缓存redis操作
Redis缓存解决访问频繁的数据计算问题

Redis缓存解决访问频繁的数据计算问题随着互联网的快速发展,大数据时代已经来临。
许多应用程序面临着访问频繁的数据计算问题。
在这种情况下,使用缓存技术可以有效提升系统性能。
Redis作为一种高性能的键值存储系统,被广泛应用于解决访问频繁的数据计算问题。
本文将重点讨论如何使用Redis缓存来解决这类问题。
一、Redis缓存的基本原理Redis是一种内存数据库,它将数据存储在内存中,以实现高速的读写性能。
常见的应用场景之一就是将热点数据缓存到Redis中,以减轻数据库的访问压力。
当需要访问数据时,应用程序首先尝试从Redis缓存中获取,如果缓存中有对应的数据,则直接返回给应用程序;如果缓存中没有对应的数据,则从后端数据库中获取,同时将数据存储到Redis缓存中,以供以后使用。
二、Redis缓存的优势1. 高速读写:Redis将数据存储在内存中,避免了磁盘IO的开销,因此具有极快的读写性能。
这对于需要频繁读写的数据计算问题非常重要。
2. 数据持久化:Redis支持数据持久化,可以在服务器重启后将数据恢复到内存中。
这样即便服务器发生故障,也能保证数据的完整性。
3. 支持多种数据结构:Redis支持多种数据结构,如字符串、哈希、列表、集合、有序集合等,使得开发人员可以根据不同的业务场景选择合适的数据结构。
三、Redis缓存的使用方法1. 确定缓存键名:在使用Redis缓存时,需要为每个缓存对象设置唯一的键名。
一般可使用业务相关的ID作为键名,如用户ID、商品ID等。
2. 设置缓存超时时间:在将数据存储到Redis缓存中时,需要设置一个适当的超时时间。
超时时间的设置应根据数据变化的频率和对数据的实时性要求来进行调整。
3. 编写缓存逻辑:在访问数据之前,首先查询Redis缓存,如果缓存中有对应的数据,则直接使用缓存中的数据;如果缓存中没有对应的数据,则从数据库中获取数据,并将数据存储到Redis缓存中。
四、Redis缓存的适用场景1. 热点数据缓存:将访问频繁的热点数据存储到Redis缓存中,可以大大提升系统的性能。
redis缓存实现原理

redis缓存实现原理Redis缓存实现原理是基于内存的键值存储系统,具有快速读写操作和持久化功能。
以下是Redis缓存的实现原理:1. 内存存储:Redis将数据存储在内存中,以实现高速读写操作。
内存存储方式使得Redis能够快速地响应请求,并处理大量并发访问。
2. 键值对存储:Redis以键值对的形式存储数据。
每个键都与一个特定的值相关联。
这种存储模型使得Redis能够将数据分组并按需访问。
3. 数据类型:Redis支持多种数据类型,包括字符串、哈希表、列表、集合和有序集合。
不同的数据类型适用于不同的应用场景,提供了灵活性和高效性。
4. 持久化:Redis提供了两种持久化方式,即RDB(Redis数据库)和AOF(Append Only File)。
RDB在指定时间间隔内将内存中的数据快照保存到硬盘上,而AOF则记录了所有对数据的修改操作,通过重放这些操作来恢复数据。
5. 缓存淘汰策略:Redis通过设置缓存的最大内存容量,当达到容量限制时会触发缓存淘汰策略。
常见的淘汰策略包括LRU(最近最少使用)、LFU(最不常使用)和随机替换等。
6. 分布式部署:Redis支持分布式部署,通过在多个节点之间共享数据进行负载均衡和高可用性。
分布式Redis使用一致性哈希算法来确定数据在哪个节点中存储,同时使用复制和故障转移机制来提高可用性。
总之,Redis缓存实现原理基于内存存储、键值对存储和多种数据类型支持。
它通过持久化、缓存淘汰策略和分布式部署等机制实现高速读写、数据可持久化和可扩展性。
Redis缓存的管道技术与批量操作

Redis缓存的管道技术与批量操作Redis是一种高性能的内存数据库,常用于缓存、存储会话信息和提供实时数据分析。
为了进一步提高Redis的性能,管道技术和批量操作成为了重要的优化手段。
一、什么是Redis缓存的管道技术Redis缓存的管道技术是指客户端将多个命令一次性发送给Redis服务器,由服务器按顺序执行这些命令,并将结果一次性返回给客户端。
这种方式减少了客户端与服务器之间的网络开销和延迟,从而提高了Redis的性能。
二、Redis缓存的管道技术的使用场景1. 批量写入/更新操作:当需要写入/更新多个键值对时,使用管道技术可以减少客户端与服务器之间的通信次数,提高写入/更新的效率。
2. 批量读取操作:当需要读取多个键值对时,使用管道技术可以将多个读取命令一次性发送给服务器,并一次性获取结果,减少网络开销和延迟。
三、Redis缓存的管道技术的优势1. 减少网络开销和延迟:使用管道技术可以将多个命令一次性发送给服务器,减少了网络往返的时间,提高了整体的响应速度。
2. 提高并发性能:由于管道技术可以一次性执行多个命令,因此在高并发的场景下,可以有效降低服务器的负载,提高系统的并发能力。
3. 简化客户端代码:使用管道技术可以将多个命令封装在一个操作中,简化了客户端操作的复杂度,提高了开发效率。
四、Redis缓存的管道技术的应用案例以下是一个批量写入操作的示例代码:```import redis# 连接Redis服务器r = redis.Redis(host='localhost', port=6379)# 创建管道对象pipe = r.pipeline()# 批量写入操作pipe.set('key1', 'value1')pipe.set('key2', 'value2')pipe.set('key3', 'value3')# 执行管道命令pipe.execute()# 关闭管道pipe.close()```以上代码使用了Redis的Python客户端库`redis`,首先创建了一个连接对象`r`,然后创建了一个管道对象`pipe`,在管道对象中依次添加了要执行的写入命令,最后使用`execute()`方法执行管道命令。
rediscache方法

