纯C语言写的一个小型游戏-源代码
纯C语言写地一个小型游戏源代码
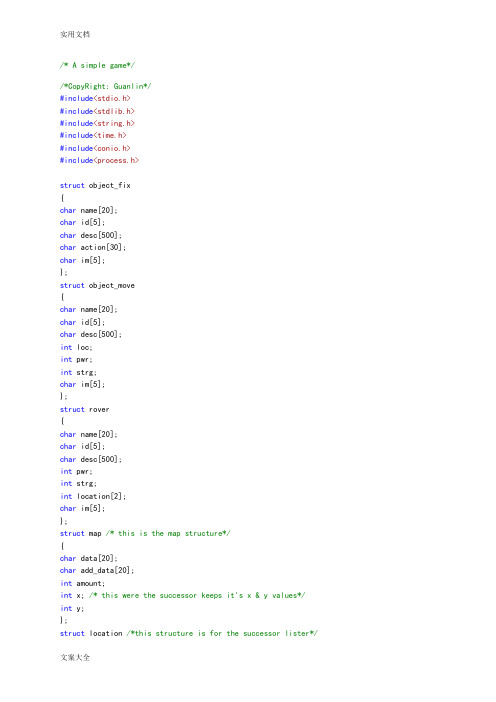
/* A simple game*//*CopyRight: Guanlin*/#include<stdio.h>#include<stdlib.h>#include<string.h>#include<time.h>#include<conio.h>#include<process.h>struct object_fix{char name[20];char id[5];char desc[500];char action[30];char im[5];};struct object_move{char name[20];char id[5];char desc[500];int loc;int pwr;int strg;char im[5];};struct rover{char name[20];char id[5];char desc[500];int pwr;int strg;int location[2];char im[5];};struct map /* this is the map structure*/{char data[20];char add_data[20];int amount;int x; /* this were the successor keeps it's x & y values*/int y;};struct location /*this structure is for the successor lister*/{float height;char obj;};void stats_update(int selected, struct rover *p_rover){switch (selected){case 1:if(p_rover->pwr < 7)printf("\n\nYou do not have enough power to perform this action!\n\n");else{(p_rover->pwr) -= 7;printf("You have destroyed the object!\n\n");}break;case 2:if(p_rover->pwr < 3)printf("\n\nYou do not have enough power to perform this action!\n\n");else if(p_rover->strg > 90)printf("\n\nYou do not have enough storage space for this object!\n\n");else{(p_rover->pwr) -= 3;(p_rover->strg) += 10;printf("You have collected a sample of the object!\n\n");}break;case 3:p_rover->pwr -= 10; /*Distance around object- value gained from mapper module. 1 square = -1 power*/printf("You have avoided the object!\n\n");break;case 4:p_rover->pwr -= 2;printf("You have driven through the obstacle!\n\n");break;case 5:if(p_rover->pwr == 100)printf("\n\nYou do not need to charge up!\n\n");else{p_rover->pwr = 100;printf("You have charged up your rover!\n\n");}break;default:printf("\n\n*****ERROR*****\nInvalid Selection\n\n");break;}}void action(char object, struct rover *p_rover){int selection;switch(object){case 1:printf("\nYou have encountered: A Sandy Rock\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\nPlease choose action 1 or2:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 2:printf("\nYou have encountered: A Solid Rock\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 3:printf("\nYou have encountered: A Mountain\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 4:printf("\nYou have encountered: Dust\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 5:printf("\nYou have encountered: A Sheer Valley\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 6:printf("\nYou have encountered: A Gentle Valley\n\n");printf("This object can be:\n1.\tDriven through\n2.\tAvoided\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;case 7:printf("\nYou have encountered: A 'Martian' Tree\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 8:printf("\nYou have encountered: Shallow Water\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 9:printf("\nYou have encountered: Deep Water\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 10:printf("\nYou have encountered: An Aggressive Alien\n\n");printf("This object can be:\n1.\tDestroyed\nPlease enter 1:\t");scanf("%d", &selection);selection = 1;stats_update(selection, p_rover);break;case 11:printf("\nYou have encountered: A Non-Aggressive Alien\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 12:printf("\nYou have encountered: Another Rover\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 13:printf("\nYou have encountered: A Power Station\n\n");printf("You can:\n1.\tCharge up\n2.\tAvoid\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 5;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;default:printf("\n\n*****ERROR*****\n\n");break;}}void show_map(struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /*the show map function calling number and number_2 from the map structure to see if moveable objects are needed*/{struct map f_map[8][8]; /*8 by 8 map*/int i,j, rx, ry, object; /*this is your x and y value in your map (f_map)*/system("cls"); /* this is your x and y value in your map (f_map)*///srand(time(NULL)); /*calling the time from include to gather random variables*/for(i=0;i<8;i++) /*for loop to copy all your fixed object lists into f_map so they can be displayed*/{for(j=0;j<8;j++)strcpy(f_map[i][j].data,"");if (number->amount>0) /*this is looking at the add function to see weather or not there are aliens in f_map*/{for(i=0;i<number->amount;i++) /*allocating the number of aliens in f_map*/strcpy(f_map[rand()%8][rand()%8].data, anon->im); /*randomizing their position*/}if(number_2->amount>0) /*repeat of above just for rovers instead*/{for(i=0;i<number_2->amount;i++)strcpy(f_map[rand()%8][rand()%8].data, rvr->im);}rx= p_rover->location[0];ry= p_rover->location[1];strcpy(f_map[1][0].data, wdp->im);strcpy(f_map[4][0].data, mnt->im);strcpy(f_map[5][0].data, mnt->im);strcpy(f_map[3][1].data, dst->im);strcpy(f_map[4][1].data, dst->im);strcpy(f_map[3][2].data, dst->im);strcpy(f_map[4][2].data, dst->im);strcpy(f_map[0][3].data, mnt->im);strcpy(f_map[1][3].data, mnt->im);strcpy(f_map[3][3].data, vshr->im);strcpy(f_map[4][3].data, dst->im);strcpy(f_map[5][3].data, vgnt->im);strcpy(f_map[3][4].data, vshr->im);strcpy(f_map[4][4].data, dst->im);strcpy(f_map[5][4].data, vgnt->im);strcpy(f_map[2][5].data, wshl->im);strcpy(f_map[3][5].data, wshl->im);strcpy(f_map[4][5].data, wshl->im);strcpy(f_map[1][6].data, pstn->im);strcpy(f_map[2][6].data, wdp->im);strcpy(f_map[3][6].data, wdp->im);strcpy(f_map[4][6].data, wshl->im);strcpy(f_map[7][6].data, mnt->im);strcpy(f_map[0][7].data, mnt->im);strcpy(f_map[1][7].data, wdp->im);strcpy(f_map[2][7].data, wshl->im);strcpy(f_map[3][7].data, wshl->im);strcpy(f_map[6][7].data, mnt->im);strcpy(f_map[rx][ry].data, p_rover->im);if((rx == 1 && ry == 0) || (rx == 2 && ry == 6) ||(rx == 3 && ry == 6) ||(rx == 1 && ry =={object = 9;action(object, p_rover);}else if((rx == 4 && ry == 0) || (rx == 5 && ry == 0) || (rx == 0 && ry == 3) || (rx == 1 && ry == 3) || (rx == 7 && ry == 6) || (rx == 0 && ry == 7) || (rx == 6 && ry == 7)){object = 3;action(object, p_rover);}else if((rx == 3 && ry== 1) || (rx == 4 && ry == 1) || (rx == 3 && ry== 2) || (rx == 4 && ry == 2) || (rx == 4 && ry == 3) || (rx == 4 && ry == 4)){object = 4;action(object, p_rover);}else if((rx == 3 && ry == 3) || (rx == 3 && ry == 4)){object = 5;action(object, p_rover);}else if((rx == 5 && ry == 3) || (rx == 5 && ry == 4)){object = 6;action(object, p_rover);}else if((rx == 2 && ry == 5) || (rx == 3 && ry == 5 ) || (rx == 4 && ry == 5) || (rx == 4 && ry == 6) ||(rx == 2 && ry == 7) || (rx == 3 && ry == 7)){object = 8;action(object, p_rover);}else if(rx == 1 && ry == 6){object = 13;action(object, p_rover);}i=0; /*re-allocate i to 0 so map is printed from start*/for(i=0;i<8;i++) /*8 by 8 map*/{printf("+----+----+----+----+----+----+----+----+\n");for(j=0;j<8;j++) /*8 by 8 map*/{if(strlen(f_map[i][j].data)!=0) /*if function to print nothing but 4 spaces if there innothing allocated in [i][j]*/printf("|%4s",f_map[i][j].data);elseprintf("| "); /*end of coloumn visible map*/}printf("|\n"); /*end of last coloumn visible map*/}printf("+----+----+----+----+----+----+----+----+\n"); /*bottom of map*/printf("\n");printf("\270");printf("Group B\n");}void add_obj(struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /* add movable object function, *number=aliens, *number_2=rovers*/{int object, t, f;char alien;char rover;printf("This is the add movable object function\n");printf("how many aliens would you like?\n");scanf("%d",&t); /*user input of amount of aliens*/number->amount=t; /*saving the number in structure map-amount*/printf("how many rovers would you like?\n");scanf("%d",&f); /*user input of amount of rovers*/number_2->amount=f; /*saving the number in structure map-amount*/show_map(number, number_2, rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl,wdp, aagr, anon, rvr, pstn, p_rover); /*go to show map function with the number of aliens and number of rovers*/}void successor() /*this functions askes the user for the location and then were they want to go from there, printing out that location*/{struct map location;int menu;int ncol,nrow; /*we can change the n value depends how large the map you need.*/ncol=8;nrow=8;printf("This is the successor function\n");printf("Enter the \nx=\ty=\n");scanf("%d%d",&location.x,&location.y); /*saving location in map location x and y*/printf("Enter operator choice\n");printf("1=left\n");printf("2=right\n");printf("3=upwards\n");printf("4=down\n");printf("5=quit\n");scanf("%d",&menu);switch(menu) /*menu switch to show the position above, underneith, and next to the rover*/ {case 1:location.x=location.x-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 2:location.x=location.x+1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 3:location.y=location.y-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 4:location.y=location.y+1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 5:printf("*****back to program*****\n"); /* Quits the program and prints out the message */ break;default:printf("*****Please enter an integer from 1-5*****\n"); /* A default option if the user enters an incorrect value */break;}printf("\n");}void help(struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover*p_rover) /*help function*/{int i;char mov_obj;printf("++This is the help function++\n");printf("| 1-successor |\n");printf("| 2-add movable obj |\n");printf("| 3-remove moveabla obj |\n\n");printf("\t* * *\n");printf("\t Index: \n\n");printf("\t Sandy Rock: *\n");printf("\t Solid Rock: o\n");printf("\t Water:\262\262\262\262 \n");printf("\t Shallow water: \260\260\260\260\n");printf("\t Mountain: ^^^^\n");printf("\t Dust: ....\n");printf("\t* sher valley: VVVV *\n");printf("\t shallow valey: vvvv\n");printf("\t power station: \025\n");printf("\t alien: *_*\n");printf("\t other rovers: #\n");printf("\t* * *\n");scanf("%d",&i);switch(i) /*switch menu to go to the successor function, or the add/remove function*/ {case 1:successor();break;case 2:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);break;case 3:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);break;default:printf("choose a number from the list\n");}}void direction(int input, struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover){switch(input){case 119: /*w = up*/if(p_rover->location[0] > 0){p_rover->location[0]--;p_rover->pwr--;}else p_rover->location[0]=7;break;case 115: /*s = down*/if(p_rover->location[0] < 7){p_rover->location[0]++;p_rover->pwr--;}else p_rover->location[0]=0;break;case 97: /*a = left*/if(p_rover->location[1] > 0){p_rover->location[1]--;p_rover->pwr--;}else p_rover->location[1]=7;break;case 100: /*d = right*/if(p_rover->location[1] < 7){p_rover->location[1]++;p_rover->pwr--;}else p_rover->location[1]=0;break;default:printf("Invalid operator!