第六章 多态性与虚函数
虚函数与多态性实验

一.实验目的及要求1.进一步熟悉类的设计、运用继承与派生机制设计派生类,合理设置数据成员和成员函数。
2.掌握通过继承、虚函数、基类的指针或引用实现动态多态性的方法。
3.理解并掌握具有纯虚函数的抽象类的作用,在各派生类中重新定义各纯虚函数的方法,以及此时实现的动态多态性。
二.实验内容在自己的文件夹下建立一个名为exp5的工程,在该工程中做如下操作:定义一个抽象类容器类,其中定义了若干纯虚函数,实现求表面积、体积、输出等功能。
由此抽象类派生出正方体、球体和圆柱体等多个派生类,根据需要定义自己的成员变量,在各个派生类中重新定义各纯虚函数,实现各自类中相应功能,各个类成员的初始化均由本类构造函数实现。
(1)在主函数中,定义容器类的指针和各个派生类的对象,使指针指向不同对象处调用相同的函数能执行不同的函数代码,从而实现动态多态性。
(2)定义一个顶层函数void TopPrint(Container &r);使得主函数中调用该函数时,根据实在参数所有的类自动调用对应类的输出函数。
(3)主函数中定义一个Container类对象,观察编译时的错误信息,从而得出什么结论三.实验程序及运行结果#include <iostream>using namespace std;class Base{public:virtual void f(){ cout << "调用Base::f()" << endl; }};class Derived: public Base{public:void f(){ cout << "调用Derived::f()" << endl; } // 虚函数};int main(void){Derived obj; // 定义派生类对象Base *p = &obj; // 基类指针p->f(); // 调用函数f()system("PAUSE");return 0;}2.#include <iostream>using namespace std; //class Base{public:virtual void Show() const{ cout << "调用Base::Show()" << endl; } // 虚函数};class Derived: public Base{public:void Show() const{ cout << "调用Derived::Show()" << endl; }};void Refers(const Base &obj) // 基类引用{obj.Show(); // 调用函数Show()}int main(void){Base obj1;Derived obj2; // 定义对象Refers(obj1); // 调用函数Refers()Refers(obj2);system("PAUSE");return 0;}3.#include <iostream>using namespace std; /class Base{private:int m;public:Base(int a): m(a){ }virtual void Show() const{ cout << m << endl; }// 虚函数};class Derived: public Base{private:int n;public:Derived(int a, int b): Base(a), n(a){ } // 构造函数void Show() const{cout << n << ","; /Base::Show(); // 调用基类的Show() }};int main(void){Base obj1(168);Derived obj2(158, 98);Base *p;p = &obj1;p->Show();p = &obj2;p->Show();p->Base::Show();system("PAUSE");return 0;}4.#include <iostream>using namespace std;class A{public:virtual void Show() const{ cout << "基类A" << endl; } };class B: public A{public:void Show() const{ cout << "派生类B" << endl; } };int main(void){B obj;A *p = &obj;p->Show();system("PAUSE");return 0;}5.#include <iostream>using namespace std;const double PI = 3.1415926;class Shape{public:virtual void Show() const = 0;static double sum;};class Circle: public Shape{private:double radius;public:Circle(double r): radius(r){ sum += PI * radius * radius; }void Show() const{cout << "圆形:" << endl;cout << "半径:" << radius << endl;cout << "面积:" << PI * radius * radius << endl;}};class Rectangle: public Shape{private:double height;double width;public:Rectangle(double h, double w): height(h), width(w){ sum += height * width; }void Show() const{cout << "矩形:" << endl;cout << "高:" << height << endl;cout << "宽:" << width << endl;cout << "面积:" << height * width << endl;}};double Shape::sum = 0;int main(void){char flag = 'Y'; 'Shape *p;while (toupper(flag) == 'Y'){cout << "请选择输入类别(1.