实验二 继承与接口实验
继承与接口实验报告

继承与接口实验报告
实验目的:掌握Java中继承与接口的基本概念和使用方法,能够灵活运用继承和接口设计类和实现多态。
实验环境:Java语言编译器和JDK环境。
实验步骤:
1. 创建一个父类Animal,包含属性name和age,以及方法eat()和sleep()。
2. 创建两个子类Cat和Dog,继承自Animal类。
在子类中重写父类的方法,并添加特有的方法和属性。
3. 创建一个接口Jumpable,包含方法jump()。
4. 在Cat类中实现Jumpable接口,使其具有跳跃的能力。
5. 创建一个测试类Test,通过实例化Cat和Dog对象,调用它们的方法进行测试。
实验结果:
1. 父类Animal成功创建,子类Cat和Dog成功继承父类,并添加特有的方法和属性。
2. 接口Jumpable成功创建,并被Cat类实现。
3. 测试类Test成功实例化Cat和Dog对象,并调用它们的方法进行测试。
实验结论:
1. 继承是Java中实现代码重用的重要手段,通过继承可以快速创建具有相似特性的子类。
2. 接口是Java中实现多态的重要手段,通过接口可以使类具有更多的行为特性。
3. 在实际开发中,应根据需求灵活运用继承和接口,设计出合理的类结构和实现多态的方式。
java实验报告——继承与接口
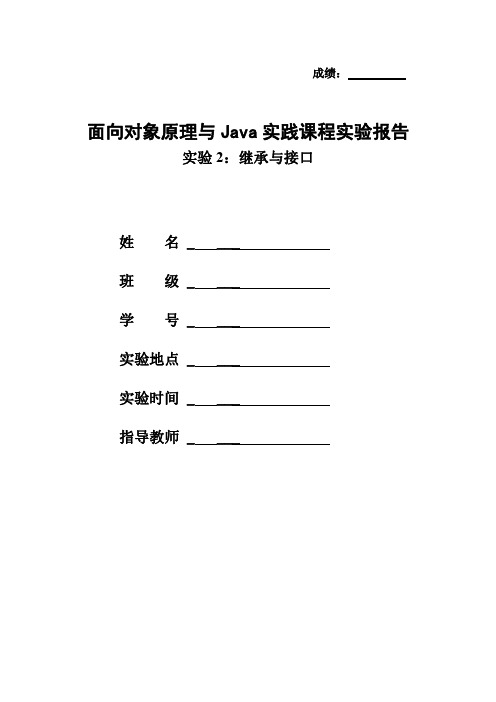
System.out.print('\t'+"平均分");
System.out.println('\t'+"成绩等级");
for(int i=0; i<pg.length;i++) {
System.out.print(pg[i].Name); System.out.print('\t'+pg[i].Stu_type); System.out.print('\t'+String.valueOf(pg[i].C_score)); System.out.print('\t'+String.valueOf(pg[i].English_score)); System.out.print('\t'+String.valueOf(pg[i].Java_score)); System.out.print('\t'+String.valueOf(pg[i].score)); System.out.println('\t'+pg[i].sco_Level); } System.out.println(); } }
String Name=""; String Stu_type=""; int C_score; int English_score; int Java_score; int score; // String sco_Level=""; Sco_Level sco_Level;
public Student(String name,String stu_type,int sco1,int sco2,int sco3) {
实验2(08) 继承

班级:姓名:学号:成绩实验二Java面向对象技术——必做实验目的:1.理解继承的概念2.掌握继承的实现3.理解继承中的覆盖现象4.理解抽象类的概念,掌握定义抽象类的方法和应用5.掌握接口的定义和实现接口的方法实验数据记录及分析(或程序及运行结果)1.按照要求完成程序:1)第一个类是图形类(Shape),含有一个成员变量color(字符串类型),一个没有参数的构造方法,以及一个有一个字符串类型参数的构造方法来初始化颜色变量,还有一个返回颜色变量值的成员方法getColor,一个toString()方法,以及一个抽象方法getArea获取面积,返回值为double类型;2)第二个类是圆形类(Circle)继承自图形类,含有一个成员变量半径r,有一个有两个参数的构造方法,来初始化颜色和半径,一个toString()方法,一个方法getArea,返回值为double,获取圆的面积值。
3)第三个类是矩形类(Rectangle)继承自图形,含有两个double类型的成员变量长a和宽b,有一个有三个参数的构造方法,来初始化颜色、长和宽,一个toString()方法,一个方法getArea,返回值为double,获取矩形的面积值。
、4)第四个类是测试类(TestShape),声明一个图形引用变量,分别指向圆形类和矩形类的实例对象,并用toString方法和getArea方法来测试方法的“多态性”(由动态绑定引起)。
2.根据要求完成程序:1)定义名称为PCI的接口,包括启动的方法start和关闭的方法stop;2)定义名称为NetworkCard 的类表示网卡,实现PCI接口,并且其在实现start方法时输出“sending data……”,在实现stop方法时输出“network stop.”3)定义名称为SoundCard 的类表示声卡,实现PCI接口;并且其在实现start方法时输出“dudu……”,在实现stop方法时输出“sound stop.”4)定义名称为MainBoard 的类表示主板,包含方法public voidusePCICard(PCI p),在方法体内通过p来启动和关闭组件5)定义一个包含main方法的Test类,在main方法内创建一个MainBoard 对象用mb来引用,创建一个NetworkCard对象用nc来引用,创建一个SoundCard对象用sc来引用,分别通过mb来使用usePCICard(PCI p)启动声卡和网卡。
最新实验二继承机制实验报告

最新实验二继承机制实验报告实验目的:本实验旨在探究二继承机制在面向对象编程中的应用和实现,通过具体的编程实践,理解多重继承的概念,掌握如何在现代编程语言中实现二继承,并分析其优缺点以及可能遇到的问题。
实验环境:- 编程语言:Python 3.8- 开发环境:PyCharm 2021- 操作系统:Windows 10实验内容:1. 设计一个基本的类层次结构,包含两个父类和一个子类,子类需要从两个父类继承属性和方法。
2. 实现二继承,确保子类能够访问和重写父类的属性和方法。
3. 通过实例化子类,测试继承后的属性和方法的正确性。
4. 分析二继承在代码重用和系统设计中的优势和潜在的问题。
实验步骤:1. 定义两个父类`ClassA`和`ClassB`,分别具有不同的属性和方法。
2. 创建一个子类`ClassC`,继承自`ClassA`和`ClassB`。
3. 在`ClassC`中重写父类的方法,以展示多态性。
4. 编写测试代码,创建`ClassC`的实例,并调用其继承和重写的方法。
5. 记录实验结果,并对比预期和实际输出。
实验结果:- `ClassC`成功继承了`ClassA`和`ClassB`的属性和方法。
- 重写的方法在`ClassC`的实例中按预期工作,展示了多态性。
- 测试代码中所有的调用均返回了正确的结果。
实验分析:二继承机制允许子类继承多个父类的属性和方法,这在某些情况下可以提高代码的复用性,使得类的设计更加灵活。
然而,它也可能导致一些问题,如菱形继承问题,其中两个父类继承自一个共同的基类,可能会导致歧义和初始化顺序的复杂性。
此外,多重继承也可能使得类的继承关系变得复杂,难以理解和维护。
结论:通过本次实验,我们成功实现了二继承机制,并通过实际测试验证了其功能。
二继承提供了一种有效的方式来组合多个类的属性和行为,但开发者在使用时需要注意避免继承结构过于复杂,以及可能出现的菱形继承问题。
在设计类时,应当权衡二继承带来的便利性和可能引入的复杂性。
继承与接口实验
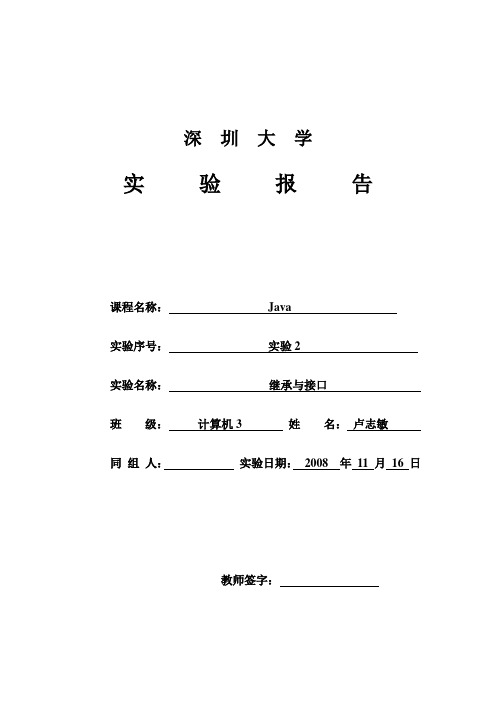
}
public void chinaGongfu() {
System.out.println("坐如钟,站如松,睡如弓");
}
}
class AmericanPeople extends People {
public void speakHello(){
BeijingPeople是ChinaPelple的子类,新增public void beijingOpera()方法。要求ChinaPeople重写父类的public void speakHello()、public void averageHeight()和public void averageWeight()方法。
System.out.println("American Average weight: 80.23 kg");
