栈的应用-迷宫问题-数据结构(C语言版)-源代码(直接运行)
C语言实验:迷宫问题(搜索,C语言实现栈、队列)

C语⾔实验:迷宫问题(搜索,C语⾔实现栈、队列)Description给定迷宫起点和终点,寻找⼀条从起点到终点的路径。
(0,1)(2,0)起点(1,1)(1,2)(1,3)(1,4)(2,0)(2,1)(2,4)(3,0)(3,1)(3,2)终点(3,4)(4,1)上图中黄⾊代表墙,⽩⾊代表通路,起点为(1,1),终点为(3,4)。
要求搜寻策略是从起点开始按照“上、下、左、右”四个⽅向寻找终点,到下⼀个点继续按照“上、下、左、右”四个⽅⾯寻找,当该结点四个⽅向都搜寻完,但还没到终点时,退回到上⼀个点,直到找到终点或者没有路径。
⽐如上图从(1,1)开始,向上(0,1)不通,向下到(2,1);到了(2,1)后继续按“上、下、左、右”四个⽅⾯寻找,上已经⾛过,向下到(3,1);到(3,1)后上已经⾛过,下和左不通,向右到(3,2);到(3,2)四个⽅⾯都不通,回到(3,1)四个⽅向都不通,再回到(2,1),(1,1);到达(1,1)后下已经⾛过,左不通,继续向右⾛,重复这个过程最后到达(3,4)。
Input第⼀⾏两个数m和n表⽰迷宫的⾏数和列数。
迷宫⼤⼩不超过100×100第⼆⾏四个数x1,y1,x2,y2分别表⽰起点和终点的坐标。
接下来是m⾏n列的数,⽤来表⽰迷宫,1表⽰墙,0表⽰通路。
Output从起点到终点所经过的路径的坐标。
如果不存在这样的路径则输出“No Path!”。
Sample Input5 61 1 3 41 1 1 1 1 11 0 0 0 0 11 0 1 1 0 11 0 0 1 0 11 1 1 1 1 1Sample Output(1 1)(1 2)(1 3)(1 4)(2 4)(3 4)1.思路:(1)若当前点是终点,dfs函数返回1;(2)若不是终点,将此点标记为1,对该点4个⽅向进⾏搜索,实现⽅式为定义int dir[4][2] = { {-1, 0}, {1, 0}, {0, -1}, {0, 1} }; 通过⼀个⼩循环: for(int i = 0; i < 4; i++) { position nextp; nextp.x = dir[i][0] + now.x;nextp.y = dir[i][1] + now.y;...... } 进⾏搜索;若该点的下⼀个点nextp不是墙,未⾛,并且没有超界则将nextp压⼊栈中,递归调⽤dfs,若此过程经过(1)判断返回了1,说明最终找到了通往终点的路,便可以返回1,结束函数,此时栈中已储存了通往终点的路径,若没有通路,则弹出栈顶元素,根据递归原理该路径上的所有点都会弹出并标记未⾛,回溯到之前的点,继续向其他⽅向搜索,直到找到终点或遍历完整个图。
数据结构栈的应用(迷宫求解)

栈的应用迷宫求解任务:可以输入一个任意大小的迷宫数据,用非递归的方法求出一条走出迷宫的路径,并将路径输出;源代码:#include<stdio.h>#include<stdlib.h>/*数据定义*/typedefenum { ERROR, OK } Status;typedefstruct{int row; //row表示"行"号int line; //line表示"列"号}PosType; //位置的元素类型typedefstruct{intord; //该通道在路径上的"序号"PosType seat; //通道块在迷宫中的"坐标位置"int di; //从此通道走向下以通道块的"方向"}SElemType; //栈的元素类型typedefstruct{SElemType * base;SElemType * top;intstacksize;}SqStack;/*函数原型说明*/Status InitStack(SqStack&S); //创建一个空栈SStatus Push(SqStack&S,SElemType&a); //插入新元素aStatus Pop(SqStack&S,SElemType&a); //删除栈顶元素,a返回其值Status StackEmpty(SqStack S); //检查是否空栈Status MazePath(int maze[12][12],SqStack&S, PosType start, PosType end); //找通路void Initmaze(int maze[12][12],intx,int y); //初始化迷宫void printmaze(int maze[12][12],intx,int y); //显示迷宫Status Pass(int maze[12][12],PosTypeCurPos); //判断当前位置是否可通void Markfoot(int maze[12][12], PosTypeCurPos); //标记当前位置不可通PosTypeNextPos(PosTy peCurPos, intDir); //进入下一位置void printpath(int maze[12][12],SqStackS,intx,inty,PosTypestart,PosType end); //显示通路/*主函数*/void main (void){PosTypestart,end;int t=0;SqStack S;int maze[12][12];do{if(t!=0)printf("\n");for(int i=0;i<20;i++){printf("* ");}if(t==0){printf("\n*\t欢迎来到迷宫世界\t * \n");printf("*\t 1.设置迷宫\t\t * \n");}else{printf("\n* 请继续选择:\t\t\t * \n");printf("*\t 1.设置迷宫\t\t * \n");}printf("*\t 2.设置迷宫路径\t\t * \n");printf("*\t 3.输出迷宫通路路径\t * \n");printf("*\t 4.结束\t\t\t *\n");t++;for(int j=0;j<20;j++){printf("* ");}printf("\n");int s;printf("请选择:");scanf("%d",&s);intaa;intx,y;switch(s){case 1://1.初始化迷宫aa=1;printf("迷宫设置:请输入\n"); //设置迷宫大小do{printf("行X(x<10)=");scanf("%d",&x);printf("列Y(y<10)=");scanf("%d",&y);if(x<1 || x>10||y<1 || y>10){printf("输入错误!\n");}}while(x<1 || x>10||y<1 || y>10);Initmaze(maze,x,y); //初始化迷宫printmaze(maze,x,y); //显示所创建的迷宫break;case 2://寻找迷宫路径if(aa==1){aa=2; //设置入口和出口do{printf("输入入口行坐标:");scanf("%d",&start.row);if(start.row>x)printf("输入错误!\n");}while(start.row>x);do{printf("输入入口列坐标:");scanf("%d",&start.line);if(start.line>y)printf("输入错误!\n");}while(start.line>y);do{printf("输入出口行坐标:");scanf("%d",&end.row);if(end.row>x)printf("输入错误!\n");}while(end.row>x);do{printf("输入出口列坐标:");scanf("%d",&end.line);if(end.line>y)printf("输入错误!\n");}while(end.line>y);}elseprintf("请先初始化迷宫!\n");printf("\n所设置入口坐标为(%d,%d),出口坐标为(%d,%d).\n",start.row,start.line,end.row,end.line);break;case 3://输出此迷宫通路路径if(aa==2){aa=3;if(MazePath(maze,S,start,end)) //若有通路,显示通路printpath(maze,S,x,y,start,end);elseprintf("\n抱歉,找不到通路!\n\n");elseprintf("请先初始化迷宫并寻找路径!\n");break;case 4:exit(0);break;default:exit(0);}}while(1);}/*若迷宫maze中从入口start到出口end的通道,则求得一条存放在栈中*/Status MazePath(int maze[12][12],SqStack&S, PosType start, PosType end){PosTypecurpos;intcurstep;SElemType e;InitStack(S);curpos = start; // 设定"当前位置"为"入口位置curstep = 1; // 探索第一步do {if (Pass(maze,curpos)) // 当前位置可通过,即是未曾走到过的通道块{Markfoot(maze,curpos); // 留下足迹e.di =1;e.ord = curstep;e.seat= curpos;Push(S,e); // 加入路径if (curpos.row==end.row&&curpos.line==end.line)return OK; // 到达终点(出口)curpos = NextPos(curpos, 1); // 下一位置是当前位置的东邻curstep++; // 探索下一步}else // 当前位置不能通过{if (!StackEmpty(S)){Pop(S,e);while (e.di==4 && !StackEmpty(S)){Markfoot(maze,e.seat); // 留下不能通过的标记,并退回一步Pop(S,e);}if (e.di<4)e.di++; // 换下一个方向探索Push(S, e);curpos = NextPos(e.seat, e.di); // 当前位置设为新方向的相邻块}}}} while (!StackEmpty(S));return ERROR;}/*初始化迷宫时间复杂度:n^2*/voidInitmaze(int maze[12][12],intx,int y){char select;do{printf("创建方式A:自动生成B:手动创建\n");label: s canf("%c",&select);if(select=='a'||select=='A') //自动生成{for(int i=1;i<x+1;i++){for(int j=1;j<y+1;j++)maze[i][j]=rand()%2;}break;}else if(select=='b'||select=='B') //手动设置{printf("按行输入%d*%d数据,0代表可通,1代表不可通(每行以Enter结束):\n",x,y);for(int i=1;i<x+1;i++){for(int j=1;j<y+1;j++)scanf("%d",&maze[i][j]);maze[i][y+1]=1;}for(i=0;i<x+2;i++)maze[x+1][i]=1;break;}else if(select=='\n')goto label; //排除Enter键的影响elseif(select!='b'||select!='B'||select!='a'||select!='A')printf("输入错误!\n");}while(select!='b'||select!='B'||select!='a'||select!='A');}/*显示迷宫时间复杂度:n^2*/voidprintmaze(int maze[12][12],intx,int y){printf("\n\n");printf("显示所建的迷宫(#表示墙):\n");printf("%c ",'#');printf("%c ",'y');for(int j=1;j<y+1;j++)printf("%d ",j);printf("%c ",'#');printf("\nx ");for(int i=1;i<y+3;i++)printf("%c ",'#');printf("\n");for(i=1;i<x+1;i++){if(i<x+1)printf("%d ",i);elseprintf("%c ",'#');printf("%c ",'#');for(int j=1;j<y+1;j++){printf("%d ",maze[i][j]);}printf("%c",'#');printf("\n");}for(i=0;i<y+3;i++)printf("%c ",'#');printf("\n");}/*输出路径时间复杂度:n^2*/voidprintpath(int maze[12][12],SqStackS,intx,inty,PosTypestart,PosType end) {printf("\n\n\001通路路径:\n");SElemType * p=S.base;while(p!