DataGrid导出EXCEL的几个方法(WebControl)
将DataGridView中的数据导出至Excel中(亲测成功)

将DataGridView中的数据导出至Excel(亲测成功)一、建立一个静态方法public static void ExportAsExcel(DataGridView dgv){OutputAsExcelFile(DGVHelper.DataGridViewToTable(dgv));}二、把DataGridView中的数据转换到DataTable中///<summary>///将DataGridView中的数据转换为DataTable包含隐藏列///</summary>///<param name="dgv"></param>///<returns></returns>public static DataTable DataGridViewToTable(DataGridView dgv){DataTable dt=new DataTable();//循环列标题名称,处理了隐藏的行不显示for(int count=0;count<dgv.Columns.Count;count++){if(dgv.Columns[count].Visible==true){dt.Columns.Add(dgv.Columns[count].HeaderText.ToString());}}//循环行,处理了隐藏的行不显示for(int count=0;count<dgv.Rows.Count;count++){DataRow dr=dt.NewRow();int curr=0;for(int countsub=0;countsub<dgv.Columns.Count;countsub++){if(dgv.Columns[countsub].Visible==true){if(dgv.Rows[count].Cells[countsub].Value!=null){dr[curr]=dgv.Rows[count].Cells[countsub].Value.ToString();}else{dr[curr]="";}curr++;}}dt.Rows.Add(dr);}return dt;}三、把DataTable中的数据导出到Excel中public static void OutputAsExcelFile(DataTable dt){if(dt.Rows.Count<=0){MessM.PromptInfo("提示","无数据!");return;}SaveFileDialog s=new SaveFileDialog{Title="保存Excel文件",Filter="Excel文件(*.xls)|*.xls",FilterIndex=1};if(s.ShowDialog()==DialogResult.OK)filePath=s.FileName;elsereturn;DTExportToExcel(dt);}///<summary>///第二步:导出dataTable到Excel///</summary>///<param name="dt"></param>private static void DTExportToExcel(DataTable dt){//第二步:导出dataTable到Excellong rowNum=dt.Rows.Count;//行数int columnNum=dt.Columns.Count;//列数Excel.Application m_xlApp=new Excel.Application{DisplayAlerts=false,//不显示更改提示Visible=false};Excel.Workbooks workbooks=m_xlApp.Workbooks;Excel.Workbook workbook=workbooks.Add(Excel.XlWBATemplate.xlWBATWorksheet);Excel.Worksheet worksheet=(Excel.Worksheet)workbook.Worksheets[1];//取得sheet1try{string[,]datas=new string[rowNum+1,columnNum];for(int i=0;i<columnNum;i++)//写入字段datas[0,i]=dt.Columns[i].Caption;//Excel.Range range=worksheet.get_Range(worksheet.Cells[1,1],worksheet.Cells[1, columnNum]);Excel.Range range=m_xlApp.Range[worksheet.Cells[1,1],worksheet.Cells[1, columnNum]];range.Interior.ColorIndex=15;//15代表灰色range.Font.Bold=true;range.Font.Size=10;int r=0;for(r=0;r<rowNum;r++){for(int i=0;i<columnNum;i++){object obj=dt.Rows[r][dt.Columns[i].ToString()];datas[r+1,i]=obj==null?"":"'"+obj.ToString().Trim();//在obj.ToString()前加单引號是为了防止自己主动转化格式}Application.DoEvents();//加入进度条}//Excel.Range fchR=worksheet.get_Range(worksheet.Cells[1,1], worksheet.Cells[rowNum+1,columnNum]);Excel.Range fchR=m_xlApp.Range[worksheet.Cells[1,1],worksheet.Cells[rowNum+1, columnNum]];fchR.Value2=datas;worksheet.Columns.EntireColumn.AutoFit();//列宽自适应。
C#DataGridView导出excel的几种方法
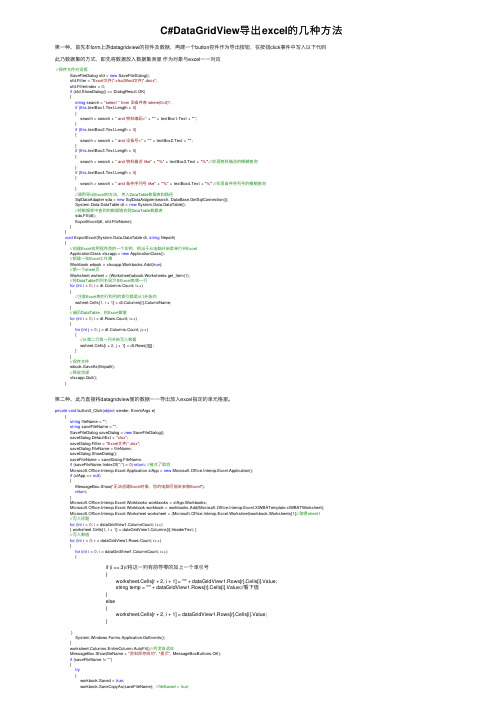
C#DataGridView导出excel的⼏种⽅法第⼀种、⾸先本form上游datagridview的控件及数据,再建⼀个button控件作为导出按钮,在按钮click事件中写⼊以下代码此乃数据集的⽅式,即先将数据放⼊数据集表⾥作为对象与excel⼀⼀对应//保存⽂件对话框SaveFileDialog sfd = new SaveFileDialog();sfd.Filter = "Excel⽂件|*.xlsx|Word⽂件|*.docx";sfd.FilterIndex = 0;if (sfd.ShowDialog() == DialogResult.OK){string search = "select * from 旧备件表 where(0=0)";if (this.textBox1.Text.Length > 0){search = search + " and 物料编码=" + "'" + textBox1.Text + "'";}if (this.textBox2.Text.Length > 0){search = search + " and 设备号=" + "'" + textBox2.Text + "'";}if (this.textBox3.Text.Length > 0){search = search + " and 物料描述 like" + "'%" + textBox3.Text + "%'";//实现物料描述的模糊查询}if (this.textBox4.Text.Length > 0){search = search + " and 备件序列号 like" + "'%" + textBox4.Text + "%'";//实现备件序列号的模糊查询}//调⽤导出Excel的⽅法,传⼊DataTable数据表和路径SqlDataAdapter sda = new SqlDataAdapter(search, DataBase.GetSqlConnection());System.Data.DataTable dt = new System.Data.DataTable();//将数据库中查到的数据填充到DataTable数据表sda.Fill(dt);ExportExcel(dt, sfd.FileName);}}void ExportExcel(System.Data.DataTable dt, string filepath){//创建Excel应⽤程序类的⼀个实例,相当于从电脑开始菜单打开ExcelApplicationClass xlsxapp = new ApplicationClass();//新建⼀张Excel⼯作簿Workbook wbook = xlsxapp.Workbooks.Add(true);//第⼀个sheet页Worksheet wsheet = (Worksheet)wbook.Worksheets.get_Item(1);//将DataTable的列名显⽰在Excel表第⼀⾏for (int i = 0; i < dt.Columns.Count; i++){//注意Excel表的⾏和列的索引都是从1开始的wsheet.Cells[1, i + 1] = dt.Columns[i].ColumnName;}//遍历DataTable,给Excel赋值for (int i = 0; i < dt.Rows.Count; i++){for (int j = 0; j < dt.Columns.Count; j++){//从第⼆⾏第⼀列开始写⼊数据wsheet.Cells[i + 2, j + 1] = dt.Rows[i][j];}}//保存⽂件wbook.SaveAs(filepath);//释放资源xlsxapp.Quit();}第⼆种、此乃直接将datagridview⾥的数据⼀⼀导出放⼊excel指定的单元格⾥。
DxDbGrid与DbGridEh表格使用及导出Excel

DxDbGrid与DbGridEh表格使用及导出Excel分类:Delphi 2011-05-16 09:47 110人阅读评论(0) 收藏举报前言:二者都是非常不错的第三方表格控件,都可实现多表头的表格及分组汇总功能;在导出 Excel 方面,个人觉得 DxDbGrid 做的比DbGridEh 出色,几乎是 Grid 原样导出, DbGridEh 导出表格模式的单元格与 Grid 显示有所出入。
一、所用版本及安装:1 、版本: DevExQuantumGrid v3.22 Pro for D7 、 EhLib 5.2.842 、安装: DevExQuantumGrid 直接 Setup.exe 就 OK ; EhLib 在Delphi7 的安装稍微啰嗦点,具体步骤参考 readme.txt 或如下步骤:(1). 将 EhLib 5.2.84 解压缩到目标目录。
(2). 打开 Delphi 7 ,将 EhLib 的 /Delphi7 子目录加到 Delphi 的 Library path 。
( 菜单操作路径为:Tools|Environment Options...|Library|Library path)(3). 将 EhLib 目标安装目录中的 common 和 DataService子目录的文件移动到 EhLib 的 /Delphi7 子目录中。
(4). 在 Delphi 7 中打开 EhLib70.dpk ,编译,但不要安装。
(5). 在 Delphi 7 中打开 DclEhLib70.dpk ,编译并安装。
(6). 组件面板中出现一个 EhLib 的组件页。
(7). 打开附带的 DEMOS ,编译并运行,测试安装成功。
二、使用 DxDbGrid1 、窗体拖入 dxDBGrid1 、 ADOConnection1 、 ADOQuery1 、DataSource1 、 Button1 、 SaveDialog1 ,然后完成数据的链接及相关控件关联,如何操作,你应该懂的;接下来完成如下图所示的一个表格:2 、双击 dxDBGrid1 ,在 Bands 栏增加 TdxTreeListBand 并填写Caption ,注意要将 dxDBGrid1 的 ShowBands 属性设置为 True 才能显示 Bands 栏;同样双击 dxDBGrid1 ,在 Columns 栏,添加多个dxGridColumn (根据需要选择不同的类型)并使其与数据库字段形成关联,涉及如下几个属性, BandIndex 选择对应的 Band 从而形成二级表头, Caption 、 FieldName 、 HeaderAlignment 、 width 等,如此完成了基本的表格设计,如果喜欢表头平滑更改 LookAndFeel 属性为 lfFlat 即可。
cxgrid数据导出到Excel方法-骆驼的日志-网易博客

cxgrid数据导出到Excel方法-骆驼的日志-网易博客将数据导出到各种格式的文件是所有表格控件必须处理的重要问题,ExpressQuantumGrid这种成熟的产品控件更不待说,它支持将表格数据导出成Excel,HTML,Text和XML四种文件格式,分别由ExportGrid4ToExcel、ExportGrid4T oHTML、ExportGrid4ToText和ExportGrid4ToXML完成。