}
public void americanBoxing() {
System.out.println("直拳钩拳");
}
}
class BeijingPeople extends ChinaPeople {
System.out.println("How do you do");
}
public void averageHeight(){
System.out.println("American Average height: 188.0 cm");
}
public void averageWeight(){
public void speakHello(){
java实验报告——继承与接口
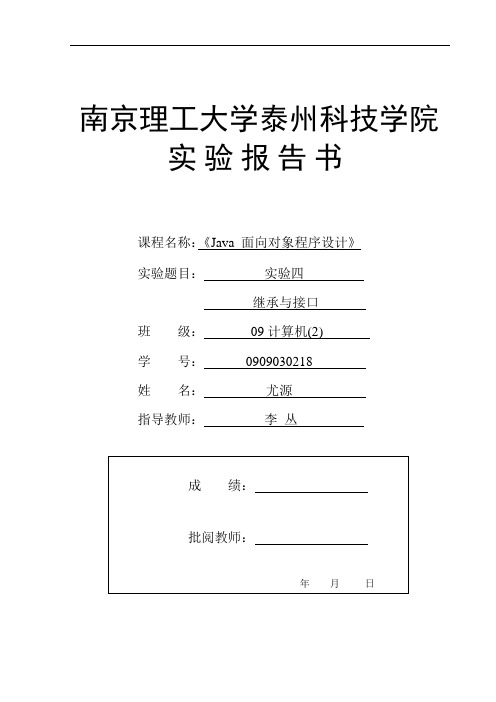
南京理工大学泰州科技学院实验报告书课程名称:《Java 面向对象程序设计》实验题目:实验四继承与接口班级:09计算机(2)学号:0909030218姓名:尤源指导教师:李丛一、实验目的1.掌握Java语言的类的继承的概念。
2.掌握Java语言中抽象类的使用。
3.掌握Java语言中接口的使用4.掌握eclipse集成开发环境的使用。
二、实验内容1.类的继承,具体要求如下:(1)定义一Person类,该类具有属性人名、年龄、身份证号等信息以及将属性信息作为字符串返回的一方法;(2)定义一Student类,让该类继承Person类,该类除了具有属性人名、年龄、身份证号等信息以外还有学号,所在学校等信息;该类也具有将属性信息作为字符串返回的一方法;(3)编写测试类,测试这两个类2.定义一个动物抽象类Animal,该类有一个抽象的方法cry();定义一个小猫类Cat,该类继承了Animal类并实现了cry()方法,当调用cry()方法时打印“小猫喵喵叫”,定义一个小狗类Dog,该类也继承了Animal类并实现了cry()方法,当调用cry()方法时打印“小狗汪汪叫”。
3. 接口的运用。
定义一接口接口名叫Usb,该接口声明了两个方法分别为start()和stop()方法,定义一U 盘类UsbDiskWriter,一照相机类Camera、一手机类Mobile,让它们都实现该接口。
三、实验步骤实验(1)编写代码实验(2)编写代码实验(3)编写代码四、实验结果实验(1)运行结果实验(2)运行结果实验(3)运行结果五、结果分析1. 子类若想调用父类的构造函数必须要用super关键字。
2.接口体中只能运用抽象类。
3.在同一个java文件中只能在入口函数的类中用public。
实验二---继承与接口实验
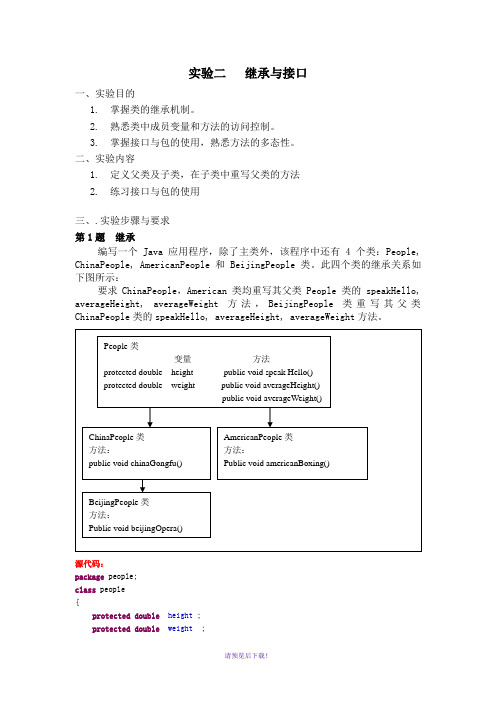
实验二继承与接口一、实验目的1.掌握类的继承机制。
2.熟悉类中成员变量和方法的访问控制。
3.掌握接口与包的使用,熟悉方法的多态性。
二、实验内容1.定义父类及子类,在子类中重写父类的方法2.练习接口与包的使用三、.实验步骤与要求第1题继承编写一个Java应用程序,除了主类外,该程序中还有4个类:People, ChinaPeople, AmericanPeople 和 BeijingPeople类。
此四个类的继承关系如下图所示:要求ChinaPeople,American类均重写其父类People类的speakHello, averageHeight, averageWeight方法,BeijingPeople类重写其父类ChinaPeople类的speakHello, averageHeight, averageWeight方法。
源代码:package people;class people{protected double height ;protected double weight ;public void speakHello() //问候语的函数{System.out.println("hello");}public void averageHeight()//人们的平均身高{height=170;System.out.println(+height);}public void averageWeight()//人们的平均体重{ weight=120;System.out.println(+weight);}}class Chinapeople extends people{public void speakHello(){System.out.println("你好");}public void averageHeight(){height=172;System.out.println(+height);}public void averageWeight(){ weight=115;System.out.println(+weight);}public void chinaGongfu()//中国功夫的方法{System.out.println("中国功夫");}}class Americanpeople extends people{public void speakHello(){System.out.println("hello");}public void averageHeight(){height=180;System.out.println(+height);}public void averageWeight(){ weight=150;System.out.println(+weight);}public void americanBoxing()//美国拳击的方法{System.out.println("americanBoxing");}}class Beijingpeople extends Chinapeople{public void speakHello(){System.out.println("北京欢迎你");}public void averageHeight(){height=168;System.out.println(+height);}public void averageWeight(){ weight=125;System.out.println(+weight);}}class Example{public static void main(String []args){people p =new people();Chinapeople c=new Chinapeople();Americanpeople a=new Americanpeople(); Beijingpeople b=new Beijingpeople();p.averageHeight();p.averageWeight();p.speakHello();c.averageHeight();c.averageWeight();c.chinaGongfu();c.speakHello();a.averageHeight();a.averageWeight();a.americanBoxing();a.speakHello();b.averageHeight();b.averageWeight();b.speakHello();}}结果截图:第2题上转型对象要求有一个abstract类,类名为Employee。
02实验二--继承和接口报告模板 (1)

publicbooleanisFilled() {
returnfilled;
}
/** Set a new filled */
publicvoidsetFilled(booleanfilled) {
this.filled= filled;
publicString getColor() {
returncolor;
}
/** Set a new color */
publicvoidsetColor(String color) {
this.color= color;
}
/** Return filled. Since filled is boolean,
2.用Eclipse工具编辑、编译、执行Java程序。
3.程序编写尽量规范化。在程序中添加适当的注释;类的命名、Field的命名、方法的命名应符合命名规则。
4.每个类都包含无参和有参的构造器。子类的构造器调用父类的构造器
实验内容
实验内容:
1.编写类之间具有继承关系的程序。
2.编写有抽象类和一般类的程序。
重写toString()方法返回三角形的字符串描述,返回值如:Triangle:side1=1.0,side2=2.0,side3=2.1
