().deriveFont(Font.BOLD, 18);initGrid();addMouseListener(new MouseAdapter() {@Overridepublic void mousePressed(MouseEvent e) {if (gameOver) {startNewGame();repaint();}}});addKeyListener(new KeyAdapter() {@Overridepublic void keyPressed(KeyEvent e) {switch (e.getKeyCode()) {case KeyEvent.VK_UP:if (dir != Dir.down)dir = Dir.up;break;case KeyEvent.VK_LEFT:if (dir != Dir.right)dir = Dir.left;break;case KeyEvent.VK_RIGHT:if (dir != Dir.left)dir = Dir.right;break;case KeyEvent.VK_DOWN:if (dir != Dir.up)dir = Dir.down;break;}repaint();}});}void startNewGame() {gameOver = false;stop();initGrid();treats = new LinkedList<>();dir = Dir.left;energy = MAX_ENERGY;if (score > hiScore)hiScore = score;score = 0;snake = new ArrayList<>();for (int x = 0; x < 7; x++)snake.add(new Point(nCols / 2 + x, nRows / 2));doaddTreat();while(treats.isEmpty());(gameThread = new Thread(this)).start();}void stop() {if (gameThread != null) {Thread tmp = gameThread;gameThread = null;tmp.interrupt();}}void initGrid() {grid = new int[nRows][nCols];for (int r = 0; r < nRows; r++) {for (int c = 0; c < nCols; c++) {if (c == 0 || c == nCols - 1 || r == 0 || r == nRows - 1)grid[r][c] = WALL;}}}@Overridepublic void run() {while (Thread.currentThread() == gameThread) {try {Thread.sleep(Math.max(75 - score, 25));} catch (InterruptedException e) {return;}if (energyUsed() || hitsWall() || hitsSnake()) {gameOver();} else {if (eatsTreat()) {score++;energy = MAX_ENERGY;growSnake();}moveSnake();addTreat();}repaint();}}boolean energyUsed() {energy -= 10;return energy <= 0;}boolean hitsWall() {Point head = snake.get(0);int nextCol = head.x + dir.x;int nextRow = head.y + dir.y;return grid[nextRow][nextCol] == WALL;}boolean hitsSnake() {Point head = snake.get(0);int nextCol = head.x + dir.x;int nextRow = head.y + dir.y;for (Point p : snake)if (p.x == nextCol && p.y == nextRow)return true;return false;}boolean eatsTreat() {Point head = snake.get(0);int nextCol = head.x + dir.x;int nextRow = head.y + dir.y;for (Point p : treats)if (p.x == nextCol && p.y == nextRow) {return treats.remove(p);}return false;}void gameOver() {gameOver = true;stop();}void moveSnake() {for (int i = snake.size() - 1; i > 0; i--) {Point p1 = snake.get(i - 1);Point p2 = snake.get(i);p2.x = p1.x;p2.y = p1.y;}Point head = snake.get(0);head.x += dir.x;head.y += dir.y;}void growSnake() {Point tail = snake.get(snake.size() - 1);int x = tail.x + dir.x;int y = tail.y + dir.y;snake.add(new Point(x, y));}void addTreat() {if (treats.size() < 3) {if (rand.nextInt(10) == 0) { // 1 in 10if (rand.nextInt(4) != 0) { // 3 in 4int x, y;while (true) {x = rand.nextInt(nCols);y = rand.nextInt(nRows);if (grid[y][x] != 0)continue;Point p = new Point(x, y);if (snake.contains(p) || treats.contains(p))continue;treats.add(p);break;}} else if (treats.size() > 1)treats.remove(0);}}}void drawGrid(Graphics2D g) {g.setColor(Color.lightGray);for (int r = 0; r < nRows; r++) {for (int c = 0; c < nCols; c++) {if (grid[r][c] == WALL)g.fillRect(c * 10, r * 10, 10, 10);}}}void drawSnake(Graphics2D g) {g.setColor(Color.blue);for (Point p : snake)g.fillRect(p.x * 10, p.y * 10, 10, 10);g.setColor(energy < 500 ? Color.red : Color.orange);Point head = snake.get(0);g.fillRect(head.x * 10, head.y * 10, 10, 10);}void drawTreats(Graphics2D g) {g.setColor(Color.green);for (Point p : treats)g.fillRect(p.x * 10, p.y * 10, 10, 10);}void drawStartScreen(Graphics2D g) {g.setColor(Color.blue);g.setFont(getFont());g.drawString("Snake", 240, 190);g.setColor(Color.orange);g.setFont(smallFont);g.drawString("(click to start)", 250, 240);}void drawScore(Graphics2D g) {int h = getHeight();g.setFont(smallFont);g.setColor(getForeground());String s = format("hiscore %d score %d", hiScore, score);g.drawString(s, 30, h - 30);g.drawString(format("energy %d", energy), getWidth() - 150, h - 30); }@Overridepublic void paintComponent(Graphics gg) {super.paintComponent(gg);Graphics2D g = (Graphics2D) gg;g.setRenderingHint(RenderingHints.KEY_ANTIALIASING,RenderingHints.VALUE_ANTIALIAS_ON);drawGrid(g);if (gameOver) {drawStartScreen(g);} else {drawSnake(g);drawTreats(g);drawScore(g);}}public static void main(String[] args) {SwingUtilities.invokeLater(() -> {JFrame f = new JFrame();f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);f.setTitle("Snake");f.setResizable(false);f.add(new Snake(), BorderLayout.CENTER);f.pack();f.setLocationRelativeTo(null);f.setVisible(true);});}}。
java经典代码(最全面)

Java实现ftp功能import .ftp.*;import .*;import java.awt.*;import java.awt.event.*;import java.applet.*;import java.io.*;public class FtpApplet extends Applet{FtpClient aftp;DataOutputStream outputs ;TelnetInputStream ins;TelnetOutputStream outs;TextArea lsArea;Label LblPrompt;Button BtnConn;Button BtnClose;TextField TxtUID;TextField TxtPWD;TextField TxtHost;int ch;public String a="没有连接主机";String hostname="";public void init () {setBackground(Color.white);setLayout(new GridBagLayout()); GridBagConstraints GBC = new GridBagConstraints( );LblPrompt = new Label("没有连接主机"); LblPrompt.setAlignment(Label.LEFT);BtnConn = new Button("连接");BtnClose = new Button("断开");BtnClose.enable(false);TxtUID = new TextField("",15);TxtPWD = new TextField("",15);TxtPWD.setEchoCharacter(’*’);TxtHost = new TextField("",20);Label LblUID = new Label("User ID:");Label LblPWD = new Label("PWD:");Label LblHost = new Label("Host:");lsArea = new TextArea(30,80); lsArea.setEditable(false);GBC.gridwidth= GridBagConstraints.REMAINDER; GBC.fill = GridBagConstraints.HORIZONTAL; ((GridBagLayout)getLayout()).setConstraints(LblPro mpt,GBC);add(LblPrompt);GBC.gridwidth=1;((GridBagLayout)getLayout()).setConstraints(LblHost ,GBC);add(LblHost);GBC.gridwidth=GridBagConstraints.REMAINDER; ((GridBagLayout)getLayout()).setConstraints(TxtHost ,GBC);add(TxtHost);GBC.gridwidth=1;((GridBagLayout)getLayout()).setConstraints(LblUID ,GBC);add(LblUID);GBC.gridwidth=1;((GridBagLayout)getLayout()).setConstraints(TxtUID ,GBC);add(TxtUID);GBC.gridwidth=1;((GridBagLayout)getLayout()).setConstraints(LblPW D,GBC);add(LblPWD);GBC.gridwidth=1;((GridBagLayout)getLayout()).setConstraints(TxtPW