=S.top){maze[p->seat.row][p->seat.line]=2; //标记为路径中的点p++;}printf("\001路径图为:\n");printf("%c ",'#');printf("%c ",'y');for(int j=1;j<y+1;j++)printf("%d ",j);printf("%c ",'#');printf("\nx ");for(int m=1;m<y+3;m++)printf("%c ",'#');printf("\n");for(int i=1;i<x+1;i++){if(i<x+1)printf("%d ",i);elseprintf("%c ",'#');printf("%c ",'#');for(int j=1;j<y+1;j++){if(maze[i][j]==2){if(i==start.row&&j==start.line||i==end.row&&j==end.line){if(i==start.row&&j==start.line)printf("i ");if(i==end.row&&j==end.line)printf("o ");}elseprintf("%c ",' ');}elseprintf("%c ",'#');}printf("%c ",'#');printf("\n");}for(i=0;i<y+3;i++)printf("%c ",'#');printf("\n\n");printf("\001路径线路为:\n");SqStack M;InitStack(M);SElemType a;while (!StackEmpty(S)){Pop(S,a);Push(M,a);}while (!StackEmpty(M)){Pop(M,a);printf("(%d,%d)",a.seat.row,a.seat.line);}printf("\n");printf("路径输出成功!\n");}/* 判断当前位置是否可通*/Status Pass(int maze[12][12],PosTypeCurPos){if(maze[CurPos.row][CurPos.line]==0)return OK; // 如果当前位置是可以通过,返回1 elsereturn ERROR; // 其它情况返回0}/* 标记当前位置不可通*/voidMarkfoot(int maze[12][12],PosTypeCurPos){maze[CurPos.row][CurPos.line]=1;}/*进入下一位置*/PosTypeNextPos(PosTy peCurPos, intDir){PosTypeReturnPos;switch (Dir){case 1:ReturnPos.row=CurPos.row;ReturnPos.line=CurPos.line+1;break;case 2:ReturnPos.row=CurPos.row+1;ReturnPos.line=CurPos.line;break;case 3:ReturnPos.row=CurPos.row;ReturnPos.line=CurPos.line-1;break;case 4:ReturnPos.row=CurPos.row-1;ReturnPos.line=CurPos.line;break;}returnReturnPos;}/*创建一个空栈S*/Status InitStack(SqStack&S){S.base=(SElemType *)malloc(100*sizeof(SElemType));if(!S.base)return ERROR;S.top=S.base;S.stacksize=100;return OK;}/*插入新元素a*/Status Push(SqStack&S,SElemType&a){*S.top++=a;return OK;}/* 删除栈顶元素,a返回其值*/Status Pop(SqStack&S,SElemType&a){if(S.top==S.base)return ERROR;a=*--S.top;return OK;}/*检查是否空栈*/Status StackEmpty(SqStack S){if(S.top==S.base)return OK;return ERROR;}。
数据结构迷宫问题源代码
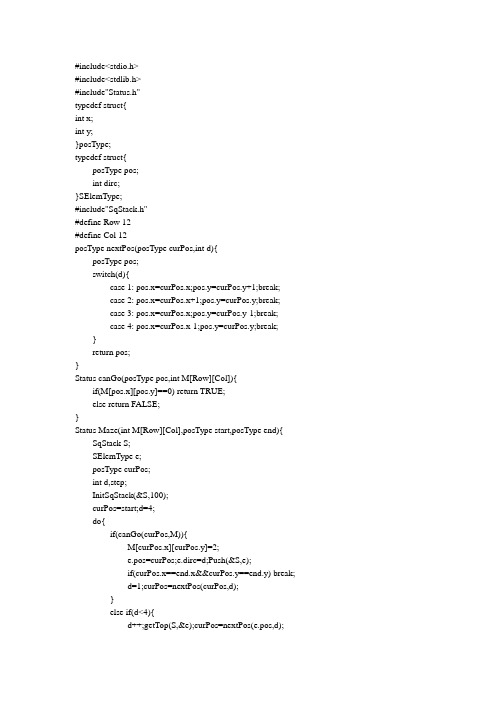
#include<stdio.h>#include<stdlib.h>#include"Status.h"typedef struct{int x;int y;}posType;typedef struct{posType pos;int dirc;}SElemType;#include"SqStack.h"#define Row 12#define Col 12posType nextPos(posType curPos,int d){posType pos;switch(d){case 1: pos.x=curPos.x;pos.y=curPos.y+1;break;case 2: pos.x=curPos.x+1;pos.y=curPos.y;break;case 3: pos.x=curPos.x;pos.y=curPos.y-1;break;case 4: pos.x=curPos.x-1;pos.y=curPos.y;break;}return pos;}Status canGo(posType pos,int M[Row][Col]){if(M[pos.x][pos.y]==0) return TRUE;else return FALSE;}Status Maze(int M[Row][Col],posType start,posType end){ SqStack S;SElemType e;posType curPos;int d,step;InitSqStack(&S,100);curPos=start;d=4;do{if(canGo(curPos,M)){M[curPos.x][curPos.y]=2;e.pos=curPos;e.dirc=d;Push(&S,e);if(curPos.x==end.x&&curPos.y==end.y) break;d=1;curPos=nextPos(curPos,d);}else if(d<4){d++;getTop(S,&e);curPos=nextPos(e.pos,d);}else if(!stackIsEmpty(S)){Pop(&S,&e);d=e.dirc;curPos=e.pos;}}while(!stackIsEmpty(S));if(!stackIsEmpty(S)){Pop(&S,&e);M[e.pos.x][e.pos.y]='e';d=e.dirc;for(step=stackLength(S);step>0;step--){Pop(&S,&e);switch(d){case 1: M[e.pos.x][e.pos.y]=26;break;case 2: M[e.pos.x][e.pos.y]=25;break;case 3: M[e.pos.x][e.pos.y]=27;break;case 4: M[e.pos.x][e.pos.y]=24;break;}d=e.dirc;}M[start.x][start.y]='s';return TRUE;}else return FALSE;}void printMaze(int M[Row][Col],posType start,posType end){int i,j;printf("迷宫:入口(%d,%d),出口(%d,%d)\n",start.x,start.y,end.x,end.y); printf("\t%3c",' ');for(i=0;i<Col;i++)printf("%2d ",i);printf("\n");for(i=0;i<Row;i++){printf("\t%2d",i);for(j=0;j<Col;j++){if(M[i][j]==1)printf("|||");else if(M[i][j]==0)printf(" ");else if(M[i][j]==2)printf(" = ");else printf(" %c",M[i][j]);}printf("\n");}printf("\n");}int main(){int M[Row][Col]={{1,1,1,1,1,1,1,1,1,1,1,1},{1,0,0,0,1,1,0,1,1,1,0,1},{1,0,1,0,0,1,0,0,1,0,0,1},{1,0,1,1,0,0,0,1,1,0,1,1},{1,0,0,1,0,1,0,0,0,0,0,1},{1,1,0,1,0,1,1,0,1,0,1,1},{1,0,0,1,0,0,0,0,1,1,1,1},{1,0,1,0,1,1,1,1,0,0,1,1},{1,0,0,0,0,1,1,0,0,0,1,1},{1,0,1,0,1,0,0,0,1,0,0,1},{1,0,0,0,0,0,1,0,0,1,0,1},{1,1,1,1,1,1,1,1,1,1,1,1} };posType start,end;start.x=1;start.y=1;end.x=10;end.y=10;printMaze(M,start,end);if(Maze(M,start,end)){printf("找到可行路径:\n");printMaze(M,start,end);}else printf("无可行路径!\n");system("pause");return 0;}。
数据结构(C语言版)实验报告(迷宫)
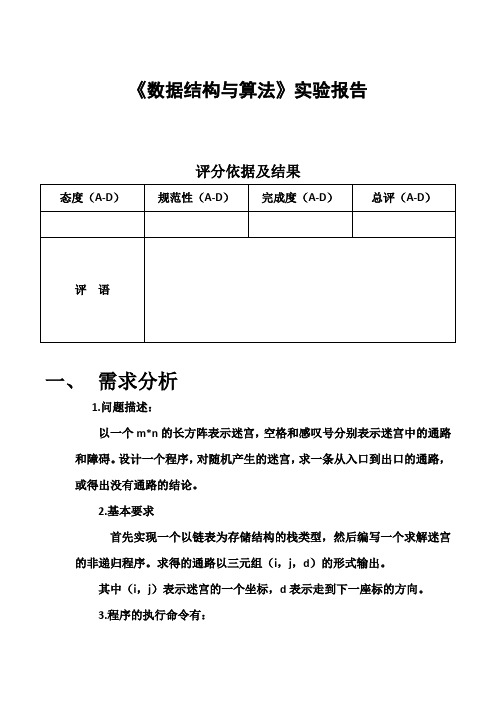
《数据结构与算法》实验报告评分依据及结果一、需求分析1.问题描述:以一个m*n的长方阵表示迷宫,空格和感叹号分别表示迷宫中的通路和障碍。
设计一个程序,对随机产生的迷宫,求一条从入口到出口的通路,或得出没有通路的结论。
2.基本要求首先实现一个以链表为存储结构的栈类型,然后编写一个求解迷宫的非递归程序。
求得的通路以三元组(i,j,d)的形式输出。
其中(i,j)表示迷宫的一个坐标,d表示走到下一座标的方向。
3.程序的执行命令有:1)输入迷宫的列数2)输入迷宫的行数二、概要设计为实现上述功能,需要以一个链表为存储结构的栈类型1.栈的顺序存储表示typedef struct{int x; /*列*/int y; /*行*/}PosType; //坐标位置类型typedef struct{int ord; //通道块在路径上的"序号"PosType seat; //通道块在迷宫中的"坐标位置"int di; //从此通道块走向下一通道块的"方向"}SElemType; //栈的元素类型typedef struct{SElemType *base;SElemType *top;int stacksize; //当前已分配的存储空间,以元素为单位}SqStack;//迷宫程序typedef struct{int lie; /*列数*/int hang; /*行数*/char a[999][999];}MazeType; /*迷宫类型*/2.基本操作int InitStack(SqStack *S)//分配空间int GetTop(SqStack *S,SElemType *e) //若栈不空,则用e返回S的栈顶元素,并返回OK;否则返回ERRORint Push(SqStack *S,SElemType *e)//插入元素e作为新的栈顶元素int Pop(SqStack *S,SElemType *e) //若栈不空,则删除S的栈顶元素,用e返回其值,并返回OK;否则返回ERRORint generatemaze( MazeType *maze)// 随机生成迷宫int Pass(MazeType *maze, PosType curpos ) //判断当前位置可否通过int FootPrint(MazeType *maze,PosType curpos) //留下足迹int MarkPrint(MazeType *maze,PosType curpos) //留下不能通过的标记PosType NextPos(PosType curpos,int di) //返回当前位置的下一位置int MazePath(MazeType *maze,PosType start,PosType end) //若迷宫有解,则求得一条存放在栈中(从栈底到栈顶),并返回OK,否则返回ERROR void PrintMaze(MazeType *maze) //打印迷宫三、详细设计//程序的头文件#include <stdio.h>#include <malloc.h>#include <stdlib.h>#include <string.h>#include <time.h>//函数的返回值#define OK 1#define ERROR 0#define NULL 0#define OVERFLOW -2#define STACK_INIT_SIZE 100#define STACKINCREMENT 10//栈的顺序存储表示typedef struct{int x; /*列*/int y; /*行*/}PosType; //坐标位置类型typedef struct{int ord; //通道块在路径上的"序号"PosType seat; //通道块在迷宫中的"坐标位置"int di; //从此通道块走向下一通道块的"方向"}SElemType; //栈的元素类型typedef struct{SElemType *base;SElemType *top;int stacksize; //当前已分配的存储空间,以元素为单位}SqStack;//基本操作int InitStack(SqStack *S){S->base=(SElemType *)malloc(STACK_INIT_SIZE * sizeof(SElemType));if(!S->base)exit(OVERFLOW);S->top=S->base;S->stacksize=STACK_INIT_SIZE;return