实验要求:
提供Triangle类的UML设计
实现该类的Java代码实现Triangle.java,并编写一个测试程序TestTriangle.java,在测试程序中创建一个Triangle对象,其边长分别为1.0,1.5和1.0,颜色为yellow,filled为true,然后显示其面积、周长、颜色以及是否被填充。
java 继承与接口 实验报告

应用数学学院专业1 班、学号_ _姓名___ __ 教师评定_________________实验题目继承与接口一、实验目的与要求实验目的:1、掌握类的继承关系。
2、掌握接口的定义与使用。
实验要求:按下列要求编写Java程序:1、定义接口Printx,其中包括一个方法printMyWay(),这个方法没有形参,返回值为空。
2、编写矩形类,矩形类要求实现Printx接口,有求面积、求周长的方法,printMyWay()方法要能显示矩形的边长、面积和周长。
3、编写正方形类作为矩形类的子类,正方形类继承了矩形类求面积和周长的方法,新增加求对角线长的方法,重写printMyWay()方法,要求该方法能显示正方形的边长、面积、周长和对角线长。
二、实验方案编辑该实验源程序如下:■LISTEN.javainterface Printx{ //定义接口public abstract void printMyWay();}class Rectangle implements Printx{ //矩形类double a,b;Rectangle(double a,double b){this.a=a;this.b=b;}public double getArea(){return a*b;}public double getLength(){return (a+b)*2.0;}public void printMyWay(){System.out.println("该矩形的长a="+a+"宽b="+b);System.out.println("面积s="+getArea());System.out.println("周长l="+getLength());}}class Square extends Rectangle{ //正方形类double c;Square(double a,double b){super(a,b);}public double getDiagonal(){c=Math.sqrt(a*a+b*b);return c;}public void printMyWay(){System.out.println("该矩形的长a="+a+"宽b="+b);System.out.println("面积s="+getArea());System.out.println("周长l="+getLength());System.out.println("对角线d="+getDiagonal());}}public class LISTEN {public static void main(String[] args) {Rectangle rectangle;Square square;rectangle=new Rectangle(11,5);square=new Square(5,5);rectangle.printMyWay();square.printMyWay();}}三、实验结果和数据处理运行上述源程序,可得如下运行结果:1、矩形长a=11 宽b=52、矩形长a=5 宽b=5四、结论接口是Java实现部分多继承功能的体现。
实训-继承与接口2

实训继承与接口2一、实训目标理解对象的上转型对象,以及多态的目的和意义,掌握多态的常用形式。
理解抽象类的意义和实际应用的一般模式,熟练掌握抽象类和抽象方法的定义以及抽象类的继承,尤其是子类是非抽象类的情况。
二、实训内容1、请按模板要求,将【代码】替换为Java程序代码。
Parent.javaabstract class Parent{abstract void grow();}Son.javaclass Son extends Parent{void grow(){System.out.println("son:我比父亲成长条件好一点!"); //重写grow()方法,输出”son:我比父亲成长条件好一点!”}void play(){System.out.println("我会踢球");}}Daughter.javaclass Daughter extends Parent{void grow(){System.out.println("daughter:我比父亲成长条件好很多!"); //重写grow ()方法,输出”daughter:我比父亲成长条件好很多!”}void dance(){System.out.println("我会跳舞");}}MyTest.javapublic class MyTest {public static void main(String[] args) {Parent p=new Son(); //将p设为为子类Son的上转型对象p.grow(); //调用重写父类中的grow()方法//p.play(); 这里会报错,因为不能调用子类新增的play()方法Son s=(Son)p; //将上转型对象进行强制转化为子类Son的对象s.play(); //调用子类中的方法Parent p2=new Daughter(); //将p设为为子类Daughter的上转型对象p2.grow(); //调用重写父类中的grow()方法Daughter d=(Daughter)p2; //将p进行强制转化为Daughter的对象d.dance(); //调用Daughter类中的dance()方法}}2、编程题【实验-继承与接口1】(1)设计一个形状类Shape,包含一个getArea()方法,该方法不包含实际语句。
类继承与接口(二)实验报告
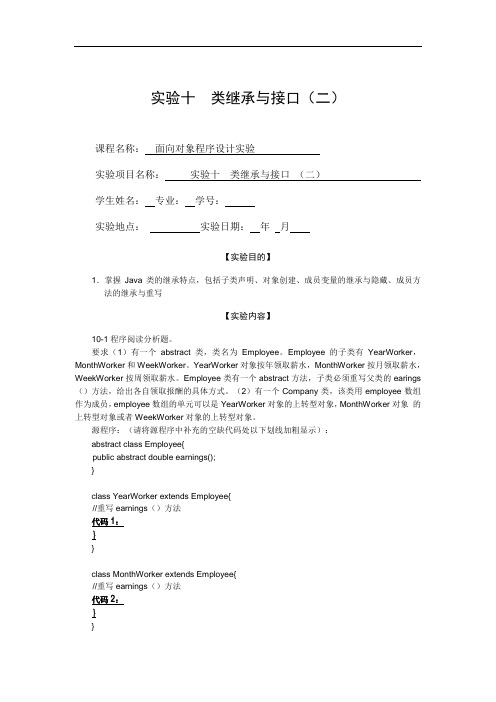
}
是否出错:错,
出错原因(如果出错):
Bbb(){ }中没有调用Aaa中的构造器,改为Bbb(){super(10);}
4.class Aaa{
private int i;
protected int j;
}
class Bbb extends Aaa{
Bbb(){
i = 10;
j = 99;
int i;
public void Aaa(int a){ i=a;}
public static void main(String[] args){
Aaa a = new Aaa(10);
}
}
是否出错:是
出错原因(如果出错):
public void Aaa(int a){ i=a;}应为构造器,不是方法,改为public Aaa(int a){ i=a;}
salaries = salaries + employee[i].earnings();
}
return salaries;
}
}
public class HardWork{
public static void main(String args[]){
Employee[] employee=new Employee[20];
public double earnings(){
return60000.0;
}
}
class WeekWorker extends Employee{
public double earnings(){
return 40000.0;
}
}
class Company{
实验二继承机制实验报告

浙江理工大学信息学院实验指导书实验名称:类的继承机制的实现学时安排:3实验类别:设计性实验实验要求:1人1组学号:姓名 ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄ ̄一、实验目的1.掌握单继承和多重继承的概念。
2.理解不同的继承类型:public、protected和private,掌握何时使用何种继承类型。
3.掌握类层次中构造函数的定义方式和建立对象时构造和析构次序二、实验原理介绍通过继承机制实现对类功能的扩展,合理设计派生类的构造函数、成员函数。
三、实验设备介绍软件需求: windows或linux下的c++编译器硬件需求: 对于硬件方面的要求,建议配置是Pentium III 450以上的CPU 处理器,64MB以上的内存,200MB的自由硬盘空间、CD-ROM驱动器、能支持24位真彩色的显示卡、彩色显示器、打印机。