D,GBC);add(TxtPWD);GBC.gridwidth=1;GBC.weightx=2;((GridBagLayout)getLayout()).setConstraints(BtnCon n,GBC);add(BtnConn);GBC.gridwidth=GridBagConstraints.REMAINDER;((GridBagLayout)getLayout()).setConstraints(BtnClos e,GBC);add(BtnClose);GBC.gridwidth=GridBagConstraints.REMAINDER; GBC.fill = GridBagConstraints.HORIZONTAL; ((GridBagLayout)getLayout()).setConstraints(lsArea, GBC);add(lsArea);}public boolean connect(String hostname, String uid,St ring pwd){this.hostname = hostname;LblPrompt.setText("正在连接,请等待.....");try{aftp =new FtpClient(hostname);aftp.login(uid,pwd);aftp.binary();showFileContents();}catch(FtpLoginException e){a="无权限与主机:"+hostname+"连接!"; LblPrompt.setText(a);return false;}catch (IOException e){a="连接主机:"+hostname+"失败!";LblPrompt.setText(a);return false;}catch(SecurityException e){a="无权限与主机:"+hostname+"连接!"; LblPrompt.setText(a);return false;}LblPrompt.setText("连接主机:"+hostname+"成功!"); return true;}public void stop(){try{aftp.closeServer(); }catch(IOException e){}}public void paint(Graphics g){}public boolean action(Event evt,Object obj){if (evt.target == BtnConn){LblPrompt.setText("正在连接,请等待.....");if (connect(TxtHost.getText(),TxtUID.getText(),TxtP WD.getText())){BtnConn.setEnabled(false);BtnClose.setEnabled(true);}return true;}if (evt.target == BtnClose){stop();BtnConn.enable(true);BtnClose.enable(false);LblPrompt.setText("与主机"+hostname+"连接已断开!");return true;}return super.action(evt,obj);}public boolean sendFile(String filepathname){ boolean result=true;if (aftp != null){LblPrompt.setText("正在粘贴文件,请耐心等待....");String contentperline;try{a="粘贴成功!";String fg =new String("\\");int index = stIndexOf(fg);String filename = filepathname.substring(index+1); File localFile ;localFile = new File(filepathname) ; RandomAccessFile sendFile = new RandomAccessFil e(filepathname,"r");//sendFile.seek(0);outs = aftp.put(filename);outputs = new DataOutputStream(outs);while (sendFile.getFilePointer() < sendFile.length() ) {ch = sendFile.read();outputs.write(ch);}outs.close();sendFile.close();}catch(IOException e){a = "粘贴失败!";result = false ;}LblPrompt.setText(a);showFileContents();}else{result = false;}return result;}public void showFileContents(){StringBuffer buf = new StringBuffer();lsArea.setText("");try{ins= aftp.list();while ((ch=ins.read())>=0){buf.append((char)ch);}lsArea.appendText(buf.toString());ins.close();} catch(IOException e){}}public static void main(String args[]){Frame f = new Frame("FTP Client");f.addWindowListener(new WindowAdapter() {public void windowClosing(WindowEvent e ){System.exit(0);}});FtpApplet ftp = new FtpApplet();ftp.init();ftp.start();f.add(ftp);f.pack();f.setVisible(true);}}Java URL编程import java.io.*;import .*;////// GetHost.java////public class GetHost{public static void main (String arg[]){if (arg.length>=1){InetAddress[] Inet;int i=1;try{for (i=1;i<=arg.length;i++){Inet = InetAddress.getAllByName(arg[i-1]);for (int j=1;j<=Inet.length;j++){System.out.print(Inet[j-1].toString());System.out.print("\n");}}}catch(UnknownHostException e){System.out.print("Unknown HostName!"