圆形2.矩形)";int select;cin >> select;switch (select){case 1:double r;cout << "输入半径:";cin >> r;p = new Circle(r);p->Show();delete p;break;case 2:double h, w;cout << "输入高:";cin >> h;cout << "输入宽:";cin >> w;p = new Rectangle(h, w);p->Show(); // 显示相关信息delete p; // 释放存储空间break;default: // 其它情况, 表示选择有误cout << "选择有误!"<< endl;break;}cout << endl << "是否继续录入信息?(Y/N)";cin >> flag;}cout << "总面积:" << Shape::sum << endl;system("PAUSE");return 0;}6.#include <iostream>using namespace std;const double PI = 3.1415926;const int NUM = 10;class Shape{public:virtual void ShowArea() const = 0;static double sum;};class Circle: public Shape{private:double radius;public:Circle(double r): radius(r){ sum += PI * radius * radius; }void ShowArea() const{ cout << "圆面积:" << PI * radius * radius << endl; }};class Rectangle: public Shape{private:double height;double width;public:Rectangle(double h, double w): height(h), width(w){ sum += height * width; }void ShowArea() const{ cout << "矩形面积:" << height * width << endl; }};class Square: public Shape{private:double length;public:Square(double a): length(a){ sum += length * length; }void ShowArea() const{ cout << "正方形面积:" <<length * length << endl; } };double Shape::sum = 0;int main(void){Shape *shape[NUM];int count = 0;while (count < NUM){cout << "请选择(1.圆形2.矩形3.正方形4.退出):";int select;cin >> select;if (select == 4) break;switch (select){case 1:double r;cout << "输入半径:";cin >> r;shape[count] = new Circle(r);shape[count]->ShowArea();count++;break;case 2:double h, w;cout << "输入高:";cin >> h;cout << "输入宽:";cin >> w;shape[count] = new Rectangle(h, w);shape[count]->ShowArea();count++;break;case 3:double a;cout << "输入边长:";cin >> a;shape[count] = new Square(a);shape[count]->ShowArea();count++;break;default:cout << "选择有误!"<< endl;break;}}cout << "总面积:" << Shape::sum << endl;for (int i = 0; i < count; i++) delete shape[i];system("PAUSE");return 0;}五.实验总结通过本次试验 我更深刻的理解了某些语句如何使用及结构体的优点 也能更加熟练的编写简单的程序了 我深知实践要比书本更加重要 今后还要多练习 在实践中学习。
C++面向对象概述

C++类定义的基本形式
C++类定义的基本形式:
class { private: <私有数据成员和私有成员函数的声明列表>; <类名>
public:
<公有数据成员和公有成员函数的声明列表>;
protected:
<保护数据成员和保护成员函数的声明列表>; };
说明:
类的定义由关键字 class 开始,其后为用户定义 的类名,花括号括起来的部分称为类体。 关键字private、public和protected称为访问权限 控制符,用来设置数据成员和成员函数的访问 属性,其默认值为private。 private 属性表示数据成员和成员函数是类的私 有成员,它们只允许被本类的成员函数访问或 调用,数据成员一般定义为private属性;
对象作为对象的成员使用。 私有数据成员hour、minute 和second只能在类的成员 函数中被访问或赋值;
类的实现:
利用 C++ 类进行面向对象编程,定义类的成员 只是完成了工作的第一步,最重要的工作是实 现定义的类。 类的实现实质上是类的成员函数的实现,即定 义类的成员函数。 成员函数的定义形式与一般函数的定义形式基 本相同,但必须在成员函数名前加上类名和作 用域限定符(::)。 成员函数的定义也可放在类体内(该函数声明之 处),这时成员函数将变成内联函数。
之间通过函数参数和全局变量进行相互联系。
结构化程序设计的特点:
与非结构化程序相比,结构化程序在调试、可读
性和可维护性等方面都有很大的改进。
代码重用性不高:以过程为中心设计新系统,除
了一些标准函数,大部分代码都必须重新编写。
c#第6章 继承与多态性

例如: 例如: class Humen { public string name; name; public string sex; sex; public string work ; } class Teacher:Humen Teacher: { public string speciality ; public string department; department; } Human是基类 Teacher是派生类 Human是基类,Teacher是派生类,它拥有基类 是基类, 是派生类, 的全部成员。 的全部成员。
派生类隐藏基类成员 :用new关键字。 new关键字 关键字。 隐藏基类的字段成员: 隐藏基类的字段成员: 派生类可以声明与基类有相同的名称和类型的字 派生类可以声明与基类有相同的名称和类型的字 段成员来隐藏基类的字段。 来隐藏基类的字段 段成员来隐藏基类的字段。这时通过派生类引用 或对象访问的是派生类的字段, 或对象访问的是派生类的字段,基类的相应成员 被屏蔽了。但是通过指向派生类对象的基类引用 被屏蔽了。但是通过指向派生类对象的基类引用 访问的则是基类的字段。 访问的则是基类的字段。 隐藏基类的方法成员: 隐藏基类的方法成员: 派生类可以声明与基类有相同的方法名称和形参 表的方法成员来隐藏基类的方法 基类的方法, 表的方法成员来隐藏基类的方法,与返回类型无 这时通过派生类引用或对象访问的是派生类 关。这时通过派生类引用或对象访问的是派生类 的方法成员,基类的相应方法成员被屏蔽了。 的方法成员,基类的相应方法成员被屏蔽了。但 是通过指向派生类对象的基类引用访问的则是基 指向派生类对象的基类引用访问的则是 是通过指向派生类对象的基类引用访问的则是基 类的成员。 类的成员。 派生类中可以通过 可以通过base关键字访问被隐藏的基 在派生类中可以通过base关键字访问被隐藏的基 类成员。 类成员。 详见例MaskBase。 详见例MaskBase。
【C++面向对象的程序设计】6多态性
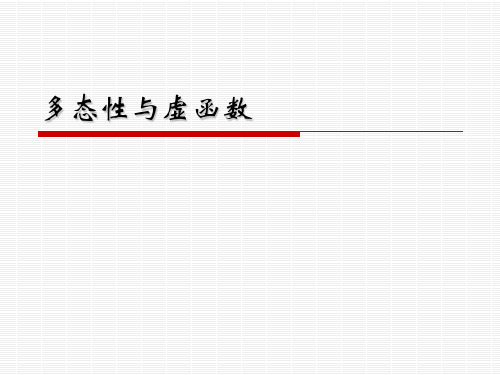
虚析构函数
析构函数的作用是对象撤销之后清理现场。 在派生类对象撤销时,一般先调用派生类的 析构函数。再调用基类的析构函数。
然而,当定义的是一个指向基类的指针变量, 使用new运算符建立临时对象时,如果基类 中有析构函数,则在使用delete析构时只会 调用基类的析构函数。
这就需要将基类中的析构函数声明为虚函数。
虚函数的声明与使用
声明虚函数的一般格式如下: virtual 函数原型;
⑴ 必须首先在基类中声明虚函数。 ⑵ 派生类中与基类虚函数原型完全相同的成员函 数,即使在说明时前面没有冠以关键字virtual也 自动成为虚函数。
声明虚函数
⑶ 只有非静态成员函数可以声明为虚函数。 ⑷ 不允许在派生类中定义与基类虚函数名字及参数 特征都相同,仅仅返回类型不同的成员函数。 编译时 出错。 ⑸ 系统把函数名相同但参数特征不同的函数视为不 同的函数。 ⑹ 通过声明虚函数来使用C++提供的多态性机制时, 派生类应该从它的基类公有派生。
构函数等内容。
本章内容
静态联编与动态联编 虚函数的声明与使用 纯虚函数和抽象类 虚析构函数
Hale Waihona Puke 静态联编与动态联编所谓联编(tinding),就是使一个计算机程序的不同部 分彼此关联的过程。
静态联编在编译阶段完成,因为所有联编过程都在程 序开始运行之前完成,因此静态联编也叫先前联编或早期 联编。
另一种情况编译程序在编译时并不确切知道应把发送 到对象的消息和实现消息的哪段具体代码联编在一起,而 是在运行时才能把函数调用与函数体联系在一起,则称为 动态联编。
动态联编的实现
C ++语言中的动态联编是通过使用虚函数表 (Virtual Function Table)来实现的,虚函数表也称 为v-表。
实验四 虚函数与多态性

实验四虚函数与多态性实验目的和要求 :1 了解多态性在 C++ 中的体现。
2 掌握虚函数的应用。
3 了解抽象类。
实验内容:定义一个基类为哺乳动物类Mammal,其中有数据成员年龄、重量、品种,有成员函数move()、speak()等,以此表示动物的行为。
由这个基类派生出狗、猫、马、猪等哺乳动物,它们有各自的行为。
编程分别使各个动物表现出不同的行为。
要求如下:1、从基类分别派生出各种动物类,通过虚函数实现不同动物表现出的不同行为。
2、今有狗: CAIRN:3岁,3kg;DORE:4岁,2kg;猫: CAT:5 岁,4kg;马: HORSE,5岁,60kg;猪: PIG,2岁,45kg。
3、设置一个 Mammal 类数组,设计一个屏幕菜单,选择不同的动物或不同的品种,则显示出动物相对应的动作,直到选择结束。
4、对应的动作中要先显示出动物的名称,然后显示年龄、重量、品种、叫声及其他特征。
实验原理:1.多态性:多态是指同样的消息被不同类型的对象接受时导致不同的行为,所谓消息是指对类的成员函数的调用,而不同的行为是指不同的实现,也就是调用不同的函数。
多态性从实现的角度来讲可以划分为两类:编译时的多态性和运行时的多态性。
编译时的多态性是在编译的过程中确定同名操作的具体操作对象,也就是通过重载函数来实现的。
运行时的的多态性是在程序运行过程中才动态地确定操作所针对的具体对象,使用虚函数来实现的。