rediscache方法
RedisCache方法是指Redis缓存中的操作方法。
Redis是一个
基于内存的数据存储系统,它提供了一些用于缓存数据的功能,可以将常用的数据存储在内存中,以提高数据读写的速度。
RedisCache方法包括以下几种常见的操作:
1. get(key): 根据给定的key获取缓存中对应的value。
2. set(key, value): 将给定的key和value存储到缓存中,如果
key已经存在,则更新value。
3. delete(key): 删除缓存中指定的key及其对应的value。
4. exists(key): 判断给定的key是否存在于缓存中。
5. expire(key, seconds): 设置给定的key在指定的秒数后过期,
即在缓存中自动删除。
6. flushall(): 清空缓存中的所有数据。
这些方法可以用于对Redis缓存进行常规的读取、写入、删除
等操作。
在实际开发中,需要根据具体的业务需求来选择合适的RedisCache方法来提高数据读写的效率和性能。
同时,需
要注意合理设置缓存的过期时间,以避免缓存数据过期导致读取错误或存储无效数据。
redis存储数据的方法
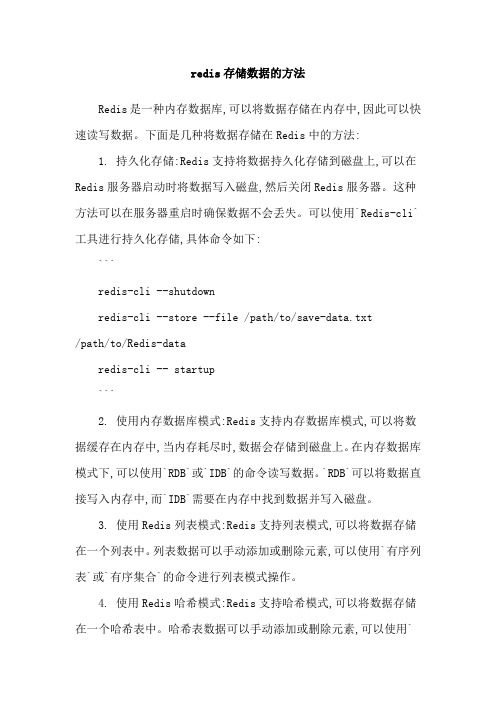
redis存储数据的方法
Redis是一种内存数据库,可以将数据存储在内存中,因此可以快速读写数据。
下面是几种将数据存储在Redis中的方法:
1. 持久化存储:Redis支持将数据持久化存储到磁盘上,可以在Redis服务器启动时将数据写入磁盘,然后关闭Redis服务器。
这种方法可以在服务器重启时确保数据不会丢失。
可以使用`Redis-cli`工具进行持久化存储,具体命令如下:
```
redis-cli --shutdown
redis-cli --store --file /path/to/save-data.txt
/path/to/Redis-data
redis-cli -- startup
```
2. 使用内存数据库模式:Redis支持内存数据库模式,可以将数据缓存在内存中,当内存耗尽时,数据会存储到磁盘上。
在内存数据库模式下,可以使用`RDB`或`IDB`的命令读写数据。
`RDB`可以将数据直接写入内存中,而`IDB`需要在内存中找到数据并写入磁盘。
3. 使用Redis列表模式:Redis支持列表模式,可以将数据存储在一个列表中。
列表数据可以手动添加或删除元素,可以使用`有序列表`或`有序集合`的命令进行列表模式操作。
4. 使用Redis哈希模式:Redis支持哈希模式,可以将数据存储在一个哈希表中。
哈希表数据可以手动添加或删除元素,可以使用`
hash`或`set`命令进行哈希模式操作。
无论使用哪种方法,都需要将数据结构设计好,以便更好地管理
和访问数据。
同时,需要注意Redis的性能,合理分配内存和磁盘资源,避免超时而挂起系统等问题。
redis cachemanager 用法