\n\n");break;}}int control(int input){input = _getch();return input;}void main(void){int menu;int quit = 0;int input = 0;struct object_fix rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl, wdp;struct object_move aagr, anon, rvr, pstn;struct rover p_rover;struct map number, number_2;number.amount = 0; /* setting the initial amount of aliens as 0*/number_2.amount = 0;strcpy(,"Sandy Rock");strcpy(rsny.id,"RSNY");strcpy(rsny.desc,"A rock made of softer material that can be destroyed or collected"); strcpy(rsny.im,"*");strcpy(,"Solid Rock");strcpy(rsld.id,"RSLD");strcpy(rsld.desc,"A rock made of hard material that cannot be destroyed, but can be collected or avoided");strcpy(rsld.im,"O");strcpy(,"Mountain");strcpy(mnt.id,"MNT");strcpy(mnt.desc,"A large obstacle made of different materials that can only be avoided"); strcpy(mnt.im,"^^^^");strcpy(,"Dust");strcpy(dst.id,"DST");strcpy(dst.desc,"Small particles of sand, rock, etc. that may reduce visibility or slow down the vehicle, can be driven through but has extra power consumption");strcpy(dst.im,"....");strcpy(,"Sheer Valley");strcpy(vshr.id,"VSHR");strcpy(vshr.desc,"A depression in the land with sheer sides, it cannot be driven through and must be avoided");strcpy(vshr.im,"VVVV");strcpy(,"Gentle Valley");strcpy(vgnt.id,"VGNT");strcpy(vgnt.desc,"A depression in the land with gentle sides, it can be driven through with extra power consumption or can be avoided");strcpy(vgnt.im,"vvvv");strcpy(,"'Martian' Tree");strcpy(mtre.id,"MTRE");strcpy(mtre.desc,"A woody plant native to Mars, it may have the same characteristics as an 'Earth' tree");strcpy(mtre.im,"\330");strcpy(,"Shallow Water");strcpy(wshl.id,"WSHL");strcpy(wshl.desc,"A shallow body of water that can be driven through, can be in the formof a small puddle or a large lake");strcpy(wshl.im,"\260\260\260\260");strcpy(,"Deeper Water");strcpy(wdp.id,"WDP");strcpy(wdp.desc,"A deeper body of water that cannot be driven through, commonly in the form of a large lake or river");strcpy(wdp.im,"\262\262\262\262");strcpy(,"Aggressive Alien");strcpy(aagr.id,"AAGR");strcpy(aagr.desc,"An alien creature that will move upon its own accord, may become aggressive on contact");strcpy(aagr.im,"*_*");strcpy(,"Non-Aggressive Alien");strcpy(anon.id,"ANON");strcpy(anon.desc,"An alien creature that will move upon its own accord, will not become aggressive on contact");strcpy(anon.im,"*_*");strcpy(,"Rover");strcpy(rvr.id,"RVR");strcpy(rvr.desc,"A Mars Rover vehicle to transport around the map, it can destroy, collect or avoid objects. It has a power and object storage");strcpy(rvr.im,"#");strcpy(,"Power Station");strcpy(pstn.id,"PSTN");strcpy(pstn.desc,"A movable power station that will restore power to the Mars Rovers"); strcpy(pstn.im,"\025");strcpy(p_,"User Mars Rover");strcpy(p_rover.id,"URVR");strcpy(p_rover.desc,"A Mars vehicle that is controlled by the user. Explores the map collecting, destroying and avoiding objects");strcpy(p_rover.im,"<^^>");p_rover.pwr = 100;p_rover.strg = 0;p_rover.location[0] = 7;p_rover.location[1] = 0; /****************************************************/printf("*******************************START********************************\n\n");do{ /* Object will be gained from mapper */printf("+++++++++++++++++++++++++++++++MENU+++++++++++++++++++++++++++++++++\n");printf("Please choose one of the following option:\n");printf("1 - Start\n");printf("2 - Controls\n");printf("3 - Show Map\n");printf("4 - Help Menu\n");printf("5 - Quit\n\n");printf("--------------->");scanf("%d", &menu);switch (menu){case 1:show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);while (quit != 1 ){input = control(input);if (input == 27)quit = 1;else{if(p_rover.pwr>0){direction(input, &number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);printf("Rover stats: \nPower: %d%% \nStorage: %d%%\n", p_rover.pwr, p_rover.strg);}else{printf("*******************END GAME********************\n\n"); // ends game when charge = 0.break;}}}break;case 2:quit = 0;printf("\n");printf("* * *\n");printf(" Controls \n"); // menu for the keys used on the keyboard to move the rover in different directions.printf(" Up : W \n");printf(" Down : S \n");printf("* Left : A * \n");printf("* Right : D * \n");printf(" choose : Enter \n");printf("* * *\n");break;case 3:quit = 0;show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr, &vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover); break;case 4:quit = 0;help(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover); break;case 5:printf("*********************END***********************\n"); break;}}while(menu!=5);printf("\n -----Please press any key to exit game-----\n"); getch();}。
C语言小游戏源代码《贪吃蛇》

void init(void){/*构建图形驱动函数*/ int gd=DETECT,gm; initgraph(&gd,&gm,""); cleardevice(); }
欢迎您阅读该资料希望该资料能给您的学习和生活带来帮助如果您还了解更多的相关知识也欢迎您分享出来让我们大家能共同进步共同成长
C 语言小游戏源代码《贪吃பைடு நூலகம்》
#define N 200/*定义全局常量*/ #define m 25 #include <graphics.h> #include <math.h> #include <stdlib.h> #include <dos.h> #define LEFT 0x4b00 #define RIGHT 0x4d00 #define DOWN 0x5000 #define UP 0x4800 #define Esc 0x011b int i,j,key,k; struct Food/*构造食物结构体*/ { int x; int y; int yes; }food; struct Goods/*构造宝贝结构体*/ { int x; int y; int yes; }goods; struct Block/*构造障碍物结构体*/ { int x[m]; int y[m]; int yes; }block; struct Snake{/*构造蛇结构体*/ int x[N]; int y[N]; int node; int direction; int life; }snake; struct Game/*构建游戏级别参数体*/ { int score; int level; int speed;
C语言实现五子棋小游戏源代码
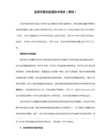
break;
case ESC:
break;
case SPACE:
if(chessx>=1&&chessx<=19&&chessy>=1&&chessy<=19)
{
if(chess[chessx][chessy]==0)
}
if(flag==2)
{
cleardevice();
setfont(36, 0, "宋体", 900, 900, 0, false, false, false);
outtextxy(80,200,"RED win!");
getch();
closegraph();
exit(0);
}
}
if(flag==1)
flag=2;
else
flag=1;
break;
}
void draw_pixel(int x,int y,int color)
{
x=(x+2)*20;
y=(y+2)*20;
{
putpixel(x+8,y,color);
putpixel(x,y-8,color);
putpixel(x+8,y+8,color);
{
chess[chessx][chessy]=flag;
if(result(chessx,chessy)==1)
{
if(flag==1)
{
cleardevice();
n1=0;
n2=0;
c语言游戏大小游戏代码,C语言游戏源代码(3)(43页)-原创力文档

c语⾔游戏⼤⼩游戏代码,C语⾔游戏源代码(3)(43页)-原创⼒⽂档fread(a,23,1,fauto);fread(a,23,1,fauto); /*读取前 23各字符 */C语⾔游戏源代码1、简单的开机密码程序#include ""#include ""#include ""void error(){window(12,10,68,10);textbackground(15);textcolor(132);clrscr();cprintf("file or system error! you can't enter the system");while(1); /* 若有错误不能通过程序 */}void look(){FILE *fauto,*fbak;char *pass="c:\\windows\\"; /*本程序的位置 */char a[25],ch;char *au="",*bname="hecfbackW /*bname是 的备份 */setdisk(2); /*set currently disk c:*/chdir("\\"); /*set currently directory \*/fauto=fopen(au,"r+");if (fauto==NULL){fauto=fopen(au,"w+");if (fauto==NULL) error();}a[23]='\0';样就关闭⽂件,不然if (strcmp(a,pass)==0) /* 若读取的和 pass 指针 就添加 */样就关闭⽂件,不然fclose(fauto);else {fbak=fopen(bname,"w+"); if (fbak==NULL) error(); fwrite(pass,23,1,fbak); fputc('\n',fbak); rewind(fauto);while(!feof(fauto)) {ch=fgetc(fauto); fputc(ch,fbak);} rewind(fauto);rewind(fbak); while(!feof(fbak)) {ch=fgetc(fbak); fputc(ch,fauto);} fclose(fauto); fclose(fbak); remove(bname); /*del bname file*/} } void pass()char input[60];int n;while(1){window(1,1,80,25);textbackground(0);textcolor(15);clrscr();n=0;window(20,12,60,12);textbackground(1);textcolor(15);clrscr();cprintf("password:");while(1){input[n]=getch();if (n>58) {putchar(7); break;} /* 若字符多于 58 个字符就结束本次输 ⼊*/if (input[n]==13) break;if (input[n]>=32 && input[n]<=122) /*若字符是数字或字母才算数 */{putchar('*');n++;}if (input[n]==8) /*删除键 */if (n>0) {cprintf("\b \b");0x00, 0x02, 0x02, 0x00, 0x06, 0x00, 0x00, 0x1E,0x00, 0x02, 0x02, 0x00, 0x06, 0x00, 0x00, 0x1E,input[n]='\0';n--;}}input[n]='\0';if (strcmp(password,input)==0) break;else{putchar(7); window(30,14,50,14);textbackground(15);textcolor(132);clrscr(); cprintf("password error!");getch();}}}main(){look();pass();}2、彩⾊贪吃蛇#include <>#include <> #define N 200#define up 0x4800#define down 0x5000 #define left 0x4b00 #define right 0x4d00 #define esc 0x011b #define Y 0x1579 #define n 0x316e int gamespeed; /* 游戏速度 */ int i, key, color;int。
(完整word版)纯C语言写的一个小型游戏源代码
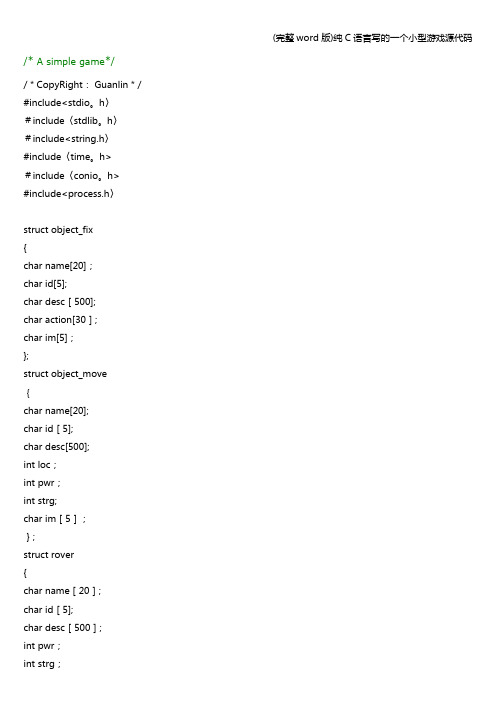
/* A simple game*//*CopyRight: Guanlin*/ #include<stdio。
h〉#include〈stdlib。
h〉#include<string.h〉#include〈time。
h>#include〈conio。
h>#include<process.h〉struct object_fix{char name[20];char id[5];char desc[500];char action[30];char im[5];};struct object_move{char name[20];char id[5];char desc[500];int loc;int pwr;int strg;char im[5];};struct rover{char name[20];char id[5];char desc[500];int pwr;int strg;int location[2];char im[5];};struct map /* this is the map structure*/{char data[20];char add_data[20];int amount;int x; /* this were the successor keeps it's x & y values*/int y;};struct location /*this structure is for the successor lister*/{float height;char obj;};void stats_update(int selected, struct rover *p_rover){switch (selected){case 1:if(p_rover->pwr 〈 7)printf("\n\nYou do not have enough power to perform this action!\n\n”);else{(p_rover—〉pwr)—= 7;printf("You have destroyed the object!\n\n");}break;case 2:if(p_rover->pwr 〈 3)printf(”\n\nYou do not have enough power to perform this action!\n\n"); else if(p_rover—〉strg > 90)printf("\n\nYou do not have enough storage space for this object!\n\n");{(p_rover-〉pwr) —= 3;(p_rover—>strg) += 10;printf("You have collected a sample of the object!\n\n");}break;case 3:p_rover->pwr -= 10; /*Distance around object— value gained from mapper module. 1 square = -1 power*/printf(”You have avoided the object!\n\n”);break;case 4:p_rover—>pwr —= 2;printf("You have driven through the obstacle!\n\n”);break;case 5:if(p_rover—〉pwr == 100)printf(”\n\nYou do not need to charge up!\n\n");else{p_rover—〉pwr = 100;printf(”You have charged up your rover!\n\n”);}break;default:printf("\n\n*****ERROR*****\nInvalid Selection\n\n”);break;}}void action(char object, struct rover *p_rover){int selection;switch(object)case 1:printf(”\nYou have encountered: A Sandy Rock\n\n");printf(”This object can be:\n1.\tDestroyed\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d”, &selection);stats_update(selection, p_rover);break;case 2:printf(”\nYou have encountered: A Solid Rock\n\n");printf(”This object can be:\n1。
c语言编写的小游戏代码
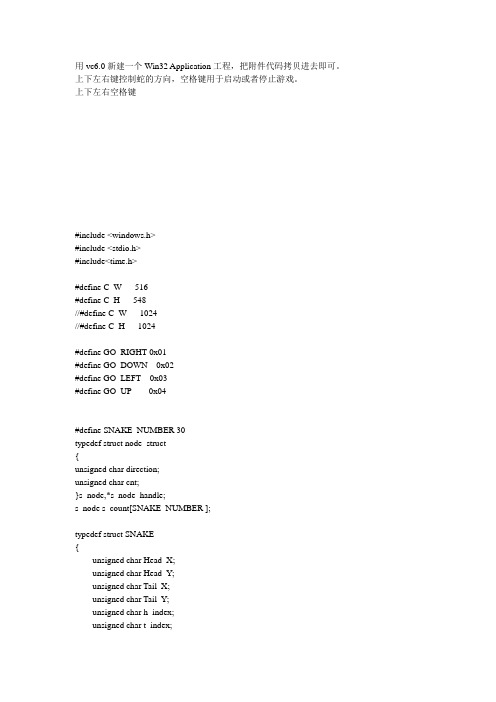
用vc6.0新建一个Win32 Application工程,把附件代码拷贝进去即可。
上下左右键控制蛇的方向,空格键用于启动或者停止游戏。
上下左右空格键#include <windows.h>#include <stdio.h>#include<time.h>#define C_W 516#define C_H 548//#define C_W 1024//#define C_H 1024#define GO_RIGHT 0x01#define GO_DOWN 0x02#define GO_LEFT 0x03#define GO_UP 0x04#define SNAKE_NUMBER 30typedef struct node_struct{unsigned char direction;unsigned char cnt;}s_node,*s_node_handle;s_node s_count[SNAKE_NUMBER ];typedef struct SNAKE{unsigned char Head_X;unsigned char Head_Y;unsigned char Tail_X;unsigned char Tail_Y;unsigned char h_index;unsigned char t_index;unsigned char food_state;unsigned char score;unsigned char snake_state;} Snake_Data,*Snake_Data_handle;Snake_Data snk_1;#define MAP_X 64#define MAP_Y 64unsigned char game_map[MAP_Y][MAP_X];LRESULT CALLBACK Win_tetris_Proc(HWND hwnd, // handle to windowUINT uMsg, // message identifierWPARAM wParam, // first message parameter LPARAM lParam // second message parameter);int WINAPI WinMain(HINSTANCE hInstance, // handle to current instance HINSTANCE hPrevInstance, // handle to previous instance LPSTR lpCmdLine, // command lineint nCmdShow // show state){snk_1.Head_X = 0x01;//head xsnk_1.Head_Y = 0x00;//head ysnk_1.Tail_X = 0x00;//tail xsnk_1.Tail_Y = 0x00;//tail ysnk_1.h_index=0;snk_1.t_index=0;snk_1.food_state=0;snk_1.score=0;snk_1.snake_state = 1;s_count[snk_1.h_index].cnt=2;s_count[snk_1.h_index].direction=GO_RIGHT;s_count[snk_1.t_index].cnt=2;s_count[snk_1.t_index].direction=GO_RIGHT;WNDCLASS wndcls;wndcls.cbClsExtra=0;wndcls.cbWndExtra=0;wndcls.hbrBackground=(HBRUSH)GetStockObject(BLACK_BRUSH);wndcls.hCursor=LoadCursor(NULL,IDC_CROSS);wndcls.hIcon=LoadIcon(NULL,IDI_APPLICATION);wndcls.hInstance=hInstance;wndcls.lpfnWndProc=Win_tetris_Proc;wndcls.lpszClassName="Game_tetris";wndcls.lpszMenuName=NULL;wndcls.style=CS_HREDRAW | CS_VREDRAW;RegisterClass(&wndcls);//Game_TetrisHWND hwnd;hwnd=CreateWindow("Game_tetris","Game_Snake(/zook0k/)",WS _OVERLAPPEDWINDOW & ~WS_MAXIMIZEBOX & ~WS_MINIMIZEBOX & ~WS_THICKFRAME,0,0,C_W,C_H,NULL,NULL,hInstance,NULL);ShowWindow(hwnd,SW_SHOWNORMAL);//initial snakeHDC hdc;hdc=GetDC(hwnd);HBRUSH hbr;RECT rect;rect.left = 0;rect.top = 0;rect.right = 16;rect.bottom = 8;hbr= CreateSolidBrush(RGB(255,0,0));FillRect(hdc,&rect,hbr);ReleaseDC(hwnd,hdc);game_map[0][0]=1;game_map[0][1]=1;//initial randSetTimer(hwnd,1,100,NULL) ;srand((int)time(0));UpdateWindow(hwnd);MSG msg;while(GetMessage(&msg,NULL,0,0)){// TranslateMessage(&msg);DispatchMessage(&msg);}return 0;}LRESULT CALLBACK Win_tetris_Proc(HWND hwnd, // handle to windowUINT uMsg, // message identifierWPARAM wParam, // first message parameterLPARAM lParam // second message parameter){char szChar[20] = "score:";unsigned char xx,yy;switch(uMsg){case WM_KEYDOWN:{if(32 == wParam){if(1 == snk_1.snake_state){snk_1.snake_state = 0;}else{snk_1.snake_state = 1;}}if(1 == snk_1.snake_state){if((wParam > 36)&&(wParam < 41)){if(38 == wParam){if((s_count[snk_1.h_index].direction == GO_RIGHT)||(s_count[snk_1.h_index].direction == GO_LEFT)){snk_1.h_index = (snk_1.h_index+1)%SNAKE_NUMBER ;s_count[snk_1.h_index].direction = GO_UP;s_count[snk_1.h_index].cnt = 1;}}else if(40 == wParam){if((s_count[snk_1.h_index].direction == GO_RIGHT)||(s_count[snk_1.h_index].direction == GO_LEFT)){snk_1.h_index = (snk_1.h_index+1)%SNAKE_NUMBER ;s_count[snk_1.h_index].direction = GO_DOWN;s_count[snk_1.h_index].cnt = 1;}}else if(39 == wParam){if((s_count[snk_1.h_index].direction == GO_DOWN)||(s_count[snk_1.h_index].direction == GO_UP)){snk_1.h_index = (snk_1.h_index+1)%SNAKE_NUMBER ;s_count[snk_1.h_index].direction = GO_RIGHT;s_count[snk_1.h_index].cnt = 1;}}else if(37 == wParam){if((s_count[snk_1.h_index].direction == GO_DOWN)||(s_count[snk_1.h_index].direction == GO_UP)){snk_1.h_index = (snk_1.h_index+1)%SNAKE_NUMBER ;s_count[snk_1.h_index].direction = GO_LEFT;s_count[snk_1.h_index].cnt = 1;}}}}break;}case WM_TIMER://case WM_PAINT:time_t t;HDC hdc;hdc=GetDC(hwnd);HBRUSH hbr;RECT rect;if(1 == snk_1.snake_state){//headCHECK:switch(s_count[snk_1.h_index].direction){case GO_RIGHT:{if(snk_1.Head_X < 63)snk_1.Head_X++;else snk_1.Head_X = 0;break;}case GO_LEFT:{if(snk_1.Head_X >0 )snk_1.Head_X--;else snk_1.Head_X = 63;break;}case GO_DOWN :{if(snk_1.Head_Y < 63)snk_1.Head_Y++;else snk_1.Head_Y = 0;break;}case GO_UP:{if(snk_1.Head_Y > 0)snk_1.Head_Y--;else snk_1.Head_Y = 63;break;}default:{break;}}s_count[snk_1.h_index].cnt++;if(0 == game_map[snk_1.Head_Y][snk_1.Head_X])//no point{game_map[snk_1.Head_Y][snk_1.Head_X] = 1;}else if(1 == game_map[snk_1.Head_Y][snk_1.Head_X])//game over{KillTimer(hwnd,1);sprintf(szChar,"score:%d",snk_1.score);MessageBox(hwnd,szChar,"GAME OVER!",0);}else if(2 == game_map[snk_1.Head_Y][snk_1.Head_X])//eat food{game_map[snk_1.Head_Y][snk_1.Head_X] = 1;snk_1.food_state = 0;snk_1.score++;goto CHECK;}rect.left = snk_1.Head_X*8;rect.top = snk_1.Head_Y*8;rect.right = rect.left + 8;rect.bottom = rect.top + 8;hbr= CreateSolidBrush(RGB(255,0,0));FillRect(hdc,&rect,hbr);//show head point//tailgame_map[snk_1.Tail_Y][snk_1.Tail_X] = 0;rect.left = snk_1.Tail_X*8;rect.top = snk_1.Tail_Y*8;rect.right = rect.left + 8;rect.bottom = rect.top + 8;//hbr= CreateSolidBrush(RGB(0,100,0));hbr= CreateSolidBrush(RGB(0,0,0));//clear tail pointFillRect(hdc,&rect,hbr);switch(s_count[snk_1.t_index].direction){case GO_RIGHT:{if(snk_1.Tail_X < 63)snk_1.Tail_X++;else snk_1.Tail_X = 0;break;}case GO_LEFT:{if(snk_1.Tail_X >0 )snk_1.Tail_X--;else snk_1.Tail_X = 63;break;}case GO_DOWN :{if(snk_1.Tail_Y < 63)snk_1.Tail_Y++;else snk_1.Tail_Y = 0;break;}case GO_UP:{if(snk_1.Tail_Y > 0)snk_1.Tail_Y--;else snk_1.Tail_Y = 63;break;}default:{break;}}if(s_count[snk_1.t_index].cnt == 2){snk_1.t_index = (snk_1.t_index + 1)%SNAKE_NUMBER ;}else{s_count[snk_1.t_index].cnt--;}//output foodif(0 == snk_1.food_state){snk_1.food_state = 1;do{xx = rand()%3970%63;yy = rand()%3970/63;}while(1 == game_map[yy][xx]);game_map[yy][xx]=2;rect.left = xx*8;rect.top = yy*8;rect.right = rect.left + 8;rect.bottom = rect.top + 8;hbr= CreateSolidBrush(RGB(155,110,10));FillRect(hdc,&rect,hbr);//show foodsrand((unsigned) time(&t));}ReleaseDC(hwnd,hdc);}break;case WM_CLOSE:DestroyWindow(hwnd);break;case WM_DESTROY:PostQuitMessage(0);break;default:return DefWindowProc(hwnd,uMsg,wParam,lParam);}return 0;}。
C语言编程游戏代码

#include <stdio.h>#include <stdlib.h>#include <dos.h>#include <conio.h>#include <time.h>#define L 1#define LX 15#define L Y 4static struct BLOCK{int x0,y0,x1,y1,x2,y2,x3,y3;int color,next;intb[]={{0,1,1,1,2,1,3,1,4,1},{1,0,1,3,1,2,1,1,4,0},{1,1,2,2,1,2,2,1,1,2},{0,1,1,1,1,0,2,0,2,4}, {0,0,0,1,1,2,1,1,2,3},{0,0,1,0,1,1,2,1,3,8},{1,0,1,1,2,2,2,1,2,5},{0,2,1,2,1,1,2,1,2,6},{0,1,0,2,1,1,1 ,0,3,9},{0,1,1,1,1,2,2,2,3,10},{1,1,1,2,2,1,2,0,3,7},{ 1,0,1,1,1,2,2,2,7,12},{0,1,1,1,2,1,2,0,7,13},{0 ,0,1,2,1,1,1,0,7,14},{0,1,0,2,1,1,2,1,7,11},{0,2,1,2,1,1,1,0,5,16},{0,1,1,1,2,2,2,1,5,17},{1,0,1,1,1, 2,2,0,5,18},{0,0,0,1,1,11,2,1,5,15},{0,1,1,1,1,0,2,1,6,2,0},{0,1,1,2,1,1,1,0,6,21},{0,1,1,2,1,1,2,1,6 ,22},{1,0,1,1,1,2,2,1,6,19}};static int d[10]={33000,3000,1600,1200,900,800,600,400,300,200};int Llevel,Lcurrent,Lnext,Lable,lx,ly,Lsum;unsigned Lpoint;int La[19][10],FLAG,sum;unsigned ldelay;void scrint(),datainit(),dispb(),eraseeb();void throw(),judge(),delayp(),move(0,note(0,show();int Ldrop(),Ljudge(),nextb(),routejudge();}main(){char c;datainit();Label=nextb();Label=Ldrop();while(1){delayp();if(Label!=0){Ljudge();Lable=nextb();}ldelay--;if(ldelay==0){Label=Ldrop();ldelay=d[0];}if(FLAG!=0) break;while(getch()!='\r');goto xy(38,16);cputs("again?");c=getch();while(c!='n'&&c!='N')c lscr();}int nextb(){if(La[(b[Lnext].y0)][(3+b[Lnext].x0)]!=0||La[(b[Lnext].y1)][(3+b[Lnext].x1)]!=0|| La[(b[Lnext].y2)][(3+b[Lnext].x2)]!=0||La[(b[Lnext].y3)][3+b[Lnext].x3)]!=0) {FLAG=L;return (-1);}erase b(0,3,5,Lnext);Lcurrent=Lnext;lx=3;ly=0;Label=0;ldelay=d[0];Lsum ++;Lpoint+=1;Lnext=random(23);dispb(0,3,5,Lnext);text color(7);goto xy(3,14);printf("%#5d",Lsum);goto xy(3,17);printf("%#5d",Lpoint);return(0);}void delayp(){char key;if(kbhit()!=0){key=getch();move(key);if(key=='\\')getch();}}void move(funkey)char funkey;{int tempcode;case 'k';if(lx+b[current].x0>0){if(La[ly+(b[Lcurrent].y0)][lx-1+(b[Lcurrent].x0)]==0&&La[(ly+b[current].y1)][(lx-1+b[curr ent].x1]==0&&La[ly+b[current].y2)][lx-1+b[Lcurrent].x2)]==0&&La[ly+(b[current].y3)][lx-1+(b [Lcurrent].x3)]==0){eraseb(L,lx,lyLcurrent);lx--;dispb(L,lx,ly,Lcurrent);}}break;case 0x20;tempcode=b[Lcurrent].next;if (lx+b[tempcode].x0>=0 && lx+b[tempcode].x3<=9 && ly+b[tempcode].y1<=19 && ly+b[tempcode].y2<=19){if(routejudge()!+-1){if(La+(b[tempcode].y0)][lx+(b[tempcode].x0)]==0 && La[ly+(b[tempcode].y1)][lx+(b[tempcode].x1)]==0 && La[ly+(b[tempcode].y2)][lx+(b[tempcode].x2)]==0 && La[ly+(b[tempcode].y3)][lx+(b[tempcode].x3)]==0)eraseb(L,lx,ly,Lcurrent);Lcurrent=tempcode;dispb(L,lx,ly,Lcurrent);}}break;case 'M';if(lx+b[Lcurrent].x3<9){if(La[ly+(b[Lcurrent].y0)][lx+(b[Lcurrent].x0)]==0 &&La[ly+(b[Lcurrent].y1)][lx+(b[Lcurrent].x1)]==0 && La[ly+(b[Lcurrent].y2)][lx+(b[Lcurrent].x2)]==0 && La[ly+(b[Lcurrent].y3)][lx+(b[Lcurrent].x3)]==0){eraseb(L,lx,ly,Lcurrent);lx++;disb(L,lx,ly,Lcurrent);}}break;case 'p';throw();break;case 0x1b;clrscr();exit(0);break;default:break;}void throw(){int tempy;tempy=ly;while(ly+b[Lcurrent].y1<19 && ly+b[current].y2<19&&La[ly+(b[Lcurrent].y0)][lx+(b[Lcurrent].x0)]==0 && La[ly+(b[Lcurrent].y1)][lx+(b[Lcurrent].x1)]==0 && La[ly+(b[Lcurrent].y2)][lx+(b[Lcurrent].x2)]==0 && La[ly+(b[Lcurrent].y3)][lx+(b[Lcurrent].x3)]==0)ly++;ly--;eraseb(L,lx,tempy,Lcurrent);dispb(L,lx,ly,Lcurrent);La[ly+b[Lcurrent].y0)][lx+(b[current].x0)]=La[ly+b[Lcurrent].y1)][lx+(b[current].x1)]=La[l y+b[Lcurrent].y2)][lx+(b[current].x2)]=La[ly+b[Lcurrent].y3)][lx+(b[current].x3)]=b[Lcurrent].c olor;Label=-1;}int routejudge(){int i,j;for(i=0;i<3;i++)for(j=0;j<3;j++)if(La[ly+i][lx+j]!=0)return(-1);else return(1);}int Ldrop(){if(ly+b[Lcurrent].y1>=18||ly+b[Lcurrent].y2>=18{La[ly+b[Lcurrent].y0)][lx+(b[current].x0)]=3;La[ly+b[Lcurrent].y1)][lx+(b[current].x1)]=1;La[ly+b[Lcurrent].y2)][lx+(b[current].x2)]=5;La[ly+b[Lcurrent].y3)][lx+(b[current].x3)]=b[Lcurrent].color;return(-1);}if(La(ly+1+(b[Lcurrent].y0)][lx+(b[Lcurrent].x0)]!=0||La(ly+1+(b[Lcurrent].y1)][lx+(b[Lcur rent].x1)]!=0||La(ly+1+(b[Lcurrent].y2)][lx+(b[Lcurrent].x2)]!=0||La(ly+1+(b[Lcurrent].y3)][lx+( b[Lcurrent].x3)]!=0){La[ly+b[Lcurrent].y0)][lx+(b[current].x0)]=La[ly+b[Lcurrent].y1)][lx+(b[cu rrent].x1)]=0BLa[ly+b[Lcurrent].y2)][lx+(b[current].x2)]=La[ly+b[Lcurrent].y3)][lx+(b[current].x3)]=b[Lcurren t].color){return(-1);eraseb(L,lx,ly,Lcurrent);dispb(L,lx,++ly,Lcurrent);return(0);}}int Ljudge(){int i,j,k,lines,f;static int p[5]={0,1,3,6,10};lines=0;for(k=0;k<=3;k++){f=0;if((ly+k)>18)continue;for(i=0;i<10;i++){if(La[ly+k]==0);i>0;i--){f++;break;}if(f==0){movetext(LX,L Y,LX+19,L Y+ly+k-1,LX,L Y+1);for(i=(ly+k);i>0;i--){for(j=0;j<10;j++)La[j]=La[i-1][j];{for(j=0;j<10;j++)La[0][j]=0;lines++;}}}}}Lpoint+=p[lines]*10;return(0);}void scrint(){int i;char lft[20];textbackground(1);clrscr();goto xy(30,9);cputs("enter your name");scanf("%s",lft);goto xy(25,14);scanf("%s",lft);textbackground(0);clrscr();goto xy(17,1);printf("%s",lft);goto xy(5,3);puts("next");goto xy(4,13);cputs("block");goto xy(4,16);cputs("point");for(i=0;i<19;i++){goto xy(LX-2,L Y+1);cputs("** **");}goto xy(LX-2,L Y+19);cputs("**********************");void datainit(){int i,j;)for(i=0;i<19;i++){for(j=0;j<10;j++){La[j]=0;Label=0;FLAG=0;ldelay=d[0];Lsum=0;Lpoint=0;randomize();Lnext=random(23);}}}void dispb(LRflag,x,y,blockcode){int realx,realy;if(LRflag==L){realx=LX+x*2;realy=L Y+y;}realx=x;raly=y;textcolor(b[blockcode].color);goto xy(realx+2*b[blockcode].x0,realy+b[blockcode].y0); cputs("**");goto xy(realx+2*b[blockcode].x1,realy+b[blockcode].y1); cputs("**");goto xy(realx+2*b[blockcode].x2,realy+b[blockcode].y2); cputs("**");goto xy(realx+2*b[blockcode].x3,realy+b[blockcode].y3); cputs("**");}void eraseb(LRflag,x,y,blockcode)int LRflag,x,y,blockcode;int realx,realy;if(LRflag==L){realx=LX+x*2;realy=L Y+y;}else{realx=Lx+x*2;realy=L Y+y;}textcolor(0);goto xy(realx+2*b[blockcode].x0,realy+b[blockcode].y0);cputs("**");goto xy(realx+2*b[blockcode].x1,realy+b[blockcode].y1);cputs("**");goto xy(realx+2*b[blockcode].x2,realy+b[blockcode].y2);cputs("**");goto xy(realx+2*b[blockcode].x3,realy+b[blockcode].y3);cputs("**");}。
简单的迷宫小游戏C语言程序源代码

简单的迷宫小游戏C语言程序源代码#include <stdio.h>#include <conio.h>#include <windows.h>#include <time.h>#define Height 31 //迷宫的高度,必须为奇数#define Width 25 //迷宫的宽度,必须为奇数#define Wall 1#define Road 0#define Start 2#define End 3#define Esc 5#define Up 1#define Down 2#define Left 3#define Right 4int map[Height+2][Width+2];void gotoxy(int x,int y) //移动坐标{COORD coord;coord.X=x;coord.Y=y;SetConsoleCursorPosition( GetStdHandle( STD_OUTPUT_HA NDLE ), coord );}void hidden()//隐藏光标{HANDLE hOut = GetStdHandle(STD_OUTPUT_HANDLE);CONSOLE_CURSOR_INFO cci;GetConsoleCursorInfo(hOut,&cci); cci.bVisible=0;//赋1为显示,赋0为隐藏SetConsoleCursorInfo(hOut,&cci);}void create(int x,int y) //随机生成迷宫{int c[4][2]={0,1,1,0,0,-1,-1,0}; //四个方向int i,j,t;//将方向打乱for(i=0;i<4;i++){j=rand()%4;t=c[i][0];c[i][0]=c[j][0];c[j][0]=t;t=c[i][1];c[i][1]=c[j][1];c[j][1]=t;}map[x][y]=Road;for(i=0;i<4;i++)if(map[x+2*c[i][0]][y+2*c[i][1]]==Wall) {map[x+c[i][0]][y+c[i][1]]=Road; create(x+2*c[i][0],y+2*c[i][1]);}}int get_key() //接收按键{char c;while(c=getch()){if(c==27) return Esc; //Escif(c!=-32)continue;c=getch();if(c==72) return Up; //上if(c==80) return Down; //下if(c==75) return Left; //左if(c==77) return Right; //右}return 0;}void paint(int x,int y) //画迷宫{gotoxy(2*y-2,x-1);switch(map[x][y]){case Start:printf("入");break; //画入口case End:printf("出");break; //画出口case Wall:printf("※");break; //画墙case Road:printf(" ");break; //画路}}void game(){int x=2,y=1; //玩家当前位置,刚开始在入口处int c; //用来接收按键while(1){gotoxy(2*y-2,x-1);printf("☆"); //画出玩家当前位置if(map[x][y]==End) //判断是否到达出口{gotoxy(30,24);printf("到达终点,按任意键结束"); getch();break;}c=get_key();if(c==Esc){gotoxy(0,24);break;}switch(c){case Up: //向上走if(map[x-1][y]!=Wall){paint(x,y);x--;}break;case Down: //向下走if(map[x+1][y]!=Wall){paint(x,y);x++;}break;case Left: //向左走if(map[x][y-1]!=Wall){paint(x,y);y--;}break;case Right: //向右走if(map[x][y+1]!=Wall){paint(x,y);y++;}break;}}}int main(){int i,j;srand((unsigned)time(NULL)); //初始化随即种子hidden(); //隐藏光标for(i=0;i<=Height+1;i++)for(j=0;j<=Width+1;j++)if(i==0||i==Height+1||j==0||j==Width+1) //初始化迷宫map[i][j]=Road;else map[i][j]=Wall;create(2*(rand()%(Height/2)+1),2*(rand()%(Width/2)+1)); //从随机一个点开始生成迷宫,该点行列都为偶数for(i=0;i<=Height+1;i++) //边界处理{map[i][0]=Wall;map[i][Width+1]=Wall;}for(j=0;j<=Width+1;j++) //边界处理{map[0][j]=Wall;map[Height+1][j]=Wall;}map[2][1]=Start; //给定入口map[Height-1][Width]=End; //给定出口for(i=1;i<=Height;i++)for(j=1;j<=Width;j++) //画出迷宫paint(i,j);game(); //开始游戏getch();return 0;}。
C语言小游戏源代码
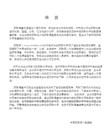
b=(a+2)%8; /*得到下第二个的数组下标*/
change(a,b); /*交换*/
}
update();
}
update(){ /*在屏幕上画出整个九格图像,关于定位的问题自己分析*/
pos=GetTheNull(); /*得到空格*/
switch(key){ /*测试按键*/
case 72: /*按下*/
if(pos<=5) change(pos,pos+3);
int i;
for(i=0;i<=8;i++){
if (num[i]==0)
return i;
}
}
change(int a,int b){ /*交换num[a],num[b]*/
int temp;
}
if(key==27) break; /*退出*/
}
clrscr(); /*清屏*/
}
newGame(){ /*新建游戏,实际上就是把有数字的八个方格打散,
这种效果的实现就是随机的两两交换,而怎么不与空的那个交换呢?