2.虚函数:虚函数是重载的另一种形式,它提供了一种更为灵活的多态性机制。
虚函数允许函数调用与函数体之间的联系在运行时才建立,也就是运行时才决定如何动作,即所谓的“动态连接”。
虚函数成员的定义:virtual 函数类型函数名(形参表)3.(1)抽象类:抽象类是一种特殊的类。
抽象类是为了抽象和设计的目的而建立的。
一个抽象类自身无法实例化,也就是说无法定义一个抽象类的对象,只能通过继承机制,生成抽象类的非抽象派生类,然后再实例化。
多态性与虚函数实验报告

多态性与虚函数实验报告实验目的:通过实验掌握多态性和虚函数的概念及使用方法,理解多态性实现原理和虚函数的应用场景。
实验原理:1.多态性:多态性是指在面向对象编程中,同一种行为或者方法可以具有多种不同形态的能力。
它是面向对象编程的核心特性之一,能够提供更加灵活和可扩展的代码结构。
多态性主要通过继承和接口来实现。
继承是指子类可以重写父类的方法,实现自己的特定行为;接口是一种约束,定义了类应该实现的方法和属性。
2.虚函数:虚函数是在基类中声明的函数,它可以在派生类中被重新定义,以实现多态性。
在类的成员函数前面加上virtual关键字,就可以将它定义为虚函数。
当使用基类指针或引用调用虚函数时,实际调用的是派生类的重写函数。
实验步骤:1. 创建一个基类Shape,包含两个成员变量color和area,并声明一个虚函数printArea(用于打印面积。
2. 创建三个派生类Circle、Rectangle和Triangle,分别继承Shape类,并重写printArea(函数。
3. 在主函数中,通过基类指针分别指向派生类的对象,并调用printArea(函数,观察多态性的效果。
实验结果与分析:在实验中,通过创建Shape类和派生类Circle、Rectangle和Triangle,可以实现对不同形状图形面积的计算和打印。
当使用基类指针调用printArea(函数时,实际调用的是派生类的重写函数,而不是基类的函数。
这就是多态性的实现,通过基类指针或引用,能够调用不同对象的同名函数,实现了对不同对象的统一操作。
通过实验1.提高代码的可扩展性和灵活性:通过多态性,可以将一类具有相似功能的对象统一管理,节省了代码的重复编写和修改成本,增强了代码的可扩展性和灵活性。
2.简化代码结构:通过虚函数,可以将各个派生类的不同行为统一命名为同一个函数,简化了代码结构,提高了代码的可读性和维护性。
3.支持动态绑定:通过运行时的动态绑定,可以根据对象的实际类型来确定调用的函数,实现了动态绑定和多态性。
C++实验指导书专业资料

C++程序设计实验指导书实验一C++程序的运营环境和运营(2学时)实验名称: C++程序的运营环境和运营实验目的:1.熟悉C与C++的编程区别;2.熟悉C++的函数重载。
实验规定:求两个数的平方和。
规定如下:1、有int, float和long型的数据各3个;2.重载SumSqure函数求两个相同类型变量的平方和。
1、实验环节:2、添加头文献#include <iostream>和名字空间using namespace std。
若要使用cin和cout标准输入输出流, 则必须添加上述两个内容。
3、构建重载SumSqure函数。
SumSqure函数的功能是对输入的两个同类型形参a, b求其平方和, 并将结果返回。
对于输入和返回的不同类型int, float, long, 其函数内部实现代码是同样的, 所以可运用函数的重载写出三个SumSqure函数。
4、main函数中的赋值。
定义int, float, long三种类型的数据, 分别调用SumSqure函数, 测试其结果。
5、调用SumSqure函数。
注意SumSqure函数重载的调用, 根据SumSqure函数的定义可知: 实参必须是同一种类型的变量才干调用不同的SumSqure, 针对不同类型求平方和。
实验二类与对象(一)(4学时)实验名称: 类与对象(一)实验目的:1.掌握类的设计;2.掌握对象的创建;3.实现一个简朴的成员函数设计。
实验规定:求3个长方体的体积, 编写一个基于对象的程序, 数据成员涉及lenth, width, height。
规定用成员函数实现以下功能:1.由键盘分别输入3个长方体的长、宽、高;2.计算长方体的体积;3.输出3个长方体的体积。
实验环节:建立三个文献, 分别存储长方体类的声明头文献, 长方体类的定义文献和main函数测试文献。
注意: 类的头文献和类的定义实现文献的命名要一致!头文献信息:头文献长方体类的声明中, 类成员变量有:lenth,width,height;类成员函数有:V olumeCalculation(), InputData()。
c++多态性与虚函数习题答案
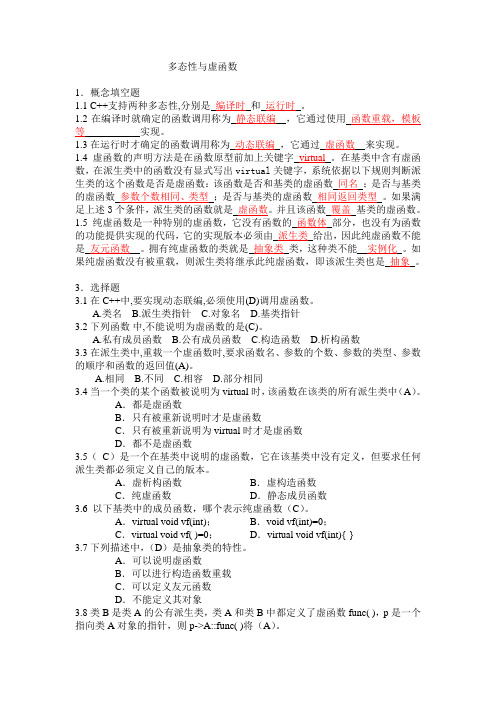
多态性与虚函数1.概念填空题1.1 C++支持两种多态性,分别是编译时和运行时。
1.2在编译时就确定的函数调用称为静态联编,它通过使用函数重载,模板等实现。
1.3在运行时才确定的函数调用称为动态联编,它通过虚函数来实现。
1.4虚函数的声明方法是在函数原型前加上关键字virtual。
在基类中含有虚函数,在派生类中的函数没有显式写出virtual关键字,系统依据以下规则判断派生类的这个函数是否是虚函数:该函数是否和基类的虚函数同名;是否与基类的虚函数参数个数相同、类型;是否与基类的虚函数相同返回类型。
如果满足上述3个条件,派生类的函数就是虚函数。
并且该函数覆盖基类的虚函数。
1.5 纯虚函数是一种特别的虚函数,它没有函数的函数体部分,也没有为函数的功能提供实现的代码,它的实现版本必须由派生类给出,因此纯虚函数不能是友元函数。
拥有纯虚函数的类就是抽象类类,这种类不能实例化。
如果纯虚函数没有被重载,则派生类将继承此纯虚函数,即该派生类也是抽象。
3.选择题3.1在C++中,要实现动态联编,必须使用(D)调用虚函数。
A.类名B.派生类指针C.对象名D.基类指针3.2下列函数中,不能说明为虚函数的是(C)。