四、实验内容实现对第一次实验结果Elevator类的功能扩展。
在Elevator类已有功能的基础上派生AdvancedElevator类。
AdvancedElevator类可以实现当多人在不同楼层等待乘坐上行或下行的同一部电梯时,能够合理的根据乘坐人的需求对电梯经停的楼层进行排序。
要求:1.为了实现上的方便性,我们假设同一组要求乘坐电梯的乘客或者都是上行,或者都是下行。
2.在主函数中对该类的功能进行测试,测试方法是首先选择在某一时间段一组要乘坐电梯的乘客是上行还是下行,然后输入组中乘客的人数及每一个乘客所在楼层和目的楼层,由AdvancedElevator类实例化后的电梯对象在运作的过程中,如果电梯是上行,则能根据乘客所在的楼层和目的楼层从下向上依次停靠;如果电梯是下行,则能根据乘客所在的楼层和目的楼层从上向下依次停靠。
3.在测试的过程中,还需要注意测试当多个用户在同一楼层或多个用户的目的楼层为同一楼层时情况的处理。
提示:为了方便描述乘客,我们可以定义一个Person类,主要描述每一个乘客所在楼层和目的楼层。
java实验,继承与接口

一、实验目的与要求实验目的:1、掌握类的继承关系。
2、掌握接口的定义与使用。
实验要求:按下列要求编写Java程序:1、定义接口Printx,其中包括一个方法printMyWay(),这个方法没有形参,返回值为空。
2、编写矩形类,矩形类要求实现Printx接口,有求面积、求周长的方法,printMyWay()方法要能显示矩形的边长、面积和周长。
3、编写正方形类作为矩形类的子类,正方形类继承了矩形类求面积和周长的方法,新增加求对角线长的方法,重写printMyWay()方法,要求该方法能显示正方形的边长、面积、周长和对角线长。
二、实验方案/** To change this template, choose Tools | Templates* and open the template in the editor.*/package 继承与接口;interface Printx{void printMyWay();}class rectangle implements Printx{double length = 0,wide=0;public rectangle(double l, double w){length=l;wide=w;}double area(){double area = length * wide;return area;}double peritemer(){double peritemer=(length+wide)*2;return peritemer;}public void printMyWay(){System.out.println("长方形边长是:"+length+" "+wide);System.out.println("长方形面积是:"+area());System.out.println("长方形周长是:"+peritemer());}}class square extends rectangle{public square(double l,double w){super(l,w);}double diagonal(){return Math.sqrt(length*length*2);}@Overridepublic void printMyWay(){System.out.println("正方形边长是:"+length);System.out.println("正方形面积是:"+area());System.out.println("正方形周长是:"+peritemer());System.out.println("正方形的对角线长是:"+diagonal());}}public class Main{static double length = 0,wide=0;public static void main(String[] args) {rectangle a=new rectangle(8,4);square b=new square(6,6);a.printMyWay();b.printMyWay();// TODO code application logic here}}三、实验结果和数据处理将长方形的长和宽设置为8和4,正方形边长设置为6,输出结果如下:四、结论接口是Java实现部分多继承功能的体现。
继承与接口实验报告
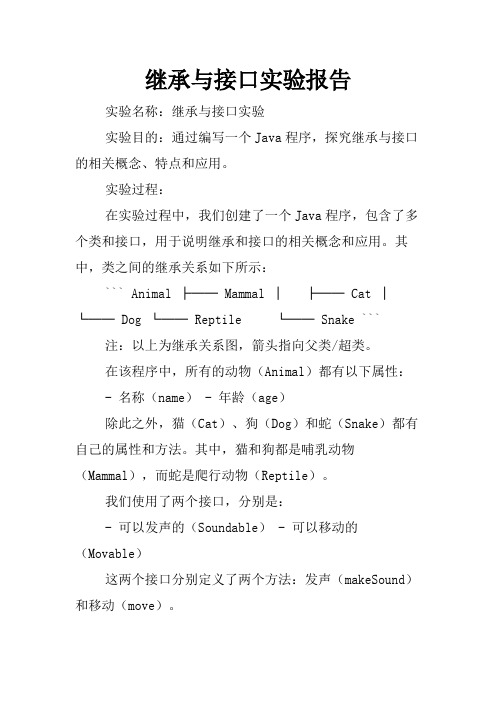
继承与接口实验报告实验名称:继承与接口实验实验目的:通过编写一个Java程序,探究继承与接口的相关概念、特点和应用。
实验过程:在实验过程中,我们创建了一个Java程序,包含了多个类和接口,用于说明继承和接口的相关概念和应用。
其中,类之间的继承关系如下所示:``` Animal ├── Mammal │ ├── Cat │ └── Dog └── Reptile └── Snake ```注:以上为继承关系图,箭头指向父类/超类。
在该程序中,所有的动物(Animal)都有以下属性:- 名称(name) - 年龄(age)除此之外,猫(Cat)、狗(Dog)和蛇(Snake)都有自己的属性和方法。
其中,猫和狗都是哺乳动物(Mammal),而蛇是爬行动物(Reptile)。
我们使用了两个接口,分别是:- 可以发声的(Soundable) - 可以移动的(Movable)这两个接口分别定义了两个方法:发声(makeSound)和移动(move)。
在这些类和接口的基础上,我们编写了一个测试类(TestAnimals),测试了每个类和接口的功能。
实验结果:- Animal类:Animal类是所有动物的“祖先”类,它包含了所有动物共有的属性。
- Mammal类:Mammal类是哺乳动物的基类,它包含了所有哺乳动物共有的属性和方法。
- Reptile类:Reptile类是爬行动物的基类,它包含了所有爬行动物共有的属性和方法。
- Cat类:Cat类继承了Mammal类,它包含了猫的属性和方法。
- Dog类:Dog类继承了Mammal类,它包含了狗的属性和方法。
- Snake类:Snake类继承了Reptile类,它包含了蛇的属性和方法。
- Soundable接口:Soundable接口定义了makeSound 方法,用于让实现了该接口的类发出声音。
- Movable接口:Movable接口定义了move方法,用于让实现了该接口的类移动。
继承与接口实验报告

继承与接口实验报告本次实验主要涉及到Java中的继承和接口的使用。
通过实验,我们可以更好地理解和掌握继承和接口的概念、特点和使用方法。
实验步骤:1. 创建一个父类Animal,其中包含成员变量name和age,以及方法eat()和sleep()。
2. 创建两个子类Cat和Dog,它们分别继承自Animal类,并分别实现自己的eat()和sleep()方法。
3. 创建一个接口Swim,其中包含一个swim()方法。
4. 在Dog类中实现Swim接口,并实现swim()方法。
5. 创建一个测试类Test,其中包含main()方法。
在main()方法中,创建一个Cat对象和一个Dog对象,并分别调用它们的eat()和sleep()方法。
6. 在main()方法中,创建一个Swim类型的变量s,将Dog对象赋值给它,并调用s的swim()方法。
实验结果:通过实验,我们成功地创建了Animal类和它的两个子类Cat和Dog,它们分别继承了Animal类的成员变量和方法,并实现了自己的eat()和sleep()方法。
同时,我们还创建了Swim接口,并在Dog类中实现了它。
在测试类Test中,我们成功地创建了Cat和Dog对象,并调用了它们的eat()和sleep()方法。
同时,我们还创建了一个Swim 类型的变量s,将Dog对象赋值给它,并调用了s的swim()方法。
实验结论:通过本次实验,我们深入理解了Java中的继承和接口的概念和使用方法。
其中,继承可以让子类继承父类的成员变量和方法,从而避免了重复编写代码的问题;而接口则可以让类实现共同的方法,提高代码的重用性和可维护性。
同时,我们还学会了如何在子类中实现接口,并在测试类中使用。
这些知识对于我们今后的Java程序开发将有很大的帮助。