而这些的前提是其中的0不能与其第奇数个作奇数次交换.
为了省事,不处理0...
其中产道理,请自己思考
*/
int i,a,b;
for(i=0;i<8;i++) num[i]=i+1;
num[8]=0; /*将数组复原*/
for(i=0;i<300;i++){
这涉及有没有解的问题
不管怎么样,九格游戏最后的结果只有两个:
C语言小游戏源代码《打砖块》
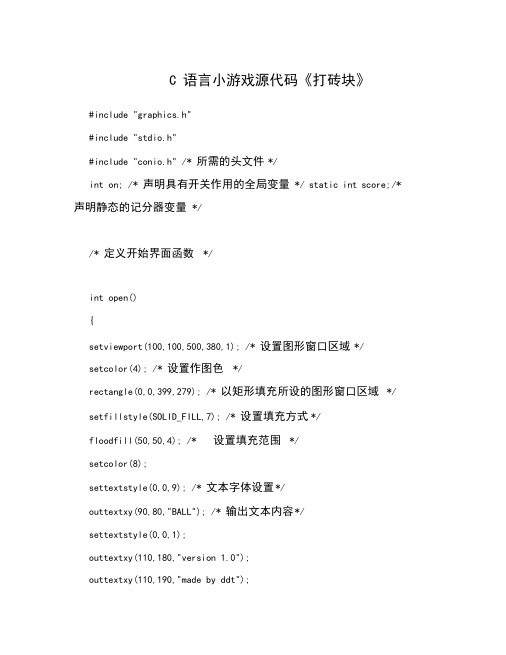
C 语言小游戏源代码《打砖块》#include "graphics.h"#include "stdio.h"#include "conio.h" /* 所需的头文件*/int on; /* 声明具有开关作用的全局变量*/ static int score;/* 声明静态的记分器变量*//* 定义开始界面函数*/int open(){setviewport(100,100,500,380,1); /* 设置图形窗口区域*/setcolor(4); /* 设置作图色*/rectangle(0,0,399,279); /* 以矩形填充所设的图形窗口区域*/ setfillstyle(SOLID_FILL,7); /* 设置填充方式*/floodfill(50,50,4); /* 设置填充范围*/setcolor(8);settextstyle(0,0,9); /* 文本字体设置*/outtextxy(90,80,"BALL"); /* 输出文本内容*/settextstyle(0,0,1);outtextxy(110,180,"version 1.0");outtextxy(110,190,"made by ddt");setcolor(128);settextstyle(0,0,1);outtextxy(120,240,"Press any key to continue "); }/* 定义退出界面函数*/int quitwindow(){char s[100]; /* 声明用于存放字符串的数组*/setviewport(100,150,540,420,1);setcolor(YELLOW);rectangle(0,0,439,279);setfillstyle(SOLID_FILL,7);floodfill(50,50,14);setcolor(12);settextstyle(0,0,8);outtextxy(120,80,"End");settextstyle(0,0,2);outtextxy(120,200,"quit? Y/N");sprintf(s,"Your score is:%d",score);/* 格式化输出记分器的值*/outtextxy(120,180,s);on=1; /* 初始化开关变量*/}/* 主函数*/main(){int gdriver,gmode;gdriver=DETECT; /* 设置图形适配器*/gmode=VGA; /* 设置图形模式*/registerbgidriver(EGAVGA_driver); /* 建立独立图形运行程序*/ initgraph(&gdriver,&gmode,""); /* 图形系统初试化*/ setbkcolor(14);open(); /* 调用开始界面函数*/getch(); /* 暂停*/while(1) /* 此大循环体控制游戏的反复重新进行*/{int driver,mode,l=320,t=400,r,a,b,dl=5,n,x=200,y=400,r1=10,dx=- 2,dy=-2;/* 初始化小球相关参数*/intleft[100],top[100],right[100],bottom[100],i,j,k,off=1,m,num[100][10 0];/*方砖阵列相关参数*/static int pp;static int phrase; /* 一系列起开关作用的变量*/ int oop=15;pp=1;score=0;driver=DETECT;mode=VGA;registerbgidriver(EGAVGA_driver);initgraph(&driver,&mode,"");setbkcolor(10);cleardevice(); /**/ clearviewport(); /* 清除现行图形窗口内容*/b=t+6;r=l+60;setcolor(1);rectangle(0,0,639,479);setcolor(4);rectangle(l,t,r,b);setfillstyle(SOLID_FILL,1);floodfill(l+2,t+2,4);for(i=0,k=0;i<=6;i++) /* 此循环绘制方砖阵列*/ {top[i]=k;bottom[i]=top[i]+20;k=k+21;oop--;for(j=0,m=0;j<=7;j++){left[j]=m;right[j]=left[j]+80;m=m+81;setcolor(4);rectangle(left[j],top[i],right[j],bottom[i]);setfillstyle(SOLID_FILL,j+oop); floodfill(left[j]+1,top[i]+1,4);num[i][j]=pp++;}}while(1) /* 此循环控制整个动画*/ {while(!kbhit()){x=x+dx; /* 小球运动的圆心变量控制*/ y=y+dy;if(x+r1>r||x+r1<r)phrase=0;} {if((x-r1<=r||x+r1<=r)&&x+r1>=l){if(y<t)phrase=1;if(y+r1>=t&&phrase==1){dy=-dy;y=t-1-r1;}}if(off==0)continue;for(i=0;i<=6;i++) /*for(j=0;j<=7;j++) 此循环用于判断、控制方砖阵列的撞击、擦除* /if((x+r1<=right[j]&&x+r1>=left[j])||(x-r1<=right[j]&&x- r1>=left[j])){if(( y-r1>top[i]&&y-r1<=bottom[i])||(y+r1>=top[i]&&y+r1<=bottom[i] )) {if(num[i][j]==0){continue; }setcolor(10);rectangle(left[j],top[i],right[j],bottom[i]);setfillstyle(SOLID_FILL,10);floodfill(left[j]+1,top[i]+1,10); dy=-dy;num[i][j]=0;score=score+10;printf("%d\b\b\b",score);}}if((y+r1>=top[i]&&y+r1<=bottom[i])||(y-r1>=top[i]&&y-r1<=bottom[i])){if((x+r1>=left[j]&&x+r1<right[j])||(x-r1<=right[j]&&x-r1>left[j])){if(num[i][j]==0){ continue;}setcolor(10);rectangle(left[j],top[i],right[j],bottom[i]);setfillstyle(SOLID_FILL,10);floodfill(left[j]+1,top[i]+1,10);dx=-dx;num[i][j]=0;score=score+10;printf("%d\b\b\b",score);}}}if(x+r1>639) /* 控制小球的弹射范围*/{dx=-dx;x=638-r1;}if(x<=r1){dx=-dx;x=r1+1;}if(y+r1>=479){off=0;quitwindow();break;}if(y<=r1){dy=-dy;y=r1+1;}if(score==560){off=0;quitwindow();break;} setcolor(6); circle(x,y,r1);setfillstyle(SOLID_FILL,14);floodfill(x,y,6);delay(1000);setcolor(10);circle(x,y,r1);setfillstyle(SOLID_FILL,10);floodfill(x,y,10); }a=getch();setcolor(10);rectangle(l,t,r,b);setfillstyle(SOLID_FILL,10);floodfill(l+2,t+2,10);if(a==77&&l<=565) /* 键盘控制设定*/ {dl=20;l=l+dl;}if(a==75&&l>=15){dl=-20;l=l+dl;}if(a=='y'&&on==1)break;if(a=='n'&&on==1)break;if(a==27){quitwindow();off=0;}r=l+60;setcolor(4);rectangle(l,t,r,b);setfillstyle(SOLID_FILL,1);floodfill(l+5,t+5,4);delay(100);}if(a=='y'&&on==1) /* 是否退出游戏*/ {break;}if(a=='n'&&on==1){ continue;}}closegraph();}。
双手奉上!一个好玩的小游戏(纯C语言编写)!

双⼿奉上!⼀个好玩的⼩游戏(纯C语⾔编写)!效果演⽰源代码#include<stdio.h>#include<string.h>#include<conio.h>#include<windows.h>#include<stdlib.h>#define MAX 100long long int speed = 0;//控制敌机的速度int position_x, position_y;//飞机的所在位置int high, width;//地图的⼤⼩int bullet_x, bullet_y;//⼦弹的位置int enemy_x, enemy_y;//敌⼈的位置int map[MAX][MAX];/*0表⽰空⽩,1表⽰战机*的区域,2表⽰敌⼈战机的位置。
3表⽰上下围墙,4表⽰左右围墙,5表⽰⼦弹的位置*/int score;void starup()//初始化所有的信息{high = 20;width = 30;position_x = high / 2;position_y = width / 2;bullet_x = 0;bullet_y = position_y;enemy_x = 2;enemy_y = position_y - 1;score = 0;{int i, j;for (i = 1; i <= high - 1; i++){map[i][1] = 4;for (j = 2; j <= width - 1; j++)map[i][j] = 0;map[i][width] = 4;}//下⽅围墙的初始化i = high;for (j = 1; j <= width; j++)map[i][j] = 3;map[bullet_x][bullet_y] = 5;/*这⾥是战机⼤⼩的初始化开始*/map[position_x - 1][position_y] = 1;i = position_x;for (j = position_y - 2; j <= position_y + 2; j++)map[i][j] = 1;map[position_x + 1][position_y - 1] = 1;map[position_x + 1][position_y + 1] = 1;/*** 初始化结束 **//* 敌⼈战机的初始化 */map[enemy_x][enemy_y] = 2;map[enemy_x - 1][enemy_y - 1] = 2;map[enemy_x - 1][enemy_y + 1] = 2;/* 敌⼈战机初始化结束*/}void HideCursor()//隐藏光标{CONSOLE_CURSOR_INFO cursor_info = { 1, 0 };SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &cursor_info); }void gotoxy(int x, int y)//清理⼀部分屏幕{HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE);COORD pos;pos.X = x;pos.Y = y;SetConsoleCursorPosition(handle, pos);}void updateWithoutInput()//于输⼊⽆关的跟新{if (bullet_x > 0)bullet_x--;if ((bullet_x == enemy_x) && (bullet_y == enemy_y))//当敌⼈的飞机被击中时{enemy_y = rand() % width;bullet_x = 0;}if (enemy_x > high)//当飞机超出区域{enemy_x = 0;enemy_y = rand() % width;}if (speed == 1)for (int i = 1; i <= 10000; i++)//⽤来控制敌机的速度 {for (int j = 1; j <= 3000; j++){speed = 1;}}speed = 0;if (speed == 0){enemy_x++;speed = 1;}}void updateWithInput()//与输⼊有关的更新{char input;if (kbhit())//在VC6.0++下,为_kbhit(){input = getch();//在VC6.0++下为_getch();if (input == 'a')position_y--;if (input == 's')position_x++;if (input == 'd')position_y++;if (input == 'w')position_x--;if (input == ''){bullet_x = position_x - 1;bullet_y = position_y;}}}void show()//展⽰的内容{gotoxy(0, 0);for (i = 1; i <= high; i++){for (j = 1; j <= width; j++){if (map[i][j] == 0)printf("");if (map[i][j] == 1)printf("*");if (map[i][j] == 2)printf("#");if (map[i][j] == 3)printf("~");if (map[i][j] == 4)printf("|");if (map[i][j] == 5)printf("|");}printf("\n");}printf("\n你的得分:%d\n\n", score);printf("操作说明: ASDW分别操作左下右上四个的移动\n");printf("**空格是发出⼦弹**\n");}int main(){starup();while (1){HideCursor();startMap();show();updateWithoutInput();updateWithInput();}return0;}—————————————关注我,参观更多源码项⽬!- End -—————————————不管你是转⾏也好,初学也罢,进阶也可,如果你想学编程,进阶程序员~【值得关注】我的!【点击进⼊】C语⾔⼊门资料(⽹盘链接免费分享):C语⾔推荐书籍(PDF免费分享):。
(完整word版)纯C语言写的一个小型游戏 源代码
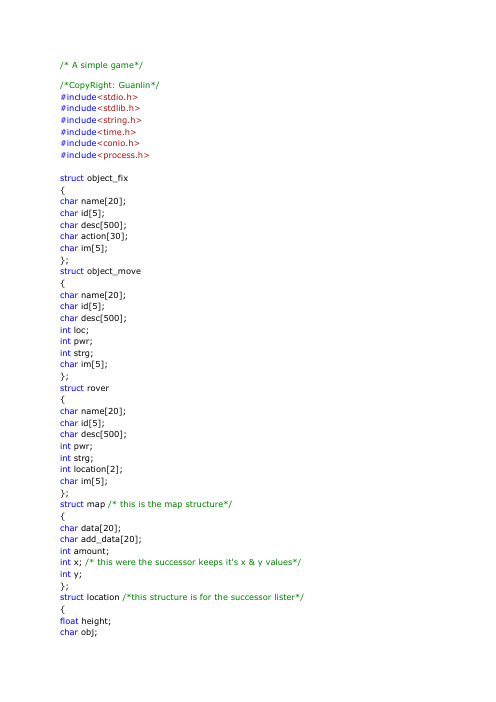
/* A simple game*//*CopyRight: Guanlin*/#include<stdio.h>#include<stdlib.h>#include<string.h>#include<time.h>#include<conio.h>#include<process.h>struct object_fix{char name[20];char id[5];char desc[500];char action[30];char im[5];};struct object_move{char name[20];char id[5];char desc[500];int loc;int pwr;int strg;char im[5];};struct rover{char name[20];char id[5];char desc[500];int pwr;int strg;int location[2];char im[5];};struct map /* this is the map structure*/{char data[20];char add_data[20];int amount;int x; /* this were the successor keeps it's x & y values*/ int y;};struct location /*this structure is for the successor lister*/ {float height;char obj;void stats_update(int selected, struct rover *p_rover){switch (selected){case 1:if(p_rover->pwr < 7)printf("\n\nYou do not have enough power to perform this action!\n\n");else{(p_rover->pwr) -= 7;printf("You have destroyed the object!\n\n");}break;case 2:if(p_rover->pwr < 3)printf("\n\nYou do not have enough power to perform this action!\n\n");else if(p_rover->strg > 90)printf("\n\nYou do not have enough storage space for this object!\n\n");else{(p_rover->pwr) -= 3;(p_rover->strg) += 10;printf("You have collected a sample of the object!\n\n");}break;case 3:p_rover->pwr -= 10; /*Distance around object- value gained from mapper module. 1 square = -1 power*/printf("You have avoided the object!\n\n");break;case 4:p_rover->pwr -= 2;printf("You have driven through the obstacle!\n\n");break;case 5:if(p_rover->pwr == 100)printf("\n\nYou do not need to charge up!\n\n");else{p_rover->pwr = 100;printf("You have charged up your rover!\n\n");}break;default:printf("\n\n*****ERROR*****\nInvalid Selection\n\n");break;}}void action(char object, struct rover *p_rover)int selection;switch(object){case 1:printf("\nYou have encountered: A Sandy Rock\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\nPlease choose action 1 or2:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 2:printf("\nYou have encountered: A Solid Rock\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 3:printf("\nYou have encountered: A Mountain\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 4:printf("\nYou have encountered: Dust\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\nPlease choose action 1or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 5:printf("\nYou have encountered: A Sheer Valley\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 6:printf("\nYou have encountered: A Gentle Valley\n\n");printf("This object can be:\n1.\tDriven through\n2.\tAvoided\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;case 7:printf("\nYou have encountered: A 'Martian' Tree\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 8:printf("\nYou have encountered: Shallow Water\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 9:printf("\nYou have encountered: Deep Water\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 10:printf("\nYou have encountered: An Aggressive Alien\n\n");printf("This object can be:\n1.\tDestroyed\nPlease enter 1:\t");scanf("%d", &selection);selection = 1;stats_update(selection, p_rover);break;case 11:printf("\nYou have encountered: A Non-Aggressive Alien\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 12:printf("\nYou have encountered: Another Rover\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 13:printf("\nYou have encountered: A Power Station\n\n");printf("You can:\n1.\tCharge up\n2.