OK;}//若栈不空,则用e返回S的栈顶元素,并返回OK;否则返回ERRORint GetTop(SqStack *S,SElemType *e){if(S->top==S->base)return ERROR;*e=*(S->top-1);return OK;}int Push(SqStack *S,SElemType *e)//插入元素e作为新的栈顶元素{if(S->top-S->base>=S->stacksize)/*栈满,追加存储空间*/{S->base=(SElemType*)realloc(S->base,(S->stacksize+STACKINCREMENT)*sizeof(SElemType));if(!S->base)exit(OVERFLOW);S->top=S->base+S->stacksize;S->stacksize+=STACKINCREMENT;}*S->top++=*e;return OK;}//若栈不空,则删除S的栈顶元素,用e返回其值,并返回OK;否则返回ERRORint Pop(SqStack *S,SElemType *e){if(S->top==S->base)return ERROR;*e=*--S->top;return OK;}int StackEmpty(SqStack *S){return(S->top==S->base);}//迷宫程序typedef struct{int lie; /*列数*/int hang; /*行数*/char a[999][999];}MazeType; /*迷宫类型*//*随机生成迷宫*/int generatemaze( MazeType *maze){int i,j;maze->a[0][0]=2;maze->a[++maze->hang][++maze->lie]=3; /*设置外墙*/maze->a[0][maze->lie]='!';maze->a[maze->hang][0]='!';for(j=1;j<maze->lie;j++){maze->a[0][j]='!';maze->a[maze->hang][j]='!';}for(i=1;i<maze->hang;i++){maze->a[i][0]='!';maze->a[i][maze->lie]='!';}srand((unsigned)time( NULL ));rand();for(i=1; i <maze->hang; i++)for(j=1;j<maze->lie;j++){if (rand()>=RAND_MAX/4) maze->a[i][j] =' '; //' ' 暗示出路else maze->a[i][j] ='!'; //'!'暗示无出路}return OK;}int Pass(MazeType *maze, PosType curpos ) //判断当前位置可否通过{if ((curpos.x < 1) || (curpos.x >= maze->lie))return ERROR;if ((curpos.y < 1) || (curpos.y >= maze->hang))return ERROR;if (maze->a[curpos.y][curpos.x]==' ')return OK;else return ERROR;}int FootPrint(MazeType *maze,PosType curpos) //留下足迹{maze->a[curpos.y][curpos.x]='*';return OK;}int MarkPrint(MazeType *maze,PosType curpos) //留下不能通过的标记{maze->a[curpos.y][curpos.x]='@';return OK;}PosType NextPos(PosType curpos,int di)//返回当前位置的下一位置{PosType pos=curpos;switch(di){case 1: //右东pos.x++;break;case 2: //下南pos.y++;break;case 3: //左西pos.x--;break;case 4: //上北pos.y--;break;}return pos;}//若迷宫有解,则求得一条存放在栈中(从栈底到栈顶),并返回OK,否则返回ERROR int MazePath(MazeType *maze,PosType start,PosType end){PosType curpos;SqStack *S=(SqStack *)malloc(sizeof(SqStack));InitStack(S);SElemType *e;e=(SElemType *)malloc(sizeof(SElemType));curpos=start; //设定当前位置为入口位置int curstep = 1; //探索第一步do {if(Pass(maze,curpos)) //当前位置可通过{FootPrint(maze,curpos);e->ord=curstep;e->seat=curpos;e->di=1;Push(S,e);if(curpos.x==end.x&&curpos.y==end.y)return (OK);curpos=NextPos(curpos,1);curstep++;}else{if(!StackEmpty(S)){Pop(S,e);while(e->di==4&&!StackEmpty(S)) //栈不空但栈顶位置四周均不通{MarkPrint(maze,e->seat);Pop(S,e);}if(e->di<4) //栈不空且栈顶位置四周有其他位置未探索{e->di++;Push(S,e);curpos=e->seat;curpos=NextPos(curpos,e->di);}}}}while(!StackEmpty(S));return ERROR;}void PrintMaze(MazeType *maze) //打印迷宫{int i,j,k,n;int c[999],d[999];for(i=0,k=0;i<=maze->hang;i++){for(j=0;j<=maze->lie;j++){printf("%c ",maze->a[i][j]);if(maze->a[i][j]=='*'){c[k]=i;d[k]=j;k++;}}printf("\n");}n=k;for(k=0;k<n;k++)printf("<%d,%d>",c[k],d[k]);printf("\n");printf("\n");}int main(){int zmg;char ch;printf(" 数据结构课程设计--迷宫问题求解\n\n");printf(" |----------------------------------------|\n");printf(" | |\n");printf(" | |\n");printf(" | |\n");printf(" | |\n");printf(" | XXXX XXXXXXXXXXXXXX |\n");printf(" | XXXXXXX |\n");printf(" |----------------------------------------|\n");getchar();do{system("cls");fflush(stdin);MazeType *maze=(MazeType *)malloc(sizeof(MazeType)); //设置迷宫的长宽不含外墙printf("请输入迷宫的列数(不含外墙时):");scanf("%d",&maze->lie);printf("请输入迷宫的行数(不含外墙时):");scanf("%d",&maze->hang);generatemaze(maze);printf("随机创建迷宫\n");PrintMaze(maze);getchar();getchar();PosType start,end;start.x=1;start.y=1;end.x=maze->lie-1;end.y=maze->hang-1;zmg=MazePath(maze,start,end);if(zmg){printf("此迷宫通路为\n");PrintMaze(maze);}elseprintf("此迷宫无通路\n"); //getchar();printf("再次尝试?(Y/N)?");scanf("%c",&ch);}while(ch=='Y'||ch=='y');return 0;}四、调试分析1.本程序界面设计合理,以空格为通路,感叹号!为障碍,笑脸为起始点,*为行走路线,心形为出口设计精巧,便于用户使用。
数据结构栈的应用(迷宫求解)

数据结构栈的应用(迷宫求解)栈的应用迷宫求解任务:可以输入一个任意大小的迷宫数据,用非递归的方法求出一条走出迷宫的路径,并将路径输出;源代码:#include#include/*数据定义*/typedefenum { ERROR, OK } Status;typedefstruct{int row; //row表示"行"号int line; //line表示"列"号}PosType; //位置的元素类型typedefstruct{intord; //该通道在路径上的"序号"PosType seat; //通道块在迷宫中的"坐标位置"int di; //从此通道走向下以通道块的"方向"}SElemType; //栈的元素类型typedefstruct{SElemType * base;SElemType * top;intstacksize;}SqStack;/*函数原型说明*/Status InitStack(SqStack&S); //创建一个空栈SStatus Push(SqStack&S,SElemType&a); //插入新元素aStatus Pop(SqStack&S,SElemType&a); //删除栈顶元素,a返回其值Status StackEmpty(SqStack S); //检查是否空栈Status MazePath(int maze[12][12],SqStack&S, PosType start, PosType end); //找通路void Initmaze(int maze[12][12],intx,int y); //初始化迷宫void printmaze(int maze[12][12],intx,int y); //显示迷宫Status Pass(int maze[12][12],PosTypeCurPos); //判断当前位置是否可通void Markfoot(int maze[12][12], PosTypeCurPos); //标记当前位置不可通PosTypeNextPos(PosTy peCurPos, intDir); //进入下一位置void printpath(int maze[12][12],SqStackS,intx,inty,PosTypestart,PosType end); //显示通路/*主函数*/void main (void){PosTypestart,end;int t=0;SqStack S;int maze[12][12];do{if(t!=0)printf("\n");for(int i=0;i<20;i++){printf("* ");}if(t==0){printf("\n*\t欢迎来到迷宫世界\t * \n"); printf("*\t 1.设置迷宫\t\t * \n");}else{printf("\n* 请继续选择:\t\t\t * \n");printf("*\t 1.设置迷宫\t\t * \n");}printf("*\t 2.设置迷宫路径\t\t * \n");printf("*\t 3.输出迷宫通路路径\t * \n"); printf("*\t 4.结束\t\t\t *\n");t++;for(int j=0;j<20;j++){printf("* ");}printf("\n");int s;printf("请选择:");scanf("%d",&s);intaa;intx,y;switch(s){case 1://1.初始化迷宫aa=1;printf("迷宫设置:请输入\n"); //设置迷宫大小do{printf("行X(x<10)=");scanf("%d",&x);printf("列Y(y<10)=");scanf("%d",&y);if(x<1 || x>10||y<1 || y>10){printf("输入错误!\n");}}while(x<1 || x>10||y<1 || y>10);Initmaze(maze,x,y); //初始化迷宫printmaze(maze,x,y); //显示所创建的迷宫break;case 2://寻找迷宫路径if(aa==1){aa=2; //设置入口和出口do{printf("输入入口行坐标:");scanf("%d",&start.row); if(start.row>x)printf("输入错误!\n");}while(start.row>x);do{printf("输入入口列坐标:");scanf("%d",&start.line); if(start.line>y)printf("输入错误!\n");}while(start.line>y);do{printf("输入出口行坐标:");scanf("%d",&end.row); if(end.row>x)printf("输入错误!\n");}while(end.row>x);do{printf("输入出口列坐标:");scanf("%d",&end.line);if(end.line>y)printf("输入错误!\n");}while(end.line>y);}elseprintf("请先初始化迷宫!