实验项目2 第2部分 继承与接口
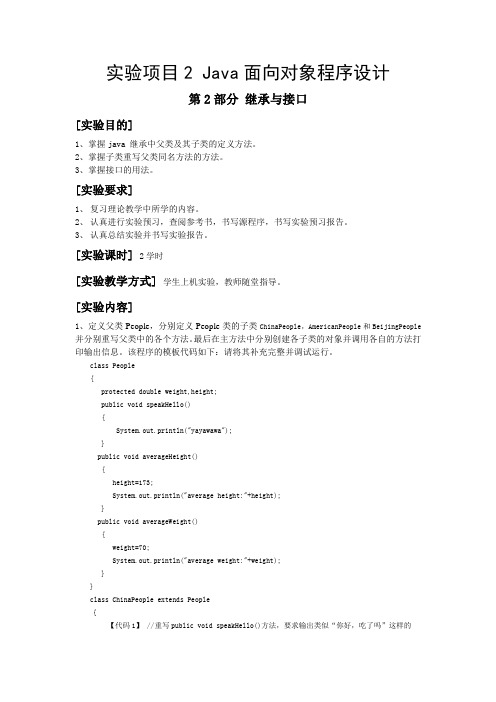
实验项目2 Java面向对象程序设计第2部分继承与接口[实验目的]1、掌握java 继承中父类及其子类的定义方法。
2、掌握子类重写父类同名方法的方法。
3、掌握接口的用法。
[实验要求]1、复习理论教学中所学的内容。
2、认真进行实验预习,查阅参考书,书写源程序,书写实验预习报告。
3、认真总结实验并书写实验报告。
[实验课时] 2学时[实验教学方式] 学生上机实验,教师随堂指导。
[实验内容]1、定义父类People,分别定义People类的子类ChinaPeople,AmericanPeople和BeijingPeople 并分别重写父类中的各个方法。
最后在主方法中分别创建各子类的对象并调用各自的方法打印输出信息。
该程序的模板代码如下:请将其补充完整并调试运行。
class People{protected double weight,height;public void speakHello(){System.out.println("yayawawa");}public void averageHeight(){height=173;System.out.println("average height:"+height);}public void averageWeight(){weight=70;System.out.println("average weight:"+weight);}}class ChinaPeople extends People{【代码1】 //重写public void speakHello()方法,要求输出类似“你好,吃了吗”这样的//汉语信息【代码2】 //重写public void averageHeight()方法,要求输出类似//“中国人的平均身高:168.78厘米”这样的汉语信息【代码3】 //重写public void averageWeight()方法,//要求输出类似“中国人的平均体重:65公斤”这样的汉语信息public void chinaGongfu(){【代码4】//输出中国武术的信息,例如:"坐如钟,站如松,睡如弓"等}}class AmericanPeople extends People{【代码5】 //重写public void speakHello()方法,要求输出类似//“How do you do”这样的英语信息。
java实验 2 (2) 继承、多态与接口
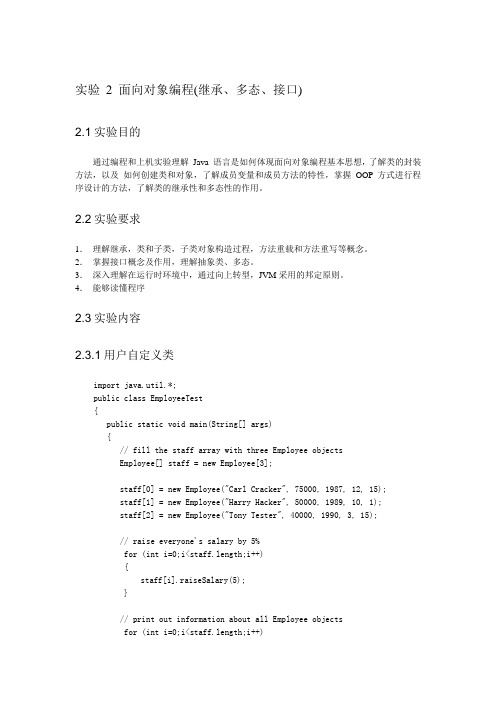
实验2 面向对象编程(继承、多态、接口)2.1实验目的通过编程和上机实验理解Java 语言是如何体现面向对象编程基本思想,了解类的封装方法,以及如何创建类和对象,了解成员变量和成员方法的特性,掌握OOP 方式进行程序设计的方法,了解类的继承性和多态性的作用。
2.2实验要求1.理解继承,类和子类,子类对象构造过程,方法重载和方法重写等概念。
2.掌握接口概念及作用,理解抽象类、多态。
3.深入理解在运行时环境中,通过向上转型,JVM采用的邦定原则。
4.能够读懂程序2.3实验内容2.3.1用户自定义类import java.util.*;public class EmployeeTest{public static void main(String[] args){// fill the staff array with three Employee objectsEmployee[] staff = new Employee[3];staff[0] = new Employee("Carl Cracker", 75000, 1987, 12, 15);staff[1] = new Employee("Harry Hacker", 50000, 1989, 10, 1);staff[2] = new Employee("Tony Tester", 40000, 1990, 3, 15);// raise everyone's salary by 5%for (int i=0;i<staff.length;i++){staff[i].raiseSalary(5);}// print out information about all Employee objectsfor (int i=0;i<staff.length;i++){System.out.println("name=" + staff[i].getName()+ ",salary=" + staff[i].getSalary()+ ",hireDay=" + staff[i].getHireDay());}}}class Employee{public Employee(String n, double s, int year, int month, int day){name = n;salary = s;GregorianCalendar calendar = new GregorianCalendar(year, month - 1, day);// GregorianCalendar uses 0 for JanuaryhireDay = calendar.getTime();}public String getName(){return name;}public double getSalary(){return salary;}public Date getHireDay(){return hireDay;}public void raiseSalary(double byPercent){double raise = salary * byPercent / 100;salary += raise;}private String name;private double salary;private Date hireDay;}2.3.2类的继承性class Employee {String name ;int salary;public Employee(String name,int salary){ = name;this.salary = salary;}public String getDetails( ){return "Name: "+name+"\nSalary: "+salary;}}class Manager extends Employee {private String department ;public Manager(String name,int salary,String department){ super(name,salary);this.department = department;}public String getDetails( ){return "Name: "+name+"\nSalary: "+salary+"\nDepartment: "+ department;}}class Secretary extends Employee{public Secretary(String name,int salary){super(name,salary);}}public class TestOverriding{public static void main(String[] srgs){Manager m = new Manager("Tom",2000,"Finance");Secretary s = new Secretary("Mary",1500);System.out.println(m.getDetails());System.out.println(s.getDetails());}}2.3.3类的多态性import java.util.