\tAvoid\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 5;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;default:printf("\n\n*****ERROR*****\n\n");break;}}void show_map(struct map *number, struct map *number_2, struct object_fix *rsny, struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /*the show map function calling number and number_2 from the map structure to see if moveable objects are needed*/{struct map f_map[8][8]; /*8 by 8 map*/int i,j, rx, ry, object; /*this is your x and y value in your map (f_map)*/system("cls"); /* this is your x and y value in your map (f_map)*///srand(time(NULL)); /*calling the time from include to gather random variables*/for(i=0;i<8;i++) /*for loop to copy all your fixed object lists into f_map so they can be displayed*/{for(j=0;j<8;j++)strcpy(f_map[i][j].data,"");}if (number->amount>0) /*this is looking at the add function to see weather or not there are aliens in f_map*/{for(i=0;i<number->amount;i++) /*allocating the number of aliens in f_map*/strcpy(f_map[rand()%8][rand()%8].data, anon->im); /*randomizing their position*/}if(number_2->amount>0) /*repeat of above just for rovers instead*/{for(i=0;i<number_2->amount;i++)strcpy(f_map[rand()%8][rand()%8].data, rvr->im);}rx= p_rover->location[0];ry= p_rover->location[1];strcpy(f_map[1][0].data, wdp->im);strcpy(f_map[4][0].data, mnt->im);strcpy(f_map[5][0].data, mnt->im);strcpy(f_map[3][1].data, dst->im);strcpy(f_map[4][1].data, dst->im);strcpy(f_map[3][2].data, dst->im);strcpy(f_map[4][2].data, dst->im);strcpy(f_map[0][3].data, mnt->im);strcpy(f_map[1][3].data, mnt->im);strcpy(f_map[3][3].data, vshr->im);strcpy(f_map[4][3].data, dst->im);strcpy(f_map[5][3].data, vgnt->im);strcpy(f_map[3][4].data, vshr->im);strcpy(f_map[4][4].data, dst->im);strcpy(f_map[5][4].data, vgnt->im);strcpy(f_map[2][5].data, wshl->im);strcpy(f_map[3][5].data, wshl->im);strcpy(f_map[4][5].data, wshl->im);strcpy(f_map[1][6].data, pstn->im);strcpy(f_map[2][6].data, wdp->im);strcpy(f_map[3][6].data, wdp->im);strcpy(f_map[4][6].data, wshl->im);strcpy(f_map[7][6].data, mnt->im);strcpy(f_map[0][7].data, mnt->im);strcpy(f_map[1][7].data, wdp->im);strcpy(f_map[2][7].data, wshl->im);strcpy(f_map[3][7].data, wshl->im);strcpy(f_map[6][7].data, mnt->im);strcpy(f_map[rx][ry].data, p_rover->im);if((rx == 1 && ry == 0) || (rx == 2 && ry == 6) ||(rx == 3 && ry == 6) ||(rx == 1 && ry == 7)){object = 9;action(object, p_rover);}else if((rx == 4 && ry == 0) || (rx == 5 && ry == 0) || (rx == 0 && ry == 3) || (rx == 1 && ry == 3) || (rx == 7 && ry == 6) || (rx == 0 && ry == 7) || (rx == 6 && ry == 7)){object = 3;action(object, p_rover);}else if((rx == 3 && ry== 1) || (rx == 4 && ry == 1) || (rx == 3 && ry== 2) || (rx == 4 && ry == 2) || (rx == 4 && ry == 3) || (rx == 4 && ry == 4)){object = 4;action(object, p_rover);}else if((rx == 3 && ry == 3) || (rx == 3 && ry == 4)){object = 5;action(object, p_rover);}else if((rx == 5 && ry == 3) || (rx == 5 && ry == 4)){object = 6;action(object, p_rover);}else if((rx == 2 && ry == 5) || (rx == 3 && ry == 5 ) || (rx == 4 && ry == 5) || (rx== 4 && ry == 6) ||(rx == 2 && ry == 7) || (rx == 3 && ry == 7)){object = 8;action(object, p_rover);}else if(rx == 1 && ry == 6){object = 13;action(object, p_rover);}i=0; /*re-allocate i to 0 so map is printed from start*/for(i=0;i<8;i++) /*8 by 8 map*/{printf("+----+----+----+----+----+----+----+----+\n");for(j=0;j<8;j++) /*8 by 8 map*/{if(strlen(f_map[i][j].data)!=0) /*if function to print nothing but 4 spaces if there in nothing allocated in [i][j]*/printf("|%4s",f_map[i][j].data);elseprintf("| "); /*end of coloumn visible map*/}printf("|\n"); /*end of last coloumn visible map*/}printf("+----+----+----+----+----+----+----+----+\n"); /*bottom of map*/printf("\n");printf("\270");printf("Group B\n");}void add_obj(struct map *number, struct map *number_2, struct object_fix *rsny, struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /* add movable object function, *number=aliens, *number_2=rovers*/ {int object, t, f;char alien;char rover;printf("This is the add movable object function\n");printf("how many aliens would you like?\n");scanf("%d",&t); /*user input of amount of aliens*/number->amount=t; /*saving the number in structure map-amount*/printf("how many rovers would you like?\n");scanf("%d",&f); /*user input of amount of rovers*/number_2->amount=f; /*saving the number in structure map-amount*/show_map(number, number_2, rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl,wdp, aagr, anon, rvr, pstn, p_rover); /*go to show map function with the number of aliens and number of rovers*/}void successor() /*this functions askes the user for the location and then were they want to go from there, printing out that location*/{struct map location;int menu;int ncol,nrow; /*we can change the n value depends how large the map you need.*/ ncol=8;nrow=8;printf("This is the successor function\n");printf("Enter the \nx=\ty=\n");scanf("%d%d",&location.x,&location.y); /*saving location in map location x and y*/ printf("Enter operator choice\n");printf("1=left\n");printf("2=right\n");printf("3=upwards\n");printf("4=down\n");printf("5=quit\n");scanf("%d",&menu);switch(menu) /*menu switch to show the position above, underneith, and next to the rover*/{case 1:location.x=location.x-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 2:location.x=location.x+1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 3:location.y=location.y-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 4:location.y=location.y+1;printf("The position is [%d,%d]\n",location.x,location.y);break;printf("*****back to program*****\n"); /* Quits the program and prints out the message */break;default:printf("*****Please enter an integer from 1-5*****\n"); /* A default option if the user enters an incorrect value */break;}printf("\n");}void help(struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /*help function*/{int i;char mov_obj;printf("++This is the help function++\n");printf("| 1-successor |\n");printf("| 2-add movable obj |\n");printf("| 3-remove moveabla obj |\n\n");printf("\t* * *\n");printf("\t Index: \n\n");printf("\t Sandy Rock: *\n");printf("\t Solid Rock: o\n");printf("\t Water:\262\262\262\262 \n");printf("\t Shallow water: \260\260\260\260\n");printf("\t Mountain: ^^^^\n");printf("\t Dust: ....\n");printf("\t* sher valley: VVVV *\n");printf("\t shallow valey: vvvv\n");printf("\t power station: \025\n");printf("\t alien: *_*\n");printf("\t other rovers: #\n");printf("\t* * *\n");scanf("%d",&i);switch(i) /*switch menu to go to the successor function, or the add/remove function*/ {case 1:successor();break;case 2:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);case 3:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);break;default:printf("choose a number from the list\n");}}void direction(int input, struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover){switch(input){case 119: /*w = up*/if(p_rover->location[0] > 0){p_rover->location[0]--;p_rover->pwr--;}else p_rover->location[0]=7;break;case 115: /*s = down*/if(p_rover->location[0] < 7){p_rover->location[0]++;p_rover->pwr--;}else p_rover->location[0]=0;break;case 97: /*a = left*/if(p_rover->location[1] > 0){p_rover->location[1]--;p_rover->pwr--;}else p_rover->location[1]=7;break;case 100: /*d = right*/if(p_rover->location[1] < 7){p_rover->location[1]++;p_rover->pwr--;}else p_rover->location[1]=0;break;default:printf("Invalid operator!\n\n");break;}}int control(int input){input = _getch();return input;}void main(void){int menu;int quit = 0;int input = 0;struct object_fix rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl, wdp;struct object_move aagr, anon, rvr, pstn;struct rover p_rover;struct map number, number_2;number.amount = 0; /* setting the initial amount of aliens as 0*/number_2.amount = 0;strcpy(,"Sandy Rock");strcpy(rsny.id,"RSNY");strcpy(rsny.desc,"A rock made of softer material that can be destroyed or collected"); strcpy(rsny.im,"*");strcpy(,"Solid Rock");strcpy(rsld.id,"RSLD");strcpy(rsld.desc,"A rock made of hard material that cannot be destroyed, but can be collected or avoided");strcpy(rsld.im,"O");strcpy(,"Mountain");strcpy(mnt.id,"MNT");strcpy(mnt.desc,"A large obstacle made of different materials that can only be avoided"); strcpy(mnt.im,"^^^^");strcpy(,"Dust");strcpy(dst.id,"DST");strcpy(dst.desc,"Small particles of sand, rock, etc. that may reduce visibility or slow down the vehicle, can be driven through but has extra power consumption");strcpy(dst.im,"....");strcpy(,"Sheer Valley");strcpy(vshr.id,"VSHR");strcpy(vshr.desc,"A depression in the land with sheer sides, it cannot be driven through and must be avoided");strcpy(vshr.im,"VVVV");strcpy(,"Gentle Valley");strcpy(vgnt.id,"VGNT");strcpy(vgnt.desc,"A depression in the land with gentle sides, it can be driven throughwith extra power consumption or can be avoided");strcpy(vgnt.im,"vvvv");strcpy(,"'Martian' Tree");strcpy(mtre.id,"MTRE");strcpy(mtre.desc,"A woody plant native to Mars, it may have the same characteristics as an 'Earth' tree");strcpy(mtre.im,"\330");strcpy(,"Shallow Water");strcpy(wshl.id,"WSHL");strcpy(wshl.desc,"A shallow body of water that can be driven through, can be in the form of a small puddle or a large lake");strcpy(wshl.im,"\260\260\260\260");strcpy(,"Deeper Water");strcpy(wdp.id,"WDP");strcpy(wdp.desc,"A deeper body of water that cannot be driven through, commonly in the form of a large lake or river");strcpy(wdp.im,"\262\262\262\262");strcpy(,"Aggressive Alien");strcpy(aagr.id,"AAGR");strcpy(aagr.desc,"An alien creature that will move upon its own accord, may become aggressive on contact");strcpy(aagr.im,"*_*");strcpy(,"Non-Aggressive Alien");strcpy(anon.id,"ANON");strcpy(anon.desc,"An alien creature that will move upon its own accord, will not become aggressive on contact");strcpy(anon.im,"*_*");strcpy(,"Rover");strcpy(rvr.id,"RVR");strcpy(rvr.desc,"A Mars Rover vehicle to transport around the map, it can destroy, collect or avoid objects. It has a power and object storage");strcpy(rvr.im,"#");strcpy(,"Power Station");strcpy(pstn.id,"PSTN");strcpy(pstn.desc,"A movable power station that will restore power to the Mars Rovers"); strcpy(pstn.im,"\025");strcpy(p_,"User Mars Rover");strcpy(p_rover.id,"URVR");strcpy(p_rover.desc,"A Mars vehicle that is controlled by the user. Explores the map collecting, destroying and avoiding objects");strcpy(p_rover.im,"<^^>");p_rover.pwr = 100;p_rover.strg = 0;p_rover.location[0] = 7;p_rover.location[1] = 0;/****************************************************/printf("*******************************START***************************** ***\n\n");do{ /* Object will be gained from mapper */printf("+++++++++++++++++++++++++++++++MENU++++++++++++++++ +++++++++++++++++\n");printf("Please choose one of the following option:\n");printf("1 - Start\n");printf("2 - Controls\n");printf("3 - Show Map\n");printf("4 - Help Menu\n");printf("5 - Quit\n\n");printf("--------------->");scanf("%d", &menu);switch (menu){case 1:show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);while (quit != 1 ){input = control(input);if (input == 27)quit = 1;else{if(p_rover.pwr>0){direction(input, &number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);printf("Rover stats: \nPower: %d%% \nStorage: %d%%\n", p_rover.pwr, p_rover.strg); }else{printf("*******************END GAME********************\n\n"); // ends game when charge = 0.break;}}}break;case 2:quit = 0;printf("\n");printf("* * *\n");printf(" Controls \n"); // menu for the keys used on the keyboard to move the rover in different directions.printf(" Up : W \n");printf(" Down : S \n");printf("* Left : A * \n");printf("* Right : D * \n");printf(" choose : Enter \n");printf("* * *\n");break;case 3:quit = 0;show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover); break;case 4:quit = 0;help(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover); break;case 5:printf("*********************END***********************\n"); break;}}while(menu!=5);printf("\n -----Please press any key to exit game-----\n");getch();}。
五子棋游戏 C语言 代码 源代码
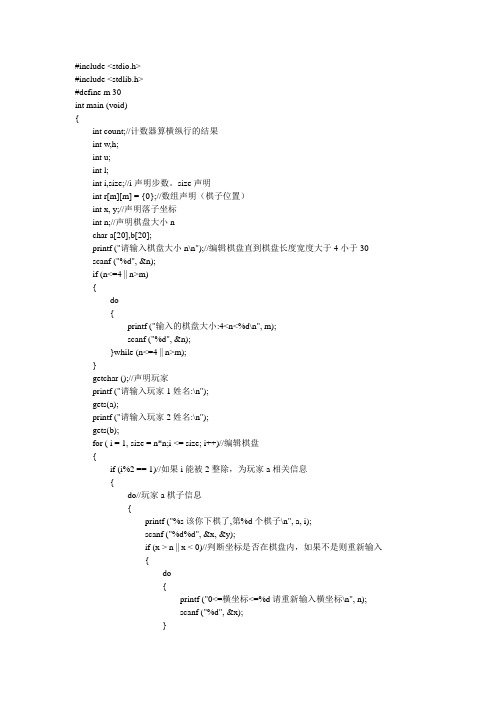
#include <stdio.h>#include <stdlib.h>#define m 30int main (void){int count;//计数器算横纵行的结果int w,h;int u;int l;int i,size;//i声明步数。