A.私有成员函数B.公有成员函数C.构造函数D.析构函数3.3在派生类中,重载一个虚函数时,要求函数名、参数的个数、参数的类型、参数的顺序和函数的返回值(A)。
A.相同B.不同C.相容D.部分相同3.4当一个类的某个函数被说明为virtual时,该函数在该类的所有派生类中(A)。
A.都是虚函数B.只有被重新说明时才是虚函数C.只有被重新说明为virtual时才是虚函数D.都不是虚函数3.5(C)是一个在基类中说明的虚函数,它在该基类中没有定义,但要求任何派生类都必须定义自己的版本。
A.虚析构函数B.虚构造函数C.纯虚函数D.静态成员函数3.6 以下基类中的成员函数,哪个表示纯虚函数(C)。
A.virtual void vf(int);B.void vf(int)=0;C.virtual void vf( )=0;D.virtual void vf(int){ }3.7下列描述中,(D)是抽象类的特性。
虚函数的用法

虚函数的用法
虚函数是面向对象编程中的一个重要概念。
它允许子类重写父类中的同名函数,以实现多态性。
在C++中,使用关键字"virtual"来声明一个函数为虚函数。
虚函数的使用有以下几个关键点:
1. 多态性:虚函数的主要作用是实现多态性。
当一个指向父类的指针或引用调用一个虚函数时,实际执行的是子类中重写的函数。
这种行为允许在运行时根据对象的实际类型来确定调用的函数。
2. 动态绑定:虚函数的调用是动态绑定的,也就是说在运行时确定具体调用的函数。
与之相反的是静态绑定,它是在编译时确定调用的函数。
动态绑定使得程序具有更大的灵活性和扩展性。
3. 虚函数表:为了实现动态绑定,编译器会为每个包含虚函数的类创建一个虚函数表(vtable)。
虚函数表是一个存储函数指针的数组,每个函数指针指向对应虚函数的实际实现。
每个对象都有一个指向其类的虚函数表的指针,通过这个指针可以实现动态调用。
4. 纯虚函数:有时候父类中的虚函数并不需要有实际的实现,而只
是为了在子类中进行重写。
这种函数被称为纯虚函数,可以通过在函数声明中添加"= 0" 来表示。
包含纯虚函数的类被称为抽象类,它只能作为基类使用,不能被实例化。
综上所述,虚函数是实现多态性的关键机制之一。
通过在父类中声明虚函数并在子类中重写,可以实现基于对象实际类型的动态绑定,提高程序的灵活性和可扩展性。
总结
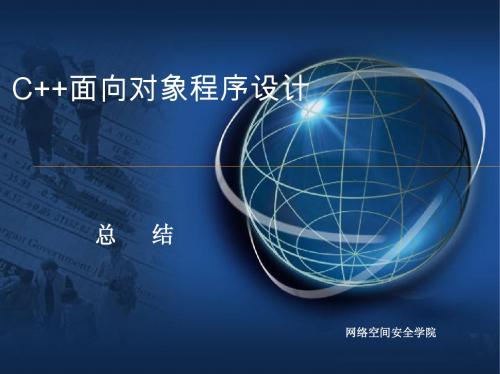
C++
11
第六章 多态性和虚函数
三、虚函数 1.虚函数的声明格式 2.虚函数的作用 3.虚函数的使用方法
4.虚析构函数
四、纯虚函数和抽象类 1.纯虚函数:作用、声明 2.抽象类
C++ 12
C++ 7
第五章 继承和派生
一、继承和派生的基本概念及实现 1.继承的基本概念:继承、派生、继承和派生的目的 2.派生类的声明 3.类成员的访问控制——继承方式 二、多继承和单继承 1.多继承和单继承 2.多继承中派生类的声明
C++
8
第五章 继承和派生
三、派生类的构造、析构函数 1.继承中的构造函数 2.继承中的析构函数 3.同名隐藏规则
C++
5
第三章 类和对象的进一步讨论
五、友元函数和友元类 友元函数和友元类:声明、使用
C++
6
第四章 运算符重载
一、运算符重载的实质和规则 1.运算符重载的实质:运算符重载的实质、必要性、实现机制 2.运算符重载的规则和限制 二、运算符重载的两种形式 1.运算符重载为成员函数 2.运算符重载为友元函数 三、类类型转换 1. 转换构造函数作用 2.类型转换函数
3.对象指针:指向对象的指针、指向对象成员的指针(数据成 员和成员函数)
C++
4.自引用指针
4
第三章 类和对象的进一步讨论
三、共用数据的保护 1.常对象、常对象成员、常成员函数、指向对象的常指针、指 向常对象的指针变量、对象的常引用 四、静态数据成员和静态成员函数 1.静态数据成员的声明和初始化 2.静态成员函数的定义和访问
C++
多态性与虚函数
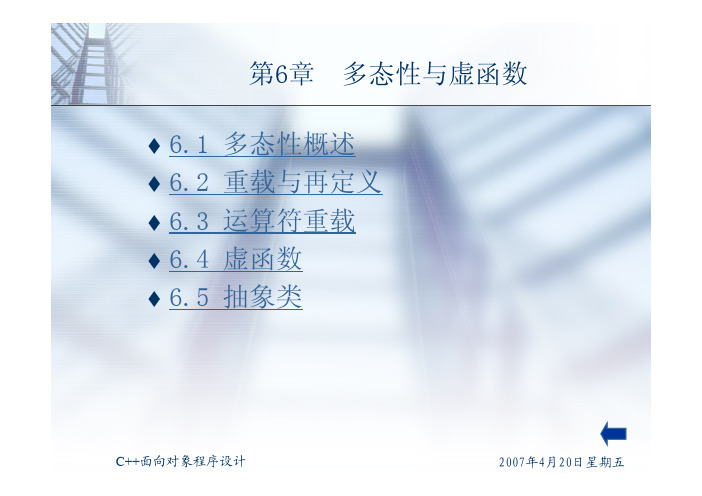
第6章多态性与虚函数6.1 多态性概述6.2 重载与再定义6.3 运算符重载6.4 虚函数6.5 抽象类6.1 多态性概述6.1.1 多态的类型 6.1.2 多态的实现6.1.1 多态的类型多态性是指同一个消息被不同类型的对象接收时产生不同的行为,特点就是一个接口,多个实现。
C++中多态性按照类型可以分为强制多态、过载多态、包含多态和参数多态四种。
z强制多态是指通过语义操作,强制数据做本不属于该类型数据的操作。
编译器内部的数据的隐式转换,比如3.0+4操作时转换成3.0+4.0就属于此种类型z重载多态是指函数重载,同名的操作在不同的环境下有不同的行为。
前面学习过的普通函数重载和将要学习的类成员函数重载、运算符重载都属于此种类型。
z包含多态是指在同一类族中定义于不同类中的同名函数的多态行为。
我们本章将要学习的虚函数就属于此种类型。
z参数多态是指功能、结构实现相同但所作用于的数据类型不同,也就是数据类型参数化的多态。
第七章中的函数模板和类模板就属于此种多态。
6.1.2 多态的实现多态是指同一个消息被不同的对象接收产生不同的行为,因此,多态在实现的时候必须确定消息的操作对象。
我们根据消息和对象相结合的时间分为两种:z在程序编译连接阶段完成的,也就是说在编译的过程中确定了消息的操作对象,我们称为静态绑定。
z在程序运行阶段完成的,也就是说在程序运行的过程中才确定消息的操作对象,我们称为动态绑定。
6.2 重载与再定义6.2.1 函数的重载 6.2.2 函数的再定义6.2.1 函数的重载函数重载是指功能相似,函数名相同但所带参数不同的一组函数。
这里的“所带参数不同”既可能是参数的数据类型不同也可能是参数的个数不同。
z普通函数的重载。
int abs(int val){return val<0? –val: val;}float abs(float val){return val>0? –val: val;}6.2.1 函数的重载z类成员函数的重载。