\n");printf("\n所设置入口坐标为(%d,%d),出口坐标为(%d,%d).\n",start.row,start.line,end.row,end.line);break;case 3://输出此迷宫通路路径if(aa==2){aa=3;if(MazePath(maze,S,start,end)) //若有通路,显示通路printpath(maze,S,x,y,start,end);elseprintf("\n抱歉,找不到通路!\n\n");elseprintf("请先初始化迷宫并寻找路径!\n");break;case 4:exit(0);break;default:exit(0);}}while(1);}/*若迷宫maze中从入口start到出口end的通道,则求得一条存放在栈中*/Status MazePath(int maze[12][12],SqStack&S, PosType start, PosType end){PosTypecurpos;intcurstep;SElemType e;InitStack(S);curpos = start; // 设定"当前位置"为"入口位置curstep = 1; // 探索第一步do {if (Pass(maze,curpos)) // 当前位置可通过,即是未曾走到过的通道块{Markfoot(maze,curpos); // 留下足迹e.di =1;e.ord = curstep;e.seat= curpos;Push(S,e); // 加入路径if (curpos.row==end.row&&curpos.line==end.line)return OK; // 到达终点(出口)curpos = NextPos(curpos, 1); // 下一位置是当前位置的东邻curstep++; // 探索下一步}else // 当前位置不能通过{if (!StackEmpty(S)){Pop(S,e);while (e.di==4 && !StackEmpty(S)){Markfoot(maze,e.seat); // 留下不能通过的标记,并退回一步Pop(S,e);}if (e.di<4)e.di++; // 换下一个方向探索Push(S, e);curpos = NextPos(e.seat, e.di); // 当前位置设为新方向的相邻块}}}} while (!StackEmpty(S));return ERROR;}/*初始化迷宫时间复杂度:n^2*/voidInitmaze(int maze[12][12],intx,int y){char select;do{printf("创建方式A:自动生成B:手动创建\n");label: s canf("%c",&select);if(select=='a'||select=='A') //自动生成{for(int i=1;i<x+1;i++)< p="">{for(int j=1;j<y+1;j++)< p="">maze[i][j]=rand()%2;}break;}else if(select=='b'||select=='B') //手动设置{printf("按行输入%d*%d数据,0代表可通,1代表不可通(每行以Enter结束):\n",x,y);for(int i=1;i<x+1;i++)< p="">{for(int j=1;j<y+1;j++)< p="">scanf("%d",&maze[i][j]);maze[i][y+1]=1;}for(i=0;i< p="">break;}else if(select=='\n')goto label; //排除Enter键的影响elseif(select!='b'||select!='B'||select!='a'||select!='A')printf("输入错误!\n");}while(select!='b'||select!='B'||select!='a'||select!='A');}/*显示迷宫时间复杂度:n^2*/voidprintmaze(int maze[12][12],intx,int y){printf("\n\n");printf("显示所建的迷宫(#表示墙):\n");printf("%c ",'#');printf("%c ",'y');for(int j=1;j<y+1;j++)< p="">printf("%d ",j);printf("%c ",'#');printf("\nx ");for(int i=1;i<y+3;i++)< p=""> printf("%c ",'#');printf("\n");for(i=1;i<x+1;i++)< p=""> {if(i<x+1)< p="">printf("%d ",i);elseprintf("%c ",'#');printf("%c ",'#');for(int j=1;j<y+1;j++)< p=""> {printf("%d ",maze[i][j]);}printf("%c",'#');printf("\n");}for(i=0;i</y+1;j++)<></x+1)<></x+1;i++)<></y+3;i++)<></y+1;j++)<><></y+1;j++)<></x+1;i++)<></y+1;j++)<></x+1;i++)<>。
C语言数据结构之迷宫问题

C语⾔数据结构之迷宫问题本⽂实例为⼤家分享了数据结构c语⾔版迷宫问题栈实现的具体代码,供⼤家参考,具体内容如下程序主要参考⾃严蔚敏⽼师的数据结构c语⾔版,在书中程序的⼤体框架下进⾏了完善。
关于迷宫问题的思路可查阅原书。
#include<iostream>using namespace std;#define MAXSIZE 10typedef int Status;typedef struct{int x;int y;}Postype;typedef struct{int ord;Postype seat;int dir;}SElemType;//栈的元素类型typedef struct{//SElemType data[MAXSIZE];SElemType* top;SElemType* base;}Stack;//栈的结构类型typedef struct{char arr[MAXSIZE][MAXSIZE];}MAZETYPE;//迷宫结构体MAZETYPE maze;void InitMaze(){maze.arr[0][0] = maze.arr[0][1] = maze.arr[0][2] = maze.arr[0][3] = maze.arr[0][4] = maze.arr[0][5] = maze.arr[0][6] = maze.arr[0][7] = maze.arr[0][8] = maze.arr[0][9] = '1'; maze.arr[1][0] = maze.arr[1][3] = maze.arr[1][7] = maze.arr[1][9] = '1';maze.arr[1][1] = maze.arr[1][2] = maze.arr[1][4] = maze.arr[1][5] = maze.arr[1][6] = maze.arr[1][8] = '0';maze.arr[2][0] = maze.arr[2][3] = maze.arr[2][7] = maze.arr[2][9] = '1';maze.arr[2][1] = maze.arr[2][2] = maze.arr[2][4] = maze.arr[2][5] = maze.arr[2][6] = maze.arr[2][8] = '0';maze.arr[3][0] = maze.arr[3][5] = maze.arr[3][6] = maze.arr[3][9] = '1';maze.arr[3][1] = maze.arr[3][2] = maze.arr[3][3] = maze.arr[3][4] = maze.arr[3][7] = maze.arr[3][8] = '0';maze.arr[4][0] = maze.arr[4][2] = maze.arr[4][3] = maze.arr[4][4] = maze.arr[4][9] = '1';maze.arr[4][1] = maze.arr[4][5] = maze.arr[4][6] = maze.arr[4][7] = maze.arr[4][8] = '0';maze.arr[5][0] = maze.arr[5][4] = maze.arr[5][9] = '1';maze.arr[5][1] = maze.arr[5][2] = maze.arr[5][3] = maze.arr[5][5] = maze.arr[5][6] = maze.arr[5][7] = maze.arr[5][8] = '0';maze.arr[6][0] = maze.arr[6][2] = maze.arr[6][6] = maze.arr[6][9] = '1';maze.arr[6][1] = maze.arr[6][3] = maze.arr[6][4] = maze.arr[6][5] = maze.arr[6][7] = maze.arr[6][8] = '0';maze.arr[7][0] = maze.arr[7][2] = maze.arr[7][3] = maze.arr[7][4] = maze.arr[7][6] = maze.arr[7][9] = '1';maze.arr[7][1] = maze.arr[7][5] = maze.arr[7][7] = maze.arr[7][8] = '0';maze.arr[8][0] = maze.arr[8][1] = maze.arr[8][9] = '0';maze.arr[8][2] = maze.arr[8][3] = maze.arr[8][4] = maze.arr[8][5] = maze.arr[8][6] = maze.arr[8][7] = maze.arr[8][8] = '0';maze.arr[9][0] = maze.arr[9][1] = maze.arr[9][2] = maze.arr[9][3] = maze.arr[9][4] = maze.arr[9][5] = maze.arr[9][6] = maze.arr[9][7] = maze.arr[9][8] = maze.arr[9][9] = '1'; }Status initStack(Stack &s){s.base = (SElemType*)malloc(MAXSIZE*sizeof(SElemType));if (!s.base) return 0;s.top = s.base;return 1;}void Push(Stack &s, SElemType e){*s.top++ = e;}void Pop(Stack &s, SElemType &e){e = *--s.top;}Status StackEmpty(Stack &s){if (s.top == s.base) return 1;else return 0;}Status Pass(Postype curpos){if (maze.arr[curpos.x][curpos.y] == '0')return 1;else return 0;}void Foot(Postype curpos){maze.arr[curpos.x][curpos.y] = '*';}void MarkPrint(Postype curpos){maze.arr[curpos.x][curpos.y] = '!';}Status StructCmp(Postype a, Postype b){if (a.x = b.x&&a.y == b.y) return 1;else return 0;}//下⼀个位置Postype NextPos(Postype CurPos, int Dir){Postype ReturnPos;switch (Dir){case 1:ReturnPos.x = CurPos.x;ReturnPos.y = CurPos.y + 1;break;case 2:ReturnPos.x = CurPos.x + 1;ReturnPos.y = CurPos.y;break;case 3:ReturnPos.x = CurPos.x;ReturnPos.y = CurPos.y - 1;break;case 4:ReturnPos.x = CurPos.x - 1;ReturnPos.y = CurPos.y;break;}return ReturnPos;}Status MazePath(Postype start, Postype end) {Stack s;SElemType e;initStack(s);Postype curpos = start;int curstep = 1;do{if (Pass(curpos)){Foot(curpos);e = { curstep, curpos, 1 };Push(s, e);if (StructCmp(curpos, end)) return 1;curpos = NextPos(curpos, 1);curstep++;}else{if (!StackEmpty(s)){Pop(s, e);while (e.dir ==4 &&!StackEmpty(s)){MarkPrint(e.seat); Pop(s, e);}if (e.dir < 4 && !StackEmpty(s)){e.dir++;Push(s, e);curpos = NextPos(e.seat, e.dir);}}}} while (!StackEmpty(s));return 0;}int main(){InitMaze();Postype s, e;s.x = s.y = 1;e.x = e.y = 8;if (MazePath(s, e))printf("迷宫成功解密!\n");elseprintf("解密失败\n");for (int i = 0; i < 10; i++){for (int j = 0; j < 10; j++){printf("%c ", maze.arr[i][j]);}printf("\n");}cout << "-=================================" << endl;for (int i = 0; i < 10; i++){for (int j = 0; j < 10; j++){if (maze.arr[i][j] == '*' || maze.arr[i][j] == '!')printf("%c ", maze.arr[i][j]);else cout << " ";}printf("\n");}}以上就是本⽂的全部内容,希望对⼤家的学习有所帮助,也希望⼤家多多⽀持。