*;//定义Shape类class Shape {void draw() {}void erase() {}}//定义Circle类class Circle extends Shape {void draw() {System.out.println("Calling Circle.draw()"); }void erase() {System.out.println("Calling Circle.erase()"); }}//定义Square类class Square extends Shape {void draw() {System.out.println("Calling Square.draw()"); }void erase() {System.out.println("Calling Square.erase()"); }}//定义Triangle类class Triangle extends Shape {void draw() {System.out.println("Calling Triangle.draw()"); }void erase() {System.out.println("Calling Triangle.erase()"); }}//包含main()的测试类public class Shapes{static void drawOneShape(Shape s){s.draw();}static void drawShapes(Shape[] ss){for(int i = 0; i < ss.length; i++){ss[i].draw();}}public static void main(String[] args) {Random rand = new Random(); //阅读JDK API 文档Shape[] s = new Shape[9];for(int i = 0; i < s.length; i++){switch(rand.nextInt(3)) { //rand.nextInt(3)随机生成0、1、2三个整数case 0: s[i] = new Circle();break;case 1: s[i] = new Square();break;case 2: s[i] = new Triangle();break;}}drawShapes(s);}}//思考题:将Shape改成接口类型,再试2.3.4 属性隐藏与方法重写//这是一个向上转型,实现多态的例子//阅读程序,理解JVM的绑定规则class Base{String var="BaseVar"; //实例变量static String staticVar="StaticBaseVar"; //静态变量void method(){ //实例方法System.out.println("Base method");}static void staticMethod(){ //静态方法System.out.println("Static Base method");}}public class Sub extends Base{String var="SubVar"; //实例变量static String staticVar="StaticSubVar"; //静态变量void method(){ //覆盖父类的method()方法System.out.println("Sub method");}static void staticMethod(){ //隐藏父类的staticMethod()方法System.out.println("Static Sub method");}String subVar="Var only belonging to Sub";void subMethod(){System.out.println("Method only belonging to Sub");}public static void main(String args[]){Base who=new Sub(); //who被声明为Base类型,引用Sub实例System.out.println("who.var="+who.var); //打印Base类的var变量System.out.println("who.staticVar="+who.staticVar); //打印Base类的staticVar变量who.method(); //打印Sub实例的method()方法whpillar;pillar;pillar;pillar;pillar;。
面向对象--类继承与接口(二)实验报告

实验十类继承与接口(二)课程名称:面向对象程序设计实验项目名称:实验十类继承与接口(二)学生姓名:专业:学号:实验地点:实验日期:年月【实验目的】1.掌握Java类的继承特点,包括子类声明、对象创建2. 掌握域的继承与隐藏的特点和用法3. 掌握方法的继承与重写的特点和用法【实验内容】一. 改错题检查下面代码是否有错,如果有错,写明错误原因,并修正错误。
(1)class Aaa{int i;Aaa(){i=-1; }Aaa(int a){ i = a;}Aaa(double d){Aaa((int)d);}}是否出错:是出错原因(如果出错):构造器调用构造器不能直接用名class Aaa{int i;Aaa(){i=-1; }Aaa(int a){ i = a;}Aaa(double d){this((int)d);}}(2)class Aaa{int i;Aaa(){i=-1; }Aaa(int a){ i = a;}Aaa(double a){int b=(int)a;this(b);}}是否出错:是出错原因(如果出错):this放第一行class Aaa{int i;Aaa(){i=-1; }Aaa(int a){ i = a;}Aaa(double a){this((int)a);}}(3)class Aaa{int i;Aaa(int a){ i = a;}}class Bbb extends Aaa{Bbb(){ }}是否出错:是出错原因(如果出错):父类没有无参构造,子类中药通过super调用父类构造class Aaa{int i;Aaa(int a){ i = a;}}class Bbb extends Aaa{Bbb(int a) {super(a);}}(4)class Aaa{private int i;protected int j;}class Bbb extends Aaa{Bbb(){i = 10;j = 99;}}是否出错:是出错原因(如果出错):private只能在自己的类中使用class Aaa{protected int i;protected int j;}class Bbb extends Aaa{Bbb(){i = 10;j = 99;}}(5)编译下面程序,程序是否出错class A{int i =100;}class B extends A{int i =10000;public static void main(String[] args){System.out.println(this.i);System.out.println(super.i);}}a)是否出错:是b)出错原因(如果出错):不能在静态方法使用this superclass A{int i =100;}class B extends A{int i =10000;public void main(String[] args){System.out.println(this.i);System.out.println(super.i);}}c)如果将上述类B的int i =10000改为static int i =10000;类A的int i =100改为static int i =100;程序是否出错?是出错原因(如果出错):不能在静态方法使用this superclass A{int i =100;}class B extends A{int i =10000;public void main(String[] args){System.out.println(this.i);System.out.println(super.i);}}d)如果将类B的main方法改为public static void main(String[] args){B b =new B();System.out.println(b.i);System.out.println((A)b.i);}程序是否出错? 