redis cachemanager 用法Redis CacheManager 是一个强大的缓存管理工具,可以用于提升应用程序的性能和响应速度。
本文将介绍 Redis CacheManager 的基本用法和一些常见的应用场景。
1. Redis CacheManager 简介Redis CacheManager 是基于 Redis 的一个高性能缓存管理器。
Redis 是一种内存数据库,具有快速读写、持久化存储和数据结构丰富等特点,非常适合用于缓存场景。
CacheManager 则是对缓存的统一管理工具,可以简化缓存的配置和管理操作。
2. Redis CacheManager 的配置要使用 Redis CacheManager,首先需在项目中引入相应的依赖库,并进行必要的配置。
以下是一个基本的配置示例:```java@Configuration@EnableCachingpublic class RedisCacheConfig extends CachingConfigurerSupport { @Beanpublic RedisCacheManager cacheManager(RedisConnectionFactory redisConnectionFactory) {RedisCacheConfiguration cacheConfiguration = RedisCacheConfiguration.defaultCacheConfig();cacheConfiguration =cacheConfiguration.serializeKeysWith(SerializationPair.fromSerializer(new StringRedisSerializer()));cacheConfiguration =cacheConfiguration.serializeValuesWith(SerializationPair.fromSerializer(ne w GenericJackson2JsonRedisSerializer()));RedisCacheManager.RedisCacheManagerBuilder builder = RedisCacheManager.RedisCacheManagerBuilder.fromConnectionFactory(r edisConnectionFactory);builder.cacheDefaults(cacheConfiguration);return builder.build();}@Beanpublic RedisTemplate<String, Object>redisTemplate(RedisConnectionFactory redisConnectionFactory) {RedisTemplate<String, Object> redisTemplate = new RedisTemplate<>();redisTemplate.setConnectionFactory(redisConnectionFactory);redisTemplate.setKeySerializer(new StringRedisSerializer());redisTemplate.setValueSerializer(newGenericJackson2JsonRedisSerializer());redisTemplate.afterPropertiesSet();return redisTemplate;}}```在上述配置中,我们首先使用 `@EnableCaching` 注解启用缓存,并通过 `@Configuration` 注解将该类声明为配置类。
javaredis缓存用法
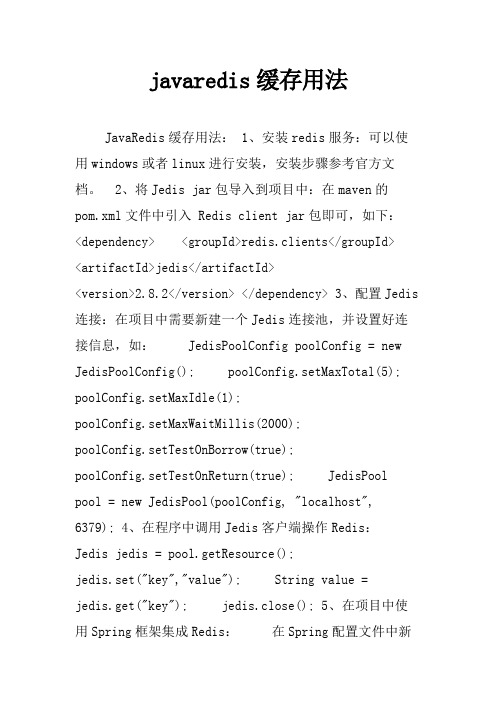
javaredis缓存用法JavaRedis缓存用法: 1、安装redis服务:可以使用windows或者linux进行安装,安装步骤参考官方文档。
2、将Jedis jar包导入到项目中:在maven的pom.xml文件中引入 Redis client jar包即可,如下:<dependency> <groupId>redis.clients</groupId> <artifactId>jedis</artifactId><version>2.8.2</version> </dependency> 3、配置Jedis连接:在项目中需要新建一个Jedis连接池,并设置好连接信息,如: JedisPoolConfig poolConfig = new JedisPoolConfig(); poolConfig.setMaxTotal(5); poolConfig.setMaxIdle(1);poolConfig.setMaxWaitMillis(2000);poolConfig.setTestOnBorrow(true);poolConfig.setTestOnReturn(true); JedisPoolpool = new JedisPool(poolConfig, "localhost", 6379); 4、在程序中调用Jedis客户端操作Redis:Jedis jedis = pool.getResource();jedis.set("key","value"); String value =jedis.get("key"); jedis.close(); 5、在项目中使用Spring框架集成Redis:在Spring配置文件中新增Redis数据源,如: <bean id="jedisPool"class="redis.clients.jedis.JedisPool"><constructor-arg index="0" ref="jedisPoolConfig"/> <constructor-arg index="1" value="localhost"/><constructor-arg index="2" value="6379"/></bean> 然后可以使用@Autowired注解来注入JedisPool,来获取 Jedis 连接,然后进行Redis操作。
rediscache 用法

rediscache 用法Redis 是一个高性能的内存数据库,提供了丰富的数据结构和功能。
其中的Redis Cache 是Redis 的一个重要功能,可以用于缓存数据,提升系统性能和响应速度。
下面我将详细介绍Redis Cache 的用法。
一、Redis Cache 的基本概念1. 缓存:将计算过的结果存储在高速存储设备中,以便后续使用,减少计算和查询的时间消耗。
2. 缓存击穿:当缓存中某个键对应的数据过期或者不存在时,大量的并发请求会直接冲击到数据库上,导致数据库压力骤增,甚至崩溃。
3. 缓存雪崩:缓存中大量的键同时过期,导致大量的并发请求都直接冲击到数据库上,导致数据库压力骤增,甚至崩溃。
4. 缓存一致性:指缓存中的数据与数据库中的数据保持一致,保证数据的正确性。
5. 缓存更新策略:指缓存中的数据何时进行更新,通过设置合适的更新策略,可以减少数据库压力,提高系统性能。
二、Redis Cache 的优势1. 高性能:Redis Cache 是基于内存的缓存技术,速度非常快,能够提供高并发的读写能力。
2. 丰富的数据结构:Redis 提供了多种数据结构,如字符串、哈希表、列表、集合、有序集合等,能够满足不同场景下的需求。
3. 持久化支持:Redis 提供了两种持久化方式,即快照和AOF日志,可以将缓存数据持久化到磁盘,避免数据丢失。
4. 高可用性:Redis 支持主从复制和哨兵机制,可以实现缓存的高可用性和故障恢复。
5. 多种应用场景:Redis 除了作为缓存使用外,还可以用作消息队列、分布式锁等,具有广泛的应用场景。
三、Redis Cache 的使用1. 安装和配置Redis:首先需要安装Redis,并进行相关的配置,如绑定IP地址、端口号、密码等。
可以通过官方网站下载安装包,然后按照文档进行配置。
2. 连接Redis:通过Redis 客户端连接Redis 服务器,在代码中指定Redis 的IP地址、端口号和密码,即可建立连接。
java中redis的redistemplate缓存用法