C++继承,虚函数与多态性专题
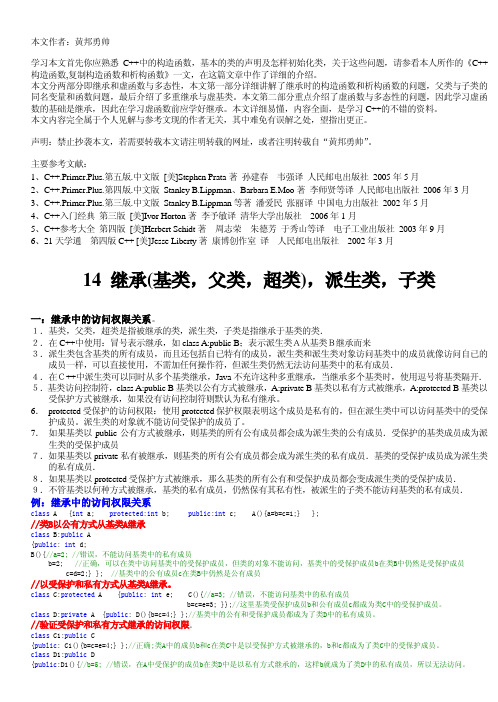
本文作者:黄邦勇帅学习本文首先你应熟悉C++中的构造函数,基本的类的声明及怎样初始化类,关于这些问题,请参看本人所作的《C++构造函数,复制构造函数和析构函数》一文,在这篇文章中作了详细的介绍。
本文分两部分即继承和虚函数与多态性,本文第一部分详细讲解了继承时的构造函数和析构函数的问题,父类与子类的同名变量和函数问题,最后介绍了多重继承与虚基类。
本文第二部分重点介绍了虚函数与多态性的问题,因此学习虚函数的基础是继承,因此在学习虚函数前应学好继承。
本文详细易懂,内容全面,是学习C++的不错的资料。
本文内容完全属于个人见解与参考文现的作者无关,其中难免有误解之处,望指出更正。
声明:禁止抄袭本文,若需要转载本文请注明转载的网址,或者注明转载自“黄邦勇帅”。
主要参考文献:1、C++.Primer.Plus.第五版.中文版[美]Stephen Prata著孙建春韦强译人民邮电出版社2005年5月2、C++.Primer.Plus.第四版.中文版Stanley B.Lippman、Barbara E.Moo著李师贤等译人民邮电出版社2006年3月3、C++.Primer.Plus.第三版.中文版Stanley B.Lippman等著潘爱民张丽译中国电力出版社2002年5月4、C++入门经典第三版[美]Ivor Horton著李予敏译清华大学出版社2006年1月5、C++参考大全第四版[美]Herbert Schidt著周志荣朱德芳于秀山等译电子工业出版社2003年9月6、21天学通第四版C++ [美]Jesse Liberty著康博创作室译人民邮电出版社2002年3月14 继承(基类,父类,超类),派生类,子类一:继承中的访问权限关系。
1.基类,父类,超类是指被继承的类,派生类,子类是指继承于基类的类.2.在C++中使用:冒号表示继承,如class A:public B;表示派生类A从基类B继承而来3.派生类包含基类的所有成员,而且还包括自已特有的成员,派生类和派生类对象访问基类中的成员就像访问自已的成员一样,可以直接使用,不需加任何操作符,但派生类仍然无法访问基类中的私有成员.4.在C++中派生类可以同时从多个基类继承,Java不充许这种多重继承,当继承多个基类时,使用逗号将基类隔开.5.基类访问控制符,class A:public B基类以公有方式被继承,A:private B基类以私有方式被继承,A:protected B基类以受保护方式被继承,如果没有访问控制符则默认为私有继承。
[09_10(2)]09本④⑤班《面向对象程序设计C++》教学进度表(程细柱)
![[09_10(2)]09本④⑤班《面向对象程序设计C++》教学进度表(程细柱)](https://img.taocdn.com/s3/m/d5e0560c7cd184254b3535fc.png)
实验4:类与对象(二)
实验5:继承和派生
实验6:多态性与虚函数
实验7:函数模板和类模板
实验8:输入输出的格式控制
实验9:文件的输入与输出
复习
考试
介绍面向对象程序设计的基本概念、基本特征、主要优点
使学生了解C++语言的特点、C++源程序的构成
使学生掌握类与对象的构成与定义
使学生掌握对象数组、静态成员与友元的特点与使用
使学生掌握if和switch选择结构、while和for循环结构及实现循环的方法
使学生掌握类和对象的定义及类成员的访问控制方式
使学生掌握对象数组与对象指针的定义与使用方法
使学生理解继承的含义,掌握派生类的定义方法和实现
使学生理解多态性的基本概念,掌握虚函数和纯虚函数的概念
使学生掌握C++中函数模板和类模板的使用方法
韶关学院09—10学年第二学期《面向对象程序设计C++》教学进度表
院(系):计算机科学系年级(班):09本④⑤班人数:113学时:72学分:4
课程编号011204700选用教材《C++面向对象程序设计》陈维兴中国铁道出版社
填表日期10年2月26日
周次
起止日期
章节
教学目标
教学形式
备注
1
2
3
4、5
6、7
使学生能用C++预定义的I/O类进行流处理
使学生掌握文件的输入输出方法
成绩评定,考试成绩质量分析
讲授
讲授
讲授
讲授
讲授
讲授
讲授
讲授
讲授
自学
辅导
辅导
《C++面向对象程序设计》 谭浩强 第六章

C++
先声明基类point类,并调试之; 再声明派生类circle类,调试之; 最后声明cylinder类,调试之。
6- 5
① 先声明基类point
#include <iostream.h> class point //声明类point {public: point (float x=0, float y=0 ); //有默认值的构造函数 void setPoint (float, float); //设置点坐标 float getX ( ) const { return x; } // 读x 值 float getY ( ) const { return y; } // 读y 值 friend ostream & operator << ( ostream &, const point &); protected: float x,y; }; // 下面定义 point类的成员函数 point :: point (float a, float b) // point的构造函数 { x = a; y = b; } void point :: setPoint (float a, float b) { x =a; y = b; } ostream &operator << (ostream &output, const point &p) { // 重载运算符<< output<<“[x=“<<p.x<<“, y=“<<p.y<<“]”<<endl; return output; }