是出错原因(如果出错):不能强制将in类型改为A如果没出错, 给出结果:上面的System.out.println(b.i)的含义:System.out.println((A)b.i) 的含义:(6)class Aaa{int i;public void Aaa(int a){ i=a;}public static void main(String[] args){Aaa a = new Aaa(10);}是否出错:是出错原因(如果出错):构造器没有返回class Aaa{int i;public Aaa(int a){ i=a;}public static void main(String[] args){Aaa a = new Aaa(10);}}(7)class Aaa{public static void main(String[] args){int[][] a=new int[5][];for( int i=0;i<a.length;i++)for(int j=0;i<a[i].length;j++){a[i][j]= (int)(100*Math.random());System.out.println(a[i][j]);}}}是否出错:出错原因(如果出错):for(int j=0;i<a[i].length;j++)出现无限循环class Aaa{public static void main(String[] args){int[][] a=new int[5][5];for( int i=0;i<a.length;i++)for(int j=0;j<a[i].length;j++){a[i][j]= (int)(100*Math.random());System.out.println(a[i][j]);}}}(8)class A{int abc(int i){return 1;} //方法1int abc(int j){return 2;} //方法2int abc(int i,int j){return 3;} //方法3void abc(int i){ } //方法4是否有错:是上面类A中定义的四个方法是否都是方法的重载,有没有重复定义的方法,如果有,那么哪几个方法是重复定义的?方法1和方法2是重复定义(9)class A{void show(){System.out.println("A类的show()");}}class B extends A{int show(){System.out.println("B类的show()");}public static void main(String[] args){B b = new B();b.show();}}上面程序中的方法覆盖是否出错:是出错原因(如果出错):如果将类B中的方法show()改为:int show(int b){System.out.println("B类的show()");return b;}程序是否出错?不出错二.程序分析设计题下面各个设计要放在各自的包中。
实验二+++继承、接口与异常处理

实验一继承、接口与异常处理一、实验目的1.掌握类的继承机制。
2.熟悉类中成员变量和方法的访问控制。
3.掌握接口与包的使用,熟悉方法的多态性。
4.掌握异常处理方法及熟悉常见异常的捕获方法。
二、实验内容1.定义父类及子类,在子类中重写父类的方法2.练习接口与包的使用3.练习捕获异常、声明异常、抛出异常的方法、熟悉try和catch子句的三、.实验步骤与要求第1题继承编写一个Java应用程序,除了主类外,该程序中还有4个类:People, ChinaPeople, AmericanPeople 和BeijingPeople类。
此四个类的继承关系如下图所示:要求ChinaPeople,American类均重写其父类People类的speakHello, averageHeight, averageWeight方法,BeijingPeople类重写其父类ChinaPeople类的speakHello, averageHeight, averageWeight方法。
第2题上转型对象要求有一个abstract类,类名为Employee。
Employee的子类有YearWorker,MonthWorker和WeekWorker。
YearWorker按年领取薪水,MonthWorker按月领取薪水,WeekWorker按周领取薪水。
Employee类有一个abstract方法:public abstract earnings();子类必须重写父类的earnings()方法,给出各自领取报酬的具体方式。
有一个Company类,该类用employee数组作为成员,employee数组的单元可以是YearWorker对象的上转型对象、MonthWorker对象的上转型对象或WeekWorker对象的上转型对象。
程序能输出Company对象一年需要支付的薪水总额。
第3题接口回调要求有一个ComputeTotalSales接口,该接口中有一个方法:public double totalSalesByYear(),有三个实现该接口的类:Television,Computer和Mobile。
实训-继承与接口2

实训继承与接口 2一、实训目标理解对象的上转型对象,以及多态的目的和意义,掌握多态的常用形式。
理解抽象类的意义和实际应用的一般模式,熟练掌握抽象类和抽象方法的定义以及抽象类的继承,尤其是子类是非抽象类的情况。
二、实训内容1、请按模板要求,将【代码】替换为Java程序代码。
Parent.javaabstract class Parent{ abstract void grow();}Son.javaclass Son extends Parent{ void grow(){System. out .println( "son: 我比父亲成长条件好一点!" ); // 重写grow ()方法,输出”son: 我比父亲成长条件好一点! ”}void play(){System. out .println(" 我会踢球" );}}Daughter.javaclass Daughter extends Parent{void grow(){System. out .println( "daughter: 我比父亲成长条件好很多!" ); // 重写grow ()方法,输出” daughter: 我比父亲成长条件好很多! ”}void dance(){System. out .println(" 我会跳舞" );}MyTest.javapublic class MyTest {public static void main(String[] args ) {Parent p=new Son();// 将p设为为子类Son的上转型对象p.grow(); // 调用重写父类中的grow ()方法//p.play(); 这里会报错,因为不能调用子类新增的play() 方法Son s =(Son) p; // 将上转型对象进行强制转化为子类Son的对象s.play(); // 调用子类中的方法Parent p2 =new Daughter(); // 将p设为为子类Daughter 的上转型对象p2 .grow();// 调用重写父类中的grow ()方法Daughter d =(Daughter) p2; // 将p进行强制转化为Daughter 的对象 d.dance(); // 调用Daughter 类中的dance ()方法【实验-继承与接口1】(1)设计一个形状类Shape,包含一个getArea() 方法,该方法不包含实际语句。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
实验二继承与接口一、实验目的1.掌握类的继承机制。
2.熟悉类中成员变量和方法的访问控制。
3.掌握接口与包的使用,熟悉方法的多态性。
二、实验内容1.定义父类及子类,在子类中重写父类的方法2.练习接口与包的使用三、.实验步骤与要求第1题继承编写一个Java应用程序,除了主类外,该程序中还有4个类:People, ChinaPeople, AmericanPeople 和BeijingPeople类。
此四个类的继承关系如下图所示:要求ChinaPeople,American类均重写其父类People类的speakHello, averageHeight, averageWeight方法,BeijingPeople类重写其父类ChinaPeople类的speakHello, averageHeight, averageWeight方法。