+arg[i-1]); }}else{System.out.print("Usage java/jview GetIp <hostname >");}}}</pre></p><p><pre><font color=red>Example 2</font><a href="GetHTML.java">download now</a>//GetHTML.java/*** This is a program which can read information from a web server.* @version 1.0 2000/01/01* @author jdeveloper**/import .*;import java.io.*;public class GetHTML {public static void main(String args[]){if (args.length < 1){System.out.println("USAGE: java GetHTML httpaddr ess");System.exit(1);}String sURLAddress = new String(args[0]);URL url = null;try{url = new URL(sURLAddress);}catch(MalformedURLException e){System.err.println(e.toString());System.exit(1);}try{ InputStream ins = url.openStream();BufferedReader breader = new BufferedReader(new InputStreamReader(ins));String info = breader.readLine();while(info != null){System.out.println(info);info = breader.readLine();}}catch(IOException e){System.err.println(e.toString());System.exit(1);}}}Java RMI编程<b>Step 1: Implements the interface of Remote Serv er as SimpleCounterServer.java</b>public interface SimpleCounterServer extends java. rmi.Remote{public int getCount() throws java.rmi.RemoteExcep tion;}Compile it with javac SimpleCounterServer.java<b>Step 2: Produce the implement file SimpleCou nterServerImpl.java as</b>import java.rmi.*;import java.rmi.server.UnicastRemoteObject;////// SimpleCounterServerImpl////public class SimpleCounterServerImplextends UnicastRemoteObjectimplements SimpleCounterServer{private int iCount;public SimpleCounterServerImpl() throws java.rmi.RemoteException{iCount = 0;}public int getCount() throws RemoteException{return ++iCount;}public static void main(String args[]){System.setSecurityManager(new RMISecurityM anager());try{SimpleCounterServerImpl server = new Simpl eCounterServerImpl();System.out.println("SimpleCounterServer crea ted");Naming.rebind("SimpleCounterServer",server); System.out.println("SimpleCounterServer regi stered");}catch(RemoteException x){x.printStackTrace();}catch(Exception x){x.printStackTrace();}}}Complile it with javac SimpleCounterServerImpl.java<b>Step 3: Produce skeleton and stub file with rmic S impleCounterServerImpl</b>You will get two class files:1.SimpleCounterServerImpl_Stub.class2.SimpleCounterServerImpl_Skel.class<b>Step 4: start rmiregistry </b><b>Step 5: java SimpleCounterServerImpl</b> <b>Step 6: Implements the Client Applet to invoke th e Remote method</b>File :SimpleCounterApplet.java asimport java.applet.Applet;import java.rmi.*;import java.awt.*;////// SimpleCounterApplet////public class SimpleCounterApplet extends Applet {String message="";String message1 = "";public void init(){setBackground(Color.white);try{SimpleCounterServer sever = (SimpleCounter Server)Naming.lookup("//"+getCodeBase().getHost ()+"/SimpleCounterServer");message1 = "//"+getCodeBase().getHost()+"/S impleCounterServer";message = String.valueOf(sever.getCount()); }catch(Exception x){x.printStackTrace();message = x.toString();}}public void paint(Graphics g){g.drawString("Number is "+message,10,10);g.drawString("Number is "+message1,10,30);}}<b>step 7 create an Html File rmi.htm : </b>< html>< body>< applet code="SimpleCounterApplet.class" width="5 00" height="150">< /applet>< /body>< /html>Java CORBA入门Below is a simple example of a CORBA program download the source file<b>1. produce a idl file like this</b>hello.idlmodule HelloApp {interface Hello {string sayHello();};};<b>2. produce stub and skeleton files through idltojav a.exe</b>idltojava hello.idlidltojava is now named as idlj.exe and is included in the JDK.