数据结构迷宫问题完整代码
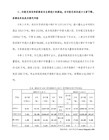
int main(int argc, char* argv[])
{
struct SqStack *p=Init();
int a[10][10]={{1,1,1,1,1,1,1,1,1,1},
{1,0,0,1,0,0,0,1,0,1},
{1,0,0,1,0,0,0,1,0,1},
printf("%d,%d,%d,%d\n",e.ord,e.seat.x,e.seat.y,e.di);
}
}
int Pass(struct PosType cur,int b[10][10])
{
if(b[cur.x][cur.y]==1)
return 0;
else return 1;
#include "stdafx.h"
#define STACK_INIT_SIZE 100
#define STACKINCREMENT 10
#include <malloc.h>
#include <stdlib.h>
struct PosType{
int x;
int y;
};
int curstep=1;
do
{
if(Pass(curpos,a))
{
FootPrint(curpos,a);
e.di=1;
e.ord=curstep;
e.seat=curpos;
Push(p,e);
printf("%d,%d,%d,%d\n",e.ord,e.seat.x,e.seat.y,e.di);
用c语言实现迷宫求解完美源代码

用c语言实现迷宫求解完美源代码#include#include#include#define TRUE 1#define FALSE 0#define OK 1#define ERROR 0#define OVERFLOW -2#define UNDERFLOW -2typedef int Status;//-----栈开始-----typedef struct{//迷宫中r行c列的位置int r;int c;}PostType;//坐标位置类型typedef struct{int ord;// 当前位置在路径上的序号PostType seat;// 当前坐标int di;// 从此通块走向下一通块的“方向”}SElemType;// 栈的元素类型//定义链式栈的存储结构struct LNode{SElemType data;//数据域struct LNode *next;//指针域};struct LStack{struct LNode *top;//栈顶指针};Status InitStack(LStack &s)//操作结果:构造一个空栈S {struct LNode *p;p=(LNode *)malloc(sizeof(LNode));if(!p){printf("分配失败,退出程序");exit(ERROR);}s.top=p;p->next=NULL;return OK;}Status StackEmpty(LStack s)//若栈s为空栈,则返回TRUE,否则FALSE{if(s.top->next==NULL) return TRUE;return FALSE;}Status Push(LStack &s,SElemType e)//插入元素e成为新的栈顶元素{struct LNode *p;p=(LNode *)malloc(sizeof(LNode));if(!p) exit(OVERFLOW);s.top->data=e;p->next=s.top;s.top=p;return OK;}Status Pop(LStack &s,SElemType &e)//删除s的栈顶元素,并且用e返回其值{struct LNode *p;if(!(s.top->next)) exit(UNDERFLOW);p=s.top;s.top=p->next;e=s.top->data;free(p);return OK;}Status DestroyStack(LStack &s)//操作结果:栈s被销毁{struct LNode *p;p=s.top;while(p){s.top=p->next;free(p);p=s.top;}return OK;}//-----栈结束------//-----迷宫开始-------#define MAXLEN 10// 迷宫包括外墙最大行列数typedef struct{int r;int c;char adr[MAXLEN][MAXLEN];// 可取' ''*' '@' '#'}MazeType;// 迷宫类型Status InitMaze(MazeType&maze){// 初始化迷宫,成功返回TRUE,否则返回FALSE int m,n,i,j;printf("输入迷宫行数和列数(包括了外墙): ");scanf("%d%d",&maze.r,&maze.c); // 输入迷宫行数和列数for(i=0;i<=maze.c+1;i++){// 迷宫行外墙maze.adr[0][i]='#';maze.adr[maze.r+1][i]='#';}//forfor(i=0;i<=maze.r+1;i++){// 迷宫列外墙maze.adr[i][0]='#';maze.adr[i][maze.c+1]='#';}for(i=1;i<=maze.r;i++)for(j=1;j<=maze.c;j++)maze.adr[i][j]=' ';// 初始化迷宫printf("输入障碍的坐标((-1 -1)结束): ");scanf("%d%d",&m,&n);// 接收障碍的坐标while(m!=-1){if(m>maze.r || n>maze.c)// 越界exit(ERROR);maze.adr[m][n]='#';// 迷宫障碍用#标记printf("输入障碍的坐标((-1,-1)结束): ");scanf("%d%d",&m,&n);}//whilereturn OK;}//InitMazeStatus Pass(MazeType maze,PostType curpos){// 当前位置可同则返回TURE,否则返回FALSEif(maze.adr[curpos.r][curpos.c]==' ')// 可通return TRUE;elsereturn FALSE;}//PassStatus FootPrint(MazeType &maze,PostType curpos) {// 若走过并且可通,则返回TRUE,否则返回FALSE maze.adr[curpos.r][curpos.c]='*';//"*"表示可通return OK;}//FootPrintPostType NextPos(PostType &curpos,int i){// 指示并返回下一位置的坐标PostType cpos;cpos=curpos;switch(i){//1.2.3.4 分别表示东南西北方向case 1 : cpos.c+=1; break;case 2 : cpos.r+=1; break;case 3 : cpos.c-=1; break;case 4 : cpos.r-=1; break;default: exit(ERROR);}return cpos;}//NextposStatus MarkPrint(MazeType &maze,PostType curpos) {// 曾走过,但不是通路标记,并返回OKmaze.adr[curpos.r][curpos.c]='@';//"@" 表示曾走过但不通return OK;}//MarkPrintStatus MazePath(MazeType &maze,PostType start,PostType end){// 若迷宫maze存在通路,则求出一条同路放在栈中,并返回TRUE,否则返回FALSE struct LStack S;PostType curpos;int curstep;// 当前序号,1,2,3,4分别表示东南西北方向SElemType e;InitStack(S);curpos=start; //设置"当前位置"为"入口位置"curstep=1;// 探索第一位printf("以三元组形式表示迷宫路径:\n");do{if(Pass(maze,curpos)){// 当前位置可以通过FootPrint(maze,curpos);// 留下足迹e.ord=curstep;e.seat=curpos;e.di=1;printf("%d %d %d-->",e.seat.r,e.seat.c,e.di);Push(S,e);// 加入路径if(curpos.r==end.r&&curpos.c==end.c)if(!DestroyStack(S))// 销毁失败exit(OVERFLOW);elsereturn TRUE; // 到达出口else{curpos=NextPos(curpos,1);// 下一位置是当前位置的东邻curstep++;// 探索下一步}//else}//ifelse{// 当前位置不通时if(!StackEmpty(S)){Pop(S,e);while(e.di==4&& !StackEmpty(S)){MarkPrint(maze,e.seat);Pop(S,e);// 留下不能通过的标记,并退一步}//whileif(e.di < 4){e.di++;// 换一个方向探索Push(S,e);curpos=NextPos(e.seat,e.di);// 设定当前位置是该方向上的相邻printf("%d %d %d-->",e.seat.r,e.seat.c,e.di);}//if}//if}//else}while(!StackEmpty(S));if(!DestroyStack(S))// 销毁栈exit(OVERFLOW);elsereturn FALSE;}//MazePathvoid PrintMaze(MazeType &maze){// 将标记路径信息的迷宫输出到终端int i,j;printf("\n输出迷宫(*表示通路):\n\n");printf("");for(i=0;i<=maze.r+1;i++)// 打印列数名printf("%4d",i);printf("\n\n");for(i=0;i<=maze.r+1;i++){printf("%2d",i);// 打印行名for(j=0;j<=maze.c+1;j++)printf("%4c",maze.adr[i][j]);// 输出迷宫当前位置的标记printf("\n\n");}}//PrintMazeint main(){// 主函数MazeType maze;PostType start,end;char cmd;do{printf("-------创建迷宫--------\n");if(!InitMaze(maze)){printf("Initialization errors\n");exit(OVERFLOW);// 初始化失败}do{// 输入迷宫入口坐标printf("\n输入迷宫入口坐标: ");scanf("%d%d",&start.r,&start.c);if(start.r>maze.r ||start.c>maze.c){printf("\nBeyond the maze\n"); continue;}}while(start.r>maze.r ||start.c>maze.c);do{// 输入迷宫出口坐标printf("\n输入迷宫出口坐标: ");scanf("%d%d",&end.r,&end.c);if(end.r>maze.r ||end.c>maze.c){printf("\nBeyond the maze\n"); continue;}}while(end.r>maze.r ||end.c>maze.c);if(!MazePath(maze,start,end))//迷宫求解printf("\nNo path from entranceto exit!\n"); elsePrintMaze(maze);// 打印路径printf("\n需要继续创建新的迷宫吗?(y/n): "); scanf("%s",&cmd);}while(cmd=='y' || cmd=='Y');}。
用栈求解迷宫问题所有路径及最短路径程序c语言

用栈求解迷宫问题所有路径及最短路径程序c语言
摘要:
1.迷宫问题的背景和意义
2.栈的基本概念和原理
3.用栈解决迷宫问题的方法
4.C 语言编程实现步骤
5.程序示例及运行结果
正文:
【1.迷宫问题的背景和意义】