以下介绍将cxgrid数据导出到Excel的ExportGrid4ToExcel方法:procedure ExportGrid4ToExcel(const AFileName: string; AGrid: TcxGrid; AExpand: Boolean = True;ASaveAll: Boolean = True; AUseNativeFormat: Boolean = True;const AFileExt: string = 'xls');参数说明:AFileName:定义导出文件的名称,不需要包含文件扩展名,否则最后一个文件扩展名将会被参数AFileExt对应的字符串替换。
AGrid:定义导出文件的来源表格,只有表格的根级可用内容才会被导出,该函数不支持主从数据的导出。
AExpand:定义是否导出来源表格中未展开的记录,默认值为true。
设置为true时,表格将在导出所有记录前展开所有未展开的数据;设置为false时,表格不会改变记录的展开状态并且只导出已展开的记录。
ASaveAll:定义是否导出来源表格中的所有记录,默认值为true。
设置为false时,将只导出当前选中的记录。
AUseNativeFormat:定义是否将来源表格中的数据格式转换为Excel的数据格式,默认值为true。
设置为false时,来源表格中的所有内容将以字符串类型被导出;设置为true时,函数尝试以下表中的对应关系将单元格编辑器转换为Excel数据格式,如果单元格内容无法转换成对应的格式(如包含非法的字符等引起的转换不成功)时,该单元格内容也将以字符串类型被导出。
asp.net里导出excel表方法汇总

HttpContext.Current.Response.Write(tw.ToString());
HttpContext.Current.Response.End();
}
用法:ToExcel(datagrid1);
4、这个用dataview ,代码好长
//
//设置报表表格为最适应宽度
//
xSt.get_Range(excel.Cells[4,2],excel.Cells[rowSum,colIndex]).Select();
xSt.get_Range(excel.Cells[4,2],excel.Cells[rowSum,colIndex]).Columns.AutoFit();
}
//
//取得表格中的数据
//
foreach(DataRowView row in dv)
{
rowIndex ++;
colIndex = 1;
foreach(DataColumn col in dv.Table.Columns)
{
colIndex ++;
//
xSt.get_Range(excel.Cells[rowSum,colSum],excel.Cells[rowSum,colIndex]).Select();
xSt.get_Range(excel.Cells[rowSum,colSum],excel.Cells[rowSum,colIndex]).Interior.ColorIndex = 19;//设置为浅黄色,共计有56种
HttpContext.Current.Response.Charset ="UTF-8";
unigui unidbgrid导出excel实例

unigui unidbgrid导出excel实例全文共四篇示例,供读者参考第一篇示例:uniGUI是一个基于Delphi的全栈Web应用开发框架,它允许开发人员通过使用Delphi IDE创建Web应用程序。
其中uniDBGrid是uniGUI框架中的一个组件,用于显示数据库中的数据并提供丰富的数据操作功能。
本文将介绍如何使用uniGUI和uniDBGrid导出Excel数据的实例。
一、uniDBGrid简介uniGUI中的uniDBGrid是一个用于显示数据库表格数据的组件,它支持多种数据源(如FireDAC、BDE、ADO等),可以根据需求实现数据的显示、编辑、筛选、排序等功能。
开发人员可以通过简单的设置和调整,快速地创建一个功能强大的数据表格。
uniGUI中的uniDBGrid组件提供了导出数据到Excel的功能,使用户可以方便地将数据库中的数据以Excel表格的形式保存或分享。
这对于需要将数据进行进一步处理或与其他人共享数据非常方便。
导出Excel功能可以将uniDBGrid中显示的数据一键导出到Excel 文件中,保留数据的格式、样式和布局。
用户可以选择导出的数据范围(全部数据、当前页数据、选中的数据),以及导出的文件格式(Excel 97-2003格式.xls、Excel 2007及以上格式.xlsx)。
下面我们以一个简单的示例来介绍如何使用uniDBGrid导出Excel 的功能。
1. 准备工作我们需要搭建一个uniGUI的项目,并添加一个uniDBGrid组件以及一个Button按钮用于触发导出操作。
在uniDBGrid的属性中,设置数据源,绑定需要显示的数据表格。
在Button的OnClick事件中编写导出Excel的代码。
2. 编写导出Excel的代码在Button的OnClick事件中添加以下代码:// 设置导出文件的格式为Excel 97-2003格式uniDBGrid.Export.FileType := ftExcel97;ShowMessage('数据已成功导出到Excel 文件: ' + ExcelFileName);end;```以上代码中,我们创建了一个TUniDBGridExport对象,并设置了导出文件的格式为Excel 97-2003格式。
delphi dbgrid 保存为excel 简单方法

delphi dbgrid 保存为excel 简单方法你可以使用以下简单方法将Delphi的DBGrid保存为Excel:1. 首先,添加Excel的引用。
在Delphi的“工具”菜单下选择“导入类型库”,然后选择Microsoft Excel并点击“创建单元”。
这将在您的Delphi项目中添加对Excel的引用。
2. 在所需的单元(例如表单或数据模块)中,添加以下单元引用:- ComObj:用于与COM对象(例如Excel)进行交互的单元。
- DBGrids:用于访问和操作DBGrid的单元。
3. 创建一个导出数据的按钮(或其他事件),然后在事件处理程序中添加以下代码:```delphiusesComObj, DBGrids;procedure TForm1.Button1Click(Sender: TObject);varExcel, Workbook, Worksheet: Variant;i, j: Integer;begin// 创建Excel对象Excel := CreateOleObject('Excel.Application');Excel.Visible := True;// 创建Workbook和WorksheetWorkbook := Excel.Workbooks.Add;Worksheet := Workbook.Worksheets[1];// 输出DBGrid的标题行for i := 0 to DBGrid1.Columns.Count - 1 doWorksheet.Cells[1, i+1].Value :=DBGrid1.Columns[i].Title.Caption;// 输出DBGrid的数据行for i := 0 to DBGrid1.DataSource.DataSet.RecordCount - 1 dobeginfor j := 0 to DBGrid1.Columns.Count - 1 doWorksheet.Cells[i+2, j+1].Value :=DBGrid1.DataSource.DataSet.FieldByName(DBGrid1.Columns[j].FieldName).AsString;DBGrid1.DataSource.DataSet.Next;end;// 保存Workbook并关闭ExcelWorkbook.SaveAs('path\to\save\file.xlsx');Workbook.Close;Excel.Quit;end;```在上述代码中,使用`CreateOleObject`函数创建了Excel对象,并将其设置为可见。
DevExpress中GridControl自定义格式导出Excel
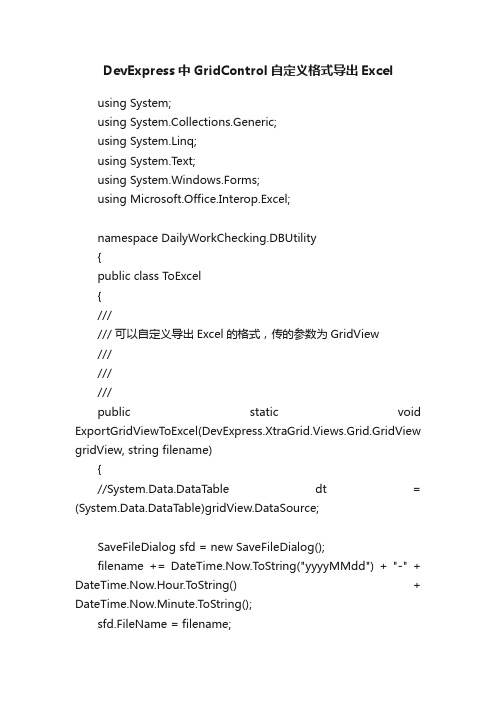
DevExpress中GridControl自定义格式导出Excelusing System;using System.Collections.Generic;using System.Linq;using System.Text;using System.Windows.Forms;using Microsoft.Office.Interop.Excel;namespace DailyWorkChecking.DBUtility{public class ToExcel{////// 可以自定义导出Excel的格式,传的参数为GridView/////////public static void ExportGridViewT oExcel(DevExpress.XtraGrid.Views.Grid.GridView gridView, string filename){//System.Data.DataTable dt = (System.Data.DataTable)gridView.DataSource;SaveFileDialog sfd = new SaveFileDialog();filename += DateTime.Now.T oString("yyyyMMdd") + "-" + DateTime.Now.Hour.ToString() + DateTime.Now.Minute.ToString();sfd.FileName = filename;sfd.Filter = "Excel files (*xls) | *.xls";sfd.RestoreDirectory = true;if (sfd.ShowDialog() == DialogResult.OK && sfd.FileName.Trim() != null){int rowIndex = 1;int colIndex = 0;int colNum = gridView.Columns.Count;System.Reflection.Missing miss = System.Reflection.Missing.Value;Microsoft.Office.Interop.Excel.Application xlapp = new Microsoft.Office.Interop.Excel.Application();xlapp.Visible = true;Microsoft.Office.Interop.Excel.Workbooks mBooks = (Microsoft.Office.Interop.Excel.Workbooks)xlapp.Workbooks;Microsoft.Office.Interop.Excel.Workbook mBook = (Microsoft.Office.Interop.Excel.Workbook)mBooks.Add(miss);Microsoft.Office.Interop.Excel.Worksheet mSheet = (Microsoft.Office.Interop.Excel.Worksheet)mBook.ActiveSheet;Microsoft.Office.Interop.Excel.Range mRange = mSheet.get_Range((object)"A1",System.Reflection.Missing.Value);//设置对齐方式mSheet.Cells.HorizontalAlignment = XlHAlign.xlHAlignCenter;//设置文字自动换行//mSheet.Cells.WrapText = true;//设置第一行高度,即标题栏((Microsoft.Office.Interop.Excel.Range)mSheet.Rows["1:1", System.Type.Missing]).RowHeight = 20;//设置数据行行高度((Microsoft.Office.Interop.Excel.Range)mSheet.Rows["2:" + gridView.RowCount + 1, System.Type.Missing]).RowHeight = 16;//设置字体大小(10号字体)mSheet.Range[mSheet.Cells[1, 1], mSheet.Cells[gridView.RowCount + 1, gridView.Columns.Count]].Font.Size = 10;//设置单元格边框Microsoft.Office.Interop.Excel.Range range1 = mSheet.Range[mSheet.Cells[1, 1], mSheet.Cells[gridView.RowCount + 1, gridView.Columns.Count]];range1.Borders.LineStyle = 1;//写标题for (int row = 1; row <= gridView.Columns.Count; row++){mSheet.Cells[1, row] = gridView.Columns[row - 1].GetTextCaption();}try{for (int i = 0; i < gridView.RowCount; i++){rowIndex++;colIndex = 0;for (int j = 0; j < gridView.Columns.Count; j++){colIndex++;mSheet.Cells[rowIndex, colIndex] = gridView.GetRowCellValue(i, gridView.Columns[j]);}}mBook.SaveAs(sfd.FileName,Microsoft.Office.Interop.Excel.XlFileFormat.xlExcel7, miss, miss, miss, miss, Microsoft.Office.Interop.Excel.XlSaveAsAccessMode.xlNoChange, miss, miss, miss, miss, miss);//return true;}catch (Exception ex){//throw new Exception(ex.Message);}finally{//mBook.Close(false, miss, miss);mBooks.Close();xlapp.Quit();System.Runtime.InteropServices.Marshal.ReleaseComObject (mRange);System.Runtime.InteropServices.Marshal.ReleaseComObject (mSheet);System.Runtime.InteropServices.Marshal.ReleaseComObject (mBook);System.Runtime.InteropServices.Marshal.ReleaseComObject (mBooks);System.Runtime.InteropServices.Marshal.ReleaseComObject (xlapp);GC.Collect();}}else{//return false;}}}}。