源代码:package people;class people{protected double height ;protected double weight ;public void speakHello() //问候语的函数{System.out.println("hello");}public void averageHeight()//人们的平均身高{height=170;System.out.println(+height);}public void averageWeight()//人们的平均体重{ weight=120;System.out.println(+weight);}}class Chinapeople extends people{public void speakHello(){System.out.println("你好");}public void averageHeight(){height=172;System.out.println(+height);}public void averageWeight(){ weight=115;System.out.println(+weight);}public void chinaGongfu()//中国功夫的方法{System.out.println("中国功夫");}}class Americanpeople extends people{public void speakHello(){System.out.println("hello");}public void averageHeight(){height=180;System.out.println(+height);}public void averageWeight(){ weight=150;System.out.println(+weight);}public void americanBoxing()//美国拳击的方法{System.out.println("americanBoxing");}}class Beijingpeople extends Chinapeople{public void speakHello(){System.out.println("北京欢迎你");}public void averageHeight(){height=168;System.out.println(+height);}public void averageWeight(){ weight=125;System.out.println(+weight);}}class Example{public static void main(String []args){people p =new people();Chinapeople c=new Chinapeople(); Americanpeople a=new Americanpeople(); Beijingpeople b=new Beijingpeople();p.averageHeight();p.averageWeight();p.speakHello();c.averageHeight();c.averageWeight();c.chinaGongfu();c.speakHello();a.averageHeight();a.averageWeight();a.americanBoxing();a.speakHello();b.averageHeight();b.averageWeight();b.speakHello();}}结果截图:第2题上转型对象要求有一个abstract类,类名为Employee。
Employee的子类有YearWorker,MonthWorker和WeekWorker。
YearWorker按年领取薪水,MonthWorker按月领取薪水,WeekWorker按周领取薪水。
Employee类有一个abstract方法:public abstract earnings();子类必须重写父类的earnings()方法,给出各自领取报酬的具体方式。
有一个Company类,该类用employee数组作为成员,employee数组的单元可以是YearWorker对象的上转型对象、MonthWorker对象的上转型对象或WeekWorker对象的上转型对象。
程序能输出Company对象一年需要支付的薪水总额。
源代码:package people;abstract class Employee{int salary;public abstract void earnings();}class YearWorker extends Employee{public void earnings(){ salary=200;System.out.println("年薪:" +salary*365);}}class MonthWorker extends Employee{public void earnings(){ salary=100;System.out.println("月薪:"+salary*30+" 一年薪水: "+salary*30*12);}}class WeekWorker extends Employee{public void earnings(){ salary=80;System.out.println("周薪:"+salary*7+" 一年薪水: "+salary*365);}}public class Company{ public static void main(String []args){Employee e[]=new Employee[3];e[0]=new YearWorker();e[0].earnings();e[1]=new MonthWorker();e[1].earnings();e[2]=new WeekWorker();e[2].earnings();}}结果截图:第3题接口回调要求有一个ComputeTotalSales接口,该接口中有一个方法:public double totalSalesByYear(),有三个实现该接口的类:Television,Computer和Mobile。
这三个类通过实现接口computeTotalSales,给出自己的年销售额。
有一个Shop类,该类用computeTotalSales数组作为成员,computeTotalSales 数组的单元可以存放Television对象的引用、Computer对象的引用或Mobile对象的引用。
程序能输出Shop对象的年销售额。
源代码:package people;interface ComputeTotalSales{public double totalSalesByYear();}class Television implements ComputeTotalSales{public double totalSalesByYear(){double sale=100;return sale*365;}}class computer implements ComputeTotalSales{public double totalSalesByYear(){double sale=200;return sale*365;}}class Mobile implements ComputeTotalSales{public double totalSalesByYear(){double sale=220;return sale*365;}}public class shop {public static void main(String []args){ComputeTotalSales c[];c=new ComputeTotalSales[3];c[0]=new Television();System.out.println("电视机的年销售量:"+c[0].totalSalesByYear());c[1]=new computer();System.out.println("电脑的年销售量:"+c[1].totalSalesByYear());c[2]=new Mobile();System.out.println("手机的年销售量:"+c[2].totalSalesByYear());}}结果截图:四、实验结果编写源程序并上机调试通过,根据实验过程填写实验报告。
提交电子版实验报告要求:1、源代码要规范、有详细的注释;每题以文件夹管理各个Java源文件;2、运行结果截图+心得体会(实验报告)。
打包命名:“学号姓名java实验几”:如“2012085111小明java实验二”。