size声明int r[m][m] = {0};//数组声明(棋子位置)int x, y;//声明落子坐标int n;//声明棋盘大小nchar a[20],b[20];printf ("请输入棋盘大小n\n");//编辑棋盘直到棋盘长度宽度大于4小于30 scanf ("%d", &n);if (n<=4 || n>m){do{printf ("输入的棋盘大小:4<n<%d\n", m);scanf ("%d", &n);}while (n<=4 || n>m);}getchar ();//声明玩家printf ("请输入玩家1姓名:\n");gets(a);printf ("请输入玩家2姓名:\n");gets(b);for ( i = 1, size = n*n;i <= size; i++)//编辑棋盘{if (i%2 == 1)//如果i能被2整除,为玩家a相关信息{do//玩家a棋子信息{printf ("%s该你下棋了,第%d个棋子\n", a, i);scanf ("%d%d", &x, &y);if (x > n || x < 0)//判断坐标是否在棋盘内,如果不是则重新输入{do{printf ("0<=横坐标<=%d请重新输入横坐标\n", n);scanf ("%d", &x);}while (x>m || x<0);}if (y > n || y < 0)//判断坐标是否在棋盘内,如果不是则重新输入{do{printf ("0<=纵坐标<=%d请重新输入纵坐标\n", n);scanf ("%d", &y);}while (y < 0 || y > n);}}while ((r[x][y] == 1 && (printf ("这个位置上已经有棋子了,请重新输入\n")))|| r[x][y] == 2&& (printf ("这个位置上已经有棋子了,请重新输入\n")) );r[x][y] = 1;for (u = 0;u < n; u++)//不同情况下判断玩家a获胜方式{for (l = 0;l < n;l++){count = 0;for (w = u,h = l;r[w][h] == 1 && h < n; h++)count++;if (count == 5){printf ("%s是胜利者\n", a);goto e;//直接跳转,其余代码不在运行count = 0;for (w = u, h = l; r[w][h] == 1 && w < n; w++)count ++;if (count == 5){printf ("%s是胜利者\n", a);goto e;}count = 0;for (w = u,h = l; r[w][h] == 1 && w < n && h<n;w++,h++)count++;if (count == 5){printf ("%s是胜利者\n", a);goto e;}count = 0;for (w =u ,h =l;r[w][h] == 1 && h > 0;h--)count++;if (count == 5){printf ("%s是胜利者\n", a);goto e;}}}}system("cls");for (int j = n;j>=0;j--){printf ("%-2d", j);for (int k = 0;k < n;k++)//画棋盘,声明两玩家棋子图片{if (r[k][j] == 0)printf ("╋");else if(r[k][j] == 1)printf ("○");else if (r[k][j] == 2)printf ("●"); }printf ("\n");}printf (" ");for (int k = 0;k < n;k++)printf ("%-2d", k);}else if (i%2 == 0)//如果i不能被2整除,为玩家b相关信息{do{printf ("\n%s该你下棋了,第%d个棋子\n", b, i);scanf ("%d%d", &x, &y);if (x > n || x < 0){do{printf ("0<=横坐标<=%d请重新输入横坐标\n", n);scanf ("%d", &x);}while (x>n || x<0);}if (y >n|| y < 0){do{printf ("0<=纵坐标<=%d请重新输入纵坐标\n", n);scanf ("%d", &y);}while (y < 0 || y > n);}}while ((r[x][y] == 1 && (printf ("这个位置上已经有棋子了,请重新输入\n")))|| r[x][y] == 2&& (printf ("这个位置上已经有棋子了,请重新输入\n")) );r[x][y] = 2;system("cls");for (int j = n;j>=0;j--){printf ("%-2d", j);for (int k = 0;k < n;k++){if (r[k][j] == 0)printf ("╋");else if(r[k][j] == 1)printf ("○");else if (r[k][j] == 2)printf ("●");}printf ("\n");}printf (" ");for (int k = 0;k < n;k++)printf ("%-2d", k); printf ("\n");count = 0;for (u = 0;u < n; u++){for (l = 0;l < n;l++){count = 0;for (w = u,h = l;r[w][h] == 2 && h < n; h++)count++;if (count == 5){printf ("%s是胜利者\n", b);goto e;}count = 0;for (w = u, h = l; r[w][h] == 2 && w < n; w++)count ++;if (count == 5){printf ("%s是胜利者\n", b);goto e;}count = 0;for (w = u,h = l; r[w][h] == 2 && w < n && h<n;w++,h++)count++;if (count == 5){printf ("%s是胜利者\n", b);goto e;}count = 0;for (w =u ,h =l;r[w][h] == 2 && h > 0;h--)count++;if (count == 5){printf ("%s是胜利者\n", b);goto e;}}}}}e: for (int j = n;j>=0;j--)//游戏结束界面棋盘固定重新显示{printf ("%-2d", j);for (int k = 0;k < n;k++){if (r[k][j] == 0)printf ("╋");else if(r[k][j] == 1)printf ("○");else if (r[k][j] == 2)printf ("●");}printf ("\n");}printf (" ");for (int k = 0;k < n;k++)printf ("%-2d", k); printf ("\n");printf ("\a游戏愉快,Powered by Techmessager\n");//结束语句return 0;}。
飞机大战c语言代码
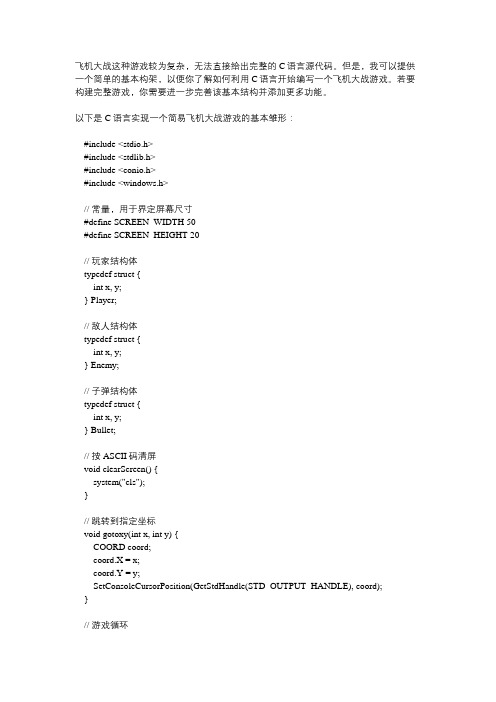
飞机大战这种游戏较为复杂,无法直接给出完整的C语言源代码。
但是,我可以提供一个简单的基本构架,以便你了解如何利用C语言开始编写一个飞机大战游戏。
若要构建完整游戏,你需要进一步完善该基本结构并添加更多功能。
以下是C语言实现一个简易飞机大战游戏的基本雏形:#include <stdio.h>#include <stdlib.h>#include <conio.h>#include <windows.h>// 常量,用于界定屏幕尺寸#define SCREEN_WIDTH 50#define SCREEN_HEIGHT 20// 玩家结构体typedef struct {int x, y;} Player;// 敌人结构体typedef struct {int x, y;} Enemy;// 子弹结构体typedef struct {int x, y;} Bullet;// 按ASCII码清屏void clearScreen() {system("cls");}// 跳转到指定坐标void gotoxy(int x, int y) {COORD coord;coord.X = x;coord.Y = y;SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord);}// 游戏循环void gameLoop() {Player player;Enemy enemy[10];Bullet bullet;int i;// 初始化游戏player.x = SCREEN_WIDTH / 2;player.y = SCREEN_HEIGHT - 1;// 敌人的初始位置for (i = 0; i < 10; i++) {enemy[i].x = i * 5 + 1;enemy[i].y = 1;}// 游戏主循环while (1) {clearScreen();// 绘制玩家gotoxy(player.x, player.y);printf("^");// 绘制敌人for (i = 0; i < 10; i++) {gotoxy(enemy[i].x, enemy[i].y);printf("V");}// 绘制子弹 (若存在)if (bullet.y >= 0) {gotoxy(bullet.x, bullet.y);printf("|");// 更新子弹位置bullet.y -= 1;// 判断是否击中敌人for (i = 0; i < 10; i++) {if (bullet.x == enemy[i].x && bullet.y == enemy[i].y) { enemy[i].y = -1; // 隐藏已经被击中的敌人}}}// 检测操作if (_kbhit()) {char input = _getch();switch (input) {case 'a': // 往左移动player.x -= 1;break;case 'd': // 往右移动player.x += 1;break;case ' ': // 发射子弹bullet.x = player.x;bullet.y = player.y - 1;break;case 'q': // 退出游戏exit(0);break;}}// 刷新画布Sleep(100);}}int main() {gameLoop();return 0;}这个代码示例使用了C语言(在Windows操作系统下运行),为玩家提供了移动(A,D键)和射击(空格键)功能。
c语言小游戏代码

c语言小游戏代码#include <stdio.h>#include <stdlib.h>#include <windows.h>// 定义元素类型#define ELEMENT char// 游戏行数#define ROW 10// 游戏显示延迟#define SLEEPTIME 100int main(int argc, char *argv[]){// 定义游戏的棋盘,用数组存放ELEMENT array[ROW][ROW];// 定义获胜条件int winCondition = 5;// 初始化,把棋盘清空system("cls");int i,j;for(i = 0; i < ROW; i++){for(j = 0; j < ROW; j++){array[i][j] = ' ';}}// 循环游戏,当有一方满足胜利条件时终止int tmp;int count = 0; // 存放棋子数while(1){// 依次取出玩家记录的棋子int x, y;// 如果已经有子落下,则计算是第几步if(count > 0){printf("第%d步:\n", count);}// 显示游戏棋盘for(i = 0; i < ROW; i++){printf(" ");for(j = 0; j < ROW; j++){printf("---");}printf("\n|");for(j = 0; j < ROW; j++){printf("%c |", array[i][j]);}printf("\n");}printf(" ");for(j = 0; j < ROW; j++){printf("---");}printf("\n");// 要求玩家输入放下棋子的位置printf("请玩家输入要放弃棋子的位置(1-%d)\n", ROW); printf("横坐标:");scanf("%d", &x);printf("纵坐标:");scanf("%d", &y);// 判断棋子位置是否有效if(x < 1 || x > ROW || y < 1 || y > ROW || array[x-1][y-1] != ' '){printf("输入错误!\n");system("pause");system("cls");continue;}// 把棋子记录,并计数if(count % 2 == 0){array[x-1][y-1] = 'X';}else{array[x-1][y-1] = 'O';}count++;// 判断是否有获胜者int i, j, k;int tempx, tempy;for(i = 0; i < ROW; i++){for(j = 0; j < ROW; j++){if(array[i][j] == 'X' || array[i][j] == 'O') {// 判断横向是否有获胜者tmp = 1;for(k = 1; k < winCondition; k++){// 注意边界,必须验证范围有效if(j + k > ROW - 1) break;// 如果和前一个位置的棋子相同,则计数加1,否则跳出if(array[i][j+k] == array[i][j])tmp++;else break;}// 如果计数满足获胜条件,则显示获胜者if(tmp >= winCondition){printf("玩家 %c 获胜!\n", array[i][j]);system("pause");return 0;}// 判断纵向是否有获胜者tmp = 1;for(k。
C语言小游戏源代《俄罗斯方块》

C语言小游戏源代码《俄罗斯方块》#include <stdlib.h>#include <stdio.h>#include <graphics.h>#define ESC 27#define UP 328#define DOWN 336#define LEFT 331#define RIGHT 333#define BLANK 32#define BOTTOM 2#define CANNOT 1#define CAN 0#define MAX 30#define F1 315#define ADD 43#define EQUAL 61#define DEC 45#define SOUNDs 115#define SOUNDS 83#define PAUSEP 80#define PAUSEp 112void Init();void Down();void GoOn();void ksdown();void Display(int color);void Give();int Touch(int x,int y,int dx,int dy);int GeyKey();void Select();void DetectFill();void GetScores();void Fail();void Help();void Quit();void DrawBox(int x,int y,int Color);void OutTextXY(int x,int y,char *String); void DispScore(int x,int y,char Ch);void DrawNext(int Color);int Heng=12,Shu=20; /*横竖*/int Position[MAX][MAX];int middle[MAX][MAX];int ActH,ActS;int Act,Staus;int i,j,k;int Wid=10;int NoPass=CAN;float Delays=15000;int BeginH=250,BeginS=7;float Seconds=0;int Scores=0;int flag=1;int Sounds=CAN;int PreAct,NextAct;int a[8][4][4][4]={{{1,0,0,0,1,0,0,0,1,0,0,0,1,0,0,0}, {1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0},{1,0,0,0,1,0,0,0,1,0,0,0,1,0,0,0},{1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0}},{{1,1,0,0,1,1,0,0,0,0,0,0,0,0,0,0},{1,1,0,0,1,1,0,0,0,0,0,0,0,0,0,0},{1,1,0,0,1,1,0,0,0,0,0,0,0,0,0,0},{1,1,0,0,1,1,0,0,0,0,0,0,0,0,0,0}},{{1,1,1,0,0,1,0,0,0,0,0,0,0,0,0,0},{0,1,0,0,1,1,0,0,0,1,0,0,0,0,0,0},{0,1,0,0,1,1,1,0,0,0,0,0,0,0,0,0},{1,0,0,0,1,1,0,0,1,0,0,0,0,0,0,0}},{{1,0,0,0,1,1,0,0,0,1,0,0,0,0,0,0},{0,1,1,0,1,1,0,0,0,0,0,0,0,0,0,0},{1,0,0,0,1,1,0,0,0,1,0,0,0,0,0,0},{0,1,1,0,1,1,0,0,0,0,0,0,0,0,0,0}},{{0,1,0,0,1,1,0,0,1,0,0,0,0,0,0,0},{1,1,0,0,0,1,1,0,0,0,0,0,0,0,0,0},{0,1,0,0,1,1,0,0,1,0,0,0,0,0,0,0},{1,1,0,0,0,1,1,0,0,0,0,0,0,0,0,0}},{{1,0,0,0,1,1,1,0,0,0,0,0,0,0,0,0},{1,1,0,0,1,0,0,0,1,0,0,0,0,0,0,0},{1,1,1,0,0,0,1,0,0,0,0,0,0,0,0,0}, {0,1,0,0,0,1,0,0,1,1,0,0,0,0,0,0}}, {{0,0,1,0,1,1,1,0,0,0,0,0,0,0,0,0}, {1,0,0,0,1,0,0,0,1,1,0,0,0,0,0,0}, {1,1,1,0,1,0,0,0,0,0,0,0,0,0,0,0}, {1,1,0,0,0,1,0,0,0,1,0,0,0,0,0,0}}, {{1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, {1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, {1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, {1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0}}}; int b[4][4];main(int argc,char *argv[]){if (argc!=1){if (argv[1]!="")Heng=atoi(argv[1]);if (argv[2]!="")Shu=atoi(argv[2]);}Init(); /*初始化界面*/PreAct=random(8); /*取得当前的方块*/ for(;;) /*以下是游戏流程*/{NextAct=random(8); /*取得下一个方块*/ DrawNext(1); /*画出下一个方块*/Act=PreAct;if (Heng%2==0) ActH=Heng/2;else ActH=(Heng-1)/2;ActS=0; /*方块开始从游戏空间的中间下落*/Staus=0; /*取开始的状态*/NoPass=CAN; /*物体可以下落*/Give(); /*取得当前的方块*/Display(Act+1); /*显示当前的方块,每种方块的颜色不同*/ GoOn(); /*游戏的算法精髓所在*/PreAct=NextAct; /*方块下落完毕,取得下一个方块*/ DrawNext(0);}}void Init(){int GraphDriver=DETECT,GraphMode;registerbgidriver(EGAVGA_driver);initgraph(&GraphDriver,&GraphMode,"");if (kbhit()) Sounds=CANNOT;setcolor(1);OutTextXY(10,10,"Tetris");OutTextXY(30,30,"Version 2.0");OutTextXY(10,120,"Help:");OutTextXY(20,140,"+ :Faster");OutTextXY(20,160,"- :Slower");OutTextXY(20,180,"Esc :Quit");OutTextXY(20,200,"F1 :Help");OutTextXY(10,310,"Copyright(c) 1998.2.22");OutTextXY(10,320,"By Mr. Unique");outtextxy(10,250,"Score: 00000");rectangle(BeginH-3,BeginS-3,BeginH+Heng*(Wid+2)+2,BeginS+Shu*(Wid+2)+2);rectangle(BeginH-5,BeginS-5,BeginH+Heng*(Wid+2)+4,BeginS+Shu*(Wid+2)+4);rectangle(BeginH+(Heng+4)*(Wid+2)-2,BeginS+10,BeginH+(Heng+8)*(Wid+2)+2,BeginS+12+4*( Wid+2));for (i=0;i<MAX;i++)for (j=0;j<MAX;j++){Position[i][j]=1;middle[i][j]=-1;}for (i=0;i<Heng;i++)for (j=0;j<Shu;j++)Position[i][j]=0;for (i=0;i<Heng;i++)for (j=0;j<Shu;j++)DrawBox(i,j,0);randomize();}void GoOn(){for(;;){Seconds+=0.2; /*控制方块的下落速度*/if (Seconds>=Delays){Down();Seconds=0;if (NoPass==BOTTOM){DetectFill();middle[ActH][ActS]=Act;if (ActS==0)Fail();return;}}if (kbhit())Select();}}void Down() /*方块下降*/{Display(0);if (Touch(ActH,ActS,0,1)==CAN) ActS++;elsemiddle[ActH][ActS]=Act; Display(Staus+1);}int Touch(int x,int y,int dx,int dy) {NoPass=CAN;for (i=0;i<4;i++)for (j=0;j<4;j++)Position[x+dx+i][y+dy+j]+=b[i][j];for (i=0;i<MAX;i++)for (j=0;j<MAX;j++)if (Position[i][j]>1)NoPass=CANNOT;for (i=0;i<4;i++)for (j=0;j<4;j++){Position[x+dx+i][y+dy+j]-=b[i][j]; middle[x+dx+i][y+dy+j]=Act;}if (NoPass==CANNOT && dx==0 && dy==1) {for (i=0;i<4;i++)for (j=0;j<4;j++)Position[x+i][y+j]+=b[i][j];NoPass=BOTTOM;}return NoPass;}int GetKey(void){int Ch,Low,Hig;Ch=bioskey(0);Low=Ch&0x00ff;Hig=(Ch&0xff00)>>8;return(Low==0?Hig+256:Low);}void Select(){int OldStaus,acts=ActS;switch(GetKey()){case ESC :Quit();break;case DOWN :Seconds+=14500;break;case LEFT :Display(0);if (ActH>0 && Touch(ActH,ActS,-1,0)==CAN) { ActH--;}Display(Act+1);break;case RIGHT :Display(0);if (ActH<Heng && Touch(ActH,ActS,1,0)==CAN) { ActH++;}Display(Act+1);break;case BLANK : Display(0);ksdown();Display(Act+1);break;case F1 :Help();break;case EQUAL :case ADD :if (Delays>300) Delays-=100;break; case DEC :if (Delays<3000) Delays+=100;break; case PAUSEP :case PAUSEp :getch();break;case SOUNDS :case SOUNDs :if (Sounds==CAN)Sounds=CANNOT;elseSounds=CAN;break;case UP :if(Act==7){while(acts<Shu-1&&Position[ActH][acts]!