.net把datatable导出到excel

.net把datatable导出到exceldsResult = BaseClass.Query(sql);#region 用NPOI方式导出,数据量过多则抛出内存溢出异常//using (System.IO.MemoryStream ms = Util.DataSetToExcel(dsResult))//{// string pathtmp = folder + name + ".xlsx";// using (System.IO.FileStream fs = new System.IO.FileStream(pathtmp, System.IO.FileMode.Create, System.IO.FileAccess.Write))// {// byte[] data = ms.ToArray();// fs.Write(data, 0, data.Length);// fs.Flush();// }//}#endregion//用数据流的方式导出//string path = folder + name + ".xlsx";//ExcelUtil.ExportT oExcel(dsResult.Tables[0], path);//用EPPlus.dll导出Excel(xlsx)string path = folder + name + ".xlsx";ExcelExport.ExportByEPPlus(table,dsResult.Tables[0], path);using System.Data;using System.IO;using erModel;using erModel;using erModel;using NPOI;using NPOI.HPSF;using NPOI.HSSF;using NPOI.HSSF.Util;using NPOI.POIFS;using NPOI.SS.Formula.Eval;using NPOI.Util;using NPOI.SS;using NPOI.DDF;using NPOI.SS.Util;using System.Collections;using System.Text.RegularExpressions;using NPOI.XSSF;using System.Text;using OfficeOpenXml;using OfficeOpenXml.Style;namespace Cis.DRGsSystem.Dal{/// <summary>/// 用NPOI插件导出Excel,如果数据量过大则抛内存溢出错误,改用数据流的方式导出Excel/// </summary>public static class Util{/// <summary>/// 拆分字符串1,2,3,4,5/// </summary>/// <param name="strid">1,2,3,4,5</param>/// <returns></returns>public static string StrArr(this string strid){string StrValue = "";if (!string.IsNullOrEmpty(strid)){string[] strarr = strid.Split(',');foreach (string item in strarr){StrValue += "'" + item.Trim() + "',";}StrValue = StrValue.Substring(0, StrValue.Length - 1);}return StrValue;}#region DataSet导出到Excel/// <summary>/// DataSet导出到Excel的MemoryStream/// </summary>/// <param name="dtSource">源DataSet</param> public static MemoryStream DataSetToExcel(DataSet ds) {XSSFWorkbook workbook = new XSSFWorkbook();for (int k = 0; k < ds.Tables.Count; k++){// HSSFSheet sheet = (HSSFSheet)workbook.CreateSheet();XSSFSheet sheet = (XSSFSheet)workbook.CreateSheet(ds.Tables[k].TableName.ToStr ing());#region 右击文件属性信息{DocumentSummaryInformation dsi = PropertySetFactory.CreateDocumentSummaryInformation();pany = "NPOI";// workbook.DocumentSummaryInformation = dsi;SummaryInformation si = PropertySetFactory.CreateSummaryInformation();si.Author = "文件作者信息"; //填加xls文件作者信息si.ApplicationName = "创建程序信息"; //填加xls文件创建程序信息stAuthor = "最后保存者信息"; //填加xls文件最后保存者信息ments = "作者信息"; //填加xls文件作者信息si.Title = "标题信息"; //填加xls文件标题信息si.Subject = "主题信息";//填加文件主题信息si.CreateDateTime = System.DateTime.Now;}#endregionXSSFCellStyle dateStyle = (XSSFCellStyle)workbook.CreateCellStyle();XSSFDataFormat format = (XSSFDataFormat)workbook.CreateDataFormat();dateStyle.DataFormat = format.GetFormat("yyyy-mm-dd");//取得列宽int rowIndex = 0;foreach (DataRow row in ds.Tables[k].Rows){#region 新建表,填充表头,填充列头,样式if (rowIndex == 0){#region 列头及样式{XSSFRow headerRow = (XSSFRow)sheet.CreateRow(0);XSSFCellStyle headStyle = (XSSFCellStyle)workbook.CreateCellStyle();//headStyle.Alignment = CellHorizontalAlignment.CENTER;XSSFFont font = (XSSFFont)workbook.CreateFont();font.FontHeightInPoints = 10;font.Boldweight = 700;headStyle.SetFont(font);foreach (DataColumn column in ds.T ables[k].Columns){headerRow.CreateCell(column.Ordinal).SetCellValue(column. ColumnName);headerRow.GetCell(column.Ordinal).CellStyle = headStyle;//设置列宽//sheet.SetColumnWidth(column.Ordinal, (arrColWidth[column.Ordinal] + 1) * 256);}}#endregionrowIndex = 1;}#endregion#region 填充内容XSSFRow dataRow = (XSSFRow)sheet.CreateRow(rowIndex);foreach (DataColumn column in ds.T ables[k].Columns){XSSFCell newCell = (XSSFCell)dataRow.CreateCell(column.Ordinal);newCell.SetCellType(CellType.String);string drValue = row[column].ToString();switch (column.DataType.ToString()){case "System.String"://字符串类型newCell.SetCellValue(drValue);break;case "System.DateTime"://日期类型System.DateTime dateV;System.DateTime.TryParse(drValue, out dateV);newCell.SetCellValue(dateV);newCell.CellStyle = dateStyle;//格式化显示break;case "System.Boolean"://布尔型bool boolV = false;bool.TryParse(drValue, out boolV); newCell.SetCellValue(boolV); break;case "System.Int16"://整型case "System.Int32":case "System.Int64":case "System.Byte":int intV = 0;int.TryParse(drValue, out intV); newCell.SetCellValue(intV); break;case "System.Decimal"://浮点型case "System.Double":double doubV = 0;double.TryParse(drValue, out doubV); newCell.SetCellValue(doubV); break;case "System.DBNull"://空值处理newCell.SetCellValue("");break;default:newCell.SetCellValue("");break;}}#endregionrowIndex++;}}using (MemoryStream ms = new MemoryStream()){workbook.Write(ms);ms.Flush();return ms;}}#endregion}/// <summary>/// 用数据流方式导出Excel/// </summary>public class ExcelUtil{/// <summary>/// 导出文件,使用文件流。
asp.net生成Excel并导出下载五种实现方法