迷宫问题是计算机科学中的一个经典问题,它涉及到图论、数据结构和算法等多个领域。
在迷宫问题中,给定一个有向图,目标是找到从起点到终点的所有路径以及最短路径。
这个问题在现实生活中也有很多应用,例如地图导航、物流路径规划等。
【2.栈的基本概念和原理】
栈是一种线性数据结构,它遵循后进先出(LIFO)的原则。
栈可以用来存储序列中的元素,也可以用来表示函数调用关系。
栈的操作通常包括入栈、出栈、获取栈顶元素等。
【3.用栈解决迷宫问题的方法】
为了解决迷宫问题,我们可以使用栈来记录遍历过程中的路径。
具体步骤如下:
1.创建一个栈,用于存储遍历过程中的路径;
2.从起点开始,将当前节点的编号入栈;
3.遍历当前节点的所有相邻节点,如果相邻节点未被访问过,则将其入栈;
4.当栈不为空时,继续执行步骤3;否则,说明已到达终点,开始回溯,找到最短路径;
5.从栈顶弹出节点,将其添加到结果路径列表中;
6.如果栈为空,说明没有找到从起点到终点的路径;否则,返回结果路径列表。
《数据结构》上机实验报告—利用栈实现迷宫求解

《数据结构》上机实验报告—利用栈实现迷宫求解实验目的:掌握栈的基本操作和迷宫求解的算法,并能够利用栈实现迷宫求解。
实验原理:迷宫求解是一个常见的路径问题,其中最常见的方法之一是采用栈来实现。
栈是一种先进后出的数据结构,适用于这种类型的问题。
实验步骤:1.创建一个迷宫对象,并初始化迷宫地图。
2.创建一个栈对象,用于存储待探索的路径。
3.将起点入栈。
4.循环执行以下步骤,直到找到一个通向终点的路径或栈为空:a)将栈顶元素出栈,并标记为已访问。
b)检查当前位置是否为终点,若是则路径已找到,结束。
c)检查当前位置的上、下、左、右四个方向的相邻位置,若未访问过且可以通行,则将其入栈。
5.若栈为空,则迷宫中不存在通向终点的路径。
实验结果:经过多次实验,发现利用栈实现迷宫求解的算法能够较快地找到一条通向终点的路径。
在实验中,迷宫的地图可通过一个二维数组表示,其中0表示可通行的路径,1表示墙壁。
实验结果显示,该算法能够正确地找出所有可行的路径,并找到最短路径。
实验结果还显示,该算法对于大型迷宫来说,解决速度相对较慢。
实验总结:通过本次实验,我掌握了利用栈实现迷宫求解的算法。
栈作为一种先进后出的数据结构,非常适合解决一些路径的问题。
通过实现迷宫求解算法,我深入了解了栈的基本操作,并学会运用栈来解决实际问题。
此外,我还了解到迷宫求解是一个复杂度较高的问题,对于大型迷宫来说,解决时间较长。
因此,在实际应用中需要权衡算法的速度和性能。
在今后的学习中,我将进一步加深对栈的理解,并掌握其他数据结构和算法。
我还将学习更多的路径算法,以便更好地解决迷宫类问题。
掌握这些知识将有助于我解决更加复杂的问题,并提升编程能力。
数据结构设计——用栈实现迷宫问题的求解

数据结构设计——⽤栈实现迷宫问题的求解求解迷宫问题1,问题描述以⼀个m*n的长⽅阵表⽰迷宫,0和1分别表⽰迷宫中的通路和障碍。
迷宫问题要求求出从⼊⼝(1,1)到出⼝(m,n)的⼀条通路,或得出没有通路的结论。
基本要求:⾸先实现⼀个以链表作存储结构的栈类型,然后编写⼀个求迷宫问题的⾮递归程序,求得的通路,其中:(i,j)指⽰迷宫中的⼀个坐标, d表⽰⾛到下⼀坐标的⽅向。
左上⾓(1,1)为⼊⼝,右下⾓(m,n)为出⼝。
2.设计思路: ⽤栈实现迷宫问题的求解;3.实验代码:栈实现迷宫求解问题:************************************************************************************************************1//maze_stack.cpp2 #include<stdio.h>3 #include<stdlib.h>4 #include<windows.h>5 #include"seqstack.h"67#define MAX_ROW 128#define MAX_COL 14910int maze[12][14] = {111, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1,121, 0, 1, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1,131, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 1,141, 0, 0, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1,151, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 1, 1,161, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 0, 1,171, 1, 0, 0, 1, 0, 1, 1, 0, 1, 0, 1, 1, 1,181, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1,191, 0, 0, 0, 0, 1 ,0 ,0, 0 ,0 ,1 ,0 ,1 ,1,201, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1,211, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1,221, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 123 };2425void print_line()26 {27 system("cls");28 printf("迷宫如下‘■’代表墙,数字或者‘☆’表⽰路径\n");29int i, j;30for (i = 0; i < MAX_ROW; i++){31for (j = 0; j < MAX_COL; j++)32if (maze[i][j] == 1) printf("■");33else if (maze[i][j] >= 3){34 printf("%2d", maze[i][j] - 2);35/*if (i == MAX_ROW-2 && j == MAX_COL-2) printf("★");36 else printf("☆");*/37 }38else printf("");39 printf("\n");40 }41 printf("已到达出⼝...\n");42 printf("可见使⽤栈求出的路径并⾮最优路径,根据我依次探索的⽅向不同,结果也将不同\n");43 }4445void visit(mark p,int sign, PSeqStack S)46 {47 Push_SeqStack(S,p);48switch (sign)49 {50case1: p.col++; Push_SeqStack(S, p); maze[p.row][p.col] = 2; break;//向右51case2: p.row++; Push_SeqStack(S, p); maze[p.row][p.col] = 2; break;//向下52case3: p.col--; Push_SeqStack(S, p); maze[p.row][p.col] = 2; break;//向左53case4: p.row--; Push_SeqStack(S, p); maze[p.row][p.col] = 2; break;//向上54 }55 }5657int main()59struct point p = { 1, 1 };60 maze[p.row][p.col] = 2;//遍历过的点设置为261 PSeqStack S = Init_SeqStack();62 Push_SeqStack(S,p);63while (!Empty_SeqStack(S))64 {65 Pop_SeqStack(S, &p);66if (p.row == MAX_ROW - 2 && p.col == MAX_COL - 2)67break;68if (p.col + 1 < MAX_COL - 1 && maze[p.row][p.col + 1] == 0)//向右69 {70 visit(p, 1, S);71continue;72 }73if (p.row + 1 < MAX_ROW - 1 && maze[p.row + 1][p.col] == 0)//向下74 {75 visit(p, 2, S);76continue;77 }78if (p.col - 1 >= 1 && maze[p.row][p.col - 1] == 0)//向左79 {80 visit(p, 3, S);81continue;82 }83if (p.row - 1 >= 1 && maze[p.row - 1][p.col] == 0)//向上84 {85 visit(p, 4, S);86continue;87 }//以上是对迷宫的四个⽅向进⾏操作88 }89if (p.row == MAX_ROW - 2 && p.col == MAX_COL - 2)//是否为出⼝90 {91int count = GetLength_SeqStack(S)+3;//为了与迷宫0,1,2的区别所以基数要以3开始92 printf("成功找到出⼝,路径倒序输出:\n");93 printf("(%d,%d)\n", p.row, p.col);94 maze[p.row][p.col] = count;95while (!Empty_SeqStack(S))//按照前驱进⾏查找96 {97 count--;98 Pop_SeqStack(S, &p);99 maze[p.row][p.col] = count;100 printf("(%d,%d)\n", p.row, p.col);101 }102 printf("3秒后打印路径......");103 Sleep(3000);104 print_line();105 }106else {107 printf("没有出路\n");108 }109 system("pause");110return0;111 }112//end maze_stack.cpp************************************************************************************************************* 1//seqstack.h2 #include<stdio.h>3 #include<stdlib.h>4#define MAXSIZE 10056 typedef struct point{7int row, col;8 }mark;910 typedef mark DataType;1112 typedef struct {13 DataType data[MAXSIZE];14int top;15 }SeqStack, * PSeqStack;1617 PSeqStack Init_SeqStack (void)18 {19 PSeqStack S;20 S = (PSeqStack)malloc(sizeof(SeqStack));21if (S)22 S->top = -1;23else24 exit(-1);25return S;26 }28int Empty_SeqStack(PSeqStack S)29 {30//return (S->top==-1);31if (S->top == -1)32return1;33else34return0;35 }3637int Push_SeqStack(PSeqStack S,DataType x)38 {39if (S->top == MAXSIZE - 1)40 {41 printf("栈满不能⼊栈\n");42return0;43 }44else45 {46 S->top++;47 S->data[S->top] = x;48return1;49 }50 }5152int Pop_SeqStack(PSeqStack S,DataType *x)53 {54if(Empty_SeqStack(S))55return0;56else57 {58 *x = S->data[S->top];59 S->top--;60return1;61 }62 }6364int GetTop_SeqStack(PSeqStack S ,DataType *x) 65 {66if(Empty_SeqStack(S))67return0;68else69 {70 *x = S->data[S->top];71return1;72 }73 }74int GetLength_SeqStack(PSeqStack S)75 {76return S->top + 1;77 }7879void Distory_SeqStack(PSeqStack *S)80 {81if(*S)82free(*S);83 *S = NULL;84 }//end seqstack.h4.运⾏结果:栈求解迷宫:。
栈在迷宫求解问题中的应用
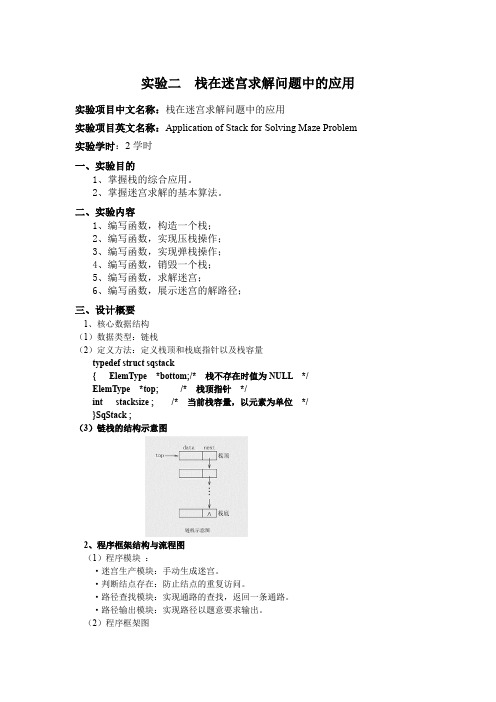
a=(SNode*)malloc(sizeof(SNode));
while(S->top!=NULL)
{
a=S->top;
S->top=S->top->next;
free(a);
}
return 0;
}
Statu readmaze(char map[SIZE][SIZE], int *row, int *col){
{