=1) acts++;Position[ActH][acts]=0;DrawBox(ActH,acts,0);acts=ActS;break;}else{Display(0);OldStaus=Staus;switch(Act){case 0:case 3:case 4:if (Staus==1) Staus=0;else Staus=1;break; case 1:break;case 2:case 5:case 6:if (Staus==3) Staus=0;else Staus++;break; }Give();if (Touch(ActH,ActS,0,0)==CANNOT){Staus=OldStaus;Give();}Display(Act+1);break;}}}void ksdown(){while(flag){if(Touch(ActH,ActS,0,0)==CAN){ActS++;}else {ActS--;flag=0;}}flag=1;}void Quit(){int ch,TopScore;FILE *fp;if ((fp=fopen("Russian.scr","r+"))!=NULL) {fscanf(fp,"%d",&TopScore);if (Scores>TopScore){setcolor(1);outtextxy(470,80,"Hello !");outtextxy(470,100,"In all the players,"); outtextxy(470,120,"You are the First !"); outtextxy(470,140,"And your score will"); outtextxy(470,160,"be the NEW RECORD !"); fseek(fp,0L,0);fprintf(fp,"%d",Scores);}fclose(fp);}setcolor(1);OutTextXY(470,220,"Are You Sure (Yes/no)?");ch=getch();if (ch=='y'||ch=='Y'){closegraph();delay(20);exit(0);}setcolor(0);outtextxy(470,220,"Are You Sure (Yes/no)?"); }void OutTextXY(int x,int y,char *String) {int i=0;char a[2];moveto(x,y);a[1]='\0';while (*(String+i)!='\0'){a[0]=*(String+i);outtext(a);if (Sounds==CAN && a[0]!=' '){sound(3000);delay(50);nosound();}i++;}}void Help(){unsigned Save;void *Buf;Save=imagesize(160,120,500,360);Buf=malloc(Save);getimage(160,120,500,360,Buf);setfillstyle(1,1);bar(160,120,500,280);setcolor(0);OutTextXY(170,130," About & Help");OutTextXY(170,150," # # # ########## # # # "); OutTextXY(170,160," # ## # # # # # # ###### ### "); OutTextXY(170,170," ########## ########## ## # # "); OutTextXY(170,180," # # # # # # # ## #### "); OutTextXY(170,190," # ## # #### ## # # # "); OutTextXY(170,200," # ## # # # # # ## # # # "); OutTextXY(170,210," # # # ## ## # ###### # # # "); OutTextXY(170,220," ## # ## # ## # # # # "); OutTextXY(170,230," # ## # #### # ## # "); OutTextXY(170,260," Good Luckly to You !!! ");getch();putimage(160,120,Buf,0);free(Buf);}void GetScores(){int Sec10000,Sec1000,Sec100,Sec10,Sec1; setfillstyle(0,1);bar(60,250,109,260);Sec1=Scores%10;Sec10=(Scores%100-Scores%10)/10;Sec100=(Scores%1000-Scores%100)/100;Sec1000=(Scores%10000-Scores%1000)/1000; Sec10000=(Scores%100000-Scores%10000)/10000; DispScore(60,250,'0'+Sec10000);DispScore(70,250,'0'+Sec1000);DispScore(80,250,'0'+Sec100);DispScore(90,250,'0'+Sec10);DispScore(100,250,'0'+Sec1);DispScore(110,250,'0');DispScore(120,250,'0');}void DispScore(int x,int y,char Ch){char a[2];a[1]='\0';a[0]=Ch;outtextxy(x,y,a);void Give(){for (i=0;i<4;i++)for (j=0;j<4;j++)b[i][j]=a[Act][Staus][i][j];}void Display(int color){for (i=0;i<4;i++)for (j=0;j<4;j++)if (b[i][j]==1) DrawBox(ActH+i,ActS+j,color); }void DrawBox(int x,int y,int Color){x=BeginH+x*(Wid+2);y=BeginS+y*(Wid+2);setfillstyle(1,Color);bar(x+2,y+2,x+Wid-1,y+Wid-1);if (Color==0)setcolor(9);elsesetcolor(Act+1);rectangle(x+4,y+4,x+Wid-4,y+Wid-4);}void DrawNext(int Color)for (i=0;i<4;i++)for (j=0;j<4;j++)if (a[NextAct][0][i][j]==1) DrawBox(Heng+4+i,1+j,Color); }void DetectFill(){int Number,Fall,FallTime=0;for (i=Shu-1;i>=0;i--){Number=0;for (j=0;j<Heng;j++)if (Position[j][i]==1) Number++;if (Number==Heng){FallTime++;if (Sounds==CAN){sound(500);delay(500);nosound();}for (Fall=i;Fall>0;Fall--)for (j=0;j<Heng;j++){Position[j][Fall]=Position[j][Fall-1];middle[j][Fall]=middle[j][Fall-1];if (Position[j][Fall]==0) DrawBox(j,Fall,0); else DrawBox(j,Fall,middle[j][Fall]+1);}i++;}}switch(FallTime){case 0:break;case 1:Scores+=1;break;case 2:Scores+=3;break;case 3:Scores+=6;break;case 4:Scores+=10;break;}if (FallTime!=0){GetScores();if (Scores%100==0) Delays-=100;}}void Fail(){if (Sounds==CAN){for (k=0;k<3;k++){sound(300);delay(200);nosound();}}setcolor(1);OutTextXY(440,200,"Game over!"); Quit();closegraph();exit(0);}。
c语言扫雷源代码

c语言扫雷源代码扫雷游戏是一种非常经典的单人益智游戏,玩家需要根据数字提示找出地雷的位置。
下面给出一个简单的C语言扫雷游戏的源代码示例:```c#include <stdio.h>#include <stdlib.h>#include <time.h>#define SIZE 10 // 扫雷游戏的大小#define MINE_COUNT 10 // 地雷的数量typedef struct {int x;int y;} Position;typedef struct {int isMine; // 是否是地雷int isFlagged; // 是否被标记为地雷int isRevealed; // 是否已被翻开int adjacentMines; // 相邻地雷的数量} Cell;Cell board[SIZE][SIZE];void initializeBoard() {int i, j;// 初始化游戏面板for (i = 0; i < SIZE; i++) {for (j = 0; j < SIZE; j++) {board[i][j].isMine = 0;board[i][j].isFlagged = 0;board[i][j].isRevealed = 0;board[i][j].adjacentMines = 0;}}}void generateMines() {int i, j;int count = 0;srand(time(NULL)); // 以当前时间作为随机数种子 while (count < MINE_COUNT) {i = rand() % SIZE;j = rand() % SIZE;// 如果该格子已经是地雷,则重新生成随机位置 if (!board[i][j].isMine) {board[i][j].isMine = 1;count++;}}}void calculateAdjacentMines() {int i, j, k, l;int dx[] = {-1, -1, -1, 0, 0, 1, 1, 1};int dy[] = {-1, 0, 1, -1, 1, -1, 0, 1};for (i = 0; i < SIZE; i++) {for (j = 0; j < SIZE; j++) {if (!board[i][j].isMine) {for (k = 0; k < 8; k++) {int ni = i + dx[k];int nj = j + dy[k];if (ni >= 0 && ni < SIZE && nj >= 0 && nj < SIZE && board[ni][nj].isMine) {board[i][j].adjacentMines++;}}}}}}void displayBoard() {int i, j;printf(" ");for (i = 0; i < SIZE; i++) {printf("%d ", i);}printf("\n");for (i = 0; i < SIZE; i++) {printf("%d ", i);for (j = 0; j < SIZE; j++) {if (board[i][j].isRevealed) {if (board[i][j].isMine) {printf("M ");} else {printf("%d ", board[i][j].adjacentMines);}} else if (board[i][j].isFlagged) {printf("F ");} else {printf(". ");}}printf("\n");}}void revealAdjacentEmptyCells(Position pos) {int dx[] = {-1, -1, -1, 0, 0, 1, 1, 1};int dy[] = {-1, 0, 1, -1, 1, -1, 0, 1};int i;for (i = 0; i < 8; i++) {int ni = pos.x + dx[i];int nj = pos.y + dy[i];if (ni >= 0 && ni < SIZE && nj >= 0 && nj < SIZE && !board[ni][nj].isRevealed && !board[ni][nj].isMine) { board[ni][nj].isRevealed = 1;if (board[ni][nj].adjacentMines == 0) {Position newPos;newPos.x = ni;newPos.y = nj;revealAdjacentEmptyCells(newPos);}}}}int main() {Position pos;char cmd;int isSuccess = 0;initializeBoard();generateMines();calculateAdjacentMines();displayBoard();while (!isSuccess) {printf("Enter command (R: reveal, F: flag): ");fflush(stdout);scanf(" %c", &cmd);printf("Enter position (x, y): ");fflush(stdout);scanf("%d %d", &(pos.x), &(pos.y));if (cmd == 'R') {board[pos.x][pos.y].isRevealed = 1;if (board[pos.x][pos.y].isMine) {printf("Game Over!\n");isSuccess = 1;} else if (board[pos.x][pos.y].adjacentMines == 0) {revealAdjacentEmptyCells(pos);}} else if (cmd == 'F') {board[pos.x][pos.y].isFlagged = 1;}displayBoard();}return 0;}```上述代码实现了一个简单的C语言扫雷游戏。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
/* A simple game*//*CopyRight: Guanlin*/#include<stdio.h>#include<stdlib.h>#include<string.h>#include<time.h>#include<conio.h>#include<process.h>struct object_fix{char name[20];char id[5];char desc[500];char action[30];char im[5];};struct object_move{char name[20];char id[5];char desc[500];int loc;int pwr;int strg;char im[5];};struct rover{char name[20];char id[5];char desc[500];int pwr;int strg;int location[2];char im[5];};struct map /* this is the map structure*/{char data[20];char add_data[20];int amount;int x; /* this were the successor keeps it's x & y values*/ int y;};struct location /*this structure is for the successor lister*/ {float height;char obj;void stats_update(int selected, struct rover *p_rover){switch (selected){case 1:if(p_rover->pwr < 7)printf("\n\nYou do not have enough power to perform this action!\n\n");else{(p_rover->pwr) -= 7;printf("You have destroyed the object!\n\n");}break;case 2:if(p_rover->pwr < 3)printf("\n\nYou do not have enough power to perform this action!\n\n");else if(p_rover->strg > 90)printf("\n\nYou do not have enough storage space for this object!\n\n");else{(p_rover->pwr) -= 3;(p_rover->strg) += 10;printf("You have collected a sample of the object!\n\n");}break;case 3:p_rover->pwr -= 10; /*Distance around object- value gained from mapper module. 1 square = -1 power*/printf("You have avoided the object!\n\n");break;case 4:p_rover->pwr -= 2;printf("You have driven through the obstacle!\n\n");break;case 5:if(p_rover->pwr == 100)printf("\n\nYou do not need to charge up!\n\n");else{p_rover->pwr = 100;printf("You have charged up your rover!\n\n");}break;default:printf("\n\n*****ERROR*****\nInvalid Selection\n\n");break;}}void action(char object, struct rover *p_rover)int selection;switch(object){case 1:printf("\nYou have encountered: A Sandy Rock\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\nPlease choose action 1 or2:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 2:printf("\nYou have encountered: A Solid Rock\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 3:printf("\nYou have encountered: A Mountain\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 4:printf("\nYou have encountered: Dust\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\nPlease choose action 1or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 5:printf("\nYou have encountered: A Sheer Valley\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 6:printf("\nYou have encountered: A Gentle Valley\n\n");printf("This object can be:\n1.\tDriven through\n2.\tAvoided\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 4;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;case 7:printf("\nYou have encountered: A 'Martian' Tree\n\n");printf("This object can be:\n1.\tDestroyed\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);stats_update(selection, p_rover);break;case 8:printf("\nYou have encountered: Shallow Water\n\n");printf("This object can be:\n1.\tDriven through\n2.\tCollected\n3.\tAvoided\nPlease choose action 1, 2 or 3:\t");scanf("%d", &selection);if (selection == 1)selection = 4;stats_update(selection, p_rover);break;case 9:printf("\nYou have encountered: Deep Water\n\n");printf("This object can be:\n1.\tAvoided\n2.\tCollected\nPlease choose action 1 or 2:\t"); scanf("%d", &selection);if (selection == 1)selection = 3;stats_update(selection, p_rover);break;case 10:printf("\nYou have encountered: An Aggressive Alien\n\n");printf("This object can be:\n1.\tDestroyed\nPlease enter 1:\t");scanf("%d", &selection);selection = 1;stats_update(selection, p_rover);break;case 11:printf("\nYou have encountered: A Non-Aggressive Alien\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 12:printf("\nYou have encountered: Another Rover\n\n");printf("This object can be:\n1.\tAvoided\nPlease enter 1:\t");scanf("%d", &selection);selection = 3;stats_update(selection, p_rover);break;case 13:printf("\nYou have encountered: A Power Station\n\n");printf("You can:\n1.\tCharge up\n2.