⽣成Excel并导出下载五种实现⽅法通过GridView(简评:⽅法⽐较简单,但是只适合⽣成格式简单的Excel,且⽆法保留VBA代码),页⾯⽆刷新aspx.cs部分复制代码代码如下:using System; using System.Collections; using System.Configuration; using System.Data; using System.Web; using System.Web.Security; using System.Web.UI; using System.Web.UI.HtmlControls; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Text; public partial class DataPage_NationDataShow : System.Web.UI.Page { private Data_Link link = new Data_Link(); private string sql; protected void Page_Load(object sender, EventArgs e) { Ajax.Utility.RegisterTypeForAjax(typeof(DataPage_NationDataShow)); } protected void btnExcel_Click(object sender, EventArgs e) { string strExcelName = "MyExcel"; strExcelName = strExcelName.Replace(@"/", ""); Data_Link link = new Data_Link(); string strSQL = this.hidParam.Value; DataSet ds = new DataSet(); ds = link.D_DataSet_Return(strSQL);//获得想要放⼊Excel的数据 gvExcel.Visible = true; gvExcel.DataSource = null; gvExcel.DataMember = ds.Tables[0].TableName; gvExcel.DataSource = ds.Tables[0]; gvExcel.DataBind(); ExportToExcel(this.Page, gvExcel, strExcelName); } protected void gvExcel_RowDataBound(object sender, GridViewRowEventArgs e) { } public override void VerifyRenderingInServerForm(Control control) { } /// <summary> /// ⼯具⽅法,Excel出⼒(解决乱码问题) /// </summary> ///<param name="page">调⽤页⾯</param> /// <param name="excel">Excel数据</param> /// <param name="fileName">⽂件名</param> public void ExportToExcel(System.Web.UI.Page page, GridView excel, string fileName) { try { foreach (GridViewRow row in excel.Rows) { for (int i = 0; i < row.Cells.Count; i++) { excel.HeaderRow.Cells[i].BackColor = System.Drawing.Color.Yellow; } } excel.Font.Size = 10; excel.AlternatingRowStyle.BackColor =System.Drawing.Color.LightCyan; excel.RowStyle.Height = 25; page.Response.AppendHeader("Content-Disposition", "attachment;filename=" + fileName); page.Response.Charset = "utf-8"; page.Response.ContentType = "application/vnd.ms-excel"; page.Response.Write("<meta http-equiv=Content-Type content=text/html;charset=utf-8>");excel.Page.EnableViewState = false; excel.Visible = true; excel.HeaderStyle.Reset(); excel.AlternatingRowStyle.Reset(); System.IO.StringWriter oStringWriter = new System.IO.StringWriter(); System.Web.UI.HtmlTextWriter oHtmlTextWriter = new System.Web.UI.HtmlTextWriter(oStringWriter); excel.RenderControl(oHtmlTextWriter);page.Response.Write(oStringWriter.ToString()); page.Response.End(); excel.DataSource = null; excel.Visible = false; } catch (Exception e) { } } }aspx部分复制代码代码如下:<head runat="server"> <script type="text/javascript"> //Excel DownLoad function excelExport(){ var hidText = document.getElementById("hidParam"); hidText.value = "some params"; document.getElementById("ExcelOutput").click(); } </script> </head> <body onload="pageInit()"> <form id="form1"runat="server"> <input type="button" value="EXCEL下载" style="width:100px;" onclick="excelExport()" id="excelBut" /><input id="hidParam" type="text" runat="server" style="display:none;"/> <asp:Button runat="server" ID="ExcelOutput"style="display:none" Text= "EXCEL出⼒" Width="0px" onclick="btnExcel_Click" UseSubmitBehavior="false"/><asp:GridView ID="gvExcel" runat="server" Height="95px" OnRowDataBound="gvExcel_RowDataBound" Visible="False"> </asp:GridView> </form> </body>在刚才的aspx.cs代码中复制代码代码如下:foreach (GridViewRow row in excel.Rows) { for (int i = 0; i < row.Cells.Count; i++) { excel.HeaderRow.Cells[i].BackColor = System.Drawing.Color.Yellow; } }这部分是给表头添加样式。
C#中datatable导出excel(三种方法)

C#中datatable导出excel(三种⽅法)⽅法⼀:(拷贝直接可以使⽤,适合⼤批量资料, 上万笔)Microsoft.Office.Interop.Excel.Application appexcel = new Microsoft.Office.Interop.Excel.Application();SaveFileDialog savefiledialog = new SaveFileDialog();System.Reflection.Missing miss = System.Reflection.Missing.Value;appexcel = new Microsoft.Office.Interop.Excel.Application();Microsoft.Office.Interop.Excel.Workbook workbookdata;Microsoft.Office.Interop.Excel.Worksheet worksheetdata;Microsoft.Office.Interop.Excel.Range rangedata;//设置对象不可见appexcel.Visible = false;System.Globalization.CultureInfo currentci = System.Threading.Thread.CurrentThread.CurrentCulture;System.Threading.Thread.CurrentThread.CurrentCulture = new System.Globalization.CultureInfo("en-us"); workbookdata = appexcel.Workbooks.Add(miss);worksheetdata = (Microsoft.Office.Interop.Excel.Worksheet)workbookdata.Worksheets.Add(miss, miss, miss, miss);//给⼯作表赋名称 = "saved";for (int i = 0; i < dt.Columns.Count; i++){worksheetdata.Cells[1, i + 1] = dt.Columns[i].ColumnName.ToString();}//因为第⼀⾏已经写了表头,所以所有数据都应该从a2开始rangedata = worksheetdata.get_Range("a2", miss);Microsoft.Office.Interop.Excel.Range xlrang = null;//irowcount为实际⾏数,最⼤⾏int irowcount = dt.Rows.Count;int iparstedrow = 0, icurrsize = 0;//ieachsize为每次写⾏的数值,可以⾃⼰设置int ieachsize = 1000;//icolumnaccount为实际列数,最⼤列数int icolumnaccount = dt.Columns.Count;//在内存中声明⼀个ieachsize×icolumnaccount的数组,ieachsize是每次最⼤存储的⾏数,icolumnaccount就是存储的实际列数object[,] objval = new object[ieachsize, icolumnaccount];icurrsize = ieachsize;while (iparstedrow < irowcount){if ((irowcount - iparstedrow) < ieachsize)icurrsize = irowcount - iparstedrow;//⽤for循环给数组赋值for (int i = 0; i < icurrsize; i++){for (int j = 0; j < icolumnaccount; j++)objval[i, j] = dt.Rows[i + iparstedrow][j].ToString();System.Windows.Forms.Application.DoEvents();}string X = "A" + ((int)(iparstedrow + 2)).ToString();string col = "";if (icolumnaccount <= 26){col = ((char)('A' + icolumnaccount - 1)).ToString() + ((int)(iparstedrow + icurrsize + 1)).ToString();}else{col = ((char)('A' + (icolumnaccount / 26 - 1))).ToString() + ((char)('A' + (icolumnaccount % 26 - 1))).ToString() + ((int)(iparstedrow + icurrsize + 1)).ToString();}xlrang = worksheetdata.get_Range(X, col);// 调⽤range的value2属性,把内存中的值赋给excelxlrang.Value2 = objval;iparstedrow = iparstedrow + icurrsize;}//保存⼯作表System.Runtime.InteropServices.Marshal.ReleaseComObject(xlrang);xlrang = null;//调⽤⽅法关闭excel进程appexcel.Visible = true;⽅法⼆:(⾃⼰建函数,适合⼤批量资料, 上万笔)using System.IO;private void dataTableToCsv(DataTable table, string file){string title = "";FileStream fs = new FileStream(file, FileMode.OpenOrCreate);//FileStream fs1 = File.Open(file, FileMode.Open, FileAccess.Read);StreamWriter sw = new StreamWriter(new BufferedStream(fs), System.Text.Encoding.Default); for (int i = 0; i < table.Columns.Count; i++){title += table.Columns[i].ColumnName + "\t"; //栏位:⾃动跳到下⼀单元格}title = title.Substring(0, title.Length - 1) + "\n";sw.Write(title);foreach (DataRow row in table.Rows){string line = "";for (int i = 0; i < table.Columns.Count; i++){line += row[i].ToString().Trim() + "\t"; //内容:⾃动跳到下⼀单元格}line = line.Substring(0, line.Length - 1) + "\n";sw.Write(line);}sw.Close();fs.Close();}dataTableToCsv(dt, @"c:\1.xls"); //调⽤函数System.Diagnostics.Process.Start(@"c:\1.xls"); //打开excel⽂件⽅法三:(可以⾃⼰调整单元格的格式,适合⼩批量的数量)//没有数据的话就不往下执⾏if (dataGridView1.Rows.Count == 0)return;//实例化⼀个Excel.Application对象Microsoft.Office.Interop.Excel.Application excel = new Microsoft.Office.Interop.Excel.Application();//让后台执⾏设置为不可见,为true的话会看到打开⼀个Excel,然后数据在往⾥写//excel.Visible = false;excel.Visible = true;//新增加⼀个⼯作簿,Workbook是直接保存,不会弹出保存对话框,加上Application会弹出保存对话框,值为false会报错 excel.Application.Workbooks.Add(true);//⽣成Excel中列头名称for (int i = 0; i < dataGridView1.Columns.Count; i++){excel.Cells[1, i + 1] = dataGridView1.Columns[i].HeaderText;}//把DataGridView当前页的数据保存在Excel中for (int i = 0; i < dataGridView1.Rows.Count - 1; i++){for (int j = 0; j < dataGridView1.Columns.Count; j++){if (dataGridView1[j, i].ValueType == typeof(string)){excel.Cells[i + 2, j + 1] = "'" + dataGridView1[j, i].Value.ToString();}else{excel.Cells[i + 2, j + 1] = dataGridView1[j, i].Value.ToString();}}}//设置禁⽌弹出保存和覆盖的询问提⽰框excel.DisplayAlerts = false;excel.AlertBeforeOverwriting = false;////保存⼯作簿//excel.Application.Workbooks.Add(true).Save();////保存excel⽂件//excel.Save("D:" + "\\KKHMD.xls");////确保Excel进程关闭//excel.Quit(); //可以直接打开⽂件//excel = null;}catch (Exception ex){MessageBox.Show(ex.Message, "错误提⽰");}Excel.output((DataTable)dataGridView1.DataSource);。
把WinForm的DataGridView的数据导出到Excel三种方法

把WinForm的DataGridView的数据导出到Excel三种方法导出WinForm的DataGridView数据到Excel有多种方法,下面将详细介绍三种常用的方法:方法一:使用Microsoft.Office.Interop.Excel库这是一种常用的方法,使用Microsoft.Office.Interop.Excel库可以直接操作Excel文件。
首先,需要在项目中添加对Microsoft Office 的引用。
然后,可以按照以下步骤导出数据:1. 创建一个Excel应用程序对象:```csharpusing Excel = Microsoft.Office.Interop.Excel;Excel.Application excelApp = new Excel.Application(;```2.创建一个工作簿对象:```csharpExcel.Workbook workbook =excelApp.Workbooks.Add(Type.Missing);```3.创建一个工作表对象:```csharpExcel.Worksheet worksheet = workbook.ActiveSheet;```4. 将DataGridView中的数据导入到Excel中:```csharpfor (int i = 0; i < dataGridView.Rows.Count; i++)for (int j = 0; j < dataGridView.Columns.Count; j++)worksheet.Cells[i + 1, j + 1] =dataGridView.Rows[i].Cells[j].Value.ToString(;}```5. 保存Excel文件并关闭Excel应用程序:```csharpworkbook.SaveAs("路径\\文件名.xlsx");excelApp.Quit(;```方法二:使用OpenXml库OpenXml是一种用于操作Office文件的开放式标准。
导出Excel的四种方法
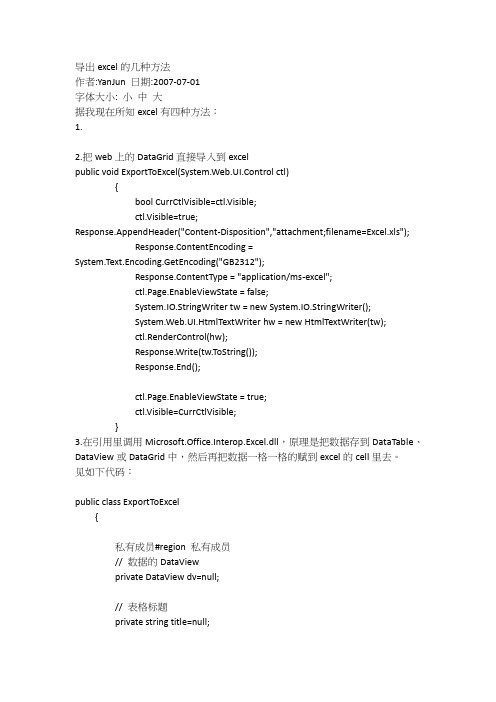
/// </summary>
public string OutFilePath
{
set
{
outFilePath=value;
}
get
{
return outFilePath;
}
}
/**//// <summary>
///输入文件ቤተ መጻሕፍቲ ባይዱ径
/// </summary>
private string InputFilePath
//
// }
#endregion
公共方法#region公共方法
/**///
public void CreateExcel()
{
int rowIndex=4;//行起始坐标
int colIndex=1;//列起始坐标
ApplicationClass myApp=null;
Workbook myBook=null;
mySheet.get_Range(mySheet.Cells[4,2],mySheet.Cells[4,colIndex]).Borders[XlBordersIndex.xlEdgeTop].Weight = XlBorderWeight.xlThick;//设置上边线加粗
mySheet.get_Range(mySheet.Cells[4,colIndex],mySheet.Cells[rowSum,colIndex]).Borders[XlBordersIndex.xlEdgeRight].Weight = XlBorderWeight.xlThick;//设置右边线加粗
mySheet.get_Range(mySheet.Cells[rowSum,colSum],mySheet.Cells[rowSum,colIndex]).Interior.ColorIndex = 19;//设置为浅黄色,共计有56种
VSGridView导出Excel合并方法详细
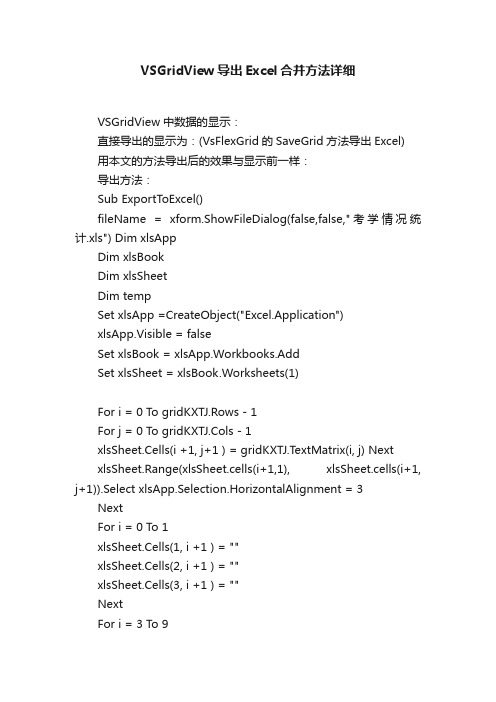
VSGridView导出Excel合并方法详细VSGridView中数据的显示:直接导出的显示为:(VsFlexGrid的SaveGrid方法导出Excel)用本文的方法导出后的效果与显示前一样:导出方法:Sub ExportToExcel()fileName = xform.ShowFileDialog(false,false,"考学情况统计.xls") Dim xlsAppDim xlsBookDim xlsSheetDim tempSet xlsApp =CreateObject("Excel.Application")xlsApp.Visible = falseSet xlsBook = xlsApp.Workbooks.AddSet xlsSheet = xlsBook.Worksheets(1)For i = 0 To gridKXTJ.Rows - 1For j = 0 To gridKXTJ.Cols - 1xlsSheet.Cells(i +1, j+1 ) = gridKXTJ.T extMatrix(i, j) NextxlsSheet.Range(xlsSheet.cells(i+1,1), xlsSheet.cells(i+1, j+1)).Select xlsApp.Selection.HorizontalAlignment = 3 NextFor i = 0 To 1xlsSheet.Cells(1, i +1 ) = ""xlsSheet.Cells(2, i +1 ) = ""xlsSheet.Cells(3, i +1 ) = ""NextFor i = 3 To 9xlsSheet.Cells(1, i ) = ""NextFor i = 5 To 8xlsSheet.Cells(2, i ) = ""NextxlsSheet.Cells(2,3)=""xlsSheet.Cells(3,3)=""xlsSheet.Cells(2,4)=""xlsSheet.Cells(3,4)=""xlsSheet.Cells(2,9)=""xlsSheet.Cells(3,9)=""xlsSheet.Range(xlsSheet.cells(1, 1), xlsSheet.cells(3, 1)).Select xlsApp.Selection.MergeCells = TruexlsApp.Selection.HorizontalAlignment = 3xlsSheet.Cells(1, 1) = "序号"xlsSheet.Range(xlsSheet.cells(1, 2), xlsSheet.cells(3, 2)).Select xlsApp.Selection.MergeCells = TruexlsApp.Selection.HorizontalAlignment = 3xlsSheet.Cells(1, 2) = "姓名"xlsSheet.Range(xlsSheet.cells(1, 3), xlsSheet.cells(1, 9)).Select xlsApp.Selection.MergeCells = True'xlsApp.Selection.HorizontalAlignment = 3xlsSheet.Cells(1, 3) = "考学"xlsSheet.Range(xlsSheet.cells(2, 3), xlsSheet.cells(3, 3)).Select xlsApp.Selection.MergeCells = TruexlsApp.Selection.HorizontalAlignment = 3xlsSheet.Cells(2, 3) = "学习得分"xlsSheet.Range(xlsSheet.cells(2, 4), xlsSheet.cells(3, 4)).Select xlsApp.Selection.MergeCells = TruexlsApp.Selection.HorizontalAlignment = 3xlsSheet.Cells(2, 4) = "考试得分"xlsSheet.Range(xlsSheet.cells(2,5), xlsSheet.cells(2, 8)).Select xlsApp.Selection.MergeCells = TruexlsApp.Selection.HorizontalAlignment = 3xlsSheet.Cells(2, 5) = "参加集中学习"xlsSheet.Range(xlsSheet.cells(2,9), xlsSheet.cells(3, 9)).Select xlsApp.Selection.MergeCells = TruexlsApp.Selection.HorizontalAlignment = 3xlsSheet.Cells(2, 9) = "扣分"xlsBook.Saveas(fileName)Set xlsSheet=NothingSet xlsBook = NothingSet xlsApp = NothingEnd Sub。
gridview --导出execl 方法及身份证号灯数据类型被转换为数字导致数据错误。及导出数据方法。
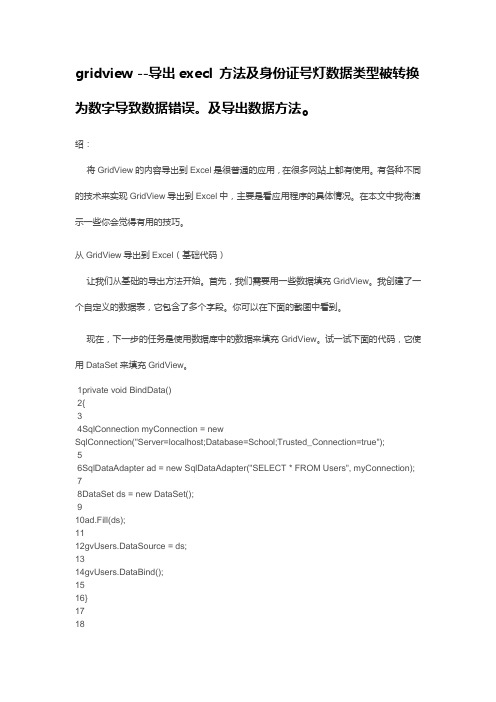
gridview --导出execl 方法及身份证号灯数据类型被转换为数字导致数据错误。
及导出数据方法。
绍:将GridView的内容导出到Excel是很普通的应用,在很多网站上都有使用。
有各种不同的技术来实现GridView导出到Excel中,主要是看应用程序的具体情况。
在本文中我将演示一些你会觉得有用的技巧。
从GridView导出到Excel(基础代码)让我们从基础的导出方法开始。