<b>3. write a server program like this </b>// HelloServer.javaimport HelloApp.*;import org.omg.CosNaming.*;import org.omg.CosNaming.NamingContextPackage. *;import org.omg.CORBA.*;import java.io.*;class HelloServant extends _HelloImplBase{public String sayHello(){return "\nHello world !!\n";}} public class HelloServer {public static void main(String args[]){try{// create and initialize the ORBORB orb = ORB.init(args, null);// create servant and register it with the ORBHelloServant helloRef = new HelloServant();orb.connect(helloRef);// get the root naming contextorg.omg.CORBA.Object objRef =orb.resolve_initial_references("NameService");NamingContext ncRef = NamingContextHelper.nar row(objRef);// bind the Object Reference in NamingNameComponent nc = new NameComponent("Hell o", "");NameComponent path[] = {nc};ncRef.rebind(path, helloRef);// wait for invocations from clientsng.Object sync = new ng.Object(); synchronized (sync) {sync.wait();}} catch (Exception e) {System.err.println("ERROR: " + e);e.printStackTrace(System.out);}}}<b>4. write a client program like this</b>// HelloClient.javaimport HelloApp.*;import org.omg.CosNaming.*;import org.omg.CORBA.*;public class HelloClient{public static void main(String args[]){try{// create and initialize the ORBORB orb = ORB.init(args, null);// get the root naming contextorg.omg.CORBA.Object objRef =orb.resolve_initial_references("NameService");NamingContext ncRef = NamingContextHelpe r.narrow(objRef);// testSystem.out.println("OK..");// resolve the Object Reference in NamingNameComponent nc = new NameComponent( "Hello", "");NameComponent path[] = {nc};Hello helloRef = HelloHelper.narrow(ncRef.re solve(path));// call the Hello server object and print results//String oldhello = stMessage();//System.out.println(oldhello);String Hello = helloRef.sayHello();System.out.println(Hello);} catch (Exception e) {System.out.println("ERROR : " + e) ;e.printStackTrace(System.out);}}}<b>5. complie these files</b>javac *.java HelloApp/*.java<b>6. run the application</b>a. first you’ve to run the Name Service prior to the others likethis c:\>tnameservb. run server c:\>java HelloServerc. run clientc:\>java HelloClient利用RamdonAccessFile来实现文件的追加RamdonAccessFile 是个很好用的类,功能十分强大,可以利用它的length()和seek()方法来轻松实现文件的追加,相信我下面这个例子是很容易看懂的,先写入十行,用length()读出长度(以byte为单位),在用seek()移动到文件末尾,继续添加,最后显示记录。
java俄罗斯方块完整代码

public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if(key==KeyEvent.VK_Q) {//如果按Q
System.exit(0);//结束Java进程
}
if(gameOver) {//如果游戏结束
T = ImageIO.read(Tetris.class.getResource("tT.png"));
I = ImageIO.read(Tetris.class.getResource("II.png"));