java中redis的redistemplate缓存用法Redis是一种高效、可扩展的内存数据库,广泛应用于缓存、消息队列和会话存储等方面。
在Java开发中,可以使用RedisTemplate来方便地操作Redis缓存。
本文将介绍Java中RedisTemplate的缓存用法。
一、RedisTemplate简介RedisTemplate是Spring Data Redis提供的一个用于操作Redis的模板类。
它封装了与Redis的连接、数据存取等操作,简化了开发人员对Redis的使用。
RedisTemplate内部使用了Jedis或Lettuce等Redis客户端来实现与Redis服务器的通信。
二、使用RedisTemplate进行缓存操作1. 引入依赖在使用RedisTemplate之前,首先需要在项目的pom.xml文件中引入相应的依赖:```<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-data-redis</artifactId></dependency>```2. 配置Redis连接信息在项目的配置文件中配置Redis的连接信息,包括主机地址、端口号、密码等。
示例配置如下:```spring.redis.host=127.0.0.1spring.redis.port=6379spring.redis.password=123456```3. 创建RedisTemplate实例在Java代码中,通过注入RedisConnectionFactory来创建RedisTemplate实例。
示例代码如下:```java@Autowiredprivate RedisTemplate<String, Object> redisTemplate;```4. 缓存数据使用RedisTemplate可以方便地进行数据缓存。
Redis缓存的使用场景

Redis缓存的使用场景Redis是一种开源、内存数据结构存储系统,支持多种数据结构,如字符串、哈希表、列表、集合、有序集合等。
由于其高性能、低延迟和可扩展性等优点,Redis在互联网应用中被广泛应用于缓存、队列、计数器、实时消息发布订阅等场景。
本文将介绍Redis缓存的使用场景。
一、页面缓存在Web应用中,页面的渲染通常是一个耗时的操作,通过使用Redis缓存可以有效减轻数据库的负载和页面的加载时间。
当用户请求一个页面时,首先检查Redis中是否已缓存了该页面,如果存在,直接返回缓存结果;如果不存在,从数据库中读取数据,并将结果保存到Redis中。
这样,下次请求同一页面时,直接从Redis中获取数据,避免了重复的数据库访问和页面渲染操作。
二、数据缓存对于频繁读取的数据,可以使用Redis缓存来提高系统的读取性能。
例如,用户的个人信息、商品的价格信息等,可以将其缓存到Redis中,减少数据库查询的次数。
当需要获取这些数据时,首先检查Redis中是否有缓存,如果不为空,则直接返回缓存结果;如果为空,则从数据库中查询,并将查询结果保存到Redis中。
三、会话缓存在Web应用中,为了保持用户的登录状态和跨页面的数据传递,通常会使用会话(Session)来存储用户相关的信息。
通过使用Redis作为会话缓存,可以有效地管理和存储会话数据。
当用户登录时,将用户的会话信息存储到Redis中,并生成一个唯一的会话ID返回给客户端。
客户端每次请求时,携带该会话ID进行身份认证。
而且Redis还提供了过期时间设置,可以自动删除过期的会话信息,提高系统的安全性和效率。
四、消息队列在分布式系统中,消息队列通常被用于解耦不同的模块,实现异步处理和削峰填谷的效果。
Redis提供了实时消息发布订阅功能,可以作为消息队列来使用。
通过将数据发布到Redis的特定频道或主题,其他系统组件可以通过订阅该频道或主题来接收消息,并进行相应的处理。
(Redis缓存)Redis哈希表与HSET HGET命令

(Redis缓存)Redis哈希表与HSET HGET命令Redis缓存技术的应用越来越广泛,其中Redis哈希表与HSET、HGET命令是常用的功能之一。
本文将详细介绍Redis哈希表以及HSET、HGET命令的使用。
一、Redis哈希表概述Redis哈希表是一种用来存储键值对的数据结构,它类似于字典或者Map。
在Redis中,每个哈希表可以存储多个字段和对应的值,字段和值之间是一一对应的关系。
二、Redis哈希表的创建与添加字段使用Redis的HSET命令可以向哈希表中添加字段和对应的值。
具体命令格式如下:HSET key field value其中,key表示哈希表的键名,field表示字段名,value表示字段对应的值。
例如,我们创建一个名为student的哈希表,并向其中添加字段名为name,值为"张三"的字段。
命令如下:HSET student name "张三"三、Redis哈希表的获取字段值使用Redis的HGET命令可以获取哈希表中指定字段的值。
具体命令格式如下:HGET key field其中,key表示哈希表的键名,field表示字段名。
例如,我们要获取名为student哈希表中字段名为name的字段值。
命令如下:HGET student name四、Redis哈希表的常用操作除了通过HSET和HGET命令来添加和获取字段及其值,Redis还提供了其他功能强大的命令,如下:1. HDEL命令:用于删除哈希表中的指定字段。
具体命令格式如下:HDEL key field2. HGETALL命令:用于获取哈希表中所有字段和对应的值。
具体命令格式如下:HGETALL key3. HINCRBY命令:用于为哈希表中的指定字段的值增加特定的增量。
具体命令格式如下:HINCRBY key field increment四、Redis哈希表的应用场景Redis哈希表广泛应用于以下场景:1. 存储用户信息:可以将用户的各项信息存储在一个哈希表中,字段名对应不同的信息,方便查询和更新。
Redis缓存的缓存原理