if(mark[path->top->next->data.seat.row-1][path->top->next->data.seat.col]==0||mark[path->top->next->data.seat.row][path->top->next->data.seat.col+1]==0||mark[path->top->next->data.seat.row+1][path->top->next->data.seat.col]==0||mark[path->top->next->data.seat.row][path->top->next->data.seat.col-1]==0)
a->data.seat.col=path->top->next->data.seat.col-1;
int stacksize ; /*当前栈容量,以元素为单位*/
}SqStack ;
(3)链栈的结构示意图
2、程序框架结构与流程图
(1)程序模块:
·迷宫生产模块:手动生成迷宫。
迷宫求解数据结构C语言版
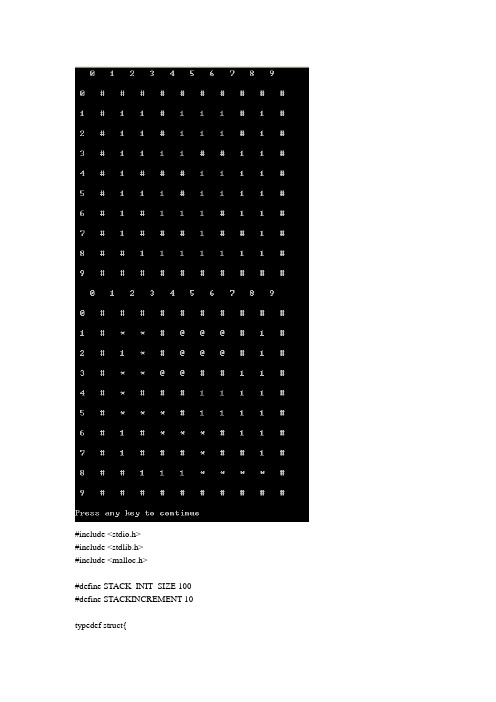
#include <stdio.h>#include <stdlib.h>#include <malloc.h>#define STACK_INIT_SIZE 100 #define STACKINCREMENT 10typedef struct{int r;int c;}PosType;//通道块坐标typedef struct {int ord;//通道块在路径上的序号PosType seat;//通道块的坐标位置int di;//下一个探索的方向}SelemType;typedef struct {int r;int c;char adr[10][10];}MazeType;//迷宫行列,内容typedef struct {SelemType * base;SelemType * top;int stacksize;}SqStack;void InitStack(SqStack &S){S.base=(SelemType*)malloc(STACK_INIT_SIZE * sizeof(SelemType));if(!S.base) exit(0);S.top=S.base;S.stacksize=STACK_INIT_SIZE;}void Push(SqStack &S,SelemType &e){if((S.top-S.base)>=S.stacksize){S.base=(SelemType*)realloc(S.base,(S.stacksize+STACKINCREMENT)* sizeof(SelemType));if(!S.base) exit(0);S.top=S.base+S.stacksize;//溢出重新定位S.stacksize+=STACKINCREMENT;}*S.top++=e;}void Pop(SqStack &S,SelemType &e){if(S.top==S.base) return;e=*--S.top;}void InitMaze(MazeType &maze){int i,j;maze.r=8;maze.c=8;for(i=0;i<10;i++){maze.adr[0][i]='#';maze.adr[9][i]='#';}for(i=0;i<10;i++){maze.adr[i][0]='#';maze.adr[i][9]='#';}for(i=1;i<9;i++)for(j=1;j<9;j++)maze.adr[i][j]='1';maze.adr[1][3]='#'; maze.adr[1][7]='#';maze.adr[2][3]='#'; maze.adr[2][7]='#';maze.adr[3][5]='#'; maze.adr[3][6]='#';maze.adr[4][2]='#'; maze.adr[4][3]='#';maze.adr[4][4]='#';maze.adr[5][4]='#';maze.adr[6][2]='#'; maze.adr[6][6]='#';maze.adr[7][2]='#'; maze.adr[7][3]='#'; maze.adr[7][4]='#'; maze.adr[7][6]='#';maze.adr[7][7]='#';maze.adr[8][1]='#';}int Pass(MazeType &maze,PosType &curpos){if(maze.adr[curpos.r][curpos.c]=='1')return 1;elsereturn 0;}void FootPrint(MazeType &maze,PosType curpos){maze.adr[curpos.r][curpos.c]='*';//已经走过的标记}PosType NextPos(PosType curpos,int i){PosType nextpos;nextpos=curpos;switch(i){case 1:nextpos.c+=1;break;case 2:nextpos.r+=1;break;case 3:nextpos.c-=1;break;case 4:nextpos.r-=1;break;default:printf("探寻方向错误!");}return nextpos;}void MarkPrint(MazeType &maze,PosType curpos){maze.adr[curpos.r][curpos.c]='@';//不通的标识}int StackEmpty(SqStack &S){if(S.base==S.top)return 1;elsereturn 0;}void MazePath(MazeType &maze,PosType start,PosType end) {SelemType e;SqStack S;InitStack(S);PosType curpos;int curstep;curpos=start;curstep=1;do{if(Pass(maze,curpos)){FootPrint(maze,curpos);//留下走过足迹*e.ord=curstep;e.seat=curpos;e.di=1;Push(S,e);//压入栈路径if(curpos.r==end.r&&curpos.c==end.c) return;curpos=NextPos(curpos,1);//探索下一步curstep++;}//ifelse{//当前位置不能通过if(!StackEmpty(S)){Pop(S,e);//top指针退后一下,指向上一步while(e.di==4&&!StackEmpty(S)){MarkPrint(maze,e.seat);//如果此步上下左右都探索过了且栈不为空留下不通过且返回一步Pop(S,e);}//while,用while是可以连续返回if(e.di<4){e.di++;Push(S,e);//换下一个方向探索curpos=NextPos(e.seat,e.di);//探索下一位置块}//if}//if}//else}while(!StackEmpty(S));}//mazepathvoid PrintMaze(MazeType &maze){int i,j;for(i=0;i<10;i++)printf("%4d",i);//输出行printf("\n\n");for(i=0;i<10;i++){printf("%2d",i);//输出列for(j=0;j<10;j++)printf("%4c",maze.adr[i][j]);printf("\n\n");}}void main(){MazeType maze;PosType start,end;InitMaze(maze);start.r=1; start.c=1;end.r=8; end.c=8;PrintMaze(maze);MazePath(maze,start,end);PrintMaze(maze);}。
C语言数据结构中求解迷宫问题实现方法

C语⾔数据结构中求解迷宫问题实现⽅法C语⾔数据结构中求解迷宫问题实现⽅法在学习数据结构栈的这⼀节遇到了求迷宫这个问题,拿来分享⼀下~⾸先求迷宫问题通常⽤的是“穷举求解” 即从⼊⼝出发,顺某⼀⽅向试探,若能⾛通,则继续往前⾛,否则原路返回,换另⼀个⽅向继续试探,直⾄⾛出去。
我们可以先建⽴⼀个8*8的迷宫其中最外侧为1的是墙int mg[M+2][N+2]={{1,1,1,1,1,1,1,1,1,1},{1,0,0,1,0,0,0,1,0,1},{1,0,0,1,0,0,0,1,0,1},{1,0,0,0,0,1,1,0,0,1},{1,0,1,1,1,0,0,0,0,1},{1,0,0,0,1,0,0,0,0,1},{1,0,1,0,0,0,1,0,0,1},{1,0,1,1,1,0,1,1,0,1},{1,1,0,0,0,0,0,0,0,1},{1,1,1,1,1,1,1,1,1,1},}如上所⽰,0对应通道⽅块,1代表墙。
对于迷宫中的每个⽅块,有上下左右4个⽅块相邻,我们规定第i⾏第j列⽅块的位置为(i,j)规定上⽅⽅块⽅位为0,顺时针⽅向递增编号。
(i,j)上⽅的即为(i-1,j),下⽅(i+1,j),左⽅(i,j-1),右⽅(i,j+1). 为了⽅⾯回溯,我们需要有进栈出栈操作,所以我们来定义:struct {int i;//当前⽅位⾏int j;//当前⽅位列int di;//下⼀个可⾛⽅位号}St[MaxSize];//栈int top=-1;//初始化栈顶指针我们来看看⽂字过程~~⾸先将⼊⼝进栈(初始⽅位为-1),在栈不空的情况下循环:取栈顶⽅块(不退栈),若该⽅块是出⼝,则退栈。
若存在这样的⽅块,则将其⽅位保存到栈顶元素中,并将这个可⾛的相邻⽅块进栈。
对应的算法:void mgpath(int x1,int y1,int x2,int y2){int i.j,di,find,k;top++;St[top].i=x1; St[top].j=y1; St[top].di=-1; mg[x1][y1]=-1;while (top>-1){i=St[top].i; j=St[top].j; di=St[top].di;if (i==x2 && j==y2){printf("迷宫路径如下:\n");for (k=0;k<=top;k++){printf("\t(%d,%d)",St[k].i,S[k].j);if ((k+1)%5==0) printf("\n"); //输出5个换⼀⾏}printf("\n"); //找到⼀条路径后结束return ;}find=0;while (di<4 && find==0){di++;switch(di){case 0: i=St[top].i-1; j=S[top].j;break;case 1: i=St[top].i; j=St[top].j+1;break;case 2: i=St[top].i+1;j=St[top].j;break;case 3: i=St[top].i; j=St[top].j-1;break;}if(mg[i] [j]==0) find=1;}if (find==1){ //找到了下⼀个可⾛⽅块St[top].di=di;//修改原栈顶的值top++; //下⼀个可⾛⽅块进栈St [top].i=i; St[top].j=j;St[top].di=-1;mg[i] [j]=-1;//避免重复⾛到该⽅块}else{ //没有路径可⾛,进⾏退栈操作mg[St[top].i] [St[top].j]=0;//让该位置变为其他路径的可⾛⽅块top--;}}printf("没有路径可⾛!\n");}当然我们也可以⽤队列去求该迷宫的最优算法,这只是⼀个⽤来理解栈的例⼦~~~感谢阅读,希望能帮助到⼤家,谢谢⼤家对本站的⽀持!。
利用栈实现迷宫求解

利用栈实现迷宫的求解
一、要解决的四个问题:
1、表示迷宫的数据结构:
设迷宫为 m 行 n 列,利用 maze[m][n]来表示一个迷宫,maze[i][j]=0 或 1; 其中:0 表示 通路,1 表示不通,当从某点向下试探时,中间点有 4 个方向可以试探,(见图)而四个角 点有 2 个方向,其它边缘点有 3 个方向,为使问题简单化我们用 maze[m+2][n+2]来表示迷 宫,而迷宫的四周的值全部为 1。这样做使问题简单了,每个点的试探方向全部为 4,不用 再判断当前点的试探方向有几个,同时与迷宫周围是墙壁这一实际问题相一致。
Push_SeqStack ( s, temp ) ; x=i ; y=j ; maze[x][y]= -1 ; if (x= =m&&y= =n) return 1 ; /*迷宫有路*/ else d=0 ; } else d++ ; } /*while (d<4)*/ } /*while (! Empty_SeqStack (s ) )*/ return 0 ;/*迷宫无路*/ } 栈中保存的就是一条迷宫的通路。
素有两个域组成,x:横坐标增量,y:纵坐标增量。Move 数组如图 3 所示。 move 数组定义如下: typedef struct { int x ; //行 int y ; //列 } item ; item move[4] ; 这样对 move 的设计会很方便地求出从某点 (x,y) 按某一方法思想如下:
(1) 栈初始化; (2) 将入口点坐标及到达该点的方向(设为-1)入栈 (3) while (栈不空)
数据结构编程迷宫(用栈的方法解决)