6Hale Waihona Puke 7③ 最后声明cylinder类
C++实验指导书
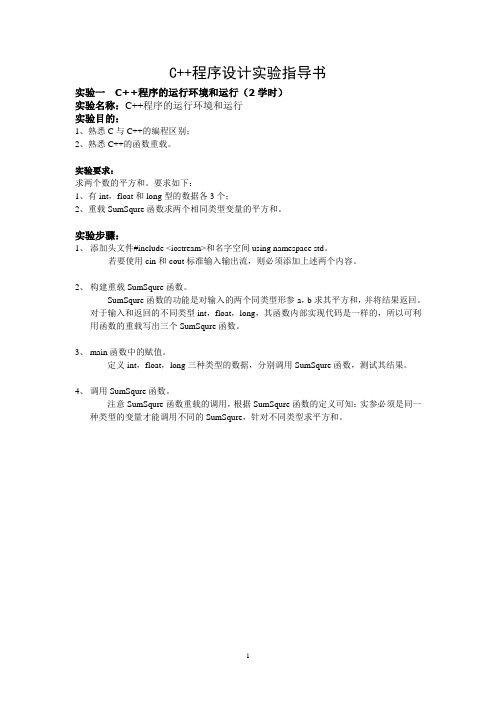
C++程序设计实验指导书实验一C++程序的运行环境和运行(2学时)实验名称:C++程序的运行环境和运行实验目的:1、熟悉C与C++的编程区别;2、熟悉C++的函数重载。
实验要求:求两个数的平方和。
要求如下:1、有int,float和long型的数据各3个;2、重载SumSqure函数求两个相同类型变量的平方和。
实验步骤:1、添加头文件#include <iostream>和名字空间using namespace std。
若要使用cin和cout标准输入输出流,则必须添加上述两个内容。
2、构建重载SumSqure函数。
SumSqure函数的功能是对输入的两个同类型形参a,b求其平方和,并将结果返回。
对于输入和返回的不同类型int,float,long,其函数内部实现代码是一样的,所以可利用函数的重载写出三个SumSqure函数。
3、main函数中的赋值。
定义int,float,long三种类型的数据,分别调用SumSqure函数,测试其结果。
4、调用SumSqure函数。
注意SumSqure函数重载的调用,根据SumSqure函数的定义可知:实参必须是同一种类型的变量才能调用不同的SumSqure,针对不同类型求平方和。
实验名称:类与对象(一)实验目的:1、掌握类的设计;2、掌握对象的创建;3、实现一个简单的成员函数设计。
实验要求:求3个长方体的体积,编写一个基于对象的程序,数据成员包括lenth,width,height。
要求用成员函数实现以下功能:1、由键盘分别输入3个长方体的长、宽、高;2、计算长方体的体积;3、输出3个长方体的体积。
实验步骤:1、建立三个文件,分别存储长方体类的声明头文件,长方体类的定义文件和main函数测试文件。
注意:类的头文件和类的定义实现文件的命名要一致!2、头文件信息:头文件长方体类的声明中,类成员变量有:lenth,width,height;类成员函数有:V olumeCalculation(), InputData()。
C++程序设计基础第6章 虚函数与多态性

6.2.1 虚函数的定义
2. 虚函数的定义 • 虚函数的定义是在基类中进行的 。 • 虚函数的定义语法格式如下:
virtual<函数类型><函数名>(形参表) {
函数体 }
12
6.2.1 虚函数的定义
3. 定义虚函数时,要注意遵循以下规则: 1)只有成员函数才能声明为虚函数,因为虚
函数仅适用于有继承关系的类对象,所以 普通函数不能声明为虚函数。 2)虚函数的声明只能出现在类声明中的函数 原型声明中,而不能出现在成员的函数体 实现上。 3)类的静态成员函数不可以定义为虚函数, 因为静态成员函数不受限于某个对象。
}
7
void main()
{
MaxClass mc(34,67,143,89);
cout<<"计算前两个数中的最大数为:“
<<mc.max(34,67)<<endl;
cout<<"计算前三个数中的最大数为:“
<<mc.max(34,67,143)<<endl;
cout<<"计算四个数中的最大数为:“
运行结果: 张晓强,园林工人 李文卓,生命科学教师
23
6.2.3 虚函数的重载
• 2. 多继承中的虚函数
【例6.8】多继承中使用虚函数例题。
#include <iostream.h>
class base1
//定义基类base1
{
public: virtual void display()
//函数定义为虚函数
运行结果:
(1) : 动物(食草/食肉). (2) : 食草动物 : 羚羊 (3) : 食草动物 : 羚羊 (4) : 食肉动物 : 老虎 (5) : 食肉动物 : 老虎 (6) : 食草动物 : 羚羊 (7) : 食肉动物 : 老虎
数据结构与程序设计考试大纲
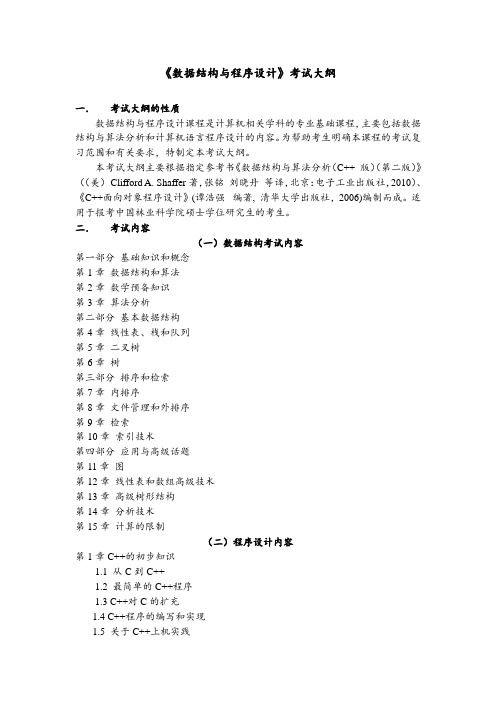
《数据结构与程序设计》考试大纲一.考试大纲的性质数据结构与程序设计课程是计算机相关学科的专业基础课程,主要包括数据结构与算法分析和计算机语言程序设计的内容。
为帮助考生明确本课程的考试复习范围和有关要求,特制定本考试大纲。
本考试大纲主要根据指定参考书《数据结构与算法分析(C++ 版)(第二版)》((美)Clifford A. Shaffer著,张铭刘晓丹等译,北京:电子工业出版社,2010)、《C++面向对象程序设计》(谭浩强编著, 清华大学出版社,2006)编制而成。
适用于报考中国林业科学院硕士学位研究生的考生。
二.考试内容(一)数据结构考试内容第一部分基础知识和概念第1章数据结构和算法第2章数学预备知识第3章算法分析第二部分基本数据结构第4章线性表、栈和队列第5章二叉树第6章树第三部分排序和检索第7章内排序第8章文件管理和外排序第9章检索第10章索引技术第四部分应用与高级话题第11章图第12章线性表和数组高级技术第13章高级树形结构第14章分析技术第15章计算的限制(二)程序设计内容第1章C++的初步知识1.1 从C到C++1.2 最简单的C++程序1.3 C++对C的扩充1.4 C++程序的编写和实现1.5 关于C++上机实践第2章类和对象第3章关于类和对象的进一步讨论第4章运算符重载第5章继承与派生第6章多态性与虚函数第7章输入输出流第8章C++工具三.考试要求数据结构与程序设计作为计算机相关专业的基础和公共课程,要求考生掌握数据结构和算法分析的基本概念、掌握常用数据结构及其分析算法,了解常用面向对象的编程语言的特点和编程规范,能熟练应用C++程序语言编写和实现简单应用程序(如数据结构与算法等)。
了解数据结构算法与计算机程序设计的关系,具有综合运用所学知识分析和解决问题的能力。
四.试卷结构数据结构与程序设计各占50%。