Redis缓存的缓存原理Redis是一种高性能的键值存储系统,被广泛应用于缓存、消息队列、数据同步等场景。
其中,Redis缓存作为其中的一个主要应用之一,可以有效地提高系统的读取性能和响应速度。
本文将介绍Redis缓存的原理和使用场景。
一、Redis缓存的概述Redis是一种基于内存的数据结构服务器,具备高效的读写能力,所以被广泛应用于缓存系统。
Redis缓存主要通过将数据存储在内存中,提高数据的读取速度,从而减轻数据库的读取压力,提高系统的性能。
二、Redis缓存的工作原理1. 缓存的读写操作Redis缓存根据业务需求将数据存储在内存中,以键值对(Key-Value)的形式存在。
当系统需要读取数据时,首先会在Redis缓存中查询是否存在相应的键值对,若存在则直接返回数据,若不存在则查询数据库并将查询结果存储到Redis缓存中。
当数据更新时,系统会更新Redis缓存中的数据,保持与数据库中数据的一致性。
2. 缓存的过期策略为了避免缓存数据过期后无法及时更新,Redis缓存引入了过期策略。
可以为每个键值对设置一个过期时间,到达过期时间后,键值对会被Redis自动删除。
过期策略可以减轻缓存空间的占用,同时保证数据的实时性。
3. 缓存的淘汰策略在Redis缓存中,当缓存空间使用满时,需要根据淘汰策略来删除一些键值对以腾出空间。
常见的淘汰策略有:LRU(Least Recently Used,最近最少使用)、LFU(Least Frequently Used,最不经常使用)等。
通过淘汰策略,可以保证缓存中存储的是最有用的数据。
三、Redis缓存的使用场景1. 页面缓存在Web应用中,经常需要缓存一些静态页面,提高页面的渲染速度。
通过Redis缓存可以将这些静态页面存储在内存中,减轻后端服务器的负载,加快页面的响应速度。
2. 数据库查询缓存数据库查询是Web应用中性能瓶颈之一,通过将查询结果存储在Redis缓存中,可以避免频繁的数据库查询操作,减少数据库的负载,提高系统的性能。
redis缓存常用的读写策略

redis缓存常用的读写策略
在使用Redis作为缓存时,有一些常用的读写策略可以帮助提高性能和减少数据访问延迟。
1. Cache-Aside模式:这是一种常见的读写策略,应用程序首先从缓存中查询数据,如果缓存中不存在,则从数据库中获取数据,并将数据存储到缓存中,以供后续查询使用。
写操作则直接更新数据库,并清除缓存中相应的数据,以保持缓存和数据库的一致性。
2. Read-Through模式:在这种策略中,应用程序直接从缓存中读取数据,如果缓存中不存在所需的数据,则通过一个缓存代理(如Dataloader)从数据库中获取数据,并将数据存储到缓存中。
这种策略可以减少数据库访问次数,提高性能。
3. Write-Through模式:在这种策略中,应用程序直接将写请求发送到缓存,缓存负责将数据更新同步到数据库。
这样可以避免直接写入数据库的开销,提高写入性能,并保持缓存和数据库的一致性。
4. Write-behind模式:这是一种异步写入策略,应用程序首先将写请求发送到缓存中,并立即响应,而不等待缓存将数据写入数据库。
缓存负责将数据异步写入数据库,可以减少写入的延迟,并在高负载情况下提高系统的吞吐量。
这些是Redis缓存常用的读写策略,选择适合的策略可以根据系统的性能需求和数据访问模式。
在实际应用中,根据业务需求,可以结合这些策略来提高系统性能和响应速度。
(Redis缓存)Redis有序集合与ZADD ZRANGE命令

(Redis缓存)Redis有序集合与ZADDZRANGE命令Redis有序集合与ZADD ZRANGE命令Redis是一个开源的内存数据结构存储系统,被广泛应用于诸多领域,其中包括缓存。
在Redis中,有序集合是一种键-值存储模型,它的特点是集合中的每个成员都有一个关联的分数(score),用于排序和去重。
本文将重点介绍Redis有序集合的基本概念,并详细说明ZADD和ZRANGE两个相关命令的用法。
一、Redis有序集合概述Redis有序集合是一种无序集合,其中集合中的每个成员都关联一个分数。
这个分数用来对集合中的成员进行排序,使得集合中的元素是有序的。
与普通的集合不同,有序集合中的成员是唯一的,即不能出现重复的成员。
有序集合在实际应用中有广泛的用途,比如排行榜、积分榜、热门文章列表等。
通过有序集合的分数,可以很方便地根据特定的需求进行排序和筛选。
在Redis中,有序集合的实现采用了一种叫做跳跃表(Skip List)的数据结构,它的插入和删除操作时间复杂度都是O(logN),查找复杂度是O(N)。
二、ZADD命令ZADD命令用于向有序集合中添加一个或多个成员,以及给每个成员关联一个分数。
其基本语法如下:ZADD key score member [score member ...]其中,key是有序集合的键名,score是要关联的分数,member是要添加的成员。
可以使用多个score member参数来一次性添加多个成员。
例如,下面的示例将向有序集合"ranklist"中添加成员"player1"、"player2"和"player3",并分别给它们关联分数100、200和300:ZADD ranklist 100 player1 200 player2 300 player3三、ZRANGE命令ZRANGE命令用于按照成员的分数从小到大的顺序,返回有序集合中指定范围内的成员。
Redis缓存技术的应用与实践

Redis缓存技术的应用与实践Redis是一款开源的key-value存储系统,其数据模型与Memcached类似,但 Redis支持复杂的数据类型,如字符串(Strings)、散列(Hashes)、列表(Lists)、集合(Sets)和有序集合(Sorted Sets)。
Redis还支持事务、Lua脚本、LRU驱动事件通知等功能。
这些功能方便使用者将Redis嵌入到各种场景中。
本篇文章主要介绍Redis缓存技术的应用与实践。
一、 Redis缓存技术的应用场景1. 热点数据缓存热点数据是指一些数据在系统中的频繁访问,读写频率高,如用户登录信息、产品信息、广告信息等。
使用Redis缓存可以减轻数据库负载,提高访问速度。
当查询请求到达系统时,先通过Redis判断缓存中是否有对应的数据。
如果存在,则直接返回数据,不访问数据库;如果不存在,则从数据库中查询出数据,并将数据存入Redis缓存,从而提高读取速度,减少数据库的压力。
2. 分布式锁Redis能够快速执行原子操作,利用这个特性可以支持分布式锁实现。
分布式锁一般用于多个客户端同时访问共享资源时的数据一致性问题。
例如系统A和系统B都对同一数据进行访问,如果没有加锁,可能会造成数据错误。
而使用Redis缓存可以帮助系统解决这个问题,避免重复操作,保证数据的正确性。
3. 会话管理会话管理是一种客户端与服务器端之间的数据交换方式,如Web应用程序的会话管理。
Redis缓存可以用来存储会话信息,提高应用的性能和可扩展性。
在会话管理中,当用户登录并验证成功后,会将用户信息存储到Redis缓存中,并返回一个Token给客户端。
客户端后续的访问请求必须带着Token,服务器会对Token 进行验证,将用户信息返回给客户端。
这样做的好处是节省服务器资源和提高系统性能。
二、 Redis缓存技术的实践1. Redis的安装和配置安装Redis需要下载最新的Redis安装包,将其解压缩到服务器指定的目录中,并运行Redis的服务端程序redis-server。
(Redis缓存)Redis排序与排序命令

(Redis缓存)Redis排序与排序命令Redis排序与排序命令Redis是一个高性能的内存数据库,它支持各种数据结构的存储与操作。
其中,排序是Redis提供的一项重要功能,通过排序可以对存储在Redis中的数据进行特定字段的排序操作。
本文将重点介绍Redis中的排序功能以及相关的排序命令。
1. 排序概述排序是指按照特定字段对存储在Redis中的数据进行排序操作,可以用于处理各种场景,比如排行榜、热门文章列表等。
Redis通过有序集合(sorted set)来实现排序功能,有序集合中的每个元素都有一个score 值,用于排序。
2. 排序命令Redis提供了多个命令用于对有序集合进行排序操作,其中最常用的命令包括:SORT、ZADD、ZCARD、ZSCORE和ZRANK等。
2.1 SORT命令SORT命令用于对指定集合进行排序操作,并返回排序后的结果。
它的基本语法如下:SORT key [BY pattern] [LIMIT offset count] [GET pattern [GET pattern ...]] [ASC|DESC] [ALPHA] [STORE destination]- key: 指定要排序的有序集合的键名。
- BY pattern: 可选参数,用于指定按照其他键的值进行排序。
- LIMIT offset count: 可选参数,用于指定排序结果的偏移量和数量。
- GET pattern: 可选参数,用于获取其他键的值,并将其与排序结果一起返回。
- ASC|DESC: 可选参数,用于指定升序或降序排序,默认为升序排序。
- ALPHA: 可选参数,用于指定按照字符串进行排序,默认按照数字排序。
- STORE destination: 可选参数,用于将排序结果保存到指定键中。
2.2 ZADD命令ZADD命令用于向有序集合中添加一个或多个元素,并为每个元素设置一个score值。
Redis缓存的数据压缩和序列化方法

Redis缓存的数据压缩和序列化方法数据压缩和序列化对于Redis缓存的性能和效率至关重要。
本文将介绍Redis缓存中常用的数据压缩和序列化方法,包括压缩算法和序列化协议的选择,以及实现这些方法的步骤。
一、数据压缩方法数据压缩是将原始数据通过特定算法进行压缩,减少数据量以提高存储和传输效率。
在Redis缓存中,常用的数据压缩方法包括Gzip和Lzf。
1. Gzip压缩方法Gzip是一种常见的压缩算法,能够有效地压缩文本和二进制数据。
在Redis中使用Gzip进行数据压缩的步骤如下:(1)安装Gzip库:首先需要在服务器上安装Gzip库,以便使用Gzip算法。
(2)配置Redis服务器:在Redis服务器的配置文件中,将压缩选项设置为启用,并指定使用Gzip算法进行压缩。
(3)压缩和解压缩数据:在应用程序中,使用Gzip算法对需要存储到Redis缓存的数据进行压缩,或者从Redis缓存中获取数据后解压缩。
2. Lzf压缩方法Lzf是一种高效的压缩算法,特别适用于压缩简单的文本数据。
在Redis中使用Lzf进行数据压缩的步骤类似于Gzip:(1)安装Lzf库:在服务器上安装Lzf库,以便使用Lzf算法。
(2)配置Redis服务器:在Redis服务器的配置文件中,将压缩选项设置为启用,并指定使用Lzf算法进行压缩。
(3)压缩和解压缩数据:在应用程序中,使用Lzf算法对需要存储到Redis缓存的数据进行压缩,或者从Redis缓存中获取数据后解压缩。
二、数据序列化方法数据序列化是将对象或数据结构转换成字节流,以便在不同的应用程序之间进行传输和存储。
在Redis缓存中,常用的数据序列化方法包括JSON、MessagePack和Protobuf。
1. JSON序列化方法JSON是一种轻量级的数据交换格式,常用于Web应用程序中的数据传输。
在Redis中使用JSON进行数据序列化的步骤如下:(1)选择合适的JSON库:根据编程语言的不同,选择适合的JSON库进行序列化和反序列化操作。
(Redis缓存)Redis集合与SADD SMEMBERS命令

(Redis缓存)Redis集合与SADDSMEMBERS命令Redis缓存:Redis集合与SADD SMEMBERS命令Redis是一种高性能的数据存储解决方案,它提供了各种数据结构,包括字符串、哈希、列表、集合和有序集合等。
在这篇文章中,我将重点介绍Redis集合的使用,以及其中的两个重要命令:SADD和SMEMBERS。
一、Redis集合的概述Redis集合是一个无序且唯一的字符串集合,它可以对字符串进行添加、删除和查询等操作。
与列表不同的是,集合中的元素是唯一的,不会出现重复的情况。
在实际应用中,Redis集合经常用于存储用户的标签、好友关系等数据。
二、SADD命令SADD命令用于向集合中添加一个或多个元素,添加的元素必须是字符串类型,并且不存在于集合中。
语法如下:SADD key member [member ...]其中,key是集合的名称,member是要添加的元素。
SADD命令可同时添加多个元素,每个元素之间用空格分隔。
例如,我们可以使用以下命令创建一个名为"tags"的集合,并向其中添加三个元素:SADD tags "redis" "cache" "database"三、SMEMBERS命令SMEMBERS命令用于返回集合中的所有元素,该命令将返回一个包含所有元素的数组。
语法如下:SMEMBERS key其中,key是要查询的集合的名称。
例如,在上述示例中,我们可以使用以下命令来获取名为"tags"的集合中的所有元素:SMEMBERS tags四、Redis集合的其他命令除了SADD和SMEMBERS命令之外,Redis还提供了丰富的集合操作命令。
这些命令包括集合的删除、元素的移除、集合的交集、并集和差集等。
- SREM命令用于从集合中移除一个或多个元素。
- SCARD命令用于获取集合中元素的数量。
redis缓存的简单操作(get、put)

redis缓存的简单操作(get、put)本⽂介绍简单的redis缓存操作,包括引⼊jedisjar包、配置redis、RedisDao需要的⼀些⼯具、向redis中放数据(put)、从redis中取数据(get)、访问redis时的逻辑⼀、引⼊jedis jar包<!-- java访问redis的jar包jedis --><dependency><groupId>redis.clients</groupId><artifactId>jedis</artifactId><version>2.7.3</version></dependency><!-- protostuff序列化依赖 --><dependency><groupId>com.dyuproject.protostuff</groupId><artifactId>protostuff-core</artifactId><version>1.0.8</version></dependency><dependency><groupId>com.dyuproject.protostuff</groupId><artifactId>protostuff-runtime</artifactId><version>1.0.8</version></dependency>注意:为什么要引⼊序列化依赖jar包protostuff?1)从redis中取出的数据是序列化的,我们需要使⽤protostuff的反序列化操作,讲序列化对象转化成我们的需要的对象2)向redis中放⼊数据时,我们需要先使⽤protostuff的序列化操作,将对象转化成序列化对象,才能放⼊redis⼆、在spring配置⽂件中注⼊redis,放⼊spring的ioc容器<!-- 注⼊redis dao --><bean id="redisDao" class="org.demo.dao.cache.RedisDao"> <constructor-arg index="0" value="localhost"></constructor-arg> <constructor-arg index="1" value="6379"></constructor-arg></bean>注意:1)这⾥的RedisDao路径是我的包路径,注意你在配置的时候应使⽤你⾃⼰的路径2)这⾥使⽤本地的redis服务localhost3)redis服务的默认端⼝是6379三、RedisDao需要的⼀些⼯具//redis连接池private final JedisPool jedisPool;//根据对象的字节码⽂件,⽣成空对象private RuntimeSchema<Object> schema = RuntimeSchema.createFrom(Object.class); //Object.class:获取对象的字节码public RedisDao(String ip, int port){jedisPool = new JedisPool(ip, port);}注意:1)RedisDao需要redis的连接池JedisPool,就好⽐JDBC的数据库连接池⼀样。
Redis缓存预热与加载

Redis缓存预热与加载Redis是一种高性能的内存缓存数据库,用于存储和查找数据以提高应用程序的响应速度。
作为一种基于键值对的存储系统,Redis可以将数据缓存在内存中,并通过快速响应请求来大大减少对后端数据库的访问。
在实际应用中,Redis缓存预热和加载是一种优化缓存性能的常用方法。
缓存预热是指在应用程序启动之前或在高峰期到来之前,提前将一些热门数据加载到Redis缓存中。
这样可以避免在实际使用过程中出现大量的缓存未命中,减少对后端数据库的高并发访问,提高系统的响应速度和吞吐量。
缓存预热和加载的具体实现方法可以通过多种途径完成。
以下是一些常用的方式:1. 手动插入:通过脚本或命令行工具手动将热门数据插入到Redis 缓存中。
这种方式需要管理员手动干预,可以根据应用需求和实际情况选择需要预热的数据。
2. 定时任务:使用定时任务工具,如Cron,可以定期从后端数据库中读取热门数据,并将其加载到Redis缓存中。
这样可以保证缓存的最新性,并且减少对数据库的直接访问。
3. 首次访问加载:在用户首次访问某个数据时,如果发现缓存未命中,则从后端数据库中读取数据,并将其保存到Redis缓存中。
这样可以避免对全部数据进行预热,只预热实际使用到的数据。
4. 数据变更时加载:当后端数据库中的数据发生变更时,可以通过监听数据库的变更事件,将被修改的数据同步到Redis缓存中。
这样可以保证缓存与数据库的一致性,同时提高系统的可用性和性能。
除了缓存预热和加载,Redis还提供了一些其他的缓存策略和技术,以进一步提高系统的性能和可扩展性。
例如,可以通过设置过期时间来自动淘汰长时间未被访问的数据,以节省内存空间;也可以通过使用数据分片,在多个Redis节点之间分布数据负载,提高系统的并发处理能力。
总而言之,Redis缓存预热和加载是一种优化缓存性能的有效手段。
通过提前将热门数据加载到Redis缓存中,可以减少对后端数据库的访问,提高应用程序的响应速度和吞吐量。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
[ConfigurationProperty("MaxReadPoolSize", IsRequired = false, DefaultValue = 15)]
public int MaxReadPoolSize
{
{
/// <summary>
/// 加载Readis配置信息
/// </summary>
private static RedisCacheConfig redisCacheConfig = RedisCacheConfig.GetRedisCacheConfig();
{
int _maxWritePoolSize = (int)base["MaxWritePoolSize"];
return _maxWritePoolSize > 0 ? _maxWritePoolSize : 15;
public string ReadServerList
{
get
{
return (string)base["ReadServerList"];
}
set
{
return (bool)base["AutoStart"];
}
set
{
base["AutoStart"] = value;
}
/// </summary>
[ConfigurationProperty("RecordeLog", IsRequired = false, DefaultValue = false)]
public bool RecordeLog
{
{
base["LocalCacheTime"] = value;
}
}
/// <summary>
/// 是否记录日志,该设置仅用于排查redis运行时出现的问题,如redis工作正常,请关闭该项
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Configuration;
namespace Cseth.WebService
{
public sealed class RedisCacheConfig : ConfigurationSection
{
base["ReadServerList"] = value;
}
}
/// <summary>
/// 最大写连接数
/// </summary>
}
set
{
base["MaxReadPoolSize"] = value;
}
}
/// <summary>
/// 是否自动重启
public int LocalCacheTime
{
get
{
return (int)base["LocalCacheTime"];
}
set
get
{
return (bool)base["RecordeLog"];
}
set
{
base["RecordeLog"] = value;
{
throw new ConfigurationErrorsException("没有找到【RedisCacheConfig】配置节点。");
}
return config;
}
安装redis服务、
配置web.config
<RedisCacheConfig WriteServerList="192.168.1.11:6379" ReadServerList="192.168.1.11:6379" MaxWritePoolSize="100" MaxReadPoolSize="100"
[ConfigurationProperty("MaxWritePoolSize", IsRequired = false, DefaultValue = 15)]
public int MaxWritePoolSize
{
get
AutoStart="true" LocalCacheTime="180" RecordeLog="true">
</RedisCacheConfig>
============================================================================================================================================
get
{
int _maxReadPoolSize = (int)base["MaxReadPoolSize"];
return _maxReadPoolSize > 0 ? _maxReadPoolSize : 15;
/// </summary>
[CutoStart", IsRequired = false, DefaultValue = true)]
public bool AutoStart
{
get
{
/// <summary>
/// 获取配置文件中的Redis配置
/// </summary>
/// <returns></returns>
public static RedisCacheConfig GetRedisCacheConfig()
{
get
{
return (string)base["WriteServerList"];
}
set
{
base["WriteServerList"] = value;
}
/// <summary>
/// 本地缓存时间,单位:秒
/// </summary>
[ConfigurationProperty("LocalCacheTime", IsRequired = false, DefaultValue = 36000)]
}
set
{
base["MaxWritePoolSize"] = value;
}
}
/// <summary>
/// 最大读连接数
}
}
/// <summary>
/// 度缓存服务器列表
/// </summary>
[ConfigurationProperty("ReadServerList", IsRequired = false)]
/// <summary>
/// 缓存客户端管理器
/// </summary>
public static PooledRedisClientManager prcm;
/// <summary>
/// 静态构造函数
/// </summary>
static RedisManager()
{
if (prcm == null)
{
CreateManager();
}
{
RedisCacheConfig config = (RedisCacheConfig)ConfigurationManager.GetSection("RedisCacheConfig");
if (config == null)
}
/// <summary>
/// 创建缓存管理器
/// </summary>
private static void CreateManager()
{
string[] writeServerList = redisCacheConfig.WriteServerList.Split(',');
/// <summary>
/// 写缓存服务器列表
/// </summary>
[ConfigurationProperty("WriteServerList", IsRequired = false)]
public string WriteServerList
}
}
}
}
========================================================================================================================================