首先,我们需要用一些数据填充GridView。
我创建了一个自定义的数据表,它包含了多个字段。
你可以在下面的截图中看到。
现在,下一步的任务是使用数据库中的数据来填充GridView。
试一试下面的代码,它使用DataSet来填充GridView。
1private void BindData()2{34SqlConnection myConnection = newSqlConnection("Server=localhost;Database=School;Trusted_Connection=true");56SqlDataAdapter ad = new SqlDataAdapter("SELECT * FROM Users", myConnection);78DataSet ds = new DataSet();910ad.Fill(ds);1112gvUsers.DataSource = ds;1314gvUsers.DataBind();1516}1718所以GridView现在已经填充了数据。
接下去的任务就是将GridView导出到Excel。
你可以在button的click事件中使用下面的代码。
1Response.ClearContent();23Response.AddHeader("content-disposition", "attachment; filename=MyExcelFile.xls");45Response.ContentType = "application/excel";67StringWriter sw = new StringWriter();89HtmlTextWriter htw = new HtmlTextWriter(sw);1011gvUsers.RenderControl(htw);1213Response.Write(sw.ToString());1415Response.End();16你同时还要重载VerifyRenderingInServerForm方法。
Web导出excel的几种方法

Web导出excel的几种方法Web导出excel的几种方法ExcelWebIE浏览器应用服务器在项目中我们经常遇到需要将报表导出成excel下载保存下来,在不同的要求和不同的资源情况下我们有不同的处理方式。
在这里我将谈谈我所遇到的几种情况和遇到的一些问题。
总的来说可以分为:服务器端生成和浏览器端生成2种方法。
一、服务器端生成就是:根据用户请求,获取相应的数据,使用poi/jxl, jacob/jawin+excel或是用数据拼html的table或是cvs纯文本的数据格式等。
然后按.xls或是.cvs格式的文件的形式返回给用户,指定Content-Type:application/vnd.ms-excel ,浏览器就会提示要下载的文件是excel文件。
一般情况下采用这种方式的话就可以根据具体要求对excel文件进行细致的处理,各方面样式和排列可以自己控制使用,而且是可以跨平台使用的。
缺点就是毕竟需要根据不同的表格进行不断的coding,服务器也有一定的压力,这里我就不进行例子解释了。
二、浏览器端生成excel文件还没有特别完善的方案,这是因为js 无法处理二进制。
大概有以下几个方案,各有利弊1.activex方式:使用js调用excel对象,传入需要的参数直接导出excel。
这种方式代码简单,速度较快,前段时间用过2种采用这种方式的方法,各有千秋:A:页面table拷贝法:var curTbl = document.getElementById(tableid);var oXL = new ActiveXObject("Excel.Application");var oWB = oXL.Workbooks.Add(); //创建AX对象excelvar oSheet = oWB.ActiveSheet; //获取workbook对象var sel = document.body.createTextRange(); //激活当前sel.moveToElementText(curTbl); //把表格中的内容移到TextRange中sel.select(); //全选TextRange中内容sel.execCommand("Copy"); //复制TextRange中内容oSheet.Paste(); //粘贴到活动的EXCEL中oXL.Visible = true; //设置excel可见属性如上代码可见,这个方法需要传入的就是table的ID,通过拷贝table复制到excel的方式执行,这个方法的特点是可见即所得,当然页面格子大小不能控制精细,只能得到当前页面展示的table部分,如table做了分页处理的话,直接导出的也只是当前页面部分。
从DBGridEh控件导出Excel

从DBGridEh控件导出Excel从DBGridEh控件导出Excel(收藏)1、调用(通过点击按钮直接导出Excel):procedure TForm3.Button2Click(Sender: TObject);varGridtoExcel: TDBGridEhToExcel;begintryGridtoExcel := TDBGridEhT oExcel.Create(nil);GridtoExcel.DBGridEh := DBGridEh1;GridtoExcel.TitleName := '收费明细表';GridtoExcel.ShowProgress := true;GridtoExcel.ShowOpenExcel := true;GridtoExcel.ExportToExcel;finallyGridtoExcel.Free;end;end;2、具体的导出函数unit Unit_DBGridEhToExcel;interfaceusesSysUtils, Variants, Classes, Graphics, Controls, Forms, Excel2000, ComObj,Dialogs, DB, DBGridEh, windows,ComCtrls,ExtCtrls;typeTDBGridEhToExcel = class(TComponent)privateFProgressForm: TForm; {进度窗体}FtempGauge: TProgressBar; {进度条}FShowProgress: Boolean; {是否显示进度窗体}FShowOpenExcel:Boolean; {是否导出后打开Excel文件}FDBGridEh: TDBGridEh;FTitleName: TCaption; {Excel文件标题} FUserName: TCaption; {制表人}procedure SetShowProgress(const Value: Boolean); {是否显示进度条}procedure SetShowOpenExcel(const Value: Boolean); {是否打开生成的Excel文件}procedure SetDBGridEh(const Value: TDBGridEh);procedure SetTitleName(const Value: TCaption); {标题名称}procedure SetUserName(const Value: TCaption); {使用人名称}procedure CreateProcessForm(AOwner: TComponent); {生成进度窗体}publicconstructor Create(AOwner: TComponent); override;destructor Destroy; override;procedure ExportT oExcel; {输出Excel文件}publishedproperty DBGridEh: TDBGridEh read FDBGridEh writeSetDBGridEh;property ShowProgress: Boolean read FShowProgress write SetShowProgress; //是否显示进度条property ShowOpenExcel: Boolean read FShowOpenExcel write SetShowOpenExcel; //是否打开Excelproperty TitleName: TCaption read FTitleName write SetTitleName;property UserName: TCaption read FUserName write SetUserName;end;implementationconstructor TDBGridEhToExcel.Create(AOwner: TComponent);begininherited Create(AOwner);FShowProgress := True;FShowOpenExcel:= True;end;procedure TDBGridEhToExcel.SetShowProgress(const Value: Boolean);beginFShowProgress := Value;end;procedure TDBGridEhT oExcel.SetDBGridEh(const Value: TDBGridEh);beginFDBGridEh := Value;end;procedure TDBGridEhT oExcel.SetTitleName(const Value: TCaption);beginFTitleName := Value;end;procedure TDBGridEhT oExcel.SetUserName(const Value: TCaption);beginFUserName := Value;end;function IsFileInUse(fName: string ): boolean;varHFileRes: HFILE;beginResult :=false;if not FileExists(fName) then exit;HFileRes :=CreateFile(pchar(fName), GENERIC_READor GENERIC_WRITE,0, nil,OPEN_EXISTING,FILE_ATTRIBUTE_NORMAL, 0);Result :=(HFileRes=INVALID_HANDLE_VALUE);if not Result thenCloseHandle(HFileRes);end;procedure TDBGridEhToExcel.ExportToExcel;XLApp: Variant;Sheet: Variant;s1, s2: string;Caption,Msg: String;Row, Col: integer;iCount, jCount: Integer;FBookMark: TBookmark;FileName: String;SaveDialog1: TSaveDialog;begin//如果数据集为空或没有打开则退出if not DBGridEh.DataSource.DataSet.Active then Exit;SaveDialog1 := TSaveDialog.Create(Nil);SaveDialog1.FileName := TitleName + '_' + FormatDateTime('YYMMDDHHmmSS', now);SaveDialog1.Filter := 'Excel文件|*.xls';if SaveDialog1.Execute thenFileName := SaveDialog1.FileName;SaveDialog1.Free;if FileName = '' then Exit;while IsFileInUse(FileName) dobeginif Application.MessageBox('目标文件使用中,请退出目标文件后点击确定继续!','注意', MB_OKCANCEL + MB_ICONWARNING) = IDOK then beginelsebeginExit;end;end;if FileExists(FileName) thenbeginMsg := '已存在文件(' + FileName + '),是否覆盖?';if Application.MessageBox(PChar(Msg), '提示', MB_YESNO + MB_ICONQUESTION + MB_DEFBUTTON2) = IDYES then begin//删除文件DeleteFile(PChar(FileName))endelseexit;end;Application.ProcessMessages;Screen.Cursor := crHourGlass;//显示进度窗体if ShowProgress thenCreateProcessForm(nil);if not VarIsEmpty(XLApp) thenbeginXLApp.DisplayAlerts := False;XLApp.Quit;VarClear(XLApp);end;//通过ole创建Excel对象tryXLApp := CreateOleObject('Excel.Application');exceptMessageDlg('创建Excel对象失败,请检查你的系统是否正确安装了Excel软件!', mtError, [mbOk], 0);Screen.Cursor := crDefault;Exit;end;//生成工作页XLApp.WorkBooks.Add[XLWBatWorksheet];XLApp.WorkBooks[1].WorkSheets[1].Name := TitleName;Sheet := XLApp.Workbooks[1].WorkSheets[TitleName];//写标题sheet.cells[1, 1] := TitleName;sheet.range[sheet.cells[1, 1], sheet.cells[1, DBGridEh.Columns.Count]].Select; //选择该列XLApp.selection.HorizontalAlignment := $FFFFEFF4; //居中XLApp.selection.MergeCells := True; //合并//写表头Row := 1;jCount := 3;for iCount := 0 to DBGridEh.Columns.Count - 1 dobeginCol := 2;Row := iCount+1;Caption := DBGridEh.Columns[iCount].Title.Caption;while POS('|', Caption) > 0 dobeginjCount := 4;s1 := Copy(Caption, 1, Pos('|',Caption)-1);if s2 = s1 thenbeginsheet.range[sheet.cells[Col, Row-1],sheet.cells[Col, Row]].Select;XLApp.selection.HorizontalAlignment := $FFFFEFF4;XLApp.selection.MergeCells := True;endelseSheet.cells[Col,Row] := Copy(Caption, 1, Pos('|',Caption)-1);Caption := Copy(Caption,Pos('|', Caption)+1, Length(Caption));Inc(Col);s2 := s1;end;Sheet.cells[Col, Row] := Caption;Inc(Row);end;//合并表头并居中if jCount = 4 thenfor iCount := 1 to DBGridEh.Columns.Count doif Sheet.cells[3, iCount].Value = '' thenbeginsheet.range[sheet.cells[2, iCount],sheet.cells[3, iCount]].Select;XLApp.selection.HorizontalAlignment := $FFFFEFF4;XLApp.selection.MergeCells := True;endelse beginsheet.cells[3, iCount].Select;XLApp.selection.HorizontalAlignment := $FFFFEFF4;end;//读取数据DBGridEh.DataSource.DataSet.DisableControls;FBookMark := DBGridEh.DataSource.DataSet.GetBookmark;DBGridEh.DataSource.DataSet.First;while not DBGridEh.DataSource.DataSet.Eof dobeginfor iCount := 1 to DBGridEh.Columns.Count dobegin//Sheet.cells[jCount,iCount] :=DBGridEh.Columns.Items[iCount-1].Field.AsString;caseDBGridEh.DataSource.DataSet.FieldByName(DBGridEh.Columns. Items[iCount-1].FieldName).DataType offtSmallint, ftInteger, ftWord, ftAutoInc, ftBytes:Sheet.cells[jCount,iCount] :=DBGridEh.Columns.Items[iCount-1].Field.asinteger;ftFloat, ftCurrency, ftBCD:Sheet.cells[jCount,iCount] :=DBGridEh.Columns.Items[iCount-1].Field.AsFloat;elseifDBGridEh.DataSource.DataSet.FieldByName(DBGridEh.Columns. Items[iCount-1].FieldName) is TBlobfield then // 此类型的字段(图像等)暂无法读取显示Sheet.cells[jCount,iCount] :=DBGridEh.Columns.Items[iCount-1].Field.AsString elseSheet.cells[jCount,iCount] :=''''+DBGridEh.Columns.Items[iCount-1].Field.AsString;end;end;Inc(jCount);//显示进度条进度过程if ShowProgress thenbeginFtempGauge.Position := DBGridEh.DataSource.DataSet.RecNo;FtempGauge.Refresh;end;DBGridEh.DataSource.DataSet.Next;end;if DBGridEh.DataSource.DataSet.BookmarkValid(FBookMark)thenDBGridEh.DataSource.DataSet.GotoBookmark(FBookMark);DBGridEh.DataSource.DataSet.EnableControls;//读取表脚if DBGridEh.FooterRowCount > 0 thenbeginfor Row := 0 to DBGridEh.FooterRowCount-1 dobeginfor Col := 0 to DBGridEh.Columns.Count-1 doSheet.cells[jCount, Col+1] := DBGridEh.GetFooterValue(Row,DBGridEh.Columns[Col]);Inc(jCount);end;end;//调整列宽// for iCount := 1 to DBGridEh.Columns.Count do// Sheet.Columns[iCount].EntireColumn.AutoFit;sheet.cells[1, 1].Select;XlApp.Workbooks[1].SaveAs(FileName);XlApp.Visible := True;XlApp := Unassigned;if ShowProgress thenFreeAndNil(FProgressForm);Screen.Cursor := crDefault;end;destructor TDBGridEhToExcel.Destroy;begininherited Destroy;end;procedure TDBGridEhT oExcel.CreateProcessForm(AOwner: TComponent);varPanel: TPanel;beginif Assigned(FProgressForm) thenexit;FProgressForm := TForm.Create(AOwner);with FProgressForm dobegintry := '宋体'; {设置字体}Font.Size := 10;BorderStyle := bsNone;Width := 300;Height := 30;BorderWidth := 1;Color := clBlack;Position := poScreenCenter;Panel := TPanel.Create(FProgressForm);with Panel dobeginParent := FProgressForm;Align := alClient;Caption := '正在导出Excel,请稍候......';Color:=$00E9E5E0;end;FtempGauge:=TProgressBar.Create(Panel);with FtempGauge dobeginParent := Panel;Align:=alClient;Min := 0;Max:= DBGridEh.DataSource.DataSet.RecordCount;Position := 0;end;exceptend;end;FProgressForm.Show;FProgressForm.Update;end;procedure TDBGridEhT oExcel.SetShowOpenExcel(const Value: Boolean);beginFShowOpenExcel:=Value;end;end.用法:先新建一个单元文件比如Unit_DBGridEhToExcel.pas,然后再在工程里面引用这个文件,再直接通过一个按钮事件调用就可以了。
C#数据导入导出Excel文件及winForm导出Execl总结

C#数据导⼊导出Excel⽂件及winForm导出Execl总结在中导出Execl有两种⽅法,⼀种是将导出的⽂件存放在服务器某个⽂件夹下⾯,然后将⽂件地址输出在浏览器上;⼀种是将⽂件直接将⽂件输出流写给浏览器。
在Response输出时,\t分隔的数据,导出execl时,等价于分列,\n等价于换⾏。
此法将html中所有的内容,如按钮,表格,图⽚等全部输出到Execl中。
复制代码代码如下:Response.Clear();Response.Buffer= true;Response.AppendHeader("Content-Disposition","attachment;filename="+DateTime.Now.ToString("yyyyMMdd")+".xls");Response.ContentEncoding=System.Text.Encoding.UTF8;Response.ContentType = "application/vnd.ms-excel";this.EnableViewState = false;这⾥我们利⽤了ContentType属性,它默认的属性为text/html,这时将输出为超⽂本,即我们常见的⽹页格式到客户端,如果改为ms-excel将将输出excel格式,也就是说以电⼦表格的格式输出到客户端,这时浏览器将提⽰你下载保存。
ContentType的属性还包括:image/JPEG;text/HTML;image/GIF;vnd.ms-excel/msword 。
同理,我们也可以输出(导出)图⽚、word⽂档等。
下⾯的⽅法,也均⽤了这个属性。
上述⽅法虽然实现了导出的功能,但同时把按钮、分页框等html中的所有输出信息导了进去。
⽽我们⼀般要导出的是数据,DataGrid控件上的数据。
VB6.0类模块实现DataGrid表格导出到Excel(原创)

VB6.0类模块实现DataGrid表格导出到Excel(原创)将VB中的DataGrid导出到Excel功能网上有很多实例,大家都可以下载后修改修改使用,但是一个程序中多次使用该代码的话,每次都需要修改里面的相关参数,较为麻烦。
作者奉行的理念是“拿来就用”,通过类模块的封装,可以很好的提高代码的重用性,只需要2行代码就可以搞定哦。
本源码复制粘贴后即可使用。
爽吧!如果你用的是其他表格控件,如MSHFlexGrid,则直接将参数中的DataGrid参数换成你的控件类型即可。
如有问题经联系作者****************一、准备工作要想使用本类模块,必须像使用其他导出Excel代码段一样,先引用Microsoft Excel Object Library 。
这是一定要注意的,否则调用该类模块将出错。
二、类模块源码类模块名称:ToExcelOption Explicit'**********************************************************'**函数名:GridToExcel'**输入:needGrid,needAdodc'** :'** :'**输出:'**功能描述:自动将DataGrid表格中的内容导出到Excel'**全局变量:见变量声明'**调用模块:'**作者:吴长平'**日期:2013-1-24 08:45:48'**修改人:吴长平'**日期:'**版本:V1.0.0'**支持:需要引用Excel Object Library'*********************************************************Public Sub GridToExcel(ByVal needGrid As DataGrid, ByVal needAdodc As ADODB.Recordset)'记录集为空时自动报错,并退出过程If needAdodc Is Nothing ThenMsgBox ("参数中的记录集为空!")Exit SubEnd If'定义整数i,jDim i As Integer, j As Integer'定义Excel应用程序对象Dim xlapp As Excel.Application'定义Excel工作薄对象Dim xlbook As Excel.Workbook'定义Excel工作表对象Dim xlsheet As Excel.Worksheet'创建Excel应用程序对象Set xlapp = CreateObject("excel.application") 'Excel应用程序对象可见xlapp.Visible = True'新建工作薄,赋值给xlbookSet xlbook = xlapp.Workbooks.Add'工作薄xlbook的第一个工作表赋值给xlsheet Set xlsheet = xlbook.Worksheets(1)'添加表头For i = 0 To needGrid.Columns.Count - 1xlsheet.Cells(1, i + 1) = needGrid.Columns(i).Caption Next i'循环向Excel表格的单元格中添加内容i = 0'一直输出到记录集的末尾Do While (needAdodc.EOF = False)For j = 0 To Int(needGrid.Columns.Count - 1)needGrid.Col = jxlsheet.Cells(i + 2, j + 1) = needGrid.TextNext j'记录集指针向下移动一条needAdodc.MoveNexti = i + 1Loop'释放Excel工作薄对象Set xlapp = Nothing'释放义Excel工作表对象Set xlbook = NothingEnd Sub三、类模块的使用。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