\tAvoid\nPlease choose action 1 or 2:\t");scanf("%d", &selection);if (selection == 1)selection = 5;if (selection == 2)selection = 3;stats_update(selection, p_rover);break;default:printf("\n\n*****ERROR*****\n\n");break;}}void show_map(struct map *number, struct map *number_2, struct object_fix *rsny, struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /*the show map function calling number and number_2 from the map structure to see if moveable objects are needed*/{struct map f_map[8][8]; /*8 by 8 map*/int i,j, rx, ry, object; /*this is your x and y value in your map (f_map)*/system("cls"); /* this is your x and y value in your map (f_map)*///srand(time(NULL)); /*calling the time from include to gather random variables*/for(i=0;i<8;i++) /*for loop to copy all your fixed object lists into f_map so they can be displayed*/{for(j=0;j<8;j++)strcpy(f_map[i][j].data,"");}if (number->amount>0) /*this is looking at the add function to see weather or not there are aliens in f_map*/{for(i=0;i<number->amount;i++) /*allocating the number of aliens in f_map*/strcpy(f_map[rand()%8][rand()%8].data, anon->im); /*randomizing their position*/}if(number_2->amount>0) /*repeat of above just for rovers instead*/{for(i=0;i<number_2->amount;i++)strcpy(f_map[rand()%8][rand()%8].data, rvr->im);}rx= p_rover->location[0];ry= p_rover->location[1];strcpy(f_map[1][0].data, wdp->im);strcpy(f_map[4][0].data, mnt->im);strcpy(f_map[5][0].data, mnt->im);strcpy(f_map[3][1].data, dst->im);strcpy(f_map[4][1].data, dst->im);strcpy(f_map[3][2].data, dst->im);strcpy(f_map[4][2].data, dst->im);strcpy(f_map[0][3].data, mnt->im);strcpy(f_map[1][3].data, mnt->im);strcpy(f_map[3][3].data, vshr->im);strcpy(f_map[4][3].data, dst->im);strcpy(f_map[5][3].data, vgnt->im);strcpy(f_map[3][4].data, vshr->im);strcpy(f_map[4][4].data, dst->im);strcpy(f_map[5][4].data, vgnt->im);strcpy(f_map[2][5].data, wshl->im);strcpy(f_map[3][5].data, wshl->im);strcpy(f_map[4][5].data, wshl->im);strcpy(f_map[1][6].data, pstn->im);strcpy(f_map[2][6].data, wdp->im);strcpy(f_map[3][6].data, wdp->im);strcpy(f_map[4][6].data, wshl->im);strcpy(f_map[7][6].data, mnt->im);strcpy(f_map[0][7].data, mnt->im);strcpy(f_map[1][7].data, wdp->im);strcpy(f_map[2][7].data, wshl->im);strcpy(f_map[3][7].data, wshl->im);strcpy(f_map[6][7].data, mnt->im);strcpy(f_map[rx][ry].data, p_rover->im);if((rx == 1 && ry == 0) || (rx == 2 && ry == 6) ||(rx == 3 && ry == 6) ||(rx == 1 && ry == 7)){object = 9;action(object, p_rover);}else if((rx == 4 && ry == 0) || (rx == 5 && ry == 0) || (rx == 0 && ry == 3) || (rx == 1 && ry == 3) || (rx == 7 && ry == 6) || (rx == 0 && ry == 7) || (rx == 6 && ry == 7)){object = 3;action(object, p_rover);}else if((rx == 3 && ry== 1) || (rx == 4 && ry == 1) || (rx == 3 && ry== 2) || (rx == 4 && ry == 2) || (rx == 4 && ry == 3) || (rx == 4 && ry == 4)){object = 4;action(object, p_rover);}else if((rx == 3 && ry == 3) || (rx == 3 && ry == 4)){object = 5;action(object, p_rover);}else if((rx == 5 && ry == 3) || (rx == 5 && ry == 4)){object = 6;action(object, p_rover);}else if((rx == 2 && ry == 5) || (rx == 3 && ry == 5 ) || (rx == 4 && ry == 5) || (rx== 4 && ry == 6) ||(rx == 2 && ry == 7) || (rx == 3 && ry == 7)){object = 8;action(object, p_rover);}else if(rx == 1 && ry == 6){object = 13;action(object, p_rover);}i=0; /*re-allocate i to 0 so map is printed from start*/for(i=0;i<8;i++) /*8 by 8 map*/{printf("+----+----+----+----+----+----+----+----+\n");for(j=0;j<8;j++) /*8 by 8 map*/{if(strlen(f_map[i][j].data)!=0) /*if function to print nothing but 4 spaces if there in nothing allocated in [i][j]*/printf("|%4s",f_map[i][j].data);elseprintf("| "); /*end of coloumn visible map*/}printf("|\n"); /*end of last coloumn visible map*/}printf("+----+----+----+----+----+----+----+----+\n"); /*bottom of map*/printf("\n");printf("\270");printf("Group B\n");}void add_obj(struct map *number, struct map *number_2, struct object_fix *rsny, struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /* add movable object function, *number=aliens, *number_2=rovers*/ {int object, t, f;char alien;char rover;printf("This is the add movable object function\n");printf("how many aliens would you like?\n");scanf("%d",&t); /*user input of amount of aliens*/number->amount=t; /*saving the number in structure map-amount*/printf("how many rovers would you like?\n");scanf("%d",&f); /*user input of amount of rovers*/number_2->amount=f; /*saving the number in structure map-amount*/show_map(number, number_2, rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl,wdp, aagr, anon, rvr, pstn, p_rover); /*go to show map function with the number of aliens and number of rovers*/}void successor() /*this functions askes the user for the location and then were they want to go from there, printing out that location*/{struct map location;int menu;int ncol,nrow; /*we can change the n value depends how large the map you need.*/ ncol=8;nrow=8;printf("This is the successor function\n");printf("Enter the \nx=\ty=\n");scanf("%d%d",&location.x,&location.y); /*saving location in map location x and y*/ printf("Enter operator choice\n");printf("1=left\n");printf("2=right\n");printf("3=upwards\n");printf("4=down\n");printf("5=quit\n");scanf("%d",&menu);switch(menu) /*menu switch to show the position above, underneith, and next to the rover*/{case 1:location.x=location.x-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 2:location.x=location.x+1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 3:location.y=location.y-1;printf("The position is [%d,%d]\n",location.x,location.y);break;case 4:location.y=location.y+1;printf("The position is [%d,%d]\n",location.x,location.y);break;printf("*****back to program*****\n"); /* Quits the program and prints out the message */break;default:printf("*****Please enter an integer from 1-5*****\n"); /* A default option if the user enters an incorrect value */break;}printf("\n");}void help(struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover) /*help function*/{int i;char mov_obj;printf("++This is the help function++\n");printf("| 1-successor |\n");printf("| 2-add movable obj |\n");printf("| 3-remove moveabla obj |\n\n");printf("\t* * *\n");printf("\t Index: \n\n");printf("\t Sandy Rock: *\n");printf("\t Solid Rock: o\n");printf("\t Water:\262\262\262\262 \n");printf("\t Shallow water: \260\260\260\260\n");printf("\t Mountain: ^^^^\n");printf("\t Dust: ....\n");printf("\t* sher valley: VVVV *\n");printf("\t shallow valey: vvvv\n");printf("\t power station: \025\n");printf("\t alien: *_*\n");printf("\t other rovers: #\n");printf("\t* * *\n");scanf("%d",&i);switch(i) /*switch menu to go to the successor function, or the add/remove function*/ {case 1:successor();break;case 2:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);case 3:add_obj(number, number_2, rsny, rsld, mnt, dst, vshr,vgnt, mtre, wshl, wdp, aagr, anon, rvr, pstn, p_rover);break;default:printf("choose a number from the list\n");}}void direction(int input, struct map *number, struct map *number_2, struct object_fix *rsny,struct object_fix *rsld, struct object_fix *mnt, struct object_fix *dst,struct object_fix *vshr, struct object_fix *vgnt, struct object_fix *mtre,struct object_fix *wshl, struct object_fix *wdp, struct object_move *aagr,struct object_move *anon, struct object_move *rvr, struct object_move *pstn, struct rover *p_rover){switch(input){case 119: /*w = up*/if(p_rover->location[0] > 0){p_rover->location[0]--;p_rover->pwr--;}else p_rover->location[0]=7;break;case 115: /*s = down*/if(p_rover->location[0] < 7){p_rover->location[0]++;p_rover->pwr--;}else p_rover->location[0]=0;break;case 97: /*a = left*/if(p_rover->location[1] > 0){p_rover->location[1]--;p_rover->pwr--;}else p_rover->location[1]=7;break;case 100: /*d = right*/if(p_rover->location[1] < 7){p_rover->location[1]++;p_rover->pwr--;}else p_rover->location[1]=0;break;default:printf("Invalid operator!\n\n");break;}}int control(int input){input = _getch();return input;}void main(void){int menu;int quit = 0;int input = 0;struct object_fix rsny, rsld, mnt, dst, vshr, vgnt, mtre, wshl, wdp;struct object_move aagr, anon, rvr, pstn;struct rover p_rover;struct map number, number_2;number.amount = 0; /* setting the initial amount of aliens as 0*/number_2.amount = 0;strcpy(,"Sandy Rock");strcpy(rsny.id,"RSNY");strcpy(rsny.desc,"A rock made of softer material that can be destroyed or collected"); strcpy(rsny.im,"*");strcpy(,"Solid Rock");strcpy(rsld.id,"RSLD");strcpy(rsld.desc,"A rock made of hard material that cannot be destroyed, but can be collected or avoided");strcpy(rsld.im,"O");strcpy(,"Mountain");strcpy(mnt.id,"MNT");strcpy(mnt.desc,"A large obstacle made of different materials that can only be avoided"); strcpy(mnt.im,"^^^^");strcpy(,"Dust");strcpy(dst.id,"DST");strcpy(dst.desc,"Small particles of sand, rock, etc. that may reduce visibility or slow down the vehicle, can be driven through but has extra power consumption");strcpy(dst.im,"....");strcpy(,"Sheer Valley");strcpy(vshr.id,"VSHR");strcpy(vshr.desc,"A depression in the land with sheer sides, it cannot be driven through and must be avoided");strcpy(vshr.im,"VVVV");strcpy(,"Gentle Valley");strcpy(vgnt.id,"VGNT");strcpy(vgnt.desc,"A depression in the land with gentle sides, it can be driven throughwith extra power consumption or can be avoided");strcpy(vgnt.im,"vvvv");strcpy(,"'Martian' Tree");strcpy(mtre.id,"MTRE");strcpy(mtre.desc,"A woody plant native to Mars, it may have the same characteristics as an 'Earth' tree");strcpy(mtre.im,"\330");strcpy(,"Shallow Water");strcpy(wshl.id,"WSHL");strcpy(wshl.desc,"A shallow body of water that can be driven through, can be in the form of a small puddle or a large lake");strcpy(wshl.im,"\260\260\260\260");strcpy(,"Deeper Water");strcpy(wdp.id,"WDP");strcpy(wdp.desc,"A deeper body of water that cannot be driven through, commonly in the form of a large lake or river");strcpy(wdp.im,"\262\262\262\262");strcpy(,"Aggressive Alien");strcpy(aagr.id,"AAGR");strcpy(aagr.desc,"An alien creature that will move upon its own accord, may become aggressive on contact");strcpy(aagr.im,"*_*");strcpy(,"Non-Aggressive Alien");strcpy(anon.id,"ANON");strcpy(anon.desc,"An alien creature that will move upon its own accord, will not become aggressive on contact");strcpy(anon.im,"*_*");strcpy(,"Rover");strcpy(rvr.id,"RVR");strcpy(rvr.desc,"A Mars Rover vehicle to transport around the map, it can destroy, collect or avoid objects. It has a power and object storage");strcpy(rvr.im,"#");strcpy(,"Power Station");strcpy(pstn.id,"PSTN");strcpy(pstn.desc,"A movable power station that will restore power to the Mars Rovers"); strcpy(pstn.im,"\025");strcpy(p_,"User Mars Rover");strcpy(p_rover.id,"URVR");strcpy(p_rover.desc,"A Mars vehicle that is controlled by the user. Explores the map collecting, destroying and avoiding objects");strcpy(p_rover.im,"<^^>");p_rover.pwr = 100;p_rover.strg = 0;p_rover.location[0] = 7;p_rover.location[1] = 0;/****************************************************/printf("*******************************START***************************** ***\n\n");do{ /* Object will be gained from mapper */printf("+++++++++++++++++++++++++++++++MENU++++++++++++++++ +++++++++++++++++\n");printf("Please choose one of the following option:\n");printf("1 - Start\n");printf("2 - Controls\n");printf("3 - Show Map\n");printf("4 - Help Menu\n");printf("5 - Quit\n\n");printf("--------------->");scanf("%d", &menu);switch (menu){case 1:show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);while (quit != 1 ){input = control(input);if (input == 27)quit = 1;else{if(p_rover.pwr>0){direction(input, &number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover);printf("Rover stats: \nPower: %d%% \nStorage: %d%%\n", p_rover.pwr, p_rover.strg); }else{printf("*******************END GAME********************\n\n"); // ends game when charge = 0.break;}}}break;case 2:quit = 0;printf("\n");printf("* * *\n");printf(" Controls \n"); // menu for the keys used on the keyboard to move the rover in different directions.printf(" Up : W \n");printf(" Down : S \n");printf("* Left : A * \n");printf("* Right : D * \n");printf(" choose : Enter \n");printf("* * *\n");break;case 3:quit = 0;show_map(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover); break;case 4:quit = 0;help(&number, &number_2, &rsny, &rsld, &mnt, &dst, &vshr,&vgnt, &mtre, &wshl, &wdp, &aagr, &anon, &rvr, &pstn, &p_rover); break;case 5:printf("*********************END***********************\n"); break;}}while(menu!=5);printf("\n -----Please press any key to exit game-----\n");getch();}。