#include <stdio.h>#define M 4 //定义行数为4#define N 4 //定义列数为4#define MaxSize 100 //定义栈最多元素个数int mg[M+2][N+2]= //迷宫的四周要加上均为1的外框{{1,1,1,1,1,1},{1,0,0,0,1,1},{1,0,1,0,0,1},{1,0,0,0,1,1},{1,1,0,0,0,1},{1,1,1,1,1,1}};struct{int i; //当前方块的行号int j; //当前方块的列号int di; //di是下一个可走相邻方位的方位号} Stack[MaxSize],Path[MaxSize]; //定义栈和存放最短路径的数组int top=-1; //初始化栈顶int count=1; //路径数计数int min=MaxSize; //最短路径长度计数void mgpath() //求解路径为:(1,1)->(M,N){int i,j,k,di,find;top++; //初始方块进入栈口Stack[top].i=1;Stack[top].j=1;Stack[top].di=-1;mg[1][1]=-1;while (top>-1) //栈不空时循环{i=Stack[top].i;j=Stack[top].j;di=Stack[top].di; //取栈顶方块if (i==M && j==N) //找到了出口,输出路径{printf("%4d:",count++);for (k=0;k<=top;k++){printf("(%d,%d)",Stack[k].i,Stack[k].j);if ((k+1)%5==0)printf("\n"); //每输出5个方块后换一行}printf("\n");if (top+1<min) //找最短路径{for (k=0;k<=top;k++)Path[k]=Stack[k];min=top+1;}mg[Stack[top].i][Stack[top].j]=0; //让该位置变为其他路径可走方块top--;i=Stack[top].i;j=Stack[top].j;di=Stack[top].di;}find=0;while (di<4 && find==0) //找(i,j)方块下一个可走方块{ di++;switch(di){case 0:i=Stack[top].i-1;j=Stack[top].j;break;case 1:i=Stack[top].i;j=Stack[top].j+1;break;case 2:i=Stack[top].i+1;j=Stack[top].j;break;case 3:i=Stack[top].i,j=Stack[top].j-1;break;}if (mg[i][j]==0) find=1;}if (find==1) //找到了一个可走的相邻方块{ Stack[top].di=di; //修改原栈顶元素的di值top++; //将可走相邻方块进栈Stack[top].i=i;Stack[top].j=j;Stack[top].di=-1;mg[i][j]=-1; //避免重复走到该方块,将其置为-1}else //没有相邻方块可走,则退栈{mg[Stack[top].i][Stack[top].j]=0; //让该位置变为其他路径可走方块top--;}}printf("最短路径如下:\n");printf("长度:%d\n",min);printf("路径:");for (k=0;k<min;k++){printf("(%d,%d)",Path[k].i,Path[k].j);if ((k+1)%5==0) printf("\n"); //每输出5个方块换一行}printf("\n");}void main(){printf("迷宫所有路径如下:\n");mgpath();}。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
#include <stdio.h>#include <stdlib.h>#include <string.h>#define STACK_INIT_SIZE 100#define INCREMENT 10typedef struct{int r;int c;}zuobiao;typedef struct{int ord; //在当前坐标上的“标号”zuobiao seat; //坐标int di; //走向下一通道的方向}lujing;typedef struct{int sz[10][10];}Maze;typedef struct SqStack{lujing *base;lujing *top;int size;}SqStack;int initStack(SqStack *s){s->base = (lujing *)malloc(STACK_INIT_SIZE*sizeof(lujing) );if(!s->base)return -1;s->top = s->base;s->size = STACK_INIT_SIZE;return 0;}int push(SqStack *s, lujing e){if(s->top - s->base >= s->size){s->base = (lujing *)realloc(s->base, (s->size+INCREMENT)*sizeof(lujing));if(!s->base)return -1;s->top = s->base+s->size;s->size += INCREMENT;}*s->top++ = e;return 0;}int pop(SqStack *s,lujing *e){if(s->top == s->base)return -1;*e = *(--s->top);return 0;}int isEmpty(SqStack *s){if(s->base == s->top)return 1;elsereturn 0;}int pass( Maze maze,zuobiao dqzb) {if (maze.sz[dqzb.r][dqzb.c]==1)return 1; // 如果当前位置是可以通过,返回1else return 0; // 否则返回0}void footPrint(Maze &maze,zuobiao dqzb) {maze.sz[dqzb.r][dqzb.c]=9;}void markPrint(Maze &maze,zuobiao dqzb) {maze.sz[dqzb.r][dqzb.c]=4;}zuobiao nextPos(zuobiao dqzb, int Dir) {zuobiao xzb;switch (Dir) {case 1:xzb.r=dqzb.r;xzb.c=dqzb.c+1;break;case 2:xzb.r=dqzb.r+1;xzb.c=dqzb.c;break;case 3:xzb.r=dqzb.r;xzb.c=dqzb.c-1;break;case 4:xzb.r=dqzb.r-1;xzb.c=dqzb.c;break;}return xzb;}int mazePath(Maze &maze, zuobiao start, zuobiao end){SqStack *s = (SqStack *)malloc(sizeof(SqStack));initStack(s);zuobiao dqzb = start; // 设定"当前位置"为"入口位置"lujing e;int curstep = 1; // 探索第一步do {if (pass(maze,dqzb)) { // 当前位置可通过,即是未曾走到过的通道块footPrint(maze,dqzb); // 留下足迹e.di =1;e.ord = curstep;e.seat= dqzb;push(s,e); // 加入路径if (dqzb.r == end.r && dqzb.c==end.c)return 0; // 到达终点(出口)dqzb = nextPos(dqzb, 1); // 下一位置是当前位置的东邻curstep++; // 探索下一步} else { // 当前位置不能通过if (!isEmpty(s)) {pop(s,&e);while (e.di==4 && !isEmpty(s)) {markPrint(maze,e.seat);pop(s,&e); // 留下不能通过的标记,并退回一步}if (e.di<4) {e.di++; // 换下一个方向探索push(s, e);dqzb = nextPos(e.seat, e.di); // 当前位置设为新方向的相邻块}}}} while (!isEmpty(s) );return -1;}void main(){printf(" *----Δ迷宫求解Δ----*\n\n");printf("---迷宫如下所示:(说明:0表示墙;1表示可以通过)");printf("\n");Maze maze; //声明mazemaze.sz[0][0]= 0;maze.sz[0][1]= 0;maze.sz[0][2]= 0;maze.sz[0][3]= 0;maze.sz[0][4]= 0;maze.sz[0][5]= 0;maze.sz[0][6]= 0;maze.sz[0][7]= 0;maze.sz[0][8]= 0;maze.sz[0][9]= 0;maze.sz[1][0]= 0;maze.sz[1][1]= 1;maze.sz[1][2]= 1;maze.sz[1][3]= 0;maze.sz[1][4]= 1;maze.sz[1][5]= 1;maze.sz[1][6]= 1;maze.sz[1][7]= 0;maze.sz[1][8]= 1;maze.sz[1][9]= 0;maze.sz[2][0]= 0;maze.sz[2][1]= 1;maze.sz[2][2]= 1;maze.sz[2][3]= 0;maze.sz[2][4]= 1;maze.sz[2][5]= 1;maze.sz[2][6]= 1;maze.sz[2][7]= 0;maze.sz[2][8]= 1;maze.sz[2][9]= 0;maze.sz[3][0]= 0;maze.sz[3][1]= 1;maze.sz[3][2]= 1;maze.sz[3][3]= 1;maze.sz[3][4]= 1;maze.sz[3][5]= 0;maze.sz[3][6]= 0;maze.sz[3][7]= 1;maze.sz[3][8]= 1;maze.sz[3][9]= 0;maze.sz[4][0]= 0;maze.sz[4][1]= 1;maze.sz[4][2]= 0;maze.sz[4][3]= 0;maze.sz[4][4]= 0;maze.sz[4][5]= 1;maze.sz[4][6]= 1;maze.sz[4][7]= 1;maze.sz[4][8]= 1;maze.sz[4][9]= 0;maze.sz[5][0]= 0;maze.sz[5][1]= 1;maze.sz[5][2]= 1;maze.sz[5][3]= 1;maze.sz[5][4]= 0;maze.sz[5][5]= 1;maze.sz[5][6]= 1;maze.sz[5][7]= 0;maze.sz[5][8]= 1;maze.sz[5][9]= 0;maze.sz[6][0]= 0;maze.sz[6][1]= 1;maze.sz[6][2]= 0;maze.sz[6][3]= 1;maze.sz[6][4]= 1;maze.sz[6][5]= 1;maze.sz[6][6]= 0;maze.sz[6][7]= 1;maze.sz[6][8]= 1;maze.sz[6][9]= 0;maze.sz[7][0]= 0;maze.sz[7][1]= 1;maze.sz[7][2]= 0;maze.sz[7][3]= 0;maze.sz[7][4]= 0;maze.sz[7][5]= 1;maze.sz[7][6]= 0;maze.sz[7][7]= 0;maze.sz[7][8]= 1;maze.sz[7][9]= 0;maze.sz[8][0]= 0;maze.sz[8][1]= 0;maze.sz[8][2]= 1;maze.sz[8][3]= 1;maze.sz[8][4]= 1;maze.sz[8][5]= 1;maze.sz[8][6]= 1;maze.sz[8][7]= 1;maze.sz[8][8]= 1;maze.sz[8][9]= 0;maze.sz[9][0]= 0;maze.sz[9][1]= 0;maze.sz[9][2]= 0;maze.sz[9][3]= 0;maze.sz[9][4]= 0;maze.sz[9][5]= 0;maze.sz[9][6]= 0;maze.sz[9][7]= 0;maze.sz[9][8]= 0;maze.sz[9][9]= 0;int i,j,sum;for (i = 0; i < 10; i++) {for (j = 0; j < 10; j++){printf("%4d",maze.sz[i][j]);sum++;}if(sum%10==0)printf("\n");}printf("\n");printf("---请输入1求解路径图:");int a;scanf("%d",&a);if(a==1){zuobiao rukou,chukou;rukou.r=1;rukou.c=1;chukou.r=8;chukou.c=8;mazePath(maze,rukou,chukou);printf("\n");printf("---图解如下所示:(说明:0表示墙;1表示可以通过;4表示走不通;9表示所走的路径)\n");for (i = 0; i < 10; i++) {for (j = 0; j < 10; j++){printf("%4d",maze.sz[i][j]);sum++;}if(sum%10==0)printf("\n");}}}。