DataGirdµ¼³öEXCELµÄ¼¸¸ö·½·¨£¨WebControl£©using System;using System.Data;using System.Text;using System.Web;using System.Web.UI;using System.Diagnostics;using System.Web.UI.WebControls;using System.Web.UI.HtmlControls;using System.Data.SqlClient;using System.Collections;namespace bookstore{/// <summary>/// myExcel µÄժҪ˵Ã÷¡£/// </summary>public class myExcel{public myExcel(){}/// <summary>/// ½«DATAGRIDµ¼³öΪEXCELÎļþ·½·¨Ò»,/// ²ÎÊýÊÇ:Òªµ¼³öµÄDATAGRIDµÄIDºÍÒª±£´æÏÂÀ´µÄEXCELÎļþÃû/// </summary>/// <param name="myPage">page</param>/// <param name="dg">datagrid</param>/// <param name="name">filename</param>private void OutExcel(Page myPage,DataGrid dg,string name) {HttpResponse Response;Response=myPage.Response;string name1="attachment;filename="+name+".xls";dg.Visible=true;Response.Clear();Response.Buffer= true;Response.Charset="GB2312";Response.AppendHeader("Content-Disposition",name1);Response.ContentEncoding=System.Text.Encoding.GetEncoding("GB2312" );Response.ContentType ="application/ms-excel";dg.EnableViewState = false;System.IO.StringWriter oStringWriter = new System.IO.StringWriter();System.Web.UI.HtmlTextWriter oHtmlTextWriter = new System.Web.UI.HtmlTextWriter(oStringWriter);dg.RenderControl(oHtmlTextWriter);Response.Write(oStringWriter.ToString());Response.End();}/// <summary>/// ½«DATAGRIDµ¼³öΪEXCELÎļþ·½·¨¶þ,/// ²ÎÊýÊÇ:Òªµ¼³öµÄDATAGRIDµÄIDºÍÒª±£´æÏÂÀ´µÄEXCELÎļþÃû/// </summary>/// <param name="myPage">page</param>/// <param name="ctl">datagrid</param>/// <param name="filename">filename</param>public void ExportToExcel(Page myPage,DataGrid ctl,string filename) {HttpResponse Response;Response=myPage.Response;bool CurrCtlVisible=ctl.Visible;ctl.Visible=true;Response.AppendHeader("Content-Disposition","attachment;filename="+filename+".xls");Response.ContentEncoding = System.Text.Encoding.GetEncoding("utf-8");Response.ContentType = "application/ms-excel";ctl.Page.EnableViewState = false;System.IO.StringWriter tw = new System.IO.StringWriter();System.Web.UI.HtmlTextWriter hw = new HtmlTextWriter(tw);ctl.RenderControl(hw);Response.Write(tw.ToString());Response.End();ctl.Page.EnableViewState = true;ctl.Visible=CurrCtlVisible;}private void DgOutExcel(Page myPage,DataGrid dg,string name){HttpResponse Response;Response=myPage.Response;string name1="attachment;filename="+name+".xls";dg.Visible=true;Response.Clear();Response.Buffer= true;Response.Charset="GB2312";Response.AppendHeader("Content-Disposition",name1);Response.ContentEncoding=System.Text.Encoding.GetEncoding("GB2312" );Response.ContentType ="application/ms-excel";dg.EnableViewState = false;System.IO.StringWriter oStringWriter = new System.IO.StringWriter();System.Web.UI.HtmlTextWriter oHtmlTextWriter = new System.Web.UI.HtmlTextWriter(oStringWriter);dg.RenderControl(oHtmlTextWriter);Response.Write(oStringWriter.ToString());Response.End();}#region µ¼³öEXCEL£¬ÓÃDATASETpublic string myExportString(DataGrid DG,DataSet ds){string HTstring="<table><tr>";string Fieldstring="";ArrayList myAL=new ArrayList();string sRows="<tr>";for(int i=0;i<DG.Columns.Count;i++){HTstring+="<td>"+DG.Columns[i].HeaderText+"</td>";Fieldstring+="<td>"+((System.Web.UI.WebControls.BoundColumn)(DG.Columns[i])).DataField+"</td>";myAL.Add(((System.Web.UI.WebControls.BoundColumn)(DG.Columns[i])).DataField);}for(int k=0;k<ds.Tables[0].Rows.Count;k++){foreach(string field in myAL){sRows+= "<td>"+ds.Tables[0].Rows[k][field]+"</td>";}sRows+="</tr>";}HTstring+="</tr>"+sRows+"</table>";return HTstring;}public void SaveToExcel(Page myPage, string myExportString,stringmyFileName){HttpResponse resp;resp=myPage.Response;resp.ContentEncoding=System.Text.Encoding.GetEncoding("GB2312");resp.AppendHeader("Content-Disposition","attachment;filename="+myFileName+".xls");resp.ContentType="application/ms-excel"; resp.Write(myExportString);resp.End();//resp.Clear();//resp.Close();}public void Export(DataGrid myDG,DataTable dt,HttpResponse response){// clean up response objectresponse.Clear();response.Charset = "";response.Charset = "UTF-8";// response.ContentEncoding = System.Text.Encoding.Default;response.ContentEncoding=System.Text.Encoding.GetEncoding("GB2312" );// set response object's mime type// response.ContentType = "application/vnd.ms-excel";response.AppendHeader("Content-Disposition","attachment;filename=m ytest.xls");System.IO.StringWriter sw = new System.IO.StringWriter();System.Web.UI.HtmlTextWriter htw = new System.Web.UI.HtmlTextWriter(sw);DataGrid dg = new DataGrid();dg.AllowPaging = false;dg.AllowSorting = false;ArrayList myAL=new ArrayList();for(int i=0;i<myDG.Columns.Count;i++){// dg.Columns.AddAt(i,myDG.Columns[i]);// dg.Columns[i].HeaderText=myDG.Columns[i].HeaderText;myAL.Add(((System.Web.UI.WebControls.BoundColumn)(myDG.Columns[i]) ).DataField);for(int k=0;k<dt.Columns.Count;k++){foreach(string field in myAL){if(dt.Columns[i].ColumnName==field){dt.Columns[i].ColumnName=myDG.Columns[i].HeaderText;}}}}dg.DataSource = dt;dg.ShowHeader = true;dg.HeaderStyle.BackColor = System.Drawing.Color.DarkGray;dg.HeaderStyle.ForeColor = System.Drawing.Color.White;dg.HeaderStyle.Font.Bold = true;dg.AlternatingItemStyle.BackColor = System.Drawing.Color.LightGray;dg.DataBind();dg.RenderControl(htw);response.Write(sw.ToString());response.End();}#endregion}}。