S = ImageIO.read(Tetris.class.getResource("SS.png"));
}else {
g.drawImage(cell.getImage(),x-1,y-1,null);//有格子贴图
}
}
}
}
//添加启动方法
public void action() {
wall = new Cell[ROWS][COLS];
//启动
startAction();
//处理键盘按下事件
KeyAdapter l = new KeyAdapter(){
if(gameOver) {
g.drawImage(overImage,0,0,null);
}
}
//画分数
private void paintScore(Graphics g) {
int x = 390;//基线位置
int y = 190;//基线位置
g.setColor(new Color(FONT_COLOR));//颜色
iec608705104协议解析java代码样例
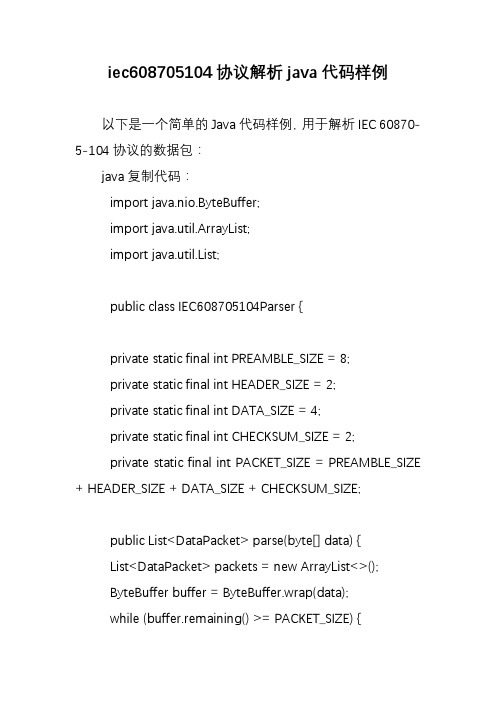
iec608705104协议解析java代码样例以下是一个简单的Java代码样例,用于解析IEC 60870-5-104协议的数据包:java复制代码:import java.nio.ByteBuffer;import java.util.ArrayList;import java.util.List;public class IEC608705104Parser {private static final int PREAMBLE_SIZE = 8;private static final int HEADER_SIZE = 2;private static final int DATA_SIZE = 4;private static final int CHECKSUM_SIZE = 2;private static final int PACKET_SIZE = PREAMBLE_SIZE + HEADER_SIZE + DATA_SIZE + CHECKSUM_SIZE;public List<DataPacket> parse(byte[] data) {List<DataPacket> packets = new ArrayList<>();ByteBuffer buffer = ByteBuffer.wrap(data);while (buffer.remaining() >= PACKET_SIZE) {byte[] preamble = new byte[PREAMBLE_SIZE];buffer.get(preamble);if (!isPreambleValid(preamble)) {break;}short header = buffer.getShort();byte[] dataBytes = new byte[DATA_SIZE];buffer.get(dataBytes);short checksum = buffer.getShort();DataPacket packet = new DataPacket(preamble, header, dataBytes, checksum);packets.add(packet);}return packets;}private boolean isPreambleValid(byte[] preamble) {for (byte b : preamble) {if (b != 0x80) {return false;}}return true;}}该代码定义了一个IEC608705104Parser类,该类包含一个parse()方法,该方法接收一个字节数组作为参数,并返回一个包含解析出的数据包的列表。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Chapter01——初识Java1.单词公共的、公有的:public静态的:static主要的:main打印:print2.编写类名为HelloWorld的的程序框架public class HelloWorld{}3.编写main()方法的框架public static void main(String[] args){}4.编写代码输出HelloWorld后并换行System.out.println(“HelloWorld”);Chapter02——变量、数据类型和运算符1.单词字符:character布尔:boolean扫描器:scanner成绩:score名字:name2.写出本章节中学习过的五种数据类型int、double、char、String、boolean3.创建扫描器对象,并接收用户输入的年龄Scanner input=new Scanner(System.in);System.out.print(“请输入年龄:”);int age=input.nextInt();4.目前有整型变量custNo,请分解出它的个位、十位、百位和千位int gewei=custNo%10;int shiwei=custNo/10%10;int baiwei=custNo/100%10;int qianwei=custNo/1000;Chapter03——选择结构(一)1.单词如果:if继续:continue随机:random数学:math打断:break2.如果张浩的Java成绩大于98分,那么老师奖励他一个MP4;否则老师罚他编码,请补全以下代码:int score=91;if(score>98){System.out.println(“奖励一个MP4”);}else{System.out.println(“惩罚进行编码”);}3.某人想买车,买什么车决定于此人在银行有多少存款。
如果此人的存款超过500万,则买凯迪拉克否则,如果此人的存款超过100万,则买帕萨特否则,如果此人的存款超过50万,则买伊兰特否则。
如果此人的存款超过10万,则买奥拓否则此人买捷安特,请补全以下代码:int money=52; //我的存款,单位:万元if(money>=500){System.out.println(“买凯迪拉克”);}else if(money>=100){System.out.println(“买帕萨特”);}else if(money>=50){System.out.println(“买伊兰特”);}else if(money>=10){System.out.println(“买奥拓”);}else{System.out.println(“买捷安特”);}4.学校举行运动会,百米赛跑成绩在10秒以内的学生有资格进决赛,根据性别分为男子组和女子组,不在10秒以内的淘汰,补全以下代码:Scanner input=new Scanner(System.in);System.out.print(“请输入比赛成绩(s):”);double score=input.nextDouble();System.out.print(“请输入性别:”);String gender=input.next();if(score<=10){if(gender.equals(“男”)){System.out.println(“进入男子组决赛!”);}else if(gender.equals(“女”)){System.out.println(“进入女子组决赛!”);}}else{System.out.println(“淘汰!”);}Chapter04——选择结构(二)1.单词退出:exit默认的:default事例、情况:case2.使用switch实现,韩嫣参加计算机编程大赛。
如果获得第1名,将参加麻省理工大学组织的1个月夏令营;如果获得第2名,将奖励惠普笔记本电脑一部;如果获得第3名,将奖励移动硬盘一个;否则,没有任何奖励。
int mingCi=1;//名次switch(mingCi){case 1:System.out.println(“参加麻省理工大学组织的1个月夏令营”);break;case 2:System.out.println(“奖励惠普笔记本电脑一部”);break;case 3:System.out.println(“奖励移动硬盘一个”);break;default:System.out.println(“没有任何奖励”);break;}3.判断键盘输入的是否是整数,如果是整数,定义整数变量接收输入数字并输出;如果不是数字,定义字符串变量接收,并输出内容。
Scanner input = new Scanner(System.in);if(input.hasNextInt()==true){System.out.println(“输入的是整数”);int i=input.nextInt();System.out.println(“输入的整数是:”+i);}else{System.out.println(“输入的不是整数”);String s=input.next();System.out.println(“输入的内容为:”+s);}4.使用switch结构实现,从键盘输入月份,控制台输出该月份有多少天。
public static void main(String[] args) {Scanner input = new Scanner(System.in);System.out.print("请输入年份:");int year = input.nextInt();System.out.print("请输入月份:");int month = input.nextInt();switch (month) {case 1:case 3:case 5:case 7:case 8:case 10:case 12:System.out.println("该月有31天");break;case 4:case 6:case 9:case 11:System.out.println("该月有30天");break;case 2:if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0) { System.out.println("该月有29天");} else {System.out.println("该月有28天");}break;default:System.out.println("输入的月份有误!");break;}}Chapter05——循环结构(一)1.单词调试:debug相等、等于:equals回答:answer数量:amount折扣:discount总共的:total付款、支付:payment2.用while循环输出100遍“好好学习,天天向上”。
int i=1;while(i<=100){System.out.println(“第”+i+”遍好好学习,天天向上!”);i++;}3.用do…while循环输出100遍“好好学习,天天向上”。
int i=1;do{System.out.println(“第”+i+”遍好好学习,天天向上!”);i++;}while(i<=100);4.使用while实现,键盘输入是否继续(y/n),如果输入y,继续循环,如果输入n,退出循环Scanner input = new Scanner(System.in);String answer=“y”;while(!answer.equals(“n”)){System.out.println(“是否继续?(y/n)”);answer=input.next();}System.out.println(“程序结束!”);Chapter06——循环结构(二)1.单词平均:average得分:score总数:sum比率、比例:rate2.使用for循环输出100遍“好好学习,天天向上”for(int i=1;i<=100;i++){System.out.println(“第”+i+“遍好好学习,天天向上!”);}3.使用while和break结合实现,键盘输入是否继续(y/n),如果输入y,继续循环,如果输入n,退出循环Scanner input = new Scanner(System.in);while(true){System.out.println(“是否继续?(y/n)”);String answer=input.next();if(answer.equals(“n”)){break;}}System.out.println(“程序结束!”);4.使用for循环和continue结合实现,从键盘输入10个学生的成绩,统计90分及以上的学生人数。
Scanner input=new Scanner(System.in);int count=0;for(int i=1;i<=10;i++){System.out.println(“请输入第”+i+“个学生的成绩:”);int score=input.nextInt();if(score<90){continue;}count++;}System.out.println(“90分及以上的学生人数为:”+count);Chapter08——数组1.单词数组:array长度:length排序:sort最大的:maximum最小的:minimum2.定义一个长度为5的字符串数组,并同时给数组元素赋值为“张三”、“李四”、“王五”、“赵六”、“田七”,使用for循环,循环输出数组元素内容。
String[] names={“张三”,“李四”,“王五”,“赵六”,“田七”};for(int i=0;i<names.length;i++){System.out.println(names[i]);}3.定义一个长度为5的整型数组,并使用for循环从键盘给5个元素赋值。
Scanner input=new Scanner(System.in);int[] nums=new int[5];for(int i=0;i<nums.length;i++){System.out.print(“第”+(i+1)+“个数:”);nums[i]=input.nextInt();}4.定义一个长度为5的整型数组,并同时赋值为4、6、3、9、2,使用for循环求出数组中最大的值。