1. 名词解释(30%)2. 简答题(40%)3. 论述题(30%)五.考试方式和时间考试方式:笔试考试时间:3小时主要参考书1.《数据结构与算法分析(C++ 版)(第二版)》,(美)Clifford A. Shaffer 著,张铭刘晓丹等译,北京:电子工业出版社,20102.《C++面向对象程序设计》,谭浩强编著, 清华大学出版社,20062。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
实验八 继承性与派生类
• 实验目的: 1、掌握继承的语法格式; 2、掌握派生类的成员访问控制属 性; • 实验内容: 1、调试习题2和4,给出实验结果;
抽象类
定义:如果一个类至少有一个纯虚函数,那么该 类成为抽象类。 规则: 1、抽象类可以有非纯虚成员; 2、抽象类不能建立对象(可以建立指针); 3、抽象类不能用作参数类型、函数返回类型或 显示转换的类型。
例
#include <iostream.h> void class Graphic{ { public: virtual void Draw()=0; }; } class Rectangle:public Graphic{ public: void Draw() {cout<<"draw a rectangle now "<<endl;} };
class Graphic{ public: virtual void draw() { cout<<" draw a graphic"<<endl; } };
问题解决
class Graphic{ void main() public: virtual void Draw() { {cout<<"draw a graphic now "<<endl;} }; Graphic * agraphic; class Rectangle:public Graphic{ Rectangle * arect=new Rectangle(); public: void Draw() agraphic=arect; {cout<<"draw a rectangle now "<<endl;} agraphic->Draw(); }; class Circle:public Graphic{ Circle * acircle=new Circle(); public: agraphic=acircle; void Draw() {cout<<"draw a circle now "<<endl;} agraphic->Draw(); };
void main() { Graphic g; g.Draw(); Rectangle * arect=new Rectangle(); arect->Draw(); Graphic *p=arect; p->Draw(); }
课后作业
1、武器中有手枪,大炮和导弹等,这些武器都 有发射功能。 请用纯虚函数和抽象类的概念来设计他们。
main() Rectangle * arect=new Rectangle(); arect->Draw();
பைடு நூலகம் 以下程序是否有错?输出 结果是什么?
#include <iostream.h> class Graphic{ public: virtual void Draw()=0 { cout<<"draw a graphic"<<endl; } }; class Rectangle:public Graphic{ public: void Draw() {cout<<"draw a rectangle now "<<endl;} };
Rectangle Draw()
void main() {
Circle Draw()
Graphic * agraphic; Rectangle * arect=new Rectangle(); agraphic=arect; agraphic->Draw(); Circle * acircle=new Circle(); agraphic=acircle; agraphic->Draw(); }
第六章 多态性与虚函数
Graphic Draw()
继承中的指针
class Graphic{ public: void Draw() {cout<<"draw a graphic now "<<endl;} }; class Rectangle:public Graphic{ public: void Draw() {cout<<"draw a rectangle now "<<endl;} }; class Circle:public Graphic{ public: void Draw() {cout<<"draw a circle now "<<endl;} };
纯虚函数
• 有的类没有或不需要实例,即从不创建 对象; • 你希望所有派生类都必须重定义自己的 虚函数 ——使用纯虚函数
纯虚函数—语法
virtual 函数类型 函数名(参数)=0; 注意:纯虚函数可以而且应该没有函数体, 因为他从来不被执行 例如:
class Graphic{ public: virtual void Draw()=0; };
}
动态绑定
动态绑定(dynamic binding):必须到程序运 行时才可确定调用的函数体。
fun base fun Virtual func Virtual func desend fun Virtual func Virtual func fun
问题
• 什么是Graphic? 画一个圆可以,但画一个图形??? 怎么画?
静态绑定
• 绑定:将一个函数调用链接上相应于函 数体代码的过程。 • 静态绑定(static banding):在编译时确 (static banding) 定函数所调用的函数体。
fun base fun desend fun fun
运行时多态性——虚函数
定义:类中具有保留字virtual的函数称为虚函数。 语法: virtual 返回类型 类名